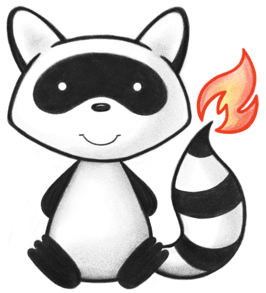
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 051 */ 052@ResourceDef(name="Claim", profile="http://hl7.org/fhir/Profile/Claim") 053public class Claim extends DomainResource { 054 055 public enum ClaimStatus { 056 /** 057 * The instance is currently in-force. 058 */ 059 ACTIVE, 060 /** 061 * The instance is withdrawn, rescinded or reversed. 062 */ 063 CANCELLED, 064 /** 065 * A new instance the contents of which is not complete. 066 */ 067 DRAFT, 068 /** 069 * The instance was entered in error. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static ClaimStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown ClaimStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: return "active"; 095 case CANCELLED: return "cancelled"; 096 case DRAFT: return "draft"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 105 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 106 case DRAFT: return "http://hl7.org/fhir/fm-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case ACTIVE: return "The instance is currently in-force."; 115 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 116 case DRAFT: return "A new instance the contents of which is not complete."; 117 case ENTEREDINERROR: return "The instance was entered in error."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ACTIVE: return "Active"; 125 case CANCELLED: return "Cancelled"; 126 case DRAFT: return "Draft"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class ClaimStatusEnumFactory implements EnumFactory<ClaimStatus> { 135 public ClaimStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("active".equals(codeString)) 140 return ClaimStatus.ACTIVE; 141 if ("cancelled".equals(codeString)) 142 return ClaimStatus.CANCELLED; 143 if ("draft".equals(codeString)) 144 return ClaimStatus.DRAFT; 145 if ("entered-in-error".equals(codeString)) 146 return ClaimStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown ClaimStatus code '"+codeString+"'"); 148 } 149 public Enumeration<ClaimStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<ClaimStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("active".equals(codeString)) 158 return new Enumeration<ClaimStatus>(this, ClaimStatus.ACTIVE); 159 if ("cancelled".equals(codeString)) 160 return new Enumeration<ClaimStatus>(this, ClaimStatus.CANCELLED); 161 if ("draft".equals(codeString)) 162 return new Enumeration<ClaimStatus>(this, ClaimStatus.DRAFT); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<ClaimStatus>(this, ClaimStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown ClaimStatus code '"+codeString+"'"); 166 } 167 public String toCode(ClaimStatus code) { 168 if (code == ClaimStatus.NULL) 169 return null; 170 if (code == ClaimStatus.ACTIVE) 171 return "active"; 172 if (code == ClaimStatus.CANCELLED) 173 return "cancelled"; 174 if (code == ClaimStatus.DRAFT) 175 return "draft"; 176 if (code == ClaimStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(ClaimStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 public enum Use { 186 /** 187 * The treatment is complete and this represents a Claim for the services. 188 */ 189 COMPLETE, 190 /** 191 * The treatment is proposed and this represents a Pre-authorization for the services. 192 */ 193 PROPOSED, 194 /** 195 * The treatment is proposed and this represents a Pre-determination for the services. 196 */ 197 EXPLORATORY, 198 /** 199 * A locally defined or otherwise resolved status. 200 */ 201 OTHER, 202 /** 203 * added to help the parsers with the generic types 204 */ 205 NULL; 206 public static Use fromCode(String codeString) throws FHIRException { 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("complete".equals(codeString)) 210 return COMPLETE; 211 if ("proposed".equals(codeString)) 212 return PROPOSED; 213 if ("exploratory".equals(codeString)) 214 return EXPLORATORY; 215 if ("other".equals(codeString)) 216 return OTHER; 217 if (Configuration.isAcceptInvalidEnums()) 218 return null; 219 else 220 throw new FHIRException("Unknown Use code '"+codeString+"'"); 221 } 222 public String toCode() { 223 switch (this) { 224 case COMPLETE: return "complete"; 225 case PROPOSED: return "proposed"; 226 case EXPLORATORY: return "exploratory"; 227 case OTHER: return "other"; 228 case NULL: return null; 229 default: return "?"; 230 } 231 } 232 public String getSystem() { 233 switch (this) { 234 case COMPLETE: return "http://hl7.org/fhir/claim-use"; 235 case PROPOSED: return "http://hl7.org/fhir/claim-use"; 236 case EXPLORATORY: return "http://hl7.org/fhir/claim-use"; 237 case OTHER: return "http://hl7.org/fhir/claim-use"; 238 case NULL: return null; 239 default: return "?"; 240 } 241 } 242 public String getDefinition() { 243 switch (this) { 244 case COMPLETE: return "The treatment is complete and this represents a Claim for the services."; 245 case PROPOSED: return "The treatment is proposed and this represents a Pre-authorization for the services."; 246 case EXPLORATORY: return "The treatment is proposed and this represents a Pre-determination for the services."; 247 case OTHER: return "A locally defined or otherwise resolved status."; 248 case NULL: return null; 249 default: return "?"; 250 } 251 } 252 public String getDisplay() { 253 switch (this) { 254 case COMPLETE: return "Complete"; 255 case PROPOSED: return "Proposed"; 256 case EXPLORATORY: return "Exploratory"; 257 case OTHER: return "Other"; 258 case NULL: return null; 259 default: return "?"; 260 } 261 } 262 } 263 264 public static class UseEnumFactory implements EnumFactory<Use> { 265 public Use fromCode(String codeString) throws IllegalArgumentException { 266 if (codeString == null || "".equals(codeString)) 267 if (codeString == null || "".equals(codeString)) 268 return null; 269 if ("complete".equals(codeString)) 270 return Use.COMPLETE; 271 if ("proposed".equals(codeString)) 272 return Use.PROPOSED; 273 if ("exploratory".equals(codeString)) 274 return Use.EXPLORATORY; 275 if ("other".equals(codeString)) 276 return Use.OTHER; 277 throw new IllegalArgumentException("Unknown Use code '"+codeString+"'"); 278 } 279 public Enumeration<Use> fromType(PrimitiveType<?> code) throws FHIRException { 280 if (code == null) 281 return null; 282 if (code.isEmpty()) 283 return new Enumeration<Use>(this); 284 String codeString = code.asStringValue(); 285 if (codeString == null || "".equals(codeString)) 286 return null; 287 if ("complete".equals(codeString)) 288 return new Enumeration<Use>(this, Use.COMPLETE); 289 if ("proposed".equals(codeString)) 290 return new Enumeration<Use>(this, Use.PROPOSED); 291 if ("exploratory".equals(codeString)) 292 return new Enumeration<Use>(this, Use.EXPLORATORY); 293 if ("other".equals(codeString)) 294 return new Enumeration<Use>(this, Use.OTHER); 295 throw new FHIRException("Unknown Use code '"+codeString+"'"); 296 } 297 public String toCode(Use code) { 298 if (code == Use.NULL) 299 return null; 300 if (code == Use.COMPLETE) 301 return "complete"; 302 if (code == Use.PROPOSED) 303 return "proposed"; 304 if (code == Use.EXPLORATORY) 305 return "exploratory"; 306 if (code == Use.OTHER) 307 return "other"; 308 return "?"; 309 } 310 public String toSystem(Use code) { 311 return code.getSystem(); 312 } 313 } 314 315 @Block() 316 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 317 /** 318 * Other claims which are related to this claim such as prior claim versions or for related services. 319 */ 320 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 321 @Description(shortDefinition="Reference to the related claim", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 322 protected Reference claim; 323 324 /** 325 * The actual object that is the target of the reference (Other claims which are related to this claim such as prior claim versions or for related services.) 326 */ 327 protected Claim claimTarget; 328 329 /** 330 * For example prior or umbrella. 331 */ 332 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 333 @Description(shortDefinition="How the reference claim is related", formalDefinition="For example prior or umbrella." ) 334 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 335 protected CodeableConcept relationship; 336 337 /** 338 * An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # . 339 */ 340 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 341 @Description(shortDefinition="Related file or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # ." ) 342 protected Identifier reference; 343 344 private static final long serialVersionUID = -379338905L; 345 346 /** 347 * Constructor 348 */ 349 public RelatedClaimComponent() { 350 super(); 351 } 352 353 /** 354 * @return {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 355 */ 356 public Reference getClaim() { 357 if (this.claim == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 360 else if (Configuration.doAutoCreate()) 361 this.claim = new Reference(); // cc 362 return this.claim; 363 } 364 365 public boolean hasClaim() { 366 return this.claim != null && !this.claim.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 371 */ 372 public RelatedClaimComponent setClaim(Reference value) { 373 this.claim = value; 374 return this; 375 } 376 377 /** 378 * @return {@link #claim} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 379 */ 380 public Claim getClaimTarget() { 381 if (this.claimTarget == null) 382 if (Configuration.errorOnAutoCreate()) 383 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 384 else if (Configuration.doAutoCreate()) 385 this.claimTarget = new Claim(); // aa 386 return this.claimTarget; 387 } 388 389 /** 390 * @param value {@link #claim} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 391 */ 392 public RelatedClaimComponent setClaimTarget(Claim value) { 393 this.claimTarget = value; 394 return this; 395 } 396 397 /** 398 * @return {@link #relationship} (For example prior or umbrella.) 399 */ 400 public CodeableConcept getRelationship() { 401 if (this.relationship == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 404 else if (Configuration.doAutoCreate()) 405 this.relationship = new CodeableConcept(); // cc 406 return this.relationship; 407 } 408 409 public boolean hasRelationship() { 410 return this.relationship != null && !this.relationship.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #relationship} (For example prior or umbrella.) 415 */ 416 public RelatedClaimComponent setRelationship(CodeableConcept value) { 417 this.relationship = value; 418 return this; 419 } 420 421 /** 422 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 423 */ 424 public Identifier getReference() { 425 if (this.reference == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 428 else if (Configuration.doAutoCreate()) 429 this.reference = new Identifier(); // cc 430 return this.reference; 431 } 432 433 public boolean hasReference() { 434 return this.reference != null && !this.reference.isEmpty(); 435 } 436 437 /** 438 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 439 */ 440 public RelatedClaimComponent setReference(Identifier value) { 441 this.reference = value; 442 return this; 443 } 444 445 protected void listChildren(List<Property> children) { 446 super.listChildren(children); 447 children.add(new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim)); 448 children.add(new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship)); 449 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference)); 450 } 451 452 @Override 453 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 454 switch (_hash) { 455 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim); 456 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship); 457 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference); 458 default: return super.getNamedProperty(_hash, _name, _checkValid); 459 } 460 461 } 462 463 @Override 464 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 465 switch (hash) { 466 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 467 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 468 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 469 default: return super.getProperty(hash, name, checkValid); 470 } 471 472 } 473 474 @Override 475 public Base setProperty(int hash, String name, Base value) throws FHIRException { 476 switch (hash) { 477 case 94742588: // claim 478 this.claim = castToReference(value); // Reference 479 return value; 480 case -261851592: // relationship 481 this.relationship = castToCodeableConcept(value); // CodeableConcept 482 return value; 483 case -925155509: // reference 484 this.reference = castToIdentifier(value); // Identifier 485 return value; 486 default: return super.setProperty(hash, name, value); 487 } 488 489 } 490 491 @Override 492 public Base setProperty(String name, Base value) throws FHIRException { 493 if (name.equals("claim")) { 494 this.claim = castToReference(value); // Reference 495 } else if (name.equals("relationship")) { 496 this.relationship = castToCodeableConcept(value); // CodeableConcept 497 } else if (name.equals("reference")) { 498 this.reference = castToIdentifier(value); // Identifier 499 } else 500 return super.setProperty(name, value); 501 return value; 502 } 503 504 @Override 505 public Base makeProperty(int hash, String name) throws FHIRException { 506 switch (hash) { 507 case 94742588: return getClaim(); 508 case -261851592: return getRelationship(); 509 case -925155509: return getReference(); 510 default: return super.makeProperty(hash, name); 511 } 512 513 } 514 515 @Override 516 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 517 switch (hash) { 518 case 94742588: /*claim*/ return new String[] {"Reference"}; 519 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 520 case -925155509: /*reference*/ return new String[] {"Identifier"}; 521 default: return super.getTypesForProperty(hash, name); 522 } 523 524 } 525 526 @Override 527 public Base addChild(String name) throws FHIRException { 528 if (name.equals("claim")) { 529 this.claim = new Reference(); 530 return this.claim; 531 } 532 else if (name.equals("relationship")) { 533 this.relationship = new CodeableConcept(); 534 return this.relationship; 535 } 536 else if (name.equals("reference")) { 537 this.reference = new Identifier(); 538 return this.reference; 539 } 540 else 541 return super.addChild(name); 542 } 543 544 public RelatedClaimComponent copy() { 545 RelatedClaimComponent dst = new RelatedClaimComponent(); 546 copyValues(dst); 547 dst.claim = claim == null ? null : claim.copy(); 548 dst.relationship = relationship == null ? null : relationship.copy(); 549 dst.reference = reference == null ? null : reference.copy(); 550 return dst; 551 } 552 553 @Override 554 public boolean equalsDeep(Base other_) { 555 if (!super.equalsDeep(other_)) 556 return false; 557 if (!(other_ instanceof RelatedClaimComponent)) 558 return false; 559 RelatedClaimComponent o = (RelatedClaimComponent) other_; 560 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 561 ; 562 } 563 564 @Override 565 public boolean equalsShallow(Base other_) { 566 if (!super.equalsShallow(other_)) 567 return false; 568 if (!(other_ instanceof RelatedClaimComponent)) 569 return false; 570 RelatedClaimComponent o = (RelatedClaimComponent) other_; 571 return true; 572 } 573 574 public boolean isEmpty() { 575 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 576 ); 577 } 578 579 public String fhirType() { 580 return "Claim.related"; 581 582 } 583 584 } 585 586 @Block() 587 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 588 /** 589 * Type of Party to be reimbursed: Subscriber, provider, other. 590 */ 591 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 592 @Description(shortDefinition="Type of party: Subscriber, Provider, other", formalDefinition="Type of Party to be reimbursed: Subscriber, provider, other." ) 593 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 594 protected CodeableConcept type; 595 596 /** 597 * organization | patient | practitioner | relatedperson. 598 */ 599 @Child(name = "resourceType", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=false) 600 @Description(shortDefinition="organization | patient | practitioner | relatedperson", formalDefinition="organization | patient | practitioner | relatedperson." ) 601 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-payee-resource-type") 602 protected Coding resourceType; 603 604 /** 605 * Party to be reimbursed: Subscriber, provider, other. 606 */ 607 @Child(name = "party", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=false) 608 @Description(shortDefinition="Party to receive the payable", formalDefinition="Party to be reimbursed: Subscriber, provider, other." ) 609 protected Reference party; 610 611 /** 612 * The actual object that is the target of the reference (Party to be reimbursed: Subscriber, provider, other.) 613 */ 614 protected Resource partyTarget; 615 616 private static final long serialVersionUID = -1395150769L; 617 618 /** 619 * Constructor 620 */ 621 public PayeeComponent() { 622 super(); 623 } 624 625 /** 626 * Constructor 627 */ 628 public PayeeComponent(CodeableConcept type) { 629 super(); 630 this.type = type; 631 } 632 633 /** 634 * @return {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 635 */ 636 public CodeableConcept getType() { 637 if (this.type == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create PayeeComponent.type"); 640 else if (Configuration.doAutoCreate()) 641 this.type = new CodeableConcept(); // cc 642 return this.type; 643 } 644 645 public boolean hasType() { 646 return this.type != null && !this.type.isEmpty(); 647 } 648 649 /** 650 * @param value {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 651 */ 652 public PayeeComponent setType(CodeableConcept value) { 653 this.type = value; 654 return this; 655 } 656 657 /** 658 * @return {@link #resourceType} (organization | patient | practitioner | relatedperson.) 659 */ 660 public Coding getResourceType() { 661 if (this.resourceType == null) 662 if (Configuration.errorOnAutoCreate()) 663 throw new Error("Attempt to auto-create PayeeComponent.resourceType"); 664 else if (Configuration.doAutoCreate()) 665 this.resourceType = new Coding(); // cc 666 return this.resourceType; 667 } 668 669 public boolean hasResourceType() { 670 return this.resourceType != null && !this.resourceType.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #resourceType} (organization | patient | practitioner | relatedperson.) 675 */ 676 public PayeeComponent setResourceType(Coding value) { 677 this.resourceType = value; 678 return this; 679 } 680 681 /** 682 * @return {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 683 */ 684 public Reference getParty() { 685 if (this.party == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create PayeeComponent.party"); 688 else if (Configuration.doAutoCreate()) 689 this.party = new Reference(); // cc 690 return this.party; 691 } 692 693 public boolean hasParty() { 694 return this.party != null && !this.party.isEmpty(); 695 } 696 697 /** 698 * @param value {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 699 */ 700 public PayeeComponent setParty(Reference value) { 701 this.party = value; 702 return this; 703 } 704 705 /** 706 * @return {@link #party} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 707 */ 708 public Resource getPartyTarget() { 709 return this.partyTarget; 710 } 711 712 /** 713 * @param value {@link #party} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 714 */ 715 public PayeeComponent setPartyTarget(Resource value) { 716 this.partyTarget = value; 717 return this; 718 } 719 720 protected void listChildren(List<Property> children) { 721 super.listChildren(children); 722 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type)); 723 children.add(new Property("resourceType", "Coding", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType)); 724 children.add(new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party)); 725 } 726 727 @Override 728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 729 switch (_hash) { 730 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type); 731 case -384364440: /*resourceType*/ return new Property("resourceType", "Coding", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType); 732 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party); 733 default: return super.getNamedProperty(_hash, _name, _checkValid); 734 } 735 736 } 737 738 @Override 739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 740 switch (hash) { 741 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 742 case -384364440: /*resourceType*/ return this.resourceType == null ? new Base[0] : new Base[] {this.resourceType}; // Coding 743 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 744 default: return super.getProperty(hash, name, checkValid); 745 } 746 747 } 748 749 @Override 750 public Base setProperty(int hash, String name, Base value) throws FHIRException { 751 switch (hash) { 752 case 3575610: // type 753 this.type = castToCodeableConcept(value); // CodeableConcept 754 return value; 755 case -384364440: // resourceType 756 this.resourceType = castToCoding(value); // Coding 757 return value; 758 case 106437350: // party 759 this.party = castToReference(value); // Reference 760 return value; 761 default: return super.setProperty(hash, name, value); 762 } 763 764 } 765 766 @Override 767 public Base setProperty(String name, Base value) throws FHIRException { 768 if (name.equals("type")) { 769 this.type = castToCodeableConcept(value); // CodeableConcept 770 } else if (name.equals("resourceType")) { 771 this.resourceType = castToCoding(value); // Coding 772 } else if (name.equals("party")) { 773 this.party = castToReference(value); // Reference 774 } else 775 return super.setProperty(name, value); 776 return value; 777 } 778 779 @Override 780 public Base makeProperty(int hash, String name) throws FHIRException { 781 switch (hash) { 782 case 3575610: return getType(); 783 case -384364440: return getResourceType(); 784 case 106437350: return getParty(); 785 default: return super.makeProperty(hash, name); 786 } 787 788 } 789 790 @Override 791 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 792 switch (hash) { 793 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 794 case -384364440: /*resourceType*/ return new String[] {"Coding"}; 795 case 106437350: /*party*/ return new String[] {"Reference"}; 796 default: return super.getTypesForProperty(hash, name); 797 } 798 799 } 800 801 @Override 802 public Base addChild(String name) throws FHIRException { 803 if (name.equals("type")) { 804 this.type = new CodeableConcept(); 805 return this.type; 806 } 807 else if (name.equals("resourceType")) { 808 this.resourceType = new Coding(); 809 return this.resourceType; 810 } 811 else if (name.equals("party")) { 812 this.party = new Reference(); 813 return this.party; 814 } 815 else 816 return super.addChild(name); 817 } 818 819 public PayeeComponent copy() { 820 PayeeComponent dst = new PayeeComponent(); 821 copyValues(dst); 822 dst.type = type == null ? null : type.copy(); 823 dst.resourceType = resourceType == null ? null : resourceType.copy(); 824 dst.party = party == null ? null : party.copy(); 825 return dst; 826 } 827 828 @Override 829 public boolean equalsDeep(Base other_) { 830 if (!super.equalsDeep(other_)) 831 return false; 832 if (!(other_ instanceof PayeeComponent)) 833 return false; 834 PayeeComponent o = (PayeeComponent) other_; 835 return compareDeep(type, o.type, true) && compareDeep(resourceType, o.resourceType, true) && compareDeep(party, o.party, true) 836 ; 837 } 838 839 @Override 840 public boolean equalsShallow(Base other_) { 841 if (!super.equalsShallow(other_)) 842 return false; 843 if (!(other_ instanceof PayeeComponent)) 844 return false; 845 PayeeComponent o = (PayeeComponent) other_; 846 return true; 847 } 848 849 public boolean isEmpty() { 850 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resourceType, party 851 ); 852 } 853 854 public String fhirType() { 855 return "Claim.payee"; 856 857 } 858 859 } 860 861 @Block() 862 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 863 /** 864 * Sequence of the careTeam which serves to order and provide a link. 865 */ 866 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 867 @Description(shortDefinition="Number to covey order of careTeam", formalDefinition="Sequence of the careTeam which serves to order and provide a link." ) 868 protected PositiveIntType sequence; 869 870 /** 871 * Member of the team who provided the overall service. 872 */ 873 @Child(name = "provider", type = {Practitioner.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 874 @Description(shortDefinition="Provider individual or organization", formalDefinition="Member of the team who provided the overall service." ) 875 protected Reference provider; 876 877 /** 878 * The actual object that is the target of the reference (Member of the team who provided the overall service.) 879 */ 880 protected Resource providerTarget; 881 882 /** 883 * The party who is billing and responsible for the claimed good or service rendered to the patient. 884 */ 885 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 886 @Description(shortDefinition="Billing provider", formalDefinition="The party who is billing and responsible for the claimed good or service rendered to the patient." ) 887 protected BooleanType responsible; 888 889 /** 890 * The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team. 891 */ 892 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 893 @Description(shortDefinition="Role on the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team." ) 894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 895 protected CodeableConcept role; 896 897 /** 898 * The qualification which is applicable for this service. 899 */ 900 @Child(name = "qualification", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 901 @Description(shortDefinition="Type, classification or Specialization", formalDefinition="The qualification which is applicable for this service." ) 902 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 903 protected CodeableConcept qualification; 904 905 private static final long serialVersionUID = 1758966968L; 906 907 /** 908 * Constructor 909 */ 910 public CareTeamComponent() { 911 super(); 912 } 913 914 /** 915 * Constructor 916 */ 917 public CareTeamComponent(PositiveIntType sequence, Reference provider) { 918 super(); 919 this.sequence = sequence; 920 this.provider = provider; 921 } 922 923 /** 924 * @return {@link #sequence} (Sequence of the careTeam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 925 */ 926 public PositiveIntType getSequenceElement() { 927 if (this.sequence == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 930 else if (Configuration.doAutoCreate()) 931 this.sequence = new PositiveIntType(); // bb 932 return this.sequence; 933 } 934 935 public boolean hasSequenceElement() { 936 return this.sequence != null && !this.sequence.isEmpty(); 937 } 938 939 public boolean hasSequence() { 940 return this.sequence != null && !this.sequence.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #sequence} (Sequence of the careTeam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 945 */ 946 public CareTeamComponent setSequenceElement(PositiveIntType value) { 947 this.sequence = value; 948 return this; 949 } 950 951 /** 952 * @return Sequence of the careTeam which serves to order and provide a link. 953 */ 954 public int getSequence() { 955 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 956 } 957 958 /** 959 * @param value Sequence of the careTeam which serves to order and provide a link. 960 */ 961 public CareTeamComponent setSequence(int value) { 962 if (this.sequence == null) 963 this.sequence = new PositiveIntType(); 964 this.sequence.setValue(value); 965 return this; 966 } 967 968 /** 969 * @return {@link #provider} (Member of the team who provided the overall service.) 970 */ 971 public Reference getProvider() { 972 if (this.provider == null) 973 if (Configuration.errorOnAutoCreate()) 974 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 975 else if (Configuration.doAutoCreate()) 976 this.provider = new Reference(); // cc 977 return this.provider; 978 } 979 980 public boolean hasProvider() { 981 return this.provider != null && !this.provider.isEmpty(); 982 } 983 984 /** 985 * @param value {@link #provider} (Member of the team who provided the overall service.) 986 */ 987 public CareTeamComponent setProvider(Reference value) { 988 this.provider = value; 989 return this; 990 } 991 992 /** 993 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Member of the team who provided the overall service.) 994 */ 995 public Resource getProviderTarget() { 996 return this.providerTarget; 997 } 998 999 /** 1000 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Member of the team who provided the overall service.) 1001 */ 1002 public CareTeamComponent setProviderTarget(Resource value) { 1003 this.providerTarget = value; 1004 return this; 1005 } 1006 1007 /** 1008 * @return {@link #responsible} (The party who is billing and responsible for the claimed good or service rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1009 */ 1010 public BooleanType getResponsibleElement() { 1011 if (this.responsible == null) 1012 if (Configuration.errorOnAutoCreate()) 1013 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1014 else if (Configuration.doAutoCreate()) 1015 this.responsible = new BooleanType(); // bb 1016 return this.responsible; 1017 } 1018 1019 public boolean hasResponsibleElement() { 1020 return this.responsible != null && !this.responsible.isEmpty(); 1021 } 1022 1023 public boolean hasResponsible() { 1024 return this.responsible != null && !this.responsible.isEmpty(); 1025 } 1026 1027 /** 1028 * @param value {@link #responsible} (The party who is billing and responsible for the claimed good or service rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1029 */ 1030 public CareTeamComponent setResponsibleElement(BooleanType value) { 1031 this.responsible = value; 1032 return this; 1033 } 1034 1035 /** 1036 * @return The party who is billing and responsible for the claimed good or service rendered to the patient. 1037 */ 1038 public boolean getResponsible() { 1039 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1040 } 1041 1042 /** 1043 * @param value The party who is billing and responsible for the claimed good or service rendered to the patient. 1044 */ 1045 public CareTeamComponent setResponsible(boolean value) { 1046 if (this.responsible == null) 1047 this.responsible = new BooleanType(); 1048 this.responsible.setValue(value); 1049 return this; 1050 } 1051 1052 /** 1053 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1054 */ 1055 public CodeableConcept getRole() { 1056 if (this.role == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1059 else if (Configuration.doAutoCreate()) 1060 this.role = new CodeableConcept(); // cc 1061 return this.role; 1062 } 1063 1064 public boolean hasRole() { 1065 return this.role != null && !this.role.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1070 */ 1071 public CareTeamComponent setRole(CodeableConcept value) { 1072 this.role = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return {@link #qualification} (The qualification which is applicable for this service.) 1078 */ 1079 public CodeableConcept getQualification() { 1080 if (this.qualification == null) 1081 if (Configuration.errorOnAutoCreate()) 1082 throw new Error("Attempt to auto-create CareTeamComponent.qualification"); 1083 else if (Configuration.doAutoCreate()) 1084 this.qualification = new CodeableConcept(); // cc 1085 return this.qualification; 1086 } 1087 1088 public boolean hasQualification() { 1089 return this.qualification != null && !this.qualification.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #qualification} (The qualification which is applicable for this service.) 1094 */ 1095 public CareTeamComponent setQualification(CodeableConcept value) { 1096 this.qualification = value; 1097 return this; 1098 } 1099 1100 protected void listChildren(List<Property> children) { 1101 super.listChildren(children); 1102 children.add(new Property("sequence", "positiveInt", "Sequence of the careTeam which serves to order and provide a link.", 0, 1, sequence)); 1103 children.add(new Property("provider", "Reference(Practitioner|Organization)", "Member of the team who provided the overall service.", 0, 1, provider)); 1104 children.add(new Property("responsible", "boolean", "The party who is billing and responsible for the claimed good or service rendered to the patient.", 0, 1, responsible)); 1105 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role)); 1106 children.add(new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification)); 1107 } 1108 1109 @Override 1110 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1111 switch (_hash) { 1112 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of the careTeam which serves to order and provide a link.", 0, 1, sequence); 1113 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|Organization)", "Member of the team who provided the overall service.", 0, 1, provider); 1114 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The party who is billing and responsible for the claimed good or service rendered to the patient.", 0, 1, responsible); 1115 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role); 1116 case -631333393: /*qualification*/ return new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification); 1117 default: return super.getNamedProperty(_hash, _name, _checkValid); 1118 } 1119 1120 } 1121 1122 @Override 1123 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1124 switch (hash) { 1125 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1126 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1127 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1128 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1129 case -631333393: /*qualification*/ return this.qualification == null ? new Base[0] : new Base[] {this.qualification}; // CodeableConcept 1130 default: return super.getProperty(hash, name, checkValid); 1131 } 1132 1133 } 1134 1135 @Override 1136 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1137 switch (hash) { 1138 case 1349547969: // sequence 1139 this.sequence = castToPositiveInt(value); // PositiveIntType 1140 return value; 1141 case -987494927: // provider 1142 this.provider = castToReference(value); // Reference 1143 return value; 1144 case 1847674614: // responsible 1145 this.responsible = castToBoolean(value); // BooleanType 1146 return value; 1147 case 3506294: // role 1148 this.role = castToCodeableConcept(value); // CodeableConcept 1149 return value; 1150 case -631333393: // qualification 1151 this.qualification = castToCodeableConcept(value); // CodeableConcept 1152 return value; 1153 default: return super.setProperty(hash, name, value); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base setProperty(String name, Base value) throws FHIRException { 1160 if (name.equals("sequence")) { 1161 this.sequence = castToPositiveInt(value); // PositiveIntType 1162 } else if (name.equals("provider")) { 1163 this.provider = castToReference(value); // Reference 1164 } else if (name.equals("responsible")) { 1165 this.responsible = castToBoolean(value); // BooleanType 1166 } else if (name.equals("role")) { 1167 this.role = castToCodeableConcept(value); // CodeableConcept 1168 } else if (name.equals("qualification")) { 1169 this.qualification = castToCodeableConcept(value); // CodeableConcept 1170 } else 1171 return super.setProperty(name, value); 1172 return value; 1173 } 1174 1175 @Override 1176 public Base makeProperty(int hash, String name) throws FHIRException { 1177 switch (hash) { 1178 case 1349547969: return getSequenceElement(); 1179 case -987494927: return getProvider(); 1180 case 1847674614: return getResponsibleElement(); 1181 case 3506294: return getRole(); 1182 case -631333393: return getQualification(); 1183 default: return super.makeProperty(hash, name); 1184 } 1185 1186 } 1187 1188 @Override 1189 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1190 switch (hash) { 1191 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1192 case -987494927: /*provider*/ return new String[] {"Reference"}; 1193 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1194 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1195 case -631333393: /*qualification*/ return new String[] {"CodeableConcept"}; 1196 default: return super.getTypesForProperty(hash, name); 1197 } 1198 1199 } 1200 1201 @Override 1202 public Base addChild(String name) throws FHIRException { 1203 if (name.equals("sequence")) { 1204 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 1205 } 1206 else if (name.equals("provider")) { 1207 this.provider = new Reference(); 1208 return this.provider; 1209 } 1210 else if (name.equals("responsible")) { 1211 throw new FHIRException("Cannot call addChild on a singleton property Claim.responsible"); 1212 } 1213 else if (name.equals("role")) { 1214 this.role = new CodeableConcept(); 1215 return this.role; 1216 } 1217 else if (name.equals("qualification")) { 1218 this.qualification = new CodeableConcept(); 1219 return this.qualification; 1220 } 1221 else 1222 return super.addChild(name); 1223 } 1224 1225 public CareTeamComponent copy() { 1226 CareTeamComponent dst = new CareTeamComponent(); 1227 copyValues(dst); 1228 dst.sequence = sequence == null ? null : sequence.copy(); 1229 dst.provider = provider == null ? null : provider.copy(); 1230 dst.responsible = responsible == null ? null : responsible.copy(); 1231 dst.role = role == null ? null : role.copy(); 1232 dst.qualification = qualification == null ? null : qualification.copy(); 1233 return dst; 1234 } 1235 1236 @Override 1237 public boolean equalsDeep(Base other_) { 1238 if (!super.equalsDeep(other_)) 1239 return false; 1240 if (!(other_ instanceof CareTeamComponent)) 1241 return false; 1242 CareTeamComponent o = (CareTeamComponent) other_; 1243 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1244 && compareDeep(role, o.role, true) && compareDeep(qualification, o.qualification, true); 1245 } 1246 1247 @Override 1248 public boolean equalsShallow(Base other_) { 1249 if (!super.equalsShallow(other_)) 1250 return false; 1251 if (!(other_ instanceof CareTeamComponent)) 1252 return false; 1253 CareTeamComponent o = (CareTeamComponent) other_; 1254 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1255 ; 1256 } 1257 1258 public boolean isEmpty() { 1259 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1260 , role, qualification); 1261 } 1262 1263 public String fhirType() { 1264 return "Claim.careTeam"; 1265 1266 } 1267 1268 } 1269 1270 @Block() 1271 public static class SpecialConditionComponent extends BackboneElement implements IBaseBackboneElement { 1272 /** 1273 * Sequence of the information element which serves to provide a link. 1274 */ 1275 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1276 @Description(shortDefinition="Information instance identifier", formalDefinition="Sequence of the information element which serves to provide a link." ) 1277 protected PositiveIntType sequence; 1278 1279 /** 1280 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 1281 */ 1282 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1283 @Description(shortDefinition="General class of information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 1284 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 1285 protected CodeableConcept category; 1286 1287 /** 1288 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication. 1289 */ 1290 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1291 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication." ) 1292 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 1293 protected CodeableConcept code; 1294 1295 /** 1296 * The date when or period to which this information refers. 1297 */ 1298 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1299 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 1300 protected Type timing; 1301 1302 /** 1303 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 1304 */ 1305 @Child(name = "value", type = {StringType.class, Quantity.class, Attachment.class, Reference.class}, order=5, min=0, max=1, modifier=false, summary=false) 1306 @Description(shortDefinition="Additional Data or supporting information", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 1307 protected Type value; 1308 1309 /** 1310 * For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content. 1311 */ 1312 @Child(name = "reason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1313 @Description(shortDefinition="Reason associated with the information", formalDefinition="For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content." ) 1314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1315 protected CodeableConcept reason; 1316 1317 private static final long serialVersionUID = -518630232L; 1318 1319 /** 1320 * Constructor 1321 */ 1322 public SpecialConditionComponent() { 1323 super(); 1324 } 1325 1326 /** 1327 * Constructor 1328 */ 1329 public SpecialConditionComponent(PositiveIntType sequence, CodeableConcept category) { 1330 super(); 1331 this.sequence = sequence; 1332 this.category = category; 1333 } 1334 1335 /** 1336 * @return {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1337 */ 1338 public PositiveIntType getSequenceElement() { 1339 if (this.sequence == null) 1340 if (Configuration.errorOnAutoCreate()) 1341 throw new Error("Attempt to auto-create SpecialConditionComponent.sequence"); 1342 else if (Configuration.doAutoCreate()) 1343 this.sequence = new PositiveIntType(); // bb 1344 return this.sequence; 1345 } 1346 1347 public boolean hasSequenceElement() { 1348 return this.sequence != null && !this.sequence.isEmpty(); 1349 } 1350 1351 public boolean hasSequence() { 1352 return this.sequence != null && !this.sequence.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1357 */ 1358 public SpecialConditionComponent setSequenceElement(PositiveIntType value) { 1359 this.sequence = value; 1360 return this; 1361 } 1362 1363 /** 1364 * @return Sequence of the information element which serves to provide a link. 1365 */ 1366 public int getSequence() { 1367 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1368 } 1369 1370 /** 1371 * @param value Sequence of the information element which serves to provide a link. 1372 */ 1373 public SpecialConditionComponent setSequence(int value) { 1374 if (this.sequence == null) 1375 this.sequence = new PositiveIntType(); 1376 this.sequence.setValue(value); 1377 return this; 1378 } 1379 1380 /** 1381 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1382 */ 1383 public CodeableConcept getCategory() { 1384 if (this.category == null) 1385 if (Configuration.errorOnAutoCreate()) 1386 throw new Error("Attempt to auto-create SpecialConditionComponent.category"); 1387 else if (Configuration.doAutoCreate()) 1388 this.category = new CodeableConcept(); // cc 1389 return this.category; 1390 } 1391 1392 public boolean hasCategory() { 1393 return this.category != null && !this.category.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1398 */ 1399 public SpecialConditionComponent setCategory(CodeableConcept value) { 1400 this.category = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 1406 */ 1407 public CodeableConcept getCode() { 1408 if (this.code == null) 1409 if (Configuration.errorOnAutoCreate()) 1410 throw new Error("Attempt to auto-create SpecialConditionComponent.code"); 1411 else if (Configuration.doAutoCreate()) 1412 this.code = new CodeableConcept(); // cc 1413 return this.code; 1414 } 1415 1416 public boolean hasCode() { 1417 return this.code != null && !this.code.isEmpty(); 1418 } 1419 1420 /** 1421 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 1422 */ 1423 public SpecialConditionComponent setCode(CodeableConcept value) { 1424 this.code = value; 1425 return this; 1426 } 1427 1428 /** 1429 * @return {@link #timing} (The date when or period to which this information refers.) 1430 */ 1431 public Type getTiming() { 1432 return this.timing; 1433 } 1434 1435 /** 1436 * @return {@link #timing} (The date when or period to which this information refers.) 1437 */ 1438 public DateType getTimingDateType() throws FHIRException { 1439 if (this.timing == null) 1440 return null; 1441 if (!(this.timing instanceof DateType)) 1442 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 1443 return (DateType) this.timing; 1444 } 1445 1446 public boolean hasTimingDateType() { 1447 return this.timing instanceof DateType; 1448 } 1449 1450 /** 1451 * @return {@link #timing} (The date when or period to which this information refers.) 1452 */ 1453 public Period getTimingPeriod() throws FHIRException { 1454 if (this.timing == null) 1455 return null; 1456 if (!(this.timing instanceof Period)) 1457 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 1458 return (Period) this.timing; 1459 } 1460 1461 public boolean hasTimingPeriod() { 1462 return this.timing instanceof Period; 1463 } 1464 1465 public boolean hasTiming() { 1466 return this.timing != null && !this.timing.isEmpty(); 1467 } 1468 1469 /** 1470 * @param value {@link #timing} (The date when or period to which this information refers.) 1471 */ 1472 public SpecialConditionComponent setTiming(Type value) throws FHIRFormatError { 1473 if (value != null && !(value instanceof DateType || value instanceof Period)) 1474 throw new FHIRFormatError("Not the right type for Claim.information.timing[x]: "+value.fhirType()); 1475 this.timing = value; 1476 return this; 1477 } 1478 1479 /** 1480 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1481 */ 1482 public Type getValue() { 1483 return this.value; 1484 } 1485 1486 /** 1487 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1488 */ 1489 public StringType getValueStringType() throws FHIRException { 1490 if (this.value == null) 1491 return null; 1492 if (!(this.value instanceof StringType)) 1493 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1494 return (StringType) this.value; 1495 } 1496 1497 public boolean hasValueStringType() { 1498 return this.value instanceof StringType; 1499 } 1500 1501 /** 1502 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1503 */ 1504 public Quantity getValueQuantity() throws FHIRException { 1505 if (this.value == null) 1506 return null; 1507 if (!(this.value instanceof Quantity)) 1508 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1509 return (Quantity) this.value; 1510 } 1511 1512 public boolean hasValueQuantity() { 1513 return this.value instanceof Quantity; 1514 } 1515 1516 /** 1517 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1518 */ 1519 public Attachment getValueAttachment() throws FHIRException { 1520 if (this.value == null) 1521 return null; 1522 if (!(this.value instanceof Attachment)) 1523 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1524 return (Attachment) this.value; 1525 } 1526 1527 public boolean hasValueAttachment() { 1528 return this.value instanceof Attachment; 1529 } 1530 1531 /** 1532 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1533 */ 1534 public Reference getValueReference() throws FHIRException { 1535 if (this.value == null) 1536 return null; 1537 if (!(this.value instanceof Reference)) 1538 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1539 return (Reference) this.value; 1540 } 1541 1542 public boolean hasValueReference() { 1543 return this.value instanceof Reference; 1544 } 1545 1546 public boolean hasValue() { 1547 return this.value != null && !this.value.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1552 */ 1553 public SpecialConditionComponent setValue(Type value) throws FHIRFormatError { 1554 if (value != null && !(value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference)) 1555 throw new FHIRFormatError("Not the right type for Claim.information.value[x]: "+value.fhirType()); 1556 this.value = value; 1557 return this; 1558 } 1559 1560 /** 1561 * @return {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1562 */ 1563 public CodeableConcept getReason() { 1564 if (this.reason == null) 1565 if (Configuration.errorOnAutoCreate()) 1566 throw new Error("Attempt to auto-create SpecialConditionComponent.reason"); 1567 else if (Configuration.doAutoCreate()) 1568 this.reason = new CodeableConcept(); // cc 1569 return this.reason; 1570 } 1571 1572 public boolean hasReason() { 1573 return this.reason != null && !this.reason.isEmpty(); 1574 } 1575 1576 /** 1577 * @param value {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1578 */ 1579 public SpecialConditionComponent setReason(CodeableConcept value) { 1580 this.reason = value; 1581 return this; 1582 } 1583 1584 protected void listChildren(List<Property> children) { 1585 super.listChildren(children); 1586 children.add(new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence)); 1587 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1588 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code)); 1589 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1590 children.add(new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1591 children.add(new Property("reason", "CodeableConcept", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason)); 1592 } 1593 1594 @Override 1595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1596 switch (_hash) { 1597 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence); 1598 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1599 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code); 1600 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1601 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1602 case 807935768: /*timingDate*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1603 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1604 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1605 case 111972721: /*value*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1606 case -1424603934: /*valueString*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1607 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1608 case -475566732: /*valueAttachment*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1609 case 1755241690: /*valueReference*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1610 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason); 1611 default: return super.getNamedProperty(_hash, _name, _checkValid); 1612 } 1613 1614 } 1615 1616 @Override 1617 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1618 switch (hash) { 1619 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1620 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1621 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1622 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 1623 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 1624 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1625 default: return super.getProperty(hash, name, checkValid); 1626 } 1627 1628 } 1629 1630 @Override 1631 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1632 switch (hash) { 1633 case 1349547969: // sequence 1634 this.sequence = castToPositiveInt(value); // PositiveIntType 1635 return value; 1636 case 50511102: // category 1637 this.category = castToCodeableConcept(value); // CodeableConcept 1638 return value; 1639 case 3059181: // code 1640 this.code = castToCodeableConcept(value); // CodeableConcept 1641 return value; 1642 case -873664438: // timing 1643 this.timing = castToType(value); // Type 1644 return value; 1645 case 111972721: // value 1646 this.value = castToType(value); // Type 1647 return value; 1648 case -934964668: // reason 1649 this.reason = castToCodeableConcept(value); // CodeableConcept 1650 return value; 1651 default: return super.setProperty(hash, name, value); 1652 } 1653 1654 } 1655 1656 @Override 1657 public Base setProperty(String name, Base value) throws FHIRException { 1658 if (name.equals("sequence")) { 1659 this.sequence = castToPositiveInt(value); // PositiveIntType 1660 } else if (name.equals("category")) { 1661 this.category = castToCodeableConcept(value); // CodeableConcept 1662 } else if (name.equals("code")) { 1663 this.code = castToCodeableConcept(value); // CodeableConcept 1664 } else if (name.equals("timing[x]")) { 1665 this.timing = castToType(value); // Type 1666 } else if (name.equals("value[x]")) { 1667 this.value = castToType(value); // Type 1668 } else if (name.equals("reason")) { 1669 this.reason = castToCodeableConcept(value); // CodeableConcept 1670 } else 1671 return super.setProperty(name, value); 1672 return value; 1673 } 1674 1675 @Override 1676 public Base makeProperty(int hash, String name) throws FHIRException { 1677 switch (hash) { 1678 case 1349547969: return getSequenceElement(); 1679 case 50511102: return getCategory(); 1680 case 3059181: return getCode(); 1681 case 164632566: return getTiming(); 1682 case -873664438: return getTiming(); 1683 case -1410166417: return getValue(); 1684 case 111972721: return getValue(); 1685 case -934964668: return getReason(); 1686 default: return super.makeProperty(hash, name); 1687 } 1688 1689 } 1690 1691 @Override 1692 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1693 switch (hash) { 1694 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1695 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1696 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1697 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1698 case 111972721: /*value*/ return new String[] {"string", "Quantity", "Attachment", "Reference"}; 1699 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1700 default: return super.getTypesForProperty(hash, name); 1701 } 1702 1703 } 1704 1705 @Override 1706 public Base addChild(String name) throws FHIRException { 1707 if (name.equals("sequence")) { 1708 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 1709 } 1710 else if (name.equals("category")) { 1711 this.category = new CodeableConcept(); 1712 return this.category; 1713 } 1714 else if (name.equals("code")) { 1715 this.code = new CodeableConcept(); 1716 return this.code; 1717 } 1718 else if (name.equals("timingDate")) { 1719 this.timing = new DateType(); 1720 return this.timing; 1721 } 1722 else if (name.equals("timingPeriod")) { 1723 this.timing = new Period(); 1724 return this.timing; 1725 } 1726 else if (name.equals("valueString")) { 1727 this.value = new StringType(); 1728 return this.value; 1729 } 1730 else if (name.equals("valueQuantity")) { 1731 this.value = new Quantity(); 1732 return this.value; 1733 } 1734 else if (name.equals("valueAttachment")) { 1735 this.value = new Attachment(); 1736 return this.value; 1737 } 1738 else if (name.equals("valueReference")) { 1739 this.value = new Reference(); 1740 return this.value; 1741 } 1742 else if (name.equals("reason")) { 1743 this.reason = new CodeableConcept(); 1744 return this.reason; 1745 } 1746 else 1747 return super.addChild(name); 1748 } 1749 1750 public SpecialConditionComponent copy() { 1751 SpecialConditionComponent dst = new SpecialConditionComponent(); 1752 copyValues(dst); 1753 dst.sequence = sequence == null ? null : sequence.copy(); 1754 dst.category = category == null ? null : category.copy(); 1755 dst.code = code == null ? null : code.copy(); 1756 dst.timing = timing == null ? null : timing.copy(); 1757 dst.value = value == null ? null : value.copy(); 1758 dst.reason = reason == null ? null : reason.copy(); 1759 return dst; 1760 } 1761 1762 @Override 1763 public boolean equalsDeep(Base other_) { 1764 if (!super.equalsDeep(other_)) 1765 return false; 1766 if (!(other_ instanceof SpecialConditionComponent)) 1767 return false; 1768 SpecialConditionComponent o = (SpecialConditionComponent) other_; 1769 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1770 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1771 ; 1772 } 1773 1774 @Override 1775 public boolean equalsShallow(Base other_) { 1776 if (!super.equalsShallow(other_)) 1777 return false; 1778 if (!(other_ instanceof SpecialConditionComponent)) 1779 return false; 1780 SpecialConditionComponent o = (SpecialConditionComponent) other_; 1781 return compareValues(sequence, o.sequence, true); 1782 } 1783 1784 public boolean isEmpty() { 1785 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1786 , timing, value, reason); 1787 } 1788 1789 public String fhirType() { 1790 return "Claim.information"; 1791 1792 } 1793 1794 } 1795 1796 @Block() 1797 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1798 /** 1799 * Sequence of diagnosis which serves to provide a link. 1800 */ 1801 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1802 @Description(shortDefinition="Number to covey order of diagnosis", formalDefinition="Sequence of diagnosis which serves to provide a link." ) 1803 protected PositiveIntType sequence; 1804 1805 /** 1806 * The diagnosis. 1807 */ 1808 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1809 @Description(shortDefinition="Patient's diagnosis", formalDefinition="The diagnosis." ) 1810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1811 protected Type diagnosis; 1812 1813 /** 1814 * The type of the Diagnosis, for example: admitting, primary, secondary, discharge. 1815 */ 1816 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1817 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="The type of the Diagnosis, for example: admitting, primary, secondary, discharge." ) 1818 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1819 protected List<CodeableConcept> type; 1820 1821 /** 1822 * The package billing code, for example DRG, based on the assigned grouping code system. 1823 */ 1824 @Child(name = "packageCode", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1825 @Description(shortDefinition="Package billing code", formalDefinition="The package billing code, for example DRG, based on the assigned grouping code system." ) 1826 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 1827 protected CodeableConcept packageCode; 1828 1829 private static final long serialVersionUID = -350960873L; 1830 1831 /** 1832 * Constructor 1833 */ 1834 public DiagnosisComponent() { 1835 super(); 1836 } 1837 1838 /** 1839 * Constructor 1840 */ 1841 public DiagnosisComponent(PositiveIntType sequence, Type diagnosis) { 1842 super(); 1843 this.sequence = sequence; 1844 this.diagnosis = diagnosis; 1845 } 1846 1847 /** 1848 * @return {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1849 */ 1850 public PositiveIntType getSequenceElement() { 1851 if (this.sequence == null) 1852 if (Configuration.errorOnAutoCreate()) 1853 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1854 else if (Configuration.doAutoCreate()) 1855 this.sequence = new PositiveIntType(); // bb 1856 return this.sequence; 1857 } 1858 1859 public boolean hasSequenceElement() { 1860 return this.sequence != null && !this.sequence.isEmpty(); 1861 } 1862 1863 public boolean hasSequence() { 1864 return this.sequence != null && !this.sequence.isEmpty(); 1865 } 1866 1867 /** 1868 * @param value {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1869 */ 1870 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1871 this.sequence = value; 1872 return this; 1873 } 1874 1875 /** 1876 * @return Sequence of diagnosis which serves to provide a link. 1877 */ 1878 public int getSequence() { 1879 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1880 } 1881 1882 /** 1883 * @param value Sequence of diagnosis which serves to provide a link. 1884 */ 1885 public DiagnosisComponent setSequence(int value) { 1886 if (this.sequence == null) 1887 this.sequence = new PositiveIntType(); 1888 this.sequence.setValue(value); 1889 return this; 1890 } 1891 1892 /** 1893 * @return {@link #diagnosis} (The diagnosis.) 1894 */ 1895 public Type getDiagnosis() { 1896 return this.diagnosis; 1897 } 1898 1899 /** 1900 * @return {@link #diagnosis} (The diagnosis.) 1901 */ 1902 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1903 if (this.diagnosis == null) 1904 return null; 1905 if (!(this.diagnosis instanceof CodeableConcept)) 1906 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1907 return (CodeableConcept) this.diagnosis; 1908 } 1909 1910 public boolean hasDiagnosisCodeableConcept() { 1911 return this.diagnosis instanceof CodeableConcept; 1912 } 1913 1914 /** 1915 * @return {@link #diagnosis} (The diagnosis.) 1916 */ 1917 public Reference getDiagnosisReference() throws FHIRException { 1918 if (this.diagnosis == null) 1919 return null; 1920 if (!(this.diagnosis instanceof Reference)) 1921 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1922 return (Reference) this.diagnosis; 1923 } 1924 1925 public boolean hasDiagnosisReference() { 1926 return this.diagnosis instanceof Reference; 1927 } 1928 1929 public boolean hasDiagnosis() { 1930 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #diagnosis} (The diagnosis.) 1935 */ 1936 public DiagnosisComponent setDiagnosis(Type value) throws FHIRFormatError { 1937 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1938 throw new FHIRFormatError("Not the right type for Claim.diagnosis.diagnosis[x]: "+value.fhirType()); 1939 this.diagnosis = value; 1940 return this; 1941 } 1942 1943 /** 1944 * @return {@link #type} (The type of the Diagnosis, for example: admitting, primary, secondary, discharge.) 1945 */ 1946 public List<CodeableConcept> getType() { 1947 if (this.type == null) 1948 this.type = new ArrayList<CodeableConcept>(); 1949 return this.type; 1950 } 1951 1952 /** 1953 * @return Returns a reference to <code>this</code> for easy method chaining 1954 */ 1955 public DiagnosisComponent setType(List<CodeableConcept> theType) { 1956 this.type = theType; 1957 return this; 1958 } 1959 1960 public boolean hasType() { 1961 if (this.type == null) 1962 return false; 1963 for (CodeableConcept item : this.type) 1964 if (!item.isEmpty()) 1965 return true; 1966 return false; 1967 } 1968 1969 public CodeableConcept addType() { //3 1970 CodeableConcept t = new CodeableConcept(); 1971 if (this.type == null) 1972 this.type = new ArrayList<CodeableConcept>(); 1973 this.type.add(t); 1974 return t; 1975 } 1976 1977 public DiagnosisComponent addType(CodeableConcept t) { //3 1978 if (t == null) 1979 return this; 1980 if (this.type == null) 1981 this.type = new ArrayList<CodeableConcept>(); 1982 this.type.add(t); 1983 return this; 1984 } 1985 1986 /** 1987 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 1988 */ 1989 public CodeableConcept getTypeFirstRep() { 1990 if (getType().isEmpty()) { 1991 addType(); 1992 } 1993 return getType().get(0); 1994 } 1995 1996 /** 1997 * @return {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 1998 */ 1999 public CodeableConcept getPackageCode() { 2000 if (this.packageCode == null) 2001 if (Configuration.errorOnAutoCreate()) 2002 throw new Error("Attempt to auto-create DiagnosisComponent.packageCode"); 2003 else if (Configuration.doAutoCreate()) 2004 this.packageCode = new CodeableConcept(); // cc 2005 return this.packageCode; 2006 } 2007 2008 public boolean hasPackageCode() { 2009 return this.packageCode != null && !this.packageCode.isEmpty(); 2010 } 2011 2012 /** 2013 * @param value {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 2014 */ 2015 public DiagnosisComponent setPackageCode(CodeableConcept value) { 2016 this.packageCode = value; 2017 return this; 2018 } 2019 2020 protected void listChildren(List<Property> children) { 2021 super.listChildren(children); 2022 children.add(new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence)); 2023 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis)); 2024 children.add(new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type)); 2025 children.add(new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode)); 2026 } 2027 2028 @Override 2029 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2030 switch (_hash) { 2031 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence); 2032 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2033 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2034 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2035 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 2036 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type); 2037 case 908444499: /*packageCode*/ return new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode); 2038 default: return super.getNamedProperty(_hash, _name, _checkValid); 2039 } 2040 2041 } 2042 2043 @Override 2044 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2045 switch (hash) { 2046 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2047 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // Type 2048 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2049 case 908444499: /*packageCode*/ return this.packageCode == null ? new Base[0] : new Base[] {this.packageCode}; // CodeableConcept 2050 default: return super.getProperty(hash, name, checkValid); 2051 } 2052 2053 } 2054 2055 @Override 2056 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2057 switch (hash) { 2058 case 1349547969: // sequence 2059 this.sequence = castToPositiveInt(value); // PositiveIntType 2060 return value; 2061 case 1196993265: // diagnosis 2062 this.diagnosis = castToType(value); // Type 2063 return value; 2064 case 3575610: // type 2065 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2066 return value; 2067 case 908444499: // packageCode 2068 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2069 return value; 2070 default: return super.setProperty(hash, name, value); 2071 } 2072 2073 } 2074 2075 @Override 2076 public Base setProperty(String name, Base value) throws FHIRException { 2077 if (name.equals("sequence")) { 2078 this.sequence = castToPositiveInt(value); // PositiveIntType 2079 } else if (name.equals("diagnosis[x]")) { 2080 this.diagnosis = castToType(value); // Type 2081 } else if (name.equals("type")) { 2082 this.getType().add(castToCodeableConcept(value)); 2083 } else if (name.equals("packageCode")) { 2084 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2085 } else 2086 return super.setProperty(name, value); 2087 return value; 2088 } 2089 2090 @Override 2091 public Base makeProperty(int hash, String name) throws FHIRException { 2092 switch (hash) { 2093 case 1349547969: return getSequenceElement(); 2094 case -1487009809: return getDiagnosis(); 2095 case 1196993265: return getDiagnosis(); 2096 case 3575610: return addType(); 2097 case 908444499: return getPackageCode(); 2098 default: return super.makeProperty(hash, name); 2099 } 2100 2101 } 2102 2103 @Override 2104 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2105 switch (hash) { 2106 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2107 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 2108 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2109 case 908444499: /*packageCode*/ return new String[] {"CodeableConcept"}; 2110 default: return super.getTypesForProperty(hash, name); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base addChild(String name) throws FHIRException { 2117 if (name.equals("sequence")) { 2118 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2119 } 2120 else if (name.equals("diagnosisCodeableConcept")) { 2121 this.diagnosis = new CodeableConcept(); 2122 return this.diagnosis; 2123 } 2124 else if (name.equals("diagnosisReference")) { 2125 this.diagnosis = new Reference(); 2126 return this.diagnosis; 2127 } 2128 else if (name.equals("type")) { 2129 return addType(); 2130 } 2131 else if (name.equals("packageCode")) { 2132 this.packageCode = new CodeableConcept(); 2133 return this.packageCode; 2134 } 2135 else 2136 return super.addChild(name); 2137 } 2138 2139 public DiagnosisComponent copy() { 2140 DiagnosisComponent dst = new DiagnosisComponent(); 2141 copyValues(dst); 2142 dst.sequence = sequence == null ? null : sequence.copy(); 2143 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2144 if (type != null) { 2145 dst.type = new ArrayList<CodeableConcept>(); 2146 for (CodeableConcept i : type) 2147 dst.type.add(i.copy()); 2148 }; 2149 dst.packageCode = packageCode == null ? null : packageCode.copy(); 2150 return dst; 2151 } 2152 2153 @Override 2154 public boolean equalsDeep(Base other_) { 2155 if (!super.equalsDeep(other_)) 2156 return false; 2157 if (!(other_ instanceof DiagnosisComponent)) 2158 return false; 2159 DiagnosisComponent o = (DiagnosisComponent) other_; 2160 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2161 && compareDeep(packageCode, o.packageCode, true); 2162 } 2163 2164 @Override 2165 public boolean equalsShallow(Base other_) { 2166 if (!super.equalsShallow(other_)) 2167 return false; 2168 if (!(other_ instanceof DiagnosisComponent)) 2169 return false; 2170 DiagnosisComponent o = (DiagnosisComponent) other_; 2171 return compareValues(sequence, o.sequence, true); 2172 } 2173 2174 public boolean isEmpty() { 2175 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2176 , packageCode); 2177 } 2178 2179 public String fhirType() { 2180 return "Claim.diagnosis"; 2181 2182 } 2183 2184 } 2185 2186 @Block() 2187 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2188 /** 2189 * Sequence of procedures which serves to order and provide a link. 2190 */ 2191 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2192 @Description(shortDefinition="Procedure sequence for reference", formalDefinition="Sequence of procedures which serves to order and provide a link." ) 2193 protected PositiveIntType sequence; 2194 2195 /** 2196 * Date and optionally time the procedure was performed . 2197 */ 2198 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2199 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed ." ) 2200 protected DateTimeType date; 2201 2202 /** 2203 * The procedure code. 2204 */ 2205 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=3, min=1, max=1, modifier=false, summary=false) 2206 @Description(shortDefinition="Patient's list of procedures performed", formalDefinition="The procedure code." ) 2207 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2208 protected Type procedure; 2209 2210 private static final long serialVersionUID = 864307347L; 2211 2212 /** 2213 * Constructor 2214 */ 2215 public ProcedureComponent() { 2216 super(); 2217 } 2218 2219 /** 2220 * Constructor 2221 */ 2222 public ProcedureComponent(PositiveIntType sequence, Type procedure) { 2223 super(); 2224 this.sequence = sequence; 2225 this.procedure = procedure; 2226 } 2227 2228 /** 2229 * @return {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2230 */ 2231 public PositiveIntType getSequenceElement() { 2232 if (this.sequence == null) 2233 if (Configuration.errorOnAutoCreate()) 2234 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2235 else if (Configuration.doAutoCreate()) 2236 this.sequence = new PositiveIntType(); // bb 2237 return this.sequence; 2238 } 2239 2240 public boolean hasSequenceElement() { 2241 return this.sequence != null && !this.sequence.isEmpty(); 2242 } 2243 2244 public boolean hasSequence() { 2245 return this.sequence != null && !this.sequence.isEmpty(); 2246 } 2247 2248 /** 2249 * @param value {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2250 */ 2251 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2252 this.sequence = value; 2253 return this; 2254 } 2255 2256 /** 2257 * @return Sequence of procedures which serves to order and provide a link. 2258 */ 2259 public int getSequence() { 2260 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2261 } 2262 2263 /** 2264 * @param value Sequence of procedures which serves to order and provide a link. 2265 */ 2266 public ProcedureComponent setSequence(int value) { 2267 if (this.sequence == null) 2268 this.sequence = new PositiveIntType(); 2269 this.sequence.setValue(value); 2270 return this; 2271 } 2272 2273 /** 2274 * @return {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2275 */ 2276 public DateTimeType getDateElement() { 2277 if (this.date == null) 2278 if (Configuration.errorOnAutoCreate()) 2279 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2280 else if (Configuration.doAutoCreate()) 2281 this.date = new DateTimeType(); // bb 2282 return this.date; 2283 } 2284 2285 public boolean hasDateElement() { 2286 return this.date != null && !this.date.isEmpty(); 2287 } 2288 2289 public boolean hasDate() { 2290 return this.date != null && !this.date.isEmpty(); 2291 } 2292 2293 /** 2294 * @param value {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2295 */ 2296 public ProcedureComponent setDateElement(DateTimeType value) { 2297 this.date = value; 2298 return this; 2299 } 2300 2301 /** 2302 * @return Date and optionally time the procedure was performed . 2303 */ 2304 public Date getDate() { 2305 return this.date == null ? null : this.date.getValue(); 2306 } 2307 2308 /** 2309 * @param value Date and optionally time the procedure was performed . 2310 */ 2311 public ProcedureComponent setDate(Date value) { 2312 if (value == null) 2313 this.date = null; 2314 else { 2315 if (this.date == null) 2316 this.date = new DateTimeType(); 2317 this.date.setValue(value); 2318 } 2319 return this; 2320 } 2321 2322 /** 2323 * @return {@link #procedure} (The procedure code.) 2324 */ 2325 public Type getProcedure() { 2326 return this.procedure; 2327 } 2328 2329 /** 2330 * @return {@link #procedure} (The procedure code.) 2331 */ 2332 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2333 if (this.procedure == null) 2334 return null; 2335 if (!(this.procedure instanceof CodeableConcept)) 2336 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2337 return (CodeableConcept) this.procedure; 2338 } 2339 2340 public boolean hasProcedureCodeableConcept() { 2341 return this.procedure instanceof CodeableConcept; 2342 } 2343 2344 /** 2345 * @return {@link #procedure} (The procedure code.) 2346 */ 2347 public Reference getProcedureReference() throws FHIRException { 2348 if (this.procedure == null) 2349 return null; 2350 if (!(this.procedure instanceof Reference)) 2351 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2352 return (Reference) this.procedure; 2353 } 2354 2355 public boolean hasProcedureReference() { 2356 return this.procedure instanceof Reference; 2357 } 2358 2359 public boolean hasProcedure() { 2360 return this.procedure != null && !this.procedure.isEmpty(); 2361 } 2362 2363 /** 2364 * @param value {@link #procedure} (The procedure code.) 2365 */ 2366 public ProcedureComponent setProcedure(Type value) throws FHIRFormatError { 2367 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2368 throw new FHIRFormatError("Not the right type for Claim.procedure.procedure[x]: "+value.fhirType()); 2369 this.procedure = value; 2370 return this; 2371 } 2372 2373 protected void listChildren(List<Property> children) { 2374 super.listChildren(children); 2375 children.add(new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence)); 2376 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date)); 2377 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure)); 2378 } 2379 2380 @Override 2381 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2382 switch (_hash) { 2383 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence); 2384 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date); 2385 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2386 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2387 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2388 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2389 default: return super.getNamedProperty(_hash, _name, _checkValid); 2390 } 2391 2392 } 2393 2394 @Override 2395 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2396 switch (hash) { 2397 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2398 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2399 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // Type 2400 default: return super.getProperty(hash, name, checkValid); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2407 switch (hash) { 2408 case 1349547969: // sequence 2409 this.sequence = castToPositiveInt(value); // PositiveIntType 2410 return value; 2411 case 3076014: // date 2412 this.date = castToDateTime(value); // DateTimeType 2413 return value; 2414 case -1095204141: // procedure 2415 this.procedure = castToType(value); // Type 2416 return value; 2417 default: return super.setProperty(hash, name, value); 2418 } 2419 2420 } 2421 2422 @Override 2423 public Base setProperty(String name, Base value) throws FHIRException { 2424 if (name.equals("sequence")) { 2425 this.sequence = castToPositiveInt(value); // PositiveIntType 2426 } else if (name.equals("date")) { 2427 this.date = castToDateTime(value); // DateTimeType 2428 } else if (name.equals("procedure[x]")) { 2429 this.procedure = castToType(value); // Type 2430 } else 2431 return super.setProperty(name, value); 2432 return value; 2433 } 2434 2435 @Override 2436 public Base makeProperty(int hash, String name) throws FHIRException { 2437 switch (hash) { 2438 case 1349547969: return getSequenceElement(); 2439 case 3076014: return getDateElement(); 2440 case 1640074445: return getProcedure(); 2441 case -1095204141: return getProcedure(); 2442 default: return super.makeProperty(hash, name); 2443 } 2444 2445 } 2446 2447 @Override 2448 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2449 switch (hash) { 2450 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2451 case 3076014: /*date*/ return new String[] {"dateTime"}; 2452 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2453 default: return super.getTypesForProperty(hash, name); 2454 } 2455 2456 } 2457 2458 @Override 2459 public Base addChild(String name) throws FHIRException { 2460 if (name.equals("sequence")) { 2461 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2462 } 2463 else if (name.equals("date")) { 2464 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 2465 } 2466 else if (name.equals("procedureCodeableConcept")) { 2467 this.procedure = new CodeableConcept(); 2468 return this.procedure; 2469 } 2470 else if (name.equals("procedureReference")) { 2471 this.procedure = new Reference(); 2472 return this.procedure; 2473 } 2474 else 2475 return super.addChild(name); 2476 } 2477 2478 public ProcedureComponent copy() { 2479 ProcedureComponent dst = new ProcedureComponent(); 2480 copyValues(dst); 2481 dst.sequence = sequence == null ? null : sequence.copy(); 2482 dst.date = date == null ? null : date.copy(); 2483 dst.procedure = procedure == null ? null : procedure.copy(); 2484 return dst; 2485 } 2486 2487 @Override 2488 public boolean equalsDeep(Base other_) { 2489 if (!super.equalsDeep(other_)) 2490 return false; 2491 if (!(other_ instanceof ProcedureComponent)) 2492 return false; 2493 ProcedureComponent o = (ProcedureComponent) other_; 2494 return compareDeep(sequence, o.sequence, true) && compareDeep(date, o.date, true) && compareDeep(procedure, o.procedure, true) 2495 ; 2496 } 2497 2498 @Override 2499 public boolean equalsShallow(Base other_) { 2500 if (!super.equalsShallow(other_)) 2501 return false; 2502 if (!(other_ instanceof ProcedureComponent)) 2503 return false; 2504 ProcedureComponent o = (ProcedureComponent) other_; 2505 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2506 } 2507 2508 public boolean isEmpty() { 2509 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, date, procedure 2510 ); 2511 } 2512 2513 public String fhirType() { 2514 return "Claim.procedure"; 2515 2516 } 2517 2518 } 2519 2520 @Block() 2521 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2522 /** 2523 * Sequence of coverage which serves to provide a link and convey coordination of benefit order. 2524 */ 2525 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2526 @Description(shortDefinition="Service instance identifier", formalDefinition="Sequence of coverage which serves to provide a link and convey coordination of benefit order." ) 2527 protected PositiveIntType sequence; 2528 2529 /** 2530 * A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 2531 */ 2532 @Child(name = "focal", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2533 @Description(shortDefinition="Is the focal Coverage", formalDefinition="A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated." ) 2534 protected BooleanType focal; 2535 2536 /** 2537 * Reference to the program or plan identification, underwriter or payor. 2538 */ 2539 @Child(name = "coverage", type = {Coverage.class}, order=3, min=1, max=1, modifier=false, summary=false) 2540 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the program or plan identification, underwriter or payor." ) 2541 protected Reference coverage; 2542 2543 /** 2544 * The actual object that is the target of the reference (Reference to the program or plan identification, underwriter or payor.) 2545 */ 2546 protected Coverage coverageTarget; 2547 2548 /** 2549 * The contract number of a business agreement which describes the terms and conditions. 2550 */ 2551 @Child(name = "businessArrangement", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2552 @Description(shortDefinition="Business agreement", formalDefinition="The contract number of a business agreement which describes the terms and conditions." ) 2553 protected StringType businessArrangement; 2554 2555 /** 2556 * A list of references from the Insurer to which these services pertain. 2557 */ 2558 @Child(name = "preAuthRef", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2559 @Description(shortDefinition="Pre-Authorization/Determination Reference", formalDefinition="A list of references from the Insurer to which these services pertain." ) 2560 protected List<StringType> preAuthRef; 2561 2562 /** 2563 * The Coverages adjudication details. 2564 */ 2565 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=6, min=0, max=1, modifier=false, summary=false) 2566 @Description(shortDefinition="Adjudication results", formalDefinition="The Coverages adjudication details." ) 2567 protected Reference claimResponse; 2568 2569 /** 2570 * The actual object that is the target of the reference (The Coverages adjudication details.) 2571 */ 2572 protected ClaimResponse claimResponseTarget; 2573 2574 private static final long serialVersionUID = -1216535489L; 2575 2576 /** 2577 * Constructor 2578 */ 2579 public InsuranceComponent() { 2580 super(); 2581 } 2582 2583 /** 2584 * Constructor 2585 */ 2586 public InsuranceComponent(PositiveIntType sequence, BooleanType focal, Reference coverage) { 2587 super(); 2588 this.sequence = sequence; 2589 this.focal = focal; 2590 this.coverage = coverage; 2591 } 2592 2593 /** 2594 * @return {@link #sequence} (Sequence of coverage which serves to provide a link and convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2595 */ 2596 public PositiveIntType getSequenceElement() { 2597 if (this.sequence == null) 2598 if (Configuration.errorOnAutoCreate()) 2599 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 2600 else if (Configuration.doAutoCreate()) 2601 this.sequence = new PositiveIntType(); // bb 2602 return this.sequence; 2603 } 2604 2605 public boolean hasSequenceElement() { 2606 return this.sequence != null && !this.sequence.isEmpty(); 2607 } 2608 2609 public boolean hasSequence() { 2610 return this.sequence != null && !this.sequence.isEmpty(); 2611 } 2612 2613 /** 2614 * @param value {@link #sequence} (Sequence of coverage which serves to provide a link and convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2615 */ 2616 public InsuranceComponent setSequenceElement(PositiveIntType value) { 2617 this.sequence = value; 2618 return this; 2619 } 2620 2621 /** 2622 * @return Sequence of coverage which serves to provide a link and convey coordination of benefit order. 2623 */ 2624 public int getSequence() { 2625 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2626 } 2627 2628 /** 2629 * @param value Sequence of coverage which serves to provide a link and convey coordination of benefit order. 2630 */ 2631 public InsuranceComponent setSequence(int value) { 2632 if (this.sequence == null) 2633 this.sequence = new PositiveIntType(); 2634 this.sequence.setValue(value); 2635 return this; 2636 } 2637 2638 /** 2639 * @return {@link #focal} (A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2640 */ 2641 public BooleanType getFocalElement() { 2642 if (this.focal == null) 2643 if (Configuration.errorOnAutoCreate()) 2644 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 2645 else if (Configuration.doAutoCreate()) 2646 this.focal = new BooleanType(); // bb 2647 return this.focal; 2648 } 2649 2650 public boolean hasFocalElement() { 2651 return this.focal != null && !this.focal.isEmpty(); 2652 } 2653 2654 public boolean hasFocal() { 2655 return this.focal != null && !this.focal.isEmpty(); 2656 } 2657 2658 /** 2659 * @param value {@link #focal} (A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2660 */ 2661 public InsuranceComponent setFocalElement(BooleanType value) { 2662 this.focal = value; 2663 return this; 2664 } 2665 2666 /** 2667 * @return A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 2668 */ 2669 public boolean getFocal() { 2670 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 2671 } 2672 2673 /** 2674 * @param value A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 2675 */ 2676 public InsuranceComponent setFocal(boolean value) { 2677 if (this.focal == null) 2678 this.focal = new BooleanType(); 2679 this.focal.setValue(value); 2680 return this; 2681 } 2682 2683 /** 2684 * @return {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2685 */ 2686 public Reference getCoverage() { 2687 if (this.coverage == null) 2688 if (Configuration.errorOnAutoCreate()) 2689 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2690 else if (Configuration.doAutoCreate()) 2691 this.coverage = new Reference(); // cc 2692 return this.coverage; 2693 } 2694 2695 public boolean hasCoverage() { 2696 return this.coverage != null && !this.coverage.isEmpty(); 2697 } 2698 2699 /** 2700 * @param value {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2701 */ 2702 public InsuranceComponent setCoverage(Reference value) { 2703 this.coverage = value; 2704 return this; 2705 } 2706 2707 /** 2708 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2709 */ 2710 public Coverage getCoverageTarget() { 2711 if (this.coverageTarget == null) 2712 if (Configuration.errorOnAutoCreate()) 2713 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2714 else if (Configuration.doAutoCreate()) 2715 this.coverageTarget = new Coverage(); // aa 2716 return this.coverageTarget; 2717 } 2718 2719 /** 2720 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2721 */ 2722 public InsuranceComponent setCoverageTarget(Coverage value) { 2723 this.coverageTarget = value; 2724 return this; 2725 } 2726 2727 /** 2728 * @return {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2729 */ 2730 public StringType getBusinessArrangementElement() { 2731 if (this.businessArrangement == null) 2732 if (Configuration.errorOnAutoCreate()) 2733 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 2734 else if (Configuration.doAutoCreate()) 2735 this.businessArrangement = new StringType(); // bb 2736 return this.businessArrangement; 2737 } 2738 2739 public boolean hasBusinessArrangementElement() { 2740 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2741 } 2742 2743 public boolean hasBusinessArrangement() { 2744 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2745 } 2746 2747 /** 2748 * @param value {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2749 */ 2750 public InsuranceComponent setBusinessArrangementElement(StringType value) { 2751 this.businessArrangement = value; 2752 return this; 2753 } 2754 2755 /** 2756 * @return The contract number of a business agreement which describes the terms and conditions. 2757 */ 2758 public String getBusinessArrangement() { 2759 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 2760 } 2761 2762 /** 2763 * @param value The contract number of a business agreement which describes the terms and conditions. 2764 */ 2765 public InsuranceComponent setBusinessArrangement(String value) { 2766 if (Utilities.noString(value)) 2767 this.businessArrangement = null; 2768 else { 2769 if (this.businessArrangement == null) 2770 this.businessArrangement = new StringType(); 2771 this.businessArrangement.setValue(value); 2772 } 2773 return this; 2774 } 2775 2776 /** 2777 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2778 */ 2779 public List<StringType> getPreAuthRef() { 2780 if (this.preAuthRef == null) 2781 this.preAuthRef = new ArrayList<StringType>(); 2782 return this.preAuthRef; 2783 } 2784 2785 /** 2786 * @return Returns a reference to <code>this</code> for easy method chaining 2787 */ 2788 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2789 this.preAuthRef = thePreAuthRef; 2790 return this; 2791 } 2792 2793 public boolean hasPreAuthRef() { 2794 if (this.preAuthRef == null) 2795 return false; 2796 for (StringType item : this.preAuthRef) 2797 if (!item.isEmpty()) 2798 return true; 2799 return false; 2800 } 2801 2802 /** 2803 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2804 */ 2805 public StringType addPreAuthRefElement() {//2 2806 StringType t = new StringType(); 2807 if (this.preAuthRef == null) 2808 this.preAuthRef = new ArrayList<StringType>(); 2809 this.preAuthRef.add(t); 2810 return t; 2811 } 2812 2813 /** 2814 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2815 */ 2816 public InsuranceComponent addPreAuthRef(String value) { //1 2817 StringType t = new StringType(); 2818 t.setValue(value); 2819 if (this.preAuthRef == null) 2820 this.preAuthRef = new ArrayList<StringType>(); 2821 this.preAuthRef.add(t); 2822 return this; 2823 } 2824 2825 /** 2826 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2827 */ 2828 public boolean hasPreAuthRef(String value) { 2829 if (this.preAuthRef == null) 2830 return false; 2831 for (StringType v : this.preAuthRef) 2832 if (v.getValue().equals(value)) // string 2833 return true; 2834 return false; 2835 } 2836 2837 /** 2838 * @return {@link #claimResponse} (The Coverages adjudication details.) 2839 */ 2840 public Reference getClaimResponse() { 2841 if (this.claimResponse == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 2844 else if (Configuration.doAutoCreate()) 2845 this.claimResponse = new Reference(); // cc 2846 return this.claimResponse; 2847 } 2848 2849 public boolean hasClaimResponse() { 2850 return this.claimResponse != null && !this.claimResponse.isEmpty(); 2851 } 2852 2853 /** 2854 * @param value {@link #claimResponse} (The Coverages adjudication details.) 2855 */ 2856 public InsuranceComponent setClaimResponse(Reference value) { 2857 this.claimResponse = value; 2858 return this; 2859 } 2860 2861 /** 2862 * @return {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Coverages adjudication details.) 2863 */ 2864 public ClaimResponse getClaimResponseTarget() { 2865 if (this.claimResponseTarget == null) 2866 if (Configuration.errorOnAutoCreate()) 2867 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 2868 else if (Configuration.doAutoCreate()) 2869 this.claimResponseTarget = new ClaimResponse(); // aa 2870 return this.claimResponseTarget; 2871 } 2872 2873 /** 2874 * @param value {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Coverages adjudication details.) 2875 */ 2876 public InsuranceComponent setClaimResponseTarget(ClaimResponse value) { 2877 this.claimResponseTarget = value; 2878 return this; 2879 } 2880 2881 protected void listChildren(List<Property> children) { 2882 super.listChildren(children); 2883 children.add(new Property("sequence", "positiveInt", "Sequence of coverage which serves to provide a link and convey coordination of benefit order.", 0, 1, sequence)); 2884 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 0, 1, focal)); 2885 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 2886 children.add(new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement)); 2887 children.add(new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 2888 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 0, 1, claimResponse)); 2889 } 2890 2891 @Override 2892 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2893 switch (_hash) { 2894 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of coverage which serves to provide a link and convey coordination of benefit order.", 0, 1, sequence); 2895 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 0, 1, focal); 2896 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 2897 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement); 2898 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 2899 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 0, 1, claimResponse); 2900 default: return super.getNamedProperty(_hash, _name, _checkValid); 2901 } 2902 2903 } 2904 2905 @Override 2906 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2907 switch (hash) { 2908 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2909 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 2910 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 2911 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 2912 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 2913 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 2914 default: return super.getProperty(hash, name, checkValid); 2915 } 2916 2917 } 2918 2919 @Override 2920 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2921 switch (hash) { 2922 case 1349547969: // sequence 2923 this.sequence = castToPositiveInt(value); // PositiveIntType 2924 return value; 2925 case 97604197: // focal 2926 this.focal = castToBoolean(value); // BooleanType 2927 return value; 2928 case -351767064: // coverage 2929 this.coverage = castToReference(value); // Reference 2930 return value; 2931 case 259920682: // businessArrangement 2932 this.businessArrangement = castToString(value); // StringType 2933 return value; 2934 case 522246568: // preAuthRef 2935 this.getPreAuthRef().add(castToString(value)); // StringType 2936 return value; 2937 case 689513629: // claimResponse 2938 this.claimResponse = castToReference(value); // Reference 2939 return value; 2940 default: return super.setProperty(hash, name, value); 2941 } 2942 2943 } 2944 2945 @Override 2946 public Base setProperty(String name, Base value) throws FHIRException { 2947 if (name.equals("sequence")) { 2948 this.sequence = castToPositiveInt(value); // PositiveIntType 2949 } else if (name.equals("focal")) { 2950 this.focal = castToBoolean(value); // BooleanType 2951 } else if (name.equals("coverage")) { 2952 this.coverage = castToReference(value); // Reference 2953 } else if (name.equals("businessArrangement")) { 2954 this.businessArrangement = castToString(value); // StringType 2955 } else if (name.equals("preAuthRef")) { 2956 this.getPreAuthRef().add(castToString(value)); 2957 } else if (name.equals("claimResponse")) { 2958 this.claimResponse = castToReference(value); // Reference 2959 } else 2960 return super.setProperty(name, value); 2961 return value; 2962 } 2963 2964 @Override 2965 public Base makeProperty(int hash, String name) throws FHIRException { 2966 switch (hash) { 2967 case 1349547969: return getSequenceElement(); 2968 case 97604197: return getFocalElement(); 2969 case -351767064: return getCoverage(); 2970 case 259920682: return getBusinessArrangementElement(); 2971 case 522246568: return addPreAuthRefElement(); 2972 case 689513629: return getClaimResponse(); 2973 default: return super.makeProperty(hash, name); 2974 } 2975 2976 } 2977 2978 @Override 2979 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2980 switch (hash) { 2981 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2982 case 97604197: /*focal*/ return new String[] {"boolean"}; 2983 case -351767064: /*coverage*/ return new String[] {"Reference"}; 2984 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 2985 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 2986 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 2987 default: return super.getTypesForProperty(hash, name); 2988 } 2989 2990 } 2991 2992 @Override 2993 public Base addChild(String name) throws FHIRException { 2994 if (name.equals("sequence")) { 2995 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2996 } 2997 else if (name.equals("focal")) { 2998 throw new FHIRException("Cannot call addChild on a singleton property Claim.focal"); 2999 } 3000 else if (name.equals("coverage")) { 3001 this.coverage = new Reference(); 3002 return this.coverage; 3003 } 3004 else if (name.equals("businessArrangement")) { 3005 throw new FHIRException("Cannot call addChild on a singleton property Claim.businessArrangement"); 3006 } 3007 else if (name.equals("preAuthRef")) { 3008 throw new FHIRException("Cannot call addChild on a singleton property Claim.preAuthRef"); 3009 } 3010 else if (name.equals("claimResponse")) { 3011 this.claimResponse = new Reference(); 3012 return this.claimResponse; 3013 } 3014 else 3015 return super.addChild(name); 3016 } 3017 3018 public InsuranceComponent copy() { 3019 InsuranceComponent dst = new InsuranceComponent(); 3020 copyValues(dst); 3021 dst.sequence = sequence == null ? null : sequence.copy(); 3022 dst.focal = focal == null ? null : focal.copy(); 3023 dst.coverage = coverage == null ? null : coverage.copy(); 3024 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 3025 if (preAuthRef != null) { 3026 dst.preAuthRef = new ArrayList<StringType>(); 3027 for (StringType i : preAuthRef) 3028 dst.preAuthRef.add(i.copy()); 3029 }; 3030 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 3031 return dst; 3032 } 3033 3034 @Override 3035 public boolean equalsDeep(Base other_) { 3036 if (!super.equalsDeep(other_)) 3037 return false; 3038 if (!(other_ instanceof InsuranceComponent)) 3039 return false; 3040 InsuranceComponent o = (InsuranceComponent) other_; 3041 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) 3042 && compareDeep(businessArrangement, o.businessArrangement, true) && compareDeep(preAuthRef, o.preAuthRef, true) 3043 && compareDeep(claimResponse, o.claimResponse, true); 3044 } 3045 3046 @Override 3047 public boolean equalsShallow(Base other_) { 3048 if (!super.equalsShallow(other_)) 3049 return false; 3050 if (!(other_ instanceof InsuranceComponent)) 3051 return false; 3052 InsuranceComponent o = (InsuranceComponent) other_; 3053 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true) 3054 && compareValues(preAuthRef, o.preAuthRef, true); 3055 } 3056 3057 public boolean isEmpty() { 3058 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, coverage 3059 , businessArrangement, preAuthRef, claimResponse); 3060 } 3061 3062 public String fhirType() { 3063 return "Claim.insurance"; 3064 3065 } 3066 3067 } 3068 3069 @Block() 3070 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 3071 /** 3072 * Date of an accident which these services are addressing. 3073 */ 3074 @Child(name = "date", type = {DateType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3075 @Description(shortDefinition="When the accident occurred\nsee information codes\nsee information codes", formalDefinition="Date of an accident which these services are addressing." ) 3076 protected DateType date; 3077 3078 /** 3079 * Type of accident: work, auto, etc. 3080 */ 3081 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3082 @Description(shortDefinition="The nature of the accident", formalDefinition="Type of accident: work, auto, etc." ) 3083 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActIncidentCode") 3084 protected CodeableConcept type; 3085 3086 /** 3087 * Accident Place. 3088 */ 3089 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 3090 @Description(shortDefinition="Accident Place", formalDefinition="Accident Place." ) 3091 protected Type location; 3092 3093 private static final long serialVersionUID = 622904984L; 3094 3095 /** 3096 * Constructor 3097 */ 3098 public AccidentComponent() { 3099 super(); 3100 } 3101 3102 /** 3103 * Constructor 3104 */ 3105 public AccidentComponent(DateType date) { 3106 super(); 3107 this.date = date; 3108 } 3109 3110 /** 3111 * @return {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3112 */ 3113 public DateType getDateElement() { 3114 if (this.date == null) 3115 if (Configuration.errorOnAutoCreate()) 3116 throw new Error("Attempt to auto-create AccidentComponent.date"); 3117 else if (Configuration.doAutoCreate()) 3118 this.date = new DateType(); // bb 3119 return this.date; 3120 } 3121 3122 public boolean hasDateElement() { 3123 return this.date != null && !this.date.isEmpty(); 3124 } 3125 3126 public boolean hasDate() { 3127 return this.date != null && !this.date.isEmpty(); 3128 } 3129 3130 /** 3131 * @param value {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3132 */ 3133 public AccidentComponent setDateElement(DateType value) { 3134 this.date = value; 3135 return this; 3136 } 3137 3138 /** 3139 * @return Date of an accident which these services are addressing. 3140 */ 3141 public Date getDate() { 3142 return this.date == null ? null : this.date.getValue(); 3143 } 3144 3145 /** 3146 * @param value Date of an accident which these services are addressing. 3147 */ 3148 public AccidentComponent setDate(Date value) { 3149 if (this.date == null) 3150 this.date = new DateType(); 3151 this.date.setValue(value); 3152 return this; 3153 } 3154 3155 /** 3156 * @return {@link #type} (Type of accident: work, auto, etc.) 3157 */ 3158 public CodeableConcept getType() { 3159 if (this.type == null) 3160 if (Configuration.errorOnAutoCreate()) 3161 throw new Error("Attempt to auto-create AccidentComponent.type"); 3162 else if (Configuration.doAutoCreate()) 3163 this.type = new CodeableConcept(); // cc 3164 return this.type; 3165 } 3166 3167 public boolean hasType() { 3168 return this.type != null && !this.type.isEmpty(); 3169 } 3170 3171 /** 3172 * @param value {@link #type} (Type of accident: work, auto, etc.) 3173 */ 3174 public AccidentComponent setType(CodeableConcept value) { 3175 this.type = value; 3176 return this; 3177 } 3178 3179 /** 3180 * @return {@link #location} (Accident Place.) 3181 */ 3182 public Type getLocation() { 3183 return this.location; 3184 } 3185 3186 /** 3187 * @return {@link #location} (Accident Place.) 3188 */ 3189 public Address getLocationAddress() throws FHIRException { 3190 if (this.location == null) 3191 return null; 3192 if (!(this.location instanceof Address)) 3193 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3194 return (Address) this.location; 3195 } 3196 3197 public boolean hasLocationAddress() { 3198 return this.location instanceof Address; 3199 } 3200 3201 /** 3202 * @return {@link #location} (Accident Place.) 3203 */ 3204 public Reference getLocationReference() throws FHIRException { 3205 if (this.location == null) 3206 return null; 3207 if (!(this.location instanceof Reference)) 3208 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3209 return (Reference) this.location; 3210 } 3211 3212 public boolean hasLocationReference() { 3213 return this.location instanceof Reference; 3214 } 3215 3216 public boolean hasLocation() { 3217 return this.location != null && !this.location.isEmpty(); 3218 } 3219 3220 /** 3221 * @param value {@link #location} (Accident Place.) 3222 */ 3223 public AccidentComponent setLocation(Type value) throws FHIRFormatError { 3224 if (value != null && !(value instanceof Address || value instanceof Reference)) 3225 throw new FHIRFormatError("Not the right type for Claim.accident.location[x]: "+value.fhirType()); 3226 this.location = value; 3227 return this; 3228 } 3229 3230 protected void listChildren(List<Property> children) { 3231 super.listChildren(children); 3232 children.add(new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date)); 3233 children.add(new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type)); 3234 children.add(new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location)); 3235 } 3236 3237 @Override 3238 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3239 switch (_hash) { 3240 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date); 3241 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type); 3242 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3243 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3244 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3245 case 755866390: /*locationReference*/ return new Property("location[x]", "Address|Reference(Location)", "Accident Place.", 0, 1, location); 3246 default: return super.getNamedProperty(_hash, _name, _checkValid); 3247 } 3248 3249 } 3250 3251 @Override 3252 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3253 switch (hash) { 3254 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3255 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3256 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 3257 default: return super.getProperty(hash, name, checkValid); 3258 } 3259 3260 } 3261 3262 @Override 3263 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3264 switch (hash) { 3265 case 3076014: // date 3266 this.date = castToDate(value); // DateType 3267 return value; 3268 case 3575610: // type 3269 this.type = castToCodeableConcept(value); // CodeableConcept 3270 return value; 3271 case 1901043637: // location 3272 this.location = castToType(value); // Type 3273 return value; 3274 default: return super.setProperty(hash, name, value); 3275 } 3276 3277 } 3278 3279 @Override 3280 public Base setProperty(String name, Base value) throws FHIRException { 3281 if (name.equals("date")) { 3282 this.date = castToDate(value); // DateType 3283 } else if (name.equals("type")) { 3284 this.type = castToCodeableConcept(value); // CodeableConcept 3285 } else if (name.equals("location[x]")) { 3286 this.location = castToType(value); // Type 3287 } else 3288 return super.setProperty(name, value); 3289 return value; 3290 } 3291 3292 @Override 3293 public Base makeProperty(int hash, String name) throws FHIRException { 3294 switch (hash) { 3295 case 3076014: return getDateElement(); 3296 case 3575610: return getType(); 3297 case 552316075: return getLocation(); 3298 case 1901043637: return getLocation(); 3299 default: return super.makeProperty(hash, name); 3300 } 3301 3302 } 3303 3304 @Override 3305 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3306 switch (hash) { 3307 case 3076014: /*date*/ return new String[] {"date"}; 3308 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3309 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 3310 default: return super.getTypesForProperty(hash, name); 3311 } 3312 3313 } 3314 3315 @Override 3316 public Base addChild(String name) throws FHIRException { 3317 if (name.equals("date")) { 3318 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 3319 } 3320 else if (name.equals("type")) { 3321 this.type = new CodeableConcept(); 3322 return this.type; 3323 } 3324 else if (name.equals("locationAddress")) { 3325 this.location = new Address(); 3326 return this.location; 3327 } 3328 else if (name.equals("locationReference")) { 3329 this.location = new Reference(); 3330 return this.location; 3331 } 3332 else 3333 return super.addChild(name); 3334 } 3335 3336 public AccidentComponent copy() { 3337 AccidentComponent dst = new AccidentComponent(); 3338 copyValues(dst); 3339 dst.date = date == null ? null : date.copy(); 3340 dst.type = type == null ? null : type.copy(); 3341 dst.location = location == null ? null : location.copy(); 3342 return dst; 3343 } 3344 3345 @Override 3346 public boolean equalsDeep(Base other_) { 3347 if (!super.equalsDeep(other_)) 3348 return false; 3349 if (!(other_ instanceof AccidentComponent)) 3350 return false; 3351 AccidentComponent o = (AccidentComponent) other_; 3352 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 3353 ; 3354 } 3355 3356 @Override 3357 public boolean equalsShallow(Base other_) { 3358 if (!super.equalsShallow(other_)) 3359 return false; 3360 if (!(other_ instanceof AccidentComponent)) 3361 return false; 3362 AccidentComponent o = (AccidentComponent) other_; 3363 return compareValues(date, o.date, true); 3364 } 3365 3366 public boolean isEmpty() { 3367 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 3368 } 3369 3370 public String fhirType() { 3371 return "Claim.accident"; 3372 3373 } 3374 3375 } 3376 3377 @Block() 3378 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 3379 /** 3380 * A service line number. 3381 */ 3382 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3383 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 3384 protected PositiveIntType sequence; 3385 3386 /** 3387 * CareTeam applicable for this service or product line. 3388 */ 3389 @Child(name = "careTeamLinkId", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3390 @Description(shortDefinition="Applicable careTeam members", formalDefinition="CareTeam applicable for this service or product line." ) 3391 protected List<PositiveIntType> careTeamLinkId; 3392 3393 /** 3394 * Diagnosis applicable for this service or product line. 3395 */ 3396 @Child(name = "diagnosisLinkId", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3397 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnosis applicable for this service or product line." ) 3398 protected List<PositiveIntType> diagnosisLinkId; 3399 3400 /** 3401 * Procedures applicable for this service or product line. 3402 */ 3403 @Child(name = "procedureLinkId", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3404 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product line." ) 3405 protected List<PositiveIntType> procedureLinkId; 3406 3407 /** 3408 * Exceptions, special conditions and supporting information pplicable for this service or product line. 3409 */ 3410 @Child(name = "informationLinkId", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3411 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information pplicable for this service or product line." ) 3412 protected List<PositiveIntType> informationLinkId; 3413 3414 /** 3415 * The type of reveneu or cost center providing the product and/or service. 3416 */ 3417 @Child(name = "revenue", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 3418 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 3419 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 3420 protected CodeableConcept revenue; 3421 3422 /** 3423 * Health Care Service Type Codes to identify the classification of service or benefits. 3424 */ 3425 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 3426 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 3427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 3428 protected CodeableConcept category; 3429 3430 /** 3431 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 3432 */ 3433 @Child(name = "service", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3434 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 3435 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3436 protected CodeableConcept service; 3437 3438 /** 3439 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 3440 */ 3441 @Child(name = "modifier", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3442 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 3443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3444 protected List<CodeableConcept> modifier; 3445 3446 /** 3447 * For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program. 3448 */ 3449 @Child(name = "programCode", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3450 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program." ) 3451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3452 protected List<CodeableConcept> programCode; 3453 3454 /** 3455 * The date or dates when the enclosed suite of services were performed or completed. 3456 */ 3457 @Child(name = "serviced", type = {DateType.class, Period.class}, order=11, min=0, max=1, modifier=false, summary=false) 3458 @Description(shortDefinition="Date or dates of Service", formalDefinition="The date or dates when the enclosed suite of services were performed or completed." ) 3459 protected Type serviced; 3460 3461 /** 3462 * Where the service was provided. 3463 */ 3464 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 3465 @Description(shortDefinition="Place of service", formalDefinition="Where the service was provided." ) 3466 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3467 protected Type location; 3468 3469 /** 3470 * The number of repetitions of a service or product. 3471 */ 3472 @Child(name = "quantity", type = {SimpleQuantity.class}, order=13, min=0, max=1, modifier=false, summary=false) 3473 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 3474 protected SimpleQuantity quantity; 3475 3476 /** 3477 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 3478 */ 3479 @Child(name = "unitPrice", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 3480 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 3481 protected Money unitPrice; 3482 3483 /** 3484 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3485 */ 3486 @Child(name = "factor", type = {DecimalType.class}, order=15, min=0, max=1, modifier=false, summary=false) 3487 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3488 protected DecimalType factor; 3489 3490 /** 3491 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 3492 */ 3493 @Child(name = "net", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3494 @Description(shortDefinition="Total item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 3495 protected Money net; 3496 3497 /** 3498 * List of Unique Device Identifiers associated with this line item. 3499 */ 3500 @Child(name = "udi", type = {Device.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3501 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 3502 protected List<Reference> udi; 3503 /** 3504 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 3505 */ 3506 protected List<Device> udiTarget; 3507 3508 3509 /** 3510 * Physical service site on the patient (limb, tooth, etc). 3511 */ 3512 @Child(name = "bodySite", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=false) 3513 @Description(shortDefinition="Service Location", formalDefinition="Physical service site on the patient (limb, tooth, etc)." ) 3514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 3515 protected CodeableConcept bodySite; 3516 3517 /** 3518 * A region or surface of the site, eg. limb region or tooth surface(s). 3519 */ 3520 @Child(name = "subSite", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3521 @Description(shortDefinition="Service Sub-location", formalDefinition="A region or surface of the site, eg. limb region or tooth surface(s)." ) 3522 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 3523 protected List<CodeableConcept> subSite; 3524 3525 /** 3526 * A billed item may include goods or services provided in multiple encounters. 3527 */ 3528 @Child(name = "encounter", type = {Encounter.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3529 @Description(shortDefinition="Encounters related to this billed item", formalDefinition="A billed item may include goods or services provided in multiple encounters." ) 3530 protected List<Reference> encounter; 3531 /** 3532 * The actual objects that are the target of the reference (A billed item may include goods or services provided in multiple encounters.) 3533 */ 3534 protected List<Encounter> encounterTarget; 3535 3536 3537 /** 3538 * Second tier of goods and services. 3539 */ 3540 @Child(name = "detail", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3541 @Description(shortDefinition="Additional items", formalDefinition="Second tier of goods and services." ) 3542 protected List<DetailComponent> detail; 3543 3544 private static final long serialVersionUID = 784765825L; 3545 3546 /** 3547 * Constructor 3548 */ 3549 public ItemComponent() { 3550 super(); 3551 } 3552 3553 /** 3554 * Constructor 3555 */ 3556 public ItemComponent(PositiveIntType sequence) { 3557 super(); 3558 this.sequence = sequence; 3559 } 3560 3561 /** 3562 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3563 */ 3564 public PositiveIntType getSequenceElement() { 3565 if (this.sequence == null) 3566 if (Configuration.errorOnAutoCreate()) 3567 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3568 else if (Configuration.doAutoCreate()) 3569 this.sequence = new PositiveIntType(); // bb 3570 return this.sequence; 3571 } 3572 3573 public boolean hasSequenceElement() { 3574 return this.sequence != null && !this.sequence.isEmpty(); 3575 } 3576 3577 public boolean hasSequence() { 3578 return this.sequence != null && !this.sequence.isEmpty(); 3579 } 3580 3581 /** 3582 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3583 */ 3584 public ItemComponent setSequenceElement(PositiveIntType value) { 3585 this.sequence = value; 3586 return this; 3587 } 3588 3589 /** 3590 * @return A service line number. 3591 */ 3592 public int getSequence() { 3593 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3594 } 3595 3596 /** 3597 * @param value A service line number. 3598 */ 3599 public ItemComponent setSequence(int value) { 3600 if (this.sequence == null) 3601 this.sequence = new PositiveIntType(); 3602 this.sequence.setValue(value); 3603 return this; 3604 } 3605 3606 /** 3607 * @return {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3608 */ 3609 public List<PositiveIntType> getCareTeamLinkId() { 3610 if (this.careTeamLinkId == null) 3611 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3612 return this.careTeamLinkId; 3613 } 3614 3615 /** 3616 * @return Returns a reference to <code>this</code> for easy method chaining 3617 */ 3618 public ItemComponent setCareTeamLinkId(List<PositiveIntType> theCareTeamLinkId) { 3619 this.careTeamLinkId = theCareTeamLinkId; 3620 return this; 3621 } 3622 3623 public boolean hasCareTeamLinkId() { 3624 if (this.careTeamLinkId == null) 3625 return false; 3626 for (PositiveIntType item : this.careTeamLinkId) 3627 if (!item.isEmpty()) 3628 return true; 3629 return false; 3630 } 3631 3632 /** 3633 * @return {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3634 */ 3635 public PositiveIntType addCareTeamLinkIdElement() {//2 3636 PositiveIntType t = new PositiveIntType(); 3637 if (this.careTeamLinkId == null) 3638 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3639 this.careTeamLinkId.add(t); 3640 return t; 3641 } 3642 3643 /** 3644 * @param value {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3645 */ 3646 public ItemComponent addCareTeamLinkId(int value) { //1 3647 PositiveIntType t = new PositiveIntType(); 3648 t.setValue(value); 3649 if (this.careTeamLinkId == null) 3650 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3651 this.careTeamLinkId.add(t); 3652 return this; 3653 } 3654 3655 /** 3656 * @param value {@link #careTeamLinkId} (CareTeam applicable for this service or product line.) 3657 */ 3658 public boolean hasCareTeamLinkId(int value) { 3659 if (this.careTeamLinkId == null) 3660 return false; 3661 for (PositiveIntType v : this.careTeamLinkId) 3662 if (v.getValue().equals(value)) // positiveInt 3663 return true; 3664 return false; 3665 } 3666 3667 /** 3668 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3669 */ 3670 public List<PositiveIntType> getDiagnosisLinkId() { 3671 if (this.diagnosisLinkId == null) 3672 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3673 return this.diagnosisLinkId; 3674 } 3675 3676 /** 3677 * @return Returns a reference to <code>this</code> for easy method chaining 3678 */ 3679 public ItemComponent setDiagnosisLinkId(List<PositiveIntType> theDiagnosisLinkId) { 3680 this.diagnosisLinkId = theDiagnosisLinkId; 3681 return this; 3682 } 3683 3684 public boolean hasDiagnosisLinkId() { 3685 if (this.diagnosisLinkId == null) 3686 return false; 3687 for (PositiveIntType item : this.diagnosisLinkId) 3688 if (!item.isEmpty()) 3689 return true; 3690 return false; 3691 } 3692 3693 /** 3694 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3695 */ 3696 public PositiveIntType addDiagnosisLinkIdElement() {//2 3697 PositiveIntType t = new PositiveIntType(); 3698 if (this.diagnosisLinkId == null) 3699 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3700 this.diagnosisLinkId.add(t); 3701 return t; 3702 } 3703 3704 /** 3705 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3706 */ 3707 public ItemComponent addDiagnosisLinkId(int value) { //1 3708 PositiveIntType t = new PositiveIntType(); 3709 t.setValue(value); 3710 if (this.diagnosisLinkId == null) 3711 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3712 this.diagnosisLinkId.add(t); 3713 return this; 3714 } 3715 3716 /** 3717 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3718 */ 3719 public boolean hasDiagnosisLinkId(int value) { 3720 if (this.diagnosisLinkId == null) 3721 return false; 3722 for (PositiveIntType v : this.diagnosisLinkId) 3723 if (v.getValue().equals(value)) // positiveInt 3724 return true; 3725 return false; 3726 } 3727 3728 /** 3729 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3730 */ 3731 public List<PositiveIntType> getProcedureLinkId() { 3732 if (this.procedureLinkId == null) 3733 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3734 return this.procedureLinkId; 3735 } 3736 3737 /** 3738 * @return Returns a reference to <code>this</code> for easy method chaining 3739 */ 3740 public ItemComponent setProcedureLinkId(List<PositiveIntType> theProcedureLinkId) { 3741 this.procedureLinkId = theProcedureLinkId; 3742 return this; 3743 } 3744 3745 public boolean hasProcedureLinkId() { 3746 if (this.procedureLinkId == null) 3747 return false; 3748 for (PositiveIntType item : this.procedureLinkId) 3749 if (!item.isEmpty()) 3750 return true; 3751 return false; 3752 } 3753 3754 /** 3755 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3756 */ 3757 public PositiveIntType addProcedureLinkIdElement() {//2 3758 PositiveIntType t = new PositiveIntType(); 3759 if (this.procedureLinkId == null) 3760 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3761 this.procedureLinkId.add(t); 3762 return t; 3763 } 3764 3765 /** 3766 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3767 */ 3768 public ItemComponent addProcedureLinkId(int value) { //1 3769 PositiveIntType t = new PositiveIntType(); 3770 t.setValue(value); 3771 if (this.procedureLinkId == null) 3772 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3773 this.procedureLinkId.add(t); 3774 return this; 3775 } 3776 3777 /** 3778 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3779 */ 3780 public boolean hasProcedureLinkId(int value) { 3781 if (this.procedureLinkId == null) 3782 return false; 3783 for (PositiveIntType v : this.procedureLinkId) 3784 if (v.getValue().equals(value)) // positiveInt 3785 return true; 3786 return false; 3787 } 3788 3789 /** 3790 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3791 */ 3792 public List<PositiveIntType> getInformationLinkId() { 3793 if (this.informationLinkId == null) 3794 this.informationLinkId = new ArrayList<PositiveIntType>(); 3795 return this.informationLinkId; 3796 } 3797 3798 /** 3799 * @return Returns a reference to <code>this</code> for easy method chaining 3800 */ 3801 public ItemComponent setInformationLinkId(List<PositiveIntType> theInformationLinkId) { 3802 this.informationLinkId = theInformationLinkId; 3803 return this; 3804 } 3805 3806 public boolean hasInformationLinkId() { 3807 if (this.informationLinkId == null) 3808 return false; 3809 for (PositiveIntType item : this.informationLinkId) 3810 if (!item.isEmpty()) 3811 return true; 3812 return false; 3813 } 3814 3815 /** 3816 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3817 */ 3818 public PositiveIntType addInformationLinkIdElement() {//2 3819 PositiveIntType t = new PositiveIntType(); 3820 if (this.informationLinkId == null) 3821 this.informationLinkId = new ArrayList<PositiveIntType>(); 3822 this.informationLinkId.add(t); 3823 return t; 3824 } 3825 3826 /** 3827 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3828 */ 3829 public ItemComponent addInformationLinkId(int value) { //1 3830 PositiveIntType t = new PositiveIntType(); 3831 t.setValue(value); 3832 if (this.informationLinkId == null) 3833 this.informationLinkId = new ArrayList<PositiveIntType>(); 3834 this.informationLinkId.add(t); 3835 return this; 3836 } 3837 3838 /** 3839 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3840 */ 3841 public boolean hasInformationLinkId(int value) { 3842 if (this.informationLinkId == null) 3843 return false; 3844 for (PositiveIntType v : this.informationLinkId) 3845 if (v.getValue().equals(value)) // positiveInt 3846 return true; 3847 return false; 3848 } 3849 3850 /** 3851 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3852 */ 3853 public CodeableConcept getRevenue() { 3854 if (this.revenue == null) 3855 if (Configuration.errorOnAutoCreate()) 3856 throw new Error("Attempt to auto-create ItemComponent.revenue"); 3857 else if (Configuration.doAutoCreate()) 3858 this.revenue = new CodeableConcept(); // cc 3859 return this.revenue; 3860 } 3861 3862 public boolean hasRevenue() { 3863 return this.revenue != null && !this.revenue.isEmpty(); 3864 } 3865 3866 /** 3867 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3868 */ 3869 public ItemComponent setRevenue(CodeableConcept value) { 3870 this.revenue = value; 3871 return this; 3872 } 3873 3874 /** 3875 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3876 */ 3877 public CodeableConcept getCategory() { 3878 if (this.category == null) 3879 if (Configuration.errorOnAutoCreate()) 3880 throw new Error("Attempt to auto-create ItemComponent.category"); 3881 else if (Configuration.doAutoCreate()) 3882 this.category = new CodeableConcept(); // cc 3883 return this.category; 3884 } 3885 3886 public boolean hasCategory() { 3887 return this.category != null && !this.category.isEmpty(); 3888 } 3889 3890 /** 3891 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3892 */ 3893 public ItemComponent setCategory(CodeableConcept value) { 3894 this.category = value; 3895 return this; 3896 } 3897 3898 /** 3899 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3900 */ 3901 public CodeableConcept getService() { 3902 if (this.service == null) 3903 if (Configuration.errorOnAutoCreate()) 3904 throw new Error("Attempt to auto-create ItemComponent.service"); 3905 else if (Configuration.doAutoCreate()) 3906 this.service = new CodeableConcept(); // cc 3907 return this.service; 3908 } 3909 3910 public boolean hasService() { 3911 return this.service != null && !this.service.isEmpty(); 3912 } 3913 3914 /** 3915 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3916 */ 3917 public ItemComponent setService(CodeableConcept value) { 3918 this.service = value; 3919 return this; 3920 } 3921 3922 /** 3923 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 3924 */ 3925 public List<CodeableConcept> getModifier() { 3926 if (this.modifier == null) 3927 this.modifier = new ArrayList<CodeableConcept>(); 3928 return this.modifier; 3929 } 3930 3931 /** 3932 * @return Returns a reference to <code>this</code> for easy method chaining 3933 */ 3934 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 3935 this.modifier = theModifier; 3936 return this; 3937 } 3938 3939 public boolean hasModifier() { 3940 if (this.modifier == null) 3941 return false; 3942 for (CodeableConcept item : this.modifier) 3943 if (!item.isEmpty()) 3944 return true; 3945 return false; 3946 } 3947 3948 public CodeableConcept addModifier() { //3 3949 CodeableConcept t = new CodeableConcept(); 3950 if (this.modifier == null) 3951 this.modifier = new ArrayList<CodeableConcept>(); 3952 this.modifier.add(t); 3953 return t; 3954 } 3955 3956 public ItemComponent addModifier(CodeableConcept t) { //3 3957 if (t == null) 3958 return this; 3959 if (this.modifier == null) 3960 this.modifier = new ArrayList<CodeableConcept>(); 3961 this.modifier.add(t); 3962 return this; 3963 } 3964 3965 /** 3966 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 3967 */ 3968 public CodeableConcept getModifierFirstRep() { 3969 if (getModifier().isEmpty()) { 3970 addModifier(); 3971 } 3972 return getModifier().get(0); 3973 } 3974 3975 /** 3976 * @return {@link #programCode} (For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program.) 3977 */ 3978 public List<CodeableConcept> getProgramCode() { 3979 if (this.programCode == null) 3980 this.programCode = new ArrayList<CodeableConcept>(); 3981 return this.programCode; 3982 } 3983 3984 /** 3985 * @return Returns a reference to <code>this</code> for easy method chaining 3986 */ 3987 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 3988 this.programCode = theProgramCode; 3989 return this; 3990 } 3991 3992 public boolean hasProgramCode() { 3993 if (this.programCode == null) 3994 return false; 3995 for (CodeableConcept item : this.programCode) 3996 if (!item.isEmpty()) 3997 return true; 3998 return false; 3999 } 4000 4001 public CodeableConcept addProgramCode() { //3 4002 CodeableConcept t = new CodeableConcept(); 4003 if (this.programCode == null) 4004 this.programCode = new ArrayList<CodeableConcept>(); 4005 this.programCode.add(t); 4006 return t; 4007 } 4008 4009 public ItemComponent addProgramCode(CodeableConcept t) { //3 4010 if (t == null) 4011 return this; 4012 if (this.programCode == null) 4013 this.programCode = new ArrayList<CodeableConcept>(); 4014 this.programCode.add(t); 4015 return this; 4016 } 4017 4018 /** 4019 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 4020 */ 4021 public CodeableConcept getProgramCodeFirstRep() { 4022 if (getProgramCode().isEmpty()) { 4023 addProgramCode(); 4024 } 4025 return getProgramCode().get(0); 4026 } 4027 4028 /** 4029 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4030 */ 4031 public Type getServiced() { 4032 return this.serviced; 4033 } 4034 4035 /** 4036 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4037 */ 4038 public DateType getServicedDateType() throws FHIRException { 4039 if (this.serviced == null) 4040 return null; 4041 if (!(this.serviced instanceof DateType)) 4042 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4043 return (DateType) this.serviced; 4044 } 4045 4046 public boolean hasServicedDateType() { 4047 return this.serviced instanceof DateType; 4048 } 4049 4050 /** 4051 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4052 */ 4053 public Period getServicedPeriod() throws FHIRException { 4054 if (this.serviced == null) 4055 return null; 4056 if (!(this.serviced instanceof Period)) 4057 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4058 return (Period) this.serviced; 4059 } 4060 4061 public boolean hasServicedPeriod() { 4062 return this.serviced instanceof Period; 4063 } 4064 4065 public boolean hasServiced() { 4066 return this.serviced != null && !this.serviced.isEmpty(); 4067 } 4068 4069 /** 4070 * @param value {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 4071 */ 4072 public ItemComponent setServiced(Type value) throws FHIRFormatError { 4073 if (value != null && !(value instanceof DateType || value instanceof Period)) 4074 throw new FHIRFormatError("Not the right type for Claim.item.serviced[x]: "+value.fhirType()); 4075 this.serviced = value; 4076 return this; 4077 } 4078 4079 /** 4080 * @return {@link #location} (Where the service was provided.) 4081 */ 4082 public Type getLocation() { 4083 return this.location; 4084 } 4085 4086 /** 4087 * @return {@link #location} (Where the service was provided.) 4088 */ 4089 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 4090 if (this.location == null) 4091 return null; 4092 if (!(this.location instanceof CodeableConcept)) 4093 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 4094 return (CodeableConcept) this.location; 4095 } 4096 4097 public boolean hasLocationCodeableConcept() { 4098 return this.location instanceof CodeableConcept; 4099 } 4100 4101 /** 4102 * @return {@link #location} (Where the service was provided.) 4103 */ 4104 public Address getLocationAddress() throws FHIRException { 4105 if (this.location == null) 4106 return null; 4107 if (!(this.location instanceof Address)) 4108 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 4109 return (Address) this.location; 4110 } 4111 4112 public boolean hasLocationAddress() { 4113 return this.location instanceof Address; 4114 } 4115 4116 /** 4117 * @return {@link #location} (Where the service was provided.) 4118 */ 4119 public Reference getLocationReference() throws FHIRException { 4120 if (this.location == null) 4121 return null; 4122 if (!(this.location instanceof Reference)) 4123 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 4124 return (Reference) this.location; 4125 } 4126 4127 public boolean hasLocationReference() { 4128 return this.location instanceof Reference; 4129 } 4130 4131 public boolean hasLocation() { 4132 return this.location != null && !this.location.isEmpty(); 4133 } 4134 4135 /** 4136 * @param value {@link #location} (Where the service was provided.) 4137 */ 4138 public ItemComponent setLocation(Type value) throws FHIRFormatError { 4139 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 4140 throw new FHIRFormatError("Not the right type for Claim.item.location[x]: "+value.fhirType()); 4141 this.location = value; 4142 return this; 4143 } 4144 4145 /** 4146 * @return {@link #quantity} (The number of repetitions of a service or product.) 4147 */ 4148 public SimpleQuantity getQuantity() { 4149 if (this.quantity == null) 4150 if (Configuration.errorOnAutoCreate()) 4151 throw new Error("Attempt to auto-create ItemComponent.quantity"); 4152 else if (Configuration.doAutoCreate()) 4153 this.quantity = new SimpleQuantity(); // cc 4154 return this.quantity; 4155 } 4156 4157 public boolean hasQuantity() { 4158 return this.quantity != null && !this.quantity.isEmpty(); 4159 } 4160 4161 /** 4162 * @param value {@link #quantity} (The number of repetitions of a service or product.) 4163 */ 4164 public ItemComponent setQuantity(SimpleQuantity value) { 4165 this.quantity = value; 4166 return this; 4167 } 4168 4169 /** 4170 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 4171 */ 4172 public Money getUnitPrice() { 4173 if (this.unitPrice == null) 4174 if (Configuration.errorOnAutoCreate()) 4175 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 4176 else if (Configuration.doAutoCreate()) 4177 this.unitPrice = new Money(); // cc 4178 return this.unitPrice; 4179 } 4180 4181 public boolean hasUnitPrice() { 4182 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4183 } 4184 4185 /** 4186 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 4187 */ 4188 public ItemComponent setUnitPrice(Money value) { 4189 this.unitPrice = value; 4190 return this; 4191 } 4192 4193 /** 4194 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4195 */ 4196 public DecimalType getFactorElement() { 4197 if (this.factor == null) 4198 if (Configuration.errorOnAutoCreate()) 4199 throw new Error("Attempt to auto-create ItemComponent.factor"); 4200 else if (Configuration.doAutoCreate()) 4201 this.factor = new DecimalType(); // bb 4202 return this.factor; 4203 } 4204 4205 public boolean hasFactorElement() { 4206 return this.factor != null && !this.factor.isEmpty(); 4207 } 4208 4209 public boolean hasFactor() { 4210 return this.factor != null && !this.factor.isEmpty(); 4211 } 4212 4213 /** 4214 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4215 */ 4216 public ItemComponent setFactorElement(DecimalType value) { 4217 this.factor = value; 4218 return this; 4219 } 4220 4221 /** 4222 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4223 */ 4224 public BigDecimal getFactor() { 4225 return this.factor == null ? null : this.factor.getValue(); 4226 } 4227 4228 /** 4229 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4230 */ 4231 public ItemComponent setFactor(BigDecimal value) { 4232 if (value == null) 4233 this.factor = null; 4234 else { 4235 if (this.factor == null) 4236 this.factor = new DecimalType(); 4237 this.factor.setValue(value); 4238 } 4239 return this; 4240 } 4241 4242 /** 4243 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4244 */ 4245 public ItemComponent setFactor(long value) { 4246 this.factor = new DecimalType(); 4247 this.factor.setValue(value); 4248 return this; 4249 } 4250 4251 /** 4252 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4253 */ 4254 public ItemComponent setFactor(double value) { 4255 this.factor = new DecimalType(); 4256 this.factor.setValue(value); 4257 return this; 4258 } 4259 4260 /** 4261 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 4262 */ 4263 public Money getNet() { 4264 if (this.net == null) 4265 if (Configuration.errorOnAutoCreate()) 4266 throw new Error("Attempt to auto-create ItemComponent.net"); 4267 else if (Configuration.doAutoCreate()) 4268 this.net = new Money(); // cc 4269 return this.net; 4270 } 4271 4272 public boolean hasNet() { 4273 return this.net != null && !this.net.isEmpty(); 4274 } 4275 4276 /** 4277 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 4278 */ 4279 public ItemComponent setNet(Money value) { 4280 this.net = value; 4281 return this; 4282 } 4283 4284 /** 4285 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 4286 */ 4287 public List<Reference> getUdi() { 4288 if (this.udi == null) 4289 this.udi = new ArrayList<Reference>(); 4290 return this.udi; 4291 } 4292 4293 /** 4294 * @return Returns a reference to <code>this</code> for easy method chaining 4295 */ 4296 public ItemComponent setUdi(List<Reference> theUdi) { 4297 this.udi = theUdi; 4298 return this; 4299 } 4300 4301 public boolean hasUdi() { 4302 if (this.udi == null) 4303 return false; 4304 for (Reference item : this.udi) 4305 if (!item.isEmpty()) 4306 return true; 4307 return false; 4308 } 4309 4310 public Reference addUdi() { //3 4311 Reference t = new Reference(); 4312 if (this.udi == null) 4313 this.udi = new ArrayList<Reference>(); 4314 this.udi.add(t); 4315 return t; 4316 } 4317 4318 public ItemComponent addUdi(Reference t) { //3 4319 if (t == null) 4320 return this; 4321 if (this.udi == null) 4322 this.udi = new ArrayList<Reference>(); 4323 this.udi.add(t); 4324 return this; 4325 } 4326 4327 /** 4328 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 4329 */ 4330 public Reference getUdiFirstRep() { 4331 if (getUdi().isEmpty()) { 4332 addUdi(); 4333 } 4334 return getUdi().get(0); 4335 } 4336 4337 /** 4338 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 4339 */ 4340 public CodeableConcept getBodySite() { 4341 if (this.bodySite == null) 4342 if (Configuration.errorOnAutoCreate()) 4343 throw new Error("Attempt to auto-create ItemComponent.bodySite"); 4344 else if (Configuration.doAutoCreate()) 4345 this.bodySite = new CodeableConcept(); // cc 4346 return this.bodySite; 4347 } 4348 4349 public boolean hasBodySite() { 4350 return this.bodySite != null && !this.bodySite.isEmpty(); 4351 } 4352 4353 /** 4354 * @param value {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 4355 */ 4356 public ItemComponent setBodySite(CodeableConcept value) { 4357 this.bodySite = value; 4358 return this; 4359 } 4360 4361 /** 4362 * @return {@link #subSite} (A region or surface of the site, eg. limb region or tooth surface(s).) 4363 */ 4364 public List<CodeableConcept> getSubSite() { 4365 if (this.subSite == null) 4366 this.subSite = new ArrayList<CodeableConcept>(); 4367 return this.subSite; 4368 } 4369 4370 /** 4371 * @return Returns a reference to <code>this</code> for easy method chaining 4372 */ 4373 public ItemComponent setSubSite(List<CodeableConcept> theSubSite) { 4374 this.subSite = theSubSite; 4375 return this; 4376 } 4377 4378 public boolean hasSubSite() { 4379 if (this.subSite == null) 4380 return false; 4381 for (CodeableConcept item : this.subSite) 4382 if (!item.isEmpty()) 4383 return true; 4384 return false; 4385 } 4386 4387 public CodeableConcept addSubSite() { //3 4388 CodeableConcept t = new CodeableConcept(); 4389 if (this.subSite == null) 4390 this.subSite = new ArrayList<CodeableConcept>(); 4391 this.subSite.add(t); 4392 return t; 4393 } 4394 4395 public ItemComponent addSubSite(CodeableConcept t) { //3 4396 if (t == null) 4397 return this; 4398 if (this.subSite == null) 4399 this.subSite = new ArrayList<CodeableConcept>(); 4400 this.subSite.add(t); 4401 return this; 4402 } 4403 4404 /** 4405 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist 4406 */ 4407 public CodeableConcept getSubSiteFirstRep() { 4408 if (getSubSite().isEmpty()) { 4409 addSubSite(); 4410 } 4411 return getSubSite().get(0); 4412 } 4413 4414 /** 4415 * @return {@link #encounter} (A billed item may include goods or services provided in multiple encounters.) 4416 */ 4417 public List<Reference> getEncounter() { 4418 if (this.encounter == null) 4419 this.encounter = new ArrayList<Reference>(); 4420 return this.encounter; 4421 } 4422 4423 /** 4424 * @return Returns a reference to <code>this</code> for easy method chaining 4425 */ 4426 public ItemComponent setEncounter(List<Reference> theEncounter) { 4427 this.encounter = theEncounter; 4428 return this; 4429 } 4430 4431 public boolean hasEncounter() { 4432 if (this.encounter == null) 4433 return false; 4434 for (Reference item : this.encounter) 4435 if (!item.isEmpty()) 4436 return true; 4437 return false; 4438 } 4439 4440 public Reference addEncounter() { //3 4441 Reference t = new Reference(); 4442 if (this.encounter == null) 4443 this.encounter = new ArrayList<Reference>(); 4444 this.encounter.add(t); 4445 return t; 4446 } 4447 4448 public ItemComponent addEncounter(Reference t) { //3 4449 if (t == null) 4450 return this; 4451 if (this.encounter == null) 4452 this.encounter = new ArrayList<Reference>(); 4453 this.encounter.add(t); 4454 return this; 4455 } 4456 4457 /** 4458 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist 4459 */ 4460 public Reference getEncounterFirstRep() { 4461 if (getEncounter().isEmpty()) { 4462 addEncounter(); 4463 } 4464 return getEncounter().get(0); 4465 } 4466 4467 /** 4468 * @return {@link #detail} (Second tier of goods and services.) 4469 */ 4470 public List<DetailComponent> getDetail() { 4471 if (this.detail == null) 4472 this.detail = new ArrayList<DetailComponent>(); 4473 return this.detail; 4474 } 4475 4476 /** 4477 * @return Returns a reference to <code>this</code> for easy method chaining 4478 */ 4479 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4480 this.detail = theDetail; 4481 return this; 4482 } 4483 4484 public boolean hasDetail() { 4485 if (this.detail == null) 4486 return false; 4487 for (DetailComponent item : this.detail) 4488 if (!item.isEmpty()) 4489 return true; 4490 return false; 4491 } 4492 4493 public DetailComponent addDetail() { //3 4494 DetailComponent t = new DetailComponent(); 4495 if (this.detail == null) 4496 this.detail = new ArrayList<DetailComponent>(); 4497 this.detail.add(t); 4498 return t; 4499 } 4500 4501 public ItemComponent addDetail(DetailComponent t) { //3 4502 if (t == null) 4503 return this; 4504 if (this.detail == null) 4505 this.detail = new ArrayList<DetailComponent>(); 4506 this.detail.add(t); 4507 return this; 4508 } 4509 4510 /** 4511 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 4512 */ 4513 public DetailComponent getDetailFirstRep() { 4514 if (getDetail().isEmpty()) { 4515 addDetail(); 4516 } 4517 return getDetail().get(0); 4518 } 4519 4520 protected void listChildren(List<Property> children) { 4521 super.listChildren(children); 4522 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 4523 children.add(new Property("careTeamLinkId", "positiveInt", "CareTeam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId)); 4524 children.add(new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId)); 4525 children.add(new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId)); 4526 children.add(new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId)); 4527 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 4528 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 4529 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 4530 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4531 children.add(new Property("programCode", "CodeableConcept", "For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4532 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 4533 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location)); 4534 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4535 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 4536 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4537 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 4538 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4539 children.add(new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite)); 4540 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 4541 children.add(new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4542 children.add(new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 4543 } 4544 4545 @Override 4546 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4547 switch (_hash) { 4548 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 4549 case -186757789: /*careTeamLinkId*/ return new Property("careTeamLinkId", "positiveInt", "CareTeam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId); 4550 case -1659207418: /*diagnosisLinkId*/ return new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId); 4551 case -532846744: /*procedureLinkId*/ return new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId); 4552 case 1965585153: /*informationLinkId*/ return new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId); 4553 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 4554 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 4555 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,RXNorm,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 4556 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 4557 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reason codes for the inclusion or covering of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 4558 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4559 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4560 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4561 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4562 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4563 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4564 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4565 case -1280020865: /*locationAddress*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4566 case 755866390: /*locationReference*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4567 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 4568 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 4569 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 4570 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 4571 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 4572 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite); 4573 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 4574 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter); 4575 case -1335224239: /*detail*/ return new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail); 4576 default: return super.getNamedProperty(_hash, _name, _checkValid); 4577 } 4578 4579 } 4580 4581 @Override 4582 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4583 switch (hash) { 4584 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 4585 case -186757789: /*careTeamLinkId*/ return this.careTeamLinkId == null ? new Base[0] : this.careTeamLinkId.toArray(new Base[this.careTeamLinkId.size()]); // PositiveIntType 4586 case -1659207418: /*diagnosisLinkId*/ return this.diagnosisLinkId == null ? new Base[0] : this.diagnosisLinkId.toArray(new Base[this.diagnosisLinkId.size()]); // PositiveIntType 4587 case -532846744: /*procedureLinkId*/ return this.procedureLinkId == null ? new Base[0] : this.procedureLinkId.toArray(new Base[this.procedureLinkId.size()]); // PositiveIntType 4588 case 1965585153: /*informationLinkId*/ return this.informationLinkId == null ? new Base[0] : this.informationLinkId.toArray(new Base[this.informationLinkId.size()]); // PositiveIntType 4589 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 4590 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4591 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 4592 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4593 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 4594 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // Type 4595 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 4596 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 4597 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 4598 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 4599 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 4600 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 4601 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 4602 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 4603 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 4604 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 4605 default: return super.getProperty(hash, name, checkValid); 4606 } 4607 4608 } 4609 4610 @Override 4611 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4612 switch (hash) { 4613 case 1349547969: // sequence 4614 this.sequence = castToPositiveInt(value); // PositiveIntType 4615 return value; 4616 case -186757789: // careTeamLinkId 4617 this.getCareTeamLinkId().add(castToPositiveInt(value)); // PositiveIntType 4618 return value; 4619 case -1659207418: // diagnosisLinkId 4620 this.getDiagnosisLinkId().add(castToPositiveInt(value)); // PositiveIntType 4621 return value; 4622 case -532846744: // procedureLinkId 4623 this.getProcedureLinkId().add(castToPositiveInt(value)); // PositiveIntType 4624 return value; 4625 case 1965585153: // informationLinkId 4626 this.getInformationLinkId().add(castToPositiveInt(value)); // PositiveIntType 4627 return value; 4628 case 1099842588: // revenue 4629 this.revenue = castToCodeableConcept(value); // CodeableConcept 4630 return value; 4631 case 50511102: // category 4632 this.category = castToCodeableConcept(value); // CodeableConcept 4633 return value; 4634 case 1984153269: // service 4635 this.service = castToCodeableConcept(value); // CodeableConcept 4636 return value; 4637 case -615513385: // modifier 4638 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 4639 return value; 4640 case 1010065041: // programCode 4641 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 4642 return value; 4643 case 1379209295: // serviced 4644 this.serviced = castToType(value); // Type 4645 return value; 4646 case 1901043637: // location 4647 this.location = castToType(value); // Type 4648 return value; 4649 case -1285004149: // quantity 4650 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4651 return value; 4652 case -486196699: // unitPrice 4653 this.unitPrice = castToMoney(value); // Money 4654 return value; 4655 case -1282148017: // factor 4656 this.factor = castToDecimal(value); // DecimalType 4657 return value; 4658 case 108957: // net 4659 this.net = castToMoney(value); // Money 4660 return value; 4661 case 115642: // udi 4662 this.getUdi().add(castToReference(value)); // Reference 4663 return value; 4664 case 1702620169: // bodySite 4665 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4666 return value; 4667 case -1868566105: // subSite 4668 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 4669 return value; 4670 case 1524132147: // encounter 4671 this.getEncounter().add(castToReference(value)); // Reference 4672 return value; 4673 case -1335224239: // detail 4674 this.getDetail().add((DetailComponent) value); // DetailComponent 4675 return value; 4676 default: return super.setProperty(hash, name, value); 4677 } 4678 4679 } 4680 4681 @Override 4682 public Base setProperty(String name, Base value) throws FHIRException { 4683 if (name.equals("sequence")) { 4684 this.sequence = castToPositiveInt(value); // PositiveIntType 4685 } else if (name.equals("careTeamLinkId")) { 4686 this.getCareTeamLinkId().add(castToPositiveInt(value)); 4687 } else if (name.equals("diagnosisLinkId")) { 4688 this.getDiagnosisLinkId().add(castToPositiveInt(value)); 4689 } else if (name.equals("procedureLinkId")) { 4690 this.getProcedureLinkId().add(castToPositiveInt(value)); 4691 } else if (name.equals("informationLinkId")) { 4692 this.getInformationLinkId().add(castToPositiveInt(value)); 4693 } else if (name.equals("revenue")) { 4694 this.revenue = castToCodeableConcept(value); // CodeableConcept 4695 } else if (name.equals("category")) { 4696 this.category = castToCodeableConcept(value); // CodeableConcept 4697 } else if (name.equals("service")) { 4698 this.service = castToCodeableConcept(value); // CodeableConcept 4699 } else if (name.equals("modifier")) { 4700 this.getModifier().add(castToCodeableConcept(value)); 4701 } else if (name.equals("programCode")) { 4702 this.getProgramCode().add(castToCodeableConcept(value)); 4703 } else if (name.equals("serviced[x]")) { 4704 this.serviced = castToType(value); // Type 4705 } else if (name.equals("location[x]")) { 4706 this.location = castToType(value); // Type 4707 } else if (name.equals("quantity")) { 4708 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4709 } else if (name.equals("unitPrice")) { 4710 this.unitPrice = castToMoney(value); // Money 4711 } else if (name.equals("factor")) { 4712 this.factor = castToDecimal(value); // DecimalType 4713 } else if (name.equals("net")) { 4714 this.net = castToMoney(value); // Money 4715 } else if (name.equals("udi")) { 4716 this.getUdi().add(castToReference(value)); 4717 } else if (name.equals("bodySite")) { 4718 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4719 } else if (name.equals("subSite")) { 4720 this.getSubSite().add(castToCodeableConcept(value)); 4721 } else if (name.equals("encounter")) { 4722 this.getEncounter().add(castToReference(value)); 4723 } else if (name.equals("detail")) { 4724 this.getDetail().add((DetailComponent) value); 4725 } else 4726 return super.setProperty(name, value); 4727 return value; 4728 } 4729 4730 @Override 4731 public Base makeProperty(int hash, String name) throws FHIRException { 4732 switch (hash) { 4733 case 1349547969: return getSequenceElement(); 4734 case -186757789: return addCareTeamLinkIdElement(); 4735 case -1659207418: return addDiagnosisLinkIdElement(); 4736 case -532846744: return addProcedureLinkIdElement(); 4737 case 1965585153: return addInformationLinkIdElement(); 4738 case 1099842588: return getRevenue(); 4739 case 50511102: return getCategory(); 4740 case 1984153269: return getService(); 4741 case -615513385: return addModifier(); 4742 case 1010065041: return addProgramCode(); 4743 case -1927922223: return getServiced(); 4744 case 1379209295: return getServiced(); 4745 case 552316075: return getLocation(); 4746 case 1901043637: return getLocation(); 4747 case -1285004149: return getQuantity(); 4748 case -486196699: return getUnitPrice(); 4749 case -1282148017: return getFactorElement(); 4750 case 108957: return getNet(); 4751 case 115642: return addUdi(); 4752 case 1702620169: return getBodySite(); 4753 case -1868566105: return addSubSite(); 4754 case 1524132147: return addEncounter(); 4755 case -1335224239: return addDetail(); 4756 default: return super.makeProperty(hash, name); 4757 } 4758 4759 } 4760 4761 @Override 4762 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4763 switch (hash) { 4764 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 4765 case -186757789: /*careTeamLinkId*/ return new String[] {"positiveInt"}; 4766 case -1659207418: /*diagnosisLinkId*/ return new String[] {"positiveInt"}; 4767 case -532846744: /*procedureLinkId*/ return new String[] {"positiveInt"}; 4768 case 1965585153: /*informationLinkId*/ return new String[] {"positiveInt"}; 4769 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 4770 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4771 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 4772 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 4773 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 4774 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 4775 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 4776 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 4777 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 4778 case -1282148017: /*factor*/ return new String[] {"decimal"}; 4779 case 108957: /*net*/ return new String[] {"Money"}; 4780 case 115642: /*udi*/ return new String[] {"Reference"}; 4781 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 4782 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 4783 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 4784 case -1335224239: /*detail*/ return new String[] {}; 4785 default: return super.getTypesForProperty(hash, name); 4786 } 4787 4788 } 4789 4790 @Override 4791 public Base addChild(String name) throws FHIRException { 4792 if (name.equals("sequence")) { 4793 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 4794 } 4795 else if (name.equals("careTeamLinkId")) { 4796 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeamLinkId"); 4797 } 4798 else if (name.equals("diagnosisLinkId")) { 4799 throw new FHIRException("Cannot call addChild on a singleton property Claim.diagnosisLinkId"); 4800 } 4801 else if (name.equals("procedureLinkId")) { 4802 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedureLinkId"); 4803 } 4804 else if (name.equals("informationLinkId")) { 4805 throw new FHIRException("Cannot call addChild on a singleton property Claim.informationLinkId"); 4806 } 4807 else if (name.equals("revenue")) { 4808 this.revenue = new CodeableConcept(); 4809 return this.revenue; 4810 } 4811 else if (name.equals("category")) { 4812 this.category = new CodeableConcept(); 4813 return this.category; 4814 } 4815 else if (name.equals("service")) { 4816 this.service = new CodeableConcept(); 4817 return this.service; 4818 } 4819 else if (name.equals("modifier")) { 4820 return addModifier(); 4821 } 4822 else if (name.equals("programCode")) { 4823 return addProgramCode(); 4824 } 4825 else if (name.equals("servicedDate")) { 4826 this.serviced = new DateType(); 4827 return this.serviced; 4828 } 4829 else if (name.equals("servicedPeriod")) { 4830 this.serviced = new Period(); 4831 return this.serviced; 4832 } 4833 else if (name.equals("locationCodeableConcept")) { 4834 this.location = new CodeableConcept(); 4835 return this.location; 4836 } 4837 else if (name.equals("locationAddress")) { 4838 this.location = new Address(); 4839 return this.location; 4840 } 4841 else if (name.equals("locationReference")) { 4842 this.location = new Reference(); 4843 return this.location; 4844 } 4845 else if (name.equals("quantity")) { 4846 this.quantity = new SimpleQuantity(); 4847 return this.quantity; 4848 } 4849 else if (name.equals("unitPrice")) { 4850 this.unitPrice = new Money(); 4851 return this.unitPrice; 4852 } 4853 else if (name.equals("factor")) { 4854 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 4855 } 4856 else if (name.equals("net")) { 4857 this.net = new Money(); 4858 return this.net; 4859 } 4860 else if (name.equals("udi")) { 4861 return addUdi(); 4862 } 4863 else if (name.equals("bodySite")) { 4864 this.bodySite = new CodeableConcept(); 4865 return this.bodySite; 4866 } 4867 else if (name.equals("subSite")) { 4868 return addSubSite(); 4869 } 4870 else if (name.equals("encounter")) { 4871 return addEncounter(); 4872 } 4873 else if (name.equals("detail")) { 4874 return addDetail(); 4875 } 4876 else 4877 return super.addChild(name); 4878 } 4879 4880 public ItemComponent copy() { 4881 ItemComponent dst = new ItemComponent(); 4882 copyValues(dst); 4883 dst.sequence = sequence == null ? null : sequence.copy(); 4884 if (careTeamLinkId != null) { 4885 dst.careTeamLinkId = new ArrayList<PositiveIntType>(); 4886 for (PositiveIntType i : careTeamLinkId) 4887 dst.careTeamLinkId.add(i.copy()); 4888 }; 4889 if (diagnosisLinkId != null) { 4890 dst.diagnosisLinkId = new ArrayList<PositiveIntType>(); 4891 for (PositiveIntType i : diagnosisLinkId) 4892 dst.diagnosisLinkId.add(i.copy()); 4893 }; 4894 if (procedureLinkId != null) { 4895 dst.procedureLinkId = new ArrayList<PositiveIntType>(); 4896 for (PositiveIntType i : procedureLinkId) 4897 dst.procedureLinkId.add(i.copy()); 4898 }; 4899 if (informationLinkId != null) { 4900 dst.informationLinkId = new ArrayList<PositiveIntType>(); 4901 for (PositiveIntType i : informationLinkId) 4902 dst.informationLinkId.add(i.copy()); 4903 }; 4904 dst.revenue = revenue == null ? null : revenue.copy(); 4905 dst.category = category == null ? null : category.copy(); 4906 dst.service = service == null ? null : service.copy(); 4907 if (modifier != null) { 4908 dst.modifier = new ArrayList<CodeableConcept>(); 4909 for (CodeableConcept i : modifier) 4910 dst.modifier.add(i.copy()); 4911 }; 4912 if (programCode != null) { 4913 dst.programCode = new ArrayList<CodeableConcept>(); 4914 for (CodeableConcept i : programCode) 4915 dst.programCode.add(i.copy()); 4916 }; 4917 dst.serviced = serviced == null ? null : serviced.copy(); 4918 dst.location = location == null ? null : location.copy(); 4919 dst.quantity = quantity == null ? null : quantity.copy(); 4920 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4921 dst.factor = factor == null ? null : factor.copy(); 4922 dst.net = net == null ? null : net.copy(); 4923 if (udi != null) { 4924 dst.udi = new ArrayList<Reference>(); 4925 for (Reference i : udi) 4926 dst.udi.add(i.copy()); 4927 }; 4928 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4929 if (subSite != null) { 4930 dst.subSite = new ArrayList<CodeableConcept>(); 4931 for (CodeableConcept i : subSite) 4932 dst.subSite.add(i.copy()); 4933 }; 4934 if (encounter != null) { 4935 dst.encounter = new ArrayList<Reference>(); 4936 for (Reference i : encounter) 4937 dst.encounter.add(i.copy()); 4938 }; 4939 if (detail != null) { 4940 dst.detail = new ArrayList<DetailComponent>(); 4941 for (DetailComponent i : detail) 4942 dst.detail.add(i.copy()); 4943 }; 4944 return dst; 4945 } 4946 4947 @Override 4948 public boolean equalsDeep(Base other_) { 4949 if (!super.equalsDeep(other_)) 4950 return false; 4951 if (!(other_ instanceof ItemComponent)) 4952 return false; 4953 ItemComponent o = (ItemComponent) other_; 4954 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamLinkId, o.careTeamLinkId, true) 4955 && compareDeep(diagnosisLinkId, o.diagnosisLinkId, true) && compareDeep(procedureLinkId, o.procedureLinkId, true) 4956 && compareDeep(informationLinkId, o.informationLinkId, true) && compareDeep(revenue, o.revenue, true) 4957 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 4958 && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) && compareDeep(location, o.location, true) 4959 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 4960 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 4961 && compareDeep(subSite, o.subSite, true) && compareDeep(encounter, o.encounter, true) && compareDeep(detail, o.detail, true) 4962 ; 4963 } 4964 4965 @Override 4966 public boolean equalsShallow(Base other_) { 4967 if (!super.equalsShallow(other_)) 4968 return false; 4969 if (!(other_ instanceof ItemComponent)) 4970 return false; 4971 ItemComponent o = (ItemComponent) other_; 4972 return compareValues(sequence, o.sequence, true) && compareValues(careTeamLinkId, o.careTeamLinkId, true) 4973 && compareValues(diagnosisLinkId, o.diagnosisLinkId, true) && compareValues(procedureLinkId, o.procedureLinkId, true) 4974 && compareValues(informationLinkId, o.informationLinkId, true) && compareValues(factor, o.factor, true) 4975 ; 4976 } 4977 4978 public boolean isEmpty() { 4979 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamLinkId, diagnosisLinkId 4980 , procedureLinkId, informationLinkId, revenue, category, service, modifier, programCode 4981 , serviced, location, quantity, unitPrice, factor, net, udi, bodySite, subSite 4982 , encounter, detail); 4983 } 4984 4985 public String fhirType() { 4986 return "Claim.item"; 4987 4988 } 4989 4990 } 4991 4992 @Block() 4993 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 4994 /** 4995 * A service line number. 4996 */ 4997 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4998 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 4999 protected PositiveIntType sequence; 5000 5001 /** 5002 * The type of reveneu or cost center providing the product and/or service. 5003 */ 5004 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 5005 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 5006 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5007 protected CodeableConcept revenue; 5008 5009 /** 5010 * Health Care Service Type Codes to identify the classification of service or benefits. 5011 */ 5012 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5013 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 5014 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 5015 protected CodeableConcept category; 5016 5017 /** 5018 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 5019 */ 5020 @Child(name = "service", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5021 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 5022 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5023 protected CodeableConcept service; 5024 5025 /** 5026 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 5027 */ 5028 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5029 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 5030 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5031 protected List<CodeableConcept> modifier; 5032 5033 /** 5034 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 5035 */ 5036 @Child(name = "programCode", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5037 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 5038 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5039 protected List<CodeableConcept> programCode; 5040 5041 /** 5042 * The number of repetitions of a service or product. 5043 */ 5044 @Child(name = "quantity", type = {SimpleQuantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 5045 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 5046 protected SimpleQuantity quantity; 5047 5048 /** 5049 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 5050 */ 5051 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 5052 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 5053 protected Money unitPrice; 5054 5055 /** 5056 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5057 */ 5058 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5059 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5060 protected DecimalType factor; 5061 5062 /** 5063 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 5064 */ 5065 @Child(name = "net", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 5066 @Description(shortDefinition="Total additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 5067 protected Money net; 5068 5069 /** 5070 * List of Unique Device Identifiers associated with this line item. 5071 */ 5072 @Child(name = "udi", type = {Device.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5073 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 5074 protected List<Reference> udi; 5075 /** 5076 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 5077 */ 5078 protected List<Device> udiTarget; 5079 5080 5081 /** 5082 * Third tier of goods and services. 5083 */ 5084 @Child(name = "subDetail", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5085 @Description(shortDefinition="Additional items", formalDefinition="Third tier of goods and services." ) 5086 protected List<SubDetailComponent> subDetail; 5087 5088 private static final long serialVersionUID = 718584656L; 5089 5090 /** 5091 * Constructor 5092 */ 5093 public DetailComponent() { 5094 super(); 5095 } 5096 5097 /** 5098 * Constructor 5099 */ 5100 public DetailComponent(PositiveIntType sequence) { 5101 super(); 5102 this.sequence = sequence; 5103 } 5104 5105 /** 5106 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5107 */ 5108 public PositiveIntType getSequenceElement() { 5109 if (this.sequence == null) 5110 if (Configuration.errorOnAutoCreate()) 5111 throw new Error("Attempt to auto-create DetailComponent.sequence"); 5112 else if (Configuration.doAutoCreate()) 5113 this.sequence = new PositiveIntType(); // bb 5114 return this.sequence; 5115 } 5116 5117 public boolean hasSequenceElement() { 5118 return this.sequence != null && !this.sequence.isEmpty(); 5119 } 5120 5121 public boolean hasSequence() { 5122 return this.sequence != null && !this.sequence.isEmpty(); 5123 } 5124 5125 /** 5126 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5127 */ 5128 public DetailComponent setSequenceElement(PositiveIntType value) { 5129 this.sequence = value; 5130 return this; 5131 } 5132 5133 /** 5134 * @return A service line number. 5135 */ 5136 public int getSequence() { 5137 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 5138 } 5139 5140 /** 5141 * @param value A service line number. 5142 */ 5143 public DetailComponent setSequence(int value) { 5144 if (this.sequence == null) 5145 this.sequence = new PositiveIntType(); 5146 this.sequence.setValue(value); 5147 return this; 5148 } 5149 5150 /** 5151 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5152 */ 5153 public CodeableConcept getRevenue() { 5154 if (this.revenue == null) 5155 if (Configuration.errorOnAutoCreate()) 5156 throw new Error("Attempt to auto-create DetailComponent.revenue"); 5157 else if (Configuration.doAutoCreate()) 5158 this.revenue = new CodeableConcept(); // cc 5159 return this.revenue; 5160 } 5161 5162 public boolean hasRevenue() { 5163 return this.revenue != null && !this.revenue.isEmpty(); 5164 } 5165 5166 /** 5167 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5168 */ 5169 public DetailComponent setRevenue(CodeableConcept value) { 5170 this.revenue = value; 5171 return this; 5172 } 5173 5174 /** 5175 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5176 */ 5177 public CodeableConcept getCategory() { 5178 if (this.category == null) 5179 if (Configuration.errorOnAutoCreate()) 5180 throw new Error("Attempt to auto-create DetailComponent.category"); 5181 else if (Configuration.doAutoCreate()) 5182 this.category = new CodeableConcept(); // cc 5183 return this.category; 5184 } 5185 5186 public boolean hasCategory() { 5187 return this.category != null && !this.category.isEmpty(); 5188 } 5189 5190 /** 5191 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5192 */ 5193 public DetailComponent setCategory(CodeableConcept value) { 5194 this.category = value; 5195 return this; 5196 } 5197 5198 /** 5199 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5200 */ 5201 public CodeableConcept getService() { 5202 if (this.service == null) 5203 if (Configuration.errorOnAutoCreate()) 5204 throw new Error("Attempt to auto-create DetailComponent.service"); 5205 else if (Configuration.doAutoCreate()) 5206 this.service = new CodeableConcept(); // cc 5207 return this.service; 5208 } 5209 5210 public boolean hasService() { 5211 return this.service != null && !this.service.isEmpty(); 5212 } 5213 5214 /** 5215 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5216 */ 5217 public DetailComponent setService(CodeableConcept value) { 5218 this.service = value; 5219 return this; 5220 } 5221 5222 /** 5223 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 5224 */ 5225 public List<CodeableConcept> getModifier() { 5226 if (this.modifier == null) 5227 this.modifier = new ArrayList<CodeableConcept>(); 5228 return this.modifier; 5229 } 5230 5231 /** 5232 * @return Returns a reference to <code>this</code> for easy method chaining 5233 */ 5234 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 5235 this.modifier = theModifier; 5236 return this; 5237 } 5238 5239 public boolean hasModifier() { 5240 if (this.modifier == null) 5241 return false; 5242 for (CodeableConcept item : this.modifier) 5243 if (!item.isEmpty()) 5244 return true; 5245 return false; 5246 } 5247 5248 public CodeableConcept addModifier() { //3 5249 CodeableConcept t = new CodeableConcept(); 5250 if (this.modifier == null) 5251 this.modifier = new ArrayList<CodeableConcept>(); 5252 this.modifier.add(t); 5253 return t; 5254 } 5255 5256 public DetailComponent addModifier(CodeableConcept t) { //3 5257 if (t == null) 5258 return this; 5259 if (this.modifier == null) 5260 this.modifier = new ArrayList<CodeableConcept>(); 5261 this.modifier.add(t); 5262 return this; 5263 } 5264 5265 /** 5266 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 5267 */ 5268 public CodeableConcept getModifierFirstRep() { 5269 if (getModifier().isEmpty()) { 5270 addModifier(); 5271 } 5272 return getModifier().get(0); 5273 } 5274 5275 /** 5276 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 5277 */ 5278 public List<CodeableConcept> getProgramCode() { 5279 if (this.programCode == null) 5280 this.programCode = new ArrayList<CodeableConcept>(); 5281 return this.programCode; 5282 } 5283 5284 /** 5285 * @return Returns a reference to <code>this</code> for easy method chaining 5286 */ 5287 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 5288 this.programCode = theProgramCode; 5289 return this; 5290 } 5291 5292 public boolean hasProgramCode() { 5293 if (this.programCode == null) 5294 return false; 5295 for (CodeableConcept item : this.programCode) 5296 if (!item.isEmpty()) 5297 return true; 5298 return false; 5299 } 5300 5301 public CodeableConcept addProgramCode() { //3 5302 CodeableConcept t = new CodeableConcept(); 5303 if (this.programCode == null) 5304 this.programCode = new ArrayList<CodeableConcept>(); 5305 this.programCode.add(t); 5306 return t; 5307 } 5308 5309 public DetailComponent addProgramCode(CodeableConcept t) { //3 5310 if (t == null) 5311 return this; 5312 if (this.programCode == null) 5313 this.programCode = new ArrayList<CodeableConcept>(); 5314 this.programCode.add(t); 5315 return this; 5316 } 5317 5318 /** 5319 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 5320 */ 5321 public CodeableConcept getProgramCodeFirstRep() { 5322 if (getProgramCode().isEmpty()) { 5323 addProgramCode(); 5324 } 5325 return getProgramCode().get(0); 5326 } 5327 5328 /** 5329 * @return {@link #quantity} (The number of repetitions of a service or product.) 5330 */ 5331 public SimpleQuantity getQuantity() { 5332 if (this.quantity == null) 5333 if (Configuration.errorOnAutoCreate()) 5334 throw new Error("Attempt to auto-create DetailComponent.quantity"); 5335 else if (Configuration.doAutoCreate()) 5336 this.quantity = new SimpleQuantity(); // cc 5337 return this.quantity; 5338 } 5339 5340 public boolean hasQuantity() { 5341 return this.quantity != null && !this.quantity.isEmpty(); 5342 } 5343 5344 /** 5345 * @param value {@link #quantity} (The number of repetitions of a service or product.) 5346 */ 5347 public DetailComponent setQuantity(SimpleQuantity value) { 5348 this.quantity = value; 5349 return this; 5350 } 5351 5352 /** 5353 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5354 */ 5355 public Money getUnitPrice() { 5356 if (this.unitPrice == null) 5357 if (Configuration.errorOnAutoCreate()) 5358 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 5359 else if (Configuration.doAutoCreate()) 5360 this.unitPrice = new Money(); // cc 5361 return this.unitPrice; 5362 } 5363 5364 public boolean hasUnitPrice() { 5365 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5366 } 5367 5368 /** 5369 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5370 */ 5371 public DetailComponent setUnitPrice(Money value) { 5372 this.unitPrice = value; 5373 return this; 5374 } 5375 5376 /** 5377 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5378 */ 5379 public DecimalType getFactorElement() { 5380 if (this.factor == null) 5381 if (Configuration.errorOnAutoCreate()) 5382 throw new Error("Attempt to auto-create DetailComponent.factor"); 5383 else if (Configuration.doAutoCreate()) 5384 this.factor = new DecimalType(); // bb 5385 return this.factor; 5386 } 5387 5388 public boolean hasFactorElement() { 5389 return this.factor != null && !this.factor.isEmpty(); 5390 } 5391 5392 public boolean hasFactor() { 5393 return this.factor != null && !this.factor.isEmpty(); 5394 } 5395 5396 /** 5397 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5398 */ 5399 public DetailComponent setFactorElement(DecimalType value) { 5400 this.factor = value; 5401 return this; 5402 } 5403 5404 /** 5405 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5406 */ 5407 public BigDecimal getFactor() { 5408 return this.factor == null ? null : this.factor.getValue(); 5409 } 5410 5411 /** 5412 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5413 */ 5414 public DetailComponent setFactor(BigDecimal value) { 5415 if (value == null) 5416 this.factor = null; 5417 else { 5418 if (this.factor == null) 5419 this.factor = new DecimalType(); 5420 this.factor.setValue(value); 5421 } 5422 return this; 5423 } 5424 5425 /** 5426 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5427 */ 5428 public DetailComponent setFactor(long value) { 5429 this.factor = new DecimalType(); 5430 this.factor.setValue(value); 5431 return this; 5432 } 5433 5434 /** 5435 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5436 */ 5437 public DetailComponent setFactor(double value) { 5438 this.factor = new DecimalType(); 5439 this.factor.setValue(value); 5440 return this; 5441 } 5442 5443 /** 5444 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5445 */ 5446 public Money getNet() { 5447 if (this.net == null) 5448 if (Configuration.errorOnAutoCreate()) 5449 throw new Error("Attempt to auto-create DetailComponent.net"); 5450 else if (Configuration.doAutoCreate()) 5451 this.net = new Money(); // cc 5452 return this.net; 5453 } 5454 5455 public boolean hasNet() { 5456 return this.net != null && !this.net.isEmpty(); 5457 } 5458 5459 /** 5460 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5461 */ 5462 public DetailComponent setNet(Money value) { 5463 this.net = value; 5464 return this; 5465 } 5466 5467 /** 5468 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 5469 */ 5470 public List<Reference> getUdi() { 5471 if (this.udi == null) 5472 this.udi = new ArrayList<Reference>(); 5473 return this.udi; 5474 } 5475 5476 /** 5477 * @return Returns a reference to <code>this</code> for easy method chaining 5478 */ 5479 public DetailComponent setUdi(List<Reference> theUdi) { 5480 this.udi = theUdi; 5481 return this; 5482 } 5483 5484 public boolean hasUdi() { 5485 if (this.udi == null) 5486 return false; 5487 for (Reference item : this.udi) 5488 if (!item.isEmpty()) 5489 return true; 5490 return false; 5491 } 5492 5493 public Reference addUdi() { //3 5494 Reference t = new Reference(); 5495 if (this.udi == null) 5496 this.udi = new ArrayList<Reference>(); 5497 this.udi.add(t); 5498 return t; 5499 } 5500 5501 public DetailComponent addUdi(Reference t) { //3 5502 if (t == null) 5503 return this; 5504 if (this.udi == null) 5505 this.udi = new ArrayList<Reference>(); 5506 this.udi.add(t); 5507 return this; 5508 } 5509 5510 /** 5511 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 5512 */ 5513 public Reference getUdiFirstRep() { 5514 if (getUdi().isEmpty()) { 5515 addUdi(); 5516 } 5517 return getUdi().get(0); 5518 } 5519 5520 /** 5521 * @return {@link #subDetail} (Third tier of goods and services.) 5522 */ 5523 public List<SubDetailComponent> getSubDetail() { 5524 if (this.subDetail == null) 5525 this.subDetail = new ArrayList<SubDetailComponent>(); 5526 return this.subDetail; 5527 } 5528 5529 /** 5530 * @return Returns a reference to <code>this</code> for easy method chaining 5531 */ 5532 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 5533 this.subDetail = theSubDetail; 5534 return this; 5535 } 5536 5537 public boolean hasSubDetail() { 5538 if (this.subDetail == null) 5539 return false; 5540 for (SubDetailComponent item : this.subDetail) 5541 if (!item.isEmpty()) 5542 return true; 5543 return false; 5544 } 5545 5546 public SubDetailComponent addSubDetail() { //3 5547 SubDetailComponent t = new SubDetailComponent(); 5548 if (this.subDetail == null) 5549 this.subDetail = new ArrayList<SubDetailComponent>(); 5550 this.subDetail.add(t); 5551 return t; 5552 } 5553 5554 public DetailComponent addSubDetail(SubDetailComponent t) { //3 5555 if (t == null) 5556 return this; 5557 if (this.subDetail == null) 5558 this.subDetail = new ArrayList<SubDetailComponent>(); 5559 this.subDetail.add(t); 5560 return this; 5561 } 5562 5563 /** 5564 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist 5565 */ 5566 public SubDetailComponent getSubDetailFirstRep() { 5567 if (getSubDetail().isEmpty()) { 5568 addSubDetail(); 5569 } 5570 return getSubDetail().get(0); 5571 } 5572 5573 protected void listChildren(List<Property> children) { 5574 super.listChildren(children); 5575 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 5576 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 5577 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 5578 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 5579 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 5580 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 5581 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 5582 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 5583 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 5584 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 5585 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 5586 children.add(new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 5587 } 5588 5589 @Override 5590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5591 switch (_hash) { 5592 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 5593 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 5594 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 5595 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 5596 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 5597 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 5598 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 5599 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 5600 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 5601 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 5602 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5603 case -828829007: /*subDetail*/ return new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 5604 default: return super.getNamedProperty(_hash, _name, _checkValid); 5605 } 5606 5607 } 5608 5609 @Override 5610 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5611 switch (hash) { 5612 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 5613 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 5614 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 5615 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 5616 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5617 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5618 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 5619 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 5620 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 5621 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 5622 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5623 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 5624 default: return super.getProperty(hash, name, checkValid); 5625 } 5626 5627 } 5628 5629 @Override 5630 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5631 switch (hash) { 5632 case 1349547969: // sequence 5633 this.sequence = castToPositiveInt(value); // PositiveIntType 5634 return value; 5635 case 1099842588: // revenue 5636 this.revenue = castToCodeableConcept(value); // CodeableConcept 5637 return value; 5638 case 50511102: // category 5639 this.category = castToCodeableConcept(value); // CodeableConcept 5640 return value; 5641 case 1984153269: // service 5642 this.service = castToCodeableConcept(value); // CodeableConcept 5643 return value; 5644 case -615513385: // modifier 5645 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5646 return value; 5647 case 1010065041: // programCode 5648 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 5649 return value; 5650 case -1285004149: // quantity 5651 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 5652 return value; 5653 case -486196699: // unitPrice 5654 this.unitPrice = castToMoney(value); // Money 5655 return value; 5656 case -1282148017: // factor 5657 this.factor = castToDecimal(value); // DecimalType 5658 return value; 5659 case 108957: // net 5660 this.net = castToMoney(value); // Money 5661 return value; 5662 case 115642: // udi 5663 this.getUdi().add(castToReference(value)); // Reference 5664 return value; 5665 case -828829007: // subDetail 5666 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 5667 return value; 5668 default: return super.setProperty(hash, name, value); 5669 } 5670 5671 } 5672 5673 @Override 5674 public Base setProperty(String name, Base value) throws FHIRException { 5675 if (name.equals("sequence")) { 5676 this.sequence = castToPositiveInt(value); // PositiveIntType 5677 } else if (name.equals("revenue")) { 5678 this.revenue = castToCodeableConcept(value); // CodeableConcept 5679 } else if (name.equals("category")) { 5680 this.category = castToCodeableConcept(value); // CodeableConcept 5681 } else if (name.equals("service")) { 5682 this.service = castToCodeableConcept(value); // CodeableConcept 5683 } else if (name.equals("modifier")) { 5684 this.getModifier().add(castToCodeableConcept(value)); 5685 } else if (name.equals("programCode")) { 5686 this.getProgramCode().add(castToCodeableConcept(value)); 5687 } else if (name.equals("quantity")) { 5688 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 5689 } else if (name.equals("unitPrice")) { 5690 this.unitPrice = castToMoney(value); // Money 5691 } else if (name.equals("factor")) { 5692 this.factor = castToDecimal(value); // DecimalType 5693 } else if (name.equals("net")) { 5694 this.net = castToMoney(value); // Money 5695 } else if (name.equals("udi")) { 5696 this.getUdi().add(castToReference(value)); 5697 } else if (name.equals("subDetail")) { 5698 this.getSubDetail().add((SubDetailComponent) value); 5699 } else 5700 return super.setProperty(name, value); 5701 return value; 5702 } 5703 5704 @Override 5705 public Base makeProperty(int hash, String name) throws FHIRException { 5706 switch (hash) { 5707 case 1349547969: return getSequenceElement(); 5708 case 1099842588: return getRevenue(); 5709 case 50511102: return getCategory(); 5710 case 1984153269: return getService(); 5711 case -615513385: return addModifier(); 5712 case 1010065041: return addProgramCode(); 5713 case -1285004149: return getQuantity(); 5714 case -486196699: return getUnitPrice(); 5715 case -1282148017: return getFactorElement(); 5716 case 108957: return getNet(); 5717 case 115642: return addUdi(); 5718 case -828829007: return addSubDetail(); 5719 default: return super.makeProperty(hash, name); 5720 } 5721 5722 } 5723 5724 @Override 5725 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5726 switch (hash) { 5727 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 5728 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 5729 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5730 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 5731 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 5732 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 5733 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 5734 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 5735 case -1282148017: /*factor*/ return new String[] {"decimal"}; 5736 case 108957: /*net*/ return new String[] {"Money"}; 5737 case 115642: /*udi*/ return new String[] {"Reference"}; 5738 case -828829007: /*subDetail*/ return new String[] {}; 5739 default: return super.getTypesForProperty(hash, name); 5740 } 5741 5742 } 5743 5744 @Override 5745 public Base addChild(String name) throws FHIRException { 5746 if (name.equals("sequence")) { 5747 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 5748 } 5749 else if (name.equals("revenue")) { 5750 this.revenue = new CodeableConcept(); 5751 return this.revenue; 5752 } 5753 else if (name.equals("category")) { 5754 this.category = new CodeableConcept(); 5755 return this.category; 5756 } 5757 else if (name.equals("service")) { 5758 this.service = new CodeableConcept(); 5759 return this.service; 5760 } 5761 else if (name.equals("modifier")) { 5762 return addModifier(); 5763 } 5764 else if (name.equals("programCode")) { 5765 return addProgramCode(); 5766 } 5767 else if (name.equals("quantity")) { 5768 this.quantity = new SimpleQuantity(); 5769 return this.quantity; 5770 } 5771 else if (name.equals("unitPrice")) { 5772 this.unitPrice = new Money(); 5773 return this.unitPrice; 5774 } 5775 else if (name.equals("factor")) { 5776 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 5777 } 5778 else if (name.equals("net")) { 5779 this.net = new Money(); 5780 return this.net; 5781 } 5782 else if (name.equals("udi")) { 5783 return addUdi(); 5784 } 5785 else if (name.equals("subDetail")) { 5786 return addSubDetail(); 5787 } 5788 else 5789 return super.addChild(name); 5790 } 5791 5792 public DetailComponent copy() { 5793 DetailComponent dst = new DetailComponent(); 5794 copyValues(dst); 5795 dst.sequence = sequence == null ? null : sequence.copy(); 5796 dst.revenue = revenue == null ? null : revenue.copy(); 5797 dst.category = category == null ? null : category.copy(); 5798 dst.service = service == null ? null : service.copy(); 5799 if (modifier != null) { 5800 dst.modifier = new ArrayList<CodeableConcept>(); 5801 for (CodeableConcept i : modifier) 5802 dst.modifier.add(i.copy()); 5803 }; 5804 if (programCode != null) { 5805 dst.programCode = new ArrayList<CodeableConcept>(); 5806 for (CodeableConcept i : programCode) 5807 dst.programCode.add(i.copy()); 5808 }; 5809 dst.quantity = quantity == null ? null : quantity.copy(); 5810 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5811 dst.factor = factor == null ? null : factor.copy(); 5812 dst.net = net == null ? null : net.copy(); 5813 if (udi != null) { 5814 dst.udi = new ArrayList<Reference>(); 5815 for (Reference i : udi) 5816 dst.udi.add(i.copy()); 5817 }; 5818 if (subDetail != null) { 5819 dst.subDetail = new ArrayList<SubDetailComponent>(); 5820 for (SubDetailComponent i : subDetail) 5821 dst.subDetail.add(i.copy()); 5822 }; 5823 return dst; 5824 } 5825 5826 @Override 5827 public boolean equalsDeep(Base other_) { 5828 if (!super.equalsDeep(other_)) 5829 return false; 5830 if (!(other_ instanceof DetailComponent)) 5831 return false; 5832 DetailComponent o = (DetailComponent) other_; 5833 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) 5834 && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 5835 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 5836 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(subDetail, o.subDetail, true) 5837 ; 5838 } 5839 5840 @Override 5841 public boolean equalsShallow(Base other_) { 5842 if (!super.equalsShallow(other_)) 5843 return false; 5844 if (!(other_ instanceof DetailComponent)) 5845 return false; 5846 DetailComponent o = (DetailComponent) other_; 5847 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 5848 } 5849 5850 public boolean isEmpty() { 5851 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category 5852 , service, modifier, programCode, quantity, unitPrice, factor, net, udi, subDetail 5853 ); 5854 } 5855 5856 public String fhirType() { 5857 return "Claim.item.detail"; 5858 5859 } 5860 5861 } 5862 5863 @Block() 5864 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 5865 /** 5866 * A service line number. 5867 */ 5868 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5869 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 5870 protected PositiveIntType sequence; 5871 5872 /** 5873 * The type of reveneu or cost center providing the product and/or service. 5874 */ 5875 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 5876 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 5877 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5878 protected CodeableConcept revenue; 5879 5880 /** 5881 * Health Care Service Type Codes to identify the classification of service or benefits. 5882 */ 5883 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5884 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 5885 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 5886 protected CodeableConcept category; 5887 5888 /** 5889 * A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). 5890 */ 5891 @Child(name = "service", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5892 @Description(shortDefinition="Billing Code", formalDefinition="A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI)." ) 5893 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5894 protected CodeableConcept service; 5895 5896 /** 5897 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 5898 */ 5899 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5900 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 5901 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5902 protected List<CodeableConcept> modifier; 5903 5904 /** 5905 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 5906 */ 5907 @Child(name = "programCode", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5908 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 5909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5910 protected List<CodeableConcept> programCode; 5911 5912 /** 5913 * The number of repetitions of a service or product. 5914 */ 5915 @Child(name = "quantity", type = {SimpleQuantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 5916 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 5917 protected SimpleQuantity quantity; 5918 5919 /** 5920 * The fee for an addittional service or product or charge. 5921 */ 5922 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 5923 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="The fee for an addittional service or product or charge." ) 5924 protected Money unitPrice; 5925 5926 /** 5927 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5928 */ 5929 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5930 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5931 protected DecimalType factor; 5932 5933 /** 5934 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 5935 */ 5936 @Child(name = "net", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 5937 @Description(shortDefinition="Net additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 5938 protected Money net; 5939 5940 /** 5941 * List of Unique Device Identifiers associated with this line item. 5942 */ 5943 @Child(name = "udi", type = {Device.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5944 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 5945 protected List<Reference> udi; 5946 /** 5947 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 5948 */ 5949 protected List<Device> udiTarget; 5950 5951 5952 private static final long serialVersionUID = -1210367567L; 5953 5954 /** 5955 * Constructor 5956 */ 5957 public SubDetailComponent() { 5958 super(); 5959 } 5960 5961 /** 5962 * Constructor 5963 */ 5964 public SubDetailComponent(PositiveIntType sequence) { 5965 super(); 5966 this.sequence = sequence; 5967 } 5968 5969 /** 5970 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5971 */ 5972 public PositiveIntType getSequenceElement() { 5973 if (this.sequence == null) 5974 if (Configuration.errorOnAutoCreate()) 5975 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 5976 else if (Configuration.doAutoCreate()) 5977 this.sequence = new PositiveIntType(); // bb 5978 return this.sequence; 5979 } 5980 5981 public boolean hasSequenceElement() { 5982 return this.sequence != null && !this.sequence.isEmpty(); 5983 } 5984 5985 public boolean hasSequence() { 5986 return this.sequence != null && !this.sequence.isEmpty(); 5987 } 5988 5989 /** 5990 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5991 */ 5992 public SubDetailComponent setSequenceElement(PositiveIntType value) { 5993 this.sequence = value; 5994 return this; 5995 } 5996 5997 /** 5998 * @return A service line number. 5999 */ 6000 public int getSequence() { 6001 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6002 } 6003 6004 /** 6005 * @param value A service line number. 6006 */ 6007 public SubDetailComponent setSequence(int value) { 6008 if (this.sequence == null) 6009 this.sequence = new PositiveIntType(); 6010 this.sequence.setValue(value); 6011 return this; 6012 } 6013 6014 /** 6015 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6016 */ 6017 public CodeableConcept getRevenue() { 6018 if (this.revenue == null) 6019 if (Configuration.errorOnAutoCreate()) 6020 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 6021 else if (Configuration.doAutoCreate()) 6022 this.revenue = new CodeableConcept(); // cc 6023 return this.revenue; 6024 } 6025 6026 public boolean hasRevenue() { 6027 return this.revenue != null && !this.revenue.isEmpty(); 6028 } 6029 6030 /** 6031 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6032 */ 6033 public SubDetailComponent setRevenue(CodeableConcept value) { 6034 this.revenue = value; 6035 return this; 6036 } 6037 6038 /** 6039 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6040 */ 6041 public CodeableConcept getCategory() { 6042 if (this.category == null) 6043 if (Configuration.errorOnAutoCreate()) 6044 throw new Error("Attempt to auto-create SubDetailComponent.category"); 6045 else if (Configuration.doAutoCreate()) 6046 this.category = new CodeableConcept(); // cc 6047 return this.category; 6048 } 6049 6050 public boolean hasCategory() { 6051 return this.category != null && !this.category.isEmpty(); 6052 } 6053 6054 /** 6055 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6056 */ 6057 public SubDetailComponent setCategory(CodeableConcept value) { 6058 this.category = value; 6059 return this; 6060 } 6061 6062 /** 6063 * @return {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6064 */ 6065 public CodeableConcept getService() { 6066 if (this.service == null) 6067 if (Configuration.errorOnAutoCreate()) 6068 throw new Error("Attempt to auto-create SubDetailComponent.service"); 6069 else if (Configuration.doAutoCreate()) 6070 this.service = new CodeableConcept(); // cc 6071 return this.service; 6072 } 6073 6074 public boolean hasService() { 6075 return this.service != null && !this.service.isEmpty(); 6076 } 6077 6078 /** 6079 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6080 */ 6081 public SubDetailComponent setService(CodeableConcept value) { 6082 this.service = value; 6083 return this; 6084 } 6085 6086 /** 6087 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 6088 */ 6089 public List<CodeableConcept> getModifier() { 6090 if (this.modifier == null) 6091 this.modifier = new ArrayList<CodeableConcept>(); 6092 return this.modifier; 6093 } 6094 6095 /** 6096 * @return Returns a reference to <code>this</code> for easy method chaining 6097 */ 6098 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 6099 this.modifier = theModifier; 6100 return this; 6101 } 6102 6103 public boolean hasModifier() { 6104 if (this.modifier == null) 6105 return false; 6106 for (CodeableConcept item : this.modifier) 6107 if (!item.isEmpty()) 6108 return true; 6109 return false; 6110 } 6111 6112 public CodeableConcept addModifier() { //3 6113 CodeableConcept t = new CodeableConcept(); 6114 if (this.modifier == null) 6115 this.modifier = new ArrayList<CodeableConcept>(); 6116 this.modifier.add(t); 6117 return t; 6118 } 6119 6120 public SubDetailComponent addModifier(CodeableConcept t) { //3 6121 if (t == null) 6122 return this; 6123 if (this.modifier == null) 6124 this.modifier = new ArrayList<CodeableConcept>(); 6125 this.modifier.add(t); 6126 return this; 6127 } 6128 6129 /** 6130 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 6131 */ 6132 public CodeableConcept getModifierFirstRep() { 6133 if (getModifier().isEmpty()) { 6134 addModifier(); 6135 } 6136 return getModifier().get(0); 6137 } 6138 6139 /** 6140 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 6141 */ 6142 public List<CodeableConcept> getProgramCode() { 6143 if (this.programCode == null) 6144 this.programCode = new ArrayList<CodeableConcept>(); 6145 return this.programCode; 6146 } 6147 6148 /** 6149 * @return Returns a reference to <code>this</code> for easy method chaining 6150 */ 6151 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6152 this.programCode = theProgramCode; 6153 return this; 6154 } 6155 6156 public boolean hasProgramCode() { 6157 if (this.programCode == null) 6158 return false; 6159 for (CodeableConcept item : this.programCode) 6160 if (!item.isEmpty()) 6161 return true; 6162 return false; 6163 } 6164 6165 public CodeableConcept addProgramCode() { //3 6166 CodeableConcept t = new CodeableConcept(); 6167 if (this.programCode == null) 6168 this.programCode = new ArrayList<CodeableConcept>(); 6169 this.programCode.add(t); 6170 return t; 6171 } 6172 6173 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 6174 if (t == null) 6175 return this; 6176 if (this.programCode == null) 6177 this.programCode = new ArrayList<CodeableConcept>(); 6178 this.programCode.add(t); 6179 return this; 6180 } 6181 6182 /** 6183 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 6184 */ 6185 public CodeableConcept getProgramCodeFirstRep() { 6186 if (getProgramCode().isEmpty()) { 6187 addProgramCode(); 6188 } 6189 return getProgramCode().get(0); 6190 } 6191 6192 /** 6193 * @return {@link #quantity} (The number of repetitions of a service or product.) 6194 */ 6195 public SimpleQuantity getQuantity() { 6196 if (this.quantity == null) 6197 if (Configuration.errorOnAutoCreate()) 6198 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 6199 else if (Configuration.doAutoCreate()) 6200 this.quantity = new SimpleQuantity(); // cc 6201 return this.quantity; 6202 } 6203 6204 public boolean hasQuantity() { 6205 return this.quantity != null && !this.quantity.isEmpty(); 6206 } 6207 6208 /** 6209 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6210 */ 6211 public SubDetailComponent setQuantity(SimpleQuantity value) { 6212 this.quantity = value; 6213 return this; 6214 } 6215 6216 /** 6217 * @return {@link #unitPrice} (The fee for an addittional service or product or charge.) 6218 */ 6219 public Money getUnitPrice() { 6220 if (this.unitPrice == null) 6221 if (Configuration.errorOnAutoCreate()) 6222 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 6223 else if (Configuration.doAutoCreate()) 6224 this.unitPrice = new Money(); // cc 6225 return this.unitPrice; 6226 } 6227 6228 public boolean hasUnitPrice() { 6229 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6230 } 6231 6232 /** 6233 * @param value {@link #unitPrice} (The fee for an addittional service or product or charge.) 6234 */ 6235 public SubDetailComponent setUnitPrice(Money value) { 6236 this.unitPrice = value; 6237 return this; 6238 } 6239 6240 /** 6241 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6242 */ 6243 public DecimalType getFactorElement() { 6244 if (this.factor == null) 6245 if (Configuration.errorOnAutoCreate()) 6246 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 6247 else if (Configuration.doAutoCreate()) 6248 this.factor = new DecimalType(); // bb 6249 return this.factor; 6250 } 6251 6252 public boolean hasFactorElement() { 6253 return this.factor != null && !this.factor.isEmpty(); 6254 } 6255 6256 public boolean hasFactor() { 6257 return this.factor != null && !this.factor.isEmpty(); 6258 } 6259 6260 /** 6261 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6262 */ 6263 public SubDetailComponent setFactorElement(DecimalType value) { 6264 this.factor = value; 6265 return this; 6266 } 6267 6268 /** 6269 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6270 */ 6271 public BigDecimal getFactor() { 6272 return this.factor == null ? null : this.factor.getValue(); 6273 } 6274 6275 /** 6276 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6277 */ 6278 public SubDetailComponent setFactor(BigDecimal value) { 6279 if (value == null) 6280 this.factor = null; 6281 else { 6282 if (this.factor == null) 6283 this.factor = new DecimalType(); 6284 this.factor.setValue(value); 6285 } 6286 return this; 6287 } 6288 6289 /** 6290 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6291 */ 6292 public SubDetailComponent setFactor(long value) { 6293 this.factor = new DecimalType(); 6294 this.factor.setValue(value); 6295 return this; 6296 } 6297 6298 /** 6299 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6300 */ 6301 public SubDetailComponent setFactor(double value) { 6302 this.factor = new DecimalType(); 6303 this.factor.setValue(value); 6304 return this; 6305 } 6306 6307 /** 6308 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6309 */ 6310 public Money getNet() { 6311 if (this.net == null) 6312 if (Configuration.errorOnAutoCreate()) 6313 throw new Error("Attempt to auto-create SubDetailComponent.net"); 6314 else if (Configuration.doAutoCreate()) 6315 this.net = new Money(); // cc 6316 return this.net; 6317 } 6318 6319 public boolean hasNet() { 6320 return this.net != null && !this.net.isEmpty(); 6321 } 6322 6323 /** 6324 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6325 */ 6326 public SubDetailComponent setNet(Money value) { 6327 this.net = value; 6328 return this; 6329 } 6330 6331 /** 6332 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 6333 */ 6334 public List<Reference> getUdi() { 6335 if (this.udi == null) 6336 this.udi = new ArrayList<Reference>(); 6337 return this.udi; 6338 } 6339 6340 /** 6341 * @return Returns a reference to <code>this</code> for easy method chaining 6342 */ 6343 public SubDetailComponent setUdi(List<Reference> theUdi) { 6344 this.udi = theUdi; 6345 return this; 6346 } 6347 6348 public boolean hasUdi() { 6349 if (this.udi == null) 6350 return false; 6351 for (Reference item : this.udi) 6352 if (!item.isEmpty()) 6353 return true; 6354 return false; 6355 } 6356 6357 public Reference addUdi() { //3 6358 Reference t = new Reference(); 6359 if (this.udi == null) 6360 this.udi = new ArrayList<Reference>(); 6361 this.udi.add(t); 6362 return t; 6363 } 6364 6365 public SubDetailComponent addUdi(Reference t) { //3 6366 if (t == null) 6367 return this; 6368 if (this.udi == null) 6369 this.udi = new ArrayList<Reference>(); 6370 this.udi.add(t); 6371 return this; 6372 } 6373 6374 /** 6375 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 6376 */ 6377 public Reference getUdiFirstRep() { 6378 if (getUdi().isEmpty()) { 6379 addUdi(); 6380 } 6381 return getUdi().get(0); 6382 } 6383 6384 protected void listChildren(List<Property> children) { 6385 super.listChildren(children); 6386 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 6387 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 6388 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 6389 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service)); 6390 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 6391 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 6392 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 6393 children.add(new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice)); 6394 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6395 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 6396 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 6397 } 6398 6399 @Override 6400 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6401 switch (_hash) { 6402 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 6403 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 6404 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 6405 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service); 6406 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 6407 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 6408 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 6409 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice); 6410 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6411 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 6412 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 6413 default: return super.getNamedProperty(_hash, _name, _checkValid); 6414 } 6415 6416 } 6417 6418 @Override 6419 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6420 switch (hash) { 6421 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 6422 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 6423 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6424 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 6425 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 6426 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 6427 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 6428 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6429 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6430 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6431 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 6432 default: return super.getProperty(hash, name, checkValid); 6433 } 6434 6435 } 6436 6437 @Override 6438 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6439 switch (hash) { 6440 case 1349547969: // sequence 6441 this.sequence = castToPositiveInt(value); // PositiveIntType 6442 return value; 6443 case 1099842588: // revenue 6444 this.revenue = castToCodeableConcept(value); // CodeableConcept 6445 return value; 6446 case 50511102: // category 6447 this.category = castToCodeableConcept(value); // CodeableConcept 6448 return value; 6449 case 1984153269: // service 6450 this.service = castToCodeableConcept(value); // CodeableConcept 6451 return value; 6452 case -615513385: // modifier 6453 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 6454 return value; 6455 case 1010065041: // programCode 6456 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 6457 return value; 6458 case -1285004149: // quantity 6459 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 6460 return value; 6461 case -486196699: // unitPrice 6462 this.unitPrice = castToMoney(value); // Money 6463 return value; 6464 case -1282148017: // factor 6465 this.factor = castToDecimal(value); // DecimalType 6466 return value; 6467 case 108957: // net 6468 this.net = castToMoney(value); // Money 6469 return value; 6470 case 115642: // udi 6471 this.getUdi().add(castToReference(value)); // Reference 6472 return value; 6473 default: return super.setProperty(hash, name, value); 6474 } 6475 6476 } 6477 6478 @Override 6479 public Base setProperty(String name, Base value) throws FHIRException { 6480 if (name.equals("sequence")) { 6481 this.sequence = castToPositiveInt(value); // PositiveIntType 6482 } else if (name.equals("revenue")) { 6483 this.revenue = castToCodeableConcept(value); // CodeableConcept 6484 } else if (name.equals("category")) { 6485 this.category = castToCodeableConcept(value); // CodeableConcept 6486 } else if (name.equals("service")) { 6487 this.service = castToCodeableConcept(value); // CodeableConcept 6488 } else if (name.equals("modifier")) { 6489 this.getModifier().add(castToCodeableConcept(value)); 6490 } else if (name.equals("programCode")) { 6491 this.getProgramCode().add(castToCodeableConcept(value)); 6492 } else if (name.equals("quantity")) { 6493 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 6494 } else if (name.equals("unitPrice")) { 6495 this.unitPrice = castToMoney(value); // Money 6496 } else if (name.equals("factor")) { 6497 this.factor = castToDecimal(value); // DecimalType 6498 } else if (name.equals("net")) { 6499 this.net = castToMoney(value); // Money 6500 } else if (name.equals("udi")) { 6501 this.getUdi().add(castToReference(value)); 6502 } else 6503 return super.setProperty(name, value); 6504 return value; 6505 } 6506 6507 @Override 6508 public Base makeProperty(int hash, String name) throws FHIRException { 6509 switch (hash) { 6510 case 1349547969: return getSequenceElement(); 6511 case 1099842588: return getRevenue(); 6512 case 50511102: return getCategory(); 6513 case 1984153269: return getService(); 6514 case -615513385: return addModifier(); 6515 case 1010065041: return addProgramCode(); 6516 case -1285004149: return getQuantity(); 6517 case -486196699: return getUnitPrice(); 6518 case -1282148017: return getFactorElement(); 6519 case 108957: return getNet(); 6520 case 115642: return addUdi(); 6521 default: return super.makeProperty(hash, name); 6522 } 6523 6524 } 6525 6526 @Override 6527 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6528 switch (hash) { 6529 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 6530 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 6531 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6532 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 6533 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 6534 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 6535 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 6536 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6537 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6538 case 108957: /*net*/ return new String[] {"Money"}; 6539 case 115642: /*udi*/ return new String[] {"Reference"}; 6540 default: return super.getTypesForProperty(hash, name); 6541 } 6542 6543 } 6544 6545 @Override 6546 public Base addChild(String name) throws FHIRException { 6547 if (name.equals("sequence")) { 6548 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 6549 } 6550 else if (name.equals("revenue")) { 6551 this.revenue = new CodeableConcept(); 6552 return this.revenue; 6553 } 6554 else if (name.equals("category")) { 6555 this.category = new CodeableConcept(); 6556 return this.category; 6557 } 6558 else if (name.equals("service")) { 6559 this.service = new CodeableConcept(); 6560 return this.service; 6561 } 6562 else if (name.equals("modifier")) { 6563 return addModifier(); 6564 } 6565 else if (name.equals("programCode")) { 6566 return addProgramCode(); 6567 } 6568 else if (name.equals("quantity")) { 6569 this.quantity = new SimpleQuantity(); 6570 return this.quantity; 6571 } 6572 else if (name.equals("unitPrice")) { 6573 this.unitPrice = new Money(); 6574 return this.unitPrice; 6575 } 6576 else if (name.equals("factor")) { 6577 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 6578 } 6579 else if (name.equals("net")) { 6580 this.net = new Money(); 6581 return this.net; 6582 } 6583 else if (name.equals("udi")) { 6584 return addUdi(); 6585 } 6586 else 6587 return super.addChild(name); 6588 } 6589 6590 public SubDetailComponent copy() { 6591 SubDetailComponent dst = new SubDetailComponent(); 6592 copyValues(dst); 6593 dst.sequence = sequence == null ? null : sequence.copy(); 6594 dst.revenue = revenue == null ? null : revenue.copy(); 6595 dst.category = category == null ? null : category.copy(); 6596 dst.service = service == null ? null : service.copy(); 6597 if (modifier != null) { 6598 dst.modifier = new ArrayList<CodeableConcept>(); 6599 for (CodeableConcept i : modifier) 6600 dst.modifier.add(i.copy()); 6601 }; 6602 if (programCode != null) { 6603 dst.programCode = new ArrayList<CodeableConcept>(); 6604 for (CodeableConcept i : programCode) 6605 dst.programCode.add(i.copy()); 6606 }; 6607 dst.quantity = quantity == null ? null : quantity.copy(); 6608 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6609 dst.factor = factor == null ? null : factor.copy(); 6610 dst.net = net == null ? null : net.copy(); 6611 if (udi != null) { 6612 dst.udi = new ArrayList<Reference>(); 6613 for (Reference i : udi) 6614 dst.udi.add(i.copy()); 6615 }; 6616 return dst; 6617 } 6618 6619 @Override 6620 public boolean equalsDeep(Base other_) { 6621 if (!super.equalsDeep(other_)) 6622 return false; 6623 if (!(other_ instanceof SubDetailComponent)) 6624 return false; 6625 SubDetailComponent o = (SubDetailComponent) other_; 6626 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) 6627 && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 6628 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 6629 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true); 6630 } 6631 6632 @Override 6633 public boolean equalsShallow(Base other_) { 6634 if (!super.equalsShallow(other_)) 6635 return false; 6636 if (!(other_ instanceof SubDetailComponent)) 6637 return false; 6638 SubDetailComponent o = (SubDetailComponent) other_; 6639 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 6640 } 6641 6642 public boolean isEmpty() { 6643 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category 6644 , service, modifier, programCode, quantity, unitPrice, factor, net, udi); 6645 } 6646 6647 public String fhirType() { 6648 return "Claim.item.detail.subDetail"; 6649 6650 } 6651 6652 } 6653 6654 /** 6655 * The business identifier for the instance: claim number, pre-determination or pre-authorization number. 6656 */ 6657 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6658 @Description(shortDefinition="Claim number", formalDefinition="The business identifier for the instance: claim number, pre-determination or pre-authorization number." ) 6659 protected List<Identifier> identifier; 6660 6661 /** 6662 * The status of the resource instance. 6663 */ 6664 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 6665 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 6666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 6667 protected Enumeration<ClaimStatus> status; 6668 6669 /** 6670 * The category of claim, eg, oral, pharmacy, vision, insitutional, professional. 6671 */ 6672 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 6673 @Description(shortDefinition="Type or discipline", formalDefinition="The category of claim, eg, oral, pharmacy, vision, insitutional, professional." ) 6674 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 6675 protected CodeableConcept type; 6676 6677 /** 6678 * A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType. 6679 */ 6680 @Child(name = "subType", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6681 @Description(shortDefinition="Finer grained claim type information", formalDefinition="A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType." ) 6682 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 6683 protected List<CodeableConcept> subType; 6684 6685 /** 6686 * Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination). 6687 */ 6688 @Child(name = "use", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 6689 @Description(shortDefinition="complete | proposed | exploratory | other", formalDefinition="Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination)." ) 6690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-use") 6691 protected Enumeration<Use> use; 6692 6693 /** 6694 * Patient Resource. 6695 */ 6696 @Child(name = "patient", type = {Patient.class}, order=5, min=0, max=1, modifier=false, summary=false) 6697 @Description(shortDefinition="The subject of the Products and Services", formalDefinition="Patient Resource." ) 6698 protected Reference patient; 6699 6700 /** 6701 * The actual object that is the target of the reference (Patient Resource.) 6702 */ 6703 protected Patient patientTarget; 6704 6705 /** 6706 * The billable period for which charges are being submitted. 6707 */ 6708 @Child(name = "billablePeriod", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=false) 6709 @Description(shortDefinition="Period for charge submission", formalDefinition="The billable period for which charges are being submitted." ) 6710 protected Period billablePeriod; 6711 6712 /** 6713 * The date when the enclosed suite of services were performed or completed. 6714 */ 6715 @Child(name = "created", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 6716 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 6717 protected DateTimeType created; 6718 6719 /** 6720 * Person who created the invoice/claim/pre-determination or pre-authorization. 6721 */ 6722 @Child(name = "enterer", type = {Practitioner.class}, order=8, min=0, max=1, modifier=false, summary=false) 6723 @Description(shortDefinition="Author", formalDefinition="Person who created the invoice/claim/pre-determination or pre-authorization." ) 6724 protected Reference enterer; 6725 6726 /** 6727 * The actual object that is the target of the reference (Person who created the invoice/claim/pre-determination or pre-authorization.) 6728 */ 6729 protected Practitioner entererTarget; 6730 6731 /** 6732 * The Insurer who is target of the request. 6733 */ 6734 @Child(name = "insurer", type = {Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 6735 @Description(shortDefinition="Target", formalDefinition="The Insurer who is target of the request." ) 6736 protected Reference insurer; 6737 6738 /** 6739 * The actual object that is the target of the reference (The Insurer who is target of the request.) 6740 */ 6741 protected Organization insurerTarget; 6742 6743 /** 6744 * The provider which is responsible for the bill, claim pre-determination, pre-authorization. 6745 */ 6746 @Child(name = "provider", type = {Practitioner.class}, order=10, min=0, max=1, modifier=false, summary=false) 6747 @Description(shortDefinition="Responsible provider", formalDefinition="The provider which is responsible for the bill, claim pre-determination, pre-authorization." ) 6748 protected Reference provider; 6749 6750 /** 6751 * The actual object that is the target of the reference (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 6752 */ 6753 protected Practitioner providerTarget; 6754 6755 /** 6756 * The organization which is responsible for the bill, claim pre-determination, pre-authorization. 6757 */ 6758 @Child(name = "organization", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=false) 6759 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the bill, claim pre-determination, pre-authorization." ) 6760 protected Reference organization; 6761 6762 /** 6763 * The actual object that is the target of the reference (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 6764 */ 6765 protected Organization organizationTarget; 6766 6767 /** 6768 * Immediate (STAT), best effort (NORMAL), deferred (DEFER). 6769 */ 6770 @Child(name = "priority", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 6771 @Description(shortDefinition="Desired processing priority", formalDefinition="Immediate (STAT), best effort (NORMAL), deferred (DEFER)." ) 6772 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 6773 protected CodeableConcept priority; 6774 6775 /** 6776 * In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested. 6777 */ 6778 @Child(name = "fundsReserve", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 6779 @Description(shortDefinition="Funds requested to be reserved", formalDefinition="In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested." ) 6780 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 6781 protected CodeableConcept fundsReserve; 6782 6783 /** 6784 * Other claims which are related to this claim such as prior claim versions or for related services. 6785 */ 6786 @Child(name = "related", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6787 @Description(shortDefinition="Related Claims which may be revelant to processing this claimn", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 6788 protected List<RelatedClaimComponent> related; 6789 6790 /** 6791 * Prescription to support the dispensing of Pharmacy or Vision products. 6792 */ 6793 @Child(name = "prescription", type = {MedicationRequest.class, VisionPrescription.class}, order=15, min=0, max=1, modifier=false, summary=false) 6794 @Description(shortDefinition="Prescription authorizing services or products", formalDefinition="Prescription to support the dispensing of Pharmacy or Vision products." ) 6795 protected Reference prescription; 6796 6797 /** 6798 * The actual object that is the target of the reference (Prescription to support the dispensing of Pharmacy or Vision products.) 6799 */ 6800 protected Resource prescriptionTarget; 6801 6802 /** 6803 * Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'. 6804 */ 6805 @Child(name = "originalPrescription", type = {MedicationRequest.class}, order=16, min=0, max=1, modifier=false, summary=false) 6806 @Description(shortDefinition="Original prescription if superceded by fulfiller", formalDefinition="Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'." ) 6807 protected Reference originalPrescription; 6808 6809 /** 6810 * The actual object that is the target of the reference (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 6811 */ 6812 protected MedicationRequest originalPrescriptionTarget; 6813 6814 /** 6815 * The party to be reimbursed for the services. 6816 */ 6817 @Child(name = "payee", type = {}, order=17, min=0, max=1, modifier=false, summary=false) 6818 @Description(shortDefinition="Party to be paid any benefits payable", formalDefinition="The party to be reimbursed for the services." ) 6819 protected PayeeComponent payee; 6820 6821 /** 6822 * The referral resource which lists the date, practitioner, reason and other supporting information. 6823 */ 6824 @Child(name = "referral", type = {ReferralRequest.class}, order=18, min=0, max=1, modifier=false, summary=false) 6825 @Description(shortDefinition="Treatment Referral", formalDefinition="The referral resource which lists the date, practitioner, reason and other supporting information." ) 6826 protected Reference referral; 6827 6828 /** 6829 * The actual object that is the target of the reference (The referral resource which lists the date, practitioner, reason and other supporting information.) 6830 */ 6831 protected ReferralRequest referralTarget; 6832 6833 /** 6834 * Facility where the services were provided. 6835 */ 6836 @Child(name = "facility", type = {Location.class}, order=19, min=0, max=1, modifier=false, summary=false) 6837 @Description(shortDefinition="Servicing Facility", formalDefinition="Facility where the services were provided." ) 6838 protected Reference facility; 6839 6840 /** 6841 * The actual object that is the target of the reference (Facility where the services were provided.) 6842 */ 6843 protected Location facilityTarget; 6844 6845 /** 6846 * The members of the team who provided the overall service as well as their role and whether responsible and qualifications. 6847 */ 6848 @Child(name = "careTeam", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6849 @Description(shortDefinition="Members of the care team", formalDefinition="The members of the team who provided the overall service as well as their role and whether responsible and qualifications." ) 6850 protected List<CareTeamComponent> careTeam; 6851 6852 /** 6853 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required. 6854 */ 6855 @Child(name = "information", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6856 @Description(shortDefinition="Exceptions, special considerations, the condition, situation, prior or concurrent issues", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required." ) 6857 protected List<SpecialConditionComponent> information; 6858 6859 /** 6860 * List of patient diagnosis for which care is sought. 6861 */ 6862 @Child(name = "diagnosis", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6863 @Description(shortDefinition="List of Diagnosis", formalDefinition="List of patient diagnosis for which care is sought." ) 6864 protected List<DiagnosisComponent> diagnosis; 6865 6866 /** 6867 * Ordered list of patient procedures performed to support the adjudication. 6868 */ 6869 @Child(name = "procedure", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6870 @Description(shortDefinition="Procedures performed", formalDefinition="Ordered list of patient procedures performed to support the adjudication." ) 6871 protected List<ProcedureComponent> procedure; 6872 6873 /** 6874 * Financial instrument by which payment information for health care. 6875 */ 6876 @Child(name = "insurance", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6877 @Description(shortDefinition="Insurance or medical plan", formalDefinition="Financial instrument by which payment information for health care." ) 6878 protected List<InsuranceComponent> insurance; 6879 6880 /** 6881 * An accident which resulted in the need for healthcare services. 6882 */ 6883 @Child(name = "accident", type = {}, order=25, min=0, max=1, modifier=false, summary=false) 6884 @Description(shortDefinition="Details about an accident", formalDefinition="An accident which resulted in the need for healthcare services." ) 6885 protected AccidentComponent accident; 6886 6887 /** 6888 * The start and optional end dates of when the patient was precluded from working due to the treatable condition(s). 6889 */ 6890 @Child(name = "employmentImpacted", type = {Period.class}, order=26, min=0, max=1, modifier=false, summary=false) 6891 @Description(shortDefinition="Period unable to work", formalDefinition="The start and optional end dates of when the patient was precluded from working due to the treatable condition(s)." ) 6892 protected Period employmentImpacted; 6893 6894 /** 6895 * The start and optional end dates of when the patient was confined to a treatment center. 6896 */ 6897 @Child(name = "hospitalization", type = {Period.class}, order=27, min=0, max=1, modifier=false, summary=false) 6898 @Description(shortDefinition="Period in hospital", formalDefinition="The start and optional end dates of when the patient was confined to a treatment center." ) 6899 protected Period hospitalization; 6900 6901 /** 6902 * First tier of goods and services. 6903 */ 6904 @Child(name = "item", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6905 @Description(shortDefinition="Goods and Services", formalDefinition="First tier of goods and services." ) 6906 protected List<ItemComponent> item; 6907 6908 /** 6909 * The total value of the claim. 6910 */ 6911 @Child(name = "total", type = {Money.class}, order=29, min=0, max=1, modifier=false, summary=false) 6912 @Description(shortDefinition="Total claim cost", formalDefinition="The total value of the claim." ) 6913 protected Money total; 6914 6915 private static final long serialVersionUID = 1731171342L; 6916 6917 /** 6918 * Constructor 6919 */ 6920 public Claim() { 6921 super(); 6922 } 6923 6924 /** 6925 * @return {@link #identifier} (The business identifier for the instance: claim number, pre-determination or pre-authorization number.) 6926 */ 6927 public List<Identifier> getIdentifier() { 6928 if (this.identifier == null) 6929 this.identifier = new ArrayList<Identifier>(); 6930 return this.identifier; 6931 } 6932 6933 /** 6934 * @return Returns a reference to <code>this</code> for easy method chaining 6935 */ 6936 public Claim setIdentifier(List<Identifier> theIdentifier) { 6937 this.identifier = theIdentifier; 6938 return this; 6939 } 6940 6941 public boolean hasIdentifier() { 6942 if (this.identifier == null) 6943 return false; 6944 for (Identifier item : this.identifier) 6945 if (!item.isEmpty()) 6946 return true; 6947 return false; 6948 } 6949 6950 public Identifier addIdentifier() { //3 6951 Identifier t = new Identifier(); 6952 if (this.identifier == null) 6953 this.identifier = new ArrayList<Identifier>(); 6954 this.identifier.add(t); 6955 return t; 6956 } 6957 6958 public Claim addIdentifier(Identifier t) { //3 6959 if (t == null) 6960 return this; 6961 if (this.identifier == null) 6962 this.identifier = new ArrayList<Identifier>(); 6963 this.identifier.add(t); 6964 return this; 6965 } 6966 6967 /** 6968 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 6969 */ 6970 public Identifier getIdentifierFirstRep() { 6971 if (getIdentifier().isEmpty()) { 6972 addIdentifier(); 6973 } 6974 return getIdentifier().get(0); 6975 } 6976 6977 /** 6978 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 6979 */ 6980 public Enumeration<ClaimStatus> getStatusElement() { 6981 if (this.status == null) 6982 if (Configuration.errorOnAutoCreate()) 6983 throw new Error("Attempt to auto-create Claim.status"); 6984 else if (Configuration.doAutoCreate()) 6985 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); // bb 6986 return this.status; 6987 } 6988 6989 public boolean hasStatusElement() { 6990 return this.status != null && !this.status.isEmpty(); 6991 } 6992 6993 public boolean hasStatus() { 6994 return this.status != null && !this.status.isEmpty(); 6995 } 6996 6997 /** 6998 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 6999 */ 7000 public Claim setStatusElement(Enumeration<ClaimStatus> value) { 7001 this.status = value; 7002 return this; 7003 } 7004 7005 /** 7006 * @return The status of the resource instance. 7007 */ 7008 public ClaimStatus getStatus() { 7009 return this.status == null ? null : this.status.getValue(); 7010 } 7011 7012 /** 7013 * @param value The status of the resource instance. 7014 */ 7015 public Claim setStatus(ClaimStatus value) { 7016 if (value == null) 7017 this.status = null; 7018 else { 7019 if (this.status == null) 7020 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); 7021 this.status.setValue(value); 7022 } 7023 return this; 7024 } 7025 7026 /** 7027 * @return {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 7028 */ 7029 public CodeableConcept getType() { 7030 if (this.type == null) 7031 if (Configuration.errorOnAutoCreate()) 7032 throw new Error("Attempt to auto-create Claim.type"); 7033 else if (Configuration.doAutoCreate()) 7034 this.type = new CodeableConcept(); // cc 7035 return this.type; 7036 } 7037 7038 public boolean hasType() { 7039 return this.type != null && !this.type.isEmpty(); 7040 } 7041 7042 /** 7043 * @param value {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 7044 */ 7045 public Claim setType(CodeableConcept value) { 7046 this.type = value; 7047 return this; 7048 } 7049 7050 /** 7051 * @return {@link #subType} (A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.) 7052 */ 7053 public List<CodeableConcept> getSubType() { 7054 if (this.subType == null) 7055 this.subType = new ArrayList<CodeableConcept>(); 7056 return this.subType; 7057 } 7058 7059 /** 7060 * @return Returns a reference to <code>this</code> for easy method chaining 7061 */ 7062 public Claim setSubType(List<CodeableConcept> theSubType) { 7063 this.subType = theSubType; 7064 return this; 7065 } 7066 7067 public boolean hasSubType() { 7068 if (this.subType == null) 7069 return false; 7070 for (CodeableConcept item : this.subType) 7071 if (!item.isEmpty()) 7072 return true; 7073 return false; 7074 } 7075 7076 public CodeableConcept addSubType() { //3 7077 CodeableConcept t = new CodeableConcept(); 7078 if (this.subType == null) 7079 this.subType = new ArrayList<CodeableConcept>(); 7080 this.subType.add(t); 7081 return t; 7082 } 7083 7084 public Claim addSubType(CodeableConcept t) { //3 7085 if (t == null) 7086 return this; 7087 if (this.subType == null) 7088 this.subType = new ArrayList<CodeableConcept>(); 7089 this.subType.add(t); 7090 return this; 7091 } 7092 7093 /** 7094 * @return The first repetition of repeating field {@link #subType}, creating it if it does not already exist 7095 */ 7096 public CodeableConcept getSubTypeFirstRep() { 7097 if (getSubType().isEmpty()) { 7098 addSubType(); 7099 } 7100 return getSubType().get(0); 7101 } 7102 7103 /** 7104 * @return {@link #use} (Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 7105 */ 7106 public Enumeration<Use> getUseElement() { 7107 if (this.use == null) 7108 if (Configuration.errorOnAutoCreate()) 7109 throw new Error("Attempt to auto-create Claim.use"); 7110 else if (Configuration.doAutoCreate()) 7111 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 7112 return this.use; 7113 } 7114 7115 public boolean hasUseElement() { 7116 return this.use != null && !this.use.isEmpty(); 7117 } 7118 7119 public boolean hasUse() { 7120 return this.use != null && !this.use.isEmpty(); 7121 } 7122 7123 /** 7124 * @param value {@link #use} (Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 7125 */ 7126 public Claim setUseElement(Enumeration<Use> value) { 7127 this.use = value; 7128 return this; 7129 } 7130 7131 /** 7132 * @return Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination). 7133 */ 7134 public Use getUse() { 7135 return this.use == null ? null : this.use.getValue(); 7136 } 7137 7138 /** 7139 * @param value Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination). 7140 */ 7141 public Claim setUse(Use value) { 7142 if (value == null) 7143 this.use = null; 7144 else { 7145 if (this.use == null) 7146 this.use = new Enumeration<Use>(new UseEnumFactory()); 7147 this.use.setValue(value); 7148 } 7149 return this; 7150 } 7151 7152 /** 7153 * @return {@link #patient} (Patient Resource.) 7154 */ 7155 public Reference getPatient() { 7156 if (this.patient == null) 7157 if (Configuration.errorOnAutoCreate()) 7158 throw new Error("Attempt to auto-create Claim.patient"); 7159 else if (Configuration.doAutoCreate()) 7160 this.patient = new Reference(); // cc 7161 return this.patient; 7162 } 7163 7164 public boolean hasPatient() { 7165 return this.patient != null && !this.patient.isEmpty(); 7166 } 7167 7168 /** 7169 * @param value {@link #patient} (Patient Resource.) 7170 */ 7171 public Claim setPatient(Reference value) { 7172 this.patient = value; 7173 return this; 7174 } 7175 7176 /** 7177 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient Resource.) 7178 */ 7179 public Patient getPatientTarget() { 7180 if (this.patientTarget == null) 7181 if (Configuration.errorOnAutoCreate()) 7182 throw new Error("Attempt to auto-create Claim.patient"); 7183 else if (Configuration.doAutoCreate()) 7184 this.patientTarget = new Patient(); // aa 7185 return this.patientTarget; 7186 } 7187 7188 /** 7189 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient Resource.) 7190 */ 7191 public Claim setPatientTarget(Patient value) { 7192 this.patientTarget = value; 7193 return this; 7194 } 7195 7196 /** 7197 * @return {@link #billablePeriod} (The billable period for which charges are being submitted.) 7198 */ 7199 public Period getBillablePeriod() { 7200 if (this.billablePeriod == null) 7201 if (Configuration.errorOnAutoCreate()) 7202 throw new Error("Attempt to auto-create Claim.billablePeriod"); 7203 else if (Configuration.doAutoCreate()) 7204 this.billablePeriod = new Period(); // cc 7205 return this.billablePeriod; 7206 } 7207 7208 public boolean hasBillablePeriod() { 7209 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 7210 } 7211 7212 /** 7213 * @param value {@link #billablePeriod} (The billable period for which charges are being submitted.) 7214 */ 7215 public Claim setBillablePeriod(Period value) { 7216 this.billablePeriod = value; 7217 return this; 7218 } 7219 7220 /** 7221 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 7222 */ 7223 public DateTimeType getCreatedElement() { 7224 if (this.created == null) 7225 if (Configuration.errorOnAutoCreate()) 7226 throw new Error("Attempt to auto-create Claim.created"); 7227 else if (Configuration.doAutoCreate()) 7228 this.created = new DateTimeType(); // bb 7229 return this.created; 7230 } 7231 7232 public boolean hasCreatedElement() { 7233 return this.created != null && !this.created.isEmpty(); 7234 } 7235 7236 public boolean hasCreated() { 7237 return this.created != null && !this.created.isEmpty(); 7238 } 7239 7240 /** 7241 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 7242 */ 7243 public Claim setCreatedElement(DateTimeType value) { 7244 this.created = value; 7245 return this; 7246 } 7247 7248 /** 7249 * @return The date when the enclosed suite of services were performed or completed. 7250 */ 7251 public Date getCreated() { 7252 return this.created == null ? null : this.created.getValue(); 7253 } 7254 7255 /** 7256 * @param value The date when the enclosed suite of services were performed or completed. 7257 */ 7258 public Claim setCreated(Date value) { 7259 if (value == null) 7260 this.created = null; 7261 else { 7262 if (this.created == null) 7263 this.created = new DateTimeType(); 7264 this.created.setValue(value); 7265 } 7266 return this; 7267 } 7268 7269 /** 7270 * @return {@link #enterer} (Person who created the invoice/claim/pre-determination or pre-authorization.) 7271 */ 7272 public Reference getEnterer() { 7273 if (this.enterer == null) 7274 if (Configuration.errorOnAutoCreate()) 7275 throw new Error("Attempt to auto-create Claim.enterer"); 7276 else if (Configuration.doAutoCreate()) 7277 this.enterer = new Reference(); // cc 7278 return this.enterer; 7279 } 7280 7281 public boolean hasEnterer() { 7282 return this.enterer != null && !this.enterer.isEmpty(); 7283 } 7284 7285 /** 7286 * @param value {@link #enterer} (Person who created the invoice/claim/pre-determination or pre-authorization.) 7287 */ 7288 public Claim setEnterer(Reference value) { 7289 this.enterer = value; 7290 return this; 7291 } 7292 7293 /** 7294 * @return {@link #enterer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Person who created the invoice/claim/pre-determination or pre-authorization.) 7295 */ 7296 public Practitioner getEntererTarget() { 7297 if (this.entererTarget == null) 7298 if (Configuration.errorOnAutoCreate()) 7299 throw new Error("Attempt to auto-create Claim.enterer"); 7300 else if (Configuration.doAutoCreate()) 7301 this.entererTarget = new Practitioner(); // aa 7302 return this.entererTarget; 7303 } 7304 7305 /** 7306 * @param value {@link #enterer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Person who created the invoice/claim/pre-determination or pre-authorization.) 7307 */ 7308 public Claim setEntererTarget(Practitioner value) { 7309 this.entererTarget = value; 7310 return this; 7311 } 7312 7313 /** 7314 * @return {@link #insurer} (The Insurer who is target of the request.) 7315 */ 7316 public Reference getInsurer() { 7317 if (this.insurer == null) 7318 if (Configuration.errorOnAutoCreate()) 7319 throw new Error("Attempt to auto-create Claim.insurer"); 7320 else if (Configuration.doAutoCreate()) 7321 this.insurer = new Reference(); // cc 7322 return this.insurer; 7323 } 7324 7325 public boolean hasInsurer() { 7326 return this.insurer != null && !this.insurer.isEmpty(); 7327 } 7328 7329 /** 7330 * @param value {@link #insurer} (The Insurer who is target of the request.) 7331 */ 7332 public Claim setInsurer(Reference value) { 7333 this.insurer = value; 7334 return this; 7335 } 7336 7337 /** 7338 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 7339 */ 7340 public Organization getInsurerTarget() { 7341 if (this.insurerTarget == null) 7342 if (Configuration.errorOnAutoCreate()) 7343 throw new Error("Attempt to auto-create Claim.insurer"); 7344 else if (Configuration.doAutoCreate()) 7345 this.insurerTarget = new Organization(); // aa 7346 return this.insurerTarget; 7347 } 7348 7349 /** 7350 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 7351 */ 7352 public Claim setInsurerTarget(Organization value) { 7353 this.insurerTarget = value; 7354 return this; 7355 } 7356 7357 /** 7358 * @return {@link #provider} (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7359 */ 7360 public Reference getProvider() { 7361 if (this.provider == null) 7362 if (Configuration.errorOnAutoCreate()) 7363 throw new Error("Attempt to auto-create Claim.provider"); 7364 else if (Configuration.doAutoCreate()) 7365 this.provider = new Reference(); // cc 7366 return this.provider; 7367 } 7368 7369 public boolean hasProvider() { 7370 return this.provider != null && !this.provider.isEmpty(); 7371 } 7372 7373 /** 7374 * @param value {@link #provider} (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7375 */ 7376 public Claim setProvider(Reference value) { 7377 this.provider = value; 7378 return this; 7379 } 7380 7381 /** 7382 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7383 */ 7384 public Practitioner getProviderTarget() { 7385 if (this.providerTarget == null) 7386 if (Configuration.errorOnAutoCreate()) 7387 throw new Error("Attempt to auto-create Claim.provider"); 7388 else if (Configuration.doAutoCreate()) 7389 this.providerTarget = new Practitioner(); // aa 7390 return this.providerTarget; 7391 } 7392 7393 /** 7394 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the bill, claim pre-determination, pre-authorization.) 7395 */ 7396 public Claim setProviderTarget(Practitioner value) { 7397 this.providerTarget = value; 7398 return this; 7399 } 7400 7401 /** 7402 * @return {@link #organization} (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7403 */ 7404 public Reference getOrganization() { 7405 if (this.organization == null) 7406 if (Configuration.errorOnAutoCreate()) 7407 throw new Error("Attempt to auto-create Claim.organization"); 7408 else if (Configuration.doAutoCreate()) 7409 this.organization = new Reference(); // cc 7410 return this.organization; 7411 } 7412 7413 public boolean hasOrganization() { 7414 return this.organization != null && !this.organization.isEmpty(); 7415 } 7416 7417 /** 7418 * @param value {@link #organization} (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7419 */ 7420 public Claim setOrganization(Reference value) { 7421 this.organization = value; 7422 return this; 7423 } 7424 7425 /** 7426 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7427 */ 7428 public Organization getOrganizationTarget() { 7429 if (this.organizationTarget == null) 7430 if (Configuration.errorOnAutoCreate()) 7431 throw new Error("Attempt to auto-create Claim.organization"); 7432 else if (Configuration.doAutoCreate()) 7433 this.organizationTarget = new Organization(); // aa 7434 return this.organizationTarget; 7435 } 7436 7437 /** 7438 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the bill, claim pre-determination, pre-authorization.) 7439 */ 7440 public Claim setOrganizationTarget(Organization value) { 7441 this.organizationTarget = value; 7442 return this; 7443 } 7444 7445 /** 7446 * @return {@link #priority} (Immediate (STAT), best effort (NORMAL), deferred (DEFER).) 7447 */ 7448 public CodeableConcept getPriority() { 7449 if (this.priority == null) 7450 if (Configuration.errorOnAutoCreate()) 7451 throw new Error("Attempt to auto-create Claim.priority"); 7452 else if (Configuration.doAutoCreate()) 7453 this.priority = new CodeableConcept(); // cc 7454 return this.priority; 7455 } 7456 7457 public boolean hasPriority() { 7458 return this.priority != null && !this.priority.isEmpty(); 7459 } 7460 7461 /** 7462 * @param value {@link #priority} (Immediate (STAT), best effort (NORMAL), deferred (DEFER).) 7463 */ 7464 public Claim setPriority(CodeableConcept value) { 7465 this.priority = value; 7466 return this; 7467 } 7468 7469 /** 7470 * @return {@link #fundsReserve} (In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.) 7471 */ 7472 public CodeableConcept getFundsReserve() { 7473 if (this.fundsReserve == null) 7474 if (Configuration.errorOnAutoCreate()) 7475 throw new Error("Attempt to auto-create Claim.fundsReserve"); 7476 else if (Configuration.doAutoCreate()) 7477 this.fundsReserve = new CodeableConcept(); // cc 7478 return this.fundsReserve; 7479 } 7480 7481 public boolean hasFundsReserve() { 7482 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 7483 } 7484 7485 /** 7486 * @param value {@link #fundsReserve} (In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.) 7487 */ 7488 public Claim setFundsReserve(CodeableConcept value) { 7489 this.fundsReserve = value; 7490 return this; 7491 } 7492 7493 /** 7494 * @return {@link #related} (Other claims which are related to this claim such as prior claim versions or for related services.) 7495 */ 7496 public List<RelatedClaimComponent> getRelated() { 7497 if (this.related == null) 7498 this.related = new ArrayList<RelatedClaimComponent>(); 7499 return this.related; 7500 } 7501 7502 /** 7503 * @return Returns a reference to <code>this</code> for easy method chaining 7504 */ 7505 public Claim setRelated(List<RelatedClaimComponent> theRelated) { 7506 this.related = theRelated; 7507 return this; 7508 } 7509 7510 public boolean hasRelated() { 7511 if (this.related == null) 7512 return false; 7513 for (RelatedClaimComponent item : this.related) 7514 if (!item.isEmpty()) 7515 return true; 7516 return false; 7517 } 7518 7519 public RelatedClaimComponent addRelated() { //3 7520 RelatedClaimComponent t = new RelatedClaimComponent(); 7521 if (this.related == null) 7522 this.related = new ArrayList<RelatedClaimComponent>(); 7523 this.related.add(t); 7524 return t; 7525 } 7526 7527 public Claim addRelated(RelatedClaimComponent t) { //3 7528 if (t == null) 7529 return this; 7530 if (this.related == null) 7531 this.related = new ArrayList<RelatedClaimComponent>(); 7532 this.related.add(t); 7533 return this; 7534 } 7535 7536 /** 7537 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist 7538 */ 7539 public RelatedClaimComponent getRelatedFirstRep() { 7540 if (getRelated().isEmpty()) { 7541 addRelated(); 7542 } 7543 return getRelated().get(0); 7544 } 7545 7546 /** 7547 * @return {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 7548 */ 7549 public Reference getPrescription() { 7550 if (this.prescription == null) 7551 if (Configuration.errorOnAutoCreate()) 7552 throw new Error("Attempt to auto-create Claim.prescription"); 7553 else if (Configuration.doAutoCreate()) 7554 this.prescription = new Reference(); // cc 7555 return this.prescription; 7556 } 7557 7558 public boolean hasPrescription() { 7559 return this.prescription != null && !this.prescription.isEmpty(); 7560 } 7561 7562 /** 7563 * @param value {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 7564 */ 7565 public Claim setPrescription(Reference value) { 7566 this.prescription = value; 7567 return this; 7568 } 7569 7570 /** 7571 * @return {@link #prescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 7572 */ 7573 public Resource getPrescriptionTarget() { 7574 return this.prescriptionTarget; 7575 } 7576 7577 /** 7578 * @param value {@link #prescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 7579 */ 7580 public Claim setPrescriptionTarget(Resource value) { 7581 this.prescriptionTarget = value; 7582 return this; 7583 } 7584 7585 /** 7586 * @return {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7587 */ 7588 public Reference getOriginalPrescription() { 7589 if (this.originalPrescription == null) 7590 if (Configuration.errorOnAutoCreate()) 7591 throw new Error("Attempt to auto-create Claim.originalPrescription"); 7592 else if (Configuration.doAutoCreate()) 7593 this.originalPrescription = new Reference(); // cc 7594 return this.originalPrescription; 7595 } 7596 7597 public boolean hasOriginalPrescription() { 7598 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 7599 } 7600 7601 /** 7602 * @param value {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7603 */ 7604 public Claim setOriginalPrescription(Reference value) { 7605 this.originalPrescription = value; 7606 return this; 7607 } 7608 7609 /** 7610 * @return {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7611 */ 7612 public MedicationRequest getOriginalPrescriptionTarget() { 7613 if (this.originalPrescriptionTarget == null) 7614 if (Configuration.errorOnAutoCreate()) 7615 throw new Error("Attempt to auto-create Claim.originalPrescription"); 7616 else if (Configuration.doAutoCreate()) 7617 this.originalPrescriptionTarget = new MedicationRequest(); // aa 7618 return this.originalPrescriptionTarget; 7619 } 7620 7621 /** 7622 * @param value {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 7623 */ 7624 public Claim setOriginalPrescriptionTarget(MedicationRequest value) { 7625 this.originalPrescriptionTarget = value; 7626 return this; 7627 } 7628 7629 /** 7630 * @return {@link #payee} (The party to be reimbursed for the services.) 7631 */ 7632 public PayeeComponent getPayee() { 7633 if (this.payee == null) 7634 if (Configuration.errorOnAutoCreate()) 7635 throw new Error("Attempt to auto-create Claim.payee"); 7636 else if (Configuration.doAutoCreate()) 7637 this.payee = new PayeeComponent(); // cc 7638 return this.payee; 7639 } 7640 7641 public boolean hasPayee() { 7642 return this.payee != null && !this.payee.isEmpty(); 7643 } 7644 7645 /** 7646 * @param value {@link #payee} (The party to be reimbursed for the services.) 7647 */ 7648 public Claim setPayee(PayeeComponent value) { 7649 this.payee = value; 7650 return this; 7651 } 7652 7653 /** 7654 * @return {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 7655 */ 7656 public Reference getReferral() { 7657 if (this.referral == null) 7658 if (Configuration.errorOnAutoCreate()) 7659 throw new Error("Attempt to auto-create Claim.referral"); 7660 else if (Configuration.doAutoCreate()) 7661 this.referral = new Reference(); // cc 7662 return this.referral; 7663 } 7664 7665 public boolean hasReferral() { 7666 return this.referral != null && !this.referral.isEmpty(); 7667 } 7668 7669 /** 7670 * @param value {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 7671 */ 7672 public Claim setReferral(Reference value) { 7673 this.referral = value; 7674 return this; 7675 } 7676 7677 /** 7678 * @return {@link #referral} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 7679 */ 7680 public ReferralRequest getReferralTarget() { 7681 if (this.referralTarget == null) 7682 if (Configuration.errorOnAutoCreate()) 7683 throw new Error("Attempt to auto-create Claim.referral"); 7684 else if (Configuration.doAutoCreate()) 7685 this.referralTarget = new ReferralRequest(); // aa 7686 return this.referralTarget; 7687 } 7688 7689 /** 7690 * @param value {@link #referral} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 7691 */ 7692 public Claim setReferralTarget(ReferralRequest value) { 7693 this.referralTarget = value; 7694 return this; 7695 } 7696 7697 /** 7698 * @return {@link #facility} (Facility where the services were provided.) 7699 */ 7700 public Reference getFacility() { 7701 if (this.facility == null) 7702 if (Configuration.errorOnAutoCreate()) 7703 throw new Error("Attempt to auto-create Claim.facility"); 7704 else if (Configuration.doAutoCreate()) 7705 this.facility = new Reference(); // cc 7706 return this.facility; 7707 } 7708 7709 public boolean hasFacility() { 7710 return this.facility != null && !this.facility.isEmpty(); 7711 } 7712 7713 /** 7714 * @param value {@link #facility} (Facility where the services were provided.) 7715 */ 7716 public Claim setFacility(Reference value) { 7717 this.facility = value; 7718 return this; 7719 } 7720 7721 /** 7722 * @return {@link #facility} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 7723 */ 7724 public Location getFacilityTarget() { 7725 if (this.facilityTarget == null) 7726 if (Configuration.errorOnAutoCreate()) 7727 throw new Error("Attempt to auto-create Claim.facility"); 7728 else if (Configuration.doAutoCreate()) 7729 this.facilityTarget = new Location(); // aa 7730 return this.facilityTarget; 7731 } 7732 7733 /** 7734 * @param value {@link #facility} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 7735 */ 7736 public Claim setFacilityTarget(Location value) { 7737 this.facilityTarget = value; 7738 return this; 7739 } 7740 7741 /** 7742 * @return {@link #careTeam} (The members of the team who provided the overall service as well as their role and whether responsible and qualifications.) 7743 */ 7744 public List<CareTeamComponent> getCareTeam() { 7745 if (this.careTeam == null) 7746 this.careTeam = new ArrayList<CareTeamComponent>(); 7747 return this.careTeam; 7748 } 7749 7750 /** 7751 * @return Returns a reference to <code>this</code> for easy method chaining 7752 */ 7753 public Claim setCareTeam(List<CareTeamComponent> theCareTeam) { 7754 this.careTeam = theCareTeam; 7755 return this; 7756 } 7757 7758 public boolean hasCareTeam() { 7759 if (this.careTeam == null) 7760 return false; 7761 for (CareTeamComponent item : this.careTeam) 7762 if (!item.isEmpty()) 7763 return true; 7764 return false; 7765 } 7766 7767 public CareTeamComponent addCareTeam() { //3 7768 CareTeamComponent t = new CareTeamComponent(); 7769 if (this.careTeam == null) 7770 this.careTeam = new ArrayList<CareTeamComponent>(); 7771 this.careTeam.add(t); 7772 return t; 7773 } 7774 7775 public Claim addCareTeam(CareTeamComponent t) { //3 7776 if (t == null) 7777 return this; 7778 if (this.careTeam == null) 7779 this.careTeam = new ArrayList<CareTeamComponent>(); 7780 this.careTeam.add(t); 7781 return this; 7782 } 7783 7784 /** 7785 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist 7786 */ 7787 public CareTeamComponent getCareTeamFirstRep() { 7788 if (getCareTeam().isEmpty()) { 7789 addCareTeam(); 7790 } 7791 return getCareTeam().get(0); 7792 } 7793 7794 /** 7795 * @return {@link #information} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.) 7796 */ 7797 public List<SpecialConditionComponent> getInformation() { 7798 if (this.information == null) 7799 this.information = new ArrayList<SpecialConditionComponent>(); 7800 return this.information; 7801 } 7802 7803 /** 7804 * @return Returns a reference to <code>this</code> for easy method chaining 7805 */ 7806 public Claim setInformation(List<SpecialConditionComponent> theInformation) { 7807 this.information = theInformation; 7808 return this; 7809 } 7810 7811 public boolean hasInformation() { 7812 if (this.information == null) 7813 return false; 7814 for (SpecialConditionComponent item : this.information) 7815 if (!item.isEmpty()) 7816 return true; 7817 return false; 7818 } 7819 7820 public SpecialConditionComponent addInformation() { //3 7821 SpecialConditionComponent t = new SpecialConditionComponent(); 7822 if (this.information == null) 7823 this.information = new ArrayList<SpecialConditionComponent>(); 7824 this.information.add(t); 7825 return t; 7826 } 7827 7828 public Claim addInformation(SpecialConditionComponent t) { //3 7829 if (t == null) 7830 return this; 7831 if (this.information == null) 7832 this.information = new ArrayList<SpecialConditionComponent>(); 7833 this.information.add(t); 7834 return this; 7835 } 7836 7837 /** 7838 * @return The first repetition of repeating field {@link #information}, creating it if it does not already exist 7839 */ 7840 public SpecialConditionComponent getInformationFirstRep() { 7841 if (getInformation().isEmpty()) { 7842 addInformation(); 7843 } 7844 return getInformation().get(0); 7845 } 7846 7847 /** 7848 * @return {@link #diagnosis} (List of patient diagnosis for which care is sought.) 7849 */ 7850 public List<DiagnosisComponent> getDiagnosis() { 7851 if (this.diagnosis == null) 7852 this.diagnosis = new ArrayList<DiagnosisComponent>(); 7853 return this.diagnosis; 7854 } 7855 7856 /** 7857 * @return Returns a reference to <code>this</code> for easy method chaining 7858 */ 7859 public Claim setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 7860 this.diagnosis = theDiagnosis; 7861 return this; 7862 } 7863 7864 public boolean hasDiagnosis() { 7865 if (this.diagnosis == null) 7866 return false; 7867 for (DiagnosisComponent item : this.diagnosis) 7868 if (!item.isEmpty()) 7869 return true; 7870 return false; 7871 } 7872 7873 public DiagnosisComponent addDiagnosis() { //3 7874 DiagnosisComponent t = new DiagnosisComponent(); 7875 if (this.diagnosis == null) 7876 this.diagnosis = new ArrayList<DiagnosisComponent>(); 7877 this.diagnosis.add(t); 7878 return t; 7879 } 7880 7881 public Claim addDiagnosis(DiagnosisComponent t) { //3 7882 if (t == null) 7883 return this; 7884 if (this.diagnosis == null) 7885 this.diagnosis = new ArrayList<DiagnosisComponent>(); 7886 this.diagnosis.add(t); 7887 return this; 7888 } 7889 7890 /** 7891 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist 7892 */ 7893 public DiagnosisComponent getDiagnosisFirstRep() { 7894 if (getDiagnosis().isEmpty()) { 7895 addDiagnosis(); 7896 } 7897 return getDiagnosis().get(0); 7898 } 7899 7900 /** 7901 * @return {@link #procedure} (Ordered list of patient procedures performed to support the adjudication.) 7902 */ 7903 public List<ProcedureComponent> getProcedure() { 7904 if (this.procedure == null) 7905 this.procedure = new ArrayList<ProcedureComponent>(); 7906 return this.procedure; 7907 } 7908 7909 /** 7910 * @return Returns a reference to <code>this</code> for easy method chaining 7911 */ 7912 public Claim setProcedure(List<ProcedureComponent> theProcedure) { 7913 this.procedure = theProcedure; 7914 return this; 7915 } 7916 7917 public boolean hasProcedure() { 7918 if (this.procedure == null) 7919 return false; 7920 for (ProcedureComponent item : this.procedure) 7921 if (!item.isEmpty()) 7922 return true; 7923 return false; 7924 } 7925 7926 public ProcedureComponent addProcedure() { //3 7927 ProcedureComponent t = new ProcedureComponent(); 7928 if (this.procedure == null) 7929 this.procedure = new ArrayList<ProcedureComponent>(); 7930 this.procedure.add(t); 7931 return t; 7932 } 7933 7934 public Claim addProcedure(ProcedureComponent t) { //3 7935 if (t == null) 7936 return this; 7937 if (this.procedure == null) 7938 this.procedure = new ArrayList<ProcedureComponent>(); 7939 this.procedure.add(t); 7940 return this; 7941 } 7942 7943 /** 7944 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist 7945 */ 7946 public ProcedureComponent getProcedureFirstRep() { 7947 if (getProcedure().isEmpty()) { 7948 addProcedure(); 7949 } 7950 return getProcedure().get(0); 7951 } 7952 7953 /** 7954 * @return {@link #insurance} (Financial instrument by which payment information for health care.) 7955 */ 7956 public List<InsuranceComponent> getInsurance() { 7957 if (this.insurance == null) 7958 this.insurance = new ArrayList<InsuranceComponent>(); 7959 return this.insurance; 7960 } 7961 7962 /** 7963 * @return Returns a reference to <code>this</code> for easy method chaining 7964 */ 7965 public Claim setInsurance(List<InsuranceComponent> theInsurance) { 7966 this.insurance = theInsurance; 7967 return this; 7968 } 7969 7970 public boolean hasInsurance() { 7971 if (this.insurance == null) 7972 return false; 7973 for (InsuranceComponent item : this.insurance) 7974 if (!item.isEmpty()) 7975 return true; 7976 return false; 7977 } 7978 7979 public InsuranceComponent addInsurance() { //3 7980 InsuranceComponent t = new InsuranceComponent(); 7981 if (this.insurance == null) 7982 this.insurance = new ArrayList<InsuranceComponent>(); 7983 this.insurance.add(t); 7984 return t; 7985 } 7986 7987 public Claim addInsurance(InsuranceComponent t) { //3 7988 if (t == null) 7989 return this; 7990 if (this.insurance == null) 7991 this.insurance = new ArrayList<InsuranceComponent>(); 7992 this.insurance.add(t); 7993 return this; 7994 } 7995 7996 /** 7997 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist 7998 */ 7999 public InsuranceComponent getInsuranceFirstRep() { 8000 if (getInsurance().isEmpty()) { 8001 addInsurance(); 8002 } 8003 return getInsurance().get(0); 8004 } 8005 8006 /** 8007 * @return {@link #accident} (An accident which resulted in the need for healthcare services.) 8008 */ 8009 public AccidentComponent getAccident() { 8010 if (this.accident == null) 8011 if (Configuration.errorOnAutoCreate()) 8012 throw new Error("Attempt to auto-create Claim.accident"); 8013 else if (Configuration.doAutoCreate()) 8014 this.accident = new AccidentComponent(); // cc 8015 return this.accident; 8016 } 8017 8018 public boolean hasAccident() { 8019 return this.accident != null && !this.accident.isEmpty(); 8020 } 8021 8022 /** 8023 * @param value {@link #accident} (An accident which resulted in the need for healthcare services.) 8024 */ 8025 public Claim setAccident(AccidentComponent value) { 8026 this.accident = value; 8027 return this; 8028 } 8029 8030 /** 8031 * @return {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 8032 */ 8033 public Period getEmploymentImpacted() { 8034 if (this.employmentImpacted == null) 8035 if (Configuration.errorOnAutoCreate()) 8036 throw new Error("Attempt to auto-create Claim.employmentImpacted"); 8037 else if (Configuration.doAutoCreate()) 8038 this.employmentImpacted = new Period(); // cc 8039 return this.employmentImpacted; 8040 } 8041 8042 public boolean hasEmploymentImpacted() { 8043 return this.employmentImpacted != null && !this.employmentImpacted.isEmpty(); 8044 } 8045 8046 /** 8047 * @param value {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 8048 */ 8049 public Claim setEmploymentImpacted(Period value) { 8050 this.employmentImpacted = value; 8051 return this; 8052 } 8053 8054 /** 8055 * @return {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 8056 */ 8057 public Period getHospitalization() { 8058 if (this.hospitalization == null) 8059 if (Configuration.errorOnAutoCreate()) 8060 throw new Error("Attempt to auto-create Claim.hospitalization"); 8061 else if (Configuration.doAutoCreate()) 8062 this.hospitalization = new Period(); // cc 8063 return this.hospitalization; 8064 } 8065 8066 public boolean hasHospitalization() { 8067 return this.hospitalization != null && !this.hospitalization.isEmpty(); 8068 } 8069 8070 /** 8071 * @param value {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 8072 */ 8073 public Claim setHospitalization(Period value) { 8074 this.hospitalization = value; 8075 return this; 8076 } 8077 8078 /** 8079 * @return {@link #item} (First tier of goods and services.) 8080 */ 8081 public List<ItemComponent> getItem() { 8082 if (this.item == null) 8083 this.item = new ArrayList<ItemComponent>(); 8084 return this.item; 8085 } 8086 8087 /** 8088 * @return Returns a reference to <code>this</code> for easy method chaining 8089 */ 8090 public Claim setItem(List<ItemComponent> theItem) { 8091 this.item = theItem; 8092 return this; 8093 } 8094 8095 public boolean hasItem() { 8096 if (this.item == null) 8097 return false; 8098 for (ItemComponent item : this.item) 8099 if (!item.isEmpty()) 8100 return true; 8101 return false; 8102 } 8103 8104 public ItemComponent addItem() { //3 8105 ItemComponent t = new ItemComponent(); 8106 if (this.item == null) 8107 this.item = new ArrayList<ItemComponent>(); 8108 this.item.add(t); 8109 return t; 8110 } 8111 8112 public Claim addItem(ItemComponent t) { //3 8113 if (t == null) 8114 return this; 8115 if (this.item == null) 8116 this.item = new ArrayList<ItemComponent>(); 8117 this.item.add(t); 8118 return this; 8119 } 8120 8121 /** 8122 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 8123 */ 8124 public ItemComponent getItemFirstRep() { 8125 if (getItem().isEmpty()) { 8126 addItem(); 8127 } 8128 return getItem().get(0); 8129 } 8130 8131 /** 8132 * @return {@link #total} (The total value of the claim.) 8133 */ 8134 public Money getTotal() { 8135 if (this.total == null) 8136 if (Configuration.errorOnAutoCreate()) 8137 throw new Error("Attempt to auto-create Claim.total"); 8138 else if (Configuration.doAutoCreate()) 8139 this.total = new Money(); // cc 8140 return this.total; 8141 } 8142 8143 public boolean hasTotal() { 8144 return this.total != null && !this.total.isEmpty(); 8145 } 8146 8147 /** 8148 * @param value {@link #total} (The total value of the claim.) 8149 */ 8150 public Claim setTotal(Money value) { 8151 this.total = value; 8152 return this; 8153 } 8154 8155 protected void listChildren(List<Property> children) { 8156 super.listChildren(children); 8157 children.add(new Property("identifier", "Identifier", "The business identifier for the instance: claim number, pre-determination or pre-authorization number.", 0, java.lang.Integer.MAX_VALUE, identifier)); 8158 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 8159 children.add(new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type)); 8160 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType)); 8161 children.add(new Property("use", "code", "Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).", 0, 1, use)); 8162 children.add(new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient)); 8163 children.add(new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod)); 8164 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 8165 children.add(new Property("enterer", "Reference(Practitioner)", "Person who created the invoice/claim/pre-determination or pre-authorization.", 0, 1, enterer)); 8166 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 8167 children.add(new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, provider)); 8168 children.add(new Property("organization", "Reference(Organization)", "The organization which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, organization)); 8169 children.add(new Property("priority", "CodeableConcept", "Immediate (STAT), best effort (NORMAL), deferred (DEFER).", 0, 1, priority)); 8170 children.add(new Property("fundsReserve", "CodeableConcept", "In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.", 0, 1, fundsReserve)); 8171 children.add(new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related)); 8172 children.add(new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription)); 8173 children.add(new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription)); 8174 children.add(new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee)); 8175 children.add(new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral)); 8176 children.add(new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 8177 children.add(new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 8178 children.add(new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information)); 8179 children.add(new Property("diagnosis", "", "List of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 8180 children.add(new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure)); 8181 children.add(new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, java.lang.Integer.MAX_VALUE, insurance)); 8182 children.add(new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident)); 8183 children.add(new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted)); 8184 children.add(new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization)); 8185 children.add(new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item)); 8186 children.add(new Property("total", "Money", "The total value of the claim.", 0, 1, total)); 8187 } 8188 8189 @Override 8190 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8191 switch (_hash) { 8192 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The business identifier for the instance: claim number, pre-determination or pre-authorization number.", 0, java.lang.Integer.MAX_VALUE, identifier); 8193 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 8194 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type); 8195 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType); 8196 case 116103: /*use*/ return new Property("use", "code", "Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).", 0, 1, use); 8197 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient); 8198 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod); 8199 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 8200 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner)", "Person who created the invoice/claim/pre-determination or pre-authorization.", 0, 1, enterer); 8201 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer); 8202 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, provider); 8203 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 1, organization); 8204 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Immediate (STAT), best effort (NORMAL), deferred (DEFER).", 0, 1, priority); 8205 case 1314609806: /*fundsReserve*/ return new Property("fundsReserve", "CodeableConcept", "In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.", 0, 1, fundsReserve); 8206 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related); 8207 case 460301338: /*prescription*/ return new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription); 8208 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription); 8209 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee); 8210 case -722568291: /*referral*/ return new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral); 8211 case 501116579: /*facility*/ return new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility); 8212 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam); 8213 case 1968600364: /*information*/ return new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information); 8214 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "List of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 8215 case -1095204141: /*procedure*/ return new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure); 8216 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, java.lang.Integer.MAX_VALUE, insurance); 8217 case -2143202801: /*accident*/ return new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident); 8218 case 1051487345: /*employmentImpacted*/ return new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted); 8219 case 1057894634: /*hospitalization*/ return new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization); 8220 case 3242771: /*item*/ return new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item); 8221 case 110549828: /*total*/ return new Property("total", "Money", "The total value of the claim.", 0, 1, total); 8222 default: return super.getNamedProperty(_hash, _name, _checkValid); 8223 } 8224 8225 } 8226 8227 @Override 8228 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8229 switch (hash) { 8230 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8231 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ClaimStatus> 8232 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 8233 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 8234 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<Use> 8235 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 8236 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 8237 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 8238 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 8239 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 8240 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 8241 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 8242 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 8243 case 1314609806: /*fundsReserve*/ return this.fundsReserve == null ? new Base[0] : new Base[] {this.fundsReserve}; // CodeableConcept 8244 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 8245 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 8246 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 8247 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 8248 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 8249 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 8250 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 8251 case 1968600364: /*information*/ return this.information == null ? new Base[0] : this.information.toArray(new Base[this.information.size()]); // SpecialConditionComponent 8252 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 8253 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 8254 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 8255 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 8256 case 1051487345: /*employmentImpacted*/ return this.employmentImpacted == null ? new Base[0] : new Base[] {this.employmentImpacted}; // Period 8257 case 1057894634: /*hospitalization*/ return this.hospitalization == null ? new Base[0] : new Base[] {this.hospitalization}; // Period 8258 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 8259 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // Money 8260 default: return super.getProperty(hash, name, checkValid); 8261 } 8262 8263 } 8264 8265 @Override 8266 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8267 switch (hash) { 8268 case -1618432855: // identifier 8269 this.getIdentifier().add(castToIdentifier(value)); // Identifier 8270 return value; 8271 case -892481550: // status 8272 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 8273 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 8274 return value; 8275 case 3575610: // type 8276 this.type = castToCodeableConcept(value); // CodeableConcept 8277 return value; 8278 case -1868521062: // subType 8279 this.getSubType().add(castToCodeableConcept(value)); // CodeableConcept 8280 return value; 8281 case 116103: // use 8282 value = new UseEnumFactory().fromType(castToCode(value)); 8283 this.use = (Enumeration) value; // Enumeration<Use> 8284 return value; 8285 case -791418107: // patient 8286 this.patient = castToReference(value); // Reference 8287 return value; 8288 case -332066046: // billablePeriod 8289 this.billablePeriod = castToPeriod(value); // Period 8290 return value; 8291 case 1028554472: // created 8292 this.created = castToDateTime(value); // DateTimeType 8293 return value; 8294 case -1591951995: // enterer 8295 this.enterer = castToReference(value); // Reference 8296 return value; 8297 case 1957615864: // insurer 8298 this.insurer = castToReference(value); // Reference 8299 return value; 8300 case -987494927: // provider 8301 this.provider = castToReference(value); // Reference 8302 return value; 8303 case 1178922291: // organization 8304 this.organization = castToReference(value); // Reference 8305 return value; 8306 case -1165461084: // priority 8307 this.priority = castToCodeableConcept(value); // CodeableConcept 8308 return value; 8309 case 1314609806: // fundsReserve 8310 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 8311 return value; 8312 case 1090493483: // related 8313 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 8314 return value; 8315 case 460301338: // prescription 8316 this.prescription = castToReference(value); // Reference 8317 return value; 8318 case -1814015861: // originalPrescription 8319 this.originalPrescription = castToReference(value); // Reference 8320 return value; 8321 case 106443592: // payee 8322 this.payee = (PayeeComponent) value; // PayeeComponent 8323 return value; 8324 case -722568291: // referral 8325 this.referral = castToReference(value); // Reference 8326 return value; 8327 case 501116579: // facility 8328 this.facility = castToReference(value); // Reference 8329 return value; 8330 case -7323378: // careTeam 8331 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 8332 return value; 8333 case 1968600364: // information 8334 this.getInformation().add((SpecialConditionComponent) value); // SpecialConditionComponent 8335 return value; 8336 case 1196993265: // diagnosis 8337 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 8338 return value; 8339 case -1095204141: // procedure 8340 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 8341 return value; 8342 case 73049818: // insurance 8343 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 8344 return value; 8345 case -2143202801: // accident 8346 this.accident = (AccidentComponent) value; // AccidentComponent 8347 return value; 8348 case 1051487345: // employmentImpacted 8349 this.employmentImpacted = castToPeriod(value); // Period 8350 return value; 8351 case 1057894634: // hospitalization 8352 this.hospitalization = castToPeriod(value); // Period 8353 return value; 8354 case 3242771: // item 8355 this.getItem().add((ItemComponent) value); // ItemComponent 8356 return value; 8357 case 110549828: // total 8358 this.total = castToMoney(value); // Money 8359 return value; 8360 default: return super.setProperty(hash, name, value); 8361 } 8362 8363 } 8364 8365 @Override 8366 public Base setProperty(String name, Base value) throws FHIRException { 8367 if (name.equals("identifier")) { 8368 this.getIdentifier().add(castToIdentifier(value)); 8369 } else if (name.equals("status")) { 8370 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 8371 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 8372 } else if (name.equals("type")) { 8373 this.type = castToCodeableConcept(value); // CodeableConcept 8374 } else if (name.equals("subType")) { 8375 this.getSubType().add(castToCodeableConcept(value)); 8376 } else if (name.equals("use")) { 8377 value = new UseEnumFactory().fromType(castToCode(value)); 8378 this.use = (Enumeration) value; // Enumeration<Use> 8379 } else if (name.equals("patient")) { 8380 this.patient = castToReference(value); // Reference 8381 } else if (name.equals("billablePeriod")) { 8382 this.billablePeriod = castToPeriod(value); // Period 8383 } else if (name.equals("created")) { 8384 this.created = castToDateTime(value); // DateTimeType 8385 } else if (name.equals("enterer")) { 8386 this.enterer = castToReference(value); // Reference 8387 } else if (name.equals("insurer")) { 8388 this.insurer = castToReference(value); // Reference 8389 } else if (name.equals("provider")) { 8390 this.provider = castToReference(value); // Reference 8391 } else if (name.equals("organization")) { 8392 this.organization = castToReference(value); // Reference 8393 } else if (name.equals("priority")) { 8394 this.priority = castToCodeableConcept(value); // CodeableConcept 8395 } else if (name.equals("fundsReserve")) { 8396 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 8397 } else if (name.equals("related")) { 8398 this.getRelated().add((RelatedClaimComponent) value); 8399 } else if (name.equals("prescription")) { 8400 this.prescription = castToReference(value); // Reference 8401 } else if (name.equals("originalPrescription")) { 8402 this.originalPrescription = castToReference(value); // Reference 8403 } else if (name.equals("payee")) { 8404 this.payee = (PayeeComponent) value; // PayeeComponent 8405 } else if (name.equals("referral")) { 8406 this.referral = castToReference(value); // Reference 8407 } else if (name.equals("facility")) { 8408 this.facility = castToReference(value); // Reference 8409 } else if (name.equals("careTeam")) { 8410 this.getCareTeam().add((CareTeamComponent) value); 8411 } else if (name.equals("information")) { 8412 this.getInformation().add((SpecialConditionComponent) value); 8413 } else if (name.equals("diagnosis")) { 8414 this.getDiagnosis().add((DiagnosisComponent) value); 8415 } else if (name.equals("procedure")) { 8416 this.getProcedure().add((ProcedureComponent) value); 8417 } else if (name.equals("insurance")) { 8418 this.getInsurance().add((InsuranceComponent) value); 8419 } else if (name.equals("accident")) { 8420 this.accident = (AccidentComponent) value; // AccidentComponent 8421 } else if (name.equals("employmentImpacted")) { 8422 this.employmentImpacted = castToPeriod(value); // Period 8423 } else if (name.equals("hospitalization")) { 8424 this.hospitalization = castToPeriod(value); // Period 8425 } else if (name.equals("item")) { 8426 this.getItem().add((ItemComponent) value); 8427 } else if (name.equals("total")) { 8428 this.total = castToMoney(value); // Money 8429 } else 8430 return super.setProperty(name, value); 8431 return value; 8432 } 8433 8434 @Override 8435 public Base makeProperty(int hash, String name) throws FHIRException { 8436 switch (hash) { 8437 case -1618432855: return addIdentifier(); 8438 case -892481550: return getStatusElement(); 8439 case 3575610: return getType(); 8440 case -1868521062: return addSubType(); 8441 case 116103: return getUseElement(); 8442 case -791418107: return getPatient(); 8443 case -332066046: return getBillablePeriod(); 8444 case 1028554472: return getCreatedElement(); 8445 case -1591951995: return getEnterer(); 8446 case 1957615864: return getInsurer(); 8447 case -987494927: return getProvider(); 8448 case 1178922291: return getOrganization(); 8449 case -1165461084: return getPriority(); 8450 case 1314609806: return getFundsReserve(); 8451 case 1090493483: return addRelated(); 8452 case 460301338: return getPrescription(); 8453 case -1814015861: return getOriginalPrescription(); 8454 case 106443592: return getPayee(); 8455 case -722568291: return getReferral(); 8456 case 501116579: return getFacility(); 8457 case -7323378: return addCareTeam(); 8458 case 1968600364: return addInformation(); 8459 case 1196993265: return addDiagnosis(); 8460 case -1095204141: return addProcedure(); 8461 case 73049818: return addInsurance(); 8462 case -2143202801: return getAccident(); 8463 case 1051487345: return getEmploymentImpacted(); 8464 case 1057894634: return getHospitalization(); 8465 case 3242771: return addItem(); 8466 case 110549828: return getTotal(); 8467 default: return super.makeProperty(hash, name); 8468 } 8469 8470 } 8471 8472 @Override 8473 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8474 switch (hash) { 8475 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 8476 case -892481550: /*status*/ return new String[] {"code"}; 8477 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 8478 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 8479 case 116103: /*use*/ return new String[] {"code"}; 8480 case -791418107: /*patient*/ return new String[] {"Reference"}; 8481 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 8482 case 1028554472: /*created*/ return new String[] {"dateTime"}; 8483 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 8484 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 8485 case -987494927: /*provider*/ return new String[] {"Reference"}; 8486 case 1178922291: /*organization*/ return new String[] {"Reference"}; 8487 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 8488 case 1314609806: /*fundsReserve*/ return new String[] {"CodeableConcept"}; 8489 case 1090493483: /*related*/ return new String[] {}; 8490 case 460301338: /*prescription*/ return new String[] {"Reference"}; 8491 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 8492 case 106443592: /*payee*/ return new String[] {}; 8493 case -722568291: /*referral*/ return new String[] {"Reference"}; 8494 case 501116579: /*facility*/ return new String[] {"Reference"}; 8495 case -7323378: /*careTeam*/ return new String[] {}; 8496 case 1968600364: /*information*/ return new String[] {}; 8497 case 1196993265: /*diagnosis*/ return new String[] {}; 8498 case -1095204141: /*procedure*/ return new String[] {}; 8499 case 73049818: /*insurance*/ return new String[] {}; 8500 case -2143202801: /*accident*/ return new String[] {}; 8501 case 1051487345: /*employmentImpacted*/ return new String[] {"Period"}; 8502 case 1057894634: /*hospitalization*/ return new String[] {"Period"}; 8503 case 3242771: /*item*/ return new String[] {}; 8504 case 110549828: /*total*/ return new String[] {"Money"}; 8505 default: return super.getTypesForProperty(hash, name); 8506 } 8507 8508 } 8509 8510 @Override 8511 public Base addChild(String name) throws FHIRException { 8512 if (name.equals("identifier")) { 8513 return addIdentifier(); 8514 } 8515 else if (name.equals("status")) { 8516 throw new FHIRException("Cannot call addChild on a singleton property Claim.status"); 8517 } 8518 else if (name.equals("type")) { 8519 this.type = new CodeableConcept(); 8520 return this.type; 8521 } 8522 else if (name.equals("subType")) { 8523 return addSubType(); 8524 } 8525 else if (name.equals("use")) { 8526 throw new FHIRException("Cannot call addChild on a singleton property Claim.use"); 8527 } 8528 else if (name.equals("patient")) { 8529 this.patient = new Reference(); 8530 return this.patient; 8531 } 8532 else if (name.equals("billablePeriod")) { 8533 this.billablePeriod = new Period(); 8534 return this.billablePeriod; 8535 } 8536 else if (name.equals("created")) { 8537 throw new FHIRException("Cannot call addChild on a singleton property Claim.created"); 8538 } 8539 else if (name.equals("enterer")) { 8540 this.enterer = new Reference(); 8541 return this.enterer; 8542 } 8543 else if (name.equals("insurer")) { 8544 this.insurer = new Reference(); 8545 return this.insurer; 8546 } 8547 else if (name.equals("provider")) { 8548 this.provider = new Reference(); 8549 return this.provider; 8550 } 8551 else if (name.equals("organization")) { 8552 this.organization = new Reference(); 8553 return this.organization; 8554 } 8555 else if (name.equals("priority")) { 8556 this.priority = new CodeableConcept(); 8557 return this.priority; 8558 } 8559 else if (name.equals("fundsReserve")) { 8560 this.fundsReserve = new CodeableConcept(); 8561 return this.fundsReserve; 8562 } 8563 else if (name.equals("related")) { 8564 return addRelated(); 8565 } 8566 else if (name.equals("prescription")) { 8567 this.prescription = new Reference(); 8568 return this.prescription; 8569 } 8570 else if (name.equals("originalPrescription")) { 8571 this.originalPrescription = new Reference(); 8572 return this.originalPrescription; 8573 } 8574 else if (name.equals("payee")) { 8575 this.payee = new PayeeComponent(); 8576 return this.payee; 8577 } 8578 else if (name.equals("referral")) { 8579 this.referral = new Reference(); 8580 return this.referral; 8581 } 8582 else if (name.equals("facility")) { 8583 this.facility = new Reference(); 8584 return this.facility; 8585 } 8586 else if (name.equals("careTeam")) { 8587 return addCareTeam(); 8588 } 8589 else if (name.equals("information")) { 8590 return addInformation(); 8591 } 8592 else if (name.equals("diagnosis")) { 8593 return addDiagnosis(); 8594 } 8595 else if (name.equals("procedure")) { 8596 return addProcedure(); 8597 } 8598 else if (name.equals("insurance")) { 8599 return addInsurance(); 8600 } 8601 else if (name.equals("accident")) { 8602 this.accident = new AccidentComponent(); 8603 return this.accident; 8604 } 8605 else if (name.equals("employmentImpacted")) { 8606 this.employmentImpacted = new Period(); 8607 return this.employmentImpacted; 8608 } 8609 else if (name.equals("hospitalization")) { 8610 this.hospitalization = new Period(); 8611 return this.hospitalization; 8612 } 8613 else if (name.equals("item")) { 8614 return addItem(); 8615 } 8616 else if (name.equals("total")) { 8617 this.total = new Money(); 8618 return this.total; 8619 } 8620 else 8621 return super.addChild(name); 8622 } 8623 8624 public String fhirType() { 8625 return "Claim"; 8626 8627 } 8628 8629 public Claim copy() { 8630 Claim dst = new Claim(); 8631 copyValues(dst); 8632 if (identifier != null) { 8633 dst.identifier = new ArrayList<Identifier>(); 8634 for (Identifier i : identifier) 8635 dst.identifier.add(i.copy()); 8636 }; 8637 dst.status = status == null ? null : status.copy(); 8638 dst.type = type == null ? null : type.copy(); 8639 if (subType != null) { 8640 dst.subType = new ArrayList<CodeableConcept>(); 8641 for (CodeableConcept i : subType) 8642 dst.subType.add(i.copy()); 8643 }; 8644 dst.use = use == null ? null : use.copy(); 8645 dst.patient = patient == null ? null : patient.copy(); 8646 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 8647 dst.created = created == null ? null : created.copy(); 8648 dst.enterer = enterer == null ? null : enterer.copy(); 8649 dst.insurer = insurer == null ? null : insurer.copy(); 8650 dst.provider = provider == null ? null : provider.copy(); 8651 dst.organization = organization == null ? null : organization.copy(); 8652 dst.priority = priority == null ? null : priority.copy(); 8653 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 8654 if (related != null) { 8655 dst.related = new ArrayList<RelatedClaimComponent>(); 8656 for (RelatedClaimComponent i : related) 8657 dst.related.add(i.copy()); 8658 }; 8659 dst.prescription = prescription == null ? null : prescription.copy(); 8660 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 8661 dst.payee = payee == null ? null : payee.copy(); 8662 dst.referral = referral == null ? null : referral.copy(); 8663 dst.facility = facility == null ? null : facility.copy(); 8664 if (careTeam != null) { 8665 dst.careTeam = new ArrayList<CareTeamComponent>(); 8666 for (CareTeamComponent i : careTeam) 8667 dst.careTeam.add(i.copy()); 8668 }; 8669 if (information != null) { 8670 dst.information = new ArrayList<SpecialConditionComponent>(); 8671 for (SpecialConditionComponent i : information) 8672 dst.information.add(i.copy()); 8673 }; 8674 if (diagnosis != null) { 8675 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 8676 for (DiagnosisComponent i : diagnosis) 8677 dst.diagnosis.add(i.copy()); 8678 }; 8679 if (procedure != null) { 8680 dst.procedure = new ArrayList<ProcedureComponent>(); 8681 for (ProcedureComponent i : procedure) 8682 dst.procedure.add(i.copy()); 8683 }; 8684 if (insurance != null) { 8685 dst.insurance = new ArrayList<InsuranceComponent>(); 8686 for (InsuranceComponent i : insurance) 8687 dst.insurance.add(i.copy()); 8688 }; 8689 dst.accident = accident == null ? null : accident.copy(); 8690 dst.employmentImpacted = employmentImpacted == null ? null : employmentImpacted.copy(); 8691 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 8692 if (item != null) { 8693 dst.item = new ArrayList<ItemComponent>(); 8694 for (ItemComponent i : item) 8695 dst.item.add(i.copy()); 8696 }; 8697 dst.total = total == null ? null : total.copy(); 8698 return dst; 8699 } 8700 8701 protected Claim typedCopy() { 8702 return copy(); 8703 } 8704 8705 @Override 8706 public boolean equalsDeep(Base other_) { 8707 if (!super.equalsDeep(other_)) 8708 return false; 8709 if (!(other_ instanceof Claim)) 8710 return false; 8711 Claim o = (Claim) other_; 8712 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 8713 && compareDeep(subType, o.subType, true) && compareDeep(use, o.use, true) && compareDeep(patient, o.patient, true) 8714 && compareDeep(billablePeriod, o.billablePeriod, true) && compareDeep(created, o.created, true) 8715 && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) && compareDeep(provider, o.provider, true) 8716 && compareDeep(organization, o.organization, true) && compareDeep(priority, o.priority, true) && compareDeep(fundsReserve, o.fundsReserve, true) 8717 && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) && compareDeep(originalPrescription, o.originalPrescription, true) 8718 && compareDeep(payee, o.payee, true) && compareDeep(referral, o.referral, true) && compareDeep(facility, o.facility, true) 8719 && compareDeep(careTeam, o.careTeam, true) && compareDeep(information, o.information, true) && compareDeep(diagnosis, o.diagnosis, true) 8720 && compareDeep(procedure, o.procedure, true) && compareDeep(insurance, o.insurance, true) && compareDeep(accident, o.accident, true) 8721 && compareDeep(employmentImpacted, o.employmentImpacted, true) && compareDeep(hospitalization, o.hospitalization, true) 8722 && compareDeep(item, o.item, true) && compareDeep(total, o.total, true); 8723 } 8724 8725 @Override 8726 public boolean equalsShallow(Base other_) { 8727 if (!super.equalsShallow(other_)) 8728 return false; 8729 if (!(other_ instanceof Claim)) 8730 return false; 8731 Claim o = (Claim) other_; 8732 return compareValues(status, o.status, true) && compareValues(use, o.use, true) && compareValues(created, o.created, true) 8733 ; 8734 } 8735 8736 public boolean isEmpty() { 8737 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 8738 , subType, use, patient, billablePeriod, created, enterer, insurer, provider 8739 , organization, priority, fundsReserve, related, prescription, originalPrescription 8740 , payee, referral, facility, careTeam, information, diagnosis, procedure, insurance 8741 , accident, employmentImpacted, hospitalization, item, total); 8742 } 8743 8744 @Override 8745 public ResourceType getResourceType() { 8746 return ResourceType.Claim; 8747 } 8748 8749 /** 8750 * Search parameter: <b>care-team</b> 8751 * <p> 8752 * Description: <b>Member of the CareTeam</b><br> 8753 * Type: <b>reference</b><br> 8754 * Path: <b>Claim.careTeam.provider</b><br> 8755 * </p> 8756 */ 8757 @SearchParamDefinition(name="care-team", path="Claim.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 8758 public static final String SP_CARE_TEAM = "care-team"; 8759 /** 8760 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 8761 * <p> 8762 * Description: <b>Member of the CareTeam</b><br> 8763 * Type: <b>reference</b><br> 8764 * Path: <b>Claim.careTeam.provider</b><br> 8765 * </p> 8766 */ 8767 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 8768 8769/** 8770 * Constant for fluent queries to be used to add include statements. Specifies 8771 * the path value of "<b>Claim:care-team</b>". 8772 */ 8773 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("Claim:care-team").toLocked(); 8774 8775 /** 8776 * Search parameter: <b>identifier</b> 8777 * <p> 8778 * Description: <b>The primary identifier of the financial resource</b><br> 8779 * Type: <b>token</b><br> 8780 * Path: <b>Claim.identifier</b><br> 8781 * </p> 8782 */ 8783 @SearchParamDefinition(name="identifier", path="Claim.identifier", description="The primary identifier of the financial resource", type="token" ) 8784 public static final String SP_IDENTIFIER = "identifier"; 8785 /** 8786 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 8787 * <p> 8788 * Description: <b>The primary identifier of the financial resource</b><br> 8789 * Type: <b>token</b><br> 8790 * Path: <b>Claim.identifier</b><br> 8791 * </p> 8792 */ 8793 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 8794 8795 /** 8796 * Search parameter: <b>use</b> 8797 * <p> 8798 * Description: <b>The kind of financial resource</b><br> 8799 * Type: <b>token</b><br> 8800 * Path: <b>Claim.use</b><br> 8801 * </p> 8802 */ 8803 @SearchParamDefinition(name="use", path="Claim.use", description="The kind of financial resource", type="token" ) 8804 public static final String SP_USE = "use"; 8805 /** 8806 * <b>Fluent Client</b> search parameter constant for <b>use</b> 8807 * <p> 8808 * Description: <b>The kind of financial resource</b><br> 8809 * Type: <b>token</b><br> 8810 * Path: <b>Claim.use</b><br> 8811 * </p> 8812 */ 8813 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_USE); 8814 8815 /** 8816 * Search parameter: <b>created</b> 8817 * <p> 8818 * Description: <b>The creation date for the Claim</b><br> 8819 * Type: <b>date</b><br> 8820 * Path: <b>Claim.created</b><br> 8821 * </p> 8822 */ 8823 @SearchParamDefinition(name="created", path="Claim.created", description="The creation date for the Claim", type="date" ) 8824 public static final String SP_CREATED = "created"; 8825 /** 8826 * <b>Fluent Client</b> search parameter constant for <b>created</b> 8827 * <p> 8828 * Description: <b>The creation date for the Claim</b><br> 8829 * Type: <b>date</b><br> 8830 * Path: <b>Claim.created</b><br> 8831 * </p> 8832 */ 8833 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 8834 8835 /** 8836 * Search parameter: <b>encounter</b> 8837 * <p> 8838 * Description: <b>Encounters associated with a billed line item</b><br> 8839 * Type: <b>reference</b><br> 8840 * Path: <b>Claim.item.encounter</b><br> 8841 * </p> 8842 */ 8843 @SearchParamDefinition(name="encounter", path="Claim.item.encounter", description="Encounters associated with a billed line item", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 8844 public static final String SP_ENCOUNTER = "encounter"; 8845 /** 8846 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 8847 * <p> 8848 * Description: <b>Encounters associated with a billed line item</b><br> 8849 * Type: <b>reference</b><br> 8850 * Path: <b>Claim.item.encounter</b><br> 8851 * </p> 8852 */ 8853 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 8854 8855/** 8856 * Constant for fluent queries to be used to add include statements. Specifies 8857 * the path value of "<b>Claim:encounter</b>". 8858 */ 8859 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Claim:encounter").toLocked(); 8860 8861 /** 8862 * Search parameter: <b>priority</b> 8863 * <p> 8864 * Description: <b>Processing priority requested</b><br> 8865 * Type: <b>token</b><br> 8866 * Path: <b>Claim.priority</b><br> 8867 * </p> 8868 */ 8869 @SearchParamDefinition(name="priority", path="Claim.priority", description="Processing priority requested", type="token" ) 8870 public static final String SP_PRIORITY = "priority"; 8871 /** 8872 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 8873 * <p> 8874 * Description: <b>Processing priority requested</b><br> 8875 * Type: <b>token</b><br> 8876 * Path: <b>Claim.priority</b><br> 8877 * </p> 8878 */ 8879 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 8880 8881 /** 8882 * Search parameter: <b>payee</b> 8883 * <p> 8884 * Description: <b>The party receiving any payment for the Claim</b><br> 8885 * Type: <b>reference</b><br> 8886 * Path: <b>Claim.payee.party</b><br> 8887 * </p> 8888 */ 8889 @SearchParamDefinition(name="payee", path="Claim.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 8890 public static final String SP_PAYEE = "payee"; 8891 /** 8892 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 8893 * <p> 8894 * Description: <b>The party receiving any payment for the Claim</b><br> 8895 * Type: <b>reference</b><br> 8896 * Path: <b>Claim.payee.party</b><br> 8897 * </p> 8898 */ 8899 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 8900 8901/** 8902 * Constant for fluent queries to be used to add include statements. Specifies 8903 * the path value of "<b>Claim:payee</b>". 8904 */ 8905 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("Claim:payee").toLocked(); 8906 8907 /** 8908 * Search parameter: <b>provider</b> 8909 * <p> 8910 * Description: <b>Provider responsible for the Claim</b><br> 8911 * Type: <b>reference</b><br> 8912 * Path: <b>Claim.provider</b><br> 8913 * </p> 8914 */ 8915 @SearchParamDefinition(name="provider", path="Claim.provider", description="Provider responsible for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 8916 public static final String SP_PROVIDER = "provider"; 8917 /** 8918 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 8919 * <p> 8920 * Description: <b>Provider responsible for the Claim</b><br> 8921 * Type: <b>reference</b><br> 8922 * Path: <b>Claim.provider</b><br> 8923 * </p> 8924 */ 8925 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 8926 8927/** 8928 * Constant for fluent queries to be used to add include statements. Specifies 8929 * the path value of "<b>Claim:provider</b>". 8930 */ 8931 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("Claim:provider").toLocked(); 8932 8933 /** 8934 * Search parameter: <b>patient</b> 8935 * <p> 8936 * Description: <b>Patient receiving the services</b><br> 8937 * Type: <b>reference</b><br> 8938 * Path: <b>Claim.patient</b><br> 8939 * </p> 8940 */ 8941 @SearchParamDefinition(name="patient", path="Claim.patient", description="Patient receiving the services", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 8942 public static final String SP_PATIENT = "patient"; 8943 /** 8944 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 8945 * <p> 8946 * Description: <b>Patient receiving the services</b><br> 8947 * Type: <b>reference</b><br> 8948 * Path: <b>Claim.patient</b><br> 8949 * </p> 8950 */ 8951 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 8952 8953/** 8954 * Constant for fluent queries to be used to add include statements. Specifies 8955 * the path value of "<b>Claim:patient</b>". 8956 */ 8957 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Claim:patient").toLocked(); 8958 8959 /** 8960 * Search parameter: <b>insurer</b> 8961 * <p> 8962 * Description: <b>The target payor/insurer for the Claim</b><br> 8963 * Type: <b>reference</b><br> 8964 * Path: <b>Claim.insurer</b><br> 8965 * </p> 8966 */ 8967 @SearchParamDefinition(name="insurer", path="Claim.insurer", description="The target payor/insurer for the Claim", type="reference", target={Organization.class } ) 8968 public static final String SP_INSURER = "insurer"; 8969 /** 8970 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 8971 * <p> 8972 * Description: <b>The target payor/insurer for the Claim</b><br> 8973 * Type: <b>reference</b><br> 8974 * Path: <b>Claim.insurer</b><br> 8975 * </p> 8976 */ 8977 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 8978 8979/** 8980 * Constant for fluent queries to be used to add include statements. Specifies 8981 * the path value of "<b>Claim:insurer</b>". 8982 */ 8983 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("Claim:insurer").toLocked(); 8984 8985 /** 8986 * Search parameter: <b>organization</b> 8987 * <p> 8988 * Description: <b>The reference to the providing organization</b><br> 8989 * Type: <b>reference</b><br> 8990 * Path: <b>Claim.organization</b><br> 8991 * </p> 8992 */ 8993 @SearchParamDefinition(name="organization", path="Claim.organization", description="The reference to the providing organization", type="reference", target={Organization.class } ) 8994 public static final String SP_ORGANIZATION = "organization"; 8995 /** 8996 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 8997 * <p> 8998 * Description: <b>The reference to the providing organization</b><br> 8999 * Type: <b>reference</b><br> 9000 * Path: <b>Claim.organization</b><br> 9001 * </p> 9002 */ 9003 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 9004 9005/** 9006 * Constant for fluent queries to be used to add include statements. Specifies 9007 * the path value of "<b>Claim:organization</b>". 9008 */ 9009 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Claim:organization").toLocked(); 9010 9011 /** 9012 * Search parameter: <b>enterer</b> 9013 * <p> 9014 * Description: <b>The party responsible for the entry of the Claim</b><br> 9015 * Type: <b>reference</b><br> 9016 * Path: <b>Claim.enterer</b><br> 9017 * </p> 9018 */ 9019 @SearchParamDefinition(name="enterer", path="Claim.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 9020 public static final String SP_ENTERER = "enterer"; 9021 /** 9022 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 9023 * <p> 9024 * Description: <b>The party responsible for the entry of the Claim</b><br> 9025 * Type: <b>reference</b><br> 9026 * Path: <b>Claim.enterer</b><br> 9027 * </p> 9028 */ 9029 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 9030 9031/** 9032 * Constant for fluent queries to be used to add include statements. Specifies 9033 * the path value of "<b>Claim:enterer</b>". 9034 */ 9035 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("Claim:enterer").toLocked(); 9036 9037 /** 9038 * Search parameter: <b>facility</b> 9039 * <p> 9040 * Description: <b>Facility responsible for the goods and services</b><br> 9041 * Type: <b>reference</b><br> 9042 * Path: <b>Claim.facility</b><br> 9043 * </p> 9044 */ 9045 @SearchParamDefinition(name="facility", path="Claim.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class } ) 9046 public static final String SP_FACILITY = "facility"; 9047 /** 9048 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 9049 * <p> 9050 * Description: <b>Facility responsible for the goods and services</b><br> 9051 * Type: <b>reference</b><br> 9052 * Path: <b>Claim.facility</b><br> 9053 * </p> 9054 */ 9055 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 9056 9057/** 9058 * Constant for fluent queries to be used to add include statements. Specifies 9059 * the path value of "<b>Claim:facility</b>". 9060 */ 9061 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("Claim:facility").toLocked(); 9062 9063 9064}