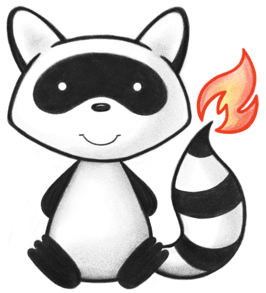
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045/** 046 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 047 */ 048@ResourceDef(name="BodySite", profile="http://hl7.org/fhir/Profile/BodySite") 049public class BodySite extends DomainResource { 050 051 /** 052 * Identifier for this instance of the anatomical location. 053 */ 054 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 055 @Description(shortDefinition="Bodysite identifier", formalDefinition="Identifier for this instance of the anatomical location." ) 056 protected List<Identifier> identifier; 057 058 /** 059 * Whether this body site is in active use. 060 */ 061 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 062 @Description(shortDefinition="Whether this body site record is in active use", formalDefinition="Whether this body site is in active use." ) 063 protected BooleanType active; 064 065 /** 066 * Named anatomical location - ideally coded where possible. 067 */ 068 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Named anatomical location", formalDefinition="Named anatomical location - ideally coded where possible." ) 070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 071 protected CodeableConcept code; 072 073 /** 074 * Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane. 075 */ 076 @Child(name = "qualifier", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 077 @Description(shortDefinition="Modification to location code", formalDefinition="Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane." ) 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bodysite-relative-location") 079 protected List<CodeableConcept> qualifier; 080 081 /** 082 * A summary, charactarization or explanation of the anatomic location. 083 */ 084 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 085 @Description(shortDefinition="Anatomical location description", formalDefinition="A summary, charactarization or explanation of the anatomic location." ) 086 protected StringType description; 087 088 /** 089 * Image or images used to identify a location. 090 */ 091 @Child(name = "image", type = {Attachment.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 092 @Description(shortDefinition="Attached images", formalDefinition="Image or images used to identify a location." ) 093 protected List<Attachment> image; 094 095 /** 096 * The person to which the body site belongs. 097 */ 098 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 099 @Description(shortDefinition="Who this is about", formalDefinition="The person to which the body site belongs." ) 100 protected Reference patient; 101 102 /** 103 * The actual object that is the target of the reference (The person to which the body site belongs.) 104 */ 105 protected Patient patientTarget; 106 107 private static final long serialVersionUID = -871837171L; 108 109 /** 110 * Constructor 111 */ 112 public BodySite() { 113 super(); 114 } 115 116 /** 117 * Constructor 118 */ 119 public BodySite(Reference patient) { 120 super(); 121 this.patient = patient; 122 } 123 124 /** 125 * @return {@link #identifier} (Identifier for this instance of the anatomical location.) 126 */ 127 public List<Identifier> getIdentifier() { 128 if (this.identifier == null) 129 this.identifier = new ArrayList<Identifier>(); 130 return this.identifier; 131 } 132 133 /** 134 * @return Returns a reference to <code>this</code> for easy method chaining 135 */ 136 public BodySite setIdentifier(List<Identifier> theIdentifier) { 137 this.identifier = theIdentifier; 138 return this; 139 } 140 141 public boolean hasIdentifier() { 142 if (this.identifier == null) 143 return false; 144 for (Identifier item : this.identifier) 145 if (!item.isEmpty()) 146 return true; 147 return false; 148 } 149 150 public Identifier addIdentifier() { //3 151 Identifier t = new Identifier(); 152 if (this.identifier == null) 153 this.identifier = new ArrayList<Identifier>(); 154 this.identifier.add(t); 155 return t; 156 } 157 158 public BodySite addIdentifier(Identifier t) { //3 159 if (t == null) 160 return this; 161 if (this.identifier == null) 162 this.identifier = new ArrayList<Identifier>(); 163 this.identifier.add(t); 164 return this; 165 } 166 167 /** 168 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 169 */ 170 public Identifier getIdentifierFirstRep() { 171 if (getIdentifier().isEmpty()) { 172 addIdentifier(); 173 } 174 return getIdentifier().get(0); 175 } 176 177 /** 178 * @return {@link #active} (Whether this body site is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 179 */ 180 public BooleanType getActiveElement() { 181 if (this.active == null) 182 if (Configuration.errorOnAutoCreate()) 183 throw new Error("Attempt to auto-create BodySite.active"); 184 else if (Configuration.doAutoCreate()) 185 this.active = new BooleanType(); // bb 186 return this.active; 187 } 188 189 public boolean hasActiveElement() { 190 return this.active != null && !this.active.isEmpty(); 191 } 192 193 public boolean hasActive() { 194 return this.active != null && !this.active.isEmpty(); 195 } 196 197 /** 198 * @param value {@link #active} (Whether this body site is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 199 */ 200 public BodySite setActiveElement(BooleanType value) { 201 this.active = value; 202 return this; 203 } 204 205 /** 206 * @return Whether this body site is in active use. 207 */ 208 public boolean getActive() { 209 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 210 } 211 212 /** 213 * @param value Whether this body site is in active use. 214 */ 215 public BodySite setActive(boolean value) { 216 if (this.active == null) 217 this.active = new BooleanType(); 218 this.active.setValue(value); 219 return this; 220 } 221 222 /** 223 * @return {@link #code} (Named anatomical location - ideally coded where possible.) 224 */ 225 public CodeableConcept getCode() { 226 if (this.code == null) 227 if (Configuration.errorOnAutoCreate()) 228 throw new Error("Attempt to auto-create BodySite.code"); 229 else if (Configuration.doAutoCreate()) 230 this.code = new CodeableConcept(); // cc 231 return this.code; 232 } 233 234 public boolean hasCode() { 235 return this.code != null && !this.code.isEmpty(); 236 } 237 238 /** 239 * @param value {@link #code} (Named anatomical location - ideally coded where possible.) 240 */ 241 public BodySite setCode(CodeableConcept value) { 242 this.code = value; 243 return this; 244 } 245 246 /** 247 * @return {@link #qualifier} (Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane.) 248 */ 249 public List<CodeableConcept> getQualifier() { 250 if (this.qualifier == null) 251 this.qualifier = new ArrayList<CodeableConcept>(); 252 return this.qualifier; 253 } 254 255 /** 256 * @return Returns a reference to <code>this</code> for easy method chaining 257 */ 258 public BodySite setQualifier(List<CodeableConcept> theQualifier) { 259 this.qualifier = theQualifier; 260 return this; 261 } 262 263 public boolean hasQualifier() { 264 if (this.qualifier == null) 265 return false; 266 for (CodeableConcept item : this.qualifier) 267 if (!item.isEmpty()) 268 return true; 269 return false; 270 } 271 272 public CodeableConcept addQualifier() { //3 273 CodeableConcept t = new CodeableConcept(); 274 if (this.qualifier == null) 275 this.qualifier = new ArrayList<CodeableConcept>(); 276 this.qualifier.add(t); 277 return t; 278 } 279 280 public BodySite addQualifier(CodeableConcept t) { //3 281 if (t == null) 282 return this; 283 if (this.qualifier == null) 284 this.qualifier = new ArrayList<CodeableConcept>(); 285 this.qualifier.add(t); 286 return this; 287 } 288 289 /** 290 * @return The first repetition of repeating field {@link #qualifier}, creating it if it does not already exist 291 */ 292 public CodeableConcept getQualifierFirstRep() { 293 if (getQualifier().isEmpty()) { 294 addQualifier(); 295 } 296 return getQualifier().get(0); 297 } 298 299 /** 300 * @return {@link #description} (A summary, charactarization or explanation of the anatomic location.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 301 */ 302 public StringType getDescriptionElement() { 303 if (this.description == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create BodySite.description"); 306 else if (Configuration.doAutoCreate()) 307 this.description = new StringType(); // bb 308 return this.description; 309 } 310 311 public boolean hasDescriptionElement() { 312 return this.description != null && !this.description.isEmpty(); 313 } 314 315 public boolean hasDescription() { 316 return this.description != null && !this.description.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #description} (A summary, charactarization or explanation of the anatomic location.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 321 */ 322 public BodySite setDescriptionElement(StringType value) { 323 this.description = value; 324 return this; 325 } 326 327 /** 328 * @return A summary, charactarization or explanation of the anatomic location. 329 */ 330 public String getDescription() { 331 return this.description == null ? null : this.description.getValue(); 332 } 333 334 /** 335 * @param value A summary, charactarization or explanation of the anatomic location. 336 */ 337 public BodySite setDescription(String value) { 338 if (Utilities.noString(value)) 339 this.description = null; 340 else { 341 if (this.description == null) 342 this.description = new StringType(); 343 this.description.setValue(value); 344 } 345 return this; 346 } 347 348 /** 349 * @return {@link #image} (Image or images used to identify a location.) 350 */ 351 public List<Attachment> getImage() { 352 if (this.image == null) 353 this.image = new ArrayList<Attachment>(); 354 return this.image; 355 } 356 357 /** 358 * @return Returns a reference to <code>this</code> for easy method chaining 359 */ 360 public BodySite setImage(List<Attachment> theImage) { 361 this.image = theImage; 362 return this; 363 } 364 365 public boolean hasImage() { 366 if (this.image == null) 367 return false; 368 for (Attachment item : this.image) 369 if (!item.isEmpty()) 370 return true; 371 return false; 372 } 373 374 public Attachment addImage() { //3 375 Attachment t = new Attachment(); 376 if (this.image == null) 377 this.image = new ArrayList<Attachment>(); 378 this.image.add(t); 379 return t; 380 } 381 382 public BodySite addImage(Attachment t) { //3 383 if (t == null) 384 return this; 385 if (this.image == null) 386 this.image = new ArrayList<Attachment>(); 387 this.image.add(t); 388 return this; 389 } 390 391 /** 392 * @return The first repetition of repeating field {@link #image}, creating it if it does not already exist 393 */ 394 public Attachment getImageFirstRep() { 395 if (getImage().isEmpty()) { 396 addImage(); 397 } 398 return getImage().get(0); 399 } 400 401 /** 402 * @return {@link #patient} (The person to which the body site belongs.) 403 */ 404 public Reference getPatient() { 405 if (this.patient == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create BodySite.patient"); 408 else if (Configuration.doAutoCreate()) 409 this.patient = new Reference(); // cc 410 return this.patient; 411 } 412 413 public boolean hasPatient() { 414 return this.patient != null && !this.patient.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #patient} (The person to which the body site belongs.) 419 */ 420 public BodySite setPatient(Reference value) { 421 this.patient = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person to which the body site belongs.) 427 */ 428 public Patient getPatientTarget() { 429 if (this.patientTarget == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create BodySite.patient"); 432 else if (Configuration.doAutoCreate()) 433 this.patientTarget = new Patient(); // aa 434 return this.patientTarget; 435 } 436 437 /** 438 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person to which the body site belongs.) 439 */ 440 public BodySite setPatientTarget(Patient value) { 441 this.patientTarget = value; 442 return this; 443 } 444 445 protected void listChildren(List<Property> children) { 446 super.listChildren(children); 447 children.add(new Property("identifier", "Identifier", "Identifier for this instance of the anatomical location.", 0, java.lang.Integer.MAX_VALUE, identifier)); 448 children.add(new Property("active", "boolean", "Whether this body site is in active use.", 0, 1, active)); 449 children.add(new Property("code", "CodeableConcept", "Named anatomical location - ideally coded where possible.", 0, 1, code)); 450 children.add(new Property("qualifier", "CodeableConcept", "Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane.", 0, java.lang.Integer.MAX_VALUE, qualifier)); 451 children.add(new Property("description", "string", "A summary, charactarization or explanation of the anatomic location.", 0, 1, description)); 452 children.add(new Property("image", "Attachment", "Image or images used to identify a location.", 0, java.lang.Integer.MAX_VALUE, image)); 453 children.add(new Property("patient", "Reference(Patient)", "The person to which the body site belongs.", 0, 1, patient)); 454 } 455 456 @Override 457 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 458 switch (_hash) { 459 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for this instance of the anatomical location.", 0, java.lang.Integer.MAX_VALUE, identifier); 460 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this body site is in active use.", 0, 1, active); 461 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Named anatomical location - ideally coded where possible.", 0, 1, code); 462 case -1247940438: /*qualifier*/ return new Property("qualifier", "CodeableConcept", "Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane.", 0, java.lang.Integer.MAX_VALUE, qualifier); 463 case -1724546052: /*description*/ return new Property("description", "string", "A summary, charactarization or explanation of the anatomic location.", 0, 1, description); 464 case 100313435: /*image*/ return new Property("image", "Attachment", "Image or images used to identify a location.", 0, java.lang.Integer.MAX_VALUE, image); 465 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The person to which the body site belongs.", 0, 1, patient); 466 default: return super.getNamedProperty(_hash, _name, _checkValid); 467 } 468 469 } 470 471 @Override 472 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 473 switch (hash) { 474 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 475 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 476 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 477 case -1247940438: /*qualifier*/ return this.qualifier == null ? new Base[0] : this.qualifier.toArray(new Base[this.qualifier.size()]); // CodeableConcept 478 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 479 case 100313435: /*image*/ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // Attachment 480 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 481 default: return super.getProperty(hash, name, checkValid); 482 } 483 484 } 485 486 @Override 487 public Base setProperty(int hash, String name, Base value) throws FHIRException { 488 switch (hash) { 489 case -1618432855: // identifier 490 this.getIdentifier().add(castToIdentifier(value)); // Identifier 491 return value; 492 case -1422950650: // active 493 this.active = castToBoolean(value); // BooleanType 494 return value; 495 case 3059181: // code 496 this.code = castToCodeableConcept(value); // CodeableConcept 497 return value; 498 case -1247940438: // qualifier 499 this.getQualifier().add(castToCodeableConcept(value)); // CodeableConcept 500 return value; 501 case -1724546052: // description 502 this.description = castToString(value); // StringType 503 return value; 504 case 100313435: // image 505 this.getImage().add(castToAttachment(value)); // Attachment 506 return value; 507 case -791418107: // patient 508 this.patient = castToReference(value); // Reference 509 return value; 510 default: return super.setProperty(hash, name, value); 511 } 512 513 } 514 515 @Override 516 public Base setProperty(String name, Base value) throws FHIRException { 517 if (name.equals("identifier")) { 518 this.getIdentifier().add(castToIdentifier(value)); 519 } else if (name.equals("active")) { 520 this.active = castToBoolean(value); // BooleanType 521 } else if (name.equals("code")) { 522 this.code = castToCodeableConcept(value); // CodeableConcept 523 } else if (name.equals("qualifier")) { 524 this.getQualifier().add(castToCodeableConcept(value)); 525 } else if (name.equals("description")) { 526 this.description = castToString(value); // StringType 527 } else if (name.equals("image")) { 528 this.getImage().add(castToAttachment(value)); 529 } else if (name.equals("patient")) { 530 this.patient = castToReference(value); // Reference 531 } else 532 return super.setProperty(name, value); 533 return value; 534 } 535 536 @Override 537 public Base makeProperty(int hash, String name) throws FHIRException { 538 switch (hash) { 539 case -1618432855: return addIdentifier(); 540 case -1422950650: return getActiveElement(); 541 case 3059181: return getCode(); 542 case -1247940438: return addQualifier(); 543 case -1724546052: return getDescriptionElement(); 544 case 100313435: return addImage(); 545 case -791418107: return getPatient(); 546 default: return super.makeProperty(hash, name); 547 } 548 549 } 550 551 @Override 552 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 553 switch (hash) { 554 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 555 case -1422950650: /*active*/ return new String[] {"boolean"}; 556 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 557 case -1247940438: /*qualifier*/ return new String[] {"CodeableConcept"}; 558 case -1724546052: /*description*/ return new String[] {"string"}; 559 case 100313435: /*image*/ return new String[] {"Attachment"}; 560 case -791418107: /*patient*/ return new String[] {"Reference"}; 561 default: return super.getTypesForProperty(hash, name); 562 } 563 564 } 565 566 @Override 567 public Base addChild(String name) throws FHIRException { 568 if (name.equals("identifier")) { 569 return addIdentifier(); 570 } 571 else if (name.equals("active")) { 572 throw new FHIRException("Cannot call addChild on a singleton property BodySite.active"); 573 } 574 else if (name.equals("code")) { 575 this.code = new CodeableConcept(); 576 return this.code; 577 } 578 else if (name.equals("qualifier")) { 579 return addQualifier(); 580 } 581 else if (name.equals("description")) { 582 throw new FHIRException("Cannot call addChild on a singleton property BodySite.description"); 583 } 584 else if (name.equals("image")) { 585 return addImage(); 586 } 587 else if (name.equals("patient")) { 588 this.patient = new Reference(); 589 return this.patient; 590 } 591 else 592 return super.addChild(name); 593 } 594 595 public String fhirType() { 596 return "BodySite"; 597 598 } 599 600 public BodySite copy() { 601 BodySite dst = new BodySite(); 602 copyValues(dst); 603 if (identifier != null) { 604 dst.identifier = new ArrayList<Identifier>(); 605 for (Identifier i : identifier) 606 dst.identifier.add(i.copy()); 607 }; 608 dst.active = active == null ? null : active.copy(); 609 dst.code = code == null ? null : code.copy(); 610 if (qualifier != null) { 611 dst.qualifier = new ArrayList<CodeableConcept>(); 612 for (CodeableConcept i : qualifier) 613 dst.qualifier.add(i.copy()); 614 }; 615 dst.description = description == null ? null : description.copy(); 616 if (image != null) { 617 dst.image = new ArrayList<Attachment>(); 618 for (Attachment i : image) 619 dst.image.add(i.copy()); 620 }; 621 dst.patient = patient == null ? null : patient.copy(); 622 return dst; 623 } 624 625 protected BodySite typedCopy() { 626 return copy(); 627 } 628 629 @Override 630 public boolean equalsDeep(Base other_) { 631 if (!super.equalsDeep(other_)) 632 return false; 633 if (!(other_ instanceof BodySite)) 634 return false; 635 BodySite o = (BodySite) other_; 636 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(code, o.code, true) 637 && compareDeep(qualifier, o.qualifier, true) && compareDeep(description, o.description, true) && compareDeep(image, o.image, true) 638 && compareDeep(patient, o.patient, true); 639 } 640 641 @Override 642 public boolean equalsShallow(Base other_) { 643 if (!super.equalsShallow(other_)) 644 return false; 645 if (!(other_ instanceof BodySite)) 646 return false; 647 BodySite o = (BodySite) other_; 648 return compareValues(active, o.active, true) && compareValues(description, o.description, true); 649 } 650 651 public boolean isEmpty() { 652 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, code 653 , qualifier, description, image, patient); 654 } 655 656 @Override 657 public ResourceType getResourceType() { 658 return ResourceType.BodySite; 659 } 660 661 /** 662 * Search parameter: <b>identifier</b> 663 * <p> 664 * Description: <b>Identifier for this instance of the anatomical location</b><br> 665 * Type: <b>token</b><br> 666 * Path: <b>BodySite.identifier</b><br> 667 * </p> 668 */ 669 @SearchParamDefinition(name="identifier", path="BodySite.identifier", description="Identifier for this instance of the anatomical location", type="token" ) 670 public static final String SP_IDENTIFIER = "identifier"; 671 /** 672 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 673 * <p> 674 * Description: <b>Identifier for this instance of the anatomical location</b><br> 675 * Type: <b>token</b><br> 676 * Path: <b>BodySite.identifier</b><br> 677 * </p> 678 */ 679 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 680 681 /** 682 * Search parameter: <b>code</b> 683 * <p> 684 * Description: <b>Named anatomical location</b><br> 685 * Type: <b>token</b><br> 686 * Path: <b>BodySite.code</b><br> 687 * </p> 688 */ 689 @SearchParamDefinition(name="code", path="BodySite.code", description="Named anatomical location", type="token" ) 690 public static final String SP_CODE = "code"; 691 /** 692 * <b>Fluent Client</b> search parameter constant for <b>code</b> 693 * <p> 694 * Description: <b>Named anatomical location</b><br> 695 * Type: <b>token</b><br> 696 * Path: <b>BodySite.code</b><br> 697 * </p> 698 */ 699 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 700 701 /** 702 * Search parameter: <b>patient</b> 703 * <p> 704 * Description: <b>Patient to whom bodysite belongs</b><br> 705 * Type: <b>reference</b><br> 706 * Path: <b>BodySite.patient</b><br> 707 * </p> 708 */ 709 @SearchParamDefinition(name="patient", path="BodySite.patient", description="Patient to whom bodysite belongs", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 710 public static final String SP_PATIENT = "patient"; 711 /** 712 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 713 * <p> 714 * Description: <b>Patient to whom bodysite belongs</b><br> 715 * Type: <b>reference</b><br> 716 * Path: <b>BodySite.patient</b><br> 717 * </p> 718 */ 719 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 720 721/** 722 * Constant for fluent queries to be used to add include statements. Specifies 723 * the path value of "<b>BodySite:patient</b>". 724 */ 725 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("BodySite:patient").toLocked(); 726 727 728}