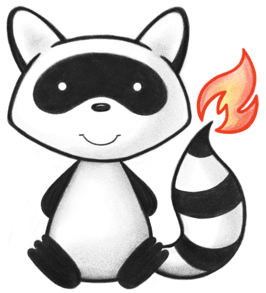
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006import java.math.BigDecimal; 007 008/* 009 Copyright (c) 2011+, HL7, Inc. 010 All rights reserved. 011 012 Redistribution and use in source and binary forms, with or without modification, 013 are permitted provided that the following conditions are met: 014 015 * Redistributions of source code must retain the above copyright notice, this 016 list of conditions and the following disclaimer. 017 * Redistributions in binary form must reproduce the above copyright notice, 018 this list of conditions and the following disclaimer in the documentation 019 and/or other materials provided with the distribution. 020 * Neither the name of HL7 nor the names of its contributors may be used to 021 endorse or promote products derived from this software without specific 022 prior written permission. 023 024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 025 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 027 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 028 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 029 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 030 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 031 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 032 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 033 POSSIBILITY OF SUCH DAMAGE. 034 035*/ 036 037// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 038import java.util.ArrayList; 039import java.util.Date; 040import java.util.List; 041 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.exceptions.FHIRFormatError; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 054 */ 055@ResourceDef(name="ChargeItem", profile="http://hl7.org/fhir/Profile/ChargeItem") 056public class ChargeItem extends DomainResource { 057 058 public enum ChargeItemStatus { 059 /** 060 * The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization 061 */ 062 PLANNED, 063 /** 064 * The charge item is ready for billing 065 */ 066 BILLABLE, 067 /** 068 * The charge item has been determined to be not billable (e.g. due to rules associated with the billing code) 069 */ 070 NOTBILLABLE, 071 /** 072 * The processing of the charge was aborted 073 */ 074 ABORTED, 075 /** 076 * The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices 077 */ 078 BILLED, 079 /** 080 * The charge item has been entered in error and should not be processed for billing 081 */ 082 ENTEREDINERROR, 083 /** 084 * The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 085 */ 086 UNKNOWN, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 public static ChargeItemStatus fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("planned".equals(codeString)) 095 return PLANNED; 096 if ("billable".equals(codeString)) 097 return BILLABLE; 098 if ("not-billable".equals(codeString)) 099 return NOTBILLABLE; 100 if ("aborted".equals(codeString)) 101 return ABORTED; 102 if ("billed".equals(codeString)) 103 return BILLED; 104 if ("entered-in-error".equals(codeString)) 105 return ENTEREDINERROR; 106 if ("unknown".equals(codeString)) 107 return UNKNOWN; 108 if (Configuration.isAcceptInvalidEnums()) 109 return null; 110 else 111 throw new FHIRException("Unknown ChargeItemStatus code '"+codeString+"'"); 112 } 113 public String toCode() { 114 switch (this) { 115 case PLANNED: return "planned"; 116 case BILLABLE: return "billable"; 117 case NOTBILLABLE: return "not-billable"; 118 case ABORTED: return "aborted"; 119 case BILLED: return "billed"; 120 case ENTEREDINERROR: return "entered-in-error"; 121 case UNKNOWN: return "unknown"; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 public String getSystem() { 127 switch (this) { 128 case PLANNED: return "http://hl7.org/fhir/chargeitem-status"; 129 case BILLABLE: return "http://hl7.org/fhir/chargeitem-status"; 130 case NOTBILLABLE: return "http://hl7.org/fhir/chargeitem-status"; 131 case ABORTED: return "http://hl7.org/fhir/chargeitem-status"; 132 case BILLED: return "http://hl7.org/fhir/chargeitem-status"; 133 case ENTEREDINERROR: return "http://hl7.org/fhir/chargeitem-status"; 134 case UNKNOWN: return "http://hl7.org/fhir/chargeitem-status"; 135 case NULL: return null; 136 default: return "?"; 137 } 138 } 139 public String getDefinition() { 140 switch (this) { 141 case PLANNED: return "The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization"; 142 case BILLABLE: return "The charge item is ready for billing"; 143 case NOTBILLABLE: return "The charge item has been determined to be not billable (e.g. due to rules associated with the billing code)"; 144 case ABORTED: return "The processing of the charge was aborted"; 145 case BILLED: return "The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices"; 146 case ENTEREDINERROR: return "The charge item has been entered in error and should not be processed for billing"; 147 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 public String getDisplay() { 153 switch (this) { 154 case PLANNED: return "Planned"; 155 case BILLABLE: return "Billable"; 156 case NOTBILLABLE: return "Not billable"; 157 case ABORTED: return "Aborted"; 158 case BILLED: return "Billed"; 159 case ENTEREDINERROR: return "Entered in Error"; 160 case UNKNOWN: return "Unknown"; 161 case NULL: return null; 162 default: return "?"; 163 } 164 } 165 } 166 167 public static class ChargeItemStatusEnumFactory implements EnumFactory<ChargeItemStatus> { 168 public ChargeItemStatus fromCode(String codeString) throws IllegalArgumentException { 169 if (codeString == null || "".equals(codeString)) 170 if (codeString == null || "".equals(codeString)) 171 return null; 172 if ("planned".equals(codeString)) 173 return ChargeItemStatus.PLANNED; 174 if ("billable".equals(codeString)) 175 return ChargeItemStatus.BILLABLE; 176 if ("not-billable".equals(codeString)) 177 return ChargeItemStatus.NOTBILLABLE; 178 if ("aborted".equals(codeString)) 179 return ChargeItemStatus.ABORTED; 180 if ("billed".equals(codeString)) 181 return ChargeItemStatus.BILLED; 182 if ("entered-in-error".equals(codeString)) 183 return ChargeItemStatus.ENTEREDINERROR; 184 if ("unknown".equals(codeString)) 185 return ChargeItemStatus.UNKNOWN; 186 throw new IllegalArgumentException("Unknown ChargeItemStatus code '"+codeString+"'"); 187 } 188 public Enumeration<ChargeItemStatus> fromType(PrimitiveType<?> code) throws FHIRException { 189 if (code == null) 190 return null; 191 if (code.isEmpty()) 192 return new Enumeration<ChargeItemStatus>(this); 193 String codeString = code.asStringValue(); 194 if (codeString == null || "".equals(codeString)) 195 return null; 196 if ("planned".equals(codeString)) 197 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.PLANNED); 198 if ("billable".equals(codeString)) 199 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLABLE); 200 if ("not-billable".equals(codeString)) 201 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NOTBILLABLE); 202 if ("aborted".equals(codeString)) 203 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ABORTED); 204 if ("billed".equals(codeString)) 205 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLED); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ENTEREDINERROR); 208 if ("unknown".equals(codeString)) 209 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.UNKNOWN); 210 throw new FHIRException("Unknown ChargeItemStatus code '"+codeString+"'"); 211 } 212 public String toCode(ChargeItemStatus code) { 213 if (code == ChargeItemStatus.NULL) 214 return null; 215 if (code == ChargeItemStatus.PLANNED) 216 return "planned"; 217 if (code == ChargeItemStatus.BILLABLE) 218 return "billable"; 219 if (code == ChargeItemStatus.NOTBILLABLE) 220 return "not-billable"; 221 if (code == ChargeItemStatus.ABORTED) 222 return "aborted"; 223 if (code == ChargeItemStatus.BILLED) 224 return "billed"; 225 if (code == ChargeItemStatus.ENTEREDINERROR) 226 return "entered-in-error"; 227 if (code == ChargeItemStatus.UNKNOWN) 228 return "unknown"; 229 return "?"; 230 } 231 public String toSystem(ChargeItemStatus code) { 232 return code.getSystem(); 233 } 234 } 235 236 @Block() 237 public static class ChargeItemParticipantComponent extends BackboneElement implements IBaseBackboneElement { 238 /** 239 * Describes the type of performance or participation(e.g. primary surgeon, anaesthesiologiest, etc.). 240 */ 241 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 242 @Description(shortDefinition="What type of performance was done", formalDefinition="Describes the type of performance or participation(e.g. primary surgeon, anaesthesiologiest, etc.)." ) 243 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 244 protected CodeableConcept role; 245 246 /** 247 * The device, practitioner, etc. who performed or participated in the service. 248 */ 249 @Child(name = "actor", type = {Practitioner.class, Organization.class, Patient.class, Device.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 250 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed or participated in the service." ) 251 protected Reference actor; 252 253 /** 254 * The actual object that is the target of the reference (The device, practitioner, etc. who performed or participated in the service.) 255 */ 256 protected Resource actorTarget; 257 258 private static final long serialVersionUID = 805521719L; 259 260 /** 261 * Constructor 262 */ 263 public ChargeItemParticipantComponent() { 264 super(); 265 } 266 267 /** 268 * Constructor 269 */ 270 public ChargeItemParticipantComponent(Reference actor) { 271 super(); 272 this.actor = actor; 273 } 274 275 /** 276 * @return {@link #role} (Describes the type of performance or participation(e.g. primary surgeon, anaesthesiologiest, etc.).) 277 */ 278 public CodeableConcept getRole() { 279 if (this.role == null) 280 if (Configuration.errorOnAutoCreate()) 281 throw new Error("Attempt to auto-create ChargeItemParticipantComponent.role"); 282 else if (Configuration.doAutoCreate()) 283 this.role = new CodeableConcept(); // cc 284 return this.role; 285 } 286 287 public boolean hasRole() { 288 return this.role != null && !this.role.isEmpty(); 289 } 290 291 /** 292 * @param value {@link #role} (Describes the type of performance or participation(e.g. primary surgeon, anaesthesiologiest, etc.).) 293 */ 294 public ChargeItemParticipantComponent setRole(CodeableConcept value) { 295 this.role = value; 296 return this; 297 } 298 299 /** 300 * @return {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 301 */ 302 public Reference getActor() { 303 if (this.actor == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create ChargeItemParticipantComponent.actor"); 306 else if (Configuration.doAutoCreate()) 307 this.actor = new Reference(); // cc 308 return this.actor; 309 } 310 311 public boolean hasActor() { 312 return this.actor != null && !this.actor.isEmpty(); 313 } 314 315 /** 316 * @param value {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 317 */ 318 public ChargeItemParticipantComponent setActor(Reference value) { 319 this.actor = value; 320 return this; 321 } 322 323 /** 324 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed or participated in the service.) 325 */ 326 public Resource getActorTarget() { 327 return this.actorTarget; 328 } 329 330 /** 331 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed or participated in the service.) 332 */ 333 public ChargeItemParticipantComponent setActorTarget(Resource value) { 334 this.actorTarget = value; 335 return this; 336 } 337 338 protected void listChildren(List<Property> children) { 339 super.listChildren(children); 340 children.add(new Property("role", "CodeableConcept", "Describes the type of performance or participation(e.g. primary surgeon, anaesthesiologiest, etc.).", 0, 1, role)); 341 children.add(new Property("actor", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor)); 342 } 343 344 @Override 345 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 346 switch (_hash) { 347 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Describes the type of performance or participation(e.g. primary surgeon, anaesthesiologiest, etc.).", 0, 1, role); 348 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor); 349 default: return super.getNamedProperty(_hash, _name, _checkValid); 350 } 351 352 } 353 354 @Override 355 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 356 switch (hash) { 357 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 358 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 359 default: return super.getProperty(hash, name, checkValid); 360 } 361 362 } 363 364 @Override 365 public Base setProperty(int hash, String name, Base value) throws FHIRException { 366 switch (hash) { 367 case 3506294: // role 368 this.role = castToCodeableConcept(value); // CodeableConcept 369 return value; 370 case 92645877: // actor 371 this.actor = castToReference(value); // Reference 372 return value; 373 default: return super.setProperty(hash, name, value); 374 } 375 376 } 377 378 @Override 379 public Base setProperty(String name, Base value) throws FHIRException { 380 if (name.equals("role")) { 381 this.role = castToCodeableConcept(value); // CodeableConcept 382 } else if (name.equals("actor")) { 383 this.actor = castToReference(value); // Reference 384 } else 385 return super.setProperty(name, value); 386 return value; 387 } 388 389 @Override 390 public Base makeProperty(int hash, String name) throws FHIRException { 391 switch (hash) { 392 case 3506294: return getRole(); 393 case 92645877: return getActor(); 394 default: return super.makeProperty(hash, name); 395 } 396 397 } 398 399 @Override 400 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 401 switch (hash) { 402 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 403 case 92645877: /*actor*/ return new String[] {"Reference"}; 404 default: return super.getTypesForProperty(hash, name); 405 } 406 407 } 408 409 @Override 410 public Base addChild(String name) throws FHIRException { 411 if (name.equals("role")) { 412 this.role = new CodeableConcept(); 413 return this.role; 414 } 415 else if (name.equals("actor")) { 416 this.actor = new Reference(); 417 return this.actor; 418 } 419 else 420 return super.addChild(name); 421 } 422 423 public ChargeItemParticipantComponent copy() { 424 ChargeItemParticipantComponent dst = new ChargeItemParticipantComponent(); 425 copyValues(dst); 426 dst.role = role == null ? null : role.copy(); 427 dst.actor = actor == null ? null : actor.copy(); 428 return dst; 429 } 430 431 @Override 432 public boolean equalsDeep(Base other_) { 433 if (!super.equalsDeep(other_)) 434 return false; 435 if (!(other_ instanceof ChargeItemParticipantComponent)) 436 return false; 437 ChargeItemParticipantComponent o = (ChargeItemParticipantComponent) other_; 438 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 439 } 440 441 @Override 442 public boolean equalsShallow(Base other_) { 443 if (!super.equalsShallow(other_)) 444 return false; 445 if (!(other_ instanceof ChargeItemParticipantComponent)) 446 return false; 447 ChargeItemParticipantComponent o = (ChargeItemParticipantComponent) other_; 448 return true; 449 } 450 451 public boolean isEmpty() { 452 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 453 } 454 455 public String fhirType() { 456 return "ChargeItem.participant"; 457 458 } 459 460 } 461 462 /** 463 * Identifiers assigned to this event performer or other systems. 464 */ 465 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 466 @Description(shortDefinition="Business Identifier for item", formalDefinition="Identifiers assigned to this event performer or other systems." ) 467 protected Identifier identifier; 468 469 /** 470 * References the source of pricing information, rules of application for the code this ChargeItem uses. 471 */ 472 @Child(name = "definition", type = {UriType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 473 @Description(shortDefinition="Defining information about the code of this charge item", formalDefinition="References the source of pricing information, rules of application for the code this ChargeItem uses." ) 474 protected List<UriType> definition; 475 476 /** 477 * The current state of the ChargeItem. 478 */ 479 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 480 @Description(shortDefinition="planned | billable | not-billable | aborted | billed | entered-in-error | unknown", formalDefinition="The current state of the ChargeItem." ) 481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chargeitem-status") 482 protected Enumeration<ChargeItemStatus> status; 483 484 /** 485 * ChargeItems can be grouped to larger ChargeItems covering the whole set. 486 */ 487 @Child(name = "partOf", type = {ChargeItem.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 488 @Description(shortDefinition="Part of referenced ChargeItem", formalDefinition="ChargeItems can be grouped to larger ChargeItems covering the whole set." ) 489 protected List<Reference> partOf; 490 /** 491 * The actual objects that are the target of the reference (ChargeItems can be grouped to larger ChargeItems covering the whole set.) 492 */ 493 protected List<ChargeItem> partOfTarget; 494 495 496 /** 497 * A code that identifies the charge, like a billing code. 498 */ 499 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 500 @Description(shortDefinition="A code that identifies the charge, like a billing code", formalDefinition="A code that identifies the charge, like a billing code." ) 501 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 502 protected CodeableConcept code; 503 504 /** 505 * The individual or set of individuals the action is being or was performed on. 506 */ 507 @Child(name = "subject", type = {Patient.class, Group.class}, order=5, min=1, max=1, modifier=false, summary=true) 508 @Description(shortDefinition="Individual service was done for/to", formalDefinition="The individual or set of individuals the action is being or was performed on." ) 509 protected Reference subject; 510 511 /** 512 * The actual object that is the target of the reference (The individual or set of individuals the action is being or was performed on.) 513 */ 514 protected Resource subjectTarget; 515 516 /** 517 * The encounter or episode of care that establishes the context for this event. 518 */ 519 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=true) 520 @Description(shortDefinition="Encounter / Episode associated with event", formalDefinition="The encounter or episode of care that establishes the context for this event." ) 521 protected Reference context; 522 523 /** 524 * The actual object that is the target of the reference (The encounter or episode of care that establishes the context for this event.) 525 */ 526 protected Resource contextTarget; 527 528 /** 529 * Date/time(s) or duration when the charged service was applied. 530 */ 531 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 532 @Description(shortDefinition="When the charged service was applied", formalDefinition="Date/time(s) or duration when the charged service was applied." ) 533 protected Type occurrence; 534 535 /** 536 * Indicates who or what performed or participated in the charged service. 537 */ 538 @Child(name = "participant", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 539 @Description(shortDefinition="Who performed charged service", formalDefinition="Indicates who or what performed or participated in the charged service." ) 540 protected List<ChargeItemParticipantComponent> participant; 541 542 /** 543 * The organization requesting the service. 544 */ 545 @Child(name = "performingOrganization", type = {Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 546 @Description(shortDefinition="Organization providing the charged sevice", formalDefinition="The organization requesting the service." ) 547 protected Reference performingOrganization; 548 549 /** 550 * The actual object that is the target of the reference (The organization requesting the service.) 551 */ 552 protected Organization performingOrganizationTarget; 553 554 /** 555 * The organization performing the service. 556 */ 557 @Child(name = "requestingOrganization", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 558 @Description(shortDefinition="Organization requesting the charged service", formalDefinition="The organization performing the service." ) 559 protected Reference requestingOrganization; 560 561 /** 562 * The actual object that is the target of the reference (The organization performing the service.) 563 */ 564 protected Organization requestingOrganizationTarget; 565 566 /** 567 * Quantity of which the charge item has been serviced. 568 */ 569 @Child(name = "quantity", type = {Quantity.class}, order=11, min=0, max=1, modifier=false, summary=true) 570 @Description(shortDefinition="Quantity of which the charge item has been serviced", formalDefinition="Quantity of which the charge item has been serviced." ) 571 protected Quantity quantity; 572 573 /** 574 * The anatomical location where the related service has been applied. 575 */ 576 @Child(name = "bodysite", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 577 @Description(shortDefinition="Anatomical location, if relevant", formalDefinition="The anatomical location where the related service has been applied." ) 578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 579 protected List<CodeableConcept> bodysite; 580 581 /** 582 * Factor overriding the factor determined by the rules associated with the code. 583 */ 584 @Child(name = "factorOverride", type = {DecimalType.class}, order=13, min=0, max=1, modifier=false, summary=false) 585 @Description(shortDefinition="Factor overriding the associated rules", formalDefinition="Factor overriding the factor determined by the rules associated with the code." ) 586 protected DecimalType factorOverride; 587 588 /** 589 * Total price of the charge overriding the list price associated with the code. 590 */ 591 @Child(name = "priceOverride", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 592 @Description(shortDefinition="Price overriding the associated rules", formalDefinition="Total price of the charge overriding the list price associated with the code." ) 593 protected Money priceOverride; 594 595 /** 596 * If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action. 597 */ 598 @Child(name = "overrideReason", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=false) 599 @Description(shortDefinition="Reason for overriding the list price/factor", formalDefinition="If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action." ) 600 protected StringType overrideReason; 601 602 /** 603 * The device, practitioner, etc. who entered the charge item. 604 */ 605 @Child(name = "enterer", type = {Practitioner.class, Organization.class, Patient.class, Device.class, RelatedPerson.class}, order=16, min=0, max=1, modifier=false, summary=true) 606 @Description(shortDefinition="Individual who was entering", formalDefinition="The device, practitioner, etc. who entered the charge item." ) 607 protected Reference enterer; 608 609 /** 610 * The actual object that is the target of the reference (The device, practitioner, etc. who entered the charge item.) 611 */ 612 protected Resource entererTarget; 613 614 /** 615 * Date the charge item was entered. 616 */ 617 @Child(name = "enteredDate", type = {DateTimeType.class}, order=17, min=0, max=1, modifier=false, summary=true) 618 @Description(shortDefinition="Date the charge item was entered", formalDefinition="Date the charge item was entered." ) 619 protected DateTimeType enteredDate; 620 621 /** 622 * Describes why the event occurred in coded or textual form. 623 */ 624 @Child(name = "reason", type = {CodeableConcept.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 625 @Description(shortDefinition="Why was the charged service rendered?", formalDefinition="Describes why the event occurred in coded or textual form." ) 626 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 627 protected List<CodeableConcept> reason; 628 629 /** 630 * Indicated the rendered service that caused this charge. 631 */ 632 @Child(name = "service", type = {DiagnosticReport.class, ImagingStudy.class, Immunization.class, MedicationAdministration.class, MedicationDispense.class, Observation.class, Procedure.class, SupplyDelivery.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 633 @Description(shortDefinition="Which rendered service is being charged?", formalDefinition="Indicated the rendered service that caused this charge." ) 634 protected List<Reference> service; 635 /** 636 * The actual objects that are the target of the reference (Indicated the rendered service that caused this charge.) 637 */ 638 protected List<Resource> serviceTarget; 639 640 641 /** 642 * Account into which this ChargeItems belongs. 643 */ 644 @Child(name = "account", type = {Account.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 645 @Description(shortDefinition="Account to place this charge", formalDefinition="Account into which this ChargeItems belongs." ) 646 protected List<Reference> account; 647 /** 648 * The actual objects that are the target of the reference (Account into which this ChargeItems belongs.) 649 */ 650 protected List<Account> accountTarget; 651 652 653 /** 654 * Comments made about the event by the performer, subject or other participants. 655 */ 656 @Child(name = "note", type = {Annotation.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 657 @Description(shortDefinition="Comments made about the ChargeItem", formalDefinition="Comments made about the event by the performer, subject or other participants." ) 658 protected List<Annotation> note; 659 660 /** 661 * Further information supporting the this charge. 662 */ 663 @Child(name = "supportingInformation", type = {Reference.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 664 @Description(shortDefinition="Further information supporting the this charge", formalDefinition="Further information supporting the this charge." ) 665 protected List<Reference> supportingInformation; 666 /** 667 * The actual objects that are the target of the reference (Further information supporting the this charge.) 668 */ 669 protected List<Resource> supportingInformationTarget; 670 671 672 private static final long serialVersionUID = 1421123938L; 673 674 /** 675 * Constructor 676 */ 677 public ChargeItem() { 678 super(); 679 } 680 681 /** 682 * Constructor 683 */ 684 public ChargeItem(Enumeration<ChargeItemStatus> status, CodeableConcept code, Reference subject) { 685 super(); 686 this.status = status; 687 this.code = code; 688 this.subject = subject; 689 } 690 691 /** 692 * @return {@link #identifier} (Identifiers assigned to this event performer or other systems.) 693 */ 694 public Identifier getIdentifier() { 695 if (this.identifier == null) 696 if (Configuration.errorOnAutoCreate()) 697 throw new Error("Attempt to auto-create ChargeItem.identifier"); 698 else if (Configuration.doAutoCreate()) 699 this.identifier = new Identifier(); // cc 700 return this.identifier; 701 } 702 703 public boolean hasIdentifier() { 704 return this.identifier != null && !this.identifier.isEmpty(); 705 } 706 707 /** 708 * @param value {@link #identifier} (Identifiers assigned to this event performer or other systems.) 709 */ 710 public ChargeItem setIdentifier(Identifier value) { 711 this.identifier = value; 712 return this; 713 } 714 715 /** 716 * @return {@link #definition} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 717 */ 718 public List<UriType> getDefinition() { 719 if (this.definition == null) 720 this.definition = new ArrayList<UriType>(); 721 return this.definition; 722 } 723 724 /** 725 * @return Returns a reference to <code>this</code> for easy method chaining 726 */ 727 public ChargeItem setDefinition(List<UriType> theDefinition) { 728 this.definition = theDefinition; 729 return this; 730 } 731 732 public boolean hasDefinition() { 733 if (this.definition == null) 734 return false; 735 for (UriType item : this.definition) 736 if (!item.isEmpty()) 737 return true; 738 return false; 739 } 740 741 /** 742 * @return {@link #definition} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 743 */ 744 public UriType addDefinitionElement() {//2 745 UriType t = new UriType(); 746 if (this.definition == null) 747 this.definition = new ArrayList<UriType>(); 748 this.definition.add(t); 749 return t; 750 } 751 752 /** 753 * @param value {@link #definition} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 754 */ 755 public ChargeItem addDefinition(String value) { //1 756 UriType t = new UriType(); 757 t.setValue(value); 758 if (this.definition == null) 759 this.definition = new ArrayList<UriType>(); 760 this.definition.add(t); 761 return this; 762 } 763 764 /** 765 * @param value {@link #definition} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 766 */ 767 public boolean hasDefinition(String value) { 768 if (this.definition == null) 769 return false; 770 for (UriType v : this.definition) 771 if (v.getValue().equals(value)) // uri 772 return true; 773 return false; 774 } 775 776 /** 777 * @return {@link #status} (The current state of the ChargeItem.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 778 */ 779 public Enumeration<ChargeItemStatus> getStatusElement() { 780 if (this.status == null) 781 if (Configuration.errorOnAutoCreate()) 782 throw new Error("Attempt to auto-create ChargeItem.status"); 783 else if (Configuration.doAutoCreate()) 784 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); // bb 785 return this.status; 786 } 787 788 public boolean hasStatusElement() { 789 return this.status != null && !this.status.isEmpty(); 790 } 791 792 public boolean hasStatus() { 793 return this.status != null && !this.status.isEmpty(); 794 } 795 796 /** 797 * @param value {@link #status} (The current state of the ChargeItem.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 798 */ 799 public ChargeItem setStatusElement(Enumeration<ChargeItemStatus> value) { 800 this.status = value; 801 return this; 802 } 803 804 /** 805 * @return The current state of the ChargeItem. 806 */ 807 public ChargeItemStatus getStatus() { 808 return this.status == null ? null : this.status.getValue(); 809 } 810 811 /** 812 * @param value The current state of the ChargeItem. 813 */ 814 public ChargeItem setStatus(ChargeItemStatus value) { 815 if (this.status == null) 816 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); 817 this.status.setValue(value); 818 return this; 819 } 820 821 /** 822 * @return {@link #partOf} (ChargeItems can be grouped to larger ChargeItems covering the whole set.) 823 */ 824 public List<Reference> getPartOf() { 825 if (this.partOf == null) 826 this.partOf = new ArrayList<Reference>(); 827 return this.partOf; 828 } 829 830 /** 831 * @return Returns a reference to <code>this</code> for easy method chaining 832 */ 833 public ChargeItem setPartOf(List<Reference> thePartOf) { 834 this.partOf = thePartOf; 835 return this; 836 } 837 838 public boolean hasPartOf() { 839 if (this.partOf == null) 840 return false; 841 for (Reference item : this.partOf) 842 if (!item.isEmpty()) 843 return true; 844 return false; 845 } 846 847 public Reference addPartOf() { //3 848 Reference t = new Reference(); 849 if (this.partOf == null) 850 this.partOf = new ArrayList<Reference>(); 851 this.partOf.add(t); 852 return t; 853 } 854 855 public ChargeItem addPartOf(Reference t) { //3 856 if (t == null) 857 return this; 858 if (this.partOf == null) 859 this.partOf = new ArrayList<Reference>(); 860 this.partOf.add(t); 861 return this; 862 } 863 864 /** 865 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 866 */ 867 public Reference getPartOfFirstRep() { 868 if (getPartOf().isEmpty()) { 869 addPartOf(); 870 } 871 return getPartOf().get(0); 872 } 873 874 /** 875 * @deprecated Use Reference#setResource(IBaseResource) instead 876 */ 877 @Deprecated 878 public List<ChargeItem> getPartOfTarget() { 879 if (this.partOfTarget == null) 880 this.partOfTarget = new ArrayList<ChargeItem>(); 881 return this.partOfTarget; 882 } 883 884 /** 885 * @deprecated Use Reference#setResource(IBaseResource) instead 886 */ 887 @Deprecated 888 public ChargeItem addPartOfTarget() { 889 ChargeItem r = new ChargeItem(); 890 if (this.partOfTarget == null) 891 this.partOfTarget = new ArrayList<ChargeItem>(); 892 this.partOfTarget.add(r); 893 return r; 894 } 895 896 /** 897 * @return {@link #code} (A code that identifies the charge, like a billing code.) 898 */ 899 public CodeableConcept getCode() { 900 if (this.code == null) 901 if (Configuration.errorOnAutoCreate()) 902 throw new Error("Attempt to auto-create ChargeItem.code"); 903 else if (Configuration.doAutoCreate()) 904 this.code = new CodeableConcept(); // cc 905 return this.code; 906 } 907 908 public boolean hasCode() { 909 return this.code != null && !this.code.isEmpty(); 910 } 911 912 /** 913 * @param value {@link #code} (A code that identifies the charge, like a billing code.) 914 */ 915 public ChargeItem setCode(CodeableConcept value) { 916 this.code = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #subject} (The individual or set of individuals the action is being or was performed on.) 922 */ 923 public Reference getSubject() { 924 if (this.subject == null) 925 if (Configuration.errorOnAutoCreate()) 926 throw new Error("Attempt to auto-create ChargeItem.subject"); 927 else if (Configuration.doAutoCreate()) 928 this.subject = new Reference(); // cc 929 return this.subject; 930 } 931 932 public boolean hasSubject() { 933 return this.subject != null && !this.subject.isEmpty(); 934 } 935 936 /** 937 * @param value {@link #subject} (The individual or set of individuals the action is being or was performed on.) 938 */ 939 public ChargeItem setSubject(Reference value) { 940 this.subject = value; 941 return this; 942 } 943 944 /** 945 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The individual or set of individuals the action is being or was performed on.) 946 */ 947 public Resource getSubjectTarget() { 948 return this.subjectTarget; 949 } 950 951 /** 952 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The individual or set of individuals the action is being or was performed on.) 953 */ 954 public ChargeItem setSubjectTarget(Resource value) { 955 this.subjectTarget = value; 956 return this; 957 } 958 959 /** 960 * @return {@link #context} (The encounter or episode of care that establishes the context for this event.) 961 */ 962 public Reference getContext() { 963 if (this.context == null) 964 if (Configuration.errorOnAutoCreate()) 965 throw new Error("Attempt to auto-create ChargeItem.context"); 966 else if (Configuration.doAutoCreate()) 967 this.context = new Reference(); // cc 968 return this.context; 969 } 970 971 public boolean hasContext() { 972 return this.context != null && !this.context.isEmpty(); 973 } 974 975 /** 976 * @param value {@link #context} (The encounter or episode of care that establishes the context for this event.) 977 */ 978 public ChargeItem setContext(Reference value) { 979 this.context = value; 980 return this; 981 } 982 983 /** 984 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this event.) 985 */ 986 public Resource getContextTarget() { 987 return this.contextTarget; 988 } 989 990 /** 991 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this event.) 992 */ 993 public ChargeItem setContextTarget(Resource value) { 994 this.contextTarget = value; 995 return this; 996 } 997 998 /** 999 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1000 */ 1001 public Type getOccurrence() { 1002 return this.occurrence; 1003 } 1004 1005 /** 1006 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1007 */ 1008 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1009 if (this.occurrence == null) 1010 return null; 1011 if (!(this.occurrence instanceof DateTimeType)) 1012 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1013 return (DateTimeType) this.occurrence; 1014 } 1015 1016 public boolean hasOccurrenceDateTimeType() { 1017 return this != null && this.occurrence instanceof DateTimeType; 1018 } 1019 1020 /** 1021 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1022 */ 1023 public Period getOccurrencePeriod() throws FHIRException { 1024 if (this.occurrence == null) 1025 return null; 1026 if (!(this.occurrence instanceof Period)) 1027 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1028 return (Period) this.occurrence; 1029 } 1030 1031 public boolean hasOccurrencePeriod() { 1032 return this != null && this.occurrence instanceof Period; 1033 } 1034 1035 /** 1036 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1037 */ 1038 public Timing getOccurrenceTiming() throws FHIRException { 1039 if (this.occurrence == null) 1040 return null; 1041 if (!(this.occurrence instanceof Timing)) 1042 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1043 return (Timing) this.occurrence; 1044 } 1045 1046 public boolean hasOccurrenceTiming() { 1047 return this != null && this.occurrence instanceof Timing; 1048 } 1049 1050 public boolean hasOccurrence() { 1051 return this.occurrence != null && !this.occurrence.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1056 */ 1057 public ChargeItem setOccurrence(Type value) throws FHIRFormatError { 1058 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1059 throw new FHIRFormatError("Not the right type for ChargeItem.occurrence[x]: "+value.fhirType()); 1060 this.occurrence = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return {@link #participant} (Indicates who or what performed or participated in the charged service.) 1066 */ 1067 public List<ChargeItemParticipantComponent> getParticipant() { 1068 if (this.participant == null) 1069 this.participant = new ArrayList<ChargeItemParticipantComponent>(); 1070 return this.participant; 1071 } 1072 1073 /** 1074 * @return Returns a reference to <code>this</code> for easy method chaining 1075 */ 1076 public ChargeItem setParticipant(List<ChargeItemParticipantComponent> theParticipant) { 1077 this.participant = theParticipant; 1078 return this; 1079 } 1080 1081 public boolean hasParticipant() { 1082 if (this.participant == null) 1083 return false; 1084 for (ChargeItemParticipantComponent item : this.participant) 1085 if (!item.isEmpty()) 1086 return true; 1087 return false; 1088 } 1089 1090 public ChargeItemParticipantComponent addParticipant() { //3 1091 ChargeItemParticipantComponent t = new ChargeItemParticipantComponent(); 1092 if (this.participant == null) 1093 this.participant = new ArrayList<ChargeItemParticipantComponent>(); 1094 this.participant.add(t); 1095 return t; 1096 } 1097 1098 public ChargeItem addParticipant(ChargeItemParticipantComponent t) { //3 1099 if (t == null) 1100 return this; 1101 if (this.participant == null) 1102 this.participant = new ArrayList<ChargeItemParticipantComponent>(); 1103 this.participant.add(t); 1104 return this; 1105 } 1106 1107 /** 1108 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 1109 */ 1110 public ChargeItemParticipantComponent getParticipantFirstRep() { 1111 if (getParticipant().isEmpty()) { 1112 addParticipant(); 1113 } 1114 return getParticipant().get(0); 1115 } 1116 1117 /** 1118 * @return {@link #performingOrganization} (The organization requesting the service.) 1119 */ 1120 public Reference getPerformingOrganization() { 1121 if (this.performingOrganization == null) 1122 if (Configuration.errorOnAutoCreate()) 1123 throw new Error("Attempt to auto-create ChargeItem.performingOrganization"); 1124 else if (Configuration.doAutoCreate()) 1125 this.performingOrganization = new Reference(); // cc 1126 return this.performingOrganization; 1127 } 1128 1129 public boolean hasPerformingOrganization() { 1130 return this.performingOrganization != null && !this.performingOrganization.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #performingOrganization} (The organization requesting the service.) 1135 */ 1136 public ChargeItem setPerformingOrganization(Reference value) { 1137 this.performingOrganization = value; 1138 return this; 1139 } 1140 1141 /** 1142 * @return {@link #performingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization requesting the service.) 1143 */ 1144 public Organization getPerformingOrganizationTarget() { 1145 if (this.performingOrganizationTarget == null) 1146 if (Configuration.errorOnAutoCreate()) 1147 throw new Error("Attempt to auto-create ChargeItem.performingOrganization"); 1148 else if (Configuration.doAutoCreate()) 1149 this.performingOrganizationTarget = new Organization(); // aa 1150 return this.performingOrganizationTarget; 1151 } 1152 1153 /** 1154 * @param value {@link #performingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization requesting the service.) 1155 */ 1156 public ChargeItem setPerformingOrganizationTarget(Organization value) { 1157 this.performingOrganizationTarget = value; 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #requestingOrganization} (The organization performing the service.) 1163 */ 1164 public Reference getRequestingOrganization() { 1165 if (this.requestingOrganization == null) 1166 if (Configuration.errorOnAutoCreate()) 1167 throw new Error("Attempt to auto-create ChargeItem.requestingOrganization"); 1168 else if (Configuration.doAutoCreate()) 1169 this.requestingOrganization = new Reference(); // cc 1170 return this.requestingOrganization; 1171 } 1172 1173 public boolean hasRequestingOrganization() { 1174 return this.requestingOrganization != null && !this.requestingOrganization.isEmpty(); 1175 } 1176 1177 /** 1178 * @param value {@link #requestingOrganization} (The organization performing the service.) 1179 */ 1180 public ChargeItem setRequestingOrganization(Reference value) { 1181 this.requestingOrganization = value; 1182 return this; 1183 } 1184 1185 /** 1186 * @return {@link #requestingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization performing the service.) 1187 */ 1188 public Organization getRequestingOrganizationTarget() { 1189 if (this.requestingOrganizationTarget == null) 1190 if (Configuration.errorOnAutoCreate()) 1191 throw new Error("Attempt to auto-create ChargeItem.requestingOrganization"); 1192 else if (Configuration.doAutoCreate()) 1193 this.requestingOrganizationTarget = new Organization(); // aa 1194 return this.requestingOrganizationTarget; 1195 } 1196 1197 /** 1198 * @param value {@link #requestingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization performing the service.) 1199 */ 1200 public ChargeItem setRequestingOrganizationTarget(Organization value) { 1201 this.requestingOrganizationTarget = value; 1202 return this; 1203 } 1204 1205 /** 1206 * @return {@link #quantity} (Quantity of which the charge item has been serviced.) 1207 */ 1208 public Quantity getQuantity() { 1209 if (this.quantity == null) 1210 if (Configuration.errorOnAutoCreate()) 1211 throw new Error("Attempt to auto-create ChargeItem.quantity"); 1212 else if (Configuration.doAutoCreate()) 1213 this.quantity = new Quantity(); // cc 1214 return this.quantity; 1215 } 1216 1217 public boolean hasQuantity() { 1218 return this.quantity != null && !this.quantity.isEmpty(); 1219 } 1220 1221 /** 1222 * @param value {@link #quantity} (Quantity of which the charge item has been serviced.) 1223 */ 1224 public ChargeItem setQuantity(Quantity value) { 1225 this.quantity = value; 1226 return this; 1227 } 1228 1229 /** 1230 * @return {@link #bodysite} (The anatomical location where the related service has been applied.) 1231 */ 1232 public List<CodeableConcept> getBodysite() { 1233 if (this.bodysite == null) 1234 this.bodysite = new ArrayList<CodeableConcept>(); 1235 return this.bodysite; 1236 } 1237 1238 /** 1239 * @return Returns a reference to <code>this</code> for easy method chaining 1240 */ 1241 public ChargeItem setBodysite(List<CodeableConcept> theBodysite) { 1242 this.bodysite = theBodysite; 1243 return this; 1244 } 1245 1246 public boolean hasBodysite() { 1247 if (this.bodysite == null) 1248 return false; 1249 for (CodeableConcept item : this.bodysite) 1250 if (!item.isEmpty()) 1251 return true; 1252 return false; 1253 } 1254 1255 public CodeableConcept addBodysite() { //3 1256 CodeableConcept t = new CodeableConcept(); 1257 if (this.bodysite == null) 1258 this.bodysite = new ArrayList<CodeableConcept>(); 1259 this.bodysite.add(t); 1260 return t; 1261 } 1262 1263 public ChargeItem addBodysite(CodeableConcept t) { //3 1264 if (t == null) 1265 return this; 1266 if (this.bodysite == null) 1267 this.bodysite = new ArrayList<CodeableConcept>(); 1268 this.bodysite.add(t); 1269 return this; 1270 } 1271 1272 /** 1273 * @return The first repetition of repeating field {@link #bodysite}, creating it if it does not already exist 1274 */ 1275 public CodeableConcept getBodysiteFirstRep() { 1276 if (getBodysite().isEmpty()) { 1277 addBodysite(); 1278 } 1279 return getBodysite().get(0); 1280 } 1281 1282 /** 1283 * @return {@link #factorOverride} (Factor overriding the factor determined by the rules associated with the code.). This is the underlying object with id, value and extensions. The accessor "getFactorOverride" gives direct access to the value 1284 */ 1285 public DecimalType getFactorOverrideElement() { 1286 if (this.factorOverride == null) 1287 if (Configuration.errorOnAutoCreate()) 1288 throw new Error("Attempt to auto-create ChargeItem.factorOverride"); 1289 else if (Configuration.doAutoCreate()) 1290 this.factorOverride = new DecimalType(); // bb 1291 return this.factorOverride; 1292 } 1293 1294 public boolean hasFactorOverrideElement() { 1295 return this.factorOverride != null && !this.factorOverride.isEmpty(); 1296 } 1297 1298 public boolean hasFactorOverride() { 1299 return this.factorOverride != null && !this.factorOverride.isEmpty(); 1300 } 1301 1302 /** 1303 * @param value {@link #factorOverride} (Factor overriding the factor determined by the rules associated with the code.). This is the underlying object with id, value and extensions. The accessor "getFactorOverride" gives direct access to the value 1304 */ 1305 public ChargeItem setFactorOverrideElement(DecimalType value) { 1306 this.factorOverride = value; 1307 return this; 1308 } 1309 1310 /** 1311 * @return Factor overriding the factor determined by the rules associated with the code. 1312 */ 1313 public BigDecimal getFactorOverride() { 1314 return this.factorOverride == null ? null : this.factorOverride.getValue(); 1315 } 1316 1317 /** 1318 * @param value Factor overriding the factor determined by the rules associated with the code. 1319 */ 1320 public ChargeItem setFactorOverride(BigDecimal value) { 1321 if (value == null) 1322 this.factorOverride = null; 1323 else { 1324 if (this.factorOverride == null) 1325 this.factorOverride = new DecimalType(); 1326 this.factorOverride.setValue(value); 1327 } 1328 return this; 1329 } 1330 1331 /** 1332 * @param value Factor overriding the factor determined by the rules associated with the code. 1333 */ 1334 public ChargeItem setFactorOverride(long value) { 1335 this.factorOverride = new DecimalType(); 1336 this.factorOverride.setValue(value); 1337 return this; 1338 } 1339 1340 /** 1341 * @param value Factor overriding the factor determined by the rules associated with the code. 1342 */ 1343 public ChargeItem setFactorOverride(double value) { 1344 this.factorOverride = new DecimalType(); 1345 this.factorOverride.setValue(value); 1346 return this; 1347 } 1348 1349 /** 1350 * @return {@link #priceOverride} (Total price of the charge overriding the list price associated with the code.) 1351 */ 1352 public Money getPriceOverride() { 1353 if (this.priceOverride == null) 1354 if (Configuration.errorOnAutoCreate()) 1355 throw new Error("Attempt to auto-create ChargeItem.priceOverride"); 1356 else if (Configuration.doAutoCreate()) 1357 this.priceOverride = new Money(); // cc 1358 return this.priceOverride; 1359 } 1360 1361 public boolean hasPriceOverride() { 1362 return this.priceOverride != null && !this.priceOverride.isEmpty(); 1363 } 1364 1365 /** 1366 * @param value {@link #priceOverride} (Total price of the charge overriding the list price associated with the code.) 1367 */ 1368 public ChargeItem setPriceOverride(Money value) { 1369 this.priceOverride = value; 1370 return this; 1371 } 1372 1373 /** 1374 * @return {@link #overrideReason} (If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.). This is the underlying object with id, value and extensions. The accessor "getOverrideReason" gives direct access to the value 1375 */ 1376 public StringType getOverrideReasonElement() { 1377 if (this.overrideReason == null) 1378 if (Configuration.errorOnAutoCreate()) 1379 throw new Error("Attempt to auto-create ChargeItem.overrideReason"); 1380 else if (Configuration.doAutoCreate()) 1381 this.overrideReason = new StringType(); // bb 1382 return this.overrideReason; 1383 } 1384 1385 public boolean hasOverrideReasonElement() { 1386 return this.overrideReason != null && !this.overrideReason.isEmpty(); 1387 } 1388 1389 public boolean hasOverrideReason() { 1390 return this.overrideReason != null && !this.overrideReason.isEmpty(); 1391 } 1392 1393 /** 1394 * @param value {@link #overrideReason} (If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.). This is the underlying object with id, value and extensions. The accessor "getOverrideReason" gives direct access to the value 1395 */ 1396 public ChargeItem setOverrideReasonElement(StringType value) { 1397 this.overrideReason = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action. 1403 */ 1404 public String getOverrideReason() { 1405 return this.overrideReason == null ? null : this.overrideReason.getValue(); 1406 } 1407 1408 /** 1409 * @param value If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action. 1410 */ 1411 public ChargeItem setOverrideReason(String value) { 1412 if (Utilities.noString(value)) 1413 this.overrideReason = null; 1414 else { 1415 if (this.overrideReason == null) 1416 this.overrideReason = new StringType(); 1417 this.overrideReason.setValue(value); 1418 } 1419 return this; 1420 } 1421 1422 /** 1423 * @return {@link #enterer} (The device, practitioner, etc. who entered the charge item.) 1424 */ 1425 public Reference getEnterer() { 1426 if (this.enterer == null) 1427 if (Configuration.errorOnAutoCreate()) 1428 throw new Error("Attempt to auto-create ChargeItem.enterer"); 1429 else if (Configuration.doAutoCreate()) 1430 this.enterer = new Reference(); // cc 1431 return this.enterer; 1432 } 1433 1434 public boolean hasEnterer() { 1435 return this.enterer != null && !this.enterer.isEmpty(); 1436 } 1437 1438 /** 1439 * @param value {@link #enterer} (The device, practitioner, etc. who entered the charge item.) 1440 */ 1441 public ChargeItem setEnterer(Reference value) { 1442 this.enterer = value; 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #enterer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who entered the charge item.) 1448 */ 1449 public Resource getEntererTarget() { 1450 return this.entererTarget; 1451 } 1452 1453 /** 1454 * @param value {@link #enterer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who entered the charge item.) 1455 */ 1456 public ChargeItem setEntererTarget(Resource value) { 1457 this.entererTarget = value; 1458 return this; 1459 } 1460 1461 /** 1462 * @return {@link #enteredDate} (Date the charge item was entered.). This is the underlying object with id, value and extensions. The accessor "getEnteredDate" gives direct access to the value 1463 */ 1464 public DateTimeType getEnteredDateElement() { 1465 if (this.enteredDate == null) 1466 if (Configuration.errorOnAutoCreate()) 1467 throw new Error("Attempt to auto-create ChargeItem.enteredDate"); 1468 else if (Configuration.doAutoCreate()) 1469 this.enteredDate = new DateTimeType(); // bb 1470 return this.enteredDate; 1471 } 1472 1473 public boolean hasEnteredDateElement() { 1474 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1475 } 1476 1477 public boolean hasEnteredDate() { 1478 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #enteredDate} (Date the charge item was entered.). This is the underlying object with id, value and extensions. The accessor "getEnteredDate" gives direct access to the value 1483 */ 1484 public ChargeItem setEnteredDateElement(DateTimeType value) { 1485 this.enteredDate = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return Date the charge item was entered. 1491 */ 1492 public Date getEnteredDate() { 1493 return this.enteredDate == null ? null : this.enteredDate.getValue(); 1494 } 1495 1496 /** 1497 * @param value Date the charge item was entered. 1498 */ 1499 public ChargeItem setEnteredDate(Date value) { 1500 if (value == null) 1501 this.enteredDate = null; 1502 else { 1503 if (this.enteredDate == null) 1504 this.enteredDate = new DateTimeType(); 1505 this.enteredDate.setValue(value); 1506 } 1507 return this; 1508 } 1509 1510 /** 1511 * @return {@link #reason} (Describes why the event occurred in coded or textual form.) 1512 */ 1513 public List<CodeableConcept> getReason() { 1514 if (this.reason == null) 1515 this.reason = new ArrayList<CodeableConcept>(); 1516 return this.reason; 1517 } 1518 1519 /** 1520 * @return Returns a reference to <code>this</code> for easy method chaining 1521 */ 1522 public ChargeItem setReason(List<CodeableConcept> theReason) { 1523 this.reason = theReason; 1524 return this; 1525 } 1526 1527 public boolean hasReason() { 1528 if (this.reason == null) 1529 return false; 1530 for (CodeableConcept item : this.reason) 1531 if (!item.isEmpty()) 1532 return true; 1533 return false; 1534 } 1535 1536 public CodeableConcept addReason() { //3 1537 CodeableConcept t = new CodeableConcept(); 1538 if (this.reason == null) 1539 this.reason = new ArrayList<CodeableConcept>(); 1540 this.reason.add(t); 1541 return t; 1542 } 1543 1544 public ChargeItem addReason(CodeableConcept t) { //3 1545 if (t == null) 1546 return this; 1547 if (this.reason == null) 1548 this.reason = new ArrayList<CodeableConcept>(); 1549 this.reason.add(t); 1550 return this; 1551 } 1552 1553 /** 1554 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 1555 */ 1556 public CodeableConcept getReasonFirstRep() { 1557 if (getReason().isEmpty()) { 1558 addReason(); 1559 } 1560 return getReason().get(0); 1561 } 1562 1563 /** 1564 * @return {@link #service} (Indicated the rendered service that caused this charge.) 1565 */ 1566 public List<Reference> getService() { 1567 if (this.service == null) 1568 this.service = new ArrayList<Reference>(); 1569 return this.service; 1570 } 1571 1572 /** 1573 * @return Returns a reference to <code>this</code> for easy method chaining 1574 */ 1575 public ChargeItem setService(List<Reference> theService) { 1576 this.service = theService; 1577 return this; 1578 } 1579 1580 public boolean hasService() { 1581 if (this.service == null) 1582 return false; 1583 for (Reference item : this.service) 1584 if (!item.isEmpty()) 1585 return true; 1586 return false; 1587 } 1588 1589 public Reference addService() { //3 1590 Reference t = new Reference(); 1591 if (this.service == null) 1592 this.service = new ArrayList<Reference>(); 1593 this.service.add(t); 1594 return t; 1595 } 1596 1597 public ChargeItem addService(Reference t) { //3 1598 if (t == null) 1599 return this; 1600 if (this.service == null) 1601 this.service = new ArrayList<Reference>(); 1602 this.service.add(t); 1603 return this; 1604 } 1605 1606 /** 1607 * @return The first repetition of repeating field {@link #service}, creating it if it does not already exist 1608 */ 1609 public Reference getServiceFirstRep() { 1610 if (getService().isEmpty()) { 1611 addService(); 1612 } 1613 return getService().get(0); 1614 } 1615 1616 /** 1617 * @deprecated Use Reference#setResource(IBaseResource) instead 1618 */ 1619 @Deprecated 1620 public List<Resource> getServiceTarget() { 1621 if (this.serviceTarget == null) 1622 this.serviceTarget = new ArrayList<Resource>(); 1623 return this.serviceTarget; 1624 } 1625 1626 /** 1627 * @return {@link #account} (Account into which this ChargeItems belongs.) 1628 */ 1629 public List<Reference> getAccount() { 1630 if (this.account == null) 1631 this.account = new ArrayList<Reference>(); 1632 return this.account; 1633 } 1634 1635 /** 1636 * @return Returns a reference to <code>this</code> for easy method chaining 1637 */ 1638 public ChargeItem setAccount(List<Reference> theAccount) { 1639 this.account = theAccount; 1640 return this; 1641 } 1642 1643 public boolean hasAccount() { 1644 if (this.account == null) 1645 return false; 1646 for (Reference item : this.account) 1647 if (!item.isEmpty()) 1648 return true; 1649 return false; 1650 } 1651 1652 public Reference addAccount() { //3 1653 Reference t = new Reference(); 1654 if (this.account == null) 1655 this.account = new ArrayList<Reference>(); 1656 this.account.add(t); 1657 return t; 1658 } 1659 1660 public ChargeItem addAccount(Reference t) { //3 1661 if (t == null) 1662 return this; 1663 if (this.account == null) 1664 this.account = new ArrayList<Reference>(); 1665 this.account.add(t); 1666 return this; 1667 } 1668 1669 /** 1670 * @return The first repetition of repeating field {@link #account}, creating it if it does not already exist 1671 */ 1672 public Reference getAccountFirstRep() { 1673 if (getAccount().isEmpty()) { 1674 addAccount(); 1675 } 1676 return getAccount().get(0); 1677 } 1678 1679 /** 1680 * @deprecated Use Reference#setResource(IBaseResource) instead 1681 */ 1682 @Deprecated 1683 public List<Account> getAccountTarget() { 1684 if (this.accountTarget == null) 1685 this.accountTarget = new ArrayList<Account>(); 1686 return this.accountTarget; 1687 } 1688 1689 /** 1690 * @deprecated Use Reference#setResource(IBaseResource) instead 1691 */ 1692 @Deprecated 1693 public Account addAccountTarget() { 1694 Account r = new Account(); 1695 if (this.accountTarget == null) 1696 this.accountTarget = new ArrayList<Account>(); 1697 this.accountTarget.add(r); 1698 return r; 1699 } 1700 1701 /** 1702 * @return {@link #note} (Comments made about the event by the performer, subject or other participants.) 1703 */ 1704 public List<Annotation> getNote() { 1705 if (this.note == null) 1706 this.note = new ArrayList<Annotation>(); 1707 return this.note; 1708 } 1709 1710 /** 1711 * @return Returns a reference to <code>this</code> for easy method chaining 1712 */ 1713 public ChargeItem setNote(List<Annotation> theNote) { 1714 this.note = theNote; 1715 return this; 1716 } 1717 1718 public boolean hasNote() { 1719 if (this.note == null) 1720 return false; 1721 for (Annotation item : this.note) 1722 if (!item.isEmpty()) 1723 return true; 1724 return false; 1725 } 1726 1727 public Annotation addNote() { //3 1728 Annotation t = new Annotation(); 1729 if (this.note == null) 1730 this.note = new ArrayList<Annotation>(); 1731 this.note.add(t); 1732 return t; 1733 } 1734 1735 public ChargeItem addNote(Annotation t) { //3 1736 if (t == null) 1737 return this; 1738 if (this.note == null) 1739 this.note = new ArrayList<Annotation>(); 1740 this.note.add(t); 1741 return this; 1742 } 1743 1744 /** 1745 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1746 */ 1747 public Annotation getNoteFirstRep() { 1748 if (getNote().isEmpty()) { 1749 addNote(); 1750 } 1751 return getNote().get(0); 1752 } 1753 1754 /** 1755 * @return {@link #supportingInformation} (Further information supporting the this charge.) 1756 */ 1757 public List<Reference> getSupportingInformation() { 1758 if (this.supportingInformation == null) 1759 this.supportingInformation = new ArrayList<Reference>(); 1760 return this.supportingInformation; 1761 } 1762 1763 /** 1764 * @return Returns a reference to <code>this</code> for easy method chaining 1765 */ 1766 public ChargeItem setSupportingInformation(List<Reference> theSupportingInformation) { 1767 this.supportingInformation = theSupportingInformation; 1768 return this; 1769 } 1770 1771 public boolean hasSupportingInformation() { 1772 if (this.supportingInformation == null) 1773 return false; 1774 for (Reference item : this.supportingInformation) 1775 if (!item.isEmpty()) 1776 return true; 1777 return false; 1778 } 1779 1780 public Reference addSupportingInformation() { //3 1781 Reference t = new Reference(); 1782 if (this.supportingInformation == null) 1783 this.supportingInformation = new ArrayList<Reference>(); 1784 this.supportingInformation.add(t); 1785 return t; 1786 } 1787 1788 public ChargeItem addSupportingInformation(Reference t) { //3 1789 if (t == null) 1790 return this; 1791 if (this.supportingInformation == null) 1792 this.supportingInformation = new ArrayList<Reference>(); 1793 this.supportingInformation.add(t); 1794 return this; 1795 } 1796 1797 /** 1798 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist 1799 */ 1800 public Reference getSupportingInformationFirstRep() { 1801 if (getSupportingInformation().isEmpty()) { 1802 addSupportingInformation(); 1803 } 1804 return getSupportingInformation().get(0); 1805 } 1806 1807 /** 1808 * @deprecated Use Reference#setResource(IBaseResource) instead 1809 */ 1810 @Deprecated 1811 public List<Resource> getSupportingInformationTarget() { 1812 if (this.supportingInformationTarget == null) 1813 this.supportingInformationTarget = new ArrayList<Resource>(); 1814 return this.supportingInformationTarget; 1815 } 1816 1817 protected void listChildren(List<Property> children) { 1818 super.listChildren(children); 1819 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this event performer or other systems.", 0, 1, identifier)); 1820 children.add(new Property("definition", "uri", "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, java.lang.Integer.MAX_VALUE, definition)); 1821 children.add(new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status)); 1822 children.add(new Property("partOf", "Reference(ChargeItem)", "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1823 children.add(new Property("code", "CodeableConcept", "A code that identifies the charge, like a billing code.", 0, 1, code)); 1824 children.add(new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals the action is being or was performed on.", 0, 1, subject)); 1825 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this event.", 0, 1, context)); 1826 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence)); 1827 children.add(new Property("participant", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, participant)); 1828 children.add(new Property("performingOrganization", "Reference(Organization)", "The organization requesting the service.", 0, 1, performingOrganization)); 1829 children.add(new Property("requestingOrganization", "Reference(Organization)", "The organization performing the service.", 0, 1, requestingOrganization)); 1830 children.add(new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 0, 1, quantity)); 1831 children.add(new Property("bodysite", "CodeableConcept", "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, bodysite)); 1832 children.add(new Property("factorOverride", "decimal", "Factor overriding the factor determined by the rules associated with the code.", 0, 1, factorOverride)); 1833 children.add(new Property("priceOverride", "Money", "Total price of the charge overriding the list price associated with the code.", 0, 1, priceOverride)); 1834 children.add(new Property("overrideReason", "string", "If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 0, 1, overrideReason)); 1835 children.add(new Property("enterer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer)); 1836 children.add(new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, enteredDate)); 1837 children.add(new Property("reason", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason)); 1838 children.add(new Property("service", "Reference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|Observation|Procedure|SupplyDelivery)", "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service)); 1839 children.add(new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 0, java.lang.Integer.MAX_VALUE, account)); 1840 children.add(new Property("note", "Annotation", "Comments made about the event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 1841 children.add(new Property("supportingInformation", "Reference(Any)", "Further information supporting the this charge.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 1842 } 1843 1844 @Override 1845 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1846 switch (_hash) { 1847 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this event performer or other systems.", 0, 1, identifier); 1848 case -1014418093: /*definition*/ return new Property("definition", "uri", "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, java.lang.Integer.MAX_VALUE, definition); 1849 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status); 1850 case -995410646: /*partOf*/ return new Property("partOf", "Reference(ChargeItem)", "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, partOf); 1851 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies the charge, like a billing code.", 0, 1, code); 1852 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals the action is being or was performed on.", 0, 1, subject); 1853 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this event.", 0, 1, context); 1854 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1855 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1856 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1857 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1858 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1859 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, participant); 1860 case 1273192628: /*performingOrganization*/ return new Property("performingOrganization", "Reference(Organization)", "The organization requesting the service.", 0, 1, performingOrganization); 1861 case 1279054790: /*requestingOrganization*/ return new Property("requestingOrganization", "Reference(Organization)", "The organization performing the service.", 0, 1, requestingOrganization); 1862 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 0, 1, quantity); 1863 case 1703573481: /*bodysite*/ return new Property("bodysite", "CodeableConcept", "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, bodysite); 1864 case -451233221: /*factorOverride*/ return new Property("factorOverride", "decimal", "Factor overriding the factor determined by the rules associated with the code.", 0, 1, factorOverride); 1865 case -216803275: /*priceOverride*/ return new Property("priceOverride", "Money", "Total price of the charge overriding the list price associated with the code.", 0, 1, priceOverride); 1866 case -742878928: /*overrideReason*/ return new Property("overrideReason", "string", "If the list price or the rule based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 0, 1, overrideReason); 1867 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer); 1868 case 555978181: /*enteredDate*/ return new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, enteredDate); 1869 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason); 1870 case 1984153269: /*service*/ return new Property("service", "Reference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|Observation|Procedure|SupplyDelivery)", "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service); 1871 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 0, java.lang.Integer.MAX_VALUE, account); 1872 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 1873 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Further information supporting the this charge.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 1874 default: return super.getNamedProperty(_hash, _name, _checkValid); 1875 } 1876 1877 } 1878 1879 @Override 1880 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1881 switch (hash) { 1882 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1883 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // UriType 1884 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ChargeItemStatus> 1885 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1886 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1887 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1888 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1889 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 1890 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // ChargeItemParticipantComponent 1891 case 1273192628: /*performingOrganization*/ return this.performingOrganization == null ? new Base[0] : new Base[] {this.performingOrganization}; // Reference 1892 case 1279054790: /*requestingOrganization*/ return this.requestingOrganization == null ? new Base[0] : new Base[] {this.requestingOrganization}; // Reference 1893 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1894 case 1703573481: /*bodysite*/ return this.bodysite == null ? new Base[0] : this.bodysite.toArray(new Base[this.bodysite.size()]); // CodeableConcept 1895 case -451233221: /*factorOverride*/ return this.factorOverride == null ? new Base[0] : new Base[] {this.factorOverride}; // DecimalType 1896 case -216803275: /*priceOverride*/ return this.priceOverride == null ? new Base[0] : new Base[] {this.priceOverride}; // Money 1897 case -742878928: /*overrideReason*/ return this.overrideReason == null ? new Base[0] : new Base[] {this.overrideReason}; // StringType 1898 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 1899 case 555978181: /*enteredDate*/ return this.enteredDate == null ? new Base[0] : new Base[] {this.enteredDate}; // DateTimeType 1900 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 1901 case 1984153269: /*service*/ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // Reference 1902 case -1177318867: /*account*/ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 1903 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1904 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 1905 default: return super.getProperty(hash, name, checkValid); 1906 } 1907 1908 } 1909 1910 @Override 1911 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1912 switch (hash) { 1913 case -1618432855: // identifier 1914 this.identifier = castToIdentifier(value); // Identifier 1915 return value; 1916 case -1014418093: // definition 1917 this.getDefinition().add(castToUri(value)); // UriType 1918 return value; 1919 case -892481550: // status 1920 value = new ChargeItemStatusEnumFactory().fromType(castToCode(value)); 1921 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 1922 return value; 1923 case -995410646: // partOf 1924 this.getPartOf().add(castToReference(value)); // Reference 1925 return value; 1926 case 3059181: // code 1927 this.code = castToCodeableConcept(value); // CodeableConcept 1928 return value; 1929 case -1867885268: // subject 1930 this.subject = castToReference(value); // Reference 1931 return value; 1932 case 951530927: // context 1933 this.context = castToReference(value); // Reference 1934 return value; 1935 case 1687874001: // occurrence 1936 this.occurrence = castToType(value); // Type 1937 return value; 1938 case 767422259: // participant 1939 this.getParticipant().add((ChargeItemParticipantComponent) value); // ChargeItemParticipantComponent 1940 return value; 1941 case 1273192628: // performingOrganization 1942 this.performingOrganization = castToReference(value); // Reference 1943 return value; 1944 case 1279054790: // requestingOrganization 1945 this.requestingOrganization = castToReference(value); // Reference 1946 return value; 1947 case -1285004149: // quantity 1948 this.quantity = castToQuantity(value); // Quantity 1949 return value; 1950 case 1703573481: // bodysite 1951 this.getBodysite().add(castToCodeableConcept(value)); // CodeableConcept 1952 return value; 1953 case -451233221: // factorOverride 1954 this.factorOverride = castToDecimal(value); // DecimalType 1955 return value; 1956 case -216803275: // priceOverride 1957 this.priceOverride = castToMoney(value); // Money 1958 return value; 1959 case -742878928: // overrideReason 1960 this.overrideReason = castToString(value); // StringType 1961 return value; 1962 case -1591951995: // enterer 1963 this.enterer = castToReference(value); // Reference 1964 return value; 1965 case 555978181: // enteredDate 1966 this.enteredDate = castToDateTime(value); // DateTimeType 1967 return value; 1968 case -934964668: // reason 1969 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 1970 return value; 1971 case 1984153269: // service 1972 this.getService().add(castToReference(value)); // Reference 1973 return value; 1974 case -1177318867: // account 1975 this.getAccount().add(castToReference(value)); // Reference 1976 return value; 1977 case 3387378: // note 1978 this.getNote().add(castToAnnotation(value)); // Annotation 1979 return value; 1980 case -1248768647: // supportingInformation 1981 this.getSupportingInformation().add(castToReference(value)); // Reference 1982 return value; 1983 default: return super.setProperty(hash, name, value); 1984 } 1985 1986 } 1987 1988 @Override 1989 public Base setProperty(String name, Base value) throws FHIRException { 1990 if (name.equals("identifier")) { 1991 this.identifier = castToIdentifier(value); // Identifier 1992 } else if (name.equals("definition")) { 1993 this.getDefinition().add(castToUri(value)); 1994 } else if (name.equals("status")) { 1995 value = new ChargeItemStatusEnumFactory().fromType(castToCode(value)); 1996 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 1997 } else if (name.equals("partOf")) { 1998 this.getPartOf().add(castToReference(value)); 1999 } else if (name.equals("code")) { 2000 this.code = castToCodeableConcept(value); // CodeableConcept 2001 } else if (name.equals("subject")) { 2002 this.subject = castToReference(value); // Reference 2003 } else if (name.equals("context")) { 2004 this.context = castToReference(value); // Reference 2005 } else if (name.equals("occurrence[x]")) { 2006 this.occurrence = castToType(value); // Type 2007 } else if (name.equals("participant")) { 2008 this.getParticipant().add((ChargeItemParticipantComponent) value); 2009 } else if (name.equals("performingOrganization")) { 2010 this.performingOrganization = castToReference(value); // Reference 2011 } else if (name.equals("requestingOrganization")) { 2012 this.requestingOrganization = castToReference(value); // Reference 2013 } else if (name.equals("quantity")) { 2014 this.quantity = castToQuantity(value); // Quantity 2015 } else if (name.equals("bodysite")) { 2016 this.getBodysite().add(castToCodeableConcept(value)); 2017 } else if (name.equals("factorOverride")) { 2018 this.factorOverride = castToDecimal(value); // DecimalType 2019 } else if (name.equals("priceOverride")) { 2020 this.priceOverride = castToMoney(value); // Money 2021 } else if (name.equals("overrideReason")) { 2022 this.overrideReason = castToString(value); // StringType 2023 } else if (name.equals("enterer")) { 2024 this.enterer = castToReference(value); // Reference 2025 } else if (name.equals("enteredDate")) { 2026 this.enteredDate = castToDateTime(value); // DateTimeType 2027 } else if (name.equals("reason")) { 2028 this.getReason().add(castToCodeableConcept(value)); 2029 } else if (name.equals("service")) { 2030 this.getService().add(castToReference(value)); 2031 } else if (name.equals("account")) { 2032 this.getAccount().add(castToReference(value)); 2033 } else if (name.equals("note")) { 2034 this.getNote().add(castToAnnotation(value)); 2035 } else if (name.equals("supportingInformation")) { 2036 this.getSupportingInformation().add(castToReference(value)); 2037 } else 2038 return super.setProperty(name, value); 2039 return value; 2040 } 2041 2042 @Override 2043 public Base makeProperty(int hash, String name) throws FHIRException { 2044 switch (hash) { 2045 case -1618432855: return getIdentifier(); 2046 case -1014418093: return addDefinitionElement(); 2047 case -892481550: return getStatusElement(); 2048 case -995410646: return addPartOf(); 2049 case 3059181: return getCode(); 2050 case -1867885268: return getSubject(); 2051 case 951530927: return getContext(); 2052 case -2022646513: return getOccurrence(); 2053 case 1687874001: return getOccurrence(); 2054 case 767422259: return addParticipant(); 2055 case 1273192628: return getPerformingOrganization(); 2056 case 1279054790: return getRequestingOrganization(); 2057 case -1285004149: return getQuantity(); 2058 case 1703573481: return addBodysite(); 2059 case -451233221: return getFactorOverrideElement(); 2060 case -216803275: return getPriceOverride(); 2061 case -742878928: return getOverrideReasonElement(); 2062 case -1591951995: return getEnterer(); 2063 case 555978181: return getEnteredDateElement(); 2064 case -934964668: return addReason(); 2065 case 1984153269: return addService(); 2066 case -1177318867: return addAccount(); 2067 case 3387378: return addNote(); 2068 case -1248768647: return addSupportingInformation(); 2069 default: return super.makeProperty(hash, name); 2070 } 2071 2072 } 2073 2074 @Override 2075 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2076 switch (hash) { 2077 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2078 case -1014418093: /*definition*/ return new String[] {"uri"}; 2079 case -892481550: /*status*/ return new String[] {"code"}; 2080 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2081 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2082 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2083 case 951530927: /*context*/ return new String[] {"Reference"}; 2084 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 2085 case 767422259: /*participant*/ return new String[] {}; 2086 case 1273192628: /*performingOrganization*/ return new String[] {"Reference"}; 2087 case 1279054790: /*requestingOrganization*/ return new String[] {"Reference"}; 2088 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 2089 case 1703573481: /*bodysite*/ return new String[] {"CodeableConcept"}; 2090 case -451233221: /*factorOverride*/ return new String[] {"decimal"}; 2091 case -216803275: /*priceOverride*/ return new String[] {"Money"}; 2092 case -742878928: /*overrideReason*/ return new String[] {"string"}; 2093 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 2094 case 555978181: /*enteredDate*/ return new String[] {"dateTime"}; 2095 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 2096 case 1984153269: /*service*/ return new String[] {"Reference"}; 2097 case -1177318867: /*account*/ return new String[] {"Reference"}; 2098 case 3387378: /*note*/ return new String[] {"Annotation"}; 2099 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 2100 default: return super.getTypesForProperty(hash, name); 2101 } 2102 2103 } 2104 2105 @Override 2106 public Base addChild(String name) throws FHIRException { 2107 if (name.equals("identifier")) { 2108 this.identifier = new Identifier(); 2109 return this.identifier; 2110 } 2111 else if (name.equals("definition")) { 2112 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.definition"); 2113 } 2114 else if (name.equals("status")) { 2115 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.status"); 2116 } 2117 else if (name.equals("partOf")) { 2118 return addPartOf(); 2119 } 2120 else if (name.equals("code")) { 2121 this.code = new CodeableConcept(); 2122 return this.code; 2123 } 2124 else if (name.equals("subject")) { 2125 this.subject = new Reference(); 2126 return this.subject; 2127 } 2128 else if (name.equals("context")) { 2129 this.context = new Reference(); 2130 return this.context; 2131 } 2132 else if (name.equals("occurrenceDateTime")) { 2133 this.occurrence = new DateTimeType(); 2134 return this.occurrence; 2135 } 2136 else if (name.equals("occurrencePeriod")) { 2137 this.occurrence = new Period(); 2138 return this.occurrence; 2139 } 2140 else if (name.equals("occurrenceTiming")) { 2141 this.occurrence = new Timing(); 2142 return this.occurrence; 2143 } 2144 else if (name.equals("participant")) { 2145 return addParticipant(); 2146 } 2147 else if (name.equals("performingOrganization")) { 2148 this.performingOrganization = new Reference(); 2149 return this.performingOrganization; 2150 } 2151 else if (name.equals("requestingOrganization")) { 2152 this.requestingOrganization = new Reference(); 2153 return this.requestingOrganization; 2154 } 2155 else if (name.equals("quantity")) { 2156 this.quantity = new Quantity(); 2157 return this.quantity; 2158 } 2159 else if (name.equals("bodysite")) { 2160 return addBodysite(); 2161 } 2162 else if (name.equals("factorOverride")) { 2163 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.factorOverride"); 2164 } 2165 else if (name.equals("priceOverride")) { 2166 this.priceOverride = new Money(); 2167 return this.priceOverride; 2168 } 2169 else if (name.equals("overrideReason")) { 2170 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.overrideReason"); 2171 } 2172 else if (name.equals("enterer")) { 2173 this.enterer = new Reference(); 2174 return this.enterer; 2175 } 2176 else if (name.equals("enteredDate")) { 2177 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.enteredDate"); 2178 } 2179 else if (name.equals("reason")) { 2180 return addReason(); 2181 } 2182 else if (name.equals("service")) { 2183 return addService(); 2184 } 2185 else if (name.equals("account")) { 2186 return addAccount(); 2187 } 2188 else if (name.equals("note")) { 2189 return addNote(); 2190 } 2191 else if (name.equals("supportingInformation")) { 2192 return addSupportingInformation(); 2193 } 2194 else 2195 return super.addChild(name); 2196 } 2197 2198 public String fhirType() { 2199 return "ChargeItem"; 2200 2201 } 2202 2203 public ChargeItem copy() { 2204 ChargeItem dst = new ChargeItem(); 2205 copyValues(dst); 2206 dst.identifier = identifier == null ? null : identifier.copy(); 2207 if (definition != null) { 2208 dst.definition = new ArrayList<UriType>(); 2209 for (UriType i : definition) 2210 dst.definition.add(i.copy()); 2211 }; 2212 dst.status = status == null ? null : status.copy(); 2213 if (partOf != null) { 2214 dst.partOf = new ArrayList<Reference>(); 2215 for (Reference i : partOf) 2216 dst.partOf.add(i.copy()); 2217 }; 2218 dst.code = code == null ? null : code.copy(); 2219 dst.subject = subject == null ? null : subject.copy(); 2220 dst.context = context == null ? null : context.copy(); 2221 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2222 if (participant != null) { 2223 dst.participant = new ArrayList<ChargeItemParticipantComponent>(); 2224 for (ChargeItemParticipantComponent i : participant) 2225 dst.participant.add(i.copy()); 2226 }; 2227 dst.performingOrganization = performingOrganization == null ? null : performingOrganization.copy(); 2228 dst.requestingOrganization = requestingOrganization == null ? null : requestingOrganization.copy(); 2229 dst.quantity = quantity == null ? null : quantity.copy(); 2230 if (bodysite != null) { 2231 dst.bodysite = new ArrayList<CodeableConcept>(); 2232 for (CodeableConcept i : bodysite) 2233 dst.bodysite.add(i.copy()); 2234 }; 2235 dst.factorOverride = factorOverride == null ? null : factorOverride.copy(); 2236 dst.priceOverride = priceOverride == null ? null : priceOverride.copy(); 2237 dst.overrideReason = overrideReason == null ? null : overrideReason.copy(); 2238 dst.enterer = enterer == null ? null : enterer.copy(); 2239 dst.enteredDate = enteredDate == null ? null : enteredDate.copy(); 2240 if (reason != null) { 2241 dst.reason = new ArrayList<CodeableConcept>(); 2242 for (CodeableConcept i : reason) 2243 dst.reason.add(i.copy()); 2244 }; 2245 if (service != null) { 2246 dst.service = new ArrayList<Reference>(); 2247 for (Reference i : service) 2248 dst.service.add(i.copy()); 2249 }; 2250 if (account != null) { 2251 dst.account = new ArrayList<Reference>(); 2252 for (Reference i : account) 2253 dst.account.add(i.copy()); 2254 }; 2255 if (note != null) { 2256 dst.note = new ArrayList<Annotation>(); 2257 for (Annotation i : note) 2258 dst.note.add(i.copy()); 2259 }; 2260 if (supportingInformation != null) { 2261 dst.supportingInformation = new ArrayList<Reference>(); 2262 for (Reference i : supportingInformation) 2263 dst.supportingInformation.add(i.copy()); 2264 }; 2265 return dst; 2266 } 2267 2268 protected ChargeItem typedCopy() { 2269 return copy(); 2270 } 2271 2272 @Override 2273 public boolean equalsDeep(Base other_) { 2274 if (!super.equalsDeep(other_)) 2275 return false; 2276 if (!(other_ instanceof ChargeItem)) 2277 return false; 2278 ChargeItem o = (ChargeItem) other_; 2279 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2280 && compareDeep(status, o.status, true) && compareDeep(partOf, o.partOf, true) && compareDeep(code, o.code, true) 2281 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(occurrence, o.occurrence, true) 2282 && compareDeep(participant, o.participant, true) && compareDeep(performingOrganization, o.performingOrganization, true) 2283 && compareDeep(requestingOrganization, o.requestingOrganization, true) && compareDeep(quantity, o.quantity, true) 2284 && compareDeep(bodysite, o.bodysite, true) && compareDeep(factorOverride, o.factorOverride, true) 2285 && compareDeep(priceOverride, o.priceOverride, true) && compareDeep(overrideReason, o.overrideReason, true) 2286 && compareDeep(enterer, o.enterer, true) && compareDeep(enteredDate, o.enteredDate, true) && compareDeep(reason, o.reason, true) 2287 && compareDeep(service, o.service, true) && compareDeep(account, o.account, true) && compareDeep(note, o.note, true) 2288 && compareDeep(supportingInformation, o.supportingInformation, true); 2289 } 2290 2291 @Override 2292 public boolean equalsShallow(Base other_) { 2293 if (!super.equalsShallow(other_)) 2294 return false; 2295 if (!(other_ instanceof ChargeItem)) 2296 return false; 2297 ChargeItem o = (ChargeItem) other_; 2298 return compareValues(definition, o.definition, true) && compareValues(status, o.status, true) && compareValues(factorOverride, o.factorOverride, true) 2299 && compareValues(overrideReason, o.overrideReason, true) && compareValues(enteredDate, o.enteredDate, true) 2300 ; 2301 } 2302 2303 public boolean isEmpty() { 2304 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, status 2305 , partOf, code, subject, context, occurrence, participant, performingOrganization 2306 , requestingOrganization, quantity, bodysite, factorOverride, priceOverride, overrideReason 2307 , enterer, enteredDate, reason, service, account, note, supportingInformation 2308 ); 2309 } 2310 2311 @Override 2312 public ResourceType getResourceType() { 2313 return ResourceType.ChargeItem; 2314 } 2315 2316 /** 2317 * Search parameter: <b>identifier</b> 2318 * <p> 2319 * Description: <b>Business Identifier for item</b><br> 2320 * Type: <b>token</b><br> 2321 * Path: <b>ChargeItem.identifier</b><br> 2322 * </p> 2323 */ 2324 @SearchParamDefinition(name="identifier", path="ChargeItem.identifier", description="Business Identifier for item", type="token" ) 2325 public static final String SP_IDENTIFIER = "identifier"; 2326 /** 2327 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2328 * <p> 2329 * Description: <b>Business Identifier for item</b><br> 2330 * Type: <b>token</b><br> 2331 * Path: <b>ChargeItem.identifier</b><br> 2332 * </p> 2333 */ 2334 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2335 2336 /** 2337 * Search parameter: <b>performing-organization</b> 2338 * <p> 2339 * Description: <b>Organization providing the charged sevice</b><br> 2340 * Type: <b>reference</b><br> 2341 * Path: <b>ChargeItem.performingOrganization</b><br> 2342 * </p> 2343 */ 2344 @SearchParamDefinition(name="performing-organization", path="ChargeItem.performingOrganization", description="Organization providing the charged sevice", type="reference", target={Organization.class } ) 2345 public static final String SP_PERFORMING_ORGANIZATION = "performing-organization"; 2346 /** 2347 * <b>Fluent Client</b> search parameter constant for <b>performing-organization</b> 2348 * <p> 2349 * Description: <b>Organization providing the charged sevice</b><br> 2350 * Type: <b>reference</b><br> 2351 * Path: <b>ChargeItem.performingOrganization</b><br> 2352 * </p> 2353 */ 2354 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMING_ORGANIZATION); 2355 2356/** 2357 * Constant for fluent queries to be used to add include statements. Specifies 2358 * the path value of "<b>ChargeItem:performing-organization</b>". 2359 */ 2360 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMING_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ChargeItem:performing-organization").toLocked(); 2361 2362 /** 2363 * Search parameter: <b>code</b> 2364 * <p> 2365 * Description: <b>A code that identifies the charge, like a billing code</b><br> 2366 * Type: <b>token</b><br> 2367 * Path: <b>ChargeItem.code</b><br> 2368 * </p> 2369 */ 2370 @SearchParamDefinition(name="code", path="ChargeItem.code", description="A code that identifies the charge, like a billing code", type="token" ) 2371 public static final String SP_CODE = "code"; 2372 /** 2373 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2374 * <p> 2375 * Description: <b>A code that identifies the charge, like a billing code</b><br> 2376 * Type: <b>token</b><br> 2377 * Path: <b>ChargeItem.code</b><br> 2378 * </p> 2379 */ 2380 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2381 2382 /** 2383 * Search parameter: <b>quantity</b> 2384 * <p> 2385 * Description: <b>Quantity of which the charge item has been serviced</b><br> 2386 * Type: <b>quantity</b><br> 2387 * Path: <b>ChargeItem.quantity</b><br> 2388 * </p> 2389 */ 2390 @SearchParamDefinition(name="quantity", path="ChargeItem.quantity", description="Quantity of which the charge item has been serviced", type="quantity" ) 2391 public static final String SP_QUANTITY = "quantity"; 2392 /** 2393 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 2394 * <p> 2395 * Description: <b>Quantity of which the charge item has been serviced</b><br> 2396 * Type: <b>quantity</b><br> 2397 * Path: <b>ChargeItem.quantity</b><br> 2398 * </p> 2399 */ 2400 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_QUANTITY); 2401 2402 /** 2403 * Search parameter: <b>subject</b> 2404 * <p> 2405 * Description: <b>Individual service was done for/to</b><br> 2406 * Type: <b>reference</b><br> 2407 * Path: <b>ChargeItem.subject</b><br> 2408 * </p> 2409 */ 2410 @SearchParamDefinition(name="subject", path="ChargeItem.subject", description="Individual service was done for/to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2411 public static final String SP_SUBJECT = "subject"; 2412 /** 2413 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2414 * <p> 2415 * Description: <b>Individual service was done for/to</b><br> 2416 * Type: <b>reference</b><br> 2417 * Path: <b>ChargeItem.subject</b><br> 2418 * </p> 2419 */ 2420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2421 2422/** 2423 * Constant for fluent queries to be used to add include statements. Specifies 2424 * the path value of "<b>ChargeItem:subject</b>". 2425 */ 2426 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ChargeItem:subject").toLocked(); 2427 2428 /** 2429 * Search parameter: <b>participant-role</b> 2430 * <p> 2431 * Description: <b>What type of performance was done</b><br> 2432 * Type: <b>token</b><br> 2433 * Path: <b>ChargeItem.participant.role</b><br> 2434 * </p> 2435 */ 2436 @SearchParamDefinition(name="participant-role", path="ChargeItem.participant.role", description="What type of performance was done", type="token" ) 2437 public static final String SP_PARTICIPANT_ROLE = "participant-role"; 2438 /** 2439 * <b>Fluent Client</b> search parameter constant for <b>participant-role</b> 2440 * <p> 2441 * Description: <b>What type of performance was done</b><br> 2442 * Type: <b>token</b><br> 2443 * Path: <b>ChargeItem.participant.role</b><br> 2444 * </p> 2445 */ 2446 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PARTICIPANT_ROLE); 2447 2448 /** 2449 * Search parameter: <b>participant-actor</b> 2450 * <p> 2451 * Description: <b>Individual who was performing</b><br> 2452 * Type: <b>reference</b><br> 2453 * Path: <b>ChargeItem.participant.actor</b><br> 2454 * </p> 2455 */ 2456 @SearchParamDefinition(name="participant-actor", path="ChargeItem.participant.actor", description="Individual who was performing", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2457 public static final String SP_PARTICIPANT_ACTOR = "participant-actor"; 2458 /** 2459 * <b>Fluent Client</b> search parameter constant for <b>participant-actor</b> 2460 * <p> 2461 * Description: <b>Individual who was performing</b><br> 2462 * Type: <b>reference</b><br> 2463 * Path: <b>ChargeItem.participant.actor</b><br> 2464 * </p> 2465 */ 2466 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT_ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT_ACTOR); 2467 2468/** 2469 * Constant for fluent queries to be used to add include statements. Specifies 2470 * the path value of "<b>ChargeItem:participant-actor</b>". 2471 */ 2472 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT_ACTOR = new ca.uhn.fhir.model.api.Include("ChargeItem:participant-actor").toLocked(); 2473 2474 /** 2475 * Search parameter: <b>occurrence</b> 2476 * <p> 2477 * Description: <b>When the charged service was applied</b><br> 2478 * Type: <b>date</b><br> 2479 * Path: <b>ChargeItem.occurrence[x]</b><br> 2480 * </p> 2481 */ 2482 @SearchParamDefinition(name="occurrence", path="ChargeItem.occurrence", description="When the charged service was applied", type="date" ) 2483 public static final String SP_OCCURRENCE = "occurrence"; 2484 /** 2485 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 2486 * <p> 2487 * Description: <b>When the charged service was applied</b><br> 2488 * Type: <b>date</b><br> 2489 * Path: <b>ChargeItem.occurrence[x]</b><br> 2490 * </p> 2491 */ 2492 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE); 2493 2494 /** 2495 * Search parameter: <b>entered-date</b> 2496 * <p> 2497 * Description: <b>Date the charge item was entered</b><br> 2498 * Type: <b>date</b><br> 2499 * Path: <b>ChargeItem.enteredDate</b><br> 2500 * </p> 2501 */ 2502 @SearchParamDefinition(name="entered-date", path="ChargeItem.enteredDate", description="Date the charge item was entered", type="date" ) 2503 public static final String SP_ENTERED_DATE = "entered-date"; 2504 /** 2505 * <b>Fluent Client</b> search parameter constant for <b>entered-date</b> 2506 * <p> 2507 * Description: <b>Date the charge item was entered</b><br> 2508 * Type: <b>date</b><br> 2509 * Path: <b>ChargeItem.enteredDate</b><br> 2510 * </p> 2511 */ 2512 public static final ca.uhn.fhir.rest.gclient.DateClientParam ENTERED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ENTERED_DATE); 2513 2514 /** 2515 * Search parameter: <b>patient</b> 2516 * <p> 2517 * Description: <b>Individual service was done for/to</b><br> 2518 * Type: <b>reference</b><br> 2519 * Path: <b>ChargeItem.subject</b><br> 2520 * </p> 2521 */ 2522 @SearchParamDefinition(name="patient", path="ChargeItem.subject", description="Individual service was done for/to", type="reference", target={Patient.class } ) 2523 public static final String SP_PATIENT = "patient"; 2524 /** 2525 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2526 * <p> 2527 * Description: <b>Individual service was done for/to</b><br> 2528 * Type: <b>reference</b><br> 2529 * Path: <b>ChargeItem.subject</b><br> 2530 * </p> 2531 */ 2532 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2533 2534/** 2535 * Constant for fluent queries to be used to add include statements. Specifies 2536 * the path value of "<b>ChargeItem:patient</b>". 2537 */ 2538 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ChargeItem:patient").toLocked(); 2539 2540 /** 2541 * Search parameter: <b>factor-override</b> 2542 * <p> 2543 * Description: <b>Factor overriding the associated rules</b><br> 2544 * Type: <b>number</b><br> 2545 * Path: <b>ChargeItem.factorOverride</b><br> 2546 * </p> 2547 */ 2548 @SearchParamDefinition(name="factor-override", path="ChargeItem.factorOverride", description="Factor overriding the associated rules", type="number" ) 2549 public static final String SP_FACTOR_OVERRIDE = "factor-override"; 2550 /** 2551 * <b>Fluent Client</b> search parameter constant for <b>factor-override</b> 2552 * <p> 2553 * Description: <b>Factor overriding the associated rules</b><br> 2554 * Type: <b>number</b><br> 2555 * Path: <b>ChargeItem.factorOverride</b><br> 2556 * </p> 2557 */ 2558 public static final ca.uhn.fhir.rest.gclient.NumberClientParam FACTOR_OVERRIDE = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_FACTOR_OVERRIDE); 2559 2560 /** 2561 * Search parameter: <b>service</b> 2562 * <p> 2563 * Description: <b>Which rendered service is being charged?</b><br> 2564 * Type: <b>reference</b><br> 2565 * Path: <b>ChargeItem.service</b><br> 2566 * </p> 2567 */ 2568 @SearchParamDefinition(name="service", path="ChargeItem.service", description="Which rendered service is being charged?", type="reference", target={DiagnosticReport.class, ImagingStudy.class, Immunization.class, MedicationAdministration.class, MedicationDispense.class, Observation.class, Procedure.class, SupplyDelivery.class } ) 2569 public static final String SP_SERVICE = "service"; 2570 /** 2571 * <b>Fluent Client</b> search parameter constant for <b>service</b> 2572 * <p> 2573 * Description: <b>Which rendered service is being charged?</b><br> 2574 * Type: <b>reference</b><br> 2575 * Path: <b>ChargeItem.service</b><br> 2576 * </p> 2577 */ 2578 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE); 2579 2580/** 2581 * Constant for fluent queries to be used to add include statements. Specifies 2582 * the path value of "<b>ChargeItem:service</b>". 2583 */ 2584 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include("ChargeItem:service").toLocked(); 2585 2586 /** 2587 * Search parameter: <b>price-override</b> 2588 * <p> 2589 * Description: <b>Price overriding the associated rules</b><br> 2590 * Type: <b>quantity</b><br> 2591 * Path: <b>ChargeItem.priceOverride</b><br> 2592 * </p> 2593 */ 2594 @SearchParamDefinition(name="price-override", path="ChargeItem.priceOverride", description="Price overriding the associated rules", type="quantity" ) 2595 public static final String SP_PRICE_OVERRIDE = "price-override"; 2596 /** 2597 * <b>Fluent Client</b> search parameter constant for <b>price-override</b> 2598 * <p> 2599 * Description: <b>Price overriding the associated rules</b><br> 2600 * Type: <b>quantity</b><br> 2601 * Path: <b>ChargeItem.priceOverride</b><br> 2602 * </p> 2603 */ 2604 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam PRICE_OVERRIDE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_PRICE_OVERRIDE); 2605 2606 /** 2607 * Search parameter: <b>context</b> 2608 * <p> 2609 * Description: <b>Encounter / Episode associated with event</b><br> 2610 * Type: <b>reference</b><br> 2611 * Path: <b>ChargeItem.context</b><br> 2612 * </p> 2613 */ 2614 @SearchParamDefinition(name="context", path="ChargeItem.context", description="Encounter / Episode associated with event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2615 public static final String SP_CONTEXT = "context"; 2616 /** 2617 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2618 * <p> 2619 * Description: <b>Encounter / Episode associated with event</b><br> 2620 * Type: <b>reference</b><br> 2621 * Path: <b>ChargeItem.context</b><br> 2622 * </p> 2623 */ 2624 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2625 2626/** 2627 * Constant for fluent queries to be used to add include statements. Specifies 2628 * the path value of "<b>ChargeItem:context</b>". 2629 */ 2630 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("ChargeItem:context").toLocked(); 2631 2632 /** 2633 * Search parameter: <b>enterer</b> 2634 * <p> 2635 * Description: <b>Individual who was entering</b><br> 2636 * Type: <b>reference</b><br> 2637 * Path: <b>ChargeItem.enterer</b><br> 2638 * </p> 2639 */ 2640 @SearchParamDefinition(name="enterer", path="ChargeItem.enterer", description="Individual who was entering", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2641 public static final String SP_ENTERER = "enterer"; 2642 /** 2643 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 2644 * <p> 2645 * Description: <b>Individual who was entering</b><br> 2646 * Type: <b>reference</b><br> 2647 * Path: <b>ChargeItem.enterer</b><br> 2648 * </p> 2649 */ 2650 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 2651 2652/** 2653 * Constant for fluent queries to be used to add include statements. Specifies 2654 * the path value of "<b>ChargeItem:enterer</b>". 2655 */ 2656 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("ChargeItem:enterer").toLocked(); 2657 2658 /** 2659 * Search parameter: <b>account</b> 2660 * <p> 2661 * Description: <b>Account to place this charge</b><br> 2662 * Type: <b>reference</b><br> 2663 * Path: <b>ChargeItem.account</b><br> 2664 * </p> 2665 */ 2666 @SearchParamDefinition(name="account", path="ChargeItem.account", description="Account to place this charge", type="reference", target={Account.class } ) 2667 public static final String SP_ACCOUNT = "account"; 2668 /** 2669 * <b>Fluent Client</b> search parameter constant for <b>account</b> 2670 * <p> 2671 * Description: <b>Account to place this charge</b><br> 2672 * Type: <b>reference</b><br> 2673 * Path: <b>ChargeItem.account</b><br> 2674 * </p> 2675 */ 2676 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACCOUNT); 2677 2678/** 2679 * Constant for fluent queries to be used to add include statements. Specifies 2680 * the path value of "<b>ChargeItem:account</b>". 2681 */ 2682 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include("ChargeItem:account").toLocked(); 2683 2684 /** 2685 * Search parameter: <b>requesting-organization</b> 2686 * <p> 2687 * Description: <b>Organization requesting the charged service</b><br> 2688 * Type: <b>reference</b><br> 2689 * Path: <b>ChargeItem.requestingOrganization</b><br> 2690 * </p> 2691 */ 2692 @SearchParamDefinition(name="requesting-organization", path="ChargeItem.requestingOrganization", description="Organization requesting the charged service", type="reference", target={Organization.class } ) 2693 public static final String SP_REQUESTING_ORGANIZATION = "requesting-organization"; 2694 /** 2695 * <b>Fluent Client</b> search parameter constant for <b>requesting-organization</b> 2696 * <p> 2697 * Description: <b>Organization requesting the charged service</b><br> 2698 * Type: <b>reference</b><br> 2699 * Path: <b>ChargeItem.requestingOrganization</b><br> 2700 * </p> 2701 */ 2702 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTING_ORGANIZATION); 2703 2704/** 2705 * Constant for fluent queries to be used to add include statements. Specifies 2706 * the path value of "<b>ChargeItem:requesting-organization</b>". 2707 */ 2708 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTING_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ChargeItem:requesting-organization").toLocked(); 2709 2710 2711}