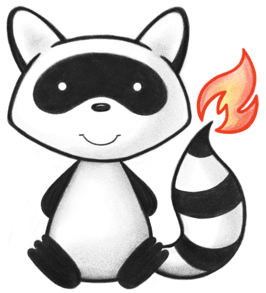
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 050 */ 051@ResourceDef(name="AuditEvent", profile="http://hl7.org/fhir/Profile/AuditEvent") 052public class AuditEvent extends DomainResource { 053 054 public enum AuditEventAction { 055 /** 056 * Create a new database object, such as placing an order. 057 */ 058 C, 059 /** 060 * Display or print data, such as a doctor census. 061 */ 062 R, 063 /** 064 * Update data, such as revise patient information. 065 */ 066 U, 067 /** 068 * Delete items, such as a doctor master file record. 069 */ 070 D, 071 /** 072 * Perform a system or application function such as log-on, program execution or use of an object's method, or perform a query/search operation. 073 */ 074 E, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static AuditEventAction fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("C".equals(codeString)) 083 return C; 084 if ("R".equals(codeString)) 085 return R; 086 if ("U".equals(codeString)) 087 return U; 088 if ("D".equals(codeString)) 089 return D; 090 if ("E".equals(codeString)) 091 return E; 092 if (Configuration.isAcceptInvalidEnums()) 093 return null; 094 else 095 throw new FHIRException("Unknown AuditEventAction code '"+codeString+"'"); 096 } 097 public String toCode() { 098 switch (this) { 099 case C: return "C"; 100 case R: return "R"; 101 case U: return "U"; 102 case D: return "D"; 103 case E: return "E"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getSystem() { 109 switch (this) { 110 case C: return "http://hl7.org/fhir/audit-event-action"; 111 case R: return "http://hl7.org/fhir/audit-event-action"; 112 case U: return "http://hl7.org/fhir/audit-event-action"; 113 case D: return "http://hl7.org/fhir/audit-event-action"; 114 case E: return "http://hl7.org/fhir/audit-event-action"; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getDefinition() { 120 switch (this) { 121 case C: return "Create a new database object, such as placing an order."; 122 case R: return "Display or print data, such as a doctor census."; 123 case U: return "Update data, such as revise patient information."; 124 case D: return "Delete items, such as a doctor master file record."; 125 case E: return "Perform a system or application function such as log-on, program execution or use of an object's method, or perform a query/search operation."; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case C: return "Create"; 133 case R: return "Read/View/Print"; 134 case U: return "Update"; 135 case D: return "Delete"; 136 case E: return "Execute"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 } 142 143 public static class AuditEventActionEnumFactory implements EnumFactory<AuditEventAction> { 144 public AuditEventAction fromCode(String codeString) throws IllegalArgumentException { 145 if (codeString == null || "".equals(codeString)) 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("C".equals(codeString)) 149 return AuditEventAction.C; 150 if ("R".equals(codeString)) 151 return AuditEventAction.R; 152 if ("U".equals(codeString)) 153 return AuditEventAction.U; 154 if ("D".equals(codeString)) 155 return AuditEventAction.D; 156 if ("E".equals(codeString)) 157 return AuditEventAction.E; 158 throw new IllegalArgumentException("Unknown AuditEventAction code '"+codeString+"'"); 159 } 160 public Enumeration<AuditEventAction> fromType(PrimitiveType<?> code) throws FHIRException { 161 if (code == null) 162 return null; 163 if (code.isEmpty()) 164 return new Enumeration<AuditEventAction>(this); 165 String codeString = code.asStringValue(); 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("C".equals(codeString)) 169 return new Enumeration<AuditEventAction>(this, AuditEventAction.C); 170 if ("R".equals(codeString)) 171 return new Enumeration<AuditEventAction>(this, AuditEventAction.R); 172 if ("U".equals(codeString)) 173 return new Enumeration<AuditEventAction>(this, AuditEventAction.U); 174 if ("D".equals(codeString)) 175 return new Enumeration<AuditEventAction>(this, AuditEventAction.D); 176 if ("E".equals(codeString)) 177 return new Enumeration<AuditEventAction>(this, AuditEventAction.E); 178 throw new FHIRException("Unknown AuditEventAction code '"+codeString+"'"); 179 } 180 public String toCode(AuditEventAction code) { 181 if (code == AuditEventAction.NULL) 182 return null; 183 if (code == AuditEventAction.C) 184 return "C"; 185 if (code == AuditEventAction.R) 186 return "R"; 187 if (code == AuditEventAction.U) 188 return "U"; 189 if (code == AuditEventAction.D) 190 return "D"; 191 if (code == AuditEventAction.E) 192 return "E"; 193 return "?"; 194 } 195 public String toSystem(AuditEventAction code) { 196 return code.getSystem(); 197 } 198 } 199 200 public enum AuditEventOutcome { 201 /** 202 * The operation completed successfully (whether with warnings or not). 203 */ 204 _0, 205 /** 206 * The action was not successful due to some kind of catered for error (often equivalent to an HTTP 400 response). 207 */ 208 _4, 209 /** 210 * The action was not successful due to some kind of unexpected error (often equivalent to an HTTP 500 response). 211 */ 212 _8, 213 /** 214 * An error of such magnitude occurred that the system is no longer available for use (i.e. the system died). 215 */ 216 _12, 217 /** 218 * added to help the parsers with the generic types 219 */ 220 NULL; 221 public static AuditEventOutcome fromCode(String codeString) throws FHIRException { 222 if (codeString == null || "".equals(codeString)) 223 return null; 224 if ("0".equals(codeString)) 225 return _0; 226 if ("4".equals(codeString)) 227 return _4; 228 if ("8".equals(codeString)) 229 return _8; 230 if ("12".equals(codeString)) 231 return _12; 232 if (Configuration.isAcceptInvalidEnums()) 233 return null; 234 else 235 throw new FHIRException("Unknown AuditEventOutcome code '"+codeString+"'"); 236 } 237 public String toCode() { 238 switch (this) { 239 case _0: return "0"; 240 case _4: return "4"; 241 case _8: return "8"; 242 case _12: return "12"; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 public String getSystem() { 248 switch (this) { 249 case _0: return "http://hl7.org/fhir/audit-event-outcome"; 250 case _4: return "http://hl7.org/fhir/audit-event-outcome"; 251 case _8: return "http://hl7.org/fhir/audit-event-outcome"; 252 case _12: return "http://hl7.org/fhir/audit-event-outcome"; 253 case NULL: return null; 254 default: return "?"; 255 } 256 } 257 public String getDefinition() { 258 switch (this) { 259 case _0: return "The operation completed successfully (whether with warnings or not)."; 260 case _4: return "The action was not successful due to some kind of catered for error (often equivalent to an HTTP 400 response)."; 261 case _8: return "The action was not successful due to some kind of unexpected error (often equivalent to an HTTP 500 response)."; 262 case _12: return "An error of such magnitude occurred that the system is no longer available for use (i.e. the system died)."; 263 case NULL: return null; 264 default: return "?"; 265 } 266 } 267 public String getDisplay() { 268 switch (this) { 269 case _0: return "Success"; 270 case _4: return "Minor failure"; 271 case _8: return "Serious failure"; 272 case _12: return "Major failure"; 273 case NULL: return null; 274 default: return "?"; 275 } 276 } 277 } 278 279 public static class AuditEventOutcomeEnumFactory implements EnumFactory<AuditEventOutcome> { 280 public AuditEventOutcome fromCode(String codeString) throws IllegalArgumentException { 281 if (codeString == null || "".equals(codeString)) 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("0".equals(codeString)) 285 return AuditEventOutcome._0; 286 if ("4".equals(codeString)) 287 return AuditEventOutcome._4; 288 if ("8".equals(codeString)) 289 return AuditEventOutcome._8; 290 if ("12".equals(codeString)) 291 return AuditEventOutcome._12; 292 throw new IllegalArgumentException("Unknown AuditEventOutcome code '"+codeString+"'"); 293 } 294 public Enumeration<AuditEventOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 295 if (code == null) 296 return null; 297 if (code.isEmpty()) 298 return new Enumeration<AuditEventOutcome>(this); 299 String codeString = code.asStringValue(); 300 if (codeString == null || "".equals(codeString)) 301 return null; 302 if ("0".equals(codeString)) 303 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._0); 304 if ("4".equals(codeString)) 305 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._4); 306 if ("8".equals(codeString)) 307 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._8); 308 if ("12".equals(codeString)) 309 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._12); 310 throw new FHIRException("Unknown AuditEventOutcome code '"+codeString+"'"); 311 } 312 public String toCode(AuditEventOutcome code) { 313 if (code == AuditEventOutcome.NULL) 314 return null; 315 if (code == AuditEventOutcome._0) 316 return "0"; 317 if (code == AuditEventOutcome._4) 318 return "4"; 319 if (code == AuditEventOutcome._8) 320 return "8"; 321 if (code == AuditEventOutcome._12) 322 return "12"; 323 return "?"; 324 } 325 public String toSystem(AuditEventOutcome code) { 326 return code.getSystem(); 327 } 328 } 329 330 public enum AuditEventAgentNetworkType { 331 /** 332 * The machine name, including DNS name. 333 */ 334 _1, 335 /** 336 * The assigned Internet Protocol (IP) address. 337 */ 338 _2, 339 /** 340 * The assigned telephone number. 341 */ 342 _3, 343 /** 344 * The assigned email address. 345 */ 346 _4, 347 /** 348 * URI (User directory, HTTP-PUT, ftp, etc.). 349 */ 350 _5, 351 /** 352 * added to help the parsers with the generic types 353 */ 354 NULL; 355 public static AuditEventAgentNetworkType fromCode(String codeString) throws FHIRException { 356 if (codeString == null || "".equals(codeString)) 357 return null; 358 if ("1".equals(codeString)) 359 return _1; 360 if ("2".equals(codeString)) 361 return _2; 362 if ("3".equals(codeString)) 363 return _3; 364 if ("4".equals(codeString)) 365 return _4; 366 if ("5".equals(codeString)) 367 return _5; 368 if (Configuration.isAcceptInvalidEnums()) 369 return null; 370 else 371 throw new FHIRException("Unknown AuditEventAgentNetworkType code '"+codeString+"'"); 372 } 373 public String toCode() { 374 switch (this) { 375 case _1: return "1"; 376 case _2: return "2"; 377 case _3: return "3"; 378 case _4: return "4"; 379 case _5: return "5"; 380 case NULL: return null; 381 default: return "?"; 382 } 383 } 384 public String getSystem() { 385 switch (this) { 386 case _1: return "http://hl7.org/fhir/network-type"; 387 case _2: return "http://hl7.org/fhir/network-type"; 388 case _3: return "http://hl7.org/fhir/network-type"; 389 case _4: return "http://hl7.org/fhir/network-type"; 390 case _5: return "http://hl7.org/fhir/network-type"; 391 case NULL: return null; 392 default: return "?"; 393 } 394 } 395 public String getDefinition() { 396 switch (this) { 397 case _1: return "The machine name, including DNS name."; 398 case _2: return "The assigned Internet Protocol (IP) address."; 399 case _3: return "The assigned telephone number."; 400 case _4: return "The assigned email address."; 401 case _5: return "URI (User directory, HTTP-PUT, ftp, etc.)."; 402 case NULL: return null; 403 default: return "?"; 404 } 405 } 406 public String getDisplay() { 407 switch (this) { 408 case _1: return "Machine Name"; 409 case _2: return "IP Address"; 410 case _3: return "Telephone Number"; 411 case _4: return "Email address"; 412 case _5: return "URI"; 413 case NULL: return null; 414 default: return "?"; 415 } 416 } 417 } 418 419 public static class AuditEventAgentNetworkTypeEnumFactory implements EnumFactory<AuditEventAgentNetworkType> { 420 public AuditEventAgentNetworkType fromCode(String codeString) throws IllegalArgumentException { 421 if (codeString == null || "".equals(codeString)) 422 if (codeString == null || "".equals(codeString)) 423 return null; 424 if ("1".equals(codeString)) 425 return AuditEventAgentNetworkType._1; 426 if ("2".equals(codeString)) 427 return AuditEventAgentNetworkType._2; 428 if ("3".equals(codeString)) 429 return AuditEventAgentNetworkType._3; 430 if ("4".equals(codeString)) 431 return AuditEventAgentNetworkType._4; 432 if ("5".equals(codeString)) 433 return AuditEventAgentNetworkType._5; 434 throw new IllegalArgumentException("Unknown AuditEventAgentNetworkType code '"+codeString+"'"); 435 } 436 public Enumeration<AuditEventAgentNetworkType> fromType(PrimitiveType<?> code) throws FHIRException { 437 if (code == null) 438 return null; 439 if (code.isEmpty()) 440 return new Enumeration<AuditEventAgentNetworkType>(this); 441 String codeString = code.asStringValue(); 442 if (codeString == null || "".equals(codeString)) 443 return null; 444 if ("1".equals(codeString)) 445 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._1); 446 if ("2".equals(codeString)) 447 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._2); 448 if ("3".equals(codeString)) 449 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._3); 450 if ("4".equals(codeString)) 451 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._4); 452 if ("5".equals(codeString)) 453 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._5); 454 throw new FHIRException("Unknown AuditEventAgentNetworkType code '"+codeString+"'"); 455 } 456 public String toCode(AuditEventAgentNetworkType code) { 457 if (code == AuditEventAgentNetworkType.NULL) 458 return null; 459 if (code == AuditEventAgentNetworkType._1) 460 return "1"; 461 if (code == AuditEventAgentNetworkType._2) 462 return "2"; 463 if (code == AuditEventAgentNetworkType._3) 464 return "3"; 465 if (code == AuditEventAgentNetworkType._4) 466 return "4"; 467 if (code == AuditEventAgentNetworkType._5) 468 return "5"; 469 return "?"; 470 } 471 public String toSystem(AuditEventAgentNetworkType code) { 472 return code.getSystem(); 473 } 474 } 475 476 @Block() 477 public static class AuditEventAgentComponent extends BackboneElement implements IBaseBackboneElement { 478 /** 479 * The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context. 480 */ 481 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 482 @Description(shortDefinition="Agent role in the event", formalDefinition="The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context." ) 483 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 484 protected List<CodeableConcept> role; 485 486 /** 487 * Direct reference to a resource that identifies the agent. 488 */ 489 @Child(name = "reference", type = {Practitioner.class, Organization.class, Device.class, Patient.class, RelatedPerson.class}, order=2, min=0, max=1, modifier=false, summary=true) 490 @Description(shortDefinition="Direct reference to resource", formalDefinition="Direct reference to a resource that identifies the agent." ) 491 protected Reference reference; 492 493 /** 494 * The actual object that is the target of the reference (Direct reference to a resource that identifies the agent.) 495 */ 496 protected Resource referenceTarget; 497 498 /** 499 * Unique identifier for the user actively participating in the event. 500 */ 501 @Child(name = "userId", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 502 @Description(shortDefinition="Unique identifier for the user", formalDefinition="Unique identifier for the user actively participating in the event." ) 503 protected Identifier userId; 504 505 /** 506 * Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available. 507 */ 508 @Child(name = "altId", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 509 @Description(shortDefinition="Alternative User id e.g. authentication", formalDefinition="Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available." ) 510 protected StringType altId; 511 512 /** 513 * Human-meaningful name for the agent. 514 */ 515 @Child(name = "name", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 516 @Description(shortDefinition="Human-meaningful name for the agent", formalDefinition="Human-meaningful name for the agent." ) 517 protected StringType name; 518 519 /** 520 * Indicator that the user is or is not the requestor, or initiator, for the event being audited. 521 */ 522 @Child(name = "requestor", type = {BooleanType.class}, order=6, min=1, max=1, modifier=false, summary=false) 523 @Description(shortDefinition="Whether user is initiator", formalDefinition="Indicator that the user is or is not the requestor, or initiator, for the event being audited." ) 524 protected BooleanType requestor; 525 526 /** 527 * Where the event occurred. 528 */ 529 @Child(name = "location", type = {Location.class}, order=7, min=0, max=1, modifier=false, summary=false) 530 @Description(shortDefinition="Where", formalDefinition="Where the event occurred." ) 531 protected Reference location; 532 533 /** 534 * The actual object that is the target of the reference (Where the event occurred.) 535 */ 536 protected Location locationTarget; 537 538 /** 539 * The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used. 540 */ 541 @Child(name = "policy", type = {UriType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 542 @Description(shortDefinition="Policy that authorized event", formalDefinition="The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used." ) 543 protected List<UriType> policy; 544 545 /** 546 * Type of media involved. Used when the event is about exporting/importing onto media. 547 */ 548 @Child(name = "media", type = {Coding.class}, order=9, min=0, max=1, modifier=false, summary=false) 549 @Description(shortDefinition="Type of media", formalDefinition="Type of media involved. Used when the event is about exporting/importing onto media." ) 550 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/dicm-405-mediatype") 551 protected Coding media; 552 553 /** 554 * Logical network location for application activity, if the activity has a network location. 555 */ 556 @Child(name = "network", type = {}, order=10, min=0, max=1, modifier=false, summary=false) 557 @Description(shortDefinition="Logical network location for application activity", formalDefinition="Logical network location for application activity, if the activity has a network location." ) 558 protected AuditEventAgentNetworkComponent network; 559 560 /** 561 * The reason (purpose of use), specific to this agent, that was used during the event being recorded. 562 */ 563 @Child(name = "purposeOfUse", type = {CodeableConcept.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 564 @Description(shortDefinition="Reason given for this user", formalDefinition="The reason (purpose of use), specific to this agent, that was used during the event being recorded." ) 565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 566 protected List<CodeableConcept> purposeOfUse; 567 568 private static final long serialVersionUID = 1205071410L; 569 570 /** 571 * Constructor 572 */ 573 public AuditEventAgentComponent() { 574 super(); 575 } 576 577 /** 578 * Constructor 579 */ 580 public AuditEventAgentComponent(BooleanType requestor) { 581 super(); 582 this.requestor = requestor; 583 } 584 585 /** 586 * @return {@link #role} (The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.) 587 */ 588 public List<CodeableConcept> getRole() { 589 if (this.role == null) 590 this.role = new ArrayList<CodeableConcept>(); 591 return this.role; 592 } 593 594 /** 595 * @return Returns a reference to <code>this</code> for easy method chaining 596 */ 597 public AuditEventAgentComponent setRole(List<CodeableConcept> theRole) { 598 this.role = theRole; 599 return this; 600 } 601 602 public boolean hasRole() { 603 if (this.role == null) 604 return false; 605 for (CodeableConcept item : this.role) 606 if (!item.isEmpty()) 607 return true; 608 return false; 609 } 610 611 public CodeableConcept addRole() { //3 612 CodeableConcept t = new CodeableConcept(); 613 if (this.role == null) 614 this.role = new ArrayList<CodeableConcept>(); 615 this.role.add(t); 616 return t; 617 } 618 619 public AuditEventAgentComponent addRole(CodeableConcept t) { //3 620 if (t == null) 621 return this; 622 if (this.role == null) 623 this.role = new ArrayList<CodeableConcept>(); 624 this.role.add(t); 625 return this; 626 } 627 628 /** 629 * @return The first repetition of repeating field {@link #role}, creating it if it does not already exist 630 */ 631 public CodeableConcept getRoleFirstRep() { 632 if (getRole().isEmpty()) { 633 addRole(); 634 } 635 return getRole().get(0); 636 } 637 638 /** 639 * @return {@link #reference} (Direct reference to a resource that identifies the agent.) 640 */ 641 public Reference getReference() { 642 if (this.reference == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create AuditEventAgentComponent.reference"); 645 else if (Configuration.doAutoCreate()) 646 this.reference = new Reference(); // cc 647 return this.reference; 648 } 649 650 public boolean hasReference() { 651 return this.reference != null && !this.reference.isEmpty(); 652 } 653 654 /** 655 * @param value {@link #reference} (Direct reference to a resource that identifies the agent.) 656 */ 657 public AuditEventAgentComponent setReference(Reference value) { 658 this.reference = value; 659 return this; 660 } 661 662 /** 663 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Direct reference to a resource that identifies the agent.) 664 */ 665 public Resource getReferenceTarget() { 666 return this.referenceTarget; 667 } 668 669 /** 670 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Direct reference to a resource that identifies the agent.) 671 */ 672 public AuditEventAgentComponent setReferenceTarget(Resource value) { 673 this.referenceTarget = value; 674 return this; 675 } 676 677 /** 678 * @return {@link #userId} (Unique identifier for the user actively participating in the event.) 679 */ 680 public Identifier getUserId() { 681 if (this.userId == null) 682 if (Configuration.errorOnAutoCreate()) 683 throw new Error("Attempt to auto-create AuditEventAgentComponent.userId"); 684 else if (Configuration.doAutoCreate()) 685 this.userId = new Identifier(); // cc 686 return this.userId; 687 } 688 689 public boolean hasUserId() { 690 return this.userId != null && !this.userId.isEmpty(); 691 } 692 693 /** 694 * @param value {@link #userId} (Unique identifier for the user actively participating in the event.) 695 */ 696 public AuditEventAgentComponent setUserId(Identifier value) { 697 this.userId = value; 698 return this; 699 } 700 701 /** 702 * @return {@link #altId} (Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.). This is the underlying object with id, value and extensions. The accessor "getAltId" gives direct access to the value 703 */ 704 public StringType getAltIdElement() { 705 if (this.altId == null) 706 if (Configuration.errorOnAutoCreate()) 707 throw new Error("Attempt to auto-create AuditEventAgentComponent.altId"); 708 else if (Configuration.doAutoCreate()) 709 this.altId = new StringType(); // bb 710 return this.altId; 711 } 712 713 public boolean hasAltIdElement() { 714 return this.altId != null && !this.altId.isEmpty(); 715 } 716 717 public boolean hasAltId() { 718 return this.altId != null && !this.altId.isEmpty(); 719 } 720 721 /** 722 * @param value {@link #altId} (Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.). This is the underlying object with id, value and extensions. The accessor "getAltId" gives direct access to the value 723 */ 724 public AuditEventAgentComponent setAltIdElement(StringType value) { 725 this.altId = value; 726 return this; 727 } 728 729 /** 730 * @return Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available. 731 */ 732 public String getAltId() { 733 return this.altId == null ? null : this.altId.getValue(); 734 } 735 736 /** 737 * @param value Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available. 738 */ 739 public AuditEventAgentComponent setAltId(String value) { 740 if (Utilities.noString(value)) 741 this.altId = null; 742 else { 743 if (this.altId == null) 744 this.altId = new StringType(); 745 this.altId.setValue(value); 746 } 747 return this; 748 } 749 750 /** 751 * @return {@link #name} (Human-meaningful name for the agent.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 752 */ 753 public StringType getNameElement() { 754 if (this.name == null) 755 if (Configuration.errorOnAutoCreate()) 756 throw new Error("Attempt to auto-create AuditEventAgentComponent.name"); 757 else if (Configuration.doAutoCreate()) 758 this.name = new StringType(); // bb 759 return this.name; 760 } 761 762 public boolean hasNameElement() { 763 return this.name != null && !this.name.isEmpty(); 764 } 765 766 public boolean hasName() { 767 return this.name != null && !this.name.isEmpty(); 768 } 769 770 /** 771 * @param value {@link #name} (Human-meaningful name for the agent.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 772 */ 773 public AuditEventAgentComponent setNameElement(StringType value) { 774 this.name = value; 775 return this; 776 } 777 778 /** 779 * @return Human-meaningful name for the agent. 780 */ 781 public String getName() { 782 return this.name == null ? null : this.name.getValue(); 783 } 784 785 /** 786 * @param value Human-meaningful name for the agent. 787 */ 788 public AuditEventAgentComponent setName(String value) { 789 if (Utilities.noString(value)) 790 this.name = null; 791 else { 792 if (this.name == null) 793 this.name = new StringType(); 794 this.name.setValue(value); 795 } 796 return this; 797 } 798 799 /** 800 * @return {@link #requestor} (Indicator that the user is or is not the requestor, or initiator, for the event being audited.). This is the underlying object with id, value and extensions. The accessor "getRequestor" gives direct access to the value 801 */ 802 public BooleanType getRequestorElement() { 803 if (this.requestor == null) 804 if (Configuration.errorOnAutoCreate()) 805 throw new Error("Attempt to auto-create AuditEventAgentComponent.requestor"); 806 else if (Configuration.doAutoCreate()) 807 this.requestor = new BooleanType(); // bb 808 return this.requestor; 809 } 810 811 public boolean hasRequestorElement() { 812 return this.requestor != null && !this.requestor.isEmpty(); 813 } 814 815 public boolean hasRequestor() { 816 return this.requestor != null && !this.requestor.isEmpty(); 817 } 818 819 /** 820 * @param value {@link #requestor} (Indicator that the user is or is not the requestor, or initiator, for the event being audited.). This is the underlying object with id, value and extensions. The accessor "getRequestor" gives direct access to the value 821 */ 822 public AuditEventAgentComponent setRequestorElement(BooleanType value) { 823 this.requestor = value; 824 return this; 825 } 826 827 /** 828 * @return Indicator that the user is or is not the requestor, or initiator, for the event being audited. 829 */ 830 public boolean getRequestor() { 831 return this.requestor == null || this.requestor.isEmpty() ? false : this.requestor.getValue(); 832 } 833 834 /** 835 * @param value Indicator that the user is or is not the requestor, or initiator, for the event being audited. 836 */ 837 public AuditEventAgentComponent setRequestor(boolean value) { 838 if (this.requestor == null) 839 this.requestor = new BooleanType(); 840 this.requestor.setValue(value); 841 return this; 842 } 843 844 /** 845 * @return {@link #location} (Where the event occurred.) 846 */ 847 public Reference getLocation() { 848 if (this.location == null) 849 if (Configuration.errorOnAutoCreate()) 850 throw new Error("Attempt to auto-create AuditEventAgentComponent.location"); 851 else if (Configuration.doAutoCreate()) 852 this.location = new Reference(); // cc 853 return this.location; 854 } 855 856 public boolean hasLocation() { 857 return this.location != null && !this.location.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #location} (Where the event occurred.) 862 */ 863 public AuditEventAgentComponent setLocation(Reference value) { 864 this.location = value; 865 return this; 866 } 867 868 /** 869 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Where the event occurred.) 870 */ 871 public Location getLocationTarget() { 872 if (this.locationTarget == null) 873 if (Configuration.errorOnAutoCreate()) 874 throw new Error("Attempt to auto-create AuditEventAgentComponent.location"); 875 else if (Configuration.doAutoCreate()) 876 this.locationTarget = new Location(); // aa 877 return this.locationTarget; 878 } 879 880 /** 881 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Where the event occurred.) 882 */ 883 public AuditEventAgentComponent setLocationTarget(Location value) { 884 this.locationTarget = value; 885 return this; 886 } 887 888 /** 889 * @return {@link #policy} (The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 890 */ 891 public List<UriType> getPolicy() { 892 if (this.policy == null) 893 this.policy = new ArrayList<UriType>(); 894 return this.policy; 895 } 896 897 /** 898 * @return Returns a reference to <code>this</code> for easy method chaining 899 */ 900 public AuditEventAgentComponent setPolicy(List<UriType> thePolicy) { 901 this.policy = thePolicy; 902 return this; 903 } 904 905 public boolean hasPolicy() { 906 if (this.policy == null) 907 return false; 908 for (UriType item : this.policy) 909 if (!item.isEmpty()) 910 return true; 911 return false; 912 } 913 914 /** 915 * @return {@link #policy} (The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 916 */ 917 public UriType addPolicyElement() {//2 918 UriType t = new UriType(); 919 if (this.policy == null) 920 this.policy = new ArrayList<UriType>(); 921 this.policy.add(t); 922 return t; 923 } 924 925 /** 926 * @param value {@link #policy} (The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 927 */ 928 public AuditEventAgentComponent addPolicy(String value) { //1 929 UriType t = new UriType(); 930 t.setValue(value); 931 if (this.policy == null) 932 this.policy = new ArrayList<UriType>(); 933 this.policy.add(t); 934 return this; 935 } 936 937 /** 938 * @param value {@link #policy} (The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 939 */ 940 public boolean hasPolicy(String value) { 941 if (this.policy == null) 942 return false; 943 for (UriType v : this.policy) 944 if (v.getValue().equals(value)) // uri 945 return true; 946 return false; 947 } 948 949 /** 950 * @return {@link #media} (Type of media involved. Used when the event is about exporting/importing onto media.) 951 */ 952 public Coding getMedia() { 953 if (this.media == null) 954 if (Configuration.errorOnAutoCreate()) 955 throw new Error("Attempt to auto-create AuditEventAgentComponent.media"); 956 else if (Configuration.doAutoCreate()) 957 this.media = new Coding(); // cc 958 return this.media; 959 } 960 961 public boolean hasMedia() { 962 return this.media != null && !this.media.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #media} (Type of media involved. Used when the event is about exporting/importing onto media.) 967 */ 968 public AuditEventAgentComponent setMedia(Coding value) { 969 this.media = value; 970 return this; 971 } 972 973 /** 974 * @return {@link #network} (Logical network location for application activity, if the activity has a network location.) 975 */ 976 public AuditEventAgentNetworkComponent getNetwork() { 977 if (this.network == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create AuditEventAgentComponent.network"); 980 else if (Configuration.doAutoCreate()) 981 this.network = new AuditEventAgentNetworkComponent(); // cc 982 return this.network; 983 } 984 985 public boolean hasNetwork() { 986 return this.network != null && !this.network.isEmpty(); 987 } 988 989 /** 990 * @param value {@link #network} (Logical network location for application activity, if the activity has a network location.) 991 */ 992 public AuditEventAgentComponent setNetwork(AuditEventAgentNetworkComponent value) { 993 this.network = value; 994 return this; 995 } 996 997 /** 998 * @return {@link #purposeOfUse} (The reason (purpose of use), specific to this agent, that was used during the event being recorded.) 999 */ 1000 public List<CodeableConcept> getPurposeOfUse() { 1001 if (this.purposeOfUse == null) 1002 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1003 return this.purposeOfUse; 1004 } 1005 1006 /** 1007 * @return Returns a reference to <code>this</code> for easy method chaining 1008 */ 1009 public AuditEventAgentComponent setPurposeOfUse(List<CodeableConcept> thePurposeOfUse) { 1010 this.purposeOfUse = thePurposeOfUse; 1011 return this; 1012 } 1013 1014 public boolean hasPurposeOfUse() { 1015 if (this.purposeOfUse == null) 1016 return false; 1017 for (CodeableConcept item : this.purposeOfUse) 1018 if (!item.isEmpty()) 1019 return true; 1020 return false; 1021 } 1022 1023 public CodeableConcept addPurposeOfUse() { //3 1024 CodeableConcept t = new CodeableConcept(); 1025 if (this.purposeOfUse == null) 1026 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1027 this.purposeOfUse.add(t); 1028 return t; 1029 } 1030 1031 public AuditEventAgentComponent addPurposeOfUse(CodeableConcept t) { //3 1032 if (t == null) 1033 return this; 1034 if (this.purposeOfUse == null) 1035 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1036 this.purposeOfUse.add(t); 1037 return this; 1038 } 1039 1040 /** 1041 * @return The first repetition of repeating field {@link #purposeOfUse}, creating it if it does not already exist 1042 */ 1043 public CodeableConcept getPurposeOfUseFirstRep() { 1044 if (getPurposeOfUse().isEmpty()) { 1045 addPurposeOfUse(); 1046 } 1047 return getPurposeOfUse().get(0); 1048 } 1049 1050 protected void listChildren(List<Property> children) { 1051 super.listChildren(children); 1052 children.add(new Property("role", "CodeableConcept", "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.", 0, java.lang.Integer.MAX_VALUE, role)); 1053 children.add(new Property("reference", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", "Direct reference to a resource that identifies the agent.", 0, 1, reference)); 1054 children.add(new Property("userId", "Identifier", "Unique identifier for the user actively participating in the event.", 0, 1, userId)); 1055 children.add(new Property("altId", "string", "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.", 0, 1, altId)); 1056 children.add(new Property("name", "string", "Human-meaningful name for the agent.", 0, 1, name)); 1057 children.add(new Property("requestor", "boolean", "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, requestor)); 1058 children.add(new Property("location", "Reference(Location)", "Where the event occurred.", 0, 1, location)); 1059 children.add(new Property("policy", "uri", "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 0, java.lang.Integer.MAX_VALUE, policy)); 1060 children.add(new Property("media", "Coding", "Type of media involved. Used when the event is about exporting/importing onto media.", 0, 1, media)); 1061 children.add(new Property("network", "", "Logical network location for application activity, if the activity has a network location.", 0, 1, network)); 1062 children.add(new Property("purposeOfUse", "CodeableConcept", "The reason (purpose of use), specific to this agent, that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, purposeOfUse)); 1063 } 1064 1065 @Override 1066 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1067 switch (_hash) { 1068 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.", 0, java.lang.Integer.MAX_VALUE, role); 1069 case -925155509: /*reference*/ return new Property("reference", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", "Direct reference to a resource that identifies the agent.", 0, 1, reference); 1070 case -836030906: /*userId*/ return new Property("userId", "Identifier", "Unique identifier for the user actively participating in the event.", 0, 1, userId); 1071 case 92912804: /*altId*/ return new Property("altId", "string", "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.", 0, 1, altId); 1072 case 3373707: /*name*/ return new Property("name", "string", "Human-meaningful name for the agent.", 0, 1, name); 1073 case 693934258: /*requestor*/ return new Property("requestor", "boolean", "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, requestor); 1074 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Where the event occurred.", 0, 1, location); 1075 case -982670030: /*policy*/ return new Property("policy", "uri", "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 0, java.lang.Integer.MAX_VALUE, policy); 1076 case 103772132: /*media*/ return new Property("media", "Coding", "Type of media involved. Used when the event is about exporting/importing onto media.", 0, 1, media); 1077 case 1843485230: /*network*/ return new Property("network", "", "Logical network location for application activity, if the activity has a network location.", 0, 1, network); 1078 case -1881902670: /*purposeOfUse*/ return new Property("purposeOfUse", "CodeableConcept", "The reason (purpose of use), specific to this agent, that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, purposeOfUse); 1079 default: return super.getNamedProperty(_hash, _name, _checkValid); 1080 } 1081 1082 } 1083 1084 @Override 1085 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1086 switch (hash) { 1087 case 3506294: /*role*/ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 1088 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1089 case -836030906: /*userId*/ return this.userId == null ? new Base[0] : new Base[] {this.userId}; // Identifier 1090 case 92912804: /*altId*/ return this.altId == null ? new Base[0] : new Base[] {this.altId}; // StringType 1091 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1092 case 693934258: /*requestor*/ return this.requestor == null ? new Base[0] : new Base[] {this.requestor}; // BooleanType 1093 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1094 case -982670030: /*policy*/ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // UriType 1095 case 103772132: /*media*/ return this.media == null ? new Base[0] : new Base[] {this.media}; // Coding 1096 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // AuditEventAgentNetworkComponent 1097 case -1881902670: /*purposeOfUse*/ return this.purposeOfUse == null ? new Base[0] : this.purposeOfUse.toArray(new Base[this.purposeOfUse.size()]); // CodeableConcept 1098 default: return super.getProperty(hash, name, checkValid); 1099 } 1100 1101 } 1102 1103 @Override 1104 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1105 switch (hash) { 1106 case 3506294: // role 1107 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 1108 return value; 1109 case -925155509: // reference 1110 this.reference = castToReference(value); // Reference 1111 return value; 1112 case -836030906: // userId 1113 this.userId = castToIdentifier(value); // Identifier 1114 return value; 1115 case 92912804: // altId 1116 this.altId = castToString(value); // StringType 1117 return value; 1118 case 3373707: // name 1119 this.name = castToString(value); // StringType 1120 return value; 1121 case 693934258: // requestor 1122 this.requestor = castToBoolean(value); // BooleanType 1123 return value; 1124 case 1901043637: // location 1125 this.location = castToReference(value); // Reference 1126 return value; 1127 case -982670030: // policy 1128 this.getPolicy().add(castToUri(value)); // UriType 1129 return value; 1130 case 103772132: // media 1131 this.media = castToCoding(value); // Coding 1132 return value; 1133 case 1843485230: // network 1134 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1135 return value; 1136 case -1881902670: // purposeOfUse 1137 this.getPurposeOfUse().add(castToCodeableConcept(value)); // CodeableConcept 1138 return value; 1139 default: return super.setProperty(hash, name, value); 1140 } 1141 1142 } 1143 1144 @Override 1145 public Base setProperty(String name, Base value) throws FHIRException { 1146 if (name.equals("role")) { 1147 this.getRole().add(castToCodeableConcept(value)); 1148 } else if (name.equals("reference")) { 1149 this.reference = castToReference(value); // Reference 1150 } else if (name.equals("userId")) { 1151 this.userId = castToIdentifier(value); // Identifier 1152 } else if (name.equals("altId")) { 1153 this.altId = castToString(value); // StringType 1154 } else if (name.equals("name")) { 1155 this.name = castToString(value); // StringType 1156 } else if (name.equals("requestor")) { 1157 this.requestor = castToBoolean(value); // BooleanType 1158 } else if (name.equals("location")) { 1159 this.location = castToReference(value); // Reference 1160 } else if (name.equals("policy")) { 1161 this.getPolicy().add(castToUri(value)); 1162 } else if (name.equals("media")) { 1163 this.media = castToCoding(value); // Coding 1164 } else if (name.equals("network")) { 1165 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1166 } else if (name.equals("purposeOfUse")) { 1167 this.getPurposeOfUse().add(castToCodeableConcept(value)); 1168 } else 1169 return super.setProperty(name, value); 1170 return value; 1171 } 1172 1173 @Override 1174 public Base makeProperty(int hash, String name) throws FHIRException { 1175 switch (hash) { 1176 case 3506294: return addRole(); 1177 case -925155509: return getReference(); 1178 case -836030906: return getUserId(); 1179 case 92912804: return getAltIdElement(); 1180 case 3373707: return getNameElement(); 1181 case 693934258: return getRequestorElement(); 1182 case 1901043637: return getLocation(); 1183 case -982670030: return addPolicyElement(); 1184 case 103772132: return getMedia(); 1185 case 1843485230: return getNetwork(); 1186 case -1881902670: return addPurposeOfUse(); 1187 default: return super.makeProperty(hash, name); 1188 } 1189 1190 } 1191 1192 @Override 1193 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1194 switch (hash) { 1195 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1196 case -925155509: /*reference*/ return new String[] {"Reference"}; 1197 case -836030906: /*userId*/ return new String[] {"Identifier"}; 1198 case 92912804: /*altId*/ return new String[] {"string"}; 1199 case 3373707: /*name*/ return new String[] {"string"}; 1200 case 693934258: /*requestor*/ return new String[] {"boolean"}; 1201 case 1901043637: /*location*/ return new String[] {"Reference"}; 1202 case -982670030: /*policy*/ return new String[] {"uri"}; 1203 case 103772132: /*media*/ return new String[] {"Coding"}; 1204 case 1843485230: /*network*/ return new String[] {}; 1205 case -1881902670: /*purposeOfUse*/ return new String[] {"CodeableConcept"}; 1206 default: return super.getTypesForProperty(hash, name); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base addChild(String name) throws FHIRException { 1213 if (name.equals("role")) { 1214 return addRole(); 1215 } 1216 else if (name.equals("reference")) { 1217 this.reference = new Reference(); 1218 return this.reference; 1219 } 1220 else if (name.equals("userId")) { 1221 this.userId = new Identifier(); 1222 return this.userId; 1223 } 1224 else if (name.equals("altId")) { 1225 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.altId"); 1226 } 1227 else if (name.equals("name")) { 1228 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 1229 } 1230 else if (name.equals("requestor")) { 1231 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.requestor"); 1232 } 1233 else if (name.equals("location")) { 1234 this.location = new Reference(); 1235 return this.location; 1236 } 1237 else if (name.equals("policy")) { 1238 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.policy"); 1239 } 1240 else if (name.equals("media")) { 1241 this.media = new Coding(); 1242 return this.media; 1243 } 1244 else if (name.equals("network")) { 1245 this.network = new AuditEventAgentNetworkComponent(); 1246 return this.network; 1247 } 1248 else if (name.equals("purposeOfUse")) { 1249 return addPurposeOfUse(); 1250 } 1251 else 1252 return super.addChild(name); 1253 } 1254 1255 public AuditEventAgentComponent copy() { 1256 AuditEventAgentComponent dst = new AuditEventAgentComponent(); 1257 copyValues(dst); 1258 if (role != null) { 1259 dst.role = new ArrayList<CodeableConcept>(); 1260 for (CodeableConcept i : role) 1261 dst.role.add(i.copy()); 1262 }; 1263 dst.reference = reference == null ? null : reference.copy(); 1264 dst.userId = userId == null ? null : userId.copy(); 1265 dst.altId = altId == null ? null : altId.copy(); 1266 dst.name = name == null ? null : name.copy(); 1267 dst.requestor = requestor == null ? null : requestor.copy(); 1268 dst.location = location == null ? null : location.copy(); 1269 if (policy != null) { 1270 dst.policy = new ArrayList<UriType>(); 1271 for (UriType i : policy) 1272 dst.policy.add(i.copy()); 1273 }; 1274 dst.media = media == null ? null : media.copy(); 1275 dst.network = network == null ? null : network.copy(); 1276 if (purposeOfUse != null) { 1277 dst.purposeOfUse = new ArrayList<CodeableConcept>(); 1278 for (CodeableConcept i : purposeOfUse) 1279 dst.purposeOfUse.add(i.copy()); 1280 }; 1281 return dst; 1282 } 1283 1284 @Override 1285 public boolean equalsDeep(Base other_) { 1286 if (!super.equalsDeep(other_)) 1287 return false; 1288 if (!(other_ instanceof AuditEventAgentComponent)) 1289 return false; 1290 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1291 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true) && compareDeep(userId, o.userId, true) 1292 && compareDeep(altId, o.altId, true) && compareDeep(name, o.name, true) && compareDeep(requestor, o.requestor, true) 1293 && compareDeep(location, o.location, true) && compareDeep(policy, o.policy, true) && compareDeep(media, o.media, true) 1294 && compareDeep(network, o.network, true) && compareDeep(purposeOfUse, o.purposeOfUse, true); 1295 } 1296 1297 @Override 1298 public boolean equalsShallow(Base other_) { 1299 if (!super.equalsShallow(other_)) 1300 return false; 1301 if (!(other_ instanceof AuditEventAgentComponent)) 1302 return false; 1303 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1304 return compareValues(altId, o.altId, true) && compareValues(name, o.name, true) && compareValues(requestor, o.requestor, true) 1305 && compareValues(policy, o.policy, true); 1306 } 1307 1308 public boolean isEmpty() { 1309 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference, userId 1310 , altId, name, requestor, location, policy, media, network, purposeOfUse); 1311 } 1312 1313 public String fhirType() { 1314 return "AuditEvent.agent"; 1315 1316 } 1317 1318 } 1319 1320 @Block() 1321 public static class AuditEventAgentNetworkComponent extends BackboneElement implements IBaseBackboneElement { 1322 /** 1323 * An identifier for the network access point of the user device for the audit event. 1324 */ 1325 @Child(name = "address", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1326 @Description(shortDefinition="Identifier for the network access point of the user device", formalDefinition="An identifier for the network access point of the user device for the audit event." ) 1327 protected StringType address; 1328 1329 /** 1330 * An identifier for the type of network access point that originated the audit event. 1331 */ 1332 @Child(name = "type", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1333 @Description(shortDefinition="The type of network access point", formalDefinition="An identifier for the type of network access point that originated the audit event." ) 1334 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/network-type") 1335 protected Enumeration<AuditEventAgentNetworkType> type; 1336 1337 private static final long serialVersionUID = -160715924L; 1338 1339 /** 1340 * Constructor 1341 */ 1342 public AuditEventAgentNetworkComponent() { 1343 super(); 1344 } 1345 1346 /** 1347 * @return {@link #address} (An identifier for the network access point of the user device for the audit event.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 1348 */ 1349 public StringType getAddressElement() { 1350 if (this.address == null) 1351 if (Configuration.errorOnAutoCreate()) 1352 throw new Error("Attempt to auto-create AuditEventAgentNetworkComponent.address"); 1353 else if (Configuration.doAutoCreate()) 1354 this.address = new StringType(); // bb 1355 return this.address; 1356 } 1357 1358 public boolean hasAddressElement() { 1359 return this.address != null && !this.address.isEmpty(); 1360 } 1361 1362 public boolean hasAddress() { 1363 return this.address != null && !this.address.isEmpty(); 1364 } 1365 1366 /** 1367 * @param value {@link #address} (An identifier for the network access point of the user device for the audit event.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 1368 */ 1369 public AuditEventAgentNetworkComponent setAddressElement(StringType value) { 1370 this.address = value; 1371 return this; 1372 } 1373 1374 /** 1375 * @return An identifier for the network access point of the user device for the audit event. 1376 */ 1377 public String getAddress() { 1378 return this.address == null ? null : this.address.getValue(); 1379 } 1380 1381 /** 1382 * @param value An identifier for the network access point of the user device for the audit event. 1383 */ 1384 public AuditEventAgentNetworkComponent setAddress(String value) { 1385 if (Utilities.noString(value)) 1386 this.address = null; 1387 else { 1388 if (this.address == null) 1389 this.address = new StringType(); 1390 this.address.setValue(value); 1391 } 1392 return this; 1393 } 1394 1395 /** 1396 * @return {@link #type} (An identifier for the type of network access point that originated the audit event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1397 */ 1398 public Enumeration<AuditEventAgentNetworkType> getTypeElement() { 1399 if (this.type == null) 1400 if (Configuration.errorOnAutoCreate()) 1401 throw new Error("Attempt to auto-create AuditEventAgentNetworkComponent.type"); 1402 else if (Configuration.doAutoCreate()) 1403 this.type = new Enumeration<AuditEventAgentNetworkType>(new AuditEventAgentNetworkTypeEnumFactory()); // bb 1404 return this.type; 1405 } 1406 1407 public boolean hasTypeElement() { 1408 return this.type != null && !this.type.isEmpty(); 1409 } 1410 1411 public boolean hasType() { 1412 return this.type != null && !this.type.isEmpty(); 1413 } 1414 1415 /** 1416 * @param value {@link #type} (An identifier for the type of network access point that originated the audit event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1417 */ 1418 public AuditEventAgentNetworkComponent setTypeElement(Enumeration<AuditEventAgentNetworkType> value) { 1419 this.type = value; 1420 return this; 1421 } 1422 1423 /** 1424 * @return An identifier for the type of network access point that originated the audit event. 1425 */ 1426 public AuditEventAgentNetworkType getType() { 1427 return this.type == null ? null : this.type.getValue(); 1428 } 1429 1430 /** 1431 * @param value An identifier for the type of network access point that originated the audit event. 1432 */ 1433 public AuditEventAgentNetworkComponent setType(AuditEventAgentNetworkType value) { 1434 if (value == null) 1435 this.type = null; 1436 else { 1437 if (this.type == null) 1438 this.type = new Enumeration<AuditEventAgentNetworkType>(new AuditEventAgentNetworkTypeEnumFactory()); 1439 this.type.setValue(value); 1440 } 1441 return this; 1442 } 1443 1444 protected void listChildren(List<Property> children) { 1445 super.listChildren(children); 1446 children.add(new Property("address", "string", "An identifier for the network access point of the user device for the audit event.", 0, 1, address)); 1447 children.add(new Property("type", "code", "An identifier for the type of network access point that originated the audit event.", 0, 1, type)); 1448 } 1449 1450 @Override 1451 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1452 switch (_hash) { 1453 case -1147692044: /*address*/ return new Property("address", "string", "An identifier for the network access point of the user device for the audit event.", 0, 1, address); 1454 case 3575610: /*type*/ return new Property("type", "code", "An identifier for the type of network access point that originated the audit event.", 0, 1, type); 1455 default: return super.getNamedProperty(_hash, _name, _checkValid); 1456 } 1457 1458 } 1459 1460 @Override 1461 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1462 switch (hash) { 1463 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // StringType 1464 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<AuditEventAgentNetworkType> 1465 default: return super.getProperty(hash, name, checkValid); 1466 } 1467 1468 } 1469 1470 @Override 1471 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1472 switch (hash) { 1473 case -1147692044: // address 1474 this.address = castToString(value); // StringType 1475 return value; 1476 case 3575610: // type 1477 value = new AuditEventAgentNetworkTypeEnumFactory().fromType(castToCode(value)); 1478 this.type = (Enumeration) value; // Enumeration<AuditEventAgentNetworkType> 1479 return value; 1480 default: return super.setProperty(hash, name, value); 1481 } 1482 1483 } 1484 1485 @Override 1486 public Base setProperty(String name, Base value) throws FHIRException { 1487 if (name.equals("address")) { 1488 this.address = castToString(value); // StringType 1489 } else if (name.equals("type")) { 1490 value = new AuditEventAgentNetworkTypeEnumFactory().fromType(castToCode(value)); 1491 this.type = (Enumeration) value; // Enumeration<AuditEventAgentNetworkType> 1492 } else 1493 return super.setProperty(name, value); 1494 return value; 1495 } 1496 1497 @Override 1498 public Base makeProperty(int hash, String name) throws FHIRException { 1499 switch (hash) { 1500 case -1147692044: return getAddressElement(); 1501 case 3575610: return getTypeElement(); 1502 default: return super.makeProperty(hash, name); 1503 } 1504 1505 } 1506 1507 @Override 1508 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1509 switch (hash) { 1510 case -1147692044: /*address*/ return new String[] {"string"}; 1511 case 3575610: /*type*/ return new String[] {"code"}; 1512 default: return super.getTypesForProperty(hash, name); 1513 } 1514 1515 } 1516 1517 @Override 1518 public Base addChild(String name) throws FHIRException { 1519 if (name.equals("address")) { 1520 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.address"); 1521 } 1522 else if (name.equals("type")) { 1523 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 1524 } 1525 else 1526 return super.addChild(name); 1527 } 1528 1529 public AuditEventAgentNetworkComponent copy() { 1530 AuditEventAgentNetworkComponent dst = new AuditEventAgentNetworkComponent(); 1531 copyValues(dst); 1532 dst.address = address == null ? null : address.copy(); 1533 dst.type = type == null ? null : type.copy(); 1534 return dst; 1535 } 1536 1537 @Override 1538 public boolean equalsDeep(Base other_) { 1539 if (!super.equalsDeep(other_)) 1540 return false; 1541 if (!(other_ instanceof AuditEventAgentNetworkComponent)) 1542 return false; 1543 AuditEventAgentNetworkComponent o = (AuditEventAgentNetworkComponent) other_; 1544 return compareDeep(address, o.address, true) && compareDeep(type, o.type, true); 1545 } 1546 1547 @Override 1548 public boolean equalsShallow(Base other_) { 1549 if (!super.equalsShallow(other_)) 1550 return false; 1551 if (!(other_ instanceof AuditEventAgentNetworkComponent)) 1552 return false; 1553 AuditEventAgentNetworkComponent o = (AuditEventAgentNetworkComponent) other_; 1554 return compareValues(address, o.address, true) && compareValues(type, o.type, true); 1555 } 1556 1557 public boolean isEmpty() { 1558 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(address, type); 1559 } 1560 1561 public String fhirType() { 1562 return "AuditEvent.agent.network"; 1563 1564 } 1565 1566 } 1567 1568 @Block() 1569 public static class AuditEventSourceComponent extends BackboneElement implements IBaseBackboneElement { 1570 /** 1571 * Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group. 1572 */ 1573 @Child(name = "site", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1574 @Description(shortDefinition="Logical source location within the enterprise", formalDefinition="Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group." ) 1575 protected StringType site; 1576 1577 /** 1578 * Identifier of the source where the event was detected. 1579 */ 1580 @Child(name = "identifier", type = {Identifier.class}, order=2, min=1, max=1, modifier=false, summary=true) 1581 @Description(shortDefinition="The identity of source detecting the event", formalDefinition="Identifier of the source where the event was detected." ) 1582 protected Identifier identifier; 1583 1584 /** 1585 * Code specifying the type of source where event originated. 1586 */ 1587 @Child(name = "type", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1588 @Description(shortDefinition="The type of source where event originated", formalDefinition="Code specifying the type of source where event originated." ) 1589 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-source-type") 1590 protected List<Coding> type; 1591 1592 private static final long serialVersionUID = -1562673890L; 1593 1594 /** 1595 * Constructor 1596 */ 1597 public AuditEventSourceComponent() { 1598 super(); 1599 } 1600 1601 /** 1602 * Constructor 1603 */ 1604 public AuditEventSourceComponent(Identifier identifier) { 1605 super(); 1606 this.identifier = identifier; 1607 } 1608 1609 /** 1610 * @return {@link #site} (Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.). This is the underlying object with id, value and extensions. The accessor "getSite" gives direct access to the value 1611 */ 1612 public StringType getSiteElement() { 1613 if (this.site == null) 1614 if (Configuration.errorOnAutoCreate()) 1615 throw new Error("Attempt to auto-create AuditEventSourceComponent.site"); 1616 else if (Configuration.doAutoCreate()) 1617 this.site = new StringType(); // bb 1618 return this.site; 1619 } 1620 1621 public boolean hasSiteElement() { 1622 return this.site != null && !this.site.isEmpty(); 1623 } 1624 1625 public boolean hasSite() { 1626 return this.site != null && !this.site.isEmpty(); 1627 } 1628 1629 /** 1630 * @param value {@link #site} (Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.). This is the underlying object with id, value and extensions. The accessor "getSite" gives direct access to the value 1631 */ 1632 public AuditEventSourceComponent setSiteElement(StringType value) { 1633 this.site = value; 1634 return this; 1635 } 1636 1637 /** 1638 * @return Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group. 1639 */ 1640 public String getSite() { 1641 return this.site == null ? null : this.site.getValue(); 1642 } 1643 1644 /** 1645 * @param value Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group. 1646 */ 1647 public AuditEventSourceComponent setSite(String value) { 1648 if (Utilities.noString(value)) 1649 this.site = null; 1650 else { 1651 if (this.site == null) 1652 this.site = new StringType(); 1653 this.site.setValue(value); 1654 } 1655 return this; 1656 } 1657 1658 /** 1659 * @return {@link #identifier} (Identifier of the source where the event was detected.) 1660 */ 1661 public Identifier getIdentifier() { 1662 if (this.identifier == null) 1663 if (Configuration.errorOnAutoCreate()) 1664 throw new Error("Attempt to auto-create AuditEventSourceComponent.identifier"); 1665 else if (Configuration.doAutoCreate()) 1666 this.identifier = new Identifier(); // cc 1667 return this.identifier; 1668 } 1669 1670 public boolean hasIdentifier() { 1671 return this.identifier != null && !this.identifier.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #identifier} (Identifier of the source where the event was detected.) 1676 */ 1677 public AuditEventSourceComponent setIdentifier(Identifier value) { 1678 this.identifier = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return {@link #type} (Code specifying the type of source where event originated.) 1684 */ 1685 public List<Coding> getType() { 1686 if (this.type == null) 1687 this.type = new ArrayList<Coding>(); 1688 return this.type; 1689 } 1690 1691 /** 1692 * @return Returns a reference to <code>this</code> for easy method chaining 1693 */ 1694 public AuditEventSourceComponent setType(List<Coding> theType) { 1695 this.type = theType; 1696 return this; 1697 } 1698 1699 public boolean hasType() { 1700 if (this.type == null) 1701 return false; 1702 for (Coding item : this.type) 1703 if (!item.isEmpty()) 1704 return true; 1705 return false; 1706 } 1707 1708 public Coding addType() { //3 1709 Coding t = new Coding(); 1710 if (this.type == null) 1711 this.type = new ArrayList<Coding>(); 1712 this.type.add(t); 1713 return t; 1714 } 1715 1716 public AuditEventSourceComponent addType(Coding t) { //3 1717 if (t == null) 1718 return this; 1719 if (this.type == null) 1720 this.type = new ArrayList<Coding>(); 1721 this.type.add(t); 1722 return this; 1723 } 1724 1725 /** 1726 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 1727 */ 1728 public Coding getTypeFirstRep() { 1729 if (getType().isEmpty()) { 1730 addType(); 1731 } 1732 return getType().get(0); 1733 } 1734 1735 protected void listChildren(List<Property> children) { 1736 super.listChildren(children); 1737 children.add(new Property("site", "string", "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 0, 1, site)); 1738 children.add(new Property("identifier", "Identifier", "Identifier of the source where the event was detected.", 0, 1, identifier)); 1739 children.add(new Property("type", "Coding", "Code specifying the type of source where event originated.", 0, java.lang.Integer.MAX_VALUE, type)); 1740 } 1741 1742 @Override 1743 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1744 switch (_hash) { 1745 case 3530567: /*site*/ return new Property("site", "string", "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 0, 1, site); 1746 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier of the source where the event was detected.", 0, 1, identifier); 1747 case 3575610: /*type*/ return new Property("type", "Coding", "Code specifying the type of source where event originated.", 0, java.lang.Integer.MAX_VALUE, type); 1748 default: return super.getNamedProperty(_hash, _name, _checkValid); 1749 } 1750 1751 } 1752 1753 @Override 1754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1755 switch (hash) { 1756 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // StringType 1757 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1758 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // Coding 1759 default: return super.getProperty(hash, name, checkValid); 1760 } 1761 1762 } 1763 1764 @Override 1765 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1766 switch (hash) { 1767 case 3530567: // site 1768 this.site = castToString(value); // StringType 1769 return value; 1770 case -1618432855: // identifier 1771 this.identifier = castToIdentifier(value); // Identifier 1772 return value; 1773 case 3575610: // type 1774 this.getType().add(castToCoding(value)); // Coding 1775 return value; 1776 default: return super.setProperty(hash, name, value); 1777 } 1778 1779 } 1780 1781 @Override 1782 public Base setProperty(String name, Base value) throws FHIRException { 1783 if (name.equals("site")) { 1784 this.site = castToString(value); // StringType 1785 } else if (name.equals("identifier")) { 1786 this.identifier = castToIdentifier(value); // Identifier 1787 } else if (name.equals("type")) { 1788 this.getType().add(castToCoding(value)); 1789 } else 1790 return super.setProperty(name, value); 1791 return value; 1792 } 1793 1794 @Override 1795 public Base makeProperty(int hash, String name) throws FHIRException { 1796 switch (hash) { 1797 case 3530567: return getSiteElement(); 1798 case -1618432855: return getIdentifier(); 1799 case 3575610: return addType(); 1800 default: return super.makeProperty(hash, name); 1801 } 1802 1803 } 1804 1805 @Override 1806 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1807 switch (hash) { 1808 case 3530567: /*site*/ return new String[] {"string"}; 1809 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1810 case 3575610: /*type*/ return new String[] {"Coding"}; 1811 default: return super.getTypesForProperty(hash, name); 1812 } 1813 1814 } 1815 1816 @Override 1817 public Base addChild(String name) throws FHIRException { 1818 if (name.equals("site")) { 1819 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.site"); 1820 } 1821 else if (name.equals("identifier")) { 1822 this.identifier = new Identifier(); 1823 return this.identifier; 1824 } 1825 else if (name.equals("type")) { 1826 return addType(); 1827 } 1828 else 1829 return super.addChild(name); 1830 } 1831 1832 public AuditEventSourceComponent copy() { 1833 AuditEventSourceComponent dst = new AuditEventSourceComponent(); 1834 copyValues(dst); 1835 dst.site = site == null ? null : site.copy(); 1836 dst.identifier = identifier == null ? null : identifier.copy(); 1837 if (type != null) { 1838 dst.type = new ArrayList<Coding>(); 1839 for (Coding i : type) 1840 dst.type.add(i.copy()); 1841 }; 1842 return dst; 1843 } 1844 1845 @Override 1846 public boolean equalsDeep(Base other_) { 1847 if (!super.equalsDeep(other_)) 1848 return false; 1849 if (!(other_ instanceof AuditEventSourceComponent)) 1850 return false; 1851 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 1852 return compareDeep(site, o.site, true) && compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 1853 ; 1854 } 1855 1856 @Override 1857 public boolean equalsShallow(Base other_) { 1858 if (!super.equalsShallow(other_)) 1859 return false; 1860 if (!(other_ instanceof AuditEventSourceComponent)) 1861 return false; 1862 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 1863 return compareValues(site, o.site, true); 1864 } 1865 1866 public boolean isEmpty() { 1867 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, identifier, type); 1868 } 1869 1870 public String fhirType() { 1871 return "AuditEvent.source"; 1872 1873 } 1874 1875 } 1876 1877 @Block() 1878 public static class AuditEventEntityComponent extends BackboneElement implements IBaseBackboneElement { 1879 /** 1880 * Identifies a specific instance of the entity. The reference should always be version specific. 1881 */ 1882 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1883 @Description(shortDefinition="Specific instance of object", formalDefinition="Identifies a specific instance of the entity. The reference should always be version specific." ) 1884 protected Identifier identifier; 1885 1886 /** 1887 * Identifies a specific instance of the entity. The reference should be version specific. 1888 */ 1889 @Child(name = "reference", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=true) 1890 @Description(shortDefinition="Specific instance of resource", formalDefinition="Identifies a specific instance of the entity. The reference should be version specific." ) 1891 protected Reference reference; 1892 1893 /** 1894 * The actual object that is the target of the reference (Identifies a specific instance of the entity. The reference should be version specific.) 1895 */ 1896 protected Resource referenceTarget; 1897 1898 /** 1899 * The type of the object that was involved in this audit event. 1900 */ 1901 @Child(name = "type", type = {Coding.class}, order=3, min=0, max=1, modifier=false, summary=false) 1902 @Description(shortDefinition="Type of entity involved", formalDefinition="The type of the object that was involved in this audit event." ) 1903 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-entity-type") 1904 protected Coding type; 1905 1906 /** 1907 * Code representing the role the entity played in the event being audited. 1908 */ 1909 @Child(name = "role", type = {Coding.class}, order=4, min=0, max=1, modifier=false, summary=false) 1910 @Description(shortDefinition="What role the entity played", formalDefinition="Code representing the role the entity played in the event being audited." ) 1911 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/object-role") 1912 protected Coding role; 1913 1914 /** 1915 * Identifier for the data life-cycle stage for the entity. 1916 */ 1917 @Child(name = "lifecycle", type = {Coding.class}, order=5, min=0, max=1, modifier=false, summary=false) 1918 @Description(shortDefinition="Life-cycle stage for the entity", formalDefinition="Identifier for the data life-cycle stage for the entity." ) 1919 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/object-lifecycle-events") 1920 protected Coding lifecycle; 1921 1922 /** 1923 * Security labels for the identified entity. 1924 */ 1925 @Child(name = "securityLabel", type = {Coding.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1926 @Description(shortDefinition="Security labels on the entity", formalDefinition="Security labels for the identified entity." ) 1927 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 1928 protected List<Coding> securityLabel; 1929 1930 /** 1931 * A name of the entity in the audit event. 1932 */ 1933 @Child(name = "name", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1934 @Description(shortDefinition="Descriptor for entity", formalDefinition="A name of the entity in the audit event." ) 1935 protected StringType name; 1936 1937 /** 1938 * Text that describes the entity in more detail. 1939 */ 1940 @Child(name = "description", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1941 @Description(shortDefinition="Descriptive text", formalDefinition="Text that describes the entity in more detail." ) 1942 protected StringType description; 1943 1944 /** 1945 * The query parameters for a query-type entities. 1946 */ 1947 @Child(name = "query", type = {Base64BinaryType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1948 @Description(shortDefinition="Query parameters", formalDefinition="The query parameters for a query-type entities." ) 1949 protected Base64BinaryType query; 1950 1951 /** 1952 * Tagged value pairs for conveying additional information about the entity. 1953 */ 1954 @Child(name = "detail", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1955 @Description(shortDefinition="Additional Information about the entity", formalDefinition="Tagged value pairs for conveying additional information about the entity." ) 1956 protected List<AuditEventEntityDetailComponent> detail; 1957 1958 private static final long serialVersionUID = -1393424632L; 1959 1960 /** 1961 * Constructor 1962 */ 1963 public AuditEventEntityComponent() { 1964 super(); 1965 } 1966 1967 /** 1968 * @return {@link #identifier} (Identifies a specific instance of the entity. The reference should always be version specific.) 1969 */ 1970 public Identifier getIdentifier() { 1971 if (this.identifier == null) 1972 if (Configuration.errorOnAutoCreate()) 1973 throw new Error("Attempt to auto-create AuditEventEntityComponent.identifier"); 1974 else if (Configuration.doAutoCreate()) 1975 this.identifier = new Identifier(); // cc 1976 return this.identifier; 1977 } 1978 1979 public boolean hasIdentifier() { 1980 return this.identifier != null && !this.identifier.isEmpty(); 1981 } 1982 1983 /** 1984 * @param value {@link #identifier} (Identifies a specific instance of the entity. The reference should always be version specific.) 1985 */ 1986 public AuditEventEntityComponent setIdentifier(Identifier value) { 1987 this.identifier = value; 1988 return this; 1989 } 1990 1991 /** 1992 * @return {@link #reference} (Identifies a specific instance of the entity. The reference should be version specific.) 1993 */ 1994 public Reference getReference() { 1995 if (this.reference == null) 1996 if (Configuration.errorOnAutoCreate()) 1997 throw new Error("Attempt to auto-create AuditEventEntityComponent.reference"); 1998 else if (Configuration.doAutoCreate()) 1999 this.reference = new Reference(); // cc 2000 return this.reference; 2001 } 2002 2003 public boolean hasReference() { 2004 return this.reference != null && !this.reference.isEmpty(); 2005 } 2006 2007 /** 2008 * @param value {@link #reference} (Identifies a specific instance of the entity. The reference should be version specific.) 2009 */ 2010 public AuditEventEntityComponent setReference(Reference value) { 2011 this.reference = value; 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies a specific instance of the entity. The reference should be version specific.) 2017 */ 2018 public Resource getReferenceTarget() { 2019 return this.referenceTarget; 2020 } 2021 2022 /** 2023 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies a specific instance of the entity. The reference should be version specific.) 2024 */ 2025 public AuditEventEntityComponent setReferenceTarget(Resource value) { 2026 this.referenceTarget = value; 2027 return this; 2028 } 2029 2030 /** 2031 * @return {@link #type} (The type of the object that was involved in this audit event.) 2032 */ 2033 public Coding getType() { 2034 if (this.type == null) 2035 if (Configuration.errorOnAutoCreate()) 2036 throw new Error("Attempt to auto-create AuditEventEntityComponent.type"); 2037 else if (Configuration.doAutoCreate()) 2038 this.type = new Coding(); // cc 2039 return this.type; 2040 } 2041 2042 public boolean hasType() { 2043 return this.type != null && !this.type.isEmpty(); 2044 } 2045 2046 /** 2047 * @param value {@link #type} (The type of the object that was involved in this audit event.) 2048 */ 2049 public AuditEventEntityComponent setType(Coding value) { 2050 this.type = value; 2051 return this; 2052 } 2053 2054 /** 2055 * @return {@link #role} (Code representing the role the entity played in the event being audited.) 2056 */ 2057 public Coding getRole() { 2058 if (this.role == null) 2059 if (Configuration.errorOnAutoCreate()) 2060 throw new Error("Attempt to auto-create AuditEventEntityComponent.role"); 2061 else if (Configuration.doAutoCreate()) 2062 this.role = new Coding(); // cc 2063 return this.role; 2064 } 2065 2066 public boolean hasRole() { 2067 return this.role != null && !this.role.isEmpty(); 2068 } 2069 2070 /** 2071 * @param value {@link #role} (Code representing the role the entity played in the event being audited.) 2072 */ 2073 public AuditEventEntityComponent setRole(Coding value) { 2074 this.role = value; 2075 return this; 2076 } 2077 2078 /** 2079 * @return {@link #lifecycle} (Identifier for the data life-cycle stage for the entity.) 2080 */ 2081 public Coding getLifecycle() { 2082 if (this.lifecycle == null) 2083 if (Configuration.errorOnAutoCreate()) 2084 throw new Error("Attempt to auto-create AuditEventEntityComponent.lifecycle"); 2085 else if (Configuration.doAutoCreate()) 2086 this.lifecycle = new Coding(); // cc 2087 return this.lifecycle; 2088 } 2089 2090 public boolean hasLifecycle() { 2091 return this.lifecycle != null && !this.lifecycle.isEmpty(); 2092 } 2093 2094 /** 2095 * @param value {@link #lifecycle} (Identifier for the data life-cycle stage for the entity.) 2096 */ 2097 public AuditEventEntityComponent setLifecycle(Coding value) { 2098 this.lifecycle = value; 2099 return this; 2100 } 2101 2102 /** 2103 * @return {@link #securityLabel} (Security labels for the identified entity.) 2104 */ 2105 public List<Coding> getSecurityLabel() { 2106 if (this.securityLabel == null) 2107 this.securityLabel = new ArrayList<Coding>(); 2108 return this.securityLabel; 2109 } 2110 2111 /** 2112 * @return Returns a reference to <code>this</code> for easy method chaining 2113 */ 2114 public AuditEventEntityComponent setSecurityLabel(List<Coding> theSecurityLabel) { 2115 this.securityLabel = theSecurityLabel; 2116 return this; 2117 } 2118 2119 public boolean hasSecurityLabel() { 2120 if (this.securityLabel == null) 2121 return false; 2122 for (Coding item : this.securityLabel) 2123 if (!item.isEmpty()) 2124 return true; 2125 return false; 2126 } 2127 2128 public Coding addSecurityLabel() { //3 2129 Coding t = new Coding(); 2130 if (this.securityLabel == null) 2131 this.securityLabel = new ArrayList<Coding>(); 2132 this.securityLabel.add(t); 2133 return t; 2134 } 2135 2136 public AuditEventEntityComponent addSecurityLabel(Coding t) { //3 2137 if (t == null) 2138 return this; 2139 if (this.securityLabel == null) 2140 this.securityLabel = new ArrayList<Coding>(); 2141 this.securityLabel.add(t); 2142 return this; 2143 } 2144 2145 /** 2146 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 2147 */ 2148 public Coding getSecurityLabelFirstRep() { 2149 if (getSecurityLabel().isEmpty()) { 2150 addSecurityLabel(); 2151 } 2152 return getSecurityLabel().get(0); 2153 } 2154 2155 /** 2156 * @return {@link #name} (A name of the entity in the audit event.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2157 */ 2158 public StringType getNameElement() { 2159 if (this.name == null) 2160 if (Configuration.errorOnAutoCreate()) 2161 throw new Error("Attempt to auto-create AuditEventEntityComponent.name"); 2162 else if (Configuration.doAutoCreate()) 2163 this.name = new StringType(); // bb 2164 return this.name; 2165 } 2166 2167 public boolean hasNameElement() { 2168 return this.name != null && !this.name.isEmpty(); 2169 } 2170 2171 public boolean hasName() { 2172 return this.name != null && !this.name.isEmpty(); 2173 } 2174 2175 /** 2176 * @param value {@link #name} (A name of the entity in the audit event.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2177 */ 2178 public AuditEventEntityComponent setNameElement(StringType value) { 2179 this.name = value; 2180 return this; 2181 } 2182 2183 /** 2184 * @return A name of the entity in the audit event. 2185 */ 2186 public String getName() { 2187 return this.name == null ? null : this.name.getValue(); 2188 } 2189 2190 /** 2191 * @param value A name of the entity in the audit event. 2192 */ 2193 public AuditEventEntityComponent setName(String value) { 2194 if (Utilities.noString(value)) 2195 this.name = null; 2196 else { 2197 if (this.name == null) 2198 this.name = new StringType(); 2199 this.name.setValue(value); 2200 } 2201 return this; 2202 } 2203 2204 /** 2205 * @return {@link #description} (Text that describes the entity in more detail.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2206 */ 2207 public StringType getDescriptionElement() { 2208 if (this.description == null) 2209 if (Configuration.errorOnAutoCreate()) 2210 throw new Error("Attempt to auto-create AuditEventEntityComponent.description"); 2211 else if (Configuration.doAutoCreate()) 2212 this.description = new StringType(); // bb 2213 return this.description; 2214 } 2215 2216 public boolean hasDescriptionElement() { 2217 return this.description != null && !this.description.isEmpty(); 2218 } 2219 2220 public boolean hasDescription() { 2221 return this.description != null && !this.description.isEmpty(); 2222 } 2223 2224 /** 2225 * @param value {@link #description} (Text that describes the entity in more detail.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2226 */ 2227 public AuditEventEntityComponent setDescriptionElement(StringType value) { 2228 this.description = value; 2229 return this; 2230 } 2231 2232 /** 2233 * @return Text that describes the entity in more detail. 2234 */ 2235 public String getDescription() { 2236 return this.description == null ? null : this.description.getValue(); 2237 } 2238 2239 /** 2240 * @param value Text that describes the entity in more detail. 2241 */ 2242 public AuditEventEntityComponent setDescription(String value) { 2243 if (Utilities.noString(value)) 2244 this.description = null; 2245 else { 2246 if (this.description == null) 2247 this.description = new StringType(); 2248 this.description.setValue(value); 2249 } 2250 return this; 2251 } 2252 2253 /** 2254 * @return {@link #query} (The query parameters for a query-type entities.). This is the underlying object with id, value and extensions. The accessor "getQuery" gives direct access to the value 2255 */ 2256 public Base64BinaryType getQueryElement() { 2257 if (this.query == null) 2258 if (Configuration.errorOnAutoCreate()) 2259 throw new Error("Attempt to auto-create AuditEventEntityComponent.query"); 2260 else if (Configuration.doAutoCreate()) 2261 this.query = new Base64BinaryType(); // bb 2262 return this.query; 2263 } 2264 2265 public boolean hasQueryElement() { 2266 return this.query != null && !this.query.isEmpty(); 2267 } 2268 2269 public boolean hasQuery() { 2270 return this.query != null && !this.query.isEmpty(); 2271 } 2272 2273 /** 2274 * @param value {@link #query} (The query parameters for a query-type entities.). This is the underlying object with id, value and extensions. The accessor "getQuery" gives direct access to the value 2275 */ 2276 public AuditEventEntityComponent setQueryElement(Base64BinaryType value) { 2277 this.query = value; 2278 return this; 2279 } 2280 2281 /** 2282 * @return The query parameters for a query-type entities. 2283 */ 2284 public byte[] getQuery() { 2285 return this.query == null ? null : this.query.getValue(); 2286 } 2287 2288 /** 2289 * @param value The query parameters for a query-type entities. 2290 */ 2291 public AuditEventEntityComponent setQuery(byte[] value) { 2292 if (value == null) 2293 this.query = null; 2294 else { 2295 if (this.query == null) 2296 this.query = new Base64BinaryType(); 2297 this.query.setValue(value); 2298 } 2299 return this; 2300 } 2301 2302 /** 2303 * @return {@link #detail} (Tagged value pairs for conveying additional information about the entity.) 2304 */ 2305 public List<AuditEventEntityDetailComponent> getDetail() { 2306 if (this.detail == null) 2307 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2308 return this.detail; 2309 } 2310 2311 /** 2312 * @return Returns a reference to <code>this</code> for easy method chaining 2313 */ 2314 public AuditEventEntityComponent setDetail(List<AuditEventEntityDetailComponent> theDetail) { 2315 this.detail = theDetail; 2316 return this; 2317 } 2318 2319 public boolean hasDetail() { 2320 if (this.detail == null) 2321 return false; 2322 for (AuditEventEntityDetailComponent item : this.detail) 2323 if (!item.isEmpty()) 2324 return true; 2325 return false; 2326 } 2327 2328 public AuditEventEntityDetailComponent addDetail() { //3 2329 AuditEventEntityDetailComponent t = new AuditEventEntityDetailComponent(); 2330 if (this.detail == null) 2331 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2332 this.detail.add(t); 2333 return t; 2334 } 2335 2336 public AuditEventEntityComponent addDetail(AuditEventEntityDetailComponent t) { //3 2337 if (t == null) 2338 return this; 2339 if (this.detail == null) 2340 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2341 this.detail.add(t); 2342 return this; 2343 } 2344 2345 /** 2346 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 2347 */ 2348 public AuditEventEntityDetailComponent getDetailFirstRep() { 2349 if (getDetail().isEmpty()) { 2350 addDetail(); 2351 } 2352 return getDetail().get(0); 2353 } 2354 2355 protected void listChildren(List<Property> children) { 2356 super.listChildren(children); 2357 children.add(new Property("identifier", "Identifier", "Identifies a specific instance of the entity. The reference should always be version specific.", 0, 1, identifier)); 2358 children.add(new Property("reference", "Reference(Any)", "Identifies a specific instance of the entity. The reference should be version specific.", 0, 1, reference)); 2359 children.add(new Property("type", "Coding", "The type of the object that was involved in this audit event.", 0, 1, type)); 2360 children.add(new Property("role", "Coding", "Code representing the role the entity played in the event being audited.", 0, 1, role)); 2361 children.add(new Property("lifecycle", "Coding", "Identifier for the data life-cycle stage for the entity.", 0, 1, lifecycle)); 2362 children.add(new Property("securityLabel", "Coding", "Security labels for the identified entity.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2363 children.add(new Property("name", "string", "A name of the entity in the audit event.", 0, 1, name)); 2364 children.add(new Property("description", "string", "Text that describes the entity in more detail.", 0, 1, description)); 2365 children.add(new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 1, query)); 2366 children.add(new Property("detail", "", "Tagged value pairs for conveying additional information about the entity.", 0, java.lang.Integer.MAX_VALUE, detail)); 2367 } 2368 2369 @Override 2370 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2371 switch (_hash) { 2372 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifies a specific instance of the entity. The reference should always be version specific.", 0, 1, identifier); 2373 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "Identifies a specific instance of the entity. The reference should be version specific.", 0, 1, reference); 2374 case 3575610: /*type*/ return new Property("type", "Coding", "The type of the object that was involved in this audit event.", 0, 1, type); 2375 case 3506294: /*role*/ return new Property("role", "Coding", "Code representing the role the entity played in the event being audited.", 0, 1, role); 2376 case -302323862: /*lifecycle*/ return new Property("lifecycle", "Coding", "Identifier for the data life-cycle stage for the entity.", 0, 1, lifecycle); 2377 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "Security labels for the identified entity.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 2378 case 3373707: /*name*/ return new Property("name", "string", "A name of the entity in the audit event.", 0, 1, name); 2379 case -1724546052: /*description*/ return new Property("description", "string", "Text that describes the entity in more detail.", 0, 1, description); 2380 case 107944136: /*query*/ return new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 1, query); 2381 case -1335224239: /*detail*/ return new Property("detail", "", "Tagged value pairs for conveying additional information about the entity.", 0, java.lang.Integer.MAX_VALUE, detail); 2382 default: return super.getNamedProperty(_hash, _name, _checkValid); 2383 } 2384 2385 } 2386 2387 @Override 2388 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2389 switch (hash) { 2390 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2391 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2392 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 2393 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // Coding 2394 case -302323862: /*lifecycle*/ return this.lifecycle == null ? new Base[0] : new Base[] {this.lifecycle}; // Coding 2395 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 2396 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2397 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2398 case 107944136: /*query*/ return this.query == null ? new Base[0] : new Base[] {this.query}; // Base64BinaryType 2399 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AuditEventEntityDetailComponent 2400 default: return super.getProperty(hash, name, checkValid); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2407 switch (hash) { 2408 case -1618432855: // identifier 2409 this.identifier = castToIdentifier(value); // Identifier 2410 return value; 2411 case -925155509: // reference 2412 this.reference = castToReference(value); // Reference 2413 return value; 2414 case 3575610: // type 2415 this.type = castToCoding(value); // Coding 2416 return value; 2417 case 3506294: // role 2418 this.role = castToCoding(value); // Coding 2419 return value; 2420 case -302323862: // lifecycle 2421 this.lifecycle = castToCoding(value); // Coding 2422 return value; 2423 case -722296940: // securityLabel 2424 this.getSecurityLabel().add(castToCoding(value)); // Coding 2425 return value; 2426 case 3373707: // name 2427 this.name = castToString(value); // StringType 2428 return value; 2429 case -1724546052: // description 2430 this.description = castToString(value); // StringType 2431 return value; 2432 case 107944136: // query 2433 this.query = castToBase64Binary(value); // Base64BinaryType 2434 return value; 2435 case -1335224239: // detail 2436 this.getDetail().add((AuditEventEntityDetailComponent) value); // AuditEventEntityDetailComponent 2437 return value; 2438 default: return super.setProperty(hash, name, value); 2439 } 2440 2441 } 2442 2443 @Override 2444 public Base setProperty(String name, Base value) throws FHIRException { 2445 if (name.equals("identifier")) { 2446 this.identifier = castToIdentifier(value); // Identifier 2447 } else if (name.equals("reference")) { 2448 this.reference = castToReference(value); // Reference 2449 } else if (name.equals("type")) { 2450 this.type = castToCoding(value); // Coding 2451 } else if (name.equals("role")) { 2452 this.role = castToCoding(value); // Coding 2453 } else if (name.equals("lifecycle")) { 2454 this.lifecycle = castToCoding(value); // Coding 2455 } else if (name.equals("securityLabel")) { 2456 this.getSecurityLabel().add(castToCoding(value)); 2457 } else if (name.equals("name")) { 2458 this.name = castToString(value); // StringType 2459 } else if (name.equals("description")) { 2460 this.description = castToString(value); // StringType 2461 } else if (name.equals("query")) { 2462 this.query = castToBase64Binary(value); // Base64BinaryType 2463 } else if (name.equals("detail")) { 2464 this.getDetail().add((AuditEventEntityDetailComponent) value); 2465 } else 2466 return super.setProperty(name, value); 2467 return value; 2468 } 2469 2470 @Override 2471 public Base makeProperty(int hash, String name) throws FHIRException { 2472 switch (hash) { 2473 case -1618432855: return getIdentifier(); 2474 case -925155509: return getReference(); 2475 case 3575610: return getType(); 2476 case 3506294: return getRole(); 2477 case -302323862: return getLifecycle(); 2478 case -722296940: return addSecurityLabel(); 2479 case 3373707: return getNameElement(); 2480 case -1724546052: return getDescriptionElement(); 2481 case 107944136: return getQueryElement(); 2482 case -1335224239: return addDetail(); 2483 default: return super.makeProperty(hash, name); 2484 } 2485 2486 } 2487 2488 @Override 2489 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2490 switch (hash) { 2491 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2492 case -925155509: /*reference*/ return new String[] {"Reference"}; 2493 case 3575610: /*type*/ return new String[] {"Coding"}; 2494 case 3506294: /*role*/ return new String[] {"Coding"}; 2495 case -302323862: /*lifecycle*/ return new String[] {"Coding"}; 2496 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 2497 case 3373707: /*name*/ return new String[] {"string"}; 2498 case -1724546052: /*description*/ return new String[] {"string"}; 2499 case 107944136: /*query*/ return new String[] {"base64Binary"}; 2500 case -1335224239: /*detail*/ return new String[] {}; 2501 default: return super.getTypesForProperty(hash, name); 2502 } 2503 2504 } 2505 2506 @Override 2507 public Base addChild(String name) throws FHIRException { 2508 if (name.equals("identifier")) { 2509 this.identifier = new Identifier(); 2510 return this.identifier; 2511 } 2512 else if (name.equals("reference")) { 2513 this.reference = new Reference(); 2514 return this.reference; 2515 } 2516 else if (name.equals("type")) { 2517 this.type = new Coding(); 2518 return this.type; 2519 } 2520 else if (name.equals("role")) { 2521 this.role = new Coding(); 2522 return this.role; 2523 } 2524 else if (name.equals("lifecycle")) { 2525 this.lifecycle = new Coding(); 2526 return this.lifecycle; 2527 } 2528 else if (name.equals("securityLabel")) { 2529 return addSecurityLabel(); 2530 } 2531 else if (name.equals("name")) { 2532 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 2533 } 2534 else if (name.equals("description")) { 2535 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.description"); 2536 } 2537 else if (name.equals("query")) { 2538 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.query"); 2539 } 2540 else if (name.equals("detail")) { 2541 return addDetail(); 2542 } 2543 else 2544 return super.addChild(name); 2545 } 2546 2547 public AuditEventEntityComponent copy() { 2548 AuditEventEntityComponent dst = new AuditEventEntityComponent(); 2549 copyValues(dst); 2550 dst.identifier = identifier == null ? null : identifier.copy(); 2551 dst.reference = reference == null ? null : reference.copy(); 2552 dst.type = type == null ? null : type.copy(); 2553 dst.role = role == null ? null : role.copy(); 2554 dst.lifecycle = lifecycle == null ? null : lifecycle.copy(); 2555 if (securityLabel != null) { 2556 dst.securityLabel = new ArrayList<Coding>(); 2557 for (Coding i : securityLabel) 2558 dst.securityLabel.add(i.copy()); 2559 }; 2560 dst.name = name == null ? null : name.copy(); 2561 dst.description = description == null ? null : description.copy(); 2562 dst.query = query == null ? null : query.copy(); 2563 if (detail != null) { 2564 dst.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2565 for (AuditEventEntityDetailComponent i : detail) 2566 dst.detail.add(i.copy()); 2567 }; 2568 return dst; 2569 } 2570 2571 @Override 2572 public boolean equalsDeep(Base other_) { 2573 if (!super.equalsDeep(other_)) 2574 return false; 2575 if (!(other_ instanceof AuditEventEntityComponent)) 2576 return false; 2577 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 2578 return compareDeep(identifier, o.identifier, true) && compareDeep(reference, o.reference, true) 2579 && compareDeep(type, o.type, true) && compareDeep(role, o.role, true) && compareDeep(lifecycle, o.lifecycle, true) 2580 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 2581 && compareDeep(query, o.query, true) && compareDeep(detail, o.detail, true); 2582 } 2583 2584 @Override 2585 public boolean equalsShallow(Base other_) { 2586 if (!super.equalsShallow(other_)) 2587 return false; 2588 if (!(other_ instanceof AuditEventEntityComponent)) 2589 return false; 2590 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 2591 return compareValues(name, o.name, true) && compareValues(description, o.description, true) && compareValues(query, o.query, true) 2592 ; 2593 } 2594 2595 public boolean isEmpty() { 2596 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, reference, type 2597 , role, lifecycle, securityLabel, name, description, query, detail); 2598 } 2599 2600 public String fhirType() { 2601 return "AuditEvent.entity"; 2602 2603 } 2604 2605 } 2606 2607 @Block() 2608 public static class AuditEventEntityDetailComponent extends BackboneElement implements IBaseBackboneElement { 2609 /** 2610 * The type of extra detail provided in the value. 2611 */ 2612 @Child(name = "type", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2613 @Description(shortDefinition="Name of the property", formalDefinition="The type of extra detail provided in the value." ) 2614 protected StringType type; 2615 2616 /** 2617 * The details, base64 encoded. Used to carry bulk information. 2618 */ 2619 @Child(name = "value", type = {Base64BinaryType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2620 @Description(shortDefinition="Property value", formalDefinition="The details, base64 encoded. Used to carry bulk information." ) 2621 protected Base64BinaryType value; 2622 2623 private static final long serialVersionUID = 11139504L; 2624 2625 /** 2626 * Constructor 2627 */ 2628 public AuditEventEntityDetailComponent() { 2629 super(); 2630 } 2631 2632 /** 2633 * Constructor 2634 */ 2635 public AuditEventEntityDetailComponent(StringType type, Base64BinaryType value) { 2636 super(); 2637 this.type = type; 2638 this.value = value; 2639 } 2640 2641 /** 2642 * @return {@link #type} (The type of extra detail provided in the value.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2643 */ 2644 public StringType getTypeElement() { 2645 if (this.type == null) 2646 if (Configuration.errorOnAutoCreate()) 2647 throw new Error("Attempt to auto-create AuditEventEntityDetailComponent.type"); 2648 else if (Configuration.doAutoCreate()) 2649 this.type = new StringType(); // bb 2650 return this.type; 2651 } 2652 2653 public boolean hasTypeElement() { 2654 return this.type != null && !this.type.isEmpty(); 2655 } 2656 2657 public boolean hasType() { 2658 return this.type != null && !this.type.isEmpty(); 2659 } 2660 2661 /** 2662 * @param value {@link #type} (The type of extra detail provided in the value.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2663 */ 2664 public AuditEventEntityDetailComponent setTypeElement(StringType value) { 2665 this.type = value; 2666 return this; 2667 } 2668 2669 /** 2670 * @return The type of extra detail provided in the value. 2671 */ 2672 public String getType() { 2673 return this.type == null ? null : this.type.getValue(); 2674 } 2675 2676 /** 2677 * @param value The type of extra detail provided in the value. 2678 */ 2679 public AuditEventEntityDetailComponent setType(String value) { 2680 if (this.type == null) 2681 this.type = new StringType(); 2682 this.type.setValue(value); 2683 return this; 2684 } 2685 2686 /** 2687 * @return {@link #value} (The details, base64 encoded. Used to carry bulk information.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2688 */ 2689 public Base64BinaryType getValueElement() { 2690 if (this.value == null) 2691 if (Configuration.errorOnAutoCreate()) 2692 throw new Error("Attempt to auto-create AuditEventEntityDetailComponent.value"); 2693 else if (Configuration.doAutoCreate()) 2694 this.value = new Base64BinaryType(); // bb 2695 return this.value; 2696 } 2697 2698 public boolean hasValueElement() { 2699 return this.value != null && !this.value.isEmpty(); 2700 } 2701 2702 public boolean hasValue() { 2703 return this.value != null && !this.value.isEmpty(); 2704 } 2705 2706 /** 2707 * @param value {@link #value} (The details, base64 encoded. Used to carry bulk information.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2708 */ 2709 public AuditEventEntityDetailComponent setValueElement(Base64BinaryType value) { 2710 this.value = value; 2711 return this; 2712 } 2713 2714 /** 2715 * @return The details, base64 encoded. Used to carry bulk information. 2716 */ 2717 public byte[] getValue() { 2718 return this.value == null ? null : this.value.getValue(); 2719 } 2720 2721 /** 2722 * @param value The details, base64 encoded. Used to carry bulk information. 2723 */ 2724 public AuditEventEntityDetailComponent setValue(byte[] value) { 2725 if (this.value == null) 2726 this.value = new Base64BinaryType(); 2727 this.value.setValue(value); 2728 return this; 2729 } 2730 2731 protected void listChildren(List<Property> children) { 2732 super.listChildren(children); 2733 children.add(new Property("type", "string", "The type of extra detail provided in the value.", 0, 1, type)); 2734 children.add(new Property("value", "base64Binary", "The details, base64 encoded. Used to carry bulk information.", 0, 1, value)); 2735 } 2736 2737 @Override 2738 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2739 switch (_hash) { 2740 case 3575610: /*type*/ return new Property("type", "string", "The type of extra detail provided in the value.", 0, 1, type); 2741 case 111972721: /*value*/ return new Property("value", "base64Binary", "The details, base64 encoded. Used to carry bulk information.", 0, 1, value); 2742 default: return super.getNamedProperty(_hash, _name, _checkValid); 2743 } 2744 2745 } 2746 2747 @Override 2748 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2749 switch (hash) { 2750 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // StringType 2751 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Base64BinaryType 2752 default: return super.getProperty(hash, name, checkValid); 2753 } 2754 2755 } 2756 2757 @Override 2758 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2759 switch (hash) { 2760 case 3575610: // type 2761 this.type = castToString(value); // StringType 2762 return value; 2763 case 111972721: // value 2764 this.value = castToBase64Binary(value); // Base64BinaryType 2765 return value; 2766 default: return super.setProperty(hash, name, value); 2767 } 2768 2769 } 2770 2771 @Override 2772 public Base setProperty(String name, Base value) throws FHIRException { 2773 if (name.equals("type")) { 2774 this.type = castToString(value); // StringType 2775 } else if (name.equals("value")) { 2776 this.value = castToBase64Binary(value); // Base64BinaryType 2777 } else 2778 return super.setProperty(name, value); 2779 return value; 2780 } 2781 2782 @Override 2783 public Base makeProperty(int hash, String name) throws FHIRException { 2784 switch (hash) { 2785 case 3575610: return getTypeElement(); 2786 case 111972721: return getValueElement(); 2787 default: return super.makeProperty(hash, name); 2788 } 2789 2790 } 2791 2792 @Override 2793 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2794 switch (hash) { 2795 case 3575610: /*type*/ return new String[] {"string"}; 2796 case 111972721: /*value*/ return new String[] {"base64Binary"}; 2797 default: return super.getTypesForProperty(hash, name); 2798 } 2799 2800 } 2801 2802 @Override 2803 public Base addChild(String name) throws FHIRException { 2804 if (name.equals("type")) { 2805 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 2806 } 2807 else if (name.equals("value")) { 2808 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.value"); 2809 } 2810 else 2811 return super.addChild(name); 2812 } 2813 2814 public AuditEventEntityDetailComponent copy() { 2815 AuditEventEntityDetailComponent dst = new AuditEventEntityDetailComponent(); 2816 copyValues(dst); 2817 dst.type = type == null ? null : type.copy(); 2818 dst.value = value == null ? null : value.copy(); 2819 return dst; 2820 } 2821 2822 @Override 2823 public boolean equalsDeep(Base other_) { 2824 if (!super.equalsDeep(other_)) 2825 return false; 2826 if (!(other_ instanceof AuditEventEntityDetailComponent)) 2827 return false; 2828 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 2829 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2830 } 2831 2832 @Override 2833 public boolean equalsShallow(Base other_) { 2834 if (!super.equalsShallow(other_)) 2835 return false; 2836 if (!(other_ instanceof AuditEventEntityDetailComponent)) 2837 return false; 2838 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 2839 return compareValues(type, o.type, true) && compareValues(value, o.value, true); 2840 } 2841 2842 public boolean isEmpty() { 2843 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2844 } 2845 2846 public String fhirType() { 2847 return "AuditEvent.entity.detail"; 2848 2849 } 2850 2851 } 2852 2853 /** 2854 * Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function. 2855 */ 2856 @Child(name = "type", type = {Coding.class}, order=0, min=1, max=1, modifier=false, summary=true) 2857 @Description(shortDefinition="Type/identifier of event", formalDefinition="Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function." ) 2858 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-type") 2859 protected Coding type; 2860 2861 /** 2862 * Identifier for the category of event. 2863 */ 2864 @Child(name = "subtype", type = {Coding.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2865 @Description(shortDefinition="More specific type/id for the event", formalDefinition="Identifier for the category of event." ) 2866 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-sub-type") 2867 protected List<Coding> subtype; 2868 2869 /** 2870 * Indicator for type of action performed during the event that generated the audit. 2871 */ 2872 @Child(name = "action", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2873 @Description(shortDefinition="Type of action performed during the event", formalDefinition="Indicator for type of action performed during the event that generated the audit." ) 2874 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-action") 2875 protected Enumeration<AuditEventAction> action; 2876 2877 /** 2878 * The time when the event occurred on the source. 2879 */ 2880 @Child(name = "recorded", type = {InstantType.class}, order=3, min=1, max=1, modifier=false, summary=true) 2881 @Description(shortDefinition="Time when the event occurred on source", formalDefinition="The time when the event occurred on the source." ) 2882 protected InstantType recorded; 2883 2884 /** 2885 * Indicates whether the event succeeded or failed. 2886 */ 2887 @Child(name = "outcome", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2888 @Description(shortDefinition="Whether the event succeeded or failed", formalDefinition="Indicates whether the event succeeded or failed." ) 2889 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-outcome") 2890 protected Enumeration<AuditEventOutcome> outcome; 2891 2892 /** 2893 * A free text description of the outcome of the event. 2894 */ 2895 @Child(name = "outcomeDesc", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2896 @Description(shortDefinition="Description of the event outcome", formalDefinition="A free text description of the outcome of the event." ) 2897 protected StringType outcomeDesc; 2898 2899 /** 2900 * The purposeOfUse (reason) that was used during the event being recorded. 2901 */ 2902 @Child(name = "purposeOfEvent", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2903 @Description(shortDefinition="The purposeOfUse of the event", formalDefinition="The purposeOfUse (reason) that was used during the event being recorded." ) 2904 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 2905 protected List<CodeableConcept> purposeOfEvent; 2906 2907 /** 2908 * An actor taking an active role in the event or activity that is logged. 2909 */ 2910 @Child(name = "agent", type = {}, order=7, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2911 @Description(shortDefinition="Actor involved in the event", formalDefinition="An actor taking an active role in the event or activity that is logged." ) 2912 protected List<AuditEventAgentComponent> agent; 2913 2914 /** 2915 * The system that is reporting the event. 2916 */ 2917 @Child(name = "source", type = {}, order=8, min=1, max=1, modifier=false, summary=false) 2918 @Description(shortDefinition="Audit Event Reporter", formalDefinition="The system that is reporting the event." ) 2919 protected AuditEventSourceComponent source; 2920 2921 /** 2922 * Specific instances of data or objects that have been accessed. 2923 */ 2924 @Child(name = "entity", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2925 @Description(shortDefinition="Data or objects used", formalDefinition="Specific instances of data or objects that have been accessed." ) 2926 protected List<AuditEventEntityComponent> entity; 2927 2928 private static final long serialVersionUID = 2102955199L; 2929 2930 /** 2931 * Constructor 2932 */ 2933 public AuditEvent() { 2934 super(); 2935 } 2936 2937 /** 2938 * Constructor 2939 */ 2940 public AuditEvent(Coding type, InstantType recorded, AuditEventSourceComponent source) { 2941 super(); 2942 this.type = type; 2943 this.recorded = recorded; 2944 this.source = source; 2945 } 2946 2947 /** 2948 * @return {@link #type} (Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.) 2949 */ 2950 public Coding getType() { 2951 if (this.type == null) 2952 if (Configuration.errorOnAutoCreate()) 2953 throw new Error("Attempt to auto-create AuditEvent.type"); 2954 else if (Configuration.doAutoCreate()) 2955 this.type = new Coding(); // cc 2956 return this.type; 2957 } 2958 2959 public boolean hasType() { 2960 return this.type != null && !this.type.isEmpty(); 2961 } 2962 2963 /** 2964 * @param value {@link #type} (Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.) 2965 */ 2966 public AuditEvent setType(Coding value) { 2967 this.type = value; 2968 return this; 2969 } 2970 2971 /** 2972 * @return {@link #subtype} (Identifier for the category of event.) 2973 */ 2974 public List<Coding> getSubtype() { 2975 if (this.subtype == null) 2976 this.subtype = new ArrayList<Coding>(); 2977 return this.subtype; 2978 } 2979 2980 /** 2981 * @return Returns a reference to <code>this</code> for easy method chaining 2982 */ 2983 public AuditEvent setSubtype(List<Coding> theSubtype) { 2984 this.subtype = theSubtype; 2985 return this; 2986 } 2987 2988 public boolean hasSubtype() { 2989 if (this.subtype == null) 2990 return false; 2991 for (Coding item : this.subtype) 2992 if (!item.isEmpty()) 2993 return true; 2994 return false; 2995 } 2996 2997 public Coding addSubtype() { //3 2998 Coding t = new Coding(); 2999 if (this.subtype == null) 3000 this.subtype = new ArrayList<Coding>(); 3001 this.subtype.add(t); 3002 return t; 3003 } 3004 3005 public AuditEvent addSubtype(Coding t) { //3 3006 if (t == null) 3007 return this; 3008 if (this.subtype == null) 3009 this.subtype = new ArrayList<Coding>(); 3010 this.subtype.add(t); 3011 return this; 3012 } 3013 3014 /** 3015 * @return The first repetition of repeating field {@link #subtype}, creating it if it does not already exist 3016 */ 3017 public Coding getSubtypeFirstRep() { 3018 if (getSubtype().isEmpty()) { 3019 addSubtype(); 3020 } 3021 return getSubtype().get(0); 3022 } 3023 3024 /** 3025 * @return {@link #action} (Indicator for type of action performed during the event that generated the audit.). This is the underlying object with id, value and extensions. The accessor "getAction" gives direct access to the value 3026 */ 3027 public Enumeration<AuditEventAction> getActionElement() { 3028 if (this.action == null) 3029 if (Configuration.errorOnAutoCreate()) 3030 throw new Error("Attempt to auto-create AuditEvent.action"); 3031 else if (Configuration.doAutoCreate()) 3032 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); // bb 3033 return this.action; 3034 } 3035 3036 public boolean hasActionElement() { 3037 return this.action != null && !this.action.isEmpty(); 3038 } 3039 3040 public boolean hasAction() { 3041 return this.action != null && !this.action.isEmpty(); 3042 } 3043 3044 /** 3045 * @param value {@link #action} (Indicator for type of action performed during the event that generated the audit.). This is the underlying object with id, value and extensions. The accessor "getAction" gives direct access to the value 3046 */ 3047 public AuditEvent setActionElement(Enumeration<AuditEventAction> value) { 3048 this.action = value; 3049 return this; 3050 } 3051 3052 /** 3053 * @return Indicator for type of action performed during the event that generated the audit. 3054 */ 3055 public AuditEventAction getAction() { 3056 return this.action == null ? null : this.action.getValue(); 3057 } 3058 3059 /** 3060 * @param value Indicator for type of action performed during the event that generated the audit. 3061 */ 3062 public AuditEvent setAction(AuditEventAction value) { 3063 if (value == null) 3064 this.action = null; 3065 else { 3066 if (this.action == null) 3067 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); 3068 this.action.setValue(value); 3069 } 3070 return this; 3071 } 3072 3073 /** 3074 * @return {@link #recorded} (The time when the event occurred on the source.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 3075 */ 3076 public InstantType getRecordedElement() { 3077 if (this.recorded == null) 3078 if (Configuration.errorOnAutoCreate()) 3079 throw new Error("Attempt to auto-create AuditEvent.recorded"); 3080 else if (Configuration.doAutoCreate()) 3081 this.recorded = new InstantType(); // bb 3082 return this.recorded; 3083 } 3084 3085 public boolean hasRecordedElement() { 3086 return this.recorded != null && !this.recorded.isEmpty(); 3087 } 3088 3089 public boolean hasRecorded() { 3090 return this.recorded != null && !this.recorded.isEmpty(); 3091 } 3092 3093 /** 3094 * @param value {@link #recorded} (The time when the event occurred on the source.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 3095 */ 3096 public AuditEvent setRecordedElement(InstantType value) { 3097 this.recorded = value; 3098 return this; 3099 } 3100 3101 /** 3102 * @return The time when the event occurred on the source. 3103 */ 3104 public Date getRecorded() { 3105 return this.recorded == null ? null : this.recorded.getValue(); 3106 } 3107 3108 /** 3109 * @param value The time when the event occurred on the source. 3110 */ 3111 public AuditEvent setRecorded(Date value) { 3112 if (this.recorded == null) 3113 this.recorded = new InstantType(); 3114 this.recorded.setValue(value); 3115 return this; 3116 } 3117 3118 /** 3119 * @return {@link #outcome} (Indicates whether the event succeeded or failed.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 3120 */ 3121 public Enumeration<AuditEventOutcome> getOutcomeElement() { 3122 if (this.outcome == null) 3123 if (Configuration.errorOnAutoCreate()) 3124 throw new Error("Attempt to auto-create AuditEvent.outcome"); 3125 else if (Configuration.doAutoCreate()) 3126 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); // bb 3127 return this.outcome; 3128 } 3129 3130 public boolean hasOutcomeElement() { 3131 return this.outcome != null && !this.outcome.isEmpty(); 3132 } 3133 3134 public boolean hasOutcome() { 3135 return this.outcome != null && !this.outcome.isEmpty(); 3136 } 3137 3138 /** 3139 * @param value {@link #outcome} (Indicates whether the event succeeded or failed.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 3140 */ 3141 public AuditEvent setOutcomeElement(Enumeration<AuditEventOutcome> value) { 3142 this.outcome = value; 3143 return this; 3144 } 3145 3146 /** 3147 * @return Indicates whether the event succeeded or failed. 3148 */ 3149 public AuditEventOutcome getOutcome() { 3150 return this.outcome == null ? null : this.outcome.getValue(); 3151 } 3152 3153 /** 3154 * @param value Indicates whether the event succeeded or failed. 3155 */ 3156 public AuditEvent setOutcome(AuditEventOutcome value) { 3157 if (value == null) 3158 this.outcome = null; 3159 else { 3160 if (this.outcome == null) 3161 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); 3162 this.outcome.setValue(value); 3163 } 3164 return this; 3165 } 3166 3167 /** 3168 * @return {@link #outcomeDesc} (A free text description of the outcome of the event.). This is the underlying object with id, value and extensions. The accessor "getOutcomeDesc" gives direct access to the value 3169 */ 3170 public StringType getOutcomeDescElement() { 3171 if (this.outcomeDesc == null) 3172 if (Configuration.errorOnAutoCreate()) 3173 throw new Error("Attempt to auto-create AuditEvent.outcomeDesc"); 3174 else if (Configuration.doAutoCreate()) 3175 this.outcomeDesc = new StringType(); // bb 3176 return this.outcomeDesc; 3177 } 3178 3179 public boolean hasOutcomeDescElement() { 3180 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 3181 } 3182 3183 public boolean hasOutcomeDesc() { 3184 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 3185 } 3186 3187 /** 3188 * @param value {@link #outcomeDesc} (A free text description of the outcome of the event.). This is the underlying object with id, value and extensions. The accessor "getOutcomeDesc" gives direct access to the value 3189 */ 3190 public AuditEvent setOutcomeDescElement(StringType value) { 3191 this.outcomeDesc = value; 3192 return this; 3193 } 3194 3195 /** 3196 * @return A free text description of the outcome of the event. 3197 */ 3198 public String getOutcomeDesc() { 3199 return this.outcomeDesc == null ? null : this.outcomeDesc.getValue(); 3200 } 3201 3202 /** 3203 * @param value A free text description of the outcome of the event. 3204 */ 3205 public AuditEvent setOutcomeDesc(String value) { 3206 if (Utilities.noString(value)) 3207 this.outcomeDesc = null; 3208 else { 3209 if (this.outcomeDesc == null) 3210 this.outcomeDesc = new StringType(); 3211 this.outcomeDesc.setValue(value); 3212 } 3213 return this; 3214 } 3215 3216 /** 3217 * @return {@link #purposeOfEvent} (The purposeOfUse (reason) that was used during the event being recorded.) 3218 */ 3219 public List<CodeableConcept> getPurposeOfEvent() { 3220 if (this.purposeOfEvent == null) 3221 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3222 return this.purposeOfEvent; 3223 } 3224 3225 /** 3226 * @return Returns a reference to <code>this</code> for easy method chaining 3227 */ 3228 public AuditEvent setPurposeOfEvent(List<CodeableConcept> thePurposeOfEvent) { 3229 this.purposeOfEvent = thePurposeOfEvent; 3230 return this; 3231 } 3232 3233 public boolean hasPurposeOfEvent() { 3234 if (this.purposeOfEvent == null) 3235 return false; 3236 for (CodeableConcept item : this.purposeOfEvent) 3237 if (!item.isEmpty()) 3238 return true; 3239 return false; 3240 } 3241 3242 public CodeableConcept addPurposeOfEvent() { //3 3243 CodeableConcept t = new CodeableConcept(); 3244 if (this.purposeOfEvent == null) 3245 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3246 this.purposeOfEvent.add(t); 3247 return t; 3248 } 3249 3250 public AuditEvent addPurposeOfEvent(CodeableConcept t) { //3 3251 if (t == null) 3252 return this; 3253 if (this.purposeOfEvent == null) 3254 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3255 this.purposeOfEvent.add(t); 3256 return this; 3257 } 3258 3259 /** 3260 * @return The first repetition of repeating field {@link #purposeOfEvent}, creating it if it does not already exist 3261 */ 3262 public CodeableConcept getPurposeOfEventFirstRep() { 3263 if (getPurposeOfEvent().isEmpty()) { 3264 addPurposeOfEvent(); 3265 } 3266 return getPurposeOfEvent().get(0); 3267 } 3268 3269 /** 3270 * @return {@link #agent} (An actor taking an active role in the event or activity that is logged.) 3271 */ 3272 public List<AuditEventAgentComponent> getAgent() { 3273 if (this.agent == null) 3274 this.agent = new ArrayList<AuditEventAgentComponent>(); 3275 return this.agent; 3276 } 3277 3278 /** 3279 * @return Returns a reference to <code>this</code> for easy method chaining 3280 */ 3281 public AuditEvent setAgent(List<AuditEventAgentComponent> theAgent) { 3282 this.agent = theAgent; 3283 return this; 3284 } 3285 3286 public boolean hasAgent() { 3287 if (this.agent == null) 3288 return false; 3289 for (AuditEventAgentComponent item : this.agent) 3290 if (!item.isEmpty()) 3291 return true; 3292 return false; 3293 } 3294 3295 public AuditEventAgentComponent addAgent() { //3 3296 AuditEventAgentComponent t = new AuditEventAgentComponent(); 3297 if (this.agent == null) 3298 this.agent = new ArrayList<AuditEventAgentComponent>(); 3299 this.agent.add(t); 3300 return t; 3301 } 3302 3303 public AuditEvent addAgent(AuditEventAgentComponent t) { //3 3304 if (t == null) 3305 return this; 3306 if (this.agent == null) 3307 this.agent = new ArrayList<AuditEventAgentComponent>(); 3308 this.agent.add(t); 3309 return this; 3310 } 3311 3312 /** 3313 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist 3314 */ 3315 public AuditEventAgentComponent getAgentFirstRep() { 3316 if (getAgent().isEmpty()) { 3317 addAgent(); 3318 } 3319 return getAgent().get(0); 3320 } 3321 3322 /** 3323 * @return {@link #source} (The system that is reporting the event.) 3324 */ 3325 public AuditEventSourceComponent getSource() { 3326 if (this.source == null) 3327 if (Configuration.errorOnAutoCreate()) 3328 throw new Error("Attempt to auto-create AuditEvent.source"); 3329 else if (Configuration.doAutoCreate()) 3330 this.source = new AuditEventSourceComponent(); // cc 3331 return this.source; 3332 } 3333 3334 public boolean hasSource() { 3335 return this.source != null && !this.source.isEmpty(); 3336 } 3337 3338 /** 3339 * @param value {@link #source} (The system that is reporting the event.) 3340 */ 3341 public AuditEvent setSource(AuditEventSourceComponent value) { 3342 this.source = value; 3343 return this; 3344 } 3345 3346 /** 3347 * @return {@link #entity} (Specific instances of data or objects that have been accessed.) 3348 */ 3349 public List<AuditEventEntityComponent> getEntity() { 3350 if (this.entity == null) 3351 this.entity = new ArrayList<AuditEventEntityComponent>(); 3352 return this.entity; 3353 } 3354 3355 /** 3356 * @return Returns a reference to <code>this</code> for easy method chaining 3357 */ 3358 public AuditEvent setEntity(List<AuditEventEntityComponent> theEntity) { 3359 this.entity = theEntity; 3360 return this; 3361 } 3362 3363 public boolean hasEntity() { 3364 if (this.entity == null) 3365 return false; 3366 for (AuditEventEntityComponent item : this.entity) 3367 if (!item.isEmpty()) 3368 return true; 3369 return false; 3370 } 3371 3372 public AuditEventEntityComponent addEntity() { //3 3373 AuditEventEntityComponent t = new AuditEventEntityComponent(); 3374 if (this.entity == null) 3375 this.entity = new ArrayList<AuditEventEntityComponent>(); 3376 this.entity.add(t); 3377 return t; 3378 } 3379 3380 public AuditEvent addEntity(AuditEventEntityComponent t) { //3 3381 if (t == null) 3382 return this; 3383 if (this.entity == null) 3384 this.entity = new ArrayList<AuditEventEntityComponent>(); 3385 this.entity.add(t); 3386 return this; 3387 } 3388 3389 /** 3390 * @return The first repetition of repeating field {@link #entity}, creating it if it does not already exist 3391 */ 3392 public AuditEventEntityComponent getEntityFirstRep() { 3393 if (getEntity().isEmpty()) { 3394 addEntity(); 3395 } 3396 return getEntity().get(0); 3397 } 3398 3399 protected void listChildren(List<Property> children) { 3400 super.listChildren(children); 3401 children.add(new Property("type", "Coding", "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.", 0, 1, type)); 3402 children.add(new Property("subtype", "Coding", "Identifier for the category of event.", 0, java.lang.Integer.MAX_VALUE, subtype)); 3403 children.add(new Property("action", "code", "Indicator for type of action performed during the event that generated the audit.", 0, 1, action)); 3404 children.add(new Property("recorded", "instant", "The time when the event occurred on the source.", 0, 1, recorded)); 3405 children.add(new Property("outcome", "code", "Indicates whether the event succeeded or failed.", 0, 1, outcome)); 3406 children.add(new Property("outcomeDesc", "string", "A free text description of the outcome of the event.", 0, 1, outcomeDesc)); 3407 children.add(new Property("purposeOfEvent", "CodeableConcept", "The purposeOfUse (reason) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, purposeOfEvent)); 3408 children.add(new Property("agent", "", "An actor taking an active role in the event or activity that is logged.", 0, java.lang.Integer.MAX_VALUE, agent)); 3409 children.add(new Property("source", "", "The system that is reporting the event.", 0, 1, source)); 3410 children.add(new Property("entity", "", "Specific instances of data or objects that have been accessed.", 0, java.lang.Integer.MAX_VALUE, entity)); 3411 } 3412 3413 @Override 3414 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3415 switch (_hash) { 3416 case 3575610: /*type*/ return new Property("type", "Coding", "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.", 0, 1, type); 3417 case -1867567750: /*subtype*/ return new Property("subtype", "Coding", "Identifier for the category of event.", 0, java.lang.Integer.MAX_VALUE, subtype); 3418 case -1422950858: /*action*/ return new Property("action", "code", "Indicator for type of action performed during the event that generated the audit.", 0, 1, action); 3419 case -799233872: /*recorded*/ return new Property("recorded", "instant", "The time when the event occurred on the source.", 0, 1, recorded); 3420 case -1106507950: /*outcome*/ return new Property("outcome", "code", "Indicates whether the event succeeded or failed.", 0, 1, outcome); 3421 case 1062502659: /*outcomeDesc*/ return new Property("outcomeDesc", "string", "A free text description of the outcome of the event.", 0, 1, outcomeDesc); 3422 case -341917691: /*purposeOfEvent*/ return new Property("purposeOfEvent", "CodeableConcept", "The purposeOfUse (reason) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, purposeOfEvent); 3423 case 92750597: /*agent*/ return new Property("agent", "", "An actor taking an active role in the event or activity that is logged.", 0, java.lang.Integer.MAX_VALUE, agent); 3424 case -896505829: /*source*/ return new Property("source", "", "The system that is reporting the event.", 0, 1, source); 3425 case -1298275357: /*entity*/ return new Property("entity", "", "Specific instances of data or objects that have been accessed.", 0, java.lang.Integer.MAX_VALUE, entity); 3426 default: return super.getNamedProperty(_hash, _name, _checkValid); 3427 } 3428 3429 } 3430 3431 @Override 3432 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3433 switch (hash) { 3434 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 3435 case -1867567750: /*subtype*/ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // Coding 3436 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // Enumeration<AuditEventAction> 3437 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // InstantType 3438 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Enumeration<AuditEventOutcome> 3439 case 1062502659: /*outcomeDesc*/ return this.outcomeDesc == null ? new Base[0] : new Base[] {this.outcomeDesc}; // StringType 3440 case -341917691: /*purposeOfEvent*/ return this.purposeOfEvent == null ? new Base[0] : this.purposeOfEvent.toArray(new Base[this.purposeOfEvent.size()]); // CodeableConcept 3441 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // AuditEventAgentComponent 3442 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // AuditEventSourceComponent 3443 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : this.entity.toArray(new Base[this.entity.size()]); // AuditEventEntityComponent 3444 default: return super.getProperty(hash, name, checkValid); 3445 } 3446 3447 } 3448 3449 @Override 3450 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3451 switch (hash) { 3452 case 3575610: // type 3453 this.type = castToCoding(value); // Coding 3454 return value; 3455 case -1867567750: // subtype 3456 this.getSubtype().add(castToCoding(value)); // Coding 3457 return value; 3458 case -1422950858: // action 3459 value = new AuditEventActionEnumFactory().fromType(castToCode(value)); 3460 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 3461 return value; 3462 case -799233872: // recorded 3463 this.recorded = castToInstant(value); // InstantType 3464 return value; 3465 case -1106507950: // outcome 3466 value = new AuditEventOutcomeEnumFactory().fromType(castToCode(value)); 3467 this.outcome = (Enumeration) value; // Enumeration<AuditEventOutcome> 3468 return value; 3469 case 1062502659: // outcomeDesc 3470 this.outcomeDesc = castToString(value); // StringType 3471 return value; 3472 case -341917691: // purposeOfEvent 3473 this.getPurposeOfEvent().add(castToCodeableConcept(value)); // CodeableConcept 3474 return value; 3475 case 92750597: // agent 3476 this.getAgent().add((AuditEventAgentComponent) value); // AuditEventAgentComponent 3477 return value; 3478 case -896505829: // source 3479 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 3480 return value; 3481 case -1298275357: // entity 3482 this.getEntity().add((AuditEventEntityComponent) value); // AuditEventEntityComponent 3483 return value; 3484 default: return super.setProperty(hash, name, value); 3485 } 3486 3487 } 3488 3489 @Override 3490 public Base setProperty(String name, Base value) throws FHIRException { 3491 if (name.equals("type")) { 3492 this.type = castToCoding(value); // Coding 3493 } else if (name.equals("subtype")) { 3494 this.getSubtype().add(castToCoding(value)); 3495 } else if (name.equals("action")) { 3496 value = new AuditEventActionEnumFactory().fromType(castToCode(value)); 3497 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 3498 } else if (name.equals("recorded")) { 3499 this.recorded = castToInstant(value); // InstantType 3500 } else if (name.equals("outcome")) { 3501 value = new AuditEventOutcomeEnumFactory().fromType(castToCode(value)); 3502 this.outcome = (Enumeration) value; // Enumeration<AuditEventOutcome> 3503 } else if (name.equals("outcomeDesc")) { 3504 this.outcomeDesc = castToString(value); // StringType 3505 } else if (name.equals("purposeOfEvent")) { 3506 this.getPurposeOfEvent().add(castToCodeableConcept(value)); 3507 } else if (name.equals("agent")) { 3508 this.getAgent().add((AuditEventAgentComponent) value); 3509 } else if (name.equals("source")) { 3510 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 3511 } else if (name.equals("entity")) { 3512 this.getEntity().add((AuditEventEntityComponent) value); 3513 } else 3514 return super.setProperty(name, value); 3515 return value; 3516 } 3517 3518 @Override 3519 public Base makeProperty(int hash, String name) throws FHIRException { 3520 switch (hash) { 3521 case 3575610: return getType(); 3522 case -1867567750: return addSubtype(); 3523 case -1422950858: return getActionElement(); 3524 case -799233872: return getRecordedElement(); 3525 case -1106507950: return getOutcomeElement(); 3526 case 1062502659: return getOutcomeDescElement(); 3527 case -341917691: return addPurposeOfEvent(); 3528 case 92750597: return addAgent(); 3529 case -896505829: return getSource(); 3530 case -1298275357: return addEntity(); 3531 default: return super.makeProperty(hash, name); 3532 } 3533 3534 } 3535 3536 @Override 3537 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3538 switch (hash) { 3539 case 3575610: /*type*/ return new String[] {"Coding"}; 3540 case -1867567750: /*subtype*/ return new String[] {"Coding"}; 3541 case -1422950858: /*action*/ return new String[] {"code"}; 3542 case -799233872: /*recorded*/ return new String[] {"instant"}; 3543 case -1106507950: /*outcome*/ return new String[] {"code"}; 3544 case 1062502659: /*outcomeDesc*/ return new String[] {"string"}; 3545 case -341917691: /*purposeOfEvent*/ return new String[] {"CodeableConcept"}; 3546 case 92750597: /*agent*/ return new String[] {}; 3547 case -896505829: /*source*/ return new String[] {}; 3548 case -1298275357: /*entity*/ return new String[] {}; 3549 default: return super.getTypesForProperty(hash, name); 3550 } 3551 3552 } 3553 3554 @Override 3555 public Base addChild(String name) throws FHIRException { 3556 if (name.equals("type")) { 3557 this.type = new Coding(); 3558 return this.type; 3559 } 3560 else if (name.equals("subtype")) { 3561 return addSubtype(); 3562 } 3563 else if (name.equals("action")) { 3564 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.action"); 3565 } 3566 else if (name.equals("recorded")) { 3567 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.recorded"); 3568 } 3569 else if (name.equals("outcome")) { 3570 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcome"); 3571 } 3572 else if (name.equals("outcomeDesc")) { 3573 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcomeDesc"); 3574 } 3575 else if (name.equals("purposeOfEvent")) { 3576 return addPurposeOfEvent(); 3577 } 3578 else if (name.equals("agent")) { 3579 return addAgent(); 3580 } 3581 else if (name.equals("source")) { 3582 this.source = new AuditEventSourceComponent(); 3583 return this.source; 3584 } 3585 else if (name.equals("entity")) { 3586 return addEntity(); 3587 } 3588 else 3589 return super.addChild(name); 3590 } 3591 3592 public String fhirType() { 3593 return "AuditEvent"; 3594 3595 } 3596 3597 public AuditEvent copy() { 3598 AuditEvent dst = new AuditEvent(); 3599 copyValues(dst); 3600 dst.type = type == null ? null : type.copy(); 3601 if (subtype != null) { 3602 dst.subtype = new ArrayList<Coding>(); 3603 for (Coding i : subtype) 3604 dst.subtype.add(i.copy()); 3605 }; 3606 dst.action = action == null ? null : action.copy(); 3607 dst.recorded = recorded == null ? null : recorded.copy(); 3608 dst.outcome = outcome == null ? null : outcome.copy(); 3609 dst.outcomeDesc = outcomeDesc == null ? null : outcomeDesc.copy(); 3610 if (purposeOfEvent != null) { 3611 dst.purposeOfEvent = new ArrayList<CodeableConcept>(); 3612 for (CodeableConcept i : purposeOfEvent) 3613 dst.purposeOfEvent.add(i.copy()); 3614 }; 3615 if (agent != null) { 3616 dst.agent = new ArrayList<AuditEventAgentComponent>(); 3617 for (AuditEventAgentComponent i : agent) 3618 dst.agent.add(i.copy()); 3619 }; 3620 dst.source = source == null ? null : source.copy(); 3621 if (entity != null) { 3622 dst.entity = new ArrayList<AuditEventEntityComponent>(); 3623 for (AuditEventEntityComponent i : entity) 3624 dst.entity.add(i.copy()); 3625 }; 3626 return dst; 3627 } 3628 3629 protected AuditEvent typedCopy() { 3630 return copy(); 3631 } 3632 3633 @Override 3634 public boolean equalsDeep(Base other_) { 3635 if (!super.equalsDeep(other_)) 3636 return false; 3637 if (!(other_ instanceof AuditEvent)) 3638 return false; 3639 AuditEvent o = (AuditEvent) other_; 3640 return compareDeep(type, o.type, true) && compareDeep(subtype, o.subtype, true) && compareDeep(action, o.action, true) 3641 && compareDeep(recorded, o.recorded, true) && compareDeep(outcome, o.outcome, true) && compareDeep(outcomeDesc, o.outcomeDesc, true) 3642 && compareDeep(purposeOfEvent, o.purposeOfEvent, true) && compareDeep(agent, o.agent, true) && compareDeep(source, o.source, true) 3643 && compareDeep(entity, o.entity, true); 3644 } 3645 3646 @Override 3647 public boolean equalsShallow(Base other_) { 3648 if (!super.equalsShallow(other_)) 3649 return false; 3650 if (!(other_ instanceof AuditEvent)) 3651 return false; 3652 AuditEvent o = (AuditEvent) other_; 3653 return compareValues(action, o.action, true) && compareValues(recorded, o.recorded, true) && compareValues(outcome, o.outcome, true) 3654 && compareValues(outcomeDesc, o.outcomeDesc, true); 3655 } 3656 3657 public boolean isEmpty() { 3658 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subtype, action, recorded 3659 , outcome, outcomeDesc, purposeOfEvent, agent, source, entity); 3660 } 3661 3662 @Override 3663 public ResourceType getResourceType() { 3664 return ResourceType.AuditEvent; 3665 } 3666 3667 /** 3668 * Search parameter: <b>date</b> 3669 * <p> 3670 * Description: <b>Time when the event occurred on source</b><br> 3671 * Type: <b>date</b><br> 3672 * Path: <b>AuditEvent.recorded</b><br> 3673 * </p> 3674 */ 3675 @SearchParamDefinition(name="date", path="AuditEvent.recorded", description="Time when the event occurred on source", type="date" ) 3676 public static final String SP_DATE = "date"; 3677 /** 3678 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3679 * <p> 3680 * Description: <b>Time when the event occurred on source</b><br> 3681 * Type: <b>date</b><br> 3682 * Path: <b>AuditEvent.recorded</b><br> 3683 * </p> 3684 */ 3685 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3686 3687 /** 3688 * Search parameter: <b>entity-type</b> 3689 * <p> 3690 * Description: <b>Type of entity involved</b><br> 3691 * Type: <b>token</b><br> 3692 * Path: <b>AuditEvent.entity.type</b><br> 3693 * </p> 3694 */ 3695 @SearchParamDefinition(name="entity-type", path="AuditEvent.entity.type", description="Type of entity involved", type="token" ) 3696 public static final String SP_ENTITY_TYPE = "entity-type"; 3697 /** 3698 * <b>Fluent Client</b> search parameter constant for <b>entity-type</b> 3699 * <p> 3700 * Description: <b>Type of entity involved</b><br> 3701 * Type: <b>token</b><br> 3702 * Path: <b>AuditEvent.entity.type</b><br> 3703 * </p> 3704 */ 3705 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ENTITY_TYPE); 3706 3707 /** 3708 * Search parameter: <b>agent</b> 3709 * <p> 3710 * Description: <b>Direct reference to resource</b><br> 3711 * Type: <b>reference</b><br> 3712 * Path: <b>AuditEvent.agent.reference</b><br> 3713 * </p> 3714 */ 3715 @SearchParamDefinition(name="agent", path="AuditEvent.agent.reference", description="Direct reference to resource", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3716 public static final String SP_AGENT = "agent"; 3717 /** 3718 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 3719 * <p> 3720 * Description: <b>Direct reference to resource</b><br> 3721 * Type: <b>reference</b><br> 3722 * Path: <b>AuditEvent.agent.reference</b><br> 3723 * </p> 3724 */ 3725 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AGENT); 3726 3727/** 3728 * Constant for fluent queries to be used to add include statements. Specifies 3729 * the path value of "<b>AuditEvent:agent</b>". 3730 */ 3731 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include("AuditEvent:agent").toLocked(); 3732 3733 /** 3734 * Search parameter: <b>address</b> 3735 * <p> 3736 * Description: <b>Identifier for the network access point of the user device</b><br> 3737 * Type: <b>string</b><br> 3738 * Path: <b>AuditEvent.agent.network.address</b><br> 3739 * </p> 3740 */ 3741 @SearchParamDefinition(name="address", path="AuditEvent.agent.network.address", description="Identifier for the network access point of the user device", type="string" ) 3742 public static final String SP_ADDRESS = "address"; 3743 /** 3744 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3745 * <p> 3746 * Description: <b>Identifier for the network access point of the user device</b><br> 3747 * Type: <b>string</b><br> 3748 * Path: <b>AuditEvent.agent.network.address</b><br> 3749 * </p> 3750 */ 3751 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 3752 3753 /** 3754 * Search parameter: <b>entity-role</b> 3755 * <p> 3756 * Description: <b>What role the entity played</b><br> 3757 * Type: <b>token</b><br> 3758 * Path: <b>AuditEvent.entity.role</b><br> 3759 * </p> 3760 */ 3761 @SearchParamDefinition(name="entity-role", path="AuditEvent.entity.role", description="What role the entity played", type="token" ) 3762 public static final String SP_ENTITY_ROLE = "entity-role"; 3763 /** 3764 * <b>Fluent Client</b> search parameter constant for <b>entity-role</b> 3765 * <p> 3766 * Description: <b>What role the entity played</b><br> 3767 * Type: <b>token</b><br> 3768 * Path: <b>AuditEvent.entity.role</b><br> 3769 * </p> 3770 */ 3771 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ENTITY_ROLE); 3772 3773 /** 3774 * Search parameter: <b>source</b> 3775 * <p> 3776 * Description: <b>The identity of source detecting the event</b><br> 3777 * Type: <b>token</b><br> 3778 * Path: <b>AuditEvent.source.identifier</b><br> 3779 * </p> 3780 */ 3781 @SearchParamDefinition(name="source", path="AuditEvent.source.identifier", description="The identity of source detecting the event", type="token" ) 3782 public static final String SP_SOURCE = "source"; 3783 /** 3784 * <b>Fluent Client</b> search parameter constant for <b>source</b> 3785 * <p> 3786 * Description: <b>The identity of source detecting the event</b><br> 3787 * Type: <b>token</b><br> 3788 * Path: <b>AuditEvent.source.identifier</b><br> 3789 * </p> 3790 */ 3791 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SOURCE); 3792 3793 /** 3794 * Search parameter: <b>type</b> 3795 * <p> 3796 * Description: <b>Type/identifier of event</b><br> 3797 * Type: <b>token</b><br> 3798 * Path: <b>AuditEvent.type</b><br> 3799 * </p> 3800 */ 3801 @SearchParamDefinition(name="type", path="AuditEvent.type", description="Type/identifier of event", type="token" ) 3802 public static final String SP_TYPE = "type"; 3803 /** 3804 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3805 * <p> 3806 * Description: <b>Type/identifier of event</b><br> 3807 * Type: <b>token</b><br> 3808 * Path: <b>AuditEvent.type</b><br> 3809 * </p> 3810 */ 3811 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3812 3813 /** 3814 * Search parameter: <b>altid</b> 3815 * <p> 3816 * Description: <b>Alternative User id e.g. authentication</b><br> 3817 * Type: <b>token</b><br> 3818 * Path: <b>AuditEvent.agent.altId</b><br> 3819 * </p> 3820 */ 3821 @SearchParamDefinition(name="altid", path="AuditEvent.agent.altId", description="Alternative User id e.g. authentication", type="token" ) 3822 public static final String SP_ALTID = "altid"; 3823 /** 3824 * <b>Fluent Client</b> search parameter constant for <b>altid</b> 3825 * <p> 3826 * Description: <b>Alternative User id e.g. authentication</b><br> 3827 * Type: <b>token</b><br> 3828 * Path: <b>AuditEvent.agent.altId</b><br> 3829 * </p> 3830 */ 3831 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ALTID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ALTID); 3832 3833 /** 3834 * Search parameter: <b>site</b> 3835 * <p> 3836 * Description: <b>Logical source location within the enterprise</b><br> 3837 * Type: <b>token</b><br> 3838 * Path: <b>AuditEvent.source.site</b><br> 3839 * </p> 3840 */ 3841 @SearchParamDefinition(name="site", path="AuditEvent.source.site", description="Logical source location within the enterprise", type="token" ) 3842 public static final String SP_SITE = "site"; 3843 /** 3844 * <b>Fluent Client</b> search parameter constant for <b>site</b> 3845 * <p> 3846 * Description: <b>Logical source location within the enterprise</b><br> 3847 * Type: <b>token</b><br> 3848 * Path: <b>AuditEvent.source.site</b><br> 3849 * </p> 3850 */ 3851 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SITE); 3852 3853 /** 3854 * Search parameter: <b>agent-name</b> 3855 * <p> 3856 * Description: <b>Human-meaningful name for the agent</b><br> 3857 * Type: <b>string</b><br> 3858 * Path: <b>AuditEvent.agent.name</b><br> 3859 * </p> 3860 */ 3861 @SearchParamDefinition(name="agent-name", path="AuditEvent.agent.name", description="Human-meaningful name for the agent", type="string" ) 3862 public static final String SP_AGENT_NAME = "agent-name"; 3863 /** 3864 * <b>Fluent Client</b> search parameter constant for <b>agent-name</b> 3865 * <p> 3866 * Description: <b>Human-meaningful name for the agent</b><br> 3867 * Type: <b>string</b><br> 3868 * Path: <b>AuditEvent.agent.name</b><br> 3869 * </p> 3870 */ 3871 public static final ca.uhn.fhir.rest.gclient.StringClientParam AGENT_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_AGENT_NAME); 3872 3873 /** 3874 * Search parameter: <b>entity-name</b> 3875 * <p> 3876 * Description: <b>Descriptor for entity</b><br> 3877 * Type: <b>string</b><br> 3878 * Path: <b>AuditEvent.entity.name</b><br> 3879 * </p> 3880 */ 3881 @SearchParamDefinition(name="entity-name", path="AuditEvent.entity.name", description="Descriptor for entity", type="string" ) 3882 public static final String SP_ENTITY_NAME = "entity-name"; 3883 /** 3884 * <b>Fluent Client</b> search parameter constant for <b>entity-name</b> 3885 * <p> 3886 * Description: <b>Descriptor for entity</b><br> 3887 * Type: <b>string</b><br> 3888 * Path: <b>AuditEvent.entity.name</b><br> 3889 * </p> 3890 */ 3891 public static final ca.uhn.fhir.rest.gclient.StringClientParam ENTITY_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ENTITY_NAME); 3892 3893 /** 3894 * Search parameter: <b>subtype</b> 3895 * <p> 3896 * Description: <b>More specific type/id for the event</b><br> 3897 * Type: <b>token</b><br> 3898 * Path: <b>AuditEvent.subtype</b><br> 3899 * </p> 3900 */ 3901 @SearchParamDefinition(name="subtype", path="AuditEvent.subtype", description="More specific type/id for the event", type="token" ) 3902 public static final String SP_SUBTYPE = "subtype"; 3903 /** 3904 * <b>Fluent Client</b> search parameter constant for <b>subtype</b> 3905 * <p> 3906 * Description: <b>More specific type/id for the event</b><br> 3907 * Type: <b>token</b><br> 3908 * Path: <b>AuditEvent.subtype</b><br> 3909 * </p> 3910 */ 3911 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUBTYPE); 3912 3913 /** 3914 * Search parameter: <b>patient</b> 3915 * <p> 3916 * Description: <b>Direct reference to resource</b><br> 3917 * Type: <b>reference</b><br> 3918 * Path: <b>AuditEvent.agent.reference, AuditEvent.entity.reference</b><br> 3919 * </p> 3920 */ 3921 @SearchParamDefinition(name="patient", path="AuditEvent.agent.reference | AuditEvent.entity.reference", description="Direct reference to resource", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 3922 public static final String SP_PATIENT = "patient"; 3923 /** 3924 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3925 * <p> 3926 * Description: <b>Direct reference to resource</b><br> 3927 * Type: <b>reference</b><br> 3928 * Path: <b>AuditEvent.agent.reference, AuditEvent.entity.reference</b><br> 3929 * </p> 3930 */ 3931 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3932 3933/** 3934 * Constant for fluent queries to be used to add include statements. Specifies 3935 * the path value of "<b>AuditEvent:patient</b>". 3936 */ 3937 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AuditEvent:patient").toLocked(); 3938 3939 /** 3940 * Search parameter: <b>action</b> 3941 * <p> 3942 * Description: <b>Type of action performed during the event</b><br> 3943 * Type: <b>token</b><br> 3944 * Path: <b>AuditEvent.action</b><br> 3945 * </p> 3946 */ 3947 @SearchParamDefinition(name="action", path="AuditEvent.action", description="Type of action performed during the event", type="token" ) 3948 public static final String SP_ACTION = "action"; 3949 /** 3950 * <b>Fluent Client</b> search parameter constant for <b>action</b> 3951 * <p> 3952 * Description: <b>Type of action performed during the event</b><br> 3953 * Type: <b>token</b><br> 3954 * Path: <b>AuditEvent.action</b><br> 3955 * </p> 3956 */ 3957 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTION); 3958 3959 /** 3960 * Search parameter: <b>agent-role</b> 3961 * <p> 3962 * Description: <b>Agent role in the event</b><br> 3963 * Type: <b>token</b><br> 3964 * Path: <b>AuditEvent.agent.role</b><br> 3965 * </p> 3966 */ 3967 @SearchParamDefinition(name="agent-role", path="AuditEvent.agent.role", description="Agent role in the event", type="token" ) 3968 public static final String SP_AGENT_ROLE = "agent-role"; 3969 /** 3970 * <b>Fluent Client</b> search parameter constant for <b>agent-role</b> 3971 * <p> 3972 * Description: <b>Agent role in the event</b><br> 3973 * Type: <b>token</b><br> 3974 * Path: <b>AuditEvent.agent.role</b><br> 3975 * </p> 3976 */ 3977 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_AGENT_ROLE); 3978 3979 /** 3980 * Search parameter: <b>user</b> 3981 * <p> 3982 * Description: <b>Unique identifier for the user</b><br> 3983 * Type: <b>token</b><br> 3984 * Path: <b>AuditEvent.agent.userId</b><br> 3985 * </p> 3986 */ 3987 @SearchParamDefinition(name="user", path="AuditEvent.agent.userId", description="Unique identifier for the user", type="token" ) 3988 public static final String SP_USER = "user"; 3989 /** 3990 * <b>Fluent Client</b> search parameter constant for <b>user</b> 3991 * <p> 3992 * Description: <b>Unique identifier for the user</b><br> 3993 * Type: <b>token</b><br> 3994 * Path: <b>AuditEvent.agent.userId</b><br> 3995 * </p> 3996 */ 3997 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_USER); 3998 3999 /** 4000 * Search parameter: <b>entity</b> 4001 * <p> 4002 * Description: <b>Specific instance of resource</b><br> 4003 * Type: <b>reference</b><br> 4004 * Path: <b>AuditEvent.entity.reference</b><br> 4005 * </p> 4006 */ 4007 @SearchParamDefinition(name="entity", path="AuditEvent.entity.reference", description="Specific instance of resource", type="reference" ) 4008 public static final String SP_ENTITY = "entity"; 4009 /** 4010 * <b>Fluent Client</b> search parameter constant for <b>entity</b> 4011 * <p> 4012 * Description: <b>Specific instance of resource</b><br> 4013 * Type: <b>reference</b><br> 4014 * Path: <b>AuditEvent.entity.reference</b><br> 4015 * </p> 4016 */ 4017 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTITY); 4018 4019/** 4020 * Constant for fluent queries to be used to add include statements. Specifies 4021 * the path value of "<b>AuditEvent:entity</b>". 4022 */ 4023 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTITY = new ca.uhn.fhir.model.api.Include("AuditEvent:entity").toLocked(); 4024 4025 /** 4026 * Search parameter: <b>entity-id</b> 4027 * <p> 4028 * Description: <b>Specific instance of object</b><br> 4029 * Type: <b>token</b><br> 4030 * Path: <b>AuditEvent.entity.identifier</b><br> 4031 * </p> 4032 */ 4033 @SearchParamDefinition(name="entity-id", path="AuditEvent.entity.identifier", description="Specific instance of object", type="token" ) 4034 public static final String SP_ENTITY_ID = "entity-id"; 4035 /** 4036 * <b>Fluent Client</b> search parameter constant for <b>entity-id</b> 4037 * <p> 4038 * Description: <b>Specific instance of object</b><br> 4039 * Type: <b>token</b><br> 4040 * Path: <b>AuditEvent.entity.identifier</b><br> 4041 * </p> 4042 */ 4043 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ENTITY_ID); 4044 4045 /** 4046 * Search parameter: <b>outcome</b> 4047 * <p> 4048 * Description: <b>Whether the event succeeded or failed</b><br> 4049 * Type: <b>token</b><br> 4050 * Path: <b>AuditEvent.outcome</b><br> 4051 * </p> 4052 */ 4053 @SearchParamDefinition(name="outcome", path="AuditEvent.outcome", description="Whether the event succeeded or failed", type="token" ) 4054 public static final String SP_OUTCOME = "outcome"; 4055 /** 4056 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 4057 * <p> 4058 * Description: <b>Whether the event succeeded or failed</b><br> 4059 * Type: <b>token</b><br> 4060 * Path: <b>AuditEvent.outcome</b><br> 4061 * </p> 4062 */ 4063 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 4064 4065 /** 4066 * Search parameter: <b>policy</b> 4067 * <p> 4068 * Description: <b>Policy that authorized event</b><br> 4069 * Type: <b>uri</b><br> 4070 * Path: <b>AuditEvent.agent.policy</b><br> 4071 * </p> 4072 */ 4073 @SearchParamDefinition(name="policy", path="AuditEvent.agent.policy", description="Policy that authorized event", type="uri" ) 4074 public static final String SP_POLICY = "policy"; 4075 /** 4076 * <b>Fluent Client</b> search parameter constant for <b>policy</b> 4077 * <p> 4078 * Description: <b>Policy that authorized event</b><br> 4079 * Type: <b>uri</b><br> 4080 * Path: <b>AuditEvent.agent.policy</b><br> 4081 * </p> 4082 */ 4083 public static final ca.uhn.fhir.rest.gclient.UriClientParam POLICY = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_POLICY); 4084 4085 4086}