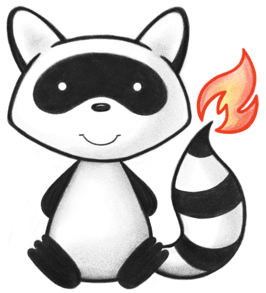
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 041import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 042import org.hl7.fhir.dstu3.model.Enumerations.SearchParamType; 043import org.hl7.fhir.dstu3.model.Enumerations.SearchParamTypeEnumFactory; 044import org.hl7.fhir.exceptions.FHIRException; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import org.hl7.fhir.instance.model.api.IBaseConformance; 047import org.hl7.fhir.utilities.Utilities; 048 049import ca.uhn.fhir.model.api.annotation.Block; 050import ca.uhn.fhir.model.api.annotation.Child; 051import ca.uhn.fhir.model.api.annotation.ChildOrder; 052import ca.uhn.fhir.model.api.annotation.Description; 053import ca.uhn.fhir.model.api.annotation.ResourceDef; 054import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 055/** 056 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 057 */ 058@ResourceDef(name="CapabilityStatement", profile="http://hl7.org/fhir/Profile/CapabilityStatement") 059@ChildOrder(names={"url", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "kind", "instantiates", "software", "implementation", "fhirVersion", "acceptUnknown", "format", "patchFormat", "implementationGuide", "profile", "rest", "messaging", "document"}) 060public class CapabilityStatement extends MetadataResource implements IBaseConformance { 061 062 public enum CapabilityStatementKind { 063 /** 064 * The CapabilityStatement instance represents the present capabilities of a specific system instance. This is the kind returned by OPTIONS for a FHIR server end-point. 065 */ 066 INSTANCE, 067 /** 068 * The CapabilityStatement instance represents the capabilities of a system or piece of software, independent of a particular installation. 069 */ 070 CAPABILITY, 071 /** 072 * The CapabilityStatement instance represents a set of requirements for other systems to meet; e.g. as part of an implementation guide or 'request for proposal'. 073 */ 074 REQUIREMENTS, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static CapabilityStatementKind fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("instance".equals(codeString)) 083 return INSTANCE; 084 if ("capability".equals(codeString)) 085 return CAPABILITY; 086 if ("requirements".equals(codeString)) 087 return REQUIREMENTS; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown CapabilityStatementKind code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case INSTANCE: return "instance"; 096 case CAPABILITY: return "capability"; 097 case REQUIREMENTS: return "requirements"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case INSTANCE: return "http://hl7.org/fhir/capability-statement-kind"; 105 case CAPABILITY: return "http://hl7.org/fhir/capability-statement-kind"; 106 case REQUIREMENTS: return "http://hl7.org/fhir/capability-statement-kind"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case INSTANCE: return "The CapabilityStatement instance represents the present capabilities of a specific system instance. This is the kind returned by OPTIONS for a FHIR server end-point."; 114 case CAPABILITY: return "The CapabilityStatement instance represents the capabilities of a system or piece of software, independent of a particular installation."; 115 case REQUIREMENTS: return "The CapabilityStatement instance represents a set of requirements for other systems to meet; e.g. as part of an implementation guide or 'request for proposal'."; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDisplay() { 121 switch (this) { 122 case INSTANCE: return "Instance"; 123 case CAPABILITY: return "Capability"; 124 case REQUIREMENTS: return "Requirements"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 } 130 131 public static class CapabilityStatementKindEnumFactory implements EnumFactory<CapabilityStatementKind> { 132 public CapabilityStatementKind fromCode(String codeString) throws IllegalArgumentException { 133 if (codeString == null || "".equals(codeString)) 134 if (codeString == null || "".equals(codeString)) 135 return null; 136 if ("instance".equals(codeString)) 137 return CapabilityStatementKind.INSTANCE; 138 if ("capability".equals(codeString)) 139 return CapabilityStatementKind.CAPABILITY; 140 if ("requirements".equals(codeString)) 141 return CapabilityStatementKind.REQUIREMENTS; 142 throw new IllegalArgumentException("Unknown CapabilityStatementKind code '"+codeString+"'"); 143 } 144 public Enumeration<CapabilityStatementKind> fromType(PrimitiveType<?> code) throws FHIRException { 145 if (code == null) 146 return null; 147 if (code.isEmpty()) 148 return new Enumeration<CapabilityStatementKind>(this); 149 String codeString = code.asStringValue(); 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("instance".equals(codeString)) 153 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.INSTANCE); 154 if ("capability".equals(codeString)) 155 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.CAPABILITY); 156 if ("requirements".equals(codeString)) 157 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.REQUIREMENTS); 158 throw new FHIRException("Unknown CapabilityStatementKind code '"+codeString+"'"); 159 } 160 public String toCode(CapabilityStatementKind code) { 161 if (code == CapabilityStatementKind.NULL) 162 return null; 163 if (code == CapabilityStatementKind.INSTANCE) 164 return "instance"; 165 if (code == CapabilityStatementKind.CAPABILITY) 166 return "capability"; 167 if (code == CapabilityStatementKind.REQUIREMENTS) 168 return "requirements"; 169 return "?"; 170 } 171 public String toSystem(CapabilityStatementKind code) { 172 return code.getSystem(); 173 } 174 } 175 176 public enum UnknownContentCode { 177 /** 178 * The application does not accept either unknown elements or extensions. 179 */ 180 NO, 181 /** 182 * The application accepts unknown extensions, but not unknown elements. 183 */ 184 EXTENSIONS, 185 /** 186 * The application accepts unknown elements, but not unknown extensions. 187 */ 188 ELEMENTS, 189 /** 190 * The application accepts unknown elements and extensions. 191 */ 192 BOTH, 193 /** 194 * added to help the parsers with the generic types 195 */ 196 NULL; 197 public static UnknownContentCode fromCode(String codeString) throws FHIRException { 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("no".equals(codeString)) 201 return NO; 202 if ("extensions".equals(codeString)) 203 return EXTENSIONS; 204 if ("elements".equals(codeString)) 205 return ELEMENTS; 206 if ("both".equals(codeString)) 207 return BOTH; 208 if (Configuration.isAcceptInvalidEnums()) 209 return null; 210 else 211 throw new FHIRException("Unknown UnknownContentCode code '"+codeString+"'"); 212 } 213 public String toCode() { 214 switch (this) { 215 case NO: return "no"; 216 case EXTENSIONS: return "extensions"; 217 case ELEMENTS: return "elements"; 218 case BOTH: return "both"; 219 case NULL: return null; 220 default: return "?"; 221 } 222 } 223 public String getSystem() { 224 switch (this) { 225 case NO: return "http://hl7.org/fhir/unknown-content-code"; 226 case EXTENSIONS: return "http://hl7.org/fhir/unknown-content-code"; 227 case ELEMENTS: return "http://hl7.org/fhir/unknown-content-code"; 228 case BOTH: return "http://hl7.org/fhir/unknown-content-code"; 229 case NULL: return null; 230 default: return "?"; 231 } 232 } 233 public String getDefinition() { 234 switch (this) { 235 case NO: return "The application does not accept either unknown elements or extensions."; 236 case EXTENSIONS: return "The application accepts unknown extensions, but not unknown elements."; 237 case ELEMENTS: return "The application accepts unknown elements, but not unknown extensions."; 238 case BOTH: return "The application accepts unknown elements and extensions."; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 public String getDisplay() { 244 switch (this) { 245 case NO: return "Neither Elements or Extensions"; 246 case EXTENSIONS: return "Unknown Extensions"; 247 case ELEMENTS: return "Unknown Elements"; 248 case BOTH: return "Unknown Elements and Extensions"; 249 case NULL: return null; 250 default: return "?"; 251 } 252 } 253 } 254 255 public static class UnknownContentCodeEnumFactory implements EnumFactory<UnknownContentCode> { 256 public UnknownContentCode fromCode(String codeString) throws IllegalArgumentException { 257 if (codeString == null || "".equals(codeString)) 258 if (codeString == null || "".equals(codeString)) 259 return null; 260 if ("no".equals(codeString)) 261 return UnknownContentCode.NO; 262 if ("extensions".equals(codeString)) 263 return UnknownContentCode.EXTENSIONS; 264 if ("elements".equals(codeString)) 265 return UnknownContentCode.ELEMENTS; 266 if ("both".equals(codeString)) 267 return UnknownContentCode.BOTH; 268 throw new IllegalArgumentException("Unknown UnknownContentCode code '"+codeString+"'"); 269 } 270 public Enumeration<UnknownContentCode> fromType(PrimitiveType<?> code) throws FHIRException { 271 if (code == null) 272 return null; 273 if (code.isEmpty()) 274 return new Enumeration<UnknownContentCode>(this); 275 String codeString = code.asStringValue(); 276 if (codeString == null || "".equals(codeString)) 277 return null; 278 if ("no".equals(codeString)) 279 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.NO); 280 if ("extensions".equals(codeString)) 281 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.EXTENSIONS); 282 if ("elements".equals(codeString)) 283 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.ELEMENTS); 284 if ("both".equals(codeString)) 285 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.BOTH); 286 throw new FHIRException("Unknown UnknownContentCode code '"+codeString+"'"); 287 } 288 public String toCode(UnknownContentCode code) { 289 if (code == UnknownContentCode.NULL) 290 return null; 291 if (code == UnknownContentCode.NO) 292 return "no"; 293 if (code == UnknownContentCode.EXTENSIONS) 294 return "extensions"; 295 if (code == UnknownContentCode.ELEMENTS) 296 return "elements"; 297 if (code == UnknownContentCode.BOTH) 298 return "both"; 299 return "?"; 300 } 301 public String toSystem(UnknownContentCode code) { 302 return code.getSystem(); 303 } 304 } 305 306 public enum RestfulCapabilityMode { 307 /** 308 * The application acts as a client for this resource. 309 */ 310 CLIENT, 311 /** 312 * The application acts as a server for this resource. 313 */ 314 SERVER, 315 /** 316 * added to help the parsers with the generic types 317 */ 318 NULL; 319 public static RestfulCapabilityMode fromCode(String codeString) throws FHIRException { 320 if (codeString == null || "".equals(codeString)) 321 return null; 322 if ("client".equals(codeString)) 323 return CLIENT; 324 if ("server".equals(codeString)) 325 return SERVER; 326 if (Configuration.isAcceptInvalidEnums()) 327 return null; 328 else 329 throw new FHIRException("Unknown RestfulCapabilityMode code '"+codeString+"'"); 330 } 331 public String toCode() { 332 switch (this) { 333 case CLIENT: return "client"; 334 case SERVER: return "server"; 335 case NULL: return null; 336 default: return "?"; 337 } 338 } 339 public String getSystem() { 340 switch (this) { 341 case CLIENT: return "http://hl7.org/fhir/restful-capability-mode"; 342 case SERVER: return "http://hl7.org/fhir/restful-capability-mode"; 343 case NULL: return null; 344 default: return "?"; 345 } 346 } 347 public String getDefinition() { 348 switch (this) { 349 case CLIENT: return "The application acts as a client for this resource."; 350 case SERVER: return "The application acts as a server for this resource."; 351 case NULL: return null; 352 default: return "?"; 353 } 354 } 355 public String getDisplay() { 356 switch (this) { 357 case CLIENT: return "Client"; 358 case SERVER: return "Server"; 359 case NULL: return null; 360 default: return "?"; 361 } 362 } 363 } 364 365 public static class RestfulCapabilityModeEnumFactory implements EnumFactory<RestfulCapabilityMode> { 366 public RestfulCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 367 if (codeString == null || "".equals(codeString)) 368 if (codeString == null || "".equals(codeString)) 369 return null; 370 if ("client".equals(codeString)) 371 return RestfulCapabilityMode.CLIENT; 372 if ("server".equals(codeString)) 373 return RestfulCapabilityMode.SERVER; 374 throw new IllegalArgumentException("Unknown RestfulCapabilityMode code '"+codeString+"'"); 375 } 376 public Enumeration<RestfulCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 377 if (code == null) 378 return null; 379 if (code.isEmpty()) 380 return new Enumeration<RestfulCapabilityMode>(this); 381 String codeString = code.asStringValue(); 382 if (codeString == null || "".equals(codeString)) 383 return null; 384 if ("client".equals(codeString)) 385 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.CLIENT); 386 if ("server".equals(codeString)) 387 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.SERVER); 388 throw new FHIRException("Unknown RestfulCapabilityMode code '"+codeString+"'"); 389 } 390 public String toCode(RestfulCapabilityMode code) { 391 if (code == RestfulCapabilityMode.NULL) 392 return null; 393 if (code == RestfulCapabilityMode.CLIENT) 394 return "client"; 395 if (code == RestfulCapabilityMode.SERVER) 396 return "server"; 397 return "?"; 398 } 399 public String toSystem(RestfulCapabilityMode code) { 400 return code.getSystem(); 401 } 402 } 403 404 public enum TypeRestfulInteraction { 405 /** 406 * null 407 */ 408 READ, 409 /** 410 * null 411 */ 412 VREAD, 413 /** 414 * null 415 */ 416 UPDATE, 417 /** 418 * null 419 */ 420 PATCH, 421 /** 422 * null 423 */ 424 DELETE, 425 /** 426 * null 427 */ 428 HISTORYINSTANCE, 429 /** 430 * null 431 */ 432 HISTORYTYPE, 433 /** 434 * null 435 */ 436 CREATE, 437 /** 438 * null 439 */ 440 SEARCHTYPE, 441 /** 442 * added to help the parsers with the generic types 443 */ 444 NULL; 445 public static TypeRestfulInteraction fromCode(String codeString) throws FHIRException { 446 if (codeString == null || "".equals(codeString)) 447 return null; 448 if ("read".equals(codeString)) 449 return READ; 450 if ("vread".equals(codeString)) 451 return VREAD; 452 if ("update".equals(codeString)) 453 return UPDATE; 454 if ("patch".equals(codeString)) 455 return PATCH; 456 if ("delete".equals(codeString)) 457 return DELETE; 458 if ("history-instance".equals(codeString)) 459 return HISTORYINSTANCE; 460 if ("history-type".equals(codeString)) 461 return HISTORYTYPE; 462 if ("create".equals(codeString)) 463 return CREATE; 464 if ("search-type".equals(codeString)) 465 return SEARCHTYPE; 466 if (Configuration.isAcceptInvalidEnums()) 467 return null; 468 else 469 throw new FHIRException("Unknown TypeRestfulInteraction code '"+codeString+"'"); 470 } 471 public String toCode() { 472 switch (this) { 473 case READ: return "read"; 474 case VREAD: return "vread"; 475 case UPDATE: return "update"; 476 case PATCH: return "patch"; 477 case DELETE: return "delete"; 478 case HISTORYINSTANCE: return "history-instance"; 479 case HISTORYTYPE: return "history-type"; 480 case CREATE: return "create"; 481 case SEARCHTYPE: return "search-type"; 482 case NULL: return null; 483 default: return "?"; 484 } 485 } 486 public String getSystem() { 487 switch (this) { 488 case READ: return "http://hl7.org/fhir/restful-interaction"; 489 case VREAD: return "http://hl7.org/fhir/restful-interaction"; 490 case UPDATE: return "http://hl7.org/fhir/restful-interaction"; 491 case PATCH: return "http://hl7.org/fhir/restful-interaction"; 492 case DELETE: return "http://hl7.org/fhir/restful-interaction"; 493 case HISTORYINSTANCE: return "http://hl7.org/fhir/restful-interaction"; 494 case HISTORYTYPE: return "http://hl7.org/fhir/restful-interaction"; 495 case CREATE: return "http://hl7.org/fhir/restful-interaction"; 496 case SEARCHTYPE: return "http://hl7.org/fhir/restful-interaction"; 497 case NULL: return null; 498 default: return "?"; 499 } 500 } 501 public String getDefinition() { 502 switch (this) { 503 case READ: return ""; 504 case VREAD: return ""; 505 case UPDATE: return ""; 506 case PATCH: return ""; 507 case DELETE: return ""; 508 case HISTORYINSTANCE: return ""; 509 case HISTORYTYPE: return ""; 510 case CREATE: return ""; 511 case SEARCHTYPE: return ""; 512 case NULL: return null; 513 default: return "?"; 514 } 515 } 516 public String getDisplay() { 517 switch (this) { 518 case READ: return "read"; 519 case VREAD: return "vread"; 520 case UPDATE: return "update"; 521 case PATCH: return "patch"; 522 case DELETE: return "delete"; 523 case HISTORYINSTANCE: return "history-instance"; 524 case HISTORYTYPE: return "history-type"; 525 case CREATE: return "create"; 526 case SEARCHTYPE: return "search-type"; 527 case NULL: return null; 528 default: return "?"; 529 } 530 } 531 } 532 533 public static class TypeRestfulInteractionEnumFactory implements EnumFactory<TypeRestfulInteraction> { 534 public TypeRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 535 if (codeString == null || "".equals(codeString)) 536 if (codeString == null || "".equals(codeString)) 537 return null; 538 if ("read".equals(codeString)) 539 return TypeRestfulInteraction.READ; 540 if ("vread".equals(codeString)) 541 return TypeRestfulInteraction.VREAD; 542 if ("update".equals(codeString)) 543 return TypeRestfulInteraction.UPDATE; 544 if ("patch".equals(codeString)) 545 return TypeRestfulInteraction.PATCH; 546 if ("delete".equals(codeString)) 547 return TypeRestfulInteraction.DELETE; 548 if ("history-instance".equals(codeString)) 549 return TypeRestfulInteraction.HISTORYINSTANCE; 550 if ("history-type".equals(codeString)) 551 return TypeRestfulInteraction.HISTORYTYPE; 552 if ("create".equals(codeString)) 553 return TypeRestfulInteraction.CREATE; 554 if ("search-type".equals(codeString)) 555 return TypeRestfulInteraction.SEARCHTYPE; 556 throw new IllegalArgumentException("Unknown TypeRestfulInteraction code '"+codeString+"'"); 557 } 558 public Enumeration<TypeRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 559 if (code == null) 560 return null; 561 if (code.isEmpty()) 562 return new Enumeration<TypeRestfulInteraction>(this); 563 String codeString = code.asStringValue(); 564 if (codeString == null || "".equals(codeString)) 565 return null; 566 if ("read".equals(codeString)) 567 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.READ); 568 if ("vread".equals(codeString)) 569 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VREAD); 570 if ("update".equals(codeString)) 571 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.UPDATE); 572 if ("patch".equals(codeString)) 573 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.PATCH); 574 if ("delete".equals(codeString)) 575 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.DELETE); 576 if ("history-instance".equals(codeString)) 577 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYINSTANCE); 578 if ("history-type".equals(codeString)) 579 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYTYPE); 580 if ("create".equals(codeString)) 581 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.CREATE); 582 if ("search-type".equals(codeString)) 583 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.SEARCHTYPE); 584 throw new FHIRException("Unknown TypeRestfulInteraction code '"+codeString+"'"); 585 } 586 public String toCode(TypeRestfulInteraction code) { 587 if (code == TypeRestfulInteraction.NULL) 588 return null; 589 if (code == TypeRestfulInteraction.READ) 590 return "read"; 591 if (code == TypeRestfulInteraction.VREAD) 592 return "vread"; 593 if (code == TypeRestfulInteraction.UPDATE) 594 return "update"; 595 if (code == TypeRestfulInteraction.PATCH) 596 return "patch"; 597 if (code == TypeRestfulInteraction.DELETE) 598 return "delete"; 599 if (code == TypeRestfulInteraction.HISTORYINSTANCE) 600 return "history-instance"; 601 if (code == TypeRestfulInteraction.HISTORYTYPE) 602 return "history-type"; 603 if (code == TypeRestfulInteraction.CREATE) 604 return "create"; 605 if (code == TypeRestfulInteraction.SEARCHTYPE) 606 return "search-type"; 607 return "?"; 608 } 609 public String toSystem(TypeRestfulInteraction code) { 610 return code.getSystem(); 611 } 612 } 613 614 public enum ResourceVersionPolicy { 615 /** 616 * VersionId meta-property is not supported (server) or used (client). 617 */ 618 NOVERSION, 619 /** 620 * VersionId meta-property is supported (server) or used (client). 621 */ 622 VERSIONED, 623 /** 624 * VersionId must be correct for updates (server) or will be specified (If-match header) for updates (client). 625 */ 626 VERSIONEDUPDATE, 627 /** 628 * added to help the parsers with the generic types 629 */ 630 NULL; 631 public static ResourceVersionPolicy fromCode(String codeString) throws FHIRException { 632 if (codeString == null || "".equals(codeString)) 633 return null; 634 if ("no-version".equals(codeString)) 635 return NOVERSION; 636 if ("versioned".equals(codeString)) 637 return VERSIONED; 638 if ("versioned-update".equals(codeString)) 639 return VERSIONEDUPDATE; 640 if (Configuration.isAcceptInvalidEnums()) 641 return null; 642 else 643 throw new FHIRException("Unknown ResourceVersionPolicy code '"+codeString+"'"); 644 } 645 public String toCode() { 646 switch (this) { 647 case NOVERSION: return "no-version"; 648 case VERSIONED: return "versioned"; 649 case VERSIONEDUPDATE: return "versioned-update"; 650 case NULL: return null; 651 default: return "?"; 652 } 653 } 654 public String getSystem() { 655 switch (this) { 656 case NOVERSION: return "http://hl7.org/fhir/versioning-policy"; 657 case VERSIONED: return "http://hl7.org/fhir/versioning-policy"; 658 case VERSIONEDUPDATE: return "http://hl7.org/fhir/versioning-policy"; 659 case NULL: return null; 660 default: return "?"; 661 } 662 } 663 public String getDefinition() { 664 switch (this) { 665 case NOVERSION: return "VersionId meta-property is not supported (server) or used (client)."; 666 case VERSIONED: return "VersionId meta-property is supported (server) or used (client)."; 667 case VERSIONEDUPDATE: return "VersionId must be correct for updates (server) or will be specified (If-match header) for updates (client)."; 668 case NULL: return null; 669 default: return "?"; 670 } 671 } 672 public String getDisplay() { 673 switch (this) { 674 case NOVERSION: return "No VersionId Support"; 675 case VERSIONED: return "Versioned"; 676 case VERSIONEDUPDATE: return "VersionId tracked fully"; 677 case NULL: return null; 678 default: return "?"; 679 } 680 } 681 } 682 683 public static class ResourceVersionPolicyEnumFactory implements EnumFactory<ResourceVersionPolicy> { 684 public ResourceVersionPolicy fromCode(String codeString) throws IllegalArgumentException { 685 if (codeString == null || "".equals(codeString)) 686 if (codeString == null || "".equals(codeString)) 687 return null; 688 if ("no-version".equals(codeString)) 689 return ResourceVersionPolicy.NOVERSION; 690 if ("versioned".equals(codeString)) 691 return ResourceVersionPolicy.VERSIONED; 692 if ("versioned-update".equals(codeString)) 693 return ResourceVersionPolicy.VERSIONEDUPDATE; 694 throw new IllegalArgumentException("Unknown ResourceVersionPolicy code '"+codeString+"'"); 695 } 696 public Enumeration<ResourceVersionPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 697 if (code == null) 698 return null; 699 if (code.isEmpty()) 700 return new Enumeration<ResourceVersionPolicy>(this); 701 String codeString = code.asStringValue(); 702 if (codeString == null || "".equals(codeString)) 703 return null; 704 if ("no-version".equals(codeString)) 705 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NOVERSION); 706 if ("versioned".equals(codeString)) 707 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONED); 708 if ("versioned-update".equals(codeString)) 709 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONEDUPDATE); 710 throw new FHIRException("Unknown ResourceVersionPolicy code '"+codeString+"'"); 711 } 712 public String toCode(ResourceVersionPolicy code) { 713 if (code == ResourceVersionPolicy.NULL) 714 return null; 715 if (code == ResourceVersionPolicy.NOVERSION) 716 return "no-version"; 717 if (code == ResourceVersionPolicy.VERSIONED) 718 return "versioned"; 719 if (code == ResourceVersionPolicy.VERSIONEDUPDATE) 720 return "versioned-update"; 721 return "?"; 722 } 723 public String toSystem(ResourceVersionPolicy code) { 724 return code.getSystem(); 725 } 726 } 727 728 public enum ConditionalReadStatus { 729 /** 730 * No support for conditional deletes. 731 */ 732 NOTSUPPORTED, 733 /** 734 * Conditional reads are supported, but only with the If-Modified-Since HTTP Header. 735 */ 736 MODIFIEDSINCE, 737 /** 738 * Conditional reads are supported, but only with the If-None-Match HTTP Header. 739 */ 740 NOTMATCH, 741 /** 742 * Conditional reads are supported, with both If-Modified-Since and If-None-Match HTTP Headers. 743 */ 744 FULLSUPPORT, 745 /** 746 * added to help the parsers with the generic types 747 */ 748 NULL; 749 public static ConditionalReadStatus fromCode(String codeString) throws FHIRException { 750 if (codeString == null || "".equals(codeString)) 751 return null; 752 if ("not-supported".equals(codeString)) 753 return NOTSUPPORTED; 754 if ("modified-since".equals(codeString)) 755 return MODIFIEDSINCE; 756 if ("not-match".equals(codeString)) 757 return NOTMATCH; 758 if ("full-support".equals(codeString)) 759 return FULLSUPPORT; 760 if (Configuration.isAcceptInvalidEnums()) 761 return null; 762 else 763 throw new FHIRException("Unknown ConditionalReadStatus code '"+codeString+"'"); 764 } 765 public String toCode() { 766 switch (this) { 767 case NOTSUPPORTED: return "not-supported"; 768 case MODIFIEDSINCE: return "modified-since"; 769 case NOTMATCH: return "not-match"; 770 case FULLSUPPORT: return "full-support"; 771 case NULL: return null; 772 default: return "?"; 773 } 774 } 775 public String getSystem() { 776 switch (this) { 777 case NOTSUPPORTED: return "http://hl7.org/fhir/conditional-read-status"; 778 case MODIFIEDSINCE: return "http://hl7.org/fhir/conditional-read-status"; 779 case NOTMATCH: return "http://hl7.org/fhir/conditional-read-status"; 780 case FULLSUPPORT: return "http://hl7.org/fhir/conditional-read-status"; 781 case NULL: return null; 782 default: return "?"; 783 } 784 } 785 public String getDefinition() { 786 switch (this) { 787 case NOTSUPPORTED: return "No support for conditional deletes."; 788 case MODIFIEDSINCE: return "Conditional reads are supported, but only with the If-Modified-Since HTTP Header."; 789 case NOTMATCH: return "Conditional reads are supported, but only with the If-None-Match HTTP Header."; 790 case FULLSUPPORT: return "Conditional reads are supported, with both If-Modified-Since and If-None-Match HTTP Headers."; 791 case NULL: return null; 792 default: return "?"; 793 } 794 } 795 public String getDisplay() { 796 switch (this) { 797 case NOTSUPPORTED: return "Not Supported"; 798 case MODIFIEDSINCE: return "If-Modified-Since"; 799 case NOTMATCH: return "If-None-Match"; 800 case FULLSUPPORT: return "Full Support"; 801 case NULL: return null; 802 default: return "?"; 803 } 804 } 805 } 806 807 public static class ConditionalReadStatusEnumFactory implements EnumFactory<ConditionalReadStatus> { 808 public ConditionalReadStatus fromCode(String codeString) throws IllegalArgumentException { 809 if (codeString == null || "".equals(codeString)) 810 if (codeString == null || "".equals(codeString)) 811 return null; 812 if ("not-supported".equals(codeString)) 813 return ConditionalReadStatus.NOTSUPPORTED; 814 if ("modified-since".equals(codeString)) 815 return ConditionalReadStatus.MODIFIEDSINCE; 816 if ("not-match".equals(codeString)) 817 return ConditionalReadStatus.NOTMATCH; 818 if ("full-support".equals(codeString)) 819 return ConditionalReadStatus.FULLSUPPORT; 820 throw new IllegalArgumentException("Unknown ConditionalReadStatus code '"+codeString+"'"); 821 } 822 public Enumeration<ConditionalReadStatus> fromType(PrimitiveType<?> code) throws FHIRException { 823 if (code == null) 824 return null; 825 if (code.isEmpty()) 826 return new Enumeration<ConditionalReadStatus>(this); 827 String codeString = code.asStringValue(); 828 if (codeString == null || "".equals(codeString)) 829 return null; 830 if ("not-supported".equals(codeString)) 831 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTSUPPORTED); 832 if ("modified-since".equals(codeString)) 833 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.MODIFIEDSINCE); 834 if ("not-match".equals(codeString)) 835 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTMATCH); 836 if ("full-support".equals(codeString)) 837 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.FULLSUPPORT); 838 throw new FHIRException("Unknown ConditionalReadStatus code '"+codeString+"'"); 839 } 840 public String toCode(ConditionalReadStatus code) { 841 if (code == ConditionalReadStatus.NULL) 842 return null; 843 if (code == ConditionalReadStatus.NOTSUPPORTED) 844 return "not-supported"; 845 if (code == ConditionalReadStatus.MODIFIEDSINCE) 846 return "modified-since"; 847 if (code == ConditionalReadStatus.NOTMATCH) 848 return "not-match"; 849 if (code == ConditionalReadStatus.FULLSUPPORT) 850 return "full-support"; 851 return "?"; 852 } 853 public String toSystem(ConditionalReadStatus code) { 854 return code.getSystem(); 855 } 856 } 857 858 public enum ConditionalDeleteStatus { 859 /** 860 * No support for conditional deletes. 861 */ 862 NOTSUPPORTED, 863 /** 864 * Conditional deletes are supported, but only single resources at a time. 865 */ 866 SINGLE, 867 /** 868 * Conditional deletes are supported, and multiple resources can be deleted in a single interaction. 869 */ 870 MULTIPLE, 871 /** 872 * added to help the parsers with the generic types 873 */ 874 NULL; 875 public static ConditionalDeleteStatus fromCode(String codeString) throws FHIRException { 876 if (codeString == null || "".equals(codeString)) 877 return null; 878 if ("not-supported".equals(codeString)) 879 return NOTSUPPORTED; 880 if ("single".equals(codeString)) 881 return SINGLE; 882 if ("multiple".equals(codeString)) 883 return MULTIPLE; 884 if (Configuration.isAcceptInvalidEnums()) 885 return null; 886 else 887 throw new FHIRException("Unknown ConditionalDeleteStatus code '"+codeString+"'"); 888 } 889 public String toCode() { 890 switch (this) { 891 case NOTSUPPORTED: return "not-supported"; 892 case SINGLE: return "single"; 893 case MULTIPLE: return "multiple"; 894 case NULL: return null; 895 default: return "?"; 896 } 897 } 898 public String getSystem() { 899 switch (this) { 900 case NOTSUPPORTED: return "http://hl7.org/fhir/conditional-delete-status"; 901 case SINGLE: return "http://hl7.org/fhir/conditional-delete-status"; 902 case MULTIPLE: return "http://hl7.org/fhir/conditional-delete-status"; 903 case NULL: return null; 904 default: return "?"; 905 } 906 } 907 public String getDefinition() { 908 switch (this) { 909 case NOTSUPPORTED: return "No support for conditional deletes."; 910 case SINGLE: return "Conditional deletes are supported, but only single resources at a time."; 911 case MULTIPLE: return "Conditional deletes are supported, and multiple resources can be deleted in a single interaction."; 912 case NULL: return null; 913 default: return "?"; 914 } 915 } 916 public String getDisplay() { 917 switch (this) { 918 case NOTSUPPORTED: return "Not Supported"; 919 case SINGLE: return "Single Deletes Supported"; 920 case MULTIPLE: return "Multiple Deletes Supported"; 921 case NULL: return null; 922 default: return "?"; 923 } 924 } 925 } 926 927 public static class ConditionalDeleteStatusEnumFactory implements EnumFactory<ConditionalDeleteStatus> { 928 public ConditionalDeleteStatus fromCode(String codeString) throws IllegalArgumentException { 929 if (codeString == null || "".equals(codeString)) 930 if (codeString == null || "".equals(codeString)) 931 return null; 932 if ("not-supported".equals(codeString)) 933 return ConditionalDeleteStatus.NOTSUPPORTED; 934 if ("single".equals(codeString)) 935 return ConditionalDeleteStatus.SINGLE; 936 if ("multiple".equals(codeString)) 937 return ConditionalDeleteStatus.MULTIPLE; 938 throw new IllegalArgumentException("Unknown ConditionalDeleteStatus code '"+codeString+"'"); 939 } 940 public Enumeration<ConditionalDeleteStatus> fromType(PrimitiveType<?> code) throws FHIRException { 941 if (code == null) 942 return null; 943 if (code.isEmpty()) 944 return new Enumeration<ConditionalDeleteStatus>(this); 945 String codeString = code.asStringValue(); 946 if (codeString == null || "".equals(codeString)) 947 return null; 948 if ("not-supported".equals(codeString)) 949 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NOTSUPPORTED); 950 if ("single".equals(codeString)) 951 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.SINGLE); 952 if ("multiple".equals(codeString)) 953 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.MULTIPLE); 954 throw new FHIRException("Unknown ConditionalDeleteStatus code '"+codeString+"'"); 955 } 956 public String toCode(ConditionalDeleteStatus code) { 957 if (code == ConditionalDeleteStatus.NULL) 958 return null; 959 if (code == ConditionalDeleteStatus.NOTSUPPORTED) 960 return "not-supported"; 961 if (code == ConditionalDeleteStatus.SINGLE) 962 return "single"; 963 if (code == ConditionalDeleteStatus.MULTIPLE) 964 return "multiple"; 965 return "?"; 966 } 967 public String toSystem(ConditionalDeleteStatus code) { 968 return code.getSystem(); 969 } 970 } 971 972 public enum ReferenceHandlingPolicy { 973 /** 974 * The server supports and populates Literal references where they are known (this code does not guarantee that all references are literal; see 'enforced') 975 */ 976 LITERAL, 977 /** 978 * The server allows logical references 979 */ 980 LOGICAL, 981 /** 982 * The server will attempt to resolve logical references to literal references (if resolution fails, the server may still accept resources; see logical) 983 */ 984 RESOLVES, 985 /** 986 * The server enforces that references have integrity - e.g. it ensures that references can always be resolved. This is typically the case for clinical record systems, but often not the case for middleware/proxy systems 987 */ 988 ENFORCED, 989 /** 990 * The server does not support references that point to other servers 991 */ 992 LOCAL, 993 /** 994 * added to help the parsers with the generic types 995 */ 996 NULL; 997 public static ReferenceHandlingPolicy fromCode(String codeString) throws FHIRException { 998 if (codeString == null || "".equals(codeString)) 999 return null; 1000 if ("literal".equals(codeString)) 1001 return LITERAL; 1002 if ("logical".equals(codeString)) 1003 return LOGICAL; 1004 if ("resolves".equals(codeString)) 1005 return RESOLVES; 1006 if ("enforced".equals(codeString)) 1007 return ENFORCED; 1008 if ("local".equals(codeString)) 1009 return LOCAL; 1010 if (Configuration.isAcceptInvalidEnums()) 1011 return null; 1012 else 1013 throw new FHIRException("Unknown ReferenceHandlingPolicy code '"+codeString+"'"); 1014 } 1015 public String toCode() { 1016 switch (this) { 1017 case LITERAL: return "literal"; 1018 case LOGICAL: return "logical"; 1019 case RESOLVES: return "resolves"; 1020 case ENFORCED: return "enforced"; 1021 case LOCAL: return "local"; 1022 case NULL: return null; 1023 default: return "?"; 1024 } 1025 } 1026 public String getSystem() { 1027 switch (this) { 1028 case LITERAL: return "http://hl7.org/fhir/reference-handling-policy"; 1029 case LOGICAL: return "http://hl7.org/fhir/reference-handling-policy"; 1030 case RESOLVES: return "http://hl7.org/fhir/reference-handling-policy"; 1031 case ENFORCED: return "http://hl7.org/fhir/reference-handling-policy"; 1032 case LOCAL: return "http://hl7.org/fhir/reference-handling-policy"; 1033 case NULL: return null; 1034 default: return "?"; 1035 } 1036 } 1037 public String getDefinition() { 1038 switch (this) { 1039 case LITERAL: return "The server supports and populates Literal references where they are known (this code does not guarantee that all references are literal; see 'enforced')"; 1040 case LOGICAL: return "The server allows logical references"; 1041 case RESOLVES: return "The server will attempt to resolve logical references to literal references (if resolution fails, the server may still accept resources; see logical)"; 1042 case ENFORCED: return "The server enforces that references have integrity - e.g. it ensures that references can always be resolved. This is typically the case for clinical record systems, but often not the case for middleware/proxy systems"; 1043 case LOCAL: return "The server does not support references that point to other servers"; 1044 case NULL: return null; 1045 default: return "?"; 1046 } 1047 } 1048 public String getDisplay() { 1049 switch (this) { 1050 case LITERAL: return "Literal References"; 1051 case LOGICAL: return "Logical References"; 1052 case RESOLVES: return "Resolves References"; 1053 case ENFORCED: return "Reference Integrity Enforced"; 1054 case LOCAL: return "Local References Only"; 1055 case NULL: return null; 1056 default: return "?"; 1057 } 1058 } 1059 } 1060 1061 public static class ReferenceHandlingPolicyEnumFactory implements EnumFactory<ReferenceHandlingPolicy> { 1062 public ReferenceHandlingPolicy fromCode(String codeString) throws IllegalArgumentException { 1063 if (codeString == null || "".equals(codeString)) 1064 if (codeString == null || "".equals(codeString)) 1065 return null; 1066 if ("literal".equals(codeString)) 1067 return ReferenceHandlingPolicy.LITERAL; 1068 if ("logical".equals(codeString)) 1069 return ReferenceHandlingPolicy.LOGICAL; 1070 if ("resolves".equals(codeString)) 1071 return ReferenceHandlingPolicy.RESOLVES; 1072 if ("enforced".equals(codeString)) 1073 return ReferenceHandlingPolicy.ENFORCED; 1074 if ("local".equals(codeString)) 1075 return ReferenceHandlingPolicy.LOCAL; 1076 throw new IllegalArgumentException("Unknown ReferenceHandlingPolicy code '"+codeString+"'"); 1077 } 1078 public Enumeration<ReferenceHandlingPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 1079 if (code == null) 1080 return null; 1081 if (code.isEmpty()) 1082 return new Enumeration<ReferenceHandlingPolicy>(this); 1083 String codeString = code.asStringValue(); 1084 if (codeString == null || "".equals(codeString)) 1085 return null; 1086 if ("literal".equals(codeString)) 1087 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LITERAL); 1088 if ("logical".equals(codeString)) 1089 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOGICAL); 1090 if ("resolves".equals(codeString)) 1091 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.RESOLVES); 1092 if ("enforced".equals(codeString)) 1093 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.ENFORCED); 1094 if ("local".equals(codeString)) 1095 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOCAL); 1096 throw new FHIRException("Unknown ReferenceHandlingPolicy code '"+codeString+"'"); 1097 } 1098 public String toCode(ReferenceHandlingPolicy code) { 1099 if (code == ReferenceHandlingPolicy.NULL) 1100 return null; 1101 if (code == ReferenceHandlingPolicy.LITERAL) 1102 return "literal"; 1103 if (code == ReferenceHandlingPolicy.LOGICAL) 1104 return "logical"; 1105 if (code == ReferenceHandlingPolicy.RESOLVES) 1106 return "resolves"; 1107 if (code == ReferenceHandlingPolicy.ENFORCED) 1108 return "enforced"; 1109 if (code == ReferenceHandlingPolicy.LOCAL) 1110 return "local"; 1111 return "?"; 1112 } 1113 public String toSystem(ReferenceHandlingPolicy code) { 1114 return code.getSystem(); 1115 } 1116 } 1117 1118 public enum SystemRestfulInteraction { 1119 /** 1120 * null 1121 */ 1122 TRANSACTION, 1123 /** 1124 * null 1125 */ 1126 BATCH, 1127 /** 1128 * null 1129 */ 1130 SEARCHSYSTEM, 1131 /** 1132 * null 1133 */ 1134 HISTORYSYSTEM, 1135 /** 1136 * added to help the parsers with the generic types 1137 */ 1138 NULL; 1139 public static SystemRestfulInteraction fromCode(String codeString) throws FHIRException { 1140 if (codeString == null || "".equals(codeString)) 1141 return null; 1142 if ("transaction".equals(codeString)) 1143 return TRANSACTION; 1144 if ("batch".equals(codeString)) 1145 return BATCH; 1146 if ("search-system".equals(codeString)) 1147 return SEARCHSYSTEM; 1148 if ("history-system".equals(codeString)) 1149 return HISTORYSYSTEM; 1150 if (Configuration.isAcceptInvalidEnums()) 1151 return null; 1152 else 1153 throw new FHIRException("Unknown SystemRestfulInteraction code '"+codeString+"'"); 1154 } 1155 public String toCode() { 1156 switch (this) { 1157 case TRANSACTION: return "transaction"; 1158 case BATCH: return "batch"; 1159 case SEARCHSYSTEM: return "search-system"; 1160 case HISTORYSYSTEM: return "history-system"; 1161 case NULL: return null; 1162 default: return "?"; 1163 } 1164 } 1165 public String getSystem() { 1166 switch (this) { 1167 case TRANSACTION: return "http://hl7.org/fhir/restful-interaction"; 1168 case BATCH: return "http://hl7.org/fhir/restful-interaction"; 1169 case SEARCHSYSTEM: return "http://hl7.org/fhir/restful-interaction"; 1170 case HISTORYSYSTEM: return "http://hl7.org/fhir/restful-interaction"; 1171 case NULL: return null; 1172 default: return "?"; 1173 } 1174 } 1175 public String getDefinition() { 1176 switch (this) { 1177 case TRANSACTION: return ""; 1178 case BATCH: return ""; 1179 case SEARCHSYSTEM: return ""; 1180 case HISTORYSYSTEM: return ""; 1181 case NULL: return null; 1182 default: return "?"; 1183 } 1184 } 1185 public String getDisplay() { 1186 switch (this) { 1187 case TRANSACTION: return "transaction"; 1188 case BATCH: return "batch"; 1189 case SEARCHSYSTEM: return "search-system"; 1190 case HISTORYSYSTEM: return "history-system"; 1191 case NULL: return null; 1192 default: return "?"; 1193 } 1194 } 1195 } 1196 1197 public static class SystemRestfulInteractionEnumFactory implements EnumFactory<SystemRestfulInteraction> { 1198 public SystemRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 1199 if (codeString == null || "".equals(codeString)) 1200 if (codeString == null || "".equals(codeString)) 1201 return null; 1202 if ("transaction".equals(codeString)) 1203 return SystemRestfulInteraction.TRANSACTION; 1204 if ("batch".equals(codeString)) 1205 return SystemRestfulInteraction.BATCH; 1206 if ("search-system".equals(codeString)) 1207 return SystemRestfulInteraction.SEARCHSYSTEM; 1208 if ("history-system".equals(codeString)) 1209 return SystemRestfulInteraction.HISTORYSYSTEM; 1210 throw new IllegalArgumentException("Unknown SystemRestfulInteraction code '"+codeString+"'"); 1211 } 1212 public Enumeration<SystemRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 1213 if (code == null) 1214 return null; 1215 if (code.isEmpty()) 1216 return new Enumeration<SystemRestfulInteraction>(this); 1217 String codeString = code.asStringValue(); 1218 if (codeString == null || "".equals(codeString)) 1219 return null; 1220 if ("transaction".equals(codeString)) 1221 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.TRANSACTION); 1222 if ("batch".equals(codeString)) 1223 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.BATCH); 1224 if ("search-system".equals(codeString)) 1225 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.SEARCHSYSTEM); 1226 if ("history-system".equals(codeString)) 1227 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.HISTORYSYSTEM); 1228 throw new FHIRException("Unknown SystemRestfulInteraction code '"+codeString+"'"); 1229 } 1230 public String toCode(SystemRestfulInteraction code) { 1231 if (code == SystemRestfulInteraction.NULL) 1232 return null; 1233 if (code == SystemRestfulInteraction.TRANSACTION) 1234 return "transaction"; 1235 if (code == SystemRestfulInteraction.BATCH) 1236 return "batch"; 1237 if (code == SystemRestfulInteraction.SEARCHSYSTEM) 1238 return "search-system"; 1239 if (code == SystemRestfulInteraction.HISTORYSYSTEM) 1240 return "history-system"; 1241 return "?"; 1242 } 1243 public String toSystem(SystemRestfulInteraction code) { 1244 return code.getSystem(); 1245 } 1246 } 1247 1248 public enum EventCapabilityMode { 1249 /** 1250 * The application sends requests and receives responses. 1251 */ 1252 SENDER, 1253 /** 1254 * The application receives requests and sends responses. 1255 */ 1256 RECEIVER, 1257 /** 1258 * added to help the parsers with the generic types 1259 */ 1260 NULL; 1261 public static EventCapabilityMode fromCode(String codeString) throws FHIRException { 1262 if (codeString == null || "".equals(codeString)) 1263 return null; 1264 if ("sender".equals(codeString)) 1265 return SENDER; 1266 if ("receiver".equals(codeString)) 1267 return RECEIVER; 1268 if (Configuration.isAcceptInvalidEnums()) 1269 return null; 1270 else 1271 throw new FHIRException("Unknown EventCapabilityMode code '"+codeString+"'"); 1272 } 1273 public String toCode() { 1274 switch (this) { 1275 case SENDER: return "sender"; 1276 case RECEIVER: return "receiver"; 1277 case NULL: return null; 1278 default: return "?"; 1279 } 1280 } 1281 public String getSystem() { 1282 switch (this) { 1283 case SENDER: return "http://hl7.org/fhir/event-capability-mode"; 1284 case RECEIVER: return "http://hl7.org/fhir/event-capability-mode"; 1285 case NULL: return null; 1286 default: return "?"; 1287 } 1288 } 1289 public String getDefinition() { 1290 switch (this) { 1291 case SENDER: return "The application sends requests and receives responses."; 1292 case RECEIVER: return "The application receives requests and sends responses."; 1293 case NULL: return null; 1294 default: return "?"; 1295 } 1296 } 1297 public String getDisplay() { 1298 switch (this) { 1299 case SENDER: return "Sender"; 1300 case RECEIVER: return "Receiver"; 1301 case NULL: return null; 1302 default: return "?"; 1303 } 1304 } 1305 } 1306 1307 public static class EventCapabilityModeEnumFactory implements EnumFactory<EventCapabilityMode> { 1308 public EventCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 1309 if (codeString == null || "".equals(codeString)) 1310 if (codeString == null || "".equals(codeString)) 1311 return null; 1312 if ("sender".equals(codeString)) 1313 return EventCapabilityMode.SENDER; 1314 if ("receiver".equals(codeString)) 1315 return EventCapabilityMode.RECEIVER; 1316 throw new IllegalArgumentException("Unknown EventCapabilityMode code '"+codeString+"'"); 1317 } 1318 public Enumeration<EventCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 1319 if (code == null) 1320 return null; 1321 if (code.isEmpty()) 1322 return new Enumeration<EventCapabilityMode>(this); 1323 String codeString = code.asStringValue(); 1324 if (codeString == null || "".equals(codeString)) 1325 return null; 1326 if ("sender".equals(codeString)) 1327 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.SENDER); 1328 if ("receiver".equals(codeString)) 1329 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.RECEIVER); 1330 throw new FHIRException("Unknown EventCapabilityMode code '"+codeString+"'"); 1331 } 1332 public String toCode(EventCapabilityMode code) { 1333 if (code == EventCapabilityMode.NULL) 1334 return null; 1335 if (code == EventCapabilityMode.SENDER) 1336 return "sender"; 1337 if (code == EventCapabilityMode.RECEIVER) 1338 return "receiver"; 1339 return "?"; 1340 } 1341 public String toSystem(EventCapabilityMode code) { 1342 return code.getSystem(); 1343 } 1344 } 1345 1346 public enum MessageSignificanceCategory { 1347 /** 1348 * The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment. 1349 */ 1350 CONSEQUENCE, 1351 /** 1352 * The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful. 1353 */ 1354 CURRENCY, 1355 /** 1356 * The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications. 1357 */ 1358 NOTIFICATION, 1359 /** 1360 * added to help the parsers with the generic types 1361 */ 1362 NULL; 1363 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 1364 if (codeString == null || "".equals(codeString)) 1365 return null; 1366 if ("Consequence".equals(codeString)) 1367 return CONSEQUENCE; 1368 if ("Currency".equals(codeString)) 1369 return CURRENCY; 1370 if ("Notification".equals(codeString)) 1371 return NOTIFICATION; 1372 if (Configuration.isAcceptInvalidEnums()) 1373 return null; 1374 else 1375 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 1376 } 1377 public String toCode() { 1378 switch (this) { 1379 case CONSEQUENCE: return "Consequence"; 1380 case CURRENCY: return "Currency"; 1381 case NOTIFICATION: return "Notification"; 1382 case NULL: return null; 1383 default: return "?"; 1384 } 1385 } 1386 public String getSystem() { 1387 switch (this) { 1388 case CONSEQUENCE: return "http://hl7.org/fhir/message-significance-category"; 1389 case CURRENCY: return "http://hl7.org/fhir/message-significance-category"; 1390 case NOTIFICATION: return "http://hl7.org/fhir/message-significance-category"; 1391 case NULL: return null; 1392 default: return "?"; 1393 } 1394 } 1395 public String getDefinition() { 1396 switch (this) { 1397 case CONSEQUENCE: return "The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment."; 1398 case CURRENCY: return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 1399 case NOTIFICATION: return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 1400 case NULL: return null; 1401 default: return "?"; 1402 } 1403 } 1404 public String getDisplay() { 1405 switch (this) { 1406 case CONSEQUENCE: return "Consequence"; 1407 case CURRENCY: return "Currency"; 1408 case NOTIFICATION: return "Notification"; 1409 case NULL: return null; 1410 default: return "?"; 1411 } 1412 } 1413 } 1414 1415 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 1416 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 1417 if (codeString == null || "".equals(codeString)) 1418 if (codeString == null || "".equals(codeString)) 1419 return null; 1420 if ("Consequence".equals(codeString)) 1421 return MessageSignificanceCategory.CONSEQUENCE; 1422 if ("Currency".equals(codeString)) 1423 return MessageSignificanceCategory.CURRENCY; 1424 if ("Notification".equals(codeString)) 1425 return MessageSignificanceCategory.NOTIFICATION; 1426 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 1427 } 1428 public Enumeration<MessageSignificanceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 1429 if (code == null) 1430 return null; 1431 if (code.isEmpty()) 1432 return new Enumeration<MessageSignificanceCategory>(this); 1433 String codeString = code.asStringValue(); 1434 if (codeString == null || "".equals(codeString)) 1435 return null; 1436 if ("Consequence".equals(codeString)) 1437 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE); 1438 if ("Currency".equals(codeString)) 1439 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY); 1440 if ("Notification".equals(codeString)) 1441 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION); 1442 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 1443 } 1444 public String toCode(MessageSignificanceCategory code) { 1445 if (code == MessageSignificanceCategory.NULL) 1446 return null; 1447 if (code == MessageSignificanceCategory.CONSEQUENCE) 1448 return "Consequence"; 1449 if (code == MessageSignificanceCategory.CURRENCY) 1450 return "Currency"; 1451 if (code == MessageSignificanceCategory.NOTIFICATION) 1452 return "Notification"; 1453 return "?"; 1454 } 1455 public String toSystem(MessageSignificanceCategory code) { 1456 return code.getSystem(); 1457 } 1458 } 1459 1460 public enum DocumentMode { 1461 /** 1462 * The application produces documents of the specified type. 1463 */ 1464 PRODUCER, 1465 /** 1466 * The application consumes documents of the specified type. 1467 */ 1468 CONSUMER, 1469 /** 1470 * added to help the parsers with the generic types 1471 */ 1472 NULL; 1473 public static DocumentMode fromCode(String codeString) throws FHIRException { 1474 if (codeString == null || "".equals(codeString)) 1475 return null; 1476 if ("producer".equals(codeString)) 1477 return PRODUCER; 1478 if ("consumer".equals(codeString)) 1479 return CONSUMER; 1480 if (Configuration.isAcceptInvalidEnums()) 1481 return null; 1482 else 1483 throw new FHIRException("Unknown DocumentMode code '"+codeString+"'"); 1484 } 1485 public String toCode() { 1486 switch (this) { 1487 case PRODUCER: return "producer"; 1488 case CONSUMER: return "consumer"; 1489 case NULL: return null; 1490 default: return "?"; 1491 } 1492 } 1493 public String getSystem() { 1494 switch (this) { 1495 case PRODUCER: return "http://hl7.org/fhir/document-mode"; 1496 case CONSUMER: return "http://hl7.org/fhir/document-mode"; 1497 case NULL: return null; 1498 default: return "?"; 1499 } 1500 } 1501 public String getDefinition() { 1502 switch (this) { 1503 case PRODUCER: return "The application produces documents of the specified type."; 1504 case CONSUMER: return "The application consumes documents of the specified type."; 1505 case NULL: return null; 1506 default: return "?"; 1507 } 1508 } 1509 public String getDisplay() { 1510 switch (this) { 1511 case PRODUCER: return "Producer"; 1512 case CONSUMER: return "Consumer"; 1513 case NULL: return null; 1514 default: return "?"; 1515 } 1516 } 1517 } 1518 1519 public static class DocumentModeEnumFactory implements EnumFactory<DocumentMode> { 1520 public DocumentMode fromCode(String codeString) throws IllegalArgumentException { 1521 if (codeString == null || "".equals(codeString)) 1522 if (codeString == null || "".equals(codeString)) 1523 return null; 1524 if ("producer".equals(codeString)) 1525 return DocumentMode.PRODUCER; 1526 if ("consumer".equals(codeString)) 1527 return DocumentMode.CONSUMER; 1528 throw new IllegalArgumentException("Unknown DocumentMode code '"+codeString+"'"); 1529 } 1530 public Enumeration<DocumentMode> fromType(PrimitiveType<?> code) throws FHIRException { 1531 if (code == null) 1532 return null; 1533 if (code.isEmpty()) 1534 return new Enumeration<DocumentMode>(this); 1535 String codeString = code.asStringValue(); 1536 if (codeString == null || "".equals(codeString)) 1537 return null; 1538 if ("producer".equals(codeString)) 1539 return new Enumeration<DocumentMode>(this, DocumentMode.PRODUCER); 1540 if ("consumer".equals(codeString)) 1541 return new Enumeration<DocumentMode>(this, DocumentMode.CONSUMER); 1542 throw new FHIRException("Unknown DocumentMode code '"+codeString+"'"); 1543 } 1544 public String toCode(DocumentMode code) { 1545 if (code == DocumentMode.NULL) 1546 return null; 1547 if (code == DocumentMode.PRODUCER) 1548 return "producer"; 1549 if (code == DocumentMode.CONSUMER) 1550 return "consumer"; 1551 return "?"; 1552 } 1553 public String toSystem(DocumentMode code) { 1554 return code.getSystem(); 1555 } 1556 } 1557 1558 @Block() 1559 public static class CapabilityStatementSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 1560 /** 1561 * Name software is known by. 1562 */ 1563 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1564 @Description(shortDefinition="A name the software is known by", formalDefinition="Name software is known by." ) 1565 protected StringType name; 1566 1567 /** 1568 * The version identifier for the software covered by this statement. 1569 */ 1570 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1571 @Description(shortDefinition="Version covered by this statement", formalDefinition="The version identifier for the software covered by this statement." ) 1572 protected StringType version; 1573 1574 /** 1575 * Date this version of the software was released. 1576 */ 1577 @Child(name = "releaseDate", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1578 @Description(shortDefinition="Date this version released", formalDefinition="Date this version of the software was released." ) 1579 protected DateTimeType releaseDate; 1580 1581 private static final long serialVersionUID = 1819769027L; 1582 1583 /** 1584 * Constructor 1585 */ 1586 public CapabilityStatementSoftwareComponent() { 1587 super(); 1588 } 1589 1590 /** 1591 * Constructor 1592 */ 1593 public CapabilityStatementSoftwareComponent(StringType name) { 1594 super(); 1595 this.name = name; 1596 } 1597 1598 /** 1599 * @return {@link #name} (Name software is known by.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1600 */ 1601 public StringType getNameElement() { 1602 if (this.name == null) 1603 if (Configuration.errorOnAutoCreate()) 1604 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.name"); 1605 else if (Configuration.doAutoCreate()) 1606 this.name = new StringType(); // bb 1607 return this.name; 1608 } 1609 1610 public boolean hasNameElement() { 1611 return this.name != null && !this.name.isEmpty(); 1612 } 1613 1614 public boolean hasName() { 1615 return this.name != null && !this.name.isEmpty(); 1616 } 1617 1618 /** 1619 * @param value {@link #name} (Name software is known by.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1620 */ 1621 public CapabilityStatementSoftwareComponent setNameElement(StringType value) { 1622 this.name = value; 1623 return this; 1624 } 1625 1626 /** 1627 * @return Name software is known by. 1628 */ 1629 public String getName() { 1630 return this.name == null ? null : this.name.getValue(); 1631 } 1632 1633 /** 1634 * @param value Name software is known by. 1635 */ 1636 public CapabilityStatementSoftwareComponent setName(String value) { 1637 if (this.name == null) 1638 this.name = new StringType(); 1639 this.name.setValue(value); 1640 return this; 1641 } 1642 1643 /** 1644 * @return {@link #version} (The version identifier for the software covered by this statement.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1645 */ 1646 public StringType getVersionElement() { 1647 if (this.version == null) 1648 if (Configuration.errorOnAutoCreate()) 1649 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.version"); 1650 else if (Configuration.doAutoCreate()) 1651 this.version = new StringType(); // bb 1652 return this.version; 1653 } 1654 1655 public boolean hasVersionElement() { 1656 return this.version != null && !this.version.isEmpty(); 1657 } 1658 1659 public boolean hasVersion() { 1660 return this.version != null && !this.version.isEmpty(); 1661 } 1662 1663 /** 1664 * @param value {@link #version} (The version identifier for the software covered by this statement.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1665 */ 1666 public CapabilityStatementSoftwareComponent setVersionElement(StringType value) { 1667 this.version = value; 1668 return this; 1669 } 1670 1671 /** 1672 * @return The version identifier for the software covered by this statement. 1673 */ 1674 public String getVersion() { 1675 return this.version == null ? null : this.version.getValue(); 1676 } 1677 1678 /** 1679 * @param value The version identifier for the software covered by this statement. 1680 */ 1681 public CapabilityStatementSoftwareComponent setVersion(String value) { 1682 if (Utilities.noString(value)) 1683 this.version = null; 1684 else { 1685 if (this.version == null) 1686 this.version = new StringType(); 1687 this.version.setValue(value); 1688 } 1689 return this; 1690 } 1691 1692 /** 1693 * @return {@link #releaseDate} (Date this version of the software was released.). This is the underlying object with id, value and extensions. The accessor "getReleaseDate" gives direct access to the value 1694 */ 1695 public DateTimeType getReleaseDateElement() { 1696 if (this.releaseDate == null) 1697 if (Configuration.errorOnAutoCreate()) 1698 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.releaseDate"); 1699 else if (Configuration.doAutoCreate()) 1700 this.releaseDate = new DateTimeType(); // bb 1701 return this.releaseDate; 1702 } 1703 1704 public boolean hasReleaseDateElement() { 1705 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1706 } 1707 1708 public boolean hasReleaseDate() { 1709 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1710 } 1711 1712 /** 1713 * @param value {@link #releaseDate} (Date this version of the software was released.). This is the underlying object with id, value and extensions. The accessor "getReleaseDate" gives direct access to the value 1714 */ 1715 public CapabilityStatementSoftwareComponent setReleaseDateElement(DateTimeType value) { 1716 this.releaseDate = value; 1717 return this; 1718 } 1719 1720 /** 1721 * @return Date this version of the software was released. 1722 */ 1723 public Date getReleaseDate() { 1724 return this.releaseDate == null ? null : this.releaseDate.getValue(); 1725 } 1726 1727 /** 1728 * @param value Date this version of the software was released. 1729 */ 1730 public CapabilityStatementSoftwareComponent setReleaseDate(Date value) { 1731 if (value == null) 1732 this.releaseDate = null; 1733 else { 1734 if (this.releaseDate == null) 1735 this.releaseDate = new DateTimeType(); 1736 this.releaseDate.setValue(value); 1737 } 1738 return this; 1739 } 1740 1741 protected void listChildren(List<Property> children) { 1742 super.listChildren(children); 1743 children.add(new Property("name", "string", "Name software is known by.", 0, 1, name)); 1744 children.add(new Property("version", "string", "The version identifier for the software covered by this statement.", 0, 1, version)); 1745 children.add(new Property("releaseDate", "dateTime", "Date this version of the software was released.", 0, 1, releaseDate)); 1746 } 1747 1748 @Override 1749 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1750 switch (_hash) { 1751 case 3373707: /*name*/ return new Property("name", "string", "Name software is known by.", 0, 1, name); 1752 case 351608024: /*version*/ return new Property("version", "string", "The version identifier for the software covered by this statement.", 0, 1, version); 1753 case 212873301: /*releaseDate*/ return new Property("releaseDate", "dateTime", "Date this version of the software was released.", 0, 1, releaseDate); 1754 default: return super.getNamedProperty(_hash, _name, _checkValid); 1755 } 1756 1757 } 1758 1759 @Override 1760 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1761 switch (hash) { 1762 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1763 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1764 case 212873301: /*releaseDate*/ return this.releaseDate == null ? new Base[0] : new Base[] {this.releaseDate}; // DateTimeType 1765 default: return super.getProperty(hash, name, checkValid); 1766 } 1767 1768 } 1769 1770 @Override 1771 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1772 switch (hash) { 1773 case 3373707: // name 1774 this.name = castToString(value); // StringType 1775 return value; 1776 case 351608024: // version 1777 this.version = castToString(value); // StringType 1778 return value; 1779 case 212873301: // releaseDate 1780 this.releaseDate = castToDateTime(value); // DateTimeType 1781 return value; 1782 default: return super.setProperty(hash, name, value); 1783 } 1784 1785 } 1786 1787 @Override 1788 public Base setProperty(String name, Base value) throws FHIRException { 1789 if (name.equals("name")) { 1790 this.name = castToString(value); // StringType 1791 } else if (name.equals("version")) { 1792 this.version = castToString(value); // StringType 1793 } else if (name.equals("releaseDate")) { 1794 this.releaseDate = castToDateTime(value); // DateTimeType 1795 } else 1796 return super.setProperty(name, value); 1797 return value; 1798 } 1799 1800 @Override 1801 public Base makeProperty(int hash, String name) throws FHIRException { 1802 switch (hash) { 1803 case 3373707: return getNameElement(); 1804 case 351608024: return getVersionElement(); 1805 case 212873301: return getReleaseDateElement(); 1806 default: return super.makeProperty(hash, name); 1807 } 1808 1809 } 1810 1811 @Override 1812 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1813 switch (hash) { 1814 case 3373707: /*name*/ return new String[] {"string"}; 1815 case 351608024: /*version*/ return new String[] {"string"}; 1816 case 212873301: /*releaseDate*/ return new String[] {"dateTime"}; 1817 default: return super.getTypesForProperty(hash, name); 1818 } 1819 1820 } 1821 1822 @Override 1823 public Base addChild(String name) throws FHIRException { 1824 if (name.equals("name")) { 1825 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 1826 } 1827 else if (name.equals("version")) { 1828 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.version"); 1829 } 1830 else if (name.equals("releaseDate")) { 1831 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.releaseDate"); 1832 } 1833 else 1834 return super.addChild(name); 1835 } 1836 1837 public CapabilityStatementSoftwareComponent copy() { 1838 CapabilityStatementSoftwareComponent dst = new CapabilityStatementSoftwareComponent(); 1839 copyValues(dst); 1840 dst.name = name == null ? null : name.copy(); 1841 dst.version = version == null ? null : version.copy(); 1842 dst.releaseDate = releaseDate == null ? null : releaseDate.copy(); 1843 return dst; 1844 } 1845 1846 @Override 1847 public boolean equalsDeep(Base other_) { 1848 if (!super.equalsDeep(other_)) 1849 return false; 1850 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1851 return false; 1852 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 1853 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true) && compareDeep(releaseDate, o.releaseDate, true) 1854 ; 1855 } 1856 1857 @Override 1858 public boolean equalsShallow(Base other_) { 1859 if (!super.equalsShallow(other_)) 1860 return false; 1861 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1862 return false; 1863 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 1864 return compareValues(name, o.name, true) && compareValues(version, o.version, true) && compareValues(releaseDate, o.releaseDate, true) 1865 ; 1866 } 1867 1868 public boolean isEmpty() { 1869 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, version, releaseDate 1870 ); 1871 } 1872 1873 public String fhirType() { 1874 return "CapabilityStatement.software"; 1875 1876 } 1877 1878 } 1879 1880 @Block() 1881 public static class CapabilityStatementImplementationComponent extends BackboneElement implements IBaseBackboneElement { 1882 /** 1883 * Information about the specific installation that this capability statement relates to. 1884 */ 1885 @Child(name = "description", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1886 @Description(shortDefinition="Describes this specific instance", formalDefinition="Information about the specific installation that this capability statement relates to." ) 1887 protected StringType description; 1888 1889 /** 1890 * An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces. 1891 */ 1892 @Child(name = "url", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1893 @Description(shortDefinition="Base URL for the installation", formalDefinition="An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces." ) 1894 protected UriType url; 1895 1896 private static final long serialVersionUID = -289238508L; 1897 1898 /** 1899 * Constructor 1900 */ 1901 public CapabilityStatementImplementationComponent() { 1902 super(); 1903 } 1904 1905 /** 1906 * Constructor 1907 */ 1908 public CapabilityStatementImplementationComponent(StringType description) { 1909 super(); 1910 this.description = description; 1911 } 1912 1913 /** 1914 * @return {@link #description} (Information about the specific installation that this capability statement relates to.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1915 */ 1916 public StringType getDescriptionElement() { 1917 if (this.description == null) 1918 if (Configuration.errorOnAutoCreate()) 1919 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.description"); 1920 else if (Configuration.doAutoCreate()) 1921 this.description = new StringType(); // bb 1922 return this.description; 1923 } 1924 1925 public boolean hasDescriptionElement() { 1926 return this.description != null && !this.description.isEmpty(); 1927 } 1928 1929 public boolean hasDescription() { 1930 return this.description != null && !this.description.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #description} (Information about the specific installation that this capability statement relates to.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1935 */ 1936 public CapabilityStatementImplementationComponent setDescriptionElement(StringType value) { 1937 this.description = value; 1938 return this; 1939 } 1940 1941 /** 1942 * @return Information about the specific installation that this capability statement relates to. 1943 */ 1944 public String getDescription() { 1945 return this.description == null ? null : this.description.getValue(); 1946 } 1947 1948 /** 1949 * @param value Information about the specific installation that this capability statement relates to. 1950 */ 1951 public CapabilityStatementImplementationComponent setDescription(String value) { 1952 if (this.description == null) 1953 this.description = new StringType(); 1954 this.description.setValue(value); 1955 return this; 1956 } 1957 1958 /** 1959 * @return {@link #url} (An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1960 */ 1961 public UriType getUrlElement() { 1962 if (this.url == null) 1963 if (Configuration.errorOnAutoCreate()) 1964 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.url"); 1965 else if (Configuration.doAutoCreate()) 1966 this.url = new UriType(); // bb 1967 return this.url; 1968 } 1969 1970 public boolean hasUrlElement() { 1971 return this.url != null && !this.url.isEmpty(); 1972 } 1973 1974 public boolean hasUrl() { 1975 return this.url != null && !this.url.isEmpty(); 1976 } 1977 1978 /** 1979 * @param value {@link #url} (An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1980 */ 1981 public CapabilityStatementImplementationComponent setUrlElement(UriType value) { 1982 this.url = value; 1983 return this; 1984 } 1985 1986 /** 1987 * @return An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces. 1988 */ 1989 public String getUrl() { 1990 return this.url == null ? null : this.url.getValue(); 1991 } 1992 1993 /** 1994 * @param value An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces. 1995 */ 1996 public CapabilityStatementImplementationComponent setUrl(String value) { 1997 if (Utilities.noString(value)) 1998 this.url = null; 1999 else { 2000 if (this.url == null) 2001 this.url = new UriType(); 2002 this.url.setValue(value); 2003 } 2004 return this; 2005 } 2006 2007 protected void listChildren(List<Property> children) { 2008 super.listChildren(children); 2009 children.add(new Property("description", "string", "Information about the specific installation that this capability statement relates to.", 0, 1, description)); 2010 children.add(new Property("url", "uri", "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 0, 1, url)); 2011 } 2012 2013 @Override 2014 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2015 switch (_hash) { 2016 case -1724546052: /*description*/ return new Property("description", "string", "Information about the specific installation that this capability statement relates to.", 0, 1, description); 2017 case 116079: /*url*/ return new Property("url", "uri", "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 0, 1, url); 2018 default: return super.getNamedProperty(_hash, _name, _checkValid); 2019 } 2020 2021 } 2022 2023 @Override 2024 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2025 switch (hash) { 2026 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2027 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2028 default: return super.getProperty(hash, name, checkValid); 2029 } 2030 2031 } 2032 2033 @Override 2034 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2035 switch (hash) { 2036 case -1724546052: // description 2037 this.description = castToString(value); // StringType 2038 return value; 2039 case 116079: // url 2040 this.url = castToUri(value); // UriType 2041 return value; 2042 default: return super.setProperty(hash, name, value); 2043 } 2044 2045 } 2046 2047 @Override 2048 public Base setProperty(String name, Base value) throws FHIRException { 2049 if (name.equals("description")) { 2050 this.description = castToString(value); // StringType 2051 } else if (name.equals("url")) { 2052 this.url = castToUri(value); // UriType 2053 } else 2054 return super.setProperty(name, value); 2055 return value; 2056 } 2057 2058 @Override 2059 public Base makeProperty(int hash, String name) throws FHIRException { 2060 switch (hash) { 2061 case -1724546052: return getDescriptionElement(); 2062 case 116079: return getUrlElement(); 2063 default: return super.makeProperty(hash, name); 2064 } 2065 2066 } 2067 2068 @Override 2069 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2070 switch (hash) { 2071 case -1724546052: /*description*/ return new String[] {"string"}; 2072 case 116079: /*url*/ return new String[] {"uri"}; 2073 default: return super.getTypesForProperty(hash, name); 2074 } 2075 2076 } 2077 2078 @Override 2079 public Base addChild(String name) throws FHIRException { 2080 if (name.equals("description")) { 2081 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 2082 } 2083 else if (name.equals("url")) { 2084 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.url"); 2085 } 2086 else 2087 return super.addChild(name); 2088 } 2089 2090 public CapabilityStatementImplementationComponent copy() { 2091 CapabilityStatementImplementationComponent dst = new CapabilityStatementImplementationComponent(); 2092 copyValues(dst); 2093 dst.description = description == null ? null : description.copy(); 2094 dst.url = url == null ? null : url.copy(); 2095 return dst; 2096 } 2097 2098 @Override 2099 public boolean equalsDeep(Base other_) { 2100 if (!super.equalsDeep(other_)) 2101 return false; 2102 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 2103 return false; 2104 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 2105 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true); 2106 } 2107 2108 @Override 2109 public boolean equalsShallow(Base other_) { 2110 if (!super.equalsShallow(other_)) 2111 return false; 2112 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 2113 return false; 2114 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 2115 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 2116 } 2117 2118 public boolean isEmpty() { 2119 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, url); 2120 } 2121 2122 public String fhirType() { 2123 return "CapabilityStatement.implementation"; 2124 2125 } 2126 2127 } 2128 2129 @Block() 2130 public static class CapabilityStatementRestComponent extends BackboneElement implements IBaseBackboneElement { 2131 /** 2132 * Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations. 2133 */ 2134 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2135 @Description(shortDefinition="client | server", formalDefinition="Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations." ) 2136 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/restful-capability-mode") 2137 protected Enumeration<RestfulCapabilityMode> mode; 2138 2139 /** 2140 * Information about the system's restful capabilities that apply across all applications, such as security. 2141 */ 2142 @Child(name = "documentation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2143 @Description(shortDefinition="General description of implementation", formalDefinition="Information about the system's restful capabilities that apply across all applications, such as security." ) 2144 protected StringType documentation; 2145 2146 /** 2147 * Information about security implementation from an interface perspective - what a client needs to know. 2148 */ 2149 @Child(name = "security", type = {}, order=3, min=0, max=1, modifier=false, summary=true) 2150 @Description(shortDefinition="Information about security of implementation", formalDefinition="Information about security implementation from an interface perspective - what a client needs to know." ) 2151 protected CapabilityStatementRestSecurityComponent security; 2152 2153 /** 2154 * A specification of the restful capabilities of the solution for a specific resource type. 2155 */ 2156 @Child(name = "resource", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2157 @Description(shortDefinition="Resource served on the REST interface", formalDefinition="A specification of the restful capabilities of the solution for a specific resource type." ) 2158 protected List<CapabilityStatementRestResourceComponent> resource; 2159 2160 /** 2161 * A specification of restful operations supported by the system. 2162 */ 2163 @Child(name = "interaction", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2164 @Description(shortDefinition="What operations are supported?", formalDefinition="A specification of restful operations supported by the system." ) 2165 protected List<SystemInteractionComponent> interaction; 2166 2167 /** 2168 * Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. 2169 */ 2170 @Child(name = "searchParam", type = {CapabilityStatementRestResourceSearchParamComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2171 @Description(shortDefinition="Search parameters for searching all resources", formalDefinition="Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation." ) 2172 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 2173 2174 /** 2175 * Definition of an operation or a named query together with its parameters and their meaning and type. 2176 */ 2177 @Child(name = "operation", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2178 @Description(shortDefinition="Definition of an operation or a custom query", formalDefinition="Definition of an operation or a named query together with its parameters and their meaning and type." ) 2179 protected List<CapabilityStatementRestOperationComponent> operation; 2180 2181 /** 2182 * An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL . 2183 */ 2184 @Child(name = "compartment", type = {UriType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2185 @Description(shortDefinition="Compartments served/used by system", formalDefinition="An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL ." ) 2186 protected List<UriType> compartment; 2187 2188 private static final long serialVersionUID = 38012979L; 2189 2190 /** 2191 * Constructor 2192 */ 2193 public CapabilityStatementRestComponent() { 2194 super(); 2195 } 2196 2197 /** 2198 * Constructor 2199 */ 2200 public CapabilityStatementRestComponent(Enumeration<RestfulCapabilityMode> mode) { 2201 super(); 2202 this.mode = mode; 2203 } 2204 2205 /** 2206 * @return {@link #mode} (Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2207 */ 2208 public Enumeration<RestfulCapabilityMode> getModeElement() { 2209 if (this.mode == null) 2210 if (Configuration.errorOnAutoCreate()) 2211 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.mode"); 2212 else if (Configuration.doAutoCreate()) 2213 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); // bb 2214 return this.mode; 2215 } 2216 2217 public boolean hasModeElement() { 2218 return this.mode != null && !this.mode.isEmpty(); 2219 } 2220 2221 public boolean hasMode() { 2222 return this.mode != null && !this.mode.isEmpty(); 2223 } 2224 2225 /** 2226 * @param value {@link #mode} (Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2227 */ 2228 public CapabilityStatementRestComponent setModeElement(Enumeration<RestfulCapabilityMode> value) { 2229 this.mode = value; 2230 return this; 2231 } 2232 2233 /** 2234 * @return Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations. 2235 */ 2236 public RestfulCapabilityMode getMode() { 2237 return this.mode == null ? null : this.mode.getValue(); 2238 } 2239 2240 /** 2241 * @param value Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations. 2242 */ 2243 public CapabilityStatementRestComponent setMode(RestfulCapabilityMode value) { 2244 if (this.mode == null) 2245 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); 2246 this.mode.setValue(value); 2247 return this; 2248 } 2249 2250 /** 2251 * @return {@link #documentation} (Information about the system's restful capabilities that apply across all applications, such as security.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2252 */ 2253 public StringType getDocumentationElement() { 2254 if (this.documentation == null) 2255 if (Configuration.errorOnAutoCreate()) 2256 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.documentation"); 2257 else if (Configuration.doAutoCreate()) 2258 this.documentation = new StringType(); // bb 2259 return this.documentation; 2260 } 2261 2262 public boolean hasDocumentationElement() { 2263 return this.documentation != null && !this.documentation.isEmpty(); 2264 } 2265 2266 public boolean hasDocumentation() { 2267 return this.documentation != null && !this.documentation.isEmpty(); 2268 } 2269 2270 /** 2271 * @param value {@link #documentation} (Information about the system's restful capabilities that apply across all applications, such as security.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2272 */ 2273 public CapabilityStatementRestComponent setDocumentationElement(StringType value) { 2274 this.documentation = value; 2275 return this; 2276 } 2277 2278 /** 2279 * @return Information about the system's restful capabilities that apply across all applications, such as security. 2280 */ 2281 public String getDocumentation() { 2282 return this.documentation == null ? null : this.documentation.getValue(); 2283 } 2284 2285 /** 2286 * @param value Information about the system's restful capabilities that apply across all applications, such as security. 2287 */ 2288 public CapabilityStatementRestComponent setDocumentation(String value) { 2289 if (Utilities.noString(value)) 2290 this.documentation = null; 2291 else { 2292 if (this.documentation == null) 2293 this.documentation = new StringType(); 2294 this.documentation.setValue(value); 2295 } 2296 return this; 2297 } 2298 2299 /** 2300 * @return {@link #security} (Information about security implementation from an interface perspective - what a client needs to know.) 2301 */ 2302 public CapabilityStatementRestSecurityComponent getSecurity() { 2303 if (this.security == null) 2304 if (Configuration.errorOnAutoCreate()) 2305 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.security"); 2306 else if (Configuration.doAutoCreate()) 2307 this.security = new CapabilityStatementRestSecurityComponent(); // cc 2308 return this.security; 2309 } 2310 2311 public boolean hasSecurity() { 2312 return this.security != null && !this.security.isEmpty(); 2313 } 2314 2315 /** 2316 * @param value {@link #security} (Information about security implementation from an interface perspective - what a client needs to know.) 2317 */ 2318 public CapabilityStatementRestComponent setSecurity(CapabilityStatementRestSecurityComponent value) { 2319 this.security = value; 2320 return this; 2321 } 2322 2323 /** 2324 * @return {@link #resource} (A specification of the restful capabilities of the solution for a specific resource type.) 2325 */ 2326 public List<CapabilityStatementRestResourceComponent> getResource() { 2327 if (this.resource == null) 2328 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2329 return this.resource; 2330 } 2331 2332 /** 2333 * @return Returns a reference to <code>this</code> for easy method chaining 2334 */ 2335 public CapabilityStatementRestComponent setResource(List<CapabilityStatementRestResourceComponent> theResource) { 2336 this.resource = theResource; 2337 return this; 2338 } 2339 2340 public boolean hasResource() { 2341 if (this.resource == null) 2342 return false; 2343 for (CapabilityStatementRestResourceComponent item : this.resource) 2344 if (!item.isEmpty()) 2345 return true; 2346 return false; 2347 } 2348 2349 public CapabilityStatementRestResourceComponent addResource() { //3 2350 CapabilityStatementRestResourceComponent t = new CapabilityStatementRestResourceComponent(); 2351 if (this.resource == null) 2352 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2353 this.resource.add(t); 2354 return t; 2355 } 2356 2357 public CapabilityStatementRestComponent addResource(CapabilityStatementRestResourceComponent t) { //3 2358 if (t == null) 2359 return this; 2360 if (this.resource == null) 2361 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2362 this.resource.add(t); 2363 return this; 2364 } 2365 2366 /** 2367 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist 2368 */ 2369 public CapabilityStatementRestResourceComponent getResourceFirstRep() { 2370 if (getResource().isEmpty()) { 2371 addResource(); 2372 } 2373 return getResource().get(0); 2374 } 2375 2376 /** 2377 * @return {@link #interaction} (A specification of restful operations supported by the system.) 2378 */ 2379 public List<SystemInteractionComponent> getInteraction() { 2380 if (this.interaction == null) 2381 this.interaction = new ArrayList<SystemInteractionComponent>(); 2382 return this.interaction; 2383 } 2384 2385 /** 2386 * @return Returns a reference to <code>this</code> for easy method chaining 2387 */ 2388 public CapabilityStatementRestComponent setInteraction(List<SystemInteractionComponent> theInteraction) { 2389 this.interaction = theInteraction; 2390 return this; 2391 } 2392 2393 public boolean hasInteraction() { 2394 if (this.interaction == null) 2395 return false; 2396 for (SystemInteractionComponent item : this.interaction) 2397 if (!item.isEmpty()) 2398 return true; 2399 return false; 2400 } 2401 2402 public SystemInteractionComponent addInteraction() { //3 2403 SystemInteractionComponent t = new SystemInteractionComponent(); 2404 if (this.interaction == null) 2405 this.interaction = new ArrayList<SystemInteractionComponent>(); 2406 this.interaction.add(t); 2407 return t; 2408 } 2409 2410 public CapabilityStatementRestComponent addInteraction(SystemInteractionComponent t) { //3 2411 if (t == null) 2412 return this; 2413 if (this.interaction == null) 2414 this.interaction = new ArrayList<SystemInteractionComponent>(); 2415 this.interaction.add(t); 2416 return this; 2417 } 2418 2419 /** 2420 * @return The first repetition of repeating field {@link #interaction}, creating it if it does not already exist 2421 */ 2422 public SystemInteractionComponent getInteractionFirstRep() { 2423 if (getInteraction().isEmpty()) { 2424 addInteraction(); 2425 } 2426 return getInteraction().get(0); 2427 } 2428 2429 /** 2430 * @return {@link #searchParam} (Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.) 2431 */ 2432 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 2433 if (this.searchParam == null) 2434 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2435 return this.searchParam; 2436 } 2437 2438 /** 2439 * @return Returns a reference to <code>this</code> for easy method chaining 2440 */ 2441 public CapabilityStatementRestComponent setSearchParam(List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 2442 this.searchParam = theSearchParam; 2443 return this; 2444 } 2445 2446 public boolean hasSearchParam() { 2447 if (this.searchParam == null) 2448 return false; 2449 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 2450 if (!item.isEmpty()) 2451 return true; 2452 return false; 2453 } 2454 2455 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { //3 2456 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 2457 if (this.searchParam == null) 2458 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2459 this.searchParam.add(t); 2460 return t; 2461 } 2462 2463 public CapabilityStatementRestComponent addSearchParam(CapabilityStatementRestResourceSearchParamComponent t) { //3 2464 if (t == null) 2465 return this; 2466 if (this.searchParam == null) 2467 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2468 this.searchParam.add(t); 2469 return this; 2470 } 2471 2472 /** 2473 * @return The first repetition of repeating field {@link #searchParam}, creating it if it does not already exist 2474 */ 2475 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 2476 if (getSearchParam().isEmpty()) { 2477 addSearchParam(); 2478 } 2479 return getSearchParam().get(0); 2480 } 2481 2482 /** 2483 * @return {@link #operation} (Definition of an operation or a named query together with its parameters and their meaning and type.) 2484 */ 2485 public List<CapabilityStatementRestOperationComponent> getOperation() { 2486 if (this.operation == null) 2487 this.operation = new ArrayList<CapabilityStatementRestOperationComponent>(); 2488 return this.operation; 2489 } 2490 2491 /** 2492 * @return Returns a reference to <code>this</code> for easy method chaining 2493 */ 2494 public CapabilityStatementRestComponent setOperation(List<CapabilityStatementRestOperationComponent> theOperation) { 2495 this.operation = theOperation; 2496 return this; 2497 } 2498 2499 public boolean hasOperation() { 2500 if (this.operation == null) 2501 return false; 2502 for (CapabilityStatementRestOperationComponent item : this.operation) 2503 if (!item.isEmpty()) 2504 return true; 2505 return false; 2506 } 2507 2508 public CapabilityStatementRestOperationComponent addOperation() { //3 2509 CapabilityStatementRestOperationComponent t = new CapabilityStatementRestOperationComponent(); 2510 if (this.operation == null) 2511 this.operation = new ArrayList<CapabilityStatementRestOperationComponent>(); 2512 this.operation.add(t); 2513 return t; 2514 } 2515 2516 public CapabilityStatementRestComponent addOperation(CapabilityStatementRestOperationComponent t) { //3 2517 if (t == null) 2518 return this; 2519 if (this.operation == null) 2520 this.operation = new ArrayList<CapabilityStatementRestOperationComponent>(); 2521 this.operation.add(t); 2522 return this; 2523 } 2524 2525 /** 2526 * @return The first repetition of repeating field {@link #operation}, creating it if it does not already exist 2527 */ 2528 public CapabilityStatementRestOperationComponent getOperationFirstRep() { 2529 if (getOperation().isEmpty()) { 2530 addOperation(); 2531 } 2532 return getOperation().get(0); 2533 } 2534 2535 /** 2536 * @return {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2537 */ 2538 public List<UriType> getCompartment() { 2539 if (this.compartment == null) 2540 this.compartment = new ArrayList<UriType>(); 2541 return this.compartment; 2542 } 2543 2544 /** 2545 * @return Returns a reference to <code>this</code> for easy method chaining 2546 */ 2547 public CapabilityStatementRestComponent setCompartment(List<UriType> theCompartment) { 2548 this.compartment = theCompartment; 2549 return this; 2550 } 2551 2552 public boolean hasCompartment() { 2553 if (this.compartment == null) 2554 return false; 2555 for (UriType item : this.compartment) 2556 if (!item.isEmpty()) 2557 return true; 2558 return false; 2559 } 2560 2561 /** 2562 * @return {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2563 */ 2564 public UriType addCompartmentElement() {//2 2565 UriType t = new UriType(); 2566 if (this.compartment == null) 2567 this.compartment = new ArrayList<UriType>(); 2568 this.compartment.add(t); 2569 return t; 2570 } 2571 2572 /** 2573 * @param value {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2574 */ 2575 public CapabilityStatementRestComponent addCompartment(String value) { //1 2576 UriType t = new UriType(); 2577 t.setValue(value); 2578 if (this.compartment == null) 2579 this.compartment = new ArrayList<UriType>(); 2580 this.compartment.add(t); 2581 return this; 2582 } 2583 2584 /** 2585 * @param value {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2586 */ 2587 public boolean hasCompartment(String value) { 2588 if (this.compartment == null) 2589 return false; 2590 for (UriType v : this.compartment) 2591 if (v.getValue().equals(value)) // uri 2592 return true; 2593 return false; 2594 } 2595 2596 protected void listChildren(List<Property> children) { 2597 super.listChildren(children); 2598 children.add(new Property("mode", "code", "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 0, 1, mode)); 2599 children.add(new Property("documentation", "string", "Information about the system's restful capabilities that apply across all applications, such as security.", 0, 1, documentation)); 2600 children.add(new Property("security", "", "Information about security implementation from an interface perspective - what a client needs to know.", 0, 1, security)); 2601 children.add(new Property("resource", "", "A specification of the restful capabilities of the solution for a specific resource type.", 0, java.lang.Integer.MAX_VALUE, resource)); 2602 children.add(new Property("interaction", "", "A specification of restful operations supported by the system.", 0, java.lang.Integer.MAX_VALUE, interaction)); 2603 children.add(new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 0, java.lang.Integer.MAX_VALUE, searchParam)); 2604 children.add(new Property("operation", "", "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, java.lang.Integer.MAX_VALUE, operation)); 2605 children.add(new Property("compartment", "uri", "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 0, java.lang.Integer.MAX_VALUE, compartment)); 2606 } 2607 2608 @Override 2609 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2610 switch (_hash) { 2611 case 3357091: /*mode*/ return new Property("mode", "code", "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 0, 1, mode); 2612 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Information about the system's restful capabilities that apply across all applications, such as security.", 0, 1, documentation); 2613 case 949122880: /*security*/ return new Property("security", "", "Information about security implementation from an interface perspective - what a client needs to know.", 0, 1, security); 2614 case -341064690: /*resource*/ return new Property("resource", "", "A specification of the restful capabilities of the solution for a specific resource type.", 0, java.lang.Integer.MAX_VALUE, resource); 2615 case 1844104722: /*interaction*/ return new Property("interaction", "", "A specification of restful operations supported by the system.", 0, java.lang.Integer.MAX_VALUE, interaction); 2616 case -553645115: /*searchParam*/ return new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 0, java.lang.Integer.MAX_VALUE, searchParam); 2617 case 1662702951: /*operation*/ return new Property("operation", "", "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, java.lang.Integer.MAX_VALUE, operation); 2618 case -397756334: /*compartment*/ return new Property("compartment", "uri", "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 0, java.lang.Integer.MAX_VALUE, compartment); 2619 default: return super.getNamedProperty(_hash, _name, _checkValid); 2620 } 2621 2622 } 2623 2624 @Override 2625 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2626 switch (hash) { 2627 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<RestfulCapabilityMode> 2628 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2629 case 949122880: /*security*/ return this.security == null ? new Base[0] : new Base[] {this.security}; // CapabilityStatementRestSecurityComponent 2630 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CapabilityStatementRestResourceComponent 2631 case 1844104722: /*interaction*/ return this.interaction == null ? new Base[0] : this.interaction.toArray(new Base[this.interaction.size()]); // SystemInteractionComponent 2632 case -553645115: /*searchParam*/ return this.searchParam == null ? new Base[0] : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 2633 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : this.operation.toArray(new Base[this.operation.size()]); // CapabilityStatementRestOperationComponent 2634 case -397756334: /*compartment*/ return this.compartment == null ? new Base[0] : this.compartment.toArray(new Base[this.compartment.size()]); // UriType 2635 default: return super.getProperty(hash, name, checkValid); 2636 } 2637 2638 } 2639 2640 @Override 2641 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2642 switch (hash) { 2643 case 3357091: // mode 2644 value = new RestfulCapabilityModeEnumFactory().fromType(castToCode(value)); 2645 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 2646 return value; 2647 case 1587405498: // documentation 2648 this.documentation = castToString(value); // StringType 2649 return value; 2650 case 949122880: // security 2651 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 2652 return value; 2653 case -341064690: // resource 2654 this.getResource().add((CapabilityStatementRestResourceComponent) value); // CapabilityStatementRestResourceComponent 2655 return value; 2656 case 1844104722: // interaction 2657 this.getInteraction().add((SystemInteractionComponent) value); // SystemInteractionComponent 2658 return value; 2659 case -553645115: // searchParam 2660 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 2661 return value; 2662 case 1662702951: // operation 2663 this.getOperation().add((CapabilityStatementRestOperationComponent) value); // CapabilityStatementRestOperationComponent 2664 return value; 2665 case -397756334: // compartment 2666 this.getCompartment().add(castToUri(value)); // UriType 2667 return value; 2668 default: return super.setProperty(hash, name, value); 2669 } 2670 2671 } 2672 2673 @Override 2674 public Base setProperty(String name, Base value) throws FHIRException { 2675 if (name.equals("mode")) { 2676 value = new RestfulCapabilityModeEnumFactory().fromType(castToCode(value)); 2677 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 2678 } else if (name.equals("documentation")) { 2679 this.documentation = castToString(value); // StringType 2680 } else if (name.equals("security")) { 2681 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 2682 } else if (name.equals("resource")) { 2683 this.getResource().add((CapabilityStatementRestResourceComponent) value); 2684 } else if (name.equals("interaction")) { 2685 this.getInteraction().add((SystemInteractionComponent) value); 2686 } else if (name.equals("searchParam")) { 2687 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 2688 } else if (name.equals("operation")) { 2689 this.getOperation().add((CapabilityStatementRestOperationComponent) value); 2690 } else if (name.equals("compartment")) { 2691 this.getCompartment().add(castToUri(value)); 2692 } else 2693 return super.setProperty(name, value); 2694 return value; 2695 } 2696 2697 @Override 2698 public Base makeProperty(int hash, String name) throws FHIRException { 2699 switch (hash) { 2700 case 3357091: return getModeElement(); 2701 case 1587405498: return getDocumentationElement(); 2702 case 949122880: return getSecurity(); 2703 case -341064690: return addResource(); 2704 case 1844104722: return addInteraction(); 2705 case -553645115: return addSearchParam(); 2706 case 1662702951: return addOperation(); 2707 case -397756334: return addCompartmentElement(); 2708 default: return super.makeProperty(hash, name); 2709 } 2710 2711 } 2712 2713 @Override 2714 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2715 switch (hash) { 2716 case 3357091: /*mode*/ return new String[] {"code"}; 2717 case 1587405498: /*documentation*/ return new String[] {"string"}; 2718 case 949122880: /*security*/ return new String[] {}; 2719 case -341064690: /*resource*/ return new String[] {}; 2720 case 1844104722: /*interaction*/ return new String[] {}; 2721 case -553645115: /*searchParam*/ return new String[] {"@CapabilityStatement.rest.resource.searchParam"}; 2722 case 1662702951: /*operation*/ return new String[] {}; 2723 case -397756334: /*compartment*/ return new String[] {"uri"}; 2724 default: return super.getTypesForProperty(hash, name); 2725 } 2726 2727 } 2728 2729 @Override 2730 public Base addChild(String name) throws FHIRException { 2731 if (name.equals("mode")) { 2732 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 2733 } 2734 else if (name.equals("documentation")) { 2735 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 2736 } 2737 else if (name.equals("security")) { 2738 this.security = new CapabilityStatementRestSecurityComponent(); 2739 return this.security; 2740 } 2741 else if (name.equals("resource")) { 2742 return addResource(); 2743 } 2744 else if (name.equals("interaction")) { 2745 return addInteraction(); 2746 } 2747 else if (name.equals("searchParam")) { 2748 return addSearchParam(); 2749 } 2750 else if (name.equals("operation")) { 2751 return addOperation(); 2752 } 2753 else if (name.equals("compartment")) { 2754 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.compartment"); 2755 } 2756 else 2757 return super.addChild(name); 2758 } 2759 2760 public CapabilityStatementRestComponent copy() { 2761 CapabilityStatementRestComponent dst = new CapabilityStatementRestComponent(); 2762 copyValues(dst); 2763 dst.mode = mode == null ? null : mode.copy(); 2764 dst.documentation = documentation == null ? null : documentation.copy(); 2765 dst.security = security == null ? null : security.copy(); 2766 if (resource != null) { 2767 dst.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2768 for (CapabilityStatementRestResourceComponent i : resource) 2769 dst.resource.add(i.copy()); 2770 }; 2771 if (interaction != null) { 2772 dst.interaction = new ArrayList<SystemInteractionComponent>(); 2773 for (SystemInteractionComponent i : interaction) 2774 dst.interaction.add(i.copy()); 2775 }; 2776 if (searchParam != null) { 2777 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2778 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 2779 dst.searchParam.add(i.copy()); 2780 }; 2781 if (operation != null) { 2782 dst.operation = new ArrayList<CapabilityStatementRestOperationComponent>(); 2783 for (CapabilityStatementRestOperationComponent i : operation) 2784 dst.operation.add(i.copy()); 2785 }; 2786 if (compartment != null) { 2787 dst.compartment = new ArrayList<UriType>(); 2788 for (UriType i : compartment) 2789 dst.compartment.add(i.copy()); 2790 }; 2791 return dst; 2792 } 2793 2794 @Override 2795 public boolean equalsDeep(Base other_) { 2796 if (!super.equalsDeep(other_)) 2797 return false; 2798 if (!(other_ instanceof CapabilityStatementRestComponent)) 2799 return false; 2800 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 2801 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) && compareDeep(security, o.security, true) 2802 && compareDeep(resource, o.resource, true) && compareDeep(interaction, o.interaction, true) && compareDeep(searchParam, o.searchParam, true) 2803 && compareDeep(operation, o.operation, true) && compareDeep(compartment, o.compartment, true); 2804 } 2805 2806 @Override 2807 public boolean equalsShallow(Base other_) { 2808 if (!super.equalsShallow(other_)) 2809 return false; 2810 if (!(other_ instanceof CapabilityStatementRestComponent)) 2811 return false; 2812 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 2813 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true) && compareValues(compartment, o.compartment, true) 2814 ; 2815 } 2816 2817 public boolean isEmpty() { 2818 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, security 2819 , resource, interaction, searchParam, operation, compartment); 2820 } 2821 2822 public String fhirType() { 2823 return "CapabilityStatement.rest"; 2824 2825 } 2826 2827 } 2828 2829 @Block() 2830 public static class CapabilityStatementRestSecurityComponent extends BackboneElement implements IBaseBackboneElement { 2831 /** 2832 * Server adds CORS headers when responding to requests - this enables javascript applications to use the server. 2833 */ 2834 @Child(name = "cors", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2835 @Description(shortDefinition="Adds CORS Headers (http://enable-cors.org/)", formalDefinition="Server adds CORS headers when responding to requests - this enables javascript applications to use the server." ) 2836 protected BooleanType cors; 2837 2838 /** 2839 * Types of security services that are supported/required by the system. 2840 */ 2841 @Child(name = "service", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2842 @Description(shortDefinition="OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", formalDefinition="Types of security services that are supported/required by the system." ) 2843 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/restful-security-service") 2844 protected List<CodeableConcept> service; 2845 2846 /** 2847 * General description of how security works. 2848 */ 2849 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2850 @Description(shortDefinition="General description of how security works", formalDefinition="General description of how security works." ) 2851 protected StringType description; 2852 2853 /** 2854 * Certificates associated with security profiles. 2855 */ 2856 @Child(name = "certificate", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2857 @Description(shortDefinition="Certificates associated with security profiles", formalDefinition="Certificates associated with security profiles." ) 2858 protected List<CapabilityStatementRestSecurityCertificateComponent> certificate; 2859 2860 private static final long serialVersionUID = 1081654002L; 2861 2862 /** 2863 * Constructor 2864 */ 2865 public CapabilityStatementRestSecurityComponent() { 2866 super(); 2867 } 2868 2869 /** 2870 * @return {@link #cors} (Server adds CORS headers when responding to requests - this enables javascript applications to use the server.). This is the underlying object with id, value and extensions. The accessor "getCors" gives direct access to the value 2871 */ 2872 public BooleanType getCorsElement() { 2873 if (this.cors == null) 2874 if (Configuration.errorOnAutoCreate()) 2875 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.cors"); 2876 else if (Configuration.doAutoCreate()) 2877 this.cors = new BooleanType(); // bb 2878 return this.cors; 2879 } 2880 2881 public boolean hasCorsElement() { 2882 return this.cors != null && !this.cors.isEmpty(); 2883 } 2884 2885 public boolean hasCors() { 2886 return this.cors != null && !this.cors.isEmpty(); 2887 } 2888 2889 /** 2890 * @param value {@link #cors} (Server adds CORS headers when responding to requests - this enables javascript applications to use the server.). This is the underlying object with id, value and extensions. The accessor "getCors" gives direct access to the value 2891 */ 2892 public CapabilityStatementRestSecurityComponent setCorsElement(BooleanType value) { 2893 this.cors = value; 2894 return this; 2895 } 2896 2897 /** 2898 * @return Server adds CORS headers when responding to requests - this enables javascript applications to use the server. 2899 */ 2900 public boolean getCors() { 2901 return this.cors == null || this.cors.isEmpty() ? false : this.cors.getValue(); 2902 } 2903 2904 /** 2905 * @param value Server adds CORS headers when responding to requests - this enables javascript applications to use the server. 2906 */ 2907 public CapabilityStatementRestSecurityComponent setCors(boolean value) { 2908 if (this.cors == null) 2909 this.cors = new BooleanType(); 2910 this.cors.setValue(value); 2911 return this; 2912 } 2913 2914 /** 2915 * @return {@link #service} (Types of security services that are supported/required by the system.) 2916 */ 2917 public List<CodeableConcept> getService() { 2918 if (this.service == null) 2919 this.service = new ArrayList<CodeableConcept>(); 2920 return this.service; 2921 } 2922 2923 /** 2924 * @return Returns a reference to <code>this</code> for easy method chaining 2925 */ 2926 public CapabilityStatementRestSecurityComponent setService(List<CodeableConcept> theService) { 2927 this.service = theService; 2928 return this; 2929 } 2930 2931 public boolean hasService() { 2932 if (this.service == null) 2933 return false; 2934 for (CodeableConcept item : this.service) 2935 if (!item.isEmpty()) 2936 return true; 2937 return false; 2938 } 2939 2940 public CodeableConcept addService() { //3 2941 CodeableConcept t = new CodeableConcept(); 2942 if (this.service == null) 2943 this.service = new ArrayList<CodeableConcept>(); 2944 this.service.add(t); 2945 return t; 2946 } 2947 2948 public CapabilityStatementRestSecurityComponent addService(CodeableConcept t) { //3 2949 if (t == null) 2950 return this; 2951 if (this.service == null) 2952 this.service = new ArrayList<CodeableConcept>(); 2953 this.service.add(t); 2954 return this; 2955 } 2956 2957 /** 2958 * @return The first repetition of repeating field {@link #service}, creating it if it does not already exist 2959 */ 2960 public CodeableConcept getServiceFirstRep() { 2961 if (getService().isEmpty()) { 2962 addService(); 2963 } 2964 return getService().get(0); 2965 } 2966 2967 /** 2968 * @return {@link #description} (General description of how security works.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2969 */ 2970 public StringType getDescriptionElement() { 2971 if (this.description == null) 2972 if (Configuration.errorOnAutoCreate()) 2973 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.description"); 2974 else if (Configuration.doAutoCreate()) 2975 this.description = new StringType(); // bb 2976 return this.description; 2977 } 2978 2979 public boolean hasDescriptionElement() { 2980 return this.description != null && !this.description.isEmpty(); 2981 } 2982 2983 public boolean hasDescription() { 2984 return this.description != null && !this.description.isEmpty(); 2985 } 2986 2987 /** 2988 * @param value {@link #description} (General description of how security works.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2989 */ 2990 public CapabilityStatementRestSecurityComponent setDescriptionElement(StringType value) { 2991 this.description = value; 2992 return this; 2993 } 2994 2995 /** 2996 * @return General description of how security works. 2997 */ 2998 public String getDescription() { 2999 return this.description == null ? null : this.description.getValue(); 3000 } 3001 3002 /** 3003 * @param value General description of how security works. 3004 */ 3005 public CapabilityStatementRestSecurityComponent setDescription(String value) { 3006 if (Utilities.noString(value)) 3007 this.description = null; 3008 else { 3009 if (this.description == null) 3010 this.description = new StringType(); 3011 this.description.setValue(value); 3012 } 3013 return this; 3014 } 3015 3016 /** 3017 * @return {@link #certificate} (Certificates associated with security profiles.) 3018 */ 3019 public List<CapabilityStatementRestSecurityCertificateComponent> getCertificate() { 3020 if (this.certificate == null) 3021 this.certificate = new ArrayList<CapabilityStatementRestSecurityCertificateComponent>(); 3022 return this.certificate; 3023 } 3024 3025 /** 3026 * @return Returns a reference to <code>this</code> for easy method chaining 3027 */ 3028 public CapabilityStatementRestSecurityComponent setCertificate(List<CapabilityStatementRestSecurityCertificateComponent> theCertificate) { 3029 this.certificate = theCertificate; 3030 return this; 3031 } 3032 3033 public boolean hasCertificate() { 3034 if (this.certificate == null) 3035 return false; 3036 for (CapabilityStatementRestSecurityCertificateComponent item : this.certificate) 3037 if (!item.isEmpty()) 3038 return true; 3039 return false; 3040 } 3041 3042 public CapabilityStatementRestSecurityCertificateComponent addCertificate() { //3 3043 CapabilityStatementRestSecurityCertificateComponent t = new CapabilityStatementRestSecurityCertificateComponent(); 3044 if (this.certificate == null) 3045 this.certificate = new ArrayList<CapabilityStatementRestSecurityCertificateComponent>(); 3046 this.certificate.add(t); 3047 return t; 3048 } 3049 3050 public CapabilityStatementRestSecurityComponent addCertificate(CapabilityStatementRestSecurityCertificateComponent t) { //3 3051 if (t == null) 3052 return this; 3053 if (this.certificate == null) 3054 this.certificate = new ArrayList<CapabilityStatementRestSecurityCertificateComponent>(); 3055 this.certificate.add(t); 3056 return this; 3057 } 3058 3059 /** 3060 * @return The first repetition of repeating field {@link #certificate}, creating it if it does not already exist 3061 */ 3062 public CapabilityStatementRestSecurityCertificateComponent getCertificateFirstRep() { 3063 if (getCertificate().isEmpty()) { 3064 addCertificate(); 3065 } 3066 return getCertificate().get(0); 3067 } 3068 3069 protected void listChildren(List<Property> children) { 3070 super.listChildren(children); 3071 children.add(new Property("cors", "boolean", "Server adds CORS headers when responding to requests - this enables javascript applications to use the server.", 0, 1, cors)); 3072 children.add(new Property("service", "CodeableConcept", "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, service)); 3073 children.add(new Property("description", "string", "General description of how security works.", 0, 1, description)); 3074 children.add(new Property("certificate", "", "Certificates associated with security profiles.", 0, java.lang.Integer.MAX_VALUE, certificate)); 3075 } 3076 3077 @Override 3078 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3079 switch (_hash) { 3080 case 3059629: /*cors*/ return new Property("cors", "boolean", "Server adds CORS headers when responding to requests - this enables javascript applications to use the server.", 0, 1, cors); 3081 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, service); 3082 case -1724546052: /*description*/ return new Property("description", "string", "General description of how security works.", 0, 1, description); 3083 case 1952399767: /*certificate*/ return new Property("certificate", "", "Certificates associated with security profiles.", 0, java.lang.Integer.MAX_VALUE, certificate); 3084 default: return super.getNamedProperty(_hash, _name, _checkValid); 3085 } 3086 3087 } 3088 3089 @Override 3090 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3091 switch (hash) { 3092 case 3059629: /*cors*/ return this.cors == null ? new Base[0] : new Base[] {this.cors}; // BooleanType 3093 case 1984153269: /*service*/ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // CodeableConcept 3094 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3095 case 1952399767: /*certificate*/ return this.certificate == null ? new Base[0] : this.certificate.toArray(new Base[this.certificate.size()]); // CapabilityStatementRestSecurityCertificateComponent 3096 default: return super.getProperty(hash, name, checkValid); 3097 } 3098 3099 } 3100 3101 @Override 3102 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3103 switch (hash) { 3104 case 3059629: // cors 3105 this.cors = castToBoolean(value); // BooleanType 3106 return value; 3107 case 1984153269: // service 3108 this.getService().add(castToCodeableConcept(value)); // CodeableConcept 3109 return value; 3110 case -1724546052: // description 3111 this.description = castToString(value); // StringType 3112 return value; 3113 case 1952399767: // certificate 3114 this.getCertificate().add((CapabilityStatementRestSecurityCertificateComponent) value); // CapabilityStatementRestSecurityCertificateComponent 3115 return value; 3116 default: return super.setProperty(hash, name, value); 3117 } 3118 3119 } 3120 3121 @Override 3122 public Base setProperty(String name, Base value) throws FHIRException { 3123 if (name.equals("cors")) { 3124 this.cors = castToBoolean(value); // BooleanType 3125 } else if (name.equals("service")) { 3126 this.getService().add(castToCodeableConcept(value)); 3127 } else if (name.equals("description")) { 3128 this.description = castToString(value); // StringType 3129 } else if (name.equals("certificate")) { 3130 this.getCertificate().add((CapabilityStatementRestSecurityCertificateComponent) value); 3131 } else 3132 return super.setProperty(name, value); 3133 return value; 3134 } 3135 3136 @Override 3137 public Base makeProperty(int hash, String name) throws FHIRException { 3138 switch (hash) { 3139 case 3059629: return getCorsElement(); 3140 case 1984153269: return addService(); 3141 case -1724546052: return getDescriptionElement(); 3142 case 1952399767: return addCertificate(); 3143 default: return super.makeProperty(hash, name); 3144 } 3145 3146 } 3147 3148 @Override 3149 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3150 switch (hash) { 3151 case 3059629: /*cors*/ return new String[] {"boolean"}; 3152 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 3153 case -1724546052: /*description*/ return new String[] {"string"}; 3154 case 1952399767: /*certificate*/ return new String[] {}; 3155 default: return super.getTypesForProperty(hash, name); 3156 } 3157 3158 } 3159 3160 @Override 3161 public Base addChild(String name) throws FHIRException { 3162 if (name.equals("cors")) { 3163 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.cors"); 3164 } 3165 else if (name.equals("service")) { 3166 return addService(); 3167 } 3168 else if (name.equals("description")) { 3169 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 3170 } 3171 else if (name.equals("certificate")) { 3172 return addCertificate(); 3173 } 3174 else 3175 return super.addChild(name); 3176 } 3177 3178 public CapabilityStatementRestSecurityComponent copy() { 3179 CapabilityStatementRestSecurityComponent dst = new CapabilityStatementRestSecurityComponent(); 3180 copyValues(dst); 3181 dst.cors = cors == null ? null : cors.copy(); 3182 if (service != null) { 3183 dst.service = new ArrayList<CodeableConcept>(); 3184 for (CodeableConcept i : service) 3185 dst.service.add(i.copy()); 3186 }; 3187 dst.description = description == null ? null : description.copy(); 3188 if (certificate != null) { 3189 dst.certificate = new ArrayList<CapabilityStatementRestSecurityCertificateComponent>(); 3190 for (CapabilityStatementRestSecurityCertificateComponent i : certificate) 3191 dst.certificate.add(i.copy()); 3192 }; 3193 return dst; 3194 } 3195 3196 @Override 3197 public boolean equalsDeep(Base other_) { 3198 if (!super.equalsDeep(other_)) 3199 return false; 3200 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 3201 return false; 3202 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 3203 return compareDeep(cors, o.cors, true) && compareDeep(service, o.service, true) && compareDeep(description, o.description, true) 3204 && compareDeep(certificate, o.certificate, true); 3205 } 3206 3207 @Override 3208 public boolean equalsShallow(Base other_) { 3209 if (!super.equalsShallow(other_)) 3210 return false; 3211 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 3212 return false; 3213 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 3214 return compareValues(cors, o.cors, true) && compareValues(description, o.description, true); 3215 } 3216 3217 public boolean isEmpty() { 3218 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(cors, service, description 3219 , certificate); 3220 } 3221 3222 public String fhirType() { 3223 return "CapabilityStatement.rest.security"; 3224 3225 } 3226 3227 } 3228 3229 @Block() 3230 public static class CapabilityStatementRestSecurityCertificateComponent extends BackboneElement implements IBaseBackboneElement { 3231 /** 3232 * Mime type for a certificate. 3233 */ 3234 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3235 @Description(shortDefinition="Mime type for certificates", formalDefinition="Mime type for a certificate." ) 3236 protected CodeType type; 3237 3238 /** 3239 * Actual certificate. 3240 */ 3241 @Child(name = "blob", type = {Base64BinaryType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3242 @Description(shortDefinition="Actual certificate", formalDefinition="Actual certificate." ) 3243 protected Base64BinaryType blob; 3244 3245 private static final long serialVersionUID = 2092655854L; 3246 3247 /** 3248 * Constructor 3249 */ 3250 public CapabilityStatementRestSecurityCertificateComponent() { 3251 super(); 3252 } 3253 3254 /** 3255 * @return {@link #type} (Mime type for a certificate.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3256 */ 3257 public CodeType getTypeElement() { 3258 if (this.type == null) 3259 if (Configuration.errorOnAutoCreate()) 3260 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityCertificateComponent.type"); 3261 else if (Configuration.doAutoCreate()) 3262 this.type = new CodeType(); // bb 3263 return this.type; 3264 } 3265 3266 public boolean hasTypeElement() { 3267 return this.type != null && !this.type.isEmpty(); 3268 } 3269 3270 public boolean hasType() { 3271 return this.type != null && !this.type.isEmpty(); 3272 } 3273 3274 /** 3275 * @param value {@link #type} (Mime type for a certificate.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3276 */ 3277 public CapabilityStatementRestSecurityCertificateComponent setTypeElement(CodeType value) { 3278 this.type = value; 3279 return this; 3280 } 3281 3282 /** 3283 * @return Mime type for a certificate. 3284 */ 3285 public String getType() { 3286 return this.type == null ? null : this.type.getValue(); 3287 } 3288 3289 /** 3290 * @param value Mime type for a certificate. 3291 */ 3292 public CapabilityStatementRestSecurityCertificateComponent setType(String value) { 3293 if (Utilities.noString(value)) 3294 this.type = null; 3295 else { 3296 if (this.type == null) 3297 this.type = new CodeType(); 3298 this.type.setValue(value); 3299 } 3300 return this; 3301 } 3302 3303 /** 3304 * @return {@link #blob} (Actual certificate.). This is the underlying object with id, value and extensions. The accessor "getBlob" gives direct access to the value 3305 */ 3306 public Base64BinaryType getBlobElement() { 3307 if (this.blob == null) 3308 if (Configuration.errorOnAutoCreate()) 3309 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityCertificateComponent.blob"); 3310 else if (Configuration.doAutoCreate()) 3311 this.blob = new Base64BinaryType(); // bb 3312 return this.blob; 3313 } 3314 3315 public boolean hasBlobElement() { 3316 return this.blob != null && !this.blob.isEmpty(); 3317 } 3318 3319 public boolean hasBlob() { 3320 return this.blob != null && !this.blob.isEmpty(); 3321 } 3322 3323 /** 3324 * @param value {@link #blob} (Actual certificate.). This is the underlying object with id, value and extensions. The accessor "getBlob" gives direct access to the value 3325 */ 3326 public CapabilityStatementRestSecurityCertificateComponent setBlobElement(Base64BinaryType value) { 3327 this.blob = value; 3328 return this; 3329 } 3330 3331 /** 3332 * @return Actual certificate. 3333 */ 3334 public byte[] getBlob() { 3335 return this.blob == null ? null : this.blob.getValue(); 3336 } 3337 3338 /** 3339 * @param value Actual certificate. 3340 */ 3341 public CapabilityStatementRestSecurityCertificateComponent setBlob(byte[] value) { 3342 if (value == null) 3343 this.blob = null; 3344 else { 3345 if (this.blob == null) 3346 this.blob = new Base64BinaryType(); 3347 this.blob.setValue(value); 3348 } 3349 return this; 3350 } 3351 3352 protected void listChildren(List<Property> children) { 3353 super.listChildren(children); 3354 children.add(new Property("type", "code", "Mime type for a certificate.", 0, 1, type)); 3355 children.add(new Property("blob", "base64Binary", "Actual certificate.", 0, 1, blob)); 3356 } 3357 3358 @Override 3359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3360 switch (_hash) { 3361 case 3575610: /*type*/ return new Property("type", "code", "Mime type for a certificate.", 0, 1, type); 3362 case 3026845: /*blob*/ return new Property("blob", "base64Binary", "Actual certificate.", 0, 1, blob); 3363 default: return super.getNamedProperty(_hash, _name, _checkValid); 3364 } 3365 3366 } 3367 3368 @Override 3369 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3370 switch (hash) { 3371 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 3372 case 3026845: /*blob*/ return this.blob == null ? new Base[0] : new Base[] {this.blob}; // Base64BinaryType 3373 default: return super.getProperty(hash, name, checkValid); 3374 } 3375 3376 } 3377 3378 @Override 3379 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3380 switch (hash) { 3381 case 3575610: // type 3382 this.type = castToCode(value); // CodeType 3383 return value; 3384 case 3026845: // blob 3385 this.blob = castToBase64Binary(value); // Base64BinaryType 3386 return value; 3387 default: return super.setProperty(hash, name, value); 3388 } 3389 3390 } 3391 3392 @Override 3393 public Base setProperty(String name, Base value) throws FHIRException { 3394 if (name.equals("type")) { 3395 this.type = castToCode(value); // CodeType 3396 } else if (name.equals("blob")) { 3397 this.blob = castToBase64Binary(value); // Base64BinaryType 3398 } else 3399 return super.setProperty(name, value); 3400 return value; 3401 } 3402 3403 @Override 3404 public Base makeProperty(int hash, String name) throws FHIRException { 3405 switch (hash) { 3406 case 3575610: return getTypeElement(); 3407 case 3026845: return getBlobElement(); 3408 default: return super.makeProperty(hash, name); 3409 } 3410 3411 } 3412 3413 @Override 3414 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3415 switch (hash) { 3416 case 3575610: /*type*/ return new String[] {"code"}; 3417 case 3026845: /*blob*/ return new String[] {"base64Binary"}; 3418 default: return super.getTypesForProperty(hash, name); 3419 } 3420 3421 } 3422 3423 @Override 3424 public Base addChild(String name) throws FHIRException { 3425 if (name.equals("type")) { 3426 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.type"); 3427 } 3428 else if (name.equals("blob")) { 3429 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.blob"); 3430 } 3431 else 3432 return super.addChild(name); 3433 } 3434 3435 public CapabilityStatementRestSecurityCertificateComponent copy() { 3436 CapabilityStatementRestSecurityCertificateComponent dst = new CapabilityStatementRestSecurityCertificateComponent(); 3437 copyValues(dst); 3438 dst.type = type == null ? null : type.copy(); 3439 dst.blob = blob == null ? null : blob.copy(); 3440 return dst; 3441 } 3442 3443 @Override 3444 public boolean equalsDeep(Base other_) { 3445 if (!super.equalsDeep(other_)) 3446 return false; 3447 if (!(other_ instanceof CapabilityStatementRestSecurityCertificateComponent)) 3448 return false; 3449 CapabilityStatementRestSecurityCertificateComponent o = (CapabilityStatementRestSecurityCertificateComponent) other_; 3450 return compareDeep(type, o.type, true) && compareDeep(blob, o.blob, true); 3451 } 3452 3453 @Override 3454 public boolean equalsShallow(Base other_) { 3455 if (!super.equalsShallow(other_)) 3456 return false; 3457 if (!(other_ instanceof CapabilityStatementRestSecurityCertificateComponent)) 3458 return false; 3459 CapabilityStatementRestSecurityCertificateComponent o = (CapabilityStatementRestSecurityCertificateComponent) other_; 3460 return compareValues(type, o.type, true) && compareValues(blob, o.blob, true); 3461 } 3462 3463 public boolean isEmpty() { 3464 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, blob); 3465 } 3466 3467 public String fhirType() { 3468 return "CapabilityStatement.rest.security.certificate"; 3469 3470 } 3471 3472 } 3473 3474 @Block() 3475 public static class CapabilityStatementRestResourceComponent extends BackboneElement implements IBaseBackboneElement { 3476 /** 3477 * A type of resource exposed via the restful interface. 3478 */ 3479 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3480 @Description(shortDefinition="A resource type that is supported", formalDefinition="A type of resource exposed via the restful interface." ) 3481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 3482 protected CodeType type; 3483 3484 /** 3485 * A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses). 3486 */ 3487 @Child(name = "profile", type = {StructureDefinition.class}, order=2, min=0, max=1, modifier=false, summary=true) 3488 @Description(shortDefinition="Base System profile for all uses of resource", formalDefinition="A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses)." ) 3489 protected Reference profile; 3490 3491 /** 3492 * The actual object that is the target of the reference (A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3493 */ 3494 protected StructureDefinition profileTarget; 3495 3496 /** 3497 * Additional information about the resource type used by the system. 3498 */ 3499 @Child(name = "documentation", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3500 @Description(shortDefinition="Additional information about the use of the resource type", formalDefinition="Additional information about the resource type used by the system." ) 3501 protected MarkdownType documentation; 3502 3503 /** 3504 * Identifies a restful operation supported by the solution. 3505 */ 3506 @Child(name = "interaction", type = {}, order=4, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3507 @Description(shortDefinition="What operations are supported?", formalDefinition="Identifies a restful operation supported by the solution." ) 3508 protected List<ResourceInteractionComponent> interaction; 3509 3510 /** 3511 * This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API. 3512 */ 3513 @Child(name = "versioning", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 3514 @Description(shortDefinition="no-version | versioned | versioned-update", formalDefinition="This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API." ) 3515 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/versioning-policy") 3516 protected Enumeration<ResourceVersionPolicy> versioning; 3517 3518 /** 3519 * A flag for whether the server is able to return past versions as part of the vRead operation. 3520 */ 3521 @Child(name = "readHistory", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=false) 3522 @Description(shortDefinition="Whether vRead can return past versions", formalDefinition="A flag for whether the server is able to return past versions as part of the vRead operation." ) 3523 protected BooleanType readHistory; 3524 3525 /** 3526 * A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server. 3527 */ 3528 @Child(name = "updateCreate", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=false) 3529 @Description(shortDefinition="If update can commit to a new identity", formalDefinition="A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server." ) 3530 protected BooleanType updateCreate; 3531 3532 /** 3533 * A flag that indicates that the server supports conditional create. 3534 */ 3535 @Child(name = "conditionalCreate", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=false) 3536 @Description(shortDefinition="If allows/uses conditional create", formalDefinition="A flag that indicates that the server supports conditional create." ) 3537 protected BooleanType conditionalCreate; 3538 3539 /** 3540 * A code that indicates how the server supports conditional read. 3541 */ 3542 @Child(name = "conditionalRead", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 3543 @Description(shortDefinition="not-supported | modified-since | not-match | full-support", formalDefinition="A code that indicates how the server supports conditional read." ) 3544 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conditional-read-status") 3545 protected Enumeration<ConditionalReadStatus> conditionalRead; 3546 3547 /** 3548 * A flag that indicates that the server supports conditional update. 3549 */ 3550 @Child(name = "conditionalUpdate", type = {BooleanType.class}, order=10, min=0, max=1, modifier=false, summary=false) 3551 @Description(shortDefinition="If allows/uses conditional update", formalDefinition="A flag that indicates that the server supports conditional update." ) 3552 protected BooleanType conditionalUpdate; 3553 3554 /** 3555 * A code that indicates how the server supports conditional delete. 3556 */ 3557 @Child(name = "conditionalDelete", type = {CodeType.class}, order=11, min=0, max=1, modifier=false, summary=false) 3558 @Description(shortDefinition="not-supported | single | multiple - how conditional delete is supported", formalDefinition="A code that indicates how the server supports conditional delete." ) 3559 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conditional-delete-status") 3560 protected Enumeration<ConditionalDeleteStatus> conditionalDelete; 3561 3562 /** 3563 * A set of flags that defines how references are supported. 3564 */ 3565 @Child(name = "referencePolicy", type = {CodeType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3566 @Description(shortDefinition="literal | logical | resolves | enforced | local", formalDefinition="A set of flags that defines how references are supported." ) 3567 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reference-handling-policy") 3568 protected List<Enumeration<ReferenceHandlingPolicy>> referencePolicy; 3569 3570 /** 3571 * A list of _include values supported by the server. 3572 */ 3573 @Child(name = "searchInclude", type = {StringType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3574 @Description(shortDefinition="_include values supported by the server", formalDefinition="A list of _include values supported by the server." ) 3575 protected List<StringType> searchInclude; 3576 3577 /** 3578 * A list of _revinclude (reverse include) values supported by the server. 3579 */ 3580 @Child(name = "searchRevInclude", type = {StringType.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3581 @Description(shortDefinition="_revinclude values supported by the server", formalDefinition="A list of _revinclude (reverse include) values supported by the server." ) 3582 protected List<StringType> searchRevInclude; 3583 3584 /** 3585 * Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. 3586 */ 3587 @Child(name = "searchParam", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3588 @Description(shortDefinition="Search parameters supported by implementation", formalDefinition="Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation." ) 3589 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 3590 3591 private static final long serialVersionUID = 1271233297L; 3592 3593 /** 3594 * Constructor 3595 */ 3596 public CapabilityStatementRestResourceComponent() { 3597 super(); 3598 } 3599 3600 /** 3601 * Constructor 3602 */ 3603 public CapabilityStatementRestResourceComponent(CodeType type) { 3604 super(); 3605 this.type = type; 3606 } 3607 3608 /** 3609 * @return {@link #type} (A type of resource exposed via the restful interface.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3610 */ 3611 public CodeType getTypeElement() { 3612 if (this.type == null) 3613 if (Configuration.errorOnAutoCreate()) 3614 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.type"); 3615 else if (Configuration.doAutoCreate()) 3616 this.type = new CodeType(); // bb 3617 return this.type; 3618 } 3619 3620 public boolean hasTypeElement() { 3621 return this.type != null && !this.type.isEmpty(); 3622 } 3623 3624 public boolean hasType() { 3625 return this.type != null && !this.type.isEmpty(); 3626 } 3627 3628 /** 3629 * @param value {@link #type} (A type of resource exposed via the restful interface.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3630 */ 3631 public CapabilityStatementRestResourceComponent setTypeElement(CodeType value) { 3632 this.type = value; 3633 return this; 3634 } 3635 3636 /** 3637 * @return A type of resource exposed via the restful interface. 3638 */ 3639 public String getType() { 3640 return this.type == null ? null : this.type.getValue(); 3641 } 3642 3643 /** 3644 * @param value A type of resource exposed via the restful interface. 3645 */ 3646 public CapabilityStatementRestResourceComponent setType(String value) { 3647 if (this.type == null) 3648 this.type = new CodeType(); 3649 this.type.setValue(value); 3650 return this; 3651 } 3652 3653 /** 3654 * @return {@link #profile} (A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3655 */ 3656 public Reference getProfile() { 3657 if (this.profile == null) 3658 if (Configuration.errorOnAutoCreate()) 3659 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.profile"); 3660 else if (Configuration.doAutoCreate()) 3661 this.profile = new Reference(); // cc 3662 return this.profile; 3663 } 3664 3665 public boolean hasProfile() { 3666 return this.profile != null && !this.profile.isEmpty(); 3667 } 3668 3669 /** 3670 * @param value {@link #profile} (A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3671 */ 3672 public CapabilityStatementRestResourceComponent setProfile(Reference value) { 3673 this.profile = value; 3674 return this; 3675 } 3676 3677 /** 3678 * @return {@link #profile} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3679 */ 3680 public StructureDefinition getProfileTarget() { 3681 if (this.profileTarget == null) 3682 if (Configuration.errorOnAutoCreate()) 3683 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.profile"); 3684 else if (Configuration.doAutoCreate()) 3685 this.profileTarget = new StructureDefinition(); // aa 3686 return this.profileTarget; 3687 } 3688 3689 /** 3690 * @param value {@link #profile} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3691 */ 3692 public CapabilityStatementRestResourceComponent setProfileTarget(StructureDefinition value) { 3693 this.profileTarget = value; 3694 return this; 3695 } 3696 3697 /** 3698 * @return {@link #documentation} (Additional information about the resource type used by the system.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 3699 */ 3700 public MarkdownType getDocumentationElement() { 3701 if (this.documentation == null) 3702 if (Configuration.errorOnAutoCreate()) 3703 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.documentation"); 3704 else if (Configuration.doAutoCreate()) 3705 this.documentation = new MarkdownType(); // bb 3706 return this.documentation; 3707 } 3708 3709 public boolean hasDocumentationElement() { 3710 return this.documentation != null && !this.documentation.isEmpty(); 3711 } 3712 3713 public boolean hasDocumentation() { 3714 return this.documentation != null && !this.documentation.isEmpty(); 3715 } 3716 3717 /** 3718 * @param value {@link #documentation} (Additional information about the resource type used by the system.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 3719 */ 3720 public CapabilityStatementRestResourceComponent setDocumentationElement(MarkdownType value) { 3721 this.documentation = value; 3722 return this; 3723 } 3724 3725 /** 3726 * @return Additional information about the resource type used by the system. 3727 */ 3728 public String getDocumentation() { 3729 return this.documentation == null ? null : this.documentation.getValue(); 3730 } 3731 3732 /** 3733 * @param value Additional information about the resource type used by the system. 3734 */ 3735 public CapabilityStatementRestResourceComponent setDocumentation(String value) { 3736 if (value == null) 3737 this.documentation = null; 3738 else { 3739 if (this.documentation == null) 3740 this.documentation = new MarkdownType(); 3741 this.documentation.setValue(value); 3742 } 3743 return this; 3744 } 3745 3746 /** 3747 * @return {@link #interaction} (Identifies a restful operation supported by the solution.) 3748 */ 3749 public List<ResourceInteractionComponent> getInteraction() { 3750 if (this.interaction == null) 3751 this.interaction = new ArrayList<ResourceInteractionComponent>(); 3752 return this.interaction; 3753 } 3754 3755 /** 3756 * @return Returns a reference to <code>this</code> for easy method chaining 3757 */ 3758 public CapabilityStatementRestResourceComponent setInteraction(List<ResourceInteractionComponent> theInteraction) { 3759 this.interaction = theInteraction; 3760 return this; 3761 } 3762 3763 public boolean hasInteraction() { 3764 if (this.interaction == null) 3765 return false; 3766 for (ResourceInteractionComponent item : this.interaction) 3767 if (!item.isEmpty()) 3768 return true; 3769 return false; 3770 } 3771 3772 public ResourceInteractionComponent addInteraction() { //3 3773 ResourceInteractionComponent t = new ResourceInteractionComponent(); 3774 if (this.interaction == null) 3775 this.interaction = new ArrayList<ResourceInteractionComponent>(); 3776 this.interaction.add(t); 3777 return t; 3778 } 3779 3780 public CapabilityStatementRestResourceComponent addInteraction(ResourceInteractionComponent t) { //3 3781 if (t == null) 3782 return this; 3783 if (this.interaction == null) 3784 this.interaction = new ArrayList<ResourceInteractionComponent>(); 3785 this.interaction.add(t); 3786 return this; 3787 } 3788 3789 /** 3790 * @return The first repetition of repeating field {@link #interaction}, creating it if it does not already exist 3791 */ 3792 public ResourceInteractionComponent getInteractionFirstRep() { 3793 if (getInteraction().isEmpty()) { 3794 addInteraction(); 3795 } 3796 return getInteraction().get(0); 3797 } 3798 3799 /** 3800 * @return {@link #versioning} (This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 3801 */ 3802 public Enumeration<ResourceVersionPolicy> getVersioningElement() { 3803 if (this.versioning == null) 3804 if (Configuration.errorOnAutoCreate()) 3805 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.versioning"); 3806 else if (Configuration.doAutoCreate()) 3807 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); // bb 3808 return this.versioning; 3809 } 3810 3811 public boolean hasVersioningElement() { 3812 return this.versioning != null && !this.versioning.isEmpty(); 3813 } 3814 3815 public boolean hasVersioning() { 3816 return this.versioning != null && !this.versioning.isEmpty(); 3817 } 3818 3819 /** 3820 * @param value {@link #versioning} (This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 3821 */ 3822 public CapabilityStatementRestResourceComponent setVersioningElement(Enumeration<ResourceVersionPolicy> value) { 3823 this.versioning = value; 3824 return this; 3825 } 3826 3827 /** 3828 * @return This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API. 3829 */ 3830 public ResourceVersionPolicy getVersioning() { 3831 return this.versioning == null ? null : this.versioning.getValue(); 3832 } 3833 3834 /** 3835 * @param value This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API. 3836 */ 3837 public CapabilityStatementRestResourceComponent setVersioning(ResourceVersionPolicy value) { 3838 if (value == null) 3839 this.versioning = null; 3840 else { 3841 if (this.versioning == null) 3842 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); 3843 this.versioning.setValue(value); 3844 } 3845 return this; 3846 } 3847 3848 /** 3849 * @return {@link #readHistory} (A flag for whether the server is able to return past versions as part of the vRead operation.). This is the underlying object with id, value and extensions. The accessor "getReadHistory" gives direct access to the value 3850 */ 3851 public BooleanType getReadHistoryElement() { 3852 if (this.readHistory == null) 3853 if (Configuration.errorOnAutoCreate()) 3854 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.readHistory"); 3855 else if (Configuration.doAutoCreate()) 3856 this.readHistory = new BooleanType(); // bb 3857 return this.readHistory; 3858 } 3859 3860 public boolean hasReadHistoryElement() { 3861 return this.readHistory != null && !this.readHistory.isEmpty(); 3862 } 3863 3864 public boolean hasReadHistory() { 3865 return this.readHistory != null && !this.readHistory.isEmpty(); 3866 } 3867 3868 /** 3869 * @param value {@link #readHistory} (A flag for whether the server is able to return past versions as part of the vRead operation.). This is the underlying object with id, value and extensions. The accessor "getReadHistory" gives direct access to the value 3870 */ 3871 public CapabilityStatementRestResourceComponent setReadHistoryElement(BooleanType value) { 3872 this.readHistory = value; 3873 return this; 3874 } 3875 3876 /** 3877 * @return A flag for whether the server is able to return past versions as part of the vRead operation. 3878 */ 3879 public boolean getReadHistory() { 3880 return this.readHistory == null || this.readHistory.isEmpty() ? false : this.readHistory.getValue(); 3881 } 3882 3883 /** 3884 * @param value A flag for whether the server is able to return past versions as part of the vRead operation. 3885 */ 3886 public CapabilityStatementRestResourceComponent setReadHistory(boolean value) { 3887 if (this.readHistory == null) 3888 this.readHistory = new BooleanType(); 3889 this.readHistory.setValue(value); 3890 return this; 3891 } 3892 3893 /** 3894 * @return {@link #updateCreate} (A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.). This is the underlying object with id, value and extensions. The accessor "getUpdateCreate" gives direct access to the value 3895 */ 3896 public BooleanType getUpdateCreateElement() { 3897 if (this.updateCreate == null) 3898 if (Configuration.errorOnAutoCreate()) 3899 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.updateCreate"); 3900 else if (Configuration.doAutoCreate()) 3901 this.updateCreate = new BooleanType(); // bb 3902 return this.updateCreate; 3903 } 3904 3905 public boolean hasUpdateCreateElement() { 3906 return this.updateCreate != null && !this.updateCreate.isEmpty(); 3907 } 3908 3909 public boolean hasUpdateCreate() { 3910 return this.updateCreate != null && !this.updateCreate.isEmpty(); 3911 } 3912 3913 /** 3914 * @param value {@link #updateCreate} (A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.). This is the underlying object with id, value and extensions. The accessor "getUpdateCreate" gives direct access to the value 3915 */ 3916 public CapabilityStatementRestResourceComponent setUpdateCreateElement(BooleanType value) { 3917 this.updateCreate = value; 3918 return this; 3919 } 3920 3921 /** 3922 * @return A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server. 3923 */ 3924 public boolean getUpdateCreate() { 3925 return this.updateCreate == null || this.updateCreate.isEmpty() ? false : this.updateCreate.getValue(); 3926 } 3927 3928 /** 3929 * @param value A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server. 3930 */ 3931 public CapabilityStatementRestResourceComponent setUpdateCreate(boolean value) { 3932 if (this.updateCreate == null) 3933 this.updateCreate = new BooleanType(); 3934 this.updateCreate.setValue(value); 3935 return this; 3936 } 3937 3938 /** 3939 * @return {@link #conditionalCreate} (A flag that indicates that the server supports conditional create.). This is the underlying object with id, value and extensions. The accessor "getConditionalCreate" gives direct access to the value 3940 */ 3941 public BooleanType getConditionalCreateElement() { 3942 if (this.conditionalCreate == null) 3943 if (Configuration.errorOnAutoCreate()) 3944 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalCreate"); 3945 else if (Configuration.doAutoCreate()) 3946 this.conditionalCreate = new BooleanType(); // bb 3947 return this.conditionalCreate; 3948 } 3949 3950 public boolean hasConditionalCreateElement() { 3951 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 3952 } 3953 3954 public boolean hasConditionalCreate() { 3955 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 3956 } 3957 3958 /** 3959 * @param value {@link #conditionalCreate} (A flag that indicates that the server supports conditional create.). This is the underlying object with id, value and extensions. The accessor "getConditionalCreate" gives direct access to the value 3960 */ 3961 public CapabilityStatementRestResourceComponent setConditionalCreateElement(BooleanType value) { 3962 this.conditionalCreate = value; 3963 return this; 3964 } 3965 3966 /** 3967 * @return A flag that indicates that the server supports conditional create. 3968 */ 3969 public boolean getConditionalCreate() { 3970 return this.conditionalCreate == null || this.conditionalCreate.isEmpty() ? false : this.conditionalCreate.getValue(); 3971 } 3972 3973 /** 3974 * @param value A flag that indicates that the server supports conditional create. 3975 */ 3976 public CapabilityStatementRestResourceComponent setConditionalCreate(boolean value) { 3977 if (this.conditionalCreate == null) 3978 this.conditionalCreate = new BooleanType(); 3979 this.conditionalCreate.setValue(value); 3980 return this; 3981 } 3982 3983 /** 3984 * @return {@link #conditionalRead} (A code that indicates how the server supports conditional read.). This is the underlying object with id, value and extensions. The accessor "getConditionalRead" gives direct access to the value 3985 */ 3986 public Enumeration<ConditionalReadStatus> getConditionalReadElement() { 3987 if (this.conditionalRead == null) 3988 if (Configuration.errorOnAutoCreate()) 3989 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalRead"); 3990 else if (Configuration.doAutoCreate()) 3991 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); // bb 3992 return this.conditionalRead; 3993 } 3994 3995 public boolean hasConditionalReadElement() { 3996 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 3997 } 3998 3999 public boolean hasConditionalRead() { 4000 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 4001 } 4002 4003 /** 4004 * @param value {@link #conditionalRead} (A code that indicates how the server supports conditional read.). This is the underlying object with id, value and extensions. The accessor "getConditionalRead" gives direct access to the value 4005 */ 4006 public CapabilityStatementRestResourceComponent setConditionalReadElement(Enumeration<ConditionalReadStatus> value) { 4007 this.conditionalRead = value; 4008 return this; 4009 } 4010 4011 /** 4012 * @return A code that indicates how the server supports conditional read. 4013 */ 4014 public ConditionalReadStatus getConditionalRead() { 4015 return this.conditionalRead == null ? null : this.conditionalRead.getValue(); 4016 } 4017 4018 /** 4019 * @param value A code that indicates how the server supports conditional read. 4020 */ 4021 public CapabilityStatementRestResourceComponent setConditionalRead(ConditionalReadStatus value) { 4022 if (value == null) 4023 this.conditionalRead = null; 4024 else { 4025 if (this.conditionalRead == null) 4026 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); 4027 this.conditionalRead.setValue(value); 4028 } 4029 return this; 4030 } 4031 4032 /** 4033 * @return {@link #conditionalUpdate} (A flag that indicates that the server supports conditional update.). This is the underlying object with id, value and extensions. The accessor "getConditionalUpdate" gives direct access to the value 4034 */ 4035 public BooleanType getConditionalUpdateElement() { 4036 if (this.conditionalUpdate == null) 4037 if (Configuration.errorOnAutoCreate()) 4038 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalUpdate"); 4039 else if (Configuration.doAutoCreate()) 4040 this.conditionalUpdate = new BooleanType(); // bb 4041 return this.conditionalUpdate; 4042 } 4043 4044 public boolean hasConditionalUpdateElement() { 4045 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4046 } 4047 4048 public boolean hasConditionalUpdate() { 4049 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4050 } 4051 4052 /** 4053 * @param value {@link #conditionalUpdate} (A flag that indicates that the server supports conditional update.). This is the underlying object with id, value and extensions. The accessor "getConditionalUpdate" gives direct access to the value 4054 */ 4055 public CapabilityStatementRestResourceComponent setConditionalUpdateElement(BooleanType value) { 4056 this.conditionalUpdate = value; 4057 return this; 4058 } 4059 4060 /** 4061 * @return A flag that indicates that the server supports conditional update. 4062 */ 4063 public boolean getConditionalUpdate() { 4064 return this.conditionalUpdate == null || this.conditionalUpdate.isEmpty() ? false : this.conditionalUpdate.getValue(); 4065 } 4066 4067 /** 4068 * @param value A flag that indicates that the server supports conditional update. 4069 */ 4070 public CapabilityStatementRestResourceComponent setConditionalUpdate(boolean value) { 4071 if (this.conditionalUpdate == null) 4072 this.conditionalUpdate = new BooleanType(); 4073 this.conditionalUpdate.setValue(value); 4074 return this; 4075 } 4076 4077 /** 4078 * @return {@link #conditionalDelete} (A code that indicates how the server supports conditional delete.). This is the underlying object with id, value and extensions. The accessor "getConditionalDelete" gives direct access to the value 4079 */ 4080 public Enumeration<ConditionalDeleteStatus> getConditionalDeleteElement() { 4081 if (this.conditionalDelete == null) 4082 if (Configuration.errorOnAutoCreate()) 4083 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalDelete"); 4084 else if (Configuration.doAutoCreate()) 4085 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); // bb 4086 return this.conditionalDelete; 4087 } 4088 4089 public boolean hasConditionalDeleteElement() { 4090 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4091 } 4092 4093 public boolean hasConditionalDelete() { 4094 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4095 } 4096 4097 /** 4098 * @param value {@link #conditionalDelete} (A code that indicates how the server supports conditional delete.). This is the underlying object with id, value and extensions. The accessor "getConditionalDelete" gives direct access to the value 4099 */ 4100 public CapabilityStatementRestResourceComponent setConditionalDeleteElement(Enumeration<ConditionalDeleteStatus> value) { 4101 this.conditionalDelete = value; 4102 return this; 4103 } 4104 4105 /** 4106 * @return A code that indicates how the server supports conditional delete. 4107 */ 4108 public ConditionalDeleteStatus getConditionalDelete() { 4109 return this.conditionalDelete == null ? null : this.conditionalDelete.getValue(); 4110 } 4111 4112 /** 4113 * @param value A code that indicates how the server supports conditional delete. 4114 */ 4115 public CapabilityStatementRestResourceComponent setConditionalDelete(ConditionalDeleteStatus value) { 4116 if (value == null) 4117 this.conditionalDelete = null; 4118 else { 4119 if (this.conditionalDelete == null) 4120 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); 4121 this.conditionalDelete.setValue(value); 4122 } 4123 return this; 4124 } 4125 4126 /** 4127 * @return {@link #referencePolicy} (A set of flags that defines how references are supported.) 4128 */ 4129 public List<Enumeration<ReferenceHandlingPolicy>> getReferencePolicy() { 4130 if (this.referencePolicy == null) 4131 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4132 return this.referencePolicy; 4133 } 4134 4135 /** 4136 * @return Returns a reference to <code>this</code> for easy method chaining 4137 */ 4138 public CapabilityStatementRestResourceComponent setReferencePolicy(List<Enumeration<ReferenceHandlingPolicy>> theReferencePolicy) { 4139 this.referencePolicy = theReferencePolicy; 4140 return this; 4141 } 4142 4143 public boolean hasReferencePolicy() { 4144 if (this.referencePolicy == null) 4145 return false; 4146 for (Enumeration<ReferenceHandlingPolicy> item : this.referencePolicy) 4147 if (!item.isEmpty()) 4148 return true; 4149 return false; 4150 } 4151 4152 /** 4153 * @return {@link #referencePolicy} (A set of flags that defines how references are supported.) 4154 */ 4155 public Enumeration<ReferenceHandlingPolicy> addReferencePolicyElement() {//2 4156 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>(new ReferenceHandlingPolicyEnumFactory()); 4157 if (this.referencePolicy == null) 4158 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4159 this.referencePolicy.add(t); 4160 return t; 4161 } 4162 4163 /** 4164 * @param value {@link #referencePolicy} (A set of flags that defines how references are supported.) 4165 */ 4166 public CapabilityStatementRestResourceComponent addReferencePolicy(ReferenceHandlingPolicy value) { //1 4167 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>(new ReferenceHandlingPolicyEnumFactory()); 4168 t.setValue(value); 4169 if (this.referencePolicy == null) 4170 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4171 this.referencePolicy.add(t); 4172 return this; 4173 } 4174 4175 /** 4176 * @param value {@link #referencePolicy} (A set of flags that defines how references are supported.) 4177 */ 4178 public boolean hasReferencePolicy(ReferenceHandlingPolicy value) { 4179 if (this.referencePolicy == null) 4180 return false; 4181 for (Enumeration<ReferenceHandlingPolicy> v : this.referencePolicy) 4182 if (v.getValue().equals(value)) // code 4183 return true; 4184 return false; 4185 } 4186 4187 /** 4188 * @return {@link #searchInclude} (A list of _include values supported by the server.) 4189 */ 4190 public List<StringType> getSearchInclude() { 4191 if (this.searchInclude == null) 4192 this.searchInclude = new ArrayList<StringType>(); 4193 return this.searchInclude; 4194 } 4195 4196 /** 4197 * @return Returns a reference to <code>this</code> for easy method chaining 4198 */ 4199 public CapabilityStatementRestResourceComponent setSearchInclude(List<StringType> theSearchInclude) { 4200 this.searchInclude = theSearchInclude; 4201 return this; 4202 } 4203 4204 public boolean hasSearchInclude() { 4205 if (this.searchInclude == null) 4206 return false; 4207 for (StringType item : this.searchInclude) 4208 if (!item.isEmpty()) 4209 return true; 4210 return false; 4211 } 4212 4213 /** 4214 * @return {@link #searchInclude} (A list of _include values supported by the server.) 4215 */ 4216 public StringType addSearchIncludeElement() {//2 4217 StringType t = new StringType(); 4218 if (this.searchInclude == null) 4219 this.searchInclude = new ArrayList<StringType>(); 4220 this.searchInclude.add(t); 4221 return t; 4222 } 4223 4224 /** 4225 * @param value {@link #searchInclude} (A list of _include values supported by the server.) 4226 */ 4227 public CapabilityStatementRestResourceComponent addSearchInclude(String value) { //1 4228 StringType t = new StringType(); 4229 t.setValue(value); 4230 if (this.searchInclude == null) 4231 this.searchInclude = new ArrayList<StringType>(); 4232 this.searchInclude.add(t); 4233 return this; 4234 } 4235 4236 /** 4237 * @param value {@link #searchInclude} (A list of _include values supported by the server.) 4238 */ 4239 public boolean hasSearchInclude(String value) { 4240 if (this.searchInclude == null) 4241 return false; 4242 for (StringType v : this.searchInclude) 4243 if (v.getValue().equals(value)) // string 4244 return true; 4245 return false; 4246 } 4247 4248 /** 4249 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 4250 */ 4251 public List<StringType> getSearchRevInclude() { 4252 if (this.searchRevInclude == null) 4253 this.searchRevInclude = new ArrayList<StringType>(); 4254 return this.searchRevInclude; 4255 } 4256 4257 /** 4258 * @return Returns a reference to <code>this</code> for easy method chaining 4259 */ 4260 public CapabilityStatementRestResourceComponent setSearchRevInclude(List<StringType> theSearchRevInclude) { 4261 this.searchRevInclude = theSearchRevInclude; 4262 return this; 4263 } 4264 4265 public boolean hasSearchRevInclude() { 4266 if (this.searchRevInclude == null) 4267 return false; 4268 for (StringType item : this.searchRevInclude) 4269 if (!item.isEmpty()) 4270 return true; 4271 return false; 4272 } 4273 4274 /** 4275 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 4276 */ 4277 public StringType addSearchRevIncludeElement() {//2 4278 StringType t = new StringType(); 4279 if (this.searchRevInclude == null) 4280 this.searchRevInclude = new ArrayList<StringType>(); 4281 this.searchRevInclude.add(t); 4282 return t; 4283 } 4284 4285 /** 4286 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 4287 */ 4288 public CapabilityStatementRestResourceComponent addSearchRevInclude(String value) { //1 4289 StringType t = new StringType(); 4290 t.setValue(value); 4291 if (this.searchRevInclude == null) 4292 this.searchRevInclude = new ArrayList<StringType>(); 4293 this.searchRevInclude.add(t); 4294 return this; 4295 } 4296 4297 /** 4298 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 4299 */ 4300 public boolean hasSearchRevInclude(String value) { 4301 if (this.searchRevInclude == null) 4302 return false; 4303 for (StringType v : this.searchRevInclude) 4304 if (v.getValue().equals(value)) // string 4305 return true; 4306 return false; 4307 } 4308 4309 /** 4310 * @return {@link #searchParam} (Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.) 4311 */ 4312 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 4313 if (this.searchParam == null) 4314 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4315 return this.searchParam; 4316 } 4317 4318 /** 4319 * @return Returns a reference to <code>this</code> for easy method chaining 4320 */ 4321 public CapabilityStatementRestResourceComponent setSearchParam(List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 4322 this.searchParam = theSearchParam; 4323 return this; 4324 } 4325 4326 public boolean hasSearchParam() { 4327 if (this.searchParam == null) 4328 return false; 4329 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 4330 if (!item.isEmpty()) 4331 return true; 4332 return false; 4333 } 4334 4335 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { //3 4336 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 4337 if (this.searchParam == null) 4338 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4339 this.searchParam.add(t); 4340 return t; 4341 } 4342 4343 public CapabilityStatementRestResourceComponent addSearchParam(CapabilityStatementRestResourceSearchParamComponent t) { //3 4344 if (t == null) 4345 return this; 4346 if (this.searchParam == null) 4347 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4348 this.searchParam.add(t); 4349 return this; 4350 } 4351 4352 /** 4353 * @return The first repetition of repeating field {@link #searchParam}, creating it if it does not already exist 4354 */ 4355 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 4356 if (getSearchParam().isEmpty()) { 4357 addSearchParam(); 4358 } 4359 return getSearchParam().get(0); 4360 } 4361 4362 protected void listChildren(List<Property> children) { 4363 super.listChildren(children); 4364 children.add(new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, type)); 4365 children.add(new Property("profile", "Reference(StructureDefinition)", "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, 1, profile)); 4366 children.add(new Property("documentation", "markdown", "Additional information about the resource type used by the system.", 0, 1, documentation)); 4367 children.add(new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, java.lang.Integer.MAX_VALUE, interaction)); 4368 children.add(new Property("versioning", "code", "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 0, 1, versioning)); 4369 children.add(new Property("readHistory", "boolean", "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, readHistory)); 4370 children.add(new Property("updateCreate", "boolean", "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 0, 1, updateCreate)); 4371 children.add(new Property("conditionalCreate", "boolean", "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate)); 4372 children.add(new Property("conditionalRead", "code", "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead)); 4373 children.add(new Property("conditionalUpdate", "boolean", "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate)); 4374 children.add(new Property("conditionalDelete", "code", "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete)); 4375 children.add(new Property("referencePolicy", "code", "A set of flags that defines how references are supported.", 0, java.lang.Integer.MAX_VALUE, referencePolicy)); 4376 children.add(new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchInclude)); 4377 children.add(new Property("searchRevInclude", "string", "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchRevInclude)); 4378 children.add(new Property("searchParam", "", "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 0, java.lang.Integer.MAX_VALUE, searchParam)); 4379 } 4380 4381 @Override 4382 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4383 switch (_hash) { 4384 case 3575610: /*type*/ return new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, type); 4385 case -309425751: /*profile*/ return new Property("profile", "Reference(StructureDefinition)", "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, 1, profile); 4386 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Additional information about the resource type used by the system.", 0, 1, documentation); 4387 case 1844104722: /*interaction*/ return new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, java.lang.Integer.MAX_VALUE, interaction); 4388 case -670487542: /*versioning*/ return new Property("versioning", "code", "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 0, 1, versioning); 4389 case 187518494: /*readHistory*/ return new Property("readHistory", "boolean", "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, readHistory); 4390 case -1400550619: /*updateCreate*/ return new Property("updateCreate", "boolean", "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 0, 1, updateCreate); 4391 case 6401826: /*conditionalCreate*/ return new Property("conditionalCreate", "boolean", "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate); 4392 case 822786364: /*conditionalRead*/ return new Property("conditionalRead", "code", "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead); 4393 case 519849711: /*conditionalUpdate*/ return new Property("conditionalUpdate", "boolean", "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate); 4394 case 23237585: /*conditionalDelete*/ return new Property("conditionalDelete", "code", "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete); 4395 case 796257373: /*referencePolicy*/ return new Property("referencePolicy", "code", "A set of flags that defines how references are supported.", 0, java.lang.Integer.MAX_VALUE, referencePolicy); 4396 case -1035904544: /*searchInclude*/ return new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchInclude); 4397 case -2123884979: /*searchRevInclude*/ return new Property("searchRevInclude", "string", "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchRevInclude); 4398 case -553645115: /*searchParam*/ return new Property("searchParam", "", "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 0, java.lang.Integer.MAX_VALUE, searchParam); 4399 default: return super.getNamedProperty(_hash, _name, _checkValid); 4400 } 4401 4402 } 4403 4404 @Override 4405 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4406 switch (hash) { 4407 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 4408 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Reference 4409 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 4410 case 1844104722: /*interaction*/ return this.interaction == null ? new Base[0] : this.interaction.toArray(new Base[this.interaction.size()]); // ResourceInteractionComponent 4411 case -670487542: /*versioning*/ return this.versioning == null ? new Base[0] : new Base[] {this.versioning}; // Enumeration<ResourceVersionPolicy> 4412 case 187518494: /*readHistory*/ return this.readHistory == null ? new Base[0] : new Base[] {this.readHistory}; // BooleanType 4413 case -1400550619: /*updateCreate*/ return this.updateCreate == null ? new Base[0] : new Base[] {this.updateCreate}; // BooleanType 4414 case 6401826: /*conditionalCreate*/ return this.conditionalCreate == null ? new Base[0] : new Base[] {this.conditionalCreate}; // BooleanType 4415 case 822786364: /*conditionalRead*/ return this.conditionalRead == null ? new Base[0] : new Base[] {this.conditionalRead}; // Enumeration<ConditionalReadStatus> 4416 case 519849711: /*conditionalUpdate*/ return this.conditionalUpdate == null ? new Base[0] : new Base[] {this.conditionalUpdate}; // BooleanType 4417 case 23237585: /*conditionalDelete*/ return this.conditionalDelete == null ? new Base[0] : new Base[] {this.conditionalDelete}; // Enumeration<ConditionalDeleteStatus> 4418 case 796257373: /*referencePolicy*/ return this.referencePolicy == null ? new Base[0] : this.referencePolicy.toArray(new Base[this.referencePolicy.size()]); // Enumeration<ReferenceHandlingPolicy> 4419 case -1035904544: /*searchInclude*/ return this.searchInclude == null ? new Base[0] : this.searchInclude.toArray(new Base[this.searchInclude.size()]); // StringType 4420 case -2123884979: /*searchRevInclude*/ return this.searchRevInclude == null ? new Base[0] : this.searchRevInclude.toArray(new Base[this.searchRevInclude.size()]); // StringType 4421 case -553645115: /*searchParam*/ return this.searchParam == null ? new Base[0] : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 4422 default: return super.getProperty(hash, name, checkValid); 4423 } 4424 4425 } 4426 4427 @Override 4428 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4429 switch (hash) { 4430 case 3575610: // type 4431 this.type = castToCode(value); // CodeType 4432 return value; 4433 case -309425751: // profile 4434 this.profile = castToReference(value); // Reference 4435 return value; 4436 case 1587405498: // documentation 4437 this.documentation = castToMarkdown(value); // MarkdownType 4438 return value; 4439 case 1844104722: // interaction 4440 this.getInteraction().add((ResourceInteractionComponent) value); // ResourceInteractionComponent 4441 return value; 4442 case -670487542: // versioning 4443 value = new ResourceVersionPolicyEnumFactory().fromType(castToCode(value)); 4444 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 4445 return value; 4446 case 187518494: // readHistory 4447 this.readHistory = castToBoolean(value); // BooleanType 4448 return value; 4449 case -1400550619: // updateCreate 4450 this.updateCreate = castToBoolean(value); // BooleanType 4451 return value; 4452 case 6401826: // conditionalCreate 4453 this.conditionalCreate = castToBoolean(value); // BooleanType 4454 return value; 4455 case 822786364: // conditionalRead 4456 value = new ConditionalReadStatusEnumFactory().fromType(castToCode(value)); 4457 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 4458 return value; 4459 case 519849711: // conditionalUpdate 4460 this.conditionalUpdate = castToBoolean(value); // BooleanType 4461 return value; 4462 case 23237585: // conditionalDelete 4463 value = new ConditionalDeleteStatusEnumFactory().fromType(castToCode(value)); 4464 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 4465 return value; 4466 case 796257373: // referencePolicy 4467 value = new ReferenceHandlingPolicyEnumFactory().fromType(castToCode(value)); 4468 this.getReferencePolicy().add((Enumeration) value); // Enumeration<ReferenceHandlingPolicy> 4469 return value; 4470 case -1035904544: // searchInclude 4471 this.getSearchInclude().add(castToString(value)); // StringType 4472 return value; 4473 case -2123884979: // searchRevInclude 4474 this.getSearchRevInclude().add(castToString(value)); // StringType 4475 return value; 4476 case -553645115: // searchParam 4477 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 4478 return value; 4479 default: return super.setProperty(hash, name, value); 4480 } 4481 4482 } 4483 4484 @Override 4485 public Base setProperty(String name, Base value) throws FHIRException { 4486 if (name.equals("type")) { 4487 this.type = castToCode(value); // CodeType 4488 } else if (name.equals("profile")) { 4489 this.profile = castToReference(value); // Reference 4490 } else if (name.equals("documentation")) { 4491 this.documentation = castToMarkdown(value); // MarkdownType 4492 } else if (name.equals("interaction")) { 4493 this.getInteraction().add((ResourceInteractionComponent) value); 4494 } else if (name.equals("versioning")) { 4495 value = new ResourceVersionPolicyEnumFactory().fromType(castToCode(value)); 4496 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 4497 } else if (name.equals("readHistory")) { 4498 this.readHistory = castToBoolean(value); // BooleanType 4499 } else if (name.equals("updateCreate")) { 4500 this.updateCreate = castToBoolean(value); // BooleanType 4501 } else if (name.equals("conditionalCreate")) { 4502 this.conditionalCreate = castToBoolean(value); // BooleanType 4503 } else if (name.equals("conditionalRead")) { 4504 value = new ConditionalReadStatusEnumFactory().fromType(castToCode(value)); 4505 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 4506 } else if (name.equals("conditionalUpdate")) { 4507 this.conditionalUpdate = castToBoolean(value); // BooleanType 4508 } else if (name.equals("conditionalDelete")) { 4509 value = new ConditionalDeleteStatusEnumFactory().fromType(castToCode(value)); 4510 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 4511 } else if (name.equals("referencePolicy")) { 4512 value = new ReferenceHandlingPolicyEnumFactory().fromType(castToCode(value)); 4513 this.getReferencePolicy().add((Enumeration) value); 4514 } else if (name.equals("searchInclude")) { 4515 this.getSearchInclude().add(castToString(value)); 4516 } else if (name.equals("searchRevInclude")) { 4517 this.getSearchRevInclude().add(castToString(value)); 4518 } else if (name.equals("searchParam")) { 4519 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 4520 } else 4521 return super.setProperty(name, value); 4522 return value; 4523 } 4524 4525 @Override 4526 public Base makeProperty(int hash, String name) throws FHIRException { 4527 switch (hash) { 4528 case 3575610: return getTypeElement(); 4529 case -309425751: return getProfile(); 4530 case 1587405498: return getDocumentationElement(); 4531 case 1844104722: return addInteraction(); 4532 case -670487542: return getVersioningElement(); 4533 case 187518494: return getReadHistoryElement(); 4534 case -1400550619: return getUpdateCreateElement(); 4535 case 6401826: return getConditionalCreateElement(); 4536 case 822786364: return getConditionalReadElement(); 4537 case 519849711: return getConditionalUpdateElement(); 4538 case 23237585: return getConditionalDeleteElement(); 4539 case 796257373: return addReferencePolicyElement(); 4540 case -1035904544: return addSearchIncludeElement(); 4541 case -2123884979: return addSearchRevIncludeElement(); 4542 case -553645115: return addSearchParam(); 4543 default: return super.makeProperty(hash, name); 4544 } 4545 4546 } 4547 4548 @Override 4549 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4550 switch (hash) { 4551 case 3575610: /*type*/ return new String[] {"code"}; 4552 case -309425751: /*profile*/ return new String[] {"Reference"}; 4553 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 4554 case 1844104722: /*interaction*/ return new String[] {}; 4555 case -670487542: /*versioning*/ return new String[] {"code"}; 4556 case 187518494: /*readHistory*/ return new String[] {"boolean"}; 4557 case -1400550619: /*updateCreate*/ return new String[] {"boolean"}; 4558 case 6401826: /*conditionalCreate*/ return new String[] {"boolean"}; 4559 case 822786364: /*conditionalRead*/ return new String[] {"code"}; 4560 case 519849711: /*conditionalUpdate*/ return new String[] {"boolean"}; 4561 case 23237585: /*conditionalDelete*/ return new String[] {"code"}; 4562 case 796257373: /*referencePolicy*/ return new String[] {"code"}; 4563 case -1035904544: /*searchInclude*/ return new String[] {"string"}; 4564 case -2123884979: /*searchRevInclude*/ return new String[] {"string"}; 4565 case -553645115: /*searchParam*/ return new String[] {}; 4566 default: return super.getTypesForProperty(hash, name); 4567 } 4568 4569 } 4570 4571 @Override 4572 public Base addChild(String name) throws FHIRException { 4573 if (name.equals("type")) { 4574 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.type"); 4575 } 4576 else if (name.equals("profile")) { 4577 this.profile = new Reference(); 4578 return this.profile; 4579 } 4580 else if (name.equals("documentation")) { 4581 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 4582 } 4583 else if (name.equals("interaction")) { 4584 return addInteraction(); 4585 } 4586 else if (name.equals("versioning")) { 4587 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.versioning"); 4588 } 4589 else if (name.equals("readHistory")) { 4590 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.readHistory"); 4591 } 4592 else if (name.equals("updateCreate")) { 4593 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.updateCreate"); 4594 } 4595 else if (name.equals("conditionalCreate")) { 4596 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalCreate"); 4597 } 4598 else if (name.equals("conditionalRead")) { 4599 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalRead"); 4600 } 4601 else if (name.equals("conditionalUpdate")) { 4602 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalUpdate"); 4603 } 4604 else if (name.equals("conditionalDelete")) { 4605 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalDelete"); 4606 } 4607 else if (name.equals("referencePolicy")) { 4608 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.referencePolicy"); 4609 } 4610 else if (name.equals("searchInclude")) { 4611 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.searchInclude"); 4612 } 4613 else if (name.equals("searchRevInclude")) { 4614 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.searchRevInclude"); 4615 } 4616 else if (name.equals("searchParam")) { 4617 return addSearchParam(); 4618 } 4619 else 4620 return super.addChild(name); 4621 } 4622 4623 public CapabilityStatementRestResourceComponent copy() { 4624 CapabilityStatementRestResourceComponent dst = new CapabilityStatementRestResourceComponent(); 4625 copyValues(dst); 4626 dst.type = type == null ? null : type.copy(); 4627 dst.profile = profile == null ? null : profile.copy(); 4628 dst.documentation = documentation == null ? null : documentation.copy(); 4629 if (interaction != null) { 4630 dst.interaction = new ArrayList<ResourceInteractionComponent>(); 4631 for (ResourceInteractionComponent i : interaction) 4632 dst.interaction.add(i.copy()); 4633 }; 4634 dst.versioning = versioning == null ? null : versioning.copy(); 4635 dst.readHistory = readHistory == null ? null : readHistory.copy(); 4636 dst.updateCreate = updateCreate == null ? null : updateCreate.copy(); 4637 dst.conditionalCreate = conditionalCreate == null ? null : conditionalCreate.copy(); 4638 dst.conditionalRead = conditionalRead == null ? null : conditionalRead.copy(); 4639 dst.conditionalUpdate = conditionalUpdate == null ? null : conditionalUpdate.copy(); 4640 dst.conditionalDelete = conditionalDelete == null ? null : conditionalDelete.copy(); 4641 if (referencePolicy != null) { 4642 dst.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4643 for (Enumeration<ReferenceHandlingPolicy> i : referencePolicy) 4644 dst.referencePolicy.add(i.copy()); 4645 }; 4646 if (searchInclude != null) { 4647 dst.searchInclude = new ArrayList<StringType>(); 4648 for (StringType i : searchInclude) 4649 dst.searchInclude.add(i.copy()); 4650 }; 4651 if (searchRevInclude != null) { 4652 dst.searchRevInclude = new ArrayList<StringType>(); 4653 for (StringType i : searchRevInclude) 4654 dst.searchRevInclude.add(i.copy()); 4655 }; 4656 if (searchParam != null) { 4657 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4658 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 4659 dst.searchParam.add(i.copy()); 4660 }; 4661 return dst; 4662 } 4663 4664 @Override 4665 public boolean equalsDeep(Base other_) { 4666 if (!super.equalsDeep(other_)) 4667 return false; 4668 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 4669 return false; 4670 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 4671 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) && compareDeep(documentation, o.documentation, true) 4672 && compareDeep(interaction, o.interaction, true) && compareDeep(versioning, o.versioning, true) 4673 && compareDeep(readHistory, o.readHistory, true) && compareDeep(updateCreate, o.updateCreate, true) 4674 && compareDeep(conditionalCreate, o.conditionalCreate, true) && compareDeep(conditionalRead, o.conditionalRead, true) 4675 && compareDeep(conditionalUpdate, o.conditionalUpdate, true) && compareDeep(conditionalDelete, o.conditionalDelete, true) 4676 && compareDeep(referencePolicy, o.referencePolicy, true) && compareDeep(searchInclude, o.searchInclude, true) 4677 && compareDeep(searchRevInclude, o.searchRevInclude, true) && compareDeep(searchParam, o.searchParam, true) 4678 ; 4679 } 4680 4681 @Override 4682 public boolean equalsShallow(Base other_) { 4683 if (!super.equalsShallow(other_)) 4684 return false; 4685 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 4686 return false; 4687 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 4688 return compareValues(type, o.type, true) && compareValues(documentation, o.documentation, true) && compareValues(versioning, o.versioning, true) 4689 && compareValues(readHistory, o.readHistory, true) && compareValues(updateCreate, o.updateCreate, true) 4690 && compareValues(conditionalCreate, o.conditionalCreate, true) && compareValues(conditionalRead, o.conditionalRead, true) 4691 && compareValues(conditionalUpdate, o.conditionalUpdate, true) && compareValues(conditionalDelete, o.conditionalDelete, true) 4692 && compareValues(referencePolicy, o.referencePolicy, true) && compareValues(searchInclude, o.searchInclude, true) 4693 && compareValues(searchRevInclude, o.searchRevInclude, true); 4694 } 4695 4696 public boolean isEmpty() { 4697 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, documentation 4698 , interaction, versioning, readHistory, updateCreate, conditionalCreate, conditionalRead 4699 , conditionalUpdate, conditionalDelete, referencePolicy, searchInclude, searchRevInclude 4700 , searchParam); 4701 } 4702 4703 public String fhirType() { 4704 return "CapabilityStatement.rest.resource"; 4705 4706 } 4707 4708 } 4709 4710 @Block() 4711 public static class ResourceInteractionComponent extends BackboneElement implements IBaseBackboneElement { 4712 /** 4713 * Coded identifier of the operation, supported by the system resource. 4714 */ 4715 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4716 @Description(shortDefinition="read | vread | update | patch | delete | history-instance | history-type | create | search-type", formalDefinition="Coded identifier of the operation, supported by the system resource." ) 4717 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/type-restful-interaction") 4718 protected Enumeration<TypeRestfulInteraction> code; 4719 4720 /** 4721 * Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'. 4722 */ 4723 @Child(name = "documentation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4724 @Description(shortDefinition="Anything special about operation behavior", formalDefinition="Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'." ) 4725 protected StringType documentation; 4726 4727 private static final long serialVersionUID = -437507806L; 4728 4729 /** 4730 * Constructor 4731 */ 4732 public ResourceInteractionComponent() { 4733 super(); 4734 } 4735 4736 /** 4737 * Constructor 4738 */ 4739 public ResourceInteractionComponent(Enumeration<TypeRestfulInteraction> code) { 4740 super(); 4741 this.code = code; 4742 } 4743 4744 /** 4745 * @return {@link #code} (Coded identifier of the operation, supported by the system resource.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4746 */ 4747 public Enumeration<TypeRestfulInteraction> getCodeElement() { 4748 if (this.code == null) 4749 if (Configuration.errorOnAutoCreate()) 4750 throw new Error("Attempt to auto-create ResourceInteractionComponent.code"); 4751 else if (Configuration.doAutoCreate()) 4752 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); // bb 4753 return this.code; 4754 } 4755 4756 public boolean hasCodeElement() { 4757 return this.code != null && !this.code.isEmpty(); 4758 } 4759 4760 public boolean hasCode() { 4761 return this.code != null && !this.code.isEmpty(); 4762 } 4763 4764 /** 4765 * @param value {@link #code} (Coded identifier of the operation, supported by the system resource.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4766 */ 4767 public ResourceInteractionComponent setCodeElement(Enumeration<TypeRestfulInteraction> value) { 4768 this.code = value; 4769 return this; 4770 } 4771 4772 /** 4773 * @return Coded identifier of the operation, supported by the system resource. 4774 */ 4775 public TypeRestfulInteraction getCode() { 4776 return this.code == null ? null : this.code.getValue(); 4777 } 4778 4779 /** 4780 * @param value Coded identifier of the operation, supported by the system resource. 4781 */ 4782 public ResourceInteractionComponent setCode(TypeRestfulInteraction value) { 4783 if (this.code == null) 4784 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); 4785 this.code.setValue(value); 4786 return this; 4787 } 4788 4789 /** 4790 * @return {@link #documentation} (Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 4791 */ 4792 public StringType getDocumentationElement() { 4793 if (this.documentation == null) 4794 if (Configuration.errorOnAutoCreate()) 4795 throw new Error("Attempt to auto-create ResourceInteractionComponent.documentation"); 4796 else if (Configuration.doAutoCreate()) 4797 this.documentation = new StringType(); // bb 4798 return this.documentation; 4799 } 4800 4801 public boolean hasDocumentationElement() { 4802 return this.documentation != null && !this.documentation.isEmpty(); 4803 } 4804 4805 public boolean hasDocumentation() { 4806 return this.documentation != null && !this.documentation.isEmpty(); 4807 } 4808 4809 /** 4810 * @param value {@link #documentation} (Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 4811 */ 4812 public ResourceInteractionComponent setDocumentationElement(StringType value) { 4813 this.documentation = value; 4814 return this; 4815 } 4816 4817 /** 4818 * @return Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'. 4819 */ 4820 public String getDocumentation() { 4821 return this.documentation == null ? null : this.documentation.getValue(); 4822 } 4823 4824 /** 4825 * @param value Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'. 4826 */ 4827 public ResourceInteractionComponent setDocumentation(String value) { 4828 if (Utilities.noString(value)) 4829 this.documentation = null; 4830 else { 4831 if (this.documentation == null) 4832 this.documentation = new StringType(); 4833 this.documentation.setValue(value); 4834 } 4835 return this; 4836 } 4837 4838 protected void listChildren(List<Property> children) { 4839 super.listChildren(children); 4840 children.add(new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 0, 1, code)); 4841 children.add(new Property("documentation", "string", "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 0, 1, documentation)); 4842 } 4843 4844 @Override 4845 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4846 switch (_hash) { 4847 case 3059181: /*code*/ return new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 0, 1, code); 4848 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 0, 1, documentation); 4849 default: return super.getNamedProperty(_hash, _name, _checkValid); 4850 } 4851 4852 } 4853 4854 @Override 4855 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4856 switch (hash) { 4857 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<TypeRestfulInteraction> 4858 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 4859 default: return super.getProperty(hash, name, checkValid); 4860 } 4861 4862 } 4863 4864 @Override 4865 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4866 switch (hash) { 4867 case 3059181: // code 4868 value = new TypeRestfulInteractionEnumFactory().fromType(castToCode(value)); 4869 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 4870 return value; 4871 case 1587405498: // documentation 4872 this.documentation = castToString(value); // StringType 4873 return value; 4874 default: return super.setProperty(hash, name, value); 4875 } 4876 4877 } 4878 4879 @Override 4880 public Base setProperty(String name, Base value) throws FHIRException { 4881 if (name.equals("code")) { 4882 value = new TypeRestfulInteractionEnumFactory().fromType(castToCode(value)); 4883 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 4884 } else if (name.equals("documentation")) { 4885 this.documentation = castToString(value); // StringType 4886 } else 4887 return super.setProperty(name, value); 4888 return value; 4889 } 4890 4891 @Override 4892 public Base makeProperty(int hash, String name) throws FHIRException { 4893 switch (hash) { 4894 case 3059181: return getCodeElement(); 4895 case 1587405498: return getDocumentationElement(); 4896 default: return super.makeProperty(hash, name); 4897 } 4898 4899 } 4900 4901 @Override 4902 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4903 switch (hash) { 4904 case 3059181: /*code*/ return new String[] {"code"}; 4905 case 1587405498: /*documentation*/ return new String[] {"string"}; 4906 default: return super.getTypesForProperty(hash, name); 4907 } 4908 4909 } 4910 4911 @Override 4912 public Base addChild(String name) throws FHIRException { 4913 if (name.equals("code")) { 4914 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.code"); 4915 } 4916 else if (name.equals("documentation")) { 4917 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 4918 } 4919 else 4920 return super.addChild(name); 4921 } 4922 4923 public ResourceInteractionComponent copy() { 4924 ResourceInteractionComponent dst = new ResourceInteractionComponent(); 4925 copyValues(dst); 4926 dst.code = code == null ? null : code.copy(); 4927 dst.documentation = documentation == null ? null : documentation.copy(); 4928 return dst; 4929 } 4930 4931 @Override 4932 public boolean equalsDeep(Base other_) { 4933 if (!super.equalsDeep(other_)) 4934 return false; 4935 if (!(other_ instanceof ResourceInteractionComponent)) 4936 return false; 4937 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 4938 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 4939 } 4940 4941 @Override 4942 public boolean equalsShallow(Base other_) { 4943 if (!super.equalsShallow(other_)) 4944 return false; 4945 if (!(other_ instanceof ResourceInteractionComponent)) 4946 return false; 4947 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 4948 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 4949 } 4950 4951 public boolean isEmpty() { 4952 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 4953 } 4954 4955 public String fhirType() { 4956 return "CapabilityStatement.rest.resource.interaction"; 4957 4958 } 4959 4960 } 4961 4962 @Block() 4963 public static class CapabilityStatementRestResourceSearchParamComponent extends BackboneElement implements IBaseBackboneElement { 4964 /** 4965 * The name of the search parameter used in the interface. 4966 */ 4967 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4968 @Description(shortDefinition="Name of search parameter", formalDefinition="The name of the search parameter used in the interface." ) 4969 protected StringType name; 4970 4971 /** 4972 * An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). 4973 */ 4974 @Child(name = "definition", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4975 @Description(shortDefinition="Source of definition for parameter", formalDefinition="An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]])." ) 4976 protected UriType definition; 4977 4978 /** 4979 * The type of value a search parameter refers to, and how the content is interpreted. 4980 */ 4981 @Child(name = "type", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 4982 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri", formalDefinition="The type of value a search parameter refers to, and how the content is interpreted." ) 4983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 4984 protected Enumeration<SearchParamType> type; 4985 4986 /** 4987 * This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms. 4988 */ 4989 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4990 @Description(shortDefinition="Server-specific usage", formalDefinition="This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms." ) 4991 protected StringType documentation; 4992 4993 private static final long serialVersionUID = 72133006L; 4994 4995 /** 4996 * Constructor 4997 */ 4998 public CapabilityStatementRestResourceSearchParamComponent() { 4999 super(); 5000 } 5001 5002 /** 5003 * Constructor 5004 */ 5005 public CapabilityStatementRestResourceSearchParamComponent(StringType name, Enumeration<SearchParamType> type) { 5006 super(); 5007 this.name = name; 5008 this.type = type; 5009 } 5010 5011 /** 5012 * @return {@link #name} (The name of the search parameter used in the interface.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5013 */ 5014 public StringType getNameElement() { 5015 if (this.name == null) 5016 if (Configuration.errorOnAutoCreate()) 5017 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.name"); 5018 else if (Configuration.doAutoCreate()) 5019 this.name = new StringType(); // bb 5020 return this.name; 5021 } 5022 5023 public boolean hasNameElement() { 5024 return this.name != null && !this.name.isEmpty(); 5025 } 5026 5027 public boolean hasName() { 5028 return this.name != null && !this.name.isEmpty(); 5029 } 5030 5031 /** 5032 * @param value {@link #name} (The name of the search parameter used in the interface.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5033 */ 5034 public CapabilityStatementRestResourceSearchParamComponent setNameElement(StringType value) { 5035 this.name = value; 5036 return this; 5037 } 5038 5039 /** 5040 * @return The name of the search parameter used in the interface. 5041 */ 5042 public String getName() { 5043 return this.name == null ? null : this.name.getValue(); 5044 } 5045 5046 /** 5047 * @param value The name of the search parameter used in the interface. 5048 */ 5049 public CapabilityStatementRestResourceSearchParamComponent setName(String value) { 5050 if (this.name == null) 5051 this.name = new StringType(); 5052 this.name.setValue(value); 5053 return this; 5054 } 5055 5056 /** 5057 * @return {@link #definition} (An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 5058 */ 5059 public UriType getDefinitionElement() { 5060 if (this.definition == null) 5061 if (Configuration.errorOnAutoCreate()) 5062 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.definition"); 5063 else if (Configuration.doAutoCreate()) 5064 this.definition = new UriType(); // bb 5065 return this.definition; 5066 } 5067 5068 public boolean hasDefinitionElement() { 5069 return this.definition != null && !this.definition.isEmpty(); 5070 } 5071 5072 public boolean hasDefinition() { 5073 return this.definition != null && !this.definition.isEmpty(); 5074 } 5075 5076 /** 5077 * @param value {@link #definition} (An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 5078 */ 5079 public CapabilityStatementRestResourceSearchParamComponent setDefinitionElement(UriType value) { 5080 this.definition = value; 5081 return this; 5082 } 5083 5084 /** 5085 * @return An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). 5086 */ 5087 public String getDefinition() { 5088 return this.definition == null ? null : this.definition.getValue(); 5089 } 5090 5091 /** 5092 * @param value An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). 5093 */ 5094 public CapabilityStatementRestResourceSearchParamComponent setDefinition(String value) { 5095 if (Utilities.noString(value)) 5096 this.definition = null; 5097 else { 5098 if (this.definition == null) 5099 this.definition = new UriType(); 5100 this.definition.setValue(value); 5101 } 5102 return this; 5103 } 5104 5105 /** 5106 * @return {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 5107 */ 5108 public Enumeration<SearchParamType> getTypeElement() { 5109 if (this.type == null) 5110 if (Configuration.errorOnAutoCreate()) 5111 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.type"); 5112 else if (Configuration.doAutoCreate()) 5113 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 5114 return this.type; 5115 } 5116 5117 public boolean hasTypeElement() { 5118 return this.type != null && !this.type.isEmpty(); 5119 } 5120 5121 public boolean hasType() { 5122 return this.type != null && !this.type.isEmpty(); 5123 } 5124 5125 /** 5126 * @param value {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 5127 */ 5128 public CapabilityStatementRestResourceSearchParamComponent setTypeElement(Enumeration<SearchParamType> value) { 5129 this.type = value; 5130 return this; 5131 } 5132 5133 /** 5134 * @return The type of value a search parameter refers to, and how the content is interpreted. 5135 */ 5136 public SearchParamType getType() { 5137 return this.type == null ? null : this.type.getValue(); 5138 } 5139 5140 /** 5141 * @param value The type of value a search parameter refers to, and how the content is interpreted. 5142 */ 5143 public CapabilityStatementRestResourceSearchParamComponent setType(SearchParamType value) { 5144 if (this.type == null) 5145 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 5146 this.type.setValue(value); 5147 return this; 5148 } 5149 5150 /** 5151 * @return {@link #documentation} (This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5152 */ 5153 public StringType getDocumentationElement() { 5154 if (this.documentation == null) 5155 if (Configuration.errorOnAutoCreate()) 5156 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.documentation"); 5157 else if (Configuration.doAutoCreate()) 5158 this.documentation = new StringType(); // bb 5159 return this.documentation; 5160 } 5161 5162 public boolean hasDocumentationElement() { 5163 return this.documentation != null && !this.documentation.isEmpty(); 5164 } 5165 5166 public boolean hasDocumentation() { 5167 return this.documentation != null && !this.documentation.isEmpty(); 5168 } 5169 5170 /** 5171 * @param value {@link #documentation} (This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5172 */ 5173 public CapabilityStatementRestResourceSearchParamComponent setDocumentationElement(StringType value) { 5174 this.documentation = value; 5175 return this; 5176 } 5177 5178 /** 5179 * @return This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms. 5180 */ 5181 public String getDocumentation() { 5182 return this.documentation == null ? null : this.documentation.getValue(); 5183 } 5184 5185 /** 5186 * @param value This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms. 5187 */ 5188 public CapabilityStatementRestResourceSearchParamComponent setDocumentation(String value) { 5189 if (Utilities.noString(value)) 5190 this.documentation = null; 5191 else { 5192 if (this.documentation == null) 5193 this.documentation = new StringType(); 5194 this.documentation.setValue(value); 5195 } 5196 return this; 5197 } 5198 5199 protected void listChildren(List<Property> children) { 5200 super.listChildren(children); 5201 children.add(new Property("name", "string", "The name of the search parameter used in the interface.", 0, 1, name)); 5202 children.add(new Property("definition", "uri", "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).", 0, 1, definition)); 5203 children.add(new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type)); 5204 children.add(new Property("documentation", "string", "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 0, 1, documentation)); 5205 } 5206 5207 @Override 5208 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5209 switch (_hash) { 5210 case 3373707: /*name*/ return new Property("name", "string", "The name of the search parameter used in the interface.", 0, 1, name); 5211 case -1014418093: /*definition*/ return new Property("definition", "uri", "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).", 0, 1, definition); 5212 case 3575610: /*type*/ return new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type); 5213 case 1587405498: /*documentation*/ return new Property("documentation", "string", "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 0, 1, documentation); 5214 default: return super.getNamedProperty(_hash, _name, _checkValid); 5215 } 5216 5217 } 5218 5219 @Override 5220 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5221 switch (hash) { 5222 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 5223 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // UriType 5224 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SearchParamType> 5225 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 5226 default: return super.getProperty(hash, name, checkValid); 5227 } 5228 5229 } 5230 5231 @Override 5232 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5233 switch (hash) { 5234 case 3373707: // name 5235 this.name = castToString(value); // StringType 5236 return value; 5237 case -1014418093: // definition 5238 this.definition = castToUri(value); // UriType 5239 return value; 5240 case 3575610: // type 5241 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 5242 this.type = (Enumeration) value; // Enumeration<SearchParamType> 5243 return value; 5244 case 1587405498: // documentation 5245 this.documentation = castToString(value); // StringType 5246 return value; 5247 default: return super.setProperty(hash, name, value); 5248 } 5249 5250 } 5251 5252 @Override 5253 public Base setProperty(String name, Base value) throws FHIRException { 5254 if (name.equals("name")) { 5255 this.name = castToString(value); // StringType 5256 } else if (name.equals("definition")) { 5257 this.definition = castToUri(value); // UriType 5258 } else if (name.equals("type")) { 5259 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 5260 this.type = (Enumeration) value; // Enumeration<SearchParamType> 5261 } else if (name.equals("documentation")) { 5262 this.documentation = castToString(value); // StringType 5263 } else 5264 return super.setProperty(name, value); 5265 return value; 5266 } 5267 5268 @Override 5269 public Base makeProperty(int hash, String name) throws FHIRException { 5270 switch (hash) { 5271 case 3373707: return getNameElement(); 5272 case -1014418093: return getDefinitionElement(); 5273 case 3575610: return getTypeElement(); 5274 case 1587405498: return getDocumentationElement(); 5275 default: return super.makeProperty(hash, name); 5276 } 5277 5278 } 5279 5280 @Override 5281 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5282 switch (hash) { 5283 case 3373707: /*name*/ return new String[] {"string"}; 5284 case -1014418093: /*definition*/ return new String[] {"uri"}; 5285 case 3575610: /*type*/ return new String[] {"code"}; 5286 case 1587405498: /*documentation*/ return new String[] {"string"}; 5287 default: return super.getTypesForProperty(hash, name); 5288 } 5289 5290 } 5291 5292 @Override 5293 public Base addChild(String name) throws FHIRException { 5294 if (name.equals("name")) { 5295 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 5296 } 5297 else if (name.equals("definition")) { 5298 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.definition"); 5299 } 5300 else if (name.equals("type")) { 5301 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.type"); 5302 } 5303 else if (name.equals("documentation")) { 5304 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 5305 } 5306 else 5307 return super.addChild(name); 5308 } 5309 5310 public CapabilityStatementRestResourceSearchParamComponent copy() { 5311 CapabilityStatementRestResourceSearchParamComponent dst = new CapabilityStatementRestResourceSearchParamComponent(); 5312 copyValues(dst); 5313 dst.name = name == null ? null : name.copy(); 5314 dst.definition = definition == null ? null : definition.copy(); 5315 dst.type = type == null ? null : type.copy(); 5316 dst.documentation = documentation == null ? null : documentation.copy(); 5317 return dst; 5318 } 5319 5320 @Override 5321 public boolean equalsDeep(Base other_) { 5322 if (!super.equalsDeep(other_)) 5323 return false; 5324 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 5325 return false; 5326 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 5327 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) && compareDeep(type, o.type, true) 5328 && compareDeep(documentation, o.documentation, true); 5329 } 5330 5331 @Override 5332 public boolean equalsShallow(Base other_) { 5333 if (!super.equalsShallow(other_)) 5334 return false; 5335 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 5336 return false; 5337 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 5338 return compareValues(name, o.name, true) && compareValues(definition, o.definition, true) && compareValues(type, o.type, true) 5339 && compareValues(documentation, o.documentation, true); 5340 } 5341 5342 public boolean isEmpty() { 5343 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition, type, documentation 5344 ); 5345 } 5346 5347 public String fhirType() { 5348 return "CapabilityStatement.rest.resource.searchParam"; 5349 5350 } 5351 5352 } 5353 5354 @Block() 5355 public static class SystemInteractionComponent extends BackboneElement implements IBaseBackboneElement { 5356 /** 5357 * A coded identifier of the operation, supported by the system. 5358 */ 5359 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5360 @Description(shortDefinition="transaction | batch | search-system | history-system", formalDefinition="A coded identifier of the operation, supported by the system." ) 5361 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/system-restful-interaction") 5362 protected Enumeration<SystemRestfulInteraction> code; 5363 5364 /** 5365 * Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented. 5366 */ 5367 @Child(name = "documentation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5368 @Description(shortDefinition="Anything special about operation behavior", formalDefinition="Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented." ) 5369 protected StringType documentation; 5370 5371 private static final long serialVersionUID = 510675287L; 5372 5373 /** 5374 * Constructor 5375 */ 5376 public SystemInteractionComponent() { 5377 super(); 5378 } 5379 5380 /** 5381 * Constructor 5382 */ 5383 public SystemInteractionComponent(Enumeration<SystemRestfulInteraction> code) { 5384 super(); 5385 this.code = code; 5386 } 5387 5388 /** 5389 * @return {@link #code} (A coded identifier of the operation, supported by the system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 5390 */ 5391 public Enumeration<SystemRestfulInteraction> getCodeElement() { 5392 if (this.code == null) 5393 if (Configuration.errorOnAutoCreate()) 5394 throw new Error("Attempt to auto-create SystemInteractionComponent.code"); 5395 else if (Configuration.doAutoCreate()) 5396 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); // bb 5397 return this.code; 5398 } 5399 5400 public boolean hasCodeElement() { 5401 return this.code != null && !this.code.isEmpty(); 5402 } 5403 5404 public boolean hasCode() { 5405 return this.code != null && !this.code.isEmpty(); 5406 } 5407 5408 /** 5409 * @param value {@link #code} (A coded identifier of the operation, supported by the system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 5410 */ 5411 public SystemInteractionComponent setCodeElement(Enumeration<SystemRestfulInteraction> value) { 5412 this.code = value; 5413 return this; 5414 } 5415 5416 /** 5417 * @return A coded identifier of the operation, supported by the system. 5418 */ 5419 public SystemRestfulInteraction getCode() { 5420 return this.code == null ? null : this.code.getValue(); 5421 } 5422 5423 /** 5424 * @param value A coded identifier of the operation, supported by the system. 5425 */ 5426 public SystemInteractionComponent setCode(SystemRestfulInteraction value) { 5427 if (this.code == null) 5428 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); 5429 this.code.setValue(value); 5430 return this; 5431 } 5432 5433 /** 5434 * @return {@link #documentation} (Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5435 */ 5436 public StringType getDocumentationElement() { 5437 if (this.documentation == null) 5438 if (Configuration.errorOnAutoCreate()) 5439 throw new Error("Attempt to auto-create SystemInteractionComponent.documentation"); 5440 else if (Configuration.doAutoCreate()) 5441 this.documentation = new StringType(); // bb 5442 return this.documentation; 5443 } 5444 5445 public boolean hasDocumentationElement() { 5446 return this.documentation != null && !this.documentation.isEmpty(); 5447 } 5448 5449 public boolean hasDocumentation() { 5450 return this.documentation != null && !this.documentation.isEmpty(); 5451 } 5452 5453 /** 5454 * @param value {@link #documentation} (Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5455 */ 5456 public SystemInteractionComponent setDocumentationElement(StringType value) { 5457 this.documentation = value; 5458 return this; 5459 } 5460 5461 /** 5462 * @return Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented. 5463 */ 5464 public String getDocumentation() { 5465 return this.documentation == null ? null : this.documentation.getValue(); 5466 } 5467 5468 /** 5469 * @param value Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented. 5470 */ 5471 public SystemInteractionComponent setDocumentation(String value) { 5472 if (Utilities.noString(value)) 5473 this.documentation = null; 5474 else { 5475 if (this.documentation == null) 5476 this.documentation = new StringType(); 5477 this.documentation.setValue(value); 5478 } 5479 return this; 5480 } 5481 5482 protected void listChildren(List<Property> children) { 5483 super.listChildren(children); 5484 children.add(new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 1, code)); 5485 children.add(new Property("documentation", "string", "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 0, 1, documentation)); 5486 } 5487 5488 @Override 5489 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5490 switch (_hash) { 5491 case 3059181: /*code*/ return new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 1, code); 5492 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 0, 1, documentation); 5493 default: return super.getNamedProperty(_hash, _name, _checkValid); 5494 } 5495 5496 } 5497 5498 @Override 5499 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5500 switch (hash) { 5501 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<SystemRestfulInteraction> 5502 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 5503 default: return super.getProperty(hash, name, checkValid); 5504 } 5505 5506 } 5507 5508 @Override 5509 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5510 switch (hash) { 5511 case 3059181: // code 5512 value = new SystemRestfulInteractionEnumFactory().fromType(castToCode(value)); 5513 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 5514 return value; 5515 case 1587405498: // documentation 5516 this.documentation = castToString(value); // StringType 5517 return value; 5518 default: return super.setProperty(hash, name, value); 5519 } 5520 5521 } 5522 5523 @Override 5524 public Base setProperty(String name, Base value) throws FHIRException { 5525 if (name.equals("code")) { 5526 value = new SystemRestfulInteractionEnumFactory().fromType(castToCode(value)); 5527 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 5528 } else if (name.equals("documentation")) { 5529 this.documentation = castToString(value); // StringType 5530 } else 5531 return super.setProperty(name, value); 5532 return value; 5533 } 5534 5535 @Override 5536 public Base makeProperty(int hash, String name) throws FHIRException { 5537 switch (hash) { 5538 case 3059181: return getCodeElement(); 5539 case 1587405498: return getDocumentationElement(); 5540 default: return super.makeProperty(hash, name); 5541 } 5542 5543 } 5544 5545 @Override 5546 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5547 switch (hash) { 5548 case 3059181: /*code*/ return new String[] {"code"}; 5549 case 1587405498: /*documentation*/ return new String[] {"string"}; 5550 default: return super.getTypesForProperty(hash, name); 5551 } 5552 5553 } 5554 5555 @Override 5556 public Base addChild(String name) throws FHIRException { 5557 if (name.equals("code")) { 5558 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.code"); 5559 } 5560 else if (name.equals("documentation")) { 5561 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 5562 } 5563 else 5564 return super.addChild(name); 5565 } 5566 5567 public SystemInteractionComponent copy() { 5568 SystemInteractionComponent dst = new SystemInteractionComponent(); 5569 copyValues(dst); 5570 dst.code = code == null ? null : code.copy(); 5571 dst.documentation = documentation == null ? null : documentation.copy(); 5572 return dst; 5573 } 5574 5575 @Override 5576 public boolean equalsDeep(Base other_) { 5577 if (!super.equalsDeep(other_)) 5578 return false; 5579 if (!(other_ instanceof SystemInteractionComponent)) 5580 return false; 5581 SystemInteractionComponent o = (SystemInteractionComponent) other_; 5582 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 5583 } 5584 5585 @Override 5586 public boolean equalsShallow(Base other_) { 5587 if (!super.equalsShallow(other_)) 5588 return false; 5589 if (!(other_ instanceof SystemInteractionComponent)) 5590 return false; 5591 SystemInteractionComponent o = (SystemInteractionComponent) other_; 5592 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 5593 } 5594 5595 public boolean isEmpty() { 5596 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 5597 } 5598 5599 public String fhirType() { 5600 return "CapabilityStatement.rest.interaction"; 5601 5602 } 5603 5604 } 5605 5606 @Block() 5607 public static class CapabilityStatementRestOperationComponent extends BackboneElement implements IBaseBackboneElement { 5608 /** 5609 * The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. 5610 */ 5611 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5612 @Description(shortDefinition="Name by which the operation/query is invoked", formalDefinition="The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called." ) 5613 protected StringType name; 5614 5615 /** 5616 * Where the formal definition can be found. 5617 */ 5618 @Child(name = "definition", type = {OperationDefinition.class}, order=2, min=1, max=1, modifier=false, summary=true) 5619 @Description(shortDefinition="The defined operation/query", formalDefinition="Where the formal definition can be found." ) 5620 protected Reference definition; 5621 5622 /** 5623 * The actual object that is the target of the reference (Where the formal definition can be found.) 5624 */ 5625 protected OperationDefinition definitionTarget; 5626 5627 private static final long serialVersionUID = 122107272L; 5628 5629 /** 5630 * Constructor 5631 */ 5632 public CapabilityStatementRestOperationComponent() { 5633 super(); 5634 } 5635 5636 /** 5637 * Constructor 5638 */ 5639 public CapabilityStatementRestOperationComponent(StringType name, Reference definition) { 5640 super(); 5641 this.name = name; 5642 this.definition = definition; 5643 } 5644 5645 /** 5646 * @return {@link #name} (The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5647 */ 5648 public StringType getNameElement() { 5649 if (this.name == null) 5650 if (Configuration.errorOnAutoCreate()) 5651 throw new Error("Attempt to auto-create CapabilityStatementRestOperationComponent.name"); 5652 else if (Configuration.doAutoCreate()) 5653 this.name = new StringType(); // bb 5654 return this.name; 5655 } 5656 5657 public boolean hasNameElement() { 5658 return this.name != null && !this.name.isEmpty(); 5659 } 5660 5661 public boolean hasName() { 5662 return this.name != null && !this.name.isEmpty(); 5663 } 5664 5665 /** 5666 * @param value {@link #name} (The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5667 */ 5668 public CapabilityStatementRestOperationComponent setNameElement(StringType value) { 5669 this.name = value; 5670 return this; 5671 } 5672 5673 /** 5674 * @return The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. 5675 */ 5676 public String getName() { 5677 return this.name == null ? null : this.name.getValue(); 5678 } 5679 5680 /** 5681 * @param value The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. 5682 */ 5683 public CapabilityStatementRestOperationComponent setName(String value) { 5684 if (this.name == null) 5685 this.name = new StringType(); 5686 this.name.setValue(value); 5687 return this; 5688 } 5689 5690 /** 5691 * @return {@link #definition} (Where the formal definition can be found.) 5692 */ 5693 public Reference getDefinition() { 5694 if (this.definition == null) 5695 if (Configuration.errorOnAutoCreate()) 5696 throw new Error("Attempt to auto-create CapabilityStatementRestOperationComponent.definition"); 5697 else if (Configuration.doAutoCreate()) 5698 this.definition = new Reference(); // cc 5699 return this.definition; 5700 } 5701 5702 public boolean hasDefinition() { 5703 return this.definition != null && !this.definition.isEmpty(); 5704 } 5705 5706 /** 5707 * @param value {@link #definition} (Where the formal definition can be found.) 5708 */ 5709 public CapabilityStatementRestOperationComponent setDefinition(Reference value) { 5710 this.definition = value; 5711 return this; 5712 } 5713 5714 /** 5715 * @return {@link #definition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Where the formal definition can be found.) 5716 */ 5717 public OperationDefinition getDefinitionTarget() { 5718 if (this.definitionTarget == null) 5719 if (Configuration.errorOnAutoCreate()) 5720 throw new Error("Attempt to auto-create CapabilityStatementRestOperationComponent.definition"); 5721 else if (Configuration.doAutoCreate()) 5722 this.definitionTarget = new OperationDefinition(); // aa 5723 return this.definitionTarget; 5724 } 5725 5726 /** 5727 * @param value {@link #definition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Where the formal definition can be found.) 5728 */ 5729 public CapabilityStatementRestOperationComponent setDefinitionTarget(OperationDefinition value) { 5730 this.definitionTarget = value; 5731 return this; 5732 } 5733 5734 protected void listChildren(List<Property> children) { 5735 super.listChildren(children); 5736 children.add(new Property("name", "string", "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.", 0, 1, name)); 5737 children.add(new Property("definition", "Reference(OperationDefinition)", "Where the formal definition can be found.", 0, 1, definition)); 5738 } 5739 5740 @Override 5741 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5742 switch (_hash) { 5743 case 3373707: /*name*/ return new Property("name", "string", "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.", 0, 1, name); 5744 case -1014418093: /*definition*/ return new Property("definition", "Reference(OperationDefinition)", "Where the formal definition can be found.", 0, 1, definition); 5745 default: return super.getNamedProperty(_hash, _name, _checkValid); 5746 } 5747 5748 } 5749 5750 @Override 5751 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5752 switch (hash) { 5753 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 5754 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Reference 5755 default: return super.getProperty(hash, name, checkValid); 5756 } 5757 5758 } 5759 5760 @Override 5761 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5762 switch (hash) { 5763 case 3373707: // name 5764 this.name = castToString(value); // StringType 5765 return value; 5766 case -1014418093: // definition 5767 this.definition = castToReference(value); // Reference 5768 return value; 5769 default: return super.setProperty(hash, name, value); 5770 } 5771 5772 } 5773 5774 @Override 5775 public Base setProperty(String name, Base value) throws FHIRException { 5776 if (name.equals("name")) { 5777 this.name = castToString(value); // StringType 5778 } else if (name.equals("definition")) { 5779 this.definition = castToReference(value); // Reference 5780 } else 5781 return super.setProperty(name, value); 5782 return value; 5783 } 5784 5785 @Override 5786 public Base makeProperty(int hash, String name) throws FHIRException { 5787 switch (hash) { 5788 case 3373707: return getNameElement(); 5789 case -1014418093: return getDefinition(); 5790 default: return super.makeProperty(hash, name); 5791 } 5792 5793 } 5794 5795 @Override 5796 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5797 switch (hash) { 5798 case 3373707: /*name*/ return new String[] {"string"}; 5799 case -1014418093: /*definition*/ return new String[] {"Reference"}; 5800 default: return super.getTypesForProperty(hash, name); 5801 } 5802 5803 } 5804 5805 @Override 5806 public Base addChild(String name) throws FHIRException { 5807 if (name.equals("name")) { 5808 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 5809 } 5810 else if (name.equals("definition")) { 5811 this.definition = new Reference(); 5812 return this.definition; 5813 } 5814 else 5815 return super.addChild(name); 5816 } 5817 5818 public CapabilityStatementRestOperationComponent copy() { 5819 CapabilityStatementRestOperationComponent dst = new CapabilityStatementRestOperationComponent(); 5820 copyValues(dst); 5821 dst.name = name == null ? null : name.copy(); 5822 dst.definition = definition == null ? null : definition.copy(); 5823 return dst; 5824 } 5825 5826 @Override 5827 public boolean equalsDeep(Base other_) { 5828 if (!super.equalsDeep(other_)) 5829 return false; 5830 if (!(other_ instanceof CapabilityStatementRestOperationComponent)) 5831 return false; 5832 CapabilityStatementRestOperationComponent o = (CapabilityStatementRestOperationComponent) other_; 5833 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true); 5834 } 5835 5836 @Override 5837 public boolean equalsShallow(Base other_) { 5838 if (!super.equalsShallow(other_)) 5839 return false; 5840 if (!(other_ instanceof CapabilityStatementRestOperationComponent)) 5841 return false; 5842 CapabilityStatementRestOperationComponent o = (CapabilityStatementRestOperationComponent) other_; 5843 return compareValues(name, o.name, true); 5844 } 5845 5846 public boolean isEmpty() { 5847 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition); 5848 } 5849 5850 public String fhirType() { 5851 return "CapabilityStatement.rest.operation"; 5852 5853 } 5854 5855 } 5856 5857 @Block() 5858 public static class CapabilityStatementMessagingComponent extends BackboneElement implements IBaseBackboneElement { 5859 /** 5860 * An endpoint (network accessible address) to which messages and/or replies are to be sent. 5861 */ 5862 @Child(name = "endpoint", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5863 @Description(shortDefinition="Where messages should be sent", formalDefinition="An endpoint (network accessible address) to which messages and/or replies are to be sent." ) 5864 protected List<CapabilityStatementMessagingEndpointComponent> endpoint; 5865 5866 /** 5867 * Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender). 5868 */ 5869 @Child(name = "reliableCache", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5870 @Description(shortDefinition="Reliable Message Cache Length (min)", formalDefinition="Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender)." ) 5871 protected UnsignedIntType reliableCache; 5872 5873 /** 5874 * Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner. 5875 */ 5876 @Child(name = "documentation", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5877 @Description(shortDefinition="Messaging interface behavior details", formalDefinition="Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner." ) 5878 protected StringType documentation; 5879 5880 /** 5881 * References to message definitions for messages this system can send or receive. 5882 */ 5883 @Child(name = "supportedMessage", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5884 @Description(shortDefinition="Messages supported by this system", formalDefinition="References to message definitions for messages this system can send or receive." ) 5885 protected List<CapabilityStatementMessagingSupportedMessageComponent> supportedMessage; 5886 5887 /** 5888 * A description of the solution's support for an event at this end-point. 5889 */ 5890 @Child(name = "event", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5891 @Description(shortDefinition="Declare support for this event", formalDefinition="A description of the solution's support for an event at this end-point." ) 5892 protected List<CapabilityStatementMessagingEventComponent> event; 5893 5894 private static final long serialVersionUID = 816243355L; 5895 5896 /** 5897 * Constructor 5898 */ 5899 public CapabilityStatementMessagingComponent() { 5900 super(); 5901 } 5902 5903 /** 5904 * @return {@link #endpoint} (An endpoint (network accessible address) to which messages and/or replies are to be sent.) 5905 */ 5906 public List<CapabilityStatementMessagingEndpointComponent> getEndpoint() { 5907 if (this.endpoint == null) 5908 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 5909 return this.endpoint; 5910 } 5911 5912 /** 5913 * @return Returns a reference to <code>this</code> for easy method chaining 5914 */ 5915 public CapabilityStatementMessagingComponent setEndpoint(List<CapabilityStatementMessagingEndpointComponent> theEndpoint) { 5916 this.endpoint = theEndpoint; 5917 return this; 5918 } 5919 5920 public boolean hasEndpoint() { 5921 if (this.endpoint == null) 5922 return false; 5923 for (CapabilityStatementMessagingEndpointComponent item : this.endpoint) 5924 if (!item.isEmpty()) 5925 return true; 5926 return false; 5927 } 5928 5929 public CapabilityStatementMessagingEndpointComponent addEndpoint() { //3 5930 CapabilityStatementMessagingEndpointComponent t = new CapabilityStatementMessagingEndpointComponent(); 5931 if (this.endpoint == null) 5932 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 5933 this.endpoint.add(t); 5934 return t; 5935 } 5936 5937 public CapabilityStatementMessagingComponent addEndpoint(CapabilityStatementMessagingEndpointComponent t) { //3 5938 if (t == null) 5939 return this; 5940 if (this.endpoint == null) 5941 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 5942 this.endpoint.add(t); 5943 return this; 5944 } 5945 5946 /** 5947 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 5948 */ 5949 public CapabilityStatementMessagingEndpointComponent getEndpointFirstRep() { 5950 if (getEndpoint().isEmpty()) { 5951 addEndpoint(); 5952 } 5953 return getEndpoint().get(0); 5954 } 5955 5956 /** 5957 * @return {@link #reliableCache} (Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).). This is the underlying object with id, value and extensions. The accessor "getReliableCache" gives direct access to the value 5958 */ 5959 public UnsignedIntType getReliableCacheElement() { 5960 if (this.reliableCache == null) 5961 if (Configuration.errorOnAutoCreate()) 5962 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.reliableCache"); 5963 else if (Configuration.doAutoCreate()) 5964 this.reliableCache = new UnsignedIntType(); // bb 5965 return this.reliableCache; 5966 } 5967 5968 public boolean hasReliableCacheElement() { 5969 return this.reliableCache != null && !this.reliableCache.isEmpty(); 5970 } 5971 5972 public boolean hasReliableCache() { 5973 return this.reliableCache != null && !this.reliableCache.isEmpty(); 5974 } 5975 5976 /** 5977 * @param value {@link #reliableCache} (Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).). This is the underlying object with id, value and extensions. The accessor "getReliableCache" gives direct access to the value 5978 */ 5979 public CapabilityStatementMessagingComponent setReliableCacheElement(UnsignedIntType value) { 5980 this.reliableCache = value; 5981 return this; 5982 } 5983 5984 /** 5985 * @return Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender). 5986 */ 5987 public int getReliableCache() { 5988 return this.reliableCache == null || this.reliableCache.isEmpty() ? 0 : this.reliableCache.getValue(); 5989 } 5990 5991 /** 5992 * @param value Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender). 5993 */ 5994 public CapabilityStatementMessagingComponent setReliableCache(int value) { 5995 if (this.reliableCache == null) 5996 this.reliableCache = new UnsignedIntType(); 5997 this.reliableCache.setValue(value); 5998 return this; 5999 } 6000 6001 /** 6002 * @return {@link #documentation} (Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 6003 */ 6004 public StringType getDocumentationElement() { 6005 if (this.documentation == null) 6006 if (Configuration.errorOnAutoCreate()) 6007 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.documentation"); 6008 else if (Configuration.doAutoCreate()) 6009 this.documentation = new StringType(); // bb 6010 return this.documentation; 6011 } 6012 6013 public boolean hasDocumentationElement() { 6014 return this.documentation != null && !this.documentation.isEmpty(); 6015 } 6016 6017 public boolean hasDocumentation() { 6018 return this.documentation != null && !this.documentation.isEmpty(); 6019 } 6020 6021 /** 6022 * @param value {@link #documentation} (Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 6023 */ 6024 public CapabilityStatementMessagingComponent setDocumentationElement(StringType value) { 6025 this.documentation = value; 6026 return this; 6027 } 6028 6029 /** 6030 * @return Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner. 6031 */ 6032 public String getDocumentation() { 6033 return this.documentation == null ? null : this.documentation.getValue(); 6034 } 6035 6036 /** 6037 * @param value Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner. 6038 */ 6039 public CapabilityStatementMessagingComponent setDocumentation(String value) { 6040 if (Utilities.noString(value)) 6041 this.documentation = null; 6042 else { 6043 if (this.documentation == null) 6044 this.documentation = new StringType(); 6045 this.documentation.setValue(value); 6046 } 6047 return this; 6048 } 6049 6050 /** 6051 * @return {@link #supportedMessage} (References to message definitions for messages this system can send or receive.) 6052 */ 6053 public List<CapabilityStatementMessagingSupportedMessageComponent> getSupportedMessage() { 6054 if (this.supportedMessage == null) 6055 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 6056 return this.supportedMessage; 6057 } 6058 6059 /** 6060 * @return Returns a reference to <code>this</code> for easy method chaining 6061 */ 6062 public CapabilityStatementMessagingComponent setSupportedMessage(List<CapabilityStatementMessagingSupportedMessageComponent> theSupportedMessage) { 6063 this.supportedMessage = theSupportedMessage; 6064 return this; 6065 } 6066 6067 public boolean hasSupportedMessage() { 6068 if (this.supportedMessage == null) 6069 return false; 6070 for (CapabilityStatementMessagingSupportedMessageComponent item : this.supportedMessage) 6071 if (!item.isEmpty()) 6072 return true; 6073 return false; 6074 } 6075 6076 public CapabilityStatementMessagingSupportedMessageComponent addSupportedMessage() { //3 6077 CapabilityStatementMessagingSupportedMessageComponent t = new CapabilityStatementMessagingSupportedMessageComponent(); 6078 if (this.supportedMessage == null) 6079 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 6080 this.supportedMessage.add(t); 6081 return t; 6082 } 6083 6084 public CapabilityStatementMessagingComponent addSupportedMessage(CapabilityStatementMessagingSupportedMessageComponent t) { //3 6085 if (t == null) 6086 return this; 6087 if (this.supportedMessage == null) 6088 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 6089 this.supportedMessage.add(t); 6090 return this; 6091 } 6092 6093 /** 6094 * @return The first repetition of repeating field {@link #supportedMessage}, creating it if it does not already exist 6095 */ 6096 public CapabilityStatementMessagingSupportedMessageComponent getSupportedMessageFirstRep() { 6097 if (getSupportedMessage().isEmpty()) { 6098 addSupportedMessage(); 6099 } 6100 return getSupportedMessage().get(0); 6101 } 6102 6103 /** 6104 * @return {@link #event} (A description of the solution's support for an event at this end-point.) 6105 */ 6106 public List<CapabilityStatementMessagingEventComponent> getEvent() { 6107 if (this.event == null) 6108 this.event = new ArrayList<CapabilityStatementMessagingEventComponent>(); 6109 return this.event; 6110 } 6111 6112 /** 6113 * @return Returns a reference to <code>this</code> for easy method chaining 6114 */ 6115 public CapabilityStatementMessagingComponent setEvent(List<CapabilityStatementMessagingEventComponent> theEvent) { 6116 this.event = theEvent; 6117 return this; 6118 } 6119 6120 public boolean hasEvent() { 6121 if (this.event == null) 6122 return false; 6123 for (CapabilityStatementMessagingEventComponent item : this.event) 6124 if (!item.isEmpty()) 6125 return true; 6126 return false; 6127 } 6128 6129 public CapabilityStatementMessagingEventComponent addEvent() { //3 6130 CapabilityStatementMessagingEventComponent t = new CapabilityStatementMessagingEventComponent(); 6131 if (this.event == null) 6132 this.event = new ArrayList<CapabilityStatementMessagingEventComponent>(); 6133 this.event.add(t); 6134 return t; 6135 } 6136 6137 public CapabilityStatementMessagingComponent addEvent(CapabilityStatementMessagingEventComponent t) { //3 6138 if (t == null) 6139 return this; 6140 if (this.event == null) 6141 this.event = new ArrayList<CapabilityStatementMessagingEventComponent>(); 6142 this.event.add(t); 6143 return this; 6144 } 6145 6146 /** 6147 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist 6148 */ 6149 public CapabilityStatementMessagingEventComponent getEventFirstRep() { 6150 if (getEvent().isEmpty()) { 6151 addEvent(); 6152 } 6153 return getEvent().get(0); 6154 } 6155 6156 protected void listChildren(List<Property> children) { 6157 super.listChildren(children); 6158 children.add(new Property("endpoint", "", "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 6159 children.add(new Property("reliableCache", "unsignedInt", "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 0, 1, reliableCache)); 6160 children.add(new Property("documentation", "string", "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 0, 1, documentation)); 6161 children.add(new Property("supportedMessage", "", "References to message definitions for messages this system can send or receive.", 0, java.lang.Integer.MAX_VALUE, supportedMessage)); 6162 children.add(new Property("event", "", "A description of the solution's support for an event at this end-point.", 0, java.lang.Integer.MAX_VALUE, event)); 6163 } 6164 6165 @Override 6166 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6167 switch (_hash) { 6168 case 1741102485: /*endpoint*/ return new Property("endpoint", "", "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, java.lang.Integer.MAX_VALUE, endpoint); 6169 case 897803608: /*reliableCache*/ return new Property("reliableCache", "unsignedInt", "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 0, 1, reliableCache); 6170 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 0, 1, documentation); 6171 case -1805139079: /*supportedMessage*/ return new Property("supportedMessage", "", "References to message definitions for messages this system can send or receive.", 0, java.lang.Integer.MAX_VALUE, supportedMessage); 6172 case 96891546: /*event*/ return new Property("event", "", "A description of the solution's support for an event at this end-point.", 0, java.lang.Integer.MAX_VALUE, event); 6173 default: return super.getNamedProperty(_hash, _name, _checkValid); 6174 } 6175 6176 } 6177 6178 @Override 6179 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6180 switch (hash) { 6181 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // CapabilityStatementMessagingEndpointComponent 6182 case 897803608: /*reliableCache*/ return this.reliableCache == null ? new Base[0] : new Base[] {this.reliableCache}; // UnsignedIntType 6183 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 6184 case -1805139079: /*supportedMessage*/ return this.supportedMessage == null ? new Base[0] : this.supportedMessage.toArray(new Base[this.supportedMessage.size()]); // CapabilityStatementMessagingSupportedMessageComponent 6185 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CapabilityStatementMessagingEventComponent 6186 default: return super.getProperty(hash, name, checkValid); 6187 } 6188 6189 } 6190 6191 @Override 6192 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6193 switch (hash) { 6194 case 1741102485: // endpoint 6195 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); // CapabilityStatementMessagingEndpointComponent 6196 return value; 6197 case 897803608: // reliableCache 6198 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 6199 return value; 6200 case 1587405498: // documentation 6201 this.documentation = castToString(value); // StringType 6202 return value; 6203 case -1805139079: // supportedMessage 6204 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); // CapabilityStatementMessagingSupportedMessageComponent 6205 return value; 6206 case 96891546: // event 6207 this.getEvent().add((CapabilityStatementMessagingEventComponent) value); // CapabilityStatementMessagingEventComponent 6208 return value; 6209 default: return super.setProperty(hash, name, value); 6210 } 6211 6212 } 6213 6214 @Override 6215 public Base setProperty(String name, Base value) throws FHIRException { 6216 if (name.equals("endpoint")) { 6217 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); 6218 } else if (name.equals("reliableCache")) { 6219 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 6220 } else if (name.equals("documentation")) { 6221 this.documentation = castToString(value); // StringType 6222 } else if (name.equals("supportedMessage")) { 6223 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); 6224 } else if (name.equals("event")) { 6225 this.getEvent().add((CapabilityStatementMessagingEventComponent) value); 6226 } else 6227 return super.setProperty(name, value); 6228 return value; 6229 } 6230 6231 @Override 6232 public Base makeProperty(int hash, String name) throws FHIRException { 6233 switch (hash) { 6234 case 1741102485: return addEndpoint(); 6235 case 897803608: return getReliableCacheElement(); 6236 case 1587405498: return getDocumentationElement(); 6237 case -1805139079: return addSupportedMessage(); 6238 case 96891546: return addEvent(); 6239 default: return super.makeProperty(hash, name); 6240 } 6241 6242 } 6243 6244 @Override 6245 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6246 switch (hash) { 6247 case 1741102485: /*endpoint*/ return new String[] {}; 6248 case 897803608: /*reliableCache*/ return new String[] {"unsignedInt"}; 6249 case 1587405498: /*documentation*/ return new String[] {"string"}; 6250 case -1805139079: /*supportedMessage*/ return new String[] {}; 6251 case 96891546: /*event*/ return new String[] {}; 6252 default: return super.getTypesForProperty(hash, name); 6253 } 6254 6255 } 6256 6257 @Override 6258 public Base addChild(String name) throws FHIRException { 6259 if (name.equals("endpoint")) { 6260 return addEndpoint(); 6261 } 6262 else if (name.equals("reliableCache")) { 6263 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.reliableCache"); 6264 } 6265 else if (name.equals("documentation")) { 6266 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 6267 } 6268 else if (name.equals("supportedMessage")) { 6269 return addSupportedMessage(); 6270 } 6271 else if (name.equals("event")) { 6272 return addEvent(); 6273 } 6274 else 6275 return super.addChild(name); 6276 } 6277 6278 public CapabilityStatementMessagingComponent copy() { 6279 CapabilityStatementMessagingComponent dst = new CapabilityStatementMessagingComponent(); 6280 copyValues(dst); 6281 if (endpoint != null) { 6282 dst.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 6283 for (CapabilityStatementMessagingEndpointComponent i : endpoint) 6284 dst.endpoint.add(i.copy()); 6285 }; 6286 dst.reliableCache = reliableCache == null ? null : reliableCache.copy(); 6287 dst.documentation = documentation == null ? null : documentation.copy(); 6288 if (supportedMessage != null) { 6289 dst.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 6290 for (CapabilityStatementMessagingSupportedMessageComponent i : supportedMessage) 6291 dst.supportedMessage.add(i.copy()); 6292 }; 6293 if (event != null) { 6294 dst.event = new ArrayList<CapabilityStatementMessagingEventComponent>(); 6295 for (CapabilityStatementMessagingEventComponent i : event) 6296 dst.event.add(i.copy()); 6297 }; 6298 return dst; 6299 } 6300 6301 @Override 6302 public boolean equalsDeep(Base other_) { 6303 if (!super.equalsDeep(other_)) 6304 return false; 6305 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 6306 return false; 6307 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 6308 return compareDeep(endpoint, o.endpoint, true) && compareDeep(reliableCache, o.reliableCache, true) 6309 && compareDeep(documentation, o.documentation, true) && compareDeep(supportedMessage, o.supportedMessage, true) 6310 && compareDeep(event, o.event, true); 6311 } 6312 6313 @Override 6314 public boolean equalsShallow(Base other_) { 6315 if (!super.equalsShallow(other_)) 6316 return false; 6317 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 6318 return false; 6319 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 6320 return compareValues(reliableCache, o.reliableCache, true) && compareValues(documentation, o.documentation, true) 6321 ; 6322 } 6323 6324 public boolean isEmpty() { 6325 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(endpoint, reliableCache, documentation 6326 , supportedMessage, event); 6327 } 6328 6329 public String fhirType() { 6330 return "CapabilityStatement.messaging"; 6331 6332 } 6333 6334 } 6335 6336 @Block() 6337 public static class CapabilityStatementMessagingEndpointComponent extends BackboneElement implements IBaseBackboneElement { 6338 /** 6339 * A list of the messaging transport protocol(s) identifiers, supported by this endpoint. 6340 */ 6341 @Child(name = "protocol", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 6342 @Description(shortDefinition="http | ftp | mllp +", formalDefinition="A list of the messaging transport protocol(s) identifiers, supported by this endpoint." ) 6343 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-transport") 6344 protected Coding protocol; 6345 6346 /** 6347 * The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier. 6348 */ 6349 @Child(name = "address", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=false) 6350 @Description(shortDefinition="Network address or identifier of the end-point", formalDefinition="The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier." ) 6351 protected UriType address; 6352 6353 private static final long serialVersionUID = 1294656428L; 6354 6355 /** 6356 * Constructor 6357 */ 6358 public CapabilityStatementMessagingEndpointComponent() { 6359 super(); 6360 } 6361 6362 /** 6363 * Constructor 6364 */ 6365 public CapabilityStatementMessagingEndpointComponent(Coding protocol, UriType address) { 6366 super(); 6367 this.protocol = protocol; 6368 this.address = address; 6369 } 6370 6371 /** 6372 * @return {@link #protocol} (A list of the messaging transport protocol(s) identifiers, supported by this endpoint.) 6373 */ 6374 public Coding getProtocol() { 6375 if (this.protocol == null) 6376 if (Configuration.errorOnAutoCreate()) 6377 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.protocol"); 6378 else if (Configuration.doAutoCreate()) 6379 this.protocol = new Coding(); // cc 6380 return this.protocol; 6381 } 6382 6383 public boolean hasProtocol() { 6384 return this.protocol != null && !this.protocol.isEmpty(); 6385 } 6386 6387 /** 6388 * @param value {@link #protocol} (A list of the messaging transport protocol(s) identifiers, supported by this endpoint.) 6389 */ 6390 public CapabilityStatementMessagingEndpointComponent setProtocol(Coding value) { 6391 this.protocol = value; 6392 return this; 6393 } 6394 6395 /** 6396 * @return {@link #address} (The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 6397 */ 6398 public UriType getAddressElement() { 6399 if (this.address == null) 6400 if (Configuration.errorOnAutoCreate()) 6401 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.address"); 6402 else if (Configuration.doAutoCreate()) 6403 this.address = new UriType(); // bb 6404 return this.address; 6405 } 6406 6407 public boolean hasAddressElement() { 6408 return this.address != null && !this.address.isEmpty(); 6409 } 6410 6411 public boolean hasAddress() { 6412 return this.address != null && !this.address.isEmpty(); 6413 } 6414 6415 /** 6416 * @param value {@link #address} (The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 6417 */ 6418 public CapabilityStatementMessagingEndpointComponent setAddressElement(UriType value) { 6419 this.address = value; 6420 return this; 6421 } 6422 6423 /** 6424 * @return The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier. 6425 */ 6426 public String getAddress() { 6427 return this.address == null ? null : this.address.getValue(); 6428 } 6429 6430 /** 6431 * @param value The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier. 6432 */ 6433 public CapabilityStatementMessagingEndpointComponent setAddress(String value) { 6434 if (this.address == null) 6435 this.address = new UriType(); 6436 this.address.setValue(value); 6437 return this; 6438 } 6439 6440 protected void listChildren(List<Property> children) { 6441 super.listChildren(children); 6442 children.add(new Property("protocol", "Coding", "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol)); 6443 children.add(new Property("address", "uri", "The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.", 0, 1, address)); 6444 } 6445 6446 @Override 6447 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6448 switch (_hash) { 6449 case -989163880: /*protocol*/ return new Property("protocol", "Coding", "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol); 6450 case -1147692044: /*address*/ return new Property("address", "uri", "The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.", 0, 1, address); 6451 default: return super.getNamedProperty(_hash, _name, _checkValid); 6452 } 6453 6454 } 6455 6456 @Override 6457 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6458 switch (hash) { 6459 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : new Base[] {this.protocol}; // Coding 6460 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // UriType 6461 default: return super.getProperty(hash, name, checkValid); 6462 } 6463 6464 } 6465 6466 @Override 6467 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6468 switch (hash) { 6469 case -989163880: // protocol 6470 this.protocol = castToCoding(value); // Coding 6471 return value; 6472 case -1147692044: // address 6473 this.address = castToUri(value); // UriType 6474 return value; 6475 default: return super.setProperty(hash, name, value); 6476 } 6477 6478 } 6479 6480 @Override 6481 public Base setProperty(String name, Base value) throws FHIRException { 6482 if (name.equals("protocol")) { 6483 this.protocol = castToCoding(value); // Coding 6484 } else if (name.equals("address")) { 6485 this.address = castToUri(value); // UriType 6486 } else 6487 return super.setProperty(name, value); 6488 return value; 6489 } 6490 6491 @Override 6492 public Base makeProperty(int hash, String name) throws FHIRException { 6493 switch (hash) { 6494 case -989163880: return getProtocol(); 6495 case -1147692044: return getAddressElement(); 6496 default: return super.makeProperty(hash, name); 6497 } 6498 6499 } 6500 6501 @Override 6502 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6503 switch (hash) { 6504 case -989163880: /*protocol*/ return new String[] {"Coding"}; 6505 case -1147692044: /*address*/ return new String[] {"uri"}; 6506 default: return super.getTypesForProperty(hash, name); 6507 } 6508 6509 } 6510 6511 @Override 6512 public Base addChild(String name) throws FHIRException { 6513 if (name.equals("protocol")) { 6514 this.protocol = new Coding(); 6515 return this.protocol; 6516 } 6517 else if (name.equals("address")) { 6518 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.address"); 6519 } 6520 else 6521 return super.addChild(name); 6522 } 6523 6524 public CapabilityStatementMessagingEndpointComponent copy() { 6525 CapabilityStatementMessagingEndpointComponent dst = new CapabilityStatementMessagingEndpointComponent(); 6526 copyValues(dst); 6527 dst.protocol = protocol == null ? null : protocol.copy(); 6528 dst.address = address == null ? null : address.copy(); 6529 return dst; 6530 } 6531 6532 @Override 6533 public boolean equalsDeep(Base other_) { 6534 if (!super.equalsDeep(other_)) 6535 return false; 6536 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 6537 return false; 6538 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 6539 return compareDeep(protocol, o.protocol, true) && compareDeep(address, o.address, true); 6540 } 6541 6542 @Override 6543 public boolean equalsShallow(Base other_) { 6544 if (!super.equalsShallow(other_)) 6545 return false; 6546 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 6547 return false; 6548 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 6549 return compareValues(address, o.address, true); 6550 } 6551 6552 public boolean isEmpty() { 6553 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(protocol, address); 6554 } 6555 6556 public String fhirType() { 6557 return "CapabilityStatement.messaging.endpoint"; 6558 6559 } 6560 6561 } 6562 6563 @Block() 6564 public static class CapabilityStatementMessagingSupportedMessageComponent extends BackboneElement implements IBaseBackboneElement { 6565 /** 6566 * The mode of this event declaration - whether application is sender or receiver. 6567 */ 6568 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 6569 @Description(shortDefinition="sender | receiver", formalDefinition="The mode of this event declaration - whether application is sender or receiver." ) 6570 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-capability-mode") 6571 protected Enumeration<EventCapabilityMode> mode; 6572 6573 /** 6574 * Points to a message definition that identifies the messaging event, message structure, allowed responses, etc. 6575 */ 6576 @Child(name = "definition", type = {MessageDefinition.class}, order=2, min=1, max=1, modifier=false, summary=true) 6577 @Description(shortDefinition="Message supported by this system", formalDefinition="Points to a message definition that identifies the messaging event, message structure, allowed responses, etc." ) 6578 protected Reference definition; 6579 6580 /** 6581 * The actual object that is the target of the reference (Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.) 6582 */ 6583 protected MessageDefinition definitionTarget; 6584 6585 private static final long serialVersionUID = -741523869L; 6586 6587 /** 6588 * Constructor 6589 */ 6590 public CapabilityStatementMessagingSupportedMessageComponent() { 6591 super(); 6592 } 6593 6594 /** 6595 * Constructor 6596 */ 6597 public CapabilityStatementMessagingSupportedMessageComponent(Enumeration<EventCapabilityMode> mode, Reference definition) { 6598 super(); 6599 this.mode = mode; 6600 this.definition = definition; 6601 } 6602 6603 /** 6604 * @return {@link #mode} (The mode of this event declaration - whether application is sender or receiver.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6605 */ 6606 public Enumeration<EventCapabilityMode> getModeElement() { 6607 if (this.mode == null) 6608 if (Configuration.errorOnAutoCreate()) 6609 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.mode"); 6610 else if (Configuration.doAutoCreate()) 6611 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); // bb 6612 return this.mode; 6613 } 6614 6615 public boolean hasModeElement() { 6616 return this.mode != null && !this.mode.isEmpty(); 6617 } 6618 6619 public boolean hasMode() { 6620 return this.mode != null && !this.mode.isEmpty(); 6621 } 6622 6623 /** 6624 * @param value {@link #mode} (The mode of this event declaration - whether application is sender or receiver.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6625 */ 6626 public CapabilityStatementMessagingSupportedMessageComponent setModeElement(Enumeration<EventCapabilityMode> value) { 6627 this.mode = value; 6628 return this; 6629 } 6630 6631 /** 6632 * @return The mode of this event declaration - whether application is sender or receiver. 6633 */ 6634 public EventCapabilityMode getMode() { 6635 return this.mode == null ? null : this.mode.getValue(); 6636 } 6637 6638 /** 6639 * @param value The mode of this event declaration - whether application is sender or receiver. 6640 */ 6641 public CapabilityStatementMessagingSupportedMessageComponent setMode(EventCapabilityMode value) { 6642 if (this.mode == null) 6643 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); 6644 this.mode.setValue(value); 6645 return this; 6646 } 6647 6648 /** 6649 * @return {@link #definition} (Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.) 6650 */ 6651 public Reference getDefinition() { 6652 if (this.definition == null) 6653 if (Configuration.errorOnAutoCreate()) 6654 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.definition"); 6655 else if (Configuration.doAutoCreate()) 6656 this.definition = new Reference(); // cc 6657 return this.definition; 6658 } 6659 6660 public boolean hasDefinition() { 6661 return this.definition != null && !this.definition.isEmpty(); 6662 } 6663 6664 /** 6665 * @param value {@link #definition} (Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.) 6666 */ 6667 public CapabilityStatementMessagingSupportedMessageComponent setDefinition(Reference value) { 6668 this.definition = value; 6669 return this; 6670 } 6671 6672 /** 6673 * @return {@link #definition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.) 6674 */ 6675 public MessageDefinition getDefinitionTarget() { 6676 if (this.definitionTarget == null) 6677 if (Configuration.errorOnAutoCreate()) 6678 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.definition"); 6679 else if (Configuration.doAutoCreate()) 6680 this.definitionTarget = new MessageDefinition(); // aa 6681 return this.definitionTarget; 6682 } 6683 6684 /** 6685 * @param value {@link #definition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.) 6686 */ 6687 public CapabilityStatementMessagingSupportedMessageComponent setDefinitionTarget(MessageDefinition value) { 6688 this.definitionTarget = value; 6689 return this; 6690 } 6691 6692 protected void listChildren(List<Property> children) { 6693 super.listChildren(children); 6694 children.add(new Property("mode", "code", "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode)); 6695 children.add(new Property("definition", "Reference(MessageDefinition)", "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 0, 1, definition)); 6696 } 6697 6698 @Override 6699 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6700 switch (_hash) { 6701 case 3357091: /*mode*/ return new Property("mode", "code", "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode); 6702 case -1014418093: /*definition*/ return new Property("definition", "Reference(MessageDefinition)", "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 0, 1, definition); 6703 default: return super.getNamedProperty(_hash, _name, _checkValid); 6704 } 6705 6706 } 6707 6708 @Override 6709 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6710 switch (hash) { 6711 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<EventCapabilityMode> 6712 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Reference 6713 default: return super.getProperty(hash, name, checkValid); 6714 } 6715 6716 } 6717 6718 @Override 6719 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6720 switch (hash) { 6721 case 3357091: // mode 6722 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 6723 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 6724 return value; 6725 case -1014418093: // definition 6726 this.definition = castToReference(value); // Reference 6727 return value; 6728 default: return super.setProperty(hash, name, value); 6729 } 6730 6731 } 6732 6733 @Override 6734 public Base setProperty(String name, Base value) throws FHIRException { 6735 if (name.equals("mode")) { 6736 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 6737 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 6738 } else if (name.equals("definition")) { 6739 this.definition = castToReference(value); // Reference 6740 } else 6741 return super.setProperty(name, value); 6742 return value; 6743 } 6744 6745 @Override 6746 public Base makeProperty(int hash, String name) throws FHIRException { 6747 switch (hash) { 6748 case 3357091: return getModeElement(); 6749 case -1014418093: return getDefinition(); 6750 default: return super.makeProperty(hash, name); 6751 } 6752 6753 } 6754 6755 @Override 6756 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6757 switch (hash) { 6758 case 3357091: /*mode*/ return new String[] {"code"}; 6759 case -1014418093: /*definition*/ return new String[] {"Reference"}; 6760 default: return super.getTypesForProperty(hash, name); 6761 } 6762 6763 } 6764 6765 @Override 6766 public Base addChild(String name) throws FHIRException { 6767 if (name.equals("mode")) { 6768 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 6769 } 6770 else if (name.equals("definition")) { 6771 this.definition = new Reference(); 6772 return this.definition; 6773 } 6774 else 6775 return super.addChild(name); 6776 } 6777 6778 public CapabilityStatementMessagingSupportedMessageComponent copy() { 6779 CapabilityStatementMessagingSupportedMessageComponent dst = new CapabilityStatementMessagingSupportedMessageComponent(); 6780 copyValues(dst); 6781 dst.mode = mode == null ? null : mode.copy(); 6782 dst.definition = definition == null ? null : definition.copy(); 6783 return dst; 6784 } 6785 6786 @Override 6787 public boolean equalsDeep(Base other_) { 6788 if (!super.equalsDeep(other_)) 6789 return false; 6790 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 6791 return false; 6792 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 6793 return compareDeep(mode, o.mode, true) && compareDeep(definition, o.definition, true); 6794 } 6795 6796 @Override 6797 public boolean equalsShallow(Base other_) { 6798 if (!super.equalsShallow(other_)) 6799 return false; 6800 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 6801 return false; 6802 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 6803 return compareValues(mode, o.mode, true); 6804 } 6805 6806 public boolean isEmpty() { 6807 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, definition); 6808 } 6809 6810 public String fhirType() { 6811 return "CapabilityStatement.messaging.supportedMessage"; 6812 6813 } 6814 6815 } 6816 6817 @Block() 6818 public static class CapabilityStatementMessagingEventComponent extends BackboneElement implements IBaseBackboneElement { 6819 /** 6820 * A coded identifier of a supported messaging event. 6821 */ 6822 @Child(name = "code", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=true) 6823 @Description(shortDefinition="Event type", formalDefinition="A coded identifier of a supported messaging event." ) 6824 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-events") 6825 protected Coding code; 6826 6827 /** 6828 * The impact of the content of the message. 6829 */ 6830 @Child(name = "category", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 6831 @Description(shortDefinition="Consequence | Currency | Notification", formalDefinition="The impact of the content of the message." ) 6832 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-significance-category") 6833 protected Enumeration<MessageSignificanceCategory> category; 6834 6835 /** 6836 * The mode of this event declaration - whether an application is a sender or receiver. 6837 */ 6838 @Child(name = "mode", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 6839 @Description(shortDefinition="sender | receiver", formalDefinition="The mode of this event declaration - whether an application is a sender or receiver." ) 6840 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-capability-mode") 6841 protected Enumeration<EventCapabilityMode> mode; 6842 6843 /** 6844 * A resource associated with the event. This is the resource that defines the event. 6845 */ 6846 @Child(name = "focus", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=false) 6847 @Description(shortDefinition="Resource that's focus of message", formalDefinition="A resource associated with the event. This is the resource that defines the event." ) 6848 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 6849 protected CodeType focus; 6850 6851 /** 6852 * Information about the request for this event. 6853 */ 6854 @Child(name = "request", type = {StructureDefinition.class}, order=5, min=1, max=1, modifier=false, summary=true) 6855 @Description(shortDefinition="Profile that describes the request", formalDefinition="Information about the request for this event." ) 6856 protected Reference request; 6857 6858 /** 6859 * The actual object that is the target of the reference (Information about the request for this event.) 6860 */ 6861 protected StructureDefinition requestTarget; 6862 6863 /** 6864 * Information about the response for this event. 6865 */ 6866 @Child(name = "response", type = {StructureDefinition.class}, order=6, min=1, max=1, modifier=false, summary=true) 6867 @Description(shortDefinition="Profile that describes the response", formalDefinition="Information about the response for this event." ) 6868 protected Reference response; 6869 6870 /** 6871 * The actual object that is the target of the reference (Information about the response for this event.) 6872 */ 6873 protected StructureDefinition responseTarget; 6874 6875 /** 6876 * Guidance on how this event is handled, such as internal system trigger points, business rules, etc. 6877 */ 6878 @Child(name = "documentation", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 6879 @Description(shortDefinition="Endpoint-specific event documentation", formalDefinition="Guidance on how this event is handled, such as internal system trigger points, business rules, etc." ) 6880 protected StringType documentation; 6881 6882 private static final long serialVersionUID = -491306017L; 6883 6884 /** 6885 * Constructor 6886 */ 6887 public CapabilityStatementMessagingEventComponent() { 6888 super(); 6889 } 6890 6891 /** 6892 * Constructor 6893 */ 6894 public CapabilityStatementMessagingEventComponent(Coding code, Enumeration<EventCapabilityMode> mode, CodeType focus, Reference request, Reference response) { 6895 super(); 6896 this.code = code; 6897 this.mode = mode; 6898 this.focus = focus; 6899 this.request = request; 6900 this.response = response; 6901 } 6902 6903 /** 6904 * @return {@link #code} (A coded identifier of a supported messaging event.) 6905 */ 6906 public Coding getCode() { 6907 if (this.code == null) 6908 if (Configuration.errorOnAutoCreate()) 6909 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.code"); 6910 else if (Configuration.doAutoCreate()) 6911 this.code = new Coding(); // cc 6912 return this.code; 6913 } 6914 6915 public boolean hasCode() { 6916 return this.code != null && !this.code.isEmpty(); 6917 } 6918 6919 /** 6920 * @param value {@link #code} (A coded identifier of a supported messaging event.) 6921 */ 6922 public CapabilityStatementMessagingEventComponent setCode(Coding value) { 6923 this.code = value; 6924 return this; 6925 } 6926 6927 /** 6928 * @return {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 6929 */ 6930 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 6931 if (this.category == null) 6932 if (Configuration.errorOnAutoCreate()) 6933 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.category"); 6934 else if (Configuration.doAutoCreate()) 6935 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 6936 return this.category; 6937 } 6938 6939 public boolean hasCategoryElement() { 6940 return this.category != null && !this.category.isEmpty(); 6941 } 6942 6943 public boolean hasCategory() { 6944 return this.category != null && !this.category.isEmpty(); 6945 } 6946 6947 /** 6948 * @param value {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 6949 */ 6950 public CapabilityStatementMessagingEventComponent setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 6951 this.category = value; 6952 return this; 6953 } 6954 6955 /** 6956 * @return The impact of the content of the message. 6957 */ 6958 public MessageSignificanceCategory getCategory() { 6959 return this.category == null ? null : this.category.getValue(); 6960 } 6961 6962 /** 6963 * @param value The impact of the content of the message. 6964 */ 6965 public CapabilityStatementMessagingEventComponent setCategory(MessageSignificanceCategory value) { 6966 if (value == null) 6967 this.category = null; 6968 else { 6969 if (this.category == null) 6970 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 6971 this.category.setValue(value); 6972 } 6973 return this; 6974 } 6975 6976 /** 6977 * @return {@link #mode} (The mode of this event declaration - whether an application is a sender or receiver.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6978 */ 6979 public Enumeration<EventCapabilityMode> getModeElement() { 6980 if (this.mode == null) 6981 if (Configuration.errorOnAutoCreate()) 6982 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.mode"); 6983 else if (Configuration.doAutoCreate()) 6984 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); // bb 6985 return this.mode; 6986 } 6987 6988 public boolean hasModeElement() { 6989 return this.mode != null && !this.mode.isEmpty(); 6990 } 6991 6992 public boolean hasMode() { 6993 return this.mode != null && !this.mode.isEmpty(); 6994 } 6995 6996 /** 6997 * @param value {@link #mode} (The mode of this event declaration - whether an application is a sender or receiver.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6998 */ 6999 public CapabilityStatementMessagingEventComponent setModeElement(Enumeration<EventCapabilityMode> value) { 7000 this.mode = value; 7001 return this; 7002 } 7003 7004 /** 7005 * @return The mode of this event declaration - whether an application is a sender or receiver. 7006 */ 7007 public EventCapabilityMode getMode() { 7008 return this.mode == null ? null : this.mode.getValue(); 7009 } 7010 7011 /** 7012 * @param value The mode of this event declaration - whether an application is a sender or receiver. 7013 */ 7014 public CapabilityStatementMessagingEventComponent setMode(EventCapabilityMode value) { 7015 if (this.mode == null) 7016 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); 7017 this.mode.setValue(value); 7018 return this; 7019 } 7020 7021 /** 7022 * @return {@link #focus} (A resource associated with the event. This is the resource that defines the event.). This is the underlying object with id, value and extensions. The accessor "getFocus" gives direct access to the value 7023 */ 7024 public CodeType getFocusElement() { 7025 if (this.focus == null) 7026 if (Configuration.errorOnAutoCreate()) 7027 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.focus"); 7028 else if (Configuration.doAutoCreate()) 7029 this.focus = new CodeType(); // bb 7030 return this.focus; 7031 } 7032 7033 public boolean hasFocusElement() { 7034 return this.focus != null && !this.focus.isEmpty(); 7035 } 7036 7037 public boolean hasFocus() { 7038 return this.focus != null && !this.focus.isEmpty(); 7039 } 7040 7041 /** 7042 * @param value {@link #focus} (A resource associated with the event. This is the resource that defines the event.). This is the underlying object with id, value and extensions. The accessor "getFocus" gives direct access to the value 7043 */ 7044 public CapabilityStatementMessagingEventComponent setFocusElement(CodeType value) { 7045 this.focus = value; 7046 return this; 7047 } 7048 7049 /** 7050 * @return A resource associated with the event. This is the resource that defines the event. 7051 */ 7052 public String getFocus() { 7053 return this.focus == null ? null : this.focus.getValue(); 7054 } 7055 7056 /** 7057 * @param value A resource associated with the event. This is the resource that defines the event. 7058 */ 7059 public CapabilityStatementMessagingEventComponent setFocus(String value) { 7060 if (this.focus == null) 7061 this.focus = new CodeType(); 7062 this.focus.setValue(value); 7063 return this; 7064 } 7065 7066 /** 7067 * @return {@link #request} (Information about the request for this event.) 7068 */ 7069 public Reference getRequest() { 7070 if (this.request == null) 7071 if (Configuration.errorOnAutoCreate()) 7072 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.request"); 7073 else if (Configuration.doAutoCreate()) 7074 this.request = new Reference(); // cc 7075 return this.request; 7076 } 7077 7078 public boolean hasRequest() { 7079 return this.request != null && !this.request.isEmpty(); 7080 } 7081 7082 /** 7083 * @param value {@link #request} (Information about the request for this event.) 7084 */ 7085 public CapabilityStatementMessagingEventComponent setRequest(Reference value) { 7086 this.request = value; 7087 return this; 7088 } 7089 7090 /** 7091 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Information about the request for this event.) 7092 */ 7093 public StructureDefinition getRequestTarget() { 7094 if (this.requestTarget == null) 7095 if (Configuration.errorOnAutoCreate()) 7096 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.request"); 7097 else if (Configuration.doAutoCreate()) 7098 this.requestTarget = new StructureDefinition(); // aa 7099 return this.requestTarget; 7100 } 7101 7102 /** 7103 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Information about the request for this event.) 7104 */ 7105 public CapabilityStatementMessagingEventComponent setRequestTarget(StructureDefinition value) { 7106 this.requestTarget = value; 7107 return this; 7108 } 7109 7110 /** 7111 * @return {@link #response} (Information about the response for this event.) 7112 */ 7113 public Reference getResponse() { 7114 if (this.response == null) 7115 if (Configuration.errorOnAutoCreate()) 7116 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.response"); 7117 else if (Configuration.doAutoCreate()) 7118 this.response = new Reference(); // cc 7119 return this.response; 7120 } 7121 7122 public boolean hasResponse() { 7123 return this.response != null && !this.response.isEmpty(); 7124 } 7125 7126 /** 7127 * @param value {@link #response} (Information about the response for this event.) 7128 */ 7129 public CapabilityStatementMessagingEventComponent setResponse(Reference value) { 7130 this.response = value; 7131 return this; 7132 } 7133 7134 /** 7135 * @return {@link #response} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Information about the response for this event.) 7136 */ 7137 public StructureDefinition getResponseTarget() { 7138 if (this.responseTarget == null) 7139 if (Configuration.errorOnAutoCreate()) 7140 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.response"); 7141 else if (Configuration.doAutoCreate()) 7142 this.responseTarget = new StructureDefinition(); // aa 7143 return this.responseTarget; 7144 } 7145 7146 /** 7147 * @param value {@link #response} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Information about the response for this event.) 7148 */ 7149 public CapabilityStatementMessagingEventComponent setResponseTarget(StructureDefinition value) { 7150 this.responseTarget = value; 7151 return this; 7152 } 7153 7154 /** 7155 * @return {@link #documentation} (Guidance on how this event is handled, such as internal system trigger points, business rules, etc.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 7156 */ 7157 public StringType getDocumentationElement() { 7158 if (this.documentation == null) 7159 if (Configuration.errorOnAutoCreate()) 7160 throw new Error("Attempt to auto-create CapabilityStatementMessagingEventComponent.documentation"); 7161 else if (Configuration.doAutoCreate()) 7162 this.documentation = new StringType(); // bb 7163 return this.documentation; 7164 } 7165 7166 public boolean hasDocumentationElement() { 7167 return this.documentation != null && !this.documentation.isEmpty(); 7168 } 7169 7170 public boolean hasDocumentation() { 7171 return this.documentation != null && !this.documentation.isEmpty(); 7172 } 7173 7174 /** 7175 * @param value {@link #documentation} (Guidance on how this event is handled, such as internal system trigger points, business rules, etc.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 7176 */ 7177 public CapabilityStatementMessagingEventComponent setDocumentationElement(StringType value) { 7178 this.documentation = value; 7179 return this; 7180 } 7181 7182 /** 7183 * @return Guidance on how this event is handled, such as internal system trigger points, business rules, etc. 7184 */ 7185 public String getDocumentation() { 7186 return this.documentation == null ? null : this.documentation.getValue(); 7187 } 7188 7189 /** 7190 * @param value Guidance on how this event is handled, such as internal system trigger points, business rules, etc. 7191 */ 7192 public CapabilityStatementMessagingEventComponent setDocumentation(String value) { 7193 if (Utilities.noString(value)) 7194 this.documentation = null; 7195 else { 7196 if (this.documentation == null) 7197 this.documentation = new StringType(); 7198 this.documentation.setValue(value); 7199 } 7200 return this; 7201 } 7202 7203 protected void listChildren(List<Property> children) { 7204 super.listChildren(children); 7205 children.add(new Property("code", "Coding", "A coded identifier of a supported messaging event.", 0, 1, code)); 7206 children.add(new Property("category", "code", "The impact of the content of the message.", 0, 1, category)); 7207 children.add(new Property("mode", "code", "The mode of this event declaration - whether an application is a sender or receiver.", 0, 1, mode)); 7208 children.add(new Property("focus", "code", "A resource associated with the event. This is the resource that defines the event.", 0, 1, focus)); 7209 children.add(new Property("request", "Reference(StructureDefinition)", "Information about the request for this event.", 0, 1, request)); 7210 children.add(new Property("response", "Reference(StructureDefinition)", "Information about the response for this event.", 0, 1, response)); 7211 children.add(new Property("documentation", "string", "Guidance on how this event is handled, such as internal system trigger points, business rules, etc.", 0, 1, documentation)); 7212 } 7213 7214 @Override 7215 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7216 switch (_hash) { 7217 case 3059181: /*code*/ return new Property("code", "Coding", "A coded identifier of a supported messaging event.", 0, 1, code); 7218 case 50511102: /*category*/ return new Property("category", "code", "The impact of the content of the message.", 0, 1, category); 7219 case 3357091: /*mode*/ return new Property("mode", "code", "The mode of this event declaration - whether an application is a sender or receiver.", 0, 1, mode); 7220 case 97604824: /*focus*/ return new Property("focus", "code", "A resource associated with the event. This is the resource that defines the event.", 0, 1, focus); 7221 case 1095692943: /*request*/ return new Property("request", "Reference(StructureDefinition)", "Information about the request for this event.", 0, 1, request); 7222 case -340323263: /*response*/ return new Property("response", "Reference(StructureDefinition)", "Information about the response for this event.", 0, 1, response); 7223 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Guidance on how this event is handled, such as internal system trigger points, business rules, etc.", 0, 1, documentation); 7224 default: return super.getNamedProperty(_hash, _name, _checkValid); 7225 } 7226 7227 } 7228 7229 @Override 7230 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7231 switch (hash) { 7232 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Coding 7233 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<MessageSignificanceCategory> 7234 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<EventCapabilityMode> 7235 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // CodeType 7236 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 7237 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Reference 7238 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 7239 default: return super.getProperty(hash, name, checkValid); 7240 } 7241 7242 } 7243 7244 @Override 7245 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7246 switch (hash) { 7247 case 3059181: // code 7248 this.code = castToCoding(value); // Coding 7249 return value; 7250 case 50511102: // category 7251 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 7252 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 7253 return value; 7254 case 3357091: // mode 7255 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 7256 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 7257 return value; 7258 case 97604824: // focus 7259 this.focus = castToCode(value); // CodeType 7260 return value; 7261 case 1095692943: // request 7262 this.request = castToReference(value); // Reference 7263 return value; 7264 case -340323263: // response 7265 this.response = castToReference(value); // Reference 7266 return value; 7267 case 1587405498: // documentation 7268 this.documentation = castToString(value); // StringType 7269 return value; 7270 default: return super.setProperty(hash, name, value); 7271 } 7272 7273 } 7274 7275 @Override 7276 public Base setProperty(String name, Base value) throws FHIRException { 7277 if (name.equals("code")) { 7278 this.code = castToCoding(value); // Coding 7279 } else if (name.equals("category")) { 7280 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 7281 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 7282 } else if (name.equals("mode")) { 7283 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 7284 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 7285 } else if (name.equals("focus")) { 7286 this.focus = castToCode(value); // CodeType 7287 } else if (name.equals("request")) { 7288 this.request = castToReference(value); // Reference 7289 } else if (name.equals("response")) { 7290 this.response = castToReference(value); // Reference 7291 } else if (name.equals("documentation")) { 7292 this.documentation = castToString(value); // StringType 7293 } else 7294 return super.setProperty(name, value); 7295 return value; 7296 } 7297 7298 @Override 7299 public Base makeProperty(int hash, String name) throws FHIRException { 7300 switch (hash) { 7301 case 3059181: return getCode(); 7302 case 50511102: return getCategoryElement(); 7303 case 3357091: return getModeElement(); 7304 case 97604824: return getFocusElement(); 7305 case 1095692943: return getRequest(); 7306 case -340323263: return getResponse(); 7307 case 1587405498: return getDocumentationElement(); 7308 default: return super.makeProperty(hash, name); 7309 } 7310 7311 } 7312 7313 @Override 7314 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7315 switch (hash) { 7316 case 3059181: /*code*/ return new String[] {"Coding"}; 7317 case 50511102: /*category*/ return new String[] {"code"}; 7318 case 3357091: /*mode*/ return new String[] {"code"}; 7319 case 97604824: /*focus*/ return new String[] {"code"}; 7320 case 1095692943: /*request*/ return new String[] {"Reference"}; 7321 case -340323263: /*response*/ return new String[] {"Reference"}; 7322 case 1587405498: /*documentation*/ return new String[] {"string"}; 7323 default: return super.getTypesForProperty(hash, name); 7324 } 7325 7326 } 7327 7328 @Override 7329 public Base addChild(String name) throws FHIRException { 7330 if (name.equals("code")) { 7331 this.code = new Coding(); 7332 return this.code; 7333 } 7334 else if (name.equals("category")) { 7335 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.category"); 7336 } 7337 else if (name.equals("mode")) { 7338 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 7339 } 7340 else if (name.equals("focus")) { 7341 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.focus"); 7342 } 7343 else if (name.equals("request")) { 7344 this.request = new Reference(); 7345 return this.request; 7346 } 7347 else if (name.equals("response")) { 7348 this.response = new Reference(); 7349 return this.response; 7350 } 7351 else if (name.equals("documentation")) { 7352 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 7353 } 7354 else 7355 return super.addChild(name); 7356 } 7357 7358 public CapabilityStatementMessagingEventComponent copy() { 7359 CapabilityStatementMessagingEventComponent dst = new CapabilityStatementMessagingEventComponent(); 7360 copyValues(dst); 7361 dst.code = code == null ? null : code.copy(); 7362 dst.category = category == null ? null : category.copy(); 7363 dst.mode = mode == null ? null : mode.copy(); 7364 dst.focus = focus == null ? null : focus.copy(); 7365 dst.request = request == null ? null : request.copy(); 7366 dst.response = response == null ? null : response.copy(); 7367 dst.documentation = documentation == null ? null : documentation.copy(); 7368 return dst; 7369 } 7370 7371 @Override 7372 public boolean equalsDeep(Base other_) { 7373 if (!super.equalsDeep(other_)) 7374 return false; 7375 if (!(other_ instanceof CapabilityStatementMessagingEventComponent)) 7376 return false; 7377 CapabilityStatementMessagingEventComponent o = (CapabilityStatementMessagingEventComponent) other_; 7378 return compareDeep(code, o.code, true) && compareDeep(category, o.category, true) && compareDeep(mode, o.mode, true) 7379 && compareDeep(focus, o.focus, true) && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 7380 && compareDeep(documentation, o.documentation, true); 7381 } 7382 7383 @Override 7384 public boolean equalsShallow(Base other_) { 7385 if (!super.equalsShallow(other_)) 7386 return false; 7387 if (!(other_ instanceof CapabilityStatementMessagingEventComponent)) 7388 return false; 7389 CapabilityStatementMessagingEventComponent o = (CapabilityStatementMessagingEventComponent) other_; 7390 return compareValues(category, o.category, true) && compareValues(mode, o.mode, true) && compareValues(focus, o.focus, true) 7391 && compareValues(documentation, o.documentation, true); 7392 } 7393 7394 public boolean isEmpty() { 7395 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, category, mode, focus 7396 , request, response, documentation); 7397 } 7398 7399 public String fhirType() { 7400 return "CapabilityStatement.messaging.event"; 7401 7402 } 7403 7404 } 7405 7406 @Block() 7407 public static class CapabilityStatementDocumentComponent extends BackboneElement implements IBaseBackboneElement { 7408 /** 7409 * Mode of this document declaration - whether an application is a producer or consumer. 7410 */ 7411 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 7412 @Description(shortDefinition="producer | consumer", formalDefinition="Mode of this document declaration - whether an application is a producer or consumer." ) 7413 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-mode") 7414 protected Enumeration<DocumentMode> mode; 7415 7416 /** 7417 * A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc. 7418 */ 7419 @Child(name = "documentation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 7420 @Description(shortDefinition="Description of document support", formalDefinition="A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc." ) 7421 protected StringType documentation; 7422 7423 /** 7424 * A constraint on a resource used in the document. 7425 */ 7426 @Child(name = "profile", type = {StructureDefinition.class}, order=3, min=1, max=1, modifier=false, summary=true) 7427 @Description(shortDefinition="Constraint on a resource used in the document", formalDefinition="A constraint on a resource used in the document." ) 7428 protected Reference profile; 7429 7430 /** 7431 * The actual object that is the target of the reference (A constraint on a resource used in the document.) 7432 */ 7433 protected StructureDefinition profileTarget; 7434 7435 private static final long serialVersionUID = -1059555053L; 7436 7437 /** 7438 * Constructor 7439 */ 7440 public CapabilityStatementDocumentComponent() { 7441 super(); 7442 } 7443 7444 /** 7445 * Constructor 7446 */ 7447 public CapabilityStatementDocumentComponent(Enumeration<DocumentMode> mode, Reference profile) { 7448 super(); 7449 this.mode = mode; 7450 this.profile = profile; 7451 } 7452 7453 /** 7454 * @return {@link #mode} (Mode of this document declaration - whether an application is a producer or consumer.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 7455 */ 7456 public Enumeration<DocumentMode> getModeElement() { 7457 if (this.mode == null) 7458 if (Configuration.errorOnAutoCreate()) 7459 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.mode"); 7460 else if (Configuration.doAutoCreate()) 7461 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); // bb 7462 return this.mode; 7463 } 7464 7465 public boolean hasModeElement() { 7466 return this.mode != null && !this.mode.isEmpty(); 7467 } 7468 7469 public boolean hasMode() { 7470 return this.mode != null && !this.mode.isEmpty(); 7471 } 7472 7473 /** 7474 * @param value {@link #mode} (Mode of this document declaration - whether an application is a producer or consumer.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 7475 */ 7476 public CapabilityStatementDocumentComponent setModeElement(Enumeration<DocumentMode> value) { 7477 this.mode = value; 7478 return this; 7479 } 7480 7481 /** 7482 * @return Mode of this document declaration - whether an application is a producer or consumer. 7483 */ 7484 public DocumentMode getMode() { 7485 return this.mode == null ? null : this.mode.getValue(); 7486 } 7487 7488 /** 7489 * @param value Mode of this document declaration - whether an application is a producer or consumer. 7490 */ 7491 public CapabilityStatementDocumentComponent setMode(DocumentMode value) { 7492 if (this.mode == null) 7493 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); 7494 this.mode.setValue(value); 7495 return this; 7496 } 7497 7498 /** 7499 * @return {@link #documentation} (A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 7500 */ 7501 public StringType getDocumentationElement() { 7502 if (this.documentation == null) 7503 if (Configuration.errorOnAutoCreate()) 7504 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.documentation"); 7505 else if (Configuration.doAutoCreate()) 7506 this.documentation = new StringType(); // bb 7507 return this.documentation; 7508 } 7509 7510 public boolean hasDocumentationElement() { 7511 return this.documentation != null && !this.documentation.isEmpty(); 7512 } 7513 7514 public boolean hasDocumentation() { 7515 return this.documentation != null && !this.documentation.isEmpty(); 7516 } 7517 7518 /** 7519 * @param value {@link #documentation} (A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 7520 */ 7521 public CapabilityStatementDocumentComponent setDocumentationElement(StringType value) { 7522 this.documentation = value; 7523 return this; 7524 } 7525 7526 /** 7527 * @return A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc. 7528 */ 7529 public String getDocumentation() { 7530 return this.documentation == null ? null : this.documentation.getValue(); 7531 } 7532 7533 /** 7534 * @param value A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc. 7535 */ 7536 public CapabilityStatementDocumentComponent setDocumentation(String value) { 7537 if (Utilities.noString(value)) 7538 this.documentation = null; 7539 else { 7540 if (this.documentation == null) 7541 this.documentation = new StringType(); 7542 this.documentation.setValue(value); 7543 } 7544 return this; 7545 } 7546 7547 /** 7548 * @return {@link #profile} (A constraint on a resource used in the document.) 7549 */ 7550 public Reference getProfile() { 7551 if (this.profile == null) 7552 if (Configuration.errorOnAutoCreate()) 7553 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.profile"); 7554 else if (Configuration.doAutoCreate()) 7555 this.profile = new Reference(); // cc 7556 return this.profile; 7557 } 7558 7559 public boolean hasProfile() { 7560 return this.profile != null && !this.profile.isEmpty(); 7561 } 7562 7563 /** 7564 * @param value {@link #profile} (A constraint on a resource used in the document.) 7565 */ 7566 public CapabilityStatementDocumentComponent setProfile(Reference value) { 7567 this.profile = value; 7568 return this; 7569 } 7570 7571 /** 7572 * @return {@link #profile} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A constraint on a resource used in the document.) 7573 */ 7574 public StructureDefinition getProfileTarget() { 7575 if (this.profileTarget == null) 7576 if (Configuration.errorOnAutoCreate()) 7577 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.profile"); 7578 else if (Configuration.doAutoCreate()) 7579 this.profileTarget = new StructureDefinition(); // aa 7580 return this.profileTarget; 7581 } 7582 7583 /** 7584 * @param value {@link #profile} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A constraint on a resource used in the document.) 7585 */ 7586 public CapabilityStatementDocumentComponent setProfileTarget(StructureDefinition value) { 7587 this.profileTarget = value; 7588 return this; 7589 } 7590 7591 protected void listChildren(List<Property> children) { 7592 super.listChildren(children); 7593 children.add(new Property("mode", "code", "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode)); 7594 children.add(new Property("documentation", "string", "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 0, 1, documentation)); 7595 children.add(new Property("profile", "Reference(StructureDefinition)", "A constraint on a resource used in the document.", 0, 1, profile)); 7596 } 7597 7598 @Override 7599 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7600 switch (_hash) { 7601 case 3357091: /*mode*/ return new Property("mode", "code", "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode); 7602 case 1587405498: /*documentation*/ return new Property("documentation", "string", "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 0, 1, documentation); 7603 case -309425751: /*profile*/ return new Property("profile", "Reference(StructureDefinition)", "A constraint on a resource used in the document.", 0, 1, profile); 7604 default: return super.getNamedProperty(_hash, _name, _checkValid); 7605 } 7606 7607 } 7608 7609 @Override 7610 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7611 switch (hash) { 7612 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<DocumentMode> 7613 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 7614 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Reference 7615 default: return super.getProperty(hash, name, checkValid); 7616 } 7617 7618 } 7619 7620 @Override 7621 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7622 switch (hash) { 7623 case 3357091: // mode 7624 value = new DocumentModeEnumFactory().fromType(castToCode(value)); 7625 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 7626 return value; 7627 case 1587405498: // documentation 7628 this.documentation = castToString(value); // StringType 7629 return value; 7630 case -309425751: // profile 7631 this.profile = castToReference(value); // Reference 7632 return value; 7633 default: return super.setProperty(hash, name, value); 7634 } 7635 7636 } 7637 7638 @Override 7639 public Base setProperty(String name, Base value) throws FHIRException { 7640 if (name.equals("mode")) { 7641 value = new DocumentModeEnumFactory().fromType(castToCode(value)); 7642 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 7643 } else if (name.equals("documentation")) { 7644 this.documentation = castToString(value); // StringType 7645 } else if (name.equals("profile")) { 7646 this.profile = castToReference(value); // Reference 7647 } else 7648 return super.setProperty(name, value); 7649 return value; 7650 } 7651 7652 @Override 7653 public Base makeProperty(int hash, String name) throws FHIRException { 7654 switch (hash) { 7655 case 3357091: return getModeElement(); 7656 case 1587405498: return getDocumentationElement(); 7657 case -309425751: return getProfile(); 7658 default: return super.makeProperty(hash, name); 7659 } 7660 7661 } 7662 7663 @Override 7664 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7665 switch (hash) { 7666 case 3357091: /*mode*/ return new String[] {"code"}; 7667 case 1587405498: /*documentation*/ return new String[] {"string"}; 7668 case -309425751: /*profile*/ return new String[] {"Reference"}; 7669 default: return super.getTypesForProperty(hash, name); 7670 } 7671 7672 } 7673 7674 @Override 7675 public Base addChild(String name) throws FHIRException { 7676 if (name.equals("mode")) { 7677 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 7678 } 7679 else if (name.equals("documentation")) { 7680 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 7681 } 7682 else if (name.equals("profile")) { 7683 this.profile = new Reference(); 7684 return this.profile; 7685 } 7686 else 7687 return super.addChild(name); 7688 } 7689 7690 public CapabilityStatementDocumentComponent copy() { 7691 CapabilityStatementDocumentComponent dst = new CapabilityStatementDocumentComponent(); 7692 copyValues(dst); 7693 dst.mode = mode == null ? null : mode.copy(); 7694 dst.documentation = documentation == null ? null : documentation.copy(); 7695 dst.profile = profile == null ? null : profile.copy(); 7696 return dst; 7697 } 7698 7699 @Override 7700 public boolean equalsDeep(Base other_) { 7701 if (!super.equalsDeep(other_)) 7702 return false; 7703 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 7704 return false; 7705 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 7706 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) && compareDeep(profile, o.profile, true) 7707 ; 7708 } 7709 7710 @Override 7711 public boolean equalsShallow(Base other_) { 7712 if (!super.equalsShallow(other_)) 7713 return false; 7714 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 7715 return false; 7716 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 7717 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true); 7718 } 7719 7720 public boolean isEmpty() { 7721 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, profile 7722 ); 7723 } 7724 7725 public String fhirType() { 7726 return "CapabilityStatement.document"; 7727 7728 } 7729 7730 } 7731 7732 /** 7733 * Explaination of why this capability statement is needed and why it has been designed as it has. 7734 */ 7735 @Child(name = "purpose", type = {MarkdownType.class}, order=0, min=0, max=1, modifier=false, summary=false) 7736 @Description(shortDefinition="Why this capability statement is defined", formalDefinition="Explaination of why this capability statement is needed and why it has been designed as it has." ) 7737 protected MarkdownType purpose; 7738 7739 /** 7740 * A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement. 7741 */ 7742 @Child(name = "copyright", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 7743 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement." ) 7744 protected MarkdownType copyright; 7745 7746 /** 7747 * The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase). 7748 */ 7749 @Child(name = "kind", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 7750 @Description(shortDefinition="instance | capability | requirements", formalDefinition="The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase)." ) 7751 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/capability-statement-kind") 7752 protected Enumeration<CapabilityStatementKind> kind; 7753 7754 /** 7755 * Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so. 7756 */ 7757 @Child(name = "instantiates", type = {UriType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7758 @Description(shortDefinition="Canonical URL of another capability statement this implements", formalDefinition="Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so." ) 7759 protected List<UriType> instantiates; 7760 7761 /** 7762 * Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation. 7763 */ 7764 @Child(name = "software", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 7765 @Description(shortDefinition="Software that is covered by this capability statement", formalDefinition="Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation." ) 7766 protected CapabilityStatementSoftwareComponent software; 7767 7768 /** 7769 * Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program. 7770 */ 7771 @Child(name = "implementation", type = {}, order=5, min=0, max=1, modifier=false, summary=true) 7772 @Description(shortDefinition="If this describes a specific instance", formalDefinition="Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program." ) 7773 protected CapabilityStatementImplementationComponent implementation; 7774 7775 /** 7776 * The version of the FHIR specification on which this capability statement is based. 7777 */ 7778 @Child(name = "fhirVersion", type = {IdType.class}, order=6, min=1, max=1, modifier=false, summary=true) 7779 @Description(shortDefinition="FHIR Version the system uses", formalDefinition="The version of the FHIR specification on which this capability statement is based." ) 7780 protected IdType fhirVersion; 7781 7782 /** 7783 * A code that indicates whether the application accepts unknown elements or extensions when reading resources. 7784 */ 7785 @Child(name = "acceptUnknown", type = {CodeType.class}, order=7, min=1, max=1, modifier=false, summary=true) 7786 @Description(shortDefinition="no | extensions | elements | both", formalDefinition="A code that indicates whether the application accepts unknown elements or extensions when reading resources." ) 7787 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/unknown-content-code") 7788 protected Enumeration<UnknownContentCode> acceptUnknown; 7789 7790 /** 7791 * A list of the formats supported by this implementation using their content types. 7792 */ 7793 @Child(name = "format", type = {CodeType.class}, order=8, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7794 @Description(shortDefinition="formats supported (xml | json | ttl | mime type)", formalDefinition="A list of the formats supported by this implementation using their content types." ) 7795 protected List<CodeType> format; 7796 7797 /** 7798 * A list of the patch formats supported by this implementation using their content types. 7799 */ 7800 @Child(name = "patchFormat", type = {CodeType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7801 @Description(shortDefinition="Patch formats supported", formalDefinition="A list of the patch formats supported by this implementation using their content types." ) 7802 protected List<CodeType> patchFormat; 7803 7804 /** 7805 * A list of implementation guides that the server does (or should) support in their entirety. 7806 */ 7807 @Child(name = "implementationGuide", type = {UriType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7808 @Description(shortDefinition="Implementation guides supported", formalDefinition="A list of implementation guides that the server does (or should) support in their entirety." ) 7809 protected List<UriType> implementationGuide; 7810 7811 /** 7812 * A list of profiles that represent different use cases supported by the system. For a server, "supported by the system" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses). 7813 */ 7814 @Child(name = "profile", type = {StructureDefinition.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7815 @Description(shortDefinition="Profiles for use cases supported", formalDefinition="A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses)." ) 7816 protected List<Reference> profile; 7817 /** 7818 * The actual objects that are the target of the reference (A list of profiles that represent different use cases supported by the system. For a server, "supported by the system" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).) 7819 */ 7820 protected List<StructureDefinition> profileTarget; 7821 7822 7823 /** 7824 * A definition of the restful capabilities of the solution, if any. 7825 */ 7826 @Child(name = "rest", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7827 @Description(shortDefinition="If the endpoint is a RESTful one", formalDefinition="A definition of the restful capabilities of the solution, if any." ) 7828 protected List<CapabilityStatementRestComponent> rest; 7829 7830 /** 7831 * A description of the messaging capabilities of the solution. 7832 */ 7833 @Child(name = "messaging", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7834 @Description(shortDefinition="If messaging is supported", formalDefinition="A description of the messaging capabilities of the solution." ) 7835 protected List<CapabilityStatementMessagingComponent> messaging; 7836 7837 /** 7838 * A document definition. 7839 */ 7840 @Child(name = "document", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7841 @Description(shortDefinition="Document definition", formalDefinition="A document definition." ) 7842 protected List<CapabilityStatementDocumentComponent> document; 7843 7844 private static final long serialVersionUID = 227177541L; 7845 7846 /** 7847 * Constructor 7848 */ 7849 public CapabilityStatement() { 7850 super(); 7851 } 7852 7853 /** 7854 * Constructor 7855 */ 7856 public CapabilityStatement(Enumeration<PublicationStatus> status, DateTimeType date, Enumeration<CapabilityStatementKind> kind, IdType fhirVersion, Enumeration<UnknownContentCode> acceptUnknown) { 7857 super(); 7858 this.status = status; 7859 this.date = date; 7860 this.kind = kind; 7861 this.fhirVersion = fhirVersion; 7862 this.acceptUnknown = acceptUnknown; 7863 } 7864 7865 /** 7866 * @return {@link #url} (An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this capability statement is (or will be) published. The URL SHOULD include the major version of the capability statement. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 7867 */ 7868 public UriType getUrlElement() { 7869 if (this.url == null) 7870 if (Configuration.errorOnAutoCreate()) 7871 throw new Error("Attempt to auto-create CapabilityStatement.url"); 7872 else if (Configuration.doAutoCreate()) 7873 this.url = new UriType(); // bb 7874 return this.url; 7875 } 7876 7877 public boolean hasUrlElement() { 7878 return this.url != null && !this.url.isEmpty(); 7879 } 7880 7881 public boolean hasUrl() { 7882 return this.url != null && !this.url.isEmpty(); 7883 } 7884 7885 /** 7886 * @param value {@link #url} (An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this capability statement is (or will be) published. The URL SHOULD include the major version of the capability statement. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 7887 */ 7888 public CapabilityStatement setUrlElement(UriType value) { 7889 this.url = value; 7890 return this; 7891 } 7892 7893 /** 7894 * @return An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this capability statement is (or will be) published. The URL SHOULD include the major version of the capability statement. For more information see [Technical and Business Versions](resource.html#versions). 7895 */ 7896 public String getUrl() { 7897 return this.url == null ? null : this.url.getValue(); 7898 } 7899 7900 /** 7901 * @param value An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this capability statement is (or will be) published. The URL SHOULD include the major version of the capability statement. For more information see [Technical and Business Versions](resource.html#versions). 7902 */ 7903 public CapabilityStatement setUrl(String value) { 7904 if (Utilities.noString(value)) 7905 this.url = null; 7906 else { 7907 if (this.url == null) 7908 this.url = new UriType(); 7909 this.url.setValue(value); 7910 } 7911 return this; 7912 } 7913 7914 /** 7915 * @return {@link #version} (The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 7916 */ 7917 public StringType getVersionElement() { 7918 if (this.version == null) 7919 if (Configuration.errorOnAutoCreate()) 7920 throw new Error("Attempt to auto-create CapabilityStatement.version"); 7921 else if (Configuration.doAutoCreate()) 7922 this.version = new StringType(); // bb 7923 return this.version; 7924 } 7925 7926 public boolean hasVersionElement() { 7927 return this.version != null && !this.version.isEmpty(); 7928 } 7929 7930 public boolean hasVersion() { 7931 return this.version != null && !this.version.isEmpty(); 7932 } 7933 7934 /** 7935 * @param value {@link #version} (The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 7936 */ 7937 public CapabilityStatement setVersionElement(StringType value) { 7938 this.version = value; 7939 return this; 7940 } 7941 7942 /** 7943 * @return The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 7944 */ 7945 public String getVersion() { 7946 return this.version == null ? null : this.version.getValue(); 7947 } 7948 7949 /** 7950 * @param value The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 7951 */ 7952 public CapabilityStatement setVersion(String value) { 7953 if (Utilities.noString(value)) 7954 this.version = null; 7955 else { 7956 if (this.version == null) 7957 this.version = new StringType(); 7958 this.version.setValue(value); 7959 } 7960 return this; 7961 } 7962 7963 /** 7964 * @return {@link #name} (A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 7965 */ 7966 public StringType getNameElement() { 7967 if (this.name == null) 7968 if (Configuration.errorOnAutoCreate()) 7969 throw new Error("Attempt to auto-create CapabilityStatement.name"); 7970 else if (Configuration.doAutoCreate()) 7971 this.name = new StringType(); // bb 7972 return this.name; 7973 } 7974 7975 public boolean hasNameElement() { 7976 return this.name != null && !this.name.isEmpty(); 7977 } 7978 7979 public boolean hasName() { 7980 return this.name != null && !this.name.isEmpty(); 7981 } 7982 7983 /** 7984 * @param value {@link #name} (A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 7985 */ 7986 public CapabilityStatement setNameElement(StringType value) { 7987 this.name = value; 7988 return this; 7989 } 7990 7991 /** 7992 * @return A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation. 7993 */ 7994 public String getName() { 7995 return this.name == null ? null : this.name.getValue(); 7996 } 7997 7998 /** 7999 * @param value A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation. 8000 */ 8001 public CapabilityStatement setName(String value) { 8002 if (Utilities.noString(value)) 8003 this.name = null; 8004 else { 8005 if (this.name == null) 8006 this.name = new StringType(); 8007 this.name.setValue(value); 8008 } 8009 return this; 8010 } 8011 8012 /** 8013 * @return {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 8014 */ 8015 public StringType getTitleElement() { 8016 if (this.title == null) 8017 if (Configuration.errorOnAutoCreate()) 8018 throw new Error("Attempt to auto-create CapabilityStatement.title"); 8019 else if (Configuration.doAutoCreate()) 8020 this.title = new StringType(); // bb 8021 return this.title; 8022 } 8023 8024 public boolean hasTitleElement() { 8025 return this.title != null && !this.title.isEmpty(); 8026 } 8027 8028 public boolean hasTitle() { 8029 return this.title != null && !this.title.isEmpty(); 8030 } 8031 8032 /** 8033 * @param value {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 8034 */ 8035 public CapabilityStatement setTitleElement(StringType value) { 8036 this.title = value; 8037 return this; 8038 } 8039 8040 /** 8041 * @return A short, descriptive, user-friendly title for the capability statement. 8042 */ 8043 public String getTitle() { 8044 return this.title == null ? null : this.title.getValue(); 8045 } 8046 8047 /** 8048 * @param value A short, descriptive, user-friendly title for the capability statement. 8049 */ 8050 public CapabilityStatement setTitle(String value) { 8051 if (Utilities.noString(value)) 8052 this.title = null; 8053 else { 8054 if (this.title == null) 8055 this.title = new StringType(); 8056 this.title.setValue(value); 8057 } 8058 return this; 8059 } 8060 8061 /** 8062 * @return {@link #status} (The status of this capability statement. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8063 */ 8064 public Enumeration<PublicationStatus> getStatusElement() { 8065 if (this.status == null) 8066 if (Configuration.errorOnAutoCreate()) 8067 throw new Error("Attempt to auto-create CapabilityStatement.status"); 8068 else if (Configuration.doAutoCreate()) 8069 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 8070 return this.status; 8071 } 8072 8073 public boolean hasStatusElement() { 8074 return this.status != null && !this.status.isEmpty(); 8075 } 8076 8077 public boolean hasStatus() { 8078 return this.status != null && !this.status.isEmpty(); 8079 } 8080 8081 /** 8082 * @param value {@link #status} (The status of this capability statement. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8083 */ 8084 public CapabilityStatement setStatusElement(Enumeration<PublicationStatus> value) { 8085 this.status = value; 8086 return this; 8087 } 8088 8089 /** 8090 * @return The status of this capability statement. Enables tracking the life-cycle of the content. 8091 */ 8092 public PublicationStatus getStatus() { 8093 return this.status == null ? null : this.status.getValue(); 8094 } 8095 8096 /** 8097 * @param value The status of this capability statement. Enables tracking the life-cycle of the content. 8098 */ 8099 public CapabilityStatement setStatus(PublicationStatus value) { 8100 if (this.status == null) 8101 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 8102 this.status.setValue(value); 8103 return this; 8104 } 8105 8106 /** 8107 * @return {@link #experimental} (A boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 8108 */ 8109 public BooleanType getExperimentalElement() { 8110 if (this.experimental == null) 8111 if (Configuration.errorOnAutoCreate()) 8112 throw new Error("Attempt to auto-create CapabilityStatement.experimental"); 8113 else if (Configuration.doAutoCreate()) 8114 this.experimental = new BooleanType(); // bb 8115 return this.experimental; 8116 } 8117 8118 public boolean hasExperimentalElement() { 8119 return this.experimental != null && !this.experimental.isEmpty(); 8120 } 8121 8122 public boolean hasExperimental() { 8123 return this.experimental != null && !this.experimental.isEmpty(); 8124 } 8125 8126 /** 8127 * @param value {@link #experimental} (A boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 8128 */ 8129 public CapabilityStatement setExperimentalElement(BooleanType value) { 8130 this.experimental = value; 8131 return this; 8132 } 8133 8134 /** 8135 * @return A boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 8136 */ 8137 public boolean getExperimental() { 8138 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 8139 } 8140 8141 /** 8142 * @param value A boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 8143 */ 8144 public CapabilityStatement setExperimental(boolean value) { 8145 if (this.experimental == null) 8146 this.experimental = new BooleanType(); 8147 this.experimental.setValue(value); 8148 return this; 8149 } 8150 8151 /** 8152 * @return {@link #date} (The date (and optionally time) when the capability statement was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 8153 */ 8154 public DateTimeType getDateElement() { 8155 if (this.date == null) 8156 if (Configuration.errorOnAutoCreate()) 8157 throw new Error("Attempt to auto-create CapabilityStatement.date"); 8158 else if (Configuration.doAutoCreate()) 8159 this.date = new DateTimeType(); // bb 8160 return this.date; 8161 } 8162 8163 public boolean hasDateElement() { 8164 return this.date != null && !this.date.isEmpty(); 8165 } 8166 8167 public boolean hasDate() { 8168 return this.date != null && !this.date.isEmpty(); 8169 } 8170 8171 /** 8172 * @param value {@link #date} (The date (and optionally time) when the capability statement was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 8173 */ 8174 public CapabilityStatement setDateElement(DateTimeType value) { 8175 this.date = value; 8176 return this; 8177 } 8178 8179 /** 8180 * @return The date (and optionally time) when the capability statement was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes. 8181 */ 8182 public Date getDate() { 8183 return this.date == null ? null : this.date.getValue(); 8184 } 8185 8186 /** 8187 * @param value The date (and optionally time) when the capability statement was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes. 8188 */ 8189 public CapabilityStatement setDate(Date value) { 8190 if (this.date == null) 8191 this.date = new DateTimeType(); 8192 this.date.setValue(value); 8193 return this; 8194 } 8195 8196 /** 8197 * @return {@link #publisher} (The name of the individual or organization that published the capability statement.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 8198 */ 8199 public StringType getPublisherElement() { 8200 if (this.publisher == null) 8201 if (Configuration.errorOnAutoCreate()) 8202 throw new Error("Attempt to auto-create CapabilityStatement.publisher"); 8203 else if (Configuration.doAutoCreate()) 8204 this.publisher = new StringType(); // bb 8205 return this.publisher; 8206 } 8207 8208 public boolean hasPublisherElement() { 8209 return this.publisher != null && !this.publisher.isEmpty(); 8210 } 8211 8212 public boolean hasPublisher() { 8213 return this.publisher != null && !this.publisher.isEmpty(); 8214 } 8215 8216 /** 8217 * @param value {@link #publisher} (The name of the individual or organization that published the capability statement.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 8218 */ 8219 public CapabilityStatement setPublisherElement(StringType value) { 8220 this.publisher = value; 8221 return this; 8222 } 8223 8224 /** 8225 * @return The name of the individual or organization that published the capability statement. 8226 */ 8227 public String getPublisher() { 8228 return this.publisher == null ? null : this.publisher.getValue(); 8229 } 8230 8231 /** 8232 * @param value The name of the individual or organization that published the capability statement. 8233 */ 8234 public CapabilityStatement setPublisher(String value) { 8235 if (Utilities.noString(value)) 8236 this.publisher = null; 8237 else { 8238 if (this.publisher == null) 8239 this.publisher = new StringType(); 8240 this.publisher.setValue(value); 8241 } 8242 return this; 8243 } 8244 8245 /** 8246 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 8247 */ 8248 public List<ContactDetail> getContact() { 8249 if (this.contact == null) 8250 this.contact = new ArrayList<ContactDetail>(); 8251 return this.contact; 8252 } 8253 8254 /** 8255 * @return Returns a reference to <code>this</code> for easy method chaining 8256 */ 8257 public CapabilityStatement setContact(List<ContactDetail> theContact) { 8258 this.contact = theContact; 8259 return this; 8260 } 8261 8262 public boolean hasContact() { 8263 if (this.contact == null) 8264 return false; 8265 for (ContactDetail item : this.contact) 8266 if (!item.isEmpty()) 8267 return true; 8268 return false; 8269 } 8270 8271 public ContactDetail addContact() { //3 8272 ContactDetail t = new ContactDetail(); 8273 if (this.contact == null) 8274 this.contact = new ArrayList<ContactDetail>(); 8275 this.contact.add(t); 8276 return t; 8277 } 8278 8279 public CapabilityStatement addContact(ContactDetail t) { //3 8280 if (t == null) 8281 return this; 8282 if (this.contact == null) 8283 this.contact = new ArrayList<ContactDetail>(); 8284 this.contact.add(t); 8285 return this; 8286 } 8287 8288 /** 8289 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 8290 */ 8291 public ContactDetail getContactFirstRep() { 8292 if (getContact().isEmpty()) { 8293 addContact(); 8294 } 8295 return getContact().get(0); 8296 } 8297 8298 /** 8299 * @return {@link #description} (A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 8300 */ 8301 public MarkdownType getDescriptionElement() { 8302 if (this.description == null) 8303 if (Configuration.errorOnAutoCreate()) 8304 throw new Error("Attempt to auto-create CapabilityStatement.description"); 8305 else if (Configuration.doAutoCreate()) 8306 this.description = new MarkdownType(); // bb 8307 return this.description; 8308 } 8309 8310 public boolean hasDescriptionElement() { 8311 return this.description != null && !this.description.isEmpty(); 8312 } 8313 8314 public boolean hasDescription() { 8315 return this.description != null && !this.description.isEmpty(); 8316 } 8317 8318 /** 8319 * @param value {@link #description} (A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 8320 */ 8321 public CapabilityStatement setDescriptionElement(MarkdownType value) { 8322 this.description = value; 8323 return this; 8324 } 8325 8326 /** 8327 * @return A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 8328 */ 8329 public String getDescription() { 8330 return this.description == null ? null : this.description.getValue(); 8331 } 8332 8333 /** 8334 * @param value A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 8335 */ 8336 public CapabilityStatement setDescription(String value) { 8337 if (value == null) 8338 this.description = null; 8339 else { 8340 if (this.description == null) 8341 this.description = new MarkdownType(); 8342 this.description.setValue(value); 8343 } 8344 return this; 8345 } 8346 8347 /** 8348 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate capability statement instances.) 8349 */ 8350 public List<UsageContext> getUseContext() { 8351 if (this.useContext == null) 8352 this.useContext = new ArrayList<UsageContext>(); 8353 return this.useContext; 8354 } 8355 8356 /** 8357 * @return Returns a reference to <code>this</code> for easy method chaining 8358 */ 8359 public CapabilityStatement setUseContext(List<UsageContext> theUseContext) { 8360 this.useContext = theUseContext; 8361 return this; 8362 } 8363 8364 public boolean hasUseContext() { 8365 if (this.useContext == null) 8366 return false; 8367 for (UsageContext item : this.useContext) 8368 if (!item.isEmpty()) 8369 return true; 8370 return false; 8371 } 8372 8373 public UsageContext addUseContext() { //3 8374 UsageContext t = new UsageContext(); 8375 if (this.useContext == null) 8376 this.useContext = new ArrayList<UsageContext>(); 8377 this.useContext.add(t); 8378 return t; 8379 } 8380 8381 public CapabilityStatement addUseContext(UsageContext t) { //3 8382 if (t == null) 8383 return this; 8384 if (this.useContext == null) 8385 this.useContext = new ArrayList<UsageContext>(); 8386 this.useContext.add(t); 8387 return this; 8388 } 8389 8390 /** 8391 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 8392 */ 8393 public UsageContext getUseContextFirstRep() { 8394 if (getUseContext().isEmpty()) { 8395 addUseContext(); 8396 } 8397 return getUseContext().get(0); 8398 } 8399 8400 /** 8401 * @return {@link #jurisdiction} (A legal or geographic region in which the capability statement is intended to be used.) 8402 */ 8403 public List<CodeableConcept> getJurisdiction() { 8404 if (this.jurisdiction == null) 8405 this.jurisdiction = new ArrayList<CodeableConcept>(); 8406 return this.jurisdiction; 8407 } 8408 8409 /** 8410 * @return Returns a reference to <code>this</code> for easy method chaining 8411 */ 8412 public CapabilityStatement setJurisdiction(List<CodeableConcept> theJurisdiction) { 8413 this.jurisdiction = theJurisdiction; 8414 return this; 8415 } 8416 8417 public boolean hasJurisdiction() { 8418 if (this.jurisdiction == null) 8419 return false; 8420 for (CodeableConcept item : this.jurisdiction) 8421 if (!item.isEmpty()) 8422 return true; 8423 return false; 8424 } 8425 8426 public CodeableConcept addJurisdiction() { //3 8427 CodeableConcept t = new CodeableConcept(); 8428 if (this.jurisdiction == null) 8429 this.jurisdiction = new ArrayList<CodeableConcept>(); 8430 this.jurisdiction.add(t); 8431 return t; 8432 } 8433 8434 public CapabilityStatement addJurisdiction(CodeableConcept t) { //3 8435 if (t == null) 8436 return this; 8437 if (this.jurisdiction == null) 8438 this.jurisdiction = new ArrayList<CodeableConcept>(); 8439 this.jurisdiction.add(t); 8440 return this; 8441 } 8442 8443 /** 8444 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 8445 */ 8446 public CodeableConcept getJurisdictionFirstRep() { 8447 if (getJurisdiction().isEmpty()) { 8448 addJurisdiction(); 8449 } 8450 return getJurisdiction().get(0); 8451 } 8452 8453 /** 8454 * @return {@link #purpose} (Explaination of why this capability statement is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 8455 */ 8456 public MarkdownType getPurposeElement() { 8457 if (this.purpose == null) 8458 if (Configuration.errorOnAutoCreate()) 8459 throw new Error("Attempt to auto-create CapabilityStatement.purpose"); 8460 else if (Configuration.doAutoCreate()) 8461 this.purpose = new MarkdownType(); // bb 8462 return this.purpose; 8463 } 8464 8465 public boolean hasPurposeElement() { 8466 return this.purpose != null && !this.purpose.isEmpty(); 8467 } 8468 8469 public boolean hasPurpose() { 8470 return this.purpose != null && !this.purpose.isEmpty(); 8471 } 8472 8473 /** 8474 * @param value {@link #purpose} (Explaination of why this capability statement is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 8475 */ 8476 public CapabilityStatement setPurposeElement(MarkdownType value) { 8477 this.purpose = value; 8478 return this; 8479 } 8480 8481 /** 8482 * @return Explaination of why this capability statement is needed and why it has been designed as it has. 8483 */ 8484 public String getPurpose() { 8485 return this.purpose == null ? null : this.purpose.getValue(); 8486 } 8487 8488 /** 8489 * @param value Explaination of why this capability statement is needed and why it has been designed as it has. 8490 */ 8491 public CapabilityStatement setPurpose(String value) { 8492 if (value == null) 8493 this.purpose = null; 8494 else { 8495 if (this.purpose == null) 8496 this.purpose = new MarkdownType(); 8497 this.purpose.setValue(value); 8498 } 8499 return this; 8500 } 8501 8502 /** 8503 * @return {@link #copyright} (A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 8504 */ 8505 public MarkdownType getCopyrightElement() { 8506 if (this.copyright == null) 8507 if (Configuration.errorOnAutoCreate()) 8508 throw new Error("Attempt to auto-create CapabilityStatement.copyright"); 8509 else if (Configuration.doAutoCreate()) 8510 this.copyright = new MarkdownType(); // bb 8511 return this.copyright; 8512 } 8513 8514 public boolean hasCopyrightElement() { 8515 return this.copyright != null && !this.copyright.isEmpty(); 8516 } 8517 8518 public boolean hasCopyright() { 8519 return this.copyright != null && !this.copyright.isEmpty(); 8520 } 8521 8522 /** 8523 * @param value {@link #copyright} (A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 8524 */ 8525 public CapabilityStatement setCopyrightElement(MarkdownType value) { 8526 this.copyright = value; 8527 return this; 8528 } 8529 8530 /** 8531 * @return A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement. 8532 */ 8533 public String getCopyright() { 8534 return this.copyright == null ? null : this.copyright.getValue(); 8535 } 8536 8537 /** 8538 * @param value A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement. 8539 */ 8540 public CapabilityStatement setCopyright(String value) { 8541 if (value == null) 8542 this.copyright = null; 8543 else { 8544 if (this.copyright == null) 8545 this.copyright = new MarkdownType(); 8546 this.copyright.setValue(value); 8547 } 8548 return this; 8549 } 8550 8551 /** 8552 * @return {@link #kind} (The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 8553 */ 8554 public Enumeration<CapabilityStatementKind> getKindElement() { 8555 if (this.kind == null) 8556 if (Configuration.errorOnAutoCreate()) 8557 throw new Error("Attempt to auto-create CapabilityStatement.kind"); 8558 else if (Configuration.doAutoCreate()) 8559 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); // bb 8560 return this.kind; 8561 } 8562 8563 public boolean hasKindElement() { 8564 return this.kind != null && !this.kind.isEmpty(); 8565 } 8566 8567 public boolean hasKind() { 8568 return this.kind != null && !this.kind.isEmpty(); 8569 } 8570 8571 /** 8572 * @param value {@link #kind} (The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 8573 */ 8574 public CapabilityStatement setKindElement(Enumeration<CapabilityStatementKind> value) { 8575 this.kind = value; 8576 return this; 8577 } 8578 8579 /** 8580 * @return The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase). 8581 */ 8582 public CapabilityStatementKind getKind() { 8583 return this.kind == null ? null : this.kind.getValue(); 8584 } 8585 8586 /** 8587 * @param value The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase). 8588 */ 8589 public CapabilityStatement setKind(CapabilityStatementKind value) { 8590 if (this.kind == null) 8591 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); 8592 this.kind.setValue(value); 8593 return this; 8594 } 8595 8596 /** 8597 * @return {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so.) 8598 */ 8599 public List<UriType> getInstantiates() { 8600 if (this.instantiates == null) 8601 this.instantiates = new ArrayList<UriType>(); 8602 return this.instantiates; 8603 } 8604 8605 /** 8606 * @return Returns a reference to <code>this</code> for easy method chaining 8607 */ 8608 public CapabilityStatement setInstantiates(List<UriType> theInstantiates) { 8609 this.instantiates = theInstantiates; 8610 return this; 8611 } 8612 8613 public boolean hasInstantiates() { 8614 if (this.instantiates == null) 8615 return false; 8616 for (UriType item : this.instantiates) 8617 if (!item.isEmpty()) 8618 return true; 8619 return false; 8620 } 8621 8622 /** 8623 * @return {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so.) 8624 */ 8625 public UriType addInstantiatesElement() {//2 8626 UriType t = new UriType(); 8627 if (this.instantiates == null) 8628 this.instantiates = new ArrayList<UriType>(); 8629 this.instantiates.add(t); 8630 return t; 8631 } 8632 8633 /** 8634 * @param value {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so.) 8635 */ 8636 public CapabilityStatement addInstantiates(String value) { //1 8637 UriType t = new UriType(); 8638 t.setValue(value); 8639 if (this.instantiates == null) 8640 this.instantiates = new ArrayList<UriType>(); 8641 this.instantiates.add(t); 8642 return this; 8643 } 8644 8645 /** 8646 * @param value {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so.) 8647 */ 8648 public boolean hasInstantiates(String value) { 8649 if (this.instantiates == null) 8650 return false; 8651 for (UriType v : this.instantiates) 8652 if (v.getValue().equals(value)) // uri 8653 return true; 8654 return false; 8655 } 8656 8657 /** 8658 * @return {@link #software} (Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.) 8659 */ 8660 public CapabilityStatementSoftwareComponent getSoftware() { 8661 if (this.software == null) 8662 if (Configuration.errorOnAutoCreate()) 8663 throw new Error("Attempt to auto-create CapabilityStatement.software"); 8664 else if (Configuration.doAutoCreate()) 8665 this.software = new CapabilityStatementSoftwareComponent(); // cc 8666 return this.software; 8667 } 8668 8669 public boolean hasSoftware() { 8670 return this.software != null && !this.software.isEmpty(); 8671 } 8672 8673 /** 8674 * @param value {@link #software} (Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.) 8675 */ 8676 public CapabilityStatement setSoftware(CapabilityStatementSoftwareComponent value) { 8677 this.software = value; 8678 return this; 8679 } 8680 8681 /** 8682 * @return {@link #implementation} (Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.) 8683 */ 8684 public CapabilityStatementImplementationComponent getImplementation() { 8685 if (this.implementation == null) 8686 if (Configuration.errorOnAutoCreate()) 8687 throw new Error("Attempt to auto-create CapabilityStatement.implementation"); 8688 else if (Configuration.doAutoCreate()) 8689 this.implementation = new CapabilityStatementImplementationComponent(); // cc 8690 return this.implementation; 8691 } 8692 8693 public boolean hasImplementation() { 8694 return this.implementation != null && !this.implementation.isEmpty(); 8695 } 8696 8697 /** 8698 * @param value {@link #implementation} (Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.) 8699 */ 8700 public CapabilityStatement setImplementation(CapabilityStatementImplementationComponent value) { 8701 this.implementation = value; 8702 return this; 8703 } 8704 8705 /** 8706 * @return {@link #fhirVersion} (The version of the FHIR specification on which this capability statement is based.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 8707 */ 8708 public IdType getFhirVersionElement() { 8709 if (this.fhirVersion == null) 8710 if (Configuration.errorOnAutoCreate()) 8711 throw new Error("Attempt to auto-create CapabilityStatement.fhirVersion"); 8712 else if (Configuration.doAutoCreate()) 8713 this.fhirVersion = new IdType(); // bb 8714 return this.fhirVersion; 8715 } 8716 8717 public boolean hasFhirVersionElement() { 8718 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8719 } 8720 8721 public boolean hasFhirVersion() { 8722 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8723 } 8724 8725 /** 8726 * @param value {@link #fhirVersion} (The version of the FHIR specification on which this capability statement is based.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 8727 */ 8728 public CapabilityStatement setFhirVersionElement(IdType value) { 8729 this.fhirVersion = value; 8730 return this; 8731 } 8732 8733 /** 8734 * @return The version of the FHIR specification on which this capability statement is based. 8735 */ 8736 public String getFhirVersion() { 8737 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 8738 } 8739 8740 /** 8741 * @param value The version of the FHIR specification on which this capability statement is based. 8742 */ 8743 public CapabilityStatement setFhirVersion(String value) { 8744 if (this.fhirVersion == null) 8745 this.fhirVersion = new IdType(); 8746 this.fhirVersion.setValue(value); 8747 return this; 8748 } 8749 8750 /** 8751 * @return {@link #acceptUnknown} (A code that indicates whether the application accepts unknown elements or extensions when reading resources.). This is the underlying object with id, value and extensions. The accessor "getAcceptUnknown" gives direct access to the value 8752 */ 8753 public Enumeration<UnknownContentCode> getAcceptUnknownElement() { 8754 if (this.acceptUnknown == null) 8755 if (Configuration.errorOnAutoCreate()) 8756 throw new Error("Attempt to auto-create CapabilityStatement.acceptUnknown"); 8757 else if (Configuration.doAutoCreate()) 8758 this.acceptUnknown = new Enumeration<UnknownContentCode>(new UnknownContentCodeEnumFactory()); // bb 8759 return this.acceptUnknown; 8760 } 8761 8762 public boolean hasAcceptUnknownElement() { 8763 return this.acceptUnknown != null && !this.acceptUnknown.isEmpty(); 8764 } 8765 8766 public boolean hasAcceptUnknown() { 8767 return this.acceptUnknown != null && !this.acceptUnknown.isEmpty(); 8768 } 8769 8770 /** 8771 * @param value {@link #acceptUnknown} (A code that indicates whether the application accepts unknown elements or extensions when reading resources.). This is the underlying object with id, value and extensions. The accessor "getAcceptUnknown" gives direct access to the value 8772 */ 8773 public CapabilityStatement setAcceptUnknownElement(Enumeration<UnknownContentCode> value) { 8774 this.acceptUnknown = value; 8775 return this; 8776 } 8777 8778 /** 8779 * @return A code that indicates whether the application accepts unknown elements or extensions when reading resources. 8780 */ 8781 public UnknownContentCode getAcceptUnknown() { 8782 return this.acceptUnknown == null ? null : this.acceptUnknown.getValue(); 8783 } 8784 8785 /** 8786 * @param value A code that indicates whether the application accepts unknown elements or extensions when reading resources. 8787 */ 8788 public CapabilityStatement setAcceptUnknown(UnknownContentCode value) { 8789 if (this.acceptUnknown == null) 8790 this.acceptUnknown = new Enumeration<UnknownContentCode>(new UnknownContentCodeEnumFactory()); 8791 this.acceptUnknown.setValue(value); 8792 return this; 8793 } 8794 8795 /** 8796 * @return {@link #format} (A list of the formats supported by this implementation using their content types.) 8797 */ 8798 public List<CodeType> getFormat() { 8799 if (this.format == null) 8800 this.format = new ArrayList<CodeType>(); 8801 return this.format; 8802 } 8803 8804 /** 8805 * @return Returns a reference to <code>this</code> for easy method chaining 8806 */ 8807 public CapabilityStatement setFormat(List<CodeType> theFormat) { 8808 this.format = theFormat; 8809 return this; 8810 } 8811 8812 public boolean hasFormat() { 8813 if (this.format == null) 8814 return false; 8815 for (CodeType item : this.format) 8816 if (!item.isEmpty()) 8817 return true; 8818 return false; 8819 } 8820 8821 /** 8822 * @return {@link #format} (A list of the formats supported by this implementation using their content types.) 8823 */ 8824 public CodeType addFormatElement() {//2 8825 CodeType t = new CodeType(); 8826 if (this.format == null) 8827 this.format = new ArrayList<CodeType>(); 8828 this.format.add(t); 8829 return t; 8830 } 8831 8832 /** 8833 * @param value {@link #format} (A list of the formats supported by this implementation using their content types.) 8834 */ 8835 public CapabilityStatement addFormat(String value) { //1 8836 CodeType t = new CodeType(); 8837 t.setValue(value); 8838 if (this.format == null) 8839 this.format = new ArrayList<CodeType>(); 8840 this.format.add(t); 8841 return this; 8842 } 8843 8844 /** 8845 * @param value {@link #format} (A list of the formats supported by this implementation using their content types.) 8846 */ 8847 public boolean hasFormat(String value) { 8848 if (this.format == null) 8849 return false; 8850 for (CodeType v : this.format) 8851 if (v.getValue().equals(value)) // code 8852 return true; 8853 return false; 8854 } 8855 8856 /** 8857 * @return {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8858 */ 8859 public List<CodeType> getPatchFormat() { 8860 if (this.patchFormat == null) 8861 this.patchFormat = new ArrayList<CodeType>(); 8862 return this.patchFormat; 8863 } 8864 8865 /** 8866 * @return Returns a reference to <code>this</code> for easy method chaining 8867 */ 8868 public CapabilityStatement setPatchFormat(List<CodeType> thePatchFormat) { 8869 this.patchFormat = thePatchFormat; 8870 return this; 8871 } 8872 8873 public boolean hasPatchFormat() { 8874 if (this.patchFormat == null) 8875 return false; 8876 for (CodeType item : this.patchFormat) 8877 if (!item.isEmpty()) 8878 return true; 8879 return false; 8880 } 8881 8882 /** 8883 * @return {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8884 */ 8885 public CodeType addPatchFormatElement() {//2 8886 CodeType t = new CodeType(); 8887 if (this.patchFormat == null) 8888 this.patchFormat = new ArrayList<CodeType>(); 8889 this.patchFormat.add(t); 8890 return t; 8891 } 8892 8893 /** 8894 * @param value {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8895 */ 8896 public CapabilityStatement addPatchFormat(String value) { //1 8897 CodeType t = new CodeType(); 8898 t.setValue(value); 8899 if (this.patchFormat == null) 8900 this.patchFormat = new ArrayList<CodeType>(); 8901 this.patchFormat.add(t); 8902 return this; 8903 } 8904 8905 /** 8906 * @param value {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8907 */ 8908 public boolean hasPatchFormat(String value) { 8909 if (this.patchFormat == null) 8910 return false; 8911 for (CodeType v : this.patchFormat) 8912 if (v.getValue().equals(value)) // code 8913 return true; 8914 return false; 8915 } 8916 8917 /** 8918 * @return {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8919 */ 8920 public List<UriType> getImplementationGuide() { 8921 if (this.implementationGuide == null) 8922 this.implementationGuide = new ArrayList<UriType>(); 8923 return this.implementationGuide; 8924 } 8925 8926 /** 8927 * @return Returns a reference to <code>this</code> for easy method chaining 8928 */ 8929 public CapabilityStatement setImplementationGuide(List<UriType> theImplementationGuide) { 8930 this.implementationGuide = theImplementationGuide; 8931 return this; 8932 } 8933 8934 public boolean hasImplementationGuide() { 8935 if (this.implementationGuide == null) 8936 return false; 8937 for (UriType item : this.implementationGuide) 8938 if (!item.isEmpty()) 8939 return true; 8940 return false; 8941 } 8942 8943 /** 8944 * @return {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8945 */ 8946 public UriType addImplementationGuideElement() {//2 8947 UriType t = new UriType(); 8948 if (this.implementationGuide == null) 8949 this.implementationGuide = new ArrayList<UriType>(); 8950 this.implementationGuide.add(t); 8951 return t; 8952 } 8953 8954 /** 8955 * @param value {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8956 */ 8957 public CapabilityStatement addImplementationGuide(String value) { //1 8958 UriType t = new UriType(); 8959 t.setValue(value); 8960 if (this.implementationGuide == null) 8961 this.implementationGuide = new ArrayList<UriType>(); 8962 this.implementationGuide.add(t); 8963 return this; 8964 } 8965 8966 /** 8967 * @param value {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8968 */ 8969 public boolean hasImplementationGuide(String value) { 8970 if (this.implementationGuide == null) 8971 return false; 8972 for (UriType v : this.implementationGuide) 8973 if (v.getValue().equals(value)) // uri 8974 return true; 8975 return false; 8976 } 8977 8978 /** 8979 * @return {@link #profile} (A list of profiles that represent different use cases supported by the system. For a server, "supported by the system" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).) 8980 */ 8981 public List<Reference> getProfile() { 8982 if (this.profile == null) 8983 this.profile = new ArrayList<Reference>(); 8984 return this.profile; 8985 } 8986 8987 /** 8988 * @return Returns a reference to <code>this</code> for easy method chaining 8989 */ 8990 public CapabilityStatement setProfile(List<Reference> theProfile) { 8991 this.profile = theProfile; 8992 return this; 8993 } 8994 8995 public boolean hasProfile() { 8996 if (this.profile == null) 8997 return false; 8998 for (Reference item : this.profile) 8999 if (!item.isEmpty()) 9000 return true; 9001 return false; 9002 } 9003 9004 public Reference addProfile() { //3 9005 Reference t = new Reference(); 9006 if (this.profile == null) 9007 this.profile = new ArrayList<Reference>(); 9008 this.profile.add(t); 9009 return t; 9010 } 9011 9012 public CapabilityStatement addProfile(Reference t) { //3 9013 if (t == null) 9014 return this; 9015 if (this.profile == null) 9016 this.profile = new ArrayList<Reference>(); 9017 this.profile.add(t); 9018 return this; 9019 } 9020 9021 /** 9022 * @return The first repetition of repeating field {@link #profile}, creating it if it does not already exist 9023 */ 9024 public Reference getProfileFirstRep() { 9025 if (getProfile().isEmpty()) { 9026 addProfile(); 9027 } 9028 return getProfile().get(0); 9029 } 9030 9031 /** 9032 * @deprecated Use Reference#setResource(IBaseResource) instead 9033 */ 9034 @Deprecated 9035 public List<StructureDefinition> getProfileTarget() { 9036 if (this.profileTarget == null) 9037 this.profileTarget = new ArrayList<StructureDefinition>(); 9038 return this.profileTarget; 9039 } 9040 9041 /** 9042 * @deprecated Use Reference#setResource(IBaseResource) instead 9043 */ 9044 @Deprecated 9045 public StructureDefinition addProfileTarget() { 9046 StructureDefinition r = new StructureDefinition(); 9047 if (this.profileTarget == null) 9048 this.profileTarget = new ArrayList<StructureDefinition>(); 9049 this.profileTarget.add(r); 9050 return r; 9051 } 9052 9053 /** 9054 * @return {@link #rest} (A definition of the restful capabilities of the solution, if any.) 9055 */ 9056 public List<CapabilityStatementRestComponent> getRest() { 9057 if (this.rest == null) 9058 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 9059 return this.rest; 9060 } 9061 9062 /** 9063 * @return Returns a reference to <code>this</code> for easy method chaining 9064 */ 9065 public CapabilityStatement setRest(List<CapabilityStatementRestComponent> theRest) { 9066 this.rest = theRest; 9067 return this; 9068 } 9069 9070 public boolean hasRest() { 9071 if (this.rest == null) 9072 return false; 9073 for (CapabilityStatementRestComponent item : this.rest) 9074 if (!item.isEmpty()) 9075 return true; 9076 return false; 9077 } 9078 9079 public CapabilityStatementRestComponent addRest() { //3 9080 CapabilityStatementRestComponent t = new CapabilityStatementRestComponent(); 9081 if (this.rest == null) 9082 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 9083 this.rest.add(t); 9084 return t; 9085 } 9086 9087 public CapabilityStatement addRest(CapabilityStatementRestComponent t) { //3 9088 if (t == null) 9089 return this; 9090 if (this.rest == null) 9091 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 9092 this.rest.add(t); 9093 return this; 9094 } 9095 9096 /** 9097 * @return The first repetition of repeating field {@link #rest}, creating it if it does not already exist 9098 */ 9099 public CapabilityStatementRestComponent getRestFirstRep() { 9100 if (getRest().isEmpty()) { 9101 addRest(); 9102 } 9103 return getRest().get(0); 9104 } 9105 9106 /** 9107 * @return {@link #messaging} (A description of the messaging capabilities of the solution.) 9108 */ 9109 public List<CapabilityStatementMessagingComponent> getMessaging() { 9110 if (this.messaging == null) 9111 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 9112 return this.messaging; 9113 } 9114 9115 /** 9116 * @return Returns a reference to <code>this</code> for easy method chaining 9117 */ 9118 public CapabilityStatement setMessaging(List<CapabilityStatementMessagingComponent> theMessaging) { 9119 this.messaging = theMessaging; 9120 return this; 9121 } 9122 9123 public boolean hasMessaging() { 9124 if (this.messaging == null) 9125 return false; 9126 for (CapabilityStatementMessagingComponent item : this.messaging) 9127 if (!item.isEmpty()) 9128 return true; 9129 return false; 9130 } 9131 9132 public CapabilityStatementMessagingComponent addMessaging() { //3 9133 CapabilityStatementMessagingComponent t = new CapabilityStatementMessagingComponent(); 9134 if (this.messaging == null) 9135 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 9136 this.messaging.add(t); 9137 return t; 9138 } 9139 9140 public CapabilityStatement addMessaging(CapabilityStatementMessagingComponent t) { //3 9141 if (t == null) 9142 return this; 9143 if (this.messaging == null) 9144 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 9145 this.messaging.add(t); 9146 return this; 9147 } 9148 9149 /** 9150 * @return The first repetition of repeating field {@link #messaging}, creating it if it does not already exist 9151 */ 9152 public CapabilityStatementMessagingComponent getMessagingFirstRep() { 9153 if (getMessaging().isEmpty()) { 9154 addMessaging(); 9155 } 9156 return getMessaging().get(0); 9157 } 9158 9159 /** 9160 * @return {@link #document} (A document definition.) 9161 */ 9162 public List<CapabilityStatementDocumentComponent> getDocument() { 9163 if (this.document == null) 9164 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 9165 return this.document; 9166 } 9167 9168 /** 9169 * @return Returns a reference to <code>this</code> for easy method chaining 9170 */ 9171 public CapabilityStatement setDocument(List<CapabilityStatementDocumentComponent> theDocument) { 9172 this.document = theDocument; 9173 return this; 9174 } 9175 9176 public boolean hasDocument() { 9177 if (this.document == null) 9178 return false; 9179 for (CapabilityStatementDocumentComponent item : this.document) 9180 if (!item.isEmpty()) 9181 return true; 9182 return false; 9183 } 9184 9185 public CapabilityStatementDocumentComponent addDocument() { //3 9186 CapabilityStatementDocumentComponent t = new CapabilityStatementDocumentComponent(); 9187 if (this.document == null) 9188 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 9189 this.document.add(t); 9190 return t; 9191 } 9192 9193 public CapabilityStatement addDocument(CapabilityStatementDocumentComponent t) { //3 9194 if (t == null) 9195 return this; 9196 if (this.document == null) 9197 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 9198 this.document.add(t); 9199 return this; 9200 } 9201 9202 /** 9203 * @return The first repetition of repeating field {@link #document}, creating it if it does not already exist 9204 */ 9205 public CapabilityStatementDocumentComponent getDocumentFirstRep() { 9206 if (getDocument().isEmpty()) { 9207 addDocument(); 9208 } 9209 return getDocument().get(0); 9210 } 9211 9212 protected void listChildren(List<Property> children) { 9213 super.listChildren(children); 9214 children.add(new Property("url", "uri", "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this capability statement is (or will be) published. The URL SHOULD include the major version of the capability statement. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 9215 children.add(new Property("version", "string", "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 9216 children.add(new Property("name", "string", "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 9217 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title)); 9218 children.add(new Property("status", "code", "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status)); 9219 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 9220 children.add(new Property("date", "dateTime", "The date (and optionally time) when the capability statement was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 0, 1, date)); 9221 children.add(new Property("publisher", "string", "The name of the individual or organization that published the capability statement.", 0, 1, publisher)); 9222 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 9223 children.add(new Property("description", "markdown", "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 0, 1, description)); 9224 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate capability statement instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 9225 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the capability statement is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 9226 children.add(new Property("purpose", "markdown", "Explaination of why this capability statement is needed and why it has been designed as it has.", 0, 1, purpose)); 9227 children.add(new Property("copyright", "markdown", "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 0, 1, copyright)); 9228 children.add(new Property("kind", "code", "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).", 0, 1, kind)); 9229 children.add(new Property("instantiates", "uri", "Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so.", 0, java.lang.Integer.MAX_VALUE, instantiates)); 9230 children.add(new Property("software", "", "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 0, 1, software)); 9231 children.add(new Property("implementation", "", "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 0, 1, implementation)); 9232 children.add(new Property("fhirVersion", "id", "The version of the FHIR specification on which this capability statement is based.", 0, 1, fhirVersion)); 9233 children.add(new Property("acceptUnknown", "code", "A code that indicates whether the application accepts unknown elements or extensions when reading resources.", 0, 1, acceptUnknown)); 9234 children.add(new Property("format", "code", "A list of the formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, format)); 9235 children.add(new Property("patchFormat", "code", "A list of the patch formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, patchFormat)); 9236 children.add(new Property("implementationGuide", "uri", "A list of implementation guides that the server does (or should) support in their entirety.", 0, java.lang.Integer.MAX_VALUE, implementationGuide)); 9237 children.add(new Property("profile", "Reference(StructureDefinition)", "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, java.lang.Integer.MAX_VALUE, profile)); 9238 children.add(new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, java.lang.Integer.MAX_VALUE, rest)); 9239 children.add(new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, java.lang.Integer.MAX_VALUE, messaging)); 9240 children.add(new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document)); 9241 } 9242 9243 @Override 9244 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9245 switch (_hash) { 9246 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this capability statement is (or will be) published. The URL SHOULD include the major version of the capability statement. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 9247 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 9248 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 9249 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title); 9250 case -892481550: /*status*/ return new Property("status", "code", "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status); 9251 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 9252 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the capability statement was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 0, 1, date); 9253 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the capability statement.", 0, 1, publisher); 9254 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 9255 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 0, 1, description); 9256 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate capability statement instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 9257 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the capability statement is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 9258 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this capability statement is needed and why it has been designed as it has.", 0, 1, purpose); 9259 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 0, 1, copyright); 9260 case 3292052: /*kind*/ return new Property("kind", "code", "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).", 0, 1, kind); 9261 case -246883639: /*instantiates*/ return new Property("instantiates", "uri", "Reference to a canonical URL of another CapabilityStatement that this software implements or uses. This capability statement is a published API description that corresponds to a business service. The rest of the capability statement does not need to repeat the details of the referenced resource, but can do so.", 0, java.lang.Integer.MAX_VALUE, instantiates); 9262 case 1319330215: /*software*/ return new Property("software", "", "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 0, 1, software); 9263 case 1683336114: /*implementation*/ return new Property("implementation", "", "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 0, 1, implementation); 9264 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "id", "The version of the FHIR specification on which this capability statement is based.", 0, 1, fhirVersion); 9265 case -1862642142: /*acceptUnknown*/ return new Property("acceptUnknown", "code", "A code that indicates whether the application accepts unknown elements or extensions when reading resources.", 0, 1, acceptUnknown); 9266 case -1268779017: /*format*/ return new Property("format", "code", "A list of the formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, format); 9267 case 172338783: /*patchFormat*/ return new Property("patchFormat", "code", "A list of the patch formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, patchFormat); 9268 case 156966506: /*implementationGuide*/ return new Property("implementationGuide", "uri", "A list of implementation guides that the server does (or should) support in their entirety.", 0, java.lang.Integer.MAX_VALUE, implementationGuide); 9269 case -309425751: /*profile*/ return new Property("profile", "Reference(StructureDefinition)", "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, java.lang.Integer.MAX_VALUE, profile); 9270 case 3496916: /*rest*/ return new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, java.lang.Integer.MAX_VALUE, rest); 9271 case -1440008444: /*messaging*/ return new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, java.lang.Integer.MAX_VALUE, messaging); 9272 case 861720859: /*document*/ return new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document); 9273 default: return super.getNamedProperty(_hash, _name, _checkValid); 9274 } 9275 9276 } 9277 9278 @Override 9279 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9280 switch (hash) { 9281 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 9282 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 9283 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 9284 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 9285 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 9286 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 9287 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 9288 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 9289 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 9290 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 9291 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 9292 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 9293 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 9294 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 9295 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<CapabilityStatementKind> 9296 case -246883639: /*instantiates*/ return this.instantiates == null ? new Base[0] : this.instantiates.toArray(new Base[this.instantiates.size()]); // UriType 9297 case 1319330215: /*software*/ return this.software == null ? new Base[0] : new Base[] {this.software}; // CapabilityStatementSoftwareComponent 9298 case 1683336114: /*implementation*/ return this.implementation == null ? new Base[0] : new Base[] {this.implementation}; // CapabilityStatementImplementationComponent 9299 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : new Base[] {this.fhirVersion}; // IdType 9300 case -1862642142: /*acceptUnknown*/ return this.acceptUnknown == null ? new Base[0] : new Base[] {this.acceptUnknown}; // Enumeration<UnknownContentCode> 9301 case -1268779017: /*format*/ return this.format == null ? new Base[0] : this.format.toArray(new Base[this.format.size()]); // CodeType 9302 case 172338783: /*patchFormat*/ return this.patchFormat == null ? new Base[0] : this.patchFormat.toArray(new Base[this.patchFormat.size()]); // CodeType 9303 case 156966506: /*implementationGuide*/ return this.implementationGuide == null ? new Base[0] : this.implementationGuide.toArray(new Base[this.implementationGuide.size()]); // UriType 9304 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // Reference 9305 case 3496916: /*rest*/ return this.rest == null ? new Base[0] : this.rest.toArray(new Base[this.rest.size()]); // CapabilityStatementRestComponent 9306 case -1440008444: /*messaging*/ return this.messaging == null ? new Base[0] : this.messaging.toArray(new Base[this.messaging.size()]); // CapabilityStatementMessagingComponent 9307 case 861720859: /*document*/ return this.document == null ? new Base[0] : this.document.toArray(new Base[this.document.size()]); // CapabilityStatementDocumentComponent 9308 default: return super.getProperty(hash, name, checkValid); 9309 } 9310 9311 } 9312 9313 @Override 9314 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9315 switch (hash) { 9316 case 116079: // url 9317 this.url = castToUri(value); // UriType 9318 return value; 9319 case 351608024: // version 9320 this.version = castToString(value); // StringType 9321 return value; 9322 case 3373707: // name 9323 this.name = castToString(value); // StringType 9324 return value; 9325 case 110371416: // title 9326 this.title = castToString(value); // StringType 9327 return value; 9328 case -892481550: // status 9329 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 9330 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 9331 return value; 9332 case -404562712: // experimental 9333 this.experimental = castToBoolean(value); // BooleanType 9334 return value; 9335 case 3076014: // date 9336 this.date = castToDateTime(value); // DateTimeType 9337 return value; 9338 case 1447404028: // publisher 9339 this.publisher = castToString(value); // StringType 9340 return value; 9341 case 951526432: // contact 9342 this.getContact().add(castToContactDetail(value)); // ContactDetail 9343 return value; 9344 case -1724546052: // description 9345 this.description = castToMarkdown(value); // MarkdownType 9346 return value; 9347 case -669707736: // useContext 9348 this.getUseContext().add(castToUsageContext(value)); // UsageContext 9349 return value; 9350 case -507075711: // jurisdiction 9351 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 9352 return value; 9353 case -220463842: // purpose 9354 this.purpose = castToMarkdown(value); // MarkdownType 9355 return value; 9356 case 1522889671: // copyright 9357 this.copyright = castToMarkdown(value); // MarkdownType 9358 return value; 9359 case 3292052: // kind 9360 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 9361 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 9362 return value; 9363 case -246883639: // instantiates 9364 this.getInstantiates().add(castToUri(value)); // UriType 9365 return value; 9366 case 1319330215: // software 9367 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 9368 return value; 9369 case 1683336114: // implementation 9370 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 9371 return value; 9372 case 461006061: // fhirVersion 9373 this.fhirVersion = castToId(value); // IdType 9374 return value; 9375 case -1862642142: // acceptUnknown 9376 value = new UnknownContentCodeEnumFactory().fromType(castToCode(value)); 9377 this.acceptUnknown = (Enumeration) value; // Enumeration<UnknownContentCode> 9378 return value; 9379 case -1268779017: // format 9380 this.getFormat().add(castToCode(value)); // CodeType 9381 return value; 9382 case 172338783: // patchFormat 9383 this.getPatchFormat().add(castToCode(value)); // CodeType 9384 return value; 9385 case 156966506: // implementationGuide 9386 this.getImplementationGuide().add(castToUri(value)); // UriType 9387 return value; 9388 case -309425751: // profile 9389 this.getProfile().add(castToReference(value)); // Reference 9390 return value; 9391 case 3496916: // rest 9392 this.getRest().add((CapabilityStatementRestComponent) value); // CapabilityStatementRestComponent 9393 return value; 9394 case -1440008444: // messaging 9395 this.getMessaging().add((CapabilityStatementMessagingComponent) value); // CapabilityStatementMessagingComponent 9396 return value; 9397 case 861720859: // document 9398 this.getDocument().add((CapabilityStatementDocumentComponent) value); // CapabilityStatementDocumentComponent 9399 return value; 9400 default: return super.setProperty(hash, name, value); 9401 } 9402 9403 } 9404 9405 @Override 9406 public Base setProperty(String name, Base value) throws FHIRException { 9407 if (name.equals("url")) { 9408 this.url = castToUri(value); // UriType 9409 } else if (name.equals("version")) { 9410 this.version = castToString(value); // StringType 9411 } else if (name.equals("name")) { 9412 this.name = castToString(value); // StringType 9413 } else if (name.equals("title")) { 9414 this.title = castToString(value); // StringType 9415 } else if (name.equals("status")) { 9416 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 9417 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 9418 } else if (name.equals("experimental")) { 9419 this.experimental = castToBoolean(value); // BooleanType 9420 } else if (name.equals("date")) { 9421 this.date = castToDateTime(value); // DateTimeType 9422 } else if (name.equals("publisher")) { 9423 this.publisher = castToString(value); // StringType 9424 } else if (name.equals("contact")) { 9425 this.getContact().add(castToContactDetail(value)); 9426 } else if (name.equals("description")) { 9427 this.description = castToMarkdown(value); // MarkdownType 9428 } else if (name.equals("useContext")) { 9429 this.getUseContext().add(castToUsageContext(value)); 9430 } else if (name.equals("jurisdiction")) { 9431 this.getJurisdiction().add(castToCodeableConcept(value)); 9432 } else if (name.equals("purpose")) { 9433 this.purpose = castToMarkdown(value); // MarkdownType 9434 } else if (name.equals("copyright")) { 9435 this.copyright = castToMarkdown(value); // MarkdownType 9436 } else if (name.equals("kind")) { 9437 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 9438 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 9439 } else if (name.equals("instantiates")) { 9440 this.getInstantiates().add(castToUri(value)); 9441 } else if (name.equals("software")) { 9442 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 9443 } else if (name.equals("implementation")) { 9444 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 9445 } else if (name.equals("fhirVersion")) { 9446 this.fhirVersion = castToId(value); // IdType 9447 } else if (name.equals("acceptUnknown")) { 9448 value = new UnknownContentCodeEnumFactory().fromType(castToCode(value)); 9449 this.acceptUnknown = (Enumeration) value; // Enumeration<UnknownContentCode> 9450 } else if (name.equals("format")) { 9451 this.getFormat().add(castToCode(value)); 9452 } else if (name.equals("patchFormat")) { 9453 this.getPatchFormat().add(castToCode(value)); 9454 } else if (name.equals("implementationGuide")) { 9455 this.getImplementationGuide().add(castToUri(value)); 9456 } else if (name.equals("profile")) { 9457 this.getProfile().add(castToReference(value)); 9458 } else if (name.equals("rest")) { 9459 this.getRest().add((CapabilityStatementRestComponent) value); 9460 } else if (name.equals("messaging")) { 9461 this.getMessaging().add((CapabilityStatementMessagingComponent) value); 9462 } else if (name.equals("document")) { 9463 this.getDocument().add((CapabilityStatementDocumentComponent) value); 9464 } else 9465 return super.setProperty(name, value); 9466 return value; 9467 } 9468 9469 @Override 9470 public Base makeProperty(int hash, String name) throws FHIRException { 9471 switch (hash) { 9472 case 116079: return getUrlElement(); 9473 case 351608024: return getVersionElement(); 9474 case 3373707: return getNameElement(); 9475 case 110371416: return getTitleElement(); 9476 case -892481550: return getStatusElement(); 9477 case -404562712: return getExperimentalElement(); 9478 case 3076014: return getDateElement(); 9479 case 1447404028: return getPublisherElement(); 9480 case 951526432: return addContact(); 9481 case -1724546052: return getDescriptionElement(); 9482 case -669707736: return addUseContext(); 9483 case -507075711: return addJurisdiction(); 9484 case -220463842: return getPurposeElement(); 9485 case 1522889671: return getCopyrightElement(); 9486 case 3292052: return getKindElement(); 9487 case -246883639: return addInstantiatesElement(); 9488 case 1319330215: return getSoftware(); 9489 case 1683336114: return getImplementation(); 9490 case 461006061: return getFhirVersionElement(); 9491 case -1862642142: return getAcceptUnknownElement(); 9492 case -1268779017: return addFormatElement(); 9493 case 172338783: return addPatchFormatElement(); 9494 case 156966506: return addImplementationGuideElement(); 9495 case -309425751: return addProfile(); 9496 case 3496916: return addRest(); 9497 case -1440008444: return addMessaging(); 9498 case 861720859: return addDocument(); 9499 default: return super.makeProperty(hash, name); 9500 } 9501 9502 } 9503 9504 @Override 9505 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9506 switch (hash) { 9507 case 116079: /*url*/ return new String[] {"uri"}; 9508 case 351608024: /*version*/ return new String[] {"string"}; 9509 case 3373707: /*name*/ return new String[] {"string"}; 9510 case 110371416: /*title*/ return new String[] {"string"}; 9511 case -892481550: /*status*/ return new String[] {"code"}; 9512 case -404562712: /*experimental*/ return new String[] {"boolean"}; 9513 case 3076014: /*date*/ return new String[] {"dateTime"}; 9514 case 1447404028: /*publisher*/ return new String[] {"string"}; 9515 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 9516 case -1724546052: /*description*/ return new String[] {"markdown"}; 9517 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 9518 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 9519 case -220463842: /*purpose*/ return new String[] {"markdown"}; 9520 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 9521 case 3292052: /*kind*/ return new String[] {"code"}; 9522 case -246883639: /*instantiates*/ return new String[] {"uri"}; 9523 case 1319330215: /*software*/ return new String[] {}; 9524 case 1683336114: /*implementation*/ return new String[] {}; 9525 case 461006061: /*fhirVersion*/ return new String[] {"id"}; 9526 case -1862642142: /*acceptUnknown*/ return new String[] {"code"}; 9527 case -1268779017: /*format*/ return new String[] {"code"}; 9528 case 172338783: /*patchFormat*/ return new String[] {"code"}; 9529 case 156966506: /*implementationGuide*/ return new String[] {"uri"}; 9530 case -309425751: /*profile*/ return new String[] {"Reference"}; 9531 case 3496916: /*rest*/ return new String[] {}; 9532 case -1440008444: /*messaging*/ return new String[] {}; 9533 case 861720859: /*document*/ return new String[] {}; 9534 default: return super.getTypesForProperty(hash, name); 9535 } 9536 9537 } 9538 9539 @Override 9540 public Base addChild(String name) throws FHIRException { 9541 if (name.equals("url")) { 9542 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.url"); 9543 } 9544 else if (name.equals("version")) { 9545 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.version"); 9546 } 9547 else if (name.equals("name")) { 9548 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 9549 } 9550 else if (name.equals("title")) { 9551 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.title"); 9552 } 9553 else if (name.equals("status")) { 9554 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.status"); 9555 } 9556 else if (name.equals("experimental")) { 9557 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.experimental"); 9558 } 9559 else if (name.equals("date")) { 9560 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.date"); 9561 } 9562 else if (name.equals("publisher")) { 9563 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.publisher"); 9564 } 9565 else if (name.equals("contact")) { 9566 return addContact(); 9567 } 9568 else if (name.equals("description")) { 9569 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 9570 } 9571 else if (name.equals("useContext")) { 9572 return addUseContext(); 9573 } 9574 else if (name.equals("jurisdiction")) { 9575 return addJurisdiction(); 9576 } 9577 else if (name.equals("purpose")) { 9578 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.purpose"); 9579 } 9580 else if (name.equals("copyright")) { 9581 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.copyright"); 9582 } 9583 else if (name.equals("kind")) { 9584 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.kind"); 9585 } 9586 else if (name.equals("instantiates")) { 9587 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.instantiates"); 9588 } 9589 else if (name.equals("software")) { 9590 this.software = new CapabilityStatementSoftwareComponent(); 9591 return this.software; 9592 } 9593 else if (name.equals("implementation")) { 9594 this.implementation = new CapabilityStatementImplementationComponent(); 9595 return this.implementation; 9596 } 9597 else if (name.equals("fhirVersion")) { 9598 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.fhirVersion"); 9599 } 9600 else if (name.equals("acceptUnknown")) { 9601 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.acceptUnknown"); 9602 } 9603 else if (name.equals("format")) { 9604 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.format"); 9605 } 9606 else if (name.equals("patchFormat")) { 9607 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.patchFormat"); 9608 } 9609 else if (name.equals("implementationGuide")) { 9610 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.implementationGuide"); 9611 } 9612 else if (name.equals("profile")) { 9613 return addProfile(); 9614 } 9615 else if (name.equals("rest")) { 9616 return addRest(); 9617 } 9618 else if (name.equals("messaging")) { 9619 return addMessaging(); 9620 } 9621 else if (name.equals("document")) { 9622 return addDocument(); 9623 } 9624 else 9625 return super.addChild(name); 9626 } 9627 9628 public String fhirType() { 9629 return "CapabilityStatement"; 9630 9631 } 9632 9633 public CapabilityStatement copy() { 9634 CapabilityStatement dst = new CapabilityStatement(); 9635 copyValues(dst); 9636 dst.url = url == null ? null : url.copy(); 9637 dst.version = version == null ? null : version.copy(); 9638 dst.name = name == null ? null : name.copy(); 9639 dst.title = title == null ? null : title.copy(); 9640 dst.status = status == null ? null : status.copy(); 9641 dst.experimental = experimental == null ? null : experimental.copy(); 9642 dst.date = date == null ? null : date.copy(); 9643 dst.publisher = publisher == null ? null : publisher.copy(); 9644 if (contact != null) { 9645 dst.contact = new ArrayList<ContactDetail>(); 9646 for (ContactDetail i : contact) 9647 dst.contact.add(i.copy()); 9648 }; 9649 dst.description = description == null ? null : description.copy(); 9650 if (useContext != null) { 9651 dst.useContext = new ArrayList<UsageContext>(); 9652 for (UsageContext i : useContext) 9653 dst.useContext.add(i.copy()); 9654 }; 9655 if (jurisdiction != null) { 9656 dst.jurisdiction = new ArrayList<CodeableConcept>(); 9657 for (CodeableConcept i : jurisdiction) 9658 dst.jurisdiction.add(i.copy()); 9659 }; 9660 dst.purpose = purpose == null ? null : purpose.copy(); 9661 dst.copyright = copyright == null ? null : copyright.copy(); 9662 dst.kind = kind == null ? null : kind.copy(); 9663 if (instantiates != null) { 9664 dst.instantiates = new ArrayList<UriType>(); 9665 for (UriType i : instantiates) 9666 dst.instantiates.add(i.copy()); 9667 }; 9668 dst.software = software == null ? null : software.copy(); 9669 dst.implementation = implementation == null ? null : implementation.copy(); 9670 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 9671 dst.acceptUnknown = acceptUnknown == null ? null : acceptUnknown.copy(); 9672 if (format != null) { 9673 dst.format = new ArrayList<CodeType>(); 9674 for (CodeType i : format) 9675 dst.format.add(i.copy()); 9676 }; 9677 if (patchFormat != null) { 9678 dst.patchFormat = new ArrayList<CodeType>(); 9679 for (CodeType i : patchFormat) 9680 dst.patchFormat.add(i.copy()); 9681 }; 9682 if (implementationGuide != null) { 9683 dst.implementationGuide = new ArrayList<UriType>(); 9684 for (UriType i : implementationGuide) 9685 dst.implementationGuide.add(i.copy()); 9686 }; 9687 if (profile != null) { 9688 dst.profile = new ArrayList<Reference>(); 9689 for (Reference i : profile) 9690 dst.profile.add(i.copy()); 9691 }; 9692 if (rest != null) { 9693 dst.rest = new ArrayList<CapabilityStatementRestComponent>(); 9694 for (CapabilityStatementRestComponent i : rest) 9695 dst.rest.add(i.copy()); 9696 }; 9697 if (messaging != null) { 9698 dst.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 9699 for (CapabilityStatementMessagingComponent i : messaging) 9700 dst.messaging.add(i.copy()); 9701 }; 9702 if (document != null) { 9703 dst.document = new ArrayList<CapabilityStatementDocumentComponent>(); 9704 for (CapabilityStatementDocumentComponent i : document) 9705 dst.document.add(i.copy()); 9706 }; 9707 return dst; 9708 } 9709 9710 protected CapabilityStatement typedCopy() { 9711 return copy(); 9712 } 9713 9714 @Override 9715 public boolean equalsDeep(Base other_) { 9716 if (!super.equalsDeep(other_)) 9717 return false; 9718 if (!(other_ instanceof CapabilityStatement)) 9719 return false; 9720 CapabilityStatement o = (CapabilityStatement) other_; 9721 return compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(kind, o.kind, true) 9722 && compareDeep(instantiates, o.instantiates, true) && compareDeep(software, o.software, true) && compareDeep(implementation, o.implementation, true) 9723 && compareDeep(fhirVersion, o.fhirVersion, true) && compareDeep(acceptUnknown, o.acceptUnknown, true) 9724 && compareDeep(format, o.format, true) && compareDeep(patchFormat, o.patchFormat, true) && compareDeep(implementationGuide, o.implementationGuide, true) 9725 && compareDeep(profile, o.profile, true) && compareDeep(rest, o.rest, true) && compareDeep(messaging, o.messaging, true) 9726 && compareDeep(document, o.document, true); 9727 } 9728 9729 @Override 9730 public boolean equalsShallow(Base other_) { 9731 if (!super.equalsShallow(other_)) 9732 return false; 9733 if (!(other_ instanceof CapabilityStatement)) 9734 return false; 9735 CapabilityStatement o = (CapabilityStatement) other_; 9736 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(kind, o.kind, true) 9737 && compareValues(instantiates, o.instantiates, true) && compareValues(fhirVersion, o.fhirVersion, true) 9738 && compareValues(acceptUnknown, o.acceptUnknown, true) && compareValues(format, o.format, true) && compareValues(patchFormat, o.patchFormat, true) 9739 && compareValues(implementationGuide, o.implementationGuide, true); 9740 } 9741 9742 public boolean isEmpty() { 9743 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, copyright, kind 9744 , instantiates, software, implementation, fhirVersion, acceptUnknown, format, patchFormat 9745 , implementationGuide, profile, rest, messaging, document); 9746 } 9747 9748 @Override 9749 public ResourceType getResourceType() { 9750 return ResourceType.CapabilityStatement; 9751 } 9752 9753 /** 9754 * Search parameter: <b>date</b> 9755 * <p> 9756 * Description: <b>The capability statement publication date</b><br> 9757 * Type: <b>date</b><br> 9758 * Path: <b>CapabilityStatement.date</b><br> 9759 * </p> 9760 */ 9761 @SearchParamDefinition(name="date", path="CapabilityStatement.date", description="The capability statement publication date", type="date" ) 9762 public static final String SP_DATE = "date"; 9763 /** 9764 * <b>Fluent Client</b> search parameter constant for <b>date</b> 9765 * <p> 9766 * Description: <b>The capability statement publication date</b><br> 9767 * Type: <b>date</b><br> 9768 * Path: <b>CapabilityStatement.date</b><br> 9769 * </p> 9770 */ 9771 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 9772 9773 /** 9774 * Search parameter: <b>resource-profile</b> 9775 * <p> 9776 * Description: <b>A profile id invoked in a capability statement</b><br> 9777 * Type: <b>reference</b><br> 9778 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 9779 * </p> 9780 */ 9781 @SearchParamDefinition(name="resource-profile", path="CapabilityStatement.rest.resource.profile", description="A profile id invoked in a capability statement", type="reference", target={StructureDefinition.class } ) 9782 public static final String SP_RESOURCE_PROFILE = "resource-profile"; 9783 /** 9784 * <b>Fluent Client</b> search parameter constant for <b>resource-profile</b> 9785 * <p> 9786 * Description: <b>A profile id invoked in a capability statement</b><br> 9787 * Type: <b>reference</b><br> 9788 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 9789 * </p> 9790 */ 9791 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESOURCE_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESOURCE_PROFILE); 9792 9793/** 9794 * Constant for fluent queries to be used to add include statements. Specifies 9795 * the path value of "<b>CapabilityStatement:resource-profile</b>". 9796 */ 9797 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESOURCE_PROFILE = new ca.uhn.fhir.model.api.Include("CapabilityStatement:resource-profile").toLocked(); 9798 9799 /** 9800 * Search parameter: <b>software</b> 9801 * <p> 9802 * Description: <b>Part of a the name of a software application</b><br> 9803 * Type: <b>string</b><br> 9804 * Path: <b>CapabilityStatement.software.name</b><br> 9805 * </p> 9806 */ 9807 @SearchParamDefinition(name="software", path="CapabilityStatement.software.name", description="Part of a the name of a software application", type="string" ) 9808 public static final String SP_SOFTWARE = "software"; 9809 /** 9810 * <b>Fluent Client</b> search parameter constant for <b>software</b> 9811 * <p> 9812 * Description: <b>Part of a the name of a software application</b><br> 9813 * Type: <b>string</b><br> 9814 * Path: <b>CapabilityStatement.software.name</b><br> 9815 * </p> 9816 */ 9817 public static final ca.uhn.fhir.rest.gclient.StringClientParam SOFTWARE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SOFTWARE); 9818 9819 /** 9820 * Search parameter: <b>resource</b> 9821 * <p> 9822 * Description: <b>Name of a resource mentioned in a capability statement</b><br> 9823 * Type: <b>token</b><br> 9824 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 9825 * </p> 9826 */ 9827 @SearchParamDefinition(name="resource", path="CapabilityStatement.rest.resource.type", description="Name of a resource mentioned in a capability statement", type="token" ) 9828 public static final String SP_RESOURCE = "resource"; 9829 /** 9830 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 9831 * <p> 9832 * Description: <b>Name of a resource mentioned in a capability statement</b><br> 9833 * Type: <b>token</b><br> 9834 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 9835 * </p> 9836 */ 9837 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESOURCE); 9838 9839 /** 9840 * Search parameter: <b>jurisdiction</b> 9841 * <p> 9842 * Description: <b>Intended jurisdiction for the capability statement</b><br> 9843 * Type: <b>token</b><br> 9844 * Path: <b>CapabilityStatement.jurisdiction</b><br> 9845 * </p> 9846 */ 9847 @SearchParamDefinition(name="jurisdiction", path="CapabilityStatement.jurisdiction", description="Intended jurisdiction for the capability statement", type="token" ) 9848 public static final String SP_JURISDICTION = "jurisdiction"; 9849 /** 9850 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 9851 * <p> 9852 * Description: <b>Intended jurisdiction for the capability statement</b><br> 9853 * Type: <b>token</b><br> 9854 * Path: <b>CapabilityStatement.jurisdiction</b><br> 9855 * </p> 9856 */ 9857 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 9858 9859 /** 9860 * Search parameter: <b>format</b> 9861 * <p> 9862 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 9863 * Type: <b>token</b><br> 9864 * Path: <b>CapabilityStatement.format</b><br> 9865 * </p> 9866 */ 9867 @SearchParamDefinition(name="format", path="CapabilityStatement.format", description="formats supported (xml | json | ttl | mime type)", type="token" ) 9868 public static final String SP_FORMAT = "format"; 9869 /** 9870 * <b>Fluent Client</b> search parameter constant for <b>format</b> 9871 * <p> 9872 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 9873 * Type: <b>token</b><br> 9874 * Path: <b>CapabilityStatement.format</b><br> 9875 * </p> 9876 */ 9877 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORMAT); 9878 9879 /** 9880 * Search parameter: <b>description</b> 9881 * <p> 9882 * Description: <b>The description of the capability statement</b><br> 9883 * Type: <b>string</b><br> 9884 * Path: <b>CapabilityStatement.description</b><br> 9885 * </p> 9886 */ 9887 @SearchParamDefinition(name="description", path="CapabilityStatement.description", description="The description of the capability statement", type="string" ) 9888 public static final String SP_DESCRIPTION = "description"; 9889 /** 9890 * <b>Fluent Client</b> search parameter constant for <b>description</b> 9891 * <p> 9892 * Description: <b>The description of the capability statement</b><br> 9893 * Type: <b>string</b><br> 9894 * Path: <b>CapabilityStatement.description</b><br> 9895 * </p> 9896 */ 9897 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 9898 9899 /** 9900 * Search parameter: <b>title</b> 9901 * <p> 9902 * Description: <b>The human-friendly name of the capability statement</b><br> 9903 * Type: <b>string</b><br> 9904 * Path: <b>CapabilityStatement.title</b><br> 9905 * </p> 9906 */ 9907 @SearchParamDefinition(name="title", path="CapabilityStatement.title", description="The human-friendly name of the capability statement", type="string" ) 9908 public static final String SP_TITLE = "title"; 9909 /** 9910 * <b>Fluent Client</b> search parameter constant for <b>title</b> 9911 * <p> 9912 * Description: <b>The human-friendly name of the capability statement</b><br> 9913 * Type: <b>string</b><br> 9914 * Path: <b>CapabilityStatement.title</b><br> 9915 * </p> 9916 */ 9917 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 9918 9919 /** 9920 * Search parameter: <b>fhirversion</b> 9921 * <p> 9922 * Description: <b>The version of FHIR</b><br> 9923 * Type: <b>token</b><br> 9924 * Path: <b>CapabilityStatement.version</b><br> 9925 * </p> 9926 */ 9927 @SearchParamDefinition(name="fhirversion", path="CapabilityStatement.version", description="The version of FHIR", type="token" ) 9928 public static final String SP_FHIRVERSION = "fhirversion"; 9929 /** 9930 * <b>Fluent Client</b> search parameter constant for <b>fhirversion</b> 9931 * <p> 9932 * Description: <b>The version of FHIR</b><br> 9933 * Type: <b>token</b><br> 9934 * Path: <b>CapabilityStatement.version</b><br> 9935 * </p> 9936 */ 9937 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FHIRVERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FHIRVERSION); 9938 9939 /** 9940 * Search parameter: <b>version</b> 9941 * <p> 9942 * Description: <b>The business version of the capability statement</b><br> 9943 * Type: <b>token</b><br> 9944 * Path: <b>CapabilityStatement.version</b><br> 9945 * </p> 9946 */ 9947 @SearchParamDefinition(name="version", path="CapabilityStatement.version", description="The business version of the capability statement", type="token" ) 9948 public static final String SP_VERSION = "version"; 9949 /** 9950 * <b>Fluent Client</b> search parameter constant for <b>version</b> 9951 * <p> 9952 * Description: <b>The business version of the capability statement</b><br> 9953 * Type: <b>token</b><br> 9954 * Path: <b>CapabilityStatement.version</b><br> 9955 * </p> 9956 */ 9957 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 9958 9959 /** 9960 * Search parameter: <b>url</b> 9961 * <p> 9962 * Description: <b>The uri that identifies the capability statement</b><br> 9963 * Type: <b>uri</b><br> 9964 * Path: <b>CapabilityStatement.url</b><br> 9965 * </p> 9966 */ 9967 @SearchParamDefinition(name="url", path="CapabilityStatement.url", description="The uri that identifies the capability statement", type="uri" ) 9968 public static final String SP_URL = "url"; 9969 /** 9970 * <b>Fluent Client</b> search parameter constant for <b>url</b> 9971 * <p> 9972 * Description: <b>The uri that identifies the capability statement</b><br> 9973 * Type: <b>uri</b><br> 9974 * Path: <b>CapabilityStatement.url</b><br> 9975 * </p> 9976 */ 9977 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 9978 9979 /** 9980 * Search parameter: <b>supported-profile</b> 9981 * <p> 9982 * Description: <b>Profiles for use cases supported</b><br> 9983 * Type: <b>reference</b><br> 9984 * Path: <b>CapabilityStatement.profile</b><br> 9985 * </p> 9986 */ 9987 @SearchParamDefinition(name="supported-profile", path="CapabilityStatement.profile", description="Profiles for use cases supported", type="reference", target={StructureDefinition.class } ) 9988 public static final String SP_SUPPORTED_PROFILE = "supported-profile"; 9989 /** 9990 * <b>Fluent Client</b> search parameter constant for <b>supported-profile</b> 9991 * <p> 9992 * Description: <b>Profiles for use cases supported</b><br> 9993 * Type: <b>reference</b><br> 9994 * Path: <b>CapabilityStatement.profile</b><br> 9995 * </p> 9996 */ 9997 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTED_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPORTED_PROFILE); 9998 9999/** 10000 * Constant for fluent queries to be used to add include statements. Specifies 10001 * the path value of "<b>CapabilityStatement:supported-profile</b>". 10002 */ 10003 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTED_PROFILE = new ca.uhn.fhir.model.api.Include("CapabilityStatement:supported-profile").toLocked(); 10004 10005 /** 10006 * Search parameter: <b>mode</b> 10007 * <p> 10008 * Description: <b>Mode - restful (server/client) or messaging (sender/receiver)</b><br> 10009 * Type: <b>token</b><br> 10010 * Path: <b>CapabilityStatement.rest.mode</b><br> 10011 * </p> 10012 */ 10013 @SearchParamDefinition(name="mode", path="CapabilityStatement.rest.mode", description="Mode - restful (server/client) or messaging (sender/receiver)", type="token" ) 10014 public static final String SP_MODE = "mode"; 10015 /** 10016 * <b>Fluent Client</b> search parameter constant for <b>mode</b> 10017 * <p> 10018 * Description: <b>Mode - restful (server/client) or messaging (sender/receiver)</b><br> 10019 * Type: <b>token</b><br> 10020 * Path: <b>CapabilityStatement.rest.mode</b><br> 10021 * </p> 10022 */ 10023 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MODE); 10024 10025 /** 10026 * Search parameter: <b>security-service</b> 10027 * <p> 10028 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates</b><br> 10029 * Type: <b>token</b><br> 10030 * Path: <b>CapabilityStatement.rest.security.service</b><br> 10031 * </p> 10032 */ 10033 @SearchParamDefinition(name="security-service", path="CapabilityStatement.rest.security.service", description="OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", type="token" ) 10034 public static final String SP_SECURITY_SERVICE = "security-service"; 10035 /** 10036 * <b>Fluent Client</b> search parameter constant for <b>security-service</b> 10037 * <p> 10038 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates</b><br> 10039 * Type: <b>token</b><br> 10040 * Path: <b>CapabilityStatement.rest.security.service</b><br> 10041 * </p> 10042 */ 10043 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_SERVICE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITY_SERVICE); 10044 10045 /** 10046 * Search parameter: <b>name</b> 10047 * <p> 10048 * Description: <b>Computationally friendly name of the capability statement</b><br> 10049 * Type: <b>string</b><br> 10050 * Path: <b>CapabilityStatement.name</b><br> 10051 * </p> 10052 */ 10053 @SearchParamDefinition(name="name", path="CapabilityStatement.name", description="Computationally friendly name of the capability statement", type="string" ) 10054 public static final String SP_NAME = "name"; 10055 /** 10056 * <b>Fluent Client</b> search parameter constant for <b>name</b> 10057 * <p> 10058 * Description: <b>Computationally friendly name of the capability statement</b><br> 10059 * Type: <b>string</b><br> 10060 * Path: <b>CapabilityStatement.name</b><br> 10061 * </p> 10062 */ 10063 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 10064 10065 /** 10066 * Search parameter: <b>publisher</b> 10067 * <p> 10068 * Description: <b>Name of the publisher of the capability statement</b><br> 10069 * Type: <b>string</b><br> 10070 * Path: <b>CapabilityStatement.publisher</b><br> 10071 * </p> 10072 */ 10073 @SearchParamDefinition(name="publisher", path="CapabilityStatement.publisher", description="Name of the publisher of the capability statement", type="string" ) 10074 public static final String SP_PUBLISHER = "publisher"; 10075 /** 10076 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 10077 * <p> 10078 * Description: <b>Name of the publisher of the capability statement</b><br> 10079 * Type: <b>string</b><br> 10080 * Path: <b>CapabilityStatement.publisher</b><br> 10081 * </p> 10082 */ 10083 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 10084 10085 /** 10086 * Search parameter: <b>event</b> 10087 * <p> 10088 * Description: <b>Event code in a capability statement</b><br> 10089 * Type: <b>token</b><br> 10090 * Path: <b>CapabilityStatement.messaging.event.code</b><br> 10091 * </p> 10092 */ 10093 @SearchParamDefinition(name="event", path="CapabilityStatement.messaging.event.code", description="Event code in a capability statement", type="token" ) 10094 public static final String SP_EVENT = "event"; 10095 /** 10096 * <b>Fluent Client</b> search parameter constant for <b>event</b> 10097 * <p> 10098 * Description: <b>Event code in a capability statement</b><br> 10099 * Type: <b>token</b><br> 10100 * Path: <b>CapabilityStatement.messaging.event.code</b><br> 10101 * </p> 10102 */ 10103 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 10104 10105 /** 10106 * Search parameter: <b>guide</b> 10107 * <p> 10108 * Description: <b>Implementation guides supported</b><br> 10109 * Type: <b>uri</b><br> 10110 * Path: <b>CapabilityStatement.implementationGuide</b><br> 10111 * </p> 10112 */ 10113 @SearchParamDefinition(name="guide", path="CapabilityStatement.implementationGuide", description="Implementation guides supported", type="uri" ) 10114 public static final String SP_GUIDE = "guide"; 10115 /** 10116 * <b>Fluent Client</b> search parameter constant for <b>guide</b> 10117 * <p> 10118 * Description: <b>Implementation guides supported</b><br> 10119 * Type: <b>uri</b><br> 10120 * Path: <b>CapabilityStatement.implementationGuide</b><br> 10121 * </p> 10122 */ 10123 public static final ca.uhn.fhir.rest.gclient.UriClientParam GUIDE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_GUIDE); 10124 10125 /** 10126 * Search parameter: <b>status</b> 10127 * <p> 10128 * Description: <b>The current status of the capability statement</b><br> 10129 * Type: <b>token</b><br> 10130 * Path: <b>CapabilityStatement.status</b><br> 10131 * </p> 10132 */ 10133 @SearchParamDefinition(name="status", path="CapabilityStatement.status", description="The current status of the capability statement", type="token" ) 10134 public static final String SP_STATUS = "status"; 10135 /** 10136 * <b>Fluent Client</b> search parameter constant for <b>status</b> 10137 * <p> 10138 * Description: <b>The current status of the capability statement</b><br> 10139 * Type: <b>token</b><br> 10140 * Path: <b>CapabilityStatement.status</b><br> 10141 * </p> 10142 */ 10143 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 10144 10145 10146}