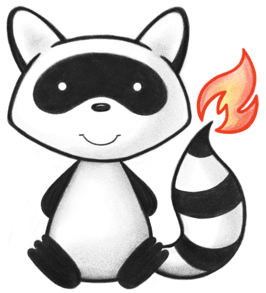
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 049 */ 050@ResourceDef(name="CareTeam", profile="http://hl7.org/fhir/Profile/CareTeam") 051public class CareTeam extends DomainResource { 052 053 public enum CareTeamStatus { 054 /** 055 * The care team has been drafted and proposed, but not yet participating in the coordination and delivery of care. 056 */ 057 PROPOSED, 058 /** 059 * The care team is currently participating in the coordination and delivery of care. 060 */ 061 ACTIVE, 062 /** 063 * The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care. 064 */ 065 SUSPENDED, 066 /** 067 * The care team was, but is no longer, participating in the coordination and delivery of care. 068 */ 069 INACTIVE, 070 /** 071 * The care team should have never existed. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 public static CareTeamStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("proposed".equals(codeString)) 082 return PROPOSED; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("suspended".equals(codeString)) 086 return SUSPENDED; 087 if ("inactive".equals(codeString)) 088 return INACTIVE; 089 if ("entered-in-error".equals(codeString)) 090 return ENTEREDINERROR; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown CareTeamStatus code '"+codeString+"'"); 095 } 096 public String toCode() { 097 switch (this) { 098 case PROPOSED: return "proposed"; 099 case ACTIVE: return "active"; 100 case SUSPENDED: return "suspended"; 101 case INACTIVE: return "inactive"; 102 case ENTEREDINERROR: return "entered-in-error"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getSystem() { 108 switch (this) { 109 case PROPOSED: return "http://hl7.org/fhir/care-team-status"; 110 case ACTIVE: return "http://hl7.org/fhir/care-team-status"; 111 case SUSPENDED: return "http://hl7.org/fhir/care-team-status"; 112 case INACTIVE: return "http://hl7.org/fhir/care-team-status"; 113 case ENTEREDINERROR: return "http://hl7.org/fhir/care-team-status"; 114 case NULL: return null; 115 default: return "?"; 116 } 117 } 118 public String getDefinition() { 119 switch (this) { 120 case PROPOSED: return "The care team has been drafted and proposed, but not yet participating in the coordination and delivery of care."; 121 case ACTIVE: return "The care team is currently participating in the coordination and delivery of care."; 122 case SUSPENDED: return "The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care."; 123 case INACTIVE: return "The care team was, but is no longer, participating in the coordination and delivery of care."; 124 case ENTEREDINERROR: return "The care team should have never existed."; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDisplay() { 130 switch (this) { 131 case PROPOSED: return "Proposed"; 132 case ACTIVE: return "Active"; 133 case SUSPENDED: return "Suspended"; 134 case INACTIVE: return "Inactive"; 135 case ENTEREDINERROR: return "Entered In Error"; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 } 141 142 public static class CareTeamStatusEnumFactory implements EnumFactory<CareTeamStatus> { 143 public CareTeamStatus fromCode(String codeString) throws IllegalArgumentException { 144 if (codeString == null || "".equals(codeString)) 145 if (codeString == null || "".equals(codeString)) 146 return null; 147 if ("proposed".equals(codeString)) 148 return CareTeamStatus.PROPOSED; 149 if ("active".equals(codeString)) 150 return CareTeamStatus.ACTIVE; 151 if ("suspended".equals(codeString)) 152 return CareTeamStatus.SUSPENDED; 153 if ("inactive".equals(codeString)) 154 return CareTeamStatus.INACTIVE; 155 if ("entered-in-error".equals(codeString)) 156 return CareTeamStatus.ENTEREDINERROR; 157 throw new IllegalArgumentException("Unknown CareTeamStatus code '"+codeString+"'"); 158 } 159 public Enumeration<CareTeamStatus> fromType(PrimitiveType<?> code) throws FHIRException { 160 if (code == null) 161 return null; 162 if (code.isEmpty()) 163 return new Enumeration<CareTeamStatus>(this); 164 String codeString = code.asStringValue(); 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("proposed".equals(codeString)) 168 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.PROPOSED); 169 if ("active".equals(codeString)) 170 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ACTIVE); 171 if ("suspended".equals(codeString)) 172 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.SUSPENDED); 173 if ("inactive".equals(codeString)) 174 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.INACTIVE); 175 if ("entered-in-error".equals(codeString)) 176 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ENTEREDINERROR); 177 throw new FHIRException("Unknown CareTeamStatus code '"+codeString+"'"); 178 } 179 public String toCode(CareTeamStatus code) { 180 if (code == CareTeamStatus.PROPOSED) 181 return "proposed"; 182 if (code == CareTeamStatus.ACTIVE) 183 return "active"; 184 if (code == CareTeamStatus.SUSPENDED) 185 return "suspended"; 186 if (code == CareTeamStatus.INACTIVE) 187 return "inactive"; 188 if (code == CareTeamStatus.ENTEREDINERROR) 189 return "entered-in-error"; 190 return "?"; 191 } 192 public String toSystem(CareTeamStatus code) { 193 return code.getSystem(); 194 } 195 } 196 197 @Block() 198 public static class CareTeamParticipantComponent extends BackboneElement implements IBaseBackboneElement { 199 /** 200 * Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc. 201 */ 202 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 203 @Description(shortDefinition="Type of involvement", formalDefinition="Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc." ) 204 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 205 protected CodeableConcept role; 206 207 /** 208 * The specific person or organization who is participating/expected to participate in the care team. 209 */ 210 @Child(name = "member", type = {Practitioner.class, RelatedPerson.class, Patient.class, Organization.class, CareTeam.class}, order=2, min=0, max=1, modifier=false, summary=true) 211 @Description(shortDefinition="Who is involved", formalDefinition="The specific person or organization who is participating/expected to participate in the care team." ) 212 protected Reference member; 213 214 /** 215 * The actual object that is the target of the reference (The specific person or organization who is participating/expected to participate in the care team.) 216 */ 217 protected Resource memberTarget; 218 219 /** 220 * The organization of the practitioner. 221 */ 222 @Child(name = "onBehalfOf", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 223 @Description(shortDefinition="Organization of the practitioner", formalDefinition="The organization of the practitioner." ) 224 protected Reference onBehalfOf; 225 226 /** 227 * The actual object that is the target of the reference (The organization of the practitioner.) 228 */ 229 protected Organization onBehalfOfTarget; 230 231 /** 232 * Indicates when the specific member or organization did (or is intended to) come into effect and end. 233 */ 234 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 235 @Description(shortDefinition="Time period of participant", formalDefinition="Indicates when the specific member or organization did (or is intended to) come into effect and end." ) 236 protected Period period; 237 238 private static final long serialVersionUID = -1363308804L; 239 240 /** 241 * Constructor 242 */ 243 public CareTeamParticipantComponent() { 244 super(); 245 } 246 247 /** 248 * @return {@link #role} (Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc.) 249 */ 250 public CodeableConcept getRole() { 251 if (this.role == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create CareTeamParticipantComponent.role"); 254 else if (Configuration.doAutoCreate()) 255 this.role = new CodeableConcept(); // cc 256 return this.role; 257 } 258 259 public boolean hasRole() { 260 return this.role != null && !this.role.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #role} (Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc.) 265 */ 266 public CareTeamParticipantComponent setRole(CodeableConcept value) { 267 this.role = value; 268 return this; 269 } 270 271 /** 272 * @return {@link #member} (The specific person or organization who is participating/expected to participate in the care team.) 273 */ 274 public Reference getMember() { 275 if (this.member == null) 276 if (Configuration.errorOnAutoCreate()) 277 throw new Error("Attempt to auto-create CareTeamParticipantComponent.member"); 278 else if (Configuration.doAutoCreate()) 279 this.member = new Reference(); // cc 280 return this.member; 281 } 282 283 public boolean hasMember() { 284 return this.member != null && !this.member.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #member} (The specific person or organization who is participating/expected to participate in the care team.) 289 */ 290 public CareTeamParticipantComponent setMember(Reference value) { 291 this.member = value; 292 return this; 293 } 294 295 /** 296 * @return {@link #member} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The specific person or organization who is participating/expected to participate in the care team.) 297 */ 298 public Resource getMemberTarget() { 299 return this.memberTarget; 300 } 301 302 /** 303 * @param value {@link #member} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The specific person or organization who is participating/expected to participate in the care team.) 304 */ 305 public CareTeamParticipantComponent setMemberTarget(Resource value) { 306 this.memberTarget = value; 307 return this; 308 } 309 310 /** 311 * @return {@link #onBehalfOf} (The organization of the practitioner.) 312 */ 313 public Reference getOnBehalfOf() { 314 if (this.onBehalfOf == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 317 else if (Configuration.doAutoCreate()) 318 this.onBehalfOf = new Reference(); // cc 319 return this.onBehalfOf; 320 } 321 322 public boolean hasOnBehalfOf() { 323 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #onBehalfOf} (The organization of the practitioner.) 328 */ 329 public CareTeamParticipantComponent setOnBehalfOf(Reference value) { 330 this.onBehalfOf = value; 331 return this; 332 } 333 334 /** 335 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization of the practitioner.) 336 */ 337 public Organization getOnBehalfOfTarget() { 338 if (this.onBehalfOfTarget == null) 339 if (Configuration.errorOnAutoCreate()) 340 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 341 else if (Configuration.doAutoCreate()) 342 this.onBehalfOfTarget = new Organization(); // aa 343 return this.onBehalfOfTarget; 344 } 345 346 /** 347 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization of the practitioner.) 348 */ 349 public CareTeamParticipantComponent setOnBehalfOfTarget(Organization value) { 350 this.onBehalfOfTarget = value; 351 return this; 352 } 353 354 /** 355 * @return {@link #period} (Indicates when the specific member or organization did (or is intended to) come into effect and end.) 356 */ 357 public Period getPeriod() { 358 if (this.period == null) 359 if (Configuration.errorOnAutoCreate()) 360 throw new Error("Attempt to auto-create CareTeamParticipantComponent.period"); 361 else if (Configuration.doAutoCreate()) 362 this.period = new Period(); // cc 363 return this.period; 364 } 365 366 public boolean hasPeriod() { 367 return this.period != null && !this.period.isEmpty(); 368 } 369 370 /** 371 * @param value {@link #period} (Indicates when the specific member or organization did (or is intended to) come into effect and end.) 372 */ 373 public CareTeamParticipantComponent setPeriod(Period value) { 374 this.period = value; 375 return this; 376 } 377 378 protected void listChildren(List<Property> children) { 379 super.listChildren(children); 380 children.add(new Property("role", "CodeableConcept", "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 0, 1, role)); 381 children.add(new Property("member", "Reference(Practitioner|RelatedPerson|Patient|Organization|CareTeam)", "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, member)); 382 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, onBehalfOf)); 383 children.add(new Property("period", "Period", "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 1, period)); 384 } 385 386 @Override 387 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 388 switch (_hash) { 389 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 0, 1, role); 390 case -1077769574: /*member*/ return new Property("member", "Reference(Practitioner|RelatedPerson|Patient|Organization|CareTeam)", "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, member); 391 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, onBehalfOf); 392 case -991726143: /*period*/ return new Property("period", "Period", "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 1, period); 393 default: return super.getNamedProperty(_hash, _name, _checkValid); 394 } 395 396 } 397 398 @Override 399 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 400 switch (hash) { 401 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 402 case -1077769574: /*member*/ return this.member == null ? new Base[0] : new Base[] {this.member}; // Reference 403 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 404 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 405 default: return super.getProperty(hash, name, checkValid); 406 } 407 408 } 409 410 @Override 411 public Base setProperty(int hash, String name, Base value) throws FHIRException { 412 switch (hash) { 413 case 3506294: // role 414 this.role = castToCodeableConcept(value); // CodeableConcept 415 return value; 416 case -1077769574: // member 417 this.member = castToReference(value); // Reference 418 return value; 419 case -14402964: // onBehalfOf 420 this.onBehalfOf = castToReference(value); // Reference 421 return value; 422 case -991726143: // period 423 this.period = castToPeriod(value); // Period 424 return value; 425 default: return super.setProperty(hash, name, value); 426 } 427 428 } 429 430 @Override 431 public Base setProperty(String name, Base value) throws FHIRException { 432 if (name.equals("role")) { 433 this.role = castToCodeableConcept(value); // CodeableConcept 434 } else if (name.equals("member")) { 435 this.member = castToReference(value); // Reference 436 } else if (name.equals("onBehalfOf")) { 437 this.onBehalfOf = castToReference(value); // Reference 438 } else if (name.equals("period")) { 439 this.period = castToPeriod(value); // Period 440 } else 441 return super.setProperty(name, value); 442 return value; 443 } 444 445 @Override 446 public Base makeProperty(int hash, String name) throws FHIRException { 447 switch (hash) { 448 case 3506294: return getRole(); 449 case -1077769574: return getMember(); 450 case -14402964: return getOnBehalfOf(); 451 case -991726143: return getPeriod(); 452 default: return super.makeProperty(hash, name); 453 } 454 455 } 456 457 @Override 458 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 459 switch (hash) { 460 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 461 case -1077769574: /*member*/ return new String[] {"Reference"}; 462 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 463 case -991726143: /*period*/ return new String[] {"Period"}; 464 default: return super.getTypesForProperty(hash, name); 465 } 466 467 } 468 469 @Override 470 public Base addChild(String name) throws FHIRException { 471 if (name.equals("role")) { 472 this.role = new CodeableConcept(); 473 return this.role; 474 } 475 else if (name.equals("member")) { 476 this.member = new Reference(); 477 return this.member; 478 } 479 else if (name.equals("onBehalfOf")) { 480 this.onBehalfOf = new Reference(); 481 return this.onBehalfOf; 482 } 483 else if (name.equals("period")) { 484 this.period = new Period(); 485 return this.period; 486 } 487 else 488 return super.addChild(name); 489 } 490 491 public CareTeamParticipantComponent copy() { 492 CareTeamParticipantComponent dst = new CareTeamParticipantComponent(); 493 copyValues(dst); 494 dst.role = role == null ? null : role.copy(); 495 dst.member = member == null ? null : member.copy(); 496 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 497 dst.period = period == null ? null : period.copy(); 498 return dst; 499 } 500 501 @Override 502 public boolean equalsDeep(Base other_) { 503 if (!super.equalsDeep(other_)) 504 return false; 505 if (!(other_ instanceof CareTeamParticipantComponent)) 506 return false; 507 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 508 return compareDeep(role, o.role, true) && compareDeep(member, o.member, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 509 && compareDeep(period, o.period, true); 510 } 511 512 @Override 513 public boolean equalsShallow(Base other_) { 514 if (!super.equalsShallow(other_)) 515 return false; 516 if (!(other_ instanceof CareTeamParticipantComponent)) 517 return false; 518 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 519 return true; 520 } 521 522 public boolean isEmpty() { 523 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, member, onBehalfOf 524 , period); 525 } 526 527 public String fhirType() { 528 return "CareTeam.participant"; 529 530 } 531 532 } 533 534 /** 535 * This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. 536 */ 537 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 538 @Description(shortDefinition="External Ids for this team", formalDefinition="This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate." ) 539 protected List<Identifier> identifier; 540 541 /** 542 * Indicates the current state of the care team. 543 */ 544 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 545 @Description(shortDefinition="proposed | active | suspended | inactive | entered-in-error", formalDefinition="Indicates the current state of the care team." ) 546 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-team-status") 547 protected Enumeration<CareTeamStatus> status; 548 549 /** 550 * Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team. 551 */ 552 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 553 @Description(shortDefinition="Type of team", formalDefinition="Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team." ) 554 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-team-category") 555 protected List<CodeableConcept> category; 556 557 /** 558 * A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 559 */ 560 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 561 @Description(shortDefinition="Name of the team, such as crisis assessment team", formalDefinition="A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams." ) 562 protected StringType name; 563 564 /** 565 * Identifies the patient or group whose intended care is handled by the team. 566 */ 567 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=0, max=1, modifier=false, summary=true) 568 @Description(shortDefinition="Who care team is for", formalDefinition="Identifies the patient or group whose intended care is handled by the team." ) 569 protected Reference subject; 570 571 /** 572 * The actual object that is the target of the reference (Identifies the patient or group whose intended care is handled by the team.) 573 */ 574 protected Resource subjectTarget; 575 576 /** 577 * The encounter or episode of care that establishes the context for this care team. 578 */ 579 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=5, min=0, max=1, modifier=false, summary=true) 580 @Description(shortDefinition="Encounter or episode associated with CareTeam", formalDefinition="The encounter or episode of care that establishes the context for this care team." ) 581 protected Reference context; 582 583 /** 584 * The actual object that is the target of the reference (The encounter or episode of care that establishes the context for this care team.) 585 */ 586 protected Resource contextTarget; 587 588 /** 589 * Indicates when the team did (or is intended to) come into effect and end. 590 */ 591 @Child(name = "period", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 592 @Description(shortDefinition="Time period team covers", formalDefinition="Indicates when the team did (or is intended to) come into effect and end." ) 593 protected Period period; 594 595 /** 596 * Identifies all people and organizations who are expected to be involved in the care team. 597 */ 598 @Child(name = "participant", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 599 @Description(shortDefinition="Members of the team", formalDefinition="Identifies all people and organizations who are expected to be involved in the care team." ) 600 protected List<CareTeamParticipantComponent> participant; 601 602 /** 603 * Describes why the care team exists. 604 */ 605 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 606 @Description(shortDefinition="Why the care team exists", formalDefinition="Describes why the care team exists." ) 607 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 608 protected List<CodeableConcept> reasonCode; 609 610 /** 611 * Condition(s) that this care team addresses. 612 */ 613 @Child(name = "reasonReference", type = {Condition.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 614 @Description(shortDefinition="Why the care team exists", formalDefinition="Condition(s) that this care team addresses." ) 615 protected List<Reference> reasonReference; 616 /** 617 * The actual objects that are the target of the reference (Condition(s) that this care team addresses.) 618 */ 619 protected List<Condition> reasonReferenceTarget; 620 621 622 /** 623 * The organization responsible for the care team. 624 */ 625 @Child(name = "managingOrganization", type = {Organization.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 626 @Description(shortDefinition="Organization responsible for the care team", formalDefinition="The organization responsible for the care team." ) 627 protected List<Reference> managingOrganization; 628 /** 629 * The actual objects that are the target of the reference (The organization responsible for the care team.) 630 */ 631 protected List<Organization> managingOrganizationTarget; 632 633 634 /** 635 * Comments made about the CareTeam. 636 */ 637 @Child(name = "note", type = {Annotation.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 638 @Description(shortDefinition="Comments made about the CareTeam", formalDefinition="Comments made about the CareTeam." ) 639 protected List<Annotation> note; 640 641 private static final long serialVersionUID = 1568354370L; 642 643 /** 644 * Constructor 645 */ 646 public CareTeam() { 647 super(); 648 } 649 650 /** 651 * @return {@link #identifier} (This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.) 652 */ 653 public List<Identifier> getIdentifier() { 654 if (this.identifier == null) 655 this.identifier = new ArrayList<Identifier>(); 656 return this.identifier; 657 } 658 659 /** 660 * @return Returns a reference to <code>this</code> for easy method chaining 661 */ 662 public CareTeam setIdentifier(List<Identifier> theIdentifier) { 663 this.identifier = theIdentifier; 664 return this; 665 } 666 667 public boolean hasIdentifier() { 668 if (this.identifier == null) 669 return false; 670 for (Identifier item : this.identifier) 671 if (!item.isEmpty()) 672 return true; 673 return false; 674 } 675 676 public Identifier addIdentifier() { //3 677 Identifier t = new Identifier(); 678 if (this.identifier == null) 679 this.identifier = new ArrayList<Identifier>(); 680 this.identifier.add(t); 681 return t; 682 } 683 684 public CareTeam addIdentifier(Identifier t) { //3 685 if (t == null) 686 return this; 687 if (this.identifier == null) 688 this.identifier = new ArrayList<Identifier>(); 689 this.identifier.add(t); 690 return this; 691 } 692 693 /** 694 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 695 */ 696 public Identifier getIdentifierFirstRep() { 697 if (getIdentifier().isEmpty()) { 698 addIdentifier(); 699 } 700 return getIdentifier().get(0); 701 } 702 703 /** 704 * @return {@link #status} (Indicates the current state of the care team.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 705 */ 706 public Enumeration<CareTeamStatus> getStatusElement() { 707 if (this.status == null) 708 if (Configuration.errorOnAutoCreate()) 709 throw new Error("Attempt to auto-create CareTeam.status"); 710 else if (Configuration.doAutoCreate()) 711 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); // bb 712 return this.status; 713 } 714 715 public boolean hasStatusElement() { 716 return this.status != null && !this.status.isEmpty(); 717 } 718 719 public boolean hasStatus() { 720 return this.status != null && !this.status.isEmpty(); 721 } 722 723 /** 724 * @param value {@link #status} (Indicates the current state of the care team.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 725 */ 726 public CareTeam setStatusElement(Enumeration<CareTeamStatus> value) { 727 this.status = value; 728 return this; 729 } 730 731 /** 732 * @return Indicates the current state of the care team. 733 */ 734 public CareTeamStatus getStatus() { 735 return this.status == null ? null : this.status.getValue(); 736 } 737 738 /** 739 * @param value Indicates the current state of the care team. 740 */ 741 public CareTeam setStatus(CareTeamStatus value) { 742 if (value == null) 743 this.status = null; 744 else { 745 if (this.status == null) 746 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); 747 this.status.setValue(value); 748 } 749 return this; 750 } 751 752 /** 753 * @return {@link #category} (Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.) 754 */ 755 public List<CodeableConcept> getCategory() { 756 if (this.category == null) 757 this.category = new ArrayList<CodeableConcept>(); 758 return this.category; 759 } 760 761 /** 762 * @return Returns a reference to <code>this</code> for easy method chaining 763 */ 764 public CareTeam setCategory(List<CodeableConcept> theCategory) { 765 this.category = theCategory; 766 return this; 767 } 768 769 public boolean hasCategory() { 770 if (this.category == null) 771 return false; 772 for (CodeableConcept item : this.category) 773 if (!item.isEmpty()) 774 return true; 775 return false; 776 } 777 778 public CodeableConcept addCategory() { //3 779 CodeableConcept t = new CodeableConcept(); 780 if (this.category == null) 781 this.category = new ArrayList<CodeableConcept>(); 782 this.category.add(t); 783 return t; 784 } 785 786 public CareTeam addCategory(CodeableConcept t) { //3 787 if (t == null) 788 return this; 789 if (this.category == null) 790 this.category = new ArrayList<CodeableConcept>(); 791 this.category.add(t); 792 return this; 793 } 794 795 /** 796 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 797 */ 798 public CodeableConcept getCategoryFirstRep() { 799 if (getCategory().isEmpty()) { 800 addCategory(); 801 } 802 return getCategory().get(0); 803 } 804 805 /** 806 * @return {@link #name} (A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 807 */ 808 public StringType getNameElement() { 809 if (this.name == null) 810 if (Configuration.errorOnAutoCreate()) 811 throw new Error("Attempt to auto-create CareTeam.name"); 812 else if (Configuration.doAutoCreate()) 813 this.name = new StringType(); // bb 814 return this.name; 815 } 816 817 public boolean hasNameElement() { 818 return this.name != null && !this.name.isEmpty(); 819 } 820 821 public boolean hasName() { 822 return this.name != null && !this.name.isEmpty(); 823 } 824 825 /** 826 * @param value {@link #name} (A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 827 */ 828 public CareTeam setNameElement(StringType value) { 829 this.name = value; 830 return this; 831 } 832 833 /** 834 * @return A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 835 */ 836 public String getName() { 837 return this.name == null ? null : this.name.getValue(); 838 } 839 840 /** 841 * @param value A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 842 */ 843 public CareTeam setName(String value) { 844 if (Utilities.noString(value)) 845 this.name = null; 846 else { 847 if (this.name == null) 848 this.name = new StringType(); 849 this.name.setValue(value); 850 } 851 return this; 852 } 853 854 /** 855 * @return {@link #subject} (Identifies the patient or group whose intended care is handled by the team.) 856 */ 857 public Reference getSubject() { 858 if (this.subject == null) 859 if (Configuration.errorOnAutoCreate()) 860 throw new Error("Attempt to auto-create CareTeam.subject"); 861 else if (Configuration.doAutoCreate()) 862 this.subject = new Reference(); // cc 863 return this.subject; 864 } 865 866 public boolean hasSubject() { 867 return this.subject != null && !this.subject.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #subject} (Identifies the patient or group whose intended care is handled by the team.) 872 */ 873 public CareTeam setSubject(Reference value) { 874 this.subject = value; 875 return this; 876 } 877 878 /** 879 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the patient or group whose intended care is handled by the team.) 880 */ 881 public Resource getSubjectTarget() { 882 return this.subjectTarget; 883 } 884 885 /** 886 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the patient or group whose intended care is handled by the team.) 887 */ 888 public CareTeam setSubjectTarget(Resource value) { 889 this.subjectTarget = value; 890 return this; 891 } 892 893 /** 894 * @return {@link #context} (The encounter or episode of care that establishes the context for this care team.) 895 */ 896 public Reference getContext() { 897 if (this.context == null) 898 if (Configuration.errorOnAutoCreate()) 899 throw new Error("Attempt to auto-create CareTeam.context"); 900 else if (Configuration.doAutoCreate()) 901 this.context = new Reference(); // cc 902 return this.context; 903 } 904 905 public boolean hasContext() { 906 return this.context != null && !this.context.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #context} (The encounter or episode of care that establishes the context for this care team.) 911 */ 912 public CareTeam setContext(Reference value) { 913 this.context = value; 914 return this; 915 } 916 917 /** 918 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this care team.) 919 */ 920 public Resource getContextTarget() { 921 return this.contextTarget; 922 } 923 924 /** 925 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this care team.) 926 */ 927 public CareTeam setContextTarget(Resource value) { 928 this.contextTarget = value; 929 return this; 930 } 931 932 /** 933 * @return {@link #period} (Indicates when the team did (or is intended to) come into effect and end.) 934 */ 935 public Period getPeriod() { 936 if (this.period == null) 937 if (Configuration.errorOnAutoCreate()) 938 throw new Error("Attempt to auto-create CareTeam.period"); 939 else if (Configuration.doAutoCreate()) 940 this.period = new Period(); // cc 941 return this.period; 942 } 943 944 public boolean hasPeriod() { 945 return this.period != null && !this.period.isEmpty(); 946 } 947 948 /** 949 * @param value {@link #period} (Indicates when the team did (or is intended to) come into effect and end.) 950 */ 951 public CareTeam setPeriod(Period value) { 952 this.period = value; 953 return this; 954 } 955 956 /** 957 * @return {@link #participant} (Identifies all people and organizations who are expected to be involved in the care team.) 958 */ 959 public List<CareTeamParticipantComponent> getParticipant() { 960 if (this.participant == null) 961 this.participant = new ArrayList<CareTeamParticipantComponent>(); 962 return this.participant; 963 } 964 965 /** 966 * @return Returns a reference to <code>this</code> for easy method chaining 967 */ 968 public CareTeam setParticipant(List<CareTeamParticipantComponent> theParticipant) { 969 this.participant = theParticipant; 970 return this; 971 } 972 973 public boolean hasParticipant() { 974 if (this.participant == null) 975 return false; 976 for (CareTeamParticipantComponent item : this.participant) 977 if (!item.isEmpty()) 978 return true; 979 return false; 980 } 981 982 public CareTeamParticipantComponent addParticipant() { //3 983 CareTeamParticipantComponent t = new CareTeamParticipantComponent(); 984 if (this.participant == null) 985 this.participant = new ArrayList<CareTeamParticipantComponent>(); 986 this.participant.add(t); 987 return t; 988 } 989 990 public CareTeam addParticipant(CareTeamParticipantComponent t) { //3 991 if (t == null) 992 return this; 993 if (this.participant == null) 994 this.participant = new ArrayList<CareTeamParticipantComponent>(); 995 this.participant.add(t); 996 return this; 997 } 998 999 /** 1000 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 1001 */ 1002 public CareTeamParticipantComponent getParticipantFirstRep() { 1003 if (getParticipant().isEmpty()) { 1004 addParticipant(); 1005 } 1006 return getParticipant().get(0); 1007 } 1008 1009 /** 1010 * @return {@link #reasonCode} (Describes why the care team exists.) 1011 */ 1012 public List<CodeableConcept> getReasonCode() { 1013 if (this.reasonCode == null) 1014 this.reasonCode = new ArrayList<CodeableConcept>(); 1015 return this.reasonCode; 1016 } 1017 1018 /** 1019 * @return Returns a reference to <code>this</code> for easy method chaining 1020 */ 1021 public CareTeam setReasonCode(List<CodeableConcept> theReasonCode) { 1022 this.reasonCode = theReasonCode; 1023 return this; 1024 } 1025 1026 public boolean hasReasonCode() { 1027 if (this.reasonCode == null) 1028 return false; 1029 for (CodeableConcept item : this.reasonCode) 1030 if (!item.isEmpty()) 1031 return true; 1032 return false; 1033 } 1034 1035 public CodeableConcept addReasonCode() { //3 1036 CodeableConcept t = new CodeableConcept(); 1037 if (this.reasonCode == null) 1038 this.reasonCode = new ArrayList<CodeableConcept>(); 1039 this.reasonCode.add(t); 1040 return t; 1041 } 1042 1043 public CareTeam addReasonCode(CodeableConcept t) { //3 1044 if (t == null) 1045 return this; 1046 if (this.reasonCode == null) 1047 this.reasonCode = new ArrayList<CodeableConcept>(); 1048 this.reasonCode.add(t); 1049 return this; 1050 } 1051 1052 /** 1053 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1054 */ 1055 public CodeableConcept getReasonCodeFirstRep() { 1056 if (getReasonCode().isEmpty()) { 1057 addReasonCode(); 1058 } 1059 return getReasonCode().get(0); 1060 } 1061 1062 /** 1063 * @return {@link #reasonReference} (Condition(s) that this care team addresses.) 1064 */ 1065 public List<Reference> getReasonReference() { 1066 if (this.reasonReference == null) 1067 this.reasonReference = new ArrayList<Reference>(); 1068 return this.reasonReference; 1069 } 1070 1071 /** 1072 * @return Returns a reference to <code>this</code> for easy method chaining 1073 */ 1074 public CareTeam setReasonReference(List<Reference> theReasonReference) { 1075 this.reasonReference = theReasonReference; 1076 return this; 1077 } 1078 1079 public boolean hasReasonReference() { 1080 if (this.reasonReference == null) 1081 return false; 1082 for (Reference item : this.reasonReference) 1083 if (!item.isEmpty()) 1084 return true; 1085 return false; 1086 } 1087 1088 public Reference addReasonReference() { //3 1089 Reference t = new Reference(); 1090 if (this.reasonReference == null) 1091 this.reasonReference = new ArrayList<Reference>(); 1092 this.reasonReference.add(t); 1093 return t; 1094 } 1095 1096 public CareTeam addReasonReference(Reference t) { //3 1097 if (t == null) 1098 return this; 1099 if (this.reasonReference == null) 1100 this.reasonReference = new ArrayList<Reference>(); 1101 this.reasonReference.add(t); 1102 return this; 1103 } 1104 1105 /** 1106 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1107 */ 1108 public Reference getReasonReferenceFirstRep() { 1109 if (getReasonReference().isEmpty()) { 1110 addReasonReference(); 1111 } 1112 return getReasonReference().get(0); 1113 } 1114 1115 /** 1116 * @deprecated Use Reference#setResource(IBaseResource) instead 1117 */ 1118 @Deprecated 1119 public List<Condition> getReasonReferenceTarget() { 1120 if (this.reasonReferenceTarget == null) 1121 this.reasonReferenceTarget = new ArrayList<Condition>(); 1122 return this.reasonReferenceTarget; 1123 } 1124 1125 /** 1126 * @deprecated Use Reference#setResource(IBaseResource) instead 1127 */ 1128 @Deprecated 1129 public Condition addReasonReferenceTarget() { 1130 Condition r = new Condition(); 1131 if (this.reasonReferenceTarget == null) 1132 this.reasonReferenceTarget = new ArrayList<Condition>(); 1133 this.reasonReferenceTarget.add(r); 1134 return r; 1135 } 1136 1137 /** 1138 * @return {@link #managingOrganization} (The organization responsible for the care team.) 1139 */ 1140 public List<Reference> getManagingOrganization() { 1141 if (this.managingOrganization == null) 1142 this.managingOrganization = new ArrayList<Reference>(); 1143 return this.managingOrganization; 1144 } 1145 1146 /** 1147 * @return Returns a reference to <code>this</code> for easy method chaining 1148 */ 1149 public CareTeam setManagingOrganization(List<Reference> theManagingOrganization) { 1150 this.managingOrganization = theManagingOrganization; 1151 return this; 1152 } 1153 1154 public boolean hasManagingOrganization() { 1155 if (this.managingOrganization == null) 1156 return false; 1157 for (Reference item : this.managingOrganization) 1158 if (!item.isEmpty()) 1159 return true; 1160 return false; 1161 } 1162 1163 public Reference addManagingOrganization() { //3 1164 Reference t = new Reference(); 1165 if (this.managingOrganization == null) 1166 this.managingOrganization = new ArrayList<Reference>(); 1167 this.managingOrganization.add(t); 1168 return t; 1169 } 1170 1171 public CareTeam addManagingOrganization(Reference t) { //3 1172 if (t == null) 1173 return this; 1174 if (this.managingOrganization == null) 1175 this.managingOrganization = new ArrayList<Reference>(); 1176 this.managingOrganization.add(t); 1177 return this; 1178 } 1179 1180 /** 1181 * @return The first repetition of repeating field {@link #managingOrganization}, creating it if it does not already exist 1182 */ 1183 public Reference getManagingOrganizationFirstRep() { 1184 if (getManagingOrganization().isEmpty()) { 1185 addManagingOrganization(); 1186 } 1187 return getManagingOrganization().get(0); 1188 } 1189 1190 /** 1191 * @deprecated Use Reference#setResource(IBaseResource) instead 1192 */ 1193 @Deprecated 1194 public List<Organization> getManagingOrganizationTarget() { 1195 if (this.managingOrganizationTarget == null) 1196 this.managingOrganizationTarget = new ArrayList<Organization>(); 1197 return this.managingOrganizationTarget; 1198 } 1199 1200 /** 1201 * @deprecated Use Reference#setResource(IBaseResource) instead 1202 */ 1203 @Deprecated 1204 public Organization addManagingOrganizationTarget() { 1205 Organization r = new Organization(); 1206 if (this.managingOrganizationTarget == null) 1207 this.managingOrganizationTarget = new ArrayList<Organization>(); 1208 this.managingOrganizationTarget.add(r); 1209 return r; 1210 } 1211 1212 /** 1213 * @return {@link #note} (Comments made about the CareTeam.) 1214 */ 1215 public List<Annotation> getNote() { 1216 if (this.note == null) 1217 this.note = new ArrayList<Annotation>(); 1218 return this.note; 1219 } 1220 1221 /** 1222 * @return Returns a reference to <code>this</code> for easy method chaining 1223 */ 1224 public CareTeam setNote(List<Annotation> theNote) { 1225 this.note = theNote; 1226 return this; 1227 } 1228 1229 public boolean hasNote() { 1230 if (this.note == null) 1231 return false; 1232 for (Annotation item : this.note) 1233 if (!item.isEmpty()) 1234 return true; 1235 return false; 1236 } 1237 1238 public Annotation addNote() { //3 1239 Annotation t = new Annotation(); 1240 if (this.note == null) 1241 this.note = new ArrayList<Annotation>(); 1242 this.note.add(t); 1243 return t; 1244 } 1245 1246 public CareTeam addNote(Annotation t) { //3 1247 if (t == null) 1248 return this; 1249 if (this.note == null) 1250 this.note = new ArrayList<Annotation>(); 1251 this.note.add(t); 1252 return this; 1253 } 1254 1255 /** 1256 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1257 */ 1258 public Annotation getNoteFirstRep() { 1259 if (getNote().isEmpty()) { 1260 addNote(); 1261 } 1262 return getNote().get(0); 1263 } 1264 1265 protected void listChildren(List<Property> children) { 1266 super.listChildren(children); 1267 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1268 children.add(new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status)); 1269 children.add(new Property("category", "CodeableConcept", "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 0, java.lang.Integer.MAX_VALUE, category)); 1270 children.add(new Property("name", "string", "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, name)); 1271 children.add(new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject)); 1272 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this care team.", 0, 1, context)); 1273 children.add(new Property("period", "Period", "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period)); 1274 children.add(new Property("participant", "", "Identifies all people and organizations who are expected to be involved in the care team.", 0, java.lang.Integer.MAX_VALUE, participant)); 1275 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1276 children.add(new Property("reasonReference", "Reference(Condition)", "Condition(s) that this care team addresses.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1277 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization)); 1278 children.add(new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note)); 1279 } 1280 1281 @Override 1282 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1283 switch (_hash) { 1284 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this care team that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier); 1285 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status); 1286 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 0, java.lang.Integer.MAX_VALUE, category); 1287 case 3373707: /*name*/ return new Property("name", "string", "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, name); 1288 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject); 1289 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this care team.", 0, 1, context); 1290 case -991726143: /*period*/ return new Property("period", "Period", "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period); 1291 case 767422259: /*participant*/ return new Property("participant", "", "Identifies all people and organizations who are expected to be involved in the care team.", 0, java.lang.Integer.MAX_VALUE, participant); 1292 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1293 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition)", "Condition(s) that this care team addresses.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1294 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization); 1295 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note); 1296 default: return super.getNamedProperty(_hash, _name, _checkValid); 1297 } 1298 1299 } 1300 1301 @Override 1302 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1303 switch (hash) { 1304 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1305 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CareTeamStatus> 1306 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1307 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1308 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1309 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1310 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1311 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // CareTeamParticipantComponent 1312 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1313 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1314 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : this.managingOrganization.toArray(new Base[this.managingOrganization.size()]); // Reference 1315 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1316 default: return super.getProperty(hash, name, checkValid); 1317 } 1318 1319 } 1320 1321 @Override 1322 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1323 switch (hash) { 1324 case -1618432855: // identifier 1325 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1326 return value; 1327 case -892481550: // status 1328 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1329 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1330 return value; 1331 case 50511102: // category 1332 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1333 return value; 1334 case 3373707: // name 1335 this.name = castToString(value); // StringType 1336 return value; 1337 case -1867885268: // subject 1338 this.subject = castToReference(value); // Reference 1339 return value; 1340 case 951530927: // context 1341 this.context = castToReference(value); // Reference 1342 return value; 1343 case -991726143: // period 1344 this.period = castToPeriod(value); // Period 1345 return value; 1346 case 767422259: // participant 1347 this.getParticipant().add((CareTeamParticipantComponent) value); // CareTeamParticipantComponent 1348 return value; 1349 case 722137681: // reasonCode 1350 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1351 return value; 1352 case -1146218137: // reasonReference 1353 this.getReasonReference().add(castToReference(value)); // Reference 1354 return value; 1355 case -2058947787: // managingOrganization 1356 this.getManagingOrganization().add(castToReference(value)); // Reference 1357 return value; 1358 case 3387378: // note 1359 this.getNote().add(castToAnnotation(value)); // Annotation 1360 return value; 1361 default: return super.setProperty(hash, name, value); 1362 } 1363 1364 } 1365 1366 @Override 1367 public Base setProperty(String name, Base value) throws FHIRException { 1368 if (name.equals("identifier")) { 1369 this.getIdentifier().add(castToIdentifier(value)); 1370 } else if (name.equals("status")) { 1371 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1372 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1373 } else if (name.equals("category")) { 1374 this.getCategory().add(castToCodeableConcept(value)); 1375 } else if (name.equals("name")) { 1376 this.name = castToString(value); // StringType 1377 } else if (name.equals("subject")) { 1378 this.subject = castToReference(value); // Reference 1379 } else if (name.equals("context")) { 1380 this.context = castToReference(value); // Reference 1381 } else if (name.equals("period")) { 1382 this.period = castToPeriod(value); // Period 1383 } else if (name.equals("participant")) { 1384 this.getParticipant().add((CareTeamParticipantComponent) value); 1385 } else if (name.equals("reasonCode")) { 1386 this.getReasonCode().add(castToCodeableConcept(value)); 1387 } else if (name.equals("reasonReference")) { 1388 this.getReasonReference().add(castToReference(value)); 1389 } else if (name.equals("managingOrganization")) { 1390 this.getManagingOrganization().add(castToReference(value)); 1391 } else if (name.equals("note")) { 1392 this.getNote().add(castToAnnotation(value)); 1393 } else 1394 return super.setProperty(name, value); 1395 return value; 1396 } 1397 1398 @Override 1399 public Base makeProperty(int hash, String name) throws FHIRException { 1400 switch (hash) { 1401 case -1618432855: return addIdentifier(); 1402 case -892481550: return getStatusElement(); 1403 case 50511102: return addCategory(); 1404 case 3373707: return getNameElement(); 1405 case -1867885268: return getSubject(); 1406 case 951530927: return getContext(); 1407 case -991726143: return getPeriod(); 1408 case 767422259: return addParticipant(); 1409 case 722137681: return addReasonCode(); 1410 case -1146218137: return addReasonReference(); 1411 case -2058947787: return addManagingOrganization(); 1412 case 3387378: return addNote(); 1413 default: return super.makeProperty(hash, name); 1414 } 1415 1416 } 1417 1418 @Override 1419 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1420 switch (hash) { 1421 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1422 case -892481550: /*status*/ return new String[] {"code"}; 1423 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1424 case 3373707: /*name*/ return new String[] {"string"}; 1425 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1426 case 951530927: /*context*/ return new String[] {"Reference"}; 1427 case -991726143: /*period*/ return new String[] {"Period"}; 1428 case 767422259: /*participant*/ return new String[] {}; 1429 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1430 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 1431 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1432 case 3387378: /*note*/ return new String[] {"Annotation"}; 1433 default: return super.getTypesForProperty(hash, name); 1434 } 1435 1436 } 1437 1438 @Override 1439 public Base addChild(String name) throws FHIRException { 1440 if (name.equals("identifier")) { 1441 return addIdentifier(); 1442 } 1443 else if (name.equals("status")) { 1444 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.status"); 1445 } 1446 else if (name.equals("category")) { 1447 return addCategory(); 1448 } 1449 else if (name.equals("name")) { 1450 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.name"); 1451 } 1452 else if (name.equals("subject")) { 1453 this.subject = new Reference(); 1454 return this.subject; 1455 } 1456 else if (name.equals("context")) { 1457 this.context = new Reference(); 1458 return this.context; 1459 } 1460 else if (name.equals("period")) { 1461 this.period = new Period(); 1462 return this.period; 1463 } 1464 else if (name.equals("participant")) { 1465 return addParticipant(); 1466 } 1467 else if (name.equals("reasonCode")) { 1468 return addReasonCode(); 1469 } 1470 else if (name.equals("reasonReference")) { 1471 return addReasonReference(); 1472 } 1473 else if (name.equals("managingOrganization")) { 1474 return addManagingOrganization(); 1475 } 1476 else if (name.equals("note")) { 1477 return addNote(); 1478 } 1479 else 1480 return super.addChild(name); 1481 } 1482 1483 public String fhirType() { 1484 return "CareTeam"; 1485 1486 } 1487 1488 public CareTeam copy() { 1489 CareTeam dst = new CareTeam(); 1490 copyValues(dst); 1491 if (identifier != null) { 1492 dst.identifier = new ArrayList<Identifier>(); 1493 for (Identifier i : identifier) 1494 dst.identifier.add(i.copy()); 1495 }; 1496 dst.status = status == null ? null : status.copy(); 1497 if (category != null) { 1498 dst.category = new ArrayList<CodeableConcept>(); 1499 for (CodeableConcept i : category) 1500 dst.category.add(i.copy()); 1501 }; 1502 dst.name = name == null ? null : name.copy(); 1503 dst.subject = subject == null ? null : subject.copy(); 1504 dst.context = context == null ? null : context.copy(); 1505 dst.period = period == null ? null : period.copy(); 1506 if (participant != null) { 1507 dst.participant = new ArrayList<CareTeamParticipantComponent>(); 1508 for (CareTeamParticipantComponent i : participant) 1509 dst.participant.add(i.copy()); 1510 }; 1511 if (reasonCode != null) { 1512 dst.reasonCode = new ArrayList<CodeableConcept>(); 1513 for (CodeableConcept i : reasonCode) 1514 dst.reasonCode.add(i.copy()); 1515 }; 1516 if (reasonReference != null) { 1517 dst.reasonReference = new ArrayList<Reference>(); 1518 for (Reference i : reasonReference) 1519 dst.reasonReference.add(i.copy()); 1520 }; 1521 if (managingOrganization != null) { 1522 dst.managingOrganization = new ArrayList<Reference>(); 1523 for (Reference i : managingOrganization) 1524 dst.managingOrganization.add(i.copy()); 1525 }; 1526 if (note != null) { 1527 dst.note = new ArrayList<Annotation>(); 1528 for (Annotation i : note) 1529 dst.note.add(i.copy()); 1530 }; 1531 return dst; 1532 } 1533 1534 protected CareTeam typedCopy() { 1535 return copy(); 1536 } 1537 1538 @Override 1539 public boolean equalsDeep(Base other_) { 1540 if (!super.equalsDeep(other_)) 1541 return false; 1542 if (!(other_ instanceof CareTeam)) 1543 return false; 1544 CareTeam o = (CareTeam) other_; 1545 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1546 && compareDeep(name, o.name, true) && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 1547 && compareDeep(period, o.period, true) && compareDeep(participant, o.participant, true) && compareDeep(reasonCode, o.reasonCode, true) 1548 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1549 && compareDeep(note, o.note, true); 1550 } 1551 1552 @Override 1553 public boolean equalsShallow(Base other_) { 1554 if (!super.equalsShallow(other_)) 1555 return false; 1556 if (!(other_ instanceof CareTeam)) 1557 return false; 1558 CareTeam o = (CareTeam) other_; 1559 return compareValues(status, o.status, true) && compareValues(name, o.name, true); 1560 } 1561 1562 public boolean isEmpty() { 1563 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1564 , name, subject, context, period, participant, reasonCode, reasonReference, managingOrganization 1565 , note); 1566 } 1567 1568 @Override 1569 public ResourceType getResourceType() { 1570 return ResourceType.CareTeam; 1571 } 1572 1573 /** 1574 * Search parameter: <b>date</b> 1575 * <p> 1576 * Description: <b>Time period team covers</b><br> 1577 * Type: <b>date</b><br> 1578 * Path: <b>CareTeam.period</b><br> 1579 * </p> 1580 */ 1581 @SearchParamDefinition(name="date", path="CareTeam.period", description="Time period team covers", type="date" ) 1582 public static final String SP_DATE = "date"; 1583 /** 1584 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1585 * <p> 1586 * Description: <b>Time period team covers</b><br> 1587 * Type: <b>date</b><br> 1588 * Path: <b>CareTeam.period</b><br> 1589 * </p> 1590 */ 1591 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1592 1593 /** 1594 * Search parameter: <b>identifier</b> 1595 * <p> 1596 * Description: <b>External Ids for this team</b><br> 1597 * Type: <b>token</b><br> 1598 * Path: <b>CareTeam.identifier</b><br> 1599 * </p> 1600 */ 1601 @SearchParamDefinition(name="identifier", path="CareTeam.identifier", description="External Ids for this team", type="token" ) 1602 public static final String SP_IDENTIFIER = "identifier"; 1603 /** 1604 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1605 * <p> 1606 * Description: <b>External Ids for this team</b><br> 1607 * Type: <b>token</b><br> 1608 * Path: <b>CareTeam.identifier</b><br> 1609 * </p> 1610 */ 1611 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1612 1613 /** 1614 * Search parameter: <b>patient</b> 1615 * <p> 1616 * Description: <b>Who care team is for</b><br> 1617 * Type: <b>reference</b><br> 1618 * Path: <b>CareTeam.subject</b><br> 1619 * </p> 1620 */ 1621 @SearchParamDefinition(name="patient", path="CareTeam.subject", description="Who care team is for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1622 public static final String SP_PATIENT = "patient"; 1623 /** 1624 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1625 * <p> 1626 * Description: <b>Who care team is for</b><br> 1627 * Type: <b>reference</b><br> 1628 * Path: <b>CareTeam.subject</b><br> 1629 * </p> 1630 */ 1631 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1632 1633/** 1634 * Constant for fluent queries to be used to add include statements. Specifies 1635 * the path value of "<b>CareTeam:patient</b>". 1636 */ 1637 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CareTeam:patient").toLocked(); 1638 1639 /** 1640 * Search parameter: <b>subject</b> 1641 * <p> 1642 * Description: <b>Who care team is for</b><br> 1643 * Type: <b>reference</b><br> 1644 * Path: <b>CareTeam.subject</b><br> 1645 * </p> 1646 */ 1647 @SearchParamDefinition(name="subject", path="CareTeam.subject", description="Who care team is for", type="reference", target={Group.class, Patient.class } ) 1648 public static final String SP_SUBJECT = "subject"; 1649 /** 1650 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1651 * <p> 1652 * Description: <b>Who care team is for</b><br> 1653 * Type: <b>reference</b><br> 1654 * Path: <b>CareTeam.subject</b><br> 1655 * </p> 1656 */ 1657 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1658 1659/** 1660 * Constant for fluent queries to be used to add include statements. Specifies 1661 * the path value of "<b>CareTeam:subject</b>". 1662 */ 1663 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("CareTeam:subject").toLocked(); 1664 1665 /** 1666 * Search parameter: <b>context</b> 1667 * <p> 1668 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1669 * Type: <b>reference</b><br> 1670 * Path: <b>CareTeam.context</b><br> 1671 * </p> 1672 */ 1673 @SearchParamDefinition(name="context", path="CareTeam.context", description="Encounter or episode associated with CareTeam", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 1674 public static final String SP_CONTEXT = "context"; 1675 /** 1676 * <b>Fluent Client</b> search parameter constant for <b>context</b> 1677 * <p> 1678 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1679 * Type: <b>reference</b><br> 1680 * Path: <b>CareTeam.context</b><br> 1681 * </p> 1682 */ 1683 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 1684 1685/** 1686 * Constant for fluent queries to be used to add include statements. Specifies 1687 * the path value of "<b>CareTeam:context</b>". 1688 */ 1689 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("CareTeam:context").toLocked(); 1690 1691 /** 1692 * Search parameter: <b>encounter</b> 1693 * <p> 1694 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1695 * Type: <b>reference</b><br> 1696 * Path: <b>CareTeam.context</b><br> 1697 * </p> 1698 */ 1699 @SearchParamDefinition(name="encounter", path="CareTeam.context", description="Encounter or episode associated with CareTeam", type="reference", target={Encounter.class } ) 1700 public static final String SP_ENCOUNTER = "encounter"; 1701 /** 1702 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1703 * <p> 1704 * Description: <b>Encounter or episode associated with CareTeam</b><br> 1705 * Type: <b>reference</b><br> 1706 * Path: <b>CareTeam.context</b><br> 1707 * </p> 1708 */ 1709 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 1710 1711/** 1712 * Constant for fluent queries to be used to add include statements. Specifies 1713 * the path value of "<b>CareTeam:encounter</b>". 1714 */ 1715 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("CareTeam:encounter").toLocked(); 1716 1717 /** 1718 * Search parameter: <b>category</b> 1719 * <p> 1720 * Description: <b>Type of team</b><br> 1721 * Type: <b>token</b><br> 1722 * Path: <b>CareTeam.category</b><br> 1723 * </p> 1724 */ 1725 @SearchParamDefinition(name="category", path="CareTeam.category", description="Type of team", type="token" ) 1726 public static final String SP_CATEGORY = "category"; 1727 /** 1728 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1729 * <p> 1730 * Description: <b>Type of team</b><br> 1731 * Type: <b>token</b><br> 1732 * Path: <b>CareTeam.category</b><br> 1733 * </p> 1734 */ 1735 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1736 1737 /** 1738 * Search parameter: <b>participant</b> 1739 * <p> 1740 * Description: <b>Who is involved</b><br> 1741 * Type: <b>reference</b><br> 1742 * Path: <b>CareTeam.participant.member</b><br> 1743 * </p> 1744 */ 1745 @SearchParamDefinition(name="participant", path="CareTeam.participant.member", description="Who is involved", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 1746 public static final String SP_PARTICIPANT = "participant"; 1747 /** 1748 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 1749 * <p> 1750 * Description: <b>Who is involved</b><br> 1751 * Type: <b>reference</b><br> 1752 * Path: <b>CareTeam.participant.member</b><br> 1753 * </p> 1754 */ 1755 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 1756 1757/** 1758 * Constant for fluent queries to be used to add include statements. Specifies 1759 * the path value of "<b>CareTeam:participant</b>". 1760 */ 1761 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("CareTeam:participant").toLocked(); 1762 1763 /** 1764 * Search parameter: <b>status</b> 1765 * <p> 1766 * Description: <b>proposed | active | suspended | inactive | entered-in-error</b><br> 1767 * Type: <b>token</b><br> 1768 * Path: <b>CareTeam.status</b><br> 1769 * </p> 1770 */ 1771 @SearchParamDefinition(name="status", path="CareTeam.status", description="proposed | active | suspended | inactive | entered-in-error", type="token" ) 1772 public static final String SP_STATUS = "status"; 1773 /** 1774 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1775 * <p> 1776 * Description: <b>proposed | active | suspended | inactive | entered-in-error</b><br> 1777 * Type: <b>token</b><br> 1778 * Path: <b>CareTeam.status</b><br> 1779 * </p> 1780 */ 1781 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1782 1783 1784}