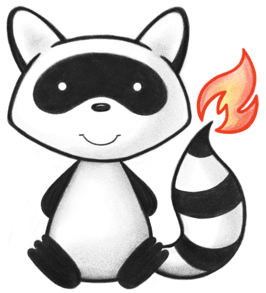
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 050 */ 051@ResourceDef(name="CarePlan", profile="http://hl7.org/fhir/Profile/CarePlan") 052public class CarePlan extends DomainResource { 053 054 public enum CarePlanStatus { 055 /** 056 * The plan is in development or awaiting use but is not yet intended to be acted upon. 057 */ 058 DRAFT, 059 /** 060 * The plan is intended to be followed and used as part of patient care. 061 */ 062 ACTIVE, 063 /** 064 * The plan has been temporarily stopped but is expected to resume in the future. 065 */ 066 SUSPENDED, 067 /** 068 * The plan is no longer in use and is not expected to be followed or used in patient care. 069 */ 070 COMPLETED, 071 /** 072 * The plan was entered in error and voided. 073 */ 074 ENTEREDINERROR, 075 /** 076 * The plan has been terminated prior to reaching completion (though it may have been replaced by a new plan). 077 */ 078 CANCELLED, 079 /** 080 * The authoring system doesn't know the current state of the care plan. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static CarePlanStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("draft".equals(codeString)) 091 return DRAFT; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("completed".equals(codeString)) 097 return COMPLETED; 098 if ("entered-in-error".equals(codeString)) 099 return ENTEREDINERROR; 100 if ("cancelled".equals(codeString)) 101 return CANCELLED; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown CarePlanStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case DRAFT: return "draft"; 112 case ACTIVE: return "active"; 113 case SUSPENDED: return "suspended"; 114 case COMPLETED: return "completed"; 115 case ENTEREDINERROR: return "entered-in-error"; 116 case CANCELLED: return "cancelled"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case DRAFT: return "http://hl7.org/fhir/care-plan-status"; 125 case ACTIVE: return "http://hl7.org/fhir/care-plan-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/care-plan-status"; 127 case COMPLETED: return "http://hl7.org/fhir/care-plan-status"; 128 case ENTEREDINERROR: return "http://hl7.org/fhir/care-plan-status"; 129 case CANCELLED: return "http://hl7.org/fhir/care-plan-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/care-plan-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case DRAFT: return "The plan is in development or awaiting use but is not yet intended to be acted upon."; 138 case ACTIVE: return "The plan is intended to be followed and used as part of patient care."; 139 case SUSPENDED: return "The plan has been temporarily stopped but is expected to resume in the future."; 140 case COMPLETED: return "The plan is no longer in use and is not expected to be followed or used in patient care."; 141 case ENTEREDINERROR: return "The plan was entered in error and voided."; 142 case CANCELLED: return "The plan has been terminated prior to reaching completion (though it may have been replaced by a new plan)."; 143 case UNKNOWN: return "The authoring system doesn't know the current state of the care plan."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case DRAFT: return "Pending"; 151 case ACTIVE: return "Active"; 152 case SUSPENDED: return "Suspended"; 153 case COMPLETED: return "Completed"; 154 case ENTEREDINERROR: return "Entered In Error"; 155 case CANCELLED: return "Cancelled"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class CarePlanStatusEnumFactory implements EnumFactory<CarePlanStatus> { 164 public CarePlanStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("draft".equals(codeString)) 169 return CarePlanStatus.DRAFT; 170 if ("active".equals(codeString)) 171 return CarePlanStatus.ACTIVE; 172 if ("suspended".equals(codeString)) 173 return CarePlanStatus.SUSPENDED; 174 if ("completed".equals(codeString)) 175 return CarePlanStatus.COMPLETED; 176 if ("entered-in-error".equals(codeString)) 177 return CarePlanStatus.ENTEREDINERROR; 178 if ("cancelled".equals(codeString)) 179 return CarePlanStatus.CANCELLED; 180 if ("unknown".equals(codeString)) 181 return CarePlanStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown CarePlanStatus code '"+codeString+"'"); 183 } 184 public Enumeration<CarePlanStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<CarePlanStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("draft".equals(codeString)) 193 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.DRAFT); 194 if ("active".equals(codeString)) 195 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ACTIVE); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.SUSPENDED); 198 if ("completed".equals(codeString)) 199 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.COMPLETED); 200 if ("entered-in-error".equals(codeString)) 201 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ENTEREDINERROR); 202 if ("cancelled".equals(codeString)) 203 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.CANCELLED); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.UNKNOWN); 206 throw new FHIRException("Unknown CarePlanStatus code '"+codeString+"'"); 207 } 208 public String toCode(CarePlanStatus code) { 209 if (code == CarePlanStatus.NULL) 210 return null; 211 if (code == CarePlanStatus.DRAFT) 212 return "draft"; 213 if (code == CarePlanStatus.ACTIVE) 214 return "active"; 215 if (code == CarePlanStatus.SUSPENDED) 216 return "suspended"; 217 if (code == CarePlanStatus.COMPLETED) 218 return "completed"; 219 if (code == CarePlanStatus.ENTEREDINERROR) 220 return "entered-in-error"; 221 if (code == CarePlanStatus.CANCELLED) 222 return "cancelled"; 223 if (code == CarePlanStatus.UNKNOWN) 224 return "unknown"; 225 return "?"; 226 } 227 public String toSystem(CarePlanStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 public enum CarePlanIntent { 233 /** 234 * The care plan is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 235 */ 236 PROPOSAL, 237 /** 238 * The care plan represents an intention to ensure something occurs without providing an authorization for others to act 239 */ 240 PLAN, 241 /** 242 * The care plan represents a request/demand and authorization for action 243 */ 244 ORDER, 245 /** 246 * The care plan represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 247 248Refer to [[[RequestGroup]]] for additional information on how this status is used 249 */ 250 OPTION, 251 /** 252 * added to help the parsers with the generic types 253 */ 254 NULL; 255 public static CarePlanIntent fromCode(String codeString) throws FHIRException { 256 if (codeString == null || "".equals(codeString)) 257 return null; 258 if ("proposal".equals(codeString)) 259 return PROPOSAL; 260 if ("plan".equals(codeString)) 261 return PLAN; 262 if ("order".equals(codeString)) 263 return ORDER; 264 if ("option".equals(codeString)) 265 return OPTION; 266 if (Configuration.isAcceptInvalidEnums()) 267 return null; 268 else 269 throw new FHIRException("Unknown CarePlanIntent code '"+codeString+"'"); 270 } 271 public String toCode() { 272 switch (this) { 273 case PROPOSAL: return "proposal"; 274 case PLAN: return "plan"; 275 case ORDER: return "order"; 276 case OPTION: return "option"; 277 case NULL: return null; 278 default: return "?"; 279 } 280 } 281 public String getSystem() { 282 switch (this) { 283 case PROPOSAL: return "http://hl7.org/fhir/care-plan-intent"; 284 case PLAN: return "http://hl7.org/fhir/care-plan-intent"; 285 case ORDER: return "http://hl7.org/fhir/care-plan-intent"; 286 case OPTION: return "http://hl7.org/fhir/care-plan-intent"; 287 case NULL: return null; 288 default: return "?"; 289 } 290 } 291 public String getDefinition() { 292 switch (this) { 293 case PROPOSAL: return "The care plan is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 294 case PLAN: return "The care plan represents an intention to ensure something occurs without providing an authorization for others to act"; 295 case ORDER: return "The care plan represents a request/demand and authorization for action"; 296 case OPTION: return "The care plan represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 297 case NULL: return null; 298 default: return "?"; 299 } 300 } 301 public String getDisplay() { 302 switch (this) { 303 case PROPOSAL: return "Proposal"; 304 case PLAN: return "Plan"; 305 case ORDER: return "Order"; 306 case OPTION: return "Option"; 307 case NULL: return null; 308 default: return "?"; 309 } 310 } 311 } 312 313 public static class CarePlanIntentEnumFactory implements EnumFactory<CarePlanIntent> { 314 public CarePlanIntent fromCode(String codeString) throws IllegalArgumentException { 315 if (codeString == null || "".equals(codeString)) 316 if (codeString == null || "".equals(codeString)) 317 return null; 318 if ("proposal".equals(codeString)) 319 return CarePlanIntent.PROPOSAL; 320 if ("plan".equals(codeString)) 321 return CarePlanIntent.PLAN; 322 if ("order".equals(codeString)) 323 return CarePlanIntent.ORDER; 324 if ("option".equals(codeString)) 325 return CarePlanIntent.OPTION; 326 throw new IllegalArgumentException("Unknown CarePlanIntent code '"+codeString+"'"); 327 } 328 public Enumeration<CarePlanIntent> fromType(PrimitiveType<?> code) throws FHIRException { 329 if (code == null) 330 return null; 331 if (code.isEmpty()) 332 return new Enumeration<CarePlanIntent>(this); 333 String codeString = code.asStringValue(); 334 if (codeString == null || "".equals(codeString)) 335 return null; 336 if ("proposal".equals(codeString)) 337 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PROPOSAL); 338 if ("plan".equals(codeString)) 339 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PLAN); 340 if ("order".equals(codeString)) 341 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.ORDER); 342 if ("option".equals(codeString)) 343 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.OPTION); 344 throw new FHIRException("Unknown CarePlanIntent code '"+codeString+"'"); 345 } 346 public String toCode(CarePlanIntent code) { 347 if (code == CarePlanIntent.NULL) 348 return null; 349 if (code == CarePlanIntent.PROPOSAL) 350 return "proposal"; 351 if (code == CarePlanIntent.PLAN) 352 return "plan"; 353 if (code == CarePlanIntent.ORDER) 354 return "order"; 355 if (code == CarePlanIntent.OPTION) 356 return "option"; 357 return "?"; 358 } 359 public String toSystem(CarePlanIntent code) { 360 return code.getSystem(); 361 } 362 } 363 364 public enum CarePlanActivityStatus { 365 /** 366 * Activity is planned but no action has yet been taken. 367 */ 368 NOTSTARTED, 369 /** 370 * Appointment or other booking has occurred but activity has not yet begun. 371 */ 372 SCHEDULED, 373 /** 374 * Activity has been started but is not yet complete. 375 */ 376 INPROGRESS, 377 /** 378 * Activity was started but has temporarily ceased with an expectation of resumption at a future time. 379 */ 380 ONHOLD, 381 /** 382 * The activities have been completed (more or less) as planned. 383 */ 384 COMPLETED, 385 /** 386 * The activities have been ended prior to completion (perhaps even before they were started). 387 */ 388 CANCELLED, 389 /** 390 * The authoring system doesn't know the current state of the activity. 391 */ 392 UNKNOWN, 393 /** 394 * added to help the parsers with the generic types 395 */ 396 NULL; 397 public static CarePlanActivityStatus fromCode(String codeString) throws FHIRException { 398 if (codeString == null || "".equals(codeString)) 399 return null; 400 if ("not-started".equals(codeString)) 401 return NOTSTARTED; 402 if ("scheduled".equals(codeString)) 403 return SCHEDULED; 404 if ("in-progress".equals(codeString)) 405 return INPROGRESS; 406 if ("on-hold".equals(codeString)) 407 return ONHOLD; 408 if ("completed".equals(codeString)) 409 return COMPLETED; 410 if ("cancelled".equals(codeString)) 411 return CANCELLED; 412 if ("unknown".equals(codeString)) 413 return UNKNOWN; 414 if (Configuration.isAcceptInvalidEnums()) 415 return null; 416 else 417 throw new FHIRException("Unknown CarePlanActivityStatus code '"+codeString+"'"); 418 } 419 public String toCode() { 420 switch (this) { 421 case NOTSTARTED: return "not-started"; 422 case SCHEDULED: return "scheduled"; 423 case INPROGRESS: return "in-progress"; 424 case ONHOLD: return "on-hold"; 425 case COMPLETED: return "completed"; 426 case CANCELLED: return "cancelled"; 427 case UNKNOWN: return "unknown"; 428 case NULL: return null; 429 default: return "?"; 430 } 431 } 432 public String getSystem() { 433 switch (this) { 434 case NOTSTARTED: return "http://hl7.org/fhir/care-plan-activity-status"; 435 case SCHEDULED: return "http://hl7.org/fhir/care-plan-activity-status"; 436 case INPROGRESS: return "http://hl7.org/fhir/care-plan-activity-status"; 437 case ONHOLD: return "http://hl7.org/fhir/care-plan-activity-status"; 438 case COMPLETED: return "http://hl7.org/fhir/care-plan-activity-status"; 439 case CANCELLED: return "http://hl7.org/fhir/care-plan-activity-status"; 440 case UNKNOWN: return "http://hl7.org/fhir/care-plan-activity-status"; 441 case NULL: return null; 442 default: return "?"; 443 } 444 } 445 public String getDefinition() { 446 switch (this) { 447 case NOTSTARTED: return "Activity is planned but no action has yet been taken."; 448 case SCHEDULED: return "Appointment or other booking has occurred but activity has not yet begun."; 449 case INPROGRESS: return "Activity has been started but is not yet complete."; 450 case ONHOLD: return "Activity was started but has temporarily ceased with an expectation of resumption at a future time."; 451 case COMPLETED: return "The activities have been completed (more or less) as planned."; 452 case CANCELLED: return "The activities have been ended prior to completion (perhaps even before they were started)."; 453 case UNKNOWN: return "The authoring system doesn't know the current state of the activity."; 454 case NULL: return null; 455 default: return "?"; 456 } 457 } 458 public String getDisplay() { 459 switch (this) { 460 case NOTSTARTED: return "Not Started"; 461 case SCHEDULED: return "Scheduled"; 462 case INPROGRESS: return "In Progress"; 463 case ONHOLD: return "On Hold"; 464 case COMPLETED: return "Completed"; 465 case CANCELLED: return "Cancelled"; 466 case UNKNOWN: return "Unknown"; 467 case NULL: return null; 468 default: return "?"; 469 } 470 } 471 } 472 473 public static class CarePlanActivityStatusEnumFactory implements EnumFactory<CarePlanActivityStatus> { 474 public CarePlanActivityStatus fromCode(String codeString) throws IllegalArgumentException { 475 if (codeString == null || "".equals(codeString)) 476 if (codeString == null || "".equals(codeString)) 477 return null; 478 if ("not-started".equals(codeString)) 479 return CarePlanActivityStatus.NOTSTARTED; 480 if ("scheduled".equals(codeString)) 481 return CarePlanActivityStatus.SCHEDULED; 482 if ("in-progress".equals(codeString)) 483 return CarePlanActivityStatus.INPROGRESS; 484 if ("on-hold".equals(codeString)) 485 return CarePlanActivityStatus.ONHOLD; 486 if ("completed".equals(codeString)) 487 return CarePlanActivityStatus.COMPLETED; 488 if ("cancelled".equals(codeString)) 489 return CarePlanActivityStatus.CANCELLED; 490 if ("unknown".equals(codeString)) 491 return CarePlanActivityStatus.UNKNOWN; 492 throw new IllegalArgumentException("Unknown CarePlanActivityStatus code '"+codeString+"'"); 493 } 494 public Enumeration<CarePlanActivityStatus> fromType(PrimitiveType<?> code) throws FHIRException { 495 if (code == null) 496 return null; 497 if (code.isEmpty()) 498 return new Enumeration<CarePlanActivityStatus>(this); 499 String codeString = code.asStringValue(); 500 if (codeString == null || "".equals(codeString)) 501 return null; 502 if ("not-started".equals(codeString)) 503 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NOTSTARTED); 504 if ("scheduled".equals(codeString)) 505 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.SCHEDULED); 506 if ("in-progress".equals(codeString)) 507 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.INPROGRESS); 508 if ("on-hold".equals(codeString)) 509 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.ONHOLD); 510 if ("completed".equals(codeString)) 511 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.COMPLETED); 512 if ("cancelled".equals(codeString)) 513 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.CANCELLED); 514 if ("unknown".equals(codeString)) 515 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.UNKNOWN); 516 throw new FHIRException("Unknown CarePlanActivityStatus code '"+codeString+"'"); 517 } 518 public String toCode(CarePlanActivityStatus code) { 519 if (code == CarePlanActivityStatus.NULL) 520 return null; 521 if (code == CarePlanActivityStatus.NOTSTARTED) 522 return "not-started"; 523 if (code == CarePlanActivityStatus.SCHEDULED) 524 return "scheduled"; 525 if (code == CarePlanActivityStatus.INPROGRESS) 526 return "in-progress"; 527 if (code == CarePlanActivityStatus.ONHOLD) 528 return "on-hold"; 529 if (code == CarePlanActivityStatus.COMPLETED) 530 return "completed"; 531 if (code == CarePlanActivityStatus.CANCELLED) 532 return "cancelled"; 533 if (code == CarePlanActivityStatus.UNKNOWN) 534 return "unknown"; 535 return "?"; 536 } 537 public String toSystem(CarePlanActivityStatus code) { 538 return code.getSystem(); 539 } 540 } 541 542 @Block() 543 public static class CarePlanActivityComponent extends BackboneElement implements IBaseBackboneElement { 544 /** 545 * Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not). 546 */ 547 @Child(name = "outcomeCodeableConcept", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 548 @Description(shortDefinition="Results of the activity", formalDefinition="Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not)." ) 549 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-activity-outcome") 550 protected List<CodeableConcept> outcomeCodeableConcept; 551 552 /** 553 * Details of the outcome or action resulting from the activity. The reference to an "event" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource). 554 */ 555 @Child(name = "outcomeReference", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 556 @Description(shortDefinition="Appointment, Encounter, Procedure, etc.", formalDefinition="Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource)." ) 557 protected List<Reference> outcomeReference; 558 /** 559 * The actual objects that are the target of the reference (Details of the outcome or action resulting from the activity. The reference to an "event" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).) 560 */ 561 protected List<Resource> outcomeReferenceTarget; 562 563 564 /** 565 * Notes about the adherence/status/progress of the activity. 566 */ 567 @Child(name = "progress", type = {Annotation.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 568 @Description(shortDefinition="Comments about the activity status/progress", formalDefinition="Notes about the adherence/status/progress of the activity." ) 569 protected List<Annotation> progress; 570 571 /** 572 * The details of the proposed activity represented in a specific resource. 573 */ 574 @Child(name = "reference", type = {Appointment.class, CommunicationRequest.class, DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, Task.class, ProcedureRequest.class, ReferralRequest.class, VisionPrescription.class, RequestGroup.class}, order=4, min=0, max=1, modifier=false, summary=false) 575 @Description(shortDefinition="Activity details defined in specific resource", formalDefinition="The details of the proposed activity represented in a specific resource." ) 576 protected Reference reference; 577 578 /** 579 * The actual object that is the target of the reference (The details of the proposed activity represented in a specific resource.) 580 */ 581 protected Resource referenceTarget; 582 583 /** 584 * A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc. 585 */ 586 @Child(name = "detail", type = {}, order=5, min=0, max=1, modifier=false, summary=false) 587 @Description(shortDefinition="In-line definition of activity", formalDefinition="A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc." ) 588 protected CarePlanActivityDetailComponent detail; 589 590 private static final long serialVersionUID = -609287300L; 591 592 /** 593 * Constructor 594 */ 595 public CarePlanActivityComponent() { 596 super(); 597 } 598 599 /** 600 * @return {@link #outcomeCodeableConcept} (Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).) 601 */ 602 public List<CodeableConcept> getOutcomeCodeableConcept() { 603 if (this.outcomeCodeableConcept == null) 604 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 605 return this.outcomeCodeableConcept; 606 } 607 608 /** 609 * @return Returns a reference to <code>this</code> for easy method chaining 610 */ 611 public CarePlanActivityComponent setOutcomeCodeableConcept(List<CodeableConcept> theOutcomeCodeableConcept) { 612 this.outcomeCodeableConcept = theOutcomeCodeableConcept; 613 return this; 614 } 615 616 public boolean hasOutcomeCodeableConcept() { 617 if (this.outcomeCodeableConcept == null) 618 return false; 619 for (CodeableConcept item : this.outcomeCodeableConcept) 620 if (!item.isEmpty()) 621 return true; 622 return false; 623 } 624 625 public CodeableConcept addOutcomeCodeableConcept() { //3 626 CodeableConcept t = new CodeableConcept(); 627 if (this.outcomeCodeableConcept == null) 628 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 629 this.outcomeCodeableConcept.add(t); 630 return t; 631 } 632 633 public CarePlanActivityComponent addOutcomeCodeableConcept(CodeableConcept t) { //3 634 if (t == null) 635 return this; 636 if (this.outcomeCodeableConcept == null) 637 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 638 this.outcomeCodeableConcept.add(t); 639 return this; 640 } 641 642 /** 643 * @return The first repetition of repeating field {@link #outcomeCodeableConcept}, creating it if it does not already exist 644 */ 645 public CodeableConcept getOutcomeCodeableConceptFirstRep() { 646 if (getOutcomeCodeableConcept().isEmpty()) { 647 addOutcomeCodeableConcept(); 648 } 649 return getOutcomeCodeableConcept().get(0); 650 } 651 652 /** 653 * @return {@link #outcomeReference} (Details of the outcome or action resulting from the activity. The reference to an "event" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).) 654 */ 655 public List<Reference> getOutcomeReference() { 656 if (this.outcomeReference == null) 657 this.outcomeReference = new ArrayList<Reference>(); 658 return this.outcomeReference; 659 } 660 661 /** 662 * @return Returns a reference to <code>this</code> for easy method chaining 663 */ 664 public CarePlanActivityComponent setOutcomeReference(List<Reference> theOutcomeReference) { 665 this.outcomeReference = theOutcomeReference; 666 return this; 667 } 668 669 public boolean hasOutcomeReference() { 670 if (this.outcomeReference == null) 671 return false; 672 for (Reference item : this.outcomeReference) 673 if (!item.isEmpty()) 674 return true; 675 return false; 676 } 677 678 public Reference addOutcomeReference() { //3 679 Reference t = new Reference(); 680 if (this.outcomeReference == null) 681 this.outcomeReference = new ArrayList<Reference>(); 682 this.outcomeReference.add(t); 683 return t; 684 } 685 686 public CarePlanActivityComponent addOutcomeReference(Reference t) { //3 687 if (t == null) 688 return this; 689 if (this.outcomeReference == null) 690 this.outcomeReference = new ArrayList<Reference>(); 691 this.outcomeReference.add(t); 692 return this; 693 } 694 695 /** 696 * @return The first repetition of repeating field {@link #outcomeReference}, creating it if it does not already exist 697 */ 698 public Reference getOutcomeReferenceFirstRep() { 699 if (getOutcomeReference().isEmpty()) { 700 addOutcomeReference(); 701 } 702 return getOutcomeReference().get(0); 703 } 704 705 /** 706 * @deprecated Use Reference#setResource(IBaseResource) instead 707 */ 708 @Deprecated 709 public List<Resource> getOutcomeReferenceTarget() { 710 if (this.outcomeReferenceTarget == null) 711 this.outcomeReferenceTarget = new ArrayList<Resource>(); 712 return this.outcomeReferenceTarget; 713 } 714 715 /** 716 * @return {@link #progress} (Notes about the adherence/status/progress of the activity.) 717 */ 718 public List<Annotation> getProgress() { 719 if (this.progress == null) 720 this.progress = new ArrayList<Annotation>(); 721 return this.progress; 722 } 723 724 /** 725 * @return Returns a reference to <code>this</code> for easy method chaining 726 */ 727 public CarePlanActivityComponent setProgress(List<Annotation> theProgress) { 728 this.progress = theProgress; 729 return this; 730 } 731 732 public boolean hasProgress() { 733 if (this.progress == null) 734 return false; 735 for (Annotation item : this.progress) 736 if (!item.isEmpty()) 737 return true; 738 return false; 739 } 740 741 public Annotation addProgress() { //3 742 Annotation t = new Annotation(); 743 if (this.progress == null) 744 this.progress = new ArrayList<Annotation>(); 745 this.progress.add(t); 746 return t; 747 } 748 749 public CarePlanActivityComponent addProgress(Annotation t) { //3 750 if (t == null) 751 return this; 752 if (this.progress == null) 753 this.progress = new ArrayList<Annotation>(); 754 this.progress.add(t); 755 return this; 756 } 757 758 /** 759 * @return The first repetition of repeating field {@link #progress}, creating it if it does not already exist 760 */ 761 public Annotation getProgressFirstRep() { 762 if (getProgress().isEmpty()) { 763 addProgress(); 764 } 765 return getProgress().get(0); 766 } 767 768 /** 769 * @return {@link #reference} (The details of the proposed activity represented in a specific resource.) 770 */ 771 public Reference getReference() { 772 if (this.reference == null) 773 if (Configuration.errorOnAutoCreate()) 774 throw new Error("Attempt to auto-create CarePlanActivityComponent.reference"); 775 else if (Configuration.doAutoCreate()) 776 this.reference = new Reference(); // cc 777 return this.reference; 778 } 779 780 public boolean hasReference() { 781 return this.reference != null && !this.reference.isEmpty(); 782 } 783 784 /** 785 * @param value {@link #reference} (The details of the proposed activity represented in a specific resource.) 786 */ 787 public CarePlanActivityComponent setReference(Reference value) { 788 this.reference = value; 789 return this; 790 } 791 792 /** 793 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The details of the proposed activity represented in a specific resource.) 794 */ 795 public Resource getReferenceTarget() { 796 return this.referenceTarget; 797 } 798 799 /** 800 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The details of the proposed activity represented in a specific resource.) 801 */ 802 public CarePlanActivityComponent setReferenceTarget(Resource value) { 803 this.referenceTarget = value; 804 return this; 805 } 806 807 /** 808 * @return {@link #detail} (A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.) 809 */ 810 public CarePlanActivityDetailComponent getDetail() { 811 if (this.detail == null) 812 if (Configuration.errorOnAutoCreate()) 813 throw new Error("Attempt to auto-create CarePlanActivityComponent.detail"); 814 else if (Configuration.doAutoCreate()) 815 this.detail = new CarePlanActivityDetailComponent(); // cc 816 return this.detail; 817 } 818 819 public boolean hasDetail() { 820 return this.detail != null && !this.detail.isEmpty(); 821 } 822 823 /** 824 * @param value {@link #detail} (A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.) 825 */ 826 public CarePlanActivityComponent setDetail(CarePlanActivityDetailComponent value) { 827 this.detail = value; 828 return this; 829 } 830 831 protected void listChildren(List<Property> children) { 832 super.listChildren(children); 833 children.add(new Property("outcomeCodeableConcept", "CodeableConcept", "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).", 0, java.lang.Integer.MAX_VALUE, outcomeCodeableConcept)); 834 children.add(new Property("outcomeReference", "Reference(Any)", "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).", 0, java.lang.Integer.MAX_VALUE, outcomeReference)); 835 children.add(new Property("progress", "Annotation", "Notes about the adherence/status/progress of the activity.", 0, java.lang.Integer.MAX_VALUE, progress)); 836 children.add(new Property("reference", "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ProcedureRequest|ReferralRequest|VisionPrescription|RequestGroup)", "The details of the proposed activity represented in a specific resource.", 0, 1, reference)); 837 children.add(new Property("detail", "", "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.", 0, 1, detail)); 838 } 839 840 @Override 841 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 842 switch (_hash) { 843 case -511913489: /*outcomeCodeableConcept*/ return new Property("outcomeCodeableConcept", "CodeableConcept", "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).", 0, java.lang.Integer.MAX_VALUE, outcomeCodeableConcept); 844 case -782273511: /*outcomeReference*/ return new Property("outcomeReference", "Reference(Any)", "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).", 0, java.lang.Integer.MAX_VALUE, outcomeReference); 845 case -1001078227: /*progress*/ return new Property("progress", "Annotation", "Notes about the adherence/status/progress of the activity.", 0, java.lang.Integer.MAX_VALUE, progress); 846 case -925155509: /*reference*/ return new Property("reference", "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ProcedureRequest|ReferralRequest|VisionPrescription|RequestGroup)", "The details of the proposed activity represented in a specific resource.", 0, 1, reference); 847 case -1335224239: /*detail*/ return new Property("detail", "", "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.", 0, 1, detail); 848 default: return super.getNamedProperty(_hash, _name, _checkValid); 849 } 850 851 } 852 853 @Override 854 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 855 switch (hash) { 856 case -511913489: /*outcomeCodeableConcept*/ return this.outcomeCodeableConcept == null ? new Base[0] : this.outcomeCodeableConcept.toArray(new Base[this.outcomeCodeableConcept.size()]); // CodeableConcept 857 case -782273511: /*outcomeReference*/ return this.outcomeReference == null ? new Base[0] : this.outcomeReference.toArray(new Base[this.outcomeReference.size()]); // Reference 858 case -1001078227: /*progress*/ return this.progress == null ? new Base[0] : this.progress.toArray(new Base[this.progress.size()]); // Annotation 859 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 860 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // CarePlanActivityDetailComponent 861 default: return super.getProperty(hash, name, checkValid); 862 } 863 864 } 865 866 @Override 867 public Base setProperty(int hash, String name, Base value) throws FHIRException { 868 switch (hash) { 869 case -511913489: // outcomeCodeableConcept 870 this.getOutcomeCodeableConcept().add(castToCodeableConcept(value)); // CodeableConcept 871 return value; 872 case -782273511: // outcomeReference 873 this.getOutcomeReference().add(castToReference(value)); // Reference 874 return value; 875 case -1001078227: // progress 876 this.getProgress().add(castToAnnotation(value)); // Annotation 877 return value; 878 case -925155509: // reference 879 this.reference = castToReference(value); // Reference 880 return value; 881 case -1335224239: // detail 882 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 883 return value; 884 default: return super.setProperty(hash, name, value); 885 } 886 887 } 888 889 @Override 890 public Base setProperty(String name, Base value) throws FHIRException { 891 if (name.equals("outcomeCodeableConcept")) { 892 this.getOutcomeCodeableConcept().add(castToCodeableConcept(value)); 893 } else if (name.equals("outcomeReference")) { 894 this.getOutcomeReference().add(castToReference(value)); 895 } else if (name.equals("progress")) { 896 this.getProgress().add(castToAnnotation(value)); 897 } else if (name.equals("reference")) { 898 this.reference = castToReference(value); // Reference 899 } else if (name.equals("detail")) { 900 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 901 } else 902 return super.setProperty(name, value); 903 return value; 904 } 905 906 @Override 907 public Base makeProperty(int hash, String name) throws FHIRException { 908 switch (hash) { 909 case -511913489: return addOutcomeCodeableConcept(); 910 case -782273511: return addOutcomeReference(); 911 case -1001078227: return addProgress(); 912 case -925155509: return getReference(); 913 case -1335224239: return getDetail(); 914 default: return super.makeProperty(hash, name); 915 } 916 917 } 918 919 @Override 920 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 921 switch (hash) { 922 case -511913489: /*outcomeCodeableConcept*/ return new String[] {"CodeableConcept"}; 923 case -782273511: /*outcomeReference*/ return new String[] {"Reference"}; 924 case -1001078227: /*progress*/ return new String[] {"Annotation"}; 925 case -925155509: /*reference*/ return new String[] {"Reference"}; 926 case -1335224239: /*detail*/ return new String[] {}; 927 default: return super.getTypesForProperty(hash, name); 928 } 929 930 } 931 932 @Override 933 public Base addChild(String name) throws FHIRException { 934 if (name.equals("outcomeCodeableConcept")) { 935 return addOutcomeCodeableConcept(); 936 } 937 else if (name.equals("outcomeReference")) { 938 return addOutcomeReference(); 939 } 940 else if (name.equals("progress")) { 941 return addProgress(); 942 } 943 else if (name.equals("reference")) { 944 this.reference = new Reference(); 945 return this.reference; 946 } 947 else if (name.equals("detail")) { 948 this.detail = new CarePlanActivityDetailComponent(); 949 return this.detail; 950 } 951 else 952 return super.addChild(name); 953 } 954 955 public CarePlanActivityComponent copy() { 956 CarePlanActivityComponent dst = new CarePlanActivityComponent(); 957 copyValues(dst); 958 if (outcomeCodeableConcept != null) { 959 dst.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 960 for (CodeableConcept i : outcomeCodeableConcept) 961 dst.outcomeCodeableConcept.add(i.copy()); 962 }; 963 if (outcomeReference != null) { 964 dst.outcomeReference = new ArrayList<Reference>(); 965 for (Reference i : outcomeReference) 966 dst.outcomeReference.add(i.copy()); 967 }; 968 if (progress != null) { 969 dst.progress = new ArrayList<Annotation>(); 970 for (Annotation i : progress) 971 dst.progress.add(i.copy()); 972 }; 973 dst.reference = reference == null ? null : reference.copy(); 974 dst.detail = detail == null ? null : detail.copy(); 975 return dst; 976 } 977 978 @Override 979 public boolean equalsDeep(Base other_) { 980 if (!super.equalsDeep(other_)) 981 return false; 982 if (!(other_ instanceof CarePlanActivityComponent)) 983 return false; 984 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 985 return compareDeep(outcomeCodeableConcept, o.outcomeCodeableConcept, true) && compareDeep(outcomeReference, o.outcomeReference, true) 986 && compareDeep(progress, o.progress, true) && compareDeep(reference, o.reference, true) && compareDeep(detail, o.detail, true) 987 ; 988 } 989 990 @Override 991 public boolean equalsShallow(Base other_) { 992 if (!super.equalsShallow(other_)) 993 return false; 994 if (!(other_ instanceof CarePlanActivityComponent)) 995 return false; 996 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 997 return true; 998 } 999 1000 public boolean isEmpty() { 1001 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(outcomeCodeableConcept, outcomeReference 1002 , progress, reference, detail); 1003 } 1004 1005 public String fhirType() { 1006 return "CarePlan.activity"; 1007 1008 } 1009 1010 } 1011 1012 @Block() 1013 public static class CarePlanActivityDetailComponent extends BackboneElement implements IBaseBackboneElement { 1014 /** 1015 * High-level categorization of the type of activity in a care plan. 1016 */ 1017 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1018 @Description(shortDefinition="diet | drug | encounter | observation | procedure | supply | other", formalDefinition="High-level categorization of the type of activity in a care plan." ) 1019 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-activity-category") 1020 protected CodeableConcept category; 1021 1022 /** 1023 * Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with. 1024 */ 1025 @Child(name = "definition", type = {PlanDefinition.class, ActivityDefinition.class, Questionnaire.class}, order=2, min=0, max=1, modifier=false, summary=false) 1026 @Description(shortDefinition="Protocol or definition", formalDefinition="Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with." ) 1027 protected Reference definition; 1028 1029 /** 1030 * The actual object that is the target of the reference (Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with.) 1031 */ 1032 protected Resource definitionTarget; 1033 1034 /** 1035 * Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter. 1036 */ 1037 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1038 @Description(shortDefinition="Detail type of activity", formalDefinition="Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter." ) 1039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-activity") 1040 protected CodeableConcept code; 1041 1042 /** 1043 * Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited. 1044 */ 1045 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1046 @Description(shortDefinition="Why activity should be done or why activity was prohibited", formalDefinition="Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited." ) 1047 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/activity-reason") 1048 protected List<CodeableConcept> reasonCode; 1049 1050 /** 1051 * Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan. 1052 */ 1053 @Child(name = "reasonReference", type = {Condition.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1054 @Description(shortDefinition="Condition triggering need for activity", formalDefinition="Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan." ) 1055 protected List<Reference> reasonReference; 1056 /** 1057 * The actual objects that are the target of the reference (Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan.) 1058 */ 1059 protected List<Condition> reasonReferenceTarget; 1060 1061 1062 /** 1063 * Internal reference that identifies the goals that this activity is intended to contribute towards meeting. 1064 */ 1065 @Child(name = "goal", type = {Goal.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1066 @Description(shortDefinition="Goals this activity relates to", formalDefinition="Internal reference that identifies the goals that this activity is intended to contribute towards meeting." ) 1067 protected List<Reference> goal; 1068 /** 1069 * The actual objects that are the target of the reference (Internal reference that identifies the goals that this activity is intended to contribute towards meeting.) 1070 */ 1071 protected List<Goal> goalTarget; 1072 1073 1074 /** 1075 * Identifies what progress is being made for the specific activity. 1076 */ 1077 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=false) 1078 @Description(shortDefinition="not-started | scheduled | in-progress | on-hold | completed | cancelled | unknown", formalDefinition="Identifies what progress is being made for the specific activity." ) 1079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-activity-status") 1080 protected Enumeration<CarePlanActivityStatus> status; 1081 1082 /** 1083 * Provides reason why the activity isn't yet started, is on hold, was cancelled, etc. 1084 */ 1085 @Child(name = "statusReason", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1086 @Description(shortDefinition="Reason for current status", formalDefinition="Provides reason why the activity isn't yet started, is on hold, was cancelled, etc." ) 1087 protected StringType statusReason; 1088 1089 /** 1090 * If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan. 1091 */ 1092 @Child(name = "prohibited", type = {BooleanType.class}, order=9, min=0, max=1, modifier=true, summary=false) 1093 @Description(shortDefinition="Do NOT do", formalDefinition="If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan." ) 1094 protected BooleanType prohibited; 1095 1096 /** 1097 * The period, timing or frequency upon which the described activity is to occur. 1098 */ 1099 @Child(name = "scheduled", type = {Timing.class, Period.class, StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 1100 @Description(shortDefinition="When activity is to occur", formalDefinition="The period, timing or frequency upon which the described activity is to occur." ) 1101 protected Type scheduled; 1102 1103 /** 1104 * Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc. 1105 */ 1106 @Child(name = "location", type = {Location.class}, order=11, min=0, max=1, modifier=false, summary=false) 1107 @Description(shortDefinition="Where it should happen", formalDefinition="Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc." ) 1108 protected Reference location; 1109 1110 /** 1111 * The actual object that is the target of the reference (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 1112 */ 1113 protected Location locationTarget; 1114 1115 /** 1116 * Identifies who's expected to be involved in the activity. 1117 */ 1118 @Child(name = "performer", type = {Practitioner.class, Organization.class, RelatedPerson.class, Patient.class, CareTeam.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1119 @Description(shortDefinition="Who will be responsible?", formalDefinition="Identifies who's expected to be involved in the activity." ) 1120 protected List<Reference> performer; 1121 /** 1122 * The actual objects that are the target of the reference (Identifies who's expected to be involved in the activity.) 1123 */ 1124 protected List<Resource> performerTarget; 1125 1126 1127 /** 1128 * Identifies the food, drug or other product to be consumed or supplied in the activity. 1129 */ 1130 @Child(name = "product", type = {CodeableConcept.class, Medication.class, Substance.class}, order=13, min=0, max=1, modifier=false, summary=false) 1131 @Description(shortDefinition="What is to be administered/supplied", formalDefinition="Identifies the food, drug or other product to be consumed or supplied in the activity." ) 1132 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 1133 protected Type product; 1134 1135 /** 1136 * Identifies the quantity expected to be consumed in a given day. 1137 */ 1138 @Child(name = "dailyAmount", type = {SimpleQuantity.class}, order=14, min=0, max=1, modifier=false, summary=false) 1139 @Description(shortDefinition="How to consume/day?", formalDefinition="Identifies the quantity expected to be consumed in a given day." ) 1140 protected SimpleQuantity dailyAmount; 1141 1142 /** 1143 * Identifies the quantity expected to be supplied, administered or consumed by the subject. 1144 */ 1145 @Child(name = "quantity", type = {SimpleQuantity.class}, order=15, min=0, max=1, modifier=false, summary=false) 1146 @Description(shortDefinition="How much to administer/supply/consume", formalDefinition="Identifies the quantity expected to be supplied, administered or consumed by the subject." ) 1147 protected SimpleQuantity quantity; 1148 1149 /** 1150 * This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc. 1151 */ 1152 @Child(name = "description", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1153 @Description(shortDefinition="Extra info describing activity to perform", formalDefinition="This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc." ) 1154 protected StringType description; 1155 1156 private static final long serialVersionUID = -549984462L; 1157 1158 /** 1159 * Constructor 1160 */ 1161 public CarePlanActivityDetailComponent() { 1162 super(); 1163 } 1164 1165 /** 1166 * Constructor 1167 */ 1168 public CarePlanActivityDetailComponent(Enumeration<CarePlanActivityStatus> status) { 1169 super(); 1170 this.status = status; 1171 } 1172 1173 /** 1174 * @return {@link #category} (High-level categorization of the type of activity in a care plan.) 1175 */ 1176 public CodeableConcept getCategory() { 1177 if (this.category == null) 1178 if (Configuration.errorOnAutoCreate()) 1179 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.category"); 1180 else if (Configuration.doAutoCreate()) 1181 this.category = new CodeableConcept(); // cc 1182 return this.category; 1183 } 1184 1185 public boolean hasCategory() { 1186 return this.category != null && !this.category.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #category} (High-level categorization of the type of activity in a care plan.) 1191 */ 1192 public CarePlanActivityDetailComponent setCategory(CodeableConcept value) { 1193 this.category = value; 1194 return this; 1195 } 1196 1197 /** 1198 * @return {@link #definition} (Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with.) 1199 */ 1200 public Reference getDefinition() { 1201 if (this.definition == null) 1202 if (Configuration.errorOnAutoCreate()) 1203 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.definition"); 1204 else if (Configuration.doAutoCreate()) 1205 this.definition = new Reference(); // cc 1206 return this.definition; 1207 } 1208 1209 public boolean hasDefinition() { 1210 return this.definition != null && !this.definition.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #definition} (Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with.) 1215 */ 1216 public CarePlanActivityDetailComponent setDefinition(Reference value) { 1217 this.definition = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #definition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with.) 1223 */ 1224 public Resource getDefinitionTarget() { 1225 return this.definitionTarget; 1226 } 1227 1228 /** 1229 * @param value {@link #definition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with.) 1230 */ 1231 public CarePlanActivityDetailComponent setDefinitionTarget(Resource value) { 1232 this.definitionTarget = value; 1233 return this; 1234 } 1235 1236 /** 1237 * @return {@link #code} (Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter.) 1238 */ 1239 public CodeableConcept getCode() { 1240 if (this.code == null) 1241 if (Configuration.errorOnAutoCreate()) 1242 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.code"); 1243 else if (Configuration.doAutoCreate()) 1244 this.code = new CodeableConcept(); // cc 1245 return this.code; 1246 } 1247 1248 public boolean hasCode() { 1249 return this.code != null && !this.code.isEmpty(); 1250 } 1251 1252 /** 1253 * @param value {@link #code} (Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter.) 1254 */ 1255 public CarePlanActivityDetailComponent setCode(CodeableConcept value) { 1256 this.code = value; 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #reasonCode} (Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.) 1262 */ 1263 public List<CodeableConcept> getReasonCode() { 1264 if (this.reasonCode == null) 1265 this.reasonCode = new ArrayList<CodeableConcept>(); 1266 return this.reasonCode; 1267 } 1268 1269 /** 1270 * @return Returns a reference to <code>this</code> for easy method chaining 1271 */ 1272 public CarePlanActivityDetailComponent setReasonCode(List<CodeableConcept> theReasonCode) { 1273 this.reasonCode = theReasonCode; 1274 return this; 1275 } 1276 1277 public boolean hasReasonCode() { 1278 if (this.reasonCode == null) 1279 return false; 1280 for (CodeableConcept item : this.reasonCode) 1281 if (!item.isEmpty()) 1282 return true; 1283 return false; 1284 } 1285 1286 public CodeableConcept addReasonCode() { //3 1287 CodeableConcept t = new CodeableConcept(); 1288 if (this.reasonCode == null) 1289 this.reasonCode = new ArrayList<CodeableConcept>(); 1290 this.reasonCode.add(t); 1291 return t; 1292 } 1293 1294 public CarePlanActivityDetailComponent addReasonCode(CodeableConcept t) { //3 1295 if (t == null) 1296 return this; 1297 if (this.reasonCode == null) 1298 this.reasonCode = new ArrayList<CodeableConcept>(); 1299 this.reasonCode.add(t); 1300 return this; 1301 } 1302 1303 /** 1304 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1305 */ 1306 public CodeableConcept getReasonCodeFirstRep() { 1307 if (getReasonCode().isEmpty()) { 1308 addReasonCode(); 1309 } 1310 return getReasonCode().get(0); 1311 } 1312 1313 /** 1314 * @return {@link #reasonReference} (Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan.) 1315 */ 1316 public List<Reference> getReasonReference() { 1317 if (this.reasonReference == null) 1318 this.reasonReference = new ArrayList<Reference>(); 1319 return this.reasonReference; 1320 } 1321 1322 /** 1323 * @return Returns a reference to <code>this</code> for easy method chaining 1324 */ 1325 public CarePlanActivityDetailComponent setReasonReference(List<Reference> theReasonReference) { 1326 this.reasonReference = theReasonReference; 1327 return this; 1328 } 1329 1330 public boolean hasReasonReference() { 1331 if (this.reasonReference == null) 1332 return false; 1333 for (Reference item : this.reasonReference) 1334 if (!item.isEmpty()) 1335 return true; 1336 return false; 1337 } 1338 1339 public Reference addReasonReference() { //3 1340 Reference t = new Reference(); 1341 if (this.reasonReference == null) 1342 this.reasonReference = new ArrayList<Reference>(); 1343 this.reasonReference.add(t); 1344 return t; 1345 } 1346 1347 public CarePlanActivityDetailComponent addReasonReference(Reference t) { //3 1348 if (t == null) 1349 return this; 1350 if (this.reasonReference == null) 1351 this.reasonReference = new ArrayList<Reference>(); 1352 this.reasonReference.add(t); 1353 return this; 1354 } 1355 1356 /** 1357 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1358 */ 1359 public Reference getReasonReferenceFirstRep() { 1360 if (getReasonReference().isEmpty()) { 1361 addReasonReference(); 1362 } 1363 return getReasonReference().get(0); 1364 } 1365 1366 /** 1367 * @deprecated Use Reference#setResource(IBaseResource) instead 1368 */ 1369 @Deprecated 1370 public List<Condition> getReasonReferenceTarget() { 1371 if (this.reasonReferenceTarget == null) 1372 this.reasonReferenceTarget = new ArrayList<Condition>(); 1373 return this.reasonReferenceTarget; 1374 } 1375 1376 /** 1377 * @deprecated Use Reference#setResource(IBaseResource) instead 1378 */ 1379 @Deprecated 1380 public Condition addReasonReferenceTarget() { 1381 Condition r = new Condition(); 1382 if (this.reasonReferenceTarget == null) 1383 this.reasonReferenceTarget = new ArrayList<Condition>(); 1384 this.reasonReferenceTarget.add(r); 1385 return r; 1386 } 1387 1388 /** 1389 * @return {@link #goal} (Internal reference that identifies the goals that this activity is intended to contribute towards meeting.) 1390 */ 1391 public List<Reference> getGoal() { 1392 if (this.goal == null) 1393 this.goal = new ArrayList<Reference>(); 1394 return this.goal; 1395 } 1396 1397 /** 1398 * @return Returns a reference to <code>this</code> for easy method chaining 1399 */ 1400 public CarePlanActivityDetailComponent setGoal(List<Reference> theGoal) { 1401 this.goal = theGoal; 1402 return this; 1403 } 1404 1405 public boolean hasGoal() { 1406 if (this.goal == null) 1407 return false; 1408 for (Reference item : this.goal) 1409 if (!item.isEmpty()) 1410 return true; 1411 return false; 1412 } 1413 1414 public Reference addGoal() { //3 1415 Reference t = new Reference(); 1416 if (this.goal == null) 1417 this.goal = new ArrayList<Reference>(); 1418 this.goal.add(t); 1419 return t; 1420 } 1421 1422 public CarePlanActivityDetailComponent addGoal(Reference t) { //3 1423 if (t == null) 1424 return this; 1425 if (this.goal == null) 1426 this.goal = new ArrayList<Reference>(); 1427 this.goal.add(t); 1428 return this; 1429 } 1430 1431 /** 1432 * @return The first repetition of repeating field {@link #goal}, creating it if it does not already exist 1433 */ 1434 public Reference getGoalFirstRep() { 1435 if (getGoal().isEmpty()) { 1436 addGoal(); 1437 } 1438 return getGoal().get(0); 1439 } 1440 1441 /** 1442 * @deprecated Use Reference#setResource(IBaseResource) instead 1443 */ 1444 @Deprecated 1445 public List<Goal> getGoalTarget() { 1446 if (this.goalTarget == null) 1447 this.goalTarget = new ArrayList<Goal>(); 1448 return this.goalTarget; 1449 } 1450 1451 /** 1452 * @deprecated Use Reference#setResource(IBaseResource) instead 1453 */ 1454 @Deprecated 1455 public Goal addGoalTarget() { 1456 Goal r = new Goal(); 1457 if (this.goalTarget == null) 1458 this.goalTarget = new ArrayList<Goal>(); 1459 this.goalTarget.add(r); 1460 return r; 1461 } 1462 1463 /** 1464 * @return {@link #status} (Identifies what progress is being made for the specific activity.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1465 */ 1466 public Enumeration<CarePlanActivityStatus> getStatusElement() { 1467 if (this.status == null) 1468 if (Configuration.errorOnAutoCreate()) 1469 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.status"); 1470 else if (Configuration.doAutoCreate()) 1471 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); // bb 1472 return this.status; 1473 } 1474 1475 public boolean hasStatusElement() { 1476 return this.status != null && !this.status.isEmpty(); 1477 } 1478 1479 public boolean hasStatus() { 1480 return this.status != null && !this.status.isEmpty(); 1481 } 1482 1483 /** 1484 * @param value {@link #status} (Identifies what progress is being made for the specific activity.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1485 */ 1486 public CarePlanActivityDetailComponent setStatusElement(Enumeration<CarePlanActivityStatus> value) { 1487 this.status = value; 1488 return this; 1489 } 1490 1491 /** 1492 * @return Identifies what progress is being made for the specific activity. 1493 */ 1494 public CarePlanActivityStatus getStatus() { 1495 return this.status == null ? null : this.status.getValue(); 1496 } 1497 1498 /** 1499 * @param value Identifies what progress is being made for the specific activity. 1500 */ 1501 public CarePlanActivityDetailComponent setStatus(CarePlanActivityStatus value) { 1502 if (this.status == null) 1503 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); 1504 this.status.setValue(value); 1505 return this; 1506 } 1507 1508 /** 1509 * @return {@link #statusReason} (Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.). This is the underlying object with id, value and extensions. The accessor "getStatusReason" gives direct access to the value 1510 */ 1511 public StringType getStatusReasonElement() { 1512 if (this.statusReason == null) 1513 if (Configuration.errorOnAutoCreate()) 1514 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.statusReason"); 1515 else if (Configuration.doAutoCreate()) 1516 this.statusReason = new StringType(); // bb 1517 return this.statusReason; 1518 } 1519 1520 public boolean hasStatusReasonElement() { 1521 return this.statusReason != null && !this.statusReason.isEmpty(); 1522 } 1523 1524 public boolean hasStatusReason() { 1525 return this.statusReason != null && !this.statusReason.isEmpty(); 1526 } 1527 1528 /** 1529 * @param value {@link #statusReason} (Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.). This is the underlying object with id, value and extensions. The accessor "getStatusReason" gives direct access to the value 1530 */ 1531 public CarePlanActivityDetailComponent setStatusReasonElement(StringType value) { 1532 this.statusReason = value; 1533 return this; 1534 } 1535 1536 /** 1537 * @return Provides reason why the activity isn't yet started, is on hold, was cancelled, etc. 1538 */ 1539 public String getStatusReason() { 1540 return this.statusReason == null ? null : this.statusReason.getValue(); 1541 } 1542 1543 /** 1544 * @param value Provides reason why the activity isn't yet started, is on hold, was cancelled, etc. 1545 */ 1546 public CarePlanActivityDetailComponent setStatusReason(String value) { 1547 if (Utilities.noString(value)) 1548 this.statusReason = null; 1549 else { 1550 if (this.statusReason == null) 1551 this.statusReason = new StringType(); 1552 this.statusReason.setValue(value); 1553 } 1554 return this; 1555 } 1556 1557 /** 1558 * @return {@link #prohibited} (If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan.). This is the underlying object with id, value and extensions. The accessor "getProhibited" gives direct access to the value 1559 */ 1560 public BooleanType getProhibitedElement() { 1561 if (this.prohibited == null) 1562 if (Configuration.errorOnAutoCreate()) 1563 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.prohibited"); 1564 else if (Configuration.doAutoCreate()) 1565 this.prohibited = new BooleanType(); // bb 1566 return this.prohibited; 1567 } 1568 1569 public boolean hasProhibitedElement() { 1570 return this.prohibited != null && !this.prohibited.isEmpty(); 1571 } 1572 1573 public boolean hasProhibited() { 1574 return this.prohibited != null && !this.prohibited.isEmpty(); 1575 } 1576 1577 /** 1578 * @param value {@link #prohibited} (If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan.). This is the underlying object with id, value and extensions. The accessor "getProhibited" gives direct access to the value 1579 */ 1580 public CarePlanActivityDetailComponent setProhibitedElement(BooleanType value) { 1581 this.prohibited = value; 1582 return this; 1583 } 1584 1585 /** 1586 * @return If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan. 1587 */ 1588 public boolean getProhibited() { 1589 return this.prohibited == null || this.prohibited.isEmpty() ? false : this.prohibited.getValue(); 1590 } 1591 1592 /** 1593 * @param value If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan. 1594 */ 1595 public CarePlanActivityDetailComponent setProhibited(boolean value) { 1596 if (this.prohibited == null) 1597 this.prohibited = new BooleanType(); 1598 this.prohibited.setValue(value); 1599 return this; 1600 } 1601 1602 /** 1603 * @return {@link #scheduled} (The period, timing or frequency upon which the described activity is to occur.) 1604 */ 1605 public Type getScheduled() { 1606 return this.scheduled; 1607 } 1608 1609 /** 1610 * @return {@link #scheduled} (The period, timing or frequency upon which the described activity is to occur.) 1611 */ 1612 public Timing getScheduledTiming() throws FHIRException { 1613 if (this.scheduled == null) 1614 return null; 1615 if (!(this.scheduled instanceof Timing)) 1616 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.scheduled.getClass().getName()+" was encountered"); 1617 return (Timing) this.scheduled; 1618 } 1619 1620 public boolean hasScheduledTiming() { 1621 return this != null && this.scheduled instanceof Timing; 1622 } 1623 1624 /** 1625 * @return {@link #scheduled} (The period, timing or frequency upon which the described activity is to occur.) 1626 */ 1627 public Period getScheduledPeriod() throws FHIRException { 1628 if (this.scheduled == null) 1629 return null; 1630 if (!(this.scheduled instanceof Period)) 1631 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.scheduled.getClass().getName()+" was encountered"); 1632 return (Period) this.scheduled; 1633 } 1634 1635 public boolean hasScheduledPeriod() { 1636 return this != null && this.scheduled instanceof Period; 1637 } 1638 1639 /** 1640 * @return {@link #scheduled} (The period, timing or frequency upon which the described activity is to occur.) 1641 */ 1642 public StringType getScheduledStringType() throws FHIRException { 1643 if (this.scheduled == null) 1644 return null; 1645 if (!(this.scheduled instanceof StringType)) 1646 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.scheduled.getClass().getName()+" was encountered"); 1647 return (StringType) this.scheduled; 1648 } 1649 1650 public boolean hasScheduledStringType() { 1651 return this != null && this.scheduled instanceof StringType; 1652 } 1653 1654 public boolean hasScheduled() { 1655 return this.scheduled != null && !this.scheduled.isEmpty(); 1656 } 1657 1658 /** 1659 * @param value {@link #scheduled} (The period, timing or frequency upon which the described activity is to occur.) 1660 */ 1661 public CarePlanActivityDetailComponent setScheduled(Type value) throws FHIRFormatError { 1662 if (value != null && !(value instanceof Timing || value instanceof Period || value instanceof StringType)) 1663 throw new FHIRFormatError("Not the right type for CarePlan.activity.detail.scheduled[x]: "+value.fhirType()); 1664 this.scheduled = value; 1665 return this; 1666 } 1667 1668 /** 1669 * @return {@link #location} (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 1670 */ 1671 public Reference getLocation() { 1672 if (this.location == null) 1673 if (Configuration.errorOnAutoCreate()) 1674 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 1675 else if (Configuration.doAutoCreate()) 1676 this.location = new Reference(); // cc 1677 return this.location; 1678 } 1679 1680 public boolean hasLocation() { 1681 return this.location != null && !this.location.isEmpty(); 1682 } 1683 1684 /** 1685 * @param value {@link #location} (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 1686 */ 1687 public CarePlanActivityDetailComponent setLocation(Reference value) { 1688 this.location = value; 1689 return this; 1690 } 1691 1692 /** 1693 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 1694 */ 1695 public Location getLocationTarget() { 1696 if (this.locationTarget == null) 1697 if (Configuration.errorOnAutoCreate()) 1698 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 1699 else if (Configuration.doAutoCreate()) 1700 this.locationTarget = new Location(); // aa 1701 return this.locationTarget; 1702 } 1703 1704 /** 1705 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 1706 */ 1707 public CarePlanActivityDetailComponent setLocationTarget(Location value) { 1708 this.locationTarget = value; 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #performer} (Identifies who's expected to be involved in the activity.) 1714 */ 1715 public List<Reference> getPerformer() { 1716 if (this.performer == null) 1717 this.performer = new ArrayList<Reference>(); 1718 return this.performer; 1719 } 1720 1721 /** 1722 * @return Returns a reference to <code>this</code> for easy method chaining 1723 */ 1724 public CarePlanActivityDetailComponent setPerformer(List<Reference> thePerformer) { 1725 this.performer = thePerformer; 1726 return this; 1727 } 1728 1729 public boolean hasPerformer() { 1730 if (this.performer == null) 1731 return false; 1732 for (Reference item : this.performer) 1733 if (!item.isEmpty()) 1734 return true; 1735 return false; 1736 } 1737 1738 public Reference addPerformer() { //3 1739 Reference t = new Reference(); 1740 if (this.performer == null) 1741 this.performer = new ArrayList<Reference>(); 1742 this.performer.add(t); 1743 return t; 1744 } 1745 1746 public CarePlanActivityDetailComponent addPerformer(Reference t) { //3 1747 if (t == null) 1748 return this; 1749 if (this.performer == null) 1750 this.performer = new ArrayList<Reference>(); 1751 this.performer.add(t); 1752 return this; 1753 } 1754 1755 /** 1756 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 1757 */ 1758 public Reference getPerformerFirstRep() { 1759 if (getPerformer().isEmpty()) { 1760 addPerformer(); 1761 } 1762 return getPerformer().get(0); 1763 } 1764 1765 /** 1766 * @deprecated Use Reference#setResource(IBaseResource) instead 1767 */ 1768 @Deprecated 1769 public List<Resource> getPerformerTarget() { 1770 if (this.performerTarget == null) 1771 this.performerTarget = new ArrayList<Resource>(); 1772 return this.performerTarget; 1773 } 1774 1775 /** 1776 * @return {@link #product} (Identifies the food, drug or other product to be consumed or supplied in the activity.) 1777 */ 1778 public Type getProduct() { 1779 return this.product; 1780 } 1781 1782 /** 1783 * @return {@link #product} (Identifies the food, drug or other product to be consumed or supplied in the activity.) 1784 */ 1785 public CodeableConcept getProductCodeableConcept() throws FHIRException { 1786 if (this.product == null) 1787 return null; 1788 if (!(this.product instanceof CodeableConcept)) 1789 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.product.getClass().getName()+" was encountered"); 1790 return (CodeableConcept) this.product; 1791 } 1792 1793 public boolean hasProductCodeableConcept() { 1794 return this != null && this.product instanceof CodeableConcept; 1795 } 1796 1797 /** 1798 * @return {@link #product} (Identifies the food, drug or other product to be consumed or supplied in the activity.) 1799 */ 1800 public Reference getProductReference() throws FHIRException { 1801 if (this.product == null) 1802 return null; 1803 if (!(this.product instanceof Reference)) 1804 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.product.getClass().getName()+" was encountered"); 1805 return (Reference) this.product; 1806 } 1807 1808 public boolean hasProductReference() { 1809 return this != null && this.product instanceof Reference; 1810 } 1811 1812 public boolean hasProduct() { 1813 return this.product != null && !this.product.isEmpty(); 1814 } 1815 1816 /** 1817 * @param value {@link #product} (Identifies the food, drug or other product to be consumed or supplied in the activity.) 1818 */ 1819 public CarePlanActivityDetailComponent setProduct(Type value) throws FHIRFormatError { 1820 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1821 throw new FHIRFormatError("Not the right type for CarePlan.activity.detail.product[x]: "+value.fhirType()); 1822 this.product = value; 1823 return this; 1824 } 1825 1826 /** 1827 * @return {@link #dailyAmount} (Identifies the quantity expected to be consumed in a given day.) 1828 */ 1829 public SimpleQuantity getDailyAmount() { 1830 if (this.dailyAmount == null) 1831 if (Configuration.errorOnAutoCreate()) 1832 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.dailyAmount"); 1833 else if (Configuration.doAutoCreate()) 1834 this.dailyAmount = new SimpleQuantity(); // cc 1835 return this.dailyAmount; 1836 } 1837 1838 public boolean hasDailyAmount() { 1839 return this.dailyAmount != null && !this.dailyAmount.isEmpty(); 1840 } 1841 1842 /** 1843 * @param value {@link #dailyAmount} (Identifies the quantity expected to be consumed in a given day.) 1844 */ 1845 public CarePlanActivityDetailComponent setDailyAmount(SimpleQuantity value) { 1846 this.dailyAmount = value; 1847 return this; 1848 } 1849 1850 /** 1851 * @return {@link #quantity} (Identifies the quantity expected to be supplied, administered or consumed by the subject.) 1852 */ 1853 public SimpleQuantity getQuantity() { 1854 if (this.quantity == null) 1855 if (Configuration.errorOnAutoCreate()) 1856 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.quantity"); 1857 else if (Configuration.doAutoCreate()) 1858 this.quantity = new SimpleQuantity(); // cc 1859 return this.quantity; 1860 } 1861 1862 public boolean hasQuantity() { 1863 return this.quantity != null && !this.quantity.isEmpty(); 1864 } 1865 1866 /** 1867 * @param value {@link #quantity} (Identifies the quantity expected to be supplied, administered or consumed by the subject.) 1868 */ 1869 public CarePlanActivityDetailComponent setQuantity(SimpleQuantity value) { 1870 this.quantity = value; 1871 return this; 1872 } 1873 1874 /** 1875 * @return {@link #description} (This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1876 */ 1877 public StringType getDescriptionElement() { 1878 if (this.description == null) 1879 if (Configuration.errorOnAutoCreate()) 1880 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.description"); 1881 else if (Configuration.doAutoCreate()) 1882 this.description = new StringType(); // bb 1883 return this.description; 1884 } 1885 1886 public boolean hasDescriptionElement() { 1887 return this.description != null && !this.description.isEmpty(); 1888 } 1889 1890 public boolean hasDescription() { 1891 return this.description != null && !this.description.isEmpty(); 1892 } 1893 1894 /** 1895 * @param value {@link #description} (This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1896 */ 1897 public CarePlanActivityDetailComponent setDescriptionElement(StringType value) { 1898 this.description = value; 1899 return this; 1900 } 1901 1902 /** 1903 * @return This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc. 1904 */ 1905 public String getDescription() { 1906 return this.description == null ? null : this.description.getValue(); 1907 } 1908 1909 /** 1910 * @param value This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc. 1911 */ 1912 public CarePlanActivityDetailComponent setDescription(String value) { 1913 if (Utilities.noString(value)) 1914 this.description = null; 1915 else { 1916 if (this.description == null) 1917 this.description = new StringType(); 1918 this.description.setValue(value); 1919 } 1920 return this; 1921 } 1922 1923 protected void listChildren(List<Property> children) { 1924 super.listChildren(children); 1925 children.add(new Property("category", "CodeableConcept", "High-level categorization of the type of activity in a care plan.", 0, 1, category)); 1926 children.add(new Property("definition", "Reference(PlanDefinition|ActivityDefinition|Questionnaire)", "Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with.", 0, 1, definition)); 1927 children.add(new Property("code", "CodeableConcept", "Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 1, code)); 1928 children.add(new Property("reasonCode", "CodeableConcept", "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1929 children.add(new Property("reasonReference", "Reference(Condition)", "Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1930 children.add(new Property("goal", "Reference(Goal)", "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.", 0, java.lang.Integer.MAX_VALUE, goal)); 1931 children.add(new Property("status", "code", "Identifies what progress is being made for the specific activity.", 0, 1, status)); 1932 children.add(new Property("statusReason", "string", "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.", 0, 1, statusReason)); 1933 children.add(new Property("prohibited", "boolean", "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan.", 0, 1, prohibited)); 1934 children.add(new Property("scheduled[x]", "Timing|Period|string", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled)); 1935 children.add(new Property("location", "Reference(Location)", "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location)); 1936 children.add(new Property("performer", "Reference(Practitioner|Organization|RelatedPerson|Patient|CareTeam)", "Identifies who's expected to be involved in the activity.", 0, java.lang.Integer.MAX_VALUE, performer)); 1937 children.add(new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product)); 1938 children.add(new Property("dailyAmount", "SimpleQuantity", "Identifies the quantity expected to be consumed in a given day.", 0, 1, dailyAmount)); 1939 children.add(new Property("quantity", "SimpleQuantity", "Identifies the quantity expected to be supplied, administered or consumed by the subject.", 0, 1, quantity)); 1940 children.add(new Property("description", "string", "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.", 0, 1, description)); 1941 } 1942 1943 @Override 1944 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1945 switch (_hash) { 1946 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "High-level categorization of the type of activity in a care plan.", 0, 1, category); 1947 case -1014418093: /*definition*/ return new Property("definition", "Reference(PlanDefinition|ActivityDefinition|Questionnaire)", "Identifies the protocol, questionnaire, guideline or other specification the planned activity should be conducted in accordance with.", 0, 1, definition); 1948 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 1, code); 1949 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1950 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition)", "Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1951 case 3178259: /*goal*/ return new Property("goal", "Reference(Goal)", "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.", 0, java.lang.Integer.MAX_VALUE, goal); 1952 case -892481550: /*status*/ return new Property("status", "code", "Identifies what progress is being made for the specific activity.", 0, 1, status); 1953 case 2051346646: /*statusReason*/ return new Property("statusReason", "string", "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.", 0, 1, statusReason); 1954 case 663275198: /*prohibited*/ return new Property("prohibited", "boolean", "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, indicates that the described activity is one that should be engaged in when following the plan.", 0, 1, prohibited); 1955 case 1162627251: /*scheduled[x]*/ return new Property("scheduled[x]", "Timing|Period|string", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 1956 case -160710483: /*scheduled*/ return new Property("scheduled[x]", "Timing|Period|string", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 1957 case 998483799: /*scheduledTiming*/ return new Property("scheduled[x]", "Timing|Period|string", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 1958 case 880422094: /*scheduledPeriod*/ return new Property("scheduled[x]", "Timing|Period|string", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 1959 case 980162334: /*scheduledString*/ return new Property("scheduled[x]", "Timing|Period|string", "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 1960 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location); 1961 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|Organization|RelatedPerson|Patient|CareTeam)", "Identifies who's expected to be involved in the activity.", 0, java.lang.Integer.MAX_VALUE, performer); 1962 case 1753005361: /*product[x]*/ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 1963 case -309474065: /*product*/ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 1964 case 906854066: /*productCodeableConcept*/ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 1965 case -669667556: /*productReference*/ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 1966 case -768908335: /*dailyAmount*/ return new Property("dailyAmount", "SimpleQuantity", "Identifies the quantity expected to be consumed in a given day.", 0, 1, dailyAmount); 1967 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "Identifies the quantity expected to be supplied, administered or consumed by the subject.", 0, 1, quantity); 1968 case -1724546052: /*description*/ return new Property("description", "string", "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.", 0, 1, description); 1969 default: return super.getNamedProperty(_hash, _name, _checkValid); 1970 } 1971 1972 } 1973 1974 @Override 1975 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1976 switch (hash) { 1977 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1978 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Reference 1979 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1980 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1981 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1982 case 3178259: /*goal*/ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 1983 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CarePlanActivityStatus> 1984 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // StringType 1985 case 663275198: /*prohibited*/ return this.prohibited == null ? new Base[0] : new Base[] {this.prohibited}; // BooleanType 1986 case -160710483: /*scheduled*/ return this.scheduled == null ? new Base[0] : new Base[] {this.scheduled}; // Type 1987 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1988 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 1989 case -309474065: /*product*/ return this.product == null ? new Base[0] : new Base[] {this.product}; // Type 1990 case -768908335: /*dailyAmount*/ return this.dailyAmount == null ? new Base[0] : new Base[] {this.dailyAmount}; // SimpleQuantity 1991 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 1992 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1993 default: return super.getProperty(hash, name, checkValid); 1994 } 1995 1996 } 1997 1998 @Override 1999 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2000 switch (hash) { 2001 case 50511102: // category 2002 this.category = castToCodeableConcept(value); // CodeableConcept 2003 return value; 2004 case -1014418093: // definition 2005 this.definition = castToReference(value); // Reference 2006 return value; 2007 case 3059181: // code 2008 this.code = castToCodeableConcept(value); // CodeableConcept 2009 return value; 2010 case 722137681: // reasonCode 2011 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2012 return value; 2013 case -1146218137: // reasonReference 2014 this.getReasonReference().add(castToReference(value)); // Reference 2015 return value; 2016 case 3178259: // goal 2017 this.getGoal().add(castToReference(value)); // Reference 2018 return value; 2019 case -892481550: // status 2020 value = new CarePlanActivityStatusEnumFactory().fromType(castToCode(value)); 2021 this.status = (Enumeration) value; // Enumeration<CarePlanActivityStatus> 2022 return value; 2023 case 2051346646: // statusReason 2024 this.statusReason = castToString(value); // StringType 2025 return value; 2026 case 663275198: // prohibited 2027 this.prohibited = castToBoolean(value); // BooleanType 2028 return value; 2029 case -160710483: // scheduled 2030 this.scheduled = castToType(value); // Type 2031 return value; 2032 case 1901043637: // location 2033 this.location = castToReference(value); // Reference 2034 return value; 2035 case 481140686: // performer 2036 this.getPerformer().add(castToReference(value)); // Reference 2037 return value; 2038 case -309474065: // product 2039 this.product = castToType(value); // Type 2040 return value; 2041 case -768908335: // dailyAmount 2042 this.dailyAmount = castToSimpleQuantity(value); // SimpleQuantity 2043 return value; 2044 case -1285004149: // quantity 2045 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2046 return value; 2047 case -1724546052: // description 2048 this.description = castToString(value); // StringType 2049 return value; 2050 default: return super.setProperty(hash, name, value); 2051 } 2052 2053 } 2054 2055 @Override 2056 public Base setProperty(String name, Base value) throws FHIRException { 2057 if (name.equals("category")) { 2058 this.category = castToCodeableConcept(value); // CodeableConcept 2059 } else if (name.equals("definition")) { 2060 this.definition = castToReference(value); // Reference 2061 } else if (name.equals("code")) { 2062 this.code = castToCodeableConcept(value); // CodeableConcept 2063 } else if (name.equals("reasonCode")) { 2064 this.getReasonCode().add(castToCodeableConcept(value)); 2065 } else if (name.equals("reasonReference")) { 2066 this.getReasonReference().add(castToReference(value)); 2067 } else if (name.equals("goal")) { 2068 this.getGoal().add(castToReference(value)); 2069 } else if (name.equals("status")) { 2070 value = new CarePlanActivityStatusEnumFactory().fromType(castToCode(value)); 2071 this.status = (Enumeration) value; // Enumeration<CarePlanActivityStatus> 2072 } else if (name.equals("statusReason")) { 2073 this.statusReason = castToString(value); // StringType 2074 } else if (name.equals("prohibited")) { 2075 this.prohibited = castToBoolean(value); // BooleanType 2076 } else if (name.equals("scheduled[x]")) { 2077 this.scheduled = castToType(value); // Type 2078 } else if (name.equals("location")) { 2079 this.location = castToReference(value); // Reference 2080 } else if (name.equals("performer")) { 2081 this.getPerformer().add(castToReference(value)); 2082 } else if (name.equals("product[x]")) { 2083 this.product = castToType(value); // Type 2084 } else if (name.equals("dailyAmount")) { 2085 this.dailyAmount = castToSimpleQuantity(value); // SimpleQuantity 2086 } else if (name.equals("quantity")) { 2087 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2088 } else if (name.equals("description")) { 2089 this.description = castToString(value); // StringType 2090 } else 2091 return super.setProperty(name, value); 2092 return value; 2093 } 2094 2095 @Override 2096 public Base makeProperty(int hash, String name) throws FHIRException { 2097 switch (hash) { 2098 case 50511102: return getCategory(); 2099 case -1014418093: return getDefinition(); 2100 case 3059181: return getCode(); 2101 case 722137681: return addReasonCode(); 2102 case -1146218137: return addReasonReference(); 2103 case 3178259: return addGoal(); 2104 case -892481550: return getStatusElement(); 2105 case 2051346646: return getStatusReasonElement(); 2106 case 663275198: return getProhibitedElement(); 2107 case 1162627251: return getScheduled(); 2108 case -160710483: return getScheduled(); 2109 case 1901043637: return getLocation(); 2110 case 481140686: return addPerformer(); 2111 case 1753005361: return getProduct(); 2112 case -309474065: return getProduct(); 2113 case -768908335: return getDailyAmount(); 2114 case -1285004149: return getQuantity(); 2115 case -1724546052: return getDescriptionElement(); 2116 default: return super.makeProperty(hash, name); 2117 } 2118 2119 } 2120 2121 @Override 2122 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2123 switch (hash) { 2124 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2125 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2126 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2127 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2128 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2129 case 3178259: /*goal*/ return new String[] {"Reference"}; 2130 case -892481550: /*status*/ return new String[] {"code"}; 2131 case 2051346646: /*statusReason*/ return new String[] {"string"}; 2132 case 663275198: /*prohibited*/ return new String[] {"boolean"}; 2133 case -160710483: /*scheduled*/ return new String[] {"Timing", "Period", "string"}; 2134 case 1901043637: /*location*/ return new String[] {"Reference"}; 2135 case 481140686: /*performer*/ return new String[] {"Reference"}; 2136 case -309474065: /*product*/ return new String[] {"CodeableConcept", "Reference"}; 2137 case -768908335: /*dailyAmount*/ return new String[] {"SimpleQuantity"}; 2138 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 2139 case -1724546052: /*description*/ return new String[] {"string"}; 2140 default: return super.getTypesForProperty(hash, name); 2141 } 2142 2143 } 2144 2145 @Override 2146 public Base addChild(String name) throws FHIRException { 2147 if (name.equals("category")) { 2148 this.category = new CodeableConcept(); 2149 return this.category; 2150 } 2151 else if (name.equals("definition")) { 2152 this.definition = new Reference(); 2153 return this.definition; 2154 } 2155 else if (name.equals("code")) { 2156 this.code = new CodeableConcept(); 2157 return this.code; 2158 } 2159 else if (name.equals("reasonCode")) { 2160 return addReasonCode(); 2161 } 2162 else if (name.equals("reasonReference")) { 2163 return addReasonReference(); 2164 } 2165 else if (name.equals("goal")) { 2166 return addGoal(); 2167 } 2168 else if (name.equals("status")) { 2169 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 2170 } 2171 else if (name.equals("statusReason")) { 2172 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.statusReason"); 2173 } 2174 else if (name.equals("prohibited")) { 2175 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.prohibited"); 2176 } 2177 else if (name.equals("scheduledTiming")) { 2178 this.scheduled = new Timing(); 2179 return this.scheduled; 2180 } 2181 else if (name.equals("scheduledPeriod")) { 2182 this.scheduled = new Period(); 2183 return this.scheduled; 2184 } 2185 else if (name.equals("scheduledString")) { 2186 this.scheduled = new StringType(); 2187 return this.scheduled; 2188 } 2189 else if (name.equals("location")) { 2190 this.location = new Reference(); 2191 return this.location; 2192 } 2193 else if (name.equals("performer")) { 2194 return addPerformer(); 2195 } 2196 else if (name.equals("productCodeableConcept")) { 2197 this.product = new CodeableConcept(); 2198 return this.product; 2199 } 2200 else if (name.equals("productReference")) { 2201 this.product = new Reference(); 2202 return this.product; 2203 } 2204 else if (name.equals("dailyAmount")) { 2205 this.dailyAmount = new SimpleQuantity(); 2206 return this.dailyAmount; 2207 } 2208 else if (name.equals("quantity")) { 2209 this.quantity = new SimpleQuantity(); 2210 return this.quantity; 2211 } 2212 else if (name.equals("description")) { 2213 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 2214 } 2215 else 2216 return super.addChild(name); 2217 } 2218 2219 public CarePlanActivityDetailComponent copy() { 2220 CarePlanActivityDetailComponent dst = new CarePlanActivityDetailComponent(); 2221 copyValues(dst); 2222 dst.category = category == null ? null : category.copy(); 2223 dst.definition = definition == null ? null : definition.copy(); 2224 dst.code = code == null ? null : code.copy(); 2225 if (reasonCode != null) { 2226 dst.reasonCode = new ArrayList<CodeableConcept>(); 2227 for (CodeableConcept i : reasonCode) 2228 dst.reasonCode.add(i.copy()); 2229 }; 2230 if (reasonReference != null) { 2231 dst.reasonReference = new ArrayList<Reference>(); 2232 for (Reference i : reasonReference) 2233 dst.reasonReference.add(i.copy()); 2234 }; 2235 if (goal != null) { 2236 dst.goal = new ArrayList<Reference>(); 2237 for (Reference i : goal) 2238 dst.goal.add(i.copy()); 2239 }; 2240 dst.status = status == null ? null : status.copy(); 2241 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2242 dst.prohibited = prohibited == null ? null : prohibited.copy(); 2243 dst.scheduled = scheduled == null ? null : scheduled.copy(); 2244 dst.location = location == null ? null : location.copy(); 2245 if (performer != null) { 2246 dst.performer = new ArrayList<Reference>(); 2247 for (Reference i : performer) 2248 dst.performer.add(i.copy()); 2249 }; 2250 dst.product = product == null ? null : product.copy(); 2251 dst.dailyAmount = dailyAmount == null ? null : dailyAmount.copy(); 2252 dst.quantity = quantity == null ? null : quantity.copy(); 2253 dst.description = description == null ? null : description.copy(); 2254 return dst; 2255 } 2256 2257 @Override 2258 public boolean equalsDeep(Base other_) { 2259 if (!super.equalsDeep(other_)) 2260 return false; 2261 if (!(other_ instanceof CarePlanActivityDetailComponent)) 2262 return false; 2263 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other_; 2264 return compareDeep(category, o.category, true) && compareDeep(definition, o.definition, true) && compareDeep(code, o.code, true) 2265 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2266 && compareDeep(goal, o.goal, true) && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 2267 && compareDeep(prohibited, o.prohibited, true) && compareDeep(scheduled, o.scheduled, true) && compareDeep(location, o.location, true) 2268 && compareDeep(performer, o.performer, true) && compareDeep(product, o.product, true) && compareDeep(dailyAmount, o.dailyAmount, true) 2269 && compareDeep(quantity, o.quantity, true) && compareDeep(description, o.description, true); 2270 } 2271 2272 @Override 2273 public boolean equalsShallow(Base other_) { 2274 if (!super.equalsShallow(other_)) 2275 return false; 2276 if (!(other_ instanceof CarePlanActivityDetailComponent)) 2277 return false; 2278 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other_; 2279 return compareValues(status, o.status, true) && compareValues(statusReason, o.statusReason, true) && compareValues(prohibited, o.prohibited, true) 2280 && compareValues(description, o.description, true); 2281 } 2282 2283 public boolean isEmpty() { 2284 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, definition, code 2285 , reasonCode, reasonReference, goal, status, statusReason, prohibited, scheduled 2286 , location, performer, product, dailyAmount, quantity, description); 2287 } 2288 2289 public String fhirType() { 2290 return "CarePlan.activity.detail"; 2291 2292 } 2293 2294 } 2295 2296 /** 2297 * This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 2298 */ 2299 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2300 @Description(shortDefinition="External Ids for this plan", formalDefinition="This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 2301 protected List<Identifier> identifier; 2302 2303 /** 2304 * Identifies the protocol, questionnaire, guideline or other specification the care plan should be conducted in accordance with. 2305 */ 2306 @Child(name = "definition", type = {PlanDefinition.class, Questionnaire.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2307 @Description(shortDefinition="Protocol or definition", formalDefinition="Identifies the protocol, questionnaire, guideline or other specification the care plan should be conducted in accordance with." ) 2308 protected List<Reference> definition; 2309 /** 2310 * The actual objects that are the target of the reference (Identifies the protocol, questionnaire, guideline or other specification the care plan should be conducted in accordance with.) 2311 */ 2312 protected List<Resource> definitionTarget; 2313 2314 2315 /** 2316 * A care plan that is fulfilled in whole or in part by this care plan. 2317 */ 2318 @Child(name = "basedOn", type = {CarePlan.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2319 @Description(shortDefinition="Fulfills care plan", formalDefinition="A care plan that is fulfilled in whole or in part by this care plan." ) 2320 protected List<Reference> basedOn; 2321 /** 2322 * The actual objects that are the target of the reference (A care plan that is fulfilled in whole or in part by this care plan.) 2323 */ 2324 protected List<CarePlan> basedOnTarget; 2325 2326 2327 /** 2328 * Completed or terminated care plan whose function is taken by this new care plan. 2329 */ 2330 @Child(name = "replaces", type = {CarePlan.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2331 @Description(shortDefinition="CarePlan replaced by this CarePlan", formalDefinition="Completed or terminated care plan whose function is taken by this new care plan." ) 2332 protected List<Reference> replaces; 2333 /** 2334 * The actual objects that are the target of the reference (Completed or terminated care plan whose function is taken by this new care plan.) 2335 */ 2336 protected List<CarePlan> replacesTarget; 2337 2338 2339 /** 2340 * A larger care plan of which this particular care plan is a component or step. 2341 */ 2342 @Child(name = "partOf", type = {CarePlan.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2343 @Description(shortDefinition="Part of referenced CarePlan", formalDefinition="A larger care plan of which this particular care plan is a component or step." ) 2344 protected List<Reference> partOf; 2345 /** 2346 * The actual objects that are the target of the reference (A larger care plan of which this particular care plan is a component or step.) 2347 */ 2348 protected List<CarePlan> partOfTarget; 2349 2350 2351 /** 2352 * Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record. 2353 */ 2354 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 2355 @Description(shortDefinition="draft | active | suspended | completed | entered-in-error | cancelled | unknown", formalDefinition="Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record." ) 2356 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-status") 2357 protected Enumeration<CarePlanStatus> status; 2358 2359 /** 2360 * Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain. 2361 */ 2362 @Child(name = "intent", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 2363 @Description(shortDefinition="proposal | plan | order | option", formalDefinition="Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain." ) 2364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-intent") 2365 protected Enumeration<CarePlanIntent> intent; 2366 2367 /** 2368 * Identifies what "kind" of plan this is to support differentiation between multiple co-existing plans; e.g. "Home health", "psychiatric", "asthma", "disease management", "wellness plan", etc. 2369 */ 2370 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2371 @Description(shortDefinition="Type of plan", formalDefinition="Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc." ) 2372 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-category") 2373 protected List<CodeableConcept> category; 2374 2375 /** 2376 * Human-friendly name for the CarePlan. 2377 */ 2378 @Child(name = "title", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 2379 @Description(shortDefinition="Human-friendly name for the CarePlan", formalDefinition="Human-friendly name for the CarePlan." ) 2380 protected StringType title; 2381 2382 /** 2383 * A description of the scope and nature of the plan. 2384 */ 2385 @Child(name = "description", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2386 @Description(shortDefinition="Summary of nature of plan", formalDefinition="A description of the scope and nature of the plan." ) 2387 protected StringType description; 2388 2389 /** 2390 * Identifies the patient or group whose intended care is described by the plan. 2391 */ 2392 @Child(name = "subject", type = {Patient.class, Group.class}, order=10, min=1, max=1, modifier=false, summary=true) 2393 @Description(shortDefinition="Who care plan is for", formalDefinition="Identifies the patient or group whose intended care is described by the plan." ) 2394 protected Reference subject; 2395 2396 /** 2397 * The actual object that is the target of the reference (Identifies the patient or group whose intended care is described by the plan.) 2398 */ 2399 protected Resource subjectTarget; 2400 2401 /** 2402 * Identifies the original context in which this particular CarePlan was created. 2403 */ 2404 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=11, min=0, max=1, modifier=false, summary=true) 2405 @Description(shortDefinition="Created in context of", formalDefinition="Identifies the original context in which this particular CarePlan was created." ) 2406 protected Reference context; 2407 2408 /** 2409 * The actual object that is the target of the reference (Identifies the original context in which this particular CarePlan was created.) 2410 */ 2411 protected Resource contextTarget; 2412 2413 /** 2414 * Indicates when the plan did (or is intended to) come into effect and end. 2415 */ 2416 @Child(name = "period", type = {Period.class}, order=12, min=0, max=1, modifier=false, summary=true) 2417 @Description(shortDefinition="Time period plan covers", formalDefinition="Indicates when the plan did (or is intended to) come into effect and end." ) 2418 protected Period period; 2419 2420 /** 2421 * Identifies the individual(s) or ogranization who is responsible for the content of the care plan. 2422 */ 2423 @Child(name = "author", type = {Patient.class, Practitioner.class, RelatedPerson.class, Organization.class, CareTeam.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2424 @Description(shortDefinition="Who is responsible for contents of the plan", formalDefinition="Identifies the individual(s) or ogranization who is responsible for the content of the care plan." ) 2425 protected List<Reference> author; 2426 /** 2427 * The actual objects that are the target of the reference (Identifies the individual(s) or ogranization who is responsible for the content of the care plan.) 2428 */ 2429 protected List<Resource> authorTarget; 2430 2431 2432 /** 2433 * Identifies all people and organizations who are expected to be involved in the care envisioned by this plan. 2434 */ 2435 @Child(name = "careTeam", type = {CareTeam.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2436 @Description(shortDefinition="Who's involved in plan?", formalDefinition="Identifies all people and organizations who are expected to be involved in the care envisioned by this plan." ) 2437 protected List<Reference> careTeam; 2438 /** 2439 * The actual objects that are the target of the reference (Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.) 2440 */ 2441 protected List<CareTeam> careTeamTarget; 2442 2443 2444 /** 2445 * Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan. 2446 */ 2447 @Child(name = "addresses", type = {Condition.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2448 @Description(shortDefinition="Health issues this plan addresses", formalDefinition="Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan." ) 2449 protected List<Reference> addresses; 2450 /** 2451 * The actual objects that are the target of the reference (Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.) 2452 */ 2453 protected List<Condition> addressesTarget; 2454 2455 2456 /** 2457 * Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc. 2458 */ 2459 @Child(name = "supportingInfo", type = {Reference.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2460 @Description(shortDefinition="Information considered as part of plan", formalDefinition="Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc." ) 2461 protected List<Reference> supportingInfo; 2462 /** 2463 * The actual objects that are the target of the reference (Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc.) 2464 */ 2465 protected List<Resource> supportingInfoTarget; 2466 2467 2468 /** 2469 * Describes the intended objective(s) of carrying out the care plan. 2470 */ 2471 @Child(name = "goal", type = {Goal.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2472 @Description(shortDefinition="Desired outcome of plan", formalDefinition="Describes the intended objective(s) of carrying out the care plan." ) 2473 protected List<Reference> goal; 2474 /** 2475 * The actual objects that are the target of the reference (Describes the intended objective(s) of carrying out the care plan.) 2476 */ 2477 protected List<Goal> goalTarget; 2478 2479 2480 /** 2481 * Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc. 2482 */ 2483 @Child(name = "activity", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2484 @Description(shortDefinition="Action to occur as part of plan", formalDefinition="Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc." ) 2485 protected List<CarePlanActivityComponent> activity; 2486 2487 /** 2488 * General notes about the care plan not covered elsewhere. 2489 */ 2490 @Child(name = "note", type = {Annotation.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2491 @Description(shortDefinition="Comments about the plan", formalDefinition="General notes about the care plan not covered elsewhere." ) 2492 protected List<Annotation> note; 2493 2494 private static final long serialVersionUID = 995943625L; 2495 2496 /** 2497 * Constructor 2498 */ 2499 public CarePlan() { 2500 super(); 2501 } 2502 2503 /** 2504 * Constructor 2505 */ 2506 public CarePlan(Enumeration<CarePlanStatus> status, Enumeration<CarePlanIntent> intent, Reference subject) { 2507 super(); 2508 this.status = status; 2509 this.intent = intent; 2510 this.subject = subject; 2511 } 2512 2513 /** 2514 * @return {@link #identifier} (This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 2515 */ 2516 public List<Identifier> getIdentifier() { 2517 if (this.identifier == null) 2518 this.identifier = new ArrayList<Identifier>(); 2519 return this.identifier; 2520 } 2521 2522 /** 2523 * @return Returns a reference to <code>this</code> for easy method chaining 2524 */ 2525 public CarePlan setIdentifier(List<Identifier> theIdentifier) { 2526 this.identifier = theIdentifier; 2527 return this; 2528 } 2529 2530 public boolean hasIdentifier() { 2531 if (this.identifier == null) 2532 return false; 2533 for (Identifier item : this.identifier) 2534 if (!item.isEmpty()) 2535 return true; 2536 return false; 2537 } 2538 2539 public Identifier addIdentifier() { //3 2540 Identifier t = new Identifier(); 2541 if (this.identifier == null) 2542 this.identifier = new ArrayList<Identifier>(); 2543 this.identifier.add(t); 2544 return t; 2545 } 2546 2547 public CarePlan addIdentifier(Identifier t) { //3 2548 if (t == null) 2549 return this; 2550 if (this.identifier == null) 2551 this.identifier = new ArrayList<Identifier>(); 2552 this.identifier.add(t); 2553 return this; 2554 } 2555 2556 /** 2557 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 2558 */ 2559 public Identifier getIdentifierFirstRep() { 2560 if (getIdentifier().isEmpty()) { 2561 addIdentifier(); 2562 } 2563 return getIdentifier().get(0); 2564 } 2565 2566 /** 2567 * @return {@link #definition} (Identifies the protocol, questionnaire, guideline or other specification the care plan should be conducted in accordance with.) 2568 */ 2569 public List<Reference> getDefinition() { 2570 if (this.definition == null) 2571 this.definition = new ArrayList<Reference>(); 2572 return this.definition; 2573 } 2574 2575 /** 2576 * @return Returns a reference to <code>this</code> for easy method chaining 2577 */ 2578 public CarePlan setDefinition(List<Reference> theDefinition) { 2579 this.definition = theDefinition; 2580 return this; 2581 } 2582 2583 public boolean hasDefinition() { 2584 if (this.definition == null) 2585 return false; 2586 for (Reference item : this.definition) 2587 if (!item.isEmpty()) 2588 return true; 2589 return false; 2590 } 2591 2592 public Reference addDefinition() { //3 2593 Reference t = new Reference(); 2594 if (this.definition == null) 2595 this.definition = new ArrayList<Reference>(); 2596 this.definition.add(t); 2597 return t; 2598 } 2599 2600 public CarePlan addDefinition(Reference t) { //3 2601 if (t == null) 2602 return this; 2603 if (this.definition == null) 2604 this.definition = new ArrayList<Reference>(); 2605 this.definition.add(t); 2606 return this; 2607 } 2608 2609 /** 2610 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 2611 */ 2612 public Reference getDefinitionFirstRep() { 2613 if (getDefinition().isEmpty()) { 2614 addDefinition(); 2615 } 2616 return getDefinition().get(0); 2617 } 2618 2619 /** 2620 * @deprecated Use Reference#setResource(IBaseResource) instead 2621 */ 2622 @Deprecated 2623 public List<Resource> getDefinitionTarget() { 2624 if (this.definitionTarget == null) 2625 this.definitionTarget = new ArrayList<Resource>(); 2626 return this.definitionTarget; 2627 } 2628 2629 /** 2630 * @return {@link #basedOn} (A care plan that is fulfilled in whole or in part by this care plan.) 2631 */ 2632 public List<Reference> getBasedOn() { 2633 if (this.basedOn == null) 2634 this.basedOn = new ArrayList<Reference>(); 2635 return this.basedOn; 2636 } 2637 2638 /** 2639 * @return Returns a reference to <code>this</code> for easy method chaining 2640 */ 2641 public CarePlan setBasedOn(List<Reference> theBasedOn) { 2642 this.basedOn = theBasedOn; 2643 return this; 2644 } 2645 2646 public boolean hasBasedOn() { 2647 if (this.basedOn == null) 2648 return false; 2649 for (Reference item : this.basedOn) 2650 if (!item.isEmpty()) 2651 return true; 2652 return false; 2653 } 2654 2655 public Reference addBasedOn() { //3 2656 Reference t = new Reference(); 2657 if (this.basedOn == null) 2658 this.basedOn = new ArrayList<Reference>(); 2659 this.basedOn.add(t); 2660 return t; 2661 } 2662 2663 public CarePlan addBasedOn(Reference t) { //3 2664 if (t == null) 2665 return this; 2666 if (this.basedOn == null) 2667 this.basedOn = new ArrayList<Reference>(); 2668 this.basedOn.add(t); 2669 return this; 2670 } 2671 2672 /** 2673 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 2674 */ 2675 public Reference getBasedOnFirstRep() { 2676 if (getBasedOn().isEmpty()) { 2677 addBasedOn(); 2678 } 2679 return getBasedOn().get(0); 2680 } 2681 2682 /** 2683 * @deprecated Use Reference#setResource(IBaseResource) instead 2684 */ 2685 @Deprecated 2686 public List<CarePlan> getBasedOnTarget() { 2687 if (this.basedOnTarget == null) 2688 this.basedOnTarget = new ArrayList<CarePlan>(); 2689 return this.basedOnTarget; 2690 } 2691 2692 /** 2693 * @deprecated Use Reference#setResource(IBaseResource) instead 2694 */ 2695 @Deprecated 2696 public CarePlan addBasedOnTarget() { 2697 CarePlan r = new CarePlan(); 2698 if (this.basedOnTarget == null) 2699 this.basedOnTarget = new ArrayList<CarePlan>(); 2700 this.basedOnTarget.add(r); 2701 return r; 2702 } 2703 2704 /** 2705 * @return {@link #replaces} (Completed or terminated care plan whose function is taken by this new care plan.) 2706 */ 2707 public List<Reference> getReplaces() { 2708 if (this.replaces == null) 2709 this.replaces = new ArrayList<Reference>(); 2710 return this.replaces; 2711 } 2712 2713 /** 2714 * @return Returns a reference to <code>this</code> for easy method chaining 2715 */ 2716 public CarePlan setReplaces(List<Reference> theReplaces) { 2717 this.replaces = theReplaces; 2718 return this; 2719 } 2720 2721 public boolean hasReplaces() { 2722 if (this.replaces == null) 2723 return false; 2724 for (Reference item : this.replaces) 2725 if (!item.isEmpty()) 2726 return true; 2727 return false; 2728 } 2729 2730 public Reference addReplaces() { //3 2731 Reference t = new Reference(); 2732 if (this.replaces == null) 2733 this.replaces = new ArrayList<Reference>(); 2734 this.replaces.add(t); 2735 return t; 2736 } 2737 2738 public CarePlan addReplaces(Reference t) { //3 2739 if (t == null) 2740 return this; 2741 if (this.replaces == null) 2742 this.replaces = new ArrayList<Reference>(); 2743 this.replaces.add(t); 2744 return this; 2745 } 2746 2747 /** 2748 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 2749 */ 2750 public Reference getReplacesFirstRep() { 2751 if (getReplaces().isEmpty()) { 2752 addReplaces(); 2753 } 2754 return getReplaces().get(0); 2755 } 2756 2757 /** 2758 * @deprecated Use Reference#setResource(IBaseResource) instead 2759 */ 2760 @Deprecated 2761 public List<CarePlan> getReplacesTarget() { 2762 if (this.replacesTarget == null) 2763 this.replacesTarget = new ArrayList<CarePlan>(); 2764 return this.replacesTarget; 2765 } 2766 2767 /** 2768 * @deprecated Use Reference#setResource(IBaseResource) instead 2769 */ 2770 @Deprecated 2771 public CarePlan addReplacesTarget() { 2772 CarePlan r = new CarePlan(); 2773 if (this.replacesTarget == null) 2774 this.replacesTarget = new ArrayList<CarePlan>(); 2775 this.replacesTarget.add(r); 2776 return r; 2777 } 2778 2779 /** 2780 * @return {@link #partOf} (A larger care plan of which this particular care plan is a component or step.) 2781 */ 2782 public List<Reference> getPartOf() { 2783 if (this.partOf == null) 2784 this.partOf = new ArrayList<Reference>(); 2785 return this.partOf; 2786 } 2787 2788 /** 2789 * @return Returns a reference to <code>this</code> for easy method chaining 2790 */ 2791 public CarePlan setPartOf(List<Reference> thePartOf) { 2792 this.partOf = thePartOf; 2793 return this; 2794 } 2795 2796 public boolean hasPartOf() { 2797 if (this.partOf == null) 2798 return false; 2799 for (Reference item : this.partOf) 2800 if (!item.isEmpty()) 2801 return true; 2802 return false; 2803 } 2804 2805 public Reference addPartOf() { //3 2806 Reference t = new Reference(); 2807 if (this.partOf == null) 2808 this.partOf = new ArrayList<Reference>(); 2809 this.partOf.add(t); 2810 return t; 2811 } 2812 2813 public CarePlan addPartOf(Reference t) { //3 2814 if (t == null) 2815 return this; 2816 if (this.partOf == null) 2817 this.partOf = new ArrayList<Reference>(); 2818 this.partOf.add(t); 2819 return this; 2820 } 2821 2822 /** 2823 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 2824 */ 2825 public Reference getPartOfFirstRep() { 2826 if (getPartOf().isEmpty()) { 2827 addPartOf(); 2828 } 2829 return getPartOf().get(0); 2830 } 2831 2832 /** 2833 * @deprecated Use Reference#setResource(IBaseResource) instead 2834 */ 2835 @Deprecated 2836 public List<CarePlan> getPartOfTarget() { 2837 if (this.partOfTarget == null) 2838 this.partOfTarget = new ArrayList<CarePlan>(); 2839 return this.partOfTarget; 2840 } 2841 2842 /** 2843 * @deprecated Use Reference#setResource(IBaseResource) instead 2844 */ 2845 @Deprecated 2846 public CarePlan addPartOfTarget() { 2847 CarePlan r = new CarePlan(); 2848 if (this.partOfTarget == null) 2849 this.partOfTarget = new ArrayList<CarePlan>(); 2850 this.partOfTarget.add(r); 2851 return r; 2852 } 2853 2854 /** 2855 * @return {@link #status} (Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2856 */ 2857 public Enumeration<CarePlanStatus> getStatusElement() { 2858 if (this.status == null) 2859 if (Configuration.errorOnAutoCreate()) 2860 throw new Error("Attempt to auto-create CarePlan.status"); 2861 else if (Configuration.doAutoCreate()) 2862 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); // bb 2863 return this.status; 2864 } 2865 2866 public boolean hasStatusElement() { 2867 return this.status != null && !this.status.isEmpty(); 2868 } 2869 2870 public boolean hasStatus() { 2871 return this.status != null && !this.status.isEmpty(); 2872 } 2873 2874 /** 2875 * @param value {@link #status} (Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2876 */ 2877 public CarePlan setStatusElement(Enumeration<CarePlanStatus> value) { 2878 this.status = value; 2879 return this; 2880 } 2881 2882 /** 2883 * @return Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record. 2884 */ 2885 public CarePlanStatus getStatus() { 2886 return this.status == null ? null : this.status.getValue(); 2887 } 2888 2889 /** 2890 * @param value Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record. 2891 */ 2892 public CarePlan setStatus(CarePlanStatus value) { 2893 if (this.status == null) 2894 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); 2895 this.status.setValue(value); 2896 return this; 2897 } 2898 2899 /** 2900 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2901 */ 2902 public Enumeration<CarePlanIntent> getIntentElement() { 2903 if (this.intent == null) 2904 if (Configuration.errorOnAutoCreate()) 2905 throw new Error("Attempt to auto-create CarePlan.intent"); 2906 else if (Configuration.doAutoCreate()) 2907 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); // bb 2908 return this.intent; 2909 } 2910 2911 public boolean hasIntentElement() { 2912 return this.intent != null && !this.intent.isEmpty(); 2913 } 2914 2915 public boolean hasIntent() { 2916 return this.intent != null && !this.intent.isEmpty(); 2917 } 2918 2919 /** 2920 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2921 */ 2922 public CarePlan setIntentElement(Enumeration<CarePlanIntent> value) { 2923 this.intent = value; 2924 return this; 2925 } 2926 2927 /** 2928 * @return Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain. 2929 */ 2930 public CarePlanIntent getIntent() { 2931 return this.intent == null ? null : this.intent.getValue(); 2932 } 2933 2934 /** 2935 * @param value Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain. 2936 */ 2937 public CarePlan setIntent(CarePlanIntent value) { 2938 if (this.intent == null) 2939 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); 2940 this.intent.setValue(value); 2941 return this; 2942 } 2943 2944 /** 2945 * @return {@link #category} (Identifies what "kind" of plan this is to support differentiation between multiple co-existing plans; e.g. "Home health", "psychiatric", "asthma", "disease management", "wellness plan", etc.) 2946 */ 2947 public List<CodeableConcept> getCategory() { 2948 if (this.category == null) 2949 this.category = new ArrayList<CodeableConcept>(); 2950 return this.category; 2951 } 2952 2953 /** 2954 * @return Returns a reference to <code>this</code> for easy method chaining 2955 */ 2956 public CarePlan setCategory(List<CodeableConcept> theCategory) { 2957 this.category = theCategory; 2958 return this; 2959 } 2960 2961 public boolean hasCategory() { 2962 if (this.category == null) 2963 return false; 2964 for (CodeableConcept item : this.category) 2965 if (!item.isEmpty()) 2966 return true; 2967 return false; 2968 } 2969 2970 public CodeableConcept addCategory() { //3 2971 CodeableConcept t = new CodeableConcept(); 2972 if (this.category == null) 2973 this.category = new ArrayList<CodeableConcept>(); 2974 this.category.add(t); 2975 return t; 2976 } 2977 2978 public CarePlan addCategory(CodeableConcept t) { //3 2979 if (t == null) 2980 return this; 2981 if (this.category == null) 2982 this.category = new ArrayList<CodeableConcept>(); 2983 this.category.add(t); 2984 return this; 2985 } 2986 2987 /** 2988 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 2989 */ 2990 public CodeableConcept getCategoryFirstRep() { 2991 if (getCategory().isEmpty()) { 2992 addCategory(); 2993 } 2994 return getCategory().get(0); 2995 } 2996 2997 /** 2998 * @return {@link #title} (Human-friendly name for the CarePlan.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2999 */ 3000 public StringType getTitleElement() { 3001 if (this.title == null) 3002 if (Configuration.errorOnAutoCreate()) 3003 throw new Error("Attempt to auto-create CarePlan.title"); 3004 else if (Configuration.doAutoCreate()) 3005 this.title = new StringType(); // bb 3006 return this.title; 3007 } 3008 3009 public boolean hasTitleElement() { 3010 return this.title != null && !this.title.isEmpty(); 3011 } 3012 3013 public boolean hasTitle() { 3014 return this.title != null && !this.title.isEmpty(); 3015 } 3016 3017 /** 3018 * @param value {@link #title} (Human-friendly name for the CarePlan.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3019 */ 3020 public CarePlan setTitleElement(StringType value) { 3021 this.title = value; 3022 return this; 3023 } 3024 3025 /** 3026 * @return Human-friendly name for the CarePlan. 3027 */ 3028 public String getTitle() { 3029 return this.title == null ? null : this.title.getValue(); 3030 } 3031 3032 /** 3033 * @param value Human-friendly name for the CarePlan. 3034 */ 3035 public CarePlan setTitle(String value) { 3036 if (Utilities.noString(value)) 3037 this.title = null; 3038 else { 3039 if (this.title == null) 3040 this.title = new StringType(); 3041 this.title.setValue(value); 3042 } 3043 return this; 3044 } 3045 3046 /** 3047 * @return {@link #description} (A description of the scope and nature of the plan.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3048 */ 3049 public StringType getDescriptionElement() { 3050 if (this.description == null) 3051 if (Configuration.errorOnAutoCreate()) 3052 throw new Error("Attempt to auto-create CarePlan.description"); 3053 else if (Configuration.doAutoCreate()) 3054 this.description = new StringType(); // bb 3055 return this.description; 3056 } 3057 3058 public boolean hasDescriptionElement() { 3059 return this.description != null && !this.description.isEmpty(); 3060 } 3061 3062 public boolean hasDescription() { 3063 return this.description != null && !this.description.isEmpty(); 3064 } 3065 3066 /** 3067 * @param value {@link #description} (A description of the scope and nature of the plan.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3068 */ 3069 public CarePlan setDescriptionElement(StringType value) { 3070 this.description = value; 3071 return this; 3072 } 3073 3074 /** 3075 * @return A description of the scope and nature of the plan. 3076 */ 3077 public String getDescription() { 3078 return this.description == null ? null : this.description.getValue(); 3079 } 3080 3081 /** 3082 * @param value A description of the scope and nature of the plan. 3083 */ 3084 public CarePlan setDescription(String value) { 3085 if (Utilities.noString(value)) 3086 this.description = null; 3087 else { 3088 if (this.description == null) 3089 this.description = new StringType(); 3090 this.description.setValue(value); 3091 } 3092 return this; 3093 } 3094 3095 /** 3096 * @return {@link #subject} (Identifies the patient or group whose intended care is described by the plan.) 3097 */ 3098 public Reference getSubject() { 3099 if (this.subject == null) 3100 if (Configuration.errorOnAutoCreate()) 3101 throw new Error("Attempt to auto-create CarePlan.subject"); 3102 else if (Configuration.doAutoCreate()) 3103 this.subject = new Reference(); // cc 3104 return this.subject; 3105 } 3106 3107 public boolean hasSubject() { 3108 return this.subject != null && !this.subject.isEmpty(); 3109 } 3110 3111 /** 3112 * @param value {@link #subject} (Identifies the patient or group whose intended care is described by the plan.) 3113 */ 3114 public CarePlan setSubject(Reference value) { 3115 this.subject = value; 3116 return this; 3117 } 3118 3119 /** 3120 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the patient or group whose intended care is described by the plan.) 3121 */ 3122 public Resource getSubjectTarget() { 3123 return this.subjectTarget; 3124 } 3125 3126 /** 3127 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the patient or group whose intended care is described by the plan.) 3128 */ 3129 public CarePlan setSubjectTarget(Resource value) { 3130 this.subjectTarget = value; 3131 return this; 3132 } 3133 3134 /** 3135 * @return {@link #context} (Identifies the original context in which this particular CarePlan was created.) 3136 */ 3137 public Reference getContext() { 3138 if (this.context == null) 3139 if (Configuration.errorOnAutoCreate()) 3140 throw new Error("Attempt to auto-create CarePlan.context"); 3141 else if (Configuration.doAutoCreate()) 3142 this.context = new Reference(); // cc 3143 return this.context; 3144 } 3145 3146 public boolean hasContext() { 3147 return this.context != null && !this.context.isEmpty(); 3148 } 3149 3150 /** 3151 * @param value {@link #context} (Identifies the original context in which this particular CarePlan was created.) 3152 */ 3153 public CarePlan setContext(Reference value) { 3154 this.context = value; 3155 return this; 3156 } 3157 3158 /** 3159 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the original context in which this particular CarePlan was created.) 3160 */ 3161 public Resource getContextTarget() { 3162 return this.contextTarget; 3163 } 3164 3165 /** 3166 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the original context in which this particular CarePlan was created.) 3167 */ 3168 public CarePlan setContextTarget(Resource value) { 3169 this.contextTarget = value; 3170 return this; 3171 } 3172 3173 /** 3174 * @return {@link #period} (Indicates when the plan did (or is intended to) come into effect and end.) 3175 */ 3176 public Period getPeriod() { 3177 if (this.period == null) 3178 if (Configuration.errorOnAutoCreate()) 3179 throw new Error("Attempt to auto-create CarePlan.period"); 3180 else if (Configuration.doAutoCreate()) 3181 this.period = new Period(); // cc 3182 return this.period; 3183 } 3184 3185 public boolean hasPeriod() { 3186 return this.period != null && !this.period.isEmpty(); 3187 } 3188 3189 /** 3190 * @param value {@link #period} (Indicates when the plan did (or is intended to) come into effect and end.) 3191 */ 3192 public CarePlan setPeriod(Period value) { 3193 this.period = value; 3194 return this; 3195 } 3196 3197 /** 3198 * @return {@link #author} (Identifies the individual(s) or ogranization who is responsible for the content of the care plan.) 3199 */ 3200 public List<Reference> getAuthor() { 3201 if (this.author == null) 3202 this.author = new ArrayList<Reference>(); 3203 return this.author; 3204 } 3205 3206 /** 3207 * @return Returns a reference to <code>this</code> for easy method chaining 3208 */ 3209 public CarePlan setAuthor(List<Reference> theAuthor) { 3210 this.author = theAuthor; 3211 return this; 3212 } 3213 3214 public boolean hasAuthor() { 3215 if (this.author == null) 3216 return false; 3217 for (Reference item : this.author) 3218 if (!item.isEmpty()) 3219 return true; 3220 return false; 3221 } 3222 3223 public Reference addAuthor() { //3 3224 Reference t = new Reference(); 3225 if (this.author == null) 3226 this.author = new ArrayList<Reference>(); 3227 this.author.add(t); 3228 return t; 3229 } 3230 3231 public CarePlan addAuthor(Reference t) { //3 3232 if (t == null) 3233 return this; 3234 if (this.author == null) 3235 this.author = new ArrayList<Reference>(); 3236 this.author.add(t); 3237 return this; 3238 } 3239 3240 /** 3241 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist 3242 */ 3243 public Reference getAuthorFirstRep() { 3244 if (getAuthor().isEmpty()) { 3245 addAuthor(); 3246 } 3247 return getAuthor().get(0); 3248 } 3249 3250 /** 3251 * @deprecated Use Reference#setResource(IBaseResource) instead 3252 */ 3253 @Deprecated 3254 public List<Resource> getAuthorTarget() { 3255 if (this.authorTarget == null) 3256 this.authorTarget = new ArrayList<Resource>(); 3257 return this.authorTarget; 3258 } 3259 3260 /** 3261 * @return {@link #careTeam} (Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.) 3262 */ 3263 public List<Reference> getCareTeam() { 3264 if (this.careTeam == null) 3265 this.careTeam = new ArrayList<Reference>(); 3266 return this.careTeam; 3267 } 3268 3269 /** 3270 * @return Returns a reference to <code>this</code> for easy method chaining 3271 */ 3272 public CarePlan setCareTeam(List<Reference> theCareTeam) { 3273 this.careTeam = theCareTeam; 3274 return this; 3275 } 3276 3277 public boolean hasCareTeam() { 3278 if (this.careTeam == null) 3279 return false; 3280 for (Reference item : this.careTeam) 3281 if (!item.isEmpty()) 3282 return true; 3283 return false; 3284 } 3285 3286 public Reference addCareTeam() { //3 3287 Reference t = new Reference(); 3288 if (this.careTeam == null) 3289 this.careTeam = new ArrayList<Reference>(); 3290 this.careTeam.add(t); 3291 return t; 3292 } 3293 3294 public CarePlan addCareTeam(Reference t) { //3 3295 if (t == null) 3296 return this; 3297 if (this.careTeam == null) 3298 this.careTeam = new ArrayList<Reference>(); 3299 this.careTeam.add(t); 3300 return this; 3301 } 3302 3303 /** 3304 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist 3305 */ 3306 public Reference getCareTeamFirstRep() { 3307 if (getCareTeam().isEmpty()) { 3308 addCareTeam(); 3309 } 3310 return getCareTeam().get(0); 3311 } 3312 3313 /** 3314 * @deprecated Use Reference#setResource(IBaseResource) instead 3315 */ 3316 @Deprecated 3317 public List<CareTeam> getCareTeamTarget() { 3318 if (this.careTeamTarget == null) 3319 this.careTeamTarget = new ArrayList<CareTeam>(); 3320 return this.careTeamTarget; 3321 } 3322 3323 /** 3324 * @deprecated Use Reference#setResource(IBaseResource) instead 3325 */ 3326 @Deprecated 3327 public CareTeam addCareTeamTarget() { 3328 CareTeam r = new CareTeam(); 3329 if (this.careTeamTarget == null) 3330 this.careTeamTarget = new ArrayList<CareTeam>(); 3331 this.careTeamTarget.add(r); 3332 return r; 3333 } 3334 3335 /** 3336 * @return {@link #addresses} (Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.) 3337 */ 3338 public List<Reference> getAddresses() { 3339 if (this.addresses == null) 3340 this.addresses = new ArrayList<Reference>(); 3341 return this.addresses; 3342 } 3343 3344 /** 3345 * @return Returns a reference to <code>this</code> for easy method chaining 3346 */ 3347 public CarePlan setAddresses(List<Reference> theAddresses) { 3348 this.addresses = theAddresses; 3349 return this; 3350 } 3351 3352 public boolean hasAddresses() { 3353 if (this.addresses == null) 3354 return false; 3355 for (Reference item : this.addresses) 3356 if (!item.isEmpty()) 3357 return true; 3358 return false; 3359 } 3360 3361 public Reference addAddresses() { //3 3362 Reference t = new Reference(); 3363 if (this.addresses == null) 3364 this.addresses = new ArrayList<Reference>(); 3365 this.addresses.add(t); 3366 return t; 3367 } 3368 3369 public CarePlan addAddresses(Reference t) { //3 3370 if (t == null) 3371 return this; 3372 if (this.addresses == null) 3373 this.addresses = new ArrayList<Reference>(); 3374 this.addresses.add(t); 3375 return this; 3376 } 3377 3378 /** 3379 * @return The first repetition of repeating field {@link #addresses}, creating it if it does not already exist 3380 */ 3381 public Reference getAddressesFirstRep() { 3382 if (getAddresses().isEmpty()) { 3383 addAddresses(); 3384 } 3385 return getAddresses().get(0); 3386 } 3387 3388 /** 3389 * @deprecated Use Reference#setResource(IBaseResource) instead 3390 */ 3391 @Deprecated 3392 public List<Condition> getAddressesTarget() { 3393 if (this.addressesTarget == null) 3394 this.addressesTarget = new ArrayList<Condition>(); 3395 return this.addressesTarget; 3396 } 3397 3398 /** 3399 * @deprecated Use Reference#setResource(IBaseResource) instead 3400 */ 3401 @Deprecated 3402 public Condition addAddressesTarget() { 3403 Condition r = new Condition(); 3404 if (this.addressesTarget == null) 3405 this.addressesTarget = new ArrayList<Condition>(); 3406 this.addressesTarget.add(r); 3407 return r; 3408 } 3409 3410 /** 3411 * @return {@link #supportingInfo} (Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc.) 3412 */ 3413 public List<Reference> getSupportingInfo() { 3414 if (this.supportingInfo == null) 3415 this.supportingInfo = new ArrayList<Reference>(); 3416 return this.supportingInfo; 3417 } 3418 3419 /** 3420 * @return Returns a reference to <code>this</code> for easy method chaining 3421 */ 3422 public CarePlan setSupportingInfo(List<Reference> theSupportingInfo) { 3423 this.supportingInfo = theSupportingInfo; 3424 return this; 3425 } 3426 3427 public boolean hasSupportingInfo() { 3428 if (this.supportingInfo == null) 3429 return false; 3430 for (Reference item : this.supportingInfo) 3431 if (!item.isEmpty()) 3432 return true; 3433 return false; 3434 } 3435 3436 public Reference addSupportingInfo() { //3 3437 Reference t = new Reference(); 3438 if (this.supportingInfo == null) 3439 this.supportingInfo = new ArrayList<Reference>(); 3440 this.supportingInfo.add(t); 3441 return t; 3442 } 3443 3444 public CarePlan addSupportingInfo(Reference t) { //3 3445 if (t == null) 3446 return this; 3447 if (this.supportingInfo == null) 3448 this.supportingInfo = new ArrayList<Reference>(); 3449 this.supportingInfo.add(t); 3450 return this; 3451 } 3452 3453 /** 3454 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 3455 */ 3456 public Reference getSupportingInfoFirstRep() { 3457 if (getSupportingInfo().isEmpty()) { 3458 addSupportingInfo(); 3459 } 3460 return getSupportingInfo().get(0); 3461 } 3462 3463 /** 3464 * @deprecated Use Reference#setResource(IBaseResource) instead 3465 */ 3466 @Deprecated 3467 public List<Resource> getSupportingInfoTarget() { 3468 if (this.supportingInfoTarget == null) 3469 this.supportingInfoTarget = new ArrayList<Resource>(); 3470 return this.supportingInfoTarget; 3471 } 3472 3473 /** 3474 * @return {@link #goal} (Describes the intended objective(s) of carrying out the care plan.) 3475 */ 3476 public List<Reference> getGoal() { 3477 if (this.goal == null) 3478 this.goal = new ArrayList<Reference>(); 3479 return this.goal; 3480 } 3481 3482 /** 3483 * @return Returns a reference to <code>this</code> for easy method chaining 3484 */ 3485 public CarePlan setGoal(List<Reference> theGoal) { 3486 this.goal = theGoal; 3487 return this; 3488 } 3489 3490 public boolean hasGoal() { 3491 if (this.goal == null) 3492 return false; 3493 for (Reference item : this.goal) 3494 if (!item.isEmpty()) 3495 return true; 3496 return false; 3497 } 3498 3499 public Reference addGoal() { //3 3500 Reference t = new Reference(); 3501 if (this.goal == null) 3502 this.goal = new ArrayList<Reference>(); 3503 this.goal.add(t); 3504 return t; 3505 } 3506 3507 public CarePlan addGoal(Reference t) { //3 3508 if (t == null) 3509 return this; 3510 if (this.goal == null) 3511 this.goal = new ArrayList<Reference>(); 3512 this.goal.add(t); 3513 return this; 3514 } 3515 3516 /** 3517 * @return The first repetition of repeating field {@link #goal}, creating it if it does not already exist 3518 */ 3519 public Reference getGoalFirstRep() { 3520 if (getGoal().isEmpty()) { 3521 addGoal(); 3522 } 3523 return getGoal().get(0); 3524 } 3525 3526 /** 3527 * @deprecated Use Reference#setResource(IBaseResource) instead 3528 */ 3529 @Deprecated 3530 public List<Goal> getGoalTarget() { 3531 if (this.goalTarget == null) 3532 this.goalTarget = new ArrayList<Goal>(); 3533 return this.goalTarget; 3534 } 3535 3536 /** 3537 * @deprecated Use Reference#setResource(IBaseResource) instead 3538 */ 3539 @Deprecated 3540 public Goal addGoalTarget() { 3541 Goal r = new Goal(); 3542 if (this.goalTarget == null) 3543 this.goalTarget = new ArrayList<Goal>(); 3544 this.goalTarget.add(r); 3545 return r; 3546 } 3547 3548 /** 3549 * @return {@link #activity} (Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.) 3550 */ 3551 public List<CarePlanActivityComponent> getActivity() { 3552 if (this.activity == null) 3553 this.activity = new ArrayList<CarePlanActivityComponent>(); 3554 return this.activity; 3555 } 3556 3557 /** 3558 * @return Returns a reference to <code>this</code> for easy method chaining 3559 */ 3560 public CarePlan setActivity(List<CarePlanActivityComponent> theActivity) { 3561 this.activity = theActivity; 3562 return this; 3563 } 3564 3565 public boolean hasActivity() { 3566 if (this.activity == null) 3567 return false; 3568 for (CarePlanActivityComponent item : this.activity) 3569 if (!item.isEmpty()) 3570 return true; 3571 return false; 3572 } 3573 3574 public CarePlanActivityComponent addActivity() { //3 3575 CarePlanActivityComponent t = new CarePlanActivityComponent(); 3576 if (this.activity == null) 3577 this.activity = new ArrayList<CarePlanActivityComponent>(); 3578 this.activity.add(t); 3579 return t; 3580 } 3581 3582 public CarePlan addActivity(CarePlanActivityComponent t) { //3 3583 if (t == null) 3584 return this; 3585 if (this.activity == null) 3586 this.activity = new ArrayList<CarePlanActivityComponent>(); 3587 this.activity.add(t); 3588 return this; 3589 } 3590 3591 /** 3592 * @return The first repetition of repeating field {@link #activity}, creating it if it does not already exist 3593 */ 3594 public CarePlanActivityComponent getActivityFirstRep() { 3595 if (getActivity().isEmpty()) { 3596 addActivity(); 3597 } 3598 return getActivity().get(0); 3599 } 3600 3601 /** 3602 * @return {@link #note} (General notes about the care plan not covered elsewhere.) 3603 */ 3604 public List<Annotation> getNote() { 3605 if (this.note == null) 3606 this.note = new ArrayList<Annotation>(); 3607 return this.note; 3608 } 3609 3610 /** 3611 * @return Returns a reference to <code>this</code> for easy method chaining 3612 */ 3613 public CarePlan setNote(List<Annotation> theNote) { 3614 this.note = theNote; 3615 return this; 3616 } 3617 3618 public boolean hasNote() { 3619 if (this.note == null) 3620 return false; 3621 for (Annotation item : this.note) 3622 if (!item.isEmpty()) 3623 return true; 3624 return false; 3625 } 3626 3627 public Annotation addNote() { //3 3628 Annotation t = new Annotation(); 3629 if (this.note == null) 3630 this.note = new ArrayList<Annotation>(); 3631 this.note.add(t); 3632 return t; 3633 } 3634 3635 public CarePlan addNote(Annotation t) { //3 3636 if (t == null) 3637 return this; 3638 if (this.note == null) 3639 this.note = new ArrayList<Annotation>(); 3640 this.note.add(t); 3641 return this; 3642 } 3643 3644 /** 3645 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 3646 */ 3647 public Annotation getNoteFirstRep() { 3648 if (getNote().isEmpty()) { 3649 addNote(); 3650 } 3651 return getNote().get(0); 3652 } 3653 3654 protected void listChildren(List<Property> children) { 3655 super.listChildren(children); 3656 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 3657 children.add(new Property("definition", "Reference(PlanDefinition|Questionnaire)", "Identifies the protocol, questionnaire, guideline or other specification the care plan should be conducted in accordance with.", 0, java.lang.Integer.MAX_VALUE, definition)); 3658 children.add(new Property("basedOn", "Reference(CarePlan)", "A care plan that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3659 children.add(new Property("replaces", "Reference(CarePlan)", "Completed or terminated care plan whose function is taken by this new care plan.", 0, java.lang.Integer.MAX_VALUE, replaces)); 3660 children.add(new Property("partOf", "Reference(CarePlan)", "A larger care plan of which this particular care plan is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 3661 children.add(new Property("status", "code", "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 0, 1, status)); 3662 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 0, 1, intent)); 3663 children.add(new Property("category", "CodeableConcept", "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 0, java.lang.Integer.MAX_VALUE, category)); 3664 children.add(new Property("title", "string", "Human-friendly name for the CarePlan.", 0, 1, title)); 3665 children.add(new Property("description", "string", "A description of the scope and nature of the plan.", 0, 1, description)); 3666 children.add(new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject)); 3667 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "Identifies the original context in which this particular CarePlan was created.", 0, 1, context)); 3668 children.add(new Property("period", "Period", "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period)); 3669 children.add(new Property("author", "Reference(Patient|Practitioner|RelatedPerson|Organization|CareTeam)", "Identifies the individual(s) or ogranization who is responsible for the content of the care plan.", 0, java.lang.Integer.MAX_VALUE, author)); 3670 children.add(new Property("careTeam", "Reference(CareTeam)", "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 3671 children.add(new Property("addresses", "Reference(Condition)", "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 0, java.lang.Integer.MAX_VALUE, addresses)); 3672 children.add(new Property("supportingInfo", "Reference(Any)", "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 3673 children.add(new Property("goal", "Reference(Goal)", "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal)); 3674 children.add(new Property("activity", "", "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.", 0, java.lang.Integer.MAX_VALUE, activity)); 3675 children.add(new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 0, java.lang.Integer.MAX_VALUE, note)); 3676 } 3677 3678 @Override 3679 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3680 switch (_hash) { 3681 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 3682 case -1014418093: /*definition*/ return new Property("definition", "Reference(PlanDefinition|Questionnaire)", "Identifies the protocol, questionnaire, guideline or other specification the care plan should be conducted in accordance with.", 0, java.lang.Integer.MAX_VALUE, definition); 3683 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan)", "A care plan that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3684 case -430332865: /*replaces*/ return new Property("replaces", "Reference(CarePlan)", "Completed or terminated care plan whose function is taken by this new care plan.", 0, java.lang.Integer.MAX_VALUE, replaces); 3685 case -995410646: /*partOf*/ return new Property("partOf", "Reference(CarePlan)", "A larger care plan of which this particular care plan is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 3686 case -892481550: /*status*/ return new Property("status", "code", "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 0, 1, status); 3687 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 0, 1, intent); 3688 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 0, java.lang.Integer.MAX_VALUE, category); 3689 case 110371416: /*title*/ return new Property("title", "string", "Human-friendly name for the CarePlan.", 0, 1, title); 3690 case -1724546052: /*description*/ return new Property("description", "string", "A description of the scope and nature of the plan.", 0, 1, description); 3691 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject); 3692 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "Identifies the original context in which this particular CarePlan was created.", 0, 1, context); 3693 case -991726143: /*period*/ return new Property("period", "Period", "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period); 3694 case -1406328437: /*author*/ return new Property("author", "Reference(Patient|Practitioner|RelatedPerson|Organization|CareTeam)", "Identifies the individual(s) or ogranization who is responsible for the content of the care plan.", 0, java.lang.Integer.MAX_VALUE, author); 3695 case -7323378: /*careTeam*/ return new Property("careTeam", "Reference(CareTeam)", "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 0, java.lang.Integer.MAX_VALUE, careTeam); 3696 case 874544034: /*addresses*/ return new Property("addresses", "Reference(Condition)", "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 0, java.lang.Integer.MAX_VALUE, addresses); 3697 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 3698 case 3178259: /*goal*/ return new Property("goal", "Reference(Goal)", "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal); 3699 case -1655966961: /*activity*/ return new Property("activity", "", "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.", 0, java.lang.Integer.MAX_VALUE, activity); 3700 case 3387378: /*note*/ return new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 0, java.lang.Integer.MAX_VALUE, note); 3701 default: return super.getNamedProperty(_hash, _name, _checkValid); 3702 } 3703 3704 } 3705 3706 @Override 3707 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3708 switch (hash) { 3709 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3710 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 3711 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3712 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 3713 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 3714 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CarePlanStatus> 3715 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<CarePlanIntent> 3716 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3717 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3718 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3719 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3720 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 3721 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3722 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 3723 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // Reference 3724 case 874544034: /*addresses*/ return this.addresses == null ? new Base[0] : this.addresses.toArray(new Base[this.addresses.size()]); // Reference 3725 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 3726 case 3178259: /*goal*/ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 3727 case -1655966961: /*activity*/ return this.activity == null ? new Base[0] : this.activity.toArray(new Base[this.activity.size()]); // CarePlanActivityComponent 3728 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3729 default: return super.getProperty(hash, name, checkValid); 3730 } 3731 3732 } 3733 3734 @Override 3735 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3736 switch (hash) { 3737 case -1618432855: // identifier 3738 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3739 return value; 3740 case -1014418093: // definition 3741 this.getDefinition().add(castToReference(value)); // Reference 3742 return value; 3743 case -332612366: // basedOn 3744 this.getBasedOn().add(castToReference(value)); // Reference 3745 return value; 3746 case -430332865: // replaces 3747 this.getReplaces().add(castToReference(value)); // Reference 3748 return value; 3749 case -995410646: // partOf 3750 this.getPartOf().add(castToReference(value)); // Reference 3751 return value; 3752 case -892481550: // status 3753 value = new CarePlanStatusEnumFactory().fromType(castToCode(value)); 3754 this.status = (Enumeration) value; // Enumeration<CarePlanStatus> 3755 return value; 3756 case -1183762788: // intent 3757 value = new CarePlanIntentEnumFactory().fromType(castToCode(value)); 3758 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 3759 return value; 3760 case 50511102: // category 3761 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3762 return value; 3763 case 110371416: // title 3764 this.title = castToString(value); // StringType 3765 return value; 3766 case -1724546052: // description 3767 this.description = castToString(value); // StringType 3768 return value; 3769 case -1867885268: // subject 3770 this.subject = castToReference(value); // Reference 3771 return value; 3772 case 951530927: // context 3773 this.context = castToReference(value); // Reference 3774 return value; 3775 case -991726143: // period 3776 this.period = castToPeriod(value); // Period 3777 return value; 3778 case -1406328437: // author 3779 this.getAuthor().add(castToReference(value)); // Reference 3780 return value; 3781 case -7323378: // careTeam 3782 this.getCareTeam().add(castToReference(value)); // Reference 3783 return value; 3784 case 874544034: // addresses 3785 this.getAddresses().add(castToReference(value)); // Reference 3786 return value; 3787 case 1922406657: // supportingInfo 3788 this.getSupportingInfo().add(castToReference(value)); // Reference 3789 return value; 3790 case 3178259: // goal 3791 this.getGoal().add(castToReference(value)); // Reference 3792 return value; 3793 case -1655966961: // activity 3794 this.getActivity().add((CarePlanActivityComponent) value); // CarePlanActivityComponent 3795 return value; 3796 case 3387378: // note 3797 this.getNote().add(castToAnnotation(value)); // Annotation 3798 return value; 3799 default: return super.setProperty(hash, name, value); 3800 } 3801 3802 } 3803 3804 @Override 3805 public Base setProperty(String name, Base value) throws FHIRException { 3806 if (name.equals("identifier")) { 3807 this.getIdentifier().add(castToIdentifier(value)); 3808 } else if (name.equals("definition")) { 3809 this.getDefinition().add(castToReference(value)); 3810 } else if (name.equals("basedOn")) { 3811 this.getBasedOn().add(castToReference(value)); 3812 } else if (name.equals("replaces")) { 3813 this.getReplaces().add(castToReference(value)); 3814 } else if (name.equals("partOf")) { 3815 this.getPartOf().add(castToReference(value)); 3816 } else if (name.equals("status")) { 3817 value = new CarePlanStatusEnumFactory().fromType(castToCode(value)); 3818 this.status = (Enumeration) value; // Enumeration<CarePlanStatus> 3819 } else if (name.equals("intent")) { 3820 value = new CarePlanIntentEnumFactory().fromType(castToCode(value)); 3821 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 3822 } else if (name.equals("category")) { 3823 this.getCategory().add(castToCodeableConcept(value)); 3824 } else if (name.equals("title")) { 3825 this.title = castToString(value); // StringType 3826 } else if (name.equals("description")) { 3827 this.description = castToString(value); // StringType 3828 } else if (name.equals("subject")) { 3829 this.subject = castToReference(value); // Reference 3830 } else if (name.equals("context")) { 3831 this.context = castToReference(value); // Reference 3832 } else if (name.equals("period")) { 3833 this.period = castToPeriod(value); // Period 3834 } else if (name.equals("author")) { 3835 this.getAuthor().add(castToReference(value)); 3836 } else if (name.equals("careTeam")) { 3837 this.getCareTeam().add(castToReference(value)); 3838 } else if (name.equals("addresses")) { 3839 this.getAddresses().add(castToReference(value)); 3840 } else if (name.equals("supportingInfo")) { 3841 this.getSupportingInfo().add(castToReference(value)); 3842 } else if (name.equals("goal")) { 3843 this.getGoal().add(castToReference(value)); 3844 } else if (name.equals("activity")) { 3845 this.getActivity().add((CarePlanActivityComponent) value); 3846 } else if (name.equals("note")) { 3847 this.getNote().add(castToAnnotation(value)); 3848 } else 3849 return super.setProperty(name, value); 3850 return value; 3851 } 3852 3853 @Override 3854 public Base makeProperty(int hash, String name) throws FHIRException { 3855 switch (hash) { 3856 case -1618432855: return addIdentifier(); 3857 case -1014418093: return addDefinition(); 3858 case -332612366: return addBasedOn(); 3859 case -430332865: return addReplaces(); 3860 case -995410646: return addPartOf(); 3861 case -892481550: return getStatusElement(); 3862 case -1183762788: return getIntentElement(); 3863 case 50511102: return addCategory(); 3864 case 110371416: return getTitleElement(); 3865 case -1724546052: return getDescriptionElement(); 3866 case -1867885268: return getSubject(); 3867 case 951530927: return getContext(); 3868 case -991726143: return getPeriod(); 3869 case -1406328437: return addAuthor(); 3870 case -7323378: return addCareTeam(); 3871 case 874544034: return addAddresses(); 3872 case 1922406657: return addSupportingInfo(); 3873 case 3178259: return addGoal(); 3874 case -1655966961: return addActivity(); 3875 case 3387378: return addNote(); 3876 default: return super.makeProperty(hash, name); 3877 } 3878 3879 } 3880 3881 @Override 3882 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3883 switch (hash) { 3884 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3885 case -1014418093: /*definition*/ return new String[] {"Reference"}; 3886 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3887 case -430332865: /*replaces*/ return new String[] {"Reference"}; 3888 case -995410646: /*partOf*/ return new String[] {"Reference"}; 3889 case -892481550: /*status*/ return new String[] {"code"}; 3890 case -1183762788: /*intent*/ return new String[] {"code"}; 3891 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3892 case 110371416: /*title*/ return new String[] {"string"}; 3893 case -1724546052: /*description*/ return new String[] {"string"}; 3894 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3895 case 951530927: /*context*/ return new String[] {"Reference"}; 3896 case -991726143: /*period*/ return new String[] {"Period"}; 3897 case -1406328437: /*author*/ return new String[] {"Reference"}; 3898 case -7323378: /*careTeam*/ return new String[] {"Reference"}; 3899 case 874544034: /*addresses*/ return new String[] {"Reference"}; 3900 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 3901 case 3178259: /*goal*/ return new String[] {"Reference"}; 3902 case -1655966961: /*activity*/ return new String[] {}; 3903 case 3387378: /*note*/ return new String[] {"Annotation"}; 3904 default: return super.getTypesForProperty(hash, name); 3905 } 3906 3907 } 3908 3909 @Override 3910 public Base addChild(String name) throws FHIRException { 3911 if (name.equals("identifier")) { 3912 return addIdentifier(); 3913 } 3914 else if (name.equals("definition")) { 3915 return addDefinition(); 3916 } 3917 else if (name.equals("basedOn")) { 3918 return addBasedOn(); 3919 } 3920 else if (name.equals("replaces")) { 3921 return addReplaces(); 3922 } 3923 else if (name.equals("partOf")) { 3924 return addPartOf(); 3925 } 3926 else if (name.equals("status")) { 3927 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 3928 } 3929 else if (name.equals("intent")) { 3930 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.intent"); 3931 } 3932 else if (name.equals("category")) { 3933 return addCategory(); 3934 } 3935 else if (name.equals("title")) { 3936 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.title"); 3937 } 3938 else if (name.equals("description")) { 3939 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 3940 } 3941 else if (name.equals("subject")) { 3942 this.subject = new Reference(); 3943 return this.subject; 3944 } 3945 else if (name.equals("context")) { 3946 this.context = new Reference(); 3947 return this.context; 3948 } 3949 else if (name.equals("period")) { 3950 this.period = new Period(); 3951 return this.period; 3952 } 3953 else if (name.equals("author")) { 3954 return addAuthor(); 3955 } 3956 else if (name.equals("careTeam")) { 3957 return addCareTeam(); 3958 } 3959 else if (name.equals("addresses")) { 3960 return addAddresses(); 3961 } 3962 else if (name.equals("supportingInfo")) { 3963 return addSupportingInfo(); 3964 } 3965 else if (name.equals("goal")) { 3966 return addGoal(); 3967 } 3968 else if (name.equals("activity")) { 3969 return addActivity(); 3970 } 3971 else if (name.equals("note")) { 3972 return addNote(); 3973 } 3974 else 3975 return super.addChild(name); 3976 } 3977 3978 public String fhirType() { 3979 return "CarePlan"; 3980 3981 } 3982 3983 public CarePlan copy() { 3984 CarePlan dst = new CarePlan(); 3985 copyValues(dst); 3986 if (identifier != null) { 3987 dst.identifier = new ArrayList<Identifier>(); 3988 for (Identifier i : identifier) 3989 dst.identifier.add(i.copy()); 3990 }; 3991 if (definition != null) { 3992 dst.definition = new ArrayList<Reference>(); 3993 for (Reference i : definition) 3994 dst.definition.add(i.copy()); 3995 }; 3996 if (basedOn != null) { 3997 dst.basedOn = new ArrayList<Reference>(); 3998 for (Reference i : basedOn) 3999 dst.basedOn.add(i.copy()); 4000 }; 4001 if (replaces != null) { 4002 dst.replaces = new ArrayList<Reference>(); 4003 for (Reference i : replaces) 4004 dst.replaces.add(i.copy()); 4005 }; 4006 if (partOf != null) { 4007 dst.partOf = new ArrayList<Reference>(); 4008 for (Reference i : partOf) 4009 dst.partOf.add(i.copy()); 4010 }; 4011 dst.status = status == null ? null : status.copy(); 4012 dst.intent = intent == null ? null : intent.copy(); 4013 if (category != null) { 4014 dst.category = new ArrayList<CodeableConcept>(); 4015 for (CodeableConcept i : category) 4016 dst.category.add(i.copy()); 4017 }; 4018 dst.title = title == null ? null : title.copy(); 4019 dst.description = description == null ? null : description.copy(); 4020 dst.subject = subject == null ? null : subject.copy(); 4021 dst.context = context == null ? null : context.copy(); 4022 dst.period = period == null ? null : period.copy(); 4023 if (author != null) { 4024 dst.author = new ArrayList<Reference>(); 4025 for (Reference i : author) 4026 dst.author.add(i.copy()); 4027 }; 4028 if (careTeam != null) { 4029 dst.careTeam = new ArrayList<Reference>(); 4030 for (Reference i : careTeam) 4031 dst.careTeam.add(i.copy()); 4032 }; 4033 if (addresses != null) { 4034 dst.addresses = new ArrayList<Reference>(); 4035 for (Reference i : addresses) 4036 dst.addresses.add(i.copy()); 4037 }; 4038 if (supportingInfo != null) { 4039 dst.supportingInfo = new ArrayList<Reference>(); 4040 for (Reference i : supportingInfo) 4041 dst.supportingInfo.add(i.copy()); 4042 }; 4043 if (goal != null) { 4044 dst.goal = new ArrayList<Reference>(); 4045 for (Reference i : goal) 4046 dst.goal.add(i.copy()); 4047 }; 4048 if (activity != null) { 4049 dst.activity = new ArrayList<CarePlanActivityComponent>(); 4050 for (CarePlanActivityComponent i : activity) 4051 dst.activity.add(i.copy()); 4052 }; 4053 if (note != null) { 4054 dst.note = new ArrayList<Annotation>(); 4055 for (Annotation i : note) 4056 dst.note.add(i.copy()); 4057 }; 4058 return dst; 4059 } 4060 4061 protected CarePlan typedCopy() { 4062 return copy(); 4063 } 4064 4065 @Override 4066 public boolean equalsDeep(Base other_) { 4067 if (!super.equalsDeep(other_)) 4068 return false; 4069 if (!(other_ instanceof CarePlan)) 4070 return false; 4071 CarePlan o = (CarePlan) other_; 4072 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 4073 && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) && compareDeep(partOf, o.partOf, true) 4074 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(category, o.category, true) 4075 && compareDeep(title, o.title, true) && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) 4076 && compareDeep(context, o.context, true) && compareDeep(period, o.period, true) && compareDeep(author, o.author, true) 4077 && compareDeep(careTeam, o.careTeam, true) && compareDeep(addresses, o.addresses, true) && compareDeep(supportingInfo, o.supportingInfo, true) 4078 && compareDeep(goal, o.goal, true) && compareDeep(activity, o.activity, true) && compareDeep(note, o.note, true) 4079 ; 4080 } 4081 4082 @Override 4083 public boolean equalsShallow(Base other_) { 4084 if (!super.equalsShallow(other_)) 4085 return false; 4086 if (!(other_ instanceof CarePlan)) 4087 return false; 4088 CarePlan o = (CarePlan) other_; 4089 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(title, o.title, true) 4090 && compareValues(description, o.description, true); 4091 } 4092 4093 public boolean isEmpty() { 4094 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 4095 , replaces, partOf, status, intent, category, title, description, subject, context 4096 , period, author, careTeam, addresses, supportingInfo, goal, activity, note 4097 ); 4098 } 4099 4100 @Override 4101 public ResourceType getResourceType() { 4102 return ResourceType.CarePlan; 4103 } 4104 4105 /** 4106 * Search parameter: <b>date</b> 4107 * <p> 4108 * Description: <b>Time period plan covers</b><br> 4109 * Type: <b>date</b><br> 4110 * Path: <b>CarePlan.period</b><br> 4111 * </p> 4112 */ 4113 @SearchParamDefinition(name="date", path="CarePlan.period", description="Time period plan covers", type="date" ) 4114 public static final String SP_DATE = "date"; 4115 /** 4116 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4117 * <p> 4118 * Description: <b>Time period plan covers</b><br> 4119 * Type: <b>date</b><br> 4120 * Path: <b>CarePlan.period</b><br> 4121 * </p> 4122 */ 4123 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4124 4125 /** 4126 * Search parameter: <b>care-team</b> 4127 * <p> 4128 * Description: <b>Who's involved in plan?</b><br> 4129 * Type: <b>reference</b><br> 4130 * Path: <b>CarePlan.careTeam</b><br> 4131 * </p> 4132 */ 4133 @SearchParamDefinition(name="care-team", path="CarePlan.careTeam", description="Who's involved in plan?", type="reference", target={CareTeam.class } ) 4134 public static final String SP_CARE_TEAM = "care-team"; 4135 /** 4136 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 4137 * <p> 4138 * Description: <b>Who's involved in plan?</b><br> 4139 * Type: <b>reference</b><br> 4140 * Path: <b>CarePlan.careTeam</b><br> 4141 * </p> 4142 */ 4143 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 4144 4145/** 4146 * Constant for fluent queries to be used to add include statements. Specifies 4147 * the path value of "<b>CarePlan:care-team</b>". 4148 */ 4149 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("CarePlan:care-team").toLocked(); 4150 4151 /** 4152 * Search parameter: <b>identifier</b> 4153 * <p> 4154 * Description: <b>External Ids for this plan</b><br> 4155 * Type: <b>token</b><br> 4156 * Path: <b>CarePlan.identifier</b><br> 4157 * </p> 4158 */ 4159 @SearchParamDefinition(name="identifier", path="CarePlan.identifier", description="External Ids for this plan", type="token" ) 4160 public static final String SP_IDENTIFIER = "identifier"; 4161 /** 4162 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4163 * <p> 4164 * Description: <b>External Ids for this plan</b><br> 4165 * Type: <b>token</b><br> 4166 * Path: <b>CarePlan.identifier</b><br> 4167 * </p> 4168 */ 4169 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4170 4171 /** 4172 * Search parameter: <b>performer</b> 4173 * <p> 4174 * Description: <b>Matches if the practitioner is listed as a performer in any of the "simple" activities. (For performers of the detailed activities, chain through the activitydetail search parameter.)</b><br> 4175 * Type: <b>reference</b><br> 4176 * Path: <b>CarePlan.activity.detail.performer</b><br> 4177 * </p> 4178 */ 4179 @SearchParamDefinition(name="performer", path="CarePlan.activity.detail.performer", description="Matches if the practitioner is listed as a performer in any of the \"simple\" activities. (For performers of the detailed activities, chain through the activitydetail search parameter.)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 4180 public static final String SP_PERFORMER = "performer"; 4181 /** 4182 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4183 * <p> 4184 * Description: <b>Matches if the practitioner is listed as a performer in any of the "simple" activities. (For performers of the detailed activities, chain through the activitydetail search parameter.)</b><br> 4185 * Type: <b>reference</b><br> 4186 * Path: <b>CarePlan.activity.detail.performer</b><br> 4187 * </p> 4188 */ 4189 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 4190 4191/** 4192 * Constant for fluent queries to be used to add include statements. Specifies 4193 * the path value of "<b>CarePlan:performer</b>". 4194 */ 4195 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("CarePlan:performer").toLocked(); 4196 4197 /** 4198 * Search parameter: <b>goal</b> 4199 * <p> 4200 * Description: <b>Desired outcome of plan</b><br> 4201 * Type: <b>reference</b><br> 4202 * Path: <b>CarePlan.goal</b><br> 4203 * </p> 4204 */ 4205 @SearchParamDefinition(name="goal", path="CarePlan.goal", description="Desired outcome of plan", type="reference", target={Goal.class } ) 4206 public static final String SP_GOAL = "goal"; 4207 /** 4208 * <b>Fluent Client</b> search parameter constant for <b>goal</b> 4209 * <p> 4210 * Description: <b>Desired outcome of plan</b><br> 4211 * Type: <b>reference</b><br> 4212 * Path: <b>CarePlan.goal</b><br> 4213 * </p> 4214 */ 4215 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GOAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GOAL); 4216 4217/** 4218 * Constant for fluent queries to be used to add include statements. Specifies 4219 * the path value of "<b>CarePlan:goal</b>". 4220 */ 4221 public static final ca.uhn.fhir.model.api.Include INCLUDE_GOAL = new ca.uhn.fhir.model.api.Include("CarePlan:goal").toLocked(); 4222 4223 /** 4224 * Search parameter: <b>subject</b> 4225 * <p> 4226 * Description: <b>Who care plan is for</b><br> 4227 * Type: <b>reference</b><br> 4228 * Path: <b>CarePlan.subject</b><br> 4229 * </p> 4230 */ 4231 @SearchParamDefinition(name="subject", path="CarePlan.subject", description="Who care plan is for", type="reference", target={Group.class, Patient.class } ) 4232 public static final String SP_SUBJECT = "subject"; 4233 /** 4234 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4235 * <p> 4236 * Description: <b>Who care plan is for</b><br> 4237 * Type: <b>reference</b><br> 4238 * Path: <b>CarePlan.subject</b><br> 4239 * </p> 4240 */ 4241 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4242 4243/** 4244 * Constant for fluent queries to be used to add include statements. Specifies 4245 * the path value of "<b>CarePlan:subject</b>". 4246 */ 4247 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("CarePlan:subject").toLocked(); 4248 4249 /** 4250 * Search parameter: <b>replaces</b> 4251 * <p> 4252 * Description: <b>CarePlan replaced by this CarePlan</b><br> 4253 * Type: <b>reference</b><br> 4254 * Path: <b>CarePlan.replaces</b><br> 4255 * </p> 4256 */ 4257 @SearchParamDefinition(name="replaces", path="CarePlan.replaces", description="CarePlan replaced by this CarePlan", type="reference", target={CarePlan.class } ) 4258 public static final String SP_REPLACES = "replaces"; 4259 /** 4260 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 4261 * <p> 4262 * Description: <b>CarePlan replaced by this CarePlan</b><br> 4263 * Type: <b>reference</b><br> 4264 * Path: <b>CarePlan.replaces</b><br> 4265 * </p> 4266 */ 4267 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 4268 4269/** 4270 * Constant for fluent queries to be used to add include statements. Specifies 4271 * the path value of "<b>CarePlan:replaces</b>". 4272 */ 4273 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("CarePlan:replaces").toLocked(); 4274 4275 /** 4276 * Search parameter: <b>part-of</b> 4277 * <p> 4278 * Description: <b>Part of referenced CarePlan</b><br> 4279 * Type: <b>reference</b><br> 4280 * Path: <b>CarePlan.partOf</b><br> 4281 * </p> 4282 */ 4283 @SearchParamDefinition(name="part-of", path="CarePlan.partOf", description="Part of referenced CarePlan", type="reference", target={CarePlan.class } ) 4284 public static final String SP_PART_OF = "part-of"; 4285 /** 4286 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4287 * <p> 4288 * Description: <b>Part of referenced CarePlan</b><br> 4289 * Type: <b>reference</b><br> 4290 * Path: <b>CarePlan.partOf</b><br> 4291 * </p> 4292 */ 4293 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 4294 4295/** 4296 * Constant for fluent queries to be used to add include statements. Specifies 4297 * the path value of "<b>CarePlan:part-of</b>". 4298 */ 4299 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("CarePlan:part-of").toLocked(); 4300 4301 /** 4302 * Search parameter: <b>encounter</b> 4303 * <p> 4304 * Description: <b>Created in context of</b><br> 4305 * Type: <b>reference</b><br> 4306 * Path: <b>CarePlan.context</b><br> 4307 * </p> 4308 */ 4309 @SearchParamDefinition(name="encounter", path="CarePlan.context", description="Created in context of", type="reference", target={Encounter.class } ) 4310 public static final String SP_ENCOUNTER = "encounter"; 4311 /** 4312 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4313 * <p> 4314 * Description: <b>Created in context of</b><br> 4315 * Type: <b>reference</b><br> 4316 * Path: <b>CarePlan.context</b><br> 4317 * </p> 4318 */ 4319 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4320 4321/** 4322 * Constant for fluent queries to be used to add include statements. Specifies 4323 * the path value of "<b>CarePlan:encounter</b>". 4324 */ 4325 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("CarePlan:encounter").toLocked(); 4326 4327 /** 4328 * Search parameter: <b>intent</b> 4329 * <p> 4330 * Description: <b>proposal | plan | order | option</b><br> 4331 * Type: <b>token</b><br> 4332 * Path: <b>CarePlan.intent</b><br> 4333 * </p> 4334 */ 4335 @SearchParamDefinition(name="intent", path="CarePlan.intent", description="proposal | plan | order | option", type="token" ) 4336 public static final String SP_INTENT = "intent"; 4337 /** 4338 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 4339 * <p> 4340 * Description: <b>proposal | plan | order | option</b><br> 4341 * Type: <b>token</b><br> 4342 * Path: <b>CarePlan.intent</b><br> 4343 * </p> 4344 */ 4345 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 4346 4347 /** 4348 * Search parameter: <b>activity-reference</b> 4349 * <p> 4350 * Description: <b>Activity details defined in specific resource</b><br> 4351 * Type: <b>reference</b><br> 4352 * Path: <b>CarePlan.activity.reference</b><br> 4353 * </p> 4354 */ 4355 @SearchParamDefinition(name="activity-reference", path="CarePlan.activity.reference", description="Activity details defined in specific resource", type="reference", target={Appointment.class, CommunicationRequest.class, DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ProcedureRequest.class, ReferralRequest.class, RequestGroup.class, Task.class, VisionPrescription.class } ) 4356 public static final String SP_ACTIVITY_REFERENCE = "activity-reference"; 4357 /** 4358 * <b>Fluent Client</b> search parameter constant for <b>activity-reference</b> 4359 * <p> 4360 * Description: <b>Activity details defined in specific resource</b><br> 4361 * Type: <b>reference</b><br> 4362 * Path: <b>CarePlan.activity.reference</b><br> 4363 * </p> 4364 */ 4365 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTIVITY_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTIVITY_REFERENCE); 4366 4367/** 4368 * Constant for fluent queries to be used to add include statements. Specifies 4369 * the path value of "<b>CarePlan:activity-reference</b>". 4370 */ 4371 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTIVITY_REFERENCE = new ca.uhn.fhir.model.api.Include("CarePlan:activity-reference").toLocked(); 4372 4373 /** 4374 * Search parameter: <b>condition</b> 4375 * <p> 4376 * Description: <b>Health issues this plan addresses</b><br> 4377 * Type: <b>reference</b><br> 4378 * Path: <b>CarePlan.addresses</b><br> 4379 * </p> 4380 */ 4381 @SearchParamDefinition(name="condition", path="CarePlan.addresses", description="Health issues this plan addresses", type="reference", target={Condition.class } ) 4382 public static final String SP_CONDITION = "condition"; 4383 /** 4384 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 4385 * <p> 4386 * Description: <b>Health issues this plan addresses</b><br> 4387 * Type: <b>reference</b><br> 4388 * Path: <b>CarePlan.addresses</b><br> 4389 * </p> 4390 */ 4391 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONDITION); 4392 4393/** 4394 * Constant for fluent queries to be used to add include statements. Specifies 4395 * the path value of "<b>CarePlan:condition</b>". 4396 */ 4397 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include("CarePlan:condition").toLocked(); 4398 4399 /** 4400 * Search parameter: <b>based-on</b> 4401 * <p> 4402 * Description: <b>Fulfills care plan</b><br> 4403 * Type: <b>reference</b><br> 4404 * Path: <b>CarePlan.basedOn</b><br> 4405 * </p> 4406 */ 4407 @SearchParamDefinition(name="based-on", path="CarePlan.basedOn", description="Fulfills care plan", type="reference", target={CarePlan.class } ) 4408 public static final String SP_BASED_ON = "based-on"; 4409 /** 4410 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 4411 * <p> 4412 * Description: <b>Fulfills care plan</b><br> 4413 * Type: <b>reference</b><br> 4414 * Path: <b>CarePlan.basedOn</b><br> 4415 * </p> 4416 */ 4417 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 4418 4419/** 4420 * Constant for fluent queries to be used to add include statements. Specifies 4421 * the path value of "<b>CarePlan:based-on</b>". 4422 */ 4423 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("CarePlan:based-on").toLocked(); 4424 4425 /** 4426 * Search parameter: <b>patient</b> 4427 * <p> 4428 * Description: <b>Who care plan is for</b><br> 4429 * Type: <b>reference</b><br> 4430 * Path: <b>CarePlan.subject</b><br> 4431 * </p> 4432 */ 4433 @SearchParamDefinition(name="patient", path="CarePlan.subject", description="Who care plan is for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 4434 public static final String SP_PATIENT = "patient"; 4435 /** 4436 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4437 * <p> 4438 * Description: <b>Who care plan is for</b><br> 4439 * Type: <b>reference</b><br> 4440 * Path: <b>CarePlan.subject</b><br> 4441 * </p> 4442 */ 4443 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4444 4445/** 4446 * Constant for fluent queries to be used to add include statements. Specifies 4447 * the path value of "<b>CarePlan:patient</b>". 4448 */ 4449 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CarePlan:patient").toLocked(); 4450 4451 /** 4452 * Search parameter: <b>context</b> 4453 * <p> 4454 * Description: <b>Created in context of</b><br> 4455 * Type: <b>reference</b><br> 4456 * Path: <b>CarePlan.context</b><br> 4457 * </p> 4458 */ 4459 @SearchParamDefinition(name="context", path="CarePlan.context", description="Created in context of", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 4460 public static final String SP_CONTEXT = "context"; 4461 /** 4462 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4463 * <p> 4464 * Description: <b>Created in context of</b><br> 4465 * Type: <b>reference</b><br> 4466 * Path: <b>CarePlan.context</b><br> 4467 * </p> 4468 */ 4469 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 4470 4471/** 4472 * Constant for fluent queries to be used to add include statements. Specifies 4473 * the path value of "<b>CarePlan:context</b>". 4474 */ 4475 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("CarePlan:context").toLocked(); 4476 4477 /** 4478 * Search parameter: <b>activity-date</b> 4479 * <p> 4480 * Description: <b>Specified date occurs within period specified by CarePlan.activity.timingSchedule</b><br> 4481 * Type: <b>date</b><br> 4482 * Path: <b>CarePlan.activity.detail.scheduled[x]</b><br> 4483 * </p> 4484 */ 4485 @SearchParamDefinition(name="activity-date", path="CarePlan.activity.detail.scheduled", description="Specified date occurs within period specified by CarePlan.activity.timingSchedule", type="date" ) 4486 public static final String SP_ACTIVITY_DATE = "activity-date"; 4487 /** 4488 * <b>Fluent Client</b> search parameter constant for <b>activity-date</b> 4489 * <p> 4490 * Description: <b>Specified date occurs within period specified by CarePlan.activity.timingSchedule</b><br> 4491 * Type: <b>date</b><br> 4492 * Path: <b>CarePlan.activity.detail.scheduled[x]</b><br> 4493 * </p> 4494 */ 4495 public static final ca.uhn.fhir.rest.gclient.DateClientParam ACTIVITY_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ACTIVITY_DATE); 4496 4497 /** 4498 * Search parameter: <b>definition</b> 4499 * <p> 4500 * Description: <b>Protocol or definition</b><br> 4501 * Type: <b>reference</b><br> 4502 * Path: <b>CarePlan.definition</b><br> 4503 * </p> 4504 */ 4505 @SearchParamDefinition(name="definition", path="CarePlan.definition", description="Protocol or definition", type="reference", target={PlanDefinition.class, Questionnaire.class } ) 4506 public static final String SP_DEFINITION = "definition"; 4507 /** 4508 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 4509 * <p> 4510 * Description: <b>Protocol or definition</b><br> 4511 * Type: <b>reference</b><br> 4512 * Path: <b>CarePlan.definition</b><br> 4513 * </p> 4514 */ 4515 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 4516 4517/** 4518 * Constant for fluent queries to be used to add include statements. Specifies 4519 * the path value of "<b>CarePlan:definition</b>". 4520 */ 4521 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("CarePlan:definition").toLocked(); 4522 4523 /** 4524 * Search parameter: <b>category</b> 4525 * <p> 4526 * Description: <b>Type of plan</b><br> 4527 * Type: <b>token</b><br> 4528 * Path: <b>CarePlan.category</b><br> 4529 * </p> 4530 */ 4531 @SearchParamDefinition(name="category", path="CarePlan.category", description="Type of plan", type="token" ) 4532 public static final String SP_CATEGORY = "category"; 4533 /** 4534 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4535 * <p> 4536 * Description: <b>Type of plan</b><br> 4537 * Type: <b>token</b><br> 4538 * Path: <b>CarePlan.category</b><br> 4539 * </p> 4540 */ 4541 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4542 4543 /** 4544 * Search parameter: <b>activity-code</b> 4545 * <p> 4546 * Description: <b>Detail type of activity</b><br> 4547 * Type: <b>token</b><br> 4548 * Path: <b>CarePlan.activity.detail.code</b><br> 4549 * </p> 4550 */ 4551 @SearchParamDefinition(name="activity-code", path="CarePlan.activity.detail.code", description="Detail type of activity", type="token" ) 4552 public static final String SP_ACTIVITY_CODE = "activity-code"; 4553 /** 4554 * <b>Fluent Client</b> search parameter constant for <b>activity-code</b> 4555 * <p> 4556 * Description: <b>Detail type of activity</b><br> 4557 * Type: <b>token</b><br> 4558 * Path: <b>CarePlan.activity.detail.code</b><br> 4559 * </p> 4560 */ 4561 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVITY_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVITY_CODE); 4562 4563 /** 4564 * Search parameter: <b>status</b> 4565 * <p> 4566 * Description: <b>draft | active | suspended | completed | entered-in-error | cancelled | unknown</b><br> 4567 * Type: <b>token</b><br> 4568 * Path: <b>CarePlan.status</b><br> 4569 * </p> 4570 */ 4571 @SearchParamDefinition(name="status", path="CarePlan.status", description="draft | active | suspended | completed | entered-in-error | cancelled | unknown", type="token" ) 4572 public static final String SP_STATUS = "status"; 4573 /** 4574 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4575 * <p> 4576 * Description: <b>draft | active | suspended | completed | entered-in-error | cancelled | unknown</b><br> 4577 * Type: <b>token</b><br> 4578 * Path: <b>CarePlan.status</b><br> 4579 * </p> 4580 */ 4581 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4582 4583 4584}