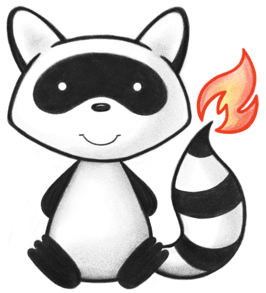
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.instance.model.api.IBaseBundle; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A container for a collection of resources. 051 */ 052@ResourceDef(name="Bundle", profile="http://hl7.org/fhir/Profile/Bundle") 053public class Bundle extends Resource implements IBaseBundle { 054 055 public enum BundleType { 056 /** 057 * The bundle is a document. The first resource is a Composition. 058 */ 059 DOCUMENT, 060 /** 061 * The bundle is a message. The first resource is a MessageHeader. 062 */ 063 MESSAGE, 064 /** 065 * The bundle is a transaction - intended to be processed by a server as an atomic commit. 066 */ 067 TRANSACTION, 068 /** 069 * The bundle is a transaction response. Because the response is a transaction response, the transaction has succeeded, and all responses are error free. 070 */ 071 TRANSACTIONRESPONSE, 072 /** 073 * The bundle is a transaction - intended to be processed by a server as a group of actions. 074 */ 075 BATCH, 076 /** 077 * The bundle is a batch response. Note that as a batch, some responses may indicate failure and others success. 078 */ 079 BATCHRESPONSE, 080 /** 081 * The bundle is a list of resources from a history interaction on a server. 082 */ 083 HISTORY, 084 /** 085 * The bundle is a list of resources returned as a result of a search/query interaction, operation, or message. 086 */ 087 SEARCHSET, 088 /** 089 * The bundle is a set of resources collected into a single package for ease of distribution. 090 */ 091 COLLECTION, 092 /** 093 * added to help the parsers with the generic types 094 */ 095 NULL; 096 public static BundleType fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("document".equals(codeString)) 100 return DOCUMENT; 101 if ("message".equals(codeString)) 102 return MESSAGE; 103 if ("transaction".equals(codeString)) 104 return TRANSACTION; 105 if ("transaction-response".equals(codeString)) 106 return TRANSACTIONRESPONSE; 107 if ("batch".equals(codeString)) 108 return BATCH; 109 if ("batch-response".equals(codeString)) 110 return BATCHRESPONSE; 111 if ("history".equals(codeString)) 112 return HISTORY; 113 if ("searchset".equals(codeString)) 114 return SEARCHSET; 115 if ("collection".equals(codeString)) 116 return COLLECTION; 117 if (Configuration.isAcceptInvalidEnums()) 118 return null; 119 else 120 throw new FHIRException("Unknown BundleType code '"+codeString+"'"); 121 } 122 public String toCode() { 123 switch (this) { 124 case DOCUMENT: return "document"; 125 case MESSAGE: return "message"; 126 case TRANSACTION: return "transaction"; 127 case TRANSACTIONRESPONSE: return "transaction-response"; 128 case BATCH: return "batch"; 129 case BATCHRESPONSE: return "batch-response"; 130 case HISTORY: return "history"; 131 case SEARCHSET: return "searchset"; 132 case COLLECTION: return "collection"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getSystem() { 138 switch (this) { 139 case DOCUMENT: return "http://hl7.org/fhir/bundle-type"; 140 case MESSAGE: return "http://hl7.org/fhir/bundle-type"; 141 case TRANSACTION: return "http://hl7.org/fhir/bundle-type"; 142 case TRANSACTIONRESPONSE: return "http://hl7.org/fhir/bundle-type"; 143 case BATCH: return "http://hl7.org/fhir/bundle-type"; 144 case BATCHRESPONSE: return "http://hl7.org/fhir/bundle-type"; 145 case HISTORY: return "http://hl7.org/fhir/bundle-type"; 146 case SEARCHSET: return "http://hl7.org/fhir/bundle-type"; 147 case COLLECTION: return "http://hl7.org/fhir/bundle-type"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 public String getDefinition() { 153 switch (this) { 154 case DOCUMENT: return "The bundle is a document. The first resource is a Composition."; 155 case MESSAGE: return "The bundle is a message. The first resource is a MessageHeader."; 156 case TRANSACTION: return "The bundle is a transaction - intended to be processed by a server as an atomic commit."; 157 case TRANSACTIONRESPONSE: return "The bundle is a transaction response. Because the response is a transaction response, the transaction has succeeded, and all responses are error free."; 158 case BATCH: return "The bundle is a transaction - intended to be processed by a server as a group of actions."; 159 case BATCHRESPONSE: return "The bundle is a batch response. Note that as a batch, some responses may indicate failure and others success."; 160 case HISTORY: return "The bundle is a list of resources from a history interaction on a server."; 161 case SEARCHSET: return "The bundle is a list of resources returned as a result of a search/query interaction, operation, or message."; 162 case COLLECTION: return "The bundle is a set of resources collected into a single package for ease of distribution."; 163 case NULL: return null; 164 default: return "?"; 165 } 166 } 167 public String getDisplay() { 168 switch (this) { 169 case DOCUMENT: return "Document"; 170 case MESSAGE: return "Message"; 171 case TRANSACTION: return "Transaction"; 172 case TRANSACTIONRESPONSE: return "Transaction Response"; 173 case BATCH: return "Batch"; 174 case BATCHRESPONSE: return "Batch Response"; 175 case HISTORY: return "History List"; 176 case SEARCHSET: return "Search Results"; 177 case COLLECTION: return "Collection"; 178 case NULL: return null; 179 default: return "?"; 180 } 181 } 182 } 183 184 public static class BundleTypeEnumFactory implements EnumFactory<BundleType> { 185 public BundleType fromCode(String codeString) throws IllegalArgumentException { 186 if (codeString == null || "".equals(codeString)) 187 if (codeString == null || "".equals(codeString)) 188 return null; 189 if ("document".equals(codeString)) 190 return BundleType.DOCUMENT; 191 if ("message".equals(codeString)) 192 return BundleType.MESSAGE; 193 if ("transaction".equals(codeString)) 194 return BundleType.TRANSACTION; 195 if ("transaction-response".equals(codeString)) 196 return BundleType.TRANSACTIONRESPONSE; 197 if ("batch".equals(codeString)) 198 return BundleType.BATCH; 199 if ("batch-response".equals(codeString)) 200 return BundleType.BATCHRESPONSE; 201 if ("history".equals(codeString)) 202 return BundleType.HISTORY; 203 if ("searchset".equals(codeString)) 204 return BundleType.SEARCHSET; 205 if ("collection".equals(codeString)) 206 return BundleType.COLLECTION; 207 throw new IllegalArgumentException("Unknown BundleType code '"+codeString+"'"); 208 } 209 public Enumeration<BundleType> fromType(PrimitiveType<?> code) throws FHIRException { 210 if (code == null) 211 return null; 212 if (code.isEmpty()) 213 return new Enumeration<BundleType>(this); 214 String codeString = code.asStringValue(); 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("document".equals(codeString)) 218 return new Enumeration<BundleType>(this, BundleType.DOCUMENT); 219 if ("message".equals(codeString)) 220 return new Enumeration<BundleType>(this, BundleType.MESSAGE); 221 if ("transaction".equals(codeString)) 222 return new Enumeration<BundleType>(this, BundleType.TRANSACTION); 223 if ("transaction-response".equals(codeString)) 224 return new Enumeration<BundleType>(this, BundleType.TRANSACTIONRESPONSE); 225 if ("batch".equals(codeString)) 226 return new Enumeration<BundleType>(this, BundleType.BATCH); 227 if ("batch-response".equals(codeString)) 228 return new Enumeration<BundleType>(this, BundleType.BATCHRESPONSE); 229 if ("history".equals(codeString)) 230 return new Enumeration<BundleType>(this, BundleType.HISTORY); 231 if ("searchset".equals(codeString)) 232 return new Enumeration<BundleType>(this, BundleType.SEARCHSET); 233 if ("collection".equals(codeString)) 234 return new Enumeration<BundleType>(this, BundleType.COLLECTION); 235 throw new FHIRException("Unknown BundleType code '"+codeString+"'"); 236 } 237 public String toCode(BundleType code) { 238 if (code == BundleType.NULL) 239 return null; 240 if (code == BundleType.DOCUMENT) 241 return "document"; 242 if (code == BundleType.MESSAGE) 243 return "message"; 244 if (code == BundleType.TRANSACTION) 245 return "transaction"; 246 if (code == BundleType.TRANSACTIONRESPONSE) 247 return "transaction-response"; 248 if (code == BundleType.BATCH) 249 return "batch"; 250 if (code == BundleType.BATCHRESPONSE) 251 return "batch-response"; 252 if (code == BundleType.HISTORY) 253 return "history"; 254 if (code == BundleType.SEARCHSET) 255 return "searchset"; 256 if (code == BundleType.COLLECTION) 257 return "collection"; 258 return "?"; 259 } 260 public String toSystem(BundleType code) { 261 return code.getSystem(); 262 } 263 } 264 265 public enum SearchEntryMode { 266 /** 267 * This resource matched the search specification. 268 */ 269 MATCH, 270 /** 271 * This resource is returned because it is referred to from another resource in the search set. 272 */ 273 INCLUDE, 274 /** 275 * An OperationOutcome that provides additional information about the processing of a search. 276 */ 277 OUTCOME, 278 /** 279 * added to help the parsers with the generic types 280 */ 281 NULL; 282 public static SearchEntryMode fromCode(String codeString) throws FHIRException { 283 if (codeString == null || "".equals(codeString)) 284 return null; 285 if ("match".equals(codeString)) 286 return MATCH; 287 if ("include".equals(codeString)) 288 return INCLUDE; 289 if ("outcome".equals(codeString)) 290 return OUTCOME; 291 if (Configuration.isAcceptInvalidEnums()) 292 return null; 293 else 294 throw new FHIRException("Unknown SearchEntryMode code '"+codeString+"'"); 295 } 296 public String toCode() { 297 switch (this) { 298 case MATCH: return "match"; 299 case INCLUDE: return "include"; 300 case OUTCOME: return "outcome"; 301 case NULL: return null; 302 default: return "?"; 303 } 304 } 305 public String getSystem() { 306 switch (this) { 307 case MATCH: return "http://hl7.org/fhir/search-entry-mode"; 308 case INCLUDE: return "http://hl7.org/fhir/search-entry-mode"; 309 case OUTCOME: return "http://hl7.org/fhir/search-entry-mode"; 310 case NULL: return null; 311 default: return "?"; 312 } 313 } 314 public String getDefinition() { 315 switch (this) { 316 case MATCH: return "This resource matched the search specification."; 317 case INCLUDE: return "This resource is returned because it is referred to from another resource in the search set."; 318 case OUTCOME: return "An OperationOutcome that provides additional information about the processing of a search."; 319 case NULL: return null; 320 default: return "?"; 321 } 322 } 323 public String getDisplay() { 324 switch (this) { 325 case MATCH: return "Match"; 326 case INCLUDE: return "Include"; 327 case OUTCOME: return "Outcome"; 328 case NULL: return null; 329 default: return "?"; 330 } 331 } 332 } 333 334 public static class SearchEntryModeEnumFactory implements EnumFactory<SearchEntryMode> { 335 public SearchEntryMode fromCode(String codeString) throws IllegalArgumentException { 336 if (codeString == null || "".equals(codeString)) 337 if (codeString == null || "".equals(codeString)) 338 return null; 339 if ("match".equals(codeString)) 340 return SearchEntryMode.MATCH; 341 if ("include".equals(codeString)) 342 return SearchEntryMode.INCLUDE; 343 if ("outcome".equals(codeString)) 344 return SearchEntryMode.OUTCOME; 345 throw new IllegalArgumentException("Unknown SearchEntryMode code '"+codeString+"'"); 346 } 347 public Enumeration<SearchEntryMode> fromType(PrimitiveType<?> code) throws FHIRException { 348 if (code == null) 349 return null; 350 if (code.isEmpty()) 351 return new Enumeration<SearchEntryMode>(this); 352 String codeString = code.asStringValue(); 353 if (codeString == null || "".equals(codeString)) 354 return null; 355 if ("match".equals(codeString)) 356 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.MATCH); 357 if ("include".equals(codeString)) 358 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.INCLUDE); 359 if ("outcome".equals(codeString)) 360 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.OUTCOME); 361 throw new FHIRException("Unknown SearchEntryMode code '"+codeString+"'"); 362 } 363 public String toCode(SearchEntryMode code) { 364 if (code == SearchEntryMode.NULL) 365 return null; 366 if (code == SearchEntryMode.MATCH) 367 return "match"; 368 if (code == SearchEntryMode.INCLUDE) 369 return "include"; 370 if (code == SearchEntryMode.OUTCOME) 371 return "outcome"; 372 return "?"; 373 } 374 public String toSystem(SearchEntryMode code) { 375 return code.getSystem(); 376 } 377 } 378 379 public enum HTTPVerb { 380 /** 381 * HTTP GET 382 */ 383 GET, 384 /** 385 * HTTP POST 386 */ 387 POST, 388 /** 389 * HTTP PUT 390 */ 391 PUT, 392 /** 393 * HTTP DELETE 394 */ 395 DELETE, 396 /** 397 * added to help the parsers with the generic types 398 */ 399 NULL; 400 public static HTTPVerb fromCode(String codeString) throws FHIRException { 401 if (codeString == null || "".equals(codeString)) 402 return null; 403 if ("GET".equals(codeString)) 404 return GET; 405 if ("POST".equals(codeString)) 406 return POST; 407 if ("PUT".equals(codeString)) 408 return PUT; 409 if ("DELETE".equals(codeString)) 410 return DELETE; 411 if (Configuration.isAcceptInvalidEnums()) 412 return null; 413 else 414 throw new FHIRException("Unknown HTTPVerb code '"+codeString+"'"); 415 } 416 public String toCode() { 417 switch (this) { 418 case GET: return "GET"; 419 case POST: return "POST"; 420 case PUT: return "PUT"; 421 case DELETE: return "DELETE"; 422 case NULL: return null; 423 default: return "?"; 424 } 425 } 426 public String getSystem() { 427 switch (this) { 428 case GET: return "http://hl7.org/fhir/http-verb"; 429 case POST: return "http://hl7.org/fhir/http-verb"; 430 case PUT: return "http://hl7.org/fhir/http-verb"; 431 case DELETE: return "http://hl7.org/fhir/http-verb"; 432 case NULL: return null; 433 default: return "?"; 434 } 435 } 436 public String getDefinition() { 437 switch (this) { 438 case GET: return "HTTP GET"; 439 case POST: return "HTTP POST"; 440 case PUT: return "HTTP PUT"; 441 case DELETE: return "HTTP DELETE"; 442 case NULL: return null; 443 default: return "?"; 444 } 445 } 446 public String getDisplay() { 447 switch (this) { 448 case GET: return "GET"; 449 case POST: return "POST"; 450 case PUT: return "PUT"; 451 case DELETE: return "DELETE"; 452 case NULL: return null; 453 default: return "?"; 454 } 455 } 456 } 457 458 public static class HTTPVerbEnumFactory implements EnumFactory<HTTPVerb> { 459 public HTTPVerb fromCode(String codeString) throws IllegalArgumentException { 460 if (codeString == null || "".equals(codeString)) 461 if (codeString == null || "".equals(codeString)) 462 return null; 463 if ("GET".equals(codeString)) 464 return HTTPVerb.GET; 465 if ("POST".equals(codeString)) 466 return HTTPVerb.POST; 467 if ("PUT".equals(codeString)) 468 return HTTPVerb.PUT; 469 if ("DELETE".equals(codeString)) 470 return HTTPVerb.DELETE; 471 throw new IllegalArgumentException("Unknown HTTPVerb code '"+codeString+"'"); 472 } 473 public Enumeration<HTTPVerb> fromType(PrimitiveType<?> code) throws FHIRException { 474 if (code == null) 475 return null; 476 if (code.isEmpty()) 477 return new Enumeration<HTTPVerb>(this); 478 String codeString = code.asStringValue(); 479 if (codeString == null || "".equals(codeString)) 480 return null; 481 if ("GET".equals(codeString)) 482 return new Enumeration<HTTPVerb>(this, HTTPVerb.GET); 483 if ("POST".equals(codeString)) 484 return new Enumeration<HTTPVerb>(this, HTTPVerb.POST); 485 if ("PUT".equals(codeString)) 486 return new Enumeration<HTTPVerb>(this, HTTPVerb.PUT); 487 if ("DELETE".equals(codeString)) 488 return new Enumeration<HTTPVerb>(this, HTTPVerb.DELETE); 489 throw new FHIRException("Unknown HTTPVerb code '"+codeString+"'"); 490 } 491 public String toCode(HTTPVerb code) { 492 if (code == HTTPVerb.NULL) 493 return null; 494 if (code == HTTPVerb.GET) 495 return "GET"; 496 if (code == HTTPVerb.POST) 497 return "POST"; 498 if (code == HTTPVerb.PUT) 499 return "PUT"; 500 if (code == HTTPVerb.DELETE) 501 return "DELETE"; 502 return "?"; 503 } 504 public String toSystem(HTTPVerb code) { 505 return code.getSystem(); 506 } 507 } 508 509 @Block() 510 public static class BundleLinkComponent extends BackboneElement implements IBaseBackboneElement { 511 /** 512 * A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 513 */ 514 @Child(name = "relation", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 515 @Description(shortDefinition="See http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1", formalDefinition="A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1)." ) 516 protected StringType relation; 517 518 /** 519 * The reference details for the link. 520 */ 521 @Child(name = "url", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 522 @Description(shortDefinition="Reference details for the link", formalDefinition="The reference details for the link." ) 523 protected UriType url; 524 525 private static final long serialVersionUID = -1010386066L; 526 527 /** 528 * Constructor 529 */ 530 public BundleLinkComponent() { 531 super(); 532 } 533 534 /** 535 * Constructor 536 */ 537 public BundleLinkComponent(StringType relation, UriType url) { 538 super(); 539 this.relation = relation; 540 this.url = url; 541 } 542 543 /** 544 * @return {@link #relation} (A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).). This is the underlying object with id, value and extensions. The accessor "getRelation" gives direct access to the value 545 */ 546 public StringType getRelationElement() { 547 if (this.relation == null) 548 if (Configuration.errorOnAutoCreate()) 549 throw new Error("Attempt to auto-create BundleLinkComponent.relation"); 550 else if (Configuration.doAutoCreate()) 551 this.relation = new StringType(); // bb 552 return this.relation; 553 } 554 555 public boolean hasRelationElement() { 556 return this.relation != null && !this.relation.isEmpty(); 557 } 558 559 public boolean hasRelation() { 560 return this.relation != null && !this.relation.isEmpty(); 561 } 562 563 /** 564 * @param value {@link #relation} (A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).). This is the underlying object with id, value and extensions. The accessor "getRelation" gives direct access to the value 565 */ 566 public BundleLinkComponent setRelationElement(StringType value) { 567 this.relation = value; 568 return this; 569 } 570 571 /** 572 * @return A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 573 */ 574 public String getRelation() { 575 return this.relation == null ? null : this.relation.getValue(); 576 } 577 578 /** 579 * @param value A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 580 */ 581 public BundleLinkComponent setRelation(String value) { 582 if (this.relation == null) 583 this.relation = new StringType(); 584 this.relation.setValue(value); 585 return this; 586 } 587 588 /** 589 * @return {@link #url} (The reference details for the link.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 590 */ 591 public UriType getUrlElement() { 592 if (this.url == null) 593 if (Configuration.errorOnAutoCreate()) 594 throw new Error("Attempt to auto-create BundleLinkComponent.url"); 595 else if (Configuration.doAutoCreate()) 596 this.url = new UriType(); // bb 597 return this.url; 598 } 599 600 public boolean hasUrlElement() { 601 return this.url != null && !this.url.isEmpty(); 602 } 603 604 public boolean hasUrl() { 605 return this.url != null && !this.url.isEmpty(); 606 } 607 608 /** 609 * @param value {@link #url} (The reference details for the link.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 610 */ 611 public BundleLinkComponent setUrlElement(UriType value) { 612 this.url = value; 613 return this; 614 } 615 616 /** 617 * @return The reference details for the link. 618 */ 619 public String getUrl() { 620 return this.url == null ? null : this.url.getValue(); 621 } 622 623 /** 624 * @param value The reference details for the link. 625 */ 626 public BundleLinkComponent setUrl(String value) { 627 if (this.url == null) 628 this.url = new UriType(); 629 this.url.setValue(value); 630 return this; 631 } 632 633 protected void listChildren(List<Property> children) { 634 super.listChildren(children); 635 children.add(new Property("relation", "string", "A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).", 0, 1, relation)); 636 children.add(new Property("url", "uri", "The reference details for the link.", 0, 1, url)); 637 } 638 639 @Override 640 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 641 switch (_hash) { 642 case -554436100: /*relation*/ return new Property("relation", "string", "A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).", 0, 1, relation); 643 case 116079: /*url*/ return new Property("url", "uri", "The reference details for the link.", 0, 1, url); 644 default: return super.getNamedProperty(_hash, _name, _checkValid); 645 } 646 647 } 648 649 @Override 650 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 651 switch (hash) { 652 case -554436100: /*relation*/ return this.relation == null ? new Base[0] : new Base[] {this.relation}; // StringType 653 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 654 default: return super.getProperty(hash, name, checkValid); 655 } 656 657 } 658 659 @Override 660 public Base setProperty(int hash, String name, Base value) throws FHIRException { 661 switch (hash) { 662 case -554436100: // relation 663 this.relation = castToString(value); // StringType 664 return value; 665 case 116079: // url 666 this.url = castToUri(value); // UriType 667 return value; 668 default: return super.setProperty(hash, name, value); 669 } 670 671 } 672 673 @Override 674 public Base setProperty(String name, Base value) throws FHIRException { 675 if (name.equals("relation")) { 676 this.relation = castToString(value); // StringType 677 } else if (name.equals("url")) { 678 this.url = castToUri(value); // UriType 679 } else 680 return super.setProperty(name, value); 681 return value; 682 } 683 684 @Override 685 public Base makeProperty(int hash, String name) throws FHIRException { 686 switch (hash) { 687 case -554436100: return getRelationElement(); 688 case 116079: return getUrlElement(); 689 default: return super.makeProperty(hash, name); 690 } 691 692 } 693 694 @Override 695 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 696 switch (hash) { 697 case -554436100: /*relation*/ return new String[] {"string"}; 698 case 116079: /*url*/ return new String[] {"uri"}; 699 default: return super.getTypesForProperty(hash, name); 700 } 701 702 } 703 704 @Override 705 public Base addChild(String name) throws FHIRException { 706 if (name.equals("relation")) { 707 throw new FHIRException("Cannot call addChild on a singleton property Bundle.relation"); 708 } 709 else if (name.equals("url")) { 710 throw new FHIRException("Cannot call addChild on a singleton property Bundle.url"); 711 } 712 else 713 return super.addChild(name); 714 } 715 716 public BundleLinkComponent copy() { 717 BundleLinkComponent dst = new BundleLinkComponent(); 718 copyValues(dst); 719 dst.relation = relation == null ? null : relation.copy(); 720 dst.url = url == null ? null : url.copy(); 721 return dst; 722 } 723 724 @Override 725 public boolean equalsDeep(Base other_) { 726 if (!super.equalsDeep(other_)) 727 return false; 728 if (!(other_ instanceof BundleLinkComponent)) 729 return false; 730 BundleLinkComponent o = (BundleLinkComponent) other_; 731 return compareDeep(relation, o.relation, true) && compareDeep(url, o.url, true); 732 } 733 734 @Override 735 public boolean equalsShallow(Base other_) { 736 if (!super.equalsShallow(other_)) 737 return false; 738 if (!(other_ instanceof BundleLinkComponent)) 739 return false; 740 BundleLinkComponent o = (BundleLinkComponent) other_; 741 return compareValues(relation, o.relation, true) && compareValues(url, o.url, true); 742 } 743 744 public boolean isEmpty() { 745 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relation, url); 746 } 747 748 public String fhirType() { 749 return "Bundle.link"; 750 751 } 752 753 } 754 755 @Block() 756 public static class BundleEntryComponent extends BackboneElement implements IBaseBackboneElement { 757 /** 758 * A series of links that provide context to this entry. 759 */ 760 @Child(name = "link", type = {BundleLinkComponent.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 761 @Description(shortDefinition="Links related to this entry", formalDefinition="A series of links that provide context to this entry." ) 762 protected List<BundleLinkComponent> link; 763 764 /** 765 * The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: 766* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle) 767* Results from operations might involve resources that are not identified. 768 */ 769 @Child(name = "fullUrl", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 770 @Description(shortDefinition="Absolute URL for resource (server address, or UUID/OID)", formalDefinition="The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: \n* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle)\n* Results from operations might involve resources that are not identified." ) 771 protected UriType fullUrl; 772 773 /** 774 * The Resources for the entry. 775 */ 776 @Child(name = "resource", type = {Resource.class}, order=3, min=0, max=1, modifier=false, summary=true) 777 @Description(shortDefinition="A resource in the bundle", formalDefinition="The Resources for the entry." ) 778 protected Resource resource; 779 780 /** 781 * Information about the search process that lead to the creation of this entry. 782 */ 783 @Child(name = "search", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 784 @Description(shortDefinition="Search related information", formalDefinition="Information about the search process that lead to the creation of this entry." ) 785 protected BundleEntrySearchComponent search; 786 787 /** 788 * Additional information about how this entry should be processed as part of a transaction. 789 */ 790 @Child(name = "request", type = {}, order=5, min=0, max=1, modifier=false, summary=true) 791 @Description(shortDefinition="Transaction Related Information", formalDefinition="Additional information about how this entry should be processed as part of a transaction." ) 792 protected BundleEntryRequestComponent request; 793 794 /** 795 * Additional information about how this entry should be processed as part of a transaction. 796 */ 797 @Child(name = "response", type = {}, order=6, min=0, max=1, modifier=false, summary=true) 798 @Description(shortDefinition="Transaction Related Information", formalDefinition="Additional information about how this entry should be processed as part of a transaction." ) 799 protected BundleEntryResponseComponent response; 800 801 private static final long serialVersionUID = 517783054L; 802 803 /** 804 * Constructor 805 */ 806 public BundleEntryComponent() { 807 super(); 808 } 809 810 /** 811 * @return {@link #link} (A series of links that provide context to this entry.) 812 */ 813 public List<BundleLinkComponent> getLink() { 814 if (this.link == null) 815 this.link = new ArrayList<BundleLinkComponent>(); 816 return this.link; 817 } 818 819 /** 820 * @return Returns a reference to <code>this</code> for easy method chaining 821 */ 822 public BundleEntryComponent setLink(List<BundleLinkComponent> theLink) { 823 this.link = theLink; 824 return this; 825 } 826 827 public boolean hasLink() { 828 if (this.link == null) 829 return false; 830 for (BundleLinkComponent item : this.link) 831 if (!item.isEmpty()) 832 return true; 833 return false; 834 } 835 836 public BundleLinkComponent addLink() { //3 837 BundleLinkComponent t = new BundleLinkComponent(); 838 if (this.link == null) 839 this.link = new ArrayList<BundleLinkComponent>(); 840 this.link.add(t); 841 return t; 842 } 843 844 public BundleEntryComponent addLink(BundleLinkComponent t) { //3 845 if (t == null) 846 return this; 847 if (this.link == null) 848 this.link = new ArrayList<BundleLinkComponent>(); 849 this.link.add(t); 850 return this; 851 } 852 853 /** 854 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist 855 */ 856 public BundleLinkComponent getLinkFirstRep() { 857 if (getLink().isEmpty()) { 858 addLink(); 859 } 860 return getLink().get(0); 861 } 862 863 /** 864 * @return {@link #fullUrl} (The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: 865* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle) 866* Results from operations might involve resources that are not identified.). This is the underlying object with id, value and extensions. The accessor "getFullUrl" gives direct access to the value 867 */ 868 public UriType getFullUrlElement() { 869 if (this.fullUrl == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create BundleEntryComponent.fullUrl"); 872 else if (Configuration.doAutoCreate()) 873 this.fullUrl = new UriType(); // bb 874 return this.fullUrl; 875 } 876 877 public boolean hasFullUrlElement() { 878 return this.fullUrl != null && !this.fullUrl.isEmpty(); 879 } 880 881 public boolean hasFullUrl() { 882 return this.fullUrl != null && !this.fullUrl.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #fullUrl} (The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: 887* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle) 888* Results from operations might involve resources that are not identified.). This is the underlying object with id, value and extensions. The accessor "getFullUrl" gives direct access to the value 889 */ 890 public BundleEntryComponent setFullUrlElement(UriType value) { 891 this.fullUrl = value; 892 return this; 893 } 894 895 /** 896 * @return The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: 897* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle) 898* Results from operations might involve resources that are not identified. 899 */ 900 public String getFullUrl() { 901 return this.fullUrl == null ? null : this.fullUrl.getValue(); 902 } 903 904 /** 905 * @param value The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: 906* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle) 907* Results from operations might involve resources that are not identified. 908 */ 909 public BundleEntryComponent setFullUrl(String value) { 910 if (Utilities.noString(value)) 911 this.fullUrl = null; 912 else { 913 if (this.fullUrl == null) 914 this.fullUrl = new UriType(); 915 this.fullUrl.setValue(value); 916 } 917 return this; 918 } 919 920 /** 921 * @return {@link #resource} (The Resources for the entry.) 922 */ 923 public Resource getResource() { 924 return this.resource; 925 } 926 927 public boolean hasResource() { 928 return this.resource != null && !this.resource.isEmpty(); 929 } 930 931 /** 932 * @param value {@link #resource} (The Resources for the entry.) 933 */ 934 public BundleEntryComponent setResource(Resource value) { 935 this.resource = value; 936 return this; 937 } 938 939 /** 940 * @return {@link #search} (Information about the search process that lead to the creation of this entry.) 941 */ 942 public BundleEntrySearchComponent getSearch() { 943 if (this.search == null) 944 if (Configuration.errorOnAutoCreate()) 945 throw new Error("Attempt to auto-create BundleEntryComponent.search"); 946 else if (Configuration.doAutoCreate()) 947 this.search = new BundleEntrySearchComponent(); // cc 948 return this.search; 949 } 950 951 public boolean hasSearch() { 952 return this.search != null && !this.search.isEmpty(); 953 } 954 955 /** 956 * @param value {@link #search} (Information about the search process that lead to the creation of this entry.) 957 */ 958 public BundleEntryComponent setSearch(BundleEntrySearchComponent value) { 959 this.search = value; 960 return this; 961 } 962 963 /** 964 * @return {@link #request} (Additional information about how this entry should be processed as part of a transaction.) 965 */ 966 public BundleEntryRequestComponent getRequest() { 967 if (this.request == null) 968 if (Configuration.errorOnAutoCreate()) 969 throw new Error("Attempt to auto-create BundleEntryComponent.request"); 970 else if (Configuration.doAutoCreate()) 971 this.request = new BundleEntryRequestComponent(); // cc 972 return this.request; 973 } 974 975 public boolean hasRequest() { 976 return this.request != null && !this.request.isEmpty(); 977 } 978 979 /** 980 * @param value {@link #request} (Additional information about how this entry should be processed as part of a transaction.) 981 */ 982 public BundleEntryComponent setRequest(BundleEntryRequestComponent value) { 983 this.request = value; 984 return this; 985 } 986 987 /** 988 * @return {@link #response} (Additional information about how this entry should be processed as part of a transaction.) 989 */ 990 public BundleEntryResponseComponent getResponse() { 991 if (this.response == null) 992 if (Configuration.errorOnAutoCreate()) 993 throw new Error("Attempt to auto-create BundleEntryComponent.response"); 994 else if (Configuration.doAutoCreate()) 995 this.response = new BundleEntryResponseComponent(); // cc 996 return this.response; 997 } 998 999 public boolean hasResponse() { 1000 return this.response != null && !this.response.isEmpty(); 1001 } 1002 1003 /** 1004 * @param value {@link #response} (Additional information about how this entry should be processed as part of a transaction.) 1005 */ 1006 public BundleEntryComponent setResponse(BundleEntryResponseComponent value) { 1007 this.response = value; 1008 return this; 1009 } 1010 1011 /** 1012 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 1013 * If no link is found which matches the given relation, returns <code>null</code>. If more than one 1014 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 1015 * 1016 * @param theRelation 1017 * The relation, such as "next", or "self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 1018 * @return Returns a matching BundleLinkComponent, or <code>null</code> 1019 * @see IBaseBundle#LINK_NEXT 1020 * @see IBaseBundle#LINK_PREV 1021 * @see IBaseBundle#LINK_SELF 1022 */ 1023 public BundleLinkComponent getLink(String theRelation) { 1024 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 1025 for (BundleLinkComponent next : getLink()) { 1026 if (theRelation.equals(next.getRelation())) { 1027 return next; 1028 } 1029 } 1030 return null; 1031 } 1032 1033 /** 1034 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 1035 * If no link is found which matches the given relation, creates a new BundleLinkComponent with the 1036 * given relation and adds it to this Bundle. If more than one 1037 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 1038 * 1039 * @param theRelation 1040 * The relation, such as "next", or "self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 1041 * @return Returns a matching BundleLinkComponent, or <code>null</code> 1042 * @see IBaseBundle#LINK_NEXT 1043 * @see IBaseBundle#LINK_PREV 1044 * @see IBaseBundle#LINK_SELF 1045 */ 1046 public BundleLinkComponent getLinkOrCreate(String theRelation) { 1047 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 1048 for (BundleLinkComponent next : getLink()) { 1049 if (theRelation.equals(next.getRelation())) { 1050 return next; 1051 } 1052 } 1053 BundleLinkComponent retVal = new BundleLinkComponent(); 1054 retVal.setRelation(theRelation); 1055 getLink().add(retVal); 1056 return retVal; 1057 } 1058 protected void listChildren(List<Property> children) { 1059 super.listChildren(children); 1060 children.add(new Property("link", "@Bundle.link", "A series of links that provide context to this entry.", 0, java.lang.Integer.MAX_VALUE, link)); 1061 children.add(new Property("fullUrl", "uri", "The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: \n* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle)\n* Results from operations might involve resources that are not identified.", 0, 1, fullUrl)); 1062 children.add(new Property("resource", "Resource", "The Resources for the entry.", 0, 1, resource)); 1063 children.add(new Property("search", "", "Information about the search process that lead to the creation of this entry.", 0, 1, search)); 1064 children.add(new Property("request", "", "Additional information about how this entry should be processed as part of a transaction.", 0, 1, request)); 1065 children.add(new Property("response", "", "Additional information about how this entry should be processed as part of a transaction.", 0, 1, response)); 1066 } 1067 1068 @Override 1069 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1070 switch (_hash) { 1071 case 3321850: /*link*/ return new Property("link", "@Bundle.link", "A series of links that provide context to this entry.", 0, java.lang.Integer.MAX_VALUE, link); 1072 case -511251360: /*fullUrl*/ return new Property("fullUrl", "uri", "The Absolute URL for the resource. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: \n* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle)\n* Results from operations might involve resources that are not identified.", 0, 1, fullUrl); 1073 case -341064690: /*resource*/ return new Property("resource", "Resource", "The Resources for the entry.", 0, 1, resource); 1074 case -906336856: /*search*/ return new Property("search", "", "Information about the search process that lead to the creation of this entry.", 0, 1, search); 1075 case 1095692943: /*request*/ return new Property("request", "", "Additional information about how this entry should be processed as part of a transaction.", 0, 1, request); 1076 case -340323263: /*response*/ return new Property("response", "", "Additional information about how this entry should be processed as part of a transaction.", 0, 1, response); 1077 default: return super.getNamedProperty(_hash, _name, _checkValid); 1078 } 1079 1080 } 1081 1082 @Override 1083 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1084 switch (hash) { 1085 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // BundleLinkComponent 1086 case -511251360: /*fullUrl*/ return this.fullUrl == null ? new Base[0] : new Base[] {this.fullUrl}; // UriType 1087 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Resource 1088 case -906336856: /*search*/ return this.search == null ? new Base[0] : new Base[] {this.search}; // BundleEntrySearchComponent 1089 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // BundleEntryRequestComponent 1090 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // BundleEntryResponseComponent 1091 default: return super.getProperty(hash, name, checkValid); 1092 } 1093 1094 } 1095 1096 @Override 1097 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1098 switch (hash) { 1099 case 3321850: // link 1100 this.getLink().add((BundleLinkComponent) value); // BundleLinkComponent 1101 return value; 1102 case -511251360: // fullUrl 1103 this.fullUrl = castToUri(value); // UriType 1104 return value; 1105 case -341064690: // resource 1106 this.resource = castToResource(value); // Resource 1107 return value; 1108 case -906336856: // search 1109 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 1110 return value; 1111 case 1095692943: // request 1112 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 1113 return value; 1114 case -340323263: // response 1115 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 1116 return value; 1117 default: return super.setProperty(hash, name, value); 1118 } 1119 1120 } 1121 1122 @Override 1123 public Base setProperty(String name, Base value) throws FHIRException { 1124 if (name.equals("link")) { 1125 this.getLink().add((BundleLinkComponent) value); 1126 } else if (name.equals("fullUrl")) { 1127 this.fullUrl = castToUri(value); // UriType 1128 } else if (name.equals("resource")) { 1129 this.resource = castToResource(value); // Resource 1130 } else if (name.equals("search")) { 1131 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 1132 } else if (name.equals("request")) { 1133 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 1134 } else if (name.equals("response")) { 1135 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 1136 } else 1137 return super.setProperty(name, value); 1138 return value; 1139 } 1140 1141 @Override 1142 public Base makeProperty(int hash, String name) throws FHIRException { 1143 switch (hash) { 1144 case 3321850: return addLink(); 1145 case -511251360: return getFullUrlElement(); 1146 case -341064690: throw new FHIRException("Cannot make property resource as it is not a complex type"); // Resource 1147 case -906336856: return getSearch(); 1148 case 1095692943: return getRequest(); 1149 case -340323263: return getResponse(); 1150 default: return super.makeProperty(hash, name); 1151 } 1152 1153 } 1154 1155 @Override 1156 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1157 switch (hash) { 1158 case 3321850: /*link*/ return new String[] {"@Bundle.link"}; 1159 case -511251360: /*fullUrl*/ return new String[] {"uri"}; 1160 case -341064690: /*resource*/ return new String[] {"Resource"}; 1161 case -906336856: /*search*/ return new String[] {}; 1162 case 1095692943: /*request*/ return new String[] {}; 1163 case -340323263: /*response*/ return new String[] {}; 1164 default: return super.getTypesForProperty(hash, name); 1165 } 1166 1167 } 1168 1169 @Override 1170 public Base addChild(String name) throws FHIRException { 1171 if (name.equals("link")) { 1172 return addLink(); 1173 } 1174 else if (name.equals("fullUrl")) { 1175 throw new FHIRException("Cannot call addChild on a singleton property Bundle.fullUrl"); 1176 } 1177 else if (name.equals("resource")) { 1178 throw new FHIRException("Cannot call addChild on an abstract type Bundle.resource"); 1179 } 1180 else if (name.equals("search")) { 1181 this.search = new BundleEntrySearchComponent(); 1182 return this.search; 1183 } 1184 else if (name.equals("request")) { 1185 this.request = new BundleEntryRequestComponent(); 1186 return this.request; 1187 } 1188 else if (name.equals("response")) { 1189 this.response = new BundleEntryResponseComponent(); 1190 return this.response; 1191 } 1192 else 1193 return super.addChild(name); 1194 } 1195 1196 public BundleEntryComponent copy() { 1197 BundleEntryComponent dst = new BundleEntryComponent(); 1198 copyValues(dst); 1199 if (link != null) { 1200 dst.link = new ArrayList<BundleLinkComponent>(); 1201 for (BundleLinkComponent i : link) 1202 dst.link.add(i.copy()); 1203 }; 1204 dst.fullUrl = fullUrl == null ? null : fullUrl.copy(); 1205 dst.resource = resource == null ? null : resource.copy(); 1206 dst.search = search == null ? null : search.copy(); 1207 dst.request = request == null ? null : request.copy(); 1208 dst.response = response == null ? null : response.copy(); 1209 return dst; 1210 } 1211 1212 @Override 1213 public boolean equalsDeep(Base other_) { 1214 if (!super.equalsDeep(other_)) 1215 return false; 1216 if (!(other_ instanceof BundleEntryComponent)) 1217 return false; 1218 BundleEntryComponent o = (BundleEntryComponent) other_; 1219 return compareDeep(link, o.link, true) && compareDeep(fullUrl, o.fullUrl, true) && compareDeep(resource, o.resource, true) 1220 && compareDeep(search, o.search, true) && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 1221 ; 1222 } 1223 1224 @Override 1225 public boolean equalsShallow(Base other_) { 1226 if (!super.equalsShallow(other_)) 1227 return false; 1228 if (!(other_ instanceof BundleEntryComponent)) 1229 return false; 1230 BundleEntryComponent o = (BundleEntryComponent) other_; 1231 return compareValues(fullUrl, o.fullUrl, true); 1232 } 1233 1234 public boolean isEmpty() { 1235 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link, fullUrl, resource 1236 , search, request, response); 1237 } 1238 1239 public String fhirType() { 1240 return "Bundle.entry"; 1241 1242 } 1243 1244 } 1245 1246 @Block() 1247 public static class BundleEntrySearchComponent extends BackboneElement implements IBaseBackboneElement { 1248 /** 1249 * Why this entry is in the result set - whether it's included as a match or because of an _include requirement. 1250 */ 1251 @Child(name = "mode", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1252 @Description(shortDefinition="match | include | outcome - why this is in the result set", formalDefinition="Why this entry is in the result set - whether it's included as a match or because of an _include requirement." ) 1253 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-entry-mode") 1254 protected Enumeration<SearchEntryMode> mode; 1255 1256 /** 1257 * When searching, the server's search ranking score for the entry. 1258 */ 1259 @Child(name = "score", type = {DecimalType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1260 @Description(shortDefinition="Search ranking (between 0 and 1)", formalDefinition="When searching, the server's search ranking score for the entry." ) 1261 protected DecimalType score; 1262 1263 private static final long serialVersionUID = 837739866L; 1264 1265 /** 1266 * Constructor 1267 */ 1268 public BundleEntrySearchComponent() { 1269 super(); 1270 } 1271 1272 /** 1273 * @return {@link #mode} (Why this entry is in the result set - whether it's included as a match or because of an _include requirement.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1274 */ 1275 public Enumeration<SearchEntryMode> getModeElement() { 1276 if (this.mode == null) 1277 if (Configuration.errorOnAutoCreate()) 1278 throw new Error("Attempt to auto-create BundleEntrySearchComponent.mode"); 1279 else if (Configuration.doAutoCreate()) 1280 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); // bb 1281 return this.mode; 1282 } 1283 1284 public boolean hasModeElement() { 1285 return this.mode != null && !this.mode.isEmpty(); 1286 } 1287 1288 public boolean hasMode() { 1289 return this.mode != null && !this.mode.isEmpty(); 1290 } 1291 1292 /** 1293 * @param value {@link #mode} (Why this entry is in the result set - whether it's included as a match or because of an _include requirement.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1294 */ 1295 public BundleEntrySearchComponent setModeElement(Enumeration<SearchEntryMode> value) { 1296 this.mode = value; 1297 return this; 1298 } 1299 1300 /** 1301 * @return Why this entry is in the result set - whether it's included as a match or because of an _include requirement. 1302 */ 1303 public SearchEntryMode getMode() { 1304 return this.mode == null ? null : this.mode.getValue(); 1305 } 1306 1307 /** 1308 * @param value Why this entry is in the result set - whether it's included as a match or because of an _include requirement. 1309 */ 1310 public BundleEntrySearchComponent setMode(SearchEntryMode value) { 1311 if (value == null) 1312 this.mode = null; 1313 else { 1314 if (this.mode == null) 1315 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); 1316 this.mode.setValue(value); 1317 } 1318 return this; 1319 } 1320 1321 /** 1322 * @return {@link #score} (When searching, the server's search ranking score for the entry.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 1323 */ 1324 public DecimalType getScoreElement() { 1325 if (this.score == null) 1326 if (Configuration.errorOnAutoCreate()) 1327 throw new Error("Attempt to auto-create BundleEntrySearchComponent.score"); 1328 else if (Configuration.doAutoCreate()) 1329 this.score = new DecimalType(); // bb 1330 return this.score; 1331 } 1332 1333 public boolean hasScoreElement() { 1334 return this.score != null && !this.score.isEmpty(); 1335 } 1336 1337 public boolean hasScore() { 1338 return this.score != null && !this.score.isEmpty(); 1339 } 1340 1341 /** 1342 * @param value {@link #score} (When searching, the server's search ranking score for the entry.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 1343 */ 1344 public BundleEntrySearchComponent setScoreElement(DecimalType value) { 1345 this.score = value; 1346 return this; 1347 } 1348 1349 /** 1350 * @return When searching, the server's search ranking score for the entry. 1351 */ 1352 public BigDecimal getScore() { 1353 return this.score == null ? null : this.score.getValue(); 1354 } 1355 1356 /** 1357 * @param value When searching, the server's search ranking score for the entry. 1358 */ 1359 public BundleEntrySearchComponent setScore(BigDecimal value) { 1360 if (value == null) 1361 this.score = null; 1362 else { 1363 if (this.score == null) 1364 this.score = new DecimalType(); 1365 this.score.setValue(value); 1366 } 1367 return this; 1368 } 1369 1370 /** 1371 * @param value When searching, the server's search ranking score for the entry. 1372 */ 1373 public BundleEntrySearchComponent setScore(long value) { 1374 this.score = new DecimalType(); 1375 this.score.setValue(value); 1376 return this; 1377 } 1378 1379 /** 1380 * @param value When searching, the server's search ranking score for the entry. 1381 */ 1382 public BundleEntrySearchComponent setScore(double value) { 1383 this.score = new DecimalType(); 1384 this.score.setValue(value); 1385 return this; 1386 } 1387 1388 protected void listChildren(List<Property> children) { 1389 super.listChildren(children); 1390 children.add(new Property("mode", "code", "Why this entry is in the result set - whether it's included as a match or because of an _include requirement.", 0, 1, mode)); 1391 children.add(new Property("score", "decimal", "When searching, the server's search ranking score for the entry.", 0, 1, score)); 1392 } 1393 1394 @Override 1395 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1396 switch (_hash) { 1397 case 3357091: /*mode*/ return new Property("mode", "code", "Why this entry is in the result set - whether it's included as a match or because of an _include requirement.", 0, 1, mode); 1398 case 109264530: /*score*/ return new Property("score", "decimal", "When searching, the server's search ranking score for the entry.", 0, 1, score); 1399 default: return super.getNamedProperty(_hash, _name, _checkValid); 1400 } 1401 1402 } 1403 1404 @Override 1405 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1406 switch (hash) { 1407 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<SearchEntryMode> 1408 case 109264530: /*score*/ return this.score == null ? new Base[0] : new Base[] {this.score}; // DecimalType 1409 default: return super.getProperty(hash, name, checkValid); 1410 } 1411 1412 } 1413 1414 @Override 1415 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1416 switch (hash) { 1417 case 3357091: // mode 1418 value = new SearchEntryModeEnumFactory().fromType(castToCode(value)); 1419 this.mode = (Enumeration) value; // Enumeration<SearchEntryMode> 1420 return value; 1421 case 109264530: // score 1422 this.score = castToDecimal(value); // DecimalType 1423 return value; 1424 default: return super.setProperty(hash, name, value); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base setProperty(String name, Base value) throws FHIRException { 1431 if (name.equals("mode")) { 1432 value = new SearchEntryModeEnumFactory().fromType(castToCode(value)); 1433 this.mode = (Enumeration) value; // Enumeration<SearchEntryMode> 1434 } else if (name.equals("score")) { 1435 this.score = castToDecimal(value); // DecimalType 1436 } else 1437 return super.setProperty(name, value); 1438 return value; 1439 } 1440 1441 @Override 1442 public Base makeProperty(int hash, String name) throws FHIRException { 1443 switch (hash) { 1444 case 3357091: return getModeElement(); 1445 case 109264530: return getScoreElement(); 1446 default: return super.makeProperty(hash, name); 1447 } 1448 1449 } 1450 1451 @Override 1452 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1453 switch (hash) { 1454 case 3357091: /*mode*/ return new String[] {"code"}; 1455 case 109264530: /*score*/ return new String[] {"decimal"}; 1456 default: return super.getTypesForProperty(hash, name); 1457 } 1458 1459 } 1460 1461 @Override 1462 public Base addChild(String name) throws FHIRException { 1463 if (name.equals("mode")) { 1464 throw new FHIRException("Cannot call addChild on a singleton property Bundle.mode"); 1465 } 1466 else if (name.equals("score")) { 1467 throw new FHIRException("Cannot call addChild on a singleton property Bundle.score"); 1468 } 1469 else 1470 return super.addChild(name); 1471 } 1472 1473 public BundleEntrySearchComponent copy() { 1474 BundleEntrySearchComponent dst = new BundleEntrySearchComponent(); 1475 copyValues(dst); 1476 dst.mode = mode == null ? null : mode.copy(); 1477 dst.score = score == null ? null : score.copy(); 1478 return dst; 1479 } 1480 1481 @Override 1482 public boolean equalsDeep(Base other_) { 1483 if (!super.equalsDeep(other_)) 1484 return false; 1485 if (!(other_ instanceof BundleEntrySearchComponent)) 1486 return false; 1487 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other_; 1488 return compareDeep(mode, o.mode, true) && compareDeep(score, o.score, true); 1489 } 1490 1491 @Override 1492 public boolean equalsShallow(Base other_) { 1493 if (!super.equalsShallow(other_)) 1494 return false; 1495 if (!(other_ instanceof BundleEntrySearchComponent)) 1496 return false; 1497 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other_; 1498 return compareValues(mode, o.mode, true) && compareValues(score, o.score, true); 1499 } 1500 1501 public boolean isEmpty() { 1502 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, score); 1503 } 1504 1505 public String fhirType() { 1506 return "Bundle.entry.search"; 1507 1508 } 1509 1510 } 1511 1512 @Block() 1513 public static class BundleEntryRequestComponent extends BackboneElement implements IBaseBackboneElement { 1514 /** 1515 * The HTTP verb for this entry in either a change history, or a transaction/ transaction response. 1516 */ 1517 @Child(name = "method", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1518 @Description(shortDefinition="GET | POST | PUT | DELETE", formalDefinition="The HTTP verb for this entry in either a change history, or a transaction/ transaction response." ) 1519 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/http-verb") 1520 protected Enumeration<HTTPVerb> method; 1521 1522 /** 1523 * The URL for this entry, relative to the root (the address to which the request is posted). 1524 */ 1525 @Child(name = "url", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1526 @Description(shortDefinition="URL for HTTP equivalent of this entry", formalDefinition="The URL for this entry, relative to the root (the address to which the request is posted)." ) 1527 protected UriType url; 1528 1529 /** 1530 * If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread). 1531 */ 1532 @Child(name = "ifNoneMatch", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1533 @Description(shortDefinition="For managing cache currency", formalDefinition="If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread)." ) 1534 protected StringType ifNoneMatch; 1535 1536 /** 1537 * Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread). 1538 */ 1539 @Child(name = "ifModifiedSince", type = {InstantType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1540 @Description(shortDefinition="For managing update contention", formalDefinition="Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread)." ) 1541 protected InstantType ifModifiedSince; 1542 1543 /** 1544 * Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency). 1545 */ 1546 @Child(name = "ifMatch", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1547 @Description(shortDefinition="For managing update contention", formalDefinition="Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency)." ) 1548 protected StringType ifMatch; 1549 1550 /** 1551 * Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?"). 1552 */ 1553 @Child(name = "ifNoneExist", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 1554 @Description(shortDefinition="For conditional creates", formalDefinition="Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\")." ) 1555 protected StringType ifNoneExist; 1556 1557 private static final long serialVersionUID = -1349769744L; 1558 1559 /** 1560 * Constructor 1561 */ 1562 public BundleEntryRequestComponent() { 1563 super(); 1564 } 1565 1566 /** 1567 * Constructor 1568 */ 1569 public BundleEntryRequestComponent(Enumeration<HTTPVerb> method, UriType url) { 1570 super(); 1571 this.method = method; 1572 this.url = url; 1573 } 1574 1575 /** 1576 * @return {@link #method} (The HTTP verb for this entry in either a change history, or a transaction/ transaction response.). This is the underlying object with id, value and extensions. The accessor "getMethod" gives direct access to the value 1577 */ 1578 public Enumeration<HTTPVerb> getMethodElement() { 1579 if (this.method == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create BundleEntryRequestComponent.method"); 1582 else if (Configuration.doAutoCreate()) 1583 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); // bb 1584 return this.method; 1585 } 1586 1587 public boolean hasMethodElement() { 1588 return this.method != null && !this.method.isEmpty(); 1589 } 1590 1591 public boolean hasMethod() { 1592 return this.method != null && !this.method.isEmpty(); 1593 } 1594 1595 /** 1596 * @param value {@link #method} (The HTTP verb for this entry in either a change history, or a transaction/ transaction response.). This is the underlying object with id, value and extensions. The accessor "getMethod" gives direct access to the value 1597 */ 1598 public BundleEntryRequestComponent setMethodElement(Enumeration<HTTPVerb> value) { 1599 this.method = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return The HTTP verb for this entry in either a change history, or a transaction/ transaction response. 1605 */ 1606 public HTTPVerb getMethod() { 1607 return this.method == null ? null : this.method.getValue(); 1608 } 1609 1610 /** 1611 * @param value The HTTP verb for this entry in either a change history, or a transaction/ transaction response. 1612 */ 1613 public BundleEntryRequestComponent setMethod(HTTPVerb value) { 1614 if (this.method == null) 1615 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); 1616 this.method.setValue(value); 1617 return this; 1618 } 1619 1620 /** 1621 * @return {@link #url} (The URL for this entry, relative to the root (the address to which the request is posted).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1622 */ 1623 public UriType getUrlElement() { 1624 if (this.url == null) 1625 if (Configuration.errorOnAutoCreate()) 1626 throw new Error("Attempt to auto-create BundleEntryRequestComponent.url"); 1627 else if (Configuration.doAutoCreate()) 1628 this.url = new UriType(); // bb 1629 return this.url; 1630 } 1631 1632 public boolean hasUrlElement() { 1633 return this.url != null && !this.url.isEmpty(); 1634 } 1635 1636 public boolean hasUrl() { 1637 return this.url != null && !this.url.isEmpty(); 1638 } 1639 1640 /** 1641 * @param value {@link #url} (The URL for this entry, relative to the root (the address to which the request is posted).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1642 */ 1643 public BundleEntryRequestComponent setUrlElement(UriType value) { 1644 this.url = value; 1645 return this; 1646 } 1647 1648 /** 1649 * @return The URL for this entry, relative to the root (the address to which the request is posted). 1650 */ 1651 public String getUrl() { 1652 return this.url == null ? null : this.url.getValue(); 1653 } 1654 1655 /** 1656 * @param value The URL for this entry, relative to the root (the address to which the request is posted). 1657 */ 1658 public BundleEntryRequestComponent setUrl(String value) { 1659 if (this.url == null) 1660 this.url = new UriType(); 1661 this.url.setValue(value); 1662 return this; 1663 } 1664 1665 /** 1666 * @return {@link #ifNoneMatch} (If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfNoneMatch" gives direct access to the value 1667 */ 1668 public StringType getIfNoneMatchElement() { 1669 if (this.ifNoneMatch == null) 1670 if (Configuration.errorOnAutoCreate()) 1671 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneMatch"); 1672 else if (Configuration.doAutoCreate()) 1673 this.ifNoneMatch = new StringType(); // bb 1674 return this.ifNoneMatch; 1675 } 1676 1677 public boolean hasIfNoneMatchElement() { 1678 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 1679 } 1680 1681 public boolean hasIfNoneMatch() { 1682 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 1683 } 1684 1685 /** 1686 * @param value {@link #ifNoneMatch} (If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfNoneMatch" gives direct access to the value 1687 */ 1688 public BundleEntryRequestComponent setIfNoneMatchElement(StringType value) { 1689 this.ifNoneMatch = value; 1690 return this; 1691 } 1692 1693 /** 1694 * @return If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread). 1695 */ 1696 public String getIfNoneMatch() { 1697 return this.ifNoneMatch == null ? null : this.ifNoneMatch.getValue(); 1698 } 1699 1700 /** 1701 * @param value If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread). 1702 */ 1703 public BundleEntryRequestComponent setIfNoneMatch(String value) { 1704 if (Utilities.noString(value)) 1705 this.ifNoneMatch = null; 1706 else { 1707 if (this.ifNoneMatch == null) 1708 this.ifNoneMatch = new StringType(); 1709 this.ifNoneMatch.setValue(value); 1710 } 1711 return this; 1712 } 1713 1714 /** 1715 * @return {@link #ifModifiedSince} (Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfModifiedSince" gives direct access to the value 1716 */ 1717 public InstantType getIfModifiedSinceElement() { 1718 if (this.ifModifiedSince == null) 1719 if (Configuration.errorOnAutoCreate()) 1720 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifModifiedSince"); 1721 else if (Configuration.doAutoCreate()) 1722 this.ifModifiedSince = new InstantType(); // bb 1723 return this.ifModifiedSince; 1724 } 1725 1726 public boolean hasIfModifiedSinceElement() { 1727 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 1728 } 1729 1730 public boolean hasIfModifiedSince() { 1731 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 1732 } 1733 1734 /** 1735 * @param value {@link #ifModifiedSince} (Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfModifiedSince" gives direct access to the value 1736 */ 1737 public BundleEntryRequestComponent setIfModifiedSinceElement(InstantType value) { 1738 this.ifModifiedSince = value; 1739 return this; 1740 } 1741 1742 /** 1743 * @return Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread). 1744 */ 1745 public Date getIfModifiedSince() { 1746 return this.ifModifiedSince == null ? null : this.ifModifiedSince.getValue(); 1747 } 1748 1749 /** 1750 * @param value Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread). 1751 */ 1752 public BundleEntryRequestComponent setIfModifiedSince(Date value) { 1753 if (value == null) 1754 this.ifModifiedSince = null; 1755 else { 1756 if (this.ifModifiedSince == null) 1757 this.ifModifiedSince = new InstantType(); 1758 this.ifModifiedSince.setValue(value); 1759 } 1760 return this; 1761 } 1762 1763 /** 1764 * @return {@link #ifMatch} (Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency).). This is the underlying object with id, value and extensions. The accessor "getIfMatch" gives direct access to the value 1765 */ 1766 public StringType getIfMatchElement() { 1767 if (this.ifMatch == null) 1768 if (Configuration.errorOnAutoCreate()) 1769 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifMatch"); 1770 else if (Configuration.doAutoCreate()) 1771 this.ifMatch = new StringType(); // bb 1772 return this.ifMatch; 1773 } 1774 1775 public boolean hasIfMatchElement() { 1776 return this.ifMatch != null && !this.ifMatch.isEmpty(); 1777 } 1778 1779 public boolean hasIfMatch() { 1780 return this.ifMatch != null && !this.ifMatch.isEmpty(); 1781 } 1782 1783 /** 1784 * @param value {@link #ifMatch} (Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency).). This is the underlying object with id, value and extensions. The accessor "getIfMatch" gives direct access to the value 1785 */ 1786 public BundleEntryRequestComponent setIfMatchElement(StringType value) { 1787 this.ifMatch = value; 1788 return this; 1789 } 1790 1791 /** 1792 * @return Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency). 1793 */ 1794 public String getIfMatch() { 1795 return this.ifMatch == null ? null : this.ifMatch.getValue(); 1796 } 1797 1798 /** 1799 * @param value Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency). 1800 */ 1801 public BundleEntryRequestComponent setIfMatch(String value) { 1802 if (Utilities.noString(value)) 1803 this.ifMatch = null; 1804 else { 1805 if (this.ifMatch == null) 1806 this.ifMatch = new StringType(); 1807 this.ifMatch.setValue(value); 1808 } 1809 return this; 1810 } 1811 1812 /** 1813 * @return {@link #ifNoneExist} (Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?").). This is the underlying object with id, value and extensions. The accessor "getIfNoneExist" gives direct access to the value 1814 */ 1815 public StringType getIfNoneExistElement() { 1816 if (this.ifNoneExist == null) 1817 if (Configuration.errorOnAutoCreate()) 1818 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneExist"); 1819 else if (Configuration.doAutoCreate()) 1820 this.ifNoneExist = new StringType(); // bb 1821 return this.ifNoneExist; 1822 } 1823 1824 public boolean hasIfNoneExistElement() { 1825 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 1826 } 1827 1828 public boolean hasIfNoneExist() { 1829 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 1830 } 1831 1832 /** 1833 * @param value {@link #ifNoneExist} (Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?").). This is the underlying object with id, value and extensions. The accessor "getIfNoneExist" gives direct access to the value 1834 */ 1835 public BundleEntryRequestComponent setIfNoneExistElement(StringType value) { 1836 this.ifNoneExist = value; 1837 return this; 1838 } 1839 1840 /** 1841 * @return Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?"). 1842 */ 1843 public String getIfNoneExist() { 1844 return this.ifNoneExist == null ? null : this.ifNoneExist.getValue(); 1845 } 1846 1847 /** 1848 * @param value Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?"). 1849 */ 1850 public BundleEntryRequestComponent setIfNoneExist(String value) { 1851 if (Utilities.noString(value)) 1852 this.ifNoneExist = null; 1853 else { 1854 if (this.ifNoneExist == null) 1855 this.ifNoneExist = new StringType(); 1856 this.ifNoneExist.setValue(value); 1857 } 1858 return this; 1859 } 1860 1861 protected void listChildren(List<Property> children) { 1862 super.listChildren(children); 1863 children.add(new Property("method", "code", "The HTTP verb for this entry in either a change history, or a transaction/ transaction response.", 0, 1, method)); 1864 children.add(new Property("url", "uri", "The URL for this entry, relative to the root (the address to which the request is posted).", 0, 1, url)); 1865 children.add(new Property("ifNoneMatch", "string", "If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifNoneMatch)); 1866 children.add(new Property("ifModifiedSince", "instant", "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifModifiedSince)); 1867 children.add(new Property("ifMatch", "string", "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).", 0, 1, ifMatch)); 1868 children.add(new Property("ifNoneExist", "string", "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").", 0, 1, ifNoneExist)); 1869 } 1870 1871 @Override 1872 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1873 switch (_hash) { 1874 case -1077554975: /*method*/ return new Property("method", "code", "The HTTP verb for this entry in either a change history, or a transaction/ transaction response.", 0, 1, method); 1875 case 116079: /*url*/ return new Property("url", "uri", "The URL for this entry, relative to the root (the address to which the request is posted).", 0, 1, url); 1876 case 171868368: /*ifNoneMatch*/ return new Property("ifNoneMatch", "string", "If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifNoneMatch); 1877 case -2061602860: /*ifModifiedSince*/ return new Property("ifModifiedSince", "instant", "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifModifiedSince); 1878 case 1692894888: /*ifMatch*/ return new Property("ifMatch", "string", "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).", 0, 1, ifMatch); 1879 case 165155330: /*ifNoneExist*/ return new Property("ifNoneExist", "string", "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").", 0, 1, ifNoneExist); 1880 default: return super.getNamedProperty(_hash, _name, _checkValid); 1881 } 1882 1883 } 1884 1885 @Override 1886 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1887 switch (hash) { 1888 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // Enumeration<HTTPVerb> 1889 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1890 case 171868368: /*ifNoneMatch*/ return this.ifNoneMatch == null ? new Base[0] : new Base[] {this.ifNoneMatch}; // StringType 1891 case -2061602860: /*ifModifiedSince*/ return this.ifModifiedSince == null ? new Base[0] : new Base[] {this.ifModifiedSince}; // InstantType 1892 case 1692894888: /*ifMatch*/ return this.ifMatch == null ? new Base[0] : new Base[] {this.ifMatch}; // StringType 1893 case 165155330: /*ifNoneExist*/ return this.ifNoneExist == null ? new Base[0] : new Base[] {this.ifNoneExist}; // StringType 1894 default: return super.getProperty(hash, name, checkValid); 1895 } 1896 1897 } 1898 1899 @Override 1900 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1901 switch (hash) { 1902 case -1077554975: // method 1903 value = new HTTPVerbEnumFactory().fromType(castToCode(value)); 1904 this.method = (Enumeration) value; // Enumeration<HTTPVerb> 1905 return value; 1906 case 116079: // url 1907 this.url = castToUri(value); // UriType 1908 return value; 1909 case 171868368: // ifNoneMatch 1910 this.ifNoneMatch = castToString(value); // StringType 1911 return value; 1912 case -2061602860: // ifModifiedSince 1913 this.ifModifiedSince = castToInstant(value); // InstantType 1914 return value; 1915 case 1692894888: // ifMatch 1916 this.ifMatch = castToString(value); // StringType 1917 return value; 1918 case 165155330: // ifNoneExist 1919 this.ifNoneExist = castToString(value); // StringType 1920 return value; 1921 default: return super.setProperty(hash, name, value); 1922 } 1923 1924 } 1925 1926 @Override 1927 public Base setProperty(String name, Base value) throws FHIRException { 1928 if (name.equals("method")) { 1929 value = new HTTPVerbEnumFactory().fromType(castToCode(value)); 1930 this.method = (Enumeration) value; // Enumeration<HTTPVerb> 1931 } else if (name.equals("url")) { 1932 this.url = castToUri(value); // UriType 1933 } else if (name.equals("ifNoneMatch")) { 1934 this.ifNoneMatch = castToString(value); // StringType 1935 } else if (name.equals("ifModifiedSince")) { 1936 this.ifModifiedSince = castToInstant(value); // InstantType 1937 } else if (name.equals("ifMatch")) { 1938 this.ifMatch = castToString(value); // StringType 1939 } else if (name.equals("ifNoneExist")) { 1940 this.ifNoneExist = castToString(value); // StringType 1941 } else 1942 return super.setProperty(name, value); 1943 return value; 1944 } 1945 1946 @Override 1947 public Base makeProperty(int hash, String name) throws FHIRException { 1948 switch (hash) { 1949 case -1077554975: return getMethodElement(); 1950 case 116079: return getUrlElement(); 1951 case 171868368: return getIfNoneMatchElement(); 1952 case -2061602860: return getIfModifiedSinceElement(); 1953 case 1692894888: return getIfMatchElement(); 1954 case 165155330: return getIfNoneExistElement(); 1955 default: return super.makeProperty(hash, name); 1956 } 1957 1958 } 1959 1960 @Override 1961 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1962 switch (hash) { 1963 case -1077554975: /*method*/ return new String[] {"code"}; 1964 case 116079: /*url*/ return new String[] {"uri"}; 1965 case 171868368: /*ifNoneMatch*/ return new String[] {"string"}; 1966 case -2061602860: /*ifModifiedSince*/ return new String[] {"instant"}; 1967 case 1692894888: /*ifMatch*/ return new String[] {"string"}; 1968 case 165155330: /*ifNoneExist*/ return new String[] {"string"}; 1969 default: return super.getTypesForProperty(hash, name); 1970 } 1971 1972 } 1973 1974 @Override 1975 public Base addChild(String name) throws FHIRException { 1976 if (name.equals("method")) { 1977 throw new FHIRException("Cannot call addChild on a singleton property Bundle.method"); 1978 } 1979 else if (name.equals("url")) { 1980 throw new FHIRException("Cannot call addChild on a singleton property Bundle.url"); 1981 } 1982 else if (name.equals("ifNoneMatch")) { 1983 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifNoneMatch"); 1984 } 1985 else if (name.equals("ifModifiedSince")) { 1986 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifModifiedSince"); 1987 } 1988 else if (name.equals("ifMatch")) { 1989 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifMatch"); 1990 } 1991 else if (name.equals("ifNoneExist")) { 1992 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifNoneExist"); 1993 } 1994 else 1995 return super.addChild(name); 1996 } 1997 1998 public BundleEntryRequestComponent copy() { 1999 BundleEntryRequestComponent dst = new BundleEntryRequestComponent(); 2000 copyValues(dst); 2001 dst.method = method == null ? null : method.copy(); 2002 dst.url = url == null ? null : url.copy(); 2003 dst.ifNoneMatch = ifNoneMatch == null ? null : ifNoneMatch.copy(); 2004 dst.ifModifiedSince = ifModifiedSince == null ? null : ifModifiedSince.copy(); 2005 dst.ifMatch = ifMatch == null ? null : ifMatch.copy(); 2006 dst.ifNoneExist = ifNoneExist == null ? null : ifNoneExist.copy(); 2007 return dst; 2008 } 2009 2010 @Override 2011 public boolean equalsDeep(Base other_) { 2012 if (!super.equalsDeep(other_)) 2013 return false; 2014 if (!(other_ instanceof BundleEntryRequestComponent)) 2015 return false; 2016 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other_; 2017 return compareDeep(method, o.method, true) && compareDeep(url, o.url, true) && compareDeep(ifNoneMatch, o.ifNoneMatch, true) 2018 && compareDeep(ifModifiedSince, o.ifModifiedSince, true) && compareDeep(ifMatch, o.ifMatch, true) 2019 && compareDeep(ifNoneExist, o.ifNoneExist, true); 2020 } 2021 2022 @Override 2023 public boolean equalsShallow(Base other_) { 2024 if (!super.equalsShallow(other_)) 2025 return false; 2026 if (!(other_ instanceof BundleEntryRequestComponent)) 2027 return false; 2028 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other_; 2029 return compareValues(method, o.method, true) && compareValues(url, o.url, true) && compareValues(ifNoneMatch, o.ifNoneMatch, true) 2030 && compareValues(ifModifiedSince, o.ifModifiedSince, true) && compareValues(ifMatch, o.ifMatch, true) 2031 && compareValues(ifNoneExist, o.ifNoneExist, true); 2032 } 2033 2034 public boolean isEmpty() { 2035 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(method, url, ifNoneMatch 2036 , ifModifiedSince, ifMatch, ifNoneExist); 2037 } 2038 2039 public String fhirType() { 2040 return "Bundle.entry.request"; 2041 2042 } 2043 2044 } 2045 2046 @Block() 2047 public static class BundleEntryResponseComponent extends BackboneElement implements IBaseBackboneElement { 2048 /** 2049 * The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code. 2050 */ 2051 @Child(name = "status", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2052 @Description(shortDefinition="Status response code (text optional)", formalDefinition="The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code." ) 2053 protected StringType status; 2054 2055 /** 2056 * The location header created by processing this operation. 2057 */ 2058 @Child(name = "location", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2059 @Description(shortDefinition="The location, if the operation returns a location", formalDefinition="The location header created by processing this operation." ) 2060 protected UriType location; 2061 2062 /** 2063 * The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)). 2064 */ 2065 @Child(name = "etag", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2066 @Description(shortDefinition="The etag for the resource (if relevant)", formalDefinition="The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency))." ) 2067 protected StringType etag; 2068 2069 /** 2070 * The date/time that the resource was modified on the server. 2071 */ 2072 @Child(name = "lastModified", type = {InstantType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2073 @Description(shortDefinition="Server's date time modified", formalDefinition="The date/time that the resource was modified on the server." ) 2074 protected InstantType lastModified; 2075 2076 /** 2077 * An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction. 2078 */ 2079 @Child(name = "outcome", type = {Resource.class}, order=5, min=0, max=1, modifier=false, summary=true) 2080 @Description(shortDefinition="OperationOutcome with hints and warnings (for batch/transaction)", formalDefinition="An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction." ) 2081 protected Resource outcome; 2082 2083 private static final long serialVersionUID = 923278008L; 2084 2085 /** 2086 * Constructor 2087 */ 2088 public BundleEntryResponseComponent() { 2089 super(); 2090 } 2091 2092 /** 2093 * Constructor 2094 */ 2095 public BundleEntryResponseComponent(StringType status) { 2096 super(); 2097 this.status = status; 2098 } 2099 2100 /** 2101 * @return {@link #status} (The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2102 */ 2103 public StringType getStatusElement() { 2104 if (this.status == null) 2105 if (Configuration.errorOnAutoCreate()) 2106 throw new Error("Attempt to auto-create BundleEntryResponseComponent.status"); 2107 else if (Configuration.doAutoCreate()) 2108 this.status = new StringType(); // bb 2109 return this.status; 2110 } 2111 2112 public boolean hasStatusElement() { 2113 return this.status != null && !this.status.isEmpty(); 2114 } 2115 2116 public boolean hasStatus() { 2117 return this.status != null && !this.status.isEmpty(); 2118 } 2119 2120 /** 2121 * @param value {@link #status} (The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2122 */ 2123 public BundleEntryResponseComponent setStatusElement(StringType value) { 2124 this.status = value; 2125 return this; 2126 } 2127 2128 /** 2129 * @return The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code. 2130 */ 2131 public String getStatus() { 2132 return this.status == null ? null : this.status.getValue(); 2133 } 2134 2135 /** 2136 * @param value The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code. 2137 */ 2138 public BundleEntryResponseComponent setStatus(String value) { 2139 if (this.status == null) 2140 this.status = new StringType(); 2141 this.status.setValue(value); 2142 return this; 2143 } 2144 2145 /** 2146 * @return {@link #location} (The location header created by processing this operation.). This is the underlying object with id, value and extensions. The accessor "getLocation" gives direct access to the value 2147 */ 2148 public UriType getLocationElement() { 2149 if (this.location == null) 2150 if (Configuration.errorOnAutoCreate()) 2151 throw new Error("Attempt to auto-create BundleEntryResponseComponent.location"); 2152 else if (Configuration.doAutoCreate()) 2153 this.location = new UriType(); // bb 2154 return this.location; 2155 } 2156 2157 public boolean hasLocationElement() { 2158 return this.location != null && !this.location.isEmpty(); 2159 } 2160 2161 public boolean hasLocation() { 2162 return this.location != null && !this.location.isEmpty(); 2163 } 2164 2165 /** 2166 * @param value {@link #location} (The location header created by processing this operation.). This is the underlying object with id, value and extensions. The accessor "getLocation" gives direct access to the value 2167 */ 2168 public BundleEntryResponseComponent setLocationElement(UriType value) { 2169 this.location = value; 2170 return this; 2171 } 2172 2173 /** 2174 * @return The location header created by processing this operation. 2175 */ 2176 public String getLocation() { 2177 return this.location == null ? null : this.location.getValue(); 2178 } 2179 2180 /** 2181 * @param value The location header created by processing this operation. 2182 */ 2183 public BundleEntryResponseComponent setLocation(String value) { 2184 if (Utilities.noString(value)) 2185 this.location = null; 2186 else { 2187 if (this.location == null) 2188 this.location = new UriType(); 2189 this.location.setValue(value); 2190 } 2191 return this; 2192 } 2193 2194 /** 2195 * @return {@link #etag} (The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).). This is the underlying object with id, value and extensions. The accessor "getEtag" gives direct access to the value 2196 */ 2197 public StringType getEtagElement() { 2198 if (this.etag == null) 2199 if (Configuration.errorOnAutoCreate()) 2200 throw new Error("Attempt to auto-create BundleEntryResponseComponent.etag"); 2201 else if (Configuration.doAutoCreate()) 2202 this.etag = new StringType(); // bb 2203 return this.etag; 2204 } 2205 2206 public boolean hasEtagElement() { 2207 return this.etag != null && !this.etag.isEmpty(); 2208 } 2209 2210 public boolean hasEtag() { 2211 return this.etag != null && !this.etag.isEmpty(); 2212 } 2213 2214 /** 2215 * @param value {@link #etag} (The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).). This is the underlying object with id, value and extensions. The accessor "getEtag" gives direct access to the value 2216 */ 2217 public BundleEntryResponseComponent setEtagElement(StringType value) { 2218 this.etag = value; 2219 return this; 2220 } 2221 2222 /** 2223 * @return The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)). 2224 */ 2225 public String getEtag() { 2226 return this.etag == null ? null : this.etag.getValue(); 2227 } 2228 2229 /** 2230 * @param value The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)). 2231 */ 2232 public BundleEntryResponseComponent setEtag(String value) { 2233 if (Utilities.noString(value)) 2234 this.etag = null; 2235 else { 2236 if (this.etag == null) 2237 this.etag = new StringType(); 2238 this.etag.setValue(value); 2239 } 2240 return this; 2241 } 2242 2243 /** 2244 * @return {@link #lastModified} (The date/time that the resource was modified on the server.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 2245 */ 2246 public InstantType getLastModifiedElement() { 2247 if (this.lastModified == null) 2248 if (Configuration.errorOnAutoCreate()) 2249 throw new Error("Attempt to auto-create BundleEntryResponseComponent.lastModified"); 2250 else if (Configuration.doAutoCreate()) 2251 this.lastModified = new InstantType(); // bb 2252 return this.lastModified; 2253 } 2254 2255 public boolean hasLastModifiedElement() { 2256 return this.lastModified != null && !this.lastModified.isEmpty(); 2257 } 2258 2259 public boolean hasLastModified() { 2260 return this.lastModified != null && !this.lastModified.isEmpty(); 2261 } 2262 2263 /** 2264 * @param value {@link #lastModified} (The date/time that the resource was modified on the server.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 2265 */ 2266 public BundleEntryResponseComponent setLastModifiedElement(InstantType value) { 2267 this.lastModified = value; 2268 return this; 2269 } 2270 2271 /** 2272 * @return The date/time that the resource was modified on the server. 2273 */ 2274 public Date getLastModified() { 2275 return this.lastModified == null ? null : this.lastModified.getValue(); 2276 } 2277 2278 /** 2279 * @param value The date/time that the resource was modified on the server. 2280 */ 2281 public BundleEntryResponseComponent setLastModified(Date value) { 2282 if (value == null) 2283 this.lastModified = null; 2284 else { 2285 if (this.lastModified == null) 2286 this.lastModified = new InstantType(); 2287 this.lastModified.setValue(value); 2288 } 2289 return this; 2290 } 2291 2292 /** 2293 * @return {@link #outcome} (An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.) 2294 */ 2295 public Resource getOutcome() { 2296 return this.outcome; 2297 } 2298 2299 public boolean hasOutcome() { 2300 return this.outcome != null && !this.outcome.isEmpty(); 2301 } 2302 2303 /** 2304 * @param value {@link #outcome} (An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.) 2305 */ 2306 public BundleEntryResponseComponent setOutcome(Resource value) { 2307 this.outcome = value; 2308 return this; 2309 } 2310 2311 protected void listChildren(List<Property> children) { 2312 super.listChildren(children); 2313 children.add(new Property("status", "string", "The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.", 0, 1, status)); 2314 children.add(new Property("location", "uri", "The location header created by processing this operation.", 0, 1, location)); 2315 children.add(new Property("etag", "string", "The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).", 0, 1, etag)); 2316 children.add(new Property("lastModified", "instant", "The date/time that the resource was modified on the server.", 0, 1, lastModified)); 2317 children.add(new Property("outcome", "Resource", "An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.", 0, 1, outcome)); 2318 } 2319 2320 @Override 2321 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2322 switch (_hash) { 2323 case -892481550: /*status*/ return new Property("status", "string", "The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.", 0, 1, status); 2324 case 1901043637: /*location*/ return new Property("location", "uri", "The location header created by processing this operation.", 0, 1, location); 2325 case 3123477: /*etag*/ return new Property("etag", "string", "The etag for the resource, it the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).", 0, 1, etag); 2326 case 1959003007: /*lastModified*/ return new Property("lastModified", "instant", "The date/time that the resource was modified on the server.", 0, 1, lastModified); 2327 case -1106507950: /*outcome*/ return new Property("outcome", "Resource", "An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.", 0, 1, outcome); 2328 default: return super.getNamedProperty(_hash, _name, _checkValid); 2329 } 2330 2331 } 2332 2333 @Override 2334 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2335 switch (hash) { 2336 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // StringType 2337 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // UriType 2338 case 3123477: /*etag*/ return this.etag == null ? new Base[0] : new Base[] {this.etag}; // StringType 2339 case 1959003007: /*lastModified*/ return this.lastModified == null ? new Base[0] : new Base[] {this.lastModified}; // InstantType 2340 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Resource 2341 default: return super.getProperty(hash, name, checkValid); 2342 } 2343 2344 } 2345 2346 @Override 2347 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2348 switch (hash) { 2349 case -892481550: // status 2350 this.status = castToString(value); // StringType 2351 return value; 2352 case 1901043637: // location 2353 this.location = castToUri(value); // UriType 2354 return value; 2355 case 3123477: // etag 2356 this.etag = castToString(value); // StringType 2357 return value; 2358 case 1959003007: // lastModified 2359 this.lastModified = castToInstant(value); // InstantType 2360 return value; 2361 case -1106507950: // outcome 2362 this.outcome = castToResource(value); // Resource 2363 return value; 2364 default: return super.setProperty(hash, name, value); 2365 } 2366 2367 } 2368 2369 @Override 2370 public Base setProperty(String name, Base value) throws FHIRException { 2371 if (name.equals("status")) { 2372 this.status = castToString(value); // StringType 2373 } else if (name.equals("location")) { 2374 this.location = castToUri(value); // UriType 2375 } else if (name.equals("etag")) { 2376 this.etag = castToString(value); // StringType 2377 } else if (name.equals("lastModified")) { 2378 this.lastModified = castToInstant(value); // InstantType 2379 } else if (name.equals("outcome")) { 2380 this.outcome = castToResource(value); // Resource 2381 } else 2382 return super.setProperty(name, value); 2383 return value; 2384 } 2385 2386 @Override 2387 public Base makeProperty(int hash, String name) throws FHIRException { 2388 switch (hash) { 2389 case -892481550: return getStatusElement(); 2390 case 1901043637: return getLocationElement(); 2391 case 3123477: return getEtagElement(); 2392 case 1959003007: return getLastModifiedElement(); 2393 case -1106507950: throw new FHIRException("Cannot make property outcome as it is not a complex type"); // Resource 2394 default: return super.makeProperty(hash, name); 2395 } 2396 2397 } 2398 2399 @Override 2400 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2401 switch (hash) { 2402 case -892481550: /*status*/ return new String[] {"string"}; 2403 case 1901043637: /*location*/ return new String[] {"uri"}; 2404 case 3123477: /*etag*/ return new String[] {"string"}; 2405 case 1959003007: /*lastModified*/ return new String[] {"instant"}; 2406 case -1106507950: /*outcome*/ return new String[] {"Resource"}; 2407 default: return super.getTypesForProperty(hash, name); 2408 } 2409 2410 } 2411 2412 @Override 2413 public Base addChild(String name) throws FHIRException { 2414 if (name.equals("status")) { 2415 throw new FHIRException("Cannot call addChild on a singleton property Bundle.status"); 2416 } 2417 else if (name.equals("location")) { 2418 throw new FHIRException("Cannot call addChild on a singleton property Bundle.location"); 2419 } 2420 else if (name.equals("etag")) { 2421 throw new FHIRException("Cannot call addChild on a singleton property Bundle.etag"); 2422 } 2423 else if (name.equals("lastModified")) { 2424 throw new FHIRException("Cannot call addChild on a singleton property Bundle.lastModified"); 2425 } 2426 else if (name.equals("outcome")) { 2427 throw new FHIRException("Cannot call addChild on an abstract type Bundle.outcome"); 2428 } 2429 else 2430 return super.addChild(name); 2431 } 2432 2433 public BundleEntryResponseComponent copy() { 2434 BundleEntryResponseComponent dst = new BundleEntryResponseComponent(); 2435 copyValues(dst); 2436 dst.status = status == null ? null : status.copy(); 2437 dst.location = location == null ? null : location.copy(); 2438 dst.etag = etag == null ? null : etag.copy(); 2439 dst.lastModified = lastModified == null ? null : lastModified.copy(); 2440 dst.outcome = outcome == null ? null : outcome.copy(); 2441 return dst; 2442 } 2443 2444 @Override 2445 public boolean equalsDeep(Base other_) { 2446 if (!super.equalsDeep(other_)) 2447 return false; 2448 if (!(other_ instanceof BundleEntryResponseComponent)) 2449 return false; 2450 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other_; 2451 return compareDeep(status, o.status, true) && compareDeep(location, o.location, true) && compareDeep(etag, o.etag, true) 2452 && compareDeep(lastModified, o.lastModified, true) && compareDeep(outcome, o.outcome, true); 2453 } 2454 2455 @Override 2456 public boolean equalsShallow(Base other_) { 2457 if (!super.equalsShallow(other_)) 2458 return false; 2459 if (!(other_ instanceof BundleEntryResponseComponent)) 2460 return false; 2461 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other_; 2462 return compareValues(status, o.status, true) && compareValues(location, o.location, true) && compareValues(etag, o.etag, true) 2463 && compareValues(lastModified, o.lastModified, true); 2464 } 2465 2466 public boolean isEmpty() { 2467 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, location, etag, lastModified 2468 , outcome); 2469 } 2470 2471 public String fhirType() { 2472 return "Bundle.entry.response"; 2473 2474 } 2475 2476 } 2477 2478 /** 2479 * A persistent identifier for the batch that won't change as a batch is copied from server to server. 2480 */ 2481 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2482 @Description(shortDefinition="Persistent identifier for the bundle", formalDefinition="A persistent identifier for the batch that won't change as a batch is copied from server to server." ) 2483 protected Identifier identifier; 2484 2485 /** 2486 * Indicates the purpose of this bundle - how it was intended to be used. 2487 */ 2488 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2489 @Description(shortDefinition="document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection", formalDefinition="Indicates the purpose of this bundle - how it was intended to be used." ) 2490 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bundle-type") 2491 protected Enumeration<BundleType> type; 2492 2493 /** 2494 * If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle). 2495 */ 2496 @Child(name = "total", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2497 @Description(shortDefinition="If search, the total number of matches", formalDefinition="If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle)." ) 2498 protected UnsignedIntType total; 2499 2500 /** 2501 * A series of links that provide context to this bundle. 2502 */ 2503 @Child(name = "link", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2504 @Description(shortDefinition="Links related to this Bundle", formalDefinition="A series of links that provide context to this bundle." ) 2505 protected List<BundleLinkComponent> link; 2506 2507 /** 2508 * An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only). 2509 */ 2510 @Child(name = "entry", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2511 @Description(shortDefinition="Entry in the bundle - will have a resource, or information", formalDefinition="An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only)." ) 2512 protected List<BundleEntryComponent> entry; 2513 2514 /** 2515 * Digital Signature - base64 encoded. XML-DSIg or a JWT. 2516 */ 2517 @Child(name = "signature", type = {Signature.class}, order=5, min=0, max=1, modifier=false, summary=true) 2518 @Description(shortDefinition="Digital Signature", formalDefinition="Digital Signature - base64 encoded. XML-DSIg or a JWT." ) 2519 protected Signature signature; 2520 2521 private static final long serialVersionUID = 982760501L; 2522 2523 /** 2524 * Constructor 2525 */ 2526 public Bundle() { 2527 super(); 2528 } 2529 2530 /** 2531 * Constructor 2532 */ 2533 public Bundle(Enumeration<BundleType> type) { 2534 super(); 2535 this.type = type; 2536 } 2537 2538 /** 2539 * @return {@link #identifier} (A persistent identifier for the batch that won't change as a batch is copied from server to server.) 2540 */ 2541 public Identifier getIdentifier() { 2542 if (this.identifier == null) 2543 if (Configuration.errorOnAutoCreate()) 2544 throw new Error("Attempt to auto-create Bundle.identifier"); 2545 else if (Configuration.doAutoCreate()) 2546 this.identifier = new Identifier(); // cc 2547 return this.identifier; 2548 } 2549 2550 public boolean hasIdentifier() { 2551 return this.identifier != null && !this.identifier.isEmpty(); 2552 } 2553 2554 /** 2555 * @param value {@link #identifier} (A persistent identifier for the batch that won't change as a batch is copied from server to server.) 2556 */ 2557 public Bundle setIdentifier(Identifier value) { 2558 this.identifier = value; 2559 return this; 2560 } 2561 2562 /** 2563 * @return {@link #type} (Indicates the purpose of this bundle - how it was intended to be used.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2564 */ 2565 public Enumeration<BundleType> getTypeElement() { 2566 if (this.type == null) 2567 if (Configuration.errorOnAutoCreate()) 2568 throw new Error("Attempt to auto-create Bundle.type"); 2569 else if (Configuration.doAutoCreate()) 2570 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); // bb 2571 return this.type; 2572 } 2573 2574 public boolean hasTypeElement() { 2575 return this.type != null && !this.type.isEmpty(); 2576 } 2577 2578 public boolean hasType() { 2579 return this.type != null && !this.type.isEmpty(); 2580 } 2581 2582 /** 2583 * @param value {@link #type} (Indicates the purpose of this bundle - how it was intended to be used.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2584 */ 2585 public Bundle setTypeElement(Enumeration<BundleType> value) { 2586 this.type = value; 2587 return this; 2588 } 2589 2590 /** 2591 * @return Indicates the purpose of this bundle - how it was intended to be used. 2592 */ 2593 public BundleType getType() { 2594 return this.type == null ? null : this.type.getValue(); 2595 } 2596 2597 /** 2598 * @param value Indicates the purpose of this bundle - how it was intended to be used. 2599 */ 2600 public Bundle setType(BundleType value) { 2601 if (this.type == null) 2602 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); 2603 this.type.setValue(value); 2604 return this; 2605 } 2606 2607 /** 2608 * @return {@link #total} (If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle).). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 2609 */ 2610 public UnsignedIntType getTotalElement() { 2611 if (this.total == null) 2612 if (Configuration.errorOnAutoCreate()) 2613 throw new Error("Attempt to auto-create Bundle.total"); 2614 else if (Configuration.doAutoCreate()) 2615 this.total = new UnsignedIntType(); // bb 2616 return this.total; 2617 } 2618 2619 public boolean hasTotalElement() { 2620 return this.total != null && !this.total.isEmpty(); 2621 } 2622 2623 public boolean hasTotal() { 2624 return this.total != null && !this.total.isEmpty(); 2625 } 2626 2627 /** 2628 * @param value {@link #total} (If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle).). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 2629 */ 2630 public Bundle setTotalElement(UnsignedIntType value) { 2631 this.total = value; 2632 return this; 2633 } 2634 2635 /** 2636 * @return If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle). 2637 */ 2638 public int getTotal() { 2639 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 2640 } 2641 2642 /** 2643 * @param value If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle). 2644 */ 2645 public Bundle setTotal(int value) { 2646 if (this.total == null) 2647 this.total = new UnsignedIntType(); 2648 this.total.setValue(value); 2649 return this; 2650 } 2651 2652 /** 2653 * @return {@link #link} (A series of links that provide context to this bundle.) 2654 */ 2655 public List<BundleLinkComponent> getLink() { 2656 if (this.link == null) 2657 this.link = new ArrayList<BundleLinkComponent>(); 2658 return this.link; 2659 } 2660 2661 /** 2662 * @return Returns a reference to <code>this</code> for easy method chaining 2663 */ 2664 public Bundle setLink(List<BundleLinkComponent> theLink) { 2665 this.link = theLink; 2666 return this; 2667 } 2668 2669 public boolean hasLink() { 2670 if (this.link == null) 2671 return false; 2672 for (BundleLinkComponent item : this.link) 2673 if (!item.isEmpty()) 2674 return true; 2675 return false; 2676 } 2677 2678 public BundleLinkComponent addLink() { //3 2679 BundleLinkComponent t = new BundleLinkComponent(); 2680 if (this.link == null) 2681 this.link = new ArrayList<BundleLinkComponent>(); 2682 this.link.add(t); 2683 return t; 2684 } 2685 2686 public Bundle addLink(BundleLinkComponent t) { //3 2687 if (t == null) 2688 return this; 2689 if (this.link == null) 2690 this.link = new ArrayList<BundleLinkComponent>(); 2691 this.link.add(t); 2692 return this; 2693 } 2694 2695 /** 2696 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist 2697 */ 2698 public BundleLinkComponent getLinkFirstRep() { 2699 if (getLink().isEmpty()) { 2700 addLink(); 2701 } 2702 return getLink().get(0); 2703 } 2704 2705 /** 2706 * @return {@link #entry} (An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only).) 2707 */ 2708 public List<BundleEntryComponent> getEntry() { 2709 if (this.entry == null) 2710 this.entry = new ArrayList<BundleEntryComponent>(); 2711 return this.entry; 2712 } 2713 2714 /** 2715 * @return Returns a reference to <code>this</code> for easy method chaining 2716 */ 2717 public Bundle setEntry(List<BundleEntryComponent> theEntry) { 2718 this.entry = theEntry; 2719 return this; 2720 } 2721 2722 public boolean hasEntry() { 2723 if (this.entry == null) 2724 return false; 2725 for (BundleEntryComponent item : this.entry) 2726 if (!item.isEmpty()) 2727 return true; 2728 return false; 2729 } 2730 2731 public BundleEntryComponent addEntry() { //3 2732 BundleEntryComponent t = new BundleEntryComponent(); 2733 if (this.entry == null) 2734 this.entry = new ArrayList<BundleEntryComponent>(); 2735 this.entry.add(t); 2736 return t; 2737 } 2738 2739 public Bundle addEntry(BundleEntryComponent t) { //3 2740 if (t == null) 2741 return this; 2742 if (this.entry == null) 2743 this.entry = new ArrayList<BundleEntryComponent>(); 2744 this.entry.add(t); 2745 return this; 2746 } 2747 2748 /** 2749 * @return The first repetition of repeating field {@link #entry}, creating it if it does not already exist 2750 */ 2751 public BundleEntryComponent getEntryFirstRep() { 2752 if (getEntry().isEmpty()) { 2753 addEntry(); 2754 } 2755 return getEntry().get(0); 2756 } 2757 2758 /** 2759 * @return {@link #signature} (Digital Signature - base64 encoded. XML-DSIg or a JWT.) 2760 */ 2761 public Signature getSignature() { 2762 if (this.signature == null) 2763 if (Configuration.errorOnAutoCreate()) 2764 throw new Error("Attempt to auto-create Bundle.signature"); 2765 else if (Configuration.doAutoCreate()) 2766 this.signature = new Signature(); // cc 2767 return this.signature; 2768 } 2769 2770 public boolean hasSignature() { 2771 return this.signature != null && !this.signature.isEmpty(); 2772 } 2773 2774 /** 2775 * @param value {@link #signature} (Digital Signature - base64 encoded. XML-DSIg or a JWT.) 2776 */ 2777 public Bundle setSignature(Signature value) { 2778 this.signature = value; 2779 return this; 2780 } 2781 2782 /** 2783 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 2784 * If no link is found which matches the given relation, returns <code>null</code>. If more than one 2785 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 2786 * 2787 * @param theRelation 2788 * The relation, such as "next", or "self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 2789 * @return Returns a matching BundleLinkComponent, or <code>null</code> 2790 * @see IBaseBundle#LINK_NEXT 2791 * @see IBaseBundle#LINK_PREV 2792 * @see IBaseBundle#LINK_SELF 2793 */ 2794 public BundleLinkComponent getLink(String theRelation) { 2795 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 2796 for (BundleLinkComponent next : getLink()) { 2797 if (theRelation.equals(next.getRelation())) { 2798 return next; 2799 } 2800 } 2801 return null; 2802 } 2803 2804 /** 2805 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 2806 * If no link is found which matches the given relation, creates a new BundleLinkComponent with the 2807 * given relation and adds it to this Bundle. If more than one 2808 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 2809 * 2810 * @param theRelation 2811 * The relation, such as "next", or "self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 2812 * @return Returns a matching BundleLinkComponent, or <code>null</code> 2813 * @see IBaseBundle#LINK_NEXT 2814 * @see IBaseBundle#LINK_PREV 2815 * @see IBaseBundle#LINK_SELF 2816 */ 2817 public BundleLinkComponent getLinkOrCreate(String theRelation) { 2818 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 2819 for (BundleLinkComponent next : getLink()) { 2820 if (theRelation.equals(next.getRelation())) { 2821 return next; 2822 } 2823 } 2824 BundleLinkComponent retVal = new BundleLinkComponent(); 2825 retVal.setRelation(theRelation); 2826 getLink().add(retVal); 2827 return retVal; 2828 } 2829 protected void listChildren(List<Property> children) { 2830 super.listChildren(children); 2831 children.add(new Property("identifier", "Identifier", "A persistent identifier for the batch that won't change as a batch is copied from server to server.", 0, 1, identifier)); 2832 children.add(new Property("type", "code", "Indicates the purpose of this bundle - how it was intended to be used.", 0, 1, type)); 2833 children.add(new Property("total", "unsignedInt", "If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle).", 0, 1, total)); 2834 children.add(new Property("link", "", "A series of links that provide context to this bundle.", 0, java.lang.Integer.MAX_VALUE, link)); 2835 children.add(new Property("entry", "", "An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only).", 0, java.lang.Integer.MAX_VALUE, entry)); 2836 children.add(new Property("signature", "Signature", "Digital Signature - base64 encoded. XML-DSIg or a JWT.", 0, 1, signature)); 2837 } 2838 2839 @Override 2840 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2841 switch (_hash) { 2842 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A persistent identifier for the batch that won't change as a batch is copied from server to server.", 0, 1, identifier); 2843 case 3575610: /*type*/ return new Property("type", "code", "Indicates the purpose of this bundle - how it was intended to be used.", 0, 1, type); 2844 case 110549828: /*total*/ return new Property("total", "unsignedInt", "If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle).", 0, 1, total); 2845 case 3321850: /*link*/ return new Property("link", "", "A series of links that provide context to this bundle.", 0, java.lang.Integer.MAX_VALUE, link); 2846 case 96667762: /*entry*/ return new Property("entry", "", "An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only).", 0, java.lang.Integer.MAX_VALUE, entry); 2847 case 1073584312: /*signature*/ return new Property("signature", "Signature", "Digital Signature - base64 encoded. XML-DSIg or a JWT.", 0, 1, signature); 2848 default: return super.getNamedProperty(_hash, _name, _checkValid); 2849 } 2850 2851 } 2852 2853 @Override 2854 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2855 switch (hash) { 2856 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2857 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<BundleType> 2858 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // UnsignedIntType 2859 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // BundleLinkComponent 2860 case 96667762: /*entry*/ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // BundleEntryComponent 2861 case 1073584312: /*signature*/ return this.signature == null ? new Base[0] : new Base[] {this.signature}; // Signature 2862 default: return super.getProperty(hash, name, checkValid); 2863 } 2864 2865 } 2866 2867 @Override 2868 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2869 switch (hash) { 2870 case -1618432855: // identifier 2871 this.identifier = castToIdentifier(value); // Identifier 2872 return value; 2873 case 3575610: // type 2874 value = new BundleTypeEnumFactory().fromType(castToCode(value)); 2875 this.type = (Enumeration) value; // Enumeration<BundleType> 2876 return value; 2877 case 110549828: // total 2878 this.total = castToUnsignedInt(value); // UnsignedIntType 2879 return value; 2880 case 3321850: // link 2881 this.getLink().add((BundleLinkComponent) value); // BundleLinkComponent 2882 return value; 2883 case 96667762: // entry 2884 this.getEntry().add((BundleEntryComponent) value); // BundleEntryComponent 2885 return value; 2886 case 1073584312: // signature 2887 this.signature = castToSignature(value); // Signature 2888 return value; 2889 default: return super.setProperty(hash, name, value); 2890 } 2891 2892 } 2893 2894 @Override 2895 public Base setProperty(String name, Base value) throws FHIRException { 2896 if (name.equals("identifier")) { 2897 this.identifier = castToIdentifier(value); // Identifier 2898 } else if (name.equals("type")) { 2899 value = new BundleTypeEnumFactory().fromType(castToCode(value)); 2900 this.type = (Enumeration) value; // Enumeration<BundleType> 2901 } else if (name.equals("total")) { 2902 this.total = castToUnsignedInt(value); // UnsignedIntType 2903 } else if (name.equals("link")) { 2904 this.getLink().add((BundleLinkComponent) value); 2905 } else if (name.equals("entry")) { 2906 this.getEntry().add((BundleEntryComponent) value); 2907 } else if (name.equals("signature")) { 2908 this.signature = castToSignature(value); // Signature 2909 } else 2910 return super.setProperty(name, value); 2911 return value; 2912 } 2913 2914 @Override 2915 public Base makeProperty(int hash, String name) throws FHIRException { 2916 switch (hash) { 2917 case -1618432855: return getIdentifier(); 2918 case 3575610: return getTypeElement(); 2919 case 110549828: return getTotalElement(); 2920 case 3321850: return addLink(); 2921 case 96667762: return addEntry(); 2922 case 1073584312: return getSignature(); 2923 default: return super.makeProperty(hash, name); 2924 } 2925 2926 } 2927 2928 @Override 2929 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2930 switch (hash) { 2931 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2932 case 3575610: /*type*/ return new String[] {"code"}; 2933 case 110549828: /*total*/ return new String[] {"unsignedInt"}; 2934 case 3321850: /*link*/ return new String[] {}; 2935 case 96667762: /*entry*/ return new String[] {}; 2936 case 1073584312: /*signature*/ return new String[] {"Signature"}; 2937 default: return super.getTypesForProperty(hash, name); 2938 } 2939 2940 } 2941 2942 @Override 2943 public Base addChild(String name) throws FHIRException { 2944 if (name.equals("identifier")) { 2945 this.identifier = new Identifier(); 2946 return this.identifier; 2947 } 2948 else if (name.equals("type")) { 2949 throw new FHIRException("Cannot call addChild on a singleton property Bundle.type"); 2950 } 2951 else if (name.equals("total")) { 2952 throw new FHIRException("Cannot call addChild on a singleton property Bundle.total"); 2953 } 2954 else if (name.equals("link")) { 2955 return addLink(); 2956 } 2957 else if (name.equals("entry")) { 2958 return addEntry(); 2959 } 2960 else if (name.equals("signature")) { 2961 this.signature = new Signature(); 2962 return this.signature; 2963 } 2964 else 2965 return super.addChild(name); 2966 } 2967 2968 public String fhirType() { 2969 return "Bundle"; 2970 2971 } 2972 2973 public Bundle copy() { 2974 Bundle dst = new Bundle(); 2975 copyValues(dst); 2976 dst.identifier = identifier == null ? null : identifier.copy(); 2977 dst.type = type == null ? null : type.copy(); 2978 dst.total = total == null ? null : total.copy(); 2979 if (link != null) { 2980 dst.link = new ArrayList<BundleLinkComponent>(); 2981 for (BundleLinkComponent i : link) 2982 dst.link.add(i.copy()); 2983 }; 2984 if (entry != null) { 2985 dst.entry = new ArrayList<BundleEntryComponent>(); 2986 for (BundleEntryComponent i : entry) 2987 dst.entry.add(i.copy()); 2988 }; 2989 dst.signature = signature == null ? null : signature.copy(); 2990 return dst; 2991 } 2992 2993 protected Bundle typedCopy() { 2994 return copy(); 2995 } 2996 2997 @Override 2998 public boolean equalsDeep(Base other_) { 2999 if (!super.equalsDeep(other_)) 3000 return false; 3001 if (!(other_ instanceof Bundle)) 3002 return false; 3003 Bundle o = (Bundle) other_; 3004 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(total, o.total, true) 3005 && compareDeep(link, o.link, true) && compareDeep(entry, o.entry, true) && compareDeep(signature, o.signature, true) 3006 ; 3007 } 3008 3009 @Override 3010 public boolean equalsShallow(Base other_) { 3011 if (!super.equalsShallow(other_)) 3012 return false; 3013 if (!(other_ instanceof Bundle)) 3014 return false; 3015 Bundle o = (Bundle) other_; 3016 return compareValues(type, o.type, true) && compareValues(total, o.total, true); 3017 } 3018 3019 public boolean isEmpty() { 3020 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, total 3021 , link, entry, signature); 3022 } 3023 3024 @Override 3025 public ResourceType getResourceType() { 3026 return ResourceType.Bundle; 3027 } 3028 3029 /** 3030 * Search parameter: <b>identifier</b> 3031 * <p> 3032 * Description: <b>Persistent identifier for the bundle</b><br> 3033 * Type: <b>token</b><br> 3034 * Path: <b>Bundle.identifier</b><br> 3035 * </p> 3036 */ 3037 @SearchParamDefinition(name="identifier", path="Bundle.identifier", description="Persistent identifier for the bundle", type="token" ) 3038 public static final String SP_IDENTIFIER = "identifier"; 3039 /** 3040 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3041 * <p> 3042 * Description: <b>Persistent identifier for the bundle</b><br> 3043 * Type: <b>token</b><br> 3044 * Path: <b>Bundle.identifier</b><br> 3045 * </p> 3046 */ 3047 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3048 3049 /** 3050 * Search parameter: <b>composition</b> 3051 * <p> 3052 * Description: <b>The first resource in the bundle, if the bundle type is "document" - this is a composition, and this parameter provides access to searches its contents</b><br> 3053 * Type: <b>reference</b><br> 3054 * Path: <b>Bundle.entry(0).resource</b><br> 3055 * </p> 3056 */ 3057 @SearchParamDefinition(name="composition", path="Bundle.entry[0].resource", description="The first resource in the bundle, if the bundle type is \"document\" - this is a composition, and this parameter provides access to searches its contents", type="reference", target={Composition.class } ) 3058 public static final String SP_COMPOSITION = "composition"; 3059 /** 3060 * <b>Fluent Client</b> search parameter constant for <b>composition</b> 3061 * <p> 3062 * Description: <b>The first resource in the bundle, if the bundle type is "document" - this is a composition, and this parameter provides access to searches its contents</b><br> 3063 * Type: <b>reference</b><br> 3064 * Path: <b>Bundle.entry(0).resource</b><br> 3065 * </p> 3066 */ 3067 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSITION); 3068 3069/** 3070 * Constant for fluent queries to be used to add include statements. Specifies 3071 * the path value of "<b>Bundle:composition</b>". 3072 */ 3073 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSITION = new ca.uhn.fhir.model.api.Include("Bundle:composition").toLocked(); 3074 3075 /** 3076 * Search parameter: <b>type</b> 3077 * <p> 3078 * Description: <b>document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection</b><br> 3079 * Type: <b>token</b><br> 3080 * Path: <b>Bundle.type</b><br> 3081 * </p> 3082 */ 3083 @SearchParamDefinition(name="type", path="Bundle.type", description="document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection", type="token" ) 3084 public static final String SP_TYPE = "type"; 3085 /** 3086 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3087 * <p> 3088 * Description: <b>document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection</b><br> 3089 * Type: <b>token</b><br> 3090 * Path: <b>Bundle.type</b><br> 3091 * </p> 3092 */ 3093 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3094 3095 /** 3096 * Search parameter: <b>message</b> 3097 * <p> 3098 * Description: <b>The first resource in the bundle, if the bundle type is "message" - this is a message header, and this parameter provides access to search its contents</b><br> 3099 * Type: <b>reference</b><br> 3100 * Path: <b>Bundle.entry(0).resource</b><br> 3101 * </p> 3102 */ 3103 @SearchParamDefinition(name="message", path="Bundle.entry[0].resource", description="The first resource in the bundle, if the bundle type is \"message\" - this is a message header, and this parameter provides access to search its contents", type="reference", target={MessageHeader.class } ) 3104 public static final String SP_MESSAGE = "message"; 3105 /** 3106 * <b>Fluent Client</b> search parameter constant for <b>message</b> 3107 * <p> 3108 * Description: <b>The first resource in the bundle, if the bundle type is "message" - this is a message header, and this parameter provides access to search its contents</b><br> 3109 * Type: <b>reference</b><br> 3110 * Path: <b>Bundle.entry(0).resource</b><br> 3111 * </p> 3112 */ 3113 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MESSAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MESSAGE); 3114 3115/** 3116 * Constant for fluent queries to be used to add include statements. Specifies 3117 * the path value of "<b>Bundle:message</b>". 3118 */ 3119 public static final ca.uhn.fhir.model.api.Include INCLUDE_MESSAGE = new ca.uhn.fhir.model.api.Include("Bundle:message").toLocked(); 3120 3121 3122}