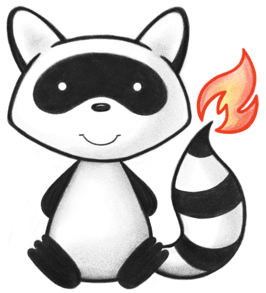
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 051 */ 052@ResourceDef(name="Claim", profile="http://hl7.org/fhir/Profile/Claim") 053public class Claim extends DomainResource { 054 055 public enum ClaimStatus { 056 /** 057 * The instance is currently in-force. 058 */ 059 ACTIVE, 060 /** 061 * The instance is withdrawn, rescinded or reversed. 062 */ 063 CANCELLED, 064 /** 065 * A new instance the contents of which is not complete. 066 */ 067 DRAFT, 068 /** 069 * The instance was entered in error. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static ClaimStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown ClaimStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: return "active"; 095 case CANCELLED: return "cancelled"; 096 case DRAFT: return "draft"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 105 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 106 case DRAFT: return "http://hl7.org/fhir/fm-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case ACTIVE: return "The instance is currently in-force."; 115 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 116 case DRAFT: return "A new instance the contents of which is not complete."; 117 case ENTEREDINERROR: return "The instance was entered in error."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ACTIVE: return "Active"; 125 case CANCELLED: return "Cancelled"; 126 case DRAFT: return "Draft"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class ClaimStatusEnumFactory implements EnumFactory<ClaimStatus> { 135 public ClaimStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("active".equals(codeString)) 140 return ClaimStatus.ACTIVE; 141 if ("cancelled".equals(codeString)) 142 return ClaimStatus.CANCELLED; 143 if ("draft".equals(codeString)) 144 return ClaimStatus.DRAFT; 145 if ("entered-in-error".equals(codeString)) 146 return ClaimStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown ClaimStatus code '"+codeString+"'"); 148 } 149 public Enumeration<ClaimStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<ClaimStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("active".equals(codeString)) 158 return new Enumeration<ClaimStatus>(this, ClaimStatus.ACTIVE); 159 if ("cancelled".equals(codeString)) 160 return new Enumeration<ClaimStatus>(this, ClaimStatus.CANCELLED); 161 if ("draft".equals(codeString)) 162 return new Enumeration<ClaimStatus>(this, ClaimStatus.DRAFT); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<ClaimStatus>(this, ClaimStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown ClaimStatus code '"+codeString+"'"); 166 } 167 public String toCode(ClaimStatus code) { 168 if (code == ClaimStatus.NULL) 169 return null; 170 if (code == ClaimStatus.ACTIVE) 171 return "active"; 172 if (code == ClaimStatus.CANCELLED) 173 return "cancelled"; 174 if (code == ClaimStatus.DRAFT) 175 return "draft"; 176 if (code == ClaimStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(ClaimStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 public enum Use { 186 /** 187 * The treatment is complete and this represents a Claim for the services. 188 */ 189 COMPLETE, 190 /** 191 * The treatment is proposed and this represents a Pre-authorization for the services. 192 */ 193 PROPOSED, 194 /** 195 * The treatment is proposed and this represents a Pre-determination for the services. 196 */ 197 EXPLORATORY, 198 /** 199 * A locally defined or otherwise resolved status. 200 */ 201 OTHER, 202 /** 203 * added to help the parsers with the generic types 204 */ 205 NULL; 206 public static Use fromCode(String codeString) throws FHIRException { 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("complete".equals(codeString)) 210 return COMPLETE; 211 if ("proposed".equals(codeString)) 212 return PROPOSED; 213 if ("exploratory".equals(codeString)) 214 return EXPLORATORY; 215 if ("other".equals(codeString)) 216 return OTHER; 217 if (Configuration.isAcceptInvalidEnums()) 218 return null; 219 else 220 throw new FHIRException("Unknown Use code '"+codeString+"'"); 221 } 222 public String toCode() { 223 switch (this) { 224 case COMPLETE: return "complete"; 225 case PROPOSED: return "proposed"; 226 case EXPLORATORY: return "exploratory"; 227 case OTHER: return "other"; 228 case NULL: return null; 229 default: return "?"; 230 } 231 } 232 public String getSystem() { 233 switch (this) { 234 case COMPLETE: return "http://hl7.org/fhir/claim-use"; 235 case PROPOSED: return "http://hl7.org/fhir/claim-use"; 236 case EXPLORATORY: return "http://hl7.org/fhir/claim-use"; 237 case OTHER: return "http://hl7.org/fhir/claim-use"; 238 case NULL: return null; 239 default: return "?"; 240 } 241 } 242 public String getDefinition() { 243 switch (this) { 244 case COMPLETE: return "The treatment is complete and this represents a Claim for the services."; 245 case PROPOSED: return "The treatment is proposed and this represents a Pre-authorization for the services."; 246 case EXPLORATORY: return "The treatment is proposed and this represents a Pre-determination for the services."; 247 case OTHER: return "A locally defined or otherwise resolved status."; 248 case NULL: return null; 249 default: return "?"; 250 } 251 } 252 public String getDisplay() { 253 switch (this) { 254 case COMPLETE: return "Complete"; 255 case PROPOSED: return "Proposed"; 256 case EXPLORATORY: return "Exploratory"; 257 case OTHER: return "Other"; 258 case NULL: return null; 259 default: return "?"; 260 } 261 } 262 } 263 264 public static class UseEnumFactory implements EnumFactory<Use> { 265 public Use fromCode(String codeString) throws IllegalArgumentException { 266 if (codeString == null || "".equals(codeString)) 267 if (codeString == null || "".equals(codeString)) 268 return null; 269 if ("complete".equals(codeString)) 270 return Use.COMPLETE; 271 if ("proposed".equals(codeString)) 272 return Use.PROPOSED; 273 if ("exploratory".equals(codeString)) 274 return Use.EXPLORATORY; 275 if ("other".equals(codeString)) 276 return Use.OTHER; 277 throw new IllegalArgumentException("Unknown Use code '"+codeString+"'"); 278 } 279 public Enumeration<Use> fromType(PrimitiveType<?> code) throws FHIRException { 280 if (code == null) 281 return null; 282 if (code.isEmpty()) 283 return new Enumeration<Use>(this); 284 String codeString = code.asStringValue(); 285 if (codeString == null || "".equals(codeString)) 286 return null; 287 if ("complete".equals(codeString)) 288 return new Enumeration<Use>(this, Use.COMPLETE); 289 if ("proposed".equals(codeString)) 290 return new Enumeration<Use>(this, Use.PROPOSED); 291 if ("exploratory".equals(codeString)) 292 return new Enumeration<Use>(this, Use.EXPLORATORY); 293 if ("other".equals(codeString)) 294 return new Enumeration<Use>(this, Use.OTHER); 295 throw new FHIRException("Unknown Use code '"+codeString+"'"); 296 } 297 public String toCode(Use code) { 298 if (code == Use.NULL) 299 return null; 300 if (code == Use.COMPLETE) 301 return "complete"; 302 if (code == Use.PROPOSED) 303 return "proposed"; 304 if (code == Use.EXPLORATORY) 305 return "exploratory"; 306 if (code == Use.OTHER) 307 return "other"; 308 return "?"; 309 } 310 public String toSystem(Use code) { 311 return code.getSystem(); 312 } 313 } 314 315 @Block() 316 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 317 /** 318 * Other claims which are related to this claim such as prior claim versions or for related services. 319 */ 320 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 321 @Description(shortDefinition="Reference to the related claim", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 322 protected Reference claim; 323 324 /** 325 * The actual object that is the target of the reference (Other claims which are related to this claim such as prior claim versions or for related services.) 326 */ 327 protected Claim claimTarget; 328 329 /** 330 * For example prior or umbrella. 331 */ 332 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 333 @Description(shortDefinition="How the reference claim is related", formalDefinition="For example prior or umbrella." ) 334 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 335 protected CodeableConcept relationship; 336 337 /** 338 * An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # . 339 */ 340 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 341 @Description(shortDefinition="Related file or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # ." ) 342 protected Identifier reference; 343 344 private static final long serialVersionUID = -379338905L; 345 346 /** 347 * Constructor 348 */ 349 public RelatedClaimComponent() { 350 super(); 351 } 352 353 /** 354 * @return {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 355 */ 356 public Reference getClaim() { 357 if (this.claim == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 360 else if (Configuration.doAutoCreate()) 361 this.claim = new Reference(); // cc 362 return this.claim; 363 } 364 365 public boolean hasClaim() { 366 return this.claim != null && !this.claim.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 371 */ 372 public RelatedClaimComponent setClaim(Reference value) { 373 this.claim = value; 374 return this; 375 } 376 377 /** 378 * @return {@link #claim} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 379 */ 380 public Claim getClaimTarget() { 381 if (this.claimTarget == null) 382 if (Configuration.errorOnAutoCreate()) 383 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 384 else if (Configuration.doAutoCreate()) 385 this.claimTarget = new Claim(); // aa 386 return this.claimTarget; 387 } 388 389 /** 390 * @param value {@link #claim} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 391 */ 392 public RelatedClaimComponent setClaimTarget(Claim value) { 393 this.claimTarget = value; 394 return this; 395 } 396 397 /** 398 * @return {@link #relationship} (For example prior or umbrella.) 399 */ 400 public CodeableConcept getRelationship() { 401 if (this.relationship == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 404 else if (Configuration.doAutoCreate()) 405 this.relationship = new CodeableConcept(); // cc 406 return this.relationship; 407 } 408 409 public boolean hasRelationship() { 410 return this.relationship != null && !this.relationship.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #relationship} (For example prior or umbrella.) 415 */ 416 public RelatedClaimComponent setRelationship(CodeableConcept value) { 417 this.relationship = value; 418 return this; 419 } 420 421 /** 422 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 423 */ 424 public Identifier getReference() { 425 if (this.reference == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 428 else if (Configuration.doAutoCreate()) 429 this.reference = new Identifier(); // cc 430 return this.reference; 431 } 432 433 public boolean hasReference() { 434 return this.reference != null && !this.reference.isEmpty(); 435 } 436 437 /** 438 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 439 */ 440 public RelatedClaimComponent setReference(Identifier value) { 441 this.reference = value; 442 return this; 443 } 444 445 protected void listChildren(List<Property> children) { 446 super.listChildren(children); 447 children.add(new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim)); 448 children.add(new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship)); 449 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference)); 450 } 451 452 @Override 453 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 454 switch (_hash) { 455 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim); 456 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship); 457 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference); 458 default: return super.getNamedProperty(_hash, _name, _checkValid); 459 } 460 461 } 462 463 @Override 464 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 465 switch (hash) { 466 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 467 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 468 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 469 default: return super.getProperty(hash, name, checkValid); 470 } 471 472 } 473 474 @Override 475 public Base setProperty(int hash, String name, Base value) throws FHIRException { 476 switch (hash) { 477 case 94742588: // claim 478 this.claim = castToReference(value); // Reference 479 return value; 480 case -261851592: // relationship 481 this.relationship = castToCodeableConcept(value); // CodeableConcept 482 return value; 483 case -925155509: // reference 484 this.reference = castToIdentifier(value); // Identifier 485 return value; 486 default: return super.setProperty(hash, name, value); 487 } 488 489 } 490 491 @Override 492 public Base setProperty(String name, Base value) throws FHIRException { 493 if (name.equals("claim")) { 494 this.claim = castToReference(value); // Reference 495 } else if (name.equals("relationship")) { 496 this.relationship = castToCodeableConcept(value); // CodeableConcept 497 } else if (name.equals("reference")) { 498 this.reference = castToIdentifier(value); // Identifier 499 } else 500 return super.setProperty(name, value); 501 return value; 502 } 503 504 @Override 505 public Base makeProperty(int hash, String name) throws FHIRException { 506 switch (hash) { 507 case 94742588: return getClaim(); 508 case -261851592: return getRelationship(); 509 case -925155509: return getReference(); 510 default: return super.makeProperty(hash, name); 511 } 512 513 } 514 515 @Override 516 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 517 switch (hash) { 518 case 94742588: /*claim*/ return new String[] {"Reference"}; 519 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 520 case -925155509: /*reference*/ return new String[] {"Identifier"}; 521 default: return super.getTypesForProperty(hash, name); 522 } 523 524 } 525 526 @Override 527 public Base addChild(String name) throws FHIRException { 528 if (name.equals("claim")) { 529 this.claim = new Reference(); 530 return this.claim; 531 } 532 else if (name.equals("relationship")) { 533 this.relationship = new CodeableConcept(); 534 return this.relationship; 535 } 536 else if (name.equals("reference")) { 537 this.reference = new Identifier(); 538 return this.reference; 539 } 540 else 541 return super.addChild(name); 542 } 543 544 public RelatedClaimComponent copy() { 545 RelatedClaimComponent dst = new RelatedClaimComponent(); 546 copyValues(dst); 547 dst.claim = claim == null ? null : claim.copy(); 548 dst.relationship = relationship == null ? null : relationship.copy(); 549 dst.reference = reference == null ? null : reference.copy(); 550 return dst; 551 } 552 553 @Override 554 public boolean equalsDeep(Base other_) { 555 if (!super.equalsDeep(other_)) 556 return false; 557 if (!(other_ instanceof RelatedClaimComponent)) 558 return false; 559 RelatedClaimComponent o = (RelatedClaimComponent) other_; 560 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 561 ; 562 } 563 564 @Override 565 public boolean equalsShallow(Base other_) { 566 if (!super.equalsShallow(other_)) 567 return false; 568 if (!(other_ instanceof RelatedClaimComponent)) 569 return false; 570 RelatedClaimComponent o = (RelatedClaimComponent) other_; 571 return true; 572 } 573 574 public boolean isEmpty() { 575 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 576 ); 577 } 578 579 public String fhirType() { 580 return "Claim.related"; 581 582 } 583 584 } 585 586 @Block() 587 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 588 /** 589 * Type of Party to be reimbursed: Subscriber, provider, other. 590 */ 591 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 592 @Description(shortDefinition="Type of party: Subscriber, Provider, other", formalDefinition="Type of Party to be reimbursed: Subscriber, provider, other." ) 593 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 594 protected CodeableConcept type; 595 596 /** 597 * organization | patient | practitioner | relatedperson. 598 */ 599 @Child(name = "resourceType", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=false) 600 @Description(shortDefinition="organization | patient | practitioner | relatedperson", formalDefinition="organization | patient | practitioner | relatedperson." ) 601 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-payee-resource-type") 602 protected Coding resourceType; 603 604 /** 605 * Party to be reimbursed: Subscriber, provider, other. 606 */ 607 @Child(name = "party", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=false) 608 @Description(shortDefinition="Party to receive the payable", formalDefinition="Party to be reimbursed: Subscriber, provider, other." ) 609 protected Reference party; 610 611 /** 612 * The actual object that is the target of the reference (Party to be reimbursed: Subscriber, provider, other.) 613 */ 614 protected Resource partyTarget; 615 616 private static final long serialVersionUID = -1395150769L; 617 618 /** 619 * Constructor 620 */ 621 public PayeeComponent() { 622 super(); 623 } 624 625 /** 626 * Constructor 627 */ 628 public PayeeComponent(CodeableConcept type) { 629 super(); 630 this.type = type; 631 } 632 633 /** 634 * @return {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 635 */ 636 public CodeableConcept getType() { 637 if (this.type == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create PayeeComponent.type"); 640 else if (Configuration.doAutoCreate()) 641 this.type = new CodeableConcept(); // cc 642 return this.type; 643 } 644 645 public boolean hasType() { 646 return this.type != null && !this.type.isEmpty(); 647 } 648 649 /** 650 * @param value {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 651 */ 652 public PayeeComponent setType(CodeableConcept value) { 653 this.type = value; 654 return this; 655 } 656 657 /** 658 * @return {@link #resourceType} (organization | patient | practitioner | relatedperson.) 659 */ 660 public Coding getResourceType() { 661 if (this.resourceType == null) 662 if (Configuration.errorOnAutoCreate()) 663 throw new Error("Attempt to auto-create PayeeComponent.resourceType"); 664 else if (Configuration.doAutoCreate()) 665 this.resourceType = new Coding(); // cc 666 return this.resourceType; 667 } 668 669 public boolean hasResourceType() { 670 return this.resourceType != null && !this.resourceType.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #resourceType} (organization | patient | practitioner | relatedperson.) 675 */ 676 public PayeeComponent setResourceType(Coding value) { 677 this.resourceType = value; 678 return this; 679 } 680 681 /** 682 * @return {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 683 */ 684 public Reference getParty() { 685 if (this.party == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create PayeeComponent.party"); 688 else if (Configuration.doAutoCreate()) 689 this.party = new Reference(); // cc 690 return this.party; 691 } 692 693 public boolean hasParty() { 694 return this.party != null && !this.party.isEmpty(); 695 } 696 697 /** 698 * @param value {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 699 */ 700 public PayeeComponent setParty(Reference value) { 701 this.party = value; 702 return this; 703 } 704 705 /** 706 * @return {@link #party} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 707 */ 708 public Resource getPartyTarget() { 709 return this.partyTarget; 710 } 711 712 /** 713 * @param value {@link #party} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 714 */ 715 public PayeeComponent setPartyTarget(Resource value) { 716 this.partyTarget = value; 717 return this; 718 } 719 720 protected void listChildren(List<Property> children) { 721 super.listChildren(children); 722 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type)); 723 children.add(new Property("resourceType", "Coding", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType)); 724 children.add(new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party)); 725 } 726 727 @Override 728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 729 switch (_hash) { 730 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type); 731 case -384364440: /*resourceType*/ return new Property("resourceType", "Coding", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType); 732 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party); 733 default: return super.getNamedProperty(_hash, _name, _checkValid); 734 } 735 736 } 737 738 @Override 739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 740 switch (hash) { 741 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 742 case -384364440: /*resourceType*/ return this.resourceType == null ? new Base[0] : new Base[] {this.resourceType}; // Coding 743 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 744 default: return super.getProperty(hash, name, checkValid); 745 } 746 747 } 748 749 @Override 750 public Base setProperty(int hash, String name, Base value) throws FHIRException { 751 switch (hash) { 752 case 3575610: // type 753 this.type = castToCodeableConcept(value); // CodeableConcept 754 return value; 755 case -384364440: // resourceType 756 this.resourceType = castToCoding(value); // Coding 757 return value; 758 case 106437350: // party 759 this.party = castToReference(value); // Reference 760 return value; 761 default: return super.setProperty(hash, name, value); 762 } 763 764 } 765 766 @Override 767 public Base setProperty(String name, Base value) throws FHIRException { 768 if (name.equals("type")) { 769 this.type = castToCodeableConcept(value); // CodeableConcept 770 } else if (name.equals("resourceType")) { 771 this.resourceType = castToCoding(value); // Coding 772 } else if (name.equals("party")) { 773 this.party = castToReference(value); // Reference 774 } else 775 return super.setProperty(name, value); 776 return value; 777 } 778 779 @Override 780 public Base makeProperty(int hash, String name) throws FHIRException { 781 switch (hash) { 782 case 3575610: return getType(); 783 case -384364440: return getResourceType(); 784 case 106437350: return getParty(); 785 default: return super.makeProperty(hash, name); 786 } 787 788 } 789 790 @Override 791 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 792 switch (hash) { 793 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 794 case -384364440: /*resourceType*/ return new String[] {"Coding"}; 795 case 106437350: /*party*/ return new String[] {"Reference"}; 796 default: return super.getTypesForProperty(hash, name); 797 } 798 799 } 800 801 @Override 802 public Base addChild(String name) throws FHIRException { 803 if (name.equals("type")) { 804 this.type = new CodeableConcept(); 805 return this.type; 806 } 807 else if (name.equals("resourceType")) { 808 this.resourceType = new Coding(); 809 return this.resourceType; 810 } 811 else if (name.equals("party")) { 812 this.party = new Reference(); 813 return this.party; 814 } 815 else 816 return super.addChild(name); 817 } 818 819 public PayeeComponent copy() { 820 PayeeComponent dst = new PayeeComponent(); 821 copyValues(dst); 822 dst.type = type == null ? null : type.copy(); 823 dst.resourceType = resourceType == null ? null : resourceType.copy(); 824 dst.party = party == null ? null : party.copy(); 825 return dst; 826 } 827 828 @Override 829 public boolean equalsDeep(Base other_) { 830 if (!super.equalsDeep(other_)) 831 return false; 832 if (!(other_ instanceof PayeeComponent)) 833 return false; 834 PayeeComponent o = (PayeeComponent) other_; 835 return compareDeep(type, o.type, true) && compareDeep(resourceType, o.resourceType, true) && compareDeep(party, o.party, true) 836 ; 837 } 838 839 @Override 840 public boolean equalsShallow(Base other_) { 841 if (!super.equalsShallow(other_)) 842 return false; 843 if (!(other_ instanceof PayeeComponent)) 844 return false; 845 PayeeComponent o = (PayeeComponent) other_; 846 return true; 847 } 848 849 public boolean isEmpty() { 850 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resourceType, party 851 ); 852 } 853 854 public String fhirType() { 855 return "Claim.payee"; 856 857 } 858 859 } 860 861 @Block() 862 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 863 /** 864 * Sequence of the careTeam which serves to order and provide a link. 865 */ 866 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 867 @Description(shortDefinition="Number to covey order of careTeam", formalDefinition="Sequence of the careTeam which serves to order and provide a link." ) 868 protected PositiveIntType sequence; 869 870 /** 871 * Member of the team who provided the overall service. 872 */ 873 @Child(name = "provider", type = {Practitioner.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 874 @Description(shortDefinition="Provider individual or organization", formalDefinition="Member of the team who provided the overall service." ) 875 protected Reference provider; 876 877 /** 878 * The actual object that is the target of the reference (Member of the team who provided the overall service.) 879 */ 880 protected Resource providerTarget; 881 882 /** 883 * The party who is billing and responsible for the claimed good or service rendered to the patient. 884 */ 885 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 886 @Description(shortDefinition="Billing provider", formalDefinition="The party who is billing and responsible for the claimed good or service rendered to the patient." ) 887 protected BooleanType responsible; 888 889 /** 890 * The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team. 891 */ 892 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 893 @Description(shortDefinition="Role on the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team." ) 894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 895 protected CodeableConcept role; 896 897 /** 898 * The qualification which is applicable for this service. 899 */ 900 @Child(name = "qualification", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 901 @Description(shortDefinition="Type, classification or Specialization", formalDefinition="The qualification which is applicable for this service." ) 902 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 903 protected CodeableConcept qualification; 904 905 private static final long serialVersionUID = 1758966968L; 906 907 /** 908 * Constructor 909 */ 910 public CareTeamComponent() { 911 super(); 912 } 913 914 /** 915 * Constructor 916 */ 917 public CareTeamComponent(PositiveIntType sequence, Reference provider) { 918 super(); 919 this.sequence = sequence; 920 this.provider = provider; 921 } 922 923 /** 924 * @return {@link #sequence} (Sequence of the careTeam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 925 */ 926 public PositiveIntType getSequenceElement() { 927 if (this.sequence == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 930 else if (Configuration.doAutoCreate()) 931 this.sequence = new PositiveIntType(); // bb 932 return this.sequence; 933 } 934 935 public boolean hasSequenceElement() { 936 return this.sequence != null && !this.sequence.isEmpty(); 937 } 938 939 public boolean hasSequence() { 940 return this.sequence != null && !this.sequence.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #sequence} (Sequence of the careTeam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 945 */ 946 public CareTeamComponent setSequenceElement(PositiveIntType value) { 947 this.sequence = value; 948 return this; 949 } 950 951 /** 952 * @return Sequence of the careTeam which serves to order and provide a link. 953 */ 954 public int getSequence() { 955 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 956 } 957 958 /** 959 * @param value Sequence of the careTeam which serves to order and provide a link. 960 */ 961 public CareTeamComponent setSequence(int value) { 962 if (this.sequence == null) 963 this.sequence = new PositiveIntType(); 964 this.sequence.setValue(value); 965 return this; 966 } 967 968 /** 969 * @return {@link #provider} (Member of the team who provided the overall service.) 970 */ 971 public Reference getProvider() { 972 if (this.provider == null) 973 if (Configuration.errorOnAutoCreate()) 974 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 975 else if (Configuration.doAutoCreate()) 976 this.provider = new Reference(); // cc 977 return this.provider; 978 } 979 980 public boolean hasProvider() { 981 return this.provider != null && !this.provider.isEmpty(); 982 } 983 984 /** 985 * @param value {@link #provider} (Member of the team who provided the overall service.) 986 */ 987 public CareTeamComponent setProvider(Reference value) { 988 this.provider = value; 989 return this; 990 } 991 992 /** 993 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Member of the team who provided the overall service.) 994 */ 995 public Resource getProviderTarget() { 996 return this.providerTarget; 997 } 998 999 /** 1000 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Member of the team who provided the overall service.) 1001 */ 1002 public CareTeamComponent setProviderTarget(Resource value) { 1003 this.providerTarget = value; 1004 return this; 1005 } 1006 1007 /** 1008 * @return {@link #responsible} (The party who is billing and responsible for the claimed good or service rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1009 */ 1010 public BooleanType getResponsibleElement() { 1011 if (this.responsible == null) 1012 if (Configuration.errorOnAutoCreate()) 1013 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1014 else if (Configuration.doAutoCreate()) 1015 this.responsible = new BooleanType(); // bb 1016 return this.responsible; 1017 } 1018 1019 public boolean hasResponsibleElement() { 1020 return this.responsible != null && !this.responsible.isEmpty(); 1021 } 1022 1023 public boolean hasResponsible() { 1024 return this.responsible != null && !this.responsible.isEmpty(); 1025 } 1026 1027 /** 1028 * @param value {@link #responsible} (The party who is billing and responsible for the claimed good or service rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1029 */ 1030 public CareTeamComponent setResponsibleElement(BooleanType value) { 1031 this.responsible = value; 1032 return this; 1033 } 1034 1035 /** 1036 * @return The party who is billing and responsible for the claimed good or service rendered to the patient. 1037 */ 1038 public boolean getResponsible() { 1039 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1040 } 1041 1042 /** 1043 * @param value The party who is billing and responsible for the claimed good or service rendered to the patient. 1044 */ 1045 public CareTeamComponent setResponsible(boolean value) { 1046 if (this.responsible == null) 1047 this.responsible = new BooleanType(); 1048 this.responsible.setValue(value); 1049 return this; 1050 } 1051 1052 /** 1053 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1054 */ 1055 public CodeableConcept getRole() { 1056 if (this.role == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1059 else if (Configuration.doAutoCreate()) 1060 this.role = new CodeableConcept(); // cc 1061 return this.role; 1062 } 1063 1064 public boolean hasRole() { 1065 return this.role != null && !this.role.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1070 */ 1071 public CareTeamComponent setRole(CodeableConcept value) { 1072 this.role = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return {@link #qualification} (The qualification which is applicable for this service.) 1078 */ 1079 public CodeableConcept getQualification() { 1080 if (this.qualification == null) 1081 if (Configuration.errorOnAutoCreate()) 1082 throw new Error("Attempt to auto-create CareTeamComponent.qualification"); 1083 else if (Configuration.doAutoCreate()) 1084 this.qualification = new CodeableConcept(); // cc 1085 return this.qualification; 1086 } 1087 1088 public boolean hasQualification() { 1089 return this.qualification != null && !this.qualification.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #qualification} (The qualification which is applicable for this service.) 1094 */ 1095 public CareTeamComponent setQualification(CodeableConcept value) { 1096 this.qualification = value; 1097 return this; 1098 } 1099 1100 protected void listChildren(List<Property> children) { 1101 super.listChildren(children); 1102 children.add(new Property("sequence", "positiveInt", "Sequence of the careTeam which serves to order and provide a link.", 0, 1, sequence)); 1103 children.add(new Property("provider", "Reference(Practitioner|Organization)", "Member of the team who provided the overall service.", 0, 1, provider)); 1104 children.add(new Property("responsible", "boolean", "The party who is billing and responsible for the claimed good or service rendered to the patient.", 0, 1, responsible)); 1105 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role)); 1106 children.add(new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification)); 1107 } 1108 1109 @Override 1110 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1111 switch (_hash) { 1112 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of the careTeam which serves to order and provide a link.", 0, 1, sequence); 1113 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|Organization)", "Member of the team who provided the overall service.", 0, 1, provider); 1114 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The party who is billing and responsible for the claimed good or service rendered to the patient.", 0, 1, responsible); 1115 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role); 1116 case -631333393: /*qualification*/ return new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification); 1117 default: return super.getNamedProperty(_hash, _name, _checkValid); 1118 } 1119 1120 } 1121 1122 @Override 1123 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1124 switch (hash) { 1125 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1126 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1127 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1128 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1129 case -631333393: /*qualification*/ return this.qualification == null ? new Base[0] : new Base[] {this.qualification}; // CodeableConcept 1130 default: return super.getProperty(hash, name, checkValid); 1131 } 1132 1133 } 1134 1135 @Override 1136 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1137 switch (hash) { 1138 case 1349547969: // sequence 1139 this.sequence = castToPositiveInt(value); // PositiveIntType 1140 return value; 1141 case -987494927: // provider 1142 this.provider = castToReference(value); // Reference 1143 return value; 1144 case 1847674614: // responsible 1145 this.responsible = castToBoolean(value); // BooleanType 1146 return value; 1147 case 3506294: // role 1148 this.role = castToCodeableConcept(value); // CodeableConcept 1149 return value; 1150 case -631333393: // qualification 1151 this.qualification = castToCodeableConcept(value); // CodeableConcept 1152 return value; 1153 default: return super.setProperty(hash, name, value); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base setProperty(String name, Base value) throws FHIRException { 1160 if (name.equals("sequence")) { 1161 this.sequence = castToPositiveInt(value); // PositiveIntType 1162 } else if (name.equals("provider")) { 1163 this.provider = castToReference(value); // Reference 1164 } else if (name.equals("responsible")) { 1165 this.responsible = castToBoolean(value); // BooleanType 1166 } else if (name.equals("role")) { 1167 this.role = castToCodeableConcept(value); // CodeableConcept 1168 } else if (name.equals("qualification")) { 1169 this.qualification = castToCodeableConcept(value); // CodeableConcept 1170 } else 1171 return super.setProperty(name, value); 1172 return value; 1173 } 1174 1175 @Override 1176 public Base makeProperty(int hash, String name) throws FHIRException { 1177 switch (hash) { 1178 case 1349547969: return getSequenceElement(); 1179 case -987494927: return getProvider(); 1180 case 1847674614: return getResponsibleElement(); 1181 case 3506294: return getRole(); 1182 case -631333393: return getQualification(); 1183 default: return super.makeProperty(hash, name); 1184 } 1185 1186 } 1187 1188 @Override 1189 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1190 switch (hash) { 1191 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1192 case -987494927: /*provider*/ return new String[] {"Reference"}; 1193 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1194 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1195 case -631333393: /*qualification*/ return new String[] {"CodeableConcept"}; 1196 default: return super.getTypesForProperty(hash, name); 1197 } 1198 1199 } 1200 1201 @Override 1202 public Base addChild(String name) throws FHIRException { 1203 if (name.equals("sequence")) { 1204 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 1205 } 1206 else if (name.equals("provider")) { 1207 this.provider = new Reference(); 1208 return this.provider; 1209 } 1210 else if (name.equals("responsible")) { 1211 throw new FHIRException("Cannot call addChild on a singleton property Claim.responsible"); 1212 } 1213 else if (name.equals("role")) { 1214 this.role = new CodeableConcept(); 1215 return this.role; 1216 } 1217 else if (name.equals("qualification")) { 1218 this.qualification = new CodeableConcept(); 1219 return this.qualification; 1220 } 1221 else 1222 return super.addChild(name); 1223 } 1224 1225 public CareTeamComponent copy() { 1226 CareTeamComponent dst = new CareTeamComponent(); 1227 copyValues(dst); 1228 dst.sequence = sequence == null ? null : sequence.copy(); 1229 dst.provider = provider == null ? null : provider.copy(); 1230 dst.responsible = responsible == null ? null : responsible.copy(); 1231 dst.role = role == null ? null : role.copy(); 1232 dst.qualification = qualification == null ? null : qualification.copy(); 1233 return dst; 1234 } 1235 1236 @Override 1237 public boolean equalsDeep(Base other_) { 1238 if (!super.equalsDeep(other_)) 1239 return false; 1240 if (!(other_ instanceof CareTeamComponent)) 1241 return false; 1242 CareTeamComponent o = (CareTeamComponent) other_; 1243 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1244 && compareDeep(role, o.role, true) && compareDeep(qualification, o.qualification, true); 1245 } 1246 1247 @Override 1248 public boolean equalsShallow(Base other_) { 1249 if (!super.equalsShallow(other_)) 1250 return false; 1251 if (!(other_ instanceof CareTeamComponent)) 1252 return false; 1253 CareTeamComponent o = (CareTeamComponent) other_; 1254 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1255 ; 1256 } 1257 1258 public boolean isEmpty() { 1259 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1260 , role, qualification); 1261 } 1262 1263 public String fhirType() { 1264 return "Claim.careTeam"; 1265 1266 } 1267 1268 } 1269 1270 @Block() 1271 public static class SpecialConditionComponent extends BackboneElement implements IBaseBackboneElement { 1272 /** 1273 * Sequence of the information element which serves to provide a link. 1274 */ 1275 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1276 @Description(shortDefinition="Information instance identifier", formalDefinition="Sequence of the information element which serves to provide a link." ) 1277 protected PositiveIntType sequence; 1278 1279 /** 1280 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 1281 */ 1282 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1283 @Description(shortDefinition="General class of information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 1284 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 1285 protected CodeableConcept category; 1286 1287 /** 1288 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication. 1289 */ 1290 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1291 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication." ) 1292 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 1293 protected CodeableConcept code; 1294 1295 /** 1296 * The date when or period to which this information refers. 1297 */ 1298 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1299 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 1300 protected Type timing; 1301 1302 /** 1303 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 1304 */ 1305 @Child(name = "value", type = {StringType.class, Quantity.class, Attachment.class, Reference.class}, order=5, min=0, max=1, modifier=false, summary=false) 1306 @Description(shortDefinition="Additional Data or supporting information", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 1307 protected Type value; 1308 1309 /** 1310 * For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content. 1311 */ 1312 @Child(name = "reason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1313 @Description(shortDefinition="Reason associated with the information", formalDefinition="For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content." ) 1314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1315 protected CodeableConcept reason; 1316 1317 private static final long serialVersionUID = -518630232L; 1318 1319 /** 1320 * Constructor 1321 */ 1322 public SpecialConditionComponent() { 1323 super(); 1324 } 1325 1326 /** 1327 * Constructor 1328 */ 1329 public SpecialConditionComponent(PositiveIntType sequence, CodeableConcept category) { 1330 super(); 1331 this.sequence = sequence; 1332 this.category = category; 1333 } 1334 1335 /** 1336 * @return {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1337 */ 1338 public PositiveIntType getSequenceElement() { 1339 if (this.sequence == null) 1340 if (Configuration.errorOnAutoCreate()) 1341 throw new Error("Attempt to auto-create SpecialConditionComponent.sequence"); 1342 else if (Configuration.doAutoCreate()) 1343 this.sequence = new PositiveIntType(); // bb 1344 return this.sequence; 1345 } 1346 1347 public boolean hasSequenceElement() { 1348 return this.sequence != null && !this.sequence.isEmpty(); 1349 } 1350 1351 public boolean hasSequence() { 1352 return this.sequence != null && !this.sequence.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1357 */ 1358 public SpecialConditionComponent setSequenceElement(PositiveIntType value) { 1359 this.sequence = value; 1360 return this; 1361 } 1362 1363 /** 1364 * @return Sequence of the information element which serves to provide a link. 1365 */ 1366 public int getSequence() { 1367 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1368 } 1369 1370 /** 1371 * @param value Sequence of the information element which serves to provide a link. 1372 */ 1373 public SpecialConditionComponent setSequence(int value) { 1374 if (this.sequence == null) 1375 this.sequence = new PositiveIntType(); 1376 this.sequence.setValue(value); 1377 return this; 1378 } 1379 1380 /** 1381 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1382 */ 1383 public CodeableConcept getCategory() { 1384 if (this.category == null) 1385 if (Configuration.errorOnAutoCreate()) 1386 throw new Error("Attempt to auto-create SpecialConditionComponent.category"); 1387 else if (Configuration.doAutoCreate()) 1388 this.category = new CodeableConcept(); // cc 1389 return this.category; 1390 } 1391 1392 public boolean hasCategory() { 1393 return this.category != null && !this.category.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1398 */ 1399 public SpecialConditionComponent setCategory(CodeableConcept value) { 1400 this.category = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 1406 */ 1407 public CodeableConcept getCode() { 1408 if (this.code == null) 1409 if (Configuration.errorOnAutoCreate()) 1410 throw new Error("Attempt to auto-create SpecialConditionComponent.code"); 1411 else if (Configuration.doAutoCreate()) 1412 this.code = new CodeableConcept(); // cc 1413 return this.code; 1414 } 1415 1416 public boolean hasCode() { 1417 return this.code != null && !this.code.isEmpty(); 1418 } 1419 1420 /** 1421 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 1422 */ 1423 public SpecialConditionComponent setCode(CodeableConcept value) { 1424 this.code = value; 1425 return this; 1426 } 1427 1428 /** 1429 * @return {@link #timing} (The date when or period to which this information refers.) 1430 */ 1431 public Type getTiming() { 1432 return this.timing; 1433 } 1434 1435 /** 1436 * @return {@link #timing} (The date when or period to which this information refers.) 1437 */ 1438 public DateType getTimingDateType() throws FHIRException { 1439 if (this.timing == null) 1440 return null; 1441 if (!(this.timing instanceof DateType)) 1442 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 1443 return (DateType) this.timing; 1444 } 1445 1446 public boolean hasTimingDateType() { 1447 return this != null && this.timing instanceof DateType; 1448 } 1449 1450 /** 1451 * @return {@link #timing} (The date when or period to which this information refers.) 1452 */ 1453 public Period getTimingPeriod() throws FHIRException { 1454 if (this.timing == null) 1455 return null; 1456 if (!(this.timing instanceof Period)) 1457 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 1458 return (Period) this.timing; 1459 } 1460 1461 public boolean hasTimingPeriod() { 1462 return this != null && this.timing instanceof Period; 1463 } 1464 1465 public boolean hasTiming() { 1466 return this.timing != null && !this.timing.isEmpty(); 1467 } 1468 1469 /** 1470 * @param value {@link #timing} (The date when or period to which this information refers.) 1471 */ 1472 public SpecialConditionComponent setTiming(Type value) throws FHIRFormatError { 1473 if (value != null && !(value instanceof DateType || value instanceof Period)) 1474 throw new FHIRFormatError("Not the right type for Claim.information.timing[x]: "+value.fhirType()); 1475 this.timing = value; 1476 return this; 1477 } 1478 1479 /** 1480 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1481 */ 1482 public Type getValue() { 1483 return this.value; 1484 } 1485 1486 /** 1487 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1488 */ 1489 public StringType getValueStringType() throws FHIRException { 1490 if (this.value == null) 1491 return null; 1492 if (!(this.value instanceof StringType)) 1493 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1494 return (StringType) this.value; 1495 } 1496 1497 public boolean hasValueStringType() { 1498 return this != null && this.value instanceof StringType; 1499 } 1500 1501 /** 1502 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1503 */ 1504 public Quantity getValueQuantity() throws FHIRException { 1505 if (this.value == null) 1506 return null; 1507 if (!(this.value instanceof Quantity)) 1508 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1509 return (Quantity) this.value; 1510 } 1511 1512 public boolean hasValueQuantity() { 1513 return this != null && this.value instanceof Quantity; 1514 } 1515 1516 /** 1517 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1518 */ 1519 public Attachment getValueAttachment() throws FHIRException { 1520 if (this.value == null) 1521 return null; 1522 if (!(this.value instanceof Attachment)) 1523 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1524 return (Attachment) this.value; 1525 } 1526 1527 public boolean hasValueAttachment() { 1528 return this != null && this.value instanceof Attachment; 1529 } 1530 1531 /** 1532 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1533 */ 1534 public Reference getValueReference() throws FHIRException { 1535 if (this.value == null) 1536 return null; 1537 if (!(this.value instanceof Reference)) 1538 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1539 return (Reference) this.value; 1540 } 1541 1542 public boolean hasValueReference() { 1543 return this != null && this.value instanceof Reference; 1544 } 1545 1546 public boolean hasValue() { 1547 return this.value != null && !this.value.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1552 */ 1553 public SpecialConditionComponent setValue(Type value) throws FHIRFormatError { 1554 if (value != null && !(value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference)) 1555 throw new FHIRFormatError("Not the right type for Claim.information.value[x]: "+value.fhirType()); 1556 this.value = value; 1557 return this; 1558 } 1559 1560 /** 1561 * @return {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1562 */ 1563 public CodeableConcept getReason() { 1564 if (this.reason == null) 1565 if (Configuration.errorOnAutoCreate()) 1566 throw new Error("Attempt to auto-create SpecialConditionComponent.reason"); 1567 else if (Configuration.doAutoCreate()) 1568 this.reason = new CodeableConcept(); // cc 1569 return this.reason; 1570 } 1571 1572 public boolean hasReason() { 1573 return this.reason != null && !this.reason.isEmpty(); 1574 } 1575 1576 /** 1577 * @param value {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1578 */ 1579 public SpecialConditionComponent setReason(CodeableConcept value) { 1580 this.reason = value; 1581 return this; 1582 } 1583 1584 protected void listChildren(List<Property> children) { 1585 super.listChildren(children); 1586 children.add(new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence)); 1587 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1588 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code)); 1589 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1590 children.add(new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1591 children.add(new Property("reason", "CodeableConcept", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason)); 1592 } 1593 1594 @Override 1595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1596 switch (_hash) { 1597 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence); 1598 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1599 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code); 1600 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1601 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1602 case 807935768: /*timingDate*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1603 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1604 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1605 case 111972721: /*value*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1606 case -1424603934: /*valueString*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1607 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1608 case -475566732: /*valueAttachment*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1609 case 1755241690: /*valueReference*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1610 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason); 1611 default: return super.getNamedProperty(_hash, _name, _checkValid); 1612 } 1613 1614 } 1615 1616 @Override 1617 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1618 switch (hash) { 1619 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1620 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1621 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1622 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 1623 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 1624 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1625 default: return super.getProperty(hash, name, checkValid); 1626 } 1627 1628 } 1629 1630 @Override 1631 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1632 switch (hash) { 1633 case 1349547969: // sequence 1634 this.sequence = castToPositiveInt(value); // PositiveIntType 1635 return value; 1636 case 50511102: // category 1637 this.category = castToCodeableConcept(value); // CodeableConcept 1638 return value; 1639 case 3059181: // code 1640 this.code = castToCodeableConcept(value); // CodeableConcept 1641 return value; 1642 case -873664438: // timing 1643 this.timing = castToType(value); // Type 1644 return value; 1645 case 111972721: // value 1646 this.value = castToType(value); // Type 1647 return value; 1648 case -934964668: // reason 1649 this.reason = castToCodeableConcept(value); // CodeableConcept 1650 return value; 1651 default: return super.setProperty(hash, name, value); 1652 } 1653 1654 } 1655 1656 @Override 1657 public Base setProperty(String name, Base value) throws FHIRException { 1658 if (name.equals("sequence")) { 1659 this.sequence = castToPositiveInt(value); // PositiveIntType 1660 } else if (name.equals("category")) { 1661 this.category = castToCodeableConcept(value); // CodeableConcept 1662 } else if (name.equals("code")) { 1663 this.code = castToCodeableConcept(value); // CodeableConcept 1664 } else if (name.equals("timing[x]")) { 1665 this.timing = castToType(value); // Type 1666 } else if (name.equals("value[x]")) { 1667 this.value = castToType(value); // Type 1668 } else if (name.equals("reason")) { 1669 this.reason = castToCodeableConcept(value); // CodeableConcept 1670 } else 1671 return super.setProperty(name, value); 1672 return value; 1673 } 1674 1675 @Override 1676 public Base makeProperty(int hash, String name) throws FHIRException { 1677 switch (hash) { 1678 case 1349547969: return getSequenceElement(); 1679 case 50511102: return getCategory(); 1680 case 3059181: return getCode(); 1681 case 164632566: return getTiming(); 1682 case -873664438: return getTiming(); 1683 case -1410166417: return getValue(); 1684 case 111972721: return getValue(); 1685 case -934964668: return getReason(); 1686 default: return super.makeProperty(hash, name); 1687 } 1688 1689 } 1690 1691 @Override 1692 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1693 switch (hash) { 1694 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1695 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1696 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1697 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1698 case 111972721: /*value*/ return new String[] {"string", "Quantity", "Attachment", "Reference"}; 1699 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1700 default: return super.getTypesForProperty(hash, name); 1701 } 1702 1703 } 1704 1705 @Override 1706 public Base addChild(String name) throws FHIRException { 1707 if (name.equals("sequence")) { 1708 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 1709 } 1710 else if (name.equals("category")) { 1711 this.category = new CodeableConcept(); 1712 return this.category; 1713 } 1714 else if (name.equals("code")) { 1715 this.code = new CodeableConcept(); 1716 return this.code; 1717 } 1718 else if (name.equals("timingDate")) { 1719 this.timing = new DateType(); 1720 return this.timing; 1721 } 1722 else if (name.equals("timingPeriod")) { 1723 this.timing = new Period(); 1724 return this.timing; 1725 } 1726 else if (name.equals("valueString")) { 1727 this.value = new StringType(); 1728 return this.value; 1729 } 1730 else if (name.equals("valueQuantity")) { 1731 this.value = new Quantity(); 1732 return this.value; 1733 } 1734 else if (name.equals("valueAttachment")) { 1735 this.value = new Attachment(); 1736 return this.value; 1737 } 1738 else if (name.equals("valueReference")) { 1739 this.value = new Reference(); 1740 return this.value; 1741 } 1742 else if (name.equals("reason")) { 1743 this.reason = new CodeableConcept(); 1744 return this.reason; 1745 } 1746 else 1747 return super.addChild(name); 1748 } 1749 1750 public SpecialConditionComponent copy() { 1751 SpecialConditionComponent dst = new SpecialConditionComponent(); 1752 copyValues(dst); 1753 dst.sequence = sequence == null ? null : sequence.copy(); 1754 dst.category = category == null ? null : category.copy(); 1755 dst.code = code == null ? null : code.copy(); 1756 dst.timing = timing == null ? null : timing.copy(); 1757 dst.value = value == null ? null : value.copy(); 1758 dst.reason = reason == null ? null : reason.copy(); 1759 return dst; 1760 } 1761 1762 @Override 1763 public boolean equalsDeep(Base other_) { 1764 if (!super.equalsDeep(other_)) 1765 return false; 1766 if (!(other_ instanceof SpecialConditionComponent)) 1767 return false; 1768 SpecialConditionComponent o = (SpecialConditionComponent) other_; 1769 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1770 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1771 ; 1772 } 1773 1774 @Override 1775 public boolean equalsShallow(Base other_) { 1776 if (!super.equalsShallow(other_)) 1777 return false; 1778 if (!(other_ instanceof SpecialConditionComponent)) 1779 return false; 1780 SpecialConditionComponent o = (SpecialConditionComponent) other_; 1781 return compareValues(sequence, o.sequence, true); 1782 } 1783 1784 public boolean isEmpty() { 1785 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1786 , timing, value, reason); 1787 } 1788 1789 public String fhirType() { 1790 return "Claim.information"; 1791 1792 } 1793 1794 } 1795 1796 @Block() 1797 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1798 /** 1799 * Sequence of diagnosis which serves to provide a link. 1800 */ 1801 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1802 @Description(shortDefinition="Number to covey order of diagnosis", formalDefinition="Sequence of diagnosis which serves to provide a link." ) 1803 protected PositiveIntType sequence; 1804 1805 /** 1806 * The diagnosis. 1807 */ 1808 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1809 @Description(shortDefinition="Patient's diagnosis", formalDefinition="The diagnosis." ) 1810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1811 protected Type diagnosis; 1812 1813 /** 1814 * The type of the Diagnosis, for example: admitting, primary, secondary, discharge. 1815 */ 1816 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1817 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="The type of the Diagnosis, for example: admitting, primary, secondary, discharge." ) 1818 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1819 protected List<CodeableConcept> type; 1820 1821 /** 1822 * The package billing code, for example DRG, based on the assigned grouping code system. 1823 */ 1824 @Child(name = "packageCode", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1825 @Description(shortDefinition="Package billing code", formalDefinition="The package billing code, for example DRG, based on the assigned grouping code system." ) 1826 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 1827 protected CodeableConcept packageCode; 1828 1829 private static final long serialVersionUID = -350960873L; 1830 1831 /** 1832 * Constructor 1833 */ 1834 public DiagnosisComponent() { 1835 super(); 1836 } 1837 1838 /** 1839 * Constructor 1840 */ 1841 public DiagnosisComponent(PositiveIntType sequence, Type diagnosis) { 1842 super(); 1843 this.sequence = sequence; 1844 this.diagnosis = diagnosis; 1845 } 1846 1847 /** 1848 * @return {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1849 */ 1850 public PositiveIntType getSequenceElement() { 1851 if (this.sequence == null) 1852 if (Configuration.errorOnAutoCreate()) 1853 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1854 else if (Configuration.doAutoCreate()) 1855 this.sequence = new PositiveIntType(); // bb 1856 return this.sequence; 1857 } 1858 1859 public boolean hasSequenceElement() { 1860 return this.sequence != null && !this.sequence.isEmpty(); 1861 } 1862 1863 public boolean hasSequence() { 1864 return this.sequence != null && !this.sequence.isEmpty(); 1865 } 1866 1867 /** 1868 * @param value {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1869 */ 1870 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1871 this.sequence = value; 1872 return this; 1873 } 1874 1875 /** 1876 * @return Sequence of diagnosis which serves to provide a link. 1877 */ 1878 public int getSequence() { 1879 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1880 } 1881 1882 /** 1883 * @param value Sequence of diagnosis which serves to provide a link. 1884 */ 1885 public DiagnosisComponent setSequence(int value) { 1886 if (this.sequence == null) 1887 this.sequence = new PositiveIntType(); 1888 this.sequence.setValue(value); 1889 return this; 1890 } 1891 1892 /** 1893 * @return {@link #diagnosis} (The diagnosis.) 1894 */ 1895 public Type getDiagnosis() { 1896 return this.diagnosis; 1897 } 1898 1899 /** 1900 * @return {@link #diagnosis} (The diagnosis.) 1901 */ 1902 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1903 if (this.diagnosis == null) 1904 return null; 1905 if (!(this.diagnosis instanceof CodeableConcept)) 1906 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1907 return (CodeableConcept) this.diagnosis; 1908 } 1909 1910 public boolean hasDiagnosisCodeableConcept() { 1911 return this != null && this.diagnosis instanceof CodeableConcept; 1912 } 1913 1914 /** 1915 * @return {@link #diagnosis} (The diagnosis.) 1916 */ 1917 public Reference getDiagnosisReference() throws FHIRException { 1918 if (this.diagnosis == null) 1919 return null; 1920 if (!(this.diagnosis instanceof Reference)) 1921 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1922 return (Reference) this.diagnosis; 1923 } 1924 1925 public boolean hasDiagnosisReference() { 1926 return this != null && this.diagnosis instanceof Reference; 1927 } 1928 1929 public boolean hasDiagnosis() { 1930 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #diagnosis} (The diagnosis.) 1935 */ 1936 public DiagnosisComponent setDiagnosis(Type value) throws FHIRFormatError { 1937 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1938 throw new FHIRFormatError("Not the right type for Claim.diagnosis.diagnosis[x]: "+value.fhirType()); 1939 this.diagnosis = value; 1940 return this; 1941 } 1942 1943 /** 1944 * @return {@link #type} (The type of the Diagnosis, for example: admitting, primary, secondary, discharge.) 1945 */ 1946 public List<CodeableConcept> getType() { 1947 if (this.type == null) 1948 this.type = new ArrayList<CodeableConcept>(); 1949 return this.type; 1950 } 1951 1952 /** 1953 * @return Returns a reference to <code>this</code> for easy method chaining 1954 */ 1955 public DiagnosisComponent setType(List<CodeableConcept> theType) { 1956 this.type = theType; 1957 return this; 1958 } 1959 1960 public boolean hasType() { 1961 if (this.type == null) 1962 return false; 1963 for (CodeableConcept item : this.type) 1964 if (!item.isEmpty()) 1965 return true; 1966 return false; 1967 } 1968 1969 public CodeableConcept addType() { //3 1970 CodeableConcept t = new CodeableConcept(); 1971 if (this.type == null) 1972 this.type = new ArrayList<CodeableConcept>(); 1973 this.type.add(t); 1974 return t; 1975 } 1976 1977 public DiagnosisComponent addType(CodeableConcept t) { //3 1978 if (t == null) 1979 return this; 1980 if (this.type == null) 1981 this.type = new ArrayList<CodeableConcept>(); 1982 this.type.add(t); 1983 return this; 1984 } 1985 1986 /** 1987 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 1988 */ 1989 public CodeableConcept getTypeFirstRep() { 1990 if (getType().isEmpty()) { 1991 addType(); 1992 } 1993 return getType().get(0); 1994 } 1995 1996 /** 1997 * @return {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 1998 */ 1999 public CodeableConcept getPackageCode() { 2000 if (this.packageCode == null) 2001 if (Configuration.errorOnAutoCreate()) 2002 throw new Error("Attempt to auto-create DiagnosisComponent.packageCode"); 2003 else if (Configuration.doAutoCreate()) 2004 this.packageCode = new CodeableConcept(); // cc 2005 return this.packageCode; 2006 } 2007 2008 public boolean hasPackageCode() { 2009 return this.packageCode != null && !this.packageCode.isEmpty(); 2010 } 2011 2012 /** 2013 * @param value {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 2014 */ 2015 public DiagnosisComponent setPackageCode(CodeableConcept value) { 2016 this.packageCode = value; 2017 return this; 2018 } 2019 2020 protected void listChildren(List<Property> children) { 2021 super.listChildren(children); 2022 children.add(new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence)); 2023 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis)); 2024 children.add(new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type)); 2025 children.add(new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode)); 2026 } 2027 2028 @Override 2029 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2030 switch (_hash) { 2031 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence); 2032 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2033 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2034 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2035 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2036 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type); 2037 case 908444499: /*packageCode*/ return new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode); 2038 default: return super.getNamedProperty(_hash, _name, _checkValid); 2039 } 2040 2041 } 2042 2043 @Override 2044 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2045 switch (hash) { 2046 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2047 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // Type 2048 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2049 case 908444499: /*packageCode*/ return this.packageCode == null ? new Base[0] : new Base[] {this.packageCode}; // CodeableConcept 2050 default: return super.getProperty(hash, name, checkValid); 2051 } 2052 2053 } 2054 2055 @Override 2056 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2057 switch (hash) { 2058 case 1349547969: // sequence 2059 this.sequence = castToPositiveInt(value); // PositiveIntType 2060 return value; 2061 case 1196993265: // diagnosis 2062 this.diagnosis = castToType(value); // Type 2063 return value; 2064 case 3575610: // type 2065 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2066 return value; 2067 case 908444499: // packageCode 2068 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2069 return value; 2070 default: return super.setProperty(hash, name, value); 2071 } 2072 2073 } 2074 2075 @Override 2076 public Base setProperty(String name, Base value) throws FHIRException { 2077 if (name.equals("sequence")) { 2078 this.sequence = castToPositiveInt(value); // PositiveIntType 2079 } else if (name.equals("diagnosis[x]")) { 2080 this.diagnosis = castToType(value); // Type 2081 } else if (name.equals("type")) { 2082 this.getType().add(castToCodeableConcept(value)); 2083 } else if (name.equals("packageCode")) { 2084 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2085 } else 2086 return super.setProperty(name, value); 2087 return value; 2088 } 2089 2090 @Override 2091 public Base makeProperty(int hash, String name) throws FHIRException { 2092 switch (hash) { 2093 case 1349547969: return getSequenceElement(); 2094 case -1487009809: return getDiagnosis(); 2095 case 1196993265: return getDiagnosis(); 2096 case 3575610: return addType(); 2097 case 908444499: return getPackageCode(); 2098 default: return super.makeProperty(hash, name); 2099 } 2100 2101 } 2102 2103 @Override 2104 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2105 switch (hash) { 2106 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2107 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 2108 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2109 case 908444499: /*packageCode*/ return new String[] {"CodeableConcept"}; 2110 default: return super.getTypesForProperty(hash, name); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base addChild(String name) throws FHIRException { 2117 if (name.equals("sequence")) { 2118 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2119 } 2120 else if (name.equals("diagnosisCodeableConcept")) { 2121 this.diagnosis = new CodeableConcept(); 2122 return this.diagnosis; 2123 } 2124 else if (name.equals("diagnosisReference")) { 2125 this.diagnosis = new Reference(); 2126 return this.diagnosis; 2127 } 2128 else if (name.equals("type")) { 2129 return addType(); 2130 } 2131 else if (name.equals("packageCode")) { 2132 this.packageCode = new CodeableConcept(); 2133 return this.packageCode; 2134 } 2135 else 2136 return super.addChild(name); 2137 } 2138 2139 public DiagnosisComponent copy() { 2140 DiagnosisComponent dst = new DiagnosisComponent(); 2141 copyValues(dst); 2142 dst.sequence = sequence == null ? null : sequence.copy(); 2143 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2144 if (type != null) { 2145 dst.type = new ArrayList<CodeableConcept>(); 2146 for (CodeableConcept i : type) 2147 dst.type.add(i.copy()); 2148 }; 2149 dst.packageCode = packageCode == null ? null : packageCode.copy(); 2150 return dst; 2151 } 2152 2153 @Override 2154 public boolean equalsDeep(Base other_) { 2155 if (!super.equalsDeep(other_)) 2156 return false; 2157 if (!(other_ instanceof DiagnosisComponent)) 2158 return false; 2159 DiagnosisComponent o = (DiagnosisComponent) other_; 2160 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2161 && compareDeep(packageCode, o.packageCode, true); 2162 } 2163 2164 @Override 2165 public boolean equalsShallow(Base other_) { 2166 if (!super.equalsShallow(other_)) 2167 return false; 2168 if (!(other_ instanceof DiagnosisComponent)) 2169 return false; 2170 DiagnosisComponent o = (DiagnosisComponent) other_; 2171 return compareValues(sequence, o.sequence, true); 2172 } 2173 2174 public boolean isEmpty() { 2175 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2176 , packageCode); 2177 } 2178 2179 public String fhirType() { 2180 return "Claim.diagnosis"; 2181 2182 } 2183 2184 } 2185 2186 @Block() 2187 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2188 /** 2189 * Sequence of procedures which serves to order and provide a link. 2190 */ 2191 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2192 @Description(shortDefinition="Procedure sequence for reference", formalDefinition="Sequence of procedures which serves to order and provide a link." ) 2193 protected PositiveIntType sequence; 2194 2195 /** 2196 * Date and optionally time the procedure was performed . 2197 */ 2198 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2199 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed ." ) 2200 protected DateTimeType date; 2201 2202 /** 2203 * The procedure code. 2204 */ 2205 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=3, min=1, max=1, modifier=false, summary=false) 2206 @Description(shortDefinition="Patient's list of procedures performed", formalDefinition="The procedure code." ) 2207 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2208 protected Type procedure; 2209 2210 private static final long serialVersionUID = 864307347L; 2211 2212 /** 2213 * Constructor 2214 */ 2215 public ProcedureComponent() { 2216 super(); 2217 } 2218 2219 /** 2220 * Constructor 2221 */ 2222 public ProcedureComponent(PositiveIntType sequence, Type procedure) { 2223 super(); 2224 this.sequence = sequence; 2225 this.procedure = procedure; 2226 } 2227 2228 /** 2229 * @return {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2230 */ 2231 public PositiveIntType getSequenceElement() { 2232 if (this.sequence == null) 2233 if (Configuration.errorOnAutoCreate()) 2234 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2235 else if (Configuration.doAutoCreate()) 2236 this.sequence = new PositiveIntType(); // bb 2237 return this.sequence; 2238 } 2239 2240 public boolean hasSequenceElement() { 2241 return this.sequence != null && !this.sequence.isEmpty(); 2242 } 2243 2244 public boolean hasSequence() { 2245 return this.sequence != null && !this.sequence.isEmpty(); 2246 } 2247 2248 /** 2249 * @param value {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2250 */ 2251 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2252 this.sequence = value; 2253 return this; 2254 } 2255 2256 /** 2257 * @return Sequence of procedures which serves to order and provide a link. 2258 */ 2259 public int getSequence() { 2260 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2261 } 2262 2263 /** 2264 * @param value Sequence of procedures which serves to order and provide a link. 2265 */ 2266 public ProcedureComponent setSequence(int value) { 2267 if (this.sequence == null) 2268 this.sequence = new PositiveIntType(); 2269 this.sequence.setValue(value); 2270 return this; 2271 } 2272 2273 /** 2274 * @return {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2275 */ 2276 public DateTimeType getDateElement() { 2277 if (this.date == null) 2278 if (Configuration.errorOnAutoCreate()) 2279 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2280 else if (Configuration.doAutoCreate()) 2281 this.date = new DateTimeType(); // bb 2282 return this.date; 2283 } 2284 2285 public boolean hasDateElement() { 2286 return this.date != null && !this.date.isEmpty(); 2287 } 2288 2289 public boolean hasDate() { 2290 return this.date != null && !this.date.isEmpty(); 2291 } 2292 2293 /** 2294 * @param value {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2295 */ 2296 public ProcedureComponent setDateElement(DateTimeType value) { 2297 this.date = value; 2298 return this; 2299 } 2300 2301 /** 2302 * @return Date and optionally time the procedure was performed . 2303 */ 2304 public Date getDate() { 2305 return this.date == null ? null : this.date.getValue(); 2306 } 2307 2308 /** 2309 * @param value Date and optionally time the procedure was performed . 2310 */ 2311 public ProcedureComponent setDate(Date value) { 2312 if (value == null) 2313 this.date = null; 2314 else { 2315 if (this.date == null) 2316 this.date = new DateTimeType(); 2317 this.date.setValue(value); 2318 } 2319 return this; 2320 } 2321 2322 /** 2323 * @return {@link #procedure} (The procedure code.) 2324 */ 2325 public Type getProcedure() { 2326 return this.procedure; 2327 } 2328 2329 /** 2330 * @return {@link #procedure} (The procedure code.) 2331 */ 2332 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2333 if (this.procedure == null) 2334 return null; 2335 if (!(this.procedure instanceof CodeableConcept)) 2336 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2337 return (CodeableConcept) this.procedure; 2338 } 2339 2340 public boolean hasProcedureCodeableConcept() { 2341 return this != null && this.procedure instanceof CodeableConcept; 2342 } 2343 2344 /** 2345 * @return {@link #procedure} (The procedure code.) 2346 */ 2347 public Reference getProcedureReference() throws FHIRException { 2348 if (this.procedure == null) 2349 return null; 2350 if (!(this.procedure instanceof Reference)) 2351 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2352 return (Reference) this.procedure; 2353 } 2354 2355 public boolean hasProcedureReference() { 2356 return this != null && this.procedure instanceof Reference; 2357 } 2358 2359 public boolean hasProcedure() { 2360 return this.procedure != null && !this.procedure.isEmpty(); 2361 } 2362 2363 /** 2364 * @param value {@link #procedure} (The procedure code.) 2365 */ 2366 public ProcedureComponent setProcedure(Type value) throws FHIRFormatError { 2367 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2368 throw new FHIRFormatError("Not the right type for Claim.procedure.procedure[x]: "+value.fhirType()); 2369 this.procedure = value; 2370 return this; 2371 } 2372 2373 protected void listChildren(List<Property> children) { 2374 super.listChildren(children); 2375 children.add(new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence)); 2376 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date)); 2377 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure)); 2378 } 2379 2380 @Override 2381 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2382 switch (_hash) { 2383 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence); 2384 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date); 2385 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2386 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2387 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2388 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2389 default: return super.getNamedProperty(_hash, _name, _checkValid); 2390 } 2391 2392 } 2393 2394 @Override 2395 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2396 switch (hash) { 2397 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2398 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2399 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // Type 2400 default: return super.getProperty(hash, name, checkValid); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2407 switch (hash) { 2408 case 1349547969: // sequence 2409 this.sequence = castToPositiveInt(value); // PositiveIntType 2410 return value; 2411 case 3076014: // date 2412 this.date = castToDateTime(value); // DateTimeType 2413 return value; 2414 case -1095204141: // procedure 2415 this.procedure = castToType(value); // Type 2416 return value; 2417 default: return super.setProperty(hash, name, value); 2418 } 2419 2420 } 2421 2422 @Override 2423 public Base setProperty(String name, Base value) throws FHIRException { 2424 if (name.equals("sequence")) { 2425 this.sequence = castToPositiveInt(value); // PositiveIntType 2426 } else if (name.equals("date")) { 2427 this.date = castToDateTime(value); // DateTimeType 2428 } else if (name.equals("procedure[x]")) { 2429 this.procedure = castToType(value); // Type 2430 } else 2431 return super.setProperty(name, value); 2432 return value; 2433 } 2434 2435 @Override 2436 public Base makeProperty(int hash, String name) throws FHIRException { 2437 switch (hash) { 2438 case 1349547969: return getSequenceElement(); 2439 case 3076014: return getDateElement(); 2440 case 1640074445: return getProcedure(); 2441 case -1095204141: return getProcedure(); 2442 default: return super.makeProperty(hash, name); 2443 } 2444 2445 } 2446 2447 @Override 2448 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2449 switch (hash) { 2450 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2451 case 3076014: /*date*/ return new String[] {"dateTime"}; 2452 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2453 default: return super.getTypesForProperty(hash, name); 2454 } 2455 2456 } 2457 2458 @Override 2459 public Base addChild(String name) throws FHIRException { 2460 if (name.equals("sequence")) { 2461 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2462 } 2463 else if (name.equals("date")) { 2464 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 2465 } 2466 else if (name.equals("procedureCodeableConcept")) { 2467 this.procedure = new CodeableConcept(); 2468 return this.procedure; 2469 } 2470 else if (name.equals("procedureReference")) { 2471 this.procedure = new Reference(); 2472 return this.procedure; 2473 } 2474 else 2475 return super.addChild(name); 2476 } 2477 2478 public ProcedureComponent copy() { 2479 ProcedureComponent dst = new ProcedureComponent(); 2480 copyValues(dst); 2481 dst.sequence = sequence == null ? null : sequence.copy(); 2482 dst.date = date == null ? null : date.copy(); 2483 dst.procedure = procedure == null ? null : procedure.copy(); 2484 return dst; 2485 } 2486 2487 @Override 2488 public boolean equalsDeep(Base other_) { 2489 if (!super.equalsDeep(other_)) 2490 return false; 2491 if (!(other_ instanceof ProcedureComponent)) 2492 return false; 2493 ProcedureComponent o = (ProcedureComponent) other_; 2494 return compareDeep(sequence, o.sequence, true) && compareDeep(date, o.date, true) && compareDeep(procedure, o.procedure, true) 2495 ; 2496 } 2497 2498 @Override 2499 public boolean equalsShallow(Base other_) { 2500 if (!super.equalsShallow(other_)) 2501 return false; 2502 if (!(other_ instanceof ProcedureComponent)) 2503 return false; 2504 ProcedureComponent o = (ProcedureComponent) other_; 2505 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2506 } 2507 2508 public boolean isEmpty() { 2509 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, date, procedure 2510 ); 2511 } 2512 2513 public String fhirType() { 2514 return "Claim.procedure"; 2515 2516 } 2517 2518 } 2519 2520 @Block() 2521 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2522 /** 2523 * Sequence of coverage which serves to provide a link and convey coordination of benefit order. 2524 */ 2525 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2526 @Description(shortDefinition="Service instance identifier", formalDefinition="Sequence of coverage which serves to provide a link and convey coordination of benefit order." ) 2527 protected PositiveIntType sequence; 2528 2529 /** 2530 * A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 2531 */ 2532 @Child(name = "focal", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2533 @Description(shortDefinition="Is the focal Coverage", formalDefinition="A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated." ) 2534 protected BooleanType focal; 2535 2536 /** 2537 * Reference to the program or plan identification, underwriter or payor. 2538 */ 2539 @Child(name = "coverage", type = {Coverage.class}, order=3, min=1, max=1, modifier=false, summary=false) 2540 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the program or plan identification, underwriter or payor." ) 2541 protected Reference coverage; 2542 2543 /** 2544 * The actual object that is the target of the reference (Reference to the program or plan identification, underwriter or payor.) 2545 */ 2546 protected Coverage coverageTarget; 2547 2548 /** 2549 * The contract number of a business agreement which describes the terms and conditions. 2550 */ 2551 @Child(name = "businessArrangement", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2552 @Description(shortDefinition="Business agreement", formalDefinition="The contract number of a business agreement which describes the terms and conditions." ) 2553 protected StringType businessArrangement; 2554 2555 /** 2556 * A list of references from the Insurer to which these services pertain. 2557 */ 2558 @Child(name = "preAuthRef", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2559 @Description(shortDefinition="Pre-Authorization/Determination Reference", formalDefinition="A list of references from the Insurer to which these services pertain." ) 2560 protected List<StringType> preAuthRef; 2561 2562 /** 2563 * The Coverages adjudication details. 2564 */ 2565 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=6, min=0, max=1, modifier=false, summary=false) 2566 @Description(shortDefinition="Adjudication results", formalDefinition="The Coverages adjudication details." ) 2567 protected Reference claimResponse; 2568 2569 /** 2570 * The actual object that is the target of the reference (The Coverages adjudication details.) 2571 */ 2572 protected ClaimResponse claimResponseTarget; 2573 2574 private static final long serialVersionUID = -1216535489L; 2575 2576 /** 2577 * Constructor 2578 */ 2579 public InsuranceComponent() { 2580 super(); 2581 } 2582 2583 /** 2584 * Constructor 2585 */ 2586 public InsuranceComponent(PositiveIntType sequence, BooleanType focal, Reference coverage) { 2587 super(); 2588 this.sequence = sequence; 2589 this.focal = focal; 2590 this.coverage = coverage; 2591 } 2592 2593 /** 2594 * @return {@link #sequence} (Sequence of coverage which serves to provide a link and convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2595 */ 2596 public PositiveIntType getSequenceElement() { 2597 if (this.sequence == null) 2598 if (Configuration.errorOnAutoCreate()) 2599 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 2600 else if (Configuration.doAutoCreate()) 2601 this.sequence = new PositiveIntType(); // bb 2602 return this.sequence; 2603 } 2604 2605 public boolean hasSequenceElement() { 2606 return this.sequence != null && !this.sequence.isEmpty(); 2607 } 2608 2609 public boolean hasSequence() { 2610 return this.sequence != null && !this.sequence.isEmpty(); 2611 } 2612 2613 /** 2614 * @param value {@link #sequence} (Sequence of coverage which serves to provide a link and convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2615 */ 2616 public InsuranceComponent setSequenceElement(PositiveIntType value) { 2617 this.sequence = value; 2618 return this; 2619 } 2620 2621 /** 2622 * @return Sequence of coverage which serves to provide a link and convey coordination of benefit order. 2623 */ 2624 public int getSequence() { 2625 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2626 } 2627 2628 /** 2629 * @param value Sequence of coverage which serves to provide a link and convey coordination of benefit order. 2630 */ 2631 public InsuranceComponent setSequence(int value) { 2632 if (this.sequence == null) 2633 this.sequence = new PositiveIntType(); 2634 this.sequence.setValue(value); 2635 return this; 2636 } 2637 2638 /** 2639 * @return {@link #focal} (A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2640 */ 2641 public BooleanType getFocalElement() { 2642 if (this.focal == null) 2643 if (Configuration.errorOnAutoCreate()) 2644 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 2645 else if (Configuration.doAutoCreate()) 2646 this.focal = new BooleanType(); // bb 2647 return this.focal; 2648 } 2649 2650 public boolean hasFocalElement() { 2651 return this.focal != null && !this.focal.isEmpty(); 2652 } 2653 2654 public boolean hasFocal() { 2655 return this.focal != null && !this.focal.isEmpty(); 2656 } 2657 2658 /** 2659 * @param value {@link #focal} (A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2660 */ 2661 public InsuranceComponent setFocalElement(BooleanType value) { 2662 this.focal = value; 2663 return this; 2664 } 2665 2666 /** 2667 * @return A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 2668 */ 2669 public boolean getFocal() { 2670 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 2671 } 2672 2673 /** 2674 * @param value A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 2675 */ 2676 public InsuranceComponent setFocal(boolean value) { 2677 if (this.focal == null) 2678 this.focal = new BooleanType(); 2679 this.focal.setValue(value); 2680 return this; 2681 } 2682 2683 /** 2684 * @return {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2685 */ 2686 public Reference getCoverage() { 2687 if (this.coverage == null) 2688 if (Configuration.errorOnAutoCreate()) 2689 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2690 else if (Configuration.doAutoCreate()) 2691 this.coverage = new Reference(); // cc 2692 return this.coverage; 2693 } 2694 2695 public boolean hasCoverage() { 2696 return this.coverage != null && !this.coverage.isEmpty(); 2697 } 2698 2699 /** 2700 * @param value {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2701 */ 2702 public InsuranceComponent setCoverage(Reference value) { 2703 this.coverage = value; 2704 return this; 2705 } 2706 2707 /** 2708 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2709 */ 2710 public Coverage getCoverageTarget() { 2711 if (this.coverageTarget == null) 2712 if (Configuration.errorOnAutoCreate()) 2713 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2714 else if (Configuration.doAutoCreate()) 2715 this.coverageTarget = new Coverage(); // aa 2716 return this.coverageTarget; 2717 } 2718 2719 /** 2720 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2721 */ 2722 public InsuranceComponent setCoverageTarget(Coverage value) { 2723 this.coverageTarget = value; 2724 return this; 2725 } 2726 2727 /** 2728 * @return {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2729 */ 2730 public StringType getBusinessArrangementElement() { 2731 if (this.businessArrangement == null) 2732 if (Configuration.errorOnAutoCreate()) 2733 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 2734 else if (Configuration.doAutoCreate()) 2735 this.businessArrangement = new StringType(); // bb 2736 return this.businessArrangement; 2737 } 2738 2739 public boolean hasBusinessArrangementElement() { 2740 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2741 } 2742 2743 public boolean hasBusinessArrangement() { 2744 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2745 } 2746 2747 /** 2748 * @param value {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2749 */ 2750 public InsuranceComponent setBusinessArrangementElement(StringType value) { 2751 this.businessArrangement = value; 2752 return this; 2753 } 2754 2755 /** 2756 * @return The contract number of a business agreement which describes the terms and conditions. 2757 */ 2758 public String getBusinessArrangement() { 2759 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 2760 } 2761 2762 /** 2763 * @param value The contract number of a business agreement which describes the terms and conditions. 2764 */ 2765 public InsuranceComponent setBusinessArrangement(String value) { 2766 if (Utilities.noString(value)) 2767 this.businessArrangement = null; 2768 else { 2769 if (this.businessArrangement == null) 2770 this.businessArrangement = new StringType(); 2771 this.businessArrangement.setValue(value); 2772 } 2773 return this; 2774 } 2775 2776 /** 2777 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2778 */ 2779 public List<StringType> getPreAuthRef() { 2780 if (this.preAuthRef == null) 2781 this.preAuthRef = new ArrayList<StringType>(); 2782 return this.preAuthRef; 2783 } 2784 2785 /** 2786 * @return Returns a reference to <code>this</code> for easy method chaining 2787 */ 2788 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2789 this.preAuthRef = thePreAuthRef; 2790 return this; 2791 } 2792 2793 public boolean hasPreAuthRef() { 2794 if (this.preAuthRef == null) 2795 return false; 2796 for (StringType item : this.preAuthRef) 2797 if (!item.isEmpty()) 2798 return true; 2799 return false; 2800 } 2801 2802 /** 2803 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2804 */ 2805 public StringType addPreAuthRefElement() {//2 2806 StringType t = new StringType(); 2807 if (this.preAuthRef == null) 2808 this.preAuthRef = new ArrayList<StringType>(); 2809 this.preAuthRef.add(t); 2810 return t; 2811 } 2812 2813 /** 2814 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2815 */ 2816 public InsuranceComponent addPreAuthRef(String value) { //1 2817 StringType t = new StringType(); 2818 t.setValue(value); 2819 if (this.preAuthRef == null) 2820 this.preAuthRef = new ArrayList<StringType>(); 2821 this.preAuthRef.add(t); 2822 return this; 2823 } 2824 2825 /** 2826 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2827 */ 2828 public boolean hasPreAuthRef(String value) { 2829 if (this.preAuthRef == null) 2830 return false; 2831 for (StringType v : this.preAuthRef) 2832 if (v.getValue().equals(value)) // string 2833 return true; 2834 return false; 2835 } 2836 2837 /** 2838 * @return {@link #claimResponse} (The Coverages adjudication details.) 2839 */ 2840 public Reference getClaimResponse() { 2841 if (this.claimResponse == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 2844 else if (Configuration.doAutoCreate()) 2845 this.claimResponse = new Reference(); // cc 2846 return this.claimResponse; 2847 } 2848 2849 public boolean hasClaimResponse() { 2850 return this.claimResponse != null && !this.claimResponse.isEmpty(); 2851 } 2852 2853 /** 2854 * @param value {@link #claimResponse} (The Coverages adjudication details.) 2855 */ 2856 public InsuranceComponent setClaimResponse(Reference value) { 2857 this.claimResponse = value; 2858 return this; 2859 } 2860 2861 /** 2862 * @return {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Coverages adjudication details.) 2863 */ 2864 public ClaimResponse getClaimResponseTarget() { 2865 if (this.claimResponseTarget == null) 2866 if (Configuration.errorOnAutoCreate()) 2867 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 2868 else if (Configuration.doAutoCreate()) 2869 this.claimResponseTarget = new ClaimResponse(); // aa 2870 return this.claimResponseTarget; 2871 } 2872 2873 /** 2874 * @param value {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Coverages adjudication details.) 2875 */ 2876 public InsuranceComponent setClaimResponseTarget(ClaimResponse value) { 2877 this.claimResponseTarget = value; 2878 return this; 2879 } 2880 2881 protected void listChildren(List<Property> children) { 2882 super.listChildren(children); 2883 children.add(new Property("sequence", "positiveInt", "Sequence of coverage which serves to provide a link and convey coordination of benefit order.", 0, 1, sequence)); 2884 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 0, 1, focal)); 2885 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 2886 children.add(new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement)); 2887 children.add(new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 2888 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 0, 1, claimResponse)); 2889 } 2890 2891 @Override 2892 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2893 switch (_hash) { 2894 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of coverage which serves to provide a link and convey coordination of benefit order.", 0, 1, sequence); 2895 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 0, 1, focal); 2896 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 2897 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement); 2898 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 2899 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 0, 1, claimResponse); 2900 default: return super.getNamedProperty(_hash, _name, _checkValid); 2901 } 2902 2903 } 2904 2905 @Override 2906 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2907 switch (hash) { 2908 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2909 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 2910 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 2911 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 2912 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 2913 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 2914 default: return super.getProperty(hash, name, checkValid); 2915 } 2916 2917 } 2918 2919 @Override 2920 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2921 switch (hash) { 2922 case 1349547969: // sequence 2923 this.sequence = castToPositiveInt(value); // PositiveIntType 2924 return value; 2925 case 97604197: // focal 2926 this.focal = castToBoolean(value); // BooleanType 2927 return value; 2928 case -351767064: // coverage 2929 this.coverage = castToReference(value); // Reference 2930 return value; 2931 case 259920682: // businessArrangement 2932 this.businessArrangement = castToString(value); // StringType 2933 return value; 2934 case 522246568: // preAuthRef 2935 this.getPreAuthRef().add(castToString(value)); // StringType 2936 return value; 2937 case 689513629: // claimResponse 2938 this.claimResponse = castToReference(value); // Reference 2939 return value; 2940 default: return super.setProperty(hash, name, value); 2941 } 2942 2943 } 2944 2945 @Override 2946 public Base setProperty(String name, Base value) throws FHIRException { 2947 if (name.equals("sequence")) { 2948 this.sequence = castToPositiveInt(value); // PositiveIntType 2949 } else if (name.equals("focal")) { 2950 this.focal = castToBoolean(value); // BooleanType 2951 } else if (name.equals("coverage")) { 2952 this.coverage = castToReference(value); // Reference 2953 } else if (name.equals("businessArrangement")) { 2954 this.businessArrangement = castToString(value); // StringType 2955 } else if (name.equals("preAuthRef")) { 2956 this.getPreAuthRef().add(castToString(value)); 2957 } else if (name.equals("claimResponse")) { 2958 this.claimResponse = castToReference(value); // Reference 2959 } else 2960 return super.setProperty(name, value); 2961 return value; 2962 } 2963 2964 @Override 2965 public Base makeProperty(int hash, String name) throws FHIRException { 2966 switch (hash) { 2967 case 1349547969: return getSequenceElement(); 2968 case 97604197: return getFocalElement(); 2969 case -351767064: return getCoverage(); 2970 case 259920682: return getBusinessArrangementElement(); 2971 case 522246568: return addPreAuthRefElement(); 2972 case 689513629: return getClaimResponse(); 2973 default: return super.makeProperty(hash, name); 2974 } 2975 2976 } 2977 2978 @Override 2979 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2980 switch (hash) { 2981 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2982 case 97604197: /*focal*/ return new String[] {"boolean"}; 2983 case -351767064: /*coverage*/ return new String[] {"Reference"}; 2984 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 2985 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 2986 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 2987 default: return super.getTypesForProperty(hash, name); 2988 } 2989 2990 } 2991 2992 @Override 2993 public Base addChild(String name) throws FHIRException { 2994 if (name.equals("sequence")) { 2995 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2996 } 2997 else if (name.equals("focal")) { 2998 throw new FHIRException("Cannot call addChild on a singleton property Claim.focal"); 2999 } 3000 else if (name.equals("coverage")) { 3001 this.coverage = new Reference(); 3002 return this.coverage; 3003 } 3004 else if (name.equals("businessArrangement")) { 3005 throw new FHIRException("Cannot call addChild on a singleton property Claim.businessArrangement"); 3006 } 3007 else if (name.equals("preAuthRef")) { 3008 throw new FHIRException("Cannot call addChild on a singleton property Claim.preAuthRef"); 3009 } 3010 else if (name.equals("claimResponse")) { 3011 this.claimResponse = new Reference(); 3012 return this.claimResponse; 3013 } 3014 else 3015 return super.addChild(name); 3016 } 3017 3018 public InsuranceComponent copy() { 3019 InsuranceComponent dst = new InsuranceComponent(); 3020 copyValues(dst); 3021 dst.sequence = sequence == null ? null : sequence.copy(); 3022 dst.focal = focal == null ? null : focal.copy(); 3023 dst.coverage = coverage == null ? null : coverage.copy(); 3024 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 3025 if (preAuthRef != null) { 3026 dst.preAuthRef = new ArrayList<StringType>(); 3027 for (StringType i : preAuthRef) 3028 dst.preAuthRef.add(i.copy()); 3029 }; 3030 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 3031 return dst; 3032 } 3033 3034 @Override 3035 public boolean equalsDeep(Base other_) { 3036 if (!super.equalsDeep(other_)) 3037 return false; 3038 if (!(other_ instanceof InsuranceComponent)) 3039 return false; 3040 InsuranceComponent o = (InsuranceComponent) other_; 3041 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) 3042 && compareDeep(businessArrangement, o.businessArrangement, true) && compareDeep(preAuthRef, o.preAuthRef, true) 3043 && compareDeep(claimResponse, o.claimResponse, true); 3044 } 3045 3046 @Override 3047 public boolean equalsShallow(Base other_) { 3048 if (!super.equalsShallow(other_)) 3049 return false; 3050 if (!(other_ instanceof InsuranceComponent)) 3051 return false; 3052 InsuranceComponent o = (InsuranceComponent) other_; 3053 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true) 3054 && compareValues(preAuthRef, o.preAuthRef, true); 3055 } 3056 3057 public boolean isEmpty() { 3058 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, coverage 3059 , businessArrangement, preAuthRef, claimResponse); 3060 } 3061 3062 public String fhirType() { 3063 return "Claim.insurance"; 3064 3065 } 3066 3067 } 3068 3069 @Block() 3070 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 3071 /** 3072 * Date of an accident which these services are addressing. 3073 */ 3074 @Child(name = "date", type = {DateType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3075 @Description(shortDefinition="When the accident occurred\nsee information codes\nsee information codes", formalDefinition="Date of an accident which these services are addressing." ) 3076 protected DateType date; 3077 3078 /** 3079 * Type of accident: work, auto, etc. 3080 */ 3081 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3082 @Description(shortDefinition="The nature of the accident", formalDefinition="Type of accident: work, auto, etc." ) 3083 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActIncidentCode") 3084 protected CodeableConcept type; 3085 3086 /** 3087 * Accident Place. 3088 */ 3089 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 3090 @Description(shortDefinition="Accident Place", formalDefinition="Accident Place." ) 3091 protected Type location; 3092 3093 private static final long serialVersionUID = 622904984L; 3094 3095 /** 3096 * Constructor 3097 */ 3098 public AccidentComponent() { 3099 super(); 3100 } 3101 3102 /** 3103 * Constructor 3104 */ 3105 public AccidentComponent(DateType date) { 3106 super(); 3107 this.date = date; 3108 } 3109 3110 /** 3111 * @return {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3112 */ 3113 public DateType getDateElement() { 3114 if (this.date == null) 3115 if (Configuration.errorOnAutoCreate()) 3116 throw new Error("Attempt to auto-create AccidentComponent.date"); 3117 else if (Configuration.doAutoCreate()) 3118 this.date = new DateType(); // bb 3119 return this.date; 3120 } 3121 3122 public boolean hasDateElement() { 3123 return this.date != null && !this.date.isEmpty(); 3124 } 3125 3126 public boolean hasDate() { 3127 return this.date != null && !this.date.isEmpty(); 3128 } 3129 3130 /** 3131 * @param value {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3132 */ 3133 public AccidentComponent setDateElement(DateType value) { 3134 this.date = value; 3135 return this; 3136 } 3137 3138 /** 3139 * @return Date of an accident which these services are addressing. 3140 */ 3141 public Date getDate() { 3142 return this.date == null ? null : this.date.getValue(); 3143 } 3144 3145 /** 3146 * @param value Date of an accident which these services are addressing. 3147 */ 3148 public AccidentComponent setDate(Date value) { 3149 if (this.date == null) 3150 this.date = new DateType(); 3151 this.date.setValue(value); 3152 return this; 3153 } 3154 3155 /** 3156 * @return {@link #type} (Type of accident: work, auto, etc.) 3157 */ 3158 public CodeableConcept getType() { 3159 if (this.type == null) 3160 if (Configuration.errorOnAutoCreate()) 3161 throw new Error("Attempt to auto-create AccidentComponent.type"); 3162 else if (Configuration.doAutoCreate()) 3163 this.type = new CodeableConcept(); // cc 3164 return this.type; 3165 } 3166 3167 public boolean hasType() { 3168 return this.type != null && !this.type.isEmpty(); 3169 } 3170 3171 /** 3172 * @param value {@link #type} (Type of accident: work, auto, etc.) 3173 */ 3174 public AccidentComponent setType(CodeableConcept value) { 3175 this.type = value; 3176 return this; 3177 } 3178 3179 /** 3180 * @return {@link #location} (Accident Place.) 3181 */ 3182 public Type getLocation() { 3183 return this.location; 3184 } 3185 3186 /** 3187 * @return {@link #location} (Accident Place.) 3188 */ 3189 public Address getLocationAddress() throws FHIRException { 3190 if (this.location == null) 3191 return null; 3192 if (!(this.location instanceof Address)) 3193 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3194 return (Address) this.location; 3195 } 3196 3197 public boolean hasLocationAddress() { 3198 return this != null && this.location instanceof Address; 3199 } 3200 3201 /** 3202 * @return {@link #location} (Accident Place.) 3203 */ 3204 public Reference getLocationReference() throws FHIRException { 3205 if (this.location == null) 3206 return null; 3207 if (!(this.location instanceof Reference)) 3208 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3209 return (Reference) this.location; 3210 } 3211 3212 public boolean hasLocationReference() { 3213 return this != null && this.location instanceof Reference; 3214 } 3215 3216 public boolean hasLocation() { 3217 return this.location != null && !this.location.isEmpty(); 3218 } 3219 3220 /** 3221 * @param value {@link #location} (Accident Place.) 3222 */ 3223 public AccidentComponent setLocation(Type value) throws FHIRFormatError { 3224 if (value != null && !(value instanceof Address || value instanceof Reference)) 3225 throw new FHIRFormatError("Not the right type for Claim.accident.location[x]: "+value.fhirType()); 3226 this.location = value; 3227 return this; 3228 } 3229 3230 protected void listChildren(List<Property> children) { 3231 super.listChildren(children); 3232 children.add(new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date)); 3233 children.add(new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type)); 3234 children.add(new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location)); 3235 } 3236 3237 @Override 3238 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3239 switch (_hash) { 3240 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date); 3241 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type); 3242 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3243 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3244 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3245 case 755866390: /*locationReference*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3246 default: return super.getNamedProperty(_hash, _name, _checkValid); 3247 } 3248 3249 } 3250 3251 @Override 3252 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3253 switch (hash) { 3254 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3255 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3256 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 3257 default: return super.getProperty(hash, name, checkValid); 3258 } 3259 3260 } 3261 3262 @Override 3263 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3264 switch (hash) { 3265 case 3076014: // date 3266 this.date = castToDate(value); // DateType 3267 return value; 3268 case 3575610: // type 3269 this.type = castToCodeableConcept(value); // CodeableConcept 3270 return value; 3271 case 1901043637: // location 3272 this.location = castToType(value); // Type 3273 return value; 3274 default: return super.setProperty(hash, name, value); 3275 } 3276 3277 } 3278 3279 @Override 3280 public Base setProperty(String name, Base value) throws FHIRException { 3281 if (name.equals("date")) { 3282 this.date = castToDate(value); // DateType 3283 } else if (name.equals("type")) { 3284 this.type = castToCodeableConcept(value); // CodeableConcept 3285 } else if (name.equals("location[x]")) { 3286 this.location = castToType(value); // Type 3287 } else 3288 return super.setProperty(name, value); 3289 return value; 3290 } 3291 3292 @Override 3293 public Base makeProperty(int hash, String name) throws FHIRException { 3294 switch (hash) { 3295 case 3076014: return getDateElement(); 3296 case 3575610: return getType(); 3297 case 552316075: return getLocation(); 3298 case 1901043637: return getLocation(); 3299 default: return super.makeProperty(hash, name); 3300 } 3301 3302 } 3303 3304 @Override 3305 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3306 switch (hash) { 3307 case 3076014: /*date*/ return new String[] {"date"}; 3308 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3309 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 3310 default: return super.getTypesForProperty(hash, name); 3311 } 3312 3313 } 3314 3315 @Override 3316 public Base addChild(String name) throws FHIRException { 3317 if (name.equals("date")) { 3318 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 3319 } 3320 else if (name.equals("type")) { 3321 this.type = new CodeableConcept(); 3322 return this.type; 3323 } 3324 else if (name.equals("locationAddress")) { 3325 this.location = new Address(); 3326 return this.location; 3327 } 3328 else if (name.equals("locationReference")) { 3329 this.location = new Reference(); 3330 return this.location; 3331 } 3332 else 3333 return super.addChild(name); 3334 } 3335 3336 public AccidentComponent copy() { 3337 AccidentComponent dst = new AccidentComponent(); 3338 copyValues(dst); 3339 dst.date = date == null ? null : date.copy(); 3340 dst.type = type == null ? null : type.copy(); 3341 dst.location = location == null ? null : location.copy(); 3342 return dst; 3343 } 3344 3345 @Override 3346 public boolean equalsDeep(Base other_) { 3347 if (!super.equalsDeep(other_)) 3348 return false; 3349 if (!(other_ instanceof AccidentComponent)) 3350 return false; 3351 AccidentComponent o = (AccidentComponent) other_; 3352 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 3353 ; 3354 } 3355 3356 @Override 3357 public boolean equalsShallow(Base other_) { 3358 if (!super.equalsShallow(other_)) 3359 return false; 3360 if (!(other_ instanceof AccidentComponent)) 3361 return false; 3362 AccidentComponent o = (AccidentComponent) other_; 3363 return compareValues(date, o.date, true); 3364 } 3365 3366 public boolean isEmpty() { 3367 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 3368 } 3369 3370 public String fhirType() { 3371 return "Claim.accident"; 3372 3373 } 3374 3375 } 3376 3377 @Block() 3378 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 3379 /** 3380 * A service line number. 3381 */ 3382 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3383 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 3384 protected PositiveIntType sequence; 3385 3386 /** 3387 * CareTeam applicable for this service or product line. 3388 */ 3389 @Child(name = "careTeamLinkId", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3390 @Description(shortDefinition="Applicable careTeam members", formalDefinition="CareTeam applicable for this service or product line." ) 3391 protected List<PositiveIntType> careTeamLinkId; 3392 3393 /** 3394 * Diagnosis applicable for this service or product line. 3395 */ 3396 @Child(name = "diagnosisLinkId", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3397 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnosis applicable for this service or product line." ) 3398 protected List<PositiveIntType> diagnosisLinkId; 3399 3400 /** 3401 * Procedures applicable for this service or product line. 3402 */ 3403 @Child(name = "procedureLinkId", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3404 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product line." ) 3405 protected List<PositiveIntType> procedureLinkId; 3406 3407 /** 3408 * Exceptions, special conditions and supporting information pplicable for this service or product line. 3409 */ 3410 @Child(name = "informationLinkId", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3411 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information pplicable for this service or product line." ) 3412 protected List<PositiveIntType> informationLinkId; 3413 3414 /** 3415 * The type of reveneu or cost center providing the product and/or service. 3416 */ 3417 @Child(name = "revenue", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 3418 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 3419 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 3420 protected CodeableConcept revenue; 3421 3422 /** 3423 * Health Care Service Type Codes to identify the classification of service or benefits. 3424 */ 3425 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 3426 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 3427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 3428 protected CodeableConcept category; 3429 3430 /** 3431 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 3432 */ 3433 @Child(name = "service", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3434 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 3435 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3436 protected CodeableConcept service; 3437 3438 /** 3439 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 3440 */ 3441 @Child(name = "modifier", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3442 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 3443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3444 protected List<CodeableConcept> modifier; 3445 3446 /** 3447 * For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program. 3448 */ 3449 @Child(name = "programCode", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3450 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program." ) 3451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3452 protected List<CodeableConcept> programCode; 3453 3454 /** 3455 * The date or dates when the enclosed suite of services were performed or completed. 3456 */ 3457 @Child(name = "serviced", type = {DateType.class, Period.class}, order=11, min=0, max=1, modifier=false, summary=false) 3458 @Description(shortDefinition="Date or dates of Service", formalDefinition="The date or dates when the enclosed suite of services were performed or completed." ) 3459 protected Type serviced; 3460 3461 /** 3462 * Where the service was provided. 3463 */ 3464 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 3465 @Description(shortDefinition="Place of service", formalDefinition="Where the service was provided." ) 3466 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3467 protected Type location; 3468 3469 /** 3470 * The number of repetitions of a service or product. 3471 */ 3472 @Child(name = "quantity", type = {SimpleQuantity.class}, order=13, min=0, max=1, modifier=false, summary=false) 3473 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 3474 protected SimpleQuantity quantity; 3475 3476 /** 3477 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 3478 */ 3479 @Child(name = "unitPrice", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 3480 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 3481 protected Money unitPrice; 3482 3483 /** 3484 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3485 */ 3486 @Child(name = "factor", type = {DecimalType.class}, order=15, min=0, max=1, modifier=false, summary=false) 3487 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3488 protected DecimalType factor; 3489 3490 /** 3491 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 3492 */ 3493 @Child(name = "net", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3494 @Description(shortDefinition="Total item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 3495 protected Money net; 3496 3497 /** 3498 * List of Unique Device Identifiers associated with this line item. 3499 */ 3500 @Child(name = "udi", type = {Device.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3501 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 3502 protected List<Reference> udi; 3503 /** 3504 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 3505 */ 3506 protected List<Device> udiTarget; 3507 3508 3509 /** 3510 * Physical service site on the patient (limb, tooth, etc). 3511 */ 3512 @Child(name = "bodySite", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=false) 3513 @Description(shortDefinition="Service Location", formalDefinition="Physical service site on the patient (limb, tooth, etc)." ) 3514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 3515 protected CodeableConcept bodySite; 3516 3517 /** 3518 * A region or surface of the site, eg. limb region or tooth surface(s). 3519 */ 3520 @Child(name = "subSite", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3521 @Description(shortDefinition="Service Sub-location", formalDefinition="A region or surface of the site, eg. limb region or tooth surface(s)." ) 3522 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 3523 protected List<CodeableConcept> subSite; 3524 3525 /** 3526 * A billed item may include goods or services provided in multiple encounters. 3527 */ 3528 @Child(name = "encounter", type = {Encounter.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3529 @Description(shortDefinition="Encounters related to this billed item", formalDefinition="A billed item may include goods or services provided in multiple encounters." ) 3530 protected List<Reference> encounter; 3531 /** 3532 * The actual objects that are the target of the reference (A billed item may include goods or services provided in multiple encounters.) 3533 */ 3534 protected List<Encounter> encounterTarget; 3535 3536 3537 /** 3538 * Second tier of goods and services. 3539 */ 3540 @Child(name = "detail", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3541 @Description(shortDefinition="Additional items", formalDefinition="Second tier of goods and services." ) 3542 protected List<DetailComponent> detail; 3543 3544 private static final long serialVersionUID = 784765825L; 3545 3546 /** 3547 * Constructor 3548 */ 3549 public ItemComponent() { 3550 super(); 3551 } 3552 3553 /** 3554 * Constructor 3555 */ 3556 public ItemComponent(PositiveIntType sequence) { 3557 super(); 3558 this.sequence = sequence; 3559 } 3560 3561 /** 3562 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3563 */ 3564 public PositiveIntType getSequenceElement() { 3565 if (this.sequence == null) 3566 if (Configuration.errorOnAutoCreate()) 3567 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3568 else if (Configuration.doAutoCreate()) 3569 this.sequence = new PositiveIntType(); // bb 3570 return this.sequence; 3571 } 3572 3573 public boolean hasSequenceElement() { 3574 return this.sequence != null && !this.sequence.isEmpty(); 3575 } 3576 3577 public boolean hasSequence() { 3578 return this.sequence != null && !this.sequence.isEmpty(); 3579 } 3580 3581 /** 3582 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3583 */ 3584 public ItemComponent setSequenceElement(PositiveIntType value) { 3585 this.sequence = value; 3586 return this; 3587 } 3588 3589 /** 3590 * @return A service line number. 3591 */ 3592 public int getSequence() { 3593 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3594 } 3595 3596 /** 3597 * @param value A service line number. 3598 */ 3599 public ItemComponent setSequence(int value) { 3600 if (this.sequence == null) 3601 this.sequence = new PositiveIntType(); 3602 this.sequence.setValue(value); 3603 return this; 3604 } 3605 3606 /** 3607 * @return {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3608 */ 3609 public List<PositiveIntType> getCareTeamLinkId() { 3610 if (this.careTeamLinkId == null) 3611 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3612 return this.careTeamLinkId; 3613 } 3614 3615 /** 3616 * @return Returns a reference to <code>this</code> for easy method chaining 3617 */ 3618 public ItemComponent setCareTeamLinkId(List<PositiveIntType> theCareTeamLinkId) { 3619 this.careTeamLinkId = theCareTeamLinkId; 3620 return this; 3621 } 3622 3623 public boolean hasCareTeamLinkId() { 3624 if (this.careTeamLinkId == null) 3625 return false; 3626 for (PositiveIntType item : this.careTeamLinkId) 3627 if (!item.isEmpty()) 3628 return true; 3629 return false; 3630 } 3631 3632 /** 3633 * @return {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3634 */ 3635 public PositiveIntType addCareTeamLinkIdElement() {//2 3636 PositiveIntType t = new PositiveIntType(); 3637 if (this.careTeamLinkId == null) 3638 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3639 this.careTeamLinkId.add(t); 3640 return t; 3641 } 3642 3643 /** 3644 * @param value {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3645 */ 3646 public ItemComponent addCareTeamLinkId(int value) { //1 3647 PositiveIntType t = new PositiveIntType(); 3648 t.setValue(value); 3649 if (this.careTeamLinkId == null) 3650 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3651 this.careTeamLinkId.add(t); 3652 return this; 3653 } 3654 3655 /** 3656 * @param value {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3657 */ 3658 public boolean hasCareTeamLinkId(int value) { 3659 if (this.careTeamLinkId == null) 3660 return false; 3661 for (PositiveIntType v : this.careTeamLinkId) 3662 if (v.getValue().equals(value)) // positiveInt 3663 return true; 3664 return false; 3665 } 3666 3667 /** 3668 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3669 */ 3670 public List<PositiveIntType> getDiagnosisLinkId() { 3671 if (this.diagnosisLinkId == null) 3672 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3673 return this.diagnosisLinkId; 3674 } 3675 3676 /** 3677 * @return Returns a reference to <code>this</code> for easy method chaining 3678 */ 3679 public ItemComponent setDiagnosisLinkId(List<PositiveIntType> theDiagnosisLinkId) { 3680 this.diagnosisLinkId = theDiagnosisLinkId; 3681 return this; 3682 } 3683 3684 public boolean hasDiagnosisLinkId() { 3685 if (this.diagnosisLinkId == null) 3686 return false; 3687 for (PositiveIntType item : this.diagnosisLinkId) 3688 if (!item.isEmpty()) 3689 return true; 3690 return false; 3691 } 3692 3693 /** 3694 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3695 */ 3696 public PositiveIntType addDiagnosisLinkIdElement() {//2 3697 PositiveIntType t = new PositiveIntType(); 3698 if (this.diagnosisLinkId == null) 3699 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3700 this.diagnosisLinkId.add(t); 3701 return t; 3702 } 3703 3704 /** 3705 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3706 */ 3707 public ItemComponent addDiagnosisLinkId(int value) { //1 3708 PositiveIntType t = new PositiveIntType(); 3709 t.setValue(value); 3710 if (this.diagnosisLinkId == null) 3711 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3712 this.diagnosisLinkId.add(t); 3713 return this; 3714 } 3715 3716 /** 3717 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3718 */ 3719 public boolean hasDiagnosisLinkId(int value) { 3720 if (this.diagnosisLinkId == null) 3721 return false; 3722 for (PositiveIntType v : this.diagnosisLinkId) 3723 if (v.getValue().equals(value)) // positiveInt 3724 return true; 3725 return false; 3726 } 3727 3728 /** 3729 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3730 */ 3731 public List<PositiveIntType> getProcedureLinkId() { 3732 if (this.procedureLinkId == null) 3733 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3734 return this.procedureLinkId; 3735 } 3736 3737 /** 3738 * @return Returns a reference to <code>this</code> for easy method chaining 3739 */ 3740 public ItemComponent setProcedureLinkId(List<PositiveIntType> theProcedureLinkId) { 3741 this.procedureLinkId = theProcedureLinkId; 3742 return this; 3743 } 3744 3745 public boolean hasProcedureLinkId() { 3746 if (this.procedureLinkId == null) 3747 return false; 3748 for (PositiveIntType item : this.procedureLinkId) 3749 if (!item.isEmpty()) 3750 return true; 3751 return false; 3752 } 3753 3754 /** 3755 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3756 */ 3757 public PositiveIntType addProcedureLinkIdElement() {//2 3758 PositiveIntType t = new PositiveIntType(); 3759 if (this.procedureLinkId == null) 3760 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3761 this.procedureLinkId.add(t); 3762 return t; 3763 } 3764 3765 /** 3766 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3767 */ 3768 public ItemComponent addProcedureLinkId(int value) { //1 3769 PositiveIntType t = new PositiveIntType(); 3770 t.setValue(value); 3771 if (this.procedureLinkId == null) 3772 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3773 this.procedureLinkId.add(t); 3774 return this; 3775 } 3776 3777 /** 3778 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3779 */ 3780 public boolean hasProcedureLinkId(int value) { 3781 if (this.procedureLinkId == null) 3782 return false; 3783 for (PositiveIntType v : this.procedureLinkId) 3784 if (v.getValue().equals(value)) // positiveInt 3785 return true; 3786 return false; 3787 } 3788 3789 /** 3790 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3791 */ 3792 public List<PositiveIntType> getInformationLinkId() { 3793 if (this.informationLinkId == null) 3794 this.informationLinkId = new ArrayList<PositiveIntType>(); 3795 return this.informationLinkId; 3796 } 3797 3798 /** 3799 * @return Returns a reference to <code>this</code> for easy method chaining 3800 */ 3801 public ItemComponent setInformationLinkId(List<PositiveIntType> theInformationLinkId) { 3802 this.informationLinkId = theInformationLinkId; 3803 return this; 3804 } 3805 3806 public boolean hasInformationLinkId() { 3807 if (this.informationLinkId == null) 3808 return false; 3809 for (PositiveIntType item : this.informationLinkId) 3810 if (!item.isEmpty()) 3811 return true; 3812 return false; 3813 } 3814 3815 /** 3816 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3817 */ 3818 public PositiveIntType addInformationLinkIdElement() {//2 3819 PositiveIntType t = new PositiveIntType(); 3820 if (this.informationLinkId == null) 3821 this.informationLinkId = new ArrayList<PositiveIntType>(); 3822 this.informationLinkId.add(t); 3823 return t; 3824 } 3825 3826 /** 3827 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3828 */ 3829 public ItemComponent addInformationLinkId(int value) { //1 3830 PositiveIntType t = new PositiveIntType(); 3831 t.setValue(value); 3832 if (this.informationLinkId == null) 3833 this.informationLinkId = new ArrayList<PositiveIntType>(); 3834 this.informationLinkId.add(t); 3835 return this; 3836 } 3837 3838 /** 3839 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3840 */ 3841 public boolean hasInformationLinkId(int value) { 3842 if (this.informationLinkId == null) 3843 return false; 3844 for (PositiveIntType v : this.informationLinkId) 3845 if (v.getValue().equals(value)) // positiveInt 3846 return true; 3847 return false; 3848 } 3849 3850 /** 3851 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3852 */ 3853 public CodeableConcept getRevenue() { 3854 if (this.revenue == null) 3855 if (Configuration.errorOnAutoCreate()) 3856 throw new Error("Attempt to auto-create ItemComponent.revenue"); 3857 else if (Configuration.doAutoCreate()) 3858 this.revenue = new CodeableConcept(); // cc 3859 return this.revenue; 3860 } 3861 3862 public boolean hasRevenue() { 3863 return this.revenue != null && !this.revenue.isEmpty(); 3864 } 3865 3866 /** 3867 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3868 */ 3869 public ItemComponent setRevenue(CodeableConcept value) { 3870 this.revenue = value; 3871 return this; 3872 } 3873 3874 /** 3875 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3876 */ 3877 public CodeableConcept getCategory() { 3878 if (this.category == null) 3879 if (Configuration.errorOnAutoCreate()) 3880 throw new Error("Attempt to auto-create ItemComponent.category"); 3881 else if (Configuration.doAutoCreate()) 3882 this.category = new CodeableConcept(); // cc 3883 return this.category; 3884 } 3885 3886 public boolean hasCategory() { 3887 return this.category != null && !this.category.isEmpty(); 3888 } 3889 3890 /** 3891 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3892 */ 3893 public ItemComponent setCategory(CodeableConcept value) { 3894 this.category = value; 3895 return this; 3896 } 3897 3898 /** 3899 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3900 */ 3901 public CodeableConcept getService() { 3902 if (this.service == null) 3903 if (Configuration.errorOnAutoCreate()) 3904 throw new Error("Attempt to auto-create ItemComponent.service"); 3905 else if (Configuration.doAutoCreate()) 3906 this.service = new CodeableConcept(); // cc 3907 return this.service; 3908 } 3909 3910 public boolean hasService() { 3911 return this.service != null && !this.service.isEmpty(); 3912 } 3913 3914 /** 3915 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3916 */ 3917 public ItemComponent setService(CodeableConcept value) { 3918 this.service = value; 3919 return this; 3920 } 3921 3922 /** 3923 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 3924 */ 3925 public List<CodeableConcept> getModifier() { 3926 if (this.modifier == null) 3927 this.modifier = new ArrayList<CodeableConcept>(); 3928 return this.modifier; 3929 } 3930 3931 /** 3932 * @return Returns a reference to <code>this</code> for easy method chaining 3933 */ 3934 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 3935 this.modifier = theModifier; 3936 return this; 3937 } 3938 3939 public boolean hasModifier() { 3940 if (this.modifier == null) 3941 return false; 3942 for (CodeableConcept item : this.modifier) 3943 if (!item.isEmpty()) 3944 return true; 3945 return false; 3946 } 3947 3948 public CodeableConcept addModifier() { //3 3949 CodeableConcept t = new CodeableConcept(); 3950 if (this.modifier == null) 3951 this.modifier = new ArrayList<CodeableConcept>(); 3952 this.modifier.add(t); 3953 return t; 3954 } 3955 3956 public ItemComponent addModifier(CodeableConcept t) { //3 3957 if (t == null) 3958 return this; 3959 if (this.modifier == null) 3960 this.modifier = new ArrayList<CodeableConcept>(); 3961 this.modifier.add(t); 3962 return this; 3963 } 3964 3965 /** 3966 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 3967 */ 3968 public CodeableConcept getModifierFirstRep() { 3969 if (getModifier().isEmpty()) { 3970 addModifier(); 3971 } 3972 return getModifier().get(0); 3973 } 3974 3975 /** 3976 * @return {@link #programCode} (For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program.) 3977 */ 3978 public List<CodeableConcept> getProgramCode() { 3979 if (this.programCode == null) 3980 this.programCode = new ArrayList<CodeableConcept>(); 3981 return this.programCode; 3982 } 3983 3984 /** 3985 * @return Returns a reference to <code>this</code> for easy method chaining 3986 */ 3987 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 3988 this.programCode = theProgramCode; 3989 return this; 3990 } 3991 3992 public boolean hasProgramCode() { 3993 if (this.programCode == null) 3994 return false; 3995 for (CodeableConcept item : this.programCode) 3996 if (!item.isEmpty()) 3997 return true; 3998 return false; 3999 } 4000 4001 public CodeableConcept addProgramCode() { //3 4002 CodeableConcept t = new CodeableConcept(); 4003 if (this.programCode == null) 4004 this.programCode = new ArrayList<CodeableConcept>(); 4005 this.programCode.add(t); 4006 return t; 4007 } 4008 4009 public ItemComponent addProgramCode(CodeableConcept t) { //3 4010 if (t == null) 4011 return this; 4012 if (this.programCode == null) 4013 this.programCode = new ArrayList<CodeableConcept>(); 4014 this.programCode.add(t); 4015 return this; 4016 } 4017 4018 /** 4019 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 4020 */ 4021 public CodeableConcept getProgramCodeFirstRep() { 4022 if (getProgramCode().isEmpty()) { 4023 addProgramCode(); 4024 } 4025 return getProgramCode().get(0); 4026 } 4027 4028 /** 4029 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4030 */ 4031 public Type getServiced() { 4032 return this.serviced; 4033 } 4034 4035 /** 4036 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4037 */ 4038 public DateType getServicedDateType() throws FHIRException { 4039 if (this.serviced == null) 4040 return null; 4041 if (!(this.serviced instanceof DateType)) 4042 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4043 return (DateType) this.serviced; 4044 } 4045 4046 public boolean hasServicedDateType() { 4047 return this != null && this.serviced instanceof DateType; 4048 } 4049 4050 /** 4051 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4052 */ 4053 public Period getServicedPeriod() throws FHIRException { 4054 if (this.serviced == null) 4055 return null; 4056 if (!(this.serviced instanceof Period)) 4057 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4058 return (Period) this.serviced; 4059 } 4060 4061 public boolean hasServicedPeriod() { 4062 return this != null && this.serviced instanceof Period; 4063 } 4064 4065 public boolean hasServiced() { 4066 return this.serviced != null && !this.serviced.isEmpty(); 4067 } 4068 4069 /** 4070 * @param value {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4071 */ 4072 public ItemComponent setServiced(Type value) throws FHIRFormatError { 4073 if (value != null && !(value instanceof DateType || value instanceof Period)) 4074 throw new FHIRFormatError("Not the right type for Claim.item.serviced[x]: "+value.fhirType()); 4075 this.serviced = value; 4076 return this; 4077 } 4078 4079 /** 4080 * @return {@link #location} (Where the service was provided.) 4081 */ 4082 public Type getLocation() { 4083 return this.location; 4084 } 4085 4086 /** 4087 * @return {@link #location} (Where the service was provided.) 4088 */ 4089 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 4090 if (this.location == null) 4091 return null; 4092 if (!(this.location instanceof CodeableConcept)) 4093 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 4094 return (CodeableConcept) this.location; 4095 } 4096 4097 public boolean hasLocationCodeableConcept() { 4098 return this != null && this.location instanceof CodeableConcept; 4099 } 4100 4101 /** 4102 * @return {@link #location} (Where the service was provided.) 4103 */ 4104 public Address getLocationAddress() throws FHIRException { 4105 if (this.location == null) 4106 return null; 4107 if (!(this.location instanceof Address)) 4108 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 4109 return (Address) this.location; 4110 } 4111 4112 public boolean hasLocationAddress() { 4113 return this != null && this.location instanceof Address; 4114 } 4115 4116 /** 4117 * @return {@link #location} (Where the service was provided.) 4118 */ 4119 public Reference getLocationReference() throws FHIRException { 4120 if (this.location == null) 4121 return null; 4122 if (!(this.location instanceof Reference)) 4123 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 4124 return (Reference) this.location; 4125 } 4126 4127 public boolean hasLocationReference() { 4128 return this != null && this.location instanceof Reference; 4129 } 4130 4131 public boolean hasLocation() { 4132 return this.location != null && !this.location.isEmpty(); 4133 } 4134 4135 /** 4136 * @param value {@link #location} (Where the service was provided.) 4137 */ 4138 public ItemComponent setLocation(Type value) throws FHIRFormatError { 4139 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 4140 throw new FHIRFormatError("Not the right type for Claim.item.location[x]: "+value.fhirType()); 4141 this.location = value; 4142 return this; 4143 } 4144 4145 /** 4146 * @return {@link #quantity} (The number of repetitions of a service or product.) 4147 */ 4148 public SimpleQuantity getQuantity() { 4149 if (this.quantity == null) 4150 if (Configuration.errorOnAutoCreate()) 4151 throw new Error("Attempt to auto-create ItemComponent.quantity"); 4152 else if (Configuration.doAutoCreate()) 4153 this.quantity = new SimpleQuantity(); // cc 4154 return this.quantity; 4155 } 4156 4157 public boolean hasQuantity() { 4158 return this.quantity != null && !this.quantity.isEmpty(); 4159 } 4160 4161 /** 4162 * @param value {@link #quantity} (The number of repetitions of a service or product.) 4163 */ 4164 public ItemComponent setQuantity(SimpleQuantity value) { 4165 this.quantity = value; 4166 return this; 4167 } 4168 4169 /** 4170 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 4171 */ 4172 public Money getUnitPrice() { 4173 if (this.unitPrice == null) 4174 if (Configuration.errorOnAutoCreate()) 4175 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 4176 else if (Configuration.doAutoCreate()) 4177 this.unitPrice = new Money(); // cc 4178 return this.unitPrice; 4179 } 4180 4181 public boolean hasUnitPrice() { 4182 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4183 } 4184 4185 /** 4186 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 4187 */ 4188 public ItemComponent setUnitPrice(Money value) { 4189 this.unitPrice = value; 4190 return this; 4191 } 4192 4193 /** 4194 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4195 */ 4196 public DecimalType getFactorElement() { 4197 if (this.factor == null) 4198 if (Configuration.errorOnAutoCreate()) 4199 throw new Error("Attempt to auto-create ItemComponent.factor"); 4200 else if (Configuration.doAutoCreate()) 4201 this.factor = new DecimalType(); // bb 4202 return this.factor; 4203 } 4204 4205 public boolean hasFactorElement() { 4206 return this.factor != null && !this.factor.isEmpty(); 4207 } 4208 4209 public boolean hasFactor() { 4210 return this.factor != null && !this.factor.isEmpty(); 4211 } 4212 4213 /** 4214 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4215 */ 4216 public ItemComponent setFactorElement(DecimalType value) { 4217 this.factor = value; 4218 return this; 4219 } 4220 4221 /** 4222 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4223 */ 4224 public BigDecimal getFactor() { 4225 return this.factor == null ? null : this.factor.getValue(); 4226 } 4227 4228 /** 4229 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4230 */ 4231 public ItemComponent setFactor(BigDecimal value) { 4232 if (value == null) 4233 this.factor = null; 4234 else { 4235 if (this.factor == null) 4236 this.factor = new DecimalType(); 4237 this.factor.setValue(value); 4238 } 4239 return this; 4240 } 4241 4242 /** 4243 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4244 */ 4245 public ItemComponent setFactor(long value) { 4246 this.factor = new DecimalType(); 4247 this.factor.setValue(value); 4248 return this; 4249 } 4250 4251 /** 4252 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4253 */ 4254 public ItemComponent setFactor(double value) { 4255 this.factor = new DecimalType(); 4256 this.factor.setValue(value); 4257 return this; 4258 } 4259 4260 /** 4261 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 4262 */ 4263 public Money getNet() { 4264 if (this.net == null) 4265 if (Configuration.errorOnAutoCreate()) 4266 throw new Error("Attempt to auto-create ItemComponent.net"); 4267 else if (Configuration.doAutoCreate()) 4268 this.net = new Money(); // cc 4269 return this.net; 4270 } 4271 4272 public boolean hasNet() { 4273 return this.net != null && !this.net.isEmpty(); 4274 } 4275 4276 /** 4277 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 4278 */ 4279 public ItemComponent setNet(Money value) { 4280 this.net = value; 4281 return this; 4282 } 4283 4284 /** 4285 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 4286 */ 4287 public List<Reference> getUdi() { 4288 if (this.udi == null) 4289 this.udi = new ArrayList<Reference>(); 4290 return this.udi; 4291 } 4292 4293 /** 4294 * @return Returns a reference to <code>this</code> for easy method chaining 4295 */ 4296 public ItemComponent setUdi(List<Reference> theUdi) { 4297 this.udi = theUdi; 4298 return this; 4299 } 4300 4301 public boolean hasUdi() { 4302 if (this.udi == null) 4303 return false; 4304 for (Reference item : this.udi) 4305 if (!item.isEmpty()) 4306 return true; 4307 return false; 4308 } 4309 4310 public Reference addUdi() { //3 4311 Reference t = new Reference(); 4312 if (this.udi == null) 4313 this.udi = new ArrayList<Reference>(); 4314 this.udi.add(t); 4315 return t; 4316 } 4317 4318 public ItemComponent addUdi(Reference t) { //3 4319 if (t == null) 4320 return this; 4321 if (this.udi == null) 4322 this.udi = new ArrayList<Reference>(); 4323 this.udi.add(t); 4324 return this; 4325 } 4326 4327 /** 4328 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 4329 */ 4330 public Reference getUdiFirstRep() { 4331 if (getUdi().isEmpty()) { 4332 addUdi(); 4333 } 4334 return getUdi().get(0); 4335 } 4336 4337 /** 4338 * @deprecated Use Reference#setResource(IBaseResource) instead 4339 */ 4340 @Deprecated 4341 public List<Device> getUdiTarget() { 4342 if (this.udiTarget == null) 4343 this.udiTarget = new ArrayList<Device>(); 4344 return this.udiTarget; 4345 } 4346 4347 /** 4348 * @deprecated Use Reference#setResource(IBaseResource) instead 4349 */ 4350 @Deprecated 4351 public Device addUdiTarget() { 4352 Device r = new Device(); 4353 if (this.udiTarget == null) 4354 this.udiTarget = new ArrayList<Device>(); 4355 this.udiTarget.add(r); 4356 return r; 4357 } 4358 4359 /** 4360 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 4361 */ 4362 public CodeableConcept getBodySite() { 4363 if (this.bodySite == null) 4364 if (Configuration.errorOnAutoCreate()) 4365 throw new Error("Attempt to auto-create ItemComponent.bodySite"); 4366 else if (Configuration.doAutoCreate()) 4367 this.bodySite = new CodeableConcept(); // cc 4368 return this.bodySite; 4369 } 4370 4371 public boolean hasBodySite() { 4372 return this.bodySite != null && !this.bodySite.isEmpty(); 4373 } 4374 4375 /** 4376 * @param value {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 4377 */ 4378 public ItemComponent setBodySite(CodeableConcept value) { 4379 this.bodySite = value; 4380 return this; 4381 } 4382 4383 /** 4384 * @return {@link #subSite} (A region or surface of the site, eg. limb region or tooth surface(s).) 4385 */ 4386 public List<CodeableConcept> getSubSite() { 4387 if (this.subSite == null) 4388 this.subSite = new ArrayList<CodeableConcept>(); 4389 return this.subSite; 4390 } 4391 4392 /** 4393 * @return Returns a reference to <code>this</code> for easy method chaining 4394 */ 4395 public ItemComponent setSubSite(List<CodeableConcept> theSubSite) { 4396 this.subSite = theSubSite; 4397 return this; 4398 } 4399 4400 public boolean hasSubSite() { 4401 if (this.subSite == null) 4402 return false; 4403 for (CodeableConcept item : this.subSite) 4404 if (!item.isEmpty()) 4405 return true; 4406 return false; 4407 } 4408 4409 public CodeableConcept addSubSite() { //3 4410 CodeableConcept t = new CodeableConcept(); 4411 if (this.subSite == null) 4412 this.subSite = new ArrayList<CodeableConcept>(); 4413 this.subSite.add(t); 4414 return t; 4415 } 4416 4417 public ItemComponent addSubSite(CodeableConcept t) { //3 4418 if (t == null) 4419 return this; 4420 if (this.subSite == null) 4421 this.subSite = new ArrayList<CodeableConcept>(); 4422 this.subSite.add(t); 4423 return this; 4424 } 4425 4426 /** 4427 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist 4428 */ 4429 public CodeableConcept getSubSiteFirstRep() { 4430 if (getSubSite().isEmpty()) { 4431 addSubSite(); 4432 } 4433 return getSubSite().get(0); 4434 } 4435 4436 /** 4437 * @return {@link #encounter} (A billed item may include goods or services provided in multiple encounters.) 4438 */ 4439 public List<Reference> getEncounter() { 4440 if (this.encounter == null) 4441 this.encounter = new ArrayList<Reference>(); 4442 return this.encounter; 4443 } 4444 4445 /** 4446 * @return Returns a reference to <code>this</code> for easy method chaining 4447 */ 4448 public ItemComponent setEncounter(List<Reference> theEncounter) { 4449 this.encounter = theEncounter; 4450 return this; 4451 } 4452 4453 public boolean hasEncounter() { 4454 if (this.encounter == null) 4455 return false; 4456 for (Reference item : this.encounter) 4457 if (!item.isEmpty()) 4458 return true; 4459 return false; 4460 } 4461 4462 public Reference addEncounter() { //3 4463 Reference t = new Reference(); 4464 if (this.encounter == null) 4465 this.encounter = new ArrayList<Reference>(); 4466 this.encounter.add(t); 4467 return t; 4468 } 4469 4470 public ItemComponent addEncounter(Reference t) { //3 4471 if (t == null) 4472 return this; 4473 if (this.encounter == null) 4474 this.encounter = new ArrayList<Reference>(); 4475 this.encounter.add(t); 4476 return this; 4477 } 4478 4479 /** 4480 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist 4481 */ 4482 public Reference getEncounterFirstRep() { 4483 if (getEncounter().isEmpty()) { 4484 addEncounter(); 4485 } 4486 return getEncounter().get(0); 4487 } 4488 4489 /** 4490 * @deprecated Use Reference#setResource(IBaseResource) instead 4491 */ 4492 @Deprecated 4493 public List<Encounter> getEncounterTarget() { 4494 if (this.encounterTarget == null) 4495 this.encounterTarget = new ArrayList<Encounter>(); 4496 return this.encounterTarget; 4497 } 4498 4499 /** 4500 * @deprecated Use Reference#setResource(IBaseResource) instead 4501 */ 4502 @Deprecated 4503 public Encounter addEncounterTarget() { 4504 Encounter r = new Encounter(); 4505 if (this.encounterTarget == null) 4506 this.encounterTarget = new ArrayList<Encounter>(); 4507 this.encounterTarget.add(r); 4508 return r; 4509 } 4510 4511 /** 4512 * @return {@link #detail} (Second tier of goods and services.) 4513 */ 4514 public List<DetailComponent> getDetail() { 4515 if (this.detail == null) 4516 this.detail = new ArrayList<DetailComponent>(); 4517 return this.detail; 4518 } 4519 4520 /** 4521 * @return Returns a reference to <code>this</code> for easy method chaining 4522 */ 4523 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4524 this.detail = theDetail; 4525 return this; 4526 } 4527 4528 public boolean hasDetail() { 4529 if (this.detail == null) 4530 return false; 4531 for (DetailComponent item : this.detail) 4532 if (!item.isEmpty()) 4533 return true; 4534 return false; 4535 } 4536 4537 public DetailComponent addDetail() { //3 4538 DetailComponent t = new DetailComponent(); 4539 if (this.detail == null) 4540 this.detail = new ArrayList<DetailComponent>(); 4541 this.detail.add(t); 4542 return t; 4543 } 4544 4545 public ItemComponent addDetail(DetailComponent t) { //3 4546 if (t == null) 4547 return this; 4548 if (this.detail == null) 4549 this.detail = new ArrayList<DetailComponent>(); 4550 this.detail.add(t); 4551 return this; 4552 } 4553 4554 /** 4555 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 4556 */ 4557 public DetailComponent getDetailFirstRep() { 4558 if (getDetail().isEmpty()) { 4559 addDetail(); 4560 } 4561 return getDetail().get(0); 4562 } 4563 4564 protected void listChildren(List<Property> children) { 4565 super.listChildren(children); 4566 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 4567 children.add(new Property("careTeamLinkId", "positiveInt", "CareTeam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId)); 4568 children.add(new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId)); 4569 children.add(new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId)); 4570 children.add(new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId)); 4571 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 4572 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 4573 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 4574 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4575 children.add(new Property("programCode", "CodeableConcept", "For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4576 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 4577 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location)); 4578 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4579 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 4580 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4581 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 4582 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4583 children.add(new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite)); 4584 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 4585 children.add(new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4586 children.add(new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 4587 } 4588 4589 @Override 4590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4591 switch (_hash) { 4592 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 4593 case -186757789: /*careTeamLinkId*/ return new Property("careTeamLinkId", "positiveInt", "CareTeam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId); 4594 case -1659207418: /*diagnosisLinkId*/ return new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId); 4595 case -532846744: /*procedureLinkId*/ return new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId); 4596 case 1965585153: /*informationLinkId*/ return new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId); 4597 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 4598 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 4599 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 4600 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 4601 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 4602 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4603 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4604 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4605 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4606 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4607 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4608 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4609 case -1280020865: /*locationAddress*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4610 case 755866390: /*locationReference*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4611 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 4612 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 4613 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 4614 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 4615 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 4616 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite); 4617 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 4618 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter); 4619 case -1335224239: /*detail*/ return new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail); 4620 default: return super.getNamedProperty(_hash, _name, _checkValid); 4621 } 4622 4623 } 4624 4625 @Override 4626 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4627 switch (hash) { 4628 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 4629 case -186757789: /*careTeamLinkId*/ return this.careTeamLinkId == null ? new Base[0] : this.careTeamLinkId.toArray(new Base[this.careTeamLinkId.size()]); // PositiveIntType 4630 case -1659207418: /*diagnosisLinkId*/ return this.diagnosisLinkId == null ? new Base[0] : this.diagnosisLinkId.toArray(new Base[this.diagnosisLinkId.size()]); // PositiveIntType 4631 case -532846744: /*procedureLinkId*/ return this.procedureLinkId == null ? new Base[0] : this.procedureLinkId.toArray(new Base[this.procedureLinkId.size()]); // PositiveIntType 4632 case 1965585153: /*informationLinkId*/ return this.informationLinkId == null ? new Base[0] : this.informationLinkId.toArray(new Base[this.informationLinkId.size()]); // PositiveIntType 4633 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 4634 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4635 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 4636 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4637 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 4638 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // Type 4639 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 4640 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 4641 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 4642 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 4643 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 4644 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 4645 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 4646 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 4647 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 4648 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 4649 default: return super.getProperty(hash, name, checkValid); 4650 } 4651 4652 } 4653 4654 @Override 4655 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4656 switch (hash) { 4657 case 1349547969: // sequence 4658 this.sequence = castToPositiveInt(value); // PositiveIntType 4659 return value; 4660 case -186757789: // careTeamLinkId 4661 this.getCareTeamLinkId().add(castToPositiveInt(value)); // PositiveIntType 4662 return value; 4663 case -1659207418: // diagnosisLinkId 4664 this.getDiagnosisLinkId().add(castToPositiveInt(value)); // PositiveIntType 4665 return value; 4666 case -532846744: // procedureLinkId 4667 this.getProcedureLinkId().add(castToPositiveInt(value)); // PositiveIntType 4668 return value; 4669 case 1965585153: // informationLinkId 4670 this.getInformationLinkId().add(castToPositiveInt(value)); // PositiveIntType 4671 return value; 4672 case 1099842588: // revenue 4673 this.revenue = castToCodeableConcept(value); // CodeableConcept 4674 return value; 4675 case 50511102: // category 4676 this.category = castToCodeableConcept(value); // CodeableConcept 4677 return value; 4678 case 1984153269: // service 4679 this.service = castToCodeableConcept(value); // CodeableConcept 4680 return value; 4681 case -615513385: // modifier 4682 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 4683 return value; 4684 case 1010065041: // programCode 4685 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 4686 return value; 4687 case 1379209295: // serviced 4688 this.serviced = castToType(value); // Type 4689 return value; 4690 case 1901043637: // location 4691 this.location = castToType(value); // Type 4692 return value; 4693 case -1285004149: // quantity 4694 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4695 return value; 4696 case -486196699: // unitPrice 4697 this.unitPrice = castToMoney(value); // Money 4698 return value; 4699 case -1282148017: // factor 4700 this.factor = castToDecimal(value); // DecimalType 4701 return value; 4702 case 108957: // net 4703 this.net = castToMoney(value); // Money 4704 return value; 4705 case 115642: // udi 4706 this.getUdi().add(castToReference(value)); // Reference 4707 return value; 4708 case 1702620169: // bodySite 4709 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4710 return value; 4711 case -1868566105: // subSite 4712 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 4713 return value; 4714 case 1524132147: // encounter 4715 this.getEncounter().add(castToReference(value)); // Reference 4716 return value; 4717 case -1335224239: // detail 4718 this.getDetail().add((DetailComponent) value); // DetailComponent 4719 return value; 4720 default: return super.setProperty(hash, name, value); 4721 } 4722 4723 } 4724 4725 @Override 4726 public Base setProperty(String name, Base value) throws FHIRException { 4727 if (name.equals("sequence")) { 4728 this.sequence = castToPositiveInt(value); // PositiveIntType 4729 } else if (name.equals("careTeamLinkId")) { 4730 this.getCareTeamLinkId().add(castToPositiveInt(value)); 4731 } else if (name.equals("diagnosisLinkId")) { 4732 this.getDiagnosisLinkId().add(castToPositiveInt(value)); 4733 } else if (name.equals("procedureLinkId")) { 4734 this.getProcedureLinkId().add(castToPositiveInt(value)); 4735 } else if (name.equals("informationLinkId")) { 4736 this.getInformationLinkId().add(castToPositiveInt(value)); 4737 } else if (name.equals("revenue")) { 4738 this.revenue = castToCodeableConcept(value); // CodeableConcept 4739 } else if (name.equals("category")) { 4740 this.category = castToCodeableConcept(value); // CodeableConcept 4741 } else if (name.equals("service")) { 4742 this.service = castToCodeableConcept(value); // CodeableConcept 4743 } else if (name.equals("modifier")) { 4744 this.getModifier().add(castToCodeableConcept(value)); 4745 } else if (name.equals("programCode")) { 4746 this.getProgramCode().add(castToCodeableConcept(value)); 4747 } else if (name.equals("serviced[x]")) { 4748 this.serviced = castToType(value); // Type 4749 } else if (name.equals("location[x]")) { 4750 this.location = castToType(value); // Type 4751 } else if (name.equals("quantity")) { 4752 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4753 } else if (name.equals("unitPrice")) { 4754 this.unitPrice = castToMoney(value); // Money 4755 } else if (name.equals("factor")) { 4756 this.factor = castToDecimal(value); // DecimalType 4757 } else if (name.equals("net")) { 4758 this.net = castToMoney(value); // Money 4759 } else if (name.equals("udi")) { 4760 this.getUdi().add(castToReference(value)); 4761 } else if (name.equals("bodySite")) { 4762 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4763 } else if (name.equals("subSite")) { 4764 this.getSubSite().add(castToCodeableConcept(value)); 4765 } else if (name.equals("encounter")) { 4766 this.getEncounter().add(castToReference(value)); 4767 } else if (name.equals("detail")) { 4768 this.getDetail().add((DetailComponent) value); 4769 } else 4770 return super.setProperty(name, value); 4771 return value; 4772 } 4773 4774 @Override 4775 public Base makeProperty(int hash, String name) throws FHIRException { 4776 switch (hash) { 4777 case 1349547969: return getSequenceElement(); 4778 case -186757789: return addCareTeamLinkIdElement(); 4779 case -1659207418: return addDiagnosisLinkIdElement(); 4780 case -532846744: return addProcedureLinkIdElement(); 4781 case 1965585153: return addInformationLinkIdElement(); 4782 case 1099842588: return getRevenue(); 4783 case 50511102: return getCategory(); 4784 case 1984153269: return getService(); 4785 case -615513385: return addModifier(); 4786 case 1010065041: return addProgramCode(); 4787 case -1927922223: return getServiced(); 4788 case 1379209295: return getServiced(); 4789 case 552316075: return getLocation(); 4790 case 1901043637: return getLocation(); 4791 case -1285004149: return getQuantity(); 4792 case -486196699: return getUnitPrice(); 4793 case -1282148017: return getFactorElement(); 4794 case 108957: return getNet(); 4795 case 115642: return addUdi(); 4796 case 1702620169: return getBodySite(); 4797 case -1868566105: return addSubSite(); 4798 case 1524132147: return addEncounter(); 4799 case -1335224239: return addDetail(); 4800 default: return super.makeProperty(hash, name); 4801 } 4802 4803 } 4804 4805 @Override 4806 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4807 switch (hash) { 4808 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 4809 case -186757789: /*careTeamLinkId*/ return new String[] {"positiveInt"}; 4810 case -1659207418: /*diagnosisLinkId*/ return new String[] {"positiveInt"}; 4811 case -532846744: /*procedureLinkId*/ return new String[] {"positiveInt"}; 4812 case 1965585153: /*informationLinkId*/ return new String[] {"positiveInt"}; 4813 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 4814 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4815 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 4816 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 4817 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 4818 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 4819 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 4820 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 4821 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 4822 case -1282148017: /*factor*/ return new String[] {"decimal"}; 4823 case 108957: /*net*/ return new String[] {"Money"}; 4824 case 115642: /*udi*/ return new String[] {"Reference"}; 4825 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 4826 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 4827 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 4828 case -1335224239: /*detail*/ return new String[] {}; 4829 default: return super.getTypesForProperty(hash, name); 4830 } 4831 4832 } 4833 4834 @Override 4835 public Base addChild(String name) throws FHIRException { 4836 if (name.equals("sequence")) { 4837 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 4838 } 4839 else if (name.equals("careTeamLinkId")) { 4840 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeamLinkId"); 4841 } 4842 else if (name.equals("diagnosisLinkId")) { 4843 throw new FHIRException("Cannot call addChild on a singleton property Claim.diagnosisLinkId"); 4844 } 4845 else if (name.equals("procedureLinkId")) { 4846 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedureLinkId"); 4847 } 4848 else if (name.equals("informationLinkId")) { 4849 throw new FHIRException("Cannot call addChild on a singleton property Claim.informationLinkId"); 4850 } 4851 else if (name.equals("revenue")) { 4852 this.revenue = new CodeableConcept(); 4853 return this.revenue; 4854 } 4855 else if (name.equals("category")) { 4856 this.category = new CodeableConcept(); 4857 return this.category; 4858 } 4859 else if (name.equals("service")) { 4860 this.service = new CodeableConcept(); 4861 return this.service; 4862 } 4863 else if (name.equals("modifier")) { 4864 return addModifier(); 4865 } 4866 else if (name.equals("programCode")) { 4867 return addProgramCode(); 4868 } 4869 else if (name.equals("servicedDate")) { 4870 this.serviced = new DateType(); 4871 return this.serviced; 4872 } 4873 else if (name.equals("servicedPeriod")) { 4874 this.serviced = new Period(); 4875 return this.serviced; 4876 } 4877 else if (name.equals("locationCodeableConcept")) { 4878 this.location = new CodeableConcept(); 4879 return this.location; 4880 } 4881 else if (name.equals("locationAddress")) { 4882 this.location = new Address(); 4883 return this.location; 4884 } 4885 else if (name.equals("locationReference")) { 4886 this.location = new Reference(); 4887 return this.location; 4888 } 4889 else if (name.equals("quantity")) { 4890 this.quantity = new SimpleQuantity(); 4891 return this.quantity; 4892 } 4893 else if (name.equals("unitPrice")) { 4894 this.unitPrice = new Money(); 4895 return this.unitPrice; 4896 } 4897 else if (name.equals("factor")) { 4898 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 4899 } 4900 else if (name.equals("net")) { 4901 this.net = new Money(); 4902 return this.net; 4903 } 4904 else if (name.equals("udi")) { 4905 return addUdi(); 4906 } 4907 else if (name.equals("bodySite")) { 4908 this.bodySite = new CodeableConcept(); 4909 return this.bodySite; 4910 } 4911 else if (name.equals("subSite")) { 4912 return addSubSite(); 4913 } 4914 else if (name.equals("encounter")) { 4915 return addEncounter(); 4916 } 4917 else if (name.equals("detail")) { 4918 return addDetail(); 4919 } 4920 else 4921 return super.addChild(name); 4922 } 4923 4924 public ItemComponent copy() { 4925 ItemComponent dst = new ItemComponent(); 4926 copyValues(dst); 4927 dst.sequence = sequence == null ? null : sequence.copy(); 4928 if (careTeamLinkId != null) { 4929 dst.careTeamLinkId = new ArrayList<PositiveIntType>(); 4930 for (PositiveIntType i : careTeamLinkId) 4931 dst.careTeamLinkId.add(i.copy()); 4932 }; 4933 if (diagnosisLinkId != null) { 4934 dst.diagnosisLinkId = new ArrayList<PositiveIntType>(); 4935 for (PositiveIntType i : diagnosisLinkId) 4936 dst.diagnosisLinkId.add(i.copy()); 4937 }; 4938 if (procedureLinkId != null) { 4939 dst.procedureLinkId = new ArrayList<PositiveIntType>(); 4940 for (PositiveIntType i : procedureLinkId) 4941 dst.procedureLinkId.add(i.copy()); 4942 }; 4943 if (informationLinkId != null) { 4944 dst.informationLinkId = new ArrayList<PositiveIntType>(); 4945 for (PositiveIntType i : informationLinkId) 4946 dst.informationLinkId.add(i.copy()); 4947 }; 4948 dst.revenue = revenue == null ? null : revenue.copy(); 4949 dst.category = category == null ? null : category.copy(); 4950 dst.service = service == null ? null : service.copy(); 4951 if (modifier != null) { 4952 dst.modifier = new ArrayList<CodeableConcept>(); 4953 for (CodeableConcept i : modifier) 4954 dst.modifier.add(i.copy()); 4955 }; 4956 if (programCode != null) { 4957 dst.programCode = new ArrayList<CodeableConcept>(); 4958 for (CodeableConcept i : programCode) 4959 dst.programCode.add(i.copy()); 4960 }; 4961 dst.serviced = serviced == null ? null : serviced.copy(); 4962 dst.location = location == null ? null : location.copy(); 4963 dst.quantity = quantity == null ? null : quantity.copy(); 4964 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4965 dst.factor = factor == null ? null : factor.copy(); 4966 dst.net = net == null ? null : net.copy(); 4967 if (udi != null) { 4968 dst.udi = new ArrayList<Reference>(); 4969 for (Reference i : udi) 4970 dst.udi.add(i.copy()); 4971 }; 4972 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4973 if (subSite != null) { 4974 dst.subSite = new ArrayList<CodeableConcept>(); 4975 for (CodeableConcept i : subSite) 4976 dst.subSite.add(i.copy()); 4977 }; 4978 if (encounter != null) { 4979 dst.encounter = new ArrayList<Reference>(); 4980 for (Reference i : encounter) 4981 dst.encounter.add(i.copy()); 4982 }; 4983 if (detail != null) { 4984 dst.detail = new ArrayList<DetailComponent>(); 4985 for (DetailComponent i : detail) 4986 dst.detail.add(i.copy()); 4987 }; 4988 return dst; 4989 } 4990 4991 @Override 4992 public boolean equalsDeep(Base other_) { 4993 if (!super.equalsDeep(other_)) 4994 return false; 4995 if (!(other_ instanceof ItemComponent)) 4996 return false; 4997 ItemComponent o = (ItemComponent) other_; 4998 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamLinkId, o.careTeamLinkId, true) 4999 && compareDeep(diagnosisLinkId, o.diagnosisLinkId, true) && compareDeep(procedureLinkId, o.procedureLinkId, true) 5000 && compareDeep(informationLinkId, o.informationLinkId, true) && compareDeep(revenue, o.revenue, true) 5001 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 5002 && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) && compareDeep(location, o.location, true) 5003 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 5004 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 5005 && compareDeep(subSite, o.subSite, true) && compareDeep(encounter, o.encounter, true) && compareDeep(detail, o.detail, true) 5006 ; 5007 } 5008 5009 @Override 5010 public boolean equalsShallow(Base other_) { 5011 if (!super.equalsShallow(other_)) 5012 return false; 5013 if (!(other_ instanceof ItemComponent)) 5014 return false; 5015 ItemComponent o = (ItemComponent) other_; 5016 return compareValues(sequence, o.sequence, true) && compareValues(careTeamLinkId, o.careTeamLinkId, true) 5017 && compareValues(diagnosisLinkId, o.diagnosisLinkId, true) && compareValues(procedureLinkId, o.procedureLinkId, true) 5018 && compareValues(informationLinkId, o.informationLinkId, true) && compareValues(factor, o.factor, true) 5019 ; 5020 } 5021 5022 public boolean isEmpty() { 5023 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamLinkId, diagnosisLinkId 5024 , procedureLinkId, informationLinkId, revenue, category, service, modifier, programCode 5025 , serviced, location, quantity, unitPrice, factor, net, udi, bodySite, subSite 5026 , encounter, detail); 5027 } 5028 5029 public String fhirType() { 5030 return "Claim.item"; 5031 5032 } 5033 5034 } 5035 5036 @Block() 5037 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 5038 /** 5039 * A service line number. 5040 */ 5041 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5042 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 5043 protected PositiveIntType sequence; 5044 5045 /** 5046 * The type of reveneu or cost center providing the product and/or service. 5047 */ 5048 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 5049 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 5050 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5051 protected CodeableConcept revenue; 5052 5053 /** 5054 * Health Care Service Type Codes to identify the classification of service or benefits. 5055 */ 5056 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5057 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 5058 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 5059 protected CodeableConcept category; 5060 5061 /** 5062 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 5063 */ 5064 @Child(name = "service", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5065 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 5066 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5067 protected CodeableConcept service; 5068 5069 /** 5070 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 5071 */ 5072 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5073 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 5074 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5075 protected List<CodeableConcept> modifier; 5076 5077 /** 5078 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 5079 */ 5080 @Child(name = "programCode", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5081 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 5082 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5083 protected List<CodeableConcept> programCode; 5084 5085 /** 5086 * The number of repetitions of a service or product. 5087 */ 5088 @Child(name = "quantity", type = {SimpleQuantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 5089 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 5090 protected SimpleQuantity quantity; 5091 5092 /** 5093 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 5094 */ 5095 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 5096 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 5097 protected Money unitPrice; 5098 5099 /** 5100 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5101 */ 5102 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5103 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5104 protected DecimalType factor; 5105 5106 /** 5107 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 5108 */ 5109 @Child(name = "net", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 5110 @Description(shortDefinition="Total additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 5111 protected Money net; 5112 5113 /** 5114 * List of Unique Device Identifiers associated with this line item. 5115 */ 5116 @Child(name = "udi", type = {Device.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5117 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 5118 protected List<Reference> udi; 5119 /** 5120 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 5121 */ 5122 protected List<Device> udiTarget; 5123 5124 5125 /** 5126 * Third tier of goods and services. 5127 */ 5128 @Child(name = "subDetail", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5129 @Description(shortDefinition="Additional items", formalDefinition="Third tier of goods and services." ) 5130 protected List<SubDetailComponent> subDetail; 5131 5132 private static final long serialVersionUID = 718584656L; 5133 5134 /** 5135 * Constructor 5136 */ 5137 public DetailComponent() { 5138 super(); 5139 } 5140 5141 /** 5142 * Constructor 5143 */ 5144 public DetailComponent(PositiveIntType sequence) { 5145 super(); 5146 this.sequence = sequence; 5147 } 5148 5149 /** 5150 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5151 */ 5152 public PositiveIntType getSequenceElement() { 5153 if (this.sequence == null) 5154 if (Configuration.errorOnAutoCreate()) 5155 throw new Error("Attempt to auto-create DetailComponent.sequence"); 5156 else if (Configuration.doAutoCreate()) 5157 this.sequence = new PositiveIntType(); // bb 5158 return this.sequence; 5159 } 5160 5161 public boolean hasSequenceElement() { 5162 return this.sequence != null && !this.sequence.isEmpty(); 5163 } 5164 5165 public boolean hasSequence() { 5166 return this.sequence != null && !this.sequence.isEmpty(); 5167 } 5168 5169 /** 5170 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5171 */ 5172 public DetailComponent setSequenceElement(PositiveIntType value) { 5173 this.sequence = value; 5174 return this; 5175 } 5176 5177 /** 5178 * @return A service line number. 5179 */ 5180 public int getSequence() { 5181 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 5182 } 5183 5184 /** 5185 * @param value A service line number. 5186 */ 5187 public DetailComponent setSequence(int value) { 5188 if (this.sequence == null) 5189 this.sequence = new PositiveIntType(); 5190 this.sequence.setValue(value); 5191 return this; 5192 } 5193 5194 /** 5195 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5196 */ 5197 public CodeableConcept getRevenue() { 5198 if (this.revenue == null) 5199 if (Configuration.errorOnAutoCreate()) 5200 throw new Error("Attempt to auto-create DetailComponent.revenue"); 5201 else if (Configuration.doAutoCreate()) 5202 this.revenue = new CodeableConcept(); // cc 5203 return this.revenue; 5204 } 5205 5206 public boolean hasRevenue() { 5207 return this.revenue != null && !this.revenue.isEmpty(); 5208 } 5209 5210 /** 5211 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5212 */ 5213 public DetailComponent setRevenue(CodeableConcept value) { 5214 this.revenue = value; 5215 return this; 5216 } 5217 5218 /** 5219 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5220 */ 5221 public CodeableConcept getCategory() { 5222 if (this.category == null) 5223 if (Configuration.errorOnAutoCreate()) 5224 throw new Error("Attempt to auto-create DetailComponent.category"); 5225 else if (Configuration.doAutoCreate()) 5226 this.category = new CodeableConcept(); // cc 5227 return this.category; 5228 } 5229 5230 public boolean hasCategory() { 5231 return this.category != null && !this.category.isEmpty(); 5232 } 5233 5234 /** 5235 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5236 */ 5237 public DetailComponent setCategory(CodeableConcept value) { 5238 this.category = value; 5239 return this; 5240 } 5241 5242 /** 5243 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5244 */ 5245 public CodeableConcept getService() { 5246 if (this.service == null) 5247 if (Configuration.errorOnAutoCreate()) 5248 throw new Error("Attempt to auto-create DetailComponent.service"); 5249 else if (Configuration.doAutoCreate()) 5250 this.service = new CodeableConcept(); // cc 5251 return this.service; 5252 } 5253 5254 public boolean hasService() { 5255 return this.service != null && !this.service.isEmpty(); 5256 } 5257 5258 /** 5259 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5260 */ 5261 public DetailComponent setService(CodeableConcept value) { 5262 this.service = value; 5263 return this; 5264 } 5265 5266 /** 5267 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 5268 */ 5269 public List<CodeableConcept> getModifier() { 5270 if (this.modifier == null) 5271 this.modifier = new ArrayList<CodeableConcept>(); 5272 return this.modifier; 5273 } 5274 5275 /** 5276 * @return Returns a reference to <code>this</code> for easy method chaining 5277 */ 5278 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 5279 this.modifier = theModifier; 5280 return this; 5281 } 5282 5283 public boolean hasModifier() { 5284 if (this.modifier == null) 5285 return false; 5286 for (CodeableConcept item : this.modifier) 5287 if (!item.isEmpty()) 5288 return true; 5289 return false; 5290 } 5291 5292 public CodeableConcept addModifier() { //3 5293 CodeableConcept t = new CodeableConcept(); 5294 if (this.modifier == null) 5295 this.modifier = new ArrayList<CodeableConcept>(); 5296 this.modifier.add(t); 5297 return t; 5298 } 5299 5300 public DetailComponent addModifier(CodeableConcept t) { //3 5301 if (t == null) 5302 return this; 5303 if (this.modifier == null) 5304 this.modifier = new ArrayList<CodeableConcept>(); 5305 this.modifier.add(t); 5306 return this; 5307 } 5308 5309 /** 5310 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 5311 */ 5312 public CodeableConcept getModifierFirstRep() { 5313 if (getModifier().isEmpty()) { 5314 addModifier(); 5315 } 5316 return getModifier().get(0); 5317 } 5318 5319 /** 5320 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 5321 */ 5322 public List<CodeableConcept> getProgramCode() { 5323 if (this.programCode == null) 5324 this.programCode = new ArrayList<CodeableConcept>(); 5325 return this.programCode; 5326 } 5327 5328 /** 5329 * @return Returns a reference to <code>this</code> for easy method chaining 5330 */ 5331 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 5332 this.programCode = theProgramCode; 5333 return this; 5334 } 5335 5336 public boolean hasProgramCode() { 5337 if (this.programCode == null) 5338 return false; 5339 for (CodeableConcept item : this.programCode) 5340 if (!item.isEmpty()) 5341 return true; 5342 return false; 5343 } 5344 5345 public CodeableConcept addProgramCode() { //3 5346 CodeableConcept t = new CodeableConcept(); 5347 if (this.programCode == null) 5348 this.programCode = new ArrayList<CodeableConcept>(); 5349 this.programCode.add(t); 5350 return t; 5351 } 5352 5353 public DetailComponent addProgramCode(CodeableConcept t) { //3 5354 if (t == null) 5355 return this; 5356 if (this.programCode == null) 5357 this.programCode = new ArrayList<CodeableConcept>(); 5358 this.programCode.add(t); 5359 return this; 5360 } 5361 5362 /** 5363 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 5364 */ 5365 public CodeableConcept getProgramCodeFirstRep() { 5366 if (getProgramCode().isEmpty()) { 5367 addProgramCode(); 5368 } 5369 return getProgramCode().get(0); 5370 } 5371 5372 /** 5373 * @return {@link #quantity} (The number of repetitions of a service or product.) 5374 */ 5375 public SimpleQuantity getQuantity() { 5376 if (this.quantity == null) 5377 if (Configuration.errorOnAutoCreate()) 5378 throw new Error("Attempt to auto-create DetailComponent.quantity"); 5379 else if (Configuration.doAutoCreate()) 5380 this.quantity = new SimpleQuantity(); // cc 5381 return this.quantity; 5382 } 5383 5384 public boolean hasQuantity() { 5385 return this.quantity != null && !this.quantity.isEmpty(); 5386 } 5387 5388 /** 5389 * @param value {@link #quantity} (The number of repetitions of a service or product.) 5390 */ 5391 public DetailComponent setQuantity(SimpleQuantity value) { 5392 this.quantity = value; 5393 return this; 5394 } 5395 5396 /** 5397 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5398 */ 5399 public Money getUnitPrice() { 5400 if (this.unitPrice == null) 5401 if (Configuration.errorOnAutoCreate()) 5402 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 5403 else if (Configuration.doAutoCreate()) 5404 this.unitPrice = new Money(); // cc 5405 return this.unitPrice; 5406 } 5407 5408 public boolean hasUnitPrice() { 5409 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5410 } 5411 5412 /** 5413 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5414 */ 5415 public DetailComponent setUnitPrice(Money value) { 5416 this.unitPrice = value; 5417 return this; 5418 } 5419 5420 /** 5421 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5422 */ 5423 public DecimalType getFactorElement() { 5424 if (this.factor == null) 5425 if (Configuration.errorOnAutoCreate()) 5426 throw new Error("Attempt to auto-create DetailComponent.factor"); 5427 else if (Configuration.doAutoCreate()) 5428 this.factor = new DecimalType(); // bb 5429 return this.factor; 5430 } 5431 5432 public boolean hasFactorElement() { 5433 return this.factor != null && !this.factor.isEmpty(); 5434 } 5435 5436 public boolean hasFactor() { 5437 return this.factor != null && !this.factor.isEmpty(); 5438 } 5439 5440 /** 5441 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5442 */ 5443 public DetailComponent setFactorElement(DecimalType value) { 5444 this.factor = value; 5445 return this; 5446 } 5447 5448 /** 5449 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5450 */ 5451 public BigDecimal getFactor() { 5452 return this.factor == null ? null : this.factor.getValue(); 5453 } 5454 5455 /** 5456 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5457 */ 5458 public DetailComponent setFactor(BigDecimal value) { 5459 if (value == null) 5460 this.factor = null; 5461 else { 5462 if (this.factor == null) 5463 this.factor = new DecimalType(); 5464 this.factor.setValue(value); 5465 } 5466 return this; 5467 } 5468 5469 /** 5470 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5471 */ 5472 public DetailComponent setFactor(long value) { 5473 this.factor = new DecimalType(); 5474 this.factor.setValue(value); 5475 return this; 5476 } 5477 5478 /** 5479 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5480 */ 5481 public DetailComponent setFactor(double value) { 5482 this.factor = new DecimalType(); 5483 this.factor.setValue(value); 5484 return this; 5485 } 5486 5487 /** 5488 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5489 */ 5490 public Money getNet() { 5491 if (this.net == null) 5492 if (Configuration.errorOnAutoCreate()) 5493 throw new Error("Attempt to auto-create DetailComponent.net"); 5494 else if (Configuration.doAutoCreate()) 5495 this.net = new Money(); // cc 5496 return this.net; 5497 } 5498 5499 public boolean hasNet() { 5500 return this.net != null && !this.net.isEmpty(); 5501 } 5502 5503 /** 5504 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5505 */ 5506 public DetailComponent setNet(Money value) { 5507 this.net = value; 5508 return this; 5509 } 5510 5511 /** 5512 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 5513 */ 5514 public List<Reference> getUdi() { 5515 if (this.udi == null) 5516 this.udi = new ArrayList<Reference>(); 5517 return this.udi; 5518 } 5519 5520 /** 5521 * @return Returns a reference to <code>this</code> for easy method chaining 5522 */ 5523 public DetailComponent setUdi(List<Reference> theUdi) { 5524 this.udi = theUdi; 5525 return this; 5526 } 5527 5528 public boolean hasUdi() { 5529 if (this.udi == null) 5530 return false; 5531 for (Reference item : this.udi) 5532 if (!item.isEmpty()) 5533 return true; 5534 return false; 5535 } 5536 5537 public Reference addUdi() { //3 5538 Reference t = new Reference(); 5539 if (this.udi == null) 5540 this.udi = new ArrayList<Reference>(); 5541 this.udi.add(t); 5542 return t; 5543 } 5544 5545 public DetailComponent addUdi(Reference t) { //3 5546 if (t == null) 5547 return this; 5548 if (this.udi == null) 5549 this.udi = new ArrayList<Reference>(); 5550 this.udi.add(t); 5551 return this; 5552 } 5553 5554 /** 5555 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 5556 */ 5557 public Reference getUdiFirstRep() { 5558 if (getUdi().isEmpty()) { 5559 addUdi(); 5560 } 5561 return getUdi().get(0); 5562 } 5563 5564 /** 5565 * @deprecated Use Reference#setResource(IBaseResource) instead 5566 */ 5567 @Deprecated 5568 public List<Device> getUdiTarget() { 5569 if (this.udiTarget == null) 5570 this.udiTarget = new ArrayList<Device>(); 5571 return this.udiTarget; 5572 } 5573 5574 /** 5575 * @deprecated Use Reference#setResource(IBaseResource) instead 5576 */ 5577 @Deprecated 5578 public Device addUdiTarget() { 5579 Device r = new Device(); 5580 if (this.udiTarget == null) 5581 this.udiTarget = new ArrayList<Device>(); 5582 this.udiTarget.add(r); 5583 return r; 5584 } 5585 5586 /** 5587 * @return {@link #subDetail} (Third tier of goods and services.) 5588 */ 5589 public List<SubDetailComponent> getSubDetail() { 5590 if (this.subDetail == null) 5591 this.subDetail = new ArrayList<SubDetailComponent>(); 5592 return this.subDetail; 5593 } 5594 5595 /** 5596 * @return Returns a reference to <code>this</code> for easy method chaining 5597 */ 5598 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 5599 this.subDetail = theSubDetail; 5600 return this; 5601 } 5602 5603 public boolean hasSubDetail() { 5604 if (this.subDetail == null) 5605 return false; 5606 for (SubDetailComponent item : this.subDetail) 5607 if (!item.isEmpty()) 5608 return true; 5609 return false; 5610 } 5611 5612 public SubDetailComponent addSubDetail() { //3 5613 SubDetailComponent t = new SubDetailComponent(); 5614 if (this.subDetail == null) 5615 this.subDetail = new ArrayList<SubDetailComponent>(); 5616 this.subDetail.add(t); 5617 return t; 5618 } 5619 5620 public DetailComponent addSubDetail(SubDetailComponent t) { //3 5621 if (t == null) 5622 return this; 5623 if (this.subDetail == null) 5624 this.subDetail = new ArrayList<SubDetailComponent>(); 5625 this.subDetail.add(t); 5626 return this; 5627 } 5628 5629 /** 5630 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist 5631 */ 5632 public SubDetailComponent getSubDetailFirstRep() { 5633 if (getSubDetail().isEmpty()) { 5634 addSubDetail(); 5635 } 5636 return getSubDetail().get(0); 5637 } 5638 5639 protected void listChildren(List<Property> children) { 5640 super.listChildren(children); 5641 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 5642 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 5643 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 5644 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 5645 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 5646 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 5647 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 5648 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 5649 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 5650 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 5651 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 5652 children.add(new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 5653 } 5654 5655 @Override 5656 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5657 switch (_hash) { 5658 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 5659 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 5660 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 5661 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 5662 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 5663 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 5664 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 5665 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 5666 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 5667 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 5668 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5669 case -828829007: /*subDetail*/ return new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 5670 default: return super.getNamedProperty(_hash, _name, _checkValid); 5671 } 5672 5673 } 5674 5675 @Override 5676 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5677 switch (hash) { 5678 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 5679 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 5680 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 5681 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 5682 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5683 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5684 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 5685 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 5686 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 5687 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 5688 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5689 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 5690 default: return super.getProperty(hash, name, checkValid); 5691 } 5692 5693 } 5694 5695 @Override 5696 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5697 switch (hash) { 5698 case 1349547969: // sequence 5699 this.sequence = castToPositiveInt(value); // PositiveIntType 5700 return value; 5701 case 1099842588: // revenue 5702 this.revenue = castToCodeableConcept(value); // CodeableConcept 5703 return value; 5704 case 50511102: // category 5705 this.category = castToCodeableConcept(value); // CodeableConcept 5706 return value; 5707 case 1984153269: // service 5708 this.service = castToCodeableConcept(value); // CodeableConcept 5709 return value; 5710 case -615513385: // modifier 5711 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5712 return value; 5713 case 1010065041: // programCode 5714 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 5715 return value; 5716 case -1285004149: // quantity 5717 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 5718 return value; 5719 case -486196699: // unitPrice 5720 this.unitPrice = castToMoney(value); // Money 5721 return value; 5722 case -1282148017: // factor 5723 this.factor = castToDecimal(value); // DecimalType 5724 return value; 5725 case 108957: // net 5726 this.net = castToMoney(value); // Money 5727 return value; 5728 case 115642: // udi 5729 this.getUdi().add(castToReference(value)); // Reference 5730 return value; 5731 case -828829007: // subDetail 5732 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 5733 return value; 5734 default: return super.setProperty(hash, name, value); 5735 } 5736 5737 } 5738 5739 @Override 5740 public Base setProperty(String name, Base value) throws FHIRException { 5741 if (name.equals("sequence")) { 5742 this.sequence = castToPositiveInt(value); // PositiveIntType 5743 } else if (name.equals("revenue")) { 5744 this.revenue = castToCodeableConcept(value); // CodeableConcept 5745 } else if (name.equals("category")) { 5746 this.category = castToCodeableConcept(value); // CodeableConcept 5747 } else if (name.equals("service")) { 5748 this.service = castToCodeableConcept(value); // CodeableConcept 5749 } else if (name.equals("modifier")) { 5750 this.getModifier().add(castToCodeableConcept(value)); 5751 } else if (name.equals("programCode")) { 5752 this.getProgramCode().add(castToCodeableConcept(value)); 5753 } else if (name.equals("quantity")) { 5754 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 5755 } else if (name.equals("unitPrice")) { 5756 this.unitPrice = castToMoney(value); // Money 5757 } else if (name.equals("factor")) { 5758 this.factor = castToDecimal(value); // DecimalType 5759 } else if (name.equals("net")) { 5760 this.net = castToMoney(value); // Money 5761 } else if (name.equals("udi")) { 5762 this.getUdi().add(castToReference(value)); 5763 } else if (name.equals("subDetail")) { 5764 this.getSubDetail().add((SubDetailComponent) value); 5765 } else 5766 return super.setProperty(name, value); 5767 return value; 5768 } 5769 5770 @Override 5771 public Base makeProperty(int hash, String name) throws FHIRException { 5772 switch (hash) { 5773 case 1349547969: return getSequenceElement(); 5774 case 1099842588: return getRevenue(); 5775 case 50511102: return getCategory(); 5776 case 1984153269: return getService(); 5777 case -615513385: return addModifier(); 5778 case 1010065041: return addProgramCode(); 5779 case -1285004149: return getQuantity(); 5780 case -486196699: return getUnitPrice(); 5781 case -1282148017: return getFactorElement(); 5782 case 108957: return getNet(); 5783 case 115642: return addUdi(); 5784 case -828829007: return addSubDetail(); 5785 default: return super.makeProperty(hash, name); 5786 } 5787 5788 } 5789 5790 @Override 5791 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5792 switch (hash) { 5793 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 5794 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 5795 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5796 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 5797 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 5798 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 5799 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 5800 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 5801 case -1282148017: /*factor*/ return new String[] {"decimal"}; 5802 case 108957: /*net*/ return new String[] {"Money"}; 5803 case 115642: /*udi*/ return new String[] {"Reference"}; 5804 case -828829007: /*subDetail*/ return new String[] {}; 5805 default: return super.getTypesForProperty(hash, name); 5806 } 5807 5808 } 5809 5810 @Override 5811 public Base addChild(String name) throws FHIRException { 5812 if (name.equals("sequence")) { 5813 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 5814 } 5815 else if (name.equals("revenue")) { 5816 this.revenue = new CodeableConcept(); 5817 return this.revenue; 5818 } 5819 else if (name.equals("category")) { 5820 this.category = new CodeableConcept(); 5821 return this.category; 5822 } 5823 else if (name.equals("service")) { 5824 this.service = new CodeableConcept(); 5825 return this.service; 5826 } 5827 else if (name.equals("modifier")) { 5828 return addModifier(); 5829 } 5830 else if (name.equals("programCode")) { 5831 return addProgramCode(); 5832 } 5833 else if (name.equals("quantity")) { 5834 this.quantity = new SimpleQuantity(); 5835 return this.quantity; 5836 } 5837 else if (name.equals("unitPrice")) { 5838 this.unitPrice = new Money(); 5839 return this.unitPrice; 5840 } 5841 else if (name.equals("factor")) { 5842 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 5843 } 5844 else if (name.equals("net")) { 5845 this.net = new Money(); 5846 return this.net; 5847 } 5848 else if (name.equals("udi")) { 5849 return addUdi(); 5850 } 5851 else if (name.equals("subDetail")) { 5852 return addSubDetail(); 5853 } 5854 else 5855 return super.addChild(name); 5856 } 5857 5858 public DetailComponent copy() { 5859 DetailComponent dst = new DetailComponent(); 5860 copyValues(dst); 5861 dst.sequence = sequence == null ? null : sequence.copy(); 5862 dst.revenue = revenue == null ? null : revenue.copy(); 5863 dst.category = category == null ? null : category.copy(); 5864 dst.service = service == null ? null : service.copy(); 5865 if (modifier != null) { 5866 dst.modifier = new ArrayList<CodeableConcept>(); 5867 for (CodeableConcept i : modifier) 5868 dst.modifier.add(i.copy()); 5869 }; 5870 if (programCode != null) { 5871 dst.programCode = new ArrayList<CodeableConcept>(); 5872 for (CodeableConcept i : programCode) 5873 dst.programCode.add(i.copy()); 5874 }; 5875 dst.quantity = quantity == null ? null : quantity.copy(); 5876 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5877 dst.factor = factor == null ? null : factor.copy(); 5878 dst.net = net == null ? null : net.copy(); 5879 if (udi != null) { 5880 dst.udi = new ArrayList<Reference>(); 5881 for (Reference i : udi) 5882 dst.udi.add(i.copy()); 5883 }; 5884 if (subDetail != null) { 5885 dst.subDetail = new ArrayList<SubDetailComponent>(); 5886 for (SubDetailComponent i : subDetail) 5887 dst.subDetail.add(i.copy()); 5888 }; 5889 return dst; 5890 } 5891 5892 @Override 5893 public boolean equalsDeep(Base other_) { 5894 if (!super.equalsDeep(other_)) 5895 return false; 5896 if (!(other_ instanceof DetailComponent)) 5897 return false; 5898 DetailComponent o = (DetailComponent) other_; 5899 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) 5900 && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 5901 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 5902 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(subDetail, o.subDetail, true) 5903 ; 5904 } 5905 5906 @Override 5907 public boolean equalsShallow(Base other_) { 5908 if (!super.equalsShallow(other_)) 5909 return false; 5910 if (!(other_ instanceof DetailComponent)) 5911 return false; 5912 DetailComponent o = (DetailComponent) other_; 5913 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 5914 } 5915 5916 public boolean isEmpty() { 5917 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category 5918 , service, modifier, programCode, quantity, unitPrice, factor, net, udi, subDetail 5919 ); 5920 } 5921 5922 public String fhirType() { 5923 return "Claim.item.detail"; 5924 5925 } 5926 5927 } 5928 5929 @Block() 5930 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 5931 /** 5932 * A service line number. 5933 */ 5934 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5935 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 5936 protected PositiveIntType sequence; 5937 5938 /** 5939 * The type of reveneu or cost center providing the product and/or service. 5940 */ 5941 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 5942 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 5943 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5944 protected CodeableConcept revenue; 5945 5946 /** 5947 * Health Care Service Type Codes to identify the classification of service or benefits. 5948 */ 5949 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5950 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 5951 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 5952 protected CodeableConcept category; 5953 5954 /** 5955 * A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). 5956 */ 5957 @Child(name = "service", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5958 @Description(shortDefinition="Billing Code", formalDefinition="A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI)." ) 5959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5960 protected CodeableConcept service; 5961 5962 /** 5963 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 5964 */ 5965 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5966 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 5967 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5968 protected List<CodeableConcept> modifier; 5969 5970 /** 5971 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 5972 */ 5973 @Child(name = "programCode", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5974 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 5975 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5976 protected List<CodeableConcept> programCode; 5977 5978 /** 5979 * The number of repetitions of a service or product. 5980 */ 5981 @Child(name = "quantity", type = {SimpleQuantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 5982 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 5983 protected SimpleQuantity quantity; 5984 5985 /** 5986 * The fee for an addittional service or product or charge. 5987 */ 5988 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 5989 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="The fee for an addittional service or product or charge." ) 5990 protected Money unitPrice; 5991 5992 /** 5993 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5994 */ 5995 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5996 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5997 protected DecimalType factor; 5998 5999 /** 6000 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 6001 */ 6002 @Child(name = "net", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 6003 @Description(shortDefinition="Net additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 6004 protected Money net; 6005 6006 /** 6007 * List of Unique Device Identifiers associated with this line item. 6008 */ 6009 @Child(name = "udi", type = {Device.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6010 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 6011 protected List<Reference> udi; 6012 /** 6013 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 6014 */ 6015 protected List<Device> udiTarget; 6016 6017 6018 private static final long serialVersionUID = -1210367567L; 6019 6020 /** 6021 * Constructor 6022 */ 6023 public SubDetailComponent() { 6024 super(); 6025 } 6026 6027 /** 6028 * Constructor 6029 */ 6030 public SubDetailComponent(PositiveIntType sequence) { 6031 super(); 6032 this.sequence = sequence; 6033 } 6034 6035 /** 6036 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6037 */ 6038 public PositiveIntType getSequenceElement() { 6039 if (this.sequence == null) 6040 if (Configuration.errorOnAutoCreate()) 6041 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 6042 else if (Configuration.doAutoCreate()) 6043 this.sequence = new PositiveIntType(); // bb 6044 return this.sequence; 6045 } 6046 6047 public boolean hasSequenceElement() { 6048 return this.sequence != null && !this.sequence.isEmpty(); 6049 } 6050 6051 public boolean hasSequence() { 6052 return this.sequence != null && !this.sequence.isEmpty(); 6053 } 6054 6055 /** 6056 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6057 */ 6058 public SubDetailComponent setSequenceElement(PositiveIntType value) { 6059 this.sequence = value; 6060 return this; 6061 } 6062 6063 /** 6064 * @return A service line number. 6065 */ 6066 public int getSequence() { 6067 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6068 } 6069 6070 /** 6071 * @param value A service line number. 6072 */ 6073 public SubDetailComponent setSequence(int value) { 6074 if (this.sequence == null) 6075 this.sequence = new PositiveIntType(); 6076 this.sequence.setValue(value); 6077 return this; 6078 } 6079 6080 /** 6081 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6082 */ 6083 public CodeableConcept getRevenue() { 6084 if (this.revenue == null) 6085 if (Configuration.errorOnAutoCreate()) 6086 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 6087 else if (Configuration.doAutoCreate()) 6088 this.revenue = new CodeableConcept(); // cc 6089 return this.revenue; 6090 } 6091 6092 public boolean hasRevenue() { 6093 return this.revenue != null && !this.revenue.isEmpty(); 6094 } 6095 6096 /** 6097 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6098 */ 6099 public SubDetailComponent setRevenue(CodeableConcept value) { 6100 this.revenue = value; 6101 return this; 6102 } 6103 6104 /** 6105 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6106 */ 6107 public CodeableConcept getCategory() { 6108 if (this.category == null) 6109 if (Configuration.errorOnAutoCreate()) 6110 throw new Error("Attempt to auto-create SubDetailComponent.category"); 6111 else if (Configuration.doAutoCreate()) 6112 this.category = new CodeableConcept(); // cc 6113 return this.category; 6114 } 6115 6116 public boolean hasCategory() { 6117 return this.category != null && !this.category.isEmpty(); 6118 } 6119 6120 /** 6121 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6122 */ 6123 public SubDetailComponent setCategory(CodeableConcept value) { 6124 this.category = value; 6125 return this; 6126 } 6127 6128 /** 6129 * @return {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6130 */ 6131 public CodeableConcept getService() { 6132 if (this.service == null) 6133 if (Configuration.errorOnAutoCreate()) 6134 throw new Error("Attempt to auto-create SubDetailComponent.service"); 6135 else if (Configuration.doAutoCreate()) 6136 this.service = new CodeableConcept(); // cc 6137 return this.service; 6138 } 6139 6140 public boolean hasService() { 6141 return this.service != null && !this.service.isEmpty(); 6142 } 6143 6144 /** 6145 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6146 */ 6147 public SubDetailComponent setService(CodeableConcept value) { 6148 this.service = value; 6149 return this; 6150 } 6151 6152 /** 6153 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 6154 */ 6155 public List<CodeableConcept> getModifier() { 6156 if (this.modifier == null) 6157 this.modifier = new ArrayList<CodeableConcept>(); 6158 return this.modifier; 6159 } 6160 6161 /** 6162 * @return Returns a reference to <code>this</code> for easy method chaining 6163 */ 6164 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 6165 this.modifier = theModifier; 6166 return this; 6167 } 6168 6169 public boolean hasModifier() { 6170 if (this.modifier == null) 6171 return false; 6172 for (CodeableConcept item : this.modifier) 6173 if (!item.isEmpty()) 6174 return true; 6175 return false; 6176 } 6177 6178 public CodeableConcept addModifier() { //3 6179 CodeableConcept t = new CodeableConcept(); 6180 if (this.modifier == null) 6181 this.modifier = new ArrayList<CodeableConcept>(); 6182 this.modifier.add(t); 6183 return t; 6184 } 6185 6186 public SubDetailComponent addModifier(CodeableConcept t) { //3 6187 if (t == null) 6188 return this; 6189 if (this.modifier == null) 6190 this.modifier = new ArrayList<CodeableConcept>(); 6191 this.modifier.add(t); 6192 return this; 6193 } 6194 6195 /** 6196 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 6197 */ 6198 public CodeableConcept getModifierFirstRep() { 6199 if (getModifier().isEmpty()) { 6200 addModifier(); 6201 } 6202 return getModifier().get(0); 6203 } 6204 6205 /** 6206 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 6207 */ 6208 public List<CodeableConcept> getProgramCode() { 6209 if (this.programCode == null) 6210 this.programCode = new ArrayList<CodeableConcept>(); 6211 return this.programCode; 6212 } 6213 6214 /** 6215 * @return Returns a reference to <code>this</code> for easy method chaining 6216 */ 6217 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6218 this.programCode = theProgramCode; 6219 return this; 6220 } 6221 6222 public boolean hasProgramCode() { 6223 if (this.programCode == null) 6224 return false; 6225 for (CodeableConcept item : this.programCode) 6226 if (!item.isEmpty()) 6227 return true; 6228 return false; 6229 } 6230 6231 public CodeableConcept addProgramCode() { //3 6232 CodeableConcept t = new CodeableConcept(); 6233 if (this.programCode == null) 6234 this.programCode = new ArrayList<CodeableConcept>(); 6235 this.programCode.add(t); 6236 return t; 6237 } 6238 6239 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 6240 if (t == null) 6241 return this; 6242 if (this.programCode == null) 6243 this.programCode = new ArrayList<CodeableConcept>(); 6244 this.programCode.add(t); 6245 return this; 6246 } 6247 6248 /** 6249 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 6250 */ 6251 public CodeableConcept getProgramCodeFirstRep() { 6252 if (getProgramCode().isEmpty()) { 6253 addProgramCode(); 6254 } 6255 return getProgramCode().get(0); 6256 } 6257 6258 /** 6259 * @return {@link #quantity} (The number of repetitions of a service or product.) 6260 */ 6261 public SimpleQuantity getQuantity() { 6262 if (this.quantity == null) 6263 if (Configuration.errorOnAutoCreate()) 6264 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 6265 else if (Configuration.doAutoCreate()) 6266 this.quantity = new SimpleQuantity(); // cc 6267 return this.quantity; 6268 } 6269 6270 public boolean hasQuantity() { 6271 return this.quantity != null && !this.quantity.isEmpty(); 6272 } 6273 6274 /** 6275 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6276 */ 6277 public SubDetailComponent setQuantity(SimpleQuantity value) { 6278 this.quantity = value; 6279 return this; 6280 } 6281 6282 /** 6283 * @return {@link #unitPrice} (The fee for an addittional service or product or charge.) 6284 */ 6285 public Money getUnitPrice() { 6286 if (this.unitPrice == null) 6287 if (Configuration.errorOnAutoCreate()) 6288 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 6289 else if (Configuration.doAutoCreate()) 6290 this.unitPrice = new Money(); // cc 6291 return this.unitPrice; 6292 } 6293 6294 public boolean hasUnitPrice() { 6295 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6296 } 6297 6298 /** 6299 * @param value {@link #unitPrice} (The fee for an addittional service or product or charge.) 6300 */ 6301 public SubDetailComponent setUnitPrice(Money value) { 6302 this.unitPrice = value; 6303 return this; 6304 } 6305 6306 /** 6307 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6308 */ 6309 public DecimalType getFactorElement() { 6310 if (this.factor == null) 6311 if (Configuration.errorOnAutoCreate()) 6312 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 6313 else if (Configuration.doAutoCreate()) 6314 this.factor = new DecimalType(); // bb 6315 return this.factor; 6316 } 6317 6318 public boolean hasFactorElement() { 6319 return this.factor != null && !this.factor.isEmpty(); 6320 } 6321 6322 public boolean hasFactor() { 6323 return this.factor != null && !this.factor.isEmpty(); 6324 } 6325 6326 /** 6327 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6328 */ 6329 public SubDetailComponent setFactorElement(DecimalType value) { 6330 this.factor = value; 6331 return this; 6332 } 6333 6334 /** 6335 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6336 */ 6337 public BigDecimal getFactor() { 6338 return this.factor == null ? null : this.factor.getValue(); 6339 } 6340 6341 /** 6342 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6343 */ 6344 public SubDetailComponent setFactor(BigDecimal value) { 6345 if (value == null) 6346 this.factor = null; 6347 else { 6348 if (this.factor == null) 6349 this.factor = new DecimalType(); 6350 this.factor.setValue(value); 6351 } 6352 return this; 6353 } 6354 6355 /** 6356 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6357 */ 6358 public SubDetailComponent setFactor(long value) { 6359 this.factor = new DecimalType(); 6360 this.factor.setValue(value); 6361 return this; 6362 } 6363 6364 /** 6365 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6366 */ 6367 public SubDetailComponent setFactor(double value) { 6368 this.factor = new DecimalType(); 6369 this.factor.setValue(value); 6370 return this; 6371 } 6372 6373 /** 6374 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6375 */ 6376 public Money getNet() { 6377 if (this.net == null) 6378 if (Configuration.errorOnAutoCreate()) 6379 throw new Error("Attempt to auto-create SubDetailComponent.net"); 6380 else if (Configuration.doAutoCreate()) 6381 this.net = new Money(); // cc 6382 return this.net; 6383 } 6384 6385 public boolean hasNet() { 6386 return this.net != null && !this.net.isEmpty(); 6387 } 6388 6389 /** 6390 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6391 */ 6392 public SubDetailComponent setNet(Money value) { 6393 this.net = value; 6394 return this; 6395 } 6396 6397 /** 6398 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 6399 */ 6400 public List<Reference> getUdi() { 6401 if (this.udi == null) 6402 this.udi = new ArrayList<Reference>(); 6403 return this.udi; 6404 } 6405 6406 /** 6407 * @return Returns a reference to <code>this</code> for easy method chaining 6408 */ 6409 public SubDetailComponent setUdi(List<Reference> theUdi) { 6410 this.udi = theUdi; 6411 return this; 6412 } 6413 6414 public boolean hasUdi() { 6415 if (this.udi == null) 6416 return false; 6417 for (Reference item : this.udi) 6418 if (!item.isEmpty()) 6419 return true; 6420 return false; 6421 } 6422 6423 public Reference addUdi() { //3 6424 Reference t = new Reference(); 6425 if (this.udi == null) 6426 this.udi = new ArrayList<Reference>(); 6427 this.udi.add(t); 6428 return t; 6429 } 6430 6431 public SubDetailComponent addUdi(Reference t) { //3 6432 if (t == null) 6433 return this; 6434 if (this.udi == null) 6435 this.udi = new ArrayList<Reference>(); 6436 this.udi.add(t); 6437 return this; 6438 } 6439 6440 /** 6441 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 6442 */ 6443 public Reference getUdiFirstRep() { 6444 if (getUdi().isEmpty()) { 6445 addUdi(); 6446 } 6447 return getUdi().get(0); 6448 } 6449 6450 /** 6451 * @deprecated Use Reference#setResource(IBaseResource) instead 6452 */ 6453 @Deprecated 6454 public List<Device> getUdiTarget() { 6455 if (this.udiTarget == null) 6456 this.udiTarget = new ArrayList<Device>(); 6457 return this.udiTarget; 6458 } 6459 6460 /** 6461 * @deprecated Use Reference#setResource(IBaseResource) instead 6462 */ 6463 @Deprecated 6464 public Device addUdiTarget() { 6465 Device r = new Device(); 6466 if (this.udiTarget == null) 6467 this.udiTarget = new ArrayList<Device>(); 6468 this.udiTarget.add(r); 6469 return r; 6470 } 6471 6472 protected void listChildren(List<Property> children) { 6473 super.listChildren(children); 6474 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 6475 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 6476 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 6477 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service)); 6478 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 6479 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 6480 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 6481 children.add(new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice)); 6482 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6483 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 6484 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 6485 } 6486 6487 @Override 6488 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6489 switch (_hash) { 6490 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 6491 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 6492 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 6493 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service); 6494 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 6495 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 6496 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 6497 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice); 6498 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6499 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 6500 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 6501 default: return super.getNamedProperty(_hash, _name, _checkValid); 6502 } 6503 6504 } 6505 6506 @Override 6507 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6508 switch (hash) { 6509 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 6510 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 6511 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6512 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 6513 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 6514 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 6515 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 6516 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6517 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6518 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6519 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 6520 default: return super.getProperty(hash, name, checkValid); 6521 } 6522 6523 } 6524 6525 @Override 6526 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6527 switch (hash) { 6528 case 1349547969: // sequence 6529 this.sequence = castToPositiveInt(value); // PositiveIntType 6530 return value; 6531 case 1099842588: // revenue 6532 this.revenue = castToCodeableConcept(value); // CodeableConcept 6533 return value; 6534 case 50511102: // category 6535 this.category = castToCodeableConcept(value); // CodeableConcept 6536 return value; 6537 case 1984153269: // service 6538 this.service = castToCodeableConcept(value); // CodeableConcept 6539 return value; 6540 case -615513385: // modifier 6541 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 6542 return value; 6543 case 1010065041: // programCode 6544 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 6545 return value; 6546 case -1285004149: // quantity 6547 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 6548 return value; 6549 case -486196699: // unitPrice 6550 this.unitPrice = castToMoney(value); // Money 6551 return value; 6552 case -1282148017: // factor 6553 this.factor = castToDecimal(value); // DecimalType 6554 return value; 6555 case 108957: // net 6556 this.net = castToMoney(value); // Money 6557 return value; 6558 case 115642: // udi 6559 this.getUdi().add(castToReference(value)); // Reference 6560 return value; 6561 default: return super.setProperty(hash, name, value); 6562 } 6563 6564 } 6565 6566 @Override 6567 public Base setProperty(String name, Base value) throws FHIRException { 6568 if (name.equals("sequence")) { 6569 this.sequence = castToPositiveInt(value); // PositiveIntType 6570 } else if (name.equals("revenue")) { 6571 this.revenue = castToCodeableConcept(value); // CodeableConcept 6572 } else if (name.equals("category")) { 6573 this.category = castToCodeableConcept(value); // CodeableConcept 6574 } else if (name.equals("service")) { 6575 this.service = castToCodeableConcept(value); // CodeableConcept 6576 } else if (name.equals("modifier")) { 6577 this.getModifier().add(castToCodeableConcept(value)); 6578 } else if (name.equals("programCode")) { 6579 this.getProgramCode().add(castToCodeableConcept(value)); 6580 } else if (name.equals("quantity")) { 6581 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 6582 } else if (name.equals("unitPrice")) { 6583 this.unitPrice = castToMoney(value); // Money 6584 } else if (name.equals("factor")) { 6585 this.factor = castToDecimal(value); // DecimalType 6586 } else if (name.equals("net")) { 6587 this.net = castToMoney(value); // Money 6588 } else if (name.equals("udi")) { 6589 this.getUdi().add(castToReference(value)); 6590 } else 6591 return super.setProperty(name, value); 6592 return value; 6593 } 6594 6595 @Override 6596 public Base makeProperty(int hash, String name) throws FHIRException { 6597 switch (hash) { 6598 case 1349547969: return getSequenceElement(); 6599 case 1099842588: return getRevenue(); 6600 case 50511102: return getCategory(); 6601 case 1984153269: return getService(); 6602 case -615513385: return addModifier(); 6603 case 1010065041: return addProgramCode(); 6604 case -1285004149: return getQuantity(); 6605 case -486196699: return getUnitPrice(); 6606 case -1282148017: return getFactorElement(); 6607 case 108957: return getNet(); 6608 case 115642: return addUdi(); 6609 default: return super.makeProperty(hash, name); 6610 } 6611 6612 } 6613 6614 @Override 6615 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6616 switch (hash) { 6617 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 6618 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 6619 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6620 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 6621 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 6622 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 6623 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 6624 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6625 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6626 case 108957: /*net*/ return new String[] {"Money"}; 6627 case 115642: /*udi*/ return new String[] {"Reference"}; 6628 default: return super.getTypesForProperty(hash, name); 6629 } 6630 6631 } 6632 6633 @Override 6634 public Base addChild(String name) throws FHIRException { 6635 if (name.equals("sequence")) { 6636 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 6637 } 6638 else if (name.equals("revenue")) { 6639 this.revenue = new CodeableConcept(); 6640 return this.revenue; 6641 } 6642 else if (name.equals("category")) { 6643 this.category = new CodeableConcept(); 6644 return this.category; 6645 } 6646 else if (name.equals("service")) { 6647 this.service = new CodeableConcept(); 6648 return this.service; 6649 } 6650 else if (name.equals("modifier")) { 6651 return addModifier(); 6652 } 6653 else if (name.equals("programCode")) { 6654 return addProgramCode(); 6655 } 6656 else if (name.equals("quantity")) { 6657 this.quantity = new SimpleQuantity(); 6658 return this.quantity; 6659 } 6660 else if (name.equals("unitPrice")) { 6661 this.unitPrice = new Money(); 6662 return this.unitPrice; 6663 } 6664 else if (name.equals("factor")) { 6665 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 6666 } 6667 else if (name.equals("net")) { 6668 this.net = new Money(); 6669 return this.net; 6670 } 6671 else if (name.equals("udi")) { 6672 return addUdi(); 6673 } 6674 else 6675 return super.addChild(name); 6676 } 6677 6678 public SubDetailComponent copy() { 6679 SubDetailComponent dst = new SubDetailComponent(); 6680 copyValues(dst); 6681 dst.sequence = sequence == null ? null : sequence.copy(); 6682 dst.revenue = revenue == null ? null : revenue.copy(); 6683 dst.category = category == null ? null : category.copy(); 6684 dst.service = service == null ? null : service.copy(); 6685 if (modifier != null) { 6686 dst.modifier = new ArrayList<CodeableConcept>(); 6687 for (CodeableConcept i : modifier) 6688 dst.modifier.add(i.copy()); 6689 }; 6690 if (programCode != null) { 6691 dst.programCode = new ArrayList<CodeableConcept>(); 6692 for (CodeableConcept i : programCode) 6693 dst.programCode.add(i.copy()); 6694 }; 6695 dst.quantity = quantity == null ? null : quantity.copy(); 6696 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6697 dst.factor = factor == null ? null : factor.copy(); 6698 dst.net = net == null ? null : net.copy(); 6699 if (udi != null) { 6700 dst.udi = new ArrayList<Reference>(); 6701 for (Reference i : udi) 6702 dst.udi.add(i.copy()); 6703 }; 6704 return dst; 6705 } 6706 6707 @Override 6708 public boolean equalsDeep(Base other_) { 6709 if (!super.equalsDeep(other_)) 6710 return false; 6711 if (!(other_ instanceof SubDetailComponent)) 6712 return false; 6713 SubDetailComponent o = (SubDetailComponent) other_; 6714 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) 6715 && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 6716 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 6717 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true); 6718 } 6719 6720 @Override 6721 public boolean equalsShallow(Base other_) { 6722 if (!super.equalsShallow(other_)) 6723 return false; 6724 if (!(other_ instanceof SubDetailComponent)) 6725 return false; 6726 SubDetailComponent o = (SubDetailComponent) other_; 6727 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 6728 } 6729 6730 public boolean isEmpty() { 6731 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category 6732 , service, modifier, programCode, quantity, unitPrice, factor, net, udi); 6733 } 6734 6735 public String fhirType() { 6736 return "Claim.item.detail.subDetail"; 6737 6738 } 6739 6740 } 6741 6742 /** 6743 * The business identifier for the instance: claim number, pre-determination or pre-authorization number. 6744 */ 6745 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6746 @Description(shortDefinition="Claim number", formalDefinition="The business identifier for the instance: claim number, pre-determination or pre-authorization number." ) 6747 protected List<Identifier> identifier; 6748 6749 /** 6750 * The status of the resource instance. 6751 */ 6752 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 6753 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 6754 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 6755 protected Enumeration<ClaimStatus> status; 6756 6757 /** 6758 * The category of claim, eg, oral, pharmacy, vision, insitutional, professional. 6759 */ 6760 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 6761 @Description(shortDefinition="Type or discipline", formalDefinition="The category of claim, eg, oral, pharmacy, vision, insitutional, professional." ) 6762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 6763 protected CodeableConcept type; 6764 6765 /** 6766 * A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType. 6767 */ 6768 @Child(name = "subType", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6769 @Description(shortDefinition="Finer grained claim type information", formalDefinition="A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType." ) 6770 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 6771 protected List<CodeableConcept> subType; 6772 6773 /** 6774 * Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination). 6775 */ 6776 @Child(name = "use", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 6777 @Description(shortDefinition="complete | proposed | exploratory | other", formalDefinition="Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination)." ) 6778 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-use") 6779 protected Enumeration<Use> use; 6780 6781 /** 6782 * Patient Resource. 6783 */ 6784 @Child(name = "patient", type = {Patient.class}, order=5, min=0, max=1, modifier=false, summary=false) 6785 @Description(shortDefinition="The subject of the Products and Services", formalDefinition="Patient Resource." ) 6786 protected Reference patient; 6787 6788 /** 6789 * The actual object that is the target of the reference (Patient Resource.) 6790 */ 6791 protected Patient patientTarget; 6792 6793 /** 6794 * The billable period for which charges are being submitted. 6795 */ 6796 @Child(name = "billablePeriod", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=false) 6797 @Description(shortDefinition="Period for charge submission", formalDefinition="The billable period for which charges are being submitted." ) 6798 protected Period billablePeriod; 6799 6800 /** 6801 * The date when the enclosed suite of services were performed or completed. 6802 */ 6803 @Child(name = "created", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 6804 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 6805 protected DateTimeType created; 6806 6807 /** 6808 * Person who created the invoice/claim/pre-determination or pre-authorization. 6809 */ 6810 @Child(name = "enterer", type = {Practitioner.class}, order=8, min=0, max=1, modifier=false, summary=false) 6811 @Description(shortDefinition="Author", formalDefinition="Person who created the invoice/claim/pre-determination or pre-authorization." ) 6812 protected Reference enterer; 6813 6814 /** 6815 * The actual object that is the target of the reference (Person who created the invoice/claim/pre-determination or pre-authorization.) 6816 */ 6817 protected Practitioner entererTarget; 6818 6819 /** 6820 * The Insurer who is target of the request. 6821 */ 6822 @Child(name = "insurer", type = {Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 6823 @Description(shortDefinition="Target", formalDefinition="The Insurer who is target of the request." ) 6824 protected Reference insurer; 6825 6826 /** 6827 * The actual object that is the target of the reference (The Insurer who is target of the request.) 6828 */ 6829 protected Organization insurerTarget; 6830 6831 /** 6832 * The provider which is responsible for the bill, claim pre-determination, pre-authorization. 6833 */ 6834 @Child(name = "provider", type = {Practitioner.class}, order=10, min=0, max=1, modifier=false, summary=false) 6835 @Description(shortDefinition="Responsible provider", formalDefinition="The provider which is responsible for the bill, claim pre-determination, pre-authorization." ) 6836 protected Reference provider; 6837 6838 /** 6839 * The actual object that is the target of the reference (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 6840 */ 6841 protected Practitioner providerTarget; 6842 6843 /** 6844 * The organization which is responsible for the bill, claim pre-determination, pre-authorization. 6845 */ 6846 @Child(name = "organization", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=false) 6847 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the bill, claim pre-determination, pre-authorization." ) 6848 protected Reference organization; 6849 6850 /** 6851 * The actual object that is the target of the reference (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 6852 */ 6853 protected Organization organizationTarget; 6854 6855 /** 6856 * Immediate (STAT), best effort (NORMAL), deferred (DEFER). 6857 */ 6858 @Child(name = "priority", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 6859 @Description(shortDefinition="Desired processing priority", formalDefinition="Immediate (STAT), best effort (NORMAL), deferred (DEFER)." ) 6860 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 6861 protected CodeableConcept priority; 6862 6863 /** 6864 * In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested. 6865 */ 6866 @Child(name = "fundsReserve", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 6867 @Description(shortDefinition="Funds requested to be reserved", formalDefinition="In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested." ) 6868 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 6869 protected CodeableConcept fundsReserve; 6870 6871 /** 6872 * Other claims which are related to this claim such as prior claim versions or for related services. 6873 */ 6874 @Child(name = "related", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6875 @Description(shortDefinition="Related Claims which may be revelant to processing this claimn", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 6876 protected List<RelatedClaimComponent> related; 6877 6878 /** 6879 * Prescription to support the dispensing of Pharmacy or Vision products. 6880 */ 6881 @Child(name = "prescription", type = {MedicationRequest.class, VisionPrescription.class}, order=15, min=0, max=1, modifier=false, summary=false) 6882 @Description(shortDefinition="Prescription authorizing services or products", formalDefinition="Prescription to support the dispensing of Pharmacy or Vision products." ) 6883 protected Reference prescription; 6884 6885 /** 6886 * The actual object that is the target of the reference (Prescription to support the dispensing of Pharmacy or Vision products.) 6887 */ 6888 protected Resource prescriptionTarget; 6889 6890 /** 6891 * Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'. 6892 */ 6893 @Child(name = "originalPrescription", type = {MedicationRequest.class}, order=16, min=0, max=1, modifier=false, summary=false) 6894 @Description(shortDefinition="Original prescription if superceded by fulfiller", formalDefinition="Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'." ) 6895 protected Reference originalPrescription; 6896 6897 /** 6898 * The actual object that is the target of the reference (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 6899 */ 6900 protected MedicationRequest originalPrescriptionTarget; 6901 6902 /** 6903 * The party to be reimbursed for the services. 6904 */ 6905 @Child(name = "payee", type = {}, order=17, min=0, max=1, modifier=false, summary=false) 6906 @Description(shortDefinition="Party to be paid any benefits payable", formalDefinition="The party to be reimbursed for the services." ) 6907 protected PayeeComponent payee; 6908 6909 /** 6910 * The referral resource which lists the date, practitioner, reason and other supporting information. 6911 */ 6912 @Child(name = "referral", type = {ReferralRequest.class}, order=18, min=0, max=1, modifier=false, summary=false) 6913 @Description(shortDefinition="Treatment Referral", formalDefinition="The referral resource which lists the date, practitioner, reason and other supporting information." ) 6914 protected Reference referral; 6915 6916 /** 6917 * The actual object that is the target of the reference (The referral resource which lists the date, practitioner, reason and other supporting information.) 6918 */ 6919 protected ReferralRequest referralTarget; 6920 6921 /** 6922 * Facility where the services were provided. 6923 */ 6924 @Child(name = "facility", type = {Location.class}, order=19, min=0, max=1, modifier=false, summary=false) 6925 @Description(shortDefinition="Servicing Facility", formalDefinition="Facility where the services were provided." ) 6926 protected Reference facility; 6927 6928 /** 6929 * The actual object that is the target of the reference (Facility where the services were provided.) 6930 */ 6931 protected Location facilityTarget; 6932 6933 /** 6934 * The members of the team who provided the overall service as well as their role and whether responsible and qualifications. 6935 */ 6936 @Child(name = "careTeam", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6937 @Description(shortDefinition="Members of the care team", formalDefinition="The members of the team who provided the overall service as well as their role and whether responsible and qualifications." ) 6938 protected List<CareTeamComponent> careTeam; 6939 6940 /** 6941 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required. 6942 */ 6943 @Child(name = "information", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6944 @Description(shortDefinition="Exceptions, special considerations, the condition, situation, prior or concurrent issues", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required." ) 6945 protected List<SpecialConditionComponent> information; 6946 6947 /** 6948 * List of patient diagnosis for which care is sought. 6949 */ 6950 @Child(name = "diagnosis", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6951 @Description(shortDefinition="List of Diagnosis", formalDefinition="List of patient diagnosis for which care is sought." ) 6952 protected List<DiagnosisComponent> diagnosis; 6953 6954 /** 6955 * Ordered list of patient procedures performed to support the adjudication. 6956 */ 6957 @Child(name = "procedure", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6958 @Description(shortDefinition="Procedures performed", formalDefinition="Ordered list of patient procedures performed to support the adjudication." ) 6959 protected List<ProcedureComponent> procedure; 6960 6961 /** 6962 * Financial instrument by which payment information for health care. 6963 */ 6964 @Child(name = "insurance", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6965 @Description(shortDefinition="Insurance or medical plan", formalDefinition="Financial instrument by which payment information for health care." ) 6966 protected List<InsuranceComponent> insurance; 6967 6968 /** 6969 * An accident which resulted in the need for healthcare services. 6970 */ 6971 @Child(name = "accident", type = {}, order=25, min=0, max=1, modifier=false, summary=false) 6972 @Description(shortDefinition="Details about an accident", formalDefinition="An accident which resulted in the need for healthcare services." ) 6973 protected AccidentComponent accident; 6974 6975 /** 6976 * The start and optional end dates of when the patient was precluded from working due to the treatable condition(s). 6977 */ 6978 @Child(name = "employmentImpacted", type = {Period.class}, order=26, min=0, max=1, modifier=false, summary=false) 6979 @Description(shortDefinition="Period unable to work", formalDefinition="The start and optional end dates of when the patient was precluded from working due to the treatable condition(s)." ) 6980 protected Period employmentImpacted; 6981 6982 /** 6983 * The start and optional end dates of when the patient was confined to a treatment center. 6984 */ 6985 @Child(name = "hospitalization", type = {Period.class}, order=27, min=0, max=1, modifier=false, summary=false) 6986 @Description(shortDefinition="Period in hospital", formalDefinition="The start and optional end dates of when the patient was confined to a treatment center." ) 6987 protected Period hospitalization; 6988 6989 /** 6990 * First tier of goods and services. 6991 */ 6992 @Child(name = "item", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6993 @Description(shortDefinition="Goods and Services", formalDefinition="First tier of goods and services." ) 6994 protected List<ItemComponent> item; 6995 6996 /** 6997 * The total value of the claim. 6998 */ 6999 @Child(name = "total", type = {Money.class}, order=29, min=0, max=1, modifier=false, summary=false) 7000 @Description(shortDefinition="Total claim cost", formalDefinition="The total value of the claim." ) 7001 protected Money total; 7002 7003 private static final long serialVersionUID = 1731171342L; 7004 7005 /** 7006 * Constructor 7007 */ 7008 public Claim() { 7009 super(); 7010 } 7011 7012 /** 7013 * @return {@link #identifier} (The business identifier for the instance: claim number, pre-determination or pre-authorization number.) 7014 */ 7015 public List<Identifier> getIdentifier() { 7016 if (this.identifier == null) 7017 this.identifier = new ArrayList<Identifier>(); 7018 return this.identifier; 7019 } 7020 7021 /** 7022 * @return Returns a reference to <code>this</code> for easy method chaining 7023 */ 7024 public Claim setIdentifier(List<Identifier> theIdentifier) { 7025 this.identifier = theIdentifier; 7026 return this; 7027 } 7028 7029 public boolean hasIdentifier() { 7030 if (this.identifier == null) 7031 return false; 7032 for (Identifier item : this.identifier) 7033 if (!item.isEmpty()) 7034 return true; 7035 return false; 7036 } 7037 7038 public Identifier addIdentifier() { //3 7039 Identifier t = new Identifier(); 7040 if (this.identifier == null) 7041 this.identifier = new ArrayList<Identifier>(); 7042 this.identifier.add(t); 7043 return t; 7044 } 7045 7046 public Claim addIdentifier(Identifier t) { //3 7047 if (t == null) 7048 return this; 7049 if (this.identifier == null) 7050 this.identifier = new ArrayList<Identifier>(); 7051 this.identifier.add(t); 7052 return this; 7053 } 7054 7055 /** 7056 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 7057 */ 7058 public Identifier getIdentifierFirstRep() { 7059 if (getIdentifier().isEmpty()) { 7060 addIdentifier(); 7061 } 7062 return getIdentifier().get(0); 7063 } 7064 7065 /** 7066 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 7067 */ 7068 public Enumeration<ClaimStatus> getStatusElement() { 7069 if (this.status == null) 7070 if (Configuration.errorOnAutoCreate()) 7071 throw new Error("Attempt to auto-create Claim.status"); 7072 else if (Configuration.doAutoCreate()) 7073 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); // bb 7074 return this.status; 7075 } 7076 7077 public boolean hasStatusElement() { 7078 return this.status != null && !this.status.isEmpty(); 7079 } 7080 7081 public boolean hasStatus() { 7082 return this.status != null && !this.status.isEmpty(); 7083 } 7084 7085 /** 7086 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 7087 */ 7088 public Claim setStatusElement(Enumeration<ClaimStatus> value) { 7089 this.status = value; 7090 return this; 7091 } 7092 7093 /** 7094 * @return The status of the resource instance. 7095 */ 7096 public ClaimStatus getStatus() { 7097 return this.status == null ? null : this.status.getValue(); 7098 } 7099 7100 /** 7101 * @param value The status of the resource instance. 7102 */ 7103 public Claim setStatus(ClaimStatus value) { 7104 if (value == null) 7105 this.status = null; 7106 else { 7107 if (this.status == null) 7108 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); 7109 this.status.setValue(value); 7110 } 7111 return this; 7112 } 7113 7114 /** 7115 * @return {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 7116 */ 7117 public CodeableConcept getType() { 7118 if (this.type == null) 7119 if (Configuration.errorOnAutoCreate()) 7120 throw new Error("Attempt to auto-create Claim.type"); 7121 else if (Configuration.doAutoCreate()) 7122 this.type = new CodeableConcept(); // cc 7123 return this.type; 7124 } 7125 7126 public boolean hasType() { 7127 return this.type != null && !this.type.isEmpty(); 7128 } 7129 7130 /** 7131 * @param value {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 7132 */ 7133 public Claim setType(CodeableConcept value) { 7134 this.type = value; 7135 return this; 7136 } 7137 7138 /** 7139 * @return {@link #subType} (A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.) 7140 */ 7141 public List<CodeableConcept> getSubType() { 7142 if (this.subType == null) 7143 this.subType = new ArrayList<CodeableConcept>(); 7144 return this.subType; 7145 } 7146 7147 /** 7148 * @return Returns a reference to <code>this</code> for easy method chaining 7149 */ 7150 public Claim setSubType(List<CodeableConcept> theSubType) { 7151 this.subType = theSubType; 7152 return this; 7153 } 7154 7155 public boolean hasSubType() { 7156 if (this.subType == null) 7157 return false; 7158 for (CodeableConcept item : this.subType) 7159 if (!item.isEmpty()) 7160 return true; 7161 return false; 7162 } 7163 7164 public CodeableConcept addSubType() { //3 7165 CodeableConcept t = new CodeableConcept(); 7166 if (this.subType == null) 7167 this.subType = new ArrayList<CodeableConcept>(); 7168 this.subType.add(t); 7169 return t; 7170 } 7171 7172 public Claim addSubType(CodeableConcept t) { //3 7173 if (t == null) 7174 return this; 7175 if (this.subType == null) 7176 this.subType = new ArrayList<CodeableConcept>(); 7177 this.subType.add(t); 7178 return this; 7179 } 7180 7181 /** 7182 * @return The first repetition of repeating field {@link #subType}, creating it if it does not already exist 7183 */ 7184 public CodeableConcept getSubTypeFirstRep() { 7185 if (getSubType().isEmpty()) { 7186 addSubType(); 7187 } 7188 return getSubType().get(0); 7189 } 7190 7191 /** 7192 * @return {@link #use} (Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 7193 */ 7194 public Enumeration<Use> getUseElement() { 7195 if (this.use == null) 7196 if (Configuration.errorOnAutoCreate()) 7197 throw new Error("Attempt to auto-create Claim.use"); 7198 else if (Configuration.doAutoCreate()) 7199 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 7200 return this.use; 7201 } 7202 7203 public boolean hasUseElement() { 7204 return this.use != null && !this.use.isEmpty(); 7205 } 7206 7207 public boolean hasUse() { 7208 return this.use != null && !this.use.isEmpty(); 7209 } 7210 7211 /** 7212 * @param value {@link #use} (Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 7213 */ 7214 public Claim setUseElement(Enumeration<Use> value) { 7215 this.use = value; 7216 return this; 7217 } 7218 7219 /** 7220 * @return Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination). 7221 */ 7222 public Use getUse() { 7223 return this.use == null ? null : this.use.getValue(); 7224 } 7225 7226 /** 7227 * @param value Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination). 7228 */ 7229 public Claim setUse(Use value) { 7230 if (value == null) 7231 this.use = null; 7232 else { 7233 if (this.use == null) 7234 this.use = new Enumeration<Use>(new UseEnumFactory()); 7235 this.use.setValue(value); 7236 } 7237 return this; 7238 } 7239 7240 /** 7241 * @return {@link #patient} (Patient Resource.) 7242 */ 7243 public Reference getPatient() { 7244 if (this.patient == null) 7245 if (Configuration.errorOnAutoCreate()) 7246 throw new Error("Attempt to auto-create Claim.patient"); 7247 else if (Configuration.doAutoCreate()) 7248 this.patient = new Reference(); // cc 7249 return this.patient; 7250 } 7251 7252 public boolean hasPatient() { 7253 return this.patient != null && !this.patient.isEmpty(); 7254 } 7255 7256 /** 7257 * @param value {@link #patient} (Patient Resource.) 7258 */ 7259 public Claim setPatient(Reference value) { 7260 this.patient = value; 7261 return this; 7262 } 7263 7264 /** 7265 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient Resource.) 7266 */ 7267 public Patient getPatientTarget() { 7268 if (this.patientTarget == null) 7269 if (Configuration.errorOnAutoCreate()) 7270 throw new Error("Attempt to auto-create Claim.patient"); 7271 else if (Configuration.doAutoCreate()) 7272 this.patientTarget = new Patient(); // aa 7273 return this.patientTarget; 7274 } 7275 7276 /** 7277 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient Resource.) 7278 */ 7279 public Claim setPatientTarget(Patient value) { 7280 this.patientTarget = value; 7281 return this; 7282 } 7283 7284 /** 7285 * @return {@link #billablePeriod} (The billable period for which charges are being submitted.) 7286 */ 7287 public Period getBillablePeriod() { 7288 if (this.billablePeriod == null) 7289 if (Configuration.errorOnAutoCreate()) 7290 throw new Error("Attempt to auto-create Claim.billablePeriod"); 7291 else if (Configuration.doAutoCreate()) 7292 this.billablePeriod = new Period(); // cc 7293 return this.billablePeriod; 7294 } 7295 7296 public boolean hasBillablePeriod() { 7297 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 7298 } 7299 7300 /** 7301 * @param value {@link #billablePeriod} (The billable period for which charges are being submitted.) 7302 */ 7303 public Claim setBillablePeriod(Period value) { 7304 this.billablePeriod = value; 7305 return this; 7306 } 7307 7308 /** 7309 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 7310 */ 7311 public DateTimeType getCreatedElement() { 7312 if (this.created == null) 7313 if (Configuration.errorOnAutoCreate()) 7314 throw new Error("Attempt to auto-create Claim.created"); 7315 else if (Configuration.doAutoCreate()) 7316 this.created = new DateTimeType(); // bb 7317 return this.created; 7318 } 7319 7320 public boolean hasCreatedElement() { 7321 return this.created != null && !this.created.isEmpty(); 7322 } 7323 7324 public boolean hasCreated() { 7325 return this.created != null && !this.created.isEmpty(); 7326 } 7327 7328 /** 7329 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 7330 */ 7331 public Claim setCreatedElement(DateTimeType value) { 7332 this.created = value; 7333 return this; 7334 } 7335 7336 /** 7337 * @return The date when the enclosed suite of services were performed or completed. 7338 */ 7339 public Date getCreated() { 7340 return this.created == null ? null : this.created.getValue(); 7341 } 7342 7343 /** 7344 * @param value The date when the enclosed suite of services were performed or completed. 7345 */ 7346 public Claim setCreated(Date value) { 7347 if (value == null) 7348 this.created = null; 7349 else { 7350 if (this.created == null) 7351 this.created = new DateTimeType(); 7352 this.created.setValue(value); 7353 } 7354 return this; 7355 } 7356 7357 /** 7358 * @return {@link #enterer} (Person who created the invoice/claim/pre-determination or pre-authorization.) 7359 */ 7360 public Reference getEnterer() { 7361 if (this.enterer == null) 7362 if (Configuration.errorOnAutoCreate()) 7363 throw new Error("Attempt to auto-create Claim.enterer"); 7364 else if (Configuration.doAutoCreate()) 7365 this.enterer = new Reference(); // cc 7366 return this.enterer; 7367 } 7368 7369 public boolean hasEnterer() { 7370 return this.enterer != null && !this.enterer.isEmpty(); 7371 } 7372 7373 /** 7374 * @param value {@link #enterer} (Person who created the invoice/claim/pre-determination or pre-authorization.) 7375 */ 7376 public Claim setEnterer(Reference value) { 7377 this.enterer = value; 7378 return this; 7379 } 7380 7381 /** 7382 * @return {@link #enterer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Person who created the invoice/claim/pre-determination or pre-authorization.) 7383 */ 7384 public Practitioner getEntererTarget() { 7385 if (this.entererTarget == null) 7386 if (Configuration.errorOnAutoCreate()) 7387 throw new Error("Attempt to auto-create Claim.enterer"); 7388 else if (Configuration.doAutoCreate()) 7389 this.entererTarget = new Practitioner(); // aa 7390 return this.entererTarget; 7391 } 7392 7393 /** 7394 * @param value {@link #enterer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Person who created the invoice/claim/pre-determination or pre-authorization.) 7395 */ 7396 public Claim setEntererTarget(Practitioner value) { 7397 this.entererTarget = value; 7398 return this; 7399 } 7400 7401 /** 7402 * @return {@link #insurer} (The Insurer who is target of the request.) 7403 */ 7404 public Reference getInsurer() { 7405 if (this.insurer == null) 7406 if (Configuration.errorOnAutoCreate()) 7407 throw new Error("Attempt to auto-create Claim.insurer"); 7408 else if (Configuration.doAutoCreate()) 7409 this.insurer = new Reference(); // cc 7410 return this.insurer; 7411 } 7412 7413 public boolean hasInsurer() { 7414 return this.insurer != null && !this.insurer.isEmpty(); 7415 } 7416 7417 /** 7418 * @param value {@link #insurer} (The Insurer who is target of the request.) 7419 */ 7420 public Claim setInsurer(Reference value) { 7421 this.insurer = value; 7422 return this; 7423 } 7424 7425 /** 7426 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 7427 */ 7428 public Organization getInsurerTarget() { 7429 if (this.insurerTarget == null) 7430 if (Configuration.errorOnAutoCreate()) 7431 throw new Error("Attempt to auto-create Claim.insurer"); 7432 else if (Configuration.doAutoCreate()) 7433 this.insurerTarget = new Organization(); // aa 7434 return this.insurerTarget; 7435 } 7436 7437 /** 7438 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 7439 */ 7440 public Claim setInsurerTarget(Organization value) { 7441 this.insurerTarget = value; 7442 return this; 7443 } 7444 7445 /** 7446 * @return {@link #provider} (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7447 */ 7448 public Reference getProvider() { 7449 if (this.provider == null) 7450 if (Configuration.errorOnAutoCreate()) 7451 throw new Error("Attempt to auto-create Claim.provider"); 7452 else if (Configuration.doAutoCreate()) 7453 this.provider = new Reference(); // cc 7454 return this.provider; 7455 } 7456 7457 public boolean hasProvider() { 7458 return this.provider != null && !this.provider.isEmpty(); 7459 } 7460 7461 /** 7462 * @param value {@link #provider} (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7463 */ 7464 public Claim setProvider(Reference value) { 7465 this.provider = value; 7466 return this; 7467 } 7468 7469 /** 7470 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7471 */ 7472 public Practitioner getProviderTarget() { 7473 if (this.providerTarget == null) 7474 if (Configuration.errorOnAutoCreate()) 7475 throw new Error("Attempt to auto-create Claim.provider"); 7476 else if (Configuration.doAutoCreate()) 7477 this.providerTarget = new Practitioner(); // aa 7478 return this.providerTarget; 7479 } 7480 7481 /** 7482 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7483 */ 7484 public Claim setProviderTarget(Practitioner value) { 7485 this.providerTarget = value; 7486 return this; 7487 } 7488 7489 /** 7490 * @return {@link #organization} (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7491 */ 7492 public Reference getOrganization() { 7493 if (this.organization == null) 7494 if (Configuration.errorOnAutoCreate()) 7495 throw new Error("Attempt to auto-create Claim.organization"); 7496 else if (Configuration.doAutoCreate()) 7497 this.organization = new Reference(); // cc 7498 return this.organization; 7499 } 7500 7501 public boolean hasOrganization() { 7502 return this.organization != null && !this.organization.isEmpty(); 7503 } 7504 7505 /** 7506 * @param value {@link #organization} (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7507 */ 7508 public Claim setOrganization(Reference value) { 7509 this.organization = value; 7510 return this; 7511 } 7512 7513 /** 7514 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7515 */ 7516 public Organization getOrganizationTarget() { 7517 if (this.organizationTarget == null) 7518 if (Configuration.errorOnAutoCreate()) 7519 throw new Error("Attempt to auto-create Claim.organization"); 7520 else if (Configuration.doAutoCreate()) 7521 this.organizationTarget = new Organization(); // aa 7522 return this.organizationTarget; 7523 } 7524 7525 /** 7526 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7527 */ 7528 public Claim setOrganizationTarget(Organization value) { 7529 this.organizationTarget = value; 7530 return this; 7531 } 7532 7533 /** 7534 * @return {@link #priority} (Immediate (STAT), best effort (NORMAL), deferred (DEFER).) 7535 */ 7536 public CodeableConcept getPriority() { 7537 if (this.priority == null) 7538 if (Configuration.errorOnAutoCreate()) 7539 throw new Error("Attempt to auto-create Claim.priority"); 7540 else if (Configuration.doAutoCreate()) 7541 this.priority = new CodeableConcept(); // cc 7542 return this.priority; 7543 } 7544 7545 public boolean hasPriority() { 7546 return this.priority != null && !this.priority.isEmpty(); 7547 } 7548 7549 /** 7550 * @param value {@link #priority} (Immediate (STAT), best effort (NORMAL), deferred (DEFER).) 7551 */ 7552 public Claim setPriority(CodeableConcept value) { 7553 this.priority = value; 7554 return this; 7555 } 7556 7557 /** 7558 * @return {@link #fundsReserve} (In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.) 7559 */ 7560 public CodeableConcept getFundsReserve() { 7561 if (this.fundsReserve == null) 7562 if (Configuration.errorOnAutoCreate()) 7563 throw new Error("Attempt to auto-create Claim.fundsReserve"); 7564 else if (Configuration.doAutoCreate()) 7565 this.fundsReserve = new CodeableConcept(); // cc 7566 return this.fundsReserve; 7567 } 7568 7569 public boolean hasFundsReserve() { 7570 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 7571 } 7572 7573 /** 7574 * @param value {@link #fundsReserve} (In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.) 7575 */ 7576 public Claim setFundsReserve(CodeableConcept value) { 7577 this.fundsReserve = value; 7578 return this; 7579 } 7580 7581 /** 7582 * @return {@link #related} (Other claims which are related to this claim such as prior claim versions or for related services.) 7583 */ 7584 public List<RelatedClaimComponent> getRelated() { 7585 if (this.related == null) 7586 this.related = new ArrayList<RelatedClaimComponent>(); 7587 return this.related; 7588 } 7589 7590 /** 7591 * @return Returns a reference to <code>this</code> for easy method chaining 7592 */ 7593 public Claim setRelated(List<RelatedClaimComponent> theRelated) { 7594 this.related = theRelated; 7595 return this; 7596 } 7597 7598 public boolean hasRelated() { 7599 if (this.related == null) 7600 return false; 7601 for (RelatedClaimComponent item : this.related) 7602 if (!item.isEmpty()) 7603 return true; 7604 return false; 7605 } 7606 7607 public RelatedClaimComponent addRelated() { //3 7608 RelatedClaimComponent t = new RelatedClaimComponent(); 7609 if (this.related == null) 7610 this.related = new ArrayList<RelatedClaimComponent>(); 7611 this.related.add(t); 7612 return t; 7613 } 7614 7615 public Claim addRelated(RelatedClaimComponent t) { //3 7616 if (t == null) 7617 return this; 7618 if (this.related == null) 7619 this.related = new ArrayList<RelatedClaimComponent>(); 7620 this.related.add(t); 7621 return this; 7622 } 7623 7624 /** 7625 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist 7626 */ 7627 public RelatedClaimComponent getRelatedFirstRep() { 7628 if (getRelated().isEmpty()) { 7629 addRelated(); 7630 } 7631 return getRelated().get(0); 7632 } 7633 7634 /** 7635 * @return {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 7636 */ 7637 public Reference getPrescription() { 7638 if (this.prescription == null) 7639 if (Configuration.errorOnAutoCreate()) 7640 throw new Error("Attempt to auto-create Claim.prescription"); 7641 else if (Configuration.doAutoCreate()) 7642 this.prescription = new Reference(); // cc 7643 return this.prescription; 7644 } 7645 7646 public boolean hasPrescription() { 7647 return this.prescription != null && !this.prescription.isEmpty(); 7648 } 7649 7650 /** 7651 * @param value {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 7652 */ 7653 public Claim setPrescription(Reference value) { 7654 this.prescription = value; 7655 return this; 7656 } 7657 7658 /** 7659 * @return {@link #prescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 7660 */ 7661 public Resource getPrescriptionTarget() { 7662 return this.prescriptionTarget; 7663 } 7664 7665 /** 7666 * @param value {@link #prescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 7667 */ 7668 public Claim setPrescriptionTarget(Resource value) { 7669 this.prescriptionTarget = value; 7670 return this; 7671 } 7672 7673 /** 7674 * @return {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7675 */ 7676 public Reference getOriginalPrescription() { 7677 if (this.originalPrescription == null) 7678 if (Configuration.errorOnAutoCreate()) 7679 throw new Error("Attempt to auto-create Claim.originalPrescription"); 7680 else if (Configuration.doAutoCreate()) 7681 this.originalPrescription = new Reference(); // cc 7682 return this.originalPrescription; 7683 } 7684 7685 public boolean hasOriginalPrescription() { 7686 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 7687 } 7688 7689 /** 7690 * @param value {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7691 */ 7692 public Claim setOriginalPrescription(Reference value) { 7693 this.originalPrescription = value; 7694 return this; 7695 } 7696 7697 /** 7698 * @return {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7699 */ 7700 public MedicationRequest getOriginalPrescriptionTarget() { 7701 if (this.originalPrescriptionTarget == null) 7702 if (Configuration.errorOnAutoCreate()) 7703 throw new Error("Attempt to auto-create Claim.originalPrescription"); 7704 else if (Configuration.doAutoCreate()) 7705 this.originalPrescriptionTarget = new MedicationRequest(); // aa 7706 return this.originalPrescriptionTarget; 7707 } 7708 7709 /** 7710 * @param value {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7711 */ 7712 public Claim setOriginalPrescriptionTarget(MedicationRequest value) { 7713 this.originalPrescriptionTarget = value; 7714 return this; 7715 } 7716 7717 /** 7718 * @return {@link #payee} (The party to be reimbursed for the services.) 7719 */ 7720 public PayeeComponent getPayee() { 7721 if (this.payee == null) 7722 if (Configuration.errorOnAutoCreate()) 7723 throw new Error("Attempt to auto-create Claim.payee"); 7724 else if (Configuration.doAutoCreate()) 7725 this.payee = new PayeeComponent(); // cc 7726 return this.payee; 7727 } 7728 7729 public boolean hasPayee() { 7730 return this.payee != null && !this.payee.isEmpty(); 7731 } 7732 7733 /** 7734 * @param value {@link #payee} (The party to be reimbursed for the services.) 7735 */ 7736 public Claim setPayee(PayeeComponent value) { 7737 this.payee = value; 7738 return this; 7739 } 7740 7741 /** 7742 * @return {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 7743 */ 7744 public Reference getReferral() { 7745 if (this.referral == null) 7746 if (Configuration.errorOnAutoCreate()) 7747 throw new Error("Attempt to auto-create Claim.referral"); 7748 else if (Configuration.doAutoCreate()) 7749 this.referral = new Reference(); // cc 7750 return this.referral; 7751 } 7752 7753 public boolean hasReferral() { 7754 return this.referral != null && !this.referral.isEmpty(); 7755 } 7756 7757 /** 7758 * @param value {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 7759 */ 7760 public Claim setReferral(Reference value) { 7761 this.referral = value; 7762 return this; 7763 } 7764 7765 /** 7766 * @return {@link #referral} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 7767 */ 7768 public ReferralRequest getReferralTarget() { 7769 if (this.referralTarget == null) 7770 if (Configuration.errorOnAutoCreate()) 7771 throw new Error("Attempt to auto-create Claim.referral"); 7772 else if (Configuration.doAutoCreate()) 7773 this.referralTarget = new ReferralRequest(); // aa 7774 return this.referralTarget; 7775 } 7776 7777 /** 7778 * @param value {@link #referral} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 7779 */ 7780 public Claim setReferralTarget(ReferralRequest value) { 7781 this.referralTarget = value; 7782 return this; 7783 } 7784 7785 /** 7786 * @return {@link #facility} (Facility where the services were provided.) 7787 */ 7788 public Reference getFacility() { 7789 if (this.facility == null) 7790 if (Configuration.errorOnAutoCreate()) 7791 throw new Error("Attempt to auto-create Claim.facility"); 7792 else if (Configuration.doAutoCreate()) 7793 this.facility = new Reference(); // cc 7794 return this.facility; 7795 } 7796 7797 public boolean hasFacility() { 7798 return this.facility != null && !this.facility.isEmpty(); 7799 } 7800 7801 /** 7802 * @param value {@link #facility} (Facility where the services were provided.) 7803 */ 7804 public Claim setFacility(Reference value) { 7805 this.facility = value; 7806 return this; 7807 } 7808 7809 /** 7810 * @return {@link #facility} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 7811 */ 7812 public Location getFacilityTarget() { 7813 if (this.facilityTarget == null) 7814 if (Configuration.errorOnAutoCreate()) 7815 throw new Error("Attempt to auto-create Claim.facility"); 7816 else if (Configuration.doAutoCreate()) 7817 this.facilityTarget = new Location(); // aa 7818 return this.facilityTarget; 7819 } 7820 7821 /** 7822 * @param value {@link #facility} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 7823 */ 7824 public Claim setFacilityTarget(Location value) { 7825 this.facilityTarget = value; 7826 return this; 7827 } 7828 7829 /** 7830 * @return {@link #careTeam} (The members of the team who provided the overall service as well as their role and whether responsible and qualifications.) 7831 */ 7832 public List<CareTeamComponent> getCareTeam() { 7833 if (this.careTeam == null) 7834 this.careTeam = new ArrayList<CareTeamComponent>(); 7835 return this.careTeam; 7836 } 7837 7838 /** 7839 * @return Returns a reference to <code>this</code> for easy method chaining 7840 */ 7841 public Claim setCareTeam(List<CareTeamComponent> theCareTeam) { 7842 this.careTeam = theCareTeam; 7843 return this; 7844 } 7845 7846 public boolean hasCareTeam() { 7847 if (this.careTeam == null) 7848 return false; 7849 for (CareTeamComponent item : this.careTeam) 7850 if (!item.isEmpty()) 7851 return true; 7852 return false; 7853 } 7854 7855 public CareTeamComponent addCareTeam() { //3 7856 CareTeamComponent t = new CareTeamComponent(); 7857 if (this.careTeam == null) 7858 this.careTeam = new ArrayList<CareTeamComponent>(); 7859 this.careTeam.add(t); 7860 return t; 7861 } 7862 7863 public Claim addCareTeam(CareTeamComponent t) { //3 7864 if (t == null) 7865 return this; 7866 if (this.careTeam == null) 7867 this.careTeam = new ArrayList<CareTeamComponent>(); 7868 this.careTeam.add(t); 7869 return this; 7870 } 7871 7872 /** 7873 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist 7874 */ 7875 public CareTeamComponent getCareTeamFirstRep() { 7876 if (getCareTeam().isEmpty()) { 7877 addCareTeam(); 7878 } 7879 return getCareTeam().get(0); 7880 } 7881 7882 /** 7883 * @return {@link #information} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.) 7884 */ 7885 public List<SpecialConditionComponent> getInformation() { 7886 if (this.information == null) 7887 this.information = new ArrayList<SpecialConditionComponent>(); 7888 return this.information; 7889 } 7890 7891 /** 7892 * @return Returns a reference to <code>this</code> for easy method chaining 7893 */ 7894 public Claim setInformation(List<SpecialConditionComponent> theInformation) { 7895 this.information = theInformation; 7896 return this; 7897 } 7898 7899 public boolean hasInformation() { 7900 if (this.information == null) 7901 return false; 7902 for (SpecialConditionComponent item : this.information) 7903 if (!item.isEmpty()) 7904 return true; 7905 return false; 7906 } 7907 7908 public SpecialConditionComponent addInformation() { //3 7909 SpecialConditionComponent t = new SpecialConditionComponent(); 7910 if (this.information == null) 7911 this.information = new ArrayList<SpecialConditionComponent>(); 7912 this.information.add(t); 7913 return t; 7914 } 7915 7916 public Claim addInformation(SpecialConditionComponent t) { //3 7917 if (t == null) 7918 return this; 7919 if (this.information == null) 7920 this.information = new ArrayList<SpecialConditionComponent>(); 7921 this.information.add(t); 7922 return this; 7923 } 7924 7925 /** 7926 * @return The first repetition of repeating field {@link #information}, creating it if it does not already exist 7927 */ 7928 public SpecialConditionComponent getInformationFirstRep() { 7929 if (getInformation().isEmpty()) { 7930 addInformation(); 7931 } 7932 return getInformation().get(0); 7933 } 7934 7935 /** 7936 * @return {@link #diagnosis} (List of patient diagnosis for which care is sought.) 7937 */ 7938 public List<DiagnosisComponent> getDiagnosis() { 7939 if (this.diagnosis == null) 7940 this.diagnosis = new ArrayList<DiagnosisComponent>(); 7941 return this.diagnosis; 7942 } 7943 7944 /** 7945 * @return Returns a reference to <code>this</code> for easy method chaining 7946 */ 7947 public Claim setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 7948 this.diagnosis = theDiagnosis; 7949 return this; 7950 } 7951 7952 public boolean hasDiagnosis() { 7953 if (this.diagnosis == null) 7954 return false; 7955 for (DiagnosisComponent item : this.diagnosis) 7956 if (!item.isEmpty()) 7957 return true; 7958 return false; 7959 } 7960 7961 public DiagnosisComponent addDiagnosis() { //3 7962 DiagnosisComponent t = new DiagnosisComponent(); 7963 if (this.diagnosis == null) 7964 this.diagnosis = new ArrayList<DiagnosisComponent>(); 7965 this.diagnosis.add(t); 7966 return t; 7967 } 7968 7969 public Claim addDiagnosis(DiagnosisComponent t) { //3 7970 if (t == null) 7971 return this; 7972 if (this.diagnosis == null) 7973 this.diagnosis = new ArrayList<DiagnosisComponent>(); 7974 this.diagnosis.add(t); 7975 return this; 7976 } 7977 7978 /** 7979 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist 7980 */ 7981 public DiagnosisComponent getDiagnosisFirstRep() { 7982 if (getDiagnosis().isEmpty()) { 7983 addDiagnosis(); 7984 } 7985 return getDiagnosis().get(0); 7986 } 7987 7988 /** 7989 * @return {@link #procedure} (Ordered list of patient procedures performed to support the adjudication.) 7990 */ 7991 public List<ProcedureComponent> getProcedure() { 7992 if (this.procedure == null) 7993 this.procedure = new ArrayList<ProcedureComponent>(); 7994 return this.procedure; 7995 } 7996 7997 /** 7998 * @return Returns a reference to <code>this</code> for easy method chaining 7999 */ 8000 public Claim setProcedure(List<ProcedureComponent> theProcedure) { 8001 this.procedure = theProcedure; 8002 return this; 8003 } 8004 8005 public boolean hasProcedure() { 8006 if (this.procedure == null) 8007 return false; 8008 for (ProcedureComponent item : this.procedure) 8009 if (!item.isEmpty()) 8010 return true; 8011 return false; 8012 } 8013 8014 public ProcedureComponent addProcedure() { //3 8015 ProcedureComponent t = new ProcedureComponent(); 8016 if (this.procedure == null) 8017 this.procedure = new ArrayList<ProcedureComponent>(); 8018 this.procedure.add(t); 8019 return t; 8020 } 8021 8022 public Claim addProcedure(ProcedureComponent t) { //3 8023 if (t == null) 8024 return this; 8025 if (this.procedure == null) 8026 this.procedure = new ArrayList<ProcedureComponent>(); 8027 this.procedure.add(t); 8028 return this; 8029 } 8030 8031 /** 8032 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist 8033 */ 8034 public ProcedureComponent getProcedureFirstRep() { 8035 if (getProcedure().isEmpty()) { 8036 addProcedure(); 8037 } 8038 return getProcedure().get(0); 8039 } 8040 8041 /** 8042 * @return {@link #insurance} (Financial instrument by which payment information for health care.) 8043 */ 8044 public List<InsuranceComponent> getInsurance() { 8045 if (this.insurance == null) 8046 this.insurance = new ArrayList<InsuranceComponent>(); 8047 return this.insurance; 8048 } 8049 8050 /** 8051 * @return Returns a reference to <code>this</code> for easy method chaining 8052 */ 8053 public Claim setInsurance(List<InsuranceComponent> theInsurance) { 8054 this.insurance = theInsurance; 8055 return this; 8056 } 8057 8058 public boolean hasInsurance() { 8059 if (this.insurance == null) 8060 return false; 8061 for (InsuranceComponent item : this.insurance) 8062 if (!item.isEmpty()) 8063 return true; 8064 return false; 8065 } 8066 8067 public InsuranceComponent addInsurance() { //3 8068 InsuranceComponent t = new InsuranceComponent(); 8069 if (this.insurance == null) 8070 this.insurance = new ArrayList<InsuranceComponent>(); 8071 this.insurance.add(t); 8072 return t; 8073 } 8074 8075 public Claim addInsurance(InsuranceComponent t) { //3 8076 if (t == null) 8077 return this; 8078 if (this.insurance == null) 8079 this.insurance = new ArrayList<InsuranceComponent>(); 8080 this.insurance.add(t); 8081 return this; 8082 } 8083 8084 /** 8085 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist 8086 */ 8087 public InsuranceComponent getInsuranceFirstRep() { 8088 if (getInsurance().isEmpty()) { 8089 addInsurance(); 8090 } 8091 return getInsurance().get(0); 8092 } 8093 8094 /** 8095 * @return {@link #accident} (An accident which resulted in the need for healthcare services.) 8096 */ 8097 public AccidentComponent getAccident() { 8098 if (this.accident == null) 8099 if (Configuration.errorOnAutoCreate()) 8100 throw new Error("Attempt to auto-create Claim.accident"); 8101 else if (Configuration.doAutoCreate()) 8102 this.accident = new AccidentComponent(); // cc 8103 return this.accident; 8104 } 8105 8106 public boolean hasAccident() { 8107 return this.accident != null && !this.accident.isEmpty(); 8108 } 8109 8110 /** 8111 * @param value {@link #accident} (An accident which resulted in the need for healthcare services.) 8112 */ 8113 public Claim setAccident(AccidentComponent value) { 8114 this.accident = value; 8115 return this; 8116 } 8117 8118 /** 8119 * @return {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 8120 */ 8121 public Period getEmploymentImpacted() { 8122 if (this.employmentImpacted == null) 8123 if (Configuration.errorOnAutoCreate()) 8124 throw new Error("Attempt to auto-create Claim.employmentImpacted"); 8125 else if (Configuration.doAutoCreate()) 8126 this.employmentImpacted = new Period(); // cc 8127 return this.employmentImpacted; 8128 } 8129 8130 public boolean hasEmploymentImpacted() { 8131 return this.employmentImpacted != null && !this.employmentImpacted.isEmpty(); 8132 } 8133 8134 /** 8135 * @param value {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 8136 */ 8137 public Claim setEmploymentImpacted(Period value) { 8138 this.employmentImpacted = value; 8139 return this; 8140 } 8141 8142 /** 8143 * @return {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 8144 */ 8145 public Period getHospitalization() { 8146 if (this.hospitalization == null) 8147 if (Configuration.errorOnAutoCreate()) 8148 throw new Error("Attempt to auto-create Claim.hospitalization"); 8149 else if (Configuration.doAutoCreate()) 8150 this.hospitalization = new Period(); // cc 8151 return this.hospitalization; 8152 } 8153 8154 public boolean hasHospitalization() { 8155 return this.hospitalization != null && !this.hospitalization.isEmpty(); 8156 } 8157 8158 /** 8159 * @param value {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 8160 */ 8161 public Claim setHospitalization(Period value) { 8162 this.hospitalization = value; 8163 return this; 8164 } 8165 8166 /** 8167 * @return {@link #item} (First tier of goods and services.) 8168 */ 8169 public List<ItemComponent> getItem() { 8170 if (this.item == null) 8171 this.item = new ArrayList<ItemComponent>(); 8172 return this.item; 8173 } 8174 8175 /** 8176 * @return Returns a reference to <code>this</code> for easy method chaining 8177 */ 8178 public Claim setItem(List<ItemComponent> theItem) { 8179 this.item = theItem; 8180 return this; 8181 } 8182 8183 public boolean hasItem() { 8184 if (this.item == null) 8185 return false; 8186 for (ItemComponent item : this.item) 8187 if (!item.isEmpty()) 8188 return true; 8189 return false; 8190 } 8191 8192 public ItemComponent addItem() { //3 8193 ItemComponent t = new ItemComponent(); 8194 if (this.item == null) 8195 this.item = new ArrayList<ItemComponent>(); 8196 this.item.add(t); 8197 return t; 8198 } 8199 8200 public Claim addItem(ItemComponent t) { //3 8201 if (t == null) 8202 return this; 8203 if (this.item == null) 8204 this.item = new ArrayList<ItemComponent>(); 8205 this.item.add(t); 8206 return this; 8207 } 8208 8209 /** 8210 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 8211 */ 8212 public ItemComponent getItemFirstRep() { 8213 if (getItem().isEmpty()) { 8214 addItem(); 8215 } 8216 return getItem().get(0); 8217 } 8218 8219 /** 8220 * @return {@link #total} (The total value of the claim.) 8221 */ 8222 public Money getTotal() { 8223 if (this.total == null) 8224 if (Configuration.errorOnAutoCreate()) 8225 throw new Error("Attempt to auto-create Claim.total"); 8226 else if (Configuration.doAutoCreate()) 8227 this.total = new Money(); // cc 8228 return this.total; 8229 } 8230 8231 public boolean hasTotal() { 8232 return this.total != null && !this.total.isEmpty(); 8233 } 8234 8235 /** 8236 * @param value {@link #total} (The total value of the claim.) 8237 */ 8238 public Claim setTotal(Money value) { 8239 this.total = value; 8240 return this; 8241 } 8242 8243 protected void listChildren(List<Property> children) { 8244 super.listChildren(children); 8245 children.add(new Property("identifier", "Identifier", "The business identifier for the instance: claim number, pre-determination or pre-authorization number.", 0, java.lang.Integer.MAX_VALUE, identifier)); 8246 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 8247 children.add(new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type)); 8248 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType)); 8249 children.add(new Property("use", "code", "Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).", 0, 1, use)); 8250 children.add(new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient)); 8251 children.add(new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod)); 8252 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 8253 children.add(new Property("enterer", "Reference(Practitioner)", "Person who created the invoice/claim/pre-determination or pre-authorization.", 0, 1, enterer)); 8254 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 8255 children.add(new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, provider)); 8256 children.add(new Property("organization", "Reference(Organization)", "The organization which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, organization)); 8257 children.add(new Property("priority", "CodeableConcept", "Immediate (STAT), best effort (NORMAL), deferred (DEFER).", 0, 1, priority)); 8258 children.add(new Property("fundsReserve", "CodeableConcept", "In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.", 0, 1, fundsReserve)); 8259 children.add(new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related)); 8260 children.add(new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription)); 8261 children.add(new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription)); 8262 children.add(new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee)); 8263 children.add(new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral)); 8264 children.add(new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 8265 children.add(new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 8266 children.add(new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information)); 8267 children.add(new Property("diagnosis", "", "List of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 8268 children.add(new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure)); 8269 children.add(new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, java.lang.Integer.MAX_VALUE, insurance)); 8270 children.add(new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident)); 8271 children.add(new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted)); 8272 children.add(new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization)); 8273 children.add(new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item)); 8274 children.add(new Property("total", "Money", "The total value of the claim.", 0, 1, total)); 8275 } 8276 8277 @Override 8278 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8279 switch (_hash) { 8280 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The business identifier for the instance: claim number, pre-determination or pre-authorization number.", 0, java.lang.Integer.MAX_VALUE, identifier); 8281 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 8282 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type); 8283 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType); 8284 case 116103: /*use*/ return new Property("use", "code", "Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).", 0, 1, use); 8285 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient); 8286 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod); 8287 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 8288 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner)", "Person who created the invoice/claim/pre-determination or pre-authorization.", 0, 1, enterer); 8289 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer); 8290 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, provider); 8291 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, organization); 8292 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Immediate (STAT), best effort (NORMAL), deferred (DEFER).", 0, 1, priority); 8293 case 1314609806: /*fundsReserve*/ return new Property("fundsReserve", "CodeableConcept", "In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.", 0, 1, fundsReserve); 8294 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related); 8295 case 460301338: /*prescription*/ return new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription); 8296 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription); 8297 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee); 8298 case -722568291: /*referral*/ return new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral); 8299 case 501116579: /*facility*/ return new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility); 8300 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam); 8301 case 1968600364: /*information*/ return new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information); 8302 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "List of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 8303 case -1095204141: /*procedure*/ return new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure); 8304 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, java.lang.Integer.MAX_VALUE, insurance); 8305 case -2143202801: /*accident*/ return new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident); 8306 case 1051487345: /*employmentImpacted*/ return new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted); 8307 case 1057894634: /*hospitalization*/ return new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization); 8308 case 3242771: /*item*/ return new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item); 8309 case 110549828: /*total*/ return new Property("total", "Money", "The total value of the claim.", 0, 1, total); 8310 default: return super.getNamedProperty(_hash, _name, _checkValid); 8311 } 8312 8313 } 8314 8315 @Override 8316 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8317 switch (hash) { 8318 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8319 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ClaimStatus> 8320 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 8321 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 8322 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<Use> 8323 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 8324 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 8325 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 8326 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 8327 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 8328 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 8329 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 8330 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 8331 case 1314609806: /*fundsReserve*/ return this.fundsReserve == null ? new Base[0] : new Base[] {this.fundsReserve}; // CodeableConcept 8332 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 8333 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 8334 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 8335 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 8336 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 8337 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 8338 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 8339 case 1968600364: /*information*/ return this.information == null ? new Base[0] : this.information.toArray(new Base[this.information.size()]); // SpecialConditionComponent 8340 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 8341 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 8342 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 8343 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 8344 case 1051487345: /*employmentImpacted*/ return this.employmentImpacted == null ? new Base[0] : new Base[] {this.employmentImpacted}; // Period 8345 case 1057894634: /*hospitalization*/ return this.hospitalization == null ? new Base[0] : new Base[] {this.hospitalization}; // Period 8346 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 8347 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // Money 8348 default: return super.getProperty(hash, name, checkValid); 8349 } 8350 8351 } 8352 8353 @Override 8354 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8355 switch (hash) { 8356 case -1618432855: // identifier 8357 this.getIdentifier().add(castToIdentifier(value)); // Identifier 8358 return value; 8359 case -892481550: // status 8360 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 8361 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 8362 return value; 8363 case 3575610: // type 8364 this.type = castToCodeableConcept(value); // CodeableConcept 8365 return value; 8366 case -1868521062: // subType 8367 this.getSubType().add(castToCodeableConcept(value)); // CodeableConcept 8368 return value; 8369 case 116103: // use 8370 value = new UseEnumFactory().fromType(castToCode(value)); 8371 this.use = (Enumeration) value; // Enumeration<Use> 8372 return value; 8373 case -791418107: // patient 8374 this.patient = castToReference(value); // Reference 8375 return value; 8376 case -332066046: // billablePeriod 8377 this.billablePeriod = castToPeriod(value); // Period 8378 return value; 8379 case 1028554472: // created 8380 this.created = castToDateTime(value); // DateTimeType 8381 return value; 8382 case -1591951995: // enterer 8383 this.enterer = castToReference(value); // Reference 8384 return value; 8385 case 1957615864: // insurer 8386 this.insurer = castToReference(value); // Reference 8387 return value; 8388 case -987494927: // provider 8389 this.provider = castToReference(value); // Reference 8390 return value; 8391 case 1178922291: // organization 8392 this.organization = castToReference(value); // Reference 8393 return value; 8394 case -1165461084: // priority 8395 this.priority = castToCodeableConcept(value); // CodeableConcept 8396 return value; 8397 case 1314609806: // fundsReserve 8398 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 8399 return value; 8400 case 1090493483: // related 8401 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 8402 return value; 8403 case 460301338: // prescription 8404 this.prescription = castToReference(value); // Reference 8405 return value; 8406 case -1814015861: // originalPrescription 8407 this.originalPrescription = castToReference(value); // Reference 8408 return value; 8409 case 106443592: // payee 8410 this.payee = (PayeeComponent) value; // PayeeComponent 8411 return value; 8412 case -722568291: // referral 8413 this.referral = castToReference(value); // Reference 8414 return value; 8415 case 501116579: // facility 8416 this.facility = castToReference(value); // Reference 8417 return value; 8418 case -7323378: // careTeam 8419 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 8420 return value; 8421 case 1968600364: // information 8422 this.getInformation().add((SpecialConditionComponent) value); // SpecialConditionComponent 8423 return value; 8424 case 1196993265: // diagnosis 8425 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 8426 return value; 8427 case -1095204141: // procedure 8428 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 8429 return value; 8430 case 73049818: // insurance 8431 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 8432 return value; 8433 case -2143202801: // accident 8434 this.accident = (AccidentComponent) value; // AccidentComponent 8435 return value; 8436 case 1051487345: // employmentImpacted 8437 this.employmentImpacted = castToPeriod(value); // Period 8438 return value; 8439 case 1057894634: // hospitalization 8440 this.hospitalization = castToPeriod(value); // Period 8441 return value; 8442 case 3242771: // item 8443 this.getItem().add((ItemComponent) value); // ItemComponent 8444 return value; 8445 case 110549828: // total 8446 this.total = castToMoney(value); // Money 8447 return value; 8448 default: return super.setProperty(hash, name, value); 8449 } 8450 8451 } 8452 8453 @Override 8454 public Base setProperty(String name, Base value) throws FHIRException { 8455 if (name.equals("identifier")) { 8456 this.getIdentifier().add(castToIdentifier(value)); 8457 } else if (name.equals("status")) { 8458 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 8459 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 8460 } else if (name.equals("type")) { 8461 this.type = castToCodeableConcept(value); // CodeableConcept 8462 } else if (name.equals("subType")) { 8463 this.getSubType().add(castToCodeableConcept(value)); 8464 } else if (name.equals("use")) { 8465 value = new UseEnumFactory().fromType(castToCode(value)); 8466 this.use = (Enumeration) value; // Enumeration<Use> 8467 } else if (name.equals("patient")) { 8468 this.patient = castToReference(value); // Reference 8469 } else if (name.equals("billablePeriod")) { 8470 this.billablePeriod = castToPeriod(value); // Period 8471 } else if (name.equals("created")) { 8472 this.created = castToDateTime(value); // DateTimeType 8473 } else if (name.equals("enterer")) { 8474 this.enterer = castToReference(value); // Reference 8475 } else if (name.equals("insurer")) { 8476 this.insurer = castToReference(value); // Reference 8477 } else if (name.equals("provider")) { 8478 this.provider = castToReference(value); // Reference 8479 } else if (name.equals("organization")) { 8480 this.organization = castToReference(value); // Reference 8481 } else if (name.equals("priority")) { 8482 this.priority = castToCodeableConcept(value); // CodeableConcept 8483 } else if (name.equals("fundsReserve")) { 8484 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 8485 } else if (name.equals("related")) { 8486 this.getRelated().add((RelatedClaimComponent) value); 8487 } else if (name.equals("prescription")) { 8488 this.prescription = castToReference(value); // Reference 8489 } else if (name.equals("originalPrescription")) { 8490 this.originalPrescription = castToReference(value); // Reference 8491 } else if (name.equals("payee")) { 8492 this.payee = (PayeeComponent) value; // PayeeComponent 8493 } else if (name.equals("referral")) { 8494 this.referral = castToReference(value); // Reference 8495 } else if (name.equals("facility")) { 8496 this.facility = castToReference(value); // Reference 8497 } else if (name.equals("careTeam")) { 8498 this.getCareTeam().add((CareTeamComponent) value); 8499 } else if (name.equals("information")) { 8500 this.getInformation().add((SpecialConditionComponent) value); 8501 } else if (name.equals("diagnosis")) { 8502 this.getDiagnosis().add((DiagnosisComponent) value); 8503 } else if (name.equals("procedure")) { 8504 this.getProcedure().add((ProcedureComponent) value); 8505 } else if (name.equals("insurance")) { 8506 this.getInsurance().add((InsuranceComponent) value); 8507 } else if (name.equals("accident")) { 8508 this.accident = (AccidentComponent) value; // AccidentComponent 8509 } else if (name.equals("employmentImpacted")) { 8510 this.employmentImpacted = castToPeriod(value); // Period 8511 } else if (name.equals("hospitalization")) { 8512 this.hospitalization = castToPeriod(value); // Period 8513 } else if (name.equals("item")) { 8514 this.getItem().add((ItemComponent) value); 8515 } else if (name.equals("total")) { 8516 this.total = castToMoney(value); // Money 8517 } else 8518 return super.setProperty(name, value); 8519 return value; 8520 } 8521 8522 @Override 8523 public Base makeProperty(int hash, String name) throws FHIRException { 8524 switch (hash) { 8525 case -1618432855: return addIdentifier(); 8526 case -892481550: return getStatusElement(); 8527 case 3575610: return getType(); 8528 case -1868521062: return addSubType(); 8529 case 116103: return getUseElement(); 8530 case -791418107: return getPatient(); 8531 case -332066046: return getBillablePeriod(); 8532 case 1028554472: return getCreatedElement(); 8533 case -1591951995: return getEnterer(); 8534 case 1957615864: return getInsurer(); 8535 case -987494927: return getProvider(); 8536 case 1178922291: return getOrganization(); 8537 case -1165461084: return getPriority(); 8538 case 1314609806: return getFundsReserve(); 8539 case 1090493483: return addRelated(); 8540 case 460301338: return getPrescription(); 8541 case -1814015861: return getOriginalPrescription(); 8542 case 106443592: return getPayee(); 8543 case -722568291: return getReferral(); 8544 case 501116579: return getFacility(); 8545 case -7323378: return addCareTeam(); 8546 case 1968600364: return addInformation(); 8547 case 1196993265: return addDiagnosis(); 8548 case -1095204141: return addProcedure(); 8549 case 73049818: return addInsurance(); 8550 case -2143202801: return getAccident(); 8551 case 1051487345: return getEmploymentImpacted(); 8552 case 1057894634: return getHospitalization(); 8553 case 3242771: return addItem(); 8554 case 110549828: return getTotal(); 8555 default: return super.makeProperty(hash, name); 8556 } 8557 8558 } 8559 8560 @Override 8561 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8562 switch (hash) { 8563 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 8564 case -892481550: /*status*/ return new String[] {"code"}; 8565 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 8566 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 8567 case 116103: /*use*/ return new String[] {"code"}; 8568 case -791418107: /*patient*/ return new String[] {"Reference"}; 8569 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 8570 case 1028554472: /*created*/ return new String[] {"dateTime"}; 8571 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 8572 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 8573 case -987494927: /*provider*/ return new String[] {"Reference"}; 8574 case 1178922291: /*organization*/ return new String[] {"Reference"}; 8575 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 8576 case 1314609806: /*fundsReserve*/ return new String[] {"CodeableConcept"}; 8577 case 1090493483: /*related*/ return new String[] {}; 8578 case 460301338: /*prescription*/ return new String[] {"Reference"}; 8579 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 8580 case 106443592: /*payee*/ return new String[] {}; 8581 case -722568291: /*referral*/ return new String[] {"Reference"}; 8582 case 501116579: /*facility*/ return new String[] {"Reference"}; 8583 case -7323378: /*careTeam*/ return new String[] {}; 8584 case 1968600364: /*information*/ return new String[] {}; 8585 case 1196993265: /*diagnosis*/ return new String[] {}; 8586 case -1095204141: /*procedure*/ return new String[] {}; 8587 case 73049818: /*insurance*/ return new String[] {}; 8588 case -2143202801: /*accident*/ return new String[] {}; 8589 case 1051487345: /*employmentImpacted*/ return new String[] {"Period"}; 8590 case 1057894634: /*hospitalization*/ return new String[] {"Period"}; 8591 case 3242771: /*item*/ return new String[] {}; 8592 case 110549828: /*total*/ return new String[] {"Money"}; 8593 default: return super.getTypesForProperty(hash, name); 8594 } 8595 8596 } 8597 8598 @Override 8599 public Base addChild(String name) throws FHIRException { 8600 if (name.equals("identifier")) { 8601 return addIdentifier(); 8602 } 8603 else if (name.equals("status")) { 8604 throw new FHIRException("Cannot call addChild on a singleton property Claim.status"); 8605 } 8606 else if (name.equals("type")) { 8607 this.type = new CodeableConcept(); 8608 return this.type; 8609 } 8610 else if (name.equals("subType")) { 8611 return addSubType(); 8612 } 8613 else if (name.equals("use")) { 8614 throw new FHIRException("Cannot call addChild on a singleton property Claim.use"); 8615 } 8616 else if (name.equals("patient")) { 8617 this.patient = new Reference(); 8618 return this.patient; 8619 } 8620 else if (name.equals("billablePeriod")) { 8621 this.billablePeriod = new Period(); 8622 return this.billablePeriod; 8623 } 8624 else if (name.equals("created")) { 8625 throw new FHIRException("Cannot call addChild on a singleton property Claim.created"); 8626 } 8627 else if (name.equals("enterer")) { 8628 this.enterer = new Reference(); 8629 return this.enterer; 8630 } 8631 else if (name.equals("insurer")) { 8632 this.insurer = new Reference(); 8633 return this.insurer; 8634 } 8635 else if (name.equals("provider")) { 8636 this.provider = new Reference(); 8637 return this.provider; 8638 } 8639 else if (name.equals("organization")) { 8640 this.organization = new Reference(); 8641 return this.organization; 8642 } 8643 else if (name.equals("priority")) { 8644 this.priority = new CodeableConcept(); 8645 return this.priority; 8646 } 8647 else if (name.equals("fundsReserve")) { 8648 this.fundsReserve = new CodeableConcept(); 8649 return this.fundsReserve; 8650 } 8651 else if (name.equals("related")) { 8652 return addRelated(); 8653 } 8654 else if (name.equals("prescription")) { 8655 this.prescription = new Reference(); 8656 return this.prescription; 8657 } 8658 else if (name.equals("originalPrescription")) { 8659 this.originalPrescription = new Reference(); 8660 return this.originalPrescription; 8661 } 8662 else if (name.equals("payee")) { 8663 this.payee = new PayeeComponent(); 8664 return this.payee; 8665 } 8666 else if (name.equals("referral")) { 8667 this.referral = new Reference(); 8668 return this.referral; 8669 } 8670 else if (name.equals("facility")) { 8671 this.facility = new Reference(); 8672 return this.facility; 8673 } 8674 else if (name.equals("careTeam")) { 8675 return addCareTeam(); 8676 } 8677 else if (name.equals("information")) { 8678 return addInformation(); 8679 } 8680 else if (name.equals("diagnosis")) { 8681 return addDiagnosis(); 8682 } 8683 else if (name.equals("procedure")) { 8684 return addProcedure(); 8685 } 8686 else if (name.equals("insurance")) { 8687 return addInsurance(); 8688 } 8689 else if (name.equals("accident")) { 8690 this.accident = new AccidentComponent(); 8691 return this.accident; 8692 } 8693 else if (name.equals("employmentImpacted")) { 8694 this.employmentImpacted = new Period(); 8695 return this.employmentImpacted; 8696 } 8697 else if (name.equals("hospitalization")) { 8698 this.hospitalization = new Period(); 8699 return this.hospitalization; 8700 } 8701 else if (name.equals("item")) { 8702 return addItem(); 8703 } 8704 else if (name.equals("total")) { 8705 this.total = new Money(); 8706 return this.total; 8707 } 8708 else 8709 return super.addChild(name); 8710 } 8711 8712 public String fhirType() { 8713 return "Claim"; 8714 8715 } 8716 8717 public Claim copy() { 8718 Claim dst = new Claim(); 8719 copyValues(dst); 8720 if (identifier != null) { 8721 dst.identifier = new ArrayList<Identifier>(); 8722 for (Identifier i : identifier) 8723 dst.identifier.add(i.copy()); 8724 }; 8725 dst.status = status == null ? null : status.copy(); 8726 dst.type = type == null ? null : type.copy(); 8727 if (subType != null) { 8728 dst.subType = new ArrayList<CodeableConcept>(); 8729 for (CodeableConcept i : subType) 8730 dst.subType.add(i.copy()); 8731 }; 8732 dst.use = use == null ? null : use.copy(); 8733 dst.patient = patient == null ? null : patient.copy(); 8734 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 8735 dst.created = created == null ? null : created.copy(); 8736 dst.enterer = enterer == null ? null : enterer.copy(); 8737 dst.insurer = insurer == null ? null : insurer.copy(); 8738 dst.provider = provider == null ? null : provider.copy(); 8739 dst.organization = organization == null ? null : organization.copy(); 8740 dst.priority = priority == null ? null : priority.copy(); 8741 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 8742 if (related != null) { 8743 dst.related = new ArrayList<RelatedClaimComponent>(); 8744 for (RelatedClaimComponent i : related) 8745 dst.related.add(i.copy()); 8746 }; 8747 dst.prescription = prescription == null ? null : prescription.copy(); 8748 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 8749 dst.payee = payee == null ? null : payee.copy(); 8750 dst.referral = referral == null ? null : referral.copy(); 8751 dst.facility = facility == null ? null : facility.copy(); 8752 if (careTeam != null) { 8753 dst.careTeam = new ArrayList<CareTeamComponent>(); 8754 for (CareTeamComponent i : careTeam) 8755 dst.careTeam.add(i.copy()); 8756 }; 8757 if (information != null) { 8758 dst.information = new ArrayList<SpecialConditionComponent>(); 8759 for (SpecialConditionComponent i : information) 8760 dst.information.add(i.copy()); 8761 }; 8762 if (diagnosis != null) { 8763 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 8764 for (DiagnosisComponent i : diagnosis) 8765 dst.diagnosis.add(i.copy()); 8766 }; 8767 if (procedure != null) { 8768 dst.procedure = new ArrayList<ProcedureComponent>(); 8769 for (ProcedureComponent i : procedure) 8770 dst.procedure.add(i.copy()); 8771 }; 8772 if (insurance != null) { 8773 dst.insurance = new ArrayList<InsuranceComponent>(); 8774 for (InsuranceComponent i : insurance) 8775 dst.insurance.add(i.copy()); 8776 }; 8777 dst.accident = accident == null ? null : accident.copy(); 8778 dst.employmentImpacted = employmentImpacted == null ? null : employmentImpacted.copy(); 8779 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 8780 if (item != null) { 8781 dst.item = new ArrayList<ItemComponent>(); 8782 for (ItemComponent i : item) 8783 dst.item.add(i.copy()); 8784 }; 8785 dst.total = total == null ? null : total.copy(); 8786 return dst; 8787 } 8788 8789 protected Claim typedCopy() { 8790 return copy(); 8791 } 8792 8793 @Override 8794 public boolean equalsDeep(Base other_) { 8795 if (!super.equalsDeep(other_)) 8796 return false; 8797 if (!(other_ instanceof Claim)) 8798 return false; 8799 Claim o = (Claim) other_; 8800 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 8801 && compareDeep(subType, o.subType, true) && compareDeep(use, o.use, true) && compareDeep(patient, o.patient, true) 8802 && compareDeep(billablePeriod, o.billablePeriod, true) && compareDeep(created, o.created, true) 8803 && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) && compareDeep(provider, o.provider, true) 8804 && compareDeep(organization, o.organization, true) && compareDeep(priority, o.priority, true) && compareDeep(fundsReserve, o.fundsReserve, true) 8805 && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) && compareDeep(originalPrescription, o.originalPrescription, true) 8806 && compareDeep(payee, o.payee, true) && compareDeep(referral, o.referral, true) && compareDeep(facility, o.facility, true) 8807 && compareDeep(careTeam, o.careTeam, true) && compareDeep(information, o.information, true) && compareDeep(diagnosis, o.diagnosis, true) 8808 && compareDeep(procedure, o.procedure, true) && compareDeep(insurance, o.insurance, true) && compareDeep(accident, o.accident, true) 8809 && compareDeep(employmentImpacted, o.employmentImpacted, true) && compareDeep(hospitalization, o.hospitalization, true) 8810 && compareDeep(item, o.item, true) && compareDeep(total, o.total, true); 8811 } 8812 8813 @Override 8814 public boolean equalsShallow(Base other_) { 8815 if (!super.equalsShallow(other_)) 8816 return false; 8817 if (!(other_ instanceof Claim)) 8818 return false; 8819 Claim o = (Claim) other_; 8820 return compareValues(status, o.status, true) && compareValues(use, o.use, true) && compareValues(created, o.created, true) 8821 ; 8822 } 8823 8824 public boolean isEmpty() { 8825 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 8826 , subType, use, patient, billablePeriod, created, enterer, insurer, provider 8827 , organization, priority, fundsReserve, related, prescription, originalPrescription 8828 , payee, referral, facility, careTeam, information, diagnosis, procedure, insurance 8829 , accident, employmentImpacted, hospitalization, item, total); 8830 } 8831 8832 @Override 8833 public ResourceType getResourceType() { 8834 return ResourceType.Claim; 8835 } 8836 8837 /** 8838 * Search parameter: <b>care-team</b> 8839 * <p> 8840 * Description: <b>Member of the CareTeam</b><br> 8841 * Type: <b>reference</b><br> 8842 * Path: <b>Claim.careTeam.provider</b><br> 8843 * </p> 8844 */ 8845 @SearchParamDefinition(name="care-team", path="Claim.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 8846 public static final String SP_CARE_TEAM = "care-team"; 8847 /** 8848 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 8849 * <p> 8850 * Description: <b>Member of the CareTeam</b><br> 8851 * Type: <b>reference</b><br> 8852 * Path: <b>Claim.careTeam.provider</b><br> 8853 * </p> 8854 */ 8855 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 8856 8857/** 8858 * Constant for fluent queries to be used to add include statements. Specifies 8859 * the path value of "<b>Claim:care-team</b>". 8860 */ 8861 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("Claim:care-team").toLocked(); 8862 8863 /** 8864 * Search parameter: <b>identifier</b> 8865 * <p> 8866 * Description: <b>The primary identifier of the financial resource</b><br> 8867 * Type: <b>token</b><br> 8868 * Path: <b>Claim.identifier</b><br> 8869 * </p> 8870 */ 8871 @SearchParamDefinition(name="identifier", path="Claim.identifier", description="The primary identifier of the financial resource", type="token" ) 8872 public static final String SP_IDENTIFIER = "identifier"; 8873 /** 8874 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 8875 * <p> 8876 * Description: <b>The primary identifier of the financial resource</b><br> 8877 * Type: <b>token</b><br> 8878 * Path: <b>Claim.identifier</b><br> 8879 * </p> 8880 */ 8881 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 8882 8883 /** 8884 * Search parameter: <b>use</b> 8885 * <p> 8886 * Description: <b>The kind of financial resource</b><br> 8887 * Type: <b>token</b><br> 8888 * Path: <b>Claim.use</b><br> 8889 * </p> 8890 */ 8891 @SearchParamDefinition(name="use", path="Claim.use", description="The kind of financial resource", type="token" ) 8892 public static final String SP_USE = "use"; 8893 /** 8894 * <b>Fluent Client</b> search parameter constant for <b>use</b> 8895 * <p> 8896 * Description: <b>The kind of financial resource</b><br> 8897 * Type: <b>token</b><br> 8898 * Path: <b>Claim.use</b><br> 8899 * </p> 8900 */ 8901 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_USE); 8902 8903 /** 8904 * Search parameter: <b>created</b> 8905 * <p> 8906 * Description: <b>The creation date for the Claim</b><br> 8907 * Type: <b>date</b><br> 8908 * Path: <b>Claim.created</b><br> 8909 * </p> 8910 */ 8911 @SearchParamDefinition(name="created", path="Claim.created", description="The creation date for the Claim", type="date" ) 8912 public static final String SP_CREATED = "created"; 8913 /** 8914 * <b>Fluent Client</b> search parameter constant for <b>created</b> 8915 * <p> 8916 * Description: <b>The creation date for the Claim</b><br> 8917 * Type: <b>date</b><br> 8918 * Path: <b>Claim.created</b><br> 8919 * </p> 8920 */ 8921 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 8922 8923 /** 8924 * Search parameter: <b>encounter</b> 8925 * <p> 8926 * Description: <b>Encounters associated with a billed line item</b><br> 8927 * Type: <b>reference</b><br> 8928 * Path: <b>Claim.item.encounter</b><br> 8929 * </p> 8930 */ 8931 @SearchParamDefinition(name="encounter", path="Claim.item.encounter", description="Encounters associated with a billed line item", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 8932 public static final String SP_ENCOUNTER = "encounter"; 8933 /** 8934 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 8935 * <p> 8936 * Description: <b>Encounters associated with a billed line item</b><br> 8937 * Type: <b>reference</b><br> 8938 * Path: <b>Claim.item.encounter</b><br> 8939 * </p> 8940 */ 8941 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 8942 8943/** 8944 * Constant for fluent queries to be used to add include statements. Specifies 8945 * the path value of "<b>Claim:encounter</b>". 8946 */ 8947 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Claim:encounter").toLocked(); 8948 8949 /** 8950 * Search parameter: <b>priority</b> 8951 * <p> 8952 * Description: <b>Processing priority requested</b><br> 8953 * Type: <b>token</b><br> 8954 * Path: <b>Claim.priority</b><br> 8955 * </p> 8956 */ 8957 @SearchParamDefinition(name="priority", path="Claim.priority", description="Processing priority requested", type="token" ) 8958 public static final String SP_PRIORITY = "priority"; 8959 /** 8960 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 8961 * <p> 8962 * Description: <b>Processing priority requested</b><br> 8963 * Type: <b>token</b><br> 8964 * Path: <b>Claim.priority</b><br> 8965 * </p> 8966 */ 8967 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 8968 8969 /** 8970 * Search parameter: <b>payee</b> 8971 * <p> 8972 * Description: <b>The party receiving any payment for the Claim</b><br> 8973 * Type: <b>reference</b><br> 8974 * Path: <b>Claim.payee.party</b><br> 8975 * </p> 8976 */ 8977 @SearchParamDefinition(name="payee", path="Claim.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 8978 public static final String SP_PAYEE = "payee"; 8979 /** 8980 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 8981 * <p> 8982 * Description: <b>The party receiving any payment for the Claim</b><br> 8983 * Type: <b>reference</b><br> 8984 * Path: <b>Claim.payee.party</b><br> 8985 * </p> 8986 */ 8987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 8988 8989/** 8990 * Constant for fluent queries to be used to add include statements. Specifies 8991 * the path value of "<b>Claim:payee</b>". 8992 */ 8993 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("Claim:payee").toLocked(); 8994 8995 /** 8996 * Search parameter: <b>provider</b> 8997 * <p> 8998 * Description: <b>Provider responsible for the Claim</b><br> 8999 * Type: <b>reference</b><br> 9000 * Path: <b>Claim.provider</b><br> 9001 * </p> 9002 */ 9003 @SearchParamDefinition(name="provider", path="Claim.provider", description="Provider responsible for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 9004 public static final String SP_PROVIDER = "provider"; 9005 /** 9006 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 9007 * <p> 9008 * Description: <b>Provider responsible for the Claim</b><br> 9009 * Type: <b>reference</b><br> 9010 * Path: <b>Claim.provider</b><br> 9011 * </p> 9012 */ 9013 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 9014 9015/** 9016 * Constant for fluent queries to be used to add include statements. Specifies 9017 * the path value of "<b>Claim:provider</b>". 9018 */ 9019 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("Claim:provider").toLocked(); 9020 9021 /** 9022 * Search parameter: <b>patient</b> 9023 * <p> 9024 * Description: <b>Patient receiving the services</b><br> 9025 * Type: <b>reference</b><br> 9026 * Path: <b>Claim.patient</b><br> 9027 * </p> 9028 */ 9029 @SearchParamDefinition(name="patient", path="Claim.patient", description="Patient receiving the services", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 9030 public static final String SP_PATIENT = "patient"; 9031 /** 9032 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 9033 * <p> 9034 * Description: <b>Patient receiving the services</b><br> 9035 * Type: <b>reference</b><br> 9036 * Path: <b>Claim.patient</b><br> 9037 * </p> 9038 */ 9039 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 9040 9041/** 9042 * Constant for fluent queries to be used to add include statements. Specifies 9043 * the path value of "<b>Claim:patient</b>". 9044 */ 9045 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Claim:patient").toLocked(); 9046 9047 /** 9048 * Search parameter: <b>insurer</b> 9049 * <p> 9050 * Description: <b>The target payor/insurer for the Claim</b><br> 9051 * Type: <b>reference</b><br> 9052 * Path: <b>Claim.insurer</b><br> 9053 * </p> 9054 */ 9055 @SearchParamDefinition(name="insurer", path="Claim.insurer", description="The target payor/insurer for the Claim", type="reference", target={Organization.class } ) 9056 public static final String SP_INSURER = "insurer"; 9057 /** 9058 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 9059 * <p> 9060 * Description: <b>The target payor/insurer for the Claim</b><br> 9061 * Type: <b>reference</b><br> 9062 * Path: <b>Claim.insurer</b><br> 9063 * </p> 9064 */ 9065 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 9066 9067/** 9068 * Constant for fluent queries to be used to add include statements. Specifies 9069 * the path value of "<b>Claim:insurer</b>". 9070 */ 9071 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("Claim:insurer").toLocked(); 9072 9073 /** 9074 * Search parameter: <b>organization</b> 9075 * <p> 9076 * Description: <b>The reference to the providing organization</b><br> 9077 * Type: <b>reference</b><br> 9078 * Path: <b>Claim.organization</b><br> 9079 * </p> 9080 */ 9081 @SearchParamDefinition(name="organization", path="Claim.organization", description="The reference to the providing organization", type="reference", target={Organization.class } ) 9082 public static final String SP_ORGANIZATION = "organization"; 9083 /** 9084 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 9085 * <p> 9086 * Description: <b>The reference to the providing organization</b><br> 9087 * Type: <b>reference</b><br> 9088 * Path: <b>Claim.organization</b><br> 9089 * </p> 9090 */ 9091 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 9092 9093/** 9094 * Constant for fluent queries to be used to add include statements. Specifies 9095 * the path value of "<b>Claim:organization</b>". 9096 */ 9097 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Claim:organization").toLocked(); 9098 9099 /** 9100 * Search parameter: <b>enterer</b> 9101 * <p> 9102 * Description: <b>The party responsible for the entry of the Claim</b><br> 9103 * Type: <b>reference</b><br> 9104 * Path: <b>Claim.enterer</b><br> 9105 * </p> 9106 */ 9107 @SearchParamDefinition(name="enterer", path="Claim.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 9108 public static final String SP_ENTERER = "enterer"; 9109 /** 9110 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 9111 * <p> 9112 * Description: <b>The party responsible for the entry of the Claim</b><br> 9113 * Type: <b>reference</b><br> 9114 * Path: <b>Claim.enterer</b><br> 9115 * </p> 9116 */ 9117 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 9118 9119/** 9120 * Constant for fluent queries to be used to add include statements. Specifies 9121 * the path value of "<b>Claim:enterer</b>". 9122 */ 9123 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("Claim:enterer").toLocked(); 9124 9125 /** 9126 * Search parameter: <b>facility</b> 9127 * <p> 9128 * Description: <b>Facility responsible for the goods and services</b><br> 9129 * Type: <b>reference</b><br> 9130 * Path: <b>Claim.facility</b><br> 9131 * </p> 9132 */ 9133 @SearchParamDefinition(name="facility", path="Claim.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class } ) 9134 public static final String SP_FACILITY = "facility"; 9135 /** 9136 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 9137 * <p> 9138 * Description: <b>Facility responsible for the goods and services</b><br> 9139 * Type: <b>reference</b><br> 9140 * Path: <b>Claim.facility</b><br> 9141 * </p> 9142 */ 9143 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 9144 9145/** 9146 * Constant for fluent queries to be used to add include statements. Specifies 9147 * the path value of "<b>Claim:facility</b>". 9148 */ 9149 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("Claim:facility").toLocked(); 9150 9151 9152}