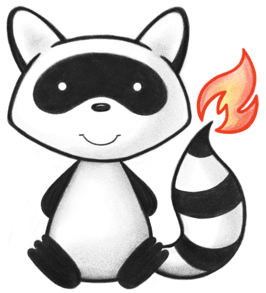
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * Base definition for all elements that are defined inside a resource - but not those in a data type. 047 */ 048@DatatypeDef(name="BackboneElement") 049public abstract class BackboneElement extends Element implements IBaseBackboneElement { 050 051 /** 052 * May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 053 */ 054 @Child(name = "modifierExtension", type = {Extension.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 055 @Description(shortDefinition="Extensions that cannot be ignored", formalDefinition="May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions." ) 056 protected List<Extension> modifierExtension; 057 058 private static final long serialVersionUID = -1431673179L; 059 060 /** 061 * Constructor 062 */ 063 public BackboneElement() { 064 super(); 065 } 066 067 /** 068 * @return {@link #modifierExtension} (May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.) 069 */ 070 public List<Extension> getModifierExtension() { 071 if (this.modifierExtension == null) 072 this.modifierExtension = new ArrayList<Extension>(); 073 return this.modifierExtension; 074 } 075 076 /** 077 * @return Returns a reference to <code>this</code> for easy method chaining 078 */ 079 public BackboneElement setModifierExtension(List<Extension> theModifierExtension) { 080 this.modifierExtension = theModifierExtension; 081 return this; 082 } 083 084 public boolean hasModifierExtension() { 085 if (this.modifierExtension == null) 086 return false; 087 for (Extension item : this.modifierExtension) 088 if (!item.isEmpty()) 089 return true; 090 return false; 091 } 092 093 public Extension addModifierExtension() { //3 094 Extension t = new Extension(); 095 if (this.modifierExtension == null) 096 this.modifierExtension = new ArrayList<Extension>(); 097 this.modifierExtension.add(t); 098 return t; 099 } 100 101 public BackboneElement addModifierExtension(Extension t) { //3 102 if (t == null) 103 return this; 104 if (this.modifierExtension == null) 105 this.modifierExtension = new ArrayList<Extension>(); 106 this.modifierExtension.add(t); 107 return this; 108 } 109 110 /** 111 * @return The first repetition of repeating field {@link #modifierExtension}, creating it if it does not already exist 112 */ 113 public Extension getModifierExtensionFirstRep() { 114 if (getModifierExtension().isEmpty()) { 115 addModifierExtension(); 116 } 117 return getModifierExtension().get(0); 118 } 119 120 protected void listChildren(List<Property> children) { 121 children.add(new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 122 } 123 124 @Override 125 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 126 switch (_hash) { 127 case -298878168: /*modifierExtension*/ return new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 0, java.lang.Integer.MAX_VALUE, modifierExtension); 128 default: return super.getNamedProperty(_hash, _name, _checkValid); 129 } 130 131 } 132 133 @Override 134 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 135 switch (hash) { 136 case -298878168: /*modifierExtension*/ return this.modifierExtension == null ? new Base[0] : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 137 default: return super.getProperty(hash, name, checkValid); 138 } 139 140 } 141 142 @Override 143 public Base setProperty(int hash, String name, Base value) throws FHIRException { 144 switch (hash) { 145 case -298878168: // modifierExtension 146 this.getModifierExtension().add(castToExtension(value)); // Extension 147 return value; 148 default: return super.setProperty(hash, name, value); 149 } 150 151 } 152 153 @Override 154 public Base setProperty(String name, Base value) throws FHIRException { 155 if (name.equals("modifierExtension")) { 156 this.getModifierExtension().add(castToExtension(value)); 157 } else 158 return super.setProperty(name, value); 159 return value; 160 } 161 162 @Override 163 public Base makeProperty(int hash, String name) throws FHIRException { 164 switch (hash) { 165 case -298878168: return addModifierExtension(); 166 default: return super.makeProperty(hash, name); 167 } 168 169 } 170 171 @Override 172 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 173 switch (hash) { 174 case -298878168: /*modifierExtension*/ return new String[] {"Extension"}; 175 default: return super.getTypesForProperty(hash, name); 176 } 177 178 } 179 180 @Override 181 public Base addChild(String name) throws FHIRException { 182 if (name.equals("modifierExtension")) { 183 return addModifierExtension(); 184 } 185 else 186 return super.addChild(name); 187 } 188 189 public String fhirType() { 190 return "BackboneElement"; 191 192 } 193 194 public abstract BackboneElement copy(); 195 196 public void copyValues(BackboneElement dst) { 197 super.copyValues(dst); 198 if (modifierExtension != null) { 199 dst.modifierExtension = new ArrayList<Extension>(); 200 for (Extension i : modifierExtension) 201 dst.modifierExtension.add(i.copy()); 202 }; 203 } 204 205 @Override 206 public boolean equalsDeep(Base other_) { 207 if (!super.equalsDeep(other_)) 208 return false; 209 if (!(other_ instanceof BackboneElement)) 210 return false; 211 BackboneElement o = (BackboneElement) other_; 212 return compareDeep(modifierExtension, o.modifierExtension, true); 213 } 214 215 @Override 216 public boolean equalsShallow(Base other_) { 217 if (!super.equalsShallow(other_)) 218 return false; 219 if (!(other_ instanceof BackboneElement)) 220 return false; 221 BackboneElement o = (BackboneElement) other_; 222 return true; 223 } 224 225 public boolean isEmpty() { 226 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifierExtension); 227 } 228 229 230}