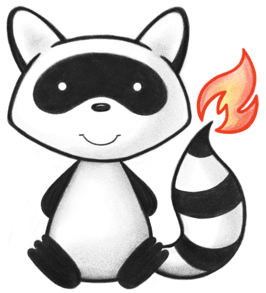
001package org.hl7.fhir.dstu3.model; 002 003import java.io.Externalizable; 004import java.io.IOException; 005import java.io.ObjectInput; 006import java.io.ObjectOutput; 007 008import org.apache.commons.codec.binary.Base64; 009import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 010import org.hl7.fhir.instance.model.api.IPrimitiveType; 011 012/* 013 Copyright (c) 2011+, HL7, Inc. 014 All rights reserved. 015 016 Redistribution and use in source and binary forms, with or without modification, 017 are permitted provided that the following conditions are met: 018 019 * Redistributions of source code must retain the above copyright notice, this 020 list of conditions and the following disclaimer. 021 * Redistributions in binary form must reproduce the above copyright notice, 022 this list of conditions and the following disclaimer in the documentation 023 and/or other materials provided with the distribution. 024 * Neither the name of HL7 nor the names of its contributors may be used to 025 endorse or promote products derived from this software without specific 026 prior written permission. 027 028 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 029 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 030 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 031 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 032 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 033 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 034 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 035 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 036 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 037 POSSIBILITY OF SUCH DAMAGE. 038 039 */ 040 041 042 043import ca.uhn.fhir.model.api.IElement; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.parser.DataFormatException; 046 047/** 048 * Primitive type "base64Binary" in FHIR: a sequence of bytes represented in base64 049 */ 050@DatatypeDef(name = "base64Binary") 051public class Base64BinaryType extends PrimitiveType<byte[]> implements IPrimitiveType<byte[]>, IBaseHasExtensions, IElement, Externalizable { 052 053 private static final long serialVersionUID = 3L; 054 private byte[] myValue; 055 056 /** 057 * Constructor 058 */ 059 public Base64BinaryType() { 060 super(); 061 } 062 063 public Base64BinaryType(byte[] theBytes) { 064 super(); 065 setValue(theBytes); 066 } 067 068 public Base64BinaryType(String theValue) { 069 super(); 070 // Null values still result in non-null instance being created 071 setValueAsString(theValue); 072 } 073 074 protected byte[] parse(String theValue) { 075 if (theValue != null) { 076 return Base64.decodeBase64(theValue.getBytes(ca.uhn.fhir.rest.api.Constants.CHARSET_UTF8)); 077 } else { 078 return null; 079 } 080 } 081 082 protected String encode(byte[] theValue) { 083 if (theValue == null) { 084 return null; 085 } 086 return new String(Base64.encodeBase64(theValue), ca.uhn.fhir.rest.api.Constants.CHARSET_UTF8); 087 } 088 089 @Override 090 public Base64BinaryType copy() { 091 return new Base64BinaryType(getValue()); 092 } 093 094 @Override 095 protected Type typedCopy() { 096 return copy(); 097 } 098 099 public String fhirType() { 100 return "base64Binary"; 101 } 102 103 @Override 104 public void writeExternal(ObjectOutput out) throws IOException { 105 out.writeObject(getValue()); 106 } 107 108 @Override 109 public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException { 110 setValue((byte[]) in.readObject()); 111 } 112 113 @Override 114 public String getValueAsString() { 115 return encode(myValue); 116 } 117 118 @Override 119 public void setValueAsString(String theValue) throws IllegalArgumentException { 120 // Null values still result in non-null instance being created 121 if (theValue != null) checkValidBase64(theValue); 122 fromStringValue(theValue); 123 setValue(parse(theValue)); 124 } 125 126 @Override 127 public byte[] getValue() { 128 return myValue; 129 } 130 131 @Override 132 public Base64BinaryType setValue(byte[] theValue) throws IllegalArgumentException { 133 myValue = theValue; 134 return (Base64BinaryType) super.setValue(theValue); 135 } 136 137 @Override 138 public boolean hasValue() { 139 return myValue != null && myValue.length > 0; 140 } 141 142 @Override 143 public boolean isEmpty() { 144 // Custom isEmpty() in order to avoid generating the text representation unneccessarily 145 return ca.uhn.fhir.util.ElementUtil.isEmpty(id, extension) && !hasValue(); 146 } 147 148 /** 149 * Checks if the passed in String is a valid {@link Base64} encoded String. Will throw a {@link DataFormatException} if not 150 * formatted correctly. 151 * 152 * @param toCheck {@link String} to check if valid {@link Base64} 153 * @throws DataFormatException 154 */ 155 public void checkValidBase64(String toCheck) throws DataFormatException { 156 if (!org.hl7.fhir.utilities.Base64.isBase64(toCheck.getBytes())) { 157 throw new DataFormatException(""); 158 } 159 } 160}