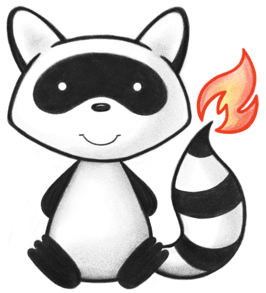
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045/** 046 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 047 */ 048@ResourceDef(name="Basic", profile="http://hl7.org/fhir/Profile/Basic") 049public class Basic extends DomainResource { 050 051 /** 052 * Identifier assigned to the resource for business purposes, outside the context of FHIR. 053 */ 054 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 055 @Description(shortDefinition="Business identifier", formalDefinition="Identifier assigned to the resource for business purposes, outside the context of FHIR." ) 056 protected List<Identifier> identifier; 057 058 /** 059 * Identifies the 'type' of resource - equivalent to the resource name for other resources. 060 */ 061 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=true, summary=true) 062 @Description(shortDefinition="Kind of Resource", formalDefinition="Identifies the 'type' of resource - equivalent to the resource name for other resources." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/basic-resource-type") 064 protected CodeableConcept code; 065 066 /** 067 * Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource. 068 */ 069 @Child(name = "subject", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="Identifies the focus of this resource", formalDefinition="Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource." ) 071 protected Reference subject; 072 073 /** 074 * The actual object that is the target of the reference (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 075 */ 076 protected Resource subjectTarget; 077 078 /** 079 * Identifies when the resource was first created. 080 */ 081 @Child(name = "created", type = {DateType.class}, order=3, min=0, max=1, modifier=false, summary=true) 082 @Description(shortDefinition="When created", formalDefinition="Identifies when the resource was first created." ) 083 protected DateType created; 084 085 /** 086 * Indicates who was responsible for creating the resource instance. 087 */ 088 @Child(name = "author", type = {Practitioner.class, Patient.class, RelatedPerson.class}, order=4, min=0, max=1, modifier=false, summary=true) 089 @Description(shortDefinition="Who created", formalDefinition="Indicates who was responsible for creating the resource instance." ) 090 protected Reference author; 091 092 /** 093 * The actual object that is the target of the reference (Indicates who was responsible for creating the resource instance.) 094 */ 095 protected Resource authorTarget; 096 097 private static final long serialVersionUID = 650756402L; 098 099 /** 100 * Constructor 101 */ 102 public Basic() { 103 super(); 104 } 105 106 /** 107 * Constructor 108 */ 109 public Basic(CodeableConcept code) { 110 super(); 111 this.code = code; 112 } 113 114 /** 115 * @return {@link #identifier} (Identifier assigned to the resource for business purposes, outside the context of FHIR.) 116 */ 117 public List<Identifier> getIdentifier() { 118 if (this.identifier == null) 119 this.identifier = new ArrayList<Identifier>(); 120 return this.identifier; 121 } 122 123 /** 124 * @return Returns a reference to <code>this</code> for easy method chaining 125 */ 126 public Basic setIdentifier(List<Identifier> theIdentifier) { 127 this.identifier = theIdentifier; 128 return this; 129 } 130 131 public boolean hasIdentifier() { 132 if (this.identifier == null) 133 return false; 134 for (Identifier item : this.identifier) 135 if (!item.isEmpty()) 136 return true; 137 return false; 138 } 139 140 public Identifier addIdentifier() { //3 141 Identifier t = new Identifier(); 142 if (this.identifier == null) 143 this.identifier = new ArrayList<Identifier>(); 144 this.identifier.add(t); 145 return t; 146 } 147 148 public Basic addIdentifier(Identifier t) { //3 149 if (t == null) 150 return this; 151 if (this.identifier == null) 152 this.identifier = new ArrayList<Identifier>(); 153 this.identifier.add(t); 154 return this; 155 } 156 157 /** 158 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 159 */ 160 public Identifier getIdentifierFirstRep() { 161 if (getIdentifier().isEmpty()) { 162 addIdentifier(); 163 } 164 return getIdentifier().get(0); 165 } 166 167 /** 168 * @return {@link #code} (Identifies the 'type' of resource - equivalent to the resource name for other resources.) 169 */ 170 public CodeableConcept getCode() { 171 if (this.code == null) 172 if (Configuration.errorOnAutoCreate()) 173 throw new Error("Attempt to auto-create Basic.code"); 174 else if (Configuration.doAutoCreate()) 175 this.code = new CodeableConcept(); // cc 176 return this.code; 177 } 178 179 public boolean hasCode() { 180 return this.code != null && !this.code.isEmpty(); 181 } 182 183 /** 184 * @param value {@link #code} (Identifies the 'type' of resource - equivalent to the resource name for other resources.) 185 */ 186 public Basic setCode(CodeableConcept value) { 187 this.code = value; 188 return this; 189 } 190 191 /** 192 * @return {@link #subject} (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 193 */ 194 public Reference getSubject() { 195 if (this.subject == null) 196 if (Configuration.errorOnAutoCreate()) 197 throw new Error("Attempt to auto-create Basic.subject"); 198 else if (Configuration.doAutoCreate()) 199 this.subject = new Reference(); // cc 200 return this.subject; 201 } 202 203 public boolean hasSubject() { 204 return this.subject != null && !this.subject.isEmpty(); 205 } 206 207 /** 208 * @param value {@link #subject} (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 209 */ 210 public Basic setSubject(Reference value) { 211 this.subject = value; 212 return this; 213 } 214 215 /** 216 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 217 */ 218 public Resource getSubjectTarget() { 219 return this.subjectTarget; 220 } 221 222 /** 223 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 224 */ 225 public Basic setSubjectTarget(Resource value) { 226 this.subjectTarget = value; 227 return this; 228 } 229 230 /** 231 * @return {@link #created} (Identifies when the resource was first created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 232 */ 233 public DateType getCreatedElement() { 234 if (this.created == null) 235 if (Configuration.errorOnAutoCreate()) 236 throw new Error("Attempt to auto-create Basic.created"); 237 else if (Configuration.doAutoCreate()) 238 this.created = new DateType(); // bb 239 return this.created; 240 } 241 242 public boolean hasCreatedElement() { 243 return this.created != null && !this.created.isEmpty(); 244 } 245 246 public boolean hasCreated() { 247 return this.created != null && !this.created.isEmpty(); 248 } 249 250 /** 251 * @param value {@link #created} (Identifies when the resource was first created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 252 */ 253 public Basic setCreatedElement(DateType value) { 254 this.created = value; 255 return this; 256 } 257 258 /** 259 * @return Identifies when the resource was first created. 260 */ 261 public Date getCreated() { 262 return this.created == null ? null : this.created.getValue(); 263 } 264 265 /** 266 * @param value Identifies when the resource was first created. 267 */ 268 public Basic setCreated(Date value) { 269 if (value == null) 270 this.created = null; 271 else { 272 if (this.created == null) 273 this.created = new DateType(); 274 this.created.setValue(value); 275 } 276 return this; 277 } 278 279 /** 280 * @return {@link #author} (Indicates who was responsible for creating the resource instance.) 281 */ 282 public Reference getAuthor() { 283 if (this.author == null) 284 if (Configuration.errorOnAutoCreate()) 285 throw new Error("Attempt to auto-create Basic.author"); 286 else if (Configuration.doAutoCreate()) 287 this.author = new Reference(); // cc 288 return this.author; 289 } 290 291 public boolean hasAuthor() { 292 return this.author != null && !this.author.isEmpty(); 293 } 294 295 /** 296 * @param value {@link #author} (Indicates who was responsible for creating the resource instance.) 297 */ 298 public Basic setAuthor(Reference value) { 299 this.author = value; 300 return this; 301 } 302 303 /** 304 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates who was responsible for creating the resource instance.) 305 */ 306 public Resource getAuthorTarget() { 307 return this.authorTarget; 308 } 309 310 /** 311 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates who was responsible for creating the resource instance.) 312 */ 313 public Basic setAuthorTarget(Resource value) { 314 this.authorTarget = value; 315 return this; 316 } 317 318 protected void listChildren(List<Property> children) { 319 super.listChildren(children); 320 children.add(new Property("identifier", "Identifier", "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier)); 321 children.add(new Property("code", "CodeableConcept", "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code)); 322 children.add(new Property("subject", "Reference(Any)", "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 1, subject)); 323 children.add(new Property("created", "date", "Identifies when the resource was first created.", 0, 1, created)); 324 children.add(new Property("author", "Reference(Practitioner|Patient|RelatedPerson)", "Indicates who was responsible for creating the resource instance.", 0, 1, author)); 325 } 326 327 @Override 328 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 329 switch (_hash) { 330 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier); 331 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code); 332 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 1, subject); 333 case 1028554472: /*created*/ return new Property("created", "date", "Identifies when the resource was first created.", 0, 1, created); 334 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Patient|RelatedPerson)", "Indicates who was responsible for creating the resource instance.", 0, 1, author); 335 default: return super.getNamedProperty(_hash, _name, _checkValid); 336 } 337 338 } 339 340 @Override 341 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 342 switch (hash) { 343 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 344 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 345 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 346 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateType 347 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 348 default: return super.getProperty(hash, name, checkValid); 349 } 350 351 } 352 353 @Override 354 public Base setProperty(int hash, String name, Base value) throws FHIRException { 355 switch (hash) { 356 case -1618432855: // identifier 357 this.getIdentifier().add(castToIdentifier(value)); // Identifier 358 return value; 359 case 3059181: // code 360 this.code = castToCodeableConcept(value); // CodeableConcept 361 return value; 362 case -1867885268: // subject 363 this.subject = castToReference(value); // Reference 364 return value; 365 case 1028554472: // created 366 this.created = castToDate(value); // DateType 367 return value; 368 case -1406328437: // author 369 this.author = castToReference(value); // Reference 370 return value; 371 default: return super.setProperty(hash, name, value); 372 } 373 374 } 375 376 @Override 377 public Base setProperty(String name, Base value) throws FHIRException { 378 if (name.equals("identifier")) { 379 this.getIdentifier().add(castToIdentifier(value)); 380 } else if (name.equals("code")) { 381 this.code = castToCodeableConcept(value); // CodeableConcept 382 } else if (name.equals("subject")) { 383 this.subject = castToReference(value); // Reference 384 } else if (name.equals("created")) { 385 this.created = castToDate(value); // DateType 386 } else if (name.equals("author")) { 387 this.author = castToReference(value); // Reference 388 } else 389 return super.setProperty(name, value); 390 return value; 391 } 392 393 @Override 394 public Base makeProperty(int hash, String name) throws FHIRException { 395 switch (hash) { 396 case -1618432855: return addIdentifier(); 397 case 3059181: return getCode(); 398 case -1867885268: return getSubject(); 399 case 1028554472: return getCreatedElement(); 400 case -1406328437: return getAuthor(); 401 default: return super.makeProperty(hash, name); 402 } 403 404 } 405 406 @Override 407 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 408 switch (hash) { 409 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 410 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 411 case -1867885268: /*subject*/ return new String[] {"Reference"}; 412 case 1028554472: /*created*/ return new String[] {"date"}; 413 case -1406328437: /*author*/ return new String[] {"Reference"}; 414 default: return super.getTypesForProperty(hash, name); 415 } 416 417 } 418 419 @Override 420 public Base addChild(String name) throws FHIRException { 421 if (name.equals("identifier")) { 422 return addIdentifier(); 423 } 424 else if (name.equals("code")) { 425 this.code = new CodeableConcept(); 426 return this.code; 427 } 428 else if (name.equals("subject")) { 429 this.subject = new Reference(); 430 return this.subject; 431 } 432 else if (name.equals("created")) { 433 throw new FHIRException("Cannot call addChild on a singleton property Basic.created"); 434 } 435 else if (name.equals("author")) { 436 this.author = new Reference(); 437 return this.author; 438 } 439 else 440 return super.addChild(name); 441 } 442 443 public String fhirType() { 444 return "Basic"; 445 446 } 447 448 public Basic copy() { 449 Basic dst = new Basic(); 450 copyValues(dst); 451 if (identifier != null) { 452 dst.identifier = new ArrayList<Identifier>(); 453 for (Identifier i : identifier) 454 dst.identifier.add(i.copy()); 455 }; 456 dst.code = code == null ? null : code.copy(); 457 dst.subject = subject == null ? null : subject.copy(); 458 dst.created = created == null ? null : created.copy(); 459 dst.author = author == null ? null : author.copy(); 460 return dst; 461 } 462 463 protected Basic typedCopy() { 464 return copy(); 465 } 466 467 @Override 468 public boolean equalsDeep(Base other_) { 469 if (!super.equalsDeep(other_)) 470 return false; 471 if (!(other_ instanceof Basic)) 472 return false; 473 Basic o = (Basic) other_; 474 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 475 && compareDeep(created, o.created, true) && compareDeep(author, o.author, true); 476 } 477 478 @Override 479 public boolean equalsShallow(Base other_) { 480 if (!super.equalsShallow(other_)) 481 return false; 482 if (!(other_ instanceof Basic)) 483 return false; 484 Basic o = (Basic) other_; 485 return compareValues(created, o.created, true); 486 } 487 488 public boolean isEmpty() { 489 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, subject 490 , created, author); 491 } 492 493 @Override 494 public ResourceType getResourceType() { 495 return ResourceType.Basic; 496 } 497 498 /** 499 * Search parameter: <b>identifier</b> 500 * <p> 501 * Description: <b>Business identifier</b><br> 502 * Type: <b>token</b><br> 503 * Path: <b>Basic.identifier</b><br> 504 * </p> 505 */ 506 @SearchParamDefinition(name="identifier", path="Basic.identifier", description="Business identifier", type="token" ) 507 public static final String SP_IDENTIFIER = "identifier"; 508 /** 509 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 510 * <p> 511 * Description: <b>Business identifier</b><br> 512 * Type: <b>token</b><br> 513 * Path: <b>Basic.identifier</b><br> 514 * </p> 515 */ 516 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 517 518 /** 519 * Search parameter: <b>code</b> 520 * <p> 521 * Description: <b>Kind of Resource</b><br> 522 * Type: <b>token</b><br> 523 * Path: <b>Basic.code</b><br> 524 * </p> 525 */ 526 @SearchParamDefinition(name="code", path="Basic.code", description="Kind of Resource", type="token" ) 527 public static final String SP_CODE = "code"; 528 /** 529 * <b>Fluent Client</b> search parameter constant for <b>code</b> 530 * <p> 531 * Description: <b>Kind of Resource</b><br> 532 * Type: <b>token</b><br> 533 * Path: <b>Basic.code</b><br> 534 * </p> 535 */ 536 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 537 538 /** 539 * Search parameter: <b>subject</b> 540 * <p> 541 * Description: <b>Identifies the focus of this resource</b><br> 542 * Type: <b>reference</b><br> 543 * Path: <b>Basic.subject</b><br> 544 * </p> 545 */ 546 @SearchParamDefinition(name="subject", path="Basic.subject", description="Identifies the focus of this resource", type="reference" ) 547 public static final String SP_SUBJECT = "subject"; 548 /** 549 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 550 * <p> 551 * Description: <b>Identifies the focus of this resource</b><br> 552 * Type: <b>reference</b><br> 553 * Path: <b>Basic.subject</b><br> 554 * </p> 555 */ 556 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 557 558/** 559 * Constant for fluent queries to be used to add include statements. Specifies 560 * the path value of "<b>Basic:subject</b>". 561 */ 562 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Basic:subject").toLocked(); 563 564 /** 565 * Search parameter: <b>created</b> 566 * <p> 567 * Description: <b>When created</b><br> 568 * Type: <b>date</b><br> 569 * Path: <b>Basic.created</b><br> 570 * </p> 571 */ 572 @SearchParamDefinition(name="created", path="Basic.created", description="When created", type="date" ) 573 public static final String SP_CREATED = "created"; 574 /** 575 * <b>Fluent Client</b> search parameter constant for <b>created</b> 576 * <p> 577 * Description: <b>When created</b><br> 578 * Type: <b>date</b><br> 579 * Path: <b>Basic.created</b><br> 580 * </p> 581 */ 582 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 583 584 /** 585 * Search parameter: <b>patient</b> 586 * <p> 587 * Description: <b>Identifies the focus of this resource</b><br> 588 * Type: <b>reference</b><br> 589 * Path: <b>Basic.subject</b><br> 590 * </p> 591 */ 592 @SearchParamDefinition(name="patient", path="Basic.subject", description="Identifies the focus of this resource", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 593 public static final String SP_PATIENT = "patient"; 594 /** 595 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 596 * <p> 597 * Description: <b>Identifies the focus of this resource</b><br> 598 * Type: <b>reference</b><br> 599 * Path: <b>Basic.subject</b><br> 600 * </p> 601 */ 602 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 603 604/** 605 * Constant for fluent queries to be used to add include statements. Specifies 606 * the path value of "<b>Basic:patient</b>". 607 */ 608 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Basic:patient").toLocked(); 609 610 /** 611 * Search parameter: <b>author</b> 612 * <p> 613 * Description: <b>Who created</b><br> 614 * Type: <b>reference</b><br> 615 * Path: <b>Basic.author</b><br> 616 * </p> 617 */ 618 @SearchParamDefinition(name="author", path="Basic.author", description="Who created", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Practitioner.class, RelatedPerson.class } ) 619 public static final String SP_AUTHOR = "author"; 620 /** 621 * <b>Fluent Client</b> search parameter constant for <b>author</b> 622 * <p> 623 * Description: <b>Who created</b><br> 624 * Type: <b>reference</b><br> 625 * Path: <b>Basic.author</b><br> 626 * </p> 627 */ 628 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 629 630/** 631 * Constant for fluent queries to be used to add include statements. Specifies 632 * the path value of "<b>Basic:author</b>". 633 */ 634 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Basic:author").toLocked(); 635 636 637}