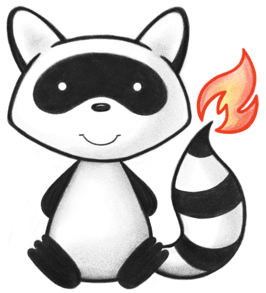
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBinary; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044/** 045 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 046 */ 047@ResourceDef(name="Binary", profile="http://hl7.org/fhir/Profile/Binary") 048public class Binary extends BaseBinary implements IBaseBinary { 049 050 /** 051 * MimeType of the binary content represented as a standard MimeType (BCP 13). 052 */ 053 @Child(name = "contentType", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 054 @Description(shortDefinition="MimeType of the binary content", formalDefinition="MimeType of the binary content represented as a standard MimeType (BCP 13)." ) 055 protected CodeType contentType; 056 057 /** 058 * Treat this binary as if it was this other resource for access control purposes. 059 */ 060 @Child(name = "securityContext", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="Access Control Management", formalDefinition="Treat this binary as if it was this other resource for access control purposes." ) 062 protected Reference securityContext; 063 064 /** 065 * The actual object that is the target of the reference (Treat this binary as if it was this other resource for access control purposes.) 066 */ 067 protected Resource securityContextTarget; 068 069 /** 070 * The actual content, base64 encoded. 071 */ 072 @Child(name = "content", type = {Base64BinaryType.class}, order=2, min=1, max=1, modifier=false, summary=false) 073 @Description(shortDefinition="The actual content", formalDefinition="The actual content, base64 encoded." ) 074 protected Base64BinaryType content; 075 076 private static final long serialVersionUID = 1111991335L; 077 078 /** 079 * Constructor 080 */ 081 public Binary() { 082 super(); 083 } 084 085 /** 086 * Constructor 087 */ 088 public Binary(CodeType contentType, Base64BinaryType content) { 089 super(); 090 this.contentType = contentType; 091 this.content = content; 092 } 093 094 /** 095 * @return {@link #contentType} (MimeType of the binary content represented as a standard MimeType (BCP 13).). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 096 */ 097 public CodeType getContentTypeElement() { 098 if (this.contentType == null) 099 if (Configuration.errorOnAutoCreate()) 100 throw new Error("Attempt to auto-create Binary.contentType"); 101 else if (Configuration.doAutoCreate()) 102 this.contentType = new CodeType(); // bb 103 return this.contentType; 104 } 105 106 public boolean hasContentTypeElement() { 107 return this.contentType != null && !this.contentType.isEmpty(); 108 } 109 110 public boolean hasContentType() { 111 return this.contentType != null && !this.contentType.isEmpty(); 112 } 113 114 /** 115 * @param value {@link #contentType} (MimeType of the binary content represented as a standard MimeType (BCP 13).). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 116 */ 117 public Binary setContentTypeElement(CodeType value) { 118 this.contentType = value; 119 return this; 120 } 121 122 /** 123 * @return MimeType of the binary content represented as a standard MimeType (BCP 13). 124 */ 125 public String getContentType() { 126 return this.contentType == null ? null : this.contentType.getValue(); 127 } 128 129 /** 130 * @param value MimeType of the binary content represented as a standard MimeType (BCP 13). 131 */ 132 public Binary setContentType(String value) { 133 if (this.contentType == null) 134 this.contentType = new CodeType(); 135 this.contentType.setValue(value); 136 return this; 137 } 138 139 /** 140 * @return {@link #securityContext} (Treat this binary as if it was this other resource for access control purposes.) 141 */ 142 public Reference getSecurityContext() { 143 if (this.securityContext == null) 144 if (Configuration.errorOnAutoCreate()) 145 throw new Error("Attempt to auto-create Binary.securityContext"); 146 else if (Configuration.doAutoCreate()) 147 this.securityContext = new Reference(); // cc 148 return this.securityContext; 149 } 150 151 public boolean hasSecurityContext() { 152 return this.securityContext != null && !this.securityContext.isEmpty(); 153 } 154 155 /** 156 * @param value {@link #securityContext} (Treat this binary as if it was this other resource for access control purposes.) 157 */ 158 public Binary setSecurityContext(Reference value) { 159 this.securityContext = value; 160 return this; 161 } 162 163 /** 164 * @return {@link #securityContext} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Treat this binary as if it was this other resource for access control purposes.) 165 */ 166 public Resource getSecurityContextTarget() { 167 return this.securityContextTarget; 168 } 169 170 /** 171 * @param value {@link #securityContext} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Treat this binary as if it was this other resource for access control purposes.) 172 */ 173 public Binary setSecurityContextTarget(Resource value) { 174 this.securityContextTarget = value; 175 return this; 176 } 177 178 /** 179 * @return {@link #content} (The actual content, base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 180 */ 181 public Base64BinaryType getContentElement() { 182 if (this.content == null) 183 if (Configuration.errorOnAutoCreate()) 184 throw new Error("Attempt to auto-create Binary.content"); 185 else if (Configuration.doAutoCreate()) 186 this.content = new Base64BinaryType(); // bb 187 return this.content; 188 } 189 190 public boolean hasContentElement() { 191 return this.content != null && !this.content.isEmpty(); 192 } 193 194 public boolean hasContent() { 195 return this.content != null && !this.content.isEmpty(); 196 } 197 198 /** 199 * @param value {@link #content} (The actual content, base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 200 */ 201 public Binary setContentElement(Base64BinaryType value) { 202 this.content = value; 203 return this; 204 } 205 206 /** 207 * @return The actual content, base64 encoded. 208 */ 209 public byte[] getContent() { 210 return this.content == null ? null : this.content.getValue(); 211 } 212 213 /** 214 * @param value The actual content, base64 encoded. 215 */ 216 public Binary setContent(byte[] value) { 217 if (this.content == null) 218 this.content = new Base64BinaryType(); 219 this.content.setValue(value); 220 return this; 221 } 222 223 protected void listChildren(List<Property> children) { 224 super.listChildren(children); 225 children.add(new Property("contentType", "code", "MimeType of the binary content represented as a standard MimeType (BCP 13).", 0, 1, contentType)); 226 children.add(new Property("securityContext", "Reference(Any)", "Treat this binary as if it was this other resource for access control purposes.", 0, 1, securityContext)); 227 children.add(new Property("content", "base64Binary", "The actual content, base64 encoded.", 0, 1, content)); 228 } 229 230 @Override 231 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 232 switch (_hash) { 233 case -389131437: /*contentType*/ return new Property("contentType", "code", "MimeType of the binary content represented as a standard MimeType (BCP 13).", 0, 1, contentType); 234 case -1622888881: /*securityContext*/ return new Property("securityContext", "Reference(Any)", "Treat this binary as if it was this other resource for access control purposes.", 0, 1, securityContext); 235 case 951530617: /*content*/ return new Property("content", "base64Binary", "The actual content, base64 encoded.", 0, 1, content); 236 default: return super.getNamedProperty(_hash, _name, _checkValid); 237 } 238 239 } 240 241 @Override 242 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 243 switch (hash) { 244 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 245 case -1622888881: /*securityContext*/ return this.securityContext == null ? new Base[0] : new Base[] {this.securityContext}; // Reference 246 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Base64BinaryType 247 default: return super.getProperty(hash, name, checkValid); 248 } 249 250 } 251 252 @Override 253 public Base setProperty(int hash, String name, Base value) throws FHIRException { 254 switch (hash) { 255 case -389131437: // contentType 256 this.contentType = castToCode(value); // CodeType 257 return value; 258 case -1622888881: // securityContext 259 this.securityContext = castToReference(value); // Reference 260 return value; 261 case 951530617: // content 262 this.content = castToBase64Binary(value); // Base64BinaryType 263 return value; 264 default: return super.setProperty(hash, name, value); 265 } 266 267 } 268 269 @Override 270 public Base setProperty(String name, Base value) throws FHIRException { 271 if (name.equals("contentType")) { 272 this.contentType = castToCode(value); // CodeType 273 } else if (name.equals("securityContext")) { 274 this.securityContext = castToReference(value); // Reference 275 } else if (name.equals("content")) { 276 this.content = castToBase64Binary(value); // Base64BinaryType 277 } else 278 return super.setProperty(name, value); 279 return value; 280 } 281 282 @Override 283 public Base makeProperty(int hash, String name) throws FHIRException { 284 switch (hash) { 285 case -389131437: return getContentTypeElement(); 286 case -1622888881: return getSecurityContext(); 287 case 951530617: return getContentElement(); 288 default: return super.makeProperty(hash, name); 289 } 290 291 } 292 293 @Override 294 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 295 switch (hash) { 296 case -389131437: /*contentType*/ return new String[] {"code"}; 297 case -1622888881: /*securityContext*/ return new String[] {"Reference"}; 298 case 951530617: /*content*/ return new String[] {"base64Binary"}; 299 default: return super.getTypesForProperty(hash, name); 300 } 301 302 } 303 304 @Override 305 public Base addChild(String name) throws FHIRException { 306 if (name.equals("contentType")) { 307 throw new FHIRException("Cannot call addChild on a singleton property Binary.contentType"); 308 } 309 else if (name.equals("securityContext")) { 310 this.securityContext = new Reference(); 311 return this.securityContext; 312 } 313 else if (name.equals("content")) { 314 throw new FHIRException("Cannot call addChild on a singleton property Binary.content"); 315 } 316 else 317 return super.addChild(name); 318 } 319 320 public String fhirType() { 321 return "Binary"; 322 323 } 324 325 public Binary copy() { 326 Binary dst = new Binary(); 327 copyValues(dst); 328 dst.contentType = contentType == null ? null : contentType.copy(); 329 dst.securityContext = securityContext == null ? null : securityContext.copy(); 330 dst.content = content == null ? null : content.copy(); 331 return dst; 332 } 333 334 protected Binary typedCopy() { 335 return copy(); 336 } 337 338 @Override 339 public boolean equalsDeep(Base other_) { 340 if (!super.equalsDeep(other_)) 341 return false; 342 if (!(other_ instanceof Binary)) 343 return false; 344 Binary o = (Binary) other_; 345 return compareDeep(contentType, o.contentType, true) && compareDeep(securityContext, o.securityContext, true) 346 && compareDeep(content, o.content, true); 347 } 348 349 @Override 350 public boolean equalsShallow(Base other_) { 351 if (!super.equalsShallow(other_)) 352 return false; 353 if (!(other_ instanceof Binary)) 354 return false; 355 Binary o = (Binary) other_; 356 return compareValues(contentType, o.contentType, true) && compareValues(content, o.content, true); 357 } 358 359 public boolean isEmpty() { 360 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(contentType, securityContext 361 , content); 362 } 363 364 @Override 365 public ResourceType getResourceType() { 366 return ResourceType.Binary; 367 } 368 369 /** 370 * Search parameter: <b>contenttype</b> 371 * <p> 372 * Description: <b>MimeType of the binary content</b><br> 373 * Type: <b>token</b><br> 374 * Path: <b>Binary.contentType</b><br> 375 * </p> 376 */ 377 @SearchParamDefinition(name="contenttype", path="Binary.contentType", description="MimeType of the binary content", type="token" ) 378 public static final String SP_CONTENTTYPE = "contenttype"; 379 /** 380 * <b>Fluent Client</b> search parameter constant for <b>contenttype</b> 381 * <p> 382 * Description: <b>MimeType of the binary content</b><br> 383 * Type: <b>token</b><br> 384 * Path: <b>Binary.contentType</b><br> 385 * </p> 386 */ 387 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENTTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTENTTYPE); 388 389 390}