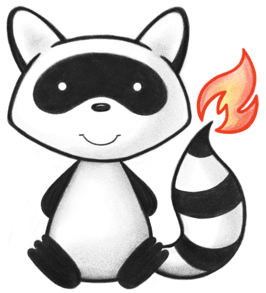
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.ChildOrder; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * A code system resource specifies a set of codes drawn from one or more code systems. 054 */ 055@ResourceDef(name="CodeSystem", profile="http://hl7.org/fhir/Profile/CodeSystem") 056@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "caseSensitive", "valueSet", "hierarchyMeaning", "compositional", "versionNeeded", "content", "count", "filter", "property", "concept"}) 057public class CodeSystem extends MetadataResource { 058 059 public enum CodeSystemHierarchyMeaning { 060 /** 061 * No particular relationship between the concepts can be assumed, except what can be determined by inspection of the definitions of the elements (possible reasons to use this: importing from a source where this is not defined, or where various parts of the hierarchy have different meanings) 062 */ 063 GROUPEDBY, 064 /** 065 * A hierarchy where the child concepts have an IS-A relationship with the parents - that is, all the properties of the parent are also true for its child concepts 066 */ 067 ISA, 068 /** 069 * Child elements list the individual parts of a composite whole (e.g. body site) 070 */ 071 PARTOF, 072 /** 073 * Child concepts in the hierarchy may have only one parent, and there is a presumption that the code system is a "closed world" meaning all things must be in the hierarchy. This results in concepts such as "not otherwise classified." 074 */ 075 CLASSIFIEDWITH, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 public static CodeSystemHierarchyMeaning fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("grouped-by".equals(codeString)) 084 return GROUPEDBY; 085 if ("is-a".equals(codeString)) 086 return ISA; 087 if ("part-of".equals(codeString)) 088 return PARTOF; 089 if ("classified-with".equals(codeString)) 090 return CLASSIFIEDWITH; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown CodeSystemHierarchyMeaning code '"+codeString+"'"); 095 } 096 public String toCode() { 097 switch (this) { 098 case GROUPEDBY: return "grouped-by"; 099 case ISA: return "is-a"; 100 case PARTOF: return "part-of"; 101 case CLASSIFIEDWITH: return "classified-with"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 switch (this) { 108 case GROUPEDBY: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 109 case ISA: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 110 case PARTOF: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 111 case CLASSIFIEDWITH: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDefinition() { 117 switch (this) { 118 case GROUPEDBY: return "No particular relationship between the concepts can be assumed, except what can be determined by inspection of the definitions of the elements (possible reasons to use this: importing from a source where this is not defined, or where various parts of the hierarchy have different meanings)"; 119 case ISA: return "A hierarchy where the child concepts have an IS-A relationship with the parents - that is, all the properties of the parent are also true for its child concepts"; 120 case PARTOF: return "Child elements list the individual parts of a composite whole (e.g. body site)"; 121 case CLASSIFIEDWITH: return "Child concepts in the hierarchy may have only one parent, and there is a presumption that the code system is a \"closed world\" meaning all things must be in the hierarchy. This results in concepts such as \"not otherwise classified.\""; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 public String getDisplay() { 127 switch (this) { 128 case GROUPEDBY: return "Grouped By"; 129 case ISA: return "Is-A"; 130 case PARTOF: return "Part Of"; 131 case CLASSIFIEDWITH: return "Classified With"; 132 case NULL: return null; 133 default: return "?"; 134 } 135 } 136 } 137 138 public static class CodeSystemHierarchyMeaningEnumFactory implements EnumFactory<CodeSystemHierarchyMeaning> { 139 public CodeSystemHierarchyMeaning fromCode(String codeString) throws IllegalArgumentException { 140 if (codeString == null || "".equals(codeString)) 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("grouped-by".equals(codeString)) 144 return CodeSystemHierarchyMeaning.GROUPEDBY; 145 if ("is-a".equals(codeString)) 146 return CodeSystemHierarchyMeaning.ISA; 147 if ("part-of".equals(codeString)) 148 return CodeSystemHierarchyMeaning.PARTOF; 149 if ("classified-with".equals(codeString)) 150 return CodeSystemHierarchyMeaning.CLASSIFIEDWITH; 151 throw new IllegalArgumentException("Unknown CodeSystemHierarchyMeaning code '"+codeString+"'"); 152 } 153 public Enumeration<CodeSystemHierarchyMeaning> fromType(PrimitiveType<?> code) throws FHIRException { 154 if (code == null) 155 return null; 156 if (code.isEmpty()) 157 return new Enumeration<CodeSystemHierarchyMeaning>(this); 158 String codeString = code.asStringValue(); 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("grouped-by".equals(codeString)) 162 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.GROUPEDBY); 163 if ("is-a".equals(codeString)) 164 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.ISA); 165 if ("part-of".equals(codeString)) 166 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.PARTOF); 167 if ("classified-with".equals(codeString)) 168 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.CLASSIFIEDWITH); 169 throw new FHIRException("Unknown CodeSystemHierarchyMeaning code '"+codeString+"'"); 170 } 171 public String toCode(CodeSystemHierarchyMeaning code) { 172 if (code == CodeSystemHierarchyMeaning.NULL) 173 return null; 174 if (code == CodeSystemHierarchyMeaning.GROUPEDBY) 175 return "grouped-by"; 176 if (code == CodeSystemHierarchyMeaning.ISA) 177 return "is-a"; 178 if (code == CodeSystemHierarchyMeaning.PARTOF) 179 return "part-of"; 180 if (code == CodeSystemHierarchyMeaning.CLASSIFIEDWITH) 181 return "classified-with"; 182 return "?"; 183 } 184 public String toSystem(CodeSystemHierarchyMeaning code) { 185 return code.getSystem(); 186 } 187 } 188 189 public enum CodeSystemContentMode { 190 /** 191 * None of the concepts defined by the code system are included in the code system resource 192 */ 193 NOTPRESENT, 194 /** 195 * A few representative concepts are included in the code system resource 196 */ 197 EXAMPLE, 198 /** 199 * A subset of the code system concepts are included in the code system resource 200 */ 201 FRAGMENT, 202 /** 203 * All the concepts defined by the code system are included in the code system resource 204 */ 205 COMPLETE, 206 /** 207 * added to help the parsers with the generic types 208 */ 209 NULL; 210 public static CodeSystemContentMode fromCode(String codeString) throws FHIRException { 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("not-present".equals(codeString)) 214 return NOTPRESENT; 215 if ("example".equals(codeString)) 216 return EXAMPLE; 217 if ("fragment".equals(codeString)) 218 return FRAGMENT; 219 if ("complete".equals(codeString)) 220 return COMPLETE; 221 if (Configuration.isAcceptInvalidEnums()) 222 return null; 223 else 224 throw new FHIRException("Unknown CodeSystemContentMode code '"+codeString+"'"); 225 } 226 public String toCode() { 227 switch (this) { 228 case NOTPRESENT: return "not-present"; 229 case EXAMPLE: return "example"; 230 case FRAGMENT: return "fragment"; 231 case COMPLETE: return "complete"; 232 case NULL: return null; 233 default: return "?"; 234 } 235 } 236 public String getSystem() { 237 switch (this) { 238 case NOTPRESENT: return "http://hl7.org/fhir/codesystem-content-mode"; 239 case EXAMPLE: return "http://hl7.org/fhir/codesystem-content-mode"; 240 case FRAGMENT: return "http://hl7.org/fhir/codesystem-content-mode"; 241 case COMPLETE: return "http://hl7.org/fhir/codesystem-content-mode"; 242 case NULL: return null; 243 default: return "?"; 244 } 245 } 246 public String getDefinition() { 247 switch (this) { 248 case NOTPRESENT: return "None of the concepts defined by the code system are included in the code system resource"; 249 case EXAMPLE: return "A few representative concepts are included in the code system resource"; 250 case FRAGMENT: return "A subset of the code system concepts are included in the code system resource"; 251 case COMPLETE: return "All the concepts defined by the code system are included in the code system resource"; 252 case NULL: return null; 253 default: return "?"; 254 } 255 } 256 public String getDisplay() { 257 switch (this) { 258 case NOTPRESENT: return "Not Present"; 259 case EXAMPLE: return "Example"; 260 case FRAGMENT: return "Fragment"; 261 case COMPLETE: return "Complete"; 262 case NULL: return null; 263 default: return "?"; 264 } 265 } 266 } 267 268 public static class CodeSystemContentModeEnumFactory implements EnumFactory<CodeSystemContentMode> { 269 public CodeSystemContentMode fromCode(String codeString) throws IllegalArgumentException { 270 if (codeString == null || "".equals(codeString)) 271 if (codeString == null || "".equals(codeString)) 272 return null; 273 if ("not-present".equals(codeString)) 274 return CodeSystemContentMode.NOTPRESENT; 275 if ("example".equals(codeString)) 276 return CodeSystemContentMode.EXAMPLE; 277 if ("fragment".equals(codeString)) 278 return CodeSystemContentMode.FRAGMENT; 279 if ("complete".equals(codeString)) 280 return CodeSystemContentMode.COMPLETE; 281 throw new IllegalArgumentException("Unknown CodeSystemContentMode code '"+codeString+"'"); 282 } 283 public Enumeration<CodeSystemContentMode> fromType(PrimitiveType<?> code) throws FHIRException { 284 if (code == null) 285 return null; 286 if (code.isEmpty()) 287 return new Enumeration<CodeSystemContentMode>(this); 288 String codeString = code.asStringValue(); 289 if (codeString == null || "".equals(codeString)) 290 return null; 291 if ("not-present".equals(codeString)) 292 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.NOTPRESENT); 293 if ("example".equals(codeString)) 294 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.EXAMPLE); 295 if ("fragment".equals(codeString)) 296 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.FRAGMENT); 297 if ("complete".equals(codeString)) 298 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.COMPLETE); 299 throw new FHIRException("Unknown CodeSystemContentMode code '"+codeString+"'"); 300 } 301 public String toCode(CodeSystemContentMode code) { 302 if (code == CodeSystemContentMode.NULL) 303 return null; 304 if (code == CodeSystemContentMode.NOTPRESENT) 305 return "not-present"; 306 if (code == CodeSystemContentMode.EXAMPLE) 307 return "example"; 308 if (code == CodeSystemContentMode.FRAGMENT) 309 return "fragment"; 310 if (code == CodeSystemContentMode.COMPLETE) 311 return "complete"; 312 return "?"; 313 } 314 public String toSystem(CodeSystemContentMode code) { 315 return code.getSystem(); 316 } 317 } 318 319 public enum FilterOperator { 320 /** 321 * The specified property of the code equals the provided value. 322 */ 323 EQUAL, 324 /** 325 * Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (i.e. include child codes) 326 */ 327 ISA, 328 /** 329 * Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself (i.e. include child codes) 330 */ 331 DESCENDENTOF, 332 /** 333 * The specified property of the code does not have an is-a relationship with the provided value. 334 */ 335 ISNOTA, 336 /** 337 * The specified property of the code matches the regex specified in the provided value. 338 */ 339 REGEX, 340 /** 341 * The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list). 342 */ 343 IN, 344 /** 345 * The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list). 346 */ 347 NOTIN, 348 /** 349 * Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (e.g. include parent codes) 350 */ 351 GENERALIZES, 352 /** 353 * The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values) 354 */ 355 EXISTS, 356 /** 357 * added to help the parsers with the generic types 358 */ 359 NULL; 360 public static FilterOperator fromCode(String codeString) throws FHIRException { 361 if (codeString == null || "".equals(codeString)) 362 return null; 363 if ("=".equals(codeString)) 364 return EQUAL; 365 if ("is-a".equals(codeString)) 366 return ISA; 367 if ("descendent-of".equals(codeString)) 368 return DESCENDENTOF; 369 if ("is-not-a".equals(codeString)) 370 return ISNOTA; 371 if ("regex".equals(codeString)) 372 return REGEX; 373 if ("in".equals(codeString)) 374 return IN; 375 if ("not-in".equals(codeString)) 376 return NOTIN; 377 if ("generalizes".equals(codeString)) 378 return GENERALIZES; 379 if ("exists".equals(codeString)) 380 return EXISTS; 381 if (Configuration.isAcceptInvalidEnums()) 382 return null; 383 else 384 throw new FHIRException("Unknown FilterOperator code '"+codeString+"'"); 385 } 386 public String toCode() { 387 switch (this) { 388 case EQUAL: return "="; 389 case ISA: return "is-a"; 390 case DESCENDENTOF: return "descendent-of"; 391 case ISNOTA: return "is-not-a"; 392 case REGEX: return "regex"; 393 case IN: return "in"; 394 case NOTIN: return "not-in"; 395 case GENERALIZES: return "generalizes"; 396 case EXISTS: return "exists"; 397 case NULL: return null; 398 default: return "?"; 399 } 400 } 401 public String getSystem() { 402 switch (this) { 403 case EQUAL: return "http://hl7.org/fhir/filter-operator"; 404 case ISA: return "http://hl7.org/fhir/filter-operator"; 405 case DESCENDENTOF: return "http://hl7.org/fhir/filter-operator"; 406 case ISNOTA: return "http://hl7.org/fhir/filter-operator"; 407 case REGEX: return "http://hl7.org/fhir/filter-operator"; 408 case IN: return "http://hl7.org/fhir/filter-operator"; 409 case NOTIN: return "http://hl7.org/fhir/filter-operator"; 410 case GENERALIZES: return "http://hl7.org/fhir/filter-operator"; 411 case EXISTS: return "http://hl7.org/fhir/filter-operator"; 412 case NULL: return null; 413 default: return "?"; 414 } 415 } 416 public String getDefinition() { 417 switch (this) { 418 case EQUAL: return "The specified property of the code equals the provided value."; 419 case ISA: return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (i.e. include child codes)"; 420 case DESCENDENTOF: return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself (i.e. include child codes)"; 421 case ISNOTA: return "The specified property of the code does not have an is-a relationship with the provided value."; 422 case REGEX: return "The specified property of the code matches the regex specified in the provided value."; 423 case IN: return "The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list)."; 424 case NOTIN: return "The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list)."; 425 case GENERALIZES: return "Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (e.g. include parent codes)"; 426 case EXISTS: return "The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values)"; 427 case NULL: return null; 428 default: return "?"; 429 } 430 } 431 public String getDisplay() { 432 switch (this) { 433 case EQUAL: return "Equals"; 434 case ISA: return "Is A (by subsumption)"; 435 case DESCENDENTOF: return "Descendent Of (by subsumption)"; 436 case ISNOTA: return "Not (Is A) (by subsumption)"; 437 case REGEX: return "Regular Expression"; 438 case IN: return "In Set"; 439 case NOTIN: return "Not in Set"; 440 case GENERALIZES: return "Generalizes (by Subsumption)"; 441 case EXISTS: return "Exists"; 442 case NULL: return null; 443 default: return "?"; 444 } 445 } 446 } 447 448 public static class FilterOperatorEnumFactory implements EnumFactory<FilterOperator> { 449 public FilterOperator fromCode(String codeString) throws IllegalArgumentException { 450 if (codeString == null || "".equals(codeString)) 451 if (codeString == null || "".equals(codeString)) 452 return null; 453 if ("=".equals(codeString)) 454 return FilterOperator.EQUAL; 455 if ("is-a".equals(codeString)) 456 return FilterOperator.ISA; 457 if ("descendent-of".equals(codeString)) 458 return FilterOperator.DESCENDENTOF; 459 if ("is-not-a".equals(codeString)) 460 return FilterOperator.ISNOTA; 461 if ("regex".equals(codeString)) 462 return FilterOperator.REGEX; 463 if ("in".equals(codeString)) 464 return FilterOperator.IN; 465 if ("not-in".equals(codeString)) 466 return FilterOperator.NOTIN; 467 if ("generalizes".equals(codeString)) 468 return FilterOperator.GENERALIZES; 469 if ("exists".equals(codeString)) 470 return FilterOperator.EXISTS; 471 throw new IllegalArgumentException("Unknown FilterOperator code '"+codeString+"'"); 472 } 473 public Enumeration<FilterOperator> fromType(PrimitiveType<?> code) throws FHIRException { 474 if (code == null) 475 return null; 476 if (code.isEmpty()) 477 return new Enumeration<FilterOperator>(this); 478 String codeString = code.asStringValue(); 479 if (codeString == null || "".equals(codeString)) 480 return null; 481 if ("=".equals(codeString)) 482 return new Enumeration<FilterOperator>(this, FilterOperator.EQUAL); 483 if ("is-a".equals(codeString)) 484 return new Enumeration<FilterOperator>(this, FilterOperator.ISA); 485 if ("descendent-of".equals(codeString)) 486 return new Enumeration<FilterOperator>(this, FilterOperator.DESCENDENTOF); 487 if ("is-not-a".equals(codeString)) 488 return new Enumeration<FilterOperator>(this, FilterOperator.ISNOTA); 489 if ("regex".equals(codeString)) 490 return new Enumeration<FilterOperator>(this, FilterOperator.REGEX); 491 if ("in".equals(codeString)) 492 return new Enumeration<FilterOperator>(this, FilterOperator.IN); 493 if ("not-in".equals(codeString)) 494 return new Enumeration<FilterOperator>(this, FilterOperator.NOTIN); 495 if ("generalizes".equals(codeString)) 496 return new Enumeration<FilterOperator>(this, FilterOperator.GENERALIZES); 497 if ("exists".equals(codeString)) 498 return new Enumeration<FilterOperator>(this, FilterOperator.EXISTS); 499 throw new FHIRException("Unknown FilterOperator code '"+codeString+"'"); 500 } 501 public String toCode(FilterOperator code) { 502 if (code == FilterOperator.NULL) 503 return null; 504 if (code == FilterOperator.EQUAL) 505 return "="; 506 if (code == FilterOperator.ISA) 507 return "is-a"; 508 if (code == FilterOperator.DESCENDENTOF) 509 return "descendent-of"; 510 if (code == FilterOperator.ISNOTA) 511 return "is-not-a"; 512 if (code == FilterOperator.REGEX) 513 return "regex"; 514 if (code == FilterOperator.IN) 515 return "in"; 516 if (code == FilterOperator.NOTIN) 517 return "not-in"; 518 if (code == FilterOperator.GENERALIZES) 519 return "generalizes"; 520 if (code == FilterOperator.EXISTS) 521 return "exists"; 522 return "?"; 523 } 524 public String toSystem(FilterOperator code) { 525 return code.getSystem(); 526 } 527 } 528 529 public enum PropertyType { 530 /** 531 * The property value is a code that identifies a concept defined in the code system 532 */ 533 CODE, 534 /** 535 * The property value is a code defined in an external code system. This may be used for translations, but is not the intent 536 */ 537 CODING, 538 /** 539 * The property value is a string 540 */ 541 STRING, 542 /** 543 * The property value is a string (often used to assign ranking values to concepts for supporting score assessments) 544 */ 545 INTEGER, 546 /** 547 * The property value is a boolean true | false 548 */ 549 BOOLEAN, 550 /** 551 * The property is a date or a date + time 552 */ 553 DATETIME, 554 /** 555 * added to help the parsers with the generic types 556 */ 557 NULL; 558 public static PropertyType fromCode(String codeString) throws FHIRException { 559 if (codeString == null || "".equals(codeString)) 560 return null; 561 if ("code".equals(codeString)) 562 return CODE; 563 if ("Coding".equals(codeString)) 564 return CODING; 565 if ("string".equals(codeString)) 566 return STRING; 567 if ("integer".equals(codeString)) 568 return INTEGER; 569 if ("boolean".equals(codeString)) 570 return BOOLEAN; 571 if ("dateTime".equals(codeString)) 572 return DATETIME; 573 if (Configuration.isAcceptInvalidEnums()) 574 return null; 575 else 576 throw new FHIRException("Unknown PropertyType code '"+codeString+"'"); 577 } 578 public String toCode() { 579 switch (this) { 580 case CODE: return "code"; 581 case CODING: return "Coding"; 582 case STRING: return "string"; 583 case INTEGER: return "integer"; 584 case BOOLEAN: return "boolean"; 585 case DATETIME: return "dateTime"; 586 case NULL: return null; 587 default: return "?"; 588 } 589 } 590 public String getSystem() { 591 switch (this) { 592 case CODE: return "http://hl7.org/fhir/concept-property-type"; 593 case CODING: return "http://hl7.org/fhir/concept-property-type"; 594 case STRING: return "http://hl7.org/fhir/concept-property-type"; 595 case INTEGER: return "http://hl7.org/fhir/concept-property-type"; 596 case BOOLEAN: return "http://hl7.org/fhir/concept-property-type"; 597 case DATETIME: return "http://hl7.org/fhir/concept-property-type"; 598 case NULL: return null; 599 default: return "?"; 600 } 601 } 602 public String getDefinition() { 603 switch (this) { 604 case CODE: return "The property value is a code that identifies a concept defined in the code system"; 605 case CODING: return "The property value is a code defined in an external code system. This may be used for translations, but is not the intent"; 606 case STRING: return "The property value is a string"; 607 case INTEGER: return "The property value is a string (often used to assign ranking values to concepts for supporting score assessments)"; 608 case BOOLEAN: return "The property value is a boolean true | false"; 609 case DATETIME: return "The property is a date or a date + time"; 610 case NULL: return null; 611 default: return "?"; 612 } 613 } 614 public String getDisplay() { 615 switch (this) { 616 case CODE: return "code (internal reference)"; 617 case CODING: return "Coding (external reference)"; 618 case STRING: return "string"; 619 case INTEGER: return "integer"; 620 case BOOLEAN: return "boolean"; 621 case DATETIME: return "dateTime"; 622 case NULL: return null; 623 default: return "?"; 624 } 625 } 626 } 627 628 public static class PropertyTypeEnumFactory implements EnumFactory<PropertyType> { 629 public PropertyType fromCode(String codeString) throws IllegalArgumentException { 630 if (codeString == null || "".equals(codeString)) 631 if (codeString == null || "".equals(codeString)) 632 return null; 633 if ("code".equals(codeString)) 634 return PropertyType.CODE; 635 if ("Coding".equals(codeString)) 636 return PropertyType.CODING; 637 if ("string".equals(codeString)) 638 return PropertyType.STRING; 639 if ("integer".equals(codeString)) 640 return PropertyType.INTEGER; 641 if ("boolean".equals(codeString)) 642 return PropertyType.BOOLEAN; 643 if ("dateTime".equals(codeString)) 644 return PropertyType.DATETIME; 645 throw new IllegalArgumentException("Unknown PropertyType code '"+codeString+"'"); 646 } 647 public Enumeration<PropertyType> fromType(PrimitiveType<?> code) throws FHIRException { 648 if (code == null) 649 return null; 650 if (code.isEmpty()) 651 return new Enumeration<PropertyType>(this); 652 String codeString = code.asStringValue(); 653 if (codeString == null || "".equals(codeString)) 654 return null; 655 if ("code".equals(codeString)) 656 return new Enumeration<PropertyType>(this, PropertyType.CODE); 657 if ("Coding".equals(codeString)) 658 return new Enumeration<PropertyType>(this, PropertyType.CODING); 659 if ("string".equals(codeString)) 660 return new Enumeration<PropertyType>(this, PropertyType.STRING); 661 if ("integer".equals(codeString)) 662 return new Enumeration<PropertyType>(this, PropertyType.INTEGER); 663 if ("boolean".equals(codeString)) 664 return new Enumeration<PropertyType>(this, PropertyType.BOOLEAN); 665 if ("dateTime".equals(codeString)) 666 return new Enumeration<PropertyType>(this, PropertyType.DATETIME); 667 throw new FHIRException("Unknown PropertyType code '"+codeString+"'"); 668 } 669 public String toCode(PropertyType code) { 670 if (code == PropertyType.NULL) 671 return null; 672 if (code == PropertyType.CODE) 673 return "code"; 674 if (code == PropertyType.CODING) 675 return "Coding"; 676 if (code == PropertyType.STRING) 677 return "string"; 678 if (code == PropertyType.INTEGER) 679 return "integer"; 680 if (code == PropertyType.BOOLEAN) 681 return "boolean"; 682 if (code == PropertyType.DATETIME) 683 return "dateTime"; 684 return "?"; 685 } 686 public String toSystem(PropertyType code) { 687 return code.getSystem(); 688 } 689 } 690 691 @Block() 692 public static class CodeSystemFilterComponent extends BackboneElement implements IBaseBackboneElement { 693 /** 694 * The code that identifies this filter when it is used in the instance. 695 */ 696 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 697 @Description(shortDefinition="Code that identifies the filter", formalDefinition="The code that identifies this filter when it is used in the instance." ) 698 protected CodeType code; 699 700 /** 701 * A description of how or why the filter is used. 702 */ 703 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 704 @Description(shortDefinition="How or why the filter is used", formalDefinition="A description of how or why the filter is used." ) 705 protected StringType description; 706 707 /** 708 * A list of operators that can be used with the filter. 709 */ 710 @Child(name = "operator", type = {CodeType.class}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 711 @Description(shortDefinition="Operators that can be used with filter", formalDefinition="A list of operators that can be used with the filter." ) 712 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/filter-operator") 713 protected List<Enumeration<FilterOperator>> operator; 714 715 /** 716 * A description of what the value for the filter should be. 717 */ 718 @Child(name = "value", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 719 @Description(shortDefinition="What to use for the value", formalDefinition="A description of what the value for the filter should be." ) 720 protected StringType value; 721 722 private static final long serialVersionUID = -1087409836L; 723 724 /** 725 * Constructor 726 */ 727 public CodeSystemFilterComponent() { 728 super(); 729 } 730 731 /** 732 * Constructor 733 */ 734 public CodeSystemFilterComponent(CodeType code, StringType value) { 735 super(); 736 this.code = code; 737 this.value = value; 738 } 739 740 /** 741 * @return {@link #code} (The code that identifies this filter when it is used in the instance.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 742 */ 743 public CodeType getCodeElement() { 744 if (this.code == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create CodeSystemFilterComponent.code"); 747 else if (Configuration.doAutoCreate()) 748 this.code = new CodeType(); // bb 749 return this.code; 750 } 751 752 public boolean hasCodeElement() { 753 return this.code != null && !this.code.isEmpty(); 754 } 755 756 public boolean hasCode() { 757 return this.code != null && !this.code.isEmpty(); 758 } 759 760 /** 761 * @param value {@link #code} (The code that identifies this filter when it is used in the instance.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 762 */ 763 public CodeSystemFilterComponent setCodeElement(CodeType value) { 764 this.code = value; 765 return this; 766 } 767 768 /** 769 * @return The code that identifies this filter when it is used in the instance. 770 */ 771 public String getCode() { 772 return this.code == null ? null : this.code.getValue(); 773 } 774 775 /** 776 * @param value The code that identifies this filter when it is used in the instance. 777 */ 778 public CodeSystemFilterComponent setCode(String value) { 779 if (this.code == null) 780 this.code = new CodeType(); 781 this.code.setValue(value); 782 return this; 783 } 784 785 /** 786 * @return {@link #description} (A description of how or why the filter is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 787 */ 788 public StringType getDescriptionElement() { 789 if (this.description == null) 790 if (Configuration.errorOnAutoCreate()) 791 throw new Error("Attempt to auto-create CodeSystemFilterComponent.description"); 792 else if (Configuration.doAutoCreate()) 793 this.description = new StringType(); // bb 794 return this.description; 795 } 796 797 public boolean hasDescriptionElement() { 798 return this.description != null && !this.description.isEmpty(); 799 } 800 801 public boolean hasDescription() { 802 return this.description != null && !this.description.isEmpty(); 803 } 804 805 /** 806 * @param value {@link #description} (A description of how or why the filter is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 807 */ 808 public CodeSystemFilterComponent setDescriptionElement(StringType value) { 809 this.description = value; 810 return this; 811 } 812 813 /** 814 * @return A description of how or why the filter is used. 815 */ 816 public String getDescription() { 817 return this.description == null ? null : this.description.getValue(); 818 } 819 820 /** 821 * @param value A description of how or why the filter is used. 822 */ 823 public CodeSystemFilterComponent setDescription(String value) { 824 if (Utilities.noString(value)) 825 this.description = null; 826 else { 827 if (this.description == null) 828 this.description = new StringType(); 829 this.description.setValue(value); 830 } 831 return this; 832 } 833 834 /** 835 * @return {@link #operator} (A list of operators that can be used with the filter.) 836 */ 837 public List<Enumeration<FilterOperator>> getOperator() { 838 if (this.operator == null) 839 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 840 return this.operator; 841 } 842 843 /** 844 * @return Returns a reference to <code>this</code> for easy method chaining 845 */ 846 public CodeSystemFilterComponent setOperator(List<Enumeration<FilterOperator>> theOperator) { 847 this.operator = theOperator; 848 return this; 849 } 850 851 public boolean hasOperator() { 852 if (this.operator == null) 853 return false; 854 for (Enumeration<FilterOperator> item : this.operator) 855 if (!item.isEmpty()) 856 return true; 857 return false; 858 } 859 860 /** 861 * @return {@link #operator} (A list of operators that can be used with the filter.) 862 */ 863 public Enumeration<FilterOperator> addOperatorElement() {//2 864 Enumeration<FilterOperator> t = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 865 if (this.operator == null) 866 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 867 this.operator.add(t); 868 return t; 869 } 870 871 /** 872 * @param value {@link #operator} (A list of operators that can be used with the filter.) 873 */ 874 public CodeSystemFilterComponent addOperator(FilterOperator value) { //1 875 Enumeration<FilterOperator> t = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 876 t.setValue(value); 877 if (this.operator == null) 878 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 879 this.operator.add(t); 880 return this; 881 } 882 883 /** 884 * @param value {@link #operator} (A list of operators that can be used with the filter.) 885 */ 886 public boolean hasOperator(FilterOperator value) { 887 if (this.operator == null) 888 return false; 889 for (Enumeration<FilterOperator> v : this.operator) 890 if (v.getValue().equals(value)) // code 891 return true; 892 return false; 893 } 894 895 /** 896 * @return {@link #value} (A description of what the value for the filter should be.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 897 */ 898 public StringType getValueElement() { 899 if (this.value == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create CodeSystemFilterComponent.value"); 902 else if (Configuration.doAutoCreate()) 903 this.value = new StringType(); // bb 904 return this.value; 905 } 906 907 public boolean hasValueElement() { 908 return this.value != null && !this.value.isEmpty(); 909 } 910 911 public boolean hasValue() { 912 return this.value != null && !this.value.isEmpty(); 913 } 914 915 /** 916 * @param value {@link #value} (A description of what the value for the filter should be.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 917 */ 918 public CodeSystemFilterComponent setValueElement(StringType value) { 919 this.value = value; 920 return this; 921 } 922 923 /** 924 * @return A description of what the value for the filter should be. 925 */ 926 public String getValue() { 927 return this.value == null ? null : this.value.getValue(); 928 } 929 930 /** 931 * @param value A description of what the value for the filter should be. 932 */ 933 public CodeSystemFilterComponent setValue(String value) { 934 if (this.value == null) 935 this.value = new StringType(); 936 this.value.setValue(value); 937 return this; 938 } 939 940 protected void listChildren(List<Property> children) { 941 super.listChildren(children); 942 children.add(new Property("code", "code", "The code that identifies this filter when it is used in the instance.", 0, 1, code)); 943 children.add(new Property("description", "string", "A description of how or why the filter is used.", 0, 1, description)); 944 children.add(new Property("operator", "code", "A list of operators that can be used with the filter.", 0, java.lang.Integer.MAX_VALUE, operator)); 945 children.add(new Property("value", "string", "A description of what the value for the filter should be.", 0, 1, value)); 946 } 947 948 @Override 949 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 950 switch (_hash) { 951 case 3059181: /*code*/ return new Property("code", "code", "The code that identifies this filter when it is used in the instance.", 0, 1, code); 952 case -1724546052: /*description*/ return new Property("description", "string", "A description of how or why the filter is used.", 0, 1, description); 953 case -500553564: /*operator*/ return new Property("operator", "code", "A list of operators that can be used with the filter.", 0, java.lang.Integer.MAX_VALUE, operator); 954 case 111972721: /*value*/ return new Property("value", "string", "A description of what the value for the filter should be.", 0, 1, value); 955 default: return super.getNamedProperty(_hash, _name, _checkValid); 956 } 957 958 } 959 960 @Override 961 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 962 switch (hash) { 963 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 964 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 965 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : this.operator.toArray(new Base[this.operator.size()]); // Enumeration<FilterOperator> 966 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 967 default: return super.getProperty(hash, name, checkValid); 968 } 969 970 } 971 972 @Override 973 public Base setProperty(int hash, String name, Base value) throws FHIRException { 974 switch (hash) { 975 case 3059181: // code 976 this.code = castToCode(value); // CodeType 977 return value; 978 case -1724546052: // description 979 this.description = castToString(value); // StringType 980 return value; 981 case -500553564: // operator 982 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 983 this.getOperator().add((Enumeration) value); // Enumeration<FilterOperator> 984 return value; 985 case 111972721: // value 986 this.value = castToString(value); // StringType 987 return value; 988 default: return super.setProperty(hash, name, value); 989 } 990 991 } 992 993 @Override 994 public Base setProperty(String name, Base value) throws FHIRException { 995 if (name.equals("code")) { 996 this.code = castToCode(value); // CodeType 997 } else if (name.equals("description")) { 998 this.description = castToString(value); // StringType 999 } else if (name.equals("operator")) { 1000 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 1001 this.getOperator().add((Enumeration) value); 1002 } else if (name.equals("value")) { 1003 this.value = castToString(value); // StringType 1004 } else 1005 return super.setProperty(name, value); 1006 return value; 1007 } 1008 1009 @Override 1010 public Base makeProperty(int hash, String name) throws FHIRException { 1011 switch (hash) { 1012 case 3059181: return getCodeElement(); 1013 case -1724546052: return getDescriptionElement(); 1014 case -500553564: return addOperatorElement(); 1015 case 111972721: return getValueElement(); 1016 default: return super.makeProperty(hash, name); 1017 } 1018 1019 } 1020 1021 @Override 1022 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1023 switch (hash) { 1024 case 3059181: /*code*/ return new String[] {"code"}; 1025 case -1724546052: /*description*/ return new String[] {"string"}; 1026 case -500553564: /*operator*/ return new String[] {"code"}; 1027 case 111972721: /*value*/ return new String[] {"string"}; 1028 default: return super.getTypesForProperty(hash, name); 1029 } 1030 1031 } 1032 1033 @Override 1034 public Base addChild(String name) throws FHIRException { 1035 if (name.equals("code")) { 1036 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 1037 } 1038 else if (name.equals("description")) { 1039 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.description"); 1040 } 1041 else if (name.equals("operator")) { 1042 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.operator"); 1043 } 1044 else if (name.equals("value")) { 1045 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.value"); 1046 } 1047 else 1048 return super.addChild(name); 1049 } 1050 1051 public CodeSystemFilterComponent copy() { 1052 CodeSystemFilterComponent dst = new CodeSystemFilterComponent(); 1053 copyValues(dst); 1054 dst.code = code == null ? null : code.copy(); 1055 dst.description = description == null ? null : description.copy(); 1056 if (operator != null) { 1057 dst.operator = new ArrayList<Enumeration<FilterOperator>>(); 1058 for (Enumeration<FilterOperator> i : operator) 1059 dst.operator.add(i.copy()); 1060 }; 1061 dst.value = value == null ? null : value.copy(); 1062 return dst; 1063 } 1064 1065 @Override 1066 public boolean equalsDeep(Base other_) { 1067 if (!super.equalsDeep(other_)) 1068 return false; 1069 if (!(other_ instanceof CodeSystemFilterComponent)) 1070 return false; 1071 CodeSystemFilterComponent o = (CodeSystemFilterComponent) other_; 1072 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true) && compareDeep(operator, o.operator, true) 1073 && compareDeep(value, o.value, true); 1074 } 1075 1076 @Override 1077 public boolean equalsShallow(Base other_) { 1078 if (!super.equalsShallow(other_)) 1079 return false; 1080 if (!(other_ instanceof CodeSystemFilterComponent)) 1081 return false; 1082 CodeSystemFilterComponent o = (CodeSystemFilterComponent) other_; 1083 return compareValues(code, o.code, true) && compareValues(description, o.description, true) && compareValues(operator, o.operator, true) 1084 && compareValues(value, o.value, true); 1085 } 1086 1087 public boolean isEmpty() { 1088 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description, operator 1089 , value); 1090 } 1091 1092 public String fhirType() { 1093 return "CodeSystem.filter"; 1094 1095 } 1096 1097 } 1098 1099 @Block() 1100 public static class PropertyComponent extends BackboneElement implements IBaseBackboneElement { 1101 /** 1102 * A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters. 1103 */ 1104 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1105 @Description(shortDefinition="Identifies the property on the concepts, and when referred to in operations", formalDefinition="A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters." ) 1106 protected CodeType code; 1107 1108 /** 1109 * Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 1110 */ 1111 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1112 @Description(shortDefinition="Formal identifier for the property", formalDefinition="Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system." ) 1113 protected UriType uri; 1114 1115 /** 1116 * A description of the property- why it is defined, and how its value might be used. 1117 */ 1118 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1119 @Description(shortDefinition="Why the property is defined, and/or what it conveys", formalDefinition="A description of the property- why it is defined, and how its value might be used." ) 1120 protected StringType description; 1121 1122 /** 1123 * The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to anotherr defined concept). 1124 */ 1125 @Child(name = "type", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1126 @Description(shortDefinition="code | Coding | string | integer | boolean | dateTime", formalDefinition="The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to anotherr defined concept)." ) 1127 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/concept-property-type") 1128 protected Enumeration<PropertyType> type; 1129 1130 private static final long serialVersionUID = -1810713373L; 1131 1132 /** 1133 * Constructor 1134 */ 1135 public PropertyComponent() { 1136 super(); 1137 } 1138 1139 /** 1140 * Constructor 1141 */ 1142 public PropertyComponent(CodeType code, Enumeration<PropertyType> type) { 1143 super(); 1144 this.code = code; 1145 this.type = type; 1146 } 1147 1148 /** 1149 * @return {@link #code} (A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1150 */ 1151 public CodeType getCodeElement() { 1152 if (this.code == null) 1153 if (Configuration.errorOnAutoCreate()) 1154 throw new Error("Attempt to auto-create PropertyComponent.code"); 1155 else if (Configuration.doAutoCreate()) 1156 this.code = new CodeType(); // bb 1157 return this.code; 1158 } 1159 1160 public boolean hasCodeElement() { 1161 return this.code != null && !this.code.isEmpty(); 1162 } 1163 1164 public boolean hasCode() { 1165 return this.code != null && !this.code.isEmpty(); 1166 } 1167 1168 /** 1169 * @param value {@link #code} (A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1170 */ 1171 public PropertyComponent setCodeElement(CodeType value) { 1172 this.code = value; 1173 return this; 1174 } 1175 1176 /** 1177 * @return A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters. 1178 */ 1179 public String getCode() { 1180 return this.code == null ? null : this.code.getValue(); 1181 } 1182 1183 /** 1184 * @param value A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters. 1185 */ 1186 public PropertyComponent setCode(String value) { 1187 if (this.code == null) 1188 this.code = new CodeType(); 1189 this.code.setValue(value); 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #uri} (Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 1195 */ 1196 public UriType getUriElement() { 1197 if (this.uri == null) 1198 if (Configuration.errorOnAutoCreate()) 1199 throw new Error("Attempt to auto-create PropertyComponent.uri"); 1200 else if (Configuration.doAutoCreate()) 1201 this.uri = new UriType(); // bb 1202 return this.uri; 1203 } 1204 1205 public boolean hasUriElement() { 1206 return this.uri != null && !this.uri.isEmpty(); 1207 } 1208 1209 public boolean hasUri() { 1210 return this.uri != null && !this.uri.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #uri} (Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 1215 */ 1216 public PropertyComponent setUriElement(UriType value) { 1217 this.uri = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 1223 */ 1224 public String getUri() { 1225 return this.uri == null ? null : this.uri.getValue(); 1226 } 1227 1228 /** 1229 * @param value Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 1230 */ 1231 public PropertyComponent setUri(String value) { 1232 if (Utilities.noString(value)) 1233 this.uri = null; 1234 else { 1235 if (this.uri == null) 1236 this.uri = new UriType(); 1237 this.uri.setValue(value); 1238 } 1239 return this; 1240 } 1241 1242 /** 1243 * @return {@link #description} (A description of the property- why it is defined, and how its value might be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1244 */ 1245 public StringType getDescriptionElement() { 1246 if (this.description == null) 1247 if (Configuration.errorOnAutoCreate()) 1248 throw new Error("Attempt to auto-create PropertyComponent.description"); 1249 else if (Configuration.doAutoCreate()) 1250 this.description = new StringType(); // bb 1251 return this.description; 1252 } 1253 1254 public boolean hasDescriptionElement() { 1255 return this.description != null && !this.description.isEmpty(); 1256 } 1257 1258 public boolean hasDescription() { 1259 return this.description != null && !this.description.isEmpty(); 1260 } 1261 1262 /** 1263 * @param value {@link #description} (A description of the property- why it is defined, and how its value might be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1264 */ 1265 public PropertyComponent setDescriptionElement(StringType value) { 1266 this.description = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return A description of the property- why it is defined, and how its value might be used. 1272 */ 1273 public String getDescription() { 1274 return this.description == null ? null : this.description.getValue(); 1275 } 1276 1277 /** 1278 * @param value A description of the property- why it is defined, and how its value might be used. 1279 */ 1280 public PropertyComponent setDescription(String value) { 1281 if (Utilities.noString(value)) 1282 this.description = null; 1283 else { 1284 if (this.description == null) 1285 this.description = new StringType(); 1286 this.description.setValue(value); 1287 } 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #type} (The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to anotherr defined concept).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1293 */ 1294 public Enumeration<PropertyType> getTypeElement() { 1295 if (this.type == null) 1296 if (Configuration.errorOnAutoCreate()) 1297 throw new Error("Attempt to auto-create PropertyComponent.type"); 1298 else if (Configuration.doAutoCreate()) 1299 this.type = new Enumeration<PropertyType>(new PropertyTypeEnumFactory()); // bb 1300 return this.type; 1301 } 1302 1303 public boolean hasTypeElement() { 1304 return this.type != null && !this.type.isEmpty(); 1305 } 1306 1307 public boolean hasType() { 1308 return this.type != null && !this.type.isEmpty(); 1309 } 1310 1311 /** 1312 * @param value {@link #type} (The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to anotherr defined concept).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1313 */ 1314 public PropertyComponent setTypeElement(Enumeration<PropertyType> value) { 1315 this.type = value; 1316 return this; 1317 } 1318 1319 /** 1320 * @return The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to anotherr defined concept). 1321 */ 1322 public PropertyType getType() { 1323 return this.type == null ? null : this.type.getValue(); 1324 } 1325 1326 /** 1327 * @param value The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to anotherr defined concept). 1328 */ 1329 public PropertyComponent setType(PropertyType value) { 1330 if (this.type == null) 1331 this.type = new Enumeration<PropertyType>(new PropertyTypeEnumFactory()); 1332 this.type.setValue(value); 1333 return this; 1334 } 1335 1336 protected void listChildren(List<Property> children) { 1337 super.listChildren(children); 1338 children.add(new Property("code", "code", "A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.", 0, 1, code)); 1339 children.add(new Property("uri", "uri", "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 0, 1, uri)); 1340 children.add(new Property("description", "string", "A description of the property- why it is defined, and how its value might be used.", 0, 1, description)); 1341 children.add(new Property("type", "code", "The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to anotherr defined concept).", 0, 1, type)); 1342 } 1343 1344 @Override 1345 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1346 switch (_hash) { 1347 case 3059181: /*code*/ return new Property("code", "code", "A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.", 0, 1, code); 1348 case 116076: /*uri*/ return new Property("uri", "uri", "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 0, 1, uri); 1349 case -1724546052: /*description*/ return new Property("description", "string", "A description of the property- why it is defined, and how its value might be used.", 0, 1, description); 1350 case 3575610: /*type*/ return new Property("type", "code", "The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to anotherr defined concept).", 0, 1, type); 1351 default: return super.getNamedProperty(_hash, _name, _checkValid); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1358 switch (hash) { 1359 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1360 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 1361 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1362 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<PropertyType> 1363 default: return super.getProperty(hash, name, checkValid); 1364 } 1365 1366 } 1367 1368 @Override 1369 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1370 switch (hash) { 1371 case 3059181: // code 1372 this.code = castToCode(value); // CodeType 1373 return value; 1374 case 116076: // uri 1375 this.uri = castToUri(value); // UriType 1376 return value; 1377 case -1724546052: // description 1378 this.description = castToString(value); // StringType 1379 return value; 1380 case 3575610: // type 1381 value = new PropertyTypeEnumFactory().fromType(castToCode(value)); 1382 this.type = (Enumeration) value; // Enumeration<PropertyType> 1383 return value; 1384 default: return super.setProperty(hash, name, value); 1385 } 1386 1387 } 1388 1389 @Override 1390 public Base setProperty(String name, Base value) throws FHIRException { 1391 if (name.equals("code")) { 1392 this.code = castToCode(value); // CodeType 1393 } else if (name.equals("uri")) { 1394 this.uri = castToUri(value); // UriType 1395 } else if (name.equals("description")) { 1396 this.description = castToString(value); // StringType 1397 } else if (name.equals("type")) { 1398 value = new PropertyTypeEnumFactory().fromType(castToCode(value)); 1399 this.type = (Enumeration) value; // Enumeration<PropertyType> 1400 } else 1401 return super.setProperty(name, value); 1402 return value; 1403 } 1404 1405 @Override 1406 public Base makeProperty(int hash, String name) throws FHIRException { 1407 switch (hash) { 1408 case 3059181: return getCodeElement(); 1409 case 116076: return getUriElement(); 1410 case -1724546052: return getDescriptionElement(); 1411 case 3575610: return getTypeElement(); 1412 default: return super.makeProperty(hash, name); 1413 } 1414 1415 } 1416 1417 @Override 1418 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1419 switch (hash) { 1420 case 3059181: /*code*/ return new String[] {"code"}; 1421 case 116076: /*uri*/ return new String[] {"uri"}; 1422 case -1724546052: /*description*/ return new String[] {"string"}; 1423 case 3575610: /*type*/ return new String[] {"code"}; 1424 default: return super.getTypesForProperty(hash, name); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base addChild(String name) throws FHIRException { 1431 if (name.equals("code")) { 1432 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 1433 } 1434 else if (name.equals("uri")) { 1435 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.uri"); 1436 } 1437 else if (name.equals("description")) { 1438 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.description"); 1439 } 1440 else if (name.equals("type")) { 1441 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.type"); 1442 } 1443 else 1444 return super.addChild(name); 1445 } 1446 1447 public PropertyComponent copy() { 1448 PropertyComponent dst = new PropertyComponent(); 1449 copyValues(dst); 1450 dst.code = code == null ? null : code.copy(); 1451 dst.uri = uri == null ? null : uri.copy(); 1452 dst.description = description == null ? null : description.copy(); 1453 dst.type = type == null ? null : type.copy(); 1454 return dst; 1455 } 1456 1457 @Override 1458 public boolean equalsDeep(Base other_) { 1459 if (!super.equalsDeep(other_)) 1460 return false; 1461 if (!(other_ instanceof PropertyComponent)) 1462 return false; 1463 PropertyComponent o = (PropertyComponent) other_; 1464 return compareDeep(code, o.code, true) && compareDeep(uri, o.uri, true) && compareDeep(description, o.description, true) 1465 && compareDeep(type, o.type, true); 1466 } 1467 1468 @Override 1469 public boolean equalsShallow(Base other_) { 1470 if (!super.equalsShallow(other_)) 1471 return false; 1472 if (!(other_ instanceof PropertyComponent)) 1473 return false; 1474 PropertyComponent o = (PropertyComponent) other_; 1475 return compareValues(code, o.code, true) && compareValues(uri, o.uri, true) && compareValues(description, o.description, true) 1476 && compareValues(type, o.type, true); 1477 } 1478 1479 public boolean isEmpty() { 1480 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, uri, description, type 1481 ); 1482 } 1483 1484 public String fhirType() { 1485 return "CodeSystem.property"; 1486 1487 } 1488 1489 } 1490 1491 @Block() 1492 public static class ConceptDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 1493 /** 1494 * A code - a text symbol - that uniquely identifies the concept within the code system. 1495 */ 1496 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1497 @Description(shortDefinition="Code that identifies concept", formalDefinition="A code - a text symbol - that uniquely identifies the concept within the code system." ) 1498 protected CodeType code; 1499 1500 /** 1501 * A human readable string that is the recommended default way to present this concept to a user. 1502 */ 1503 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1504 @Description(shortDefinition="Text to display to the user", formalDefinition="A human readable string that is the recommended default way to present this concept to a user." ) 1505 protected StringType display; 1506 1507 /** 1508 * The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept. 1509 */ 1510 @Child(name = "definition", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1511 @Description(shortDefinition="Formal definition", formalDefinition="The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept." ) 1512 protected StringType definition; 1513 1514 /** 1515 * Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc. 1516 */ 1517 @Child(name = "designation", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1518 @Description(shortDefinition="Additional representations for the concept", formalDefinition="Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc." ) 1519 protected List<ConceptDefinitionDesignationComponent> designation; 1520 1521 /** 1522 * A property value for this concept. 1523 */ 1524 @Child(name = "property", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1525 @Description(shortDefinition="Property value for the concept", formalDefinition="A property value for this concept." ) 1526 protected List<ConceptPropertyComponent> property; 1527 1528 /** 1529 * Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning. 1530 */ 1531 @Child(name = "concept", type = {ConceptDefinitionComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1532 @Description(shortDefinition="Child Concepts (is-a/contains/categorizes)", formalDefinition="Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning." ) 1533 protected List<ConceptDefinitionComponent> concept; 1534 1535 private static final long serialVersionUID = 878320988L; 1536 1537 /** 1538 * Constructor 1539 */ 1540 public ConceptDefinitionComponent() { 1541 super(); 1542 } 1543 1544 /** 1545 * Constructor 1546 */ 1547 public ConceptDefinitionComponent(CodeType code) { 1548 super(); 1549 this.code = code; 1550 } 1551 1552 /** 1553 * @return {@link #code} (A code - a text symbol - that uniquely identifies the concept within the code system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1554 */ 1555 public CodeType getCodeElement() { 1556 if (this.code == null) 1557 if (Configuration.errorOnAutoCreate()) 1558 throw new Error("Attempt to auto-create ConceptDefinitionComponent.code"); 1559 else if (Configuration.doAutoCreate()) 1560 this.code = new CodeType(); // bb 1561 return this.code; 1562 } 1563 1564 public boolean hasCodeElement() { 1565 return this.code != null && !this.code.isEmpty(); 1566 } 1567 1568 public boolean hasCode() { 1569 return this.code != null && !this.code.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #code} (A code - a text symbol - that uniquely identifies the concept within the code system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1574 */ 1575 public ConceptDefinitionComponent setCodeElement(CodeType value) { 1576 this.code = value; 1577 return this; 1578 } 1579 1580 /** 1581 * @return A code - a text symbol - that uniquely identifies the concept within the code system. 1582 */ 1583 public String getCode() { 1584 return this.code == null ? null : this.code.getValue(); 1585 } 1586 1587 /** 1588 * @param value A code - a text symbol - that uniquely identifies the concept within the code system. 1589 */ 1590 public ConceptDefinitionComponent setCode(String value) { 1591 if (this.code == null) 1592 this.code = new CodeType(); 1593 this.code.setValue(value); 1594 return this; 1595 } 1596 1597 /** 1598 * @return {@link #display} (A human readable string that is the recommended default way to present this concept to a user.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1599 */ 1600 public StringType getDisplayElement() { 1601 if (this.display == null) 1602 if (Configuration.errorOnAutoCreate()) 1603 throw new Error("Attempt to auto-create ConceptDefinitionComponent.display"); 1604 else if (Configuration.doAutoCreate()) 1605 this.display = new StringType(); // bb 1606 return this.display; 1607 } 1608 1609 public boolean hasDisplayElement() { 1610 return this.display != null && !this.display.isEmpty(); 1611 } 1612 1613 public boolean hasDisplay() { 1614 return this.display != null && !this.display.isEmpty(); 1615 } 1616 1617 /** 1618 * @param value {@link #display} (A human readable string that is the recommended default way to present this concept to a user.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1619 */ 1620 public ConceptDefinitionComponent setDisplayElement(StringType value) { 1621 this.display = value; 1622 return this; 1623 } 1624 1625 /** 1626 * @return A human readable string that is the recommended default way to present this concept to a user. 1627 */ 1628 public String getDisplay() { 1629 return this.display == null ? null : this.display.getValue(); 1630 } 1631 1632 /** 1633 * @param value A human readable string that is the recommended default way to present this concept to a user. 1634 */ 1635 public ConceptDefinitionComponent setDisplay(String value) { 1636 if (Utilities.noString(value)) 1637 this.display = null; 1638 else { 1639 if (this.display == null) 1640 this.display = new StringType(); 1641 this.display.setValue(value); 1642 } 1643 return this; 1644 } 1645 1646 /** 1647 * @return {@link #definition} (The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1648 */ 1649 public StringType getDefinitionElement() { 1650 if (this.definition == null) 1651 if (Configuration.errorOnAutoCreate()) 1652 throw new Error("Attempt to auto-create ConceptDefinitionComponent.definition"); 1653 else if (Configuration.doAutoCreate()) 1654 this.definition = new StringType(); // bb 1655 return this.definition; 1656 } 1657 1658 public boolean hasDefinitionElement() { 1659 return this.definition != null && !this.definition.isEmpty(); 1660 } 1661 1662 public boolean hasDefinition() { 1663 return this.definition != null && !this.definition.isEmpty(); 1664 } 1665 1666 /** 1667 * @param value {@link #definition} (The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1668 */ 1669 public ConceptDefinitionComponent setDefinitionElement(StringType value) { 1670 this.definition = value; 1671 return this; 1672 } 1673 1674 /** 1675 * @return The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept. 1676 */ 1677 public String getDefinition() { 1678 return this.definition == null ? null : this.definition.getValue(); 1679 } 1680 1681 /** 1682 * @param value The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept. 1683 */ 1684 public ConceptDefinitionComponent setDefinition(String value) { 1685 if (Utilities.noString(value)) 1686 this.definition = null; 1687 else { 1688 if (this.definition == null) 1689 this.definition = new StringType(); 1690 this.definition.setValue(value); 1691 } 1692 return this; 1693 } 1694 1695 /** 1696 * @return {@link #designation} (Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.) 1697 */ 1698 public List<ConceptDefinitionDesignationComponent> getDesignation() { 1699 if (this.designation == null) 1700 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1701 return this.designation; 1702 } 1703 1704 /** 1705 * @return Returns a reference to <code>this</code> for easy method chaining 1706 */ 1707 public ConceptDefinitionComponent setDesignation(List<ConceptDefinitionDesignationComponent> theDesignation) { 1708 this.designation = theDesignation; 1709 return this; 1710 } 1711 1712 public boolean hasDesignation() { 1713 if (this.designation == null) 1714 return false; 1715 for (ConceptDefinitionDesignationComponent item : this.designation) 1716 if (!item.isEmpty()) 1717 return true; 1718 return false; 1719 } 1720 1721 public ConceptDefinitionDesignationComponent addDesignation() { //3 1722 ConceptDefinitionDesignationComponent t = new ConceptDefinitionDesignationComponent(); 1723 if (this.designation == null) 1724 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1725 this.designation.add(t); 1726 return t; 1727 } 1728 1729 public ConceptDefinitionComponent addDesignation(ConceptDefinitionDesignationComponent t) { //3 1730 if (t == null) 1731 return this; 1732 if (this.designation == null) 1733 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1734 this.designation.add(t); 1735 return this; 1736 } 1737 1738 /** 1739 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist 1740 */ 1741 public ConceptDefinitionDesignationComponent getDesignationFirstRep() { 1742 if (getDesignation().isEmpty()) { 1743 addDesignation(); 1744 } 1745 return getDesignation().get(0); 1746 } 1747 1748 /** 1749 * @return {@link #property} (A property value for this concept.) 1750 */ 1751 public List<ConceptPropertyComponent> getProperty() { 1752 if (this.property == null) 1753 this.property = new ArrayList<ConceptPropertyComponent>(); 1754 return this.property; 1755 } 1756 1757 /** 1758 * @return Returns a reference to <code>this</code> for easy method chaining 1759 */ 1760 public ConceptDefinitionComponent setProperty(List<ConceptPropertyComponent> theProperty) { 1761 this.property = theProperty; 1762 return this; 1763 } 1764 1765 public boolean hasProperty() { 1766 if (this.property == null) 1767 return false; 1768 for (ConceptPropertyComponent item : this.property) 1769 if (!item.isEmpty()) 1770 return true; 1771 return false; 1772 } 1773 1774 public ConceptPropertyComponent addProperty() { //3 1775 ConceptPropertyComponent t = new ConceptPropertyComponent(); 1776 if (this.property == null) 1777 this.property = new ArrayList<ConceptPropertyComponent>(); 1778 this.property.add(t); 1779 return t; 1780 } 1781 1782 public ConceptDefinitionComponent addProperty(ConceptPropertyComponent t) { //3 1783 if (t == null) 1784 return this; 1785 if (this.property == null) 1786 this.property = new ArrayList<ConceptPropertyComponent>(); 1787 this.property.add(t); 1788 return this; 1789 } 1790 1791 /** 1792 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist 1793 */ 1794 public ConceptPropertyComponent getPropertyFirstRep() { 1795 if (getProperty().isEmpty()) { 1796 addProperty(); 1797 } 1798 return getProperty().get(0); 1799 } 1800 1801 /** 1802 * @return {@link #concept} (Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.) 1803 */ 1804 public List<ConceptDefinitionComponent> getConcept() { 1805 if (this.concept == null) 1806 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1807 return this.concept; 1808 } 1809 1810 /** 1811 * @return Returns a reference to <code>this</code> for easy method chaining 1812 */ 1813 public ConceptDefinitionComponent setConcept(List<ConceptDefinitionComponent> theConcept) { 1814 this.concept = theConcept; 1815 return this; 1816 } 1817 1818 public boolean hasConcept() { 1819 if (this.concept == null) 1820 return false; 1821 for (ConceptDefinitionComponent item : this.concept) 1822 if (!item.isEmpty()) 1823 return true; 1824 return false; 1825 } 1826 1827 public ConceptDefinitionComponent addConcept() { //3 1828 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 1829 if (this.concept == null) 1830 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1831 this.concept.add(t); 1832 return t; 1833 } 1834 1835 public ConceptDefinitionComponent addConcept(ConceptDefinitionComponent t) { //3 1836 if (t == null) 1837 return this; 1838 if (this.concept == null) 1839 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1840 this.concept.add(t); 1841 return this; 1842 } 1843 1844 /** 1845 * @return The first repetition of repeating field {@link #concept}, creating it if it does not already exist 1846 */ 1847 public ConceptDefinitionComponent getConceptFirstRep() { 1848 if (getConcept().isEmpty()) { 1849 addConcept(); 1850 } 1851 return getConcept().get(0); 1852 } 1853 1854 protected void listChildren(List<Property> children) { 1855 super.listChildren(children); 1856 children.add(new Property("code", "code", "A code - a text symbol - that uniquely identifies the concept within the code system.", 0, 1, code)); 1857 children.add(new Property("display", "string", "A human readable string that is the recommended default way to present this concept to a user.", 0, 1, display)); 1858 children.add(new Property("definition", "string", "The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.", 0, 1, definition)); 1859 children.add(new Property("designation", "", "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation)); 1860 children.add(new Property("property", "", "A property value for this concept.", 0, java.lang.Integer.MAX_VALUE, property)); 1861 children.add(new Property("concept", "@CodeSystem.concept", "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.", 0, java.lang.Integer.MAX_VALUE, concept)); 1862 } 1863 1864 @Override 1865 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1866 switch (_hash) { 1867 case 3059181: /*code*/ return new Property("code", "code", "A code - a text symbol - that uniquely identifies the concept within the code system.", 0, 1, code); 1868 case 1671764162: /*display*/ return new Property("display", "string", "A human readable string that is the recommended default way to present this concept to a user.", 0, 1, display); 1869 case -1014418093: /*definition*/ return new Property("definition", "string", "The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.", 0, 1, definition); 1870 case -900931593: /*designation*/ return new Property("designation", "", "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation); 1871 case -993141291: /*property*/ return new Property("property", "", "A property value for this concept.", 0, java.lang.Integer.MAX_VALUE, property); 1872 case 951024232: /*concept*/ return new Property("concept", "@CodeSystem.concept", "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.", 0, java.lang.Integer.MAX_VALUE, concept); 1873 default: return super.getNamedProperty(_hash, _name, _checkValid); 1874 } 1875 1876 } 1877 1878 @Override 1879 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1880 switch (hash) { 1881 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1882 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 1883 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // StringType 1884 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // ConceptDefinitionDesignationComponent 1885 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // ConceptPropertyComponent 1886 case 951024232: /*concept*/ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptDefinitionComponent 1887 default: return super.getProperty(hash, name, checkValid); 1888 } 1889 1890 } 1891 1892 @Override 1893 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1894 switch (hash) { 1895 case 3059181: // code 1896 this.code = castToCode(value); // CodeType 1897 return value; 1898 case 1671764162: // display 1899 this.display = castToString(value); // StringType 1900 return value; 1901 case -1014418093: // definition 1902 this.definition = castToString(value); // StringType 1903 return value; 1904 case -900931593: // designation 1905 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); // ConceptDefinitionDesignationComponent 1906 return value; 1907 case -993141291: // property 1908 this.getProperty().add((ConceptPropertyComponent) value); // ConceptPropertyComponent 1909 return value; 1910 case 951024232: // concept 1911 this.getConcept().add((ConceptDefinitionComponent) value); // ConceptDefinitionComponent 1912 return value; 1913 default: return super.setProperty(hash, name, value); 1914 } 1915 1916 } 1917 1918 @Override 1919 public Base setProperty(String name, Base value) throws FHIRException { 1920 if (name.equals("code")) { 1921 this.code = castToCode(value); // CodeType 1922 } else if (name.equals("display")) { 1923 this.display = castToString(value); // StringType 1924 } else if (name.equals("definition")) { 1925 this.definition = castToString(value); // StringType 1926 } else if (name.equals("designation")) { 1927 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); 1928 } else if (name.equals("property")) { 1929 this.getProperty().add((ConceptPropertyComponent) value); 1930 } else if (name.equals("concept")) { 1931 this.getConcept().add((ConceptDefinitionComponent) value); 1932 } else 1933 return super.setProperty(name, value); 1934 return value; 1935 } 1936 1937 @Override 1938 public Base makeProperty(int hash, String name) throws FHIRException { 1939 switch (hash) { 1940 case 3059181: return getCodeElement(); 1941 case 1671764162: return getDisplayElement(); 1942 case -1014418093: return getDefinitionElement(); 1943 case -900931593: return addDesignation(); 1944 case -993141291: return addProperty(); 1945 case 951024232: return addConcept(); 1946 default: return super.makeProperty(hash, name); 1947 } 1948 1949 } 1950 1951 @Override 1952 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1953 switch (hash) { 1954 case 3059181: /*code*/ return new String[] {"code"}; 1955 case 1671764162: /*display*/ return new String[] {"string"}; 1956 case -1014418093: /*definition*/ return new String[] {"string"}; 1957 case -900931593: /*designation*/ return new String[] {}; 1958 case -993141291: /*property*/ return new String[] {}; 1959 case 951024232: /*concept*/ return new String[] {"@CodeSystem.concept"}; 1960 default: return super.getTypesForProperty(hash, name); 1961 } 1962 1963 } 1964 1965 @Override 1966 public Base addChild(String name) throws FHIRException { 1967 if (name.equals("code")) { 1968 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 1969 } 1970 else if (name.equals("display")) { 1971 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.display"); 1972 } 1973 else if (name.equals("definition")) { 1974 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.definition"); 1975 } 1976 else if (name.equals("designation")) { 1977 return addDesignation(); 1978 } 1979 else if (name.equals("property")) { 1980 return addProperty(); 1981 } 1982 else if (name.equals("concept")) { 1983 return addConcept(); 1984 } 1985 else 1986 return super.addChild(name); 1987 } 1988 1989 public ConceptDefinitionComponent copy() { 1990 ConceptDefinitionComponent dst = new ConceptDefinitionComponent(); 1991 copyValues(dst); 1992 dst.code = code == null ? null : code.copy(); 1993 dst.display = display == null ? null : display.copy(); 1994 dst.definition = definition == null ? null : definition.copy(); 1995 if (designation != null) { 1996 dst.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1997 for (ConceptDefinitionDesignationComponent i : designation) 1998 dst.designation.add(i.copy()); 1999 }; 2000 if (property != null) { 2001 dst.property = new ArrayList<ConceptPropertyComponent>(); 2002 for (ConceptPropertyComponent i : property) 2003 dst.property.add(i.copy()); 2004 }; 2005 if (concept != null) { 2006 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 2007 for (ConceptDefinitionComponent i : concept) 2008 dst.concept.add(i.copy()); 2009 }; 2010 return dst; 2011 } 2012 2013 @Override 2014 public boolean equalsDeep(Base other_) { 2015 if (!super.equalsDeep(other_)) 2016 return false; 2017 if (!(other_ instanceof ConceptDefinitionComponent)) 2018 return false; 2019 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other_; 2020 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(definition, o.definition, true) 2021 && compareDeep(designation, o.designation, true) && compareDeep(property, o.property, true) && compareDeep(concept, o.concept, true) 2022 ; 2023 } 2024 2025 @Override 2026 public boolean equalsShallow(Base other_) { 2027 if (!super.equalsShallow(other_)) 2028 return false; 2029 if (!(other_ instanceof ConceptDefinitionComponent)) 2030 return false; 2031 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other_; 2032 return compareValues(code, o.code, true) && compareValues(display, o.display, true) && compareValues(definition, o.definition, true) 2033 ; 2034 } 2035 2036 public boolean isEmpty() { 2037 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, definition 2038 , designation, property, concept); 2039 } 2040 2041 public String fhirType() { 2042 return "CodeSystem.concept"; 2043 2044 } 2045 2046 } 2047 2048 @Block() 2049 public static class ConceptDefinitionDesignationComponent extends BackboneElement implements IBaseBackboneElement { 2050 /** 2051 * The language this designation is defined for. 2052 */ 2053 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2054 @Description(shortDefinition="Human language of the designation", formalDefinition="The language this designation is defined for." ) 2055 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 2056 protected CodeType language; 2057 2058 /** 2059 * A code that details how this designation would be used. 2060 */ 2061 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=false) 2062 @Description(shortDefinition="Details how this designation would be used", formalDefinition="A code that details how this designation would be used." ) 2063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 2064 protected Coding use; 2065 2066 /** 2067 * The text value for this designation. 2068 */ 2069 @Child(name = "value", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=false) 2070 @Description(shortDefinition="The text value for this designation", formalDefinition="The text value for this designation." ) 2071 protected StringType value; 2072 2073 private static final long serialVersionUID = 1515662414L; 2074 2075 /** 2076 * Constructor 2077 */ 2078 public ConceptDefinitionDesignationComponent() { 2079 super(); 2080 } 2081 2082 /** 2083 * Constructor 2084 */ 2085 public ConceptDefinitionDesignationComponent(StringType value) { 2086 super(); 2087 this.value = value; 2088 } 2089 2090 /** 2091 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 2092 */ 2093 public CodeType getLanguageElement() { 2094 if (this.language == null) 2095 if (Configuration.errorOnAutoCreate()) 2096 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.language"); 2097 else if (Configuration.doAutoCreate()) 2098 this.language = new CodeType(); // bb 2099 return this.language; 2100 } 2101 2102 public boolean hasLanguageElement() { 2103 return this.language != null && !this.language.isEmpty(); 2104 } 2105 2106 public boolean hasLanguage() { 2107 return this.language != null && !this.language.isEmpty(); 2108 } 2109 2110 /** 2111 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 2112 */ 2113 public ConceptDefinitionDesignationComponent setLanguageElement(CodeType value) { 2114 this.language = value; 2115 return this; 2116 } 2117 2118 /** 2119 * @return The language this designation is defined for. 2120 */ 2121 public String getLanguage() { 2122 return this.language == null ? null : this.language.getValue(); 2123 } 2124 2125 /** 2126 * @param value The language this designation is defined for. 2127 */ 2128 public ConceptDefinitionDesignationComponent setLanguage(String value) { 2129 if (Utilities.noString(value)) 2130 this.language = null; 2131 else { 2132 if (this.language == null) 2133 this.language = new CodeType(); 2134 this.language.setValue(value); 2135 } 2136 return this; 2137 } 2138 2139 /** 2140 * @return {@link #use} (A code that details how this designation would be used.) 2141 */ 2142 public Coding getUse() { 2143 if (this.use == null) 2144 if (Configuration.errorOnAutoCreate()) 2145 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.use"); 2146 else if (Configuration.doAutoCreate()) 2147 this.use = new Coding(); // cc 2148 return this.use; 2149 } 2150 2151 public boolean hasUse() { 2152 return this.use != null && !this.use.isEmpty(); 2153 } 2154 2155 /** 2156 * @param value {@link #use} (A code that details how this designation would be used.) 2157 */ 2158 public ConceptDefinitionDesignationComponent setUse(Coding value) { 2159 this.use = value; 2160 return this; 2161 } 2162 2163 /** 2164 * @return {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2165 */ 2166 public StringType getValueElement() { 2167 if (this.value == null) 2168 if (Configuration.errorOnAutoCreate()) 2169 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.value"); 2170 else if (Configuration.doAutoCreate()) 2171 this.value = new StringType(); // bb 2172 return this.value; 2173 } 2174 2175 public boolean hasValueElement() { 2176 return this.value != null && !this.value.isEmpty(); 2177 } 2178 2179 public boolean hasValue() { 2180 return this.value != null && !this.value.isEmpty(); 2181 } 2182 2183 /** 2184 * @param value {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2185 */ 2186 public ConceptDefinitionDesignationComponent setValueElement(StringType value) { 2187 this.value = value; 2188 return this; 2189 } 2190 2191 /** 2192 * @return The text value for this designation. 2193 */ 2194 public String getValue() { 2195 return this.value == null ? null : this.value.getValue(); 2196 } 2197 2198 /** 2199 * @param value The text value for this designation. 2200 */ 2201 public ConceptDefinitionDesignationComponent setValue(String value) { 2202 if (this.value == null) 2203 this.value = new StringType(); 2204 this.value.setValue(value); 2205 return this; 2206 } 2207 2208 protected void listChildren(List<Property> children) { 2209 super.listChildren(children); 2210 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 2211 children.add(new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, use)); 2212 children.add(new Property("value", "string", "The text value for this designation.", 0, 1, value)); 2213 } 2214 2215 @Override 2216 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2217 switch (_hash) { 2218 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 2219 case 116103: /*use*/ return new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, use); 2220 case 111972721: /*value*/ return new Property("value", "string", "The text value for this designation.", 0, 1, value); 2221 default: return super.getNamedProperty(_hash, _name, _checkValid); 2222 } 2223 2224 } 2225 2226 @Override 2227 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2228 switch (hash) { 2229 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 2230 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 2231 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 2232 default: return super.getProperty(hash, name, checkValid); 2233 } 2234 2235 } 2236 2237 @Override 2238 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2239 switch (hash) { 2240 case -1613589672: // language 2241 this.language = castToCode(value); // CodeType 2242 return value; 2243 case 116103: // use 2244 this.use = castToCoding(value); // Coding 2245 return value; 2246 case 111972721: // value 2247 this.value = castToString(value); // StringType 2248 return value; 2249 default: return super.setProperty(hash, name, value); 2250 } 2251 2252 } 2253 2254 @Override 2255 public Base setProperty(String name, Base value) throws FHIRException { 2256 if (name.equals("language")) { 2257 this.language = castToCode(value); // CodeType 2258 } else if (name.equals("use")) { 2259 this.use = castToCoding(value); // Coding 2260 } else if (name.equals("value")) { 2261 this.value = castToString(value); // StringType 2262 } else 2263 return super.setProperty(name, value); 2264 return value; 2265 } 2266 2267 @Override 2268 public Base makeProperty(int hash, String name) throws FHIRException { 2269 switch (hash) { 2270 case -1613589672: return getLanguageElement(); 2271 case 116103: return getUse(); 2272 case 111972721: return getValueElement(); 2273 default: return super.makeProperty(hash, name); 2274 } 2275 2276 } 2277 2278 @Override 2279 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2280 switch (hash) { 2281 case -1613589672: /*language*/ return new String[] {"code"}; 2282 case 116103: /*use*/ return new String[] {"Coding"}; 2283 case 111972721: /*value*/ return new String[] {"string"}; 2284 default: return super.getTypesForProperty(hash, name); 2285 } 2286 2287 } 2288 2289 @Override 2290 public Base addChild(String name) throws FHIRException { 2291 if (name.equals("language")) { 2292 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.language"); 2293 } 2294 else if (name.equals("use")) { 2295 this.use = new Coding(); 2296 return this.use; 2297 } 2298 else if (name.equals("value")) { 2299 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.value"); 2300 } 2301 else 2302 return super.addChild(name); 2303 } 2304 2305 public ConceptDefinitionDesignationComponent copy() { 2306 ConceptDefinitionDesignationComponent dst = new ConceptDefinitionDesignationComponent(); 2307 copyValues(dst); 2308 dst.language = language == null ? null : language.copy(); 2309 dst.use = use == null ? null : use.copy(); 2310 dst.value = value == null ? null : value.copy(); 2311 return dst; 2312 } 2313 2314 @Override 2315 public boolean equalsDeep(Base other_) { 2316 if (!super.equalsDeep(other_)) 2317 return false; 2318 if (!(other_ instanceof ConceptDefinitionDesignationComponent)) 2319 return false; 2320 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other_; 2321 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true) && compareDeep(value, o.value, true) 2322 ; 2323 } 2324 2325 @Override 2326 public boolean equalsShallow(Base other_) { 2327 if (!super.equalsShallow(other_)) 2328 return false; 2329 if (!(other_ instanceof ConceptDefinitionDesignationComponent)) 2330 return false; 2331 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other_; 2332 return compareValues(language, o.language, true) && compareValues(value, o.value, true); 2333 } 2334 2335 public boolean isEmpty() { 2336 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use, value); 2337 } 2338 2339 public String fhirType() { 2340 return "CodeSystem.concept.designation"; 2341 2342 } 2343 2344 } 2345 2346 @Block() 2347 public static class ConceptPropertyComponent extends BackboneElement implements IBaseBackboneElement { 2348 /** 2349 * A code that is a reference to CodeSystem.property.code. 2350 */ 2351 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2352 @Description(shortDefinition="Reference to CodeSystem.property.code", formalDefinition="A code that is a reference to CodeSystem.property.code." ) 2353 protected CodeType code; 2354 2355 /** 2356 * The value of this property. 2357 */ 2358 @Child(name = "value", type = {CodeType.class, Coding.class, StringType.class, IntegerType.class, BooleanType.class, DateTimeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2359 @Description(shortDefinition="Value of the property for this concept", formalDefinition="The value of this property." ) 2360 protected Type value; 2361 2362 private static final long serialVersionUID = 1742812311L; 2363 2364 /** 2365 * Constructor 2366 */ 2367 public ConceptPropertyComponent() { 2368 super(); 2369 } 2370 2371 /** 2372 * Constructor 2373 */ 2374 public ConceptPropertyComponent(CodeType code, Type value) { 2375 super(); 2376 this.code = code; 2377 this.value = value; 2378 } 2379 2380 /** 2381 * @return {@link #code} (A code that is a reference to CodeSystem.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2382 */ 2383 public CodeType getCodeElement() { 2384 if (this.code == null) 2385 if (Configuration.errorOnAutoCreate()) 2386 throw new Error("Attempt to auto-create ConceptPropertyComponent.code"); 2387 else if (Configuration.doAutoCreate()) 2388 this.code = new CodeType(); // bb 2389 return this.code; 2390 } 2391 2392 public boolean hasCodeElement() { 2393 return this.code != null && !this.code.isEmpty(); 2394 } 2395 2396 public boolean hasCode() { 2397 return this.code != null && !this.code.isEmpty(); 2398 } 2399 2400 /** 2401 * @param value {@link #code} (A code that is a reference to CodeSystem.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2402 */ 2403 public ConceptPropertyComponent setCodeElement(CodeType value) { 2404 this.code = value; 2405 return this; 2406 } 2407 2408 /** 2409 * @return A code that is a reference to CodeSystem.property.code. 2410 */ 2411 public String getCode() { 2412 return this.code == null ? null : this.code.getValue(); 2413 } 2414 2415 /** 2416 * @param value A code that is a reference to CodeSystem.property.code. 2417 */ 2418 public ConceptPropertyComponent setCode(String value) { 2419 if (this.code == null) 2420 this.code = new CodeType(); 2421 this.code.setValue(value); 2422 return this; 2423 } 2424 2425 /** 2426 * @return {@link #value} (The value of this property.) 2427 */ 2428 public Type getValue() { 2429 return this.value; 2430 } 2431 2432 /** 2433 * @return {@link #value} (The value of this property.) 2434 */ 2435 public CodeType getValueCodeType() throws FHIRException { 2436 if (this.value == null) 2437 return null; 2438 if (!(this.value instanceof CodeType)) 2439 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2440 return (CodeType) this.value; 2441 } 2442 2443 public boolean hasValueCodeType() { 2444 return this.value instanceof CodeType; 2445 } 2446 2447 /** 2448 * @return {@link #value} (The value of this property.) 2449 */ 2450 public Coding getValueCoding() throws FHIRException { 2451 if (this.value == null) 2452 return null; 2453 if (!(this.value instanceof Coding)) 2454 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 2455 return (Coding) this.value; 2456 } 2457 2458 public boolean hasValueCoding() { 2459 return this.value instanceof Coding; 2460 } 2461 2462 /** 2463 * @return {@link #value} (The value of this property.) 2464 */ 2465 public StringType getValueStringType() throws FHIRException { 2466 if (this.value == null) 2467 return null; 2468 if (!(this.value instanceof StringType)) 2469 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2470 return (StringType) this.value; 2471 } 2472 2473 public boolean hasValueStringType() { 2474 return this.value instanceof StringType; 2475 } 2476 2477 /** 2478 * @return {@link #value} (The value of this property.) 2479 */ 2480 public IntegerType getValueIntegerType() throws FHIRException { 2481 if (this.value == null) 2482 return null; 2483 if (!(this.value instanceof IntegerType)) 2484 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2485 return (IntegerType) this.value; 2486 } 2487 2488 public boolean hasValueIntegerType() { 2489 return this.value instanceof IntegerType; 2490 } 2491 2492 /** 2493 * @return {@link #value} (The value of this property.) 2494 */ 2495 public BooleanType getValueBooleanType() throws FHIRException { 2496 if (this.value == null) 2497 return null; 2498 if (!(this.value instanceof BooleanType)) 2499 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2500 return (BooleanType) this.value; 2501 } 2502 2503 public boolean hasValueBooleanType() { 2504 return this.value instanceof BooleanType; 2505 } 2506 2507 /** 2508 * @return {@link #value} (The value of this property.) 2509 */ 2510 public DateTimeType getValueDateTimeType() throws FHIRException { 2511 if (this.value == null) 2512 return null; 2513 if (!(this.value instanceof DateTimeType)) 2514 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2515 return (DateTimeType) this.value; 2516 } 2517 2518 public boolean hasValueDateTimeType() { 2519 return this.value instanceof DateTimeType; 2520 } 2521 2522 public boolean hasValue() { 2523 return this.value != null && !this.value.isEmpty(); 2524 } 2525 2526 /** 2527 * @param value {@link #value} (The value of this property.) 2528 */ 2529 public ConceptPropertyComponent setValue(Type value) throws FHIRFormatError { 2530 if (value != null && !(value instanceof CodeType || value instanceof Coding || value instanceof StringType || value instanceof IntegerType || value instanceof BooleanType || value instanceof DateTimeType)) 2531 throw new FHIRFormatError("Not the right type for CodeSystem.concept.property.value[x]: "+value.fhirType()); 2532 this.value = value; 2533 return this; 2534 } 2535 2536 protected void listChildren(List<Property> children) { 2537 super.listChildren(children); 2538 children.add(new Property("code", "code", "A code that is a reference to CodeSystem.property.code.", 0, 1, code)); 2539 children.add(new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value)); 2540 } 2541 2542 @Override 2543 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2544 switch (_hash) { 2545 case 3059181: /*code*/ return new Property("code", "code", "A code that is a reference to CodeSystem.property.code.", 0, 1, code); 2546 case -1410166417: /*value[x]*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2547 case 111972721: /*value*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2548 case -766209282: /*valueCode*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2549 case -1887705029: /*valueCoding*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2550 case -1424603934: /*valueString*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2551 case -1668204915: /*valueInteger*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2552 case 733421943: /*valueBoolean*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2553 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime", "The value of this property.", 0, 1, value); 2554 default: return super.getNamedProperty(_hash, _name, _checkValid); 2555 } 2556 2557 } 2558 2559 @Override 2560 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2561 switch (hash) { 2562 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2563 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 2564 default: return super.getProperty(hash, name, checkValid); 2565 } 2566 2567 } 2568 2569 @Override 2570 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2571 switch (hash) { 2572 case 3059181: // code 2573 this.code = castToCode(value); // CodeType 2574 return value; 2575 case 111972721: // value 2576 this.value = castToType(value); // Type 2577 return value; 2578 default: return super.setProperty(hash, name, value); 2579 } 2580 2581 } 2582 2583 @Override 2584 public Base setProperty(String name, Base value) throws FHIRException { 2585 if (name.equals("code")) { 2586 this.code = castToCode(value); // CodeType 2587 } else if (name.equals("value[x]")) { 2588 this.value = castToType(value); // Type 2589 } else 2590 return super.setProperty(name, value); 2591 return value; 2592 } 2593 2594 @Override 2595 public Base makeProperty(int hash, String name) throws FHIRException { 2596 switch (hash) { 2597 case 3059181: return getCodeElement(); 2598 case -1410166417: return getValue(); 2599 case 111972721: return getValue(); 2600 default: return super.makeProperty(hash, name); 2601 } 2602 2603 } 2604 2605 @Override 2606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2607 switch (hash) { 2608 case 3059181: /*code*/ return new String[] {"code"}; 2609 case 111972721: /*value*/ return new String[] {"code", "Coding", "string", "integer", "boolean", "dateTime"}; 2610 default: return super.getTypesForProperty(hash, name); 2611 } 2612 2613 } 2614 2615 @Override 2616 public Base addChild(String name) throws FHIRException { 2617 if (name.equals("code")) { 2618 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 2619 } 2620 else if (name.equals("valueCode")) { 2621 this.value = new CodeType(); 2622 return this.value; 2623 } 2624 else if (name.equals("valueCoding")) { 2625 this.value = new Coding(); 2626 return this.value; 2627 } 2628 else if (name.equals("valueString")) { 2629 this.value = new StringType(); 2630 return this.value; 2631 } 2632 else if (name.equals("valueInteger")) { 2633 this.value = new IntegerType(); 2634 return this.value; 2635 } 2636 else if (name.equals("valueBoolean")) { 2637 this.value = new BooleanType(); 2638 return this.value; 2639 } 2640 else if (name.equals("valueDateTime")) { 2641 this.value = new DateTimeType(); 2642 return this.value; 2643 } 2644 else 2645 return super.addChild(name); 2646 } 2647 2648 public ConceptPropertyComponent copy() { 2649 ConceptPropertyComponent dst = new ConceptPropertyComponent(); 2650 copyValues(dst); 2651 dst.code = code == null ? null : code.copy(); 2652 dst.value = value == null ? null : value.copy(); 2653 return dst; 2654 } 2655 2656 @Override 2657 public boolean equalsDeep(Base other_) { 2658 if (!super.equalsDeep(other_)) 2659 return false; 2660 if (!(other_ instanceof ConceptPropertyComponent)) 2661 return false; 2662 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 2663 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 2664 } 2665 2666 @Override 2667 public boolean equalsShallow(Base other_) { 2668 if (!super.equalsShallow(other_)) 2669 return false; 2670 if (!(other_ instanceof ConceptPropertyComponent)) 2671 return false; 2672 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 2673 return compareValues(code, o.code, true); 2674 } 2675 2676 public boolean isEmpty() { 2677 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 2678 } 2679 2680 public String fhirType() { 2681 return "CodeSystem.concept.property"; 2682 2683 } 2684 2685 } 2686 2687 /** 2688 * A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance. 2689 */ 2690 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2691 @Description(shortDefinition="Additional identifier for the code system", formalDefinition="A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2692 protected Identifier identifier; 2693 2694 /** 2695 * Explaination of why this code system is needed and why it has been designed as it has. 2696 */ 2697 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2698 @Description(shortDefinition="Why this code system is defined", formalDefinition="Explaination of why this code system is needed and why it has been designed as it has." ) 2699 protected MarkdownType purpose; 2700 2701 /** 2702 * A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system. 2703 */ 2704 @Child(name = "copyright", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2705 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system." ) 2706 protected MarkdownType copyright; 2707 2708 /** 2709 * If code comparison is case sensitive when codes within this system are compared to each other. 2710 */ 2711 @Child(name = "caseSensitive", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2712 @Description(shortDefinition="If code comparison is case sensitive", formalDefinition="If code comparison is case sensitive when codes within this system are compared to each other." ) 2713 protected BooleanType caseSensitive; 2714 2715 /** 2716 * Canonical URL of value set that contains the entire code system. 2717 */ 2718 @Child(name = "valueSet", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2719 @Description(shortDefinition="Canonical URL for value set with entire code system", formalDefinition="Canonical URL of value set that contains the entire code system." ) 2720 protected UriType valueSet; 2721 2722 /** 2723 * The meaning of the hierarchy of concepts. 2724 */ 2725 @Child(name = "hierarchyMeaning", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2726 @Description(shortDefinition="grouped-by | is-a | part-of | classified-with", formalDefinition="The meaning of the hierarchy of concepts." ) 2727 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/codesystem-hierarchy-meaning") 2728 protected Enumeration<CodeSystemHierarchyMeaning> hierarchyMeaning; 2729 2730 /** 2731 * True If code system defines a post-composition grammar. 2732 */ 2733 @Child(name = "compositional", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2734 @Description(shortDefinition="If code system defines a post-composition grammar", formalDefinition="True If code system defines a post-composition grammar." ) 2735 protected BooleanType compositional; 2736 2737 /** 2738 * This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system. 2739 */ 2740 @Child(name = "versionNeeded", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 2741 @Description(shortDefinition="If definitions are not stable", formalDefinition="This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system." ) 2742 protected BooleanType versionNeeded; 2743 2744 /** 2745 * How much of the content of the code system - the concepts and codes it defines - are represented in this resource. 2746 */ 2747 @Child(name = "content", type = {CodeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 2748 @Description(shortDefinition="not-present | example | fragment | complete", formalDefinition="How much of the content of the code system - the concepts and codes it defines - are represented in this resource." ) 2749 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/codesystem-content-mode") 2750 protected Enumeration<CodeSystemContentMode> content; 2751 2752 /** 2753 * The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts. 2754 */ 2755 @Child(name = "count", type = {UnsignedIntType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2756 @Description(shortDefinition="Total concepts in the code system", formalDefinition="The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts." ) 2757 protected UnsignedIntType count; 2758 2759 /** 2760 * A filter that can be used in a value set compose statement when selecting concepts using a filter. 2761 */ 2762 @Child(name = "filter", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2763 @Description(shortDefinition="Filter that can be used in a value set", formalDefinition="A filter that can be used in a value set compose statement when selecting concepts using a filter." ) 2764 protected List<CodeSystemFilterComponent> filter; 2765 2766 /** 2767 * A property defines an additional slot through which additional information can be provided about a concept. 2768 */ 2769 @Child(name = "property", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2770 @Description(shortDefinition="Additional information supplied about each concept", formalDefinition="A property defines an additional slot through which additional information can be provided about a concept." ) 2771 protected List<PropertyComponent> property; 2772 2773 /** 2774 * Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meaning of the hierarchical relationships are. 2775 */ 2776 @Child(name = "concept", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2777 @Description(shortDefinition="Concepts in the code system", formalDefinition="Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meaning of the hierarchical relationships are." ) 2778 protected List<ConceptDefinitionComponent> concept; 2779 2780 private static final long serialVersionUID = -1344546572L; 2781 2782 /** 2783 * Constructor 2784 */ 2785 public CodeSystem() { 2786 super(); 2787 } 2788 2789 /** 2790 * Constructor 2791 */ 2792 public CodeSystem(Enumeration<PublicationStatus> status, Enumeration<CodeSystemContentMode> content) { 2793 super(); 2794 this.status = status; 2795 this.content = content; 2796 } 2797 2798 /** 2799 * @return {@link #url} (An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this code system is (or will be) published. The URL SHOULD include the major version of the code system. For more information see [Technical and Business Versions](resource.html#versions). This is used in [Coding]{datatypes.html#Coding}.system.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2800 */ 2801 public UriType getUrlElement() { 2802 if (this.url == null) 2803 if (Configuration.errorOnAutoCreate()) 2804 throw new Error("Attempt to auto-create CodeSystem.url"); 2805 else if (Configuration.doAutoCreate()) 2806 this.url = new UriType(); // bb 2807 return this.url; 2808 } 2809 2810 public boolean hasUrlElement() { 2811 return this.url != null && !this.url.isEmpty(); 2812 } 2813 2814 public boolean hasUrl() { 2815 return this.url != null && !this.url.isEmpty(); 2816 } 2817 2818 /** 2819 * @param value {@link #url} (An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this code system is (or will be) published. The URL SHOULD include the major version of the code system. For more information see [Technical and Business Versions](resource.html#versions). This is used in [Coding]{datatypes.html#Coding}.system.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2820 */ 2821 public CodeSystem setUrlElement(UriType value) { 2822 this.url = value; 2823 return this; 2824 } 2825 2826 /** 2827 * @return An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this code system is (or will be) published. The URL SHOULD include the major version of the code system. For more information see [Technical and Business Versions](resource.html#versions). This is used in [Coding]{datatypes.html#Coding}.system. 2828 */ 2829 public String getUrl() { 2830 return this.url == null ? null : this.url.getValue(); 2831 } 2832 2833 /** 2834 * @param value An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this code system is (or will be) published. The URL SHOULD include the major version of the code system. For more information see [Technical and Business Versions](resource.html#versions). This is used in [Coding]{datatypes.html#Coding}.system. 2835 */ 2836 public CodeSystem setUrl(String value) { 2837 if (Utilities.noString(value)) 2838 this.url = null; 2839 else { 2840 if (this.url == null) 2841 this.url = new UriType(); 2842 this.url.setValue(value); 2843 } 2844 return this; 2845 } 2846 2847 /** 2848 * @return {@link #identifier} (A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2849 */ 2850 public Identifier getIdentifier() { 2851 if (this.identifier == null) 2852 if (Configuration.errorOnAutoCreate()) 2853 throw new Error("Attempt to auto-create CodeSystem.identifier"); 2854 else if (Configuration.doAutoCreate()) 2855 this.identifier = new Identifier(); // cc 2856 return this.identifier; 2857 } 2858 2859 public boolean hasIdentifier() { 2860 return this.identifier != null && !this.identifier.isEmpty(); 2861 } 2862 2863 /** 2864 * @param value {@link #identifier} (A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2865 */ 2866 public CodeSystem setIdentifier(Identifier value) { 2867 this.identifier = value; 2868 return this; 2869 } 2870 2871 /** 2872 * @return {@link #version} (The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding]{datatypes.html#Coding}.version.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2873 */ 2874 public StringType getVersionElement() { 2875 if (this.version == null) 2876 if (Configuration.errorOnAutoCreate()) 2877 throw new Error("Attempt to auto-create CodeSystem.version"); 2878 else if (Configuration.doAutoCreate()) 2879 this.version = new StringType(); // bb 2880 return this.version; 2881 } 2882 2883 public boolean hasVersionElement() { 2884 return this.version != null && !this.version.isEmpty(); 2885 } 2886 2887 public boolean hasVersion() { 2888 return this.version != null && !this.version.isEmpty(); 2889 } 2890 2891 /** 2892 * @param value {@link #version} (The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding]{datatypes.html#Coding}.version.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2893 */ 2894 public CodeSystem setVersionElement(StringType value) { 2895 this.version = value; 2896 return this; 2897 } 2898 2899 /** 2900 * @return The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding]{datatypes.html#Coding}.version. 2901 */ 2902 public String getVersion() { 2903 return this.version == null ? null : this.version.getValue(); 2904 } 2905 2906 /** 2907 * @param value The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding]{datatypes.html#Coding}.version. 2908 */ 2909 public CodeSystem setVersion(String value) { 2910 if (Utilities.noString(value)) 2911 this.version = null; 2912 else { 2913 if (this.version == null) 2914 this.version = new StringType(); 2915 this.version.setValue(value); 2916 } 2917 return this; 2918 } 2919 2920 /** 2921 * @return {@link #name} (A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2922 */ 2923 public StringType getNameElement() { 2924 if (this.name == null) 2925 if (Configuration.errorOnAutoCreate()) 2926 throw new Error("Attempt to auto-create CodeSystem.name"); 2927 else if (Configuration.doAutoCreate()) 2928 this.name = new StringType(); // bb 2929 return this.name; 2930 } 2931 2932 public boolean hasNameElement() { 2933 return this.name != null && !this.name.isEmpty(); 2934 } 2935 2936 public boolean hasName() { 2937 return this.name != null && !this.name.isEmpty(); 2938 } 2939 2940 /** 2941 * @param value {@link #name} (A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2942 */ 2943 public CodeSystem setNameElement(StringType value) { 2944 this.name = value; 2945 return this; 2946 } 2947 2948 /** 2949 * @return A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2950 */ 2951 public String getName() { 2952 return this.name == null ? null : this.name.getValue(); 2953 } 2954 2955 /** 2956 * @param value A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2957 */ 2958 public CodeSystem setName(String value) { 2959 if (Utilities.noString(value)) 2960 this.name = null; 2961 else { 2962 if (this.name == null) 2963 this.name = new StringType(); 2964 this.name.setValue(value); 2965 } 2966 return this; 2967 } 2968 2969 /** 2970 * @return {@link #title} (A short, descriptive, user-friendly title for the code system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2971 */ 2972 public StringType getTitleElement() { 2973 if (this.title == null) 2974 if (Configuration.errorOnAutoCreate()) 2975 throw new Error("Attempt to auto-create CodeSystem.title"); 2976 else if (Configuration.doAutoCreate()) 2977 this.title = new StringType(); // bb 2978 return this.title; 2979 } 2980 2981 public boolean hasTitleElement() { 2982 return this.title != null && !this.title.isEmpty(); 2983 } 2984 2985 public boolean hasTitle() { 2986 return this.title != null && !this.title.isEmpty(); 2987 } 2988 2989 /** 2990 * @param value {@link #title} (A short, descriptive, user-friendly title for the code system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2991 */ 2992 public CodeSystem setTitleElement(StringType value) { 2993 this.title = value; 2994 return this; 2995 } 2996 2997 /** 2998 * @return A short, descriptive, user-friendly title for the code system. 2999 */ 3000 public String getTitle() { 3001 return this.title == null ? null : this.title.getValue(); 3002 } 3003 3004 /** 3005 * @param value A short, descriptive, user-friendly title for the code system. 3006 */ 3007 public CodeSystem setTitle(String value) { 3008 if (Utilities.noString(value)) 3009 this.title = null; 3010 else { 3011 if (this.title == null) 3012 this.title = new StringType(); 3013 this.title.setValue(value); 3014 } 3015 return this; 3016 } 3017 3018 /** 3019 * @return {@link #status} (The status of this code system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3020 */ 3021 public Enumeration<PublicationStatus> getStatusElement() { 3022 if (this.status == null) 3023 if (Configuration.errorOnAutoCreate()) 3024 throw new Error("Attempt to auto-create CodeSystem.status"); 3025 else if (Configuration.doAutoCreate()) 3026 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3027 return this.status; 3028 } 3029 3030 public boolean hasStatusElement() { 3031 return this.status != null && !this.status.isEmpty(); 3032 } 3033 3034 public boolean hasStatus() { 3035 return this.status != null && !this.status.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #status} (The status of this code system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3040 */ 3041 public CodeSystem setStatusElement(Enumeration<PublicationStatus> value) { 3042 this.status = value; 3043 return this; 3044 } 3045 3046 /** 3047 * @return The status of this code system. Enables tracking the life-cycle of the content. 3048 */ 3049 public PublicationStatus getStatus() { 3050 return this.status == null ? null : this.status.getValue(); 3051 } 3052 3053 /** 3054 * @param value The status of this code system. Enables tracking the life-cycle of the content. 3055 */ 3056 public CodeSystem setStatus(PublicationStatus value) { 3057 if (this.status == null) 3058 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3059 this.status.setValue(value); 3060 return this; 3061 } 3062 3063 /** 3064 * @return {@link #experimental} (A boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3065 */ 3066 public BooleanType getExperimentalElement() { 3067 if (this.experimental == null) 3068 if (Configuration.errorOnAutoCreate()) 3069 throw new Error("Attempt to auto-create CodeSystem.experimental"); 3070 else if (Configuration.doAutoCreate()) 3071 this.experimental = new BooleanType(); // bb 3072 return this.experimental; 3073 } 3074 3075 public boolean hasExperimentalElement() { 3076 return this.experimental != null && !this.experimental.isEmpty(); 3077 } 3078 3079 public boolean hasExperimental() { 3080 return this.experimental != null && !this.experimental.isEmpty(); 3081 } 3082 3083 /** 3084 * @param value {@link #experimental} (A boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3085 */ 3086 public CodeSystem setExperimentalElement(BooleanType value) { 3087 this.experimental = value; 3088 return this; 3089 } 3090 3091 /** 3092 * @return A boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3093 */ 3094 public boolean getExperimental() { 3095 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3096 } 3097 3098 /** 3099 * @param value A boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3100 */ 3101 public CodeSystem setExperimental(boolean value) { 3102 if (this.experimental == null) 3103 this.experimental = new BooleanType(); 3104 this.experimental.setValue(value); 3105 return this; 3106 } 3107 3108 /** 3109 * @return {@link #date} (The date (and optionally time) when the code system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3110 */ 3111 public DateTimeType getDateElement() { 3112 if (this.date == null) 3113 if (Configuration.errorOnAutoCreate()) 3114 throw new Error("Attempt to auto-create CodeSystem.date"); 3115 else if (Configuration.doAutoCreate()) 3116 this.date = new DateTimeType(); // bb 3117 return this.date; 3118 } 3119 3120 public boolean hasDateElement() { 3121 return this.date != null && !this.date.isEmpty(); 3122 } 3123 3124 public boolean hasDate() { 3125 return this.date != null && !this.date.isEmpty(); 3126 } 3127 3128 /** 3129 * @param value {@link #date} (The date (and optionally time) when the code system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3130 */ 3131 public CodeSystem setDateElement(DateTimeType value) { 3132 this.date = value; 3133 return this; 3134 } 3135 3136 /** 3137 * @return The date (and optionally time) when the code system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes. 3138 */ 3139 public Date getDate() { 3140 return this.date == null ? null : this.date.getValue(); 3141 } 3142 3143 /** 3144 * @param value The date (and optionally time) when the code system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes. 3145 */ 3146 public CodeSystem setDate(Date value) { 3147 if (value == null) 3148 this.date = null; 3149 else { 3150 if (this.date == null) 3151 this.date = new DateTimeType(); 3152 this.date.setValue(value); 3153 } 3154 return this; 3155 } 3156 3157 /** 3158 * @return {@link #publisher} (The name of the individual or organization that published the code system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3159 */ 3160 public StringType getPublisherElement() { 3161 if (this.publisher == null) 3162 if (Configuration.errorOnAutoCreate()) 3163 throw new Error("Attempt to auto-create CodeSystem.publisher"); 3164 else if (Configuration.doAutoCreate()) 3165 this.publisher = new StringType(); // bb 3166 return this.publisher; 3167 } 3168 3169 public boolean hasPublisherElement() { 3170 return this.publisher != null && !this.publisher.isEmpty(); 3171 } 3172 3173 public boolean hasPublisher() { 3174 return this.publisher != null && !this.publisher.isEmpty(); 3175 } 3176 3177 /** 3178 * @param value {@link #publisher} (The name of the individual or organization that published the code system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3179 */ 3180 public CodeSystem setPublisherElement(StringType value) { 3181 this.publisher = value; 3182 return this; 3183 } 3184 3185 /** 3186 * @return The name of the individual or organization that published the code system. 3187 */ 3188 public String getPublisher() { 3189 return this.publisher == null ? null : this.publisher.getValue(); 3190 } 3191 3192 /** 3193 * @param value The name of the individual or organization that published the code system. 3194 */ 3195 public CodeSystem setPublisher(String value) { 3196 if (Utilities.noString(value)) 3197 this.publisher = null; 3198 else { 3199 if (this.publisher == null) 3200 this.publisher = new StringType(); 3201 this.publisher.setValue(value); 3202 } 3203 return this; 3204 } 3205 3206 /** 3207 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3208 */ 3209 public List<ContactDetail> getContact() { 3210 if (this.contact == null) 3211 this.contact = new ArrayList<ContactDetail>(); 3212 return this.contact; 3213 } 3214 3215 /** 3216 * @return Returns a reference to <code>this</code> for easy method chaining 3217 */ 3218 public CodeSystem setContact(List<ContactDetail> theContact) { 3219 this.contact = theContact; 3220 return this; 3221 } 3222 3223 public boolean hasContact() { 3224 if (this.contact == null) 3225 return false; 3226 for (ContactDetail item : this.contact) 3227 if (!item.isEmpty()) 3228 return true; 3229 return false; 3230 } 3231 3232 public ContactDetail addContact() { //3 3233 ContactDetail t = new ContactDetail(); 3234 if (this.contact == null) 3235 this.contact = new ArrayList<ContactDetail>(); 3236 this.contact.add(t); 3237 return t; 3238 } 3239 3240 public CodeSystem addContact(ContactDetail t) { //3 3241 if (t == null) 3242 return this; 3243 if (this.contact == null) 3244 this.contact = new ArrayList<ContactDetail>(); 3245 this.contact.add(t); 3246 return this; 3247 } 3248 3249 /** 3250 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 3251 */ 3252 public ContactDetail getContactFirstRep() { 3253 if (getContact().isEmpty()) { 3254 addContact(); 3255 } 3256 return getContact().get(0); 3257 } 3258 3259 /** 3260 * @return {@link #description} (A free text natural language description of the code system from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3261 */ 3262 public MarkdownType getDescriptionElement() { 3263 if (this.description == null) 3264 if (Configuration.errorOnAutoCreate()) 3265 throw new Error("Attempt to auto-create CodeSystem.description"); 3266 else if (Configuration.doAutoCreate()) 3267 this.description = new MarkdownType(); // bb 3268 return this.description; 3269 } 3270 3271 public boolean hasDescriptionElement() { 3272 return this.description != null && !this.description.isEmpty(); 3273 } 3274 3275 public boolean hasDescription() { 3276 return this.description != null && !this.description.isEmpty(); 3277 } 3278 3279 /** 3280 * @param value {@link #description} (A free text natural language description of the code system from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3281 */ 3282 public CodeSystem setDescriptionElement(MarkdownType value) { 3283 this.description = value; 3284 return this; 3285 } 3286 3287 /** 3288 * @return A free text natural language description of the code system from a consumer's perspective. 3289 */ 3290 public String getDescription() { 3291 return this.description == null ? null : this.description.getValue(); 3292 } 3293 3294 /** 3295 * @param value A free text natural language description of the code system from a consumer's perspective. 3296 */ 3297 public CodeSystem setDescription(String value) { 3298 if (value == null) 3299 this.description = null; 3300 else { 3301 if (this.description == null) 3302 this.description = new MarkdownType(); 3303 this.description.setValue(value); 3304 } 3305 return this; 3306 } 3307 3308 /** 3309 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate code system instances.) 3310 */ 3311 public List<UsageContext> getUseContext() { 3312 if (this.useContext == null) 3313 this.useContext = new ArrayList<UsageContext>(); 3314 return this.useContext; 3315 } 3316 3317 /** 3318 * @return Returns a reference to <code>this</code> for easy method chaining 3319 */ 3320 public CodeSystem setUseContext(List<UsageContext> theUseContext) { 3321 this.useContext = theUseContext; 3322 return this; 3323 } 3324 3325 public boolean hasUseContext() { 3326 if (this.useContext == null) 3327 return false; 3328 for (UsageContext item : this.useContext) 3329 if (!item.isEmpty()) 3330 return true; 3331 return false; 3332 } 3333 3334 public UsageContext addUseContext() { //3 3335 UsageContext t = new UsageContext(); 3336 if (this.useContext == null) 3337 this.useContext = new ArrayList<UsageContext>(); 3338 this.useContext.add(t); 3339 return t; 3340 } 3341 3342 public CodeSystem addUseContext(UsageContext t) { //3 3343 if (t == null) 3344 return this; 3345 if (this.useContext == null) 3346 this.useContext = new ArrayList<UsageContext>(); 3347 this.useContext.add(t); 3348 return this; 3349 } 3350 3351 /** 3352 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 3353 */ 3354 public UsageContext getUseContextFirstRep() { 3355 if (getUseContext().isEmpty()) { 3356 addUseContext(); 3357 } 3358 return getUseContext().get(0); 3359 } 3360 3361 /** 3362 * @return {@link #jurisdiction} (A legal or geographic region in which the code system is intended to be used.) 3363 */ 3364 public List<CodeableConcept> getJurisdiction() { 3365 if (this.jurisdiction == null) 3366 this.jurisdiction = new ArrayList<CodeableConcept>(); 3367 return this.jurisdiction; 3368 } 3369 3370 /** 3371 * @return Returns a reference to <code>this</code> for easy method chaining 3372 */ 3373 public CodeSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 3374 this.jurisdiction = theJurisdiction; 3375 return this; 3376 } 3377 3378 public boolean hasJurisdiction() { 3379 if (this.jurisdiction == null) 3380 return false; 3381 for (CodeableConcept item : this.jurisdiction) 3382 if (!item.isEmpty()) 3383 return true; 3384 return false; 3385 } 3386 3387 public CodeableConcept addJurisdiction() { //3 3388 CodeableConcept t = new CodeableConcept(); 3389 if (this.jurisdiction == null) 3390 this.jurisdiction = new ArrayList<CodeableConcept>(); 3391 this.jurisdiction.add(t); 3392 return t; 3393 } 3394 3395 public CodeSystem addJurisdiction(CodeableConcept t) { //3 3396 if (t == null) 3397 return this; 3398 if (this.jurisdiction == null) 3399 this.jurisdiction = new ArrayList<CodeableConcept>(); 3400 this.jurisdiction.add(t); 3401 return this; 3402 } 3403 3404 /** 3405 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 3406 */ 3407 public CodeableConcept getJurisdictionFirstRep() { 3408 if (getJurisdiction().isEmpty()) { 3409 addJurisdiction(); 3410 } 3411 return getJurisdiction().get(0); 3412 } 3413 3414 /** 3415 * @return {@link #purpose} (Explaination of why this code system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3416 */ 3417 public MarkdownType getPurposeElement() { 3418 if (this.purpose == null) 3419 if (Configuration.errorOnAutoCreate()) 3420 throw new Error("Attempt to auto-create CodeSystem.purpose"); 3421 else if (Configuration.doAutoCreate()) 3422 this.purpose = new MarkdownType(); // bb 3423 return this.purpose; 3424 } 3425 3426 public boolean hasPurposeElement() { 3427 return this.purpose != null && !this.purpose.isEmpty(); 3428 } 3429 3430 public boolean hasPurpose() { 3431 return this.purpose != null && !this.purpose.isEmpty(); 3432 } 3433 3434 /** 3435 * @param value {@link #purpose} (Explaination of why this code system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3436 */ 3437 public CodeSystem setPurposeElement(MarkdownType value) { 3438 this.purpose = value; 3439 return this; 3440 } 3441 3442 /** 3443 * @return Explaination of why this code system is needed and why it has been designed as it has. 3444 */ 3445 public String getPurpose() { 3446 return this.purpose == null ? null : this.purpose.getValue(); 3447 } 3448 3449 /** 3450 * @param value Explaination of why this code system is needed and why it has been designed as it has. 3451 */ 3452 public CodeSystem setPurpose(String value) { 3453 if (value == null) 3454 this.purpose = null; 3455 else { 3456 if (this.purpose == null) 3457 this.purpose = new MarkdownType(); 3458 this.purpose.setValue(value); 3459 } 3460 return this; 3461 } 3462 3463 /** 3464 * @return {@link #copyright} (A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3465 */ 3466 public MarkdownType getCopyrightElement() { 3467 if (this.copyright == null) 3468 if (Configuration.errorOnAutoCreate()) 3469 throw new Error("Attempt to auto-create CodeSystem.copyright"); 3470 else if (Configuration.doAutoCreate()) 3471 this.copyright = new MarkdownType(); // bb 3472 return this.copyright; 3473 } 3474 3475 public boolean hasCopyrightElement() { 3476 return this.copyright != null && !this.copyright.isEmpty(); 3477 } 3478 3479 public boolean hasCopyright() { 3480 return this.copyright != null && !this.copyright.isEmpty(); 3481 } 3482 3483 /** 3484 * @param value {@link #copyright} (A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3485 */ 3486 public CodeSystem setCopyrightElement(MarkdownType value) { 3487 this.copyright = value; 3488 return this; 3489 } 3490 3491 /** 3492 * @return A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system. 3493 */ 3494 public String getCopyright() { 3495 return this.copyright == null ? null : this.copyright.getValue(); 3496 } 3497 3498 /** 3499 * @param value A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system. 3500 */ 3501 public CodeSystem setCopyright(String value) { 3502 if (value == null) 3503 this.copyright = null; 3504 else { 3505 if (this.copyright == null) 3506 this.copyright = new MarkdownType(); 3507 this.copyright.setValue(value); 3508 } 3509 return this; 3510 } 3511 3512 /** 3513 * @return {@link #caseSensitive} (If code comparison is case sensitive when codes within this system are compared to each other.). This is the underlying object with id, value and extensions. The accessor "getCaseSensitive" gives direct access to the value 3514 */ 3515 public BooleanType getCaseSensitiveElement() { 3516 if (this.caseSensitive == null) 3517 if (Configuration.errorOnAutoCreate()) 3518 throw new Error("Attempt to auto-create CodeSystem.caseSensitive"); 3519 else if (Configuration.doAutoCreate()) 3520 this.caseSensitive = new BooleanType(); // bb 3521 return this.caseSensitive; 3522 } 3523 3524 public boolean hasCaseSensitiveElement() { 3525 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 3526 } 3527 3528 public boolean hasCaseSensitive() { 3529 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 3530 } 3531 3532 /** 3533 * @param value {@link #caseSensitive} (If code comparison is case sensitive when codes within this system are compared to each other.). This is the underlying object with id, value and extensions. The accessor "getCaseSensitive" gives direct access to the value 3534 */ 3535 public CodeSystem setCaseSensitiveElement(BooleanType value) { 3536 this.caseSensitive = value; 3537 return this; 3538 } 3539 3540 /** 3541 * @return If code comparison is case sensitive when codes within this system are compared to each other. 3542 */ 3543 public boolean getCaseSensitive() { 3544 return this.caseSensitive == null || this.caseSensitive.isEmpty() ? false : this.caseSensitive.getValue(); 3545 } 3546 3547 /** 3548 * @param value If code comparison is case sensitive when codes within this system are compared to each other. 3549 */ 3550 public CodeSystem setCaseSensitive(boolean value) { 3551 if (this.caseSensitive == null) 3552 this.caseSensitive = new BooleanType(); 3553 this.caseSensitive.setValue(value); 3554 return this; 3555 } 3556 3557 /** 3558 * @return {@link #valueSet} (Canonical URL of value set that contains the entire code system.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 3559 */ 3560 public UriType getValueSetElement() { 3561 if (this.valueSet == null) 3562 if (Configuration.errorOnAutoCreate()) 3563 throw new Error("Attempt to auto-create CodeSystem.valueSet"); 3564 else if (Configuration.doAutoCreate()) 3565 this.valueSet = new UriType(); // bb 3566 return this.valueSet; 3567 } 3568 3569 public boolean hasValueSetElement() { 3570 return this.valueSet != null && !this.valueSet.isEmpty(); 3571 } 3572 3573 public boolean hasValueSet() { 3574 return this.valueSet != null && !this.valueSet.isEmpty(); 3575 } 3576 3577 /** 3578 * @param value {@link #valueSet} (Canonical URL of value set that contains the entire code system.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 3579 */ 3580 public CodeSystem setValueSetElement(UriType value) { 3581 this.valueSet = value; 3582 return this; 3583 } 3584 3585 /** 3586 * @return Canonical URL of value set that contains the entire code system. 3587 */ 3588 public String getValueSet() { 3589 return this.valueSet == null ? null : this.valueSet.getValue(); 3590 } 3591 3592 /** 3593 * @param value Canonical URL of value set that contains the entire code system. 3594 */ 3595 public CodeSystem setValueSet(String value) { 3596 if (Utilities.noString(value)) 3597 this.valueSet = null; 3598 else { 3599 if (this.valueSet == null) 3600 this.valueSet = new UriType(); 3601 this.valueSet.setValue(value); 3602 } 3603 return this; 3604 } 3605 3606 /** 3607 * @return {@link #hierarchyMeaning} (The meaning of the hierarchy of concepts.). This is the underlying object with id, value and extensions. The accessor "getHierarchyMeaning" gives direct access to the value 3608 */ 3609 public Enumeration<CodeSystemHierarchyMeaning> getHierarchyMeaningElement() { 3610 if (this.hierarchyMeaning == null) 3611 if (Configuration.errorOnAutoCreate()) 3612 throw new Error("Attempt to auto-create CodeSystem.hierarchyMeaning"); 3613 else if (Configuration.doAutoCreate()) 3614 this.hierarchyMeaning = new Enumeration<CodeSystemHierarchyMeaning>(new CodeSystemHierarchyMeaningEnumFactory()); // bb 3615 return this.hierarchyMeaning; 3616 } 3617 3618 public boolean hasHierarchyMeaningElement() { 3619 return this.hierarchyMeaning != null && !this.hierarchyMeaning.isEmpty(); 3620 } 3621 3622 public boolean hasHierarchyMeaning() { 3623 return this.hierarchyMeaning != null && !this.hierarchyMeaning.isEmpty(); 3624 } 3625 3626 /** 3627 * @param value {@link #hierarchyMeaning} (The meaning of the hierarchy of concepts.). This is the underlying object with id, value and extensions. The accessor "getHierarchyMeaning" gives direct access to the value 3628 */ 3629 public CodeSystem setHierarchyMeaningElement(Enumeration<CodeSystemHierarchyMeaning> value) { 3630 this.hierarchyMeaning = value; 3631 return this; 3632 } 3633 3634 /** 3635 * @return The meaning of the hierarchy of concepts. 3636 */ 3637 public CodeSystemHierarchyMeaning getHierarchyMeaning() { 3638 return this.hierarchyMeaning == null ? null : this.hierarchyMeaning.getValue(); 3639 } 3640 3641 /** 3642 * @param value The meaning of the hierarchy of concepts. 3643 */ 3644 public CodeSystem setHierarchyMeaning(CodeSystemHierarchyMeaning value) { 3645 if (value == null) 3646 this.hierarchyMeaning = null; 3647 else { 3648 if (this.hierarchyMeaning == null) 3649 this.hierarchyMeaning = new Enumeration<CodeSystemHierarchyMeaning>(new CodeSystemHierarchyMeaningEnumFactory()); 3650 this.hierarchyMeaning.setValue(value); 3651 } 3652 return this; 3653 } 3654 3655 /** 3656 * @return {@link #compositional} (True If code system defines a post-composition grammar.). This is the underlying object with id, value and extensions. The accessor "getCompositional" gives direct access to the value 3657 */ 3658 public BooleanType getCompositionalElement() { 3659 if (this.compositional == null) 3660 if (Configuration.errorOnAutoCreate()) 3661 throw new Error("Attempt to auto-create CodeSystem.compositional"); 3662 else if (Configuration.doAutoCreate()) 3663 this.compositional = new BooleanType(); // bb 3664 return this.compositional; 3665 } 3666 3667 public boolean hasCompositionalElement() { 3668 return this.compositional != null && !this.compositional.isEmpty(); 3669 } 3670 3671 public boolean hasCompositional() { 3672 return this.compositional != null && !this.compositional.isEmpty(); 3673 } 3674 3675 /** 3676 * @param value {@link #compositional} (True If code system defines a post-composition grammar.). This is the underlying object with id, value and extensions. The accessor "getCompositional" gives direct access to the value 3677 */ 3678 public CodeSystem setCompositionalElement(BooleanType value) { 3679 this.compositional = value; 3680 return this; 3681 } 3682 3683 /** 3684 * @return True If code system defines a post-composition grammar. 3685 */ 3686 public boolean getCompositional() { 3687 return this.compositional == null || this.compositional.isEmpty() ? false : this.compositional.getValue(); 3688 } 3689 3690 /** 3691 * @param value True If code system defines a post-composition grammar. 3692 */ 3693 public CodeSystem setCompositional(boolean value) { 3694 if (this.compositional == null) 3695 this.compositional = new BooleanType(); 3696 this.compositional.setValue(value); 3697 return this; 3698 } 3699 3700 /** 3701 * @return {@link #versionNeeded} (This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system.). This is the underlying object with id, value and extensions. The accessor "getVersionNeeded" gives direct access to the value 3702 */ 3703 public BooleanType getVersionNeededElement() { 3704 if (this.versionNeeded == null) 3705 if (Configuration.errorOnAutoCreate()) 3706 throw new Error("Attempt to auto-create CodeSystem.versionNeeded"); 3707 else if (Configuration.doAutoCreate()) 3708 this.versionNeeded = new BooleanType(); // bb 3709 return this.versionNeeded; 3710 } 3711 3712 public boolean hasVersionNeededElement() { 3713 return this.versionNeeded != null && !this.versionNeeded.isEmpty(); 3714 } 3715 3716 public boolean hasVersionNeeded() { 3717 return this.versionNeeded != null && !this.versionNeeded.isEmpty(); 3718 } 3719 3720 /** 3721 * @param value {@link #versionNeeded} (This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system.). This is the underlying object with id, value and extensions. The accessor "getVersionNeeded" gives direct access to the value 3722 */ 3723 public CodeSystem setVersionNeededElement(BooleanType value) { 3724 this.versionNeeded = value; 3725 return this; 3726 } 3727 3728 /** 3729 * @return This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system. 3730 */ 3731 public boolean getVersionNeeded() { 3732 return this.versionNeeded == null || this.versionNeeded.isEmpty() ? false : this.versionNeeded.getValue(); 3733 } 3734 3735 /** 3736 * @param value This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system. 3737 */ 3738 public CodeSystem setVersionNeeded(boolean value) { 3739 if (this.versionNeeded == null) 3740 this.versionNeeded = new BooleanType(); 3741 this.versionNeeded.setValue(value); 3742 return this; 3743 } 3744 3745 /** 3746 * @return {@link #content} (How much of the content of the code system - the concepts and codes it defines - are represented in this resource.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 3747 */ 3748 public Enumeration<CodeSystemContentMode> getContentElement() { 3749 if (this.content == null) 3750 if (Configuration.errorOnAutoCreate()) 3751 throw new Error("Attempt to auto-create CodeSystem.content"); 3752 else if (Configuration.doAutoCreate()) 3753 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); // bb 3754 return this.content; 3755 } 3756 3757 public boolean hasContentElement() { 3758 return this.content != null && !this.content.isEmpty(); 3759 } 3760 3761 public boolean hasContent() { 3762 return this.content != null && !this.content.isEmpty(); 3763 } 3764 3765 /** 3766 * @param value {@link #content} (How much of the content of the code system - the concepts and codes it defines - are represented in this resource.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 3767 */ 3768 public CodeSystem setContentElement(Enumeration<CodeSystemContentMode> value) { 3769 this.content = value; 3770 return this; 3771 } 3772 3773 /** 3774 * @return How much of the content of the code system - the concepts and codes it defines - are represented in this resource. 3775 */ 3776 public CodeSystemContentMode getContent() { 3777 return this.content == null ? null : this.content.getValue(); 3778 } 3779 3780 /** 3781 * @param value How much of the content of the code system - the concepts and codes it defines - are represented in this resource. 3782 */ 3783 public CodeSystem setContent(CodeSystemContentMode value) { 3784 if (this.content == null) 3785 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); 3786 this.content.setValue(value); 3787 return this; 3788 } 3789 3790 /** 3791 * @return {@link #count} (The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 3792 */ 3793 public UnsignedIntType getCountElement() { 3794 if (this.count == null) 3795 if (Configuration.errorOnAutoCreate()) 3796 throw new Error("Attempt to auto-create CodeSystem.count"); 3797 else if (Configuration.doAutoCreate()) 3798 this.count = new UnsignedIntType(); // bb 3799 return this.count; 3800 } 3801 3802 public boolean hasCountElement() { 3803 return this.count != null && !this.count.isEmpty(); 3804 } 3805 3806 public boolean hasCount() { 3807 return this.count != null && !this.count.isEmpty(); 3808 } 3809 3810 /** 3811 * @param value {@link #count} (The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 3812 */ 3813 public CodeSystem setCountElement(UnsignedIntType value) { 3814 this.count = value; 3815 return this; 3816 } 3817 3818 /** 3819 * @return The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts. 3820 */ 3821 public int getCount() { 3822 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 3823 } 3824 3825 /** 3826 * @param value The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts. 3827 */ 3828 public CodeSystem setCount(int value) { 3829 if (this.count == null) 3830 this.count = new UnsignedIntType(); 3831 this.count.setValue(value); 3832 return this; 3833 } 3834 3835 /** 3836 * @return {@link #filter} (A filter that can be used in a value set compose statement when selecting concepts using a filter.) 3837 */ 3838 public List<CodeSystemFilterComponent> getFilter() { 3839 if (this.filter == null) 3840 this.filter = new ArrayList<CodeSystemFilterComponent>(); 3841 return this.filter; 3842 } 3843 3844 /** 3845 * @return Returns a reference to <code>this</code> for easy method chaining 3846 */ 3847 public CodeSystem setFilter(List<CodeSystemFilterComponent> theFilter) { 3848 this.filter = theFilter; 3849 return this; 3850 } 3851 3852 public boolean hasFilter() { 3853 if (this.filter == null) 3854 return false; 3855 for (CodeSystemFilterComponent item : this.filter) 3856 if (!item.isEmpty()) 3857 return true; 3858 return false; 3859 } 3860 3861 public CodeSystemFilterComponent addFilter() { //3 3862 CodeSystemFilterComponent t = new CodeSystemFilterComponent(); 3863 if (this.filter == null) 3864 this.filter = new ArrayList<CodeSystemFilterComponent>(); 3865 this.filter.add(t); 3866 return t; 3867 } 3868 3869 public CodeSystem addFilter(CodeSystemFilterComponent t) { //3 3870 if (t == null) 3871 return this; 3872 if (this.filter == null) 3873 this.filter = new ArrayList<CodeSystemFilterComponent>(); 3874 this.filter.add(t); 3875 return this; 3876 } 3877 3878 /** 3879 * @return The first repetition of repeating field {@link #filter}, creating it if it does not already exist 3880 */ 3881 public CodeSystemFilterComponent getFilterFirstRep() { 3882 if (getFilter().isEmpty()) { 3883 addFilter(); 3884 } 3885 return getFilter().get(0); 3886 } 3887 3888 /** 3889 * @return {@link #property} (A property defines an additional slot through which additional information can be provided about a concept.) 3890 */ 3891 public List<PropertyComponent> getProperty() { 3892 if (this.property == null) 3893 this.property = new ArrayList<PropertyComponent>(); 3894 return this.property; 3895 } 3896 3897 /** 3898 * @return Returns a reference to <code>this</code> for easy method chaining 3899 */ 3900 public CodeSystem setProperty(List<PropertyComponent> theProperty) { 3901 this.property = theProperty; 3902 return this; 3903 } 3904 3905 public boolean hasProperty() { 3906 if (this.property == null) 3907 return false; 3908 for (PropertyComponent item : this.property) 3909 if (!item.isEmpty()) 3910 return true; 3911 return false; 3912 } 3913 3914 public PropertyComponent addProperty() { //3 3915 PropertyComponent t = new PropertyComponent(); 3916 if (this.property == null) 3917 this.property = new ArrayList<PropertyComponent>(); 3918 this.property.add(t); 3919 return t; 3920 } 3921 3922 public CodeSystem addProperty(PropertyComponent t) { //3 3923 if (t == null) 3924 return this; 3925 if (this.property == null) 3926 this.property = new ArrayList<PropertyComponent>(); 3927 this.property.add(t); 3928 return this; 3929 } 3930 3931 /** 3932 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist 3933 */ 3934 public PropertyComponent getPropertyFirstRep() { 3935 if (getProperty().isEmpty()) { 3936 addProperty(); 3937 } 3938 return getProperty().get(0); 3939 } 3940 3941 /** 3942 * @return {@link #concept} (Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meaning of the hierarchical relationships are.) 3943 */ 3944 public List<ConceptDefinitionComponent> getConcept() { 3945 if (this.concept == null) 3946 this.concept = new ArrayList<ConceptDefinitionComponent>(); 3947 return this.concept; 3948 } 3949 3950 /** 3951 * @return Returns a reference to <code>this</code> for easy method chaining 3952 */ 3953 public CodeSystem setConcept(List<ConceptDefinitionComponent> theConcept) { 3954 this.concept = theConcept; 3955 return this; 3956 } 3957 3958 public boolean hasConcept() { 3959 if (this.concept == null) 3960 return false; 3961 for (ConceptDefinitionComponent item : this.concept) 3962 if (!item.isEmpty()) 3963 return true; 3964 return false; 3965 } 3966 3967 public ConceptDefinitionComponent addConcept() { //3 3968 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 3969 if (this.concept == null) 3970 this.concept = new ArrayList<ConceptDefinitionComponent>(); 3971 this.concept.add(t); 3972 return t; 3973 } 3974 3975 public CodeSystem addConcept(ConceptDefinitionComponent t) { //3 3976 if (t == null) 3977 return this; 3978 if (this.concept == null) 3979 this.concept = new ArrayList<ConceptDefinitionComponent>(); 3980 this.concept.add(t); 3981 return this; 3982 } 3983 3984 /** 3985 * @return The first repetition of repeating field {@link #concept}, creating it if it does not already exist 3986 */ 3987 public ConceptDefinitionComponent getConceptFirstRep() { 3988 if (getConcept().isEmpty()) { 3989 addConcept(); 3990 } 3991 return getConcept().get(0); 3992 } 3993 3994 protected void listChildren(List<Property> children) { 3995 super.listChildren(children); 3996 children.add(new Property("url", "uri", "An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this code system is (or will be) published. The URL SHOULD include the major version of the code system. For more information see [Technical and Business Versions](resource.html#versions). This is used in [Coding]{datatypes.html#Coding}.system.", 0, 1, url)); 3997 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier)); 3998 children.add(new Property("version", "string", "The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding]{datatypes.html#Coding}.version.", 0, 1, version)); 3999 children.add(new Property("name", "string", "A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 4000 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the code system.", 0, 1, title)); 4001 children.add(new Property("status", "code", "The status of this code system. Enables tracking the life-cycle of the content.", 0, 1, status)); 4002 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 4003 children.add(new Property("date", "dateTime", "The date (and optionally time) when the code system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.", 0, 1, date)); 4004 children.add(new Property("publisher", "string", "The name of the individual or organization that published the code system.", 0, 1, publisher)); 4005 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4006 children.add(new Property("description", "markdown", "A free text natural language description of the code system from a consumer's perspective.", 0, 1, description)); 4007 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate code system instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4008 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the code system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 4009 children.add(new Property("purpose", "markdown", "Explaination of why this code system is needed and why it has been designed as it has.", 0, 1, purpose)); 4010 children.add(new Property("copyright", "markdown", "A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.", 0, 1, copyright)); 4011 children.add(new Property("caseSensitive", "boolean", "If code comparison is case sensitive when codes within this system are compared to each other.", 0, 1, caseSensitive)); 4012 children.add(new Property("valueSet", "uri", "Canonical URL of value set that contains the entire code system.", 0, 1, valueSet)); 4013 children.add(new Property("hierarchyMeaning", "code", "The meaning of the hierarchy of concepts.", 0, 1, hierarchyMeaning)); 4014 children.add(new Property("compositional", "boolean", "True If code system defines a post-composition grammar.", 0, 1, compositional)); 4015 children.add(new Property("versionNeeded", "boolean", "This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system.", 0, 1, versionNeeded)); 4016 children.add(new Property("content", "code", "How much of the content of the code system - the concepts and codes it defines - are represented in this resource.", 0, 1, content)); 4017 children.add(new Property("count", "unsignedInt", "The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts.", 0, 1, count)); 4018 children.add(new Property("filter", "", "A filter that can be used in a value set compose statement when selecting concepts using a filter.", 0, java.lang.Integer.MAX_VALUE, filter)); 4019 children.add(new Property("property", "", "A property defines an additional slot through which additional information can be provided about a concept.", 0, java.lang.Integer.MAX_VALUE, property)); 4020 children.add(new Property("concept", "", "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meaning of the hierarchical relationships are.", 0, java.lang.Integer.MAX_VALUE, concept)); 4021 } 4022 4023 @Override 4024 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4025 switch (_hash) { 4026 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this code system is (or will be) published. The URL SHOULD include the major version of the code system. For more information see [Technical and Business Versions](resource.html#versions). This is used in [Coding]{datatypes.html#Coding}.system.", 0, 1, url); 4027 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier); 4028 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding]{datatypes.html#Coding}.version.", 0, 1, version); 4029 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 4030 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the code system.", 0, 1, title); 4031 case -892481550: /*status*/ return new Property("status", "code", "The status of this code system. Enables tracking the life-cycle of the content.", 0, 1, status); 4032 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 4033 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the code system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.", 0, 1, date); 4034 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the code system.", 0, 1, publisher); 4035 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4036 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the code system from a consumer's perspective.", 0, 1, description); 4037 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate code system instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4038 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the code system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 4039 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this code system is needed and why it has been designed as it has.", 0, 1, purpose); 4040 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.", 0, 1, copyright); 4041 case -35616442: /*caseSensitive*/ return new Property("caseSensitive", "boolean", "If code comparison is case sensitive when codes within this system are compared to each other.", 0, 1, caseSensitive); 4042 case -1410174671: /*valueSet*/ return new Property("valueSet", "uri", "Canonical URL of value set that contains the entire code system.", 0, 1, valueSet); 4043 case 1913078280: /*hierarchyMeaning*/ return new Property("hierarchyMeaning", "code", "The meaning of the hierarchy of concepts.", 0, 1, hierarchyMeaning); 4044 case 1248023381: /*compositional*/ return new Property("compositional", "boolean", "True If code system defines a post-composition grammar.", 0, 1, compositional); 4045 case 617270957: /*versionNeeded*/ return new Property("versionNeeded", "boolean", "This flag is used to signify that the code system has not (or does not) maintain the definitions, and a version must be specified when referencing this code system.", 0, 1, versionNeeded); 4046 case 951530617: /*content*/ return new Property("content", "code", "How much of the content of the code system - the concepts and codes it defines - are represented in this resource.", 0, 1, content); 4047 case 94851343: /*count*/ return new Property("count", "unsignedInt", "The total number of concepts defined by the code system. Where the code system has a compositional grammar, the count refers to the number of base (primitive) concepts.", 0, 1, count); 4048 case -1274492040: /*filter*/ return new Property("filter", "", "A filter that can be used in a value set compose statement when selecting concepts using a filter.", 0, java.lang.Integer.MAX_VALUE, filter); 4049 case -993141291: /*property*/ return new Property("property", "", "A property defines an additional slot through which additional information can be provided about a concept.", 0, java.lang.Integer.MAX_VALUE, property); 4050 case 951024232: /*concept*/ return new Property("concept", "", "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meaning of the hierarchical relationships are.", 0, java.lang.Integer.MAX_VALUE, concept); 4051 default: return super.getNamedProperty(_hash, _name, _checkValid); 4052 } 4053 4054 } 4055 4056 @Override 4057 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4058 switch (hash) { 4059 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4060 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 4061 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4062 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4063 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4064 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4065 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4066 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4067 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4068 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4069 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4070 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4071 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4072 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4073 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4074 case -35616442: /*caseSensitive*/ return this.caseSensitive == null ? new Base[0] : new Base[] {this.caseSensitive}; // BooleanType 4075 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // UriType 4076 case 1913078280: /*hierarchyMeaning*/ return this.hierarchyMeaning == null ? new Base[0] : new Base[] {this.hierarchyMeaning}; // Enumeration<CodeSystemHierarchyMeaning> 4077 case 1248023381: /*compositional*/ return this.compositional == null ? new Base[0] : new Base[] {this.compositional}; // BooleanType 4078 case 617270957: /*versionNeeded*/ return this.versionNeeded == null ? new Base[0] : new Base[] {this.versionNeeded}; // BooleanType 4079 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Enumeration<CodeSystemContentMode> 4080 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // UnsignedIntType 4081 case -1274492040: /*filter*/ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // CodeSystemFilterComponent 4082 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // PropertyComponent 4083 case 951024232: /*concept*/ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptDefinitionComponent 4084 default: return super.getProperty(hash, name, checkValid); 4085 } 4086 4087 } 4088 4089 @Override 4090 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4091 switch (hash) { 4092 case 116079: // url 4093 this.url = castToUri(value); // UriType 4094 return value; 4095 case -1618432855: // identifier 4096 this.identifier = castToIdentifier(value); // Identifier 4097 return value; 4098 case 351608024: // version 4099 this.version = castToString(value); // StringType 4100 return value; 4101 case 3373707: // name 4102 this.name = castToString(value); // StringType 4103 return value; 4104 case 110371416: // title 4105 this.title = castToString(value); // StringType 4106 return value; 4107 case -892481550: // status 4108 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4109 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4110 return value; 4111 case -404562712: // experimental 4112 this.experimental = castToBoolean(value); // BooleanType 4113 return value; 4114 case 3076014: // date 4115 this.date = castToDateTime(value); // DateTimeType 4116 return value; 4117 case 1447404028: // publisher 4118 this.publisher = castToString(value); // StringType 4119 return value; 4120 case 951526432: // contact 4121 this.getContact().add(castToContactDetail(value)); // ContactDetail 4122 return value; 4123 case -1724546052: // description 4124 this.description = castToMarkdown(value); // MarkdownType 4125 return value; 4126 case -669707736: // useContext 4127 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4128 return value; 4129 case -507075711: // jurisdiction 4130 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4131 return value; 4132 case -220463842: // purpose 4133 this.purpose = castToMarkdown(value); // MarkdownType 4134 return value; 4135 case 1522889671: // copyright 4136 this.copyright = castToMarkdown(value); // MarkdownType 4137 return value; 4138 case -35616442: // caseSensitive 4139 this.caseSensitive = castToBoolean(value); // BooleanType 4140 return value; 4141 case -1410174671: // valueSet 4142 this.valueSet = castToUri(value); // UriType 4143 return value; 4144 case 1913078280: // hierarchyMeaning 4145 value = new CodeSystemHierarchyMeaningEnumFactory().fromType(castToCode(value)); 4146 this.hierarchyMeaning = (Enumeration) value; // Enumeration<CodeSystemHierarchyMeaning> 4147 return value; 4148 case 1248023381: // compositional 4149 this.compositional = castToBoolean(value); // BooleanType 4150 return value; 4151 case 617270957: // versionNeeded 4152 this.versionNeeded = castToBoolean(value); // BooleanType 4153 return value; 4154 case 951530617: // content 4155 value = new CodeSystemContentModeEnumFactory().fromType(castToCode(value)); 4156 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 4157 return value; 4158 case 94851343: // count 4159 this.count = castToUnsignedInt(value); // UnsignedIntType 4160 return value; 4161 case -1274492040: // filter 4162 this.getFilter().add((CodeSystemFilterComponent) value); // CodeSystemFilterComponent 4163 return value; 4164 case -993141291: // property 4165 this.getProperty().add((PropertyComponent) value); // PropertyComponent 4166 return value; 4167 case 951024232: // concept 4168 this.getConcept().add((ConceptDefinitionComponent) value); // ConceptDefinitionComponent 4169 return value; 4170 default: return super.setProperty(hash, name, value); 4171 } 4172 4173 } 4174 4175 @Override 4176 public Base setProperty(String name, Base value) throws FHIRException { 4177 if (name.equals("url")) { 4178 this.url = castToUri(value); // UriType 4179 } else if (name.equals("identifier")) { 4180 this.identifier = castToIdentifier(value); // Identifier 4181 } else if (name.equals("version")) { 4182 this.version = castToString(value); // StringType 4183 } else if (name.equals("name")) { 4184 this.name = castToString(value); // StringType 4185 } else if (name.equals("title")) { 4186 this.title = castToString(value); // StringType 4187 } else if (name.equals("status")) { 4188 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4189 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4190 } else if (name.equals("experimental")) { 4191 this.experimental = castToBoolean(value); // BooleanType 4192 } else if (name.equals("date")) { 4193 this.date = castToDateTime(value); // DateTimeType 4194 } else if (name.equals("publisher")) { 4195 this.publisher = castToString(value); // StringType 4196 } else if (name.equals("contact")) { 4197 this.getContact().add(castToContactDetail(value)); 4198 } else if (name.equals("description")) { 4199 this.description = castToMarkdown(value); // MarkdownType 4200 } else if (name.equals("useContext")) { 4201 this.getUseContext().add(castToUsageContext(value)); 4202 } else if (name.equals("jurisdiction")) { 4203 this.getJurisdiction().add(castToCodeableConcept(value)); 4204 } else if (name.equals("purpose")) { 4205 this.purpose = castToMarkdown(value); // MarkdownType 4206 } else if (name.equals("copyright")) { 4207 this.copyright = castToMarkdown(value); // MarkdownType 4208 } else if (name.equals("caseSensitive")) { 4209 this.caseSensitive = castToBoolean(value); // BooleanType 4210 } else if (name.equals("valueSet")) { 4211 this.valueSet = castToUri(value); // UriType 4212 } else if (name.equals("hierarchyMeaning")) { 4213 value = new CodeSystemHierarchyMeaningEnumFactory().fromType(castToCode(value)); 4214 this.hierarchyMeaning = (Enumeration) value; // Enumeration<CodeSystemHierarchyMeaning> 4215 } else if (name.equals("compositional")) { 4216 this.compositional = castToBoolean(value); // BooleanType 4217 } else if (name.equals("versionNeeded")) { 4218 this.versionNeeded = castToBoolean(value); // BooleanType 4219 } else if (name.equals("content")) { 4220 value = new CodeSystemContentModeEnumFactory().fromType(castToCode(value)); 4221 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 4222 } else if (name.equals("count")) { 4223 this.count = castToUnsignedInt(value); // UnsignedIntType 4224 } else if (name.equals("filter")) { 4225 this.getFilter().add((CodeSystemFilterComponent) value); 4226 } else if (name.equals("property")) { 4227 this.getProperty().add((PropertyComponent) value); 4228 } else if (name.equals("concept")) { 4229 this.getConcept().add((ConceptDefinitionComponent) value); 4230 } else 4231 return super.setProperty(name, value); 4232 return value; 4233 } 4234 4235 @Override 4236 public Base makeProperty(int hash, String name) throws FHIRException { 4237 switch (hash) { 4238 case 116079: return getUrlElement(); 4239 case -1618432855: return getIdentifier(); 4240 case 351608024: return getVersionElement(); 4241 case 3373707: return getNameElement(); 4242 case 110371416: return getTitleElement(); 4243 case -892481550: return getStatusElement(); 4244 case -404562712: return getExperimentalElement(); 4245 case 3076014: return getDateElement(); 4246 case 1447404028: return getPublisherElement(); 4247 case 951526432: return addContact(); 4248 case -1724546052: return getDescriptionElement(); 4249 case -669707736: return addUseContext(); 4250 case -507075711: return addJurisdiction(); 4251 case -220463842: return getPurposeElement(); 4252 case 1522889671: return getCopyrightElement(); 4253 case -35616442: return getCaseSensitiveElement(); 4254 case -1410174671: return getValueSetElement(); 4255 case 1913078280: return getHierarchyMeaningElement(); 4256 case 1248023381: return getCompositionalElement(); 4257 case 617270957: return getVersionNeededElement(); 4258 case 951530617: return getContentElement(); 4259 case 94851343: return getCountElement(); 4260 case -1274492040: return addFilter(); 4261 case -993141291: return addProperty(); 4262 case 951024232: return addConcept(); 4263 default: return super.makeProperty(hash, name); 4264 } 4265 4266 } 4267 4268 @Override 4269 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4270 switch (hash) { 4271 case 116079: /*url*/ return new String[] {"uri"}; 4272 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4273 case 351608024: /*version*/ return new String[] {"string"}; 4274 case 3373707: /*name*/ return new String[] {"string"}; 4275 case 110371416: /*title*/ return new String[] {"string"}; 4276 case -892481550: /*status*/ return new String[] {"code"}; 4277 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4278 case 3076014: /*date*/ return new String[] {"dateTime"}; 4279 case 1447404028: /*publisher*/ return new String[] {"string"}; 4280 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4281 case -1724546052: /*description*/ return new String[] {"markdown"}; 4282 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4283 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4284 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4285 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4286 case -35616442: /*caseSensitive*/ return new String[] {"boolean"}; 4287 case -1410174671: /*valueSet*/ return new String[] {"uri"}; 4288 case 1913078280: /*hierarchyMeaning*/ return new String[] {"code"}; 4289 case 1248023381: /*compositional*/ return new String[] {"boolean"}; 4290 case 617270957: /*versionNeeded*/ return new String[] {"boolean"}; 4291 case 951530617: /*content*/ return new String[] {"code"}; 4292 case 94851343: /*count*/ return new String[] {"unsignedInt"}; 4293 case -1274492040: /*filter*/ return new String[] {}; 4294 case -993141291: /*property*/ return new String[] {}; 4295 case 951024232: /*concept*/ return new String[] {}; 4296 default: return super.getTypesForProperty(hash, name); 4297 } 4298 4299 } 4300 4301 @Override 4302 public Base addChild(String name) throws FHIRException { 4303 if (name.equals("url")) { 4304 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.url"); 4305 } 4306 else if (name.equals("identifier")) { 4307 this.identifier = new Identifier(); 4308 return this.identifier; 4309 } 4310 else if (name.equals("version")) { 4311 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.version"); 4312 } 4313 else if (name.equals("name")) { 4314 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.name"); 4315 } 4316 else if (name.equals("title")) { 4317 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.title"); 4318 } 4319 else if (name.equals("status")) { 4320 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.status"); 4321 } 4322 else if (name.equals("experimental")) { 4323 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.experimental"); 4324 } 4325 else if (name.equals("date")) { 4326 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.date"); 4327 } 4328 else if (name.equals("publisher")) { 4329 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.publisher"); 4330 } 4331 else if (name.equals("contact")) { 4332 return addContact(); 4333 } 4334 else if (name.equals("description")) { 4335 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.description"); 4336 } 4337 else if (name.equals("useContext")) { 4338 return addUseContext(); 4339 } 4340 else if (name.equals("jurisdiction")) { 4341 return addJurisdiction(); 4342 } 4343 else if (name.equals("purpose")) { 4344 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.purpose"); 4345 } 4346 else if (name.equals("copyright")) { 4347 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.copyright"); 4348 } 4349 else if (name.equals("caseSensitive")) { 4350 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.caseSensitive"); 4351 } 4352 else if (name.equals("valueSet")) { 4353 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.valueSet"); 4354 } 4355 else if (name.equals("hierarchyMeaning")) { 4356 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.hierarchyMeaning"); 4357 } 4358 else if (name.equals("compositional")) { 4359 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.compositional"); 4360 } 4361 else if (name.equals("versionNeeded")) { 4362 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.versionNeeded"); 4363 } 4364 else if (name.equals("content")) { 4365 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.content"); 4366 } 4367 else if (name.equals("count")) { 4368 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.count"); 4369 } 4370 else if (name.equals("filter")) { 4371 return addFilter(); 4372 } 4373 else if (name.equals("property")) { 4374 return addProperty(); 4375 } 4376 else if (name.equals("concept")) { 4377 return addConcept(); 4378 } 4379 else 4380 return super.addChild(name); 4381 } 4382 4383 public String fhirType() { 4384 return "CodeSystem"; 4385 4386 } 4387 4388 public CodeSystem copy() { 4389 CodeSystem dst = new CodeSystem(); 4390 copyValues(dst); 4391 dst.url = url == null ? null : url.copy(); 4392 dst.identifier = identifier == null ? null : identifier.copy(); 4393 dst.version = version == null ? null : version.copy(); 4394 dst.name = name == null ? null : name.copy(); 4395 dst.title = title == null ? null : title.copy(); 4396 dst.status = status == null ? null : status.copy(); 4397 dst.experimental = experimental == null ? null : experimental.copy(); 4398 dst.date = date == null ? null : date.copy(); 4399 dst.publisher = publisher == null ? null : publisher.copy(); 4400 if (contact != null) { 4401 dst.contact = new ArrayList<ContactDetail>(); 4402 for (ContactDetail i : contact) 4403 dst.contact.add(i.copy()); 4404 }; 4405 dst.description = description == null ? null : description.copy(); 4406 if (useContext != null) { 4407 dst.useContext = new ArrayList<UsageContext>(); 4408 for (UsageContext i : useContext) 4409 dst.useContext.add(i.copy()); 4410 }; 4411 if (jurisdiction != null) { 4412 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4413 for (CodeableConcept i : jurisdiction) 4414 dst.jurisdiction.add(i.copy()); 4415 }; 4416 dst.purpose = purpose == null ? null : purpose.copy(); 4417 dst.copyright = copyright == null ? null : copyright.copy(); 4418 dst.caseSensitive = caseSensitive == null ? null : caseSensitive.copy(); 4419 dst.valueSet = valueSet == null ? null : valueSet.copy(); 4420 dst.hierarchyMeaning = hierarchyMeaning == null ? null : hierarchyMeaning.copy(); 4421 dst.compositional = compositional == null ? null : compositional.copy(); 4422 dst.versionNeeded = versionNeeded == null ? null : versionNeeded.copy(); 4423 dst.content = content == null ? null : content.copy(); 4424 dst.count = count == null ? null : count.copy(); 4425 if (filter != null) { 4426 dst.filter = new ArrayList<CodeSystemFilterComponent>(); 4427 for (CodeSystemFilterComponent i : filter) 4428 dst.filter.add(i.copy()); 4429 }; 4430 if (property != null) { 4431 dst.property = new ArrayList<PropertyComponent>(); 4432 for (PropertyComponent i : property) 4433 dst.property.add(i.copy()); 4434 }; 4435 if (concept != null) { 4436 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 4437 for (ConceptDefinitionComponent i : concept) 4438 dst.concept.add(i.copy()); 4439 }; 4440 return dst; 4441 } 4442 4443 protected CodeSystem typedCopy() { 4444 return copy(); 4445 } 4446 4447 @Override 4448 public boolean equalsDeep(Base other_) { 4449 if (!super.equalsDeep(other_)) 4450 return false; 4451 if (!(other_ instanceof CodeSystem)) 4452 return false; 4453 CodeSystem o = (CodeSystem) other_; 4454 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 4455 && compareDeep(caseSensitive, o.caseSensitive, true) && compareDeep(valueSet, o.valueSet, true) 4456 && compareDeep(hierarchyMeaning, o.hierarchyMeaning, true) && compareDeep(compositional, o.compositional, true) 4457 && compareDeep(versionNeeded, o.versionNeeded, true) && compareDeep(content, o.content, true) && compareDeep(count, o.count, true) 4458 && compareDeep(filter, o.filter, true) && compareDeep(property, o.property, true) && compareDeep(concept, o.concept, true) 4459 ; 4460 } 4461 4462 @Override 4463 public boolean equalsShallow(Base other_) { 4464 if (!super.equalsShallow(other_)) 4465 return false; 4466 if (!(other_ instanceof CodeSystem)) 4467 return false; 4468 CodeSystem o = (CodeSystem) other_; 4469 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(caseSensitive, o.caseSensitive, true) 4470 && compareValues(valueSet, o.valueSet, true) && compareValues(hierarchyMeaning, o.hierarchyMeaning, true) 4471 && compareValues(compositional, o.compositional, true) && compareValues(versionNeeded, o.versionNeeded, true) 4472 && compareValues(content, o.content, true) && compareValues(count, o.count, true); 4473 } 4474 4475 public boolean isEmpty() { 4476 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright 4477 , caseSensitive, valueSet, hierarchyMeaning, compositional, versionNeeded, content 4478 , count, filter, property, concept); 4479 } 4480 4481 @Override 4482 public ResourceType getResourceType() { 4483 return ResourceType.CodeSystem; 4484 } 4485 4486 /** 4487 * Search parameter: <b>date</b> 4488 * <p> 4489 * Description: <b>The code system publication date</b><br> 4490 * Type: <b>date</b><br> 4491 * Path: <b>CodeSystem.date</b><br> 4492 * </p> 4493 */ 4494 @SearchParamDefinition(name="date", path="CodeSystem.date", description="The code system publication date", type="date" ) 4495 public static final String SP_DATE = "date"; 4496 /** 4497 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4498 * <p> 4499 * Description: <b>The code system publication date</b><br> 4500 * Type: <b>date</b><br> 4501 * Path: <b>CodeSystem.date</b><br> 4502 * </p> 4503 */ 4504 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4505 4506 /** 4507 * Search parameter: <b>identifier</b> 4508 * <p> 4509 * Description: <b>External identifier for the code system</b><br> 4510 * Type: <b>token</b><br> 4511 * Path: <b>CodeSystem.identifier</b><br> 4512 * </p> 4513 */ 4514 @SearchParamDefinition(name="identifier", path="CodeSystem.identifier", description="External identifier for the code system", type="token" ) 4515 public static final String SP_IDENTIFIER = "identifier"; 4516 /** 4517 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4518 * <p> 4519 * Description: <b>External identifier for the code system</b><br> 4520 * Type: <b>token</b><br> 4521 * Path: <b>CodeSystem.identifier</b><br> 4522 * </p> 4523 */ 4524 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4525 4526 /** 4527 * Search parameter: <b>code</b> 4528 * <p> 4529 * Description: <b>A code defined in the code system</b><br> 4530 * Type: <b>token</b><br> 4531 * Path: <b>CodeSystem.concept.code</b><br> 4532 * </p> 4533 */ 4534 @SearchParamDefinition(name="code", path="CodeSystem.concept.code", description="A code defined in the code system", type="token" ) 4535 public static final String SP_CODE = "code"; 4536 /** 4537 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4538 * <p> 4539 * Description: <b>A code defined in the code system</b><br> 4540 * Type: <b>token</b><br> 4541 * Path: <b>CodeSystem.concept.code</b><br> 4542 * </p> 4543 */ 4544 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4545 4546 /** 4547 * Search parameter: <b>content-mode</b> 4548 * <p> 4549 * Description: <b>not-present | example | fragment | complete</b><br> 4550 * Type: <b>token</b><br> 4551 * Path: <b>CodeSystem.content</b><br> 4552 * </p> 4553 */ 4554 @SearchParamDefinition(name="content-mode", path="CodeSystem.content", description="not-present | example | fragment | complete", type="token" ) 4555 public static final String SP_CONTENT_MODE = "content-mode"; 4556 /** 4557 * <b>Fluent Client</b> search parameter constant for <b>content-mode</b> 4558 * <p> 4559 * Description: <b>not-present | example | fragment | complete</b><br> 4560 * Type: <b>token</b><br> 4561 * Path: <b>CodeSystem.content</b><br> 4562 * </p> 4563 */ 4564 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENT_MODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTENT_MODE); 4565 4566 /** 4567 * Search parameter: <b>jurisdiction</b> 4568 * <p> 4569 * Description: <b>Intended jurisdiction for the code system</b><br> 4570 * Type: <b>token</b><br> 4571 * Path: <b>CodeSystem.jurisdiction</b><br> 4572 * </p> 4573 */ 4574 @SearchParamDefinition(name="jurisdiction", path="CodeSystem.jurisdiction", description="Intended jurisdiction for the code system", type="token" ) 4575 public static final String SP_JURISDICTION = "jurisdiction"; 4576 /** 4577 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4578 * <p> 4579 * Description: <b>Intended jurisdiction for the code system</b><br> 4580 * Type: <b>token</b><br> 4581 * Path: <b>CodeSystem.jurisdiction</b><br> 4582 * </p> 4583 */ 4584 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4585 4586 /** 4587 * Search parameter: <b>description</b> 4588 * <p> 4589 * Description: <b>The description of the code system</b><br> 4590 * Type: <b>string</b><br> 4591 * Path: <b>CodeSystem.description</b><br> 4592 * </p> 4593 */ 4594 @SearchParamDefinition(name="description", path="CodeSystem.description", description="The description of the code system", type="string" ) 4595 public static final String SP_DESCRIPTION = "description"; 4596 /** 4597 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4598 * <p> 4599 * Description: <b>The description of the code system</b><br> 4600 * Type: <b>string</b><br> 4601 * Path: <b>CodeSystem.description</b><br> 4602 * </p> 4603 */ 4604 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4605 4606 /** 4607 * Search parameter: <b>language</b> 4608 * <p> 4609 * Description: <b>A language in which a designation is provided</b><br> 4610 * Type: <b>token</b><br> 4611 * Path: <b>CodeSystem.concept.designation.language</b><br> 4612 * </p> 4613 */ 4614 @SearchParamDefinition(name="language", path="CodeSystem.concept.designation.language", description="A language in which a designation is provided", type="token" ) 4615 public static final String SP_LANGUAGE = "language"; 4616 /** 4617 * <b>Fluent Client</b> search parameter constant for <b>language</b> 4618 * <p> 4619 * Description: <b>A language in which a designation is provided</b><br> 4620 * Type: <b>token</b><br> 4621 * Path: <b>CodeSystem.concept.designation.language</b><br> 4622 * </p> 4623 */ 4624 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LANGUAGE); 4625 4626 /** 4627 * Search parameter: <b>title</b> 4628 * <p> 4629 * Description: <b>The human-friendly name of the code system</b><br> 4630 * Type: <b>string</b><br> 4631 * Path: <b>CodeSystem.title</b><br> 4632 * </p> 4633 */ 4634 @SearchParamDefinition(name="title", path="CodeSystem.title", description="The human-friendly name of the code system", type="string" ) 4635 public static final String SP_TITLE = "title"; 4636 /** 4637 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4638 * <p> 4639 * Description: <b>The human-friendly name of the code system</b><br> 4640 * Type: <b>string</b><br> 4641 * Path: <b>CodeSystem.title</b><br> 4642 * </p> 4643 */ 4644 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4645 4646 /** 4647 * Search parameter: <b>version</b> 4648 * <p> 4649 * Description: <b>The business version of the code system</b><br> 4650 * Type: <b>token</b><br> 4651 * Path: <b>CodeSystem.version</b><br> 4652 * </p> 4653 */ 4654 @SearchParamDefinition(name="version", path="CodeSystem.version", description="The business version of the code system", type="token" ) 4655 public static final String SP_VERSION = "version"; 4656 /** 4657 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4658 * <p> 4659 * Description: <b>The business version of the code system</b><br> 4660 * Type: <b>token</b><br> 4661 * Path: <b>CodeSystem.version</b><br> 4662 * </p> 4663 */ 4664 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4665 4666 /** 4667 * Search parameter: <b>url</b> 4668 * <p> 4669 * Description: <b>The uri that identifies the code system</b><br> 4670 * Type: <b>uri</b><br> 4671 * Path: <b>CodeSystem.url</b><br> 4672 * </p> 4673 */ 4674 @SearchParamDefinition(name="url", path="CodeSystem.url", description="The uri that identifies the code system", type="uri" ) 4675 public static final String SP_URL = "url"; 4676 /** 4677 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4678 * <p> 4679 * Description: <b>The uri that identifies the code system</b><br> 4680 * Type: <b>uri</b><br> 4681 * Path: <b>CodeSystem.url</b><br> 4682 * </p> 4683 */ 4684 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4685 4686 /** 4687 * Search parameter: <b>system</b> 4688 * <p> 4689 * Description: <b>The system for any codes defined by this code system (same as 'url')</b><br> 4690 * Type: <b>uri</b><br> 4691 * Path: <b>CodeSystem.url</b><br> 4692 * </p> 4693 */ 4694 @SearchParamDefinition(name="system", path="CodeSystem.url", description="The system for any codes defined by this code system (same as 'url')", type="uri" ) 4695 public static final String SP_SYSTEM = "system"; 4696 /** 4697 * <b>Fluent Client</b> search parameter constant for <b>system</b> 4698 * <p> 4699 * Description: <b>The system for any codes defined by this code system (same as 'url')</b><br> 4700 * Type: <b>uri</b><br> 4701 * Path: <b>CodeSystem.url</b><br> 4702 * </p> 4703 */ 4704 public static final ca.uhn.fhir.rest.gclient.UriClientParam SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SYSTEM); 4705 4706 /** 4707 * Search parameter: <b>name</b> 4708 * <p> 4709 * Description: <b>Computationally friendly name of the code system</b><br> 4710 * Type: <b>string</b><br> 4711 * Path: <b>CodeSystem.name</b><br> 4712 * </p> 4713 */ 4714 @SearchParamDefinition(name="name", path="CodeSystem.name", description="Computationally friendly name of the code system", type="string" ) 4715 public static final String SP_NAME = "name"; 4716 /** 4717 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4718 * <p> 4719 * Description: <b>Computationally friendly name of the code system</b><br> 4720 * Type: <b>string</b><br> 4721 * Path: <b>CodeSystem.name</b><br> 4722 * </p> 4723 */ 4724 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4725 4726 /** 4727 * Search parameter: <b>publisher</b> 4728 * <p> 4729 * Description: <b>Name of the publisher of the code system</b><br> 4730 * Type: <b>string</b><br> 4731 * Path: <b>CodeSystem.publisher</b><br> 4732 * </p> 4733 */ 4734 @SearchParamDefinition(name="publisher", path="CodeSystem.publisher", description="Name of the publisher of the code system", type="string" ) 4735 public static final String SP_PUBLISHER = "publisher"; 4736 /** 4737 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4738 * <p> 4739 * Description: <b>Name of the publisher of the code system</b><br> 4740 * Type: <b>string</b><br> 4741 * Path: <b>CodeSystem.publisher</b><br> 4742 * </p> 4743 */ 4744 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4745 4746 /** 4747 * Search parameter: <b>status</b> 4748 * <p> 4749 * Description: <b>The current status of the code system</b><br> 4750 * Type: <b>token</b><br> 4751 * Path: <b>CodeSystem.status</b><br> 4752 * </p> 4753 */ 4754 @SearchParamDefinition(name="status", path="CodeSystem.status", description="The current status of the code system", type="token" ) 4755 public static final String SP_STATUS = "status"; 4756 /** 4757 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4758 * <p> 4759 * Description: <b>The current status of the code system</b><br> 4760 * Type: <b>token</b><br> 4761 * Path: <b>CodeSystem.status</b><br> 4762 * </p> 4763 */ 4764 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4765 4766 4767}