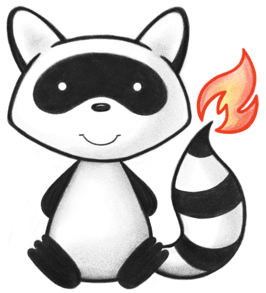
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * This resource provides the adjudication details from the processing of a Claim resource. 050 */ 051@ResourceDef(name="ClaimResponse", profile="http://hl7.org/fhir/Profile/ClaimResponse") 052public class ClaimResponse extends DomainResource { 053 054 public enum ClaimResponseStatus { 055 /** 056 * The instance is currently in-force. 057 */ 058 ACTIVE, 059 /** 060 * The instance is withdrawn, rescinded or reversed. 061 */ 062 CANCELLED, 063 /** 064 * A new instance the contents of which is not complete. 065 */ 066 DRAFT, 067 /** 068 * The instance was entered in error. 069 */ 070 ENTEREDINERROR, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static ClaimResponseStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("active".equals(codeString)) 079 return ACTIVE; 080 if ("cancelled".equals(codeString)) 081 return CANCELLED; 082 if ("draft".equals(codeString)) 083 return DRAFT; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown ClaimResponseStatus code '"+codeString+"'"); 090 } 091 public String toCode() { 092 switch (this) { 093 case ACTIVE: return "active"; 094 case CANCELLED: return "cancelled"; 095 case DRAFT: return "draft"; 096 case ENTEREDINERROR: return "entered-in-error"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getSystem() { 102 switch (this) { 103 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 104 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 105 case DRAFT: return "http://hl7.org/fhir/fm-status"; 106 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case ACTIVE: return "The instance is currently in-force."; 114 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 115 case DRAFT: return "A new instance the contents of which is not complete."; 116 case ENTEREDINERROR: return "The instance was entered in error."; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDisplay() { 122 switch (this) { 123 case ACTIVE: return "Active"; 124 case CANCELLED: return "Cancelled"; 125 case DRAFT: return "Draft"; 126 case ENTEREDINERROR: return "Entered in Error"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 } 132 133 public static class ClaimResponseStatusEnumFactory implements EnumFactory<ClaimResponseStatus> { 134 public ClaimResponseStatus fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("active".equals(codeString)) 139 return ClaimResponseStatus.ACTIVE; 140 if ("cancelled".equals(codeString)) 141 return ClaimResponseStatus.CANCELLED; 142 if ("draft".equals(codeString)) 143 return ClaimResponseStatus.DRAFT; 144 if ("entered-in-error".equals(codeString)) 145 return ClaimResponseStatus.ENTEREDINERROR; 146 throw new IllegalArgumentException("Unknown ClaimResponseStatus code '"+codeString+"'"); 147 } 148 public Enumeration<ClaimResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 149 if (code == null) 150 return null; 151 if (code.isEmpty()) 152 return new Enumeration<ClaimResponseStatus>(this); 153 String codeString = code.asStringValue(); 154 if (codeString == null || "".equals(codeString)) 155 return null; 156 if ("active".equals(codeString)) 157 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.ACTIVE); 158 if ("cancelled".equals(codeString)) 159 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.CANCELLED); 160 if ("draft".equals(codeString)) 161 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.DRAFT); 162 if ("entered-in-error".equals(codeString)) 163 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.ENTEREDINERROR); 164 throw new FHIRException("Unknown ClaimResponseStatus code '"+codeString+"'"); 165 } 166 public String toCode(ClaimResponseStatus code) { 167 if (code == ClaimResponseStatus.NULL) 168 return null; 169 if (code == ClaimResponseStatus.ACTIVE) 170 return "active"; 171 if (code == ClaimResponseStatus.CANCELLED) 172 return "cancelled"; 173 if (code == ClaimResponseStatus.DRAFT) 174 return "draft"; 175 if (code == ClaimResponseStatus.ENTEREDINERROR) 176 return "entered-in-error"; 177 return "?"; 178 } 179 public String toSystem(ClaimResponseStatus code) { 180 return code.getSystem(); 181 } 182 } 183 184 @Block() 185 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 186 /** 187 * A service line number. 188 */ 189 @Child(name = "sequenceLinkId", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 191 protected PositiveIntType sequenceLinkId; 192 193 /** 194 * A list of note references to the notes provided below. 195 */ 196 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 197 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 198 protected List<PositiveIntType> noteNumber; 199 200 /** 201 * The adjudication results. 202 */ 203 @Child(name = "adjudication", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 204 @Description(shortDefinition="Adjudication details", formalDefinition="The adjudication results." ) 205 protected List<AdjudicationComponent> adjudication; 206 207 /** 208 * The second tier service adjudications for submitted services. 209 */ 210 @Child(name = "detail", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 211 @Description(shortDefinition="Detail line items", formalDefinition="The second tier service adjudications for submitted services." ) 212 protected List<ItemDetailComponent> detail; 213 214 private static final long serialVersionUID = -1282041398L; 215 216 /** 217 * Constructor 218 */ 219 public ItemComponent() { 220 super(); 221 } 222 223 /** 224 * Constructor 225 */ 226 public ItemComponent(PositiveIntType sequenceLinkId) { 227 super(); 228 this.sequenceLinkId = sequenceLinkId; 229 } 230 231 /** 232 * @return {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 233 */ 234 public PositiveIntType getSequenceLinkIdElement() { 235 if (this.sequenceLinkId == null) 236 if (Configuration.errorOnAutoCreate()) 237 throw new Error("Attempt to auto-create ItemComponent.sequenceLinkId"); 238 else if (Configuration.doAutoCreate()) 239 this.sequenceLinkId = new PositiveIntType(); // bb 240 return this.sequenceLinkId; 241 } 242 243 public boolean hasSequenceLinkIdElement() { 244 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 245 } 246 247 public boolean hasSequenceLinkId() { 248 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 249 } 250 251 /** 252 * @param value {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 253 */ 254 public ItemComponent setSequenceLinkIdElement(PositiveIntType value) { 255 this.sequenceLinkId = value; 256 return this; 257 } 258 259 /** 260 * @return A service line number. 261 */ 262 public int getSequenceLinkId() { 263 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 264 } 265 266 /** 267 * @param value A service line number. 268 */ 269 public ItemComponent setSequenceLinkId(int value) { 270 if (this.sequenceLinkId == null) 271 this.sequenceLinkId = new PositiveIntType(); 272 this.sequenceLinkId.setValue(value); 273 return this; 274 } 275 276 /** 277 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 278 */ 279 public List<PositiveIntType> getNoteNumber() { 280 if (this.noteNumber == null) 281 this.noteNumber = new ArrayList<PositiveIntType>(); 282 return this.noteNumber; 283 } 284 285 /** 286 * @return Returns a reference to <code>this</code> for easy method chaining 287 */ 288 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 289 this.noteNumber = theNoteNumber; 290 return this; 291 } 292 293 public boolean hasNoteNumber() { 294 if (this.noteNumber == null) 295 return false; 296 for (PositiveIntType item : this.noteNumber) 297 if (!item.isEmpty()) 298 return true; 299 return false; 300 } 301 302 /** 303 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 304 */ 305 public PositiveIntType addNoteNumberElement() {//2 306 PositiveIntType t = new PositiveIntType(); 307 if (this.noteNumber == null) 308 this.noteNumber = new ArrayList<PositiveIntType>(); 309 this.noteNumber.add(t); 310 return t; 311 } 312 313 /** 314 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 315 */ 316 public ItemComponent addNoteNumber(int value) { //1 317 PositiveIntType t = new PositiveIntType(); 318 t.setValue(value); 319 if (this.noteNumber == null) 320 this.noteNumber = new ArrayList<PositiveIntType>(); 321 this.noteNumber.add(t); 322 return this; 323 } 324 325 /** 326 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 327 */ 328 public boolean hasNoteNumber(int value) { 329 if (this.noteNumber == null) 330 return false; 331 for (PositiveIntType v : this.noteNumber) 332 if (v.getValue().equals(value)) // positiveInt 333 return true; 334 return false; 335 } 336 337 /** 338 * @return {@link #adjudication} (The adjudication results.) 339 */ 340 public List<AdjudicationComponent> getAdjudication() { 341 if (this.adjudication == null) 342 this.adjudication = new ArrayList<AdjudicationComponent>(); 343 return this.adjudication; 344 } 345 346 /** 347 * @return Returns a reference to <code>this</code> for easy method chaining 348 */ 349 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 350 this.adjudication = theAdjudication; 351 return this; 352 } 353 354 public boolean hasAdjudication() { 355 if (this.adjudication == null) 356 return false; 357 for (AdjudicationComponent item : this.adjudication) 358 if (!item.isEmpty()) 359 return true; 360 return false; 361 } 362 363 public AdjudicationComponent addAdjudication() { //3 364 AdjudicationComponent t = new AdjudicationComponent(); 365 if (this.adjudication == null) 366 this.adjudication = new ArrayList<AdjudicationComponent>(); 367 this.adjudication.add(t); 368 return t; 369 } 370 371 public ItemComponent addAdjudication(AdjudicationComponent t) { //3 372 if (t == null) 373 return this; 374 if (this.adjudication == null) 375 this.adjudication = new ArrayList<AdjudicationComponent>(); 376 this.adjudication.add(t); 377 return this; 378 } 379 380 /** 381 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 382 */ 383 public AdjudicationComponent getAdjudicationFirstRep() { 384 if (getAdjudication().isEmpty()) { 385 addAdjudication(); 386 } 387 return getAdjudication().get(0); 388 } 389 390 /** 391 * @return {@link #detail} (The second tier service adjudications for submitted services.) 392 */ 393 public List<ItemDetailComponent> getDetail() { 394 if (this.detail == null) 395 this.detail = new ArrayList<ItemDetailComponent>(); 396 return this.detail; 397 } 398 399 /** 400 * @return Returns a reference to <code>this</code> for easy method chaining 401 */ 402 public ItemComponent setDetail(List<ItemDetailComponent> theDetail) { 403 this.detail = theDetail; 404 return this; 405 } 406 407 public boolean hasDetail() { 408 if (this.detail == null) 409 return false; 410 for (ItemDetailComponent item : this.detail) 411 if (!item.isEmpty()) 412 return true; 413 return false; 414 } 415 416 public ItemDetailComponent addDetail() { //3 417 ItemDetailComponent t = new ItemDetailComponent(); 418 if (this.detail == null) 419 this.detail = new ArrayList<ItemDetailComponent>(); 420 this.detail.add(t); 421 return t; 422 } 423 424 public ItemComponent addDetail(ItemDetailComponent t) { //3 425 if (t == null) 426 return this; 427 if (this.detail == null) 428 this.detail = new ArrayList<ItemDetailComponent>(); 429 this.detail.add(t); 430 return this; 431 } 432 433 /** 434 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 435 */ 436 public ItemDetailComponent getDetailFirstRep() { 437 if (getDetail().isEmpty()) { 438 addDetail(); 439 } 440 return getDetail().get(0); 441 } 442 443 protected void listChildren(List<Property> children) { 444 super.listChildren(children); 445 children.add(new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 1, sequenceLinkId)); 446 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 447 children.add(new Property("adjudication", "", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 448 children.add(new Property("detail", "", "The second tier service adjudications for submitted services.", 0, java.lang.Integer.MAX_VALUE, detail)); 449 } 450 451 @Override 452 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 453 switch (_hash) { 454 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 1, sequenceLinkId); 455 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 456 case -231349275: /*adjudication*/ return new Property("adjudication", "", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 457 case -1335224239: /*detail*/ return new Property("detail", "", "The second tier service adjudications for submitted services.", 0, java.lang.Integer.MAX_VALUE, detail); 458 default: return super.getNamedProperty(_hash, _name, _checkValid); 459 } 460 461 } 462 463 @Override 464 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 465 switch (hash) { 466 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : new Base[] {this.sequenceLinkId}; // PositiveIntType 467 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 468 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 469 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // ItemDetailComponent 470 default: return super.getProperty(hash, name, checkValid); 471 } 472 473 } 474 475 @Override 476 public Base setProperty(int hash, String name, Base value) throws FHIRException { 477 switch (hash) { 478 case -1422298666: // sequenceLinkId 479 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 480 return value; 481 case -1110033957: // noteNumber 482 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 483 return value; 484 case -231349275: // adjudication 485 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 486 return value; 487 case -1335224239: // detail 488 this.getDetail().add((ItemDetailComponent) value); // ItemDetailComponent 489 return value; 490 default: return super.setProperty(hash, name, value); 491 } 492 493 } 494 495 @Override 496 public Base setProperty(String name, Base value) throws FHIRException { 497 if (name.equals("sequenceLinkId")) { 498 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 499 } else if (name.equals("noteNumber")) { 500 this.getNoteNumber().add(castToPositiveInt(value)); 501 } else if (name.equals("adjudication")) { 502 this.getAdjudication().add((AdjudicationComponent) value); 503 } else if (name.equals("detail")) { 504 this.getDetail().add((ItemDetailComponent) value); 505 } else 506 return super.setProperty(name, value); 507 return value; 508 } 509 510 @Override 511 public Base makeProperty(int hash, String name) throws FHIRException { 512 switch (hash) { 513 case -1422298666: return getSequenceLinkIdElement(); 514 case -1110033957: return addNoteNumberElement(); 515 case -231349275: return addAdjudication(); 516 case -1335224239: return addDetail(); 517 default: return super.makeProperty(hash, name); 518 } 519 520 } 521 522 @Override 523 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 524 switch (hash) { 525 case -1422298666: /*sequenceLinkId*/ return new String[] {"positiveInt"}; 526 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 527 case -231349275: /*adjudication*/ return new String[] {}; 528 case -1335224239: /*detail*/ return new String[] {}; 529 default: return super.getTypesForProperty(hash, name); 530 } 531 532 } 533 534 @Override 535 public Base addChild(String name) throws FHIRException { 536 if (name.equals("sequenceLinkId")) { 537 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 538 } 539 else if (name.equals("noteNumber")) { 540 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 541 } 542 else if (name.equals("adjudication")) { 543 return addAdjudication(); 544 } 545 else if (name.equals("detail")) { 546 return addDetail(); 547 } 548 else 549 return super.addChild(name); 550 } 551 552 public ItemComponent copy() { 553 ItemComponent dst = new ItemComponent(); 554 copyValues(dst); 555 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 556 if (noteNumber != null) { 557 dst.noteNumber = new ArrayList<PositiveIntType>(); 558 for (PositiveIntType i : noteNumber) 559 dst.noteNumber.add(i.copy()); 560 }; 561 if (adjudication != null) { 562 dst.adjudication = new ArrayList<AdjudicationComponent>(); 563 for (AdjudicationComponent i : adjudication) 564 dst.adjudication.add(i.copy()); 565 }; 566 if (detail != null) { 567 dst.detail = new ArrayList<ItemDetailComponent>(); 568 for (ItemDetailComponent i : detail) 569 dst.detail.add(i.copy()); 570 }; 571 return dst; 572 } 573 574 @Override 575 public boolean equalsDeep(Base other_) { 576 if (!super.equalsDeep(other_)) 577 return false; 578 if (!(other_ instanceof ItemComponent)) 579 return false; 580 ItemComponent o = (ItemComponent) other_; 581 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(noteNumber, o.noteNumber, true) 582 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 583 } 584 585 @Override 586 public boolean equalsShallow(Base other_) { 587 if (!super.equalsShallow(other_)) 588 return false; 589 if (!(other_ instanceof ItemComponent)) 590 return false; 591 ItemComponent o = (ItemComponent) other_; 592 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(noteNumber, o.noteNumber, true) 593 ; 594 } 595 596 public boolean isEmpty() { 597 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId, noteNumber 598 , adjudication, detail); 599 } 600 601 public String fhirType() { 602 return "ClaimResponse.item"; 603 604 } 605 606 } 607 608 @Block() 609 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 610 /** 611 * Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc. 612 */ 613 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 614 @Description(shortDefinition="Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition="Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc." ) 615 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 616 protected CodeableConcept category; 617 618 /** 619 * Adjudication reason such as limit reached. 620 */ 621 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 622 @Description(shortDefinition="Explanation of Adjudication outcome", formalDefinition="Adjudication reason such as limit reached." ) 623 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-reason") 624 protected CodeableConcept reason; 625 626 /** 627 * Monetary amount associated with the code. 628 */ 629 @Child(name = "amount", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 630 @Description(shortDefinition="Monetary amount", formalDefinition="Monetary amount associated with the code." ) 631 protected Money amount; 632 633 /** 634 * A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 635 */ 636 @Child(name = "value", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 637 @Description(shortDefinition="Non-monetary value", formalDefinition="A non-monetary value for example a percentage. Mutually exclusive to the amount element above." ) 638 protected DecimalType value; 639 640 private static final long serialVersionUID = 1559898786L; 641 642 /** 643 * Constructor 644 */ 645 public AdjudicationComponent() { 646 super(); 647 } 648 649 /** 650 * Constructor 651 */ 652 public AdjudicationComponent(CodeableConcept category) { 653 super(); 654 this.category = category; 655 } 656 657 /** 658 * @return {@link #category} (Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.) 659 */ 660 public CodeableConcept getCategory() { 661 if (this.category == null) 662 if (Configuration.errorOnAutoCreate()) 663 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 664 else if (Configuration.doAutoCreate()) 665 this.category = new CodeableConcept(); // cc 666 return this.category; 667 } 668 669 public boolean hasCategory() { 670 return this.category != null && !this.category.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #category} (Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.) 675 */ 676 public AdjudicationComponent setCategory(CodeableConcept value) { 677 this.category = value; 678 return this; 679 } 680 681 /** 682 * @return {@link #reason} (Adjudication reason such as limit reached.) 683 */ 684 public CodeableConcept getReason() { 685 if (this.reason == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 688 else if (Configuration.doAutoCreate()) 689 this.reason = new CodeableConcept(); // cc 690 return this.reason; 691 } 692 693 public boolean hasReason() { 694 return this.reason != null && !this.reason.isEmpty(); 695 } 696 697 /** 698 * @param value {@link #reason} (Adjudication reason such as limit reached.) 699 */ 700 public AdjudicationComponent setReason(CodeableConcept value) { 701 this.reason = value; 702 return this; 703 } 704 705 /** 706 * @return {@link #amount} (Monetary amount associated with the code.) 707 */ 708 public Money getAmount() { 709 if (this.amount == null) 710 if (Configuration.errorOnAutoCreate()) 711 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 712 else if (Configuration.doAutoCreate()) 713 this.amount = new Money(); // cc 714 return this.amount; 715 } 716 717 public boolean hasAmount() { 718 return this.amount != null && !this.amount.isEmpty(); 719 } 720 721 /** 722 * @param value {@link #amount} (Monetary amount associated with the code.) 723 */ 724 public AdjudicationComponent setAmount(Money value) { 725 this.amount = value; 726 return this; 727 } 728 729 /** 730 * @return {@link #value} (A non-monetary value for example a percentage. Mutually exclusive to the amount element above.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 731 */ 732 public DecimalType getValueElement() { 733 if (this.value == null) 734 if (Configuration.errorOnAutoCreate()) 735 throw new Error("Attempt to auto-create AdjudicationComponent.value"); 736 else if (Configuration.doAutoCreate()) 737 this.value = new DecimalType(); // bb 738 return this.value; 739 } 740 741 public boolean hasValueElement() { 742 return this.value != null && !this.value.isEmpty(); 743 } 744 745 public boolean hasValue() { 746 return this.value != null && !this.value.isEmpty(); 747 } 748 749 /** 750 * @param value {@link #value} (A non-monetary value for example a percentage. Mutually exclusive to the amount element above.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 751 */ 752 public AdjudicationComponent setValueElement(DecimalType value) { 753 this.value = value; 754 return this; 755 } 756 757 /** 758 * @return A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 759 */ 760 public BigDecimal getValue() { 761 return this.value == null ? null : this.value.getValue(); 762 } 763 764 /** 765 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 766 */ 767 public AdjudicationComponent setValue(BigDecimal value) { 768 if (value == null) 769 this.value = null; 770 else { 771 if (this.value == null) 772 this.value = new DecimalType(); 773 this.value.setValue(value); 774 } 775 return this; 776 } 777 778 /** 779 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 780 */ 781 public AdjudicationComponent setValue(long value) { 782 this.value = new DecimalType(); 783 this.value.setValue(value); 784 return this; 785 } 786 787 /** 788 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 789 */ 790 public AdjudicationComponent setValue(double value) { 791 this.value = new DecimalType(); 792 this.value.setValue(value); 793 return this; 794 } 795 796 protected void listChildren(List<Property> children) { 797 super.listChildren(children); 798 children.add(new Property("category", "CodeableConcept", "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.", 0, 1, category)); 799 children.add(new Property("reason", "CodeableConcept", "Adjudication reason such as limit reached.", 0, 1, reason)); 800 children.add(new Property("amount", "Money", "Monetary amount associated with the code.", 0, 1, amount)); 801 children.add(new Property("value", "decimal", "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1, value)); 802 } 803 804 @Override 805 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 806 switch (_hash) { 807 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.", 0, 1, category); 808 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Adjudication reason such as limit reached.", 0, 1, reason); 809 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monetary amount associated with the code.", 0, 1, amount); 810 case 111972721: /*value*/ return new Property("value", "decimal", "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1, value); 811 default: return super.getNamedProperty(_hash, _name, _checkValid); 812 } 813 814 } 815 816 @Override 817 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 818 switch (hash) { 819 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 820 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 821 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 822 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DecimalType 823 default: return super.getProperty(hash, name, checkValid); 824 } 825 826 } 827 828 @Override 829 public Base setProperty(int hash, String name, Base value) throws FHIRException { 830 switch (hash) { 831 case 50511102: // category 832 this.category = castToCodeableConcept(value); // CodeableConcept 833 return value; 834 case -934964668: // reason 835 this.reason = castToCodeableConcept(value); // CodeableConcept 836 return value; 837 case -1413853096: // amount 838 this.amount = castToMoney(value); // Money 839 return value; 840 case 111972721: // value 841 this.value = castToDecimal(value); // DecimalType 842 return value; 843 default: return super.setProperty(hash, name, value); 844 } 845 846 } 847 848 @Override 849 public Base setProperty(String name, Base value) throws FHIRException { 850 if (name.equals("category")) { 851 this.category = castToCodeableConcept(value); // CodeableConcept 852 } else if (name.equals("reason")) { 853 this.reason = castToCodeableConcept(value); // CodeableConcept 854 } else if (name.equals("amount")) { 855 this.amount = castToMoney(value); // Money 856 } else if (name.equals("value")) { 857 this.value = castToDecimal(value); // DecimalType 858 } else 859 return super.setProperty(name, value); 860 return value; 861 } 862 863 @Override 864 public Base makeProperty(int hash, String name) throws FHIRException { 865 switch (hash) { 866 case 50511102: return getCategory(); 867 case -934964668: return getReason(); 868 case -1413853096: return getAmount(); 869 case 111972721: return getValueElement(); 870 default: return super.makeProperty(hash, name); 871 } 872 873 } 874 875 @Override 876 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 877 switch (hash) { 878 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 879 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 880 case -1413853096: /*amount*/ return new String[] {"Money"}; 881 case 111972721: /*value*/ return new String[] {"decimal"}; 882 default: return super.getTypesForProperty(hash, name); 883 } 884 885 } 886 887 @Override 888 public Base addChild(String name) throws FHIRException { 889 if (name.equals("category")) { 890 this.category = new CodeableConcept(); 891 return this.category; 892 } 893 else if (name.equals("reason")) { 894 this.reason = new CodeableConcept(); 895 return this.reason; 896 } 897 else if (name.equals("amount")) { 898 this.amount = new Money(); 899 return this.amount; 900 } 901 else if (name.equals("value")) { 902 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 903 } 904 else 905 return super.addChild(name); 906 } 907 908 public AdjudicationComponent copy() { 909 AdjudicationComponent dst = new AdjudicationComponent(); 910 copyValues(dst); 911 dst.category = category == null ? null : category.copy(); 912 dst.reason = reason == null ? null : reason.copy(); 913 dst.amount = amount == null ? null : amount.copy(); 914 dst.value = value == null ? null : value.copy(); 915 return dst; 916 } 917 918 @Override 919 public boolean equalsDeep(Base other_) { 920 if (!super.equalsDeep(other_)) 921 return false; 922 if (!(other_ instanceof AdjudicationComponent)) 923 return false; 924 AdjudicationComponent o = (AdjudicationComponent) other_; 925 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) && compareDeep(amount, o.amount, true) 926 && compareDeep(value, o.value, true); 927 } 928 929 @Override 930 public boolean equalsShallow(Base other_) { 931 if (!super.equalsShallow(other_)) 932 return false; 933 if (!(other_ instanceof AdjudicationComponent)) 934 return false; 935 AdjudicationComponent o = (AdjudicationComponent) other_; 936 return compareValues(value, o.value, true); 937 } 938 939 public boolean isEmpty() { 940 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount 941 , value); 942 } 943 944 public String fhirType() { 945 return "ClaimResponse.item.adjudication"; 946 947 } 948 949 } 950 951 @Block() 952 public static class ItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 953 /** 954 * A service line number. 955 */ 956 @Child(name = "sequenceLinkId", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 957 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 958 protected PositiveIntType sequenceLinkId; 959 960 /** 961 * A list of note references to the notes provided below. 962 */ 963 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 964 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 965 protected List<PositiveIntType> noteNumber; 966 967 /** 968 * The adjudications results. 969 */ 970 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 971 @Description(shortDefinition="Detail level adjudication details", formalDefinition="The adjudications results." ) 972 protected List<AdjudicationComponent> adjudication; 973 974 /** 975 * The third tier service adjudications for submitted services. 976 */ 977 @Child(name = "subDetail", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 978 @Description(shortDefinition="Subdetail line items", formalDefinition="The third tier service adjudications for submitted services." ) 979 protected List<SubDetailComponent> subDetail; 980 981 private static final long serialVersionUID = -1245557773L; 982 983 /** 984 * Constructor 985 */ 986 public ItemDetailComponent() { 987 super(); 988 } 989 990 /** 991 * Constructor 992 */ 993 public ItemDetailComponent(PositiveIntType sequenceLinkId) { 994 super(); 995 this.sequenceLinkId = sequenceLinkId; 996 } 997 998 /** 999 * @return {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 1000 */ 1001 public PositiveIntType getSequenceLinkIdElement() { 1002 if (this.sequenceLinkId == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create ItemDetailComponent.sequenceLinkId"); 1005 else if (Configuration.doAutoCreate()) 1006 this.sequenceLinkId = new PositiveIntType(); // bb 1007 return this.sequenceLinkId; 1008 } 1009 1010 public boolean hasSequenceLinkIdElement() { 1011 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 1012 } 1013 1014 public boolean hasSequenceLinkId() { 1015 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 1020 */ 1021 public ItemDetailComponent setSequenceLinkIdElement(PositiveIntType value) { 1022 this.sequenceLinkId = value; 1023 return this; 1024 } 1025 1026 /** 1027 * @return A service line number. 1028 */ 1029 public int getSequenceLinkId() { 1030 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 1031 } 1032 1033 /** 1034 * @param value A service line number. 1035 */ 1036 public ItemDetailComponent setSequenceLinkId(int value) { 1037 if (this.sequenceLinkId == null) 1038 this.sequenceLinkId = new PositiveIntType(); 1039 this.sequenceLinkId.setValue(value); 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 1045 */ 1046 public List<PositiveIntType> getNoteNumber() { 1047 if (this.noteNumber == null) 1048 this.noteNumber = new ArrayList<PositiveIntType>(); 1049 return this.noteNumber; 1050 } 1051 1052 /** 1053 * @return Returns a reference to <code>this</code> for easy method chaining 1054 */ 1055 public ItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 1056 this.noteNumber = theNoteNumber; 1057 return this; 1058 } 1059 1060 public boolean hasNoteNumber() { 1061 if (this.noteNumber == null) 1062 return false; 1063 for (PositiveIntType item : this.noteNumber) 1064 if (!item.isEmpty()) 1065 return true; 1066 return false; 1067 } 1068 1069 /** 1070 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 1071 */ 1072 public PositiveIntType addNoteNumberElement() {//2 1073 PositiveIntType t = new PositiveIntType(); 1074 if (this.noteNumber == null) 1075 this.noteNumber = new ArrayList<PositiveIntType>(); 1076 this.noteNumber.add(t); 1077 return t; 1078 } 1079 1080 /** 1081 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 1082 */ 1083 public ItemDetailComponent addNoteNumber(int value) { //1 1084 PositiveIntType t = new PositiveIntType(); 1085 t.setValue(value); 1086 if (this.noteNumber == null) 1087 this.noteNumber = new ArrayList<PositiveIntType>(); 1088 this.noteNumber.add(t); 1089 return this; 1090 } 1091 1092 /** 1093 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 1094 */ 1095 public boolean hasNoteNumber(int value) { 1096 if (this.noteNumber == null) 1097 return false; 1098 for (PositiveIntType v : this.noteNumber) 1099 if (v.getValue().equals(value)) // positiveInt 1100 return true; 1101 return false; 1102 } 1103 1104 /** 1105 * @return {@link #adjudication} (The adjudications results.) 1106 */ 1107 public List<AdjudicationComponent> getAdjudication() { 1108 if (this.adjudication == null) 1109 this.adjudication = new ArrayList<AdjudicationComponent>(); 1110 return this.adjudication; 1111 } 1112 1113 /** 1114 * @return Returns a reference to <code>this</code> for easy method chaining 1115 */ 1116 public ItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 1117 this.adjudication = theAdjudication; 1118 return this; 1119 } 1120 1121 public boolean hasAdjudication() { 1122 if (this.adjudication == null) 1123 return false; 1124 for (AdjudicationComponent item : this.adjudication) 1125 if (!item.isEmpty()) 1126 return true; 1127 return false; 1128 } 1129 1130 public AdjudicationComponent addAdjudication() { //3 1131 AdjudicationComponent t = new AdjudicationComponent(); 1132 if (this.adjudication == null) 1133 this.adjudication = new ArrayList<AdjudicationComponent>(); 1134 this.adjudication.add(t); 1135 return t; 1136 } 1137 1138 public ItemDetailComponent addAdjudication(AdjudicationComponent t) { //3 1139 if (t == null) 1140 return this; 1141 if (this.adjudication == null) 1142 this.adjudication = new ArrayList<AdjudicationComponent>(); 1143 this.adjudication.add(t); 1144 return this; 1145 } 1146 1147 /** 1148 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 1149 */ 1150 public AdjudicationComponent getAdjudicationFirstRep() { 1151 if (getAdjudication().isEmpty()) { 1152 addAdjudication(); 1153 } 1154 return getAdjudication().get(0); 1155 } 1156 1157 /** 1158 * @return {@link #subDetail} (The third tier service adjudications for submitted services.) 1159 */ 1160 public List<SubDetailComponent> getSubDetail() { 1161 if (this.subDetail == null) 1162 this.subDetail = new ArrayList<SubDetailComponent>(); 1163 return this.subDetail; 1164 } 1165 1166 /** 1167 * @return Returns a reference to <code>this</code> for easy method chaining 1168 */ 1169 public ItemDetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 1170 this.subDetail = theSubDetail; 1171 return this; 1172 } 1173 1174 public boolean hasSubDetail() { 1175 if (this.subDetail == null) 1176 return false; 1177 for (SubDetailComponent item : this.subDetail) 1178 if (!item.isEmpty()) 1179 return true; 1180 return false; 1181 } 1182 1183 public SubDetailComponent addSubDetail() { //3 1184 SubDetailComponent t = new SubDetailComponent(); 1185 if (this.subDetail == null) 1186 this.subDetail = new ArrayList<SubDetailComponent>(); 1187 this.subDetail.add(t); 1188 return t; 1189 } 1190 1191 public ItemDetailComponent addSubDetail(SubDetailComponent t) { //3 1192 if (t == null) 1193 return this; 1194 if (this.subDetail == null) 1195 this.subDetail = new ArrayList<SubDetailComponent>(); 1196 this.subDetail.add(t); 1197 return this; 1198 } 1199 1200 /** 1201 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist 1202 */ 1203 public SubDetailComponent getSubDetailFirstRep() { 1204 if (getSubDetail().isEmpty()) { 1205 addSubDetail(); 1206 } 1207 return getSubDetail().get(0); 1208 } 1209 1210 protected void listChildren(List<Property> children) { 1211 super.listChildren(children); 1212 children.add(new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 1, sequenceLinkId)); 1213 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 1214 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 1215 children.add(new Property("subDetail", "", "The third tier service adjudications for submitted services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 1216 } 1217 1218 @Override 1219 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1220 switch (_hash) { 1221 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 1, sequenceLinkId); 1222 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 1223 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 1224 case -828829007: /*subDetail*/ return new Property("subDetail", "", "The third tier service adjudications for submitted services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 1225 default: return super.getNamedProperty(_hash, _name, _checkValid); 1226 } 1227 1228 } 1229 1230 @Override 1231 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1232 switch (hash) { 1233 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : new Base[] {this.sequenceLinkId}; // PositiveIntType 1234 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 1235 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 1236 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 1237 default: return super.getProperty(hash, name, checkValid); 1238 } 1239 1240 } 1241 1242 @Override 1243 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1244 switch (hash) { 1245 case -1422298666: // sequenceLinkId 1246 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 1247 return value; 1248 case -1110033957: // noteNumber 1249 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 1250 return value; 1251 case -231349275: // adjudication 1252 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 1253 return value; 1254 case -828829007: // subDetail 1255 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 1256 return value; 1257 default: return super.setProperty(hash, name, value); 1258 } 1259 1260 } 1261 1262 @Override 1263 public Base setProperty(String name, Base value) throws FHIRException { 1264 if (name.equals("sequenceLinkId")) { 1265 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 1266 } else if (name.equals("noteNumber")) { 1267 this.getNoteNumber().add(castToPositiveInt(value)); 1268 } else if (name.equals("adjudication")) { 1269 this.getAdjudication().add((AdjudicationComponent) value); 1270 } else if (name.equals("subDetail")) { 1271 this.getSubDetail().add((SubDetailComponent) value); 1272 } else 1273 return super.setProperty(name, value); 1274 return value; 1275 } 1276 1277 @Override 1278 public Base makeProperty(int hash, String name) throws FHIRException { 1279 switch (hash) { 1280 case -1422298666: return getSequenceLinkIdElement(); 1281 case -1110033957: return addNoteNumberElement(); 1282 case -231349275: return addAdjudication(); 1283 case -828829007: return addSubDetail(); 1284 default: return super.makeProperty(hash, name); 1285 } 1286 1287 } 1288 1289 @Override 1290 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1291 switch (hash) { 1292 case -1422298666: /*sequenceLinkId*/ return new String[] {"positiveInt"}; 1293 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 1294 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 1295 case -828829007: /*subDetail*/ return new String[] {}; 1296 default: return super.getTypesForProperty(hash, name); 1297 } 1298 1299 } 1300 1301 @Override 1302 public Base addChild(String name) throws FHIRException { 1303 if (name.equals("sequenceLinkId")) { 1304 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 1305 } 1306 else if (name.equals("noteNumber")) { 1307 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 1308 } 1309 else if (name.equals("adjudication")) { 1310 return addAdjudication(); 1311 } 1312 else if (name.equals("subDetail")) { 1313 return addSubDetail(); 1314 } 1315 else 1316 return super.addChild(name); 1317 } 1318 1319 public ItemDetailComponent copy() { 1320 ItemDetailComponent dst = new ItemDetailComponent(); 1321 copyValues(dst); 1322 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 1323 if (noteNumber != null) { 1324 dst.noteNumber = new ArrayList<PositiveIntType>(); 1325 for (PositiveIntType i : noteNumber) 1326 dst.noteNumber.add(i.copy()); 1327 }; 1328 if (adjudication != null) { 1329 dst.adjudication = new ArrayList<AdjudicationComponent>(); 1330 for (AdjudicationComponent i : adjudication) 1331 dst.adjudication.add(i.copy()); 1332 }; 1333 if (subDetail != null) { 1334 dst.subDetail = new ArrayList<SubDetailComponent>(); 1335 for (SubDetailComponent i : subDetail) 1336 dst.subDetail.add(i.copy()); 1337 }; 1338 return dst; 1339 } 1340 1341 @Override 1342 public boolean equalsDeep(Base other_) { 1343 if (!super.equalsDeep(other_)) 1344 return false; 1345 if (!(other_ instanceof ItemDetailComponent)) 1346 return false; 1347 ItemDetailComponent o = (ItemDetailComponent) other_; 1348 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(noteNumber, o.noteNumber, true) 1349 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true) 1350 ; 1351 } 1352 1353 @Override 1354 public boolean equalsShallow(Base other_) { 1355 if (!super.equalsShallow(other_)) 1356 return false; 1357 if (!(other_ instanceof ItemDetailComponent)) 1358 return false; 1359 ItemDetailComponent o = (ItemDetailComponent) other_; 1360 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(noteNumber, o.noteNumber, true) 1361 ; 1362 } 1363 1364 public boolean isEmpty() { 1365 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId, noteNumber 1366 , adjudication, subDetail); 1367 } 1368 1369 public String fhirType() { 1370 return "ClaimResponse.item.detail"; 1371 1372 } 1373 1374 } 1375 1376 @Block() 1377 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 1378 /** 1379 * A service line number. 1380 */ 1381 @Child(name = "sequenceLinkId", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1382 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 1383 protected PositiveIntType sequenceLinkId; 1384 1385 /** 1386 * A list of note references to the notes provided below. 1387 */ 1388 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1389 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 1390 protected List<PositiveIntType> noteNumber; 1391 1392 /** 1393 * The adjudications results. 1394 */ 1395 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1396 @Description(shortDefinition="Subdetail level adjudication details", formalDefinition="The adjudications results." ) 1397 protected List<AdjudicationComponent> adjudication; 1398 1399 private static final long serialVersionUID = 1770463342L; 1400 1401 /** 1402 * Constructor 1403 */ 1404 public SubDetailComponent() { 1405 super(); 1406 } 1407 1408 /** 1409 * Constructor 1410 */ 1411 public SubDetailComponent(PositiveIntType sequenceLinkId) { 1412 super(); 1413 this.sequenceLinkId = sequenceLinkId; 1414 } 1415 1416 /** 1417 * @return {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 1418 */ 1419 public PositiveIntType getSequenceLinkIdElement() { 1420 if (this.sequenceLinkId == null) 1421 if (Configuration.errorOnAutoCreate()) 1422 throw new Error("Attempt to auto-create SubDetailComponent.sequenceLinkId"); 1423 else if (Configuration.doAutoCreate()) 1424 this.sequenceLinkId = new PositiveIntType(); // bb 1425 return this.sequenceLinkId; 1426 } 1427 1428 public boolean hasSequenceLinkIdElement() { 1429 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 1430 } 1431 1432 public boolean hasSequenceLinkId() { 1433 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 1434 } 1435 1436 /** 1437 * @param value {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 1438 */ 1439 public SubDetailComponent setSequenceLinkIdElement(PositiveIntType value) { 1440 this.sequenceLinkId = value; 1441 return this; 1442 } 1443 1444 /** 1445 * @return A service line number. 1446 */ 1447 public int getSequenceLinkId() { 1448 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 1449 } 1450 1451 /** 1452 * @param value A service line number. 1453 */ 1454 public SubDetailComponent setSequenceLinkId(int value) { 1455 if (this.sequenceLinkId == null) 1456 this.sequenceLinkId = new PositiveIntType(); 1457 this.sequenceLinkId.setValue(value); 1458 return this; 1459 } 1460 1461 /** 1462 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 1463 */ 1464 public List<PositiveIntType> getNoteNumber() { 1465 if (this.noteNumber == null) 1466 this.noteNumber = new ArrayList<PositiveIntType>(); 1467 return this.noteNumber; 1468 } 1469 1470 /** 1471 * @return Returns a reference to <code>this</code> for easy method chaining 1472 */ 1473 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 1474 this.noteNumber = theNoteNumber; 1475 return this; 1476 } 1477 1478 public boolean hasNoteNumber() { 1479 if (this.noteNumber == null) 1480 return false; 1481 for (PositiveIntType item : this.noteNumber) 1482 if (!item.isEmpty()) 1483 return true; 1484 return false; 1485 } 1486 1487 /** 1488 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 1489 */ 1490 public PositiveIntType addNoteNumberElement() {//2 1491 PositiveIntType t = new PositiveIntType(); 1492 if (this.noteNumber == null) 1493 this.noteNumber = new ArrayList<PositiveIntType>(); 1494 this.noteNumber.add(t); 1495 return t; 1496 } 1497 1498 /** 1499 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 1500 */ 1501 public SubDetailComponent addNoteNumber(int value) { //1 1502 PositiveIntType t = new PositiveIntType(); 1503 t.setValue(value); 1504 if (this.noteNumber == null) 1505 this.noteNumber = new ArrayList<PositiveIntType>(); 1506 this.noteNumber.add(t); 1507 return this; 1508 } 1509 1510 /** 1511 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 1512 */ 1513 public boolean hasNoteNumber(int value) { 1514 if (this.noteNumber == null) 1515 return false; 1516 for (PositiveIntType v : this.noteNumber) 1517 if (v.getValue().equals(value)) // positiveInt 1518 return true; 1519 return false; 1520 } 1521 1522 /** 1523 * @return {@link #adjudication} (The adjudications results.) 1524 */ 1525 public List<AdjudicationComponent> getAdjudication() { 1526 if (this.adjudication == null) 1527 this.adjudication = new ArrayList<AdjudicationComponent>(); 1528 return this.adjudication; 1529 } 1530 1531 /** 1532 * @return Returns a reference to <code>this</code> for easy method chaining 1533 */ 1534 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 1535 this.adjudication = theAdjudication; 1536 return this; 1537 } 1538 1539 public boolean hasAdjudication() { 1540 if (this.adjudication == null) 1541 return false; 1542 for (AdjudicationComponent item : this.adjudication) 1543 if (!item.isEmpty()) 1544 return true; 1545 return false; 1546 } 1547 1548 public AdjudicationComponent addAdjudication() { //3 1549 AdjudicationComponent t = new AdjudicationComponent(); 1550 if (this.adjudication == null) 1551 this.adjudication = new ArrayList<AdjudicationComponent>(); 1552 this.adjudication.add(t); 1553 return t; 1554 } 1555 1556 public SubDetailComponent addAdjudication(AdjudicationComponent t) { //3 1557 if (t == null) 1558 return this; 1559 if (this.adjudication == null) 1560 this.adjudication = new ArrayList<AdjudicationComponent>(); 1561 this.adjudication.add(t); 1562 return this; 1563 } 1564 1565 /** 1566 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 1567 */ 1568 public AdjudicationComponent getAdjudicationFirstRep() { 1569 if (getAdjudication().isEmpty()) { 1570 addAdjudication(); 1571 } 1572 return getAdjudication().get(0); 1573 } 1574 1575 protected void listChildren(List<Property> children) { 1576 super.listChildren(children); 1577 children.add(new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 1, sequenceLinkId)); 1578 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 1579 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 1580 } 1581 1582 @Override 1583 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1584 switch (_hash) { 1585 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 1, sequenceLinkId); 1586 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 1587 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 1588 default: return super.getNamedProperty(_hash, _name, _checkValid); 1589 } 1590 1591 } 1592 1593 @Override 1594 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1595 switch (hash) { 1596 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : new Base[] {this.sequenceLinkId}; // PositiveIntType 1597 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 1598 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 1599 default: return super.getProperty(hash, name, checkValid); 1600 } 1601 1602 } 1603 1604 @Override 1605 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1606 switch (hash) { 1607 case -1422298666: // sequenceLinkId 1608 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 1609 return value; 1610 case -1110033957: // noteNumber 1611 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 1612 return value; 1613 case -231349275: // adjudication 1614 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 1615 return value; 1616 default: return super.setProperty(hash, name, value); 1617 } 1618 1619 } 1620 1621 @Override 1622 public Base setProperty(String name, Base value) throws FHIRException { 1623 if (name.equals("sequenceLinkId")) { 1624 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 1625 } else if (name.equals("noteNumber")) { 1626 this.getNoteNumber().add(castToPositiveInt(value)); 1627 } else if (name.equals("adjudication")) { 1628 this.getAdjudication().add((AdjudicationComponent) value); 1629 } else 1630 return super.setProperty(name, value); 1631 return value; 1632 } 1633 1634 @Override 1635 public Base makeProperty(int hash, String name) throws FHIRException { 1636 switch (hash) { 1637 case -1422298666: return getSequenceLinkIdElement(); 1638 case -1110033957: return addNoteNumberElement(); 1639 case -231349275: return addAdjudication(); 1640 default: return super.makeProperty(hash, name); 1641 } 1642 1643 } 1644 1645 @Override 1646 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1647 switch (hash) { 1648 case -1422298666: /*sequenceLinkId*/ return new String[] {"positiveInt"}; 1649 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 1650 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 1651 default: return super.getTypesForProperty(hash, name); 1652 } 1653 1654 } 1655 1656 @Override 1657 public Base addChild(String name) throws FHIRException { 1658 if (name.equals("sequenceLinkId")) { 1659 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 1660 } 1661 else if (name.equals("noteNumber")) { 1662 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 1663 } 1664 else if (name.equals("adjudication")) { 1665 return addAdjudication(); 1666 } 1667 else 1668 return super.addChild(name); 1669 } 1670 1671 public SubDetailComponent copy() { 1672 SubDetailComponent dst = new SubDetailComponent(); 1673 copyValues(dst); 1674 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 1675 if (noteNumber != null) { 1676 dst.noteNumber = new ArrayList<PositiveIntType>(); 1677 for (PositiveIntType i : noteNumber) 1678 dst.noteNumber.add(i.copy()); 1679 }; 1680 if (adjudication != null) { 1681 dst.adjudication = new ArrayList<AdjudicationComponent>(); 1682 for (AdjudicationComponent i : adjudication) 1683 dst.adjudication.add(i.copy()); 1684 }; 1685 return dst; 1686 } 1687 1688 @Override 1689 public boolean equalsDeep(Base other_) { 1690 if (!super.equalsDeep(other_)) 1691 return false; 1692 if (!(other_ instanceof SubDetailComponent)) 1693 return false; 1694 SubDetailComponent o = (SubDetailComponent) other_; 1695 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(noteNumber, o.noteNumber, true) 1696 && compareDeep(adjudication, o.adjudication, true); 1697 } 1698 1699 @Override 1700 public boolean equalsShallow(Base other_) { 1701 if (!super.equalsShallow(other_)) 1702 return false; 1703 if (!(other_ instanceof SubDetailComponent)) 1704 return false; 1705 SubDetailComponent o = (SubDetailComponent) other_; 1706 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(noteNumber, o.noteNumber, true) 1707 ; 1708 } 1709 1710 public boolean isEmpty() { 1711 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId, noteNumber 1712 , adjudication); 1713 } 1714 1715 public String fhirType() { 1716 return "ClaimResponse.item.detail.subDetail"; 1717 1718 } 1719 1720 } 1721 1722 @Block() 1723 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 1724 /** 1725 * List of input service items which this service line is intended to replace. 1726 */ 1727 @Child(name = "sequenceLinkId", type = {PositiveIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1728 @Description(shortDefinition="Service instances", formalDefinition="List of input service items which this service line is intended to replace." ) 1729 protected List<PositiveIntType> sequenceLinkId; 1730 1731 /** 1732 * The type of reveneu or cost center providing the product and/or service. 1733 */ 1734 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1735 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 1736 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 1737 protected CodeableConcept revenue; 1738 1739 /** 1740 * Health Care Service Type Codes to identify the classification of service or benefits. 1741 */ 1742 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1743 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 1744 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 1745 protected CodeableConcept category; 1746 1747 /** 1748 * A code to indicate the Professional Service or Product supplied. 1749 */ 1750 @Child(name = "service", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1751 @Description(shortDefinition="Group, Service or Product", formalDefinition="A code to indicate the Professional Service or Product supplied." ) 1752 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 1753 protected CodeableConcept service; 1754 1755 /** 1756 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 1757 */ 1758 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1759 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 1760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 1761 protected List<CodeableConcept> modifier; 1762 1763 /** 1764 * The fee charged for the professional service or product.. 1765 */ 1766 @Child(name = "fee", type = {Money.class}, order=6, min=0, max=1, modifier=false, summary=false) 1767 @Description(shortDefinition="Professional fee or Product charge", formalDefinition="The fee charged for the professional service or product.." ) 1768 protected Money fee; 1769 1770 /** 1771 * A list of note references to the notes provided below. 1772 */ 1773 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1774 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 1775 protected List<PositiveIntType> noteNumber; 1776 1777 /** 1778 * The adjudications results. 1779 */ 1780 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1781 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudications results." ) 1782 protected List<AdjudicationComponent> adjudication; 1783 1784 /** 1785 * The second tier service adjudications for payor added services. 1786 */ 1787 @Child(name = "detail", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1788 @Description(shortDefinition="Added items details", formalDefinition="The second tier service adjudications for payor added services." ) 1789 protected List<AddedItemsDetailComponent> detail; 1790 1791 private static final long serialVersionUID = 1969703165L; 1792 1793 /** 1794 * Constructor 1795 */ 1796 public AddedItemComponent() { 1797 super(); 1798 } 1799 1800 /** 1801 * @return {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 1802 */ 1803 public List<PositiveIntType> getSequenceLinkId() { 1804 if (this.sequenceLinkId == null) 1805 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 1806 return this.sequenceLinkId; 1807 } 1808 1809 /** 1810 * @return Returns a reference to <code>this</code> for easy method chaining 1811 */ 1812 public AddedItemComponent setSequenceLinkId(List<PositiveIntType> theSequenceLinkId) { 1813 this.sequenceLinkId = theSequenceLinkId; 1814 return this; 1815 } 1816 1817 public boolean hasSequenceLinkId() { 1818 if (this.sequenceLinkId == null) 1819 return false; 1820 for (PositiveIntType item : this.sequenceLinkId) 1821 if (!item.isEmpty()) 1822 return true; 1823 return false; 1824 } 1825 1826 /** 1827 * @return {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 1828 */ 1829 public PositiveIntType addSequenceLinkIdElement() {//2 1830 PositiveIntType t = new PositiveIntType(); 1831 if (this.sequenceLinkId == null) 1832 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 1833 this.sequenceLinkId.add(t); 1834 return t; 1835 } 1836 1837 /** 1838 * @param value {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 1839 */ 1840 public AddedItemComponent addSequenceLinkId(int value) { //1 1841 PositiveIntType t = new PositiveIntType(); 1842 t.setValue(value); 1843 if (this.sequenceLinkId == null) 1844 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 1845 this.sequenceLinkId.add(t); 1846 return this; 1847 } 1848 1849 /** 1850 * @param value {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 1851 */ 1852 public boolean hasSequenceLinkId(int value) { 1853 if (this.sequenceLinkId == null) 1854 return false; 1855 for (PositiveIntType v : this.sequenceLinkId) 1856 if (v.getValue().equals(value)) // positiveInt 1857 return true; 1858 return false; 1859 } 1860 1861 /** 1862 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 1863 */ 1864 public CodeableConcept getRevenue() { 1865 if (this.revenue == null) 1866 if (Configuration.errorOnAutoCreate()) 1867 throw new Error("Attempt to auto-create AddedItemComponent.revenue"); 1868 else if (Configuration.doAutoCreate()) 1869 this.revenue = new CodeableConcept(); // cc 1870 return this.revenue; 1871 } 1872 1873 public boolean hasRevenue() { 1874 return this.revenue != null && !this.revenue.isEmpty(); 1875 } 1876 1877 /** 1878 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 1879 */ 1880 public AddedItemComponent setRevenue(CodeableConcept value) { 1881 this.revenue = value; 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 1887 */ 1888 public CodeableConcept getCategory() { 1889 if (this.category == null) 1890 if (Configuration.errorOnAutoCreate()) 1891 throw new Error("Attempt to auto-create AddedItemComponent.category"); 1892 else if (Configuration.doAutoCreate()) 1893 this.category = new CodeableConcept(); // cc 1894 return this.category; 1895 } 1896 1897 public boolean hasCategory() { 1898 return this.category != null && !this.category.isEmpty(); 1899 } 1900 1901 /** 1902 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 1903 */ 1904 public AddedItemComponent setCategory(CodeableConcept value) { 1905 this.category = value; 1906 return this; 1907 } 1908 1909 /** 1910 * @return {@link #service} (A code to indicate the Professional Service or Product supplied.) 1911 */ 1912 public CodeableConcept getService() { 1913 if (this.service == null) 1914 if (Configuration.errorOnAutoCreate()) 1915 throw new Error("Attempt to auto-create AddedItemComponent.service"); 1916 else if (Configuration.doAutoCreate()) 1917 this.service = new CodeableConcept(); // cc 1918 return this.service; 1919 } 1920 1921 public boolean hasService() { 1922 return this.service != null && !this.service.isEmpty(); 1923 } 1924 1925 /** 1926 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied.) 1927 */ 1928 public AddedItemComponent setService(CodeableConcept value) { 1929 this.service = value; 1930 return this; 1931 } 1932 1933 /** 1934 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 1935 */ 1936 public List<CodeableConcept> getModifier() { 1937 if (this.modifier == null) 1938 this.modifier = new ArrayList<CodeableConcept>(); 1939 return this.modifier; 1940 } 1941 1942 /** 1943 * @return Returns a reference to <code>this</code> for easy method chaining 1944 */ 1945 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 1946 this.modifier = theModifier; 1947 return this; 1948 } 1949 1950 public boolean hasModifier() { 1951 if (this.modifier == null) 1952 return false; 1953 for (CodeableConcept item : this.modifier) 1954 if (!item.isEmpty()) 1955 return true; 1956 return false; 1957 } 1958 1959 public CodeableConcept addModifier() { //3 1960 CodeableConcept t = new CodeableConcept(); 1961 if (this.modifier == null) 1962 this.modifier = new ArrayList<CodeableConcept>(); 1963 this.modifier.add(t); 1964 return t; 1965 } 1966 1967 public AddedItemComponent addModifier(CodeableConcept t) { //3 1968 if (t == null) 1969 return this; 1970 if (this.modifier == null) 1971 this.modifier = new ArrayList<CodeableConcept>(); 1972 this.modifier.add(t); 1973 return this; 1974 } 1975 1976 /** 1977 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 1978 */ 1979 public CodeableConcept getModifierFirstRep() { 1980 if (getModifier().isEmpty()) { 1981 addModifier(); 1982 } 1983 return getModifier().get(0); 1984 } 1985 1986 /** 1987 * @return {@link #fee} (The fee charged for the professional service or product..) 1988 */ 1989 public Money getFee() { 1990 if (this.fee == null) 1991 if (Configuration.errorOnAutoCreate()) 1992 throw new Error("Attempt to auto-create AddedItemComponent.fee"); 1993 else if (Configuration.doAutoCreate()) 1994 this.fee = new Money(); // cc 1995 return this.fee; 1996 } 1997 1998 public boolean hasFee() { 1999 return this.fee != null && !this.fee.isEmpty(); 2000 } 2001 2002 /** 2003 * @param value {@link #fee} (The fee charged for the professional service or product..) 2004 */ 2005 public AddedItemComponent setFee(Money value) { 2006 this.fee = value; 2007 return this; 2008 } 2009 2010 /** 2011 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 2012 */ 2013 public List<PositiveIntType> getNoteNumber() { 2014 if (this.noteNumber == null) 2015 this.noteNumber = new ArrayList<PositiveIntType>(); 2016 return this.noteNumber; 2017 } 2018 2019 /** 2020 * @return Returns a reference to <code>this</code> for easy method chaining 2021 */ 2022 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 2023 this.noteNumber = theNoteNumber; 2024 return this; 2025 } 2026 2027 public boolean hasNoteNumber() { 2028 if (this.noteNumber == null) 2029 return false; 2030 for (PositiveIntType item : this.noteNumber) 2031 if (!item.isEmpty()) 2032 return true; 2033 return false; 2034 } 2035 2036 /** 2037 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 2038 */ 2039 public PositiveIntType addNoteNumberElement() {//2 2040 PositiveIntType t = new PositiveIntType(); 2041 if (this.noteNumber == null) 2042 this.noteNumber = new ArrayList<PositiveIntType>(); 2043 this.noteNumber.add(t); 2044 return t; 2045 } 2046 2047 /** 2048 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 2049 */ 2050 public AddedItemComponent addNoteNumber(int value) { //1 2051 PositiveIntType t = new PositiveIntType(); 2052 t.setValue(value); 2053 if (this.noteNumber == null) 2054 this.noteNumber = new ArrayList<PositiveIntType>(); 2055 this.noteNumber.add(t); 2056 return this; 2057 } 2058 2059 /** 2060 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 2061 */ 2062 public boolean hasNoteNumber(int value) { 2063 if (this.noteNumber == null) 2064 return false; 2065 for (PositiveIntType v : this.noteNumber) 2066 if (v.getValue().equals(value)) // positiveInt 2067 return true; 2068 return false; 2069 } 2070 2071 /** 2072 * @return {@link #adjudication} (The adjudications results.) 2073 */ 2074 public List<AdjudicationComponent> getAdjudication() { 2075 if (this.adjudication == null) 2076 this.adjudication = new ArrayList<AdjudicationComponent>(); 2077 return this.adjudication; 2078 } 2079 2080 /** 2081 * @return Returns a reference to <code>this</code> for easy method chaining 2082 */ 2083 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 2084 this.adjudication = theAdjudication; 2085 return this; 2086 } 2087 2088 public boolean hasAdjudication() { 2089 if (this.adjudication == null) 2090 return false; 2091 for (AdjudicationComponent item : this.adjudication) 2092 if (!item.isEmpty()) 2093 return true; 2094 return false; 2095 } 2096 2097 public AdjudicationComponent addAdjudication() { //3 2098 AdjudicationComponent t = new AdjudicationComponent(); 2099 if (this.adjudication == null) 2100 this.adjudication = new ArrayList<AdjudicationComponent>(); 2101 this.adjudication.add(t); 2102 return t; 2103 } 2104 2105 public AddedItemComponent addAdjudication(AdjudicationComponent t) { //3 2106 if (t == null) 2107 return this; 2108 if (this.adjudication == null) 2109 this.adjudication = new ArrayList<AdjudicationComponent>(); 2110 this.adjudication.add(t); 2111 return this; 2112 } 2113 2114 /** 2115 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 2116 */ 2117 public AdjudicationComponent getAdjudicationFirstRep() { 2118 if (getAdjudication().isEmpty()) { 2119 addAdjudication(); 2120 } 2121 return getAdjudication().get(0); 2122 } 2123 2124 /** 2125 * @return {@link #detail} (The second tier service adjudications for payor added services.) 2126 */ 2127 public List<AddedItemsDetailComponent> getDetail() { 2128 if (this.detail == null) 2129 this.detail = new ArrayList<AddedItemsDetailComponent>(); 2130 return this.detail; 2131 } 2132 2133 /** 2134 * @return Returns a reference to <code>this</code> for easy method chaining 2135 */ 2136 public AddedItemComponent setDetail(List<AddedItemsDetailComponent> theDetail) { 2137 this.detail = theDetail; 2138 return this; 2139 } 2140 2141 public boolean hasDetail() { 2142 if (this.detail == null) 2143 return false; 2144 for (AddedItemsDetailComponent item : this.detail) 2145 if (!item.isEmpty()) 2146 return true; 2147 return false; 2148 } 2149 2150 public AddedItemsDetailComponent addDetail() { //3 2151 AddedItemsDetailComponent t = new AddedItemsDetailComponent(); 2152 if (this.detail == null) 2153 this.detail = new ArrayList<AddedItemsDetailComponent>(); 2154 this.detail.add(t); 2155 return t; 2156 } 2157 2158 public AddedItemComponent addDetail(AddedItemsDetailComponent t) { //3 2159 if (t == null) 2160 return this; 2161 if (this.detail == null) 2162 this.detail = new ArrayList<AddedItemsDetailComponent>(); 2163 this.detail.add(t); 2164 return this; 2165 } 2166 2167 /** 2168 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 2169 */ 2170 public AddedItemsDetailComponent getDetailFirstRep() { 2171 if (getDetail().isEmpty()) { 2172 addDetail(); 2173 } 2174 return getDetail().get(0); 2175 } 2176 2177 protected void listChildren(List<Property> children) { 2178 super.listChildren(children); 2179 children.add(new Property("sequenceLinkId", "positiveInt", "List of input service items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, sequenceLinkId)); 2180 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 2181 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 2182 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied.", 0, 1, service)); 2183 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 2184 children.add(new Property("fee", "Money", "The fee charged for the professional service or product..", 0, 1, fee)); 2185 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 2186 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 2187 children.add(new Property("detail", "", "The second tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail)); 2188 } 2189 2190 @Override 2191 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2192 switch (_hash) { 2193 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "positiveInt", "List of input service items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, sequenceLinkId); 2194 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 2195 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 2196 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied.", 0, 1, service); 2197 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 2198 case 101254: /*fee*/ return new Property("fee", "Money", "The fee charged for the professional service or product..", 0, 1, fee); 2199 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 2200 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 2201 case -1335224239: /*detail*/ return new Property("detail", "", "The second tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 2202 default: return super.getNamedProperty(_hash, _name, _checkValid); 2203 } 2204 2205 } 2206 2207 @Override 2208 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2209 switch (hash) { 2210 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : this.sequenceLinkId.toArray(new Base[this.sequenceLinkId.size()]); // PositiveIntType 2211 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 2212 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2213 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 2214 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 2215 case 101254: /*fee*/ return this.fee == null ? new Base[0] : new Base[] {this.fee}; // Money 2216 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 2217 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 2218 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemsDetailComponent 2219 default: return super.getProperty(hash, name, checkValid); 2220 } 2221 2222 } 2223 2224 @Override 2225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2226 switch (hash) { 2227 case -1422298666: // sequenceLinkId 2228 this.getSequenceLinkId().add(castToPositiveInt(value)); // PositiveIntType 2229 return value; 2230 case 1099842588: // revenue 2231 this.revenue = castToCodeableConcept(value); // CodeableConcept 2232 return value; 2233 case 50511102: // category 2234 this.category = castToCodeableConcept(value); // CodeableConcept 2235 return value; 2236 case 1984153269: // service 2237 this.service = castToCodeableConcept(value); // CodeableConcept 2238 return value; 2239 case -615513385: // modifier 2240 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 2241 return value; 2242 case 101254: // fee 2243 this.fee = castToMoney(value); // Money 2244 return value; 2245 case -1110033957: // noteNumber 2246 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 2247 return value; 2248 case -231349275: // adjudication 2249 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 2250 return value; 2251 case -1335224239: // detail 2252 this.getDetail().add((AddedItemsDetailComponent) value); // AddedItemsDetailComponent 2253 return value; 2254 default: return super.setProperty(hash, name, value); 2255 } 2256 2257 } 2258 2259 @Override 2260 public Base setProperty(String name, Base value) throws FHIRException { 2261 if (name.equals("sequenceLinkId")) { 2262 this.getSequenceLinkId().add(castToPositiveInt(value)); 2263 } else if (name.equals("revenue")) { 2264 this.revenue = castToCodeableConcept(value); // CodeableConcept 2265 } else if (name.equals("category")) { 2266 this.category = castToCodeableConcept(value); // CodeableConcept 2267 } else if (name.equals("service")) { 2268 this.service = castToCodeableConcept(value); // CodeableConcept 2269 } else if (name.equals("modifier")) { 2270 this.getModifier().add(castToCodeableConcept(value)); 2271 } else if (name.equals("fee")) { 2272 this.fee = castToMoney(value); // Money 2273 } else if (name.equals("noteNumber")) { 2274 this.getNoteNumber().add(castToPositiveInt(value)); 2275 } else if (name.equals("adjudication")) { 2276 this.getAdjudication().add((AdjudicationComponent) value); 2277 } else if (name.equals("detail")) { 2278 this.getDetail().add((AddedItemsDetailComponent) value); 2279 } else 2280 return super.setProperty(name, value); 2281 return value; 2282 } 2283 2284 @Override 2285 public Base makeProperty(int hash, String name) throws FHIRException { 2286 switch (hash) { 2287 case -1422298666: return addSequenceLinkIdElement(); 2288 case 1099842588: return getRevenue(); 2289 case 50511102: return getCategory(); 2290 case 1984153269: return getService(); 2291 case -615513385: return addModifier(); 2292 case 101254: return getFee(); 2293 case -1110033957: return addNoteNumberElement(); 2294 case -231349275: return addAdjudication(); 2295 case -1335224239: return addDetail(); 2296 default: return super.makeProperty(hash, name); 2297 } 2298 2299 } 2300 2301 @Override 2302 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2303 switch (hash) { 2304 case -1422298666: /*sequenceLinkId*/ return new String[] {"positiveInt"}; 2305 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 2306 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2307 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 2308 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 2309 case 101254: /*fee*/ return new String[] {"Money"}; 2310 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 2311 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 2312 case -1335224239: /*detail*/ return new String[] {}; 2313 default: return super.getTypesForProperty(hash, name); 2314 } 2315 2316 } 2317 2318 @Override 2319 public Base addChild(String name) throws FHIRException { 2320 if (name.equals("sequenceLinkId")) { 2321 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 2322 } 2323 else if (name.equals("revenue")) { 2324 this.revenue = new CodeableConcept(); 2325 return this.revenue; 2326 } 2327 else if (name.equals("category")) { 2328 this.category = new CodeableConcept(); 2329 return this.category; 2330 } 2331 else if (name.equals("service")) { 2332 this.service = new CodeableConcept(); 2333 return this.service; 2334 } 2335 else if (name.equals("modifier")) { 2336 return addModifier(); 2337 } 2338 else if (name.equals("fee")) { 2339 this.fee = new Money(); 2340 return this.fee; 2341 } 2342 else if (name.equals("noteNumber")) { 2343 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 2344 } 2345 else if (name.equals("adjudication")) { 2346 return addAdjudication(); 2347 } 2348 else if (name.equals("detail")) { 2349 return addDetail(); 2350 } 2351 else 2352 return super.addChild(name); 2353 } 2354 2355 public AddedItemComponent copy() { 2356 AddedItemComponent dst = new AddedItemComponent(); 2357 copyValues(dst); 2358 if (sequenceLinkId != null) { 2359 dst.sequenceLinkId = new ArrayList<PositiveIntType>(); 2360 for (PositiveIntType i : sequenceLinkId) 2361 dst.sequenceLinkId.add(i.copy()); 2362 }; 2363 dst.revenue = revenue == null ? null : revenue.copy(); 2364 dst.category = category == null ? null : category.copy(); 2365 dst.service = service == null ? null : service.copy(); 2366 if (modifier != null) { 2367 dst.modifier = new ArrayList<CodeableConcept>(); 2368 for (CodeableConcept i : modifier) 2369 dst.modifier.add(i.copy()); 2370 }; 2371 dst.fee = fee == null ? null : fee.copy(); 2372 if (noteNumber != null) { 2373 dst.noteNumber = new ArrayList<PositiveIntType>(); 2374 for (PositiveIntType i : noteNumber) 2375 dst.noteNumber.add(i.copy()); 2376 }; 2377 if (adjudication != null) { 2378 dst.adjudication = new ArrayList<AdjudicationComponent>(); 2379 for (AdjudicationComponent i : adjudication) 2380 dst.adjudication.add(i.copy()); 2381 }; 2382 if (detail != null) { 2383 dst.detail = new ArrayList<AddedItemsDetailComponent>(); 2384 for (AddedItemsDetailComponent i : detail) 2385 dst.detail.add(i.copy()); 2386 }; 2387 return dst; 2388 } 2389 2390 @Override 2391 public boolean equalsDeep(Base other_) { 2392 if (!super.equalsDeep(other_)) 2393 return false; 2394 if (!(other_ instanceof AddedItemComponent)) 2395 return false; 2396 AddedItemComponent o = (AddedItemComponent) other_; 2397 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(revenue, o.revenue, true) 2398 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 2399 && compareDeep(fee, o.fee, true) && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 2400 && compareDeep(detail, o.detail, true); 2401 } 2402 2403 @Override 2404 public boolean equalsShallow(Base other_) { 2405 if (!super.equalsShallow(other_)) 2406 return false; 2407 if (!(other_ instanceof AddedItemComponent)) 2408 return false; 2409 AddedItemComponent o = (AddedItemComponent) other_; 2410 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(noteNumber, o.noteNumber, true) 2411 ; 2412 } 2413 2414 public boolean isEmpty() { 2415 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId, revenue, category 2416 , service, modifier, fee, noteNumber, adjudication, detail); 2417 } 2418 2419 public String fhirType() { 2420 return "ClaimResponse.addItem"; 2421 2422 } 2423 2424 } 2425 2426 @Block() 2427 public static class AddedItemsDetailComponent extends BackboneElement implements IBaseBackboneElement { 2428 /** 2429 * The type of reveneu or cost center providing the product and/or service. 2430 */ 2431 @Child(name = "revenue", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 2432 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 2433 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 2434 protected CodeableConcept revenue; 2435 2436 /** 2437 * Health Care Service Type Codes to identify the classification of service or benefits. 2438 */ 2439 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2440 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 2441 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 2442 protected CodeableConcept category; 2443 2444 /** 2445 * A code to indicate the Professional Service or Product supplied. 2446 */ 2447 @Child(name = "service", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 2448 @Description(shortDefinition="Service or Product", formalDefinition="A code to indicate the Professional Service or Product supplied." ) 2449 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 2450 protected CodeableConcept service; 2451 2452 /** 2453 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 2454 */ 2455 @Child(name = "modifier", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2456 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 2457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 2458 protected List<CodeableConcept> modifier; 2459 2460 /** 2461 * The fee charged for the professional service or product.. 2462 */ 2463 @Child(name = "fee", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 2464 @Description(shortDefinition="Professional fee or Product charge", formalDefinition="The fee charged for the professional service or product.." ) 2465 protected Money fee; 2466 2467 /** 2468 * A list of note references to the notes provided below. 2469 */ 2470 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2471 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 2472 protected List<PositiveIntType> noteNumber; 2473 2474 /** 2475 * The adjudications results. 2476 */ 2477 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2478 @Description(shortDefinition="Added items detail adjudication", formalDefinition="The adjudications results." ) 2479 protected List<AdjudicationComponent> adjudication; 2480 2481 private static final long serialVersionUID = -311484980L; 2482 2483 /** 2484 * Constructor 2485 */ 2486 public AddedItemsDetailComponent() { 2487 super(); 2488 } 2489 2490 /** 2491 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 2492 */ 2493 public CodeableConcept getRevenue() { 2494 if (this.revenue == null) 2495 if (Configuration.errorOnAutoCreate()) 2496 throw new Error("Attempt to auto-create AddedItemsDetailComponent.revenue"); 2497 else if (Configuration.doAutoCreate()) 2498 this.revenue = new CodeableConcept(); // cc 2499 return this.revenue; 2500 } 2501 2502 public boolean hasRevenue() { 2503 return this.revenue != null && !this.revenue.isEmpty(); 2504 } 2505 2506 /** 2507 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 2508 */ 2509 public AddedItemsDetailComponent setRevenue(CodeableConcept value) { 2510 this.revenue = value; 2511 return this; 2512 } 2513 2514 /** 2515 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 2516 */ 2517 public CodeableConcept getCategory() { 2518 if (this.category == null) 2519 if (Configuration.errorOnAutoCreate()) 2520 throw new Error("Attempt to auto-create AddedItemsDetailComponent.category"); 2521 else if (Configuration.doAutoCreate()) 2522 this.category = new CodeableConcept(); // cc 2523 return this.category; 2524 } 2525 2526 public boolean hasCategory() { 2527 return this.category != null && !this.category.isEmpty(); 2528 } 2529 2530 /** 2531 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 2532 */ 2533 public AddedItemsDetailComponent setCategory(CodeableConcept value) { 2534 this.category = value; 2535 return this; 2536 } 2537 2538 /** 2539 * @return {@link #service} (A code to indicate the Professional Service or Product supplied.) 2540 */ 2541 public CodeableConcept getService() { 2542 if (this.service == null) 2543 if (Configuration.errorOnAutoCreate()) 2544 throw new Error("Attempt to auto-create AddedItemsDetailComponent.service"); 2545 else if (Configuration.doAutoCreate()) 2546 this.service = new CodeableConcept(); // cc 2547 return this.service; 2548 } 2549 2550 public boolean hasService() { 2551 return this.service != null && !this.service.isEmpty(); 2552 } 2553 2554 /** 2555 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied.) 2556 */ 2557 public AddedItemsDetailComponent setService(CodeableConcept value) { 2558 this.service = value; 2559 return this; 2560 } 2561 2562 /** 2563 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 2564 */ 2565 public List<CodeableConcept> getModifier() { 2566 if (this.modifier == null) 2567 this.modifier = new ArrayList<CodeableConcept>(); 2568 return this.modifier; 2569 } 2570 2571 /** 2572 * @return Returns a reference to <code>this</code> for easy method chaining 2573 */ 2574 public AddedItemsDetailComponent setModifier(List<CodeableConcept> theModifier) { 2575 this.modifier = theModifier; 2576 return this; 2577 } 2578 2579 public boolean hasModifier() { 2580 if (this.modifier == null) 2581 return false; 2582 for (CodeableConcept item : this.modifier) 2583 if (!item.isEmpty()) 2584 return true; 2585 return false; 2586 } 2587 2588 public CodeableConcept addModifier() { //3 2589 CodeableConcept t = new CodeableConcept(); 2590 if (this.modifier == null) 2591 this.modifier = new ArrayList<CodeableConcept>(); 2592 this.modifier.add(t); 2593 return t; 2594 } 2595 2596 public AddedItemsDetailComponent addModifier(CodeableConcept t) { //3 2597 if (t == null) 2598 return this; 2599 if (this.modifier == null) 2600 this.modifier = new ArrayList<CodeableConcept>(); 2601 this.modifier.add(t); 2602 return this; 2603 } 2604 2605 /** 2606 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 2607 */ 2608 public CodeableConcept getModifierFirstRep() { 2609 if (getModifier().isEmpty()) { 2610 addModifier(); 2611 } 2612 return getModifier().get(0); 2613 } 2614 2615 /** 2616 * @return {@link #fee} (The fee charged for the professional service or product..) 2617 */ 2618 public Money getFee() { 2619 if (this.fee == null) 2620 if (Configuration.errorOnAutoCreate()) 2621 throw new Error("Attempt to auto-create AddedItemsDetailComponent.fee"); 2622 else if (Configuration.doAutoCreate()) 2623 this.fee = new Money(); // cc 2624 return this.fee; 2625 } 2626 2627 public boolean hasFee() { 2628 return this.fee != null && !this.fee.isEmpty(); 2629 } 2630 2631 /** 2632 * @param value {@link #fee} (The fee charged for the professional service or product..) 2633 */ 2634 public AddedItemsDetailComponent setFee(Money value) { 2635 this.fee = value; 2636 return this; 2637 } 2638 2639 /** 2640 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 2641 */ 2642 public List<PositiveIntType> getNoteNumber() { 2643 if (this.noteNumber == null) 2644 this.noteNumber = new ArrayList<PositiveIntType>(); 2645 return this.noteNumber; 2646 } 2647 2648 /** 2649 * @return Returns a reference to <code>this</code> for easy method chaining 2650 */ 2651 public AddedItemsDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 2652 this.noteNumber = theNoteNumber; 2653 return this; 2654 } 2655 2656 public boolean hasNoteNumber() { 2657 if (this.noteNumber == null) 2658 return false; 2659 for (PositiveIntType item : this.noteNumber) 2660 if (!item.isEmpty()) 2661 return true; 2662 return false; 2663 } 2664 2665 /** 2666 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 2667 */ 2668 public PositiveIntType addNoteNumberElement() {//2 2669 PositiveIntType t = new PositiveIntType(); 2670 if (this.noteNumber == null) 2671 this.noteNumber = new ArrayList<PositiveIntType>(); 2672 this.noteNumber.add(t); 2673 return t; 2674 } 2675 2676 /** 2677 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 2678 */ 2679 public AddedItemsDetailComponent addNoteNumber(int value) { //1 2680 PositiveIntType t = new PositiveIntType(); 2681 t.setValue(value); 2682 if (this.noteNumber == null) 2683 this.noteNumber = new ArrayList<PositiveIntType>(); 2684 this.noteNumber.add(t); 2685 return this; 2686 } 2687 2688 /** 2689 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 2690 */ 2691 public boolean hasNoteNumber(int value) { 2692 if (this.noteNumber == null) 2693 return false; 2694 for (PositiveIntType v : this.noteNumber) 2695 if (v.getValue().equals(value)) // positiveInt 2696 return true; 2697 return false; 2698 } 2699 2700 /** 2701 * @return {@link #adjudication} (The adjudications results.) 2702 */ 2703 public List<AdjudicationComponent> getAdjudication() { 2704 if (this.adjudication == null) 2705 this.adjudication = new ArrayList<AdjudicationComponent>(); 2706 return this.adjudication; 2707 } 2708 2709 /** 2710 * @return Returns a reference to <code>this</code> for easy method chaining 2711 */ 2712 public AddedItemsDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 2713 this.adjudication = theAdjudication; 2714 return this; 2715 } 2716 2717 public boolean hasAdjudication() { 2718 if (this.adjudication == null) 2719 return false; 2720 for (AdjudicationComponent item : this.adjudication) 2721 if (!item.isEmpty()) 2722 return true; 2723 return false; 2724 } 2725 2726 public AdjudicationComponent addAdjudication() { //3 2727 AdjudicationComponent t = new AdjudicationComponent(); 2728 if (this.adjudication == null) 2729 this.adjudication = new ArrayList<AdjudicationComponent>(); 2730 this.adjudication.add(t); 2731 return t; 2732 } 2733 2734 public AddedItemsDetailComponent addAdjudication(AdjudicationComponent t) { //3 2735 if (t == null) 2736 return this; 2737 if (this.adjudication == null) 2738 this.adjudication = new ArrayList<AdjudicationComponent>(); 2739 this.adjudication.add(t); 2740 return this; 2741 } 2742 2743 /** 2744 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 2745 */ 2746 public AdjudicationComponent getAdjudicationFirstRep() { 2747 if (getAdjudication().isEmpty()) { 2748 addAdjudication(); 2749 } 2750 return getAdjudication().get(0); 2751 } 2752 2753 protected void listChildren(List<Property> children) { 2754 super.listChildren(children); 2755 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 2756 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 2757 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied.", 0, 1, service)); 2758 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 2759 children.add(new Property("fee", "Money", "The fee charged for the professional service or product..", 0, 1, fee)); 2760 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 2761 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 2762 } 2763 2764 @Override 2765 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2766 switch (_hash) { 2767 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 2768 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 2769 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied.", 0, 1, service); 2770 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 2771 case 101254: /*fee*/ return new Property("fee", "Money", "The fee charged for the professional service or product..", 0, 1, fee); 2772 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 2773 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 2774 default: return super.getNamedProperty(_hash, _name, _checkValid); 2775 } 2776 2777 } 2778 2779 @Override 2780 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2781 switch (hash) { 2782 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 2783 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2784 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 2785 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 2786 case 101254: /*fee*/ return this.fee == null ? new Base[0] : new Base[] {this.fee}; // Money 2787 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 2788 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 2789 default: return super.getProperty(hash, name, checkValid); 2790 } 2791 2792 } 2793 2794 @Override 2795 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2796 switch (hash) { 2797 case 1099842588: // revenue 2798 this.revenue = castToCodeableConcept(value); // CodeableConcept 2799 return value; 2800 case 50511102: // category 2801 this.category = castToCodeableConcept(value); // CodeableConcept 2802 return value; 2803 case 1984153269: // service 2804 this.service = castToCodeableConcept(value); // CodeableConcept 2805 return value; 2806 case -615513385: // modifier 2807 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 2808 return value; 2809 case 101254: // fee 2810 this.fee = castToMoney(value); // Money 2811 return value; 2812 case -1110033957: // noteNumber 2813 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 2814 return value; 2815 case -231349275: // adjudication 2816 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 2817 return value; 2818 default: return super.setProperty(hash, name, value); 2819 } 2820 2821 } 2822 2823 @Override 2824 public Base setProperty(String name, Base value) throws FHIRException { 2825 if (name.equals("revenue")) { 2826 this.revenue = castToCodeableConcept(value); // CodeableConcept 2827 } else if (name.equals("category")) { 2828 this.category = castToCodeableConcept(value); // CodeableConcept 2829 } else if (name.equals("service")) { 2830 this.service = castToCodeableConcept(value); // CodeableConcept 2831 } else if (name.equals("modifier")) { 2832 this.getModifier().add(castToCodeableConcept(value)); 2833 } else if (name.equals("fee")) { 2834 this.fee = castToMoney(value); // Money 2835 } else if (name.equals("noteNumber")) { 2836 this.getNoteNumber().add(castToPositiveInt(value)); 2837 } else if (name.equals("adjudication")) { 2838 this.getAdjudication().add((AdjudicationComponent) value); 2839 } else 2840 return super.setProperty(name, value); 2841 return value; 2842 } 2843 2844 @Override 2845 public Base makeProperty(int hash, String name) throws FHIRException { 2846 switch (hash) { 2847 case 1099842588: return getRevenue(); 2848 case 50511102: return getCategory(); 2849 case 1984153269: return getService(); 2850 case -615513385: return addModifier(); 2851 case 101254: return getFee(); 2852 case -1110033957: return addNoteNumberElement(); 2853 case -231349275: return addAdjudication(); 2854 default: return super.makeProperty(hash, name); 2855 } 2856 2857 } 2858 2859 @Override 2860 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2861 switch (hash) { 2862 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 2863 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2864 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 2865 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 2866 case 101254: /*fee*/ return new String[] {"Money"}; 2867 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 2868 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 2869 default: return super.getTypesForProperty(hash, name); 2870 } 2871 2872 } 2873 2874 @Override 2875 public Base addChild(String name) throws FHIRException { 2876 if (name.equals("revenue")) { 2877 this.revenue = new CodeableConcept(); 2878 return this.revenue; 2879 } 2880 else if (name.equals("category")) { 2881 this.category = new CodeableConcept(); 2882 return this.category; 2883 } 2884 else if (name.equals("service")) { 2885 this.service = new CodeableConcept(); 2886 return this.service; 2887 } 2888 else if (name.equals("modifier")) { 2889 return addModifier(); 2890 } 2891 else if (name.equals("fee")) { 2892 this.fee = new Money(); 2893 return this.fee; 2894 } 2895 else if (name.equals("noteNumber")) { 2896 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 2897 } 2898 else if (name.equals("adjudication")) { 2899 return addAdjudication(); 2900 } 2901 else 2902 return super.addChild(name); 2903 } 2904 2905 public AddedItemsDetailComponent copy() { 2906 AddedItemsDetailComponent dst = new AddedItemsDetailComponent(); 2907 copyValues(dst); 2908 dst.revenue = revenue == null ? null : revenue.copy(); 2909 dst.category = category == null ? null : category.copy(); 2910 dst.service = service == null ? null : service.copy(); 2911 if (modifier != null) { 2912 dst.modifier = new ArrayList<CodeableConcept>(); 2913 for (CodeableConcept i : modifier) 2914 dst.modifier.add(i.copy()); 2915 }; 2916 dst.fee = fee == null ? null : fee.copy(); 2917 if (noteNumber != null) { 2918 dst.noteNumber = new ArrayList<PositiveIntType>(); 2919 for (PositiveIntType i : noteNumber) 2920 dst.noteNumber.add(i.copy()); 2921 }; 2922 if (adjudication != null) { 2923 dst.adjudication = new ArrayList<AdjudicationComponent>(); 2924 for (AdjudicationComponent i : adjudication) 2925 dst.adjudication.add(i.copy()); 2926 }; 2927 return dst; 2928 } 2929 2930 @Override 2931 public boolean equalsDeep(Base other_) { 2932 if (!super.equalsDeep(other_)) 2933 return false; 2934 if (!(other_ instanceof AddedItemsDetailComponent)) 2935 return false; 2936 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other_; 2937 return compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) 2938 && compareDeep(modifier, o.modifier, true) && compareDeep(fee, o.fee, true) && compareDeep(noteNumber, o.noteNumber, true) 2939 && compareDeep(adjudication, o.adjudication, true); 2940 } 2941 2942 @Override 2943 public boolean equalsShallow(Base other_) { 2944 if (!super.equalsShallow(other_)) 2945 return false; 2946 if (!(other_ instanceof AddedItemsDetailComponent)) 2947 return false; 2948 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other_; 2949 return compareValues(noteNumber, o.noteNumber, true); 2950 } 2951 2952 public boolean isEmpty() { 2953 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(revenue, category, service 2954 , modifier, fee, noteNumber, adjudication); 2955 } 2956 2957 public String fhirType() { 2958 return "ClaimResponse.addItem.detail"; 2959 2960 } 2961 2962 } 2963 2964 @Block() 2965 public static class ErrorComponent extends BackboneElement implements IBaseBackboneElement { 2966 /** 2967 * The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere. 2968 */ 2969 @Child(name = "sequenceLinkId", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2970 @Description(shortDefinition="Item sequence number", formalDefinition="The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere." ) 2971 protected PositiveIntType sequenceLinkId; 2972 2973 /** 2974 * The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition. 2975 */ 2976 @Child(name = "detailSequenceLinkId", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2977 @Description(shortDefinition="Detail sequence number", formalDefinition="The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition." ) 2978 protected PositiveIntType detailSequenceLinkId; 2979 2980 /** 2981 * The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition. 2982 */ 2983 @Child(name = "subdetailSequenceLinkId", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2984 @Description(shortDefinition="Subdetail sequence number", formalDefinition="The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition." ) 2985 protected PositiveIntType subdetailSequenceLinkId; 2986 2987 /** 2988 * An error code,from a specified code system, which details why the claim could not be adjudicated. 2989 */ 2990 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=false) 2991 @Description(shortDefinition="Error code detailing processing issues", formalDefinition="An error code,from a specified code system, which details why the claim could not be adjudicated." ) 2992 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-error") 2993 protected CodeableConcept code; 2994 2995 private static final long serialVersionUID = -1379670472L; 2996 2997 /** 2998 * Constructor 2999 */ 3000 public ErrorComponent() { 3001 super(); 3002 } 3003 3004 /** 3005 * Constructor 3006 */ 3007 public ErrorComponent(CodeableConcept code) { 3008 super(); 3009 this.code = code; 3010 } 3011 3012 /** 3013 * @return {@link #sequenceLinkId} (The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 3014 */ 3015 public PositiveIntType getSequenceLinkIdElement() { 3016 if (this.sequenceLinkId == null) 3017 if (Configuration.errorOnAutoCreate()) 3018 throw new Error("Attempt to auto-create ErrorComponent.sequenceLinkId"); 3019 else if (Configuration.doAutoCreate()) 3020 this.sequenceLinkId = new PositiveIntType(); // bb 3021 return this.sequenceLinkId; 3022 } 3023 3024 public boolean hasSequenceLinkIdElement() { 3025 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 3026 } 3027 3028 public boolean hasSequenceLinkId() { 3029 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 3030 } 3031 3032 /** 3033 * @param value {@link #sequenceLinkId} (The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 3034 */ 3035 public ErrorComponent setSequenceLinkIdElement(PositiveIntType value) { 3036 this.sequenceLinkId = value; 3037 return this; 3038 } 3039 3040 /** 3041 * @return The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere. 3042 */ 3043 public int getSequenceLinkId() { 3044 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 3045 } 3046 3047 /** 3048 * @param value The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere. 3049 */ 3050 public ErrorComponent setSequenceLinkId(int value) { 3051 if (this.sequenceLinkId == null) 3052 this.sequenceLinkId = new PositiveIntType(); 3053 this.sequenceLinkId.setValue(value); 3054 return this; 3055 } 3056 3057 /** 3058 * @return {@link #detailSequenceLinkId} (The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.). This is the underlying object with id, value and extensions. The accessor "getDetailSequenceLinkId" gives direct access to the value 3059 */ 3060 public PositiveIntType getDetailSequenceLinkIdElement() { 3061 if (this.detailSequenceLinkId == null) 3062 if (Configuration.errorOnAutoCreate()) 3063 throw new Error("Attempt to auto-create ErrorComponent.detailSequenceLinkId"); 3064 else if (Configuration.doAutoCreate()) 3065 this.detailSequenceLinkId = new PositiveIntType(); // bb 3066 return this.detailSequenceLinkId; 3067 } 3068 3069 public boolean hasDetailSequenceLinkIdElement() { 3070 return this.detailSequenceLinkId != null && !this.detailSequenceLinkId.isEmpty(); 3071 } 3072 3073 public boolean hasDetailSequenceLinkId() { 3074 return this.detailSequenceLinkId != null && !this.detailSequenceLinkId.isEmpty(); 3075 } 3076 3077 /** 3078 * @param value {@link #detailSequenceLinkId} (The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.). This is the underlying object with id, value and extensions. The accessor "getDetailSequenceLinkId" gives direct access to the value 3079 */ 3080 public ErrorComponent setDetailSequenceLinkIdElement(PositiveIntType value) { 3081 this.detailSequenceLinkId = value; 3082 return this; 3083 } 3084 3085 /** 3086 * @return The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition. 3087 */ 3088 public int getDetailSequenceLinkId() { 3089 return this.detailSequenceLinkId == null || this.detailSequenceLinkId.isEmpty() ? 0 : this.detailSequenceLinkId.getValue(); 3090 } 3091 3092 /** 3093 * @param value The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition. 3094 */ 3095 public ErrorComponent setDetailSequenceLinkId(int value) { 3096 if (this.detailSequenceLinkId == null) 3097 this.detailSequenceLinkId = new PositiveIntType(); 3098 this.detailSequenceLinkId.setValue(value); 3099 return this; 3100 } 3101 3102 /** 3103 * @return {@link #subdetailSequenceLinkId} (The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.). This is the underlying object with id, value and extensions. The accessor "getSubdetailSequenceLinkId" gives direct access to the value 3104 */ 3105 public PositiveIntType getSubdetailSequenceLinkIdElement() { 3106 if (this.subdetailSequenceLinkId == null) 3107 if (Configuration.errorOnAutoCreate()) 3108 throw new Error("Attempt to auto-create ErrorComponent.subdetailSequenceLinkId"); 3109 else if (Configuration.doAutoCreate()) 3110 this.subdetailSequenceLinkId = new PositiveIntType(); // bb 3111 return this.subdetailSequenceLinkId; 3112 } 3113 3114 public boolean hasSubdetailSequenceLinkIdElement() { 3115 return this.subdetailSequenceLinkId != null && !this.subdetailSequenceLinkId.isEmpty(); 3116 } 3117 3118 public boolean hasSubdetailSequenceLinkId() { 3119 return this.subdetailSequenceLinkId != null && !this.subdetailSequenceLinkId.isEmpty(); 3120 } 3121 3122 /** 3123 * @param value {@link #subdetailSequenceLinkId} (The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.). This is the underlying object with id, value and extensions. The accessor "getSubdetailSequenceLinkId" gives direct access to the value 3124 */ 3125 public ErrorComponent setSubdetailSequenceLinkIdElement(PositiveIntType value) { 3126 this.subdetailSequenceLinkId = value; 3127 return this; 3128 } 3129 3130 /** 3131 * @return The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition. 3132 */ 3133 public int getSubdetailSequenceLinkId() { 3134 return this.subdetailSequenceLinkId == null || this.subdetailSequenceLinkId.isEmpty() ? 0 : this.subdetailSequenceLinkId.getValue(); 3135 } 3136 3137 /** 3138 * @param value The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition. 3139 */ 3140 public ErrorComponent setSubdetailSequenceLinkId(int value) { 3141 if (this.subdetailSequenceLinkId == null) 3142 this.subdetailSequenceLinkId = new PositiveIntType(); 3143 this.subdetailSequenceLinkId.setValue(value); 3144 return this; 3145 } 3146 3147 /** 3148 * @return {@link #code} (An error code,from a specified code system, which details why the claim could not be adjudicated.) 3149 */ 3150 public CodeableConcept getCode() { 3151 if (this.code == null) 3152 if (Configuration.errorOnAutoCreate()) 3153 throw new Error("Attempt to auto-create ErrorComponent.code"); 3154 else if (Configuration.doAutoCreate()) 3155 this.code = new CodeableConcept(); // cc 3156 return this.code; 3157 } 3158 3159 public boolean hasCode() { 3160 return this.code != null && !this.code.isEmpty(); 3161 } 3162 3163 /** 3164 * @param value {@link #code} (An error code,from a specified code system, which details why the claim could not be adjudicated.) 3165 */ 3166 public ErrorComponent setCode(CodeableConcept value) { 3167 this.code = value; 3168 return this; 3169 } 3170 3171 protected void listChildren(List<Property> children) { 3172 super.listChildren(children); 3173 children.add(new Property("sequenceLinkId", "positiveInt", "The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere.", 0, 1, sequenceLinkId)); 3174 children.add(new Property("detailSequenceLinkId", "positiveInt", "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.", 0, 1, detailSequenceLinkId)); 3175 children.add(new Property("subdetailSequenceLinkId", "positiveInt", "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.", 0, 1, subdetailSequenceLinkId)); 3176 children.add(new Property("code", "CodeableConcept", "An error code,from a specified code system, which details why the claim could not be adjudicated.", 0, 1, code)); 3177 } 3178 3179 @Override 3180 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3181 switch (_hash) { 3182 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "positiveInt", "The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere.", 0, 1, sequenceLinkId); 3183 case 516748423: /*detailSequenceLinkId*/ return new Property("detailSequenceLinkId", "positiveInt", "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.", 0, 1, detailSequenceLinkId); 3184 case -1061088569: /*subdetailSequenceLinkId*/ return new Property("subdetailSequenceLinkId", "positiveInt", "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.", 0, 1, subdetailSequenceLinkId); 3185 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "An error code,from a specified code system, which details why the claim could not be adjudicated.", 0, 1, code); 3186 default: return super.getNamedProperty(_hash, _name, _checkValid); 3187 } 3188 3189 } 3190 3191 @Override 3192 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3193 switch (hash) { 3194 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : new Base[] {this.sequenceLinkId}; // PositiveIntType 3195 case 516748423: /*detailSequenceLinkId*/ return this.detailSequenceLinkId == null ? new Base[0] : new Base[] {this.detailSequenceLinkId}; // PositiveIntType 3196 case -1061088569: /*subdetailSequenceLinkId*/ return this.subdetailSequenceLinkId == null ? new Base[0] : new Base[] {this.subdetailSequenceLinkId}; // PositiveIntType 3197 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3198 default: return super.getProperty(hash, name, checkValid); 3199 } 3200 3201 } 3202 3203 @Override 3204 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3205 switch (hash) { 3206 case -1422298666: // sequenceLinkId 3207 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 3208 return value; 3209 case 516748423: // detailSequenceLinkId 3210 this.detailSequenceLinkId = castToPositiveInt(value); // PositiveIntType 3211 return value; 3212 case -1061088569: // subdetailSequenceLinkId 3213 this.subdetailSequenceLinkId = castToPositiveInt(value); // PositiveIntType 3214 return value; 3215 case 3059181: // code 3216 this.code = castToCodeableConcept(value); // CodeableConcept 3217 return value; 3218 default: return super.setProperty(hash, name, value); 3219 } 3220 3221 } 3222 3223 @Override 3224 public Base setProperty(String name, Base value) throws FHIRException { 3225 if (name.equals("sequenceLinkId")) { 3226 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 3227 } else if (name.equals("detailSequenceLinkId")) { 3228 this.detailSequenceLinkId = castToPositiveInt(value); // PositiveIntType 3229 } else if (name.equals("subdetailSequenceLinkId")) { 3230 this.subdetailSequenceLinkId = castToPositiveInt(value); // PositiveIntType 3231 } else if (name.equals("code")) { 3232 this.code = castToCodeableConcept(value); // CodeableConcept 3233 } else 3234 return super.setProperty(name, value); 3235 return value; 3236 } 3237 3238 @Override 3239 public Base makeProperty(int hash, String name) throws FHIRException { 3240 switch (hash) { 3241 case -1422298666: return getSequenceLinkIdElement(); 3242 case 516748423: return getDetailSequenceLinkIdElement(); 3243 case -1061088569: return getSubdetailSequenceLinkIdElement(); 3244 case 3059181: return getCode(); 3245 default: return super.makeProperty(hash, name); 3246 } 3247 3248 } 3249 3250 @Override 3251 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3252 switch (hash) { 3253 case -1422298666: /*sequenceLinkId*/ return new String[] {"positiveInt"}; 3254 case 516748423: /*detailSequenceLinkId*/ return new String[] {"positiveInt"}; 3255 case -1061088569: /*subdetailSequenceLinkId*/ return new String[] {"positiveInt"}; 3256 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3257 default: return super.getTypesForProperty(hash, name); 3258 } 3259 3260 } 3261 3262 @Override 3263 public Base addChild(String name) throws FHIRException { 3264 if (name.equals("sequenceLinkId")) { 3265 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 3266 } 3267 else if (name.equals("detailSequenceLinkId")) { 3268 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequenceLinkId"); 3269 } 3270 else if (name.equals("subdetailSequenceLinkId")) { 3271 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subdetailSequenceLinkId"); 3272 } 3273 else if (name.equals("code")) { 3274 this.code = new CodeableConcept(); 3275 return this.code; 3276 } 3277 else 3278 return super.addChild(name); 3279 } 3280 3281 public ErrorComponent copy() { 3282 ErrorComponent dst = new ErrorComponent(); 3283 copyValues(dst); 3284 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 3285 dst.detailSequenceLinkId = detailSequenceLinkId == null ? null : detailSequenceLinkId.copy(); 3286 dst.subdetailSequenceLinkId = subdetailSequenceLinkId == null ? null : subdetailSequenceLinkId.copy(); 3287 dst.code = code == null ? null : code.copy(); 3288 return dst; 3289 } 3290 3291 @Override 3292 public boolean equalsDeep(Base other_) { 3293 if (!super.equalsDeep(other_)) 3294 return false; 3295 if (!(other_ instanceof ErrorComponent)) 3296 return false; 3297 ErrorComponent o = (ErrorComponent) other_; 3298 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(detailSequenceLinkId, o.detailSequenceLinkId, true) 3299 && compareDeep(subdetailSequenceLinkId, o.subdetailSequenceLinkId, true) && compareDeep(code, o.code, true) 3300 ; 3301 } 3302 3303 @Override 3304 public boolean equalsShallow(Base other_) { 3305 if (!super.equalsShallow(other_)) 3306 return false; 3307 if (!(other_ instanceof ErrorComponent)) 3308 return false; 3309 ErrorComponent o = (ErrorComponent) other_; 3310 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(detailSequenceLinkId, o.detailSequenceLinkId, true) 3311 && compareValues(subdetailSequenceLinkId, o.subdetailSequenceLinkId, true); 3312 } 3313 3314 public boolean isEmpty() { 3315 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId, detailSequenceLinkId 3316 , subdetailSequenceLinkId, code); 3317 } 3318 3319 public String fhirType() { 3320 return "ClaimResponse.error"; 3321 3322 } 3323 3324 } 3325 3326 @Block() 3327 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 3328 /** 3329 * Whether this represents partial or complete payment of the claim. 3330 */ 3331 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 3332 @Description(shortDefinition="Partial or Complete", formalDefinition="Whether this represents partial or complete payment of the claim." ) 3333 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-paymenttype") 3334 protected CodeableConcept type; 3335 3336 /** 3337 * Adjustment to the payment of this transaction which is not related to adjudication of this transaction. 3338 */ 3339 @Child(name = "adjustment", type = {Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 3340 @Description(shortDefinition="Payment adjustment for non-Claim issues", formalDefinition="Adjustment to the payment of this transaction which is not related to adjudication of this transaction." ) 3341 protected Money adjustment; 3342 3343 /** 3344 * Reason for the payment adjustment. 3345 */ 3346 @Child(name = "adjustmentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 3347 @Description(shortDefinition="Explanation for the non-claim adjustment", formalDefinition="Reason for the payment adjustment." ) 3348 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 3349 protected CodeableConcept adjustmentReason; 3350 3351 /** 3352 * Estimated payment data. 3353 */ 3354 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3355 @Description(shortDefinition="Expected data of Payment", formalDefinition="Estimated payment data." ) 3356 protected DateType date; 3357 3358 /** 3359 * Payable less any payment adjustment. 3360 */ 3361 @Child(name = "amount", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 3362 @Description(shortDefinition="Payable amount after adjustment", formalDefinition="Payable less any payment adjustment." ) 3363 protected Money amount; 3364 3365 /** 3366 * Payment identifier. 3367 */ 3368 @Child(name = "identifier", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 3369 @Description(shortDefinition="Identifier of the payment instrument", formalDefinition="Payment identifier." ) 3370 protected Identifier identifier; 3371 3372 private static final long serialVersionUID = 1539906026L; 3373 3374 /** 3375 * Constructor 3376 */ 3377 public PaymentComponent() { 3378 super(); 3379 } 3380 3381 /** 3382 * @return {@link #type} (Whether this represents partial or complete payment of the claim.) 3383 */ 3384 public CodeableConcept getType() { 3385 if (this.type == null) 3386 if (Configuration.errorOnAutoCreate()) 3387 throw new Error("Attempt to auto-create PaymentComponent.type"); 3388 else if (Configuration.doAutoCreate()) 3389 this.type = new CodeableConcept(); // cc 3390 return this.type; 3391 } 3392 3393 public boolean hasType() { 3394 return this.type != null && !this.type.isEmpty(); 3395 } 3396 3397 /** 3398 * @param value {@link #type} (Whether this represents partial or complete payment of the claim.) 3399 */ 3400 public PaymentComponent setType(CodeableConcept value) { 3401 this.type = value; 3402 return this; 3403 } 3404 3405 /** 3406 * @return {@link #adjustment} (Adjustment to the payment of this transaction which is not related to adjudication of this transaction.) 3407 */ 3408 public Money getAdjustment() { 3409 if (this.adjustment == null) 3410 if (Configuration.errorOnAutoCreate()) 3411 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 3412 else if (Configuration.doAutoCreate()) 3413 this.adjustment = new Money(); // cc 3414 return this.adjustment; 3415 } 3416 3417 public boolean hasAdjustment() { 3418 return this.adjustment != null && !this.adjustment.isEmpty(); 3419 } 3420 3421 /** 3422 * @param value {@link #adjustment} (Adjustment to the payment of this transaction which is not related to adjudication of this transaction.) 3423 */ 3424 public PaymentComponent setAdjustment(Money value) { 3425 this.adjustment = value; 3426 return this; 3427 } 3428 3429 /** 3430 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 3431 */ 3432 public CodeableConcept getAdjustmentReason() { 3433 if (this.adjustmentReason == null) 3434 if (Configuration.errorOnAutoCreate()) 3435 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 3436 else if (Configuration.doAutoCreate()) 3437 this.adjustmentReason = new CodeableConcept(); // cc 3438 return this.adjustmentReason; 3439 } 3440 3441 public boolean hasAdjustmentReason() { 3442 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 3443 } 3444 3445 /** 3446 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 3447 */ 3448 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 3449 this.adjustmentReason = value; 3450 return this; 3451 } 3452 3453 /** 3454 * @return {@link #date} (Estimated payment data.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3455 */ 3456 public DateType getDateElement() { 3457 if (this.date == null) 3458 if (Configuration.errorOnAutoCreate()) 3459 throw new Error("Attempt to auto-create PaymentComponent.date"); 3460 else if (Configuration.doAutoCreate()) 3461 this.date = new DateType(); // bb 3462 return this.date; 3463 } 3464 3465 public boolean hasDateElement() { 3466 return this.date != null && !this.date.isEmpty(); 3467 } 3468 3469 public boolean hasDate() { 3470 return this.date != null && !this.date.isEmpty(); 3471 } 3472 3473 /** 3474 * @param value {@link #date} (Estimated payment data.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3475 */ 3476 public PaymentComponent setDateElement(DateType value) { 3477 this.date = value; 3478 return this; 3479 } 3480 3481 /** 3482 * @return Estimated payment data. 3483 */ 3484 public Date getDate() { 3485 return this.date == null ? null : this.date.getValue(); 3486 } 3487 3488 /** 3489 * @param value Estimated payment data. 3490 */ 3491 public PaymentComponent setDate(Date value) { 3492 if (value == null) 3493 this.date = null; 3494 else { 3495 if (this.date == null) 3496 this.date = new DateType(); 3497 this.date.setValue(value); 3498 } 3499 return this; 3500 } 3501 3502 /** 3503 * @return {@link #amount} (Payable less any payment adjustment.) 3504 */ 3505 public Money getAmount() { 3506 if (this.amount == null) 3507 if (Configuration.errorOnAutoCreate()) 3508 throw new Error("Attempt to auto-create PaymentComponent.amount"); 3509 else if (Configuration.doAutoCreate()) 3510 this.amount = new Money(); // cc 3511 return this.amount; 3512 } 3513 3514 public boolean hasAmount() { 3515 return this.amount != null && !this.amount.isEmpty(); 3516 } 3517 3518 /** 3519 * @param value {@link #amount} (Payable less any payment adjustment.) 3520 */ 3521 public PaymentComponent setAmount(Money value) { 3522 this.amount = value; 3523 return this; 3524 } 3525 3526 /** 3527 * @return {@link #identifier} (Payment identifier.) 3528 */ 3529 public Identifier getIdentifier() { 3530 if (this.identifier == null) 3531 if (Configuration.errorOnAutoCreate()) 3532 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 3533 else if (Configuration.doAutoCreate()) 3534 this.identifier = new Identifier(); // cc 3535 return this.identifier; 3536 } 3537 3538 public boolean hasIdentifier() { 3539 return this.identifier != null && !this.identifier.isEmpty(); 3540 } 3541 3542 /** 3543 * @param value {@link #identifier} (Payment identifier.) 3544 */ 3545 public PaymentComponent setIdentifier(Identifier value) { 3546 this.identifier = value; 3547 return this; 3548 } 3549 3550 protected void listChildren(List<Property> children) { 3551 super.listChildren(children); 3552 children.add(new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the claim.", 0, 1, type)); 3553 children.add(new Property("adjustment", "Money", "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.", 0, 1, adjustment)); 3554 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason)); 3555 children.add(new Property("date", "date", "Estimated payment data.", 0, 1, date)); 3556 children.add(new Property("amount", "Money", "Payable less any payment adjustment.", 0, 1, amount)); 3557 children.add(new Property("identifier", "Identifier", "Payment identifier.", 0, 1, identifier)); 3558 } 3559 3560 @Override 3561 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3562 switch (_hash) { 3563 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the claim.", 0, 1, type); 3564 case 1977085293: /*adjustment*/ return new Property("adjustment", "Money", "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.", 0, 1, adjustment); 3565 case -1255938543: /*adjustmentReason*/ return new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason); 3566 case 3076014: /*date*/ return new Property("date", "date", "Estimated payment data.", 0, 1, date); 3567 case -1413853096: /*amount*/ return new Property("amount", "Money", "Payable less any payment adjustment.", 0, 1, amount); 3568 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Payment identifier.", 0, 1, identifier); 3569 default: return super.getNamedProperty(_hash, _name, _checkValid); 3570 } 3571 3572 } 3573 3574 @Override 3575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3576 switch (hash) { 3577 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3578 case 1977085293: /*adjustment*/ return this.adjustment == null ? new Base[0] : new Base[] {this.adjustment}; // Money 3579 case -1255938543: /*adjustmentReason*/ return this.adjustmentReason == null ? new Base[0] : new Base[] {this.adjustmentReason}; // CodeableConcept 3580 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3581 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 3582 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3583 default: return super.getProperty(hash, name, checkValid); 3584 } 3585 3586 } 3587 3588 @Override 3589 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3590 switch (hash) { 3591 case 3575610: // type 3592 this.type = castToCodeableConcept(value); // CodeableConcept 3593 return value; 3594 case 1977085293: // adjustment 3595 this.adjustment = castToMoney(value); // Money 3596 return value; 3597 case -1255938543: // adjustmentReason 3598 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 3599 return value; 3600 case 3076014: // date 3601 this.date = castToDate(value); // DateType 3602 return value; 3603 case -1413853096: // amount 3604 this.amount = castToMoney(value); // Money 3605 return value; 3606 case -1618432855: // identifier 3607 this.identifier = castToIdentifier(value); // Identifier 3608 return value; 3609 default: return super.setProperty(hash, name, value); 3610 } 3611 3612 } 3613 3614 @Override 3615 public Base setProperty(String name, Base value) throws FHIRException { 3616 if (name.equals("type")) { 3617 this.type = castToCodeableConcept(value); // CodeableConcept 3618 } else if (name.equals("adjustment")) { 3619 this.adjustment = castToMoney(value); // Money 3620 } else if (name.equals("adjustmentReason")) { 3621 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 3622 } else if (name.equals("date")) { 3623 this.date = castToDate(value); // DateType 3624 } else if (name.equals("amount")) { 3625 this.amount = castToMoney(value); // Money 3626 } else if (name.equals("identifier")) { 3627 this.identifier = castToIdentifier(value); // Identifier 3628 } else 3629 return super.setProperty(name, value); 3630 return value; 3631 } 3632 3633 @Override 3634 public Base makeProperty(int hash, String name) throws FHIRException { 3635 switch (hash) { 3636 case 3575610: return getType(); 3637 case 1977085293: return getAdjustment(); 3638 case -1255938543: return getAdjustmentReason(); 3639 case 3076014: return getDateElement(); 3640 case -1413853096: return getAmount(); 3641 case -1618432855: return getIdentifier(); 3642 default: return super.makeProperty(hash, name); 3643 } 3644 3645 } 3646 3647 @Override 3648 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3649 switch (hash) { 3650 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3651 case 1977085293: /*adjustment*/ return new String[] {"Money"}; 3652 case -1255938543: /*adjustmentReason*/ return new String[] {"CodeableConcept"}; 3653 case 3076014: /*date*/ return new String[] {"date"}; 3654 case -1413853096: /*amount*/ return new String[] {"Money"}; 3655 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3656 default: return super.getTypesForProperty(hash, name); 3657 } 3658 3659 } 3660 3661 @Override 3662 public Base addChild(String name) throws FHIRException { 3663 if (name.equals("type")) { 3664 this.type = new CodeableConcept(); 3665 return this.type; 3666 } 3667 else if (name.equals("adjustment")) { 3668 this.adjustment = new Money(); 3669 return this.adjustment; 3670 } 3671 else if (name.equals("adjustmentReason")) { 3672 this.adjustmentReason = new CodeableConcept(); 3673 return this.adjustmentReason; 3674 } 3675 else if (name.equals("date")) { 3676 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.date"); 3677 } 3678 else if (name.equals("amount")) { 3679 this.amount = new Money(); 3680 return this.amount; 3681 } 3682 else if (name.equals("identifier")) { 3683 this.identifier = new Identifier(); 3684 return this.identifier; 3685 } 3686 else 3687 return super.addChild(name); 3688 } 3689 3690 public PaymentComponent copy() { 3691 PaymentComponent dst = new PaymentComponent(); 3692 copyValues(dst); 3693 dst.type = type == null ? null : type.copy(); 3694 dst.adjustment = adjustment == null ? null : adjustment.copy(); 3695 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 3696 dst.date = date == null ? null : date.copy(); 3697 dst.amount = amount == null ? null : amount.copy(); 3698 dst.identifier = identifier == null ? null : identifier.copy(); 3699 return dst; 3700 } 3701 3702 @Override 3703 public boolean equalsDeep(Base other_) { 3704 if (!super.equalsDeep(other_)) 3705 return false; 3706 if (!(other_ instanceof PaymentComponent)) 3707 return false; 3708 PaymentComponent o = (PaymentComponent) other_; 3709 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) && compareDeep(adjustmentReason, o.adjustmentReason, true) 3710 && compareDeep(date, o.date, true) && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true) 3711 ; 3712 } 3713 3714 @Override 3715 public boolean equalsShallow(Base other_) { 3716 if (!super.equalsShallow(other_)) 3717 return false; 3718 if (!(other_ instanceof PaymentComponent)) 3719 return false; 3720 PaymentComponent o = (PaymentComponent) other_; 3721 return compareValues(date, o.date, true); 3722 } 3723 3724 public boolean isEmpty() { 3725 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason 3726 , date, amount, identifier); 3727 } 3728 3729 public String fhirType() { 3730 return "ClaimResponse.payment"; 3731 3732 } 3733 3734 } 3735 3736 @Block() 3737 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 3738 /** 3739 * An integer associated with each note which may be referred to from each service line item. 3740 */ 3741 @Child(name = "number", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3742 @Description(shortDefinition="Sequence Number for this note", formalDefinition="An integer associated with each note which may be referred to from each service line item." ) 3743 protected PositiveIntType number; 3744 3745 /** 3746 * The note purpose: Print/Display. 3747 */ 3748 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3749 @Description(shortDefinition="display | print | printoper", formalDefinition="The note purpose: Print/Display." ) 3750 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 3751 protected CodeableConcept type; 3752 3753 /** 3754 * The note text. 3755 */ 3756 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3757 @Description(shortDefinition="Note explanatory text", formalDefinition="The note text." ) 3758 protected StringType text; 3759 3760 /** 3761 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English. 3762 */ 3763 @Child(name = "language", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 3764 @Description(shortDefinition="Language if different from the resource", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English." ) 3765 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 3766 protected CodeableConcept language; 3767 3768 private static final long serialVersionUID = -944255449L; 3769 3770 /** 3771 * Constructor 3772 */ 3773 public NoteComponent() { 3774 super(); 3775 } 3776 3777 /** 3778 * @return {@link #number} (An integer associated with each note which may be referred to from each service line item.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 3779 */ 3780 public PositiveIntType getNumberElement() { 3781 if (this.number == null) 3782 if (Configuration.errorOnAutoCreate()) 3783 throw new Error("Attempt to auto-create NoteComponent.number"); 3784 else if (Configuration.doAutoCreate()) 3785 this.number = new PositiveIntType(); // bb 3786 return this.number; 3787 } 3788 3789 public boolean hasNumberElement() { 3790 return this.number != null && !this.number.isEmpty(); 3791 } 3792 3793 public boolean hasNumber() { 3794 return this.number != null && !this.number.isEmpty(); 3795 } 3796 3797 /** 3798 * @param value {@link #number} (An integer associated with each note which may be referred to from each service line item.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 3799 */ 3800 public NoteComponent setNumberElement(PositiveIntType value) { 3801 this.number = value; 3802 return this; 3803 } 3804 3805 /** 3806 * @return An integer associated with each note which may be referred to from each service line item. 3807 */ 3808 public int getNumber() { 3809 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 3810 } 3811 3812 /** 3813 * @param value An integer associated with each note which may be referred to from each service line item. 3814 */ 3815 public NoteComponent setNumber(int value) { 3816 if (this.number == null) 3817 this.number = new PositiveIntType(); 3818 this.number.setValue(value); 3819 return this; 3820 } 3821 3822 /** 3823 * @return {@link #type} (The note purpose: Print/Display.) 3824 */ 3825 public CodeableConcept getType() { 3826 if (this.type == null) 3827 if (Configuration.errorOnAutoCreate()) 3828 throw new Error("Attempt to auto-create NoteComponent.type"); 3829 else if (Configuration.doAutoCreate()) 3830 this.type = new CodeableConcept(); // cc 3831 return this.type; 3832 } 3833 3834 public boolean hasType() { 3835 return this.type != null && !this.type.isEmpty(); 3836 } 3837 3838 /** 3839 * @param value {@link #type} (The note purpose: Print/Display.) 3840 */ 3841 public NoteComponent setType(CodeableConcept value) { 3842 this.type = value; 3843 return this; 3844 } 3845 3846 /** 3847 * @return {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 3848 */ 3849 public StringType getTextElement() { 3850 if (this.text == null) 3851 if (Configuration.errorOnAutoCreate()) 3852 throw new Error("Attempt to auto-create NoteComponent.text"); 3853 else if (Configuration.doAutoCreate()) 3854 this.text = new StringType(); // bb 3855 return this.text; 3856 } 3857 3858 public boolean hasTextElement() { 3859 return this.text != null && !this.text.isEmpty(); 3860 } 3861 3862 public boolean hasText() { 3863 return this.text != null && !this.text.isEmpty(); 3864 } 3865 3866 /** 3867 * @param value {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 3868 */ 3869 public NoteComponent setTextElement(StringType value) { 3870 this.text = value; 3871 return this; 3872 } 3873 3874 /** 3875 * @return The note text. 3876 */ 3877 public String getText() { 3878 return this.text == null ? null : this.text.getValue(); 3879 } 3880 3881 /** 3882 * @param value The note text. 3883 */ 3884 public NoteComponent setText(String value) { 3885 if (Utilities.noString(value)) 3886 this.text = null; 3887 else { 3888 if (this.text == null) 3889 this.text = new StringType(); 3890 this.text.setValue(value); 3891 } 3892 return this; 3893 } 3894 3895 /** 3896 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 3897 */ 3898 public CodeableConcept getLanguage() { 3899 if (this.language == null) 3900 if (Configuration.errorOnAutoCreate()) 3901 throw new Error("Attempt to auto-create NoteComponent.language"); 3902 else if (Configuration.doAutoCreate()) 3903 this.language = new CodeableConcept(); // cc 3904 return this.language; 3905 } 3906 3907 public boolean hasLanguage() { 3908 return this.language != null && !this.language.isEmpty(); 3909 } 3910 3911 /** 3912 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 3913 */ 3914 public NoteComponent setLanguage(CodeableConcept value) { 3915 this.language = value; 3916 return this; 3917 } 3918 3919 protected void listChildren(List<Property> children) { 3920 super.listChildren(children); 3921 children.add(new Property("number", "positiveInt", "An integer associated with each note which may be referred to from each service line item.", 0, 1, number)); 3922 children.add(new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type)); 3923 children.add(new Property("text", "string", "The note text.", 0, 1, text)); 3924 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language)); 3925 } 3926 3927 @Override 3928 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3929 switch (_hash) { 3930 case -1034364087: /*number*/ return new Property("number", "positiveInt", "An integer associated with each note which may be referred to from each service line item.", 0, 1, number); 3931 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type); 3932 case 3556653: /*text*/ return new Property("text", "string", "The note text.", 0, 1, text); 3933 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language); 3934 default: return super.getNamedProperty(_hash, _name, _checkValid); 3935 } 3936 3937 } 3938 3939 @Override 3940 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3941 switch (hash) { 3942 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // PositiveIntType 3943 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3944 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 3945 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 3946 default: return super.getProperty(hash, name, checkValid); 3947 } 3948 3949 } 3950 3951 @Override 3952 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3953 switch (hash) { 3954 case -1034364087: // number 3955 this.number = castToPositiveInt(value); // PositiveIntType 3956 return value; 3957 case 3575610: // type 3958 this.type = castToCodeableConcept(value); // CodeableConcept 3959 return value; 3960 case 3556653: // text 3961 this.text = castToString(value); // StringType 3962 return value; 3963 case -1613589672: // language 3964 this.language = castToCodeableConcept(value); // CodeableConcept 3965 return value; 3966 default: return super.setProperty(hash, name, value); 3967 } 3968 3969 } 3970 3971 @Override 3972 public Base setProperty(String name, Base value) throws FHIRException { 3973 if (name.equals("number")) { 3974 this.number = castToPositiveInt(value); // PositiveIntType 3975 } else if (name.equals("type")) { 3976 this.type = castToCodeableConcept(value); // CodeableConcept 3977 } else if (name.equals("text")) { 3978 this.text = castToString(value); // StringType 3979 } else if (name.equals("language")) { 3980 this.language = castToCodeableConcept(value); // CodeableConcept 3981 } else 3982 return super.setProperty(name, value); 3983 return value; 3984 } 3985 3986 @Override 3987 public Base makeProperty(int hash, String name) throws FHIRException { 3988 switch (hash) { 3989 case -1034364087: return getNumberElement(); 3990 case 3575610: return getType(); 3991 case 3556653: return getTextElement(); 3992 case -1613589672: return getLanguage(); 3993 default: return super.makeProperty(hash, name); 3994 } 3995 3996 } 3997 3998 @Override 3999 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4000 switch (hash) { 4001 case -1034364087: /*number*/ return new String[] {"positiveInt"}; 4002 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4003 case 3556653: /*text*/ return new String[] {"string"}; 4004 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 4005 default: return super.getTypesForProperty(hash, name); 4006 } 4007 4008 } 4009 4010 @Override 4011 public Base addChild(String name) throws FHIRException { 4012 if (name.equals("number")) { 4013 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.number"); 4014 } 4015 else if (name.equals("type")) { 4016 this.type = new CodeableConcept(); 4017 return this.type; 4018 } 4019 else if (name.equals("text")) { 4020 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.text"); 4021 } 4022 else if (name.equals("language")) { 4023 this.language = new CodeableConcept(); 4024 return this.language; 4025 } 4026 else 4027 return super.addChild(name); 4028 } 4029 4030 public NoteComponent copy() { 4031 NoteComponent dst = new NoteComponent(); 4032 copyValues(dst); 4033 dst.number = number == null ? null : number.copy(); 4034 dst.type = type == null ? null : type.copy(); 4035 dst.text = text == null ? null : text.copy(); 4036 dst.language = language == null ? null : language.copy(); 4037 return dst; 4038 } 4039 4040 @Override 4041 public boolean equalsDeep(Base other_) { 4042 if (!super.equalsDeep(other_)) 4043 return false; 4044 if (!(other_ instanceof NoteComponent)) 4045 return false; 4046 NoteComponent o = (NoteComponent) other_; 4047 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 4048 && compareDeep(language, o.language, true); 4049 } 4050 4051 @Override 4052 public boolean equalsShallow(Base other_) { 4053 if (!super.equalsShallow(other_)) 4054 return false; 4055 if (!(other_ instanceof NoteComponent)) 4056 return false; 4057 NoteComponent o = (NoteComponent) other_; 4058 return compareValues(number, o.number, true) && compareValues(text, o.text, true); 4059 } 4060 4061 public boolean isEmpty() { 4062 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language 4063 ); 4064 } 4065 4066 public String fhirType() { 4067 return "ClaimResponse.processNote"; 4068 4069 } 4070 4071 } 4072 4073 @Block() 4074 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 4075 /** 4076 * A service line item. 4077 */ 4078 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4079 @Description(shortDefinition="Service instance identifier", formalDefinition="A service line item." ) 4080 protected PositiveIntType sequence; 4081 4082 /** 4083 * The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 4084 */ 4085 @Child(name = "focal", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 4086 @Description(shortDefinition="Is the focal Coverage", formalDefinition="The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated." ) 4087 protected BooleanType focal; 4088 4089 /** 4090 * Reference to the program or plan identification, underwriter or payor. 4091 */ 4092 @Child(name = "coverage", type = {Coverage.class}, order=3, min=1, max=1, modifier=false, summary=false) 4093 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the program or plan identification, underwriter or payor." ) 4094 protected Reference coverage; 4095 4096 /** 4097 * The actual object that is the target of the reference (Reference to the program or plan identification, underwriter or payor.) 4098 */ 4099 protected Coverage coverageTarget; 4100 4101 /** 4102 * The contract number of a business agreement which describes the terms and conditions. 4103 */ 4104 @Child(name = "businessArrangement", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4105 @Description(shortDefinition="Business agreement", formalDefinition="The contract number of a business agreement which describes the terms and conditions." ) 4106 protected StringType businessArrangement; 4107 4108 /** 4109 * A list of references from the Insurer to which these services pertain. 4110 */ 4111 @Child(name = "preAuthRef", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4112 @Description(shortDefinition="Pre-Authorization/Determination Reference", formalDefinition="A list of references from the Insurer to which these services pertain." ) 4113 protected List<StringType> preAuthRef; 4114 4115 /** 4116 * The Coverages adjudication details. 4117 */ 4118 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=6, min=0, max=1, modifier=false, summary=false) 4119 @Description(shortDefinition="Adjudication results", formalDefinition="The Coverages adjudication details." ) 4120 protected Reference claimResponse; 4121 4122 /** 4123 * The actual object that is the target of the reference (The Coverages adjudication details.) 4124 */ 4125 protected ClaimResponse claimResponseTarget; 4126 4127 private static final long serialVersionUID = -1216535489L; 4128 4129 /** 4130 * Constructor 4131 */ 4132 public InsuranceComponent() { 4133 super(); 4134 } 4135 4136 /** 4137 * Constructor 4138 */ 4139 public InsuranceComponent(PositiveIntType sequence, BooleanType focal, Reference coverage) { 4140 super(); 4141 this.sequence = sequence; 4142 this.focal = focal; 4143 this.coverage = coverage; 4144 } 4145 4146 /** 4147 * @return {@link #sequence} (A service line item.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 4148 */ 4149 public PositiveIntType getSequenceElement() { 4150 if (this.sequence == null) 4151 if (Configuration.errorOnAutoCreate()) 4152 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 4153 else if (Configuration.doAutoCreate()) 4154 this.sequence = new PositiveIntType(); // bb 4155 return this.sequence; 4156 } 4157 4158 public boolean hasSequenceElement() { 4159 return this.sequence != null && !this.sequence.isEmpty(); 4160 } 4161 4162 public boolean hasSequence() { 4163 return this.sequence != null && !this.sequence.isEmpty(); 4164 } 4165 4166 /** 4167 * @param value {@link #sequence} (A service line item.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 4168 */ 4169 public InsuranceComponent setSequenceElement(PositiveIntType value) { 4170 this.sequence = value; 4171 return this; 4172 } 4173 4174 /** 4175 * @return A service line item. 4176 */ 4177 public int getSequence() { 4178 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 4179 } 4180 4181 /** 4182 * @param value A service line item. 4183 */ 4184 public InsuranceComponent setSequence(int value) { 4185 if (this.sequence == null) 4186 this.sequence = new PositiveIntType(); 4187 this.sequence.setValue(value); 4188 return this; 4189 } 4190 4191 /** 4192 * @return {@link #focal} (The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 4193 */ 4194 public BooleanType getFocalElement() { 4195 if (this.focal == null) 4196 if (Configuration.errorOnAutoCreate()) 4197 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 4198 else if (Configuration.doAutoCreate()) 4199 this.focal = new BooleanType(); // bb 4200 return this.focal; 4201 } 4202 4203 public boolean hasFocalElement() { 4204 return this.focal != null && !this.focal.isEmpty(); 4205 } 4206 4207 public boolean hasFocal() { 4208 return this.focal != null && !this.focal.isEmpty(); 4209 } 4210 4211 /** 4212 * @param value {@link #focal} (The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 4213 */ 4214 public InsuranceComponent setFocalElement(BooleanType value) { 4215 this.focal = value; 4216 return this; 4217 } 4218 4219 /** 4220 * @return The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 4221 */ 4222 public boolean getFocal() { 4223 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 4224 } 4225 4226 /** 4227 * @param value The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated. 4228 */ 4229 public InsuranceComponent setFocal(boolean value) { 4230 if (this.focal == null) 4231 this.focal = new BooleanType(); 4232 this.focal.setValue(value); 4233 return this; 4234 } 4235 4236 /** 4237 * @return {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 4238 */ 4239 public Reference getCoverage() { 4240 if (this.coverage == null) 4241 if (Configuration.errorOnAutoCreate()) 4242 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 4243 else if (Configuration.doAutoCreate()) 4244 this.coverage = new Reference(); // cc 4245 return this.coverage; 4246 } 4247 4248 public boolean hasCoverage() { 4249 return this.coverage != null && !this.coverage.isEmpty(); 4250 } 4251 4252 /** 4253 * @param value {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 4254 */ 4255 public InsuranceComponent setCoverage(Reference value) { 4256 this.coverage = value; 4257 return this; 4258 } 4259 4260 /** 4261 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 4262 */ 4263 public Coverage getCoverageTarget() { 4264 if (this.coverageTarget == null) 4265 if (Configuration.errorOnAutoCreate()) 4266 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 4267 else if (Configuration.doAutoCreate()) 4268 this.coverageTarget = new Coverage(); // aa 4269 return this.coverageTarget; 4270 } 4271 4272 /** 4273 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 4274 */ 4275 public InsuranceComponent setCoverageTarget(Coverage value) { 4276 this.coverageTarget = value; 4277 return this; 4278 } 4279 4280 /** 4281 * @return {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 4282 */ 4283 public StringType getBusinessArrangementElement() { 4284 if (this.businessArrangement == null) 4285 if (Configuration.errorOnAutoCreate()) 4286 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 4287 else if (Configuration.doAutoCreate()) 4288 this.businessArrangement = new StringType(); // bb 4289 return this.businessArrangement; 4290 } 4291 4292 public boolean hasBusinessArrangementElement() { 4293 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 4294 } 4295 4296 public boolean hasBusinessArrangement() { 4297 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 4298 } 4299 4300 /** 4301 * @param value {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 4302 */ 4303 public InsuranceComponent setBusinessArrangementElement(StringType value) { 4304 this.businessArrangement = value; 4305 return this; 4306 } 4307 4308 /** 4309 * @return The contract number of a business agreement which describes the terms and conditions. 4310 */ 4311 public String getBusinessArrangement() { 4312 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 4313 } 4314 4315 /** 4316 * @param value The contract number of a business agreement which describes the terms and conditions. 4317 */ 4318 public InsuranceComponent setBusinessArrangement(String value) { 4319 if (Utilities.noString(value)) 4320 this.businessArrangement = null; 4321 else { 4322 if (this.businessArrangement == null) 4323 this.businessArrangement = new StringType(); 4324 this.businessArrangement.setValue(value); 4325 } 4326 return this; 4327 } 4328 4329 /** 4330 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 4331 */ 4332 public List<StringType> getPreAuthRef() { 4333 if (this.preAuthRef == null) 4334 this.preAuthRef = new ArrayList<StringType>(); 4335 return this.preAuthRef; 4336 } 4337 4338 /** 4339 * @return Returns a reference to <code>this</code> for easy method chaining 4340 */ 4341 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 4342 this.preAuthRef = thePreAuthRef; 4343 return this; 4344 } 4345 4346 public boolean hasPreAuthRef() { 4347 if (this.preAuthRef == null) 4348 return false; 4349 for (StringType item : this.preAuthRef) 4350 if (!item.isEmpty()) 4351 return true; 4352 return false; 4353 } 4354 4355 /** 4356 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 4357 */ 4358 public StringType addPreAuthRefElement() {//2 4359 StringType t = new StringType(); 4360 if (this.preAuthRef == null) 4361 this.preAuthRef = new ArrayList<StringType>(); 4362 this.preAuthRef.add(t); 4363 return t; 4364 } 4365 4366 /** 4367 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 4368 */ 4369 public InsuranceComponent addPreAuthRef(String value) { //1 4370 StringType t = new StringType(); 4371 t.setValue(value); 4372 if (this.preAuthRef == null) 4373 this.preAuthRef = new ArrayList<StringType>(); 4374 this.preAuthRef.add(t); 4375 return this; 4376 } 4377 4378 /** 4379 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 4380 */ 4381 public boolean hasPreAuthRef(String value) { 4382 if (this.preAuthRef == null) 4383 return false; 4384 for (StringType v : this.preAuthRef) 4385 if (v.getValue().equals(value)) // string 4386 return true; 4387 return false; 4388 } 4389 4390 /** 4391 * @return {@link #claimResponse} (The Coverages adjudication details.) 4392 */ 4393 public Reference getClaimResponse() { 4394 if (this.claimResponse == null) 4395 if (Configuration.errorOnAutoCreate()) 4396 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 4397 else if (Configuration.doAutoCreate()) 4398 this.claimResponse = new Reference(); // cc 4399 return this.claimResponse; 4400 } 4401 4402 public boolean hasClaimResponse() { 4403 return this.claimResponse != null && !this.claimResponse.isEmpty(); 4404 } 4405 4406 /** 4407 * @param value {@link #claimResponse} (The Coverages adjudication details.) 4408 */ 4409 public InsuranceComponent setClaimResponse(Reference value) { 4410 this.claimResponse = value; 4411 return this; 4412 } 4413 4414 /** 4415 * @return {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Coverages adjudication details.) 4416 */ 4417 public ClaimResponse getClaimResponseTarget() { 4418 if (this.claimResponseTarget == null) 4419 if (Configuration.errorOnAutoCreate()) 4420 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 4421 else if (Configuration.doAutoCreate()) 4422 this.claimResponseTarget = new ClaimResponse(); // aa 4423 return this.claimResponseTarget; 4424 } 4425 4426 /** 4427 * @param value {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Coverages adjudication details.) 4428 */ 4429 public InsuranceComponent setClaimResponseTarget(ClaimResponse value) { 4430 this.claimResponseTarget = value; 4431 return this; 4432 } 4433 4434 protected void listChildren(List<Property> children) { 4435 super.listChildren(children); 4436 children.add(new Property("sequence", "positiveInt", "A service line item.", 0, 1, sequence)); 4437 children.add(new Property("focal", "boolean", "The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 0, 1, focal)); 4438 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 4439 children.add(new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement)); 4440 children.add(new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 4441 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 0, 1, claimResponse)); 4442 } 4443 4444 @Override 4445 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4446 switch (_hash) { 4447 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line item.", 0, 1, sequence); 4448 case 97604197: /*focal*/ return new Property("focal", "boolean", "The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 0, 1, focal); 4449 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 4450 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement); 4451 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 4452 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 0, 1, claimResponse); 4453 default: return super.getNamedProperty(_hash, _name, _checkValid); 4454 } 4455 4456 } 4457 4458 @Override 4459 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4460 switch (hash) { 4461 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 4462 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 4463 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 4464 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 4465 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 4466 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 4467 default: return super.getProperty(hash, name, checkValid); 4468 } 4469 4470 } 4471 4472 @Override 4473 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4474 switch (hash) { 4475 case 1349547969: // sequence 4476 this.sequence = castToPositiveInt(value); // PositiveIntType 4477 return value; 4478 case 97604197: // focal 4479 this.focal = castToBoolean(value); // BooleanType 4480 return value; 4481 case -351767064: // coverage 4482 this.coverage = castToReference(value); // Reference 4483 return value; 4484 case 259920682: // businessArrangement 4485 this.businessArrangement = castToString(value); // StringType 4486 return value; 4487 case 522246568: // preAuthRef 4488 this.getPreAuthRef().add(castToString(value)); // StringType 4489 return value; 4490 case 689513629: // claimResponse 4491 this.claimResponse = castToReference(value); // Reference 4492 return value; 4493 default: return super.setProperty(hash, name, value); 4494 } 4495 4496 } 4497 4498 @Override 4499 public Base setProperty(String name, Base value) throws FHIRException { 4500 if (name.equals("sequence")) { 4501 this.sequence = castToPositiveInt(value); // PositiveIntType 4502 } else if (name.equals("focal")) { 4503 this.focal = castToBoolean(value); // BooleanType 4504 } else if (name.equals("coverage")) { 4505 this.coverage = castToReference(value); // Reference 4506 } else if (name.equals("businessArrangement")) { 4507 this.businessArrangement = castToString(value); // StringType 4508 } else if (name.equals("preAuthRef")) { 4509 this.getPreAuthRef().add(castToString(value)); 4510 } else if (name.equals("claimResponse")) { 4511 this.claimResponse = castToReference(value); // Reference 4512 } else 4513 return super.setProperty(name, value); 4514 return value; 4515 } 4516 4517 @Override 4518 public Base makeProperty(int hash, String name) throws FHIRException { 4519 switch (hash) { 4520 case 1349547969: return getSequenceElement(); 4521 case 97604197: return getFocalElement(); 4522 case -351767064: return getCoverage(); 4523 case 259920682: return getBusinessArrangementElement(); 4524 case 522246568: return addPreAuthRefElement(); 4525 case 689513629: return getClaimResponse(); 4526 default: return super.makeProperty(hash, name); 4527 } 4528 4529 } 4530 4531 @Override 4532 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4533 switch (hash) { 4534 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 4535 case 97604197: /*focal*/ return new String[] {"boolean"}; 4536 case -351767064: /*coverage*/ return new String[] {"Reference"}; 4537 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 4538 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 4539 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 4540 default: return super.getTypesForProperty(hash, name); 4541 } 4542 4543 } 4544 4545 @Override 4546 public Base addChild(String name) throws FHIRException { 4547 if (name.equals("sequence")) { 4548 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequence"); 4549 } 4550 else if (name.equals("focal")) { 4551 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.focal"); 4552 } 4553 else if (name.equals("coverage")) { 4554 this.coverage = new Reference(); 4555 return this.coverage; 4556 } 4557 else if (name.equals("businessArrangement")) { 4558 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.businessArrangement"); 4559 } 4560 else if (name.equals("preAuthRef")) { 4561 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.preAuthRef"); 4562 } 4563 else if (name.equals("claimResponse")) { 4564 this.claimResponse = new Reference(); 4565 return this.claimResponse; 4566 } 4567 else 4568 return super.addChild(name); 4569 } 4570 4571 public InsuranceComponent copy() { 4572 InsuranceComponent dst = new InsuranceComponent(); 4573 copyValues(dst); 4574 dst.sequence = sequence == null ? null : sequence.copy(); 4575 dst.focal = focal == null ? null : focal.copy(); 4576 dst.coverage = coverage == null ? null : coverage.copy(); 4577 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 4578 if (preAuthRef != null) { 4579 dst.preAuthRef = new ArrayList<StringType>(); 4580 for (StringType i : preAuthRef) 4581 dst.preAuthRef.add(i.copy()); 4582 }; 4583 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 4584 return dst; 4585 } 4586 4587 @Override 4588 public boolean equalsDeep(Base other_) { 4589 if (!super.equalsDeep(other_)) 4590 return false; 4591 if (!(other_ instanceof InsuranceComponent)) 4592 return false; 4593 InsuranceComponent o = (InsuranceComponent) other_; 4594 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) 4595 && compareDeep(businessArrangement, o.businessArrangement, true) && compareDeep(preAuthRef, o.preAuthRef, true) 4596 && compareDeep(claimResponse, o.claimResponse, true); 4597 } 4598 4599 @Override 4600 public boolean equalsShallow(Base other_) { 4601 if (!super.equalsShallow(other_)) 4602 return false; 4603 if (!(other_ instanceof InsuranceComponent)) 4604 return false; 4605 InsuranceComponent o = (InsuranceComponent) other_; 4606 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true) 4607 && compareValues(preAuthRef, o.preAuthRef, true); 4608 } 4609 4610 public boolean isEmpty() { 4611 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, coverage 4612 , businessArrangement, preAuthRef, claimResponse); 4613 } 4614 4615 public String fhirType() { 4616 return "ClaimResponse.insurance"; 4617 4618 } 4619 4620 } 4621 4622 /** 4623 * The Response business identifier. 4624 */ 4625 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4626 @Description(shortDefinition="Response number", formalDefinition="The Response business identifier." ) 4627 protected List<Identifier> identifier; 4628 4629 /** 4630 * The status of the resource instance. 4631 */ 4632 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 4633 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 4634 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 4635 protected Enumeration<ClaimResponseStatus> status; 4636 4637 /** 4638 * Patient Resource. 4639 */ 4640 @Child(name = "patient", type = {Patient.class}, order=2, min=0, max=1, modifier=false, summary=false) 4641 @Description(shortDefinition="The subject of the Products and Services", formalDefinition="Patient Resource." ) 4642 protected Reference patient; 4643 4644 /** 4645 * The actual object that is the target of the reference (Patient Resource.) 4646 */ 4647 protected Patient patientTarget; 4648 4649 /** 4650 * The date when the enclosed suite of services were performed or completed. 4651 */ 4652 @Child(name = "created", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4653 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 4654 protected DateTimeType created; 4655 4656 /** 4657 * The Insurer who produced this adjudicated response. 4658 */ 4659 @Child(name = "insurer", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 4660 @Description(shortDefinition="Insurance issuing organization", formalDefinition="The Insurer who produced this adjudicated response." ) 4661 protected Reference insurer; 4662 4663 /** 4664 * The actual object that is the target of the reference (The Insurer who produced this adjudicated response.) 4665 */ 4666 protected Organization insurerTarget; 4667 4668 /** 4669 * The practitioner who is responsible for the services rendered to the patient. 4670 */ 4671 @Child(name = "requestProvider", type = {Practitioner.class}, order=5, min=0, max=1, modifier=false, summary=false) 4672 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 4673 protected Reference requestProvider; 4674 4675 /** 4676 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 4677 */ 4678 protected Practitioner requestProviderTarget; 4679 4680 /** 4681 * The organization which is responsible for the services rendered to the patient. 4682 */ 4683 @Child(name = "requestOrganization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 4684 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 4685 protected Reference requestOrganization; 4686 4687 /** 4688 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 4689 */ 4690 protected Organization requestOrganizationTarget; 4691 4692 /** 4693 * Original request resource referrence. 4694 */ 4695 @Child(name = "request", type = {Claim.class}, order=7, min=0, max=1, modifier=false, summary=false) 4696 @Description(shortDefinition="Id of resource triggering adjudication", formalDefinition="Original request resource referrence." ) 4697 protected Reference request; 4698 4699 /** 4700 * The actual object that is the target of the reference (Original request resource referrence.) 4701 */ 4702 protected Claim requestTarget; 4703 4704 /** 4705 * Processing outcome errror, partial or complete processing. 4706 */ 4707 @Child(name = "outcome", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 4708 @Description(shortDefinition="complete | error | partial", formalDefinition="Processing outcome errror, partial or complete processing." ) 4709 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/remittance-outcome") 4710 protected CodeableConcept outcome; 4711 4712 /** 4713 * A description of the status of the adjudication. 4714 */ 4715 @Child(name = "disposition", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 4716 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication." ) 4717 protected StringType disposition; 4718 4719 /** 4720 * Party to be reimbursed: Subscriber, provider, other. 4721 */ 4722 @Child(name = "payeeType", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 4723 @Description(shortDefinition="Party to be paid any benefits payable", formalDefinition="Party to be reimbursed: Subscriber, provider, other." ) 4724 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 4725 protected CodeableConcept payeeType; 4726 4727 /** 4728 * The first tier service adjudications for submitted services. 4729 */ 4730 @Child(name = "item", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4731 @Description(shortDefinition="Line items", formalDefinition="The first tier service adjudications for submitted services." ) 4732 protected List<ItemComponent> item; 4733 4734 /** 4735 * The first tier service adjudications for payor added services. 4736 */ 4737 @Child(name = "addItem", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4738 @Description(shortDefinition="Insurer added line items", formalDefinition="The first tier service adjudications for payor added services." ) 4739 protected List<AddedItemComponent> addItem; 4740 4741 /** 4742 * Mutually exclusive with Services Provided (Item). 4743 */ 4744 @Child(name = "error", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4745 @Description(shortDefinition="Processing errors", formalDefinition="Mutually exclusive with Services Provided (Item)." ) 4746 protected List<ErrorComponent> error; 4747 4748 /** 4749 * The total cost of the services reported. 4750 */ 4751 @Child(name = "totalCost", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 4752 @Description(shortDefinition="Total Cost of service from the Claim", formalDefinition="The total cost of the services reported." ) 4753 protected Money totalCost; 4754 4755 /** 4756 * The amount of deductible applied which was not allocated to any particular service line. 4757 */ 4758 @Child(name = "unallocDeductable", type = {Money.class}, order=15, min=0, max=1, modifier=false, summary=false) 4759 @Description(shortDefinition="Unallocated deductible", formalDefinition="The amount of deductible applied which was not allocated to any particular service line." ) 4760 protected Money unallocDeductable; 4761 4762 /** 4763 * Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible). 4764 */ 4765 @Child(name = "totalBenefit", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 4766 @Description(shortDefinition="Total benefit payable for the Claim", formalDefinition="Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible)." ) 4767 protected Money totalBenefit; 4768 4769 /** 4770 * Payment details for the claim if the claim has been paid. 4771 */ 4772 @Child(name = "payment", type = {}, order=17, min=0, max=1, modifier=false, summary=false) 4773 @Description(shortDefinition="Payment details, if paid", formalDefinition="Payment details for the claim if the claim has been paid." ) 4774 protected PaymentComponent payment; 4775 4776 /** 4777 * Status of funds reservation (For provider, for Patient, None). 4778 */ 4779 @Child(name = "reserved", type = {Coding.class}, order=18, min=0, max=1, modifier=false, summary=false) 4780 @Description(shortDefinition="Funds reserved status", formalDefinition="Status of funds reservation (For provider, for Patient, None)." ) 4781 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 4782 protected Coding reserved; 4783 4784 /** 4785 * The form to be used for printing the content. 4786 */ 4787 @Child(name = "form", type = {CodeableConcept.class}, order=19, min=0, max=1, modifier=false, summary=false) 4788 @Description(shortDefinition="Printed Form Identifier", formalDefinition="The form to be used for printing the content." ) 4789 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 4790 protected CodeableConcept form; 4791 4792 /** 4793 * Note text. 4794 */ 4795 @Child(name = "processNote", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4796 @Description(shortDefinition="Processing notes", formalDefinition="Note text." ) 4797 protected List<NoteComponent> processNote; 4798 4799 /** 4800 * Request for additional supporting or authorizing information, such as: documents, images or resources. 4801 */ 4802 @Child(name = "communicationRequest", type = {CommunicationRequest.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4803 @Description(shortDefinition="Request for additional information", formalDefinition="Request for additional supporting or authorizing information, such as: documents, images or resources." ) 4804 protected List<Reference> communicationRequest; 4805 /** 4806 * The actual objects that are the target of the reference (Request for additional supporting or authorizing information, such as: documents, images or resources.) 4807 */ 4808 protected List<CommunicationRequest> communicationRequestTarget; 4809 4810 4811 /** 4812 * Financial instrument by which payment information for health care. 4813 */ 4814 @Child(name = "insurance", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4815 @Description(shortDefinition="Insurance or medical plan", formalDefinition="Financial instrument by which payment information for health care." ) 4816 protected List<InsuranceComponent> insurance; 4817 4818 private static final long serialVersionUID = -488591755L; 4819 4820 /** 4821 * Constructor 4822 */ 4823 public ClaimResponse() { 4824 super(); 4825 } 4826 4827 /** 4828 * @return {@link #identifier} (The Response business identifier.) 4829 */ 4830 public List<Identifier> getIdentifier() { 4831 if (this.identifier == null) 4832 this.identifier = new ArrayList<Identifier>(); 4833 return this.identifier; 4834 } 4835 4836 /** 4837 * @return Returns a reference to <code>this</code> for easy method chaining 4838 */ 4839 public ClaimResponse setIdentifier(List<Identifier> theIdentifier) { 4840 this.identifier = theIdentifier; 4841 return this; 4842 } 4843 4844 public boolean hasIdentifier() { 4845 if (this.identifier == null) 4846 return false; 4847 for (Identifier item : this.identifier) 4848 if (!item.isEmpty()) 4849 return true; 4850 return false; 4851 } 4852 4853 public Identifier addIdentifier() { //3 4854 Identifier t = new Identifier(); 4855 if (this.identifier == null) 4856 this.identifier = new ArrayList<Identifier>(); 4857 this.identifier.add(t); 4858 return t; 4859 } 4860 4861 public ClaimResponse addIdentifier(Identifier t) { //3 4862 if (t == null) 4863 return this; 4864 if (this.identifier == null) 4865 this.identifier = new ArrayList<Identifier>(); 4866 this.identifier.add(t); 4867 return this; 4868 } 4869 4870 /** 4871 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 4872 */ 4873 public Identifier getIdentifierFirstRep() { 4874 if (getIdentifier().isEmpty()) { 4875 addIdentifier(); 4876 } 4877 return getIdentifier().get(0); 4878 } 4879 4880 /** 4881 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4882 */ 4883 public Enumeration<ClaimResponseStatus> getStatusElement() { 4884 if (this.status == null) 4885 if (Configuration.errorOnAutoCreate()) 4886 throw new Error("Attempt to auto-create ClaimResponse.status"); 4887 else if (Configuration.doAutoCreate()) 4888 this.status = new Enumeration<ClaimResponseStatus>(new ClaimResponseStatusEnumFactory()); // bb 4889 return this.status; 4890 } 4891 4892 public boolean hasStatusElement() { 4893 return this.status != null && !this.status.isEmpty(); 4894 } 4895 4896 public boolean hasStatus() { 4897 return this.status != null && !this.status.isEmpty(); 4898 } 4899 4900 /** 4901 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4902 */ 4903 public ClaimResponse setStatusElement(Enumeration<ClaimResponseStatus> value) { 4904 this.status = value; 4905 return this; 4906 } 4907 4908 /** 4909 * @return The status of the resource instance. 4910 */ 4911 public ClaimResponseStatus getStatus() { 4912 return this.status == null ? null : this.status.getValue(); 4913 } 4914 4915 /** 4916 * @param value The status of the resource instance. 4917 */ 4918 public ClaimResponse setStatus(ClaimResponseStatus value) { 4919 if (value == null) 4920 this.status = null; 4921 else { 4922 if (this.status == null) 4923 this.status = new Enumeration<ClaimResponseStatus>(new ClaimResponseStatusEnumFactory()); 4924 this.status.setValue(value); 4925 } 4926 return this; 4927 } 4928 4929 /** 4930 * @return {@link #patient} (Patient Resource.) 4931 */ 4932 public Reference getPatient() { 4933 if (this.patient == null) 4934 if (Configuration.errorOnAutoCreate()) 4935 throw new Error("Attempt to auto-create ClaimResponse.patient"); 4936 else if (Configuration.doAutoCreate()) 4937 this.patient = new Reference(); // cc 4938 return this.patient; 4939 } 4940 4941 public boolean hasPatient() { 4942 return this.patient != null && !this.patient.isEmpty(); 4943 } 4944 4945 /** 4946 * @param value {@link #patient} (Patient Resource.) 4947 */ 4948 public ClaimResponse setPatient(Reference value) { 4949 this.patient = value; 4950 return this; 4951 } 4952 4953 /** 4954 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient Resource.) 4955 */ 4956 public Patient getPatientTarget() { 4957 if (this.patientTarget == null) 4958 if (Configuration.errorOnAutoCreate()) 4959 throw new Error("Attempt to auto-create ClaimResponse.patient"); 4960 else if (Configuration.doAutoCreate()) 4961 this.patientTarget = new Patient(); // aa 4962 return this.patientTarget; 4963 } 4964 4965 /** 4966 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient Resource.) 4967 */ 4968 public ClaimResponse setPatientTarget(Patient value) { 4969 this.patientTarget = value; 4970 return this; 4971 } 4972 4973 /** 4974 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 4975 */ 4976 public DateTimeType getCreatedElement() { 4977 if (this.created == null) 4978 if (Configuration.errorOnAutoCreate()) 4979 throw new Error("Attempt to auto-create ClaimResponse.created"); 4980 else if (Configuration.doAutoCreate()) 4981 this.created = new DateTimeType(); // bb 4982 return this.created; 4983 } 4984 4985 public boolean hasCreatedElement() { 4986 return this.created != null && !this.created.isEmpty(); 4987 } 4988 4989 public boolean hasCreated() { 4990 return this.created != null && !this.created.isEmpty(); 4991 } 4992 4993 /** 4994 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 4995 */ 4996 public ClaimResponse setCreatedElement(DateTimeType value) { 4997 this.created = value; 4998 return this; 4999 } 5000 5001 /** 5002 * @return The date when the enclosed suite of services were performed or completed. 5003 */ 5004 public Date getCreated() { 5005 return this.created == null ? null : this.created.getValue(); 5006 } 5007 5008 /** 5009 * @param value The date when the enclosed suite of services were performed or completed. 5010 */ 5011 public ClaimResponse setCreated(Date value) { 5012 if (value == null) 5013 this.created = null; 5014 else { 5015 if (this.created == null) 5016 this.created = new DateTimeType(); 5017 this.created.setValue(value); 5018 } 5019 return this; 5020 } 5021 5022 /** 5023 * @return {@link #insurer} (The Insurer who produced this adjudicated response.) 5024 */ 5025 public Reference getInsurer() { 5026 if (this.insurer == null) 5027 if (Configuration.errorOnAutoCreate()) 5028 throw new Error("Attempt to auto-create ClaimResponse.insurer"); 5029 else if (Configuration.doAutoCreate()) 5030 this.insurer = new Reference(); // cc 5031 return this.insurer; 5032 } 5033 5034 public boolean hasInsurer() { 5035 return this.insurer != null && !this.insurer.isEmpty(); 5036 } 5037 5038 /** 5039 * @param value {@link #insurer} (The Insurer who produced this adjudicated response.) 5040 */ 5041 public ClaimResponse setInsurer(Reference value) { 5042 this.insurer = value; 5043 return this; 5044 } 5045 5046 /** 5047 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 5048 */ 5049 public Organization getInsurerTarget() { 5050 if (this.insurerTarget == null) 5051 if (Configuration.errorOnAutoCreate()) 5052 throw new Error("Attempt to auto-create ClaimResponse.insurer"); 5053 else if (Configuration.doAutoCreate()) 5054 this.insurerTarget = new Organization(); // aa 5055 return this.insurerTarget; 5056 } 5057 5058 /** 5059 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 5060 */ 5061 public ClaimResponse setInsurerTarget(Organization value) { 5062 this.insurerTarget = value; 5063 return this; 5064 } 5065 5066 /** 5067 * @return {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 5068 */ 5069 public Reference getRequestProvider() { 5070 if (this.requestProvider == null) 5071 if (Configuration.errorOnAutoCreate()) 5072 throw new Error("Attempt to auto-create ClaimResponse.requestProvider"); 5073 else if (Configuration.doAutoCreate()) 5074 this.requestProvider = new Reference(); // cc 5075 return this.requestProvider; 5076 } 5077 5078 public boolean hasRequestProvider() { 5079 return this.requestProvider != null && !this.requestProvider.isEmpty(); 5080 } 5081 5082 /** 5083 * @param value {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 5084 */ 5085 public ClaimResponse setRequestProvider(Reference value) { 5086 this.requestProvider = value; 5087 return this; 5088 } 5089 5090 /** 5091 * @return {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 5092 */ 5093 public Practitioner getRequestProviderTarget() { 5094 if (this.requestProviderTarget == null) 5095 if (Configuration.errorOnAutoCreate()) 5096 throw new Error("Attempt to auto-create ClaimResponse.requestProvider"); 5097 else if (Configuration.doAutoCreate()) 5098 this.requestProviderTarget = new Practitioner(); // aa 5099 return this.requestProviderTarget; 5100 } 5101 5102 /** 5103 * @param value {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 5104 */ 5105 public ClaimResponse setRequestProviderTarget(Practitioner value) { 5106 this.requestProviderTarget = value; 5107 return this; 5108 } 5109 5110 /** 5111 * @return {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 5112 */ 5113 public Reference getRequestOrganization() { 5114 if (this.requestOrganization == null) 5115 if (Configuration.errorOnAutoCreate()) 5116 throw new Error("Attempt to auto-create ClaimResponse.requestOrganization"); 5117 else if (Configuration.doAutoCreate()) 5118 this.requestOrganization = new Reference(); // cc 5119 return this.requestOrganization; 5120 } 5121 5122 public boolean hasRequestOrganization() { 5123 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 5124 } 5125 5126 /** 5127 * @param value {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 5128 */ 5129 public ClaimResponse setRequestOrganization(Reference value) { 5130 this.requestOrganization = value; 5131 return this; 5132 } 5133 5134 /** 5135 * @return {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 5136 */ 5137 public Organization getRequestOrganizationTarget() { 5138 if (this.requestOrganizationTarget == null) 5139 if (Configuration.errorOnAutoCreate()) 5140 throw new Error("Attempt to auto-create ClaimResponse.requestOrganization"); 5141 else if (Configuration.doAutoCreate()) 5142 this.requestOrganizationTarget = new Organization(); // aa 5143 return this.requestOrganizationTarget; 5144 } 5145 5146 /** 5147 * @param value {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 5148 */ 5149 public ClaimResponse setRequestOrganizationTarget(Organization value) { 5150 this.requestOrganizationTarget = value; 5151 return this; 5152 } 5153 5154 /** 5155 * @return {@link #request} (Original request resource referrence.) 5156 */ 5157 public Reference getRequest() { 5158 if (this.request == null) 5159 if (Configuration.errorOnAutoCreate()) 5160 throw new Error("Attempt to auto-create ClaimResponse.request"); 5161 else if (Configuration.doAutoCreate()) 5162 this.request = new Reference(); // cc 5163 return this.request; 5164 } 5165 5166 public boolean hasRequest() { 5167 return this.request != null && !this.request.isEmpty(); 5168 } 5169 5170 /** 5171 * @param value {@link #request} (Original request resource referrence.) 5172 */ 5173 public ClaimResponse setRequest(Reference value) { 5174 this.request = value; 5175 return this; 5176 } 5177 5178 /** 5179 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original request resource referrence.) 5180 */ 5181 public Claim getRequestTarget() { 5182 if (this.requestTarget == null) 5183 if (Configuration.errorOnAutoCreate()) 5184 throw new Error("Attempt to auto-create ClaimResponse.request"); 5185 else if (Configuration.doAutoCreate()) 5186 this.requestTarget = new Claim(); // aa 5187 return this.requestTarget; 5188 } 5189 5190 /** 5191 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original request resource referrence.) 5192 */ 5193 public ClaimResponse setRequestTarget(Claim value) { 5194 this.requestTarget = value; 5195 return this; 5196 } 5197 5198 /** 5199 * @return {@link #outcome} (Processing outcome errror, partial or complete processing.) 5200 */ 5201 public CodeableConcept getOutcome() { 5202 if (this.outcome == null) 5203 if (Configuration.errorOnAutoCreate()) 5204 throw new Error("Attempt to auto-create ClaimResponse.outcome"); 5205 else if (Configuration.doAutoCreate()) 5206 this.outcome = new CodeableConcept(); // cc 5207 return this.outcome; 5208 } 5209 5210 public boolean hasOutcome() { 5211 return this.outcome != null && !this.outcome.isEmpty(); 5212 } 5213 5214 /** 5215 * @param value {@link #outcome} (Processing outcome errror, partial or complete processing.) 5216 */ 5217 public ClaimResponse setOutcome(CodeableConcept value) { 5218 this.outcome = value; 5219 return this; 5220 } 5221 5222 /** 5223 * @return {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 5224 */ 5225 public StringType getDispositionElement() { 5226 if (this.disposition == null) 5227 if (Configuration.errorOnAutoCreate()) 5228 throw new Error("Attempt to auto-create ClaimResponse.disposition"); 5229 else if (Configuration.doAutoCreate()) 5230 this.disposition = new StringType(); // bb 5231 return this.disposition; 5232 } 5233 5234 public boolean hasDispositionElement() { 5235 return this.disposition != null && !this.disposition.isEmpty(); 5236 } 5237 5238 public boolean hasDisposition() { 5239 return this.disposition != null && !this.disposition.isEmpty(); 5240 } 5241 5242 /** 5243 * @param value {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 5244 */ 5245 public ClaimResponse setDispositionElement(StringType value) { 5246 this.disposition = value; 5247 return this; 5248 } 5249 5250 /** 5251 * @return A description of the status of the adjudication. 5252 */ 5253 public String getDisposition() { 5254 return this.disposition == null ? null : this.disposition.getValue(); 5255 } 5256 5257 /** 5258 * @param value A description of the status of the adjudication. 5259 */ 5260 public ClaimResponse setDisposition(String value) { 5261 if (Utilities.noString(value)) 5262 this.disposition = null; 5263 else { 5264 if (this.disposition == null) 5265 this.disposition = new StringType(); 5266 this.disposition.setValue(value); 5267 } 5268 return this; 5269 } 5270 5271 /** 5272 * @return {@link #payeeType} (Party to be reimbursed: Subscriber, provider, other.) 5273 */ 5274 public CodeableConcept getPayeeType() { 5275 if (this.payeeType == null) 5276 if (Configuration.errorOnAutoCreate()) 5277 throw new Error("Attempt to auto-create ClaimResponse.payeeType"); 5278 else if (Configuration.doAutoCreate()) 5279 this.payeeType = new CodeableConcept(); // cc 5280 return this.payeeType; 5281 } 5282 5283 public boolean hasPayeeType() { 5284 return this.payeeType != null && !this.payeeType.isEmpty(); 5285 } 5286 5287 /** 5288 * @param value {@link #payeeType} (Party to be reimbursed: Subscriber, provider, other.) 5289 */ 5290 public ClaimResponse setPayeeType(CodeableConcept value) { 5291 this.payeeType = value; 5292 return this; 5293 } 5294 5295 /** 5296 * @return {@link #item} (The first tier service adjudications for submitted services.) 5297 */ 5298 public List<ItemComponent> getItem() { 5299 if (this.item == null) 5300 this.item = new ArrayList<ItemComponent>(); 5301 return this.item; 5302 } 5303 5304 /** 5305 * @return Returns a reference to <code>this</code> for easy method chaining 5306 */ 5307 public ClaimResponse setItem(List<ItemComponent> theItem) { 5308 this.item = theItem; 5309 return this; 5310 } 5311 5312 public boolean hasItem() { 5313 if (this.item == null) 5314 return false; 5315 for (ItemComponent item : this.item) 5316 if (!item.isEmpty()) 5317 return true; 5318 return false; 5319 } 5320 5321 public ItemComponent addItem() { //3 5322 ItemComponent t = new ItemComponent(); 5323 if (this.item == null) 5324 this.item = new ArrayList<ItemComponent>(); 5325 this.item.add(t); 5326 return t; 5327 } 5328 5329 public ClaimResponse addItem(ItemComponent t) { //3 5330 if (t == null) 5331 return this; 5332 if (this.item == null) 5333 this.item = new ArrayList<ItemComponent>(); 5334 this.item.add(t); 5335 return this; 5336 } 5337 5338 /** 5339 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 5340 */ 5341 public ItemComponent getItemFirstRep() { 5342 if (getItem().isEmpty()) { 5343 addItem(); 5344 } 5345 return getItem().get(0); 5346 } 5347 5348 /** 5349 * @return {@link #addItem} (The first tier service adjudications for payor added services.) 5350 */ 5351 public List<AddedItemComponent> getAddItem() { 5352 if (this.addItem == null) 5353 this.addItem = new ArrayList<AddedItemComponent>(); 5354 return this.addItem; 5355 } 5356 5357 /** 5358 * @return Returns a reference to <code>this</code> for easy method chaining 5359 */ 5360 public ClaimResponse setAddItem(List<AddedItemComponent> theAddItem) { 5361 this.addItem = theAddItem; 5362 return this; 5363 } 5364 5365 public boolean hasAddItem() { 5366 if (this.addItem == null) 5367 return false; 5368 for (AddedItemComponent item : this.addItem) 5369 if (!item.isEmpty()) 5370 return true; 5371 return false; 5372 } 5373 5374 public AddedItemComponent addAddItem() { //3 5375 AddedItemComponent t = new AddedItemComponent(); 5376 if (this.addItem == null) 5377 this.addItem = new ArrayList<AddedItemComponent>(); 5378 this.addItem.add(t); 5379 return t; 5380 } 5381 5382 public ClaimResponse addAddItem(AddedItemComponent t) { //3 5383 if (t == null) 5384 return this; 5385 if (this.addItem == null) 5386 this.addItem = new ArrayList<AddedItemComponent>(); 5387 this.addItem.add(t); 5388 return this; 5389 } 5390 5391 /** 5392 * @return The first repetition of repeating field {@link #addItem}, creating it if it does not already exist 5393 */ 5394 public AddedItemComponent getAddItemFirstRep() { 5395 if (getAddItem().isEmpty()) { 5396 addAddItem(); 5397 } 5398 return getAddItem().get(0); 5399 } 5400 5401 /** 5402 * @return {@link #error} (Mutually exclusive with Services Provided (Item).) 5403 */ 5404 public List<ErrorComponent> getError() { 5405 if (this.error == null) 5406 this.error = new ArrayList<ErrorComponent>(); 5407 return this.error; 5408 } 5409 5410 /** 5411 * @return Returns a reference to <code>this</code> for easy method chaining 5412 */ 5413 public ClaimResponse setError(List<ErrorComponent> theError) { 5414 this.error = theError; 5415 return this; 5416 } 5417 5418 public boolean hasError() { 5419 if (this.error == null) 5420 return false; 5421 for (ErrorComponent item : this.error) 5422 if (!item.isEmpty()) 5423 return true; 5424 return false; 5425 } 5426 5427 public ErrorComponent addError() { //3 5428 ErrorComponent t = new ErrorComponent(); 5429 if (this.error == null) 5430 this.error = new ArrayList<ErrorComponent>(); 5431 this.error.add(t); 5432 return t; 5433 } 5434 5435 public ClaimResponse addError(ErrorComponent t) { //3 5436 if (t == null) 5437 return this; 5438 if (this.error == null) 5439 this.error = new ArrayList<ErrorComponent>(); 5440 this.error.add(t); 5441 return this; 5442 } 5443 5444 /** 5445 * @return The first repetition of repeating field {@link #error}, creating it if it does not already exist 5446 */ 5447 public ErrorComponent getErrorFirstRep() { 5448 if (getError().isEmpty()) { 5449 addError(); 5450 } 5451 return getError().get(0); 5452 } 5453 5454 /** 5455 * @return {@link #totalCost} (The total cost of the services reported.) 5456 */ 5457 public Money getTotalCost() { 5458 if (this.totalCost == null) 5459 if (Configuration.errorOnAutoCreate()) 5460 throw new Error("Attempt to auto-create ClaimResponse.totalCost"); 5461 else if (Configuration.doAutoCreate()) 5462 this.totalCost = new Money(); // cc 5463 return this.totalCost; 5464 } 5465 5466 public boolean hasTotalCost() { 5467 return this.totalCost != null && !this.totalCost.isEmpty(); 5468 } 5469 5470 /** 5471 * @param value {@link #totalCost} (The total cost of the services reported.) 5472 */ 5473 public ClaimResponse setTotalCost(Money value) { 5474 this.totalCost = value; 5475 return this; 5476 } 5477 5478 /** 5479 * @return {@link #unallocDeductable} (The amount of deductible applied which was not allocated to any particular service line.) 5480 */ 5481 public Money getUnallocDeductable() { 5482 if (this.unallocDeductable == null) 5483 if (Configuration.errorOnAutoCreate()) 5484 throw new Error("Attempt to auto-create ClaimResponse.unallocDeductable"); 5485 else if (Configuration.doAutoCreate()) 5486 this.unallocDeductable = new Money(); // cc 5487 return this.unallocDeductable; 5488 } 5489 5490 public boolean hasUnallocDeductable() { 5491 return this.unallocDeductable != null && !this.unallocDeductable.isEmpty(); 5492 } 5493 5494 /** 5495 * @param value {@link #unallocDeductable} (The amount of deductible applied which was not allocated to any particular service line.) 5496 */ 5497 public ClaimResponse setUnallocDeductable(Money value) { 5498 this.unallocDeductable = value; 5499 return this; 5500 } 5501 5502 /** 5503 * @return {@link #totalBenefit} (Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible).) 5504 */ 5505 public Money getTotalBenefit() { 5506 if (this.totalBenefit == null) 5507 if (Configuration.errorOnAutoCreate()) 5508 throw new Error("Attempt to auto-create ClaimResponse.totalBenefit"); 5509 else if (Configuration.doAutoCreate()) 5510 this.totalBenefit = new Money(); // cc 5511 return this.totalBenefit; 5512 } 5513 5514 public boolean hasTotalBenefit() { 5515 return this.totalBenefit != null && !this.totalBenefit.isEmpty(); 5516 } 5517 5518 /** 5519 * @param value {@link #totalBenefit} (Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible).) 5520 */ 5521 public ClaimResponse setTotalBenefit(Money value) { 5522 this.totalBenefit = value; 5523 return this; 5524 } 5525 5526 /** 5527 * @return {@link #payment} (Payment details for the claim if the claim has been paid.) 5528 */ 5529 public PaymentComponent getPayment() { 5530 if (this.payment == null) 5531 if (Configuration.errorOnAutoCreate()) 5532 throw new Error("Attempt to auto-create ClaimResponse.payment"); 5533 else if (Configuration.doAutoCreate()) 5534 this.payment = new PaymentComponent(); // cc 5535 return this.payment; 5536 } 5537 5538 public boolean hasPayment() { 5539 return this.payment != null && !this.payment.isEmpty(); 5540 } 5541 5542 /** 5543 * @param value {@link #payment} (Payment details for the claim if the claim has been paid.) 5544 */ 5545 public ClaimResponse setPayment(PaymentComponent value) { 5546 this.payment = value; 5547 return this; 5548 } 5549 5550 /** 5551 * @return {@link #reserved} (Status of funds reservation (For provider, for Patient, None).) 5552 */ 5553 public Coding getReserved() { 5554 if (this.reserved == null) 5555 if (Configuration.errorOnAutoCreate()) 5556 throw new Error("Attempt to auto-create ClaimResponse.reserved"); 5557 else if (Configuration.doAutoCreate()) 5558 this.reserved = new Coding(); // cc 5559 return this.reserved; 5560 } 5561 5562 public boolean hasReserved() { 5563 return this.reserved != null && !this.reserved.isEmpty(); 5564 } 5565 5566 /** 5567 * @param value {@link #reserved} (Status of funds reservation (For provider, for Patient, None).) 5568 */ 5569 public ClaimResponse setReserved(Coding value) { 5570 this.reserved = value; 5571 return this; 5572 } 5573 5574 /** 5575 * @return {@link #form} (The form to be used for printing the content.) 5576 */ 5577 public CodeableConcept getForm() { 5578 if (this.form == null) 5579 if (Configuration.errorOnAutoCreate()) 5580 throw new Error("Attempt to auto-create ClaimResponse.form"); 5581 else if (Configuration.doAutoCreate()) 5582 this.form = new CodeableConcept(); // cc 5583 return this.form; 5584 } 5585 5586 public boolean hasForm() { 5587 return this.form != null && !this.form.isEmpty(); 5588 } 5589 5590 /** 5591 * @param value {@link #form} (The form to be used for printing the content.) 5592 */ 5593 public ClaimResponse setForm(CodeableConcept value) { 5594 this.form = value; 5595 return this; 5596 } 5597 5598 /** 5599 * @return {@link #processNote} (Note text.) 5600 */ 5601 public List<NoteComponent> getProcessNote() { 5602 if (this.processNote == null) 5603 this.processNote = new ArrayList<NoteComponent>(); 5604 return this.processNote; 5605 } 5606 5607 /** 5608 * @return Returns a reference to <code>this</code> for easy method chaining 5609 */ 5610 public ClaimResponse setProcessNote(List<NoteComponent> theProcessNote) { 5611 this.processNote = theProcessNote; 5612 return this; 5613 } 5614 5615 public boolean hasProcessNote() { 5616 if (this.processNote == null) 5617 return false; 5618 for (NoteComponent item : this.processNote) 5619 if (!item.isEmpty()) 5620 return true; 5621 return false; 5622 } 5623 5624 public NoteComponent addProcessNote() { //3 5625 NoteComponent t = new NoteComponent(); 5626 if (this.processNote == null) 5627 this.processNote = new ArrayList<NoteComponent>(); 5628 this.processNote.add(t); 5629 return t; 5630 } 5631 5632 public ClaimResponse addProcessNote(NoteComponent t) { //3 5633 if (t == null) 5634 return this; 5635 if (this.processNote == null) 5636 this.processNote = new ArrayList<NoteComponent>(); 5637 this.processNote.add(t); 5638 return this; 5639 } 5640 5641 /** 5642 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist 5643 */ 5644 public NoteComponent getProcessNoteFirstRep() { 5645 if (getProcessNote().isEmpty()) { 5646 addProcessNote(); 5647 } 5648 return getProcessNote().get(0); 5649 } 5650 5651 /** 5652 * @return {@link #communicationRequest} (Request for additional supporting or authorizing information, such as: documents, images or resources.) 5653 */ 5654 public List<Reference> getCommunicationRequest() { 5655 if (this.communicationRequest == null) 5656 this.communicationRequest = new ArrayList<Reference>(); 5657 return this.communicationRequest; 5658 } 5659 5660 /** 5661 * @return Returns a reference to <code>this</code> for easy method chaining 5662 */ 5663 public ClaimResponse setCommunicationRequest(List<Reference> theCommunicationRequest) { 5664 this.communicationRequest = theCommunicationRequest; 5665 return this; 5666 } 5667 5668 public boolean hasCommunicationRequest() { 5669 if (this.communicationRequest == null) 5670 return false; 5671 for (Reference item : this.communicationRequest) 5672 if (!item.isEmpty()) 5673 return true; 5674 return false; 5675 } 5676 5677 public Reference addCommunicationRequest() { //3 5678 Reference t = new Reference(); 5679 if (this.communicationRequest == null) 5680 this.communicationRequest = new ArrayList<Reference>(); 5681 this.communicationRequest.add(t); 5682 return t; 5683 } 5684 5685 public ClaimResponse addCommunicationRequest(Reference t) { //3 5686 if (t == null) 5687 return this; 5688 if (this.communicationRequest == null) 5689 this.communicationRequest = new ArrayList<Reference>(); 5690 this.communicationRequest.add(t); 5691 return this; 5692 } 5693 5694 /** 5695 * @return The first repetition of repeating field {@link #communicationRequest}, creating it if it does not already exist 5696 */ 5697 public Reference getCommunicationRequestFirstRep() { 5698 if (getCommunicationRequest().isEmpty()) { 5699 addCommunicationRequest(); 5700 } 5701 return getCommunicationRequest().get(0); 5702 } 5703 5704 /** 5705 * @return {@link #insurance} (Financial instrument by which payment information for health care.) 5706 */ 5707 public List<InsuranceComponent> getInsurance() { 5708 if (this.insurance == null) 5709 this.insurance = new ArrayList<InsuranceComponent>(); 5710 return this.insurance; 5711 } 5712 5713 /** 5714 * @return Returns a reference to <code>this</code> for easy method chaining 5715 */ 5716 public ClaimResponse setInsurance(List<InsuranceComponent> theInsurance) { 5717 this.insurance = theInsurance; 5718 return this; 5719 } 5720 5721 public boolean hasInsurance() { 5722 if (this.insurance == null) 5723 return false; 5724 for (InsuranceComponent item : this.insurance) 5725 if (!item.isEmpty()) 5726 return true; 5727 return false; 5728 } 5729 5730 public InsuranceComponent addInsurance() { //3 5731 InsuranceComponent t = new InsuranceComponent(); 5732 if (this.insurance == null) 5733 this.insurance = new ArrayList<InsuranceComponent>(); 5734 this.insurance.add(t); 5735 return t; 5736 } 5737 5738 public ClaimResponse addInsurance(InsuranceComponent t) { //3 5739 if (t == null) 5740 return this; 5741 if (this.insurance == null) 5742 this.insurance = new ArrayList<InsuranceComponent>(); 5743 this.insurance.add(t); 5744 return this; 5745 } 5746 5747 /** 5748 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist 5749 */ 5750 public InsuranceComponent getInsuranceFirstRep() { 5751 if (getInsurance().isEmpty()) { 5752 addInsurance(); 5753 } 5754 return getInsurance().get(0); 5755 } 5756 5757 protected void listChildren(List<Property> children) { 5758 super.listChildren(children); 5759 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 5760 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 5761 children.add(new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient)); 5762 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 5763 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, insurer)); 5764 children.add(new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider)); 5765 children.add(new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization)); 5766 children.add(new Property("request", "Reference(Claim)", "Original request resource referrence.", 0, 1, request)); 5767 children.add(new Property("outcome", "CodeableConcept", "Processing outcome errror, partial or complete processing.", 0, 1, outcome)); 5768 children.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 5769 children.add(new Property("payeeType", "CodeableConcept", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, payeeType)); 5770 children.add(new Property("item", "", "The first tier service adjudications for submitted services.", 0, java.lang.Integer.MAX_VALUE, item)); 5771 children.add(new Property("addItem", "", "The first tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, addItem)); 5772 children.add(new Property("error", "", "Mutually exclusive with Services Provided (Item).", 0, java.lang.Integer.MAX_VALUE, error)); 5773 children.add(new Property("totalCost", "Money", "The total cost of the services reported.", 0, 1, totalCost)); 5774 children.add(new Property("unallocDeductable", "Money", "The amount of deductible applied which was not allocated to any particular service line.", 0, 1, unallocDeductable)); 5775 children.add(new Property("totalBenefit", "Money", "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible).", 0, 1, totalBenefit)); 5776 children.add(new Property("payment", "", "Payment details for the claim if the claim has been paid.", 0, 1, payment)); 5777 children.add(new Property("reserved", "Coding", "Status of funds reservation (For provider, for Patient, None).", 0, 1, reserved)); 5778 children.add(new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form)); 5779 children.add(new Property("processNote", "", "Note text.", 0, java.lang.Integer.MAX_VALUE, processNote)); 5780 children.add(new Property("communicationRequest", "Reference(CommunicationRequest)", "Request for additional supporting or authorizing information, such as: documents, images or resources.", 0, java.lang.Integer.MAX_VALUE, communicationRequest)); 5781 children.add(new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, java.lang.Integer.MAX_VALUE, insurance)); 5782 } 5783 5784 @Override 5785 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5786 switch (_hash) { 5787 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 5788 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 5789 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient); 5790 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 5791 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, insurer); 5792 case 1601527200: /*requestProvider*/ return new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider); 5793 case 599053666: /*requestOrganization*/ return new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization); 5794 case 1095692943: /*request*/ return new Property("request", "Reference(Claim)", "Original request resource referrence.", 0, 1, request); 5795 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Processing outcome errror, partial or complete processing.", 0, 1, outcome); 5796 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition); 5797 case -316321118: /*payeeType*/ return new Property("payeeType", "CodeableConcept", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, payeeType); 5798 case 3242771: /*item*/ return new Property("item", "", "The first tier service adjudications for submitted services.", 0, java.lang.Integer.MAX_VALUE, item); 5799 case -1148899500: /*addItem*/ return new Property("addItem", "", "The first tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, addItem); 5800 case 96784904: /*error*/ return new Property("error", "", "Mutually exclusive with Services Provided (Item).", 0, java.lang.Integer.MAX_VALUE, error); 5801 case -577782479: /*totalCost*/ return new Property("totalCost", "Money", "The total cost of the services reported.", 0, 1, totalCost); 5802 case 2096309753: /*unallocDeductable*/ return new Property("unallocDeductable", "Money", "The amount of deductible applied which was not allocated to any particular service line.", 0, 1, unallocDeductable); 5803 case 332332211: /*totalBenefit*/ return new Property("totalBenefit", "Money", "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible).", 0, 1, totalBenefit); 5804 case -786681338: /*payment*/ return new Property("payment", "", "Payment details for the claim if the claim has been paid.", 0, 1, payment); 5805 case -350385368: /*reserved*/ return new Property("reserved", "Coding", "Status of funds reservation (For provider, for Patient, None).", 0, 1, reserved); 5806 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form); 5807 case 202339073: /*processNote*/ return new Property("processNote", "", "Note text.", 0, java.lang.Integer.MAX_VALUE, processNote); 5808 case -2071896615: /*communicationRequest*/ return new Property("communicationRequest", "Reference(CommunicationRequest)", "Request for additional supporting or authorizing information, such as: documents, images or resources.", 0, java.lang.Integer.MAX_VALUE, communicationRequest); 5809 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, java.lang.Integer.MAX_VALUE, insurance); 5810 default: return super.getNamedProperty(_hash, _name, _checkValid); 5811 } 5812 5813 } 5814 5815 @Override 5816 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5817 switch (hash) { 5818 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5819 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ClaimResponseStatus> 5820 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 5821 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 5822 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 5823 case 1601527200: /*requestProvider*/ return this.requestProvider == null ? new Base[0] : new Base[] {this.requestProvider}; // Reference 5824 case 599053666: /*requestOrganization*/ return this.requestOrganization == null ? new Base[0] : new Base[] {this.requestOrganization}; // Reference 5825 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 5826 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 5827 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 5828 case -316321118: /*payeeType*/ return this.payeeType == null ? new Base[0] : new Base[] {this.payeeType}; // CodeableConcept 5829 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 5830 case -1148899500: /*addItem*/ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 5831 case 96784904: /*error*/ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // ErrorComponent 5832 case -577782479: /*totalCost*/ return this.totalCost == null ? new Base[0] : new Base[] {this.totalCost}; // Money 5833 case 2096309753: /*unallocDeductable*/ return this.unallocDeductable == null ? new Base[0] : new Base[] {this.unallocDeductable}; // Money 5834 case 332332211: /*totalBenefit*/ return this.totalBenefit == null ? new Base[0] : new Base[] {this.totalBenefit}; // Money 5835 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // PaymentComponent 5836 case -350385368: /*reserved*/ return this.reserved == null ? new Base[0] : new Base[] {this.reserved}; // Coding 5837 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 5838 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 5839 case -2071896615: /*communicationRequest*/ return this.communicationRequest == null ? new Base[0] : this.communicationRequest.toArray(new Base[this.communicationRequest.size()]); // Reference 5840 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 5841 default: return super.getProperty(hash, name, checkValid); 5842 } 5843 5844 } 5845 5846 @Override 5847 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5848 switch (hash) { 5849 case -1618432855: // identifier 5850 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5851 return value; 5852 case -892481550: // status 5853 value = new ClaimResponseStatusEnumFactory().fromType(castToCode(value)); 5854 this.status = (Enumeration) value; // Enumeration<ClaimResponseStatus> 5855 return value; 5856 case -791418107: // patient 5857 this.patient = castToReference(value); // Reference 5858 return value; 5859 case 1028554472: // created 5860 this.created = castToDateTime(value); // DateTimeType 5861 return value; 5862 case 1957615864: // insurer 5863 this.insurer = castToReference(value); // Reference 5864 return value; 5865 case 1601527200: // requestProvider 5866 this.requestProvider = castToReference(value); // Reference 5867 return value; 5868 case 599053666: // requestOrganization 5869 this.requestOrganization = castToReference(value); // Reference 5870 return value; 5871 case 1095692943: // request 5872 this.request = castToReference(value); // Reference 5873 return value; 5874 case -1106507950: // outcome 5875 this.outcome = castToCodeableConcept(value); // CodeableConcept 5876 return value; 5877 case 583380919: // disposition 5878 this.disposition = castToString(value); // StringType 5879 return value; 5880 case -316321118: // payeeType 5881 this.payeeType = castToCodeableConcept(value); // CodeableConcept 5882 return value; 5883 case 3242771: // item 5884 this.getItem().add((ItemComponent) value); // ItemComponent 5885 return value; 5886 case -1148899500: // addItem 5887 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 5888 return value; 5889 case 96784904: // error 5890 this.getError().add((ErrorComponent) value); // ErrorComponent 5891 return value; 5892 case -577782479: // totalCost 5893 this.totalCost = castToMoney(value); // Money 5894 return value; 5895 case 2096309753: // unallocDeductable 5896 this.unallocDeductable = castToMoney(value); // Money 5897 return value; 5898 case 332332211: // totalBenefit 5899 this.totalBenefit = castToMoney(value); // Money 5900 return value; 5901 case -786681338: // payment 5902 this.payment = (PaymentComponent) value; // PaymentComponent 5903 return value; 5904 case -350385368: // reserved 5905 this.reserved = castToCoding(value); // Coding 5906 return value; 5907 case 3148996: // form 5908 this.form = castToCodeableConcept(value); // CodeableConcept 5909 return value; 5910 case 202339073: // processNote 5911 this.getProcessNote().add((NoteComponent) value); // NoteComponent 5912 return value; 5913 case -2071896615: // communicationRequest 5914 this.getCommunicationRequest().add(castToReference(value)); // Reference 5915 return value; 5916 case 73049818: // insurance 5917 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 5918 return value; 5919 default: return super.setProperty(hash, name, value); 5920 } 5921 5922 } 5923 5924 @Override 5925 public Base setProperty(String name, Base value) throws FHIRException { 5926 if (name.equals("identifier")) { 5927 this.getIdentifier().add(castToIdentifier(value)); 5928 } else if (name.equals("status")) { 5929 value = new ClaimResponseStatusEnumFactory().fromType(castToCode(value)); 5930 this.status = (Enumeration) value; // Enumeration<ClaimResponseStatus> 5931 } else if (name.equals("patient")) { 5932 this.patient = castToReference(value); // Reference 5933 } else if (name.equals("created")) { 5934 this.created = castToDateTime(value); // DateTimeType 5935 } else if (name.equals("insurer")) { 5936 this.insurer = castToReference(value); // Reference 5937 } else if (name.equals("requestProvider")) { 5938 this.requestProvider = castToReference(value); // Reference 5939 } else if (name.equals("requestOrganization")) { 5940 this.requestOrganization = castToReference(value); // Reference 5941 } else if (name.equals("request")) { 5942 this.request = castToReference(value); // Reference 5943 } else if (name.equals("outcome")) { 5944 this.outcome = castToCodeableConcept(value); // CodeableConcept 5945 } else if (name.equals("disposition")) { 5946 this.disposition = castToString(value); // StringType 5947 } else if (name.equals("payeeType")) { 5948 this.payeeType = castToCodeableConcept(value); // CodeableConcept 5949 } else if (name.equals("item")) { 5950 this.getItem().add((ItemComponent) value); 5951 } else if (name.equals("addItem")) { 5952 this.getAddItem().add((AddedItemComponent) value); 5953 } else if (name.equals("error")) { 5954 this.getError().add((ErrorComponent) value); 5955 } else if (name.equals("totalCost")) { 5956 this.totalCost = castToMoney(value); // Money 5957 } else if (name.equals("unallocDeductable")) { 5958 this.unallocDeductable = castToMoney(value); // Money 5959 } else if (name.equals("totalBenefit")) { 5960 this.totalBenefit = castToMoney(value); // Money 5961 } else if (name.equals("payment")) { 5962 this.payment = (PaymentComponent) value; // PaymentComponent 5963 } else if (name.equals("reserved")) { 5964 this.reserved = castToCoding(value); // Coding 5965 } else if (name.equals("form")) { 5966 this.form = castToCodeableConcept(value); // CodeableConcept 5967 } else if (name.equals("processNote")) { 5968 this.getProcessNote().add((NoteComponent) value); 5969 } else if (name.equals("communicationRequest")) { 5970 this.getCommunicationRequest().add(castToReference(value)); 5971 } else if (name.equals("insurance")) { 5972 this.getInsurance().add((InsuranceComponent) value); 5973 } else 5974 return super.setProperty(name, value); 5975 return value; 5976 } 5977 5978 @Override 5979 public Base makeProperty(int hash, String name) throws FHIRException { 5980 switch (hash) { 5981 case -1618432855: return addIdentifier(); 5982 case -892481550: return getStatusElement(); 5983 case -791418107: return getPatient(); 5984 case 1028554472: return getCreatedElement(); 5985 case 1957615864: return getInsurer(); 5986 case 1601527200: return getRequestProvider(); 5987 case 599053666: return getRequestOrganization(); 5988 case 1095692943: return getRequest(); 5989 case -1106507950: return getOutcome(); 5990 case 583380919: return getDispositionElement(); 5991 case -316321118: return getPayeeType(); 5992 case 3242771: return addItem(); 5993 case -1148899500: return addAddItem(); 5994 case 96784904: return addError(); 5995 case -577782479: return getTotalCost(); 5996 case 2096309753: return getUnallocDeductable(); 5997 case 332332211: return getTotalBenefit(); 5998 case -786681338: return getPayment(); 5999 case -350385368: return getReserved(); 6000 case 3148996: return getForm(); 6001 case 202339073: return addProcessNote(); 6002 case -2071896615: return addCommunicationRequest(); 6003 case 73049818: return addInsurance(); 6004 default: return super.makeProperty(hash, name); 6005 } 6006 6007 } 6008 6009 @Override 6010 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6011 switch (hash) { 6012 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6013 case -892481550: /*status*/ return new String[] {"code"}; 6014 case -791418107: /*patient*/ return new String[] {"Reference"}; 6015 case 1028554472: /*created*/ return new String[] {"dateTime"}; 6016 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 6017 case 1601527200: /*requestProvider*/ return new String[] {"Reference"}; 6018 case 599053666: /*requestOrganization*/ return new String[] {"Reference"}; 6019 case 1095692943: /*request*/ return new String[] {"Reference"}; 6020 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 6021 case 583380919: /*disposition*/ return new String[] {"string"}; 6022 case -316321118: /*payeeType*/ return new String[] {"CodeableConcept"}; 6023 case 3242771: /*item*/ return new String[] {}; 6024 case -1148899500: /*addItem*/ return new String[] {}; 6025 case 96784904: /*error*/ return new String[] {}; 6026 case -577782479: /*totalCost*/ return new String[] {"Money"}; 6027 case 2096309753: /*unallocDeductable*/ return new String[] {"Money"}; 6028 case 332332211: /*totalBenefit*/ return new String[] {"Money"}; 6029 case -786681338: /*payment*/ return new String[] {}; 6030 case -350385368: /*reserved*/ return new String[] {"Coding"}; 6031 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 6032 case 202339073: /*processNote*/ return new String[] {}; 6033 case -2071896615: /*communicationRequest*/ return new String[] {"Reference"}; 6034 case 73049818: /*insurance*/ return new String[] {}; 6035 default: return super.getTypesForProperty(hash, name); 6036 } 6037 6038 } 6039 6040 @Override 6041 public Base addChild(String name) throws FHIRException { 6042 if (name.equals("identifier")) { 6043 return addIdentifier(); 6044 } 6045 else if (name.equals("status")) { 6046 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.status"); 6047 } 6048 else if (name.equals("patient")) { 6049 this.patient = new Reference(); 6050 return this.patient; 6051 } 6052 else if (name.equals("created")) { 6053 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.created"); 6054 } 6055 else if (name.equals("insurer")) { 6056 this.insurer = new Reference(); 6057 return this.insurer; 6058 } 6059 else if (name.equals("requestProvider")) { 6060 this.requestProvider = new Reference(); 6061 return this.requestProvider; 6062 } 6063 else if (name.equals("requestOrganization")) { 6064 this.requestOrganization = new Reference(); 6065 return this.requestOrganization; 6066 } 6067 else if (name.equals("request")) { 6068 this.request = new Reference(); 6069 return this.request; 6070 } 6071 else if (name.equals("outcome")) { 6072 this.outcome = new CodeableConcept(); 6073 return this.outcome; 6074 } 6075 else if (name.equals("disposition")) { 6076 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.disposition"); 6077 } 6078 else if (name.equals("payeeType")) { 6079 this.payeeType = new CodeableConcept(); 6080 return this.payeeType; 6081 } 6082 else if (name.equals("item")) { 6083 return addItem(); 6084 } 6085 else if (name.equals("addItem")) { 6086 return addAddItem(); 6087 } 6088 else if (name.equals("error")) { 6089 return addError(); 6090 } 6091 else if (name.equals("totalCost")) { 6092 this.totalCost = new Money(); 6093 return this.totalCost; 6094 } 6095 else if (name.equals("unallocDeductable")) { 6096 this.unallocDeductable = new Money(); 6097 return this.unallocDeductable; 6098 } 6099 else if (name.equals("totalBenefit")) { 6100 this.totalBenefit = new Money(); 6101 return this.totalBenefit; 6102 } 6103 else if (name.equals("payment")) { 6104 this.payment = new PaymentComponent(); 6105 return this.payment; 6106 } 6107 else if (name.equals("reserved")) { 6108 this.reserved = new Coding(); 6109 return this.reserved; 6110 } 6111 else if (name.equals("form")) { 6112 this.form = new CodeableConcept(); 6113 return this.form; 6114 } 6115 else if (name.equals("processNote")) { 6116 return addProcessNote(); 6117 } 6118 else if (name.equals("communicationRequest")) { 6119 return addCommunicationRequest(); 6120 } 6121 else if (name.equals("insurance")) { 6122 return addInsurance(); 6123 } 6124 else 6125 return super.addChild(name); 6126 } 6127 6128 public String fhirType() { 6129 return "ClaimResponse"; 6130 6131 } 6132 6133 public ClaimResponse copy() { 6134 ClaimResponse dst = new ClaimResponse(); 6135 copyValues(dst); 6136 if (identifier != null) { 6137 dst.identifier = new ArrayList<Identifier>(); 6138 for (Identifier i : identifier) 6139 dst.identifier.add(i.copy()); 6140 }; 6141 dst.status = status == null ? null : status.copy(); 6142 dst.patient = patient == null ? null : patient.copy(); 6143 dst.created = created == null ? null : created.copy(); 6144 dst.insurer = insurer == null ? null : insurer.copy(); 6145 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 6146 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 6147 dst.request = request == null ? null : request.copy(); 6148 dst.outcome = outcome == null ? null : outcome.copy(); 6149 dst.disposition = disposition == null ? null : disposition.copy(); 6150 dst.payeeType = payeeType == null ? null : payeeType.copy(); 6151 if (item != null) { 6152 dst.item = new ArrayList<ItemComponent>(); 6153 for (ItemComponent i : item) 6154 dst.item.add(i.copy()); 6155 }; 6156 if (addItem != null) { 6157 dst.addItem = new ArrayList<AddedItemComponent>(); 6158 for (AddedItemComponent i : addItem) 6159 dst.addItem.add(i.copy()); 6160 }; 6161 if (error != null) { 6162 dst.error = new ArrayList<ErrorComponent>(); 6163 for (ErrorComponent i : error) 6164 dst.error.add(i.copy()); 6165 }; 6166 dst.totalCost = totalCost == null ? null : totalCost.copy(); 6167 dst.unallocDeductable = unallocDeductable == null ? null : unallocDeductable.copy(); 6168 dst.totalBenefit = totalBenefit == null ? null : totalBenefit.copy(); 6169 dst.payment = payment == null ? null : payment.copy(); 6170 dst.reserved = reserved == null ? null : reserved.copy(); 6171 dst.form = form == null ? null : form.copy(); 6172 if (processNote != null) { 6173 dst.processNote = new ArrayList<NoteComponent>(); 6174 for (NoteComponent i : processNote) 6175 dst.processNote.add(i.copy()); 6176 }; 6177 if (communicationRequest != null) { 6178 dst.communicationRequest = new ArrayList<Reference>(); 6179 for (Reference i : communicationRequest) 6180 dst.communicationRequest.add(i.copy()); 6181 }; 6182 if (insurance != null) { 6183 dst.insurance = new ArrayList<InsuranceComponent>(); 6184 for (InsuranceComponent i : insurance) 6185 dst.insurance.add(i.copy()); 6186 }; 6187 return dst; 6188 } 6189 6190 protected ClaimResponse typedCopy() { 6191 return copy(); 6192 } 6193 6194 @Override 6195 public boolean equalsDeep(Base other_) { 6196 if (!super.equalsDeep(other_)) 6197 return false; 6198 if (!(other_ instanceof ClaimResponse)) 6199 return false; 6200 ClaimResponse o = (ClaimResponse) other_; 6201 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(patient, o.patient, true) 6202 && compareDeep(created, o.created, true) && compareDeep(insurer, o.insurer, true) && compareDeep(requestProvider, o.requestProvider, true) 6203 && compareDeep(requestOrganization, o.requestOrganization, true) && compareDeep(request, o.request, true) 6204 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) && compareDeep(payeeType, o.payeeType, true) 6205 && compareDeep(item, o.item, true) && compareDeep(addItem, o.addItem, true) && compareDeep(error, o.error, true) 6206 && compareDeep(totalCost, o.totalCost, true) && compareDeep(unallocDeductable, o.unallocDeductable, true) 6207 && compareDeep(totalBenefit, o.totalBenefit, true) && compareDeep(payment, o.payment, true) && compareDeep(reserved, o.reserved, true) 6208 && compareDeep(form, o.form, true) && compareDeep(processNote, o.processNote, true) && compareDeep(communicationRequest, o.communicationRequest, true) 6209 && compareDeep(insurance, o.insurance, true); 6210 } 6211 6212 @Override 6213 public boolean equalsShallow(Base other_) { 6214 if (!super.equalsShallow(other_)) 6215 return false; 6216 if (!(other_ instanceof ClaimResponse)) 6217 return false; 6218 ClaimResponse o = (ClaimResponse) other_; 6219 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(disposition, o.disposition, true) 6220 ; 6221 } 6222 6223 public boolean isEmpty() { 6224 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, patient 6225 , created, insurer, requestProvider, requestOrganization, request, outcome, disposition 6226 , payeeType, item, addItem, error, totalCost, unallocDeductable, totalBenefit 6227 , payment, reserved, form, processNote, communicationRequest, insurance); 6228 } 6229 6230 @Override 6231 public ResourceType getResourceType() { 6232 return ResourceType.ClaimResponse; 6233 } 6234 6235 /** 6236 * Search parameter: <b>identifier</b> 6237 * <p> 6238 * Description: <b>The identity of the claimresponse</b><br> 6239 * Type: <b>token</b><br> 6240 * Path: <b>ClaimResponse.identifier</b><br> 6241 * </p> 6242 */ 6243 @SearchParamDefinition(name="identifier", path="ClaimResponse.identifier", description="The identity of the claimresponse", type="token" ) 6244 public static final String SP_IDENTIFIER = "identifier"; 6245 /** 6246 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6247 * <p> 6248 * Description: <b>The identity of the claimresponse</b><br> 6249 * Type: <b>token</b><br> 6250 * Path: <b>ClaimResponse.identifier</b><br> 6251 * </p> 6252 */ 6253 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6254 6255 /** 6256 * Search parameter: <b>request</b> 6257 * <p> 6258 * Description: <b>The claim reference</b><br> 6259 * Type: <b>reference</b><br> 6260 * Path: <b>ClaimResponse.request</b><br> 6261 * </p> 6262 */ 6263 @SearchParamDefinition(name="request", path="ClaimResponse.request", description="The claim reference", type="reference", target={Claim.class } ) 6264 public static final String SP_REQUEST = "request"; 6265 /** 6266 * <b>Fluent Client</b> search parameter constant for <b>request</b> 6267 * <p> 6268 * Description: <b>The claim reference</b><br> 6269 * Type: <b>reference</b><br> 6270 * Path: <b>ClaimResponse.request</b><br> 6271 * </p> 6272 */ 6273 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 6274 6275/** 6276 * Constant for fluent queries to be used to add include statements. Specifies 6277 * the path value of "<b>ClaimResponse:request</b>". 6278 */ 6279 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("ClaimResponse:request").toLocked(); 6280 6281 /** 6282 * Search parameter: <b>disposition</b> 6283 * <p> 6284 * Description: <b>The contents of the disposition message</b><br> 6285 * Type: <b>string</b><br> 6286 * Path: <b>ClaimResponse.disposition</b><br> 6287 * </p> 6288 */ 6289 @SearchParamDefinition(name="disposition", path="ClaimResponse.disposition", description="The contents of the disposition message", type="string" ) 6290 public static final String SP_DISPOSITION = "disposition"; 6291 /** 6292 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 6293 * <p> 6294 * Description: <b>The contents of the disposition message</b><br> 6295 * Type: <b>string</b><br> 6296 * Path: <b>ClaimResponse.disposition</b><br> 6297 * </p> 6298 */ 6299 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 6300 6301 /** 6302 * Search parameter: <b>insurer</b> 6303 * <p> 6304 * Description: <b>The organization who generated this resource</b><br> 6305 * Type: <b>reference</b><br> 6306 * Path: <b>ClaimResponse.insurer</b><br> 6307 * </p> 6308 */ 6309 @SearchParamDefinition(name="insurer", path="ClaimResponse.insurer", description="The organization who generated this resource", type="reference", target={Organization.class } ) 6310 public static final String SP_INSURER = "insurer"; 6311 /** 6312 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 6313 * <p> 6314 * Description: <b>The organization who generated this resource</b><br> 6315 * Type: <b>reference</b><br> 6316 * Path: <b>ClaimResponse.insurer</b><br> 6317 * </p> 6318 */ 6319 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 6320 6321/** 6322 * Constant for fluent queries to be used to add include statements. Specifies 6323 * the path value of "<b>ClaimResponse:insurer</b>". 6324 */ 6325 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("ClaimResponse:insurer").toLocked(); 6326 6327 /** 6328 * Search parameter: <b>created</b> 6329 * <p> 6330 * Description: <b>The creation date</b><br> 6331 * Type: <b>date</b><br> 6332 * Path: <b>ClaimResponse.created</b><br> 6333 * </p> 6334 */ 6335 @SearchParamDefinition(name="created", path="ClaimResponse.created", description="The creation date", type="date" ) 6336 public static final String SP_CREATED = "created"; 6337 /** 6338 * <b>Fluent Client</b> search parameter constant for <b>created</b> 6339 * <p> 6340 * Description: <b>The creation date</b><br> 6341 * Type: <b>date</b><br> 6342 * Path: <b>ClaimResponse.created</b><br> 6343 * </p> 6344 */ 6345 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 6346 6347 /** 6348 * Search parameter: <b>patient</b> 6349 * <p> 6350 * Description: <b>The subject of care.</b><br> 6351 * Type: <b>reference</b><br> 6352 * Path: <b>ClaimResponse.patient</b><br> 6353 * </p> 6354 */ 6355 @SearchParamDefinition(name="patient", path="ClaimResponse.patient", description="The subject of care.", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 6356 public static final String SP_PATIENT = "patient"; 6357 /** 6358 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6359 * <p> 6360 * Description: <b>The subject of care.</b><br> 6361 * Type: <b>reference</b><br> 6362 * Path: <b>ClaimResponse.patient</b><br> 6363 * </p> 6364 */ 6365 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 6366 6367/** 6368 * Constant for fluent queries to be used to add include statements. Specifies 6369 * the path value of "<b>ClaimResponse:patient</b>". 6370 */ 6371 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ClaimResponse:patient").toLocked(); 6372 6373 /** 6374 * Search parameter: <b>payment-date</b> 6375 * <p> 6376 * Description: <b>The expected paymentDate</b><br> 6377 * Type: <b>date</b><br> 6378 * Path: <b>ClaimResponse.payment.date</b><br> 6379 * </p> 6380 */ 6381 @SearchParamDefinition(name="payment-date", path="ClaimResponse.payment.date", description="The expected paymentDate", type="date" ) 6382 public static final String SP_PAYMENT_DATE = "payment-date"; 6383 /** 6384 * <b>Fluent Client</b> search parameter constant for <b>payment-date</b> 6385 * <p> 6386 * Description: <b>The expected paymentDate</b><br> 6387 * Type: <b>date</b><br> 6388 * Path: <b>ClaimResponse.payment.date</b><br> 6389 * </p> 6390 */ 6391 public static final ca.uhn.fhir.rest.gclient.DateClientParam PAYMENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PAYMENT_DATE); 6392 6393 /** 6394 * Search parameter: <b>request-provider</b> 6395 * <p> 6396 * Description: <b>The Provider of the claim</b><br> 6397 * Type: <b>reference</b><br> 6398 * Path: <b>ClaimResponse.requestProvider</b><br> 6399 * </p> 6400 */ 6401 @SearchParamDefinition(name="request-provider", path="ClaimResponse.requestProvider", description="The Provider of the claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 6402 public static final String SP_REQUEST_PROVIDER = "request-provider"; 6403 /** 6404 * <b>Fluent Client</b> search parameter constant for <b>request-provider</b> 6405 * <p> 6406 * Description: <b>The Provider of the claim</b><br> 6407 * Type: <b>reference</b><br> 6408 * Path: <b>ClaimResponse.requestProvider</b><br> 6409 * </p> 6410 */ 6411 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST_PROVIDER); 6412 6413/** 6414 * Constant for fluent queries to be used to add include statements. Specifies 6415 * the path value of "<b>ClaimResponse:request-provider</b>". 6416 */ 6417 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST_PROVIDER = new ca.uhn.fhir.model.api.Include("ClaimResponse:request-provider").toLocked(); 6418 6419 /** 6420 * Search parameter: <b>outcome</b> 6421 * <p> 6422 * Description: <b>The processing outcome</b><br> 6423 * Type: <b>token</b><br> 6424 * Path: <b>ClaimResponse.outcome</b><br> 6425 * </p> 6426 */ 6427 @SearchParamDefinition(name="outcome", path="ClaimResponse.outcome", description="The processing outcome", type="token" ) 6428 public static final String SP_OUTCOME = "outcome"; 6429 /** 6430 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 6431 * <p> 6432 * Description: <b>The processing outcome</b><br> 6433 * Type: <b>token</b><br> 6434 * Path: <b>ClaimResponse.outcome</b><br> 6435 * </p> 6436 */ 6437 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 6438 6439 6440}