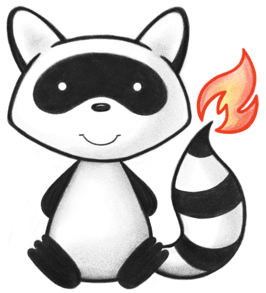
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 052 */ 053@ResourceDef(name="ClinicalImpression", profile="http://hl7.org/fhir/Profile/ClinicalImpression") 054public class ClinicalImpression extends DomainResource { 055 056 public enum ClinicalImpressionStatus { 057 /** 058 * The assessment is still on-going and results are not yet final. 059 */ 060 DRAFT, 061 /** 062 * The assessment is done and the results are final. 063 */ 064 COMPLETED, 065 /** 066 * This assessment was never actually done and the record is erroneous (e.g. Wrong patient). 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static ClinicalImpressionStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("draft".equals(codeString)) 077 return DRAFT; 078 if ("completed".equals(codeString)) 079 return COMPLETED; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown ClinicalImpressionStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case DRAFT: return "draft"; 090 case COMPLETED: return "completed"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case DRAFT: return "http://hl7.org/fhir/clinical-impression-status"; 099 case COMPLETED: return "http://hl7.org/fhir/clinical-impression-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/clinical-impression-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case DRAFT: return "The assessment is still on-going and results are not yet final."; 108 case COMPLETED: return "The assessment is done and the results are final."; 109 case ENTEREDINERROR: return "This assessment was never actually done and the record is erroneous (e.g. Wrong patient)."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case DRAFT: return "In progress"; 117 case COMPLETED: return "Completed"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class ClinicalImpressionStatusEnumFactory implements EnumFactory<ClinicalImpressionStatus> { 126 public ClinicalImpressionStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("draft".equals(codeString)) 131 return ClinicalImpressionStatus.DRAFT; 132 if ("completed".equals(codeString)) 133 return ClinicalImpressionStatus.COMPLETED; 134 if ("entered-in-error".equals(codeString)) 135 return ClinicalImpressionStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown ClinicalImpressionStatus code '"+codeString+"'"); 137 } 138 public Enumeration<ClinicalImpressionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<ClinicalImpressionStatus>(this); 143 String codeString = code.asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("draft".equals(codeString)) 147 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.DRAFT); 148 if ("completed".equals(codeString)) 149 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.COMPLETED); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.ENTEREDINERROR); 152 throw new FHIRException("Unknown ClinicalImpressionStatus code '"+codeString+"'"); 153 } 154 public String toCode(ClinicalImpressionStatus code) { 155 if (code == ClinicalImpressionStatus.NULL) 156 return null; 157 if (code == ClinicalImpressionStatus.DRAFT) 158 return "draft"; 159 if (code == ClinicalImpressionStatus.COMPLETED) 160 return "completed"; 161 if (code == ClinicalImpressionStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(ClinicalImpressionStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class ClinicalImpressionInvestigationComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * A name/code for the group ("set") of investigations. Typically, this will be something like "signs", "symptoms", "clinical", "diagnostic", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used. 174 */ 175 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="A name/code for the set", formalDefinition="A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/investigation-sets") 178 protected CodeableConcept code; 179 180 /** 181 * A record of a specific investigation that was undertaken. 182 */ 183 @Child(name = "item", type = {Observation.class, QuestionnaireResponse.class, FamilyMemberHistory.class, DiagnosticReport.class, RiskAssessment.class, ImagingStudy.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 184 @Description(shortDefinition="Record of a specific investigation", formalDefinition="A record of a specific investigation that was undertaken." ) 185 protected List<Reference> item; 186 /** 187 * The actual objects that are the target of the reference (A record of a specific investigation that was undertaken.) 188 */ 189 protected List<Resource> itemTarget; 190 191 192 private static final long serialVersionUID = -301363326L; 193 194 /** 195 * Constructor 196 */ 197 public ClinicalImpressionInvestigationComponent() { 198 super(); 199 } 200 201 /** 202 * Constructor 203 */ 204 public ClinicalImpressionInvestigationComponent(CodeableConcept code) { 205 super(); 206 this.code = code; 207 } 208 209 /** 210 * @return {@link #code} (A name/code for the group ("set") of investigations. Typically, this will be something like "signs", "symptoms", "clinical", "diagnostic", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used.) 211 */ 212 public CodeableConcept getCode() { 213 if (this.code == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create ClinicalImpressionInvestigationComponent.code"); 216 else if (Configuration.doAutoCreate()) 217 this.code = new CodeableConcept(); // cc 218 return this.code; 219 } 220 221 public boolean hasCode() { 222 return this.code != null && !this.code.isEmpty(); 223 } 224 225 /** 226 * @param value {@link #code} (A name/code for the group ("set") of investigations. Typically, this will be something like "signs", "symptoms", "clinical", "diagnostic", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used.) 227 */ 228 public ClinicalImpressionInvestigationComponent setCode(CodeableConcept value) { 229 this.code = value; 230 return this; 231 } 232 233 /** 234 * @return {@link #item} (A record of a specific investigation that was undertaken.) 235 */ 236 public List<Reference> getItem() { 237 if (this.item == null) 238 this.item = new ArrayList<Reference>(); 239 return this.item; 240 } 241 242 /** 243 * @return Returns a reference to <code>this</code> for easy method chaining 244 */ 245 public ClinicalImpressionInvestigationComponent setItem(List<Reference> theItem) { 246 this.item = theItem; 247 return this; 248 } 249 250 public boolean hasItem() { 251 if (this.item == null) 252 return false; 253 for (Reference item : this.item) 254 if (!item.isEmpty()) 255 return true; 256 return false; 257 } 258 259 public Reference addItem() { //3 260 Reference t = new Reference(); 261 if (this.item == null) 262 this.item = new ArrayList<Reference>(); 263 this.item.add(t); 264 return t; 265 } 266 267 public ClinicalImpressionInvestigationComponent addItem(Reference t) { //3 268 if (t == null) 269 return this; 270 if (this.item == null) 271 this.item = new ArrayList<Reference>(); 272 this.item.add(t); 273 return this; 274 } 275 276 /** 277 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 278 */ 279 public Reference getItemFirstRep() { 280 if (getItem().isEmpty()) { 281 addItem(); 282 } 283 return getItem().get(0); 284 } 285 286 /** 287 * @deprecated Use Reference#setResource(IBaseResource) instead 288 */ 289 @Deprecated 290 public List<Resource> getItemTarget() { 291 if (this.itemTarget == null) 292 this.itemTarget = new ArrayList<Resource>(); 293 return this.itemTarget; 294 } 295 296 protected void listChildren(List<Property> children) { 297 super.listChildren(children); 298 children.add(new Property("code", "CodeableConcept", "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used.", 0, 1, code)); 299 children.add(new Property("item", "Reference(Observation|QuestionnaireResponse|FamilyMemberHistory|DiagnosticReport|RiskAssessment|ImagingStudy)", "A record of a specific investigation that was undertaken.", 0, java.lang.Integer.MAX_VALUE, item)); 300 } 301 302 @Override 303 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 304 switch (_hash) { 305 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used.", 0, 1, code); 306 case 3242771: /*item*/ return new Property("item", "Reference(Observation|QuestionnaireResponse|FamilyMemberHistory|DiagnosticReport|RiskAssessment|ImagingStudy)", "A record of a specific investigation that was undertaken.", 0, java.lang.Integer.MAX_VALUE, item); 307 default: return super.getNamedProperty(_hash, _name, _checkValid); 308 } 309 310 } 311 312 @Override 313 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 314 switch (hash) { 315 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 316 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // Reference 317 default: return super.getProperty(hash, name, checkValid); 318 } 319 320 } 321 322 @Override 323 public Base setProperty(int hash, String name, Base value) throws FHIRException { 324 switch (hash) { 325 case 3059181: // code 326 this.code = castToCodeableConcept(value); // CodeableConcept 327 return value; 328 case 3242771: // item 329 this.getItem().add(castToReference(value)); // Reference 330 return value; 331 default: return super.setProperty(hash, name, value); 332 } 333 334 } 335 336 @Override 337 public Base setProperty(String name, Base value) throws FHIRException { 338 if (name.equals("code")) { 339 this.code = castToCodeableConcept(value); // CodeableConcept 340 } else if (name.equals("item")) { 341 this.getItem().add(castToReference(value)); 342 } else 343 return super.setProperty(name, value); 344 return value; 345 } 346 347 @Override 348 public Base makeProperty(int hash, String name) throws FHIRException { 349 switch (hash) { 350 case 3059181: return getCode(); 351 case 3242771: return addItem(); 352 default: return super.makeProperty(hash, name); 353 } 354 355 } 356 357 @Override 358 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 359 switch (hash) { 360 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 361 case 3242771: /*item*/ return new String[] {"Reference"}; 362 default: return super.getTypesForProperty(hash, name); 363 } 364 365 } 366 367 @Override 368 public Base addChild(String name) throws FHIRException { 369 if (name.equals("code")) { 370 this.code = new CodeableConcept(); 371 return this.code; 372 } 373 else if (name.equals("item")) { 374 return addItem(); 375 } 376 else 377 return super.addChild(name); 378 } 379 380 public ClinicalImpressionInvestigationComponent copy() { 381 ClinicalImpressionInvestigationComponent dst = new ClinicalImpressionInvestigationComponent(); 382 copyValues(dst); 383 dst.code = code == null ? null : code.copy(); 384 if (item != null) { 385 dst.item = new ArrayList<Reference>(); 386 for (Reference i : item) 387 dst.item.add(i.copy()); 388 }; 389 return dst; 390 } 391 392 @Override 393 public boolean equalsDeep(Base other_) { 394 if (!super.equalsDeep(other_)) 395 return false; 396 if (!(other_ instanceof ClinicalImpressionInvestigationComponent)) 397 return false; 398 ClinicalImpressionInvestigationComponent o = (ClinicalImpressionInvestigationComponent) other_; 399 return compareDeep(code, o.code, true) && compareDeep(item, o.item, true); 400 } 401 402 @Override 403 public boolean equalsShallow(Base other_) { 404 if (!super.equalsShallow(other_)) 405 return false; 406 if (!(other_ instanceof ClinicalImpressionInvestigationComponent)) 407 return false; 408 ClinicalImpressionInvestigationComponent o = (ClinicalImpressionInvestigationComponent) other_; 409 return true; 410 } 411 412 public boolean isEmpty() { 413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, item); 414 } 415 416 public String fhirType() { 417 return "ClinicalImpression.investigation"; 418 419 } 420 421 } 422 423 @Block() 424 public static class ClinicalImpressionFindingComponent extends BackboneElement implements IBaseBackboneElement { 425 /** 426 * Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions. 427 */ 428 @Child(name = "item", type = {CodeableConcept.class, Condition.class, Observation.class}, order=1, min=1, max=1, modifier=false, summary=false) 429 @Description(shortDefinition="What was found", formalDefinition="Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions." ) 430 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 431 protected Type item; 432 433 /** 434 * Which investigations support finding or diagnosis. 435 */ 436 @Child(name = "basis", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 437 @Description(shortDefinition="Which investigations support finding", formalDefinition="Which investigations support finding or diagnosis." ) 438 protected StringType basis; 439 440 private static final long serialVersionUID = 1690728236L; 441 442 /** 443 * Constructor 444 */ 445 public ClinicalImpressionFindingComponent() { 446 super(); 447 } 448 449 /** 450 * Constructor 451 */ 452 public ClinicalImpressionFindingComponent(Type item) { 453 super(); 454 this.item = item; 455 } 456 457 /** 458 * @return {@link #item} (Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.) 459 */ 460 public Type getItem() { 461 return this.item; 462 } 463 464 /** 465 * @return {@link #item} (Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.) 466 */ 467 public CodeableConcept getItemCodeableConcept() throws FHIRException { 468 if (this.item == null) 469 return null; 470 if (!(this.item instanceof CodeableConcept)) 471 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 472 return (CodeableConcept) this.item; 473 } 474 475 public boolean hasItemCodeableConcept() { 476 return this != null && this.item instanceof CodeableConcept; 477 } 478 479 /** 480 * @return {@link #item} (Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.) 481 */ 482 public Reference getItemReference() throws FHIRException { 483 if (this.item == null) 484 return null; 485 if (!(this.item instanceof Reference)) 486 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 487 return (Reference) this.item; 488 } 489 490 public boolean hasItemReference() { 491 return this != null && this.item instanceof Reference; 492 } 493 494 public boolean hasItem() { 495 return this.item != null && !this.item.isEmpty(); 496 } 497 498 /** 499 * @param value {@link #item} (Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.) 500 */ 501 public ClinicalImpressionFindingComponent setItem(Type value) throws FHIRFormatError { 502 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 503 throw new FHIRFormatError("Not the right type for ClinicalImpression.finding.item[x]: "+value.fhirType()); 504 this.item = value; 505 return this; 506 } 507 508 /** 509 * @return {@link #basis} (Which investigations support finding or diagnosis.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 510 */ 511 public StringType getBasisElement() { 512 if (this.basis == null) 513 if (Configuration.errorOnAutoCreate()) 514 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.basis"); 515 else if (Configuration.doAutoCreate()) 516 this.basis = new StringType(); // bb 517 return this.basis; 518 } 519 520 public boolean hasBasisElement() { 521 return this.basis != null && !this.basis.isEmpty(); 522 } 523 524 public boolean hasBasis() { 525 return this.basis != null && !this.basis.isEmpty(); 526 } 527 528 /** 529 * @param value {@link #basis} (Which investigations support finding or diagnosis.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 530 */ 531 public ClinicalImpressionFindingComponent setBasisElement(StringType value) { 532 this.basis = value; 533 return this; 534 } 535 536 /** 537 * @return Which investigations support finding or diagnosis. 538 */ 539 public String getBasis() { 540 return this.basis == null ? null : this.basis.getValue(); 541 } 542 543 /** 544 * @param value Which investigations support finding or diagnosis. 545 */ 546 public ClinicalImpressionFindingComponent setBasis(String value) { 547 if (Utilities.noString(value)) 548 this.basis = null; 549 else { 550 if (this.basis == null) 551 this.basis = new StringType(); 552 this.basis.setValue(value); 553 } 554 return this; 555 } 556 557 protected void listChildren(List<Property> children) { 558 super.listChildren(children); 559 children.add(new Property("item[x]", "CodeableConcept|Reference(Condition|Observation)", "Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, item)); 560 children.add(new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, basis)); 561 } 562 563 @Override 564 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 565 switch (_hash) { 566 case 2116201613: /*item[x]*/ return new Property("item[x]", "CodeableConcept|Reference(Condition|Observation)", "Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, item); 567 case 3242771: /*item*/ return new Property("item[x]", "CodeableConcept|Reference(Condition|Observation)", "Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, item); 568 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept|Reference(Condition|Observation)", "Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, item); 569 case 1376364920: /*itemReference*/ return new Property("item[x]", "CodeableConcept|Reference(Condition|Observation)", "Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, item); 570 case 93508670: /*basis*/ return new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, basis); 571 default: return super.getNamedProperty(_hash, _name, _checkValid); 572 } 573 574 } 575 576 @Override 577 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 578 switch (hash) { 579 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // Type 580 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : new Base[] {this.basis}; // StringType 581 default: return super.getProperty(hash, name, checkValid); 582 } 583 584 } 585 586 @Override 587 public Base setProperty(int hash, String name, Base value) throws FHIRException { 588 switch (hash) { 589 case 3242771: // item 590 this.item = castToType(value); // Type 591 return value; 592 case 93508670: // basis 593 this.basis = castToString(value); // StringType 594 return value; 595 default: return super.setProperty(hash, name, value); 596 } 597 598 } 599 600 @Override 601 public Base setProperty(String name, Base value) throws FHIRException { 602 if (name.equals("item[x]")) { 603 this.item = castToType(value); // Type 604 } else if (name.equals("basis")) { 605 this.basis = castToString(value); // StringType 606 } else 607 return super.setProperty(name, value); 608 return value; 609 } 610 611 @Override 612 public Base makeProperty(int hash, String name) throws FHIRException { 613 switch (hash) { 614 case 2116201613: return getItem(); 615 case 3242771: return getItem(); 616 case 93508670: return getBasisElement(); 617 default: return super.makeProperty(hash, name); 618 } 619 620 } 621 622 @Override 623 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 624 switch (hash) { 625 case 3242771: /*item*/ return new String[] {"CodeableConcept", "Reference"}; 626 case 93508670: /*basis*/ return new String[] {"string"}; 627 default: return super.getTypesForProperty(hash, name); 628 } 629 630 } 631 632 @Override 633 public Base addChild(String name) throws FHIRException { 634 if (name.equals("itemCodeableConcept")) { 635 this.item = new CodeableConcept(); 636 return this.item; 637 } 638 else if (name.equals("itemReference")) { 639 this.item = new Reference(); 640 return this.item; 641 } 642 else if (name.equals("basis")) { 643 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.basis"); 644 } 645 else 646 return super.addChild(name); 647 } 648 649 public ClinicalImpressionFindingComponent copy() { 650 ClinicalImpressionFindingComponent dst = new ClinicalImpressionFindingComponent(); 651 copyValues(dst); 652 dst.item = item == null ? null : item.copy(); 653 dst.basis = basis == null ? null : basis.copy(); 654 return dst; 655 } 656 657 @Override 658 public boolean equalsDeep(Base other_) { 659 if (!super.equalsDeep(other_)) 660 return false; 661 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 662 return false; 663 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 664 return compareDeep(item, o.item, true) && compareDeep(basis, o.basis, true); 665 } 666 667 @Override 668 public boolean equalsShallow(Base other_) { 669 if (!super.equalsShallow(other_)) 670 return false; 671 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 672 return false; 673 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 674 return compareValues(basis, o.basis, true); 675 } 676 677 public boolean isEmpty() { 678 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, basis); 679 } 680 681 public String fhirType() { 682 return "ClinicalImpression.finding"; 683 684 } 685 686 } 687 688 /** 689 * A unique identifier assigned to the clinical impression that remains consistent regardless of what server the impression is stored on. 690 */ 691 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 692 @Description(shortDefinition="Business identifier", formalDefinition="A unique identifier assigned to the clinical impression that remains consistent regardless of what server the impression is stored on." ) 693 protected List<Identifier> identifier; 694 695 /** 696 * Identifies the workflow status of the assessment. 697 */ 698 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 699 @Description(shortDefinition="draft | completed | entered-in-error", formalDefinition="Identifies the workflow status of the assessment." ) 700 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-impression-status") 701 protected Enumeration<ClinicalImpressionStatus> status; 702 703 /** 704 * Categorizes the type of clinical assessment performed. 705 */ 706 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 707 @Description(shortDefinition="Kind of assessment performed", formalDefinition="Categorizes the type of clinical assessment performed." ) 708 protected CodeableConcept code; 709 710 /** 711 * A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it. 712 */ 713 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 714 @Description(shortDefinition="Why/how the assessment was performed", formalDefinition="A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it." ) 715 protected StringType description; 716 717 /** 718 * The patient or group of individuals assessed as part of this record. 719 */ 720 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=1, max=1, modifier=false, summary=true) 721 @Description(shortDefinition="Patient or group assessed", formalDefinition="The patient or group of individuals assessed as part of this record." ) 722 protected Reference subject; 723 724 /** 725 * The actual object that is the target of the reference (The patient or group of individuals assessed as part of this record.) 726 */ 727 protected Resource subjectTarget; 728 729 /** 730 * The encounter or episode of care this impression was created as part of. 731 */ 732 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=5, min=0, max=1, modifier=false, summary=true) 733 @Description(shortDefinition="Encounter or Episode created from", formalDefinition="The encounter or episode of care this impression was created as part of." ) 734 protected Reference context; 735 736 /** 737 * The actual object that is the target of the reference (The encounter or episode of care this impression was created as part of.) 738 */ 739 protected Resource contextTarget; 740 741 /** 742 * The point in time or period over which the subject was assessed. 743 */ 744 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 745 @Description(shortDefinition="Time of assessment", formalDefinition="The point in time or period over which the subject was assessed." ) 746 protected Type effective; 747 748 /** 749 * Indicates when the documentation of the assessment was complete. 750 */ 751 @Child(name = "date", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 752 @Description(shortDefinition="When the assessment was documented", formalDefinition="Indicates when the documentation of the assessment was complete." ) 753 protected DateTimeType date; 754 755 /** 756 * The clinician performing the assessment. 757 */ 758 @Child(name = "assessor", type = {Practitioner.class}, order=8, min=0, max=1, modifier=false, summary=true) 759 @Description(shortDefinition="The clinician performing the assessment", formalDefinition="The clinician performing the assessment." ) 760 protected Reference assessor; 761 762 /** 763 * The actual object that is the target of the reference (The clinician performing the assessment.) 764 */ 765 protected Practitioner assessorTarget; 766 767 /** 768 * A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes. 769 */ 770 @Child(name = "previous", type = {ClinicalImpression.class}, order=9, min=0, max=1, modifier=false, summary=false) 771 @Description(shortDefinition="Reference to last assessment", formalDefinition="A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes." ) 772 protected Reference previous; 773 774 /** 775 * The actual object that is the target of the reference (A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.) 776 */ 777 protected ClinicalImpression previousTarget; 778 779 /** 780 * This a list of the relevant problems/conditions for a patient. 781 */ 782 @Child(name = "problem", type = {Condition.class, AllergyIntolerance.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 783 @Description(shortDefinition="Relevant impressions of patient state", formalDefinition="This a list of the relevant problems/conditions for a patient." ) 784 protected List<Reference> problem; 785 /** 786 * The actual objects that are the target of the reference (This a list of the relevant problems/conditions for a patient.) 787 */ 788 protected List<Resource> problemTarget; 789 790 791 /** 792 * One or more sets of investigations (signs, symptions, etc.). The actual grouping of investigations vary greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes. 793 */ 794 @Child(name = "investigation", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 795 @Description(shortDefinition="One or more sets of investigations (signs, symptions, etc.)", formalDefinition="One or more sets of investigations (signs, symptions, etc.). The actual grouping of investigations vary greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes." ) 796 protected List<ClinicalImpressionInvestigationComponent> investigation; 797 798 /** 799 * Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis. 800 */ 801 @Child(name = "protocol", type = {UriType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 802 @Description(shortDefinition="Clinical Protocol followed", formalDefinition="Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis." ) 803 protected List<UriType> protocol; 804 805 /** 806 * A text summary of the investigations and the diagnosis. 807 */ 808 @Child(name = "summary", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 809 @Description(shortDefinition="Summary of the assessment", formalDefinition="A text summary of the investigations and the diagnosis." ) 810 protected StringType summary; 811 812 /** 813 * Specific findings or diagnoses that was considered likely or relevant to ongoing treatment. 814 */ 815 @Child(name = "finding", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 816 @Description(shortDefinition="Possible or likely findings and diagnoses", formalDefinition="Specific findings or diagnoses that was considered likely or relevant to ongoing treatment." ) 817 protected List<ClinicalImpressionFindingComponent> finding; 818 819 /** 820 * Estimate of likely outcome. 821 */ 822 @Child(name = "prognosisCodeableConcept", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 823 @Description(shortDefinition="Estimate of likely outcome", formalDefinition="Estimate of likely outcome." ) 824 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinicalimpression-prognosis") 825 protected List<CodeableConcept> prognosisCodeableConcept; 826 827 /** 828 * RiskAssessment expressing likely outcome. 829 */ 830 @Child(name = "prognosisReference", type = {RiskAssessment.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 831 @Description(shortDefinition="RiskAssessment expressing likely outcome", formalDefinition="RiskAssessment expressing likely outcome." ) 832 protected List<Reference> prognosisReference; 833 /** 834 * The actual objects that are the target of the reference (RiskAssessment expressing likely outcome.) 835 */ 836 protected List<RiskAssessment> prognosisReferenceTarget; 837 838 839 /** 840 * Action taken as part of assessment procedure. 841 */ 842 @Child(name = "action", type = {ReferralRequest.class, ProcedureRequest.class, Procedure.class, MedicationRequest.class, Appointment.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 843 @Description(shortDefinition="Action taken as part of assessment procedure", formalDefinition="Action taken as part of assessment procedure." ) 844 protected List<Reference> action; 845 /** 846 * The actual objects that are the target of the reference (Action taken as part of assessment procedure.) 847 */ 848 protected List<Resource> actionTarget; 849 850 851 /** 852 * Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear. 853 */ 854 @Child(name = "note", type = {Annotation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 855 @Description(shortDefinition="Comments made about the ClinicalImpression", formalDefinition="Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear." ) 856 protected List<Annotation> note; 857 858 private static final long serialVersionUID = -1626670747L; 859 860 /** 861 * Constructor 862 */ 863 public ClinicalImpression() { 864 super(); 865 } 866 867 /** 868 * Constructor 869 */ 870 public ClinicalImpression(Enumeration<ClinicalImpressionStatus> status, Reference subject) { 871 super(); 872 this.status = status; 873 this.subject = subject; 874 } 875 876 /** 877 * @return {@link #identifier} (A unique identifier assigned to the clinical impression that remains consistent regardless of what server the impression is stored on.) 878 */ 879 public List<Identifier> getIdentifier() { 880 if (this.identifier == null) 881 this.identifier = new ArrayList<Identifier>(); 882 return this.identifier; 883 } 884 885 /** 886 * @return Returns a reference to <code>this</code> for easy method chaining 887 */ 888 public ClinicalImpression setIdentifier(List<Identifier> theIdentifier) { 889 this.identifier = theIdentifier; 890 return this; 891 } 892 893 public boolean hasIdentifier() { 894 if (this.identifier == null) 895 return false; 896 for (Identifier item : this.identifier) 897 if (!item.isEmpty()) 898 return true; 899 return false; 900 } 901 902 public Identifier addIdentifier() { //3 903 Identifier t = new Identifier(); 904 if (this.identifier == null) 905 this.identifier = new ArrayList<Identifier>(); 906 this.identifier.add(t); 907 return t; 908 } 909 910 public ClinicalImpression addIdentifier(Identifier t) { //3 911 if (t == null) 912 return this; 913 if (this.identifier == null) 914 this.identifier = new ArrayList<Identifier>(); 915 this.identifier.add(t); 916 return this; 917 } 918 919 /** 920 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 921 */ 922 public Identifier getIdentifierFirstRep() { 923 if (getIdentifier().isEmpty()) { 924 addIdentifier(); 925 } 926 return getIdentifier().get(0); 927 } 928 929 /** 930 * @return {@link #status} (Identifies the workflow status of the assessment.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 931 */ 932 public Enumeration<ClinicalImpressionStatus> getStatusElement() { 933 if (this.status == null) 934 if (Configuration.errorOnAutoCreate()) 935 throw new Error("Attempt to auto-create ClinicalImpression.status"); 936 else if (Configuration.doAutoCreate()) 937 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); // bb 938 return this.status; 939 } 940 941 public boolean hasStatusElement() { 942 return this.status != null && !this.status.isEmpty(); 943 } 944 945 public boolean hasStatus() { 946 return this.status != null && !this.status.isEmpty(); 947 } 948 949 /** 950 * @param value {@link #status} (Identifies the workflow status of the assessment.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 951 */ 952 public ClinicalImpression setStatusElement(Enumeration<ClinicalImpressionStatus> value) { 953 this.status = value; 954 return this; 955 } 956 957 /** 958 * @return Identifies the workflow status of the assessment. 959 */ 960 public ClinicalImpressionStatus getStatus() { 961 return this.status == null ? null : this.status.getValue(); 962 } 963 964 /** 965 * @param value Identifies the workflow status of the assessment. 966 */ 967 public ClinicalImpression setStatus(ClinicalImpressionStatus value) { 968 if (this.status == null) 969 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); 970 this.status.setValue(value); 971 return this; 972 } 973 974 /** 975 * @return {@link #code} (Categorizes the type of clinical assessment performed.) 976 */ 977 public CodeableConcept getCode() { 978 if (this.code == null) 979 if (Configuration.errorOnAutoCreate()) 980 throw new Error("Attempt to auto-create ClinicalImpression.code"); 981 else if (Configuration.doAutoCreate()) 982 this.code = new CodeableConcept(); // cc 983 return this.code; 984 } 985 986 public boolean hasCode() { 987 return this.code != null && !this.code.isEmpty(); 988 } 989 990 /** 991 * @param value {@link #code} (Categorizes the type of clinical assessment performed.) 992 */ 993 public ClinicalImpression setCode(CodeableConcept value) { 994 this.code = value; 995 return this; 996 } 997 998 /** 999 * @return {@link #description} (A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1000 */ 1001 public StringType getDescriptionElement() { 1002 if (this.description == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create ClinicalImpression.description"); 1005 else if (Configuration.doAutoCreate()) 1006 this.description = new StringType(); // bb 1007 return this.description; 1008 } 1009 1010 public boolean hasDescriptionElement() { 1011 return this.description != null && !this.description.isEmpty(); 1012 } 1013 1014 public boolean hasDescription() { 1015 return this.description != null && !this.description.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #description} (A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1020 */ 1021 public ClinicalImpression setDescriptionElement(StringType value) { 1022 this.description = value; 1023 return this; 1024 } 1025 1026 /** 1027 * @return A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it. 1028 */ 1029 public String getDescription() { 1030 return this.description == null ? null : this.description.getValue(); 1031 } 1032 1033 /** 1034 * @param value A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it. 1035 */ 1036 public ClinicalImpression setDescription(String value) { 1037 if (Utilities.noString(value)) 1038 this.description = null; 1039 else { 1040 if (this.description == null) 1041 this.description = new StringType(); 1042 this.description.setValue(value); 1043 } 1044 return this; 1045 } 1046 1047 /** 1048 * @return {@link #subject} (The patient or group of individuals assessed as part of this record.) 1049 */ 1050 public Reference getSubject() { 1051 if (this.subject == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create ClinicalImpression.subject"); 1054 else if (Configuration.doAutoCreate()) 1055 this.subject = new Reference(); // cc 1056 return this.subject; 1057 } 1058 1059 public boolean hasSubject() { 1060 return this.subject != null && !this.subject.isEmpty(); 1061 } 1062 1063 /** 1064 * @param value {@link #subject} (The patient or group of individuals assessed as part of this record.) 1065 */ 1066 public ClinicalImpression setSubject(Reference value) { 1067 this.subject = value; 1068 return this; 1069 } 1070 1071 /** 1072 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient or group of individuals assessed as part of this record.) 1073 */ 1074 public Resource getSubjectTarget() { 1075 return this.subjectTarget; 1076 } 1077 1078 /** 1079 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient or group of individuals assessed as part of this record.) 1080 */ 1081 public ClinicalImpression setSubjectTarget(Resource value) { 1082 this.subjectTarget = value; 1083 return this; 1084 } 1085 1086 /** 1087 * @return {@link #context} (The encounter or episode of care this impression was created as part of.) 1088 */ 1089 public Reference getContext() { 1090 if (this.context == null) 1091 if (Configuration.errorOnAutoCreate()) 1092 throw new Error("Attempt to auto-create ClinicalImpression.context"); 1093 else if (Configuration.doAutoCreate()) 1094 this.context = new Reference(); // cc 1095 return this.context; 1096 } 1097 1098 public boolean hasContext() { 1099 return this.context != null && !this.context.isEmpty(); 1100 } 1101 1102 /** 1103 * @param value {@link #context} (The encounter or episode of care this impression was created as part of.) 1104 */ 1105 public ClinicalImpression setContext(Reference value) { 1106 this.context = value; 1107 return this; 1108 } 1109 1110 /** 1111 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care this impression was created as part of.) 1112 */ 1113 public Resource getContextTarget() { 1114 return this.contextTarget; 1115 } 1116 1117 /** 1118 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care this impression was created as part of.) 1119 */ 1120 public ClinicalImpression setContextTarget(Resource value) { 1121 this.contextTarget = value; 1122 return this; 1123 } 1124 1125 /** 1126 * @return {@link #effective} (The point in time or period over which the subject was assessed.) 1127 */ 1128 public Type getEffective() { 1129 return this.effective; 1130 } 1131 1132 /** 1133 * @return {@link #effective} (The point in time or period over which the subject was assessed.) 1134 */ 1135 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1136 if (this.effective == null) 1137 return null; 1138 if (!(this.effective instanceof DateTimeType)) 1139 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 1140 return (DateTimeType) this.effective; 1141 } 1142 1143 public boolean hasEffectiveDateTimeType() { 1144 return this != null && this.effective instanceof DateTimeType; 1145 } 1146 1147 /** 1148 * @return {@link #effective} (The point in time or period over which the subject was assessed.) 1149 */ 1150 public Period getEffectivePeriod() throws FHIRException { 1151 if (this.effective == null) 1152 return null; 1153 if (!(this.effective instanceof Period)) 1154 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 1155 return (Period) this.effective; 1156 } 1157 1158 public boolean hasEffectivePeriod() { 1159 return this != null && this.effective instanceof Period; 1160 } 1161 1162 public boolean hasEffective() { 1163 return this.effective != null && !this.effective.isEmpty(); 1164 } 1165 1166 /** 1167 * @param value {@link #effective} (The point in time or period over which the subject was assessed.) 1168 */ 1169 public ClinicalImpression setEffective(Type value) throws FHIRFormatError { 1170 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1171 throw new FHIRFormatError("Not the right type for ClinicalImpression.effective[x]: "+value.fhirType()); 1172 this.effective = value; 1173 return this; 1174 } 1175 1176 /** 1177 * @return {@link #date} (Indicates when the documentation of the assessment was complete.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1178 */ 1179 public DateTimeType getDateElement() { 1180 if (this.date == null) 1181 if (Configuration.errorOnAutoCreate()) 1182 throw new Error("Attempt to auto-create ClinicalImpression.date"); 1183 else if (Configuration.doAutoCreate()) 1184 this.date = new DateTimeType(); // bb 1185 return this.date; 1186 } 1187 1188 public boolean hasDateElement() { 1189 return this.date != null && !this.date.isEmpty(); 1190 } 1191 1192 public boolean hasDate() { 1193 return this.date != null && !this.date.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #date} (Indicates when the documentation of the assessment was complete.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1198 */ 1199 public ClinicalImpression setDateElement(DateTimeType value) { 1200 this.date = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return Indicates when the documentation of the assessment was complete. 1206 */ 1207 public Date getDate() { 1208 return this.date == null ? null : this.date.getValue(); 1209 } 1210 1211 /** 1212 * @param value Indicates when the documentation of the assessment was complete. 1213 */ 1214 public ClinicalImpression setDate(Date value) { 1215 if (value == null) 1216 this.date = null; 1217 else { 1218 if (this.date == null) 1219 this.date = new DateTimeType(); 1220 this.date.setValue(value); 1221 } 1222 return this; 1223 } 1224 1225 /** 1226 * @return {@link #assessor} (The clinician performing the assessment.) 1227 */ 1228 public Reference getAssessor() { 1229 if (this.assessor == null) 1230 if (Configuration.errorOnAutoCreate()) 1231 throw new Error("Attempt to auto-create ClinicalImpression.assessor"); 1232 else if (Configuration.doAutoCreate()) 1233 this.assessor = new Reference(); // cc 1234 return this.assessor; 1235 } 1236 1237 public boolean hasAssessor() { 1238 return this.assessor != null && !this.assessor.isEmpty(); 1239 } 1240 1241 /** 1242 * @param value {@link #assessor} (The clinician performing the assessment.) 1243 */ 1244 public ClinicalImpression setAssessor(Reference value) { 1245 this.assessor = value; 1246 return this; 1247 } 1248 1249 /** 1250 * @return {@link #assessor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The clinician performing the assessment.) 1251 */ 1252 public Practitioner getAssessorTarget() { 1253 if (this.assessorTarget == null) 1254 if (Configuration.errorOnAutoCreate()) 1255 throw new Error("Attempt to auto-create ClinicalImpression.assessor"); 1256 else if (Configuration.doAutoCreate()) 1257 this.assessorTarget = new Practitioner(); // aa 1258 return this.assessorTarget; 1259 } 1260 1261 /** 1262 * @param value {@link #assessor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The clinician performing the assessment.) 1263 */ 1264 public ClinicalImpression setAssessorTarget(Practitioner value) { 1265 this.assessorTarget = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return {@link #previous} (A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.) 1271 */ 1272 public Reference getPrevious() { 1273 if (this.previous == null) 1274 if (Configuration.errorOnAutoCreate()) 1275 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1276 else if (Configuration.doAutoCreate()) 1277 this.previous = new Reference(); // cc 1278 return this.previous; 1279 } 1280 1281 public boolean hasPrevious() { 1282 return this.previous != null && !this.previous.isEmpty(); 1283 } 1284 1285 /** 1286 * @param value {@link #previous} (A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.) 1287 */ 1288 public ClinicalImpression setPrevious(Reference value) { 1289 this.previous = value; 1290 return this; 1291 } 1292 1293 /** 1294 * @return {@link #previous} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.) 1295 */ 1296 public ClinicalImpression getPreviousTarget() { 1297 if (this.previousTarget == null) 1298 if (Configuration.errorOnAutoCreate()) 1299 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1300 else if (Configuration.doAutoCreate()) 1301 this.previousTarget = new ClinicalImpression(); // aa 1302 return this.previousTarget; 1303 } 1304 1305 /** 1306 * @param value {@link #previous} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.) 1307 */ 1308 public ClinicalImpression setPreviousTarget(ClinicalImpression value) { 1309 this.previousTarget = value; 1310 return this; 1311 } 1312 1313 /** 1314 * @return {@link #problem} (This a list of the relevant problems/conditions for a patient.) 1315 */ 1316 public List<Reference> getProblem() { 1317 if (this.problem == null) 1318 this.problem = new ArrayList<Reference>(); 1319 return this.problem; 1320 } 1321 1322 /** 1323 * @return Returns a reference to <code>this</code> for easy method chaining 1324 */ 1325 public ClinicalImpression setProblem(List<Reference> theProblem) { 1326 this.problem = theProblem; 1327 return this; 1328 } 1329 1330 public boolean hasProblem() { 1331 if (this.problem == null) 1332 return false; 1333 for (Reference item : this.problem) 1334 if (!item.isEmpty()) 1335 return true; 1336 return false; 1337 } 1338 1339 public Reference addProblem() { //3 1340 Reference t = new Reference(); 1341 if (this.problem == null) 1342 this.problem = new ArrayList<Reference>(); 1343 this.problem.add(t); 1344 return t; 1345 } 1346 1347 public ClinicalImpression addProblem(Reference t) { //3 1348 if (t == null) 1349 return this; 1350 if (this.problem == null) 1351 this.problem = new ArrayList<Reference>(); 1352 this.problem.add(t); 1353 return this; 1354 } 1355 1356 /** 1357 * @return The first repetition of repeating field {@link #problem}, creating it if it does not already exist 1358 */ 1359 public Reference getProblemFirstRep() { 1360 if (getProblem().isEmpty()) { 1361 addProblem(); 1362 } 1363 return getProblem().get(0); 1364 } 1365 1366 /** 1367 * @deprecated Use Reference#setResource(IBaseResource) instead 1368 */ 1369 @Deprecated 1370 public List<Resource> getProblemTarget() { 1371 if (this.problemTarget == null) 1372 this.problemTarget = new ArrayList<Resource>(); 1373 return this.problemTarget; 1374 } 1375 1376 /** 1377 * @return {@link #investigation} (One or more sets of investigations (signs, symptions, etc.). The actual grouping of investigations vary greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.) 1378 */ 1379 public List<ClinicalImpressionInvestigationComponent> getInvestigation() { 1380 if (this.investigation == null) 1381 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1382 return this.investigation; 1383 } 1384 1385 /** 1386 * @return Returns a reference to <code>this</code> for easy method chaining 1387 */ 1388 public ClinicalImpression setInvestigation(List<ClinicalImpressionInvestigationComponent> theInvestigation) { 1389 this.investigation = theInvestigation; 1390 return this; 1391 } 1392 1393 public boolean hasInvestigation() { 1394 if (this.investigation == null) 1395 return false; 1396 for (ClinicalImpressionInvestigationComponent item : this.investigation) 1397 if (!item.isEmpty()) 1398 return true; 1399 return false; 1400 } 1401 1402 public ClinicalImpressionInvestigationComponent addInvestigation() { //3 1403 ClinicalImpressionInvestigationComponent t = new ClinicalImpressionInvestigationComponent(); 1404 if (this.investigation == null) 1405 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1406 this.investigation.add(t); 1407 return t; 1408 } 1409 1410 public ClinicalImpression addInvestigation(ClinicalImpressionInvestigationComponent t) { //3 1411 if (t == null) 1412 return this; 1413 if (this.investigation == null) 1414 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1415 this.investigation.add(t); 1416 return this; 1417 } 1418 1419 /** 1420 * @return The first repetition of repeating field {@link #investigation}, creating it if it does not already exist 1421 */ 1422 public ClinicalImpressionInvestigationComponent getInvestigationFirstRep() { 1423 if (getInvestigation().isEmpty()) { 1424 addInvestigation(); 1425 } 1426 return getInvestigation().get(0); 1427 } 1428 1429 /** 1430 * @return {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 1431 */ 1432 public List<UriType> getProtocol() { 1433 if (this.protocol == null) 1434 this.protocol = new ArrayList<UriType>(); 1435 return this.protocol; 1436 } 1437 1438 /** 1439 * @return Returns a reference to <code>this</code> for easy method chaining 1440 */ 1441 public ClinicalImpression setProtocol(List<UriType> theProtocol) { 1442 this.protocol = theProtocol; 1443 return this; 1444 } 1445 1446 public boolean hasProtocol() { 1447 if (this.protocol == null) 1448 return false; 1449 for (UriType item : this.protocol) 1450 if (!item.isEmpty()) 1451 return true; 1452 return false; 1453 } 1454 1455 /** 1456 * @return {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 1457 */ 1458 public UriType addProtocolElement() {//2 1459 UriType t = new UriType(); 1460 if (this.protocol == null) 1461 this.protocol = new ArrayList<UriType>(); 1462 this.protocol.add(t); 1463 return t; 1464 } 1465 1466 /** 1467 * @param value {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 1468 */ 1469 public ClinicalImpression addProtocol(String value) { //1 1470 UriType t = new UriType(); 1471 t.setValue(value); 1472 if (this.protocol == null) 1473 this.protocol = new ArrayList<UriType>(); 1474 this.protocol.add(t); 1475 return this; 1476 } 1477 1478 /** 1479 * @param value {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 1480 */ 1481 public boolean hasProtocol(String value) { 1482 if (this.protocol == null) 1483 return false; 1484 for (UriType v : this.protocol) 1485 if (v.getValue().equals(value)) // uri 1486 return true; 1487 return false; 1488 } 1489 1490 /** 1491 * @return {@link #summary} (A text summary of the investigations and the diagnosis.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 1492 */ 1493 public StringType getSummaryElement() { 1494 if (this.summary == null) 1495 if (Configuration.errorOnAutoCreate()) 1496 throw new Error("Attempt to auto-create ClinicalImpression.summary"); 1497 else if (Configuration.doAutoCreate()) 1498 this.summary = new StringType(); // bb 1499 return this.summary; 1500 } 1501 1502 public boolean hasSummaryElement() { 1503 return this.summary != null && !this.summary.isEmpty(); 1504 } 1505 1506 public boolean hasSummary() { 1507 return this.summary != null && !this.summary.isEmpty(); 1508 } 1509 1510 /** 1511 * @param value {@link #summary} (A text summary of the investigations and the diagnosis.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 1512 */ 1513 public ClinicalImpression setSummaryElement(StringType value) { 1514 this.summary = value; 1515 return this; 1516 } 1517 1518 /** 1519 * @return A text summary of the investigations and the diagnosis. 1520 */ 1521 public String getSummary() { 1522 return this.summary == null ? null : this.summary.getValue(); 1523 } 1524 1525 /** 1526 * @param value A text summary of the investigations and the diagnosis. 1527 */ 1528 public ClinicalImpression setSummary(String value) { 1529 if (Utilities.noString(value)) 1530 this.summary = null; 1531 else { 1532 if (this.summary == null) 1533 this.summary = new StringType(); 1534 this.summary.setValue(value); 1535 } 1536 return this; 1537 } 1538 1539 /** 1540 * @return {@link #finding} (Specific findings or diagnoses that was considered likely or relevant to ongoing treatment.) 1541 */ 1542 public List<ClinicalImpressionFindingComponent> getFinding() { 1543 if (this.finding == null) 1544 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1545 return this.finding; 1546 } 1547 1548 /** 1549 * @return Returns a reference to <code>this</code> for easy method chaining 1550 */ 1551 public ClinicalImpression setFinding(List<ClinicalImpressionFindingComponent> theFinding) { 1552 this.finding = theFinding; 1553 return this; 1554 } 1555 1556 public boolean hasFinding() { 1557 if (this.finding == null) 1558 return false; 1559 for (ClinicalImpressionFindingComponent item : this.finding) 1560 if (!item.isEmpty()) 1561 return true; 1562 return false; 1563 } 1564 1565 public ClinicalImpressionFindingComponent addFinding() { //3 1566 ClinicalImpressionFindingComponent t = new ClinicalImpressionFindingComponent(); 1567 if (this.finding == null) 1568 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1569 this.finding.add(t); 1570 return t; 1571 } 1572 1573 public ClinicalImpression addFinding(ClinicalImpressionFindingComponent t) { //3 1574 if (t == null) 1575 return this; 1576 if (this.finding == null) 1577 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1578 this.finding.add(t); 1579 return this; 1580 } 1581 1582 /** 1583 * @return The first repetition of repeating field {@link #finding}, creating it if it does not already exist 1584 */ 1585 public ClinicalImpressionFindingComponent getFindingFirstRep() { 1586 if (getFinding().isEmpty()) { 1587 addFinding(); 1588 } 1589 return getFinding().get(0); 1590 } 1591 1592 /** 1593 * @return {@link #prognosisCodeableConcept} (Estimate of likely outcome.) 1594 */ 1595 public List<CodeableConcept> getPrognosisCodeableConcept() { 1596 if (this.prognosisCodeableConcept == null) 1597 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1598 return this.prognosisCodeableConcept; 1599 } 1600 1601 /** 1602 * @return Returns a reference to <code>this</code> for easy method chaining 1603 */ 1604 public ClinicalImpression setPrognosisCodeableConcept(List<CodeableConcept> thePrognosisCodeableConcept) { 1605 this.prognosisCodeableConcept = thePrognosisCodeableConcept; 1606 return this; 1607 } 1608 1609 public boolean hasPrognosisCodeableConcept() { 1610 if (this.prognosisCodeableConcept == null) 1611 return false; 1612 for (CodeableConcept item : this.prognosisCodeableConcept) 1613 if (!item.isEmpty()) 1614 return true; 1615 return false; 1616 } 1617 1618 public CodeableConcept addPrognosisCodeableConcept() { //3 1619 CodeableConcept t = new CodeableConcept(); 1620 if (this.prognosisCodeableConcept == null) 1621 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1622 this.prognosisCodeableConcept.add(t); 1623 return t; 1624 } 1625 1626 public ClinicalImpression addPrognosisCodeableConcept(CodeableConcept t) { //3 1627 if (t == null) 1628 return this; 1629 if (this.prognosisCodeableConcept == null) 1630 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1631 this.prognosisCodeableConcept.add(t); 1632 return this; 1633 } 1634 1635 /** 1636 * @return The first repetition of repeating field {@link #prognosisCodeableConcept}, creating it if it does not already exist 1637 */ 1638 public CodeableConcept getPrognosisCodeableConceptFirstRep() { 1639 if (getPrognosisCodeableConcept().isEmpty()) { 1640 addPrognosisCodeableConcept(); 1641 } 1642 return getPrognosisCodeableConcept().get(0); 1643 } 1644 1645 /** 1646 * @return {@link #prognosisReference} (RiskAssessment expressing likely outcome.) 1647 */ 1648 public List<Reference> getPrognosisReference() { 1649 if (this.prognosisReference == null) 1650 this.prognosisReference = new ArrayList<Reference>(); 1651 return this.prognosisReference; 1652 } 1653 1654 /** 1655 * @return Returns a reference to <code>this</code> for easy method chaining 1656 */ 1657 public ClinicalImpression setPrognosisReference(List<Reference> thePrognosisReference) { 1658 this.prognosisReference = thePrognosisReference; 1659 return this; 1660 } 1661 1662 public boolean hasPrognosisReference() { 1663 if (this.prognosisReference == null) 1664 return false; 1665 for (Reference item : this.prognosisReference) 1666 if (!item.isEmpty()) 1667 return true; 1668 return false; 1669 } 1670 1671 public Reference addPrognosisReference() { //3 1672 Reference t = new Reference(); 1673 if (this.prognosisReference == null) 1674 this.prognosisReference = new ArrayList<Reference>(); 1675 this.prognosisReference.add(t); 1676 return t; 1677 } 1678 1679 public ClinicalImpression addPrognosisReference(Reference t) { //3 1680 if (t == null) 1681 return this; 1682 if (this.prognosisReference == null) 1683 this.prognosisReference = new ArrayList<Reference>(); 1684 this.prognosisReference.add(t); 1685 return this; 1686 } 1687 1688 /** 1689 * @return The first repetition of repeating field {@link #prognosisReference}, creating it if it does not already exist 1690 */ 1691 public Reference getPrognosisReferenceFirstRep() { 1692 if (getPrognosisReference().isEmpty()) { 1693 addPrognosisReference(); 1694 } 1695 return getPrognosisReference().get(0); 1696 } 1697 1698 /** 1699 * @deprecated Use Reference#setResource(IBaseResource) instead 1700 */ 1701 @Deprecated 1702 public List<RiskAssessment> getPrognosisReferenceTarget() { 1703 if (this.prognosisReferenceTarget == null) 1704 this.prognosisReferenceTarget = new ArrayList<RiskAssessment>(); 1705 return this.prognosisReferenceTarget; 1706 } 1707 1708 /** 1709 * @deprecated Use Reference#setResource(IBaseResource) instead 1710 */ 1711 @Deprecated 1712 public RiskAssessment addPrognosisReferenceTarget() { 1713 RiskAssessment r = new RiskAssessment(); 1714 if (this.prognosisReferenceTarget == null) 1715 this.prognosisReferenceTarget = new ArrayList<RiskAssessment>(); 1716 this.prognosisReferenceTarget.add(r); 1717 return r; 1718 } 1719 1720 /** 1721 * @return {@link #action} (Action taken as part of assessment procedure.) 1722 */ 1723 public List<Reference> getAction() { 1724 if (this.action == null) 1725 this.action = new ArrayList<Reference>(); 1726 return this.action; 1727 } 1728 1729 /** 1730 * @return Returns a reference to <code>this</code> for easy method chaining 1731 */ 1732 public ClinicalImpression setAction(List<Reference> theAction) { 1733 this.action = theAction; 1734 return this; 1735 } 1736 1737 public boolean hasAction() { 1738 if (this.action == null) 1739 return false; 1740 for (Reference item : this.action) 1741 if (!item.isEmpty()) 1742 return true; 1743 return false; 1744 } 1745 1746 public Reference addAction() { //3 1747 Reference t = new Reference(); 1748 if (this.action == null) 1749 this.action = new ArrayList<Reference>(); 1750 this.action.add(t); 1751 return t; 1752 } 1753 1754 public ClinicalImpression addAction(Reference t) { //3 1755 if (t == null) 1756 return this; 1757 if (this.action == null) 1758 this.action = new ArrayList<Reference>(); 1759 this.action.add(t); 1760 return this; 1761 } 1762 1763 /** 1764 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 1765 */ 1766 public Reference getActionFirstRep() { 1767 if (getAction().isEmpty()) { 1768 addAction(); 1769 } 1770 return getAction().get(0); 1771 } 1772 1773 /** 1774 * @deprecated Use Reference#setResource(IBaseResource) instead 1775 */ 1776 @Deprecated 1777 public List<Resource> getActionTarget() { 1778 if (this.actionTarget == null) 1779 this.actionTarget = new ArrayList<Resource>(); 1780 return this.actionTarget; 1781 } 1782 1783 /** 1784 * @return {@link #note} (Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.) 1785 */ 1786 public List<Annotation> getNote() { 1787 if (this.note == null) 1788 this.note = new ArrayList<Annotation>(); 1789 return this.note; 1790 } 1791 1792 /** 1793 * @return Returns a reference to <code>this</code> for easy method chaining 1794 */ 1795 public ClinicalImpression setNote(List<Annotation> theNote) { 1796 this.note = theNote; 1797 return this; 1798 } 1799 1800 public boolean hasNote() { 1801 if (this.note == null) 1802 return false; 1803 for (Annotation item : this.note) 1804 if (!item.isEmpty()) 1805 return true; 1806 return false; 1807 } 1808 1809 public Annotation addNote() { //3 1810 Annotation t = new Annotation(); 1811 if (this.note == null) 1812 this.note = new ArrayList<Annotation>(); 1813 this.note.add(t); 1814 return t; 1815 } 1816 1817 public ClinicalImpression addNote(Annotation t) { //3 1818 if (t == null) 1819 return this; 1820 if (this.note == null) 1821 this.note = new ArrayList<Annotation>(); 1822 this.note.add(t); 1823 return this; 1824 } 1825 1826 /** 1827 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1828 */ 1829 public Annotation getNoteFirstRep() { 1830 if (getNote().isEmpty()) { 1831 addNote(); 1832 } 1833 return getNote().get(0); 1834 } 1835 1836 protected void listChildren(List<Property> children) { 1837 super.listChildren(children); 1838 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to the clinical impression that remains consistent regardless of what server the impression is stored on.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1839 children.add(new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, status)); 1840 children.add(new Property("code", "CodeableConcept", "Categorizes the type of clinical assessment performed.", 0, 1, code)); 1841 children.add(new Property("description", "string", "A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it.", 0, 1, description)); 1842 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group of individuals assessed as part of this record.", 0, 1, subject)); 1843 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care this impression was created as part of.", 0, 1, context)); 1844 children.add(new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective)); 1845 children.add(new Property("date", "dateTime", "Indicates when the documentation of the assessment was complete.", 0, 1, date)); 1846 children.add(new Property("assessor", "Reference(Practitioner)", "The clinician performing the assessment.", 0, 1, assessor)); 1847 children.add(new Property("previous", "Reference(ClinicalImpression)", "A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 0, 1, previous)); 1848 children.add(new Property("problem", "Reference(Condition|AllergyIntolerance)", "This a list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem)); 1849 children.add(new Property("investigation", "", "One or more sets of investigations (signs, symptions, etc.). The actual grouping of investigations vary greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.", 0, java.lang.Integer.MAX_VALUE, investigation)); 1850 children.add(new Property("protocol", "uri", "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 0, java.lang.Integer.MAX_VALUE, protocol)); 1851 children.add(new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 0, 1, summary)); 1852 children.add(new Property("finding", "", "Specific findings or diagnoses that was considered likely or relevant to ongoing treatment.", 0, java.lang.Integer.MAX_VALUE, finding)); 1853 children.add(new Property("prognosisCodeableConcept", "CodeableConcept", "Estimate of likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisCodeableConcept)); 1854 children.add(new Property("prognosisReference", "Reference(RiskAssessment)", "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference)); 1855 children.add(new Property("action", "Reference(ReferralRequest|ProcedureRequest|Procedure|MedicationRequest|Appointment)", "Action taken as part of assessment procedure.", 0, java.lang.Integer.MAX_VALUE, action)); 1856 children.add(new Property("note", "Annotation", "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 0, java.lang.Integer.MAX_VALUE, note)); 1857 } 1858 1859 @Override 1860 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1861 switch (_hash) { 1862 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to the clinical impression that remains consistent regardless of what server the impression is stored on.", 0, java.lang.Integer.MAX_VALUE, identifier); 1863 case -892481550: /*status*/ return new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, status); 1864 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Categorizes the type of clinical assessment performed.", 0, 1, code); 1865 case -1724546052: /*description*/ return new Property("description", "string", "A summary of the context and/or cause of the assessment - why / where was it performed, and what patient events/status prompted it.", 0, 1, description); 1866 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group of individuals assessed as part of this record.", 0, 1, subject); 1867 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care this impression was created as part of.", 0, 1, context); 1868 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1869 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1870 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1871 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1872 case 3076014: /*date*/ return new Property("date", "dateTime", "Indicates when the documentation of the assessment was complete.", 0, 1, date); 1873 case -373213113: /*assessor*/ return new Property("assessor", "Reference(Practitioner)", "The clinician performing the assessment.", 0, 1, assessor); 1874 case -1273775369: /*previous*/ return new Property("previous", "Reference(ClinicalImpression)", "A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 0, 1, previous); 1875 case -309542241: /*problem*/ return new Property("problem", "Reference(Condition|AllergyIntolerance)", "This a list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem); 1876 case 956015362: /*investigation*/ return new Property("investigation", "", "One or more sets of investigations (signs, symptions, etc.). The actual grouping of investigations vary greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.", 0, java.lang.Integer.MAX_VALUE, investigation); 1877 case -989163880: /*protocol*/ return new Property("protocol", "uri", "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 0, java.lang.Integer.MAX_VALUE, protocol); 1878 case -1857640538: /*summary*/ return new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 0, 1, summary); 1879 case -853173367: /*finding*/ return new Property("finding", "", "Specific findings or diagnoses that was considered likely or relevant to ongoing treatment.", 0, java.lang.Integer.MAX_VALUE, finding); 1880 case -676337953: /*prognosisCodeableConcept*/ return new Property("prognosisCodeableConcept", "CodeableConcept", "Estimate of likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisCodeableConcept); 1881 case -587137783: /*prognosisReference*/ return new Property("prognosisReference", "Reference(RiskAssessment)", "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference); 1882 case -1422950858: /*action*/ return new Property("action", "Reference(ReferralRequest|ProcedureRequest|Procedure|MedicationRequest|Appointment)", "Action taken as part of assessment procedure.", 0, java.lang.Integer.MAX_VALUE, action); 1883 case 3387378: /*note*/ return new Property("note", "Annotation", "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 0, java.lang.Integer.MAX_VALUE, note); 1884 default: return super.getNamedProperty(_hash, _name, _checkValid); 1885 } 1886 1887 } 1888 1889 @Override 1890 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1891 switch (hash) { 1892 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1893 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ClinicalImpressionStatus> 1894 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1895 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1896 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1897 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1898 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // Type 1899 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1900 case -373213113: /*assessor*/ return this.assessor == null ? new Base[0] : new Base[] {this.assessor}; // Reference 1901 case -1273775369: /*previous*/ return this.previous == null ? new Base[0] : new Base[] {this.previous}; // Reference 1902 case -309542241: /*problem*/ return this.problem == null ? new Base[0] : this.problem.toArray(new Base[this.problem.size()]); // Reference 1903 case 956015362: /*investigation*/ return this.investigation == null ? new Base[0] : this.investigation.toArray(new Base[this.investigation.size()]); // ClinicalImpressionInvestigationComponent 1904 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // UriType 1905 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : new Base[] {this.summary}; // StringType 1906 case -853173367: /*finding*/ return this.finding == null ? new Base[0] : this.finding.toArray(new Base[this.finding.size()]); // ClinicalImpressionFindingComponent 1907 case -676337953: /*prognosisCodeableConcept*/ return this.prognosisCodeableConcept == null ? new Base[0] : this.prognosisCodeableConcept.toArray(new Base[this.prognosisCodeableConcept.size()]); // CodeableConcept 1908 case -587137783: /*prognosisReference*/ return this.prognosisReference == null ? new Base[0] : this.prognosisReference.toArray(new Base[this.prognosisReference.size()]); // Reference 1909 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // Reference 1910 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1911 default: return super.getProperty(hash, name, checkValid); 1912 } 1913 1914 } 1915 1916 @Override 1917 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1918 switch (hash) { 1919 case -1618432855: // identifier 1920 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1921 return value; 1922 case -892481550: // status 1923 value = new ClinicalImpressionStatusEnumFactory().fromType(castToCode(value)); 1924 this.status = (Enumeration) value; // Enumeration<ClinicalImpressionStatus> 1925 return value; 1926 case 3059181: // code 1927 this.code = castToCodeableConcept(value); // CodeableConcept 1928 return value; 1929 case -1724546052: // description 1930 this.description = castToString(value); // StringType 1931 return value; 1932 case -1867885268: // subject 1933 this.subject = castToReference(value); // Reference 1934 return value; 1935 case 951530927: // context 1936 this.context = castToReference(value); // Reference 1937 return value; 1938 case -1468651097: // effective 1939 this.effective = castToType(value); // Type 1940 return value; 1941 case 3076014: // date 1942 this.date = castToDateTime(value); // DateTimeType 1943 return value; 1944 case -373213113: // assessor 1945 this.assessor = castToReference(value); // Reference 1946 return value; 1947 case -1273775369: // previous 1948 this.previous = castToReference(value); // Reference 1949 return value; 1950 case -309542241: // problem 1951 this.getProblem().add(castToReference(value)); // Reference 1952 return value; 1953 case 956015362: // investigation 1954 this.getInvestigation().add((ClinicalImpressionInvestigationComponent) value); // ClinicalImpressionInvestigationComponent 1955 return value; 1956 case -989163880: // protocol 1957 this.getProtocol().add(castToUri(value)); // UriType 1958 return value; 1959 case -1857640538: // summary 1960 this.summary = castToString(value); // StringType 1961 return value; 1962 case -853173367: // finding 1963 this.getFinding().add((ClinicalImpressionFindingComponent) value); // ClinicalImpressionFindingComponent 1964 return value; 1965 case -676337953: // prognosisCodeableConcept 1966 this.getPrognosisCodeableConcept().add(castToCodeableConcept(value)); // CodeableConcept 1967 return value; 1968 case -587137783: // prognosisReference 1969 this.getPrognosisReference().add(castToReference(value)); // Reference 1970 return value; 1971 case -1422950858: // action 1972 this.getAction().add(castToReference(value)); // Reference 1973 return value; 1974 case 3387378: // note 1975 this.getNote().add(castToAnnotation(value)); // Annotation 1976 return value; 1977 default: return super.setProperty(hash, name, value); 1978 } 1979 1980 } 1981 1982 @Override 1983 public Base setProperty(String name, Base value) throws FHIRException { 1984 if (name.equals("identifier")) { 1985 this.getIdentifier().add(castToIdentifier(value)); 1986 } else if (name.equals("status")) { 1987 value = new ClinicalImpressionStatusEnumFactory().fromType(castToCode(value)); 1988 this.status = (Enumeration) value; // Enumeration<ClinicalImpressionStatus> 1989 } else if (name.equals("code")) { 1990 this.code = castToCodeableConcept(value); // CodeableConcept 1991 } else if (name.equals("description")) { 1992 this.description = castToString(value); // StringType 1993 } else if (name.equals("subject")) { 1994 this.subject = castToReference(value); // Reference 1995 } else if (name.equals("context")) { 1996 this.context = castToReference(value); // Reference 1997 } else if (name.equals("effective[x]")) { 1998 this.effective = castToType(value); // Type 1999 } else if (name.equals("date")) { 2000 this.date = castToDateTime(value); // DateTimeType 2001 } else if (name.equals("assessor")) { 2002 this.assessor = castToReference(value); // Reference 2003 } else if (name.equals("previous")) { 2004 this.previous = castToReference(value); // Reference 2005 } else if (name.equals("problem")) { 2006 this.getProblem().add(castToReference(value)); 2007 } else if (name.equals("investigation")) { 2008 this.getInvestigation().add((ClinicalImpressionInvestigationComponent) value); 2009 } else if (name.equals("protocol")) { 2010 this.getProtocol().add(castToUri(value)); 2011 } else if (name.equals("summary")) { 2012 this.summary = castToString(value); // StringType 2013 } else if (name.equals("finding")) { 2014 this.getFinding().add((ClinicalImpressionFindingComponent) value); 2015 } else if (name.equals("prognosisCodeableConcept")) { 2016 this.getPrognosisCodeableConcept().add(castToCodeableConcept(value)); 2017 } else if (name.equals("prognosisReference")) { 2018 this.getPrognosisReference().add(castToReference(value)); 2019 } else if (name.equals("action")) { 2020 this.getAction().add(castToReference(value)); 2021 } else if (name.equals("note")) { 2022 this.getNote().add(castToAnnotation(value)); 2023 } else 2024 return super.setProperty(name, value); 2025 return value; 2026 } 2027 2028 @Override 2029 public Base makeProperty(int hash, String name) throws FHIRException { 2030 switch (hash) { 2031 case -1618432855: return addIdentifier(); 2032 case -892481550: return getStatusElement(); 2033 case 3059181: return getCode(); 2034 case -1724546052: return getDescriptionElement(); 2035 case -1867885268: return getSubject(); 2036 case 951530927: return getContext(); 2037 case 247104889: return getEffective(); 2038 case -1468651097: return getEffective(); 2039 case 3076014: return getDateElement(); 2040 case -373213113: return getAssessor(); 2041 case -1273775369: return getPrevious(); 2042 case -309542241: return addProblem(); 2043 case 956015362: return addInvestigation(); 2044 case -989163880: return addProtocolElement(); 2045 case -1857640538: return getSummaryElement(); 2046 case -853173367: return addFinding(); 2047 case -676337953: return addPrognosisCodeableConcept(); 2048 case -587137783: return addPrognosisReference(); 2049 case -1422950858: return addAction(); 2050 case 3387378: return addNote(); 2051 default: return super.makeProperty(hash, name); 2052 } 2053 2054 } 2055 2056 @Override 2057 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2058 switch (hash) { 2059 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2060 case -892481550: /*status*/ return new String[] {"code"}; 2061 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2062 case -1724546052: /*description*/ return new String[] {"string"}; 2063 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2064 case 951530927: /*context*/ return new String[] {"Reference"}; 2065 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 2066 case 3076014: /*date*/ return new String[] {"dateTime"}; 2067 case -373213113: /*assessor*/ return new String[] {"Reference"}; 2068 case -1273775369: /*previous*/ return new String[] {"Reference"}; 2069 case -309542241: /*problem*/ return new String[] {"Reference"}; 2070 case 956015362: /*investigation*/ return new String[] {}; 2071 case -989163880: /*protocol*/ return new String[] {"uri"}; 2072 case -1857640538: /*summary*/ return new String[] {"string"}; 2073 case -853173367: /*finding*/ return new String[] {}; 2074 case -676337953: /*prognosisCodeableConcept*/ return new String[] {"CodeableConcept"}; 2075 case -587137783: /*prognosisReference*/ return new String[] {"Reference"}; 2076 case -1422950858: /*action*/ return new String[] {"Reference"}; 2077 case 3387378: /*note*/ return new String[] {"Annotation"}; 2078 default: return super.getTypesForProperty(hash, name); 2079 } 2080 2081 } 2082 2083 @Override 2084 public Base addChild(String name) throws FHIRException { 2085 if (name.equals("identifier")) { 2086 return addIdentifier(); 2087 } 2088 else if (name.equals("status")) { 2089 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.status"); 2090 } 2091 else if (name.equals("code")) { 2092 this.code = new CodeableConcept(); 2093 return this.code; 2094 } 2095 else if (name.equals("description")) { 2096 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.description"); 2097 } 2098 else if (name.equals("subject")) { 2099 this.subject = new Reference(); 2100 return this.subject; 2101 } 2102 else if (name.equals("context")) { 2103 this.context = new Reference(); 2104 return this.context; 2105 } 2106 else if (name.equals("effectiveDateTime")) { 2107 this.effective = new DateTimeType(); 2108 return this.effective; 2109 } 2110 else if (name.equals("effectivePeriod")) { 2111 this.effective = new Period(); 2112 return this.effective; 2113 } 2114 else if (name.equals("date")) { 2115 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.date"); 2116 } 2117 else if (name.equals("assessor")) { 2118 this.assessor = new Reference(); 2119 return this.assessor; 2120 } 2121 else if (name.equals("previous")) { 2122 this.previous = new Reference(); 2123 return this.previous; 2124 } 2125 else if (name.equals("problem")) { 2126 return addProblem(); 2127 } 2128 else if (name.equals("investigation")) { 2129 return addInvestigation(); 2130 } 2131 else if (name.equals("protocol")) { 2132 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.protocol"); 2133 } 2134 else if (name.equals("summary")) { 2135 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.summary"); 2136 } 2137 else if (name.equals("finding")) { 2138 return addFinding(); 2139 } 2140 else if (name.equals("prognosisCodeableConcept")) { 2141 return addPrognosisCodeableConcept(); 2142 } 2143 else if (name.equals("prognosisReference")) { 2144 return addPrognosisReference(); 2145 } 2146 else if (name.equals("action")) { 2147 return addAction(); 2148 } 2149 else if (name.equals("note")) { 2150 return addNote(); 2151 } 2152 else 2153 return super.addChild(name); 2154 } 2155 2156 public String fhirType() { 2157 return "ClinicalImpression"; 2158 2159 } 2160 2161 public ClinicalImpression copy() { 2162 ClinicalImpression dst = new ClinicalImpression(); 2163 copyValues(dst); 2164 if (identifier != null) { 2165 dst.identifier = new ArrayList<Identifier>(); 2166 for (Identifier i : identifier) 2167 dst.identifier.add(i.copy()); 2168 }; 2169 dst.status = status == null ? null : status.copy(); 2170 dst.code = code == null ? null : code.copy(); 2171 dst.description = description == null ? null : description.copy(); 2172 dst.subject = subject == null ? null : subject.copy(); 2173 dst.context = context == null ? null : context.copy(); 2174 dst.effective = effective == null ? null : effective.copy(); 2175 dst.date = date == null ? null : date.copy(); 2176 dst.assessor = assessor == null ? null : assessor.copy(); 2177 dst.previous = previous == null ? null : previous.copy(); 2178 if (problem != null) { 2179 dst.problem = new ArrayList<Reference>(); 2180 for (Reference i : problem) 2181 dst.problem.add(i.copy()); 2182 }; 2183 if (investigation != null) { 2184 dst.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 2185 for (ClinicalImpressionInvestigationComponent i : investigation) 2186 dst.investigation.add(i.copy()); 2187 }; 2188 if (protocol != null) { 2189 dst.protocol = new ArrayList<UriType>(); 2190 for (UriType i : protocol) 2191 dst.protocol.add(i.copy()); 2192 }; 2193 dst.summary = summary == null ? null : summary.copy(); 2194 if (finding != null) { 2195 dst.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 2196 for (ClinicalImpressionFindingComponent i : finding) 2197 dst.finding.add(i.copy()); 2198 }; 2199 if (prognosisCodeableConcept != null) { 2200 dst.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 2201 for (CodeableConcept i : prognosisCodeableConcept) 2202 dst.prognosisCodeableConcept.add(i.copy()); 2203 }; 2204 if (prognosisReference != null) { 2205 dst.prognosisReference = new ArrayList<Reference>(); 2206 for (Reference i : prognosisReference) 2207 dst.prognosisReference.add(i.copy()); 2208 }; 2209 if (action != null) { 2210 dst.action = new ArrayList<Reference>(); 2211 for (Reference i : action) 2212 dst.action.add(i.copy()); 2213 }; 2214 if (note != null) { 2215 dst.note = new ArrayList<Annotation>(); 2216 for (Annotation i : note) 2217 dst.note.add(i.copy()); 2218 }; 2219 return dst; 2220 } 2221 2222 protected ClinicalImpression typedCopy() { 2223 return copy(); 2224 } 2225 2226 @Override 2227 public boolean equalsDeep(Base other_) { 2228 if (!super.equalsDeep(other_)) 2229 return false; 2230 if (!(other_ instanceof ClinicalImpression)) 2231 return false; 2232 ClinicalImpression o = (ClinicalImpression) other_; 2233 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(code, o.code, true) 2234 && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 2235 && compareDeep(effective, o.effective, true) && compareDeep(date, o.date, true) && compareDeep(assessor, o.assessor, true) 2236 && compareDeep(previous, o.previous, true) && compareDeep(problem, o.problem, true) && compareDeep(investigation, o.investigation, true) 2237 && compareDeep(protocol, o.protocol, true) && compareDeep(summary, o.summary, true) && compareDeep(finding, o.finding, true) 2238 && compareDeep(prognosisCodeableConcept, o.prognosisCodeableConcept, true) && compareDeep(prognosisReference, o.prognosisReference, true) 2239 && compareDeep(action, o.action, true) && compareDeep(note, o.note, true); 2240 } 2241 2242 @Override 2243 public boolean equalsShallow(Base other_) { 2244 if (!super.equalsShallow(other_)) 2245 return false; 2246 if (!(other_ instanceof ClinicalImpression)) 2247 return false; 2248 ClinicalImpression o = (ClinicalImpression) other_; 2249 return compareValues(status, o.status, true) && compareValues(description, o.description, true) && compareValues(date, o.date, true) 2250 && compareValues(protocol, o.protocol, true) && compareValues(summary, o.summary, true); 2251 } 2252 2253 public boolean isEmpty() { 2254 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, code 2255 , description, subject, context, effective, date, assessor, previous, problem 2256 , investigation, protocol, summary, finding, prognosisCodeableConcept, prognosisReference 2257 , action, note); 2258 } 2259 2260 @Override 2261 public ResourceType getResourceType() { 2262 return ResourceType.ClinicalImpression; 2263 } 2264 2265 /** 2266 * Search parameter: <b>date</b> 2267 * <p> 2268 * Description: <b>When the assessment was documented</b><br> 2269 * Type: <b>date</b><br> 2270 * Path: <b>ClinicalImpression.date</b><br> 2271 * </p> 2272 */ 2273 @SearchParamDefinition(name="date", path="ClinicalImpression.date", description="When the assessment was documented", type="date" ) 2274 public static final String SP_DATE = "date"; 2275 /** 2276 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2277 * <p> 2278 * Description: <b>When the assessment was documented</b><br> 2279 * Type: <b>date</b><br> 2280 * Path: <b>ClinicalImpression.date</b><br> 2281 * </p> 2282 */ 2283 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2284 2285 /** 2286 * Search parameter: <b>identifier</b> 2287 * <p> 2288 * Description: <b>Business identifier</b><br> 2289 * Type: <b>token</b><br> 2290 * Path: <b>ClinicalImpression.identifier</b><br> 2291 * </p> 2292 */ 2293 @SearchParamDefinition(name="identifier", path="ClinicalImpression.identifier", description="Business identifier", type="token" ) 2294 public static final String SP_IDENTIFIER = "identifier"; 2295 /** 2296 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2297 * <p> 2298 * Description: <b>Business identifier</b><br> 2299 * Type: <b>token</b><br> 2300 * Path: <b>ClinicalImpression.identifier</b><br> 2301 * </p> 2302 */ 2303 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2304 2305 /** 2306 * Search parameter: <b>previous</b> 2307 * <p> 2308 * Description: <b>Reference to last assessment</b><br> 2309 * Type: <b>reference</b><br> 2310 * Path: <b>ClinicalImpression.previous</b><br> 2311 * </p> 2312 */ 2313 @SearchParamDefinition(name="previous", path="ClinicalImpression.previous", description="Reference to last assessment", type="reference", target={ClinicalImpression.class } ) 2314 public static final String SP_PREVIOUS = "previous"; 2315 /** 2316 * <b>Fluent Client</b> search parameter constant for <b>previous</b> 2317 * <p> 2318 * Description: <b>Reference to last assessment</b><br> 2319 * Type: <b>reference</b><br> 2320 * Path: <b>ClinicalImpression.previous</b><br> 2321 * </p> 2322 */ 2323 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREVIOUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREVIOUS); 2324 2325/** 2326 * Constant for fluent queries to be used to add include statements. Specifies 2327 * the path value of "<b>ClinicalImpression:previous</b>". 2328 */ 2329 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREVIOUS = new ca.uhn.fhir.model.api.Include("ClinicalImpression:previous").toLocked(); 2330 2331 /** 2332 * Search parameter: <b>finding-code</b> 2333 * <p> 2334 * Description: <b>What was found</b><br> 2335 * Type: <b>token</b><br> 2336 * Path: <b>ClinicalImpression.finding.item[x]</b><br> 2337 * </p> 2338 */ 2339 @SearchParamDefinition(name="finding-code", path="ClinicalImpression.finding.item.as(CodeableConcept)", description="What was found", type="token" ) 2340 public static final String SP_FINDING_CODE = "finding-code"; 2341 /** 2342 * <b>Fluent Client</b> search parameter constant for <b>finding-code</b> 2343 * <p> 2344 * Description: <b>What was found</b><br> 2345 * Type: <b>token</b><br> 2346 * Path: <b>ClinicalImpression.finding.item[x]</b><br> 2347 * </p> 2348 */ 2349 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FINDING_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FINDING_CODE); 2350 2351 /** 2352 * Search parameter: <b>assessor</b> 2353 * <p> 2354 * Description: <b>The clinician performing the assessment</b><br> 2355 * Type: <b>reference</b><br> 2356 * Path: <b>ClinicalImpression.assessor</b><br> 2357 * </p> 2358 */ 2359 @SearchParamDefinition(name="assessor", path="ClinicalImpression.assessor", description="The clinician performing the assessment", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2360 public static final String SP_ASSESSOR = "assessor"; 2361 /** 2362 * <b>Fluent Client</b> search parameter constant for <b>assessor</b> 2363 * <p> 2364 * Description: <b>The clinician performing the assessment</b><br> 2365 * Type: <b>reference</b><br> 2366 * Path: <b>ClinicalImpression.assessor</b><br> 2367 * </p> 2368 */ 2369 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ASSESSOR); 2370 2371/** 2372 * Constant for fluent queries to be used to add include statements. Specifies 2373 * the path value of "<b>ClinicalImpression:assessor</b>". 2374 */ 2375 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSESSOR = new ca.uhn.fhir.model.api.Include("ClinicalImpression:assessor").toLocked(); 2376 2377 /** 2378 * Search parameter: <b>subject</b> 2379 * <p> 2380 * Description: <b>Patient or group assessed</b><br> 2381 * Type: <b>reference</b><br> 2382 * Path: <b>ClinicalImpression.subject</b><br> 2383 * </p> 2384 */ 2385 @SearchParamDefinition(name="subject", path="ClinicalImpression.subject", description="Patient or group assessed", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2386 public static final String SP_SUBJECT = "subject"; 2387 /** 2388 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2389 * <p> 2390 * Description: <b>Patient or group assessed</b><br> 2391 * Type: <b>reference</b><br> 2392 * Path: <b>ClinicalImpression.subject</b><br> 2393 * </p> 2394 */ 2395 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2396 2397/** 2398 * Constant for fluent queries to be used to add include statements. Specifies 2399 * the path value of "<b>ClinicalImpression:subject</b>". 2400 */ 2401 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ClinicalImpression:subject").toLocked(); 2402 2403 /** 2404 * Search parameter: <b>finding-ref</b> 2405 * <p> 2406 * Description: <b>What was found</b><br> 2407 * Type: <b>reference</b><br> 2408 * Path: <b>ClinicalImpression.finding.item[x]</b><br> 2409 * </p> 2410 */ 2411 @SearchParamDefinition(name="finding-ref", path="ClinicalImpression.finding.item.as(Reference)", description="What was found", type="reference", target={Condition.class, Observation.class } ) 2412 public static final String SP_FINDING_REF = "finding-ref"; 2413 /** 2414 * <b>Fluent Client</b> search parameter constant for <b>finding-ref</b> 2415 * <p> 2416 * Description: <b>What was found</b><br> 2417 * Type: <b>reference</b><br> 2418 * Path: <b>ClinicalImpression.finding.item[x]</b><br> 2419 * </p> 2420 */ 2421 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FINDING_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FINDING_REF); 2422 2423/** 2424 * Constant for fluent queries to be used to add include statements. Specifies 2425 * the path value of "<b>ClinicalImpression:finding-ref</b>". 2426 */ 2427 public static final ca.uhn.fhir.model.api.Include INCLUDE_FINDING_REF = new ca.uhn.fhir.model.api.Include("ClinicalImpression:finding-ref").toLocked(); 2428 2429 /** 2430 * Search parameter: <b>problem</b> 2431 * <p> 2432 * Description: <b>Relevant impressions of patient state</b><br> 2433 * Type: <b>reference</b><br> 2434 * Path: <b>ClinicalImpression.problem</b><br> 2435 * </p> 2436 */ 2437 @SearchParamDefinition(name="problem", path="ClinicalImpression.problem", description="Relevant impressions of patient state", type="reference", target={AllergyIntolerance.class, Condition.class } ) 2438 public static final String SP_PROBLEM = "problem"; 2439 /** 2440 * <b>Fluent Client</b> search parameter constant for <b>problem</b> 2441 * <p> 2442 * Description: <b>Relevant impressions of patient state</b><br> 2443 * Type: <b>reference</b><br> 2444 * Path: <b>ClinicalImpression.problem</b><br> 2445 * </p> 2446 */ 2447 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROBLEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROBLEM); 2448 2449/** 2450 * Constant for fluent queries to be used to add include statements. Specifies 2451 * the path value of "<b>ClinicalImpression:problem</b>". 2452 */ 2453 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROBLEM = new ca.uhn.fhir.model.api.Include("ClinicalImpression:problem").toLocked(); 2454 2455 /** 2456 * Search parameter: <b>patient</b> 2457 * <p> 2458 * Description: <b>Patient or group assessed</b><br> 2459 * Type: <b>reference</b><br> 2460 * Path: <b>ClinicalImpression.subject</b><br> 2461 * </p> 2462 */ 2463 @SearchParamDefinition(name="patient", path="ClinicalImpression.subject", description="Patient or group assessed", type="reference", target={Patient.class } ) 2464 public static final String SP_PATIENT = "patient"; 2465 /** 2466 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2467 * <p> 2468 * Description: <b>Patient or group assessed</b><br> 2469 * Type: <b>reference</b><br> 2470 * Path: <b>ClinicalImpression.subject</b><br> 2471 * </p> 2472 */ 2473 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2474 2475/** 2476 * Constant for fluent queries to be used to add include statements. Specifies 2477 * the path value of "<b>ClinicalImpression:patient</b>". 2478 */ 2479 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ClinicalImpression:patient").toLocked(); 2480 2481 /** 2482 * Search parameter: <b>context</b> 2483 * <p> 2484 * Description: <b>Encounter or Episode created from</b><br> 2485 * Type: <b>reference</b><br> 2486 * Path: <b>ClinicalImpression.context</b><br> 2487 * </p> 2488 */ 2489 @SearchParamDefinition(name="context", path="ClinicalImpression.context", description="Encounter or Episode created from", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2490 public static final String SP_CONTEXT = "context"; 2491 /** 2492 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2493 * <p> 2494 * Description: <b>Encounter or Episode created from</b><br> 2495 * Type: <b>reference</b><br> 2496 * Path: <b>ClinicalImpression.context</b><br> 2497 * </p> 2498 */ 2499 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2500 2501/** 2502 * Constant for fluent queries to be used to add include statements. Specifies 2503 * the path value of "<b>ClinicalImpression:context</b>". 2504 */ 2505 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("ClinicalImpression:context").toLocked(); 2506 2507 /** 2508 * Search parameter: <b>investigation</b> 2509 * <p> 2510 * Description: <b>Record of a specific investigation</b><br> 2511 * Type: <b>reference</b><br> 2512 * Path: <b>ClinicalImpression.investigation.item</b><br> 2513 * </p> 2514 */ 2515 @SearchParamDefinition(name="investigation", path="ClinicalImpression.investigation.item", description="Record of a specific investigation", type="reference", target={DiagnosticReport.class, FamilyMemberHistory.class, ImagingStudy.class, Observation.class, QuestionnaireResponse.class, RiskAssessment.class } ) 2516 public static final String SP_INVESTIGATION = "investigation"; 2517 /** 2518 * <b>Fluent Client</b> search parameter constant for <b>investigation</b> 2519 * <p> 2520 * Description: <b>Record of a specific investigation</b><br> 2521 * Type: <b>reference</b><br> 2522 * Path: <b>ClinicalImpression.investigation.item</b><br> 2523 * </p> 2524 */ 2525 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INVESTIGATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INVESTIGATION); 2526 2527/** 2528 * Constant for fluent queries to be used to add include statements. Specifies 2529 * the path value of "<b>ClinicalImpression:investigation</b>". 2530 */ 2531 public static final ca.uhn.fhir.model.api.Include INCLUDE_INVESTIGATION = new ca.uhn.fhir.model.api.Include("ClinicalImpression:investigation").toLocked(); 2532 2533 /** 2534 * Search parameter: <b>action</b> 2535 * <p> 2536 * Description: <b>Action taken as part of assessment procedure</b><br> 2537 * Type: <b>reference</b><br> 2538 * Path: <b>ClinicalImpression.action</b><br> 2539 * </p> 2540 */ 2541 @SearchParamDefinition(name="action", path="ClinicalImpression.action", description="Action taken as part of assessment procedure", type="reference", target={Appointment.class, MedicationRequest.class, Procedure.class, ProcedureRequest.class, ReferralRequest.class } ) 2542 public static final String SP_ACTION = "action"; 2543 /** 2544 * <b>Fluent Client</b> search parameter constant for <b>action</b> 2545 * <p> 2546 * Description: <b>Action taken as part of assessment procedure</b><br> 2547 * Type: <b>reference</b><br> 2548 * Path: <b>ClinicalImpression.action</b><br> 2549 * </p> 2550 */ 2551 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTION); 2552 2553/** 2554 * Constant for fluent queries to be used to add include statements. Specifies 2555 * the path value of "<b>ClinicalImpression:action</b>". 2556 */ 2557 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTION = new ca.uhn.fhir.model.api.Include("ClinicalImpression:action").toLocked(); 2558 2559 /** 2560 * Search parameter: <b>status</b> 2561 * <p> 2562 * Description: <b>draft | completed | entered-in-error</b><br> 2563 * Type: <b>token</b><br> 2564 * Path: <b>ClinicalImpression.status</b><br> 2565 * </p> 2566 */ 2567 @SearchParamDefinition(name="status", path="ClinicalImpression.status", description="draft | completed | entered-in-error", type="token" ) 2568 public static final String SP_STATUS = "status"; 2569 /** 2570 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2571 * <p> 2572 * Description: <b>draft | completed | entered-in-error</b><br> 2573 * Type: <b>token</b><br> 2574 * Path: <b>ClinicalImpression.status</b><br> 2575 * </p> 2576 */ 2577 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2578 2579 2580}