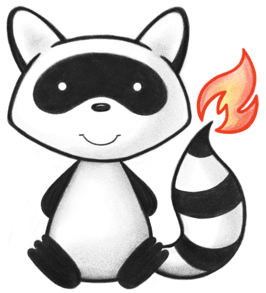
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.Date; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * For referring to data content defined in other formats. 047 */ 048@DatatypeDef(name="Attachment") 049public class Attachment extends Type implements ICompositeType { 050 051 /** 052 * Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate. 053 */ 054 @Child(name = "contentType", type = {CodeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 055 @Description(shortDefinition="Mime type of the content, with charset etc.", formalDefinition="Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate." ) 056 protected CodeType contentType; 057 058 /** 059 * The human language of the content. The value can be any valid value according to BCP 47. 060 */ 061 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Human language of the content (BCP-47)", formalDefinition="The human language of the content. The value can be any valid value according to BCP 47." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 064 protected CodeType language; 065 066 /** 067 * The actual data of the attachment - a sequence of bytes. In XML, represented using base64. 068 */ 069 @Child(name = "data", type = {Base64BinaryType.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Data inline, base64ed", formalDefinition="The actual data of the attachment - a sequence of bytes. In XML, represented using base64." ) 071 protected Base64BinaryType data; 072 073 /** 074 * An alternative location where the data can be accessed. 075 */ 076 @Child(name = "url", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=true) 077 @Description(shortDefinition="Uri where the data can be found", formalDefinition="An alternative location where the data can be accessed." ) 078 protected UriType url; 079 080 /** 081 * The number of bytes of data that make up this attachment (before base64 encoding, if that is done). 082 */ 083 @Child(name = "size", type = {UnsignedIntType.class}, order=4, min=0, max=1, modifier=false, summary=true) 084 @Description(shortDefinition="Number of bytes of content (if url provided)", formalDefinition="The number of bytes of data that make up this attachment (before base64 encoding, if that is done)." ) 085 protected UnsignedIntType size; 086 087 /** 088 * The calculated hash of the data using SHA-1. Represented using base64. 089 */ 090 @Child(name = "hash", type = {Base64BinaryType.class}, order=5, min=0, max=1, modifier=false, summary=true) 091 @Description(shortDefinition="Hash of the data (sha-1, base64ed)", formalDefinition="The calculated hash of the data using SHA-1. Represented using base64." ) 092 protected Base64BinaryType hash; 093 094 /** 095 * A label or set of text to display in place of the data. 096 */ 097 @Child(name = "title", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 098 @Description(shortDefinition="Label to display in place of the data", formalDefinition="A label or set of text to display in place of the data." ) 099 protected StringType title; 100 101 /** 102 * The date that the attachment was first created. 103 */ 104 @Child(name = "creation", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 105 @Description(shortDefinition="Date attachment was first created", formalDefinition="The date that the attachment was first created." ) 106 protected DateTimeType creation; 107 108 private static final long serialVersionUID = 581007080L; 109 110 /** 111 * Constructor 112 */ 113 public Attachment() { 114 super(); 115 } 116 117 /** 118 * @return {@link #contentType} (Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 119 */ 120 public CodeType getContentTypeElement() { 121 if (this.contentType == null) 122 if (Configuration.errorOnAutoCreate()) 123 throw new Error("Attempt to auto-create Attachment.contentType"); 124 else if (Configuration.doAutoCreate()) 125 this.contentType = new CodeType(); // bb 126 return this.contentType; 127 } 128 129 public boolean hasContentTypeElement() { 130 return this.contentType != null && !this.contentType.isEmpty(); 131 } 132 133 public boolean hasContentType() { 134 return this.contentType != null && !this.contentType.isEmpty(); 135 } 136 137 /** 138 * @param value {@link #contentType} (Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 139 */ 140 public Attachment setContentTypeElement(CodeType value) { 141 this.contentType = value; 142 return this; 143 } 144 145 /** 146 * @return Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate. 147 */ 148 public String getContentType() { 149 return this.contentType == null ? null : this.contentType.getValue(); 150 } 151 152 /** 153 * @param value Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate. 154 */ 155 public Attachment setContentType(String value) { 156 if (Utilities.noString(value)) 157 this.contentType = null; 158 else { 159 if (this.contentType == null) 160 this.contentType = new CodeType(); 161 this.contentType.setValue(value); 162 } 163 return this; 164 } 165 166 /** 167 * @return {@link #language} (The human language of the content. The value can be any valid value according to BCP 47.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 168 */ 169 public CodeType getLanguageElement() { 170 if (this.language == null) 171 if (Configuration.errorOnAutoCreate()) 172 throw new Error("Attempt to auto-create Attachment.language"); 173 else if (Configuration.doAutoCreate()) 174 this.language = new CodeType(); // bb 175 return this.language; 176 } 177 178 public boolean hasLanguageElement() { 179 return this.language != null && !this.language.isEmpty(); 180 } 181 182 public boolean hasLanguage() { 183 return this.language != null && !this.language.isEmpty(); 184 } 185 186 /** 187 * @param value {@link #language} (The human language of the content. The value can be any valid value according to BCP 47.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 188 */ 189 public Attachment setLanguageElement(CodeType value) { 190 this.language = value; 191 return this; 192 } 193 194 /** 195 * @return The human language of the content. The value can be any valid value according to BCP 47. 196 */ 197 public String getLanguage() { 198 return this.language == null ? null : this.language.getValue(); 199 } 200 201 /** 202 * @param value The human language of the content. The value can be any valid value according to BCP 47. 203 */ 204 public Attachment setLanguage(String value) { 205 if (Utilities.noString(value)) 206 this.language = null; 207 else { 208 if (this.language == null) 209 this.language = new CodeType(); 210 this.language.setValue(value); 211 } 212 return this; 213 } 214 215 /** 216 * @return {@link #data} (The actual data of the attachment - a sequence of bytes. In XML, represented using base64.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 217 */ 218 public Base64BinaryType getDataElement() { 219 if (this.data == null) 220 if (Configuration.errorOnAutoCreate()) 221 throw new Error("Attempt to auto-create Attachment.data"); 222 else if (Configuration.doAutoCreate()) 223 this.data = new Base64BinaryType(); // bb 224 return this.data; 225 } 226 227 public boolean hasDataElement() { 228 return this.data != null && !this.data.isEmpty(); 229 } 230 231 public boolean hasData() { 232 return this.data != null && !this.data.isEmpty(); 233 } 234 235 /** 236 * @param value {@link #data} (The actual data of the attachment - a sequence of bytes. In XML, represented using base64.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 237 */ 238 public Attachment setDataElement(Base64BinaryType value) { 239 this.data = value; 240 return this; 241 } 242 243 /** 244 * @return The actual data of the attachment - a sequence of bytes. In XML, represented using base64. 245 */ 246 public byte[] getData() { 247 return this.data == null ? null : this.data.getValue(); 248 } 249 250 /** 251 * @param value The actual data of the attachment - a sequence of bytes. In XML, represented using base64. 252 */ 253 public Attachment setData(byte[] value) { 254 if (value == null) 255 this.data = null; 256 else { 257 if (this.data == null) 258 this.data = new Base64BinaryType(); 259 this.data.setValue(value); 260 } 261 return this; 262 } 263 264 /** 265 * @return {@link #url} (An alternative location where the data can be accessed.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 266 */ 267 public UriType getUrlElement() { 268 if (this.url == null) 269 if (Configuration.errorOnAutoCreate()) 270 throw new Error("Attempt to auto-create Attachment.url"); 271 else if (Configuration.doAutoCreate()) 272 this.url = new UriType(); // bb 273 return this.url; 274 } 275 276 public boolean hasUrlElement() { 277 return this.url != null && !this.url.isEmpty(); 278 } 279 280 public boolean hasUrl() { 281 return this.url != null && !this.url.isEmpty(); 282 } 283 284 /** 285 * @param value {@link #url} (An alternative location where the data can be accessed.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 286 */ 287 public Attachment setUrlElement(UriType value) { 288 this.url = value; 289 return this; 290 } 291 292 /** 293 * @return An alternative location where the data can be accessed. 294 */ 295 public String getUrl() { 296 return this.url == null ? null : this.url.getValue(); 297 } 298 299 /** 300 * @param value An alternative location where the data can be accessed. 301 */ 302 public Attachment setUrl(String value) { 303 if (Utilities.noString(value)) 304 this.url = null; 305 else { 306 if (this.url == null) 307 this.url = new UriType(); 308 this.url.setValue(value); 309 } 310 return this; 311 } 312 313 /** 314 * @return {@link #size} (The number of bytes of data that make up this attachment (before base64 encoding, if that is done).). This is the underlying object with id, value and extensions. The accessor "getSize" gives direct access to the value 315 */ 316 public UnsignedIntType getSizeElement() { 317 if (this.size == null) 318 if (Configuration.errorOnAutoCreate()) 319 throw new Error("Attempt to auto-create Attachment.size"); 320 else if (Configuration.doAutoCreate()) 321 this.size = new UnsignedIntType(); // bb 322 return this.size; 323 } 324 325 public boolean hasSizeElement() { 326 return this.size != null && !this.size.isEmpty(); 327 } 328 329 public boolean hasSize() { 330 return this.size != null && !this.size.isEmpty(); 331 } 332 333 /** 334 * @param value {@link #size} (The number of bytes of data that make up this attachment (before base64 encoding, if that is done).). This is the underlying object with id, value and extensions. The accessor "getSize" gives direct access to the value 335 */ 336 public Attachment setSizeElement(UnsignedIntType value) { 337 this.size = value; 338 return this; 339 } 340 341 /** 342 * @return The number of bytes of data that make up this attachment (before base64 encoding, if that is done). 343 */ 344 public int getSize() { 345 return this.size == null || this.size.isEmpty() ? 0 : this.size.getValue(); 346 } 347 348 /** 349 * @param value The number of bytes of data that make up this attachment (before base64 encoding, if that is done). 350 */ 351 public Attachment setSize(int value) { 352 if (this.size == null) 353 this.size = new UnsignedIntType(); 354 this.size.setValue(value); 355 return this; 356 } 357 358 /** 359 * @return {@link #hash} (The calculated hash of the data using SHA-1. Represented using base64.). This is the underlying object with id, value and extensions. The accessor "getHash" gives direct access to the value 360 */ 361 public Base64BinaryType getHashElement() { 362 if (this.hash == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create Attachment.hash"); 365 else if (Configuration.doAutoCreate()) 366 this.hash = new Base64BinaryType(); // bb 367 return this.hash; 368 } 369 370 public boolean hasHashElement() { 371 return this.hash != null && !this.hash.isEmpty(); 372 } 373 374 public boolean hasHash() { 375 return this.hash != null && !this.hash.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #hash} (The calculated hash of the data using SHA-1. Represented using base64.). This is the underlying object with id, value and extensions. The accessor "getHash" gives direct access to the value 380 */ 381 public Attachment setHashElement(Base64BinaryType value) { 382 this.hash = value; 383 return this; 384 } 385 386 /** 387 * @return The calculated hash of the data using SHA-1. Represented using base64. 388 */ 389 public byte[] getHash() { 390 return this.hash == null ? null : this.hash.getValue(); 391 } 392 393 /** 394 * @param value The calculated hash of the data using SHA-1. Represented using base64. 395 */ 396 public Attachment setHash(byte[] value) { 397 if (value == null) 398 this.hash = null; 399 else { 400 if (this.hash == null) 401 this.hash = new Base64BinaryType(); 402 this.hash.setValue(value); 403 } 404 return this; 405 } 406 407 /** 408 * @return {@link #title} (A label or set of text to display in place of the data.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 409 */ 410 public StringType getTitleElement() { 411 if (this.title == null) 412 if (Configuration.errorOnAutoCreate()) 413 throw new Error("Attempt to auto-create Attachment.title"); 414 else if (Configuration.doAutoCreate()) 415 this.title = new StringType(); // bb 416 return this.title; 417 } 418 419 public boolean hasTitleElement() { 420 return this.title != null && !this.title.isEmpty(); 421 } 422 423 public boolean hasTitle() { 424 return this.title != null && !this.title.isEmpty(); 425 } 426 427 /** 428 * @param value {@link #title} (A label or set of text to display in place of the data.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 429 */ 430 public Attachment setTitleElement(StringType value) { 431 this.title = value; 432 return this; 433 } 434 435 /** 436 * @return A label or set of text to display in place of the data. 437 */ 438 public String getTitle() { 439 return this.title == null ? null : this.title.getValue(); 440 } 441 442 /** 443 * @param value A label or set of text to display in place of the data. 444 */ 445 public Attachment setTitle(String value) { 446 if (Utilities.noString(value)) 447 this.title = null; 448 else { 449 if (this.title == null) 450 this.title = new StringType(); 451 this.title.setValue(value); 452 } 453 return this; 454 } 455 456 /** 457 * @return {@link #creation} (The date that the attachment was first created.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 458 */ 459 public DateTimeType getCreationElement() { 460 if (this.creation == null) 461 if (Configuration.errorOnAutoCreate()) 462 throw new Error("Attempt to auto-create Attachment.creation"); 463 else if (Configuration.doAutoCreate()) 464 this.creation = new DateTimeType(); // bb 465 return this.creation; 466 } 467 468 public boolean hasCreationElement() { 469 return this.creation != null && !this.creation.isEmpty(); 470 } 471 472 public boolean hasCreation() { 473 return this.creation != null && !this.creation.isEmpty(); 474 } 475 476 /** 477 * @param value {@link #creation} (The date that the attachment was first created.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 478 */ 479 public Attachment setCreationElement(DateTimeType value) { 480 this.creation = value; 481 return this; 482 } 483 484 /** 485 * @return The date that the attachment was first created. 486 */ 487 public Date getCreation() { 488 return this.creation == null ? null : this.creation.getValue(); 489 } 490 491 /** 492 * @param value The date that the attachment was first created. 493 */ 494 public Attachment setCreation(Date value) { 495 if (value == null) 496 this.creation = null; 497 else { 498 if (this.creation == null) 499 this.creation = new DateTimeType(); 500 this.creation.setValue(value); 501 } 502 return this; 503 } 504 505 protected void listChildren(List<Property> children) { 506 super.listChildren(children); 507 children.add(new Property("contentType", "code", "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.", 0, 1, contentType)); 508 children.add(new Property("language", "code", "The human language of the content. The value can be any valid value according to BCP 47.", 0, 1, language)); 509 children.add(new Property("data", "base64Binary", "The actual data of the attachment - a sequence of bytes. In XML, represented using base64.", 0, 1, data)); 510 children.add(new Property("url", "uri", "An alternative location where the data can be accessed.", 0, 1, url)); 511 children.add(new Property("size", "unsignedInt", "The number of bytes of data that make up this attachment (before base64 encoding, if that is done).", 0, 1, size)); 512 children.add(new Property("hash", "base64Binary", "The calculated hash of the data using SHA-1. Represented using base64.", 0, 1, hash)); 513 children.add(new Property("title", "string", "A label or set of text to display in place of the data.", 0, 1, title)); 514 children.add(new Property("creation", "dateTime", "The date that the attachment was first created.", 0, 1, creation)); 515 } 516 517 @Override 518 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 519 switch (_hash) { 520 case -389131437: /*contentType*/ return new Property("contentType", "code", "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.", 0, 1, contentType); 521 case -1613589672: /*language*/ return new Property("language", "code", "The human language of the content. The value can be any valid value according to BCP 47.", 0, 1, language); 522 case 3076010: /*data*/ return new Property("data", "base64Binary", "The actual data of the attachment - a sequence of bytes. In XML, represented using base64.", 0, 1, data); 523 case 116079: /*url*/ return new Property("url", "uri", "An alternative location where the data can be accessed.", 0, 1, url); 524 case 3530753: /*size*/ return new Property("size", "unsignedInt", "The number of bytes of data that make up this attachment (before base64 encoding, if that is done).", 0, 1, size); 525 case 3195150: /*hash*/ return new Property("hash", "base64Binary", "The calculated hash of the data using SHA-1. Represented using base64.", 0, 1, hash); 526 case 110371416: /*title*/ return new Property("title", "string", "A label or set of text to display in place of the data.", 0, 1, title); 527 case 1820421855: /*creation*/ return new Property("creation", "dateTime", "The date that the attachment was first created.", 0, 1, creation); 528 default: return super.getNamedProperty(_hash, _name, _checkValid); 529 } 530 531 } 532 533 @Override 534 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 535 switch (hash) { 536 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 537 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 538 case 3076010: /*data*/ return this.data == null ? new Base[0] : new Base[] {this.data}; // Base64BinaryType 539 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 540 case 3530753: /*size*/ return this.size == null ? new Base[0] : new Base[] {this.size}; // UnsignedIntType 541 case 3195150: /*hash*/ return this.hash == null ? new Base[0] : new Base[] {this.hash}; // Base64BinaryType 542 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 543 case 1820421855: /*creation*/ return this.creation == null ? new Base[0] : new Base[] {this.creation}; // DateTimeType 544 default: return super.getProperty(hash, name, checkValid); 545 } 546 547 } 548 549 @Override 550 public Base setProperty(int hash, String name, Base value) throws FHIRException { 551 switch (hash) { 552 case -389131437: // contentType 553 this.contentType = castToCode(value); // CodeType 554 return value; 555 case -1613589672: // language 556 this.language = castToCode(value); // CodeType 557 return value; 558 case 3076010: // data 559 this.data = castToBase64Binary(value); // Base64BinaryType 560 return value; 561 case 116079: // url 562 this.url = castToUri(value); // UriType 563 return value; 564 case 3530753: // size 565 this.size = castToUnsignedInt(value); // UnsignedIntType 566 return value; 567 case 3195150: // hash 568 this.hash = castToBase64Binary(value); // Base64BinaryType 569 return value; 570 case 110371416: // title 571 this.title = castToString(value); // StringType 572 return value; 573 case 1820421855: // creation 574 this.creation = castToDateTime(value); // DateTimeType 575 return value; 576 default: return super.setProperty(hash, name, value); 577 } 578 579 } 580 581 @Override 582 public Base setProperty(String name, Base value) throws FHIRException { 583 if (name.equals("contentType")) { 584 this.contentType = castToCode(value); // CodeType 585 } else if (name.equals("language")) { 586 this.language = castToCode(value); // CodeType 587 } else if (name.equals("data")) { 588 this.data = castToBase64Binary(value); // Base64BinaryType 589 } else if (name.equals("url")) { 590 this.url = castToUri(value); // UriType 591 } else if (name.equals("size")) { 592 this.size = castToUnsignedInt(value); // UnsignedIntType 593 } else if (name.equals("hash")) { 594 this.hash = castToBase64Binary(value); // Base64BinaryType 595 } else if (name.equals("title")) { 596 this.title = castToString(value); // StringType 597 } else if (name.equals("creation")) { 598 this.creation = castToDateTime(value); // DateTimeType 599 } else 600 return super.setProperty(name, value); 601 return value; 602 } 603 604 @Override 605 public Base makeProperty(int hash, String name) throws FHIRException { 606 switch (hash) { 607 case -389131437: return getContentTypeElement(); 608 case -1613589672: return getLanguageElement(); 609 case 3076010: return getDataElement(); 610 case 116079: return getUrlElement(); 611 case 3530753: return getSizeElement(); 612 case 3195150: return getHashElement(); 613 case 110371416: return getTitleElement(); 614 case 1820421855: return getCreationElement(); 615 default: return super.makeProperty(hash, name); 616 } 617 618 } 619 620 @Override 621 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 622 switch (hash) { 623 case -389131437: /*contentType*/ return new String[] {"code"}; 624 case -1613589672: /*language*/ return new String[] {"code"}; 625 case 3076010: /*data*/ return new String[] {"base64Binary"}; 626 case 116079: /*url*/ return new String[] {"uri"}; 627 case 3530753: /*size*/ return new String[] {"unsignedInt"}; 628 case 3195150: /*hash*/ return new String[] {"base64Binary"}; 629 case 110371416: /*title*/ return new String[] {"string"}; 630 case 1820421855: /*creation*/ return new String[] {"dateTime"}; 631 default: return super.getTypesForProperty(hash, name); 632 } 633 634 } 635 636 @Override 637 public Base addChild(String name) throws FHIRException { 638 if (name.equals("contentType")) { 639 throw new FHIRException("Cannot call addChild on a singleton property Attachment.contentType"); 640 } 641 else if (name.equals("language")) { 642 throw new FHIRException("Cannot call addChild on a singleton property Attachment.language"); 643 } 644 else if (name.equals("data")) { 645 throw new FHIRException("Cannot call addChild on a singleton property Attachment.data"); 646 } 647 else if (name.equals("url")) { 648 throw new FHIRException("Cannot call addChild on a singleton property Attachment.url"); 649 } 650 else if (name.equals("size")) { 651 throw new FHIRException("Cannot call addChild on a singleton property Attachment.size"); 652 } 653 else if (name.equals("hash")) { 654 throw new FHIRException("Cannot call addChild on a singleton property Attachment.hash"); 655 } 656 else if (name.equals("title")) { 657 throw new FHIRException("Cannot call addChild on a singleton property Attachment.title"); 658 } 659 else if (name.equals("creation")) { 660 throw new FHIRException("Cannot call addChild on a singleton property Attachment.creation"); 661 } 662 else 663 return super.addChild(name); 664 } 665 666 public String fhirType() { 667 return "Attachment"; 668 669 } 670 671 public Attachment copy() { 672 Attachment dst = new Attachment(); 673 copyValues(dst); 674 dst.contentType = contentType == null ? null : contentType.copy(); 675 dst.language = language == null ? null : language.copy(); 676 dst.data = data == null ? null : data.copy(); 677 dst.url = url == null ? null : url.copy(); 678 dst.size = size == null ? null : size.copy(); 679 dst.hash = hash == null ? null : hash.copy(); 680 dst.title = title == null ? null : title.copy(); 681 dst.creation = creation == null ? null : creation.copy(); 682 return dst; 683 } 684 685 protected Attachment typedCopy() { 686 return copy(); 687 } 688 689 @Override 690 public boolean equalsDeep(Base other_) { 691 if (!super.equalsDeep(other_)) 692 return false; 693 if (!(other_ instanceof Attachment)) 694 return false; 695 Attachment o = (Attachment) other_; 696 return compareDeep(contentType, o.contentType, true) && compareDeep(language, o.language, true) 697 && compareDeep(data, o.data, true) && compareDeep(url, o.url, true) && compareDeep(size, o.size, true) 698 && compareDeep(hash, o.hash, true) && compareDeep(title, o.title, true) && compareDeep(creation, o.creation, true) 699 ; 700 } 701 702 @Override 703 public boolean equalsShallow(Base other_) { 704 if (!super.equalsShallow(other_)) 705 return false; 706 if (!(other_ instanceof Attachment)) 707 return false; 708 Attachment o = (Attachment) other_; 709 return compareValues(contentType, o.contentType, true) && compareValues(language, o.language, true) 710 && compareValues(data, o.data, true) && compareValues(url, o.url, true) && compareValues(size, o.size, true) 711 && compareValues(hash, o.hash, true) && compareValues(title, o.title, true) && compareValues(creation, o.creation, true) 712 ; 713 } 714 715 public boolean isEmpty() { 716 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(contentType, language, data 717 , url, size, hash, title, creation); 718 } 719 720 721}