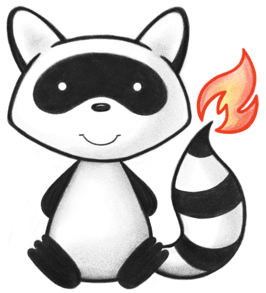
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 049 */ 050@ResourceDef(name="CareTeam", profile="http://hl7.org/fhir/Profile/CareTeam") 051public class CareTeam extends DomainResource { 052 053 public enum CareTeamStatus { 054 /** 055 * The care team has been drafted and proposed, but not yet participating in the coordination and delivery of care. 056 */ 057 PROPOSED, 058 /** 059 * The care team is currently participating in the coordination and delivery of care. 060 */ 061 ACTIVE, 062 /** 063 * The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care. 064 */ 065 SUSPENDED, 066 /** 067 * The care team was, but is no longer, participating in the coordination and delivery of care. 068 */ 069 INACTIVE, 070 /** 071 * The care team should have never existed. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 public static CareTeamStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("proposed".equals(codeString)) 082 return PROPOSED; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("suspended".equals(codeString)) 086 return SUSPENDED; 087 if ("inactive".equals(codeString)) 088 return INACTIVE; 089 if ("entered-in-error".equals(codeString)) 090 return ENTEREDINERROR; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown CareTeamStatus code '"+codeString+"'"); 095 } 096 public String toCode() { 097 switch (this) { 098 case PROPOSED: return "proposed"; 099 case ACTIVE: return "active"; 100 case SUSPENDED: return "suspended"; 101 case INACTIVE: return "inactive"; 102 case ENTEREDINERROR: return "entered-in-error"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getSystem() { 108 switch (this) { 109 case PROPOSED: return "http://hl7.org/fhir/care-team-status"; 110 case ACTIVE: return "http://hl7.org/fhir/care-team-status"; 111 case SUSPENDED: return "http://hl7.org/fhir/care-team-status"; 112 case INACTIVE: return "http://hl7.org/fhir/care-team-status"; 113 case ENTEREDINERROR: return "http://hl7.org/fhir/care-team-status"; 114 case NULL: return null; 115 default: return "?"; 116 } 117 } 118 public String getDefinition() { 119 switch (this) { 120 case PROPOSED: return "The care team has been drafted and proposed, but not yet participating in the coordination and delivery of care."; 121 case ACTIVE: return "The care team is currently participating in the coordination and delivery of care."; 122 case SUSPENDED: return "The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care."; 123 case INACTIVE: return "The care team was, but is no longer, participating in the coordination and delivery of care."; 124 case ENTEREDINERROR: return "The care team should have never existed."; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDisplay() { 130 switch (this) { 131 case PROPOSED: return "Proposed"; 132 case ACTIVE: return "Active"; 133 case SUSPENDED: return "Suspended"; 134 case INACTIVE: return "Inactive"; 135 case ENTEREDINERROR: return "Entered In Error"; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 } 141 142 public static class CareTeamStatusEnumFactory implements EnumFactory<CareTeamStatus> { 143 public CareTeamStatus fromCode(String codeString) throws IllegalArgumentException { 144 if (codeString == null || "".equals(codeString)) 145 if (codeString == null || "".equals(codeString)) 146 return null; 147 if ("proposed".equals(codeString)) 148 return CareTeamStatus.PROPOSED; 149 if ("active".equals(codeString)) 150 return CareTeamStatus.ACTIVE; 151 if ("suspended".equals(codeString)) 152 return CareTeamStatus.SUSPENDED; 153 if ("inactive".equals(codeString)) 154 return CareTeamStatus.INACTIVE; 155 if ("entered-in-error".equals(codeString)) 156 return CareTeamStatus.ENTEREDINERROR; 157 throw new IllegalArgumentException("Unknown CareTeamStatus code '"+codeString+"'"); 158 } 159 public Enumeration<CareTeamStatus> fromType(PrimitiveType<?> code) throws FHIRException { 160 if (code == null) 161 return null; 162 if (code.isEmpty()) 163 return new Enumeration<CareTeamStatus>(this); 164 String codeString = code.asStringValue(); 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("proposed".equals(codeString)) 168 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.PROPOSED); 169 if ("active".equals(codeString)) 170 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ACTIVE); 171 if ("suspended".equals(codeString)) 172 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.SUSPENDED); 173 if ("inactive".equals(codeString)) 174 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.INACTIVE); 175 if ("entered-in-error".equals(codeString)) 176 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ENTEREDINERROR); 177 throw new FHIRException("Unknown CareTeamStatus code '"+codeString+"'"); 178 } 179 public String toCode(CareTeamStatus code) { 180 if (code == CareTeamStatus.NULL) 181 return null; 182 if (code == CareTeamStatus.PROPOSED) 183 return "proposed"; 184 if (code == CareTeamStatus.ACTIVE) 185 return "active"; 186 if (code == CareTeamStatus.SUSPENDED) 187 return "suspended"; 188 if (code == CareTeamStatus.INACTIVE) 189 return "inactive"; 190 if (code == CareTeamStatus.ENTEREDINERROR) 191 return "entered-in-error"; 192 return "?"; 193 } 194 public String toSystem(CareTeamStatus code) { 195 return code.getSystem(); 196 } 197 } 198 199 @Block() 200 public static class CareTeamParticipantComponent extends BackboneElement implements IBaseBackboneElement { 201 /** 202 * Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc. 203 */ 204 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 205 @Description(shortDefinition="Type of involvement", formalDefinition="Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc." ) 206 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 207 protected CodeableConcept role; 208 209 /** 210 * The specific person or organization who is participating/expected to participate in the care team. 211 */ 212 @Child(name = "member", type = {Practitioner.class, RelatedPerson.class, Patient.class, Organization.class, CareTeam.class}, order=2, min=0, max=1, modifier=false, summary=true) 213 @Description(shortDefinition="Who is involved", formalDefinition="The specific person or organization who is participating/expected to participate in the care team." ) 214 protected Reference member; 215 216 /** 217 * The actual object that is the target of the reference (The specific person or organization who is participating/expected to participate in the care team.) 218 */ 219 protected Resource memberTarget; 220 221 /** 222 * The organization of the practitioner. 223 */ 224 @Child(name = "onBehalfOf", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 225 @Description(shortDefinition="Organization of the practitioner", formalDefinition="The organization of the practitioner." ) 226 protected Reference onBehalfOf; 227 228 /** 229 * The actual object that is the target of the reference (The organization of the practitioner.) 230 */ 231 protected Organization onBehalfOfTarget; 232 233 /** 234 * Indicates when the specific member or organization did (or is intended to) come into effect and end. 235 */ 236 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 237 @Description(shortDefinition="Time period of participant", formalDefinition="Indicates when the specific member or organization did (or is intended to) come into effect and end." ) 238 protected Period period; 239 240 private static final long serialVersionUID = -1363308804L; 241 242 /** 243 * Constructor 244 */ 245 public CareTeamParticipantComponent() { 246 super(); 247 } 248 249 /** 250 * @return {@link #role} (Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc.) 251 */ 252 public CodeableConcept getRole() { 253 if (this.role == null) 254 if (Configuration.errorOnAutoCreate()) 255 throw new Error("Attempt to auto-create CareTeamParticipantComponent.role"); 256 else if (Configuration.doAutoCreate()) 257 this.role = new CodeableConcept(); // cc 258 return this.role; 259 } 260 261 public boolean hasRole() { 262 return this.role != null && !this.role.isEmpty(); 263 } 264 265 /** 266 * @param value {@link #role} (Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc.) 267 */ 268 public CareTeamParticipantComponent setRole(CodeableConcept value) { 269 this.role = value; 270 return this; 271 } 272 273 /** 274 * @return {@link #member} (The specific person or organization who is participating/expected to participate in the care team.) 275 */ 276 public Reference getMember() { 277 if (this.member == null) 278 if (Configuration.errorOnAutoCreate()) 279 throw new Error("Attempt to auto-create CareTeamParticipantComponent.member"); 280 else if (Configuration.doAutoCreate()) 281 this.member = new Reference(); // cc 282 return this.member; 283 } 284 285 public boolean hasMember() { 286 return this.member != null && !this.member.isEmpty(); 287 } 288 289 /** 290 * @param value {@link #member} (The specific person or organization who is participating/expected to participate in the care team.) 291 */ 292 public CareTeamParticipantComponent setMember(Reference value) { 293 this.member = value; 294 return this; 295 } 296 297 /** 298 * @return {@link #member} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The specific person or organization who is participating/expected to participate in the care team.) 299 */ 300 public Resource getMemberTarget() { 301 return this.memberTarget; 302 } 303 304 /** 305 * @param value {@link #member} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The specific person or organization who is participating/expected to participate in the care team.) 306 */ 307 public CareTeamParticipantComponent setMemberTarget(Resource value) { 308 this.memberTarget = value; 309 return this; 310 } 311 312 /** 313 * @return {@link #onBehalfOf} (The organization of the practitioner.) 314 */ 315 public Reference getOnBehalfOf() { 316 if (this.onBehalfOf == null) 317 if (Configuration.errorOnAutoCreate()) 318 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 319 else if (Configuration.doAutoCreate()) 320 this.onBehalfOf = new Reference(); // cc 321 return this.onBehalfOf; 322 } 323 324 public boolean hasOnBehalfOf() { 325 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #onBehalfOf} (The organization of the practitioner.) 330 */ 331 public CareTeamParticipantComponent setOnBehalfOf(Reference value) { 332 this.onBehalfOf = value; 333 return this; 334 } 335 336 /** 337 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization of the practitioner.) 338 */ 339 public Organization getOnBehalfOfTarget() { 340 if (this.onBehalfOfTarget == null) 341 if (Configuration.errorOnAutoCreate()) 342 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 343 else if (Configuration.doAutoCreate()) 344 this.onBehalfOfTarget = new Organization(); // aa 345 return this.onBehalfOfTarget; 346 } 347 348 /** 349 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization of the practitioner.) 350 */ 351 public CareTeamParticipantComponent setOnBehalfOfTarget(Organization value) { 352 this.onBehalfOfTarget = value; 353 return this; 354 } 355 356 /** 357 * @return {@link #period} (Indicates when the specific member or organization did (or is intended to) come into effect and end.) 358 */ 359 public Period getPeriod() { 360 if (this.period == null) 361 if (Configuration.errorOnAutoCreate()) 362 throw new Error("Attempt to auto-create CareTeamParticipantComponent.period"); 363 else if (Configuration.doAutoCreate()) 364 this.period = new Period(); // cc 365 return this.period; 366 } 367 368 public boolean hasPeriod() { 369 return this.period != null && !this.period.isEmpty(); 370 } 371 372 /** 373 * @param value {@link #period} (Indicates when the specific member or organization did (or is intended to) come into effect and end.) 374 */ 375 public CareTeamParticipantComponent setPeriod(Period value) { 376 this.period = value; 377 return this; 378 } 379 380 protected void listChildren(List<Property> children) { 381 super.listChildren(children); 382 children.add(new Property("role", "CodeableConcept", "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 0, 1, role)); 383 children.add(new Property("member", "Reference(Practitioner|RelatedPerson|Patient|Organization|CareTeam)", "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, member)); 384 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, onBehalfOf)); 385 children.add(new Property("period", "Period", "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 1, period)); 386 } 387 388 @Override 389 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 390 switch (_hash) { 391 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 0, 1, role); 392 case -1077769574: /*member*/ return new Property("member", "Reference(Practitioner|RelatedPerson|Patient|Organization|CareTeam)", "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, member); 393 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, onBehalfOf); 394 case -991726143: /*period*/ return new Property("period", "Period", "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 1, period); 395 default: return super.getNamedProperty(_hash, _name, _checkValid); 396 } 397 398 } 399 400 @Override 401 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 402 switch (hash) { 403 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 404 case -1077769574: /*member*/ return this.member == null ? new Base[0] : new Base[] {this.member}; // Reference 405 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 406 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 407 default: return super.getProperty(hash, name, checkValid); 408 } 409 410 } 411 412 @Override 413 public Base setProperty(int hash, String name, Base value) throws FHIRException { 414 switch (hash) { 415 case 3506294: // role 416 this.role = castToCodeableConcept(value); // CodeableConcept 417 return value; 418 case -1077769574: // member 419 this.member = castToReference(value); // Reference 420 return value; 421 case -14402964: // onBehalfOf 422 this.onBehalfOf = castToReference(value); // Reference 423 return value; 424 case -991726143: // period 425 this.period = castToPeriod(value); // Period 426 return value; 427 default: return super.setProperty(hash, name, value); 428 } 429 430 } 431 432 @Override 433 public Base setProperty(String name, Base value) throws FHIRException { 434 if (name.equals("role")) { 435 this.role = castToCodeableConcept(value); // CodeableConcept 436 } else if (name.equals("member")) { 437 this.member = castToReference(value); // Reference 438 } else if (name.equals("onBehalfOf")) { 439 this.onBehalfOf = castToReference(value); // Reference 440 } else if (name.equals("period")) { 441 this.period = castToPeriod(value); // Period 442 } else 443 return super.setProperty(name, value); 444 return value; 445 } 446 447 @Override 448 public Base makeProperty(int hash, String name) throws FHIRException { 449 switch (hash) { 450 case 3506294: return getRole(); 451 case -1077769574: return getMember(); 452 case -14402964: return getOnBehalfOf(); 453 case -991726143: return getPeriod(); 454 default: return super.makeProperty(hash, name); 455 } 456 457 } 458 459 @Override 460 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 461 switch (hash) { 462 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 463 case -1077769574: /*member*/ return new String[] {"Reference"}; 464 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 465 case -991726143: /*period*/ return new String[] {"Period"}; 466 default: return super.getTypesForProperty(hash, name); 467 } 468 469 } 470 471 @Override 472 public Base addChild(String name) throws FHIRException { 473 if (name.equals("role")) { 474 this.role = new CodeableConcept(); 475 return this.role; 476 } 477 else if (name.equals("member")) { 478 this.member = new Reference(); 479 return this.member; 480 } 481 else if (name.equals("onBehalfOf")) { 482 this.onBehalfOf = new Reference(); 483 return this.onBehalfOf; 484 } 485 else if (name.equals("period")) { 486 this.period = new Period(); 487 return this.period; 488 } 489 else 490 return super.addChild(name); 491 } 492 493 public CareTeamParticipantComponent copy() { 494 CareTeamParticipantComponent dst = new CareTeamParticipantComponent(); 495 copyValues(dst); 496 dst.role = role == null ? null : role.copy(); 497 dst.member = member == null ? null : member.copy(); 498 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 499 dst.period = period == null ? null : period.copy(); 500 return dst; 501 } 502 503 @Override 504 public boolean equalsDeep(Base other_) { 505 if (!super.equalsDeep(other_)) 506 return false; 507 if (!(other_ instanceof CareTeamParticipantComponent)) 508 return false; 509 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 510 return compareDeep(role, o.role, true) && compareDeep(member, o.member, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 511 && compareDeep(period, o.period, true); 512 } 513 514 @Override 515 public boolean equalsShallow(Base other_) { 516 if (!super.equalsShallow(other_)) 517 return false; 518 if (!(other_ instanceof CareTeamParticipantComponent)) 519 return false; 520 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 521 return true; 522 } 523 524 public boolean isEmpty() { 525 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, member, onBehalfOf 526 , period); 527 } 528 529 public String fhirType() { 530 return "CareTeam.participant"; 531 532 } 533 534 } 535 536 /** 537 * This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. 538 */ 539 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 540 @Description(shortDefinition="External Ids for this team", formalDefinition="This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate." ) 541 protected List<Identifier> identifier; 542 543 /** 544 * Indicates the current state of the care team. 545 */ 546 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 547 @Description(shortDefinition="proposed | active | suspended | inactive | entered-in-error", formalDefinition="Indicates the current state of the care team." ) 548 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-team-status") 549 protected Enumeration<CareTeamStatus> status; 550 551 /** 552 * Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team. 553 */ 554 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 555 @Description(shortDefinition="Type of team", formalDefinition="Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team." ) 556 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-team-category") 557 protected List<CodeableConcept> category; 558 559 /** 560 * A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 561 */ 562 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 563 @Description(shortDefinition="Name of the team, such as crisis assessment team", formalDefinition="A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams." ) 564 protected StringType name; 565 566 /** 567 * Identifies the patient or group whose intended care is handled by the team. 568 */ 569 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=0, max=1, modifier=false, summary=true) 570 @Description(shortDefinition="Who care team is for", formalDefinition="Identifies the patient or group whose intended care is handled by the team." ) 571 protected Reference subject; 572 573 /** 574 * The actual object that is the target of the reference (Identifies the patient or group whose intended care is handled by the team.) 575 */ 576 protected Resource subjectTarget; 577 578 /** 579 * The encounter or episode of care that establishes the context for this care team. 580 */ 581 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=5, min=0, max=1, modifier=false, summary=true) 582 @Description(shortDefinition="Encounter or episode associated with CareTeam", formalDefinition="The encounter or episode of care that establishes the context for this care team." ) 583 protected Reference context; 584 585 /** 586 * The actual object that is the target of the reference (The encounter or episode of care that establishes the context for this care team.) 587 */ 588 protected Resource contextTarget; 589 590 /** 591 * Indicates when the team did (or is intended to) come into effect and end. 592 */ 593 @Child(name = "period", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 594 @Description(shortDefinition="Time period team covers", formalDefinition="Indicates when the team did (or is intended to) come into effect and end." ) 595 protected Period period; 596 597 /** 598 * Identifies all people and organizations who are expected to be involved in the care team. 599 */ 600 @Child(name = "participant", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 601 @Description(shortDefinition="Members of the team", formalDefinition="Identifies all people and organizations who are expected to be involved in the care team." ) 602 protected List<CareTeamParticipantComponent> participant; 603 604 /** 605 * Describes why the care team exists. 606 */ 607 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 608 @Description(shortDefinition="Why the care team exists", formalDefinition="Describes why the care team exists." ) 609 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 610 protected List<CodeableConcept> reasonCode; 611 612 /** 613 * Condition(s) that this care team addresses. 614 */ 615 @Child(name = "reasonReference", type = {Condition.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 616 @Description(shortDefinition="Why the care team exists", formalDefinition="Condition(s) that this care team addresses." ) 617 protected List<Reference> reasonReference; 618 /** 619 * The actual objects that are the target of the reference (Condition(s) that this care team addresses.) 620 */ 621 protected List<Condition> reasonReferenceTarget; 622 623 624 /** 625 * The organization responsible for the care team. 626 */ 627 @Child(name = "managingOrganization", type = {Organization.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 628 @Description(shortDefinition="Organization responsible for the care team", formalDefinition="The organization responsible for the care team." ) 629 protected List<Reference> managingOrganization; 630 /** 631 * The actual objects that are the target of the reference (The organization responsible for the care team.) 632 */ 633 protected List<Organization> managingOrganizationTarget; 634 635 636 /** 637 * Comments made about the CareTeam. 638 */ 639 @Child(name = "note", type = {Annotation.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 640 @Description(shortDefinition="Comments made about the CareTeam", formalDefinition="Comments made about the CareTeam." ) 641 protected List<Annotation> note; 642 643 private static final long serialVersionUID = 1568354370L; 644 645 /** 646 * Constructor 647 */ 648 public CareTeam() { 649 super(); 650 } 651 652 /** 653 * @return {@link #identifier} (This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.) 654 */ 655 public List<Identifier> getIdentifier() { 656 if (this.identifier == null) 657 this.identifier = new ArrayList<Identifier>(); 658 return this.identifier; 659 } 660 661 /** 662 * @return Returns a reference to <code>this</code> for easy method chaining 663 */ 664 public CareTeam setIdentifier(List<Identifier> theIdentifier) { 665 this.identifier = theIdentifier; 666 return this; 667 } 668 669 public boolean hasIdentifier() { 670 if (this.identifier == null) 671 return false; 672 for (Identifier item : this.identifier) 673 if (!item.isEmpty()) 674 return true; 675 return false; 676 } 677 678 public Identifier addIdentifier() { //3 679 Identifier t = new Identifier(); 680 if (this.identifier == null) 681 this.identifier = new ArrayList<Identifier>(); 682 this.identifier.add(t); 683 return t; 684 } 685 686 public CareTeam addIdentifier(Identifier t) { //3 687 if (t == null) 688 return this; 689 if (this.identifier == null) 690 this.identifier = new ArrayList<Identifier>(); 691 this.identifier.add(t); 692 return this; 693 } 694 695 /** 696 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 697 */ 698 public Identifier getIdentifierFirstRep() { 699 if (getIdentifier().isEmpty()) { 700 addIdentifier(); 701 } 702 return getIdentifier().get(0); 703 } 704 705 /** 706 * @return {@link #status} (Indicates the current state of the care team.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 707 */ 708 public Enumeration<CareTeamStatus> getStatusElement() { 709 if (this.status == null) 710 if (Configuration.errorOnAutoCreate()) 711 throw new Error("Attempt to auto-create CareTeam.status"); 712 else if (Configuration.doAutoCreate()) 713 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); // bb 714 return this.status; 715 } 716 717 public boolean hasStatusElement() { 718 return this.status != null && !this.status.isEmpty(); 719 } 720 721 public boolean hasStatus() { 722 return this.status != null && !this.status.isEmpty(); 723 } 724 725 /** 726 * @param value {@link #status} (Indicates the current state of the care team.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 727 */ 728 public CareTeam setStatusElement(Enumeration<CareTeamStatus> value) { 729 this.status = value; 730 return this; 731 } 732 733 /** 734 * @return Indicates the current state of the care team. 735 */ 736 public CareTeamStatus getStatus() { 737 return this.status == null ? null : this.status.getValue(); 738 } 739 740 /** 741 * @param value Indicates the current state of the care team. 742 */ 743 public CareTeam setStatus(CareTeamStatus value) { 744 if (value == null) 745 this.status = null; 746 else { 747 if (this.status == null) 748 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); 749 this.status.setValue(value); 750 } 751 return this; 752 } 753 754 /** 755 * @return {@link #category} (Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.) 756 */ 757 public List<CodeableConcept> getCategory() { 758 if (this.category == null) 759 this.category = new ArrayList<CodeableConcept>(); 760 return this.category; 761 } 762 763 /** 764 * @return Returns a reference to <code>this</code> for easy method chaining 765 */ 766 public CareTeam setCategory(List<CodeableConcept> theCategory) { 767 this.category = theCategory; 768 return this; 769 } 770 771 public boolean hasCategory() { 772 if (this.category == null) 773 return false; 774 for (CodeableConcept item : this.category) 775 if (!item.isEmpty()) 776 return true; 777 return false; 778 } 779 780 public CodeableConcept addCategory() { //3 781 CodeableConcept t = new CodeableConcept(); 782 if (this.category == null) 783 this.category = new ArrayList<CodeableConcept>(); 784 this.category.add(t); 785 return t; 786 } 787 788 public CareTeam addCategory(CodeableConcept t) { //3 789 if (t == null) 790 return this; 791 if (this.category == null) 792 this.category = new ArrayList<CodeableConcept>(); 793 this.category.add(t); 794 return this; 795 } 796 797 /** 798 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 799 */ 800 public CodeableConcept getCategoryFirstRep() { 801 if (getCategory().isEmpty()) { 802 addCategory(); 803 } 804 return getCategory().get(0); 805 } 806 807 /** 808 * @return {@link #name} (A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 809 */ 810 public StringType getNameElement() { 811 if (this.name == null) 812 if (Configuration.errorOnAutoCreate()) 813 throw new Error("Attempt to auto-create CareTeam.name"); 814 else if (Configuration.doAutoCreate()) 815 this.name = new StringType(); // bb 816 return this.name; 817 } 818 819 public boolean hasNameElement() { 820 return this.name != null && !this.name.isEmpty(); 821 } 822 823 public boolean hasName() { 824 return this.name != null && !this.name.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #name} (A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 829 */ 830 public CareTeam setNameElement(StringType value) { 831 this.name = value; 832 return this; 833 } 834 835 /** 836 * @return A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 837 */ 838 public String getName() { 839 return this.name == null ? null : this.name.getValue(); 840 } 841 842 /** 843 * @param value A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 844 */ 845 public CareTeam setName(String value) { 846 if (Utilities.noString(value)) 847 this.name = null; 848 else { 849 if (this.name == null) 850 this.name = new StringType(); 851 this.name.setValue(value); 852 } 853 return this; 854 } 855 856 /** 857 * @return {@link #subject} (Identifies the patient or group whose intended care is handled by the team.) 858 */ 859 public Reference getSubject() { 860 if (this.subject == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create CareTeam.subject"); 863 else if (Configuration.doAutoCreate()) 864 this.subject = new Reference(); // cc 865 return this.subject; 866 } 867 868 public boolean hasSubject() { 869 return this.subject != null && !this.subject.isEmpty(); 870 } 871 872 /** 873 * @param value {@link #subject} (Identifies the patient or group whose intended care is handled by the team.) 874 */ 875 public CareTeam setSubject(Reference value) { 876 this.subject = value; 877 return this; 878 } 879 880 /** 881 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the patient or group whose intended care is handled by the team.) 882 */ 883 public Resource getSubjectTarget() { 884 return this.subjectTarget; 885 } 886 887 /** 888 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the patient or group whose intended care is handled by the team.) 889 */ 890 public CareTeam setSubjectTarget(Resource value) { 891 this.subjectTarget = value; 892 return this; 893 } 894 895 /** 896 * @return {@link #context} (The encounter or episode of care that establishes the context for this care team.) 897 */ 898 public Reference getContext() { 899 if (this.context == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create CareTeam.context"); 902 else if (Configuration.doAutoCreate()) 903 this.context = new Reference(); // cc 904 return this.context; 905 } 906 907 public boolean hasContext() { 908 return this.context != null && !this.context.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #context} (The encounter or episode of care that establishes the context for this care team.) 913 */ 914 public CareTeam setContext(Reference value) { 915 this.context = value; 916 return this; 917 } 918 919 /** 920 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this care team.) 921 */ 922 public Resource getContextTarget() { 923 return this.contextTarget; 924 } 925 926 /** 927 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this care team.) 928 */ 929 public CareTeam setContextTarget(Resource value) { 930 this.contextTarget = value; 931 return this; 932 } 933 934 /** 935 * @return {@link #period} (Indicates when the team did (or is intended to) come into effect and end.) 936 */ 937 public Period getPeriod() { 938 if (this.period == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create CareTeam.period"); 941 else if (Configuration.doAutoCreate()) 942 this.period = new Period(); // cc 943 return this.period; 944 } 945 946 public boolean hasPeriod() { 947 return this.period != null && !this.period.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #period} (Indicates when the team did (or is intended to) come into effect and end.) 952 */ 953 public CareTeam setPeriod(Period value) { 954 this.period = value; 955 return this; 956 } 957 958 /** 959 * @return {@link #participant} (Identifies all people and organizations who are expected to be involved in the care team.) 960 */ 961 public List<CareTeamParticipantComponent> getParticipant() { 962 if (this.participant == null) 963 this.participant = new ArrayList<CareTeamParticipantComponent>(); 964 return this.participant; 965 } 966 967 /** 968 * @return Returns a reference to <code>this</code> for easy method chaining 969 */ 970 public CareTeam setParticipant(List<CareTeamParticipantComponent> theParticipant) { 971 this.participant = theParticipant; 972 return this; 973 } 974 975 public boolean hasParticipant() { 976 if (this.participant == null) 977 return false; 978 for (CareTeamParticipantComponent item : this.participant) 979 if (!item.isEmpty()) 980 return true; 981 return false; 982 } 983 984 public CareTeamParticipantComponent addParticipant() { //3 985 CareTeamParticipantComponent t = new CareTeamParticipantComponent(); 986 if (this.participant == null) 987 this.participant = new ArrayList<CareTeamParticipantComponent>(); 988 this.participant.add(t); 989 return t; 990 } 991 992 public CareTeam addParticipant(CareTeamParticipantComponent t) { //3 993 if (t == null) 994 return this; 995 if (this.participant == null) 996 this.participant = new ArrayList<CareTeamParticipantComponent>(); 997 this.participant.add(t); 998 return this; 999 } 1000 1001 /** 1002 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 1003 */ 1004 public CareTeamParticipantComponent getParticipantFirstRep() { 1005 if (getParticipant().isEmpty()) { 1006 addParticipant(); 1007 } 1008 return getParticipant().get(0); 1009 } 1010 1011 /** 1012 * @return {@link #reasonCode} (Describes why the care team exists.) 1013 */ 1014 public List<CodeableConcept> getReasonCode() { 1015 if (this.reasonCode == null) 1016 this.reasonCode = new ArrayList<CodeableConcept>(); 1017 return this.reasonCode; 1018 } 1019 1020 /** 1021 * @return Returns a reference to <code>this</code> for easy method chaining 1022 */ 1023 public CareTeam setReasonCode(List<CodeableConcept> theReasonCode) { 1024 this.reasonCode = theReasonCode; 1025 return this; 1026 } 1027 1028 public boolean hasReasonCode() { 1029 if (this.reasonCode == null) 1030 return false; 1031 for (CodeableConcept item : this.reasonCode) 1032 if (!item.isEmpty()) 1033 return true; 1034 return false; 1035 } 1036 1037 public CodeableConcept addReasonCode() { //3 1038 CodeableConcept t = new CodeableConcept(); 1039 if (this.reasonCode == null) 1040 this.reasonCode = new ArrayList<CodeableConcept>(); 1041 this.reasonCode.add(t); 1042 return t; 1043 } 1044 1045 public CareTeam addReasonCode(CodeableConcept t) { //3 1046 if (t == null) 1047 return this; 1048 if (this.reasonCode == null) 1049 this.reasonCode = new ArrayList<CodeableConcept>(); 1050 this.reasonCode.add(t); 1051 return this; 1052 } 1053 1054 /** 1055 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1056 */ 1057 public CodeableConcept getReasonCodeFirstRep() { 1058 if (getReasonCode().isEmpty()) { 1059 addReasonCode(); 1060 } 1061 return getReasonCode().get(0); 1062 } 1063 1064 /** 1065 * @return {@link #reasonReference} (Condition(s) that this care team addresses.) 1066 */ 1067 public List<Reference> getReasonReference() { 1068 if (this.reasonReference == null) 1069 this.reasonReference = new ArrayList<Reference>(); 1070 return this.reasonReference; 1071 } 1072 1073 /** 1074 * @return Returns a reference to <code>this</code> for easy method chaining 1075 */ 1076 public CareTeam setReasonReference(List<Reference> theReasonReference) { 1077 this.reasonReference = theReasonReference; 1078 return this; 1079 } 1080 1081 public boolean hasReasonReference() { 1082 if (this.reasonReference == null) 1083 return false; 1084 for (Reference item : this.reasonReference) 1085 if (!item.isEmpty()) 1086 return true; 1087 return false; 1088 } 1089 1090 public Reference addReasonReference() { //3 1091 Reference t = new Reference(); 1092 if (this.reasonReference == null) 1093 this.reasonReference = new ArrayList<Reference>(); 1094 this.reasonReference.add(t); 1095 return t; 1096 } 1097 1098 public CareTeam addReasonReference(Reference t) { //3 1099 if (t == null) 1100 return this; 1101 if (this.reasonReference == null) 1102 this.reasonReference = new ArrayList<Reference>(); 1103 this.reasonReference.add(t); 1104 return this; 1105 } 1106 1107 /** 1108 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1109 */ 1110 public Reference getReasonReferenceFirstRep() { 1111 if (getReasonReference().isEmpty()) { 1112 addReasonReference(); 1113 } 1114 return getReasonReference().get(0); 1115 } 1116 1117 /** 1118 * @deprecated Use Reference#setResource(IBaseResource) instead 1119 */ 1120 @Deprecated 1121 public List<Condition> getReasonReferenceTarget() { 1122 if (this.reasonReferenceTarget == null) 1123 this.reasonReferenceTarget = new ArrayList<Condition>(); 1124 return this.reasonReferenceTarget; 1125 } 1126 1127 /** 1128 * @deprecated Use Reference#setResource(IBaseResource) instead 1129 */ 1130 @Deprecated 1131 public Condition addReasonReferenceTarget() { 1132 Condition r = new Condition(); 1133 if (this.reasonReferenceTarget == null) 1134 this.reasonReferenceTarget = new ArrayList<Condition>(); 1135 this.reasonReferenceTarget.add(r); 1136 return r; 1137 } 1138 1139 /** 1140 * @return {@link #managingOrganization} (The organization responsible for the care team.) 1141 */ 1142 public List<Reference> getManagingOrganization() { 1143 if (this.managingOrganization == null) 1144 this.managingOrganization = new ArrayList<Reference>(); 1145 return this.managingOrganization; 1146 } 1147 1148 /** 1149 * @return Returns a reference to <code>this</code> for easy method chaining 1150 */ 1151 public CareTeam setManagingOrganization(List<Reference> theManagingOrganization) { 1152 this.managingOrganization = theManagingOrganization; 1153 return this; 1154 } 1155 1156 public boolean hasManagingOrganization() { 1157 if (this.managingOrganization == null) 1158 return false; 1159 for (Reference item : this.managingOrganization) 1160 if (!item.isEmpty()) 1161 return true; 1162 return false; 1163 } 1164 1165 public Reference addManagingOrganization() { //3 1166 Reference t = new Reference(); 1167 if (this.managingOrganization == null) 1168 this.managingOrganization = new ArrayList<Reference>(); 1169 this.managingOrganization.add(t); 1170 return t; 1171 } 1172 1173 public CareTeam addManagingOrganization(Reference t) { //3 1174 if (t == null) 1175 return this; 1176 if (this.managingOrganization == null) 1177 this.managingOrganization = new ArrayList<Reference>(); 1178 this.managingOrganization.add(t); 1179 return this; 1180 } 1181 1182 /** 1183 * @return The first repetition of repeating field {@link #managingOrganization}, creating it if it does not already exist 1184 */ 1185 public Reference getManagingOrganizationFirstRep() { 1186 if (getManagingOrganization().isEmpty()) { 1187 addManagingOrganization(); 1188 } 1189 return getManagingOrganization().get(0); 1190 } 1191 1192 /** 1193 * @deprecated Use Reference#setResource(IBaseResource) instead 1194 */ 1195 @Deprecated 1196 public List<Organization> getManagingOrganizationTarget() { 1197 if (this.managingOrganizationTarget == null) 1198 this.managingOrganizationTarget = new ArrayList<Organization>(); 1199 return this.managingOrganizationTarget; 1200 } 1201 1202 /** 1203 * @deprecated Use Reference#setResource(IBaseResource) instead 1204 */ 1205 @Deprecated 1206 public Organization addManagingOrganizationTarget() { 1207 Organization r = new Organization(); 1208 if (this.managingOrganizationTarget == null) 1209 this.managingOrganizationTarget = new ArrayList<Organization>(); 1210 this.managingOrganizationTarget.add(r); 1211 return r; 1212 } 1213 1214 /** 1215 * @return {@link #note} (Comments made about the CareTeam.) 1216 */ 1217 public List<Annotation> getNote() { 1218 if (this.note == null) 1219 this.note = new ArrayList<Annotation>(); 1220 return this.note; 1221 } 1222 1223 /** 1224 * @return Returns a reference to <code>this</code> for easy method chaining 1225 */ 1226 public CareTeam setNote(List<Annotation> theNote) { 1227 this.note = theNote; 1228 return this; 1229 } 1230 1231 public boolean hasNote() { 1232 if (this.note == null) 1233 return false; 1234 for (Annotation item : this.note) 1235 if (!item.isEmpty()) 1236 return true; 1237 return false; 1238 } 1239 1240 public Annotation addNote() { //3 1241 Annotation t = new Annotation(); 1242 if (this.note == null) 1243 this.note = new ArrayList<Annotation>(); 1244 this.note.add(t); 1245 return t; 1246 } 1247 1248 public CareTeam addNote(Annotation t) { //3 1249 if (t == null) 1250 return this; 1251 if (this.note == null) 1252 this.note = new ArrayList<Annotation>(); 1253 this.note.add(t); 1254 return this; 1255 } 1256 1257 /** 1258 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1259 */ 1260 public Annotation getNoteFirstRep() { 1261 if (getNote().isEmpty()) { 1262 addNote(); 1263 } 1264 return getNote().get(0); 1265 } 1266 1267 protected void listChildren(List<Property> children) { 1268 super.listChildren(children); 1269 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1270 children.add(new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status)); 1271 children.add(new Property("category", "CodeableConcept", "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 0, java.lang.Integer.MAX_VALUE, category)); 1272 children.add(new Property("name", "string", "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, name)); 1273 children.add(new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject)); 1274 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this care team.", 0, 1, context)); 1275 children.add(new Property("period", "Period", "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period)); 1276 children.add(new Property("participant", "", "Identifies all people and organizations who are expected to be involved in the care team.", 0, java.lang.Integer.MAX_VALUE, participant)); 1277 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1278 children.add(new Property("reasonReference", "Reference(Condition)", "Condition(s) that this care team addresses.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1279 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization)); 1280 children.add(new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note)); 1281 } 1282 1283 @Override 1284 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1285 switch (_hash) { 1286 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier); 1287 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status); 1288 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 0, java.lang.Integer.MAX_VALUE, category); 1289 case 3373707: /*name*/ return new Property("name", "string", "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, name); 1290 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject); 1291 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this care team.", 0, 1, context); 1292 case -991726143: /*period*/ return new Property("period", "Period", "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period); 1293 case 767422259: /*participant*/ return new Property("participant", "", "Identifies all people and organizations who are expected to be involved in the care team.", 0, java.lang.Integer.MAX_VALUE, participant); 1294 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1295 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition)", "Condition(s) that this care team addresses.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1296 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization); 1297 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note); 1298 default: return super.getNamedProperty(_hash, _name, _checkValid); 1299 } 1300 1301 } 1302 1303 @Override 1304 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1305 switch (hash) { 1306 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1307 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CareTeamStatus> 1308 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1309 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1310 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1311 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1312 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1313 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // CareTeamParticipantComponent 1314 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1315 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1316 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : this.managingOrganization.toArray(new Base[this.managingOrganization.size()]); // Reference 1317 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1318 default: return super.getProperty(hash, name, checkValid); 1319 } 1320 1321 } 1322 1323 @Override 1324 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1325 switch (hash) { 1326 case -1618432855: // identifier 1327 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1328 return value; 1329 case -892481550: // status 1330 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1331 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1332 return value; 1333 case 50511102: // category 1334 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1335 return value; 1336 case 3373707: // name 1337 this.name = castToString(value); // StringType 1338 return value; 1339 case -1867885268: // subject 1340 this.subject = castToReference(value); // Reference 1341 return value; 1342 case 951530927: // context 1343 this.context = castToReference(value); // Reference 1344 return value; 1345 case -991726143: // period 1346 this.period = castToPeriod(value); // Period 1347 return value; 1348 case 767422259: // participant 1349 this.getParticipant().add((CareTeamParticipantComponent) value); // CareTeamParticipantComponent 1350 return value; 1351 case 722137681: // reasonCode 1352 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1353 return value; 1354 case -1146218137: // reasonReference 1355 this.getReasonReference().add(castToReference(value)); // Reference 1356 return value; 1357 case -2058947787: // managingOrganization 1358 this.getManagingOrganization().add(castToReference(value)); // Reference 1359 return value; 1360 case 3387378: // note 1361 this.getNote().add(castToAnnotation(value)); // Annotation 1362 return value; 1363 default: return super.setProperty(hash, name, value); 1364 } 1365 1366 } 1367 1368 @Override 1369 public Base setProperty(String name, Base value) throws FHIRException { 1370 if (name.equals("identifier")) { 1371 this.getIdentifier().add(castToIdentifier(value)); 1372 } else if (name.equals("status")) { 1373 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1374 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1375 } else if (name.equals("category")) { 1376 this.getCategory().add(castToCodeableConcept(value)); 1377 } else if (name.equals("name")) { 1378 this.name = castToString(value); // StringType 1379 } else if (name.equals("subject")) { 1380 this.subject = castToReference(value); // Reference 1381 } else if (name.equals("context")) { 1382 this.context = castToReference(value); // Reference 1383 } else if (name.equals("period")) { 1384 this.period = castToPeriod(value); // Period 1385 } else if (name.equals("participant")) { 1386 this.getParticipant().add((CareTeamParticipantComponent) value); 1387 } else if (name.equals("reasonCode")) { 1388 this.getReasonCode().add(castToCodeableConcept(value)); 1389 } else if (name.equals("reasonReference")) { 1390 this.getReasonReference().add(castToReference(value)); 1391 } else if (name.equals("managingOrganization")) { 1392 this.getManagingOrganization().add(castToReference(value)); 1393 } else if (name.equals("note")) { 1394 this.getNote().add(castToAnnotation(value)); 1395 } else 1396 return super.setProperty(name, value); 1397 return value; 1398 } 1399 1400 @Override 1401 public Base makeProperty(int hash, String name) throws FHIRException { 1402 switch (hash) { 1403 case -1618432855: return addIdentifier(); 1404 case -892481550: return getStatusElement(); 1405 case 50511102: return addCategory(); 1406 case 3373707: return getNameElement(); 1407 case -1867885268: return getSubject(); 1408 case 951530927: return getContext(); 1409 case -991726143: return getPeriod(); 1410 case 767422259: return addParticipant(); 1411 case 722137681: return addReasonCode(); 1412 case -1146218137: return addReasonReference(); 1413 case -2058947787: return addManagingOrganization(); 1414 case 3387378: return addNote(); 1415 default: return super.makeProperty(hash, name); 1416 } 1417 1418 } 1419 1420 @Override 1421 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1422 switch (hash) { 1423 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1424 case -892481550: /*status*/ return new String[] {"code"}; 1425 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1426 case 3373707: /*name*/ return new String[] {"string"}; 1427 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1428 case 951530927: /*context*/ return new String[] {"Reference"}; 1429 case -991726143: /*period*/ return new String[] {"Period"}; 1430 case 767422259: /*participant*/ return new String[] {}; 1431 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1432 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 1433 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1434 case 3387378: /*note*/ return new String[] {"Annotation"}; 1435 default: return super.getTypesForProperty(hash, name); 1436 } 1437 1438 } 1439 1440 @Override 1441 public Base addChild(String name) throws FHIRException { 1442 if (name.equals("identifier")) { 1443 return addIdentifier(); 1444 } 1445 else if (name.equals("status")) { 1446 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.status"); 1447 } 1448 else if (name.equals("category")) { 1449 return addCategory(); 1450 } 1451 else if (name.equals("name")) { 1452 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.name"); 1453 } 1454 else if (name.equals("subject")) { 1455 this.subject = new Reference(); 1456 return this.subject; 1457 } 1458 else if (name.equals("context")) { 1459 this.context = new Reference(); 1460 return this.context; 1461 } 1462 else if (name.equals("period")) { 1463 this.period = new Period(); 1464 return this.period; 1465 } 1466 else if (name.equals("participant")) { 1467 return addParticipant(); 1468 } 1469 else if (name.equals("reasonCode")) { 1470 return addReasonCode(); 1471 } 1472 else if (name.equals("reasonReference")) { 1473 return addReasonReference(); 1474 } 1475 else if (name.equals("managingOrganization")) { 1476 return addManagingOrganization(); 1477 } 1478 else if (name.equals("note")) { 1479 return addNote(); 1480 } 1481 else 1482 return super.addChild(name); 1483 } 1484 1485 public String fhirType() { 1486 return "CareTeam"; 1487 1488 } 1489 1490 public CareTeam copy() { 1491 CareTeam dst = new CareTeam(); 1492 copyValues(dst); 1493 if (identifier != null) { 1494 dst.identifier = new ArrayList<Identifier>(); 1495 for (Identifier i : identifier) 1496 dst.identifier.add(i.copy()); 1497 }; 1498 dst.status = status == null ? null : status.copy(); 1499 if (category != null) { 1500 dst.category = new ArrayList<CodeableConcept>(); 1501 for (CodeableConcept i : category) 1502 dst.category.add(i.copy()); 1503 }; 1504 dst.name = name == null ? null : name.copy(); 1505 dst.subject = subject == null ? null : subject.copy(); 1506 dst.context = context == null ? null : context.copy(); 1507 dst.period = period == null ? null : period.copy(); 1508 if (participant != null) { 1509 dst.participant = new ArrayList<CareTeamParticipantComponent>(); 1510 for (CareTeamParticipantComponent i : participant) 1511 dst.participant.add(i.copy()); 1512 }; 1513 if (reasonCode != null) { 1514 dst.reasonCode = new ArrayList<CodeableConcept>(); 1515 for (CodeableConcept i : reasonCode) 1516 dst.reasonCode.add(i.copy()); 1517 }; 1518 if (reasonReference != null) { 1519 dst.reasonReference = new ArrayList<Reference>(); 1520 for (Reference i : reasonReference) 1521 dst.reasonReference.add(i.copy()); 1522 }; 1523 if (managingOrganization != null) { 1524 dst.managingOrganization = new ArrayList<Reference>(); 1525 for (Reference i : managingOrganization) 1526 dst.managingOrganization.add(i.copy()); 1527 }; 1528 if (note != null) { 1529 dst.note = new ArrayList<Annotation>(); 1530 for (Annotation i : note) 1531 dst.note.add(i.copy()); 1532 }; 1533 return dst; 1534 } 1535 1536 protected CareTeam typedCopy() { 1537 return copy(); 1538 } 1539 1540 @Override 1541 public boolean equalsDeep(Base other_) { 1542 if (!super.equalsDeep(other_)) 1543 return false; 1544 if (!(other_ instanceof CareTeam)) 1545 return false; 1546 CareTeam o = (CareTeam) other_; 1547 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1548 && compareDeep(name, o.name, true) && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 1549 && compareDeep(period, o.period, true) && compareDeep(participant, o.participant, true) && compareDeep(reasonCode, o.reasonCode, true) 1550 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1551 && compareDeep(note, o.note, true); 1552 } 1553 1554 @Override 1555 public boolean equalsShallow(Base other_) { 1556 if (!super.equalsShallow(other_)) 1557 return false; 1558 if (!(other_ instanceof CareTeam)) 1559 return false; 1560 CareTeam o = (CareTeam) other_; 1561 return compareValues(status, o.status, true) && compareValues(name, o.name, true); 1562 } 1563 1564 public boolean isEmpty() { 1565 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1566 , name, subject, context, period, participant, reasonCode, reasonReference, managingOrganization 1567 , note); 1568 } 1569 1570 @Override 1571 public ResourceType getResourceType() { 1572 return ResourceType.CareTeam; 1573 } 1574 1575 /** 1576 * Search parameter: <b>date</b> 1577 * <p> 1578 * Description: <b>Time period team covers</b><br> 1579 * Type: <b>date</b><br> 1580 * Path: <b>CareTeam.period</b><br> 1581 * </p> 1582 */ 1583 @SearchParamDefinition(name="date", path="CareTeam.period", description="Time period team covers", type="date" ) 1584 public static final String SP_DATE = "date"; 1585 /** 1586 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1587 * <p> 1588 * Description: <b>Time period team covers</b><br> 1589 * Type: <b>date</b><br> 1590 * Path: <b>CareTeam.period</b><br> 1591 * </p> 1592 */ 1593 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1594 1595 /** 1596 * Search parameter: <b>identifier</b> 1597 * <p> 1598 * Description: <b>External Ids for this team</b><br> 1599 * Type: <b>token</b><br> 1600 * Path: <b>CareTeam.identifier</b><br> 1601 * </p> 1602 */ 1603 @SearchParamDefinition(name="identifier", path="CareTeam.identifier", description="External Ids for this team", type="token" ) 1604 public static final String SP_IDENTIFIER = "identifier"; 1605 /** 1606 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1607 * <p> 1608 * Description: <b>External Ids for this team</b><br> 1609 * Type: <b>token</b><br> 1610 * Path: <b>CareTeam.identifier</b><br> 1611 * </p> 1612 */ 1613 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1614 1615 /** 1616 * Search parameter: <b>patient</b> 1617 * <p> 1618 * Description: <b>Who care team is for</b><br> 1619 * Type: <b>reference</b><br> 1620 * Path: <b>CareTeam.subject</b><br> 1621 * </p> 1622 */ 1623 @SearchParamDefinition(name="patient", path="CareTeam.subject", description="Who care team is for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1624 public static final String SP_PATIENT = "patient"; 1625 /** 1626 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1627 * <p> 1628 * Description: <b>Who care team is for</b><br> 1629 * Type: <b>reference</b><br> 1630 * Path: <b>CareTeam.subject</b><br> 1631 * </p> 1632 */ 1633 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1634 1635/** 1636 * Constant for fluent queries to be used to add include statements. Specifies 1637 * the path value of "<b>CareTeam:patient</b>". 1638 */ 1639 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CareTeam:patient").toLocked(); 1640 1641 /** 1642 * Search parameter: <b>subject</b> 1643 * <p> 1644 * Description: <b>Who care team is for</b><br> 1645 * Type: <b>reference</b><br> 1646 * Path: <b>CareTeam.subject</b><br> 1647 * </p> 1648 */ 1649 @SearchParamDefinition(name="subject", path="CareTeam.subject", description="Who care team is for", type="reference", target={Group.class, Patient.class } ) 1650 public static final String SP_SUBJECT = "subject"; 1651 /** 1652 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1653 * <p> 1654 * Description: <b>Who care team is for</b><br> 1655 * Type: <b>reference</b><br> 1656 * Path: <b>CareTeam.subject</b><br> 1657 * </p> 1658 */ 1659 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1660 1661/** 1662 * Constant for fluent queries to be used to add include statements. Specifies 1663 * the path value of "<b>CareTeam:subject</b>". 1664 */ 1665 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("CareTeam:subject").toLocked(); 1666 1667 /** 1668 * Search parameter: <b>context</b> 1669 * <p> 1670 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1671 * Type: <b>reference</b><br> 1672 * Path: <b>CareTeam.context</b><br> 1673 * </p> 1674 */ 1675 @SearchParamDefinition(name="context", path="CareTeam.context", description="Encounter or episode associated with CareTeam", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 1676 public static final String SP_CONTEXT = "context"; 1677 /** 1678 * <b>Fluent Client</b> search parameter constant for <b>context</b> 1679 * <p> 1680 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1681 * Type: <b>reference</b><br> 1682 * Path: <b>CareTeam.context</b><br> 1683 * </p> 1684 */ 1685 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 1686 1687/** 1688 * Constant for fluent queries to be used to add include statements. Specifies 1689 * the path value of "<b>CareTeam:context</b>". 1690 */ 1691 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("CareTeam:context").toLocked(); 1692 1693 /** 1694 * Search parameter: <b>encounter</b> 1695 * <p> 1696 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1697 * Type: <b>reference</b><br> 1698 * Path: <b>CareTeam.context</b><br> 1699 * </p> 1700 */ 1701 @SearchParamDefinition(name="encounter", path="CareTeam.context", description="Encounter or episode associated with CareTeam", type="reference", target={Encounter.class } ) 1702 public static final String SP_ENCOUNTER = "encounter"; 1703 /** 1704 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1705 * <p> 1706 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1707 * Type: <b>reference</b><br> 1708 * Path: <b>CareTeam.context</b><br> 1709 * </p> 1710 */ 1711 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 1712 1713/** 1714 * Constant for fluent queries to be used to add include statements. Specifies 1715 * the path value of "<b>CareTeam:encounter</b>". 1716 */ 1717 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("CareTeam:encounter").toLocked(); 1718 1719 /** 1720 * Search parameter: <b>category</b> 1721 * <p> 1722 * Description: <b>Type of team</b><br> 1723 * Type: <b>token</b><br> 1724 * Path: <b>CareTeam.category</b><br> 1725 * </p> 1726 */ 1727 @SearchParamDefinition(name="category", path="CareTeam.category", description="Type of team", type="token" ) 1728 public static final String SP_CATEGORY = "category"; 1729 /** 1730 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1731 * <p> 1732 * Description: <b>Type of team</b><br> 1733 * Type: <b>token</b><br> 1734 * Path: <b>CareTeam.category</b><br> 1735 * </p> 1736 */ 1737 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1738 1739 /** 1740 * Search parameter: <b>participant</b> 1741 * <p> 1742 * Description: <b>Who is involved</b><br> 1743 * Type: <b>reference</b><br> 1744 * Path: <b>CareTeam.participant.member</b><br> 1745 * </p> 1746 */ 1747 @SearchParamDefinition(name="participant", path="CareTeam.participant.member", description="Who is involved", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 1748 public static final String SP_PARTICIPANT = "participant"; 1749 /** 1750 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 1751 * <p> 1752 * Description: <b>Who is involved</b><br> 1753 * Type: <b>reference</b><br> 1754 * Path: <b>CareTeam.participant.member</b><br> 1755 * </p> 1756 */ 1757 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 1758 1759/** 1760 * Constant for fluent queries to be used to add include statements. Specifies 1761 * the path value of "<b>CareTeam:participant</b>". 1762 */ 1763 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("CareTeam:participant").toLocked(); 1764 1765 /** 1766 * Search parameter: <b>status</b> 1767 * <p> 1768 * Description: <b>proposed | active | suspended | inactive | entered-in-error</b><br> 1769 * Type: <b>token</b><br> 1770 * Path: <b>CareTeam.status</b><br> 1771 * </p> 1772 */ 1773 @SearchParamDefinition(name="status", path="CareTeam.status", description="proposed | active | suspended | inactive | entered-in-error", type="token" ) 1774 public static final String SP_STATUS = "status"; 1775 /** 1776 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1777 * <p> 1778 * Description: <b>proposed | active | suspended | inactive | entered-in-error</b><br> 1779 * Type: <b>token</b><br> 1780 * Path: <b>CareTeam.status</b><br> 1781 * </p> 1782 */ 1783 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1784 1785 1786}