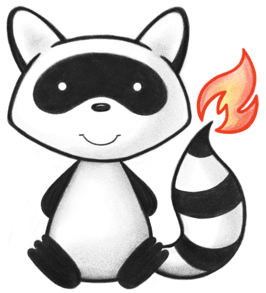
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseCoding; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046/** 047 * A reference to a code defined by a terminology system. 048 */ 049@DatatypeDef(name="Coding") 050public class Coding extends Type implements IBaseCoding, ICompositeType { 051 052 /** 053 * The identification of the code system that defines the meaning of the symbol in the code. 054 */ 055 @Child(name = "system", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 056 @Description(shortDefinition="Identity of the terminology system", formalDefinition="The identification of the code system that defines the meaning of the symbol in the code." ) 057 protected UriType system; 058 059 /** 060 * The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 061 */ 062 @Child(name = "version", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 063 @Description(shortDefinition="Version of the system - if relevant", formalDefinition="The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged." ) 064 protected StringType version; 065 066 /** 067 * A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination). 068 */ 069 @Child(name = "code", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="Symbol in syntax defined by the system", formalDefinition="A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination)." ) 071 protected CodeType code; 072 073 /** 074 * A representation of the meaning of the code in the system, following the rules of the system. 075 */ 076 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 077 @Description(shortDefinition="Representation defined by the system", formalDefinition="A representation of the meaning of the code in the system, following the rules of the system." ) 078 protected StringType display; 079 080 /** 081 * Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays). 082 */ 083 @Child(name = "userSelected", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 084 @Description(shortDefinition="If this coding was chosen directly by the user", formalDefinition="Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays)." ) 085 protected BooleanType userSelected; 086 087 private static final long serialVersionUID = -1417514061L; 088 089 /** 090 * Constructor 091 */ 092 public Coding() { 093 super(); 094 } 095 096 /** 097 * Convenience constructor 098 * 099 * @param theSystem The {@link #setSystem(String) code system} 100 * @param theCode The {@link #setCode(String) code} 101 * @param theDisplay The {@link #setDisplay(String) human readable display} 102 */ 103 public Coding(String theSystem, String theCode, String theDisplay) { 104 setSystem(theSystem); 105 setCode(theCode); 106 setDisplay(theDisplay); 107 } 108 /** 109 * @return {@link #system} (The identification of the code system that defines the meaning of the symbol in the code.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 110 */ 111 public UriType getSystemElement() { 112 if (this.system == null) 113 if (Configuration.errorOnAutoCreate()) 114 throw new Error("Attempt to auto-create Coding.system"); 115 else if (Configuration.doAutoCreate()) 116 this.system = new UriType(); // bb 117 return this.system; 118 } 119 120 public boolean hasSystemElement() { 121 return this.system != null && !this.system.isEmpty(); 122 } 123 124 public boolean hasSystem() { 125 return this.system != null && !this.system.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #system} (The identification of the code system that defines the meaning of the symbol in the code.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 130 */ 131 public Coding setSystemElement(UriType value) { 132 this.system = value; 133 return this; 134 } 135 136 /** 137 * @return The identification of the code system that defines the meaning of the symbol in the code. 138 */ 139 public String getSystem() { 140 return this.system == null ? null : this.system.getValue(); 141 } 142 143 /** 144 * @param value The identification of the code system that defines the meaning of the symbol in the code. 145 */ 146 public Coding setSystem(String value) { 147 if (Utilities.noString(value)) 148 this.system = null; 149 else { 150 if (this.system == null) 151 this.system = new UriType(); 152 this.system.setValue(value); 153 } 154 return this; 155 } 156 157 /** 158 * @return {@link #version} (The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 159 */ 160 public StringType getVersionElement() { 161 if (this.version == null) 162 if (Configuration.errorOnAutoCreate()) 163 throw new Error("Attempt to auto-create Coding.version"); 164 else if (Configuration.doAutoCreate()) 165 this.version = new StringType(); // bb 166 return this.version; 167 } 168 169 public boolean hasVersionElement() { 170 return this.version != null && !this.version.isEmpty(); 171 } 172 173 public boolean hasVersion() { 174 return this.version != null && !this.version.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #version} (The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 179 */ 180 public Coding setVersionElement(StringType value) { 181 this.version = value; 182 return this; 183 } 184 185 /** 186 * @return The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 187 */ 188 public String getVersion() { 189 return this.version == null ? null : this.version.getValue(); 190 } 191 192 /** 193 * @param value The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 194 */ 195 public Coding setVersion(String value) { 196 if (Utilities.noString(value)) 197 this.version = null; 198 else { 199 if (this.version == null) 200 this.version = new StringType(); 201 this.version.setValue(value); 202 } 203 return this; 204 } 205 206 /** 207 * @return {@link #code} (A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 208 */ 209 public CodeType getCodeElement() { 210 if (this.code == null) 211 if (Configuration.errorOnAutoCreate()) 212 throw new Error("Attempt to auto-create Coding.code"); 213 else if (Configuration.doAutoCreate()) 214 this.code = new CodeType(); // bb 215 return this.code; 216 } 217 218 public boolean hasCodeElement() { 219 return this.code != null && !this.code.isEmpty(); 220 } 221 222 public boolean hasCode() { 223 return this.code != null && !this.code.isEmpty(); 224 } 225 226 /** 227 * @param value {@link #code} (A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 228 */ 229 public Coding setCodeElement(CodeType value) { 230 this.code = value; 231 return this; 232 } 233 234 /** 235 * @return A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination). 236 */ 237 public String getCode() { 238 return this.code == null ? null : this.code.getValue(); 239 } 240 241 /** 242 * @param value A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination). 243 */ 244 public Coding setCode(String value) { 245 if (Utilities.noString(value)) 246 this.code = null; 247 else { 248 if (this.code == null) 249 this.code = new CodeType(); 250 this.code.setValue(value); 251 } 252 return this; 253 } 254 255 /** 256 * @return {@link #display} (A representation of the meaning of the code in the system, following the rules of the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 257 */ 258 public StringType getDisplayElement() { 259 if (this.display == null) 260 if (Configuration.errorOnAutoCreate()) 261 throw new Error("Attempt to auto-create Coding.display"); 262 else if (Configuration.doAutoCreate()) 263 this.display = new StringType(); // bb 264 return this.display; 265 } 266 267 public boolean hasDisplayElement() { 268 return this.display != null && !this.display.isEmpty(); 269 } 270 271 public boolean hasDisplay() { 272 return this.display != null && !this.display.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #display} (A representation of the meaning of the code in the system, following the rules of the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 277 */ 278 public Coding setDisplayElement(StringType value) { 279 this.display = value; 280 return this; 281 } 282 283 /** 284 * @return A representation of the meaning of the code in the system, following the rules of the system. 285 */ 286 public String getDisplay() { 287 return this.display == null ? null : this.display.getValue(); 288 } 289 290 /** 291 * @param value A representation of the meaning of the code in the system, following the rules of the system. 292 */ 293 public Coding setDisplay(String value) { 294 if (Utilities.noString(value)) 295 this.display = null; 296 else { 297 if (this.display == null) 298 this.display = new StringType(); 299 this.display.setValue(value); 300 } 301 return this; 302 } 303 304 /** 305 * @return {@link #userSelected} (Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays).). This is the underlying object with id, value and extensions. The accessor "getUserSelected" gives direct access to the value 306 */ 307 public BooleanType getUserSelectedElement() { 308 if (this.userSelected == null) 309 if (Configuration.errorOnAutoCreate()) 310 throw new Error("Attempt to auto-create Coding.userSelected"); 311 else if (Configuration.doAutoCreate()) 312 this.userSelected = new BooleanType(); // bb 313 return this.userSelected; 314 } 315 316 public boolean hasUserSelectedElement() { 317 return this.userSelected != null && !this.userSelected.isEmpty(); 318 } 319 320 public boolean hasUserSelected() { 321 return this.userSelected != null && !this.userSelected.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #userSelected} (Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays).). This is the underlying object with id, value and extensions. The accessor "getUserSelected" gives direct access to the value 326 */ 327 public Coding setUserSelectedElement(BooleanType value) { 328 this.userSelected = value; 329 return this; 330 } 331 332 /** 333 * @return Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays). 334 */ 335 public boolean getUserSelected() { 336 return this.userSelected == null || this.userSelected.isEmpty() ? false : this.userSelected.getValue(); 337 } 338 339 /** 340 * @param value Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays). 341 */ 342 public Coding setUserSelected(boolean value) { 343 if (this.userSelected == null) 344 this.userSelected = new BooleanType(); 345 this.userSelected.setValue(value); 346 return this; 347 } 348 349 protected void listChildren(List<Property> children) { 350 super.listChildren(children); 351 children.add(new Property("system", "uri", "The identification of the code system that defines the meaning of the symbol in the code.", 0, 1, system)); 352 children.add(new Property("version", "string", "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 0, 1, version)); 353 children.add(new Property("code", "code", "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).", 0, 1, code)); 354 children.add(new Property("display", "string", "A representation of the meaning of the code in the system, following the rules of the system.", 0, 1, display)); 355 children.add(new Property("userSelected", "boolean", "Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays).", 0, 1, userSelected)); 356 } 357 358 @Override 359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 360 switch (_hash) { 361 case -887328209: /*system*/ return new Property("system", "uri", "The identification of the code system that defines the meaning of the symbol in the code.", 0, 1, system); 362 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 0, 1, version); 363 case 3059181: /*code*/ return new Property("code", "code", "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).", 0, 1, code); 364 case 1671764162: /*display*/ return new Property("display", "string", "A representation of the meaning of the code in the system, following the rules of the system.", 0, 1, display); 365 case 423643014: /*userSelected*/ return new Property("userSelected", "boolean", "Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays).", 0, 1, userSelected); 366 default: return super.getNamedProperty(_hash, _name, _checkValid); 367 } 368 369 } 370 371 @Override 372 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 373 switch (hash) { 374 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 375 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 376 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 377 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 378 case 423643014: /*userSelected*/ return this.userSelected == null ? new Base[0] : new Base[] {this.userSelected}; // BooleanType 379 default: return super.getProperty(hash, name, checkValid); 380 } 381 382 } 383 384 @Override 385 public Base setProperty(int hash, String name, Base value) throws FHIRException { 386 switch (hash) { 387 case -887328209: // system 388 this.system = castToUri(value); // UriType 389 return value; 390 case 351608024: // version 391 this.version = castToString(value); // StringType 392 return value; 393 case 3059181: // code 394 this.code = castToCode(value); // CodeType 395 return value; 396 case 1671764162: // display 397 this.display = castToString(value); // StringType 398 return value; 399 case 423643014: // userSelected 400 this.userSelected = castToBoolean(value); // BooleanType 401 return value; 402 default: return super.setProperty(hash, name, value); 403 } 404 405 } 406 407 @Override 408 public Base setProperty(String name, Base value) throws FHIRException { 409 if (name.equals("system")) { 410 this.system = castToUri(value); // UriType 411 } else if (name.equals("version")) { 412 this.version = castToString(value); // StringType 413 } else if (name.equals("code")) { 414 this.code = castToCode(value); // CodeType 415 } else if (name.equals("display")) { 416 this.display = castToString(value); // StringType 417 } else if (name.equals("userSelected")) { 418 this.userSelected = castToBoolean(value); // BooleanType 419 } else 420 return super.setProperty(name, value); 421 return value; 422 } 423 424 @Override 425 public Base makeProperty(int hash, String name) throws FHIRException { 426 switch (hash) { 427 case -887328209: return getSystemElement(); 428 case 351608024: return getVersionElement(); 429 case 3059181: return getCodeElement(); 430 case 1671764162: return getDisplayElement(); 431 case 423643014: return getUserSelectedElement(); 432 default: return super.makeProperty(hash, name); 433 } 434 435 } 436 437 @Override 438 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 439 switch (hash) { 440 case -887328209: /*system*/ return new String[] {"uri"}; 441 case 351608024: /*version*/ return new String[] {"string"}; 442 case 3059181: /*code*/ return new String[] {"code"}; 443 case 1671764162: /*display*/ return new String[] {"string"}; 444 case 423643014: /*userSelected*/ return new String[] {"boolean"}; 445 default: return super.getTypesForProperty(hash, name); 446 } 447 448 } 449 450 @Override 451 public Base addChild(String name) throws FHIRException { 452 if (name.equals("system")) { 453 throw new FHIRException("Cannot call addChild on a singleton property Coding.system"); 454 } 455 else if (name.equals("version")) { 456 throw new FHIRException("Cannot call addChild on a singleton property Coding.version"); 457 } 458 else if (name.equals("code")) { 459 throw new FHIRException("Cannot call addChild on a singleton property Coding.code"); 460 } 461 else if (name.equals("display")) { 462 throw new FHIRException("Cannot call addChild on a singleton property Coding.display"); 463 } 464 else if (name.equals("userSelected")) { 465 throw new FHIRException("Cannot call addChild on a singleton property Coding.userSelected"); 466 } 467 else 468 return super.addChild(name); 469 } 470 471 public String fhirType() { 472 return "Coding"; 473 474 } 475 476 public Coding copy() { 477 Coding dst = new Coding(); 478 copyValues(dst); 479 dst.system = system == null ? null : system.copy(); 480 dst.version = version == null ? null : version.copy(); 481 dst.code = code == null ? null : code.copy(); 482 dst.display = display == null ? null : display.copy(); 483 dst.userSelected = userSelected == null ? null : userSelected.copy(); 484 return dst; 485 } 486 487 protected Coding typedCopy() { 488 return copy(); 489 } 490 491 @Override 492 public boolean equalsDeep(Base other_) { 493 if (!super.equalsDeep(other_)) 494 return false; 495 if (!(other_ instanceof Coding)) 496 return false; 497 Coding o = (Coding) other_; 498 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) && compareDeep(code, o.code, true) 499 && compareDeep(display, o.display, true) && compareDeep(userSelected, o.userSelected, true); 500 } 501 502 @Override 503 public boolean equalsShallow(Base other_) { 504 if (!super.equalsShallow(other_)) 505 return false; 506 if (!(other_ instanceof Coding)) 507 return false; 508 Coding o = (Coding) other_; 509 return compareValues(system, o.system, true) && compareValues(version, o.version, true) && compareValues(code, o.code, true) 510 && compareValues(display, o.display, true) && compareValues(userSelected, o.userSelected, true); 511 } 512 513 public boolean isEmpty() { 514 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, code, display 515 , userSelected); 516 } 517 518 519}