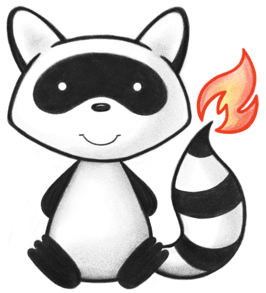
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 050 */ 051@ResourceDef(name="Communication", profile="http://hl7.org/fhir/Profile/Communication") 052public class Communication extends DomainResource { 053 054 public enum CommunicationStatus { 055 /** 056 * The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes. 057 */ 058 PREPARATION, 059 /** 060 * The event is currently occurring 061 */ 062 INPROGRESS, 063 /** 064 * The event has been temporarily stopped but is expected to resume in the future 065 */ 066 SUSPENDED, 067 /** 068 * The event was prior to the full completion of the intended actions 069 */ 070 ABORTED, 071 /** 072 * The event has now concluded 073 */ 074 COMPLETED, 075 /** 076 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static CommunicationStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("preparation".equals(codeString)) 091 return PREPARATION; 092 if ("in-progress".equals(codeString)) 093 return INPROGRESS; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("aborted".equals(codeString)) 097 return ABORTED; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown CommunicationStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case PREPARATION: return "preparation"; 112 case INPROGRESS: return "in-progress"; 113 case SUSPENDED: return "suspended"; 114 case ABORTED: return "aborted"; 115 case COMPLETED: return "completed"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case PREPARATION: return "http://hl7.org/fhir/event-status"; 125 case INPROGRESS: return "http://hl7.org/fhir/event-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/event-status"; 127 case ABORTED: return "http://hl7.org/fhir/event-status"; 128 case COMPLETED: return "http://hl7.org/fhir/event-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/event-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/event-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case PREPARATION: return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 138 case INPROGRESS: return "The event is currently occurring"; 139 case SUSPENDED: return "The event has been temporarily stopped but is expected to resume in the future"; 140 case ABORTED: return "The event was prior to the full completion of the intended actions"; 141 case COMPLETED: return "The event has now concluded"; 142 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 143 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case PREPARATION: return "Preparation"; 151 case INPROGRESS: return "In Progress"; 152 case SUSPENDED: return "Suspended"; 153 case ABORTED: return "Aborted"; 154 case COMPLETED: return "Completed"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class CommunicationStatusEnumFactory implements EnumFactory<CommunicationStatus> { 164 public CommunicationStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("preparation".equals(codeString)) 169 return CommunicationStatus.PREPARATION; 170 if ("in-progress".equals(codeString)) 171 return CommunicationStatus.INPROGRESS; 172 if ("suspended".equals(codeString)) 173 return CommunicationStatus.SUSPENDED; 174 if ("aborted".equals(codeString)) 175 return CommunicationStatus.ABORTED; 176 if ("completed".equals(codeString)) 177 return CommunicationStatus.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return CommunicationStatus.ENTEREDINERROR; 180 if ("unknown".equals(codeString)) 181 return CommunicationStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown CommunicationStatus code '"+codeString+"'"); 183 } 184 public Enumeration<CommunicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<CommunicationStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("preparation".equals(codeString)) 193 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.PREPARATION); 194 if ("in-progress".equals(codeString)) 195 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.INPROGRESS); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.SUSPENDED); 198 if ("aborted".equals(codeString)) 199 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.ABORTED); 200 if ("completed".equals(codeString)) 201 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.COMPLETED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.ENTEREDINERROR); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.UNKNOWN); 206 throw new FHIRException("Unknown CommunicationStatus code '"+codeString+"'"); 207 } 208 public String toCode(CommunicationStatus code) { 209 if (code == CommunicationStatus.NULL) 210 return null; 211 if (code == CommunicationStatus.PREPARATION) 212 return "preparation"; 213 if (code == CommunicationStatus.INPROGRESS) 214 return "in-progress"; 215 if (code == CommunicationStatus.SUSPENDED) 216 return "suspended"; 217 if (code == CommunicationStatus.ABORTED) 218 return "aborted"; 219 if (code == CommunicationStatus.COMPLETED) 220 return "completed"; 221 if (code == CommunicationStatus.ENTEREDINERROR) 222 return "entered-in-error"; 223 if (code == CommunicationStatus.UNKNOWN) 224 return "unknown"; 225 return "?"; 226 } 227 public String toSystem(CommunicationStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 @Block() 233 public static class CommunicationPayloadComponent extends BackboneElement implements IBaseBackboneElement { 234 /** 235 * A communicated content (or for multi-part communications, one portion of the communication). 236 */ 237 @Child(name = "content", type = {StringType.class, Attachment.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 238 @Description(shortDefinition="Message part content", formalDefinition="A communicated content (or for multi-part communications, one portion of the communication)." ) 239 protected Type content; 240 241 private static final long serialVersionUID = -1763459053L; 242 243 /** 244 * Constructor 245 */ 246 public CommunicationPayloadComponent() { 247 super(); 248 } 249 250 /** 251 * Constructor 252 */ 253 public CommunicationPayloadComponent(Type content) { 254 super(); 255 this.content = content; 256 } 257 258 /** 259 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 260 */ 261 public Type getContent() { 262 return this.content; 263 } 264 265 /** 266 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 267 */ 268 public StringType getContentStringType() throws FHIRException { 269 if (this.content == null) 270 return null; 271 if (!(this.content instanceof StringType)) 272 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.content.getClass().getName()+" was encountered"); 273 return (StringType) this.content; 274 } 275 276 public boolean hasContentStringType() { 277 return this.content instanceof StringType; 278 } 279 280 /** 281 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 282 */ 283 public Attachment getContentAttachment() throws FHIRException { 284 if (this.content == null) 285 return null; 286 if (!(this.content instanceof Attachment)) 287 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 288 return (Attachment) this.content; 289 } 290 291 public boolean hasContentAttachment() { 292 return this.content instanceof Attachment; 293 } 294 295 /** 296 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 297 */ 298 public Reference getContentReference() throws FHIRException { 299 if (this.content == null) 300 return null; 301 if (!(this.content instanceof Reference)) 302 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 303 return (Reference) this.content; 304 } 305 306 public boolean hasContentReference() { 307 return this.content instanceof Reference; 308 } 309 310 public boolean hasContent() { 311 return this.content != null && !this.content.isEmpty(); 312 } 313 314 /** 315 * @param value {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 316 */ 317 public CommunicationPayloadComponent setContent(Type value) throws FHIRFormatError { 318 if (value != null && !(value instanceof StringType || value instanceof Attachment || value instanceof Reference)) 319 throw new FHIRFormatError("Not the right type for Communication.payload.content[x]: "+value.fhirType()); 320 this.content = value; 321 return this; 322 } 323 324 protected void listChildren(List<Property> children) { 325 super.listChildren(children); 326 children.add(new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content)); 327 } 328 329 @Override 330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 331 switch (_hash) { 332 case 264548711: /*content[x]*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 333 case 951530617: /*content*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 334 case -326336022: /*contentString*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 335 case -702028164: /*contentAttachment*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 336 case 1193747154: /*contentReference*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 337 default: return super.getNamedProperty(_hash, _name, _checkValid); 338 } 339 340 } 341 342 @Override 343 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 344 switch (hash) { 345 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Type 346 default: return super.getProperty(hash, name, checkValid); 347 } 348 349 } 350 351 @Override 352 public Base setProperty(int hash, String name, Base value) throws FHIRException { 353 switch (hash) { 354 case 951530617: // content 355 this.content = castToType(value); // Type 356 return value; 357 default: return super.setProperty(hash, name, value); 358 } 359 360 } 361 362 @Override 363 public Base setProperty(String name, Base value) throws FHIRException { 364 if (name.equals("content[x]")) { 365 this.content = castToType(value); // Type 366 } else 367 return super.setProperty(name, value); 368 return value; 369 } 370 371 @Override 372 public Base makeProperty(int hash, String name) throws FHIRException { 373 switch (hash) { 374 case 264548711: return getContent(); 375 case 951530617: return getContent(); 376 default: return super.makeProperty(hash, name); 377 } 378 379 } 380 381 @Override 382 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 383 switch (hash) { 384 case 951530617: /*content*/ return new String[] {"string", "Attachment", "Reference"}; 385 default: return super.getTypesForProperty(hash, name); 386 } 387 388 } 389 390 @Override 391 public Base addChild(String name) throws FHIRException { 392 if (name.equals("contentString")) { 393 this.content = new StringType(); 394 return this.content; 395 } 396 else if (name.equals("contentAttachment")) { 397 this.content = new Attachment(); 398 return this.content; 399 } 400 else if (name.equals("contentReference")) { 401 this.content = new Reference(); 402 return this.content; 403 } 404 else 405 return super.addChild(name); 406 } 407 408 public CommunicationPayloadComponent copy() { 409 CommunicationPayloadComponent dst = new CommunicationPayloadComponent(); 410 copyValues(dst); 411 dst.content = content == null ? null : content.copy(); 412 return dst; 413 } 414 415 @Override 416 public boolean equalsDeep(Base other_) { 417 if (!super.equalsDeep(other_)) 418 return false; 419 if (!(other_ instanceof CommunicationPayloadComponent)) 420 return false; 421 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 422 return compareDeep(content, o.content, true); 423 } 424 425 @Override 426 public boolean equalsShallow(Base other_) { 427 if (!super.equalsShallow(other_)) 428 return false; 429 if (!(other_ instanceof CommunicationPayloadComponent)) 430 return false; 431 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 432 return true; 433 } 434 435 public boolean isEmpty() { 436 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 437 } 438 439 public String fhirType() { 440 return "Communication.payload"; 441 442 } 443 444 } 445 446 /** 447 * Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 448 */ 449 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 450 @Description(shortDefinition="Unique identifier", formalDefinition="Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 451 protected List<Identifier> identifier; 452 453 /** 454 * A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event. 455 */ 456 @Child(name = "definition", type = {PlanDefinition.class, ActivityDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 457 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event." ) 458 protected List<Reference> definition; 459 /** 460 * The actual objects that are the target of the reference (A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.) 461 */ 462 protected List<Resource> definitionTarget; 463 464 465 /** 466 * An order, proposal or plan fulfilled in whole or in part by this Communication. 467 */ 468 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 469 @Description(shortDefinition="Request fulfilled by this communication", formalDefinition="An order, proposal or plan fulfilled in whole or in part by this Communication." ) 470 protected List<Reference> basedOn; 471 /** 472 * The actual objects that are the target of the reference (An order, proposal or plan fulfilled in whole or in part by this Communication.) 473 */ 474 protected List<Resource> basedOnTarget; 475 476 477 /** 478 * Part of this action. 479 */ 480 @Child(name = "partOf", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 481 @Description(shortDefinition="Part of this action", formalDefinition="Part of this action." ) 482 protected List<Reference> partOf; 483 /** 484 * The actual objects that are the target of the reference (Part of this action.) 485 */ 486 protected List<Resource> partOfTarget; 487 488 489 /** 490 * The status of the transmission. 491 */ 492 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 493 @Description(shortDefinition="preparation | in-progress | suspended | aborted | completed | entered-in-error", formalDefinition="The status of the transmission." ) 494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-status") 495 protected Enumeration<CommunicationStatus> status; 496 497 /** 498 * If true, indicates that the described communication event did not actually occur. 499 */ 500 @Child(name = "notDone", type = {BooleanType.class}, order=5, min=0, max=1, modifier=true, summary=true) 501 @Description(shortDefinition="Communication did not occur", formalDefinition="If true, indicates that the described communication event did not actually occur." ) 502 protected BooleanType notDone; 503 504 /** 505 * Describes why the communication event did not occur in coded and/or textual form. 506 */ 507 @Child(name = "notDoneReason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 508 @Description(shortDefinition="Why communication did not occur", formalDefinition="Describes why the communication event did not occur in coded and/or textual form." ) 509 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-not-done-reason") 510 protected CodeableConcept notDoneReason; 511 512 /** 513 * The type of message conveyed such as alert, notification, reminder, instruction, etc. 514 */ 515 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 516 @Description(shortDefinition="Message category", formalDefinition="The type of message conveyed such as alert, notification, reminder, instruction, etc." ) 517 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-category") 518 protected List<CodeableConcept> category; 519 520 /** 521 * A channel that was used for this communication (e.g. email, fax). 522 */ 523 @Child(name = "medium", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 524 @Description(shortDefinition="A channel of communication", formalDefinition="A channel that was used for this communication (e.g. email, fax)." ) 525 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ParticipationMode") 526 protected List<CodeableConcept> medium; 527 528 /** 529 * The patient or group that was the focus of this communication. 530 */ 531 @Child(name = "subject", type = {Patient.class, Group.class}, order=9, min=0, max=1, modifier=false, summary=true) 532 @Description(shortDefinition="Focus of message", formalDefinition="The patient or group that was the focus of this communication." ) 533 protected Reference subject; 534 535 /** 536 * The actual object that is the target of the reference (The patient or group that was the focus of this communication.) 537 */ 538 protected Resource subjectTarget; 539 540 /** 541 * The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time). 542 */ 543 @Child(name = "recipient", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, Group.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 544 @Description(shortDefinition="Message recipient", formalDefinition="The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time)." ) 545 protected List<Reference> recipient; 546 /** 547 * The actual objects that are the target of the reference (The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).) 548 */ 549 protected List<Resource> recipientTarget; 550 551 552 /** 553 * The resources which were responsible for or related to producing this communication. 554 */ 555 @Child(name = "topic", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 556 @Description(shortDefinition="Focal resources", formalDefinition="The resources which were responsible for or related to producing this communication." ) 557 protected List<Reference> topic; 558 /** 559 * The actual objects that are the target of the reference (The resources which were responsible for or related to producing this communication.) 560 */ 561 protected List<Resource> topicTarget; 562 563 564 /** 565 * The encounter within which the communication was sent. 566 */ 567 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=12, min=0, max=1, modifier=false, summary=true) 568 @Description(shortDefinition="Encounter or episode leading to message", formalDefinition="The encounter within which the communication was sent." ) 569 protected Reference context; 570 571 /** 572 * The actual object that is the target of the reference (The encounter within which the communication was sent.) 573 */ 574 protected Resource contextTarget; 575 576 /** 577 * The time when this communication was sent. 578 */ 579 @Child(name = "sent", type = {DateTimeType.class}, order=13, min=0, max=1, modifier=false, summary=false) 580 @Description(shortDefinition="When sent", formalDefinition="The time when this communication was sent." ) 581 protected DateTimeType sent; 582 583 /** 584 * The time when this communication arrived at the destination. 585 */ 586 @Child(name = "received", type = {DateTimeType.class}, order=14, min=0, max=1, modifier=false, summary=false) 587 @Description(shortDefinition="When received", formalDefinition="The time when this communication arrived at the destination." ) 588 protected DateTimeType received; 589 590 /** 591 * The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication. 592 */ 593 @Child(name = "sender", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=15, min=0, max=1, modifier=false, summary=false) 594 @Description(shortDefinition="Message sender", formalDefinition="The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication." ) 595 protected Reference sender; 596 597 /** 598 * The actual object that is the target of the reference (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 599 */ 600 protected Resource senderTarget; 601 602 /** 603 * The reason or justification for the communication. 604 */ 605 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 606 @Description(shortDefinition="Indication for message", formalDefinition="The reason or justification for the communication." ) 607 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 608 protected List<CodeableConcept> reasonCode; 609 610 /** 611 * Indicates another resource whose existence justifies this communication. 612 */ 613 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 614 @Description(shortDefinition="Why was communication done?", formalDefinition="Indicates another resource whose existence justifies this communication." ) 615 protected List<Reference> reasonReference; 616 /** 617 * The actual objects that are the target of the reference (Indicates another resource whose existence justifies this communication.) 618 */ 619 protected List<Resource> reasonReferenceTarget; 620 621 622 /** 623 * Text, attachment(s), or resource(s) that was communicated to the recipient. 624 */ 625 @Child(name = "payload", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 626 @Description(shortDefinition="Message payload", formalDefinition="Text, attachment(s), or resource(s) that was communicated to the recipient." ) 627 protected List<CommunicationPayloadComponent> payload; 628 629 /** 630 * Additional notes or commentary about the communication by the sender, receiver or other interested parties. 631 */ 632 @Child(name = "note", type = {Annotation.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 633 @Description(shortDefinition="Comments made about the communication", formalDefinition="Additional notes or commentary about the communication by the sender, receiver or other interested parties." ) 634 protected List<Annotation> note; 635 636 private static final long serialVersionUID = -1362735590L; 637 638 /** 639 * Constructor 640 */ 641 public Communication() { 642 super(); 643 } 644 645 /** 646 * Constructor 647 */ 648 public Communication(Enumeration<CommunicationStatus> status) { 649 super(); 650 this.status = status; 651 } 652 653 /** 654 * @return {@link #identifier} (Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 655 */ 656 public List<Identifier> getIdentifier() { 657 if (this.identifier == null) 658 this.identifier = new ArrayList<Identifier>(); 659 return this.identifier; 660 } 661 662 /** 663 * @return Returns a reference to <code>this</code> for easy method chaining 664 */ 665 public Communication setIdentifier(List<Identifier> theIdentifier) { 666 this.identifier = theIdentifier; 667 return this; 668 } 669 670 public boolean hasIdentifier() { 671 if (this.identifier == null) 672 return false; 673 for (Identifier item : this.identifier) 674 if (!item.isEmpty()) 675 return true; 676 return false; 677 } 678 679 public Identifier addIdentifier() { //3 680 Identifier t = new Identifier(); 681 if (this.identifier == null) 682 this.identifier = new ArrayList<Identifier>(); 683 this.identifier.add(t); 684 return t; 685 } 686 687 public Communication addIdentifier(Identifier t) { //3 688 if (t == null) 689 return this; 690 if (this.identifier == null) 691 this.identifier = new ArrayList<Identifier>(); 692 this.identifier.add(t); 693 return this; 694 } 695 696 /** 697 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 698 */ 699 public Identifier getIdentifierFirstRep() { 700 if (getIdentifier().isEmpty()) { 701 addIdentifier(); 702 } 703 return getIdentifier().get(0); 704 } 705 706 /** 707 * @return {@link #definition} (A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.) 708 */ 709 public List<Reference> getDefinition() { 710 if (this.definition == null) 711 this.definition = new ArrayList<Reference>(); 712 return this.definition; 713 } 714 715 /** 716 * @return Returns a reference to <code>this</code> for easy method chaining 717 */ 718 public Communication setDefinition(List<Reference> theDefinition) { 719 this.definition = theDefinition; 720 return this; 721 } 722 723 public boolean hasDefinition() { 724 if (this.definition == null) 725 return false; 726 for (Reference item : this.definition) 727 if (!item.isEmpty()) 728 return true; 729 return false; 730 } 731 732 public Reference addDefinition() { //3 733 Reference t = new Reference(); 734 if (this.definition == null) 735 this.definition = new ArrayList<Reference>(); 736 this.definition.add(t); 737 return t; 738 } 739 740 public Communication addDefinition(Reference t) { //3 741 if (t == null) 742 return this; 743 if (this.definition == null) 744 this.definition = new ArrayList<Reference>(); 745 this.definition.add(t); 746 return this; 747 } 748 749 /** 750 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 751 */ 752 public Reference getDefinitionFirstRep() { 753 if (getDefinition().isEmpty()) { 754 addDefinition(); 755 } 756 return getDefinition().get(0); 757 } 758 759 /** 760 * @return {@link #basedOn} (An order, proposal or plan fulfilled in whole or in part by this Communication.) 761 */ 762 public List<Reference> getBasedOn() { 763 if (this.basedOn == null) 764 this.basedOn = new ArrayList<Reference>(); 765 return this.basedOn; 766 } 767 768 /** 769 * @return Returns a reference to <code>this</code> for easy method chaining 770 */ 771 public Communication setBasedOn(List<Reference> theBasedOn) { 772 this.basedOn = theBasedOn; 773 return this; 774 } 775 776 public boolean hasBasedOn() { 777 if (this.basedOn == null) 778 return false; 779 for (Reference item : this.basedOn) 780 if (!item.isEmpty()) 781 return true; 782 return false; 783 } 784 785 public Reference addBasedOn() { //3 786 Reference t = new Reference(); 787 if (this.basedOn == null) 788 this.basedOn = new ArrayList<Reference>(); 789 this.basedOn.add(t); 790 return t; 791 } 792 793 public Communication addBasedOn(Reference t) { //3 794 if (t == null) 795 return this; 796 if (this.basedOn == null) 797 this.basedOn = new ArrayList<Reference>(); 798 this.basedOn.add(t); 799 return this; 800 } 801 802 /** 803 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 804 */ 805 public Reference getBasedOnFirstRep() { 806 if (getBasedOn().isEmpty()) { 807 addBasedOn(); 808 } 809 return getBasedOn().get(0); 810 } 811 812 /** 813 * @return {@link #partOf} (Part of this action.) 814 */ 815 public List<Reference> getPartOf() { 816 if (this.partOf == null) 817 this.partOf = new ArrayList<Reference>(); 818 return this.partOf; 819 } 820 821 /** 822 * @return Returns a reference to <code>this</code> for easy method chaining 823 */ 824 public Communication setPartOf(List<Reference> thePartOf) { 825 this.partOf = thePartOf; 826 return this; 827 } 828 829 public boolean hasPartOf() { 830 if (this.partOf == null) 831 return false; 832 for (Reference item : this.partOf) 833 if (!item.isEmpty()) 834 return true; 835 return false; 836 } 837 838 public Reference addPartOf() { //3 839 Reference t = new Reference(); 840 if (this.partOf == null) 841 this.partOf = new ArrayList<Reference>(); 842 this.partOf.add(t); 843 return t; 844 } 845 846 public Communication addPartOf(Reference t) { //3 847 if (t == null) 848 return this; 849 if (this.partOf == null) 850 this.partOf = new ArrayList<Reference>(); 851 this.partOf.add(t); 852 return this; 853 } 854 855 /** 856 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 857 */ 858 public Reference getPartOfFirstRep() { 859 if (getPartOf().isEmpty()) { 860 addPartOf(); 861 } 862 return getPartOf().get(0); 863 } 864 865 /** 866 * @return {@link #status} (The status of the transmission.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 867 */ 868 public Enumeration<CommunicationStatus> getStatusElement() { 869 if (this.status == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create Communication.status"); 872 else if (Configuration.doAutoCreate()) 873 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); // bb 874 return this.status; 875 } 876 877 public boolean hasStatusElement() { 878 return this.status != null && !this.status.isEmpty(); 879 } 880 881 public boolean hasStatus() { 882 return this.status != null && !this.status.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #status} (The status of the transmission.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 887 */ 888 public Communication setStatusElement(Enumeration<CommunicationStatus> value) { 889 this.status = value; 890 return this; 891 } 892 893 /** 894 * @return The status of the transmission. 895 */ 896 public CommunicationStatus getStatus() { 897 return this.status == null ? null : this.status.getValue(); 898 } 899 900 /** 901 * @param value The status of the transmission. 902 */ 903 public Communication setStatus(CommunicationStatus value) { 904 if (this.status == null) 905 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); 906 this.status.setValue(value); 907 return this; 908 } 909 910 /** 911 * @return {@link #notDone} (If true, indicates that the described communication event did not actually occur.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 912 */ 913 public BooleanType getNotDoneElement() { 914 if (this.notDone == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create Communication.notDone"); 917 else if (Configuration.doAutoCreate()) 918 this.notDone = new BooleanType(); // bb 919 return this.notDone; 920 } 921 922 public boolean hasNotDoneElement() { 923 return this.notDone != null && !this.notDone.isEmpty(); 924 } 925 926 public boolean hasNotDone() { 927 return this.notDone != null && !this.notDone.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #notDone} (If true, indicates that the described communication event did not actually occur.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 932 */ 933 public Communication setNotDoneElement(BooleanType value) { 934 this.notDone = value; 935 return this; 936 } 937 938 /** 939 * @return If true, indicates that the described communication event did not actually occur. 940 */ 941 public boolean getNotDone() { 942 return this.notDone == null || this.notDone.isEmpty() ? false : this.notDone.getValue(); 943 } 944 945 /** 946 * @param value If true, indicates that the described communication event did not actually occur. 947 */ 948 public Communication setNotDone(boolean value) { 949 if (this.notDone == null) 950 this.notDone = new BooleanType(); 951 this.notDone.setValue(value); 952 return this; 953 } 954 955 /** 956 * @return {@link #notDoneReason} (Describes why the communication event did not occur in coded and/or textual form.) 957 */ 958 public CodeableConcept getNotDoneReason() { 959 if (this.notDoneReason == null) 960 if (Configuration.errorOnAutoCreate()) 961 throw new Error("Attempt to auto-create Communication.notDoneReason"); 962 else if (Configuration.doAutoCreate()) 963 this.notDoneReason = new CodeableConcept(); // cc 964 return this.notDoneReason; 965 } 966 967 public boolean hasNotDoneReason() { 968 return this.notDoneReason != null && !this.notDoneReason.isEmpty(); 969 } 970 971 /** 972 * @param value {@link #notDoneReason} (Describes why the communication event did not occur in coded and/or textual form.) 973 */ 974 public Communication setNotDoneReason(CodeableConcept value) { 975 this.notDoneReason = value; 976 return this; 977 } 978 979 /** 980 * @return {@link #category} (The type of message conveyed such as alert, notification, reminder, instruction, etc.) 981 */ 982 public List<CodeableConcept> getCategory() { 983 if (this.category == null) 984 this.category = new ArrayList<CodeableConcept>(); 985 return this.category; 986 } 987 988 /** 989 * @return Returns a reference to <code>this</code> for easy method chaining 990 */ 991 public Communication setCategory(List<CodeableConcept> theCategory) { 992 this.category = theCategory; 993 return this; 994 } 995 996 public boolean hasCategory() { 997 if (this.category == null) 998 return false; 999 for (CodeableConcept item : this.category) 1000 if (!item.isEmpty()) 1001 return true; 1002 return false; 1003 } 1004 1005 public CodeableConcept addCategory() { //3 1006 CodeableConcept t = new CodeableConcept(); 1007 if (this.category == null) 1008 this.category = new ArrayList<CodeableConcept>(); 1009 this.category.add(t); 1010 return t; 1011 } 1012 1013 public Communication addCategory(CodeableConcept t) { //3 1014 if (t == null) 1015 return this; 1016 if (this.category == null) 1017 this.category = new ArrayList<CodeableConcept>(); 1018 this.category.add(t); 1019 return this; 1020 } 1021 1022 /** 1023 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 1024 */ 1025 public CodeableConcept getCategoryFirstRep() { 1026 if (getCategory().isEmpty()) { 1027 addCategory(); 1028 } 1029 return getCategory().get(0); 1030 } 1031 1032 /** 1033 * @return {@link #medium} (A channel that was used for this communication (e.g. email, fax).) 1034 */ 1035 public List<CodeableConcept> getMedium() { 1036 if (this.medium == null) 1037 this.medium = new ArrayList<CodeableConcept>(); 1038 return this.medium; 1039 } 1040 1041 /** 1042 * @return Returns a reference to <code>this</code> for easy method chaining 1043 */ 1044 public Communication setMedium(List<CodeableConcept> theMedium) { 1045 this.medium = theMedium; 1046 return this; 1047 } 1048 1049 public boolean hasMedium() { 1050 if (this.medium == null) 1051 return false; 1052 for (CodeableConcept item : this.medium) 1053 if (!item.isEmpty()) 1054 return true; 1055 return false; 1056 } 1057 1058 public CodeableConcept addMedium() { //3 1059 CodeableConcept t = new CodeableConcept(); 1060 if (this.medium == null) 1061 this.medium = new ArrayList<CodeableConcept>(); 1062 this.medium.add(t); 1063 return t; 1064 } 1065 1066 public Communication addMedium(CodeableConcept t) { //3 1067 if (t == null) 1068 return this; 1069 if (this.medium == null) 1070 this.medium = new ArrayList<CodeableConcept>(); 1071 this.medium.add(t); 1072 return this; 1073 } 1074 1075 /** 1076 * @return The first repetition of repeating field {@link #medium}, creating it if it does not already exist 1077 */ 1078 public CodeableConcept getMediumFirstRep() { 1079 if (getMedium().isEmpty()) { 1080 addMedium(); 1081 } 1082 return getMedium().get(0); 1083 } 1084 1085 /** 1086 * @return {@link #subject} (The patient or group that was the focus of this communication.) 1087 */ 1088 public Reference getSubject() { 1089 if (this.subject == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create Communication.subject"); 1092 else if (Configuration.doAutoCreate()) 1093 this.subject = new Reference(); // cc 1094 return this.subject; 1095 } 1096 1097 public boolean hasSubject() { 1098 return this.subject != null && !this.subject.isEmpty(); 1099 } 1100 1101 /** 1102 * @param value {@link #subject} (The patient or group that was the focus of this communication.) 1103 */ 1104 public Communication setSubject(Reference value) { 1105 this.subject = value; 1106 return this; 1107 } 1108 1109 /** 1110 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient or group that was the focus of this communication.) 1111 */ 1112 public Resource getSubjectTarget() { 1113 return this.subjectTarget; 1114 } 1115 1116 /** 1117 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient or group that was the focus of this communication.) 1118 */ 1119 public Communication setSubjectTarget(Resource value) { 1120 this.subjectTarget = value; 1121 return this; 1122 } 1123 1124 /** 1125 * @return {@link #recipient} (The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).) 1126 */ 1127 public List<Reference> getRecipient() { 1128 if (this.recipient == null) 1129 this.recipient = new ArrayList<Reference>(); 1130 return this.recipient; 1131 } 1132 1133 /** 1134 * @return Returns a reference to <code>this</code> for easy method chaining 1135 */ 1136 public Communication setRecipient(List<Reference> theRecipient) { 1137 this.recipient = theRecipient; 1138 return this; 1139 } 1140 1141 public boolean hasRecipient() { 1142 if (this.recipient == null) 1143 return false; 1144 for (Reference item : this.recipient) 1145 if (!item.isEmpty()) 1146 return true; 1147 return false; 1148 } 1149 1150 public Reference addRecipient() { //3 1151 Reference t = new Reference(); 1152 if (this.recipient == null) 1153 this.recipient = new ArrayList<Reference>(); 1154 this.recipient.add(t); 1155 return t; 1156 } 1157 1158 public Communication addRecipient(Reference t) { //3 1159 if (t == null) 1160 return this; 1161 if (this.recipient == null) 1162 this.recipient = new ArrayList<Reference>(); 1163 this.recipient.add(t); 1164 return this; 1165 } 1166 1167 /** 1168 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist 1169 */ 1170 public Reference getRecipientFirstRep() { 1171 if (getRecipient().isEmpty()) { 1172 addRecipient(); 1173 } 1174 return getRecipient().get(0); 1175 } 1176 1177 /** 1178 * @return {@link #topic} (The resources which were responsible for or related to producing this communication.) 1179 */ 1180 public List<Reference> getTopic() { 1181 if (this.topic == null) 1182 this.topic = new ArrayList<Reference>(); 1183 return this.topic; 1184 } 1185 1186 /** 1187 * @return Returns a reference to <code>this</code> for easy method chaining 1188 */ 1189 public Communication setTopic(List<Reference> theTopic) { 1190 this.topic = theTopic; 1191 return this; 1192 } 1193 1194 public boolean hasTopic() { 1195 if (this.topic == null) 1196 return false; 1197 for (Reference item : this.topic) 1198 if (!item.isEmpty()) 1199 return true; 1200 return false; 1201 } 1202 1203 public Reference addTopic() { //3 1204 Reference t = new Reference(); 1205 if (this.topic == null) 1206 this.topic = new ArrayList<Reference>(); 1207 this.topic.add(t); 1208 return t; 1209 } 1210 1211 public Communication addTopic(Reference t) { //3 1212 if (t == null) 1213 return this; 1214 if (this.topic == null) 1215 this.topic = new ArrayList<Reference>(); 1216 this.topic.add(t); 1217 return this; 1218 } 1219 1220 /** 1221 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 1222 */ 1223 public Reference getTopicFirstRep() { 1224 if (getTopic().isEmpty()) { 1225 addTopic(); 1226 } 1227 return getTopic().get(0); 1228 } 1229 1230 /** 1231 * @return {@link #context} (The encounter within which the communication was sent.) 1232 */ 1233 public Reference getContext() { 1234 if (this.context == null) 1235 if (Configuration.errorOnAutoCreate()) 1236 throw new Error("Attempt to auto-create Communication.context"); 1237 else if (Configuration.doAutoCreate()) 1238 this.context = new Reference(); // cc 1239 return this.context; 1240 } 1241 1242 public boolean hasContext() { 1243 return this.context != null && !this.context.isEmpty(); 1244 } 1245 1246 /** 1247 * @param value {@link #context} (The encounter within which the communication was sent.) 1248 */ 1249 public Communication setContext(Reference value) { 1250 this.context = value; 1251 return this; 1252 } 1253 1254 /** 1255 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter within which the communication was sent.) 1256 */ 1257 public Resource getContextTarget() { 1258 return this.contextTarget; 1259 } 1260 1261 /** 1262 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter within which the communication was sent.) 1263 */ 1264 public Communication setContextTarget(Resource value) { 1265 this.contextTarget = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return {@link #sent} (The time when this communication was sent.). This is the underlying object with id, value and extensions. The accessor "getSent" gives direct access to the value 1271 */ 1272 public DateTimeType getSentElement() { 1273 if (this.sent == null) 1274 if (Configuration.errorOnAutoCreate()) 1275 throw new Error("Attempt to auto-create Communication.sent"); 1276 else if (Configuration.doAutoCreate()) 1277 this.sent = new DateTimeType(); // bb 1278 return this.sent; 1279 } 1280 1281 public boolean hasSentElement() { 1282 return this.sent != null && !this.sent.isEmpty(); 1283 } 1284 1285 public boolean hasSent() { 1286 return this.sent != null && !this.sent.isEmpty(); 1287 } 1288 1289 /** 1290 * @param value {@link #sent} (The time when this communication was sent.). This is the underlying object with id, value and extensions. The accessor "getSent" gives direct access to the value 1291 */ 1292 public Communication setSentElement(DateTimeType value) { 1293 this.sent = value; 1294 return this; 1295 } 1296 1297 /** 1298 * @return The time when this communication was sent. 1299 */ 1300 public Date getSent() { 1301 return this.sent == null ? null : this.sent.getValue(); 1302 } 1303 1304 /** 1305 * @param value The time when this communication was sent. 1306 */ 1307 public Communication setSent(Date value) { 1308 if (value == null) 1309 this.sent = null; 1310 else { 1311 if (this.sent == null) 1312 this.sent = new DateTimeType(); 1313 this.sent.setValue(value); 1314 } 1315 return this; 1316 } 1317 1318 /** 1319 * @return {@link #received} (The time when this communication arrived at the destination.). This is the underlying object with id, value and extensions. The accessor "getReceived" gives direct access to the value 1320 */ 1321 public DateTimeType getReceivedElement() { 1322 if (this.received == null) 1323 if (Configuration.errorOnAutoCreate()) 1324 throw new Error("Attempt to auto-create Communication.received"); 1325 else if (Configuration.doAutoCreate()) 1326 this.received = new DateTimeType(); // bb 1327 return this.received; 1328 } 1329 1330 public boolean hasReceivedElement() { 1331 return this.received != null && !this.received.isEmpty(); 1332 } 1333 1334 public boolean hasReceived() { 1335 return this.received != null && !this.received.isEmpty(); 1336 } 1337 1338 /** 1339 * @param value {@link #received} (The time when this communication arrived at the destination.). This is the underlying object with id, value and extensions. The accessor "getReceived" gives direct access to the value 1340 */ 1341 public Communication setReceivedElement(DateTimeType value) { 1342 this.received = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return The time when this communication arrived at the destination. 1348 */ 1349 public Date getReceived() { 1350 return this.received == null ? null : this.received.getValue(); 1351 } 1352 1353 /** 1354 * @param value The time when this communication arrived at the destination. 1355 */ 1356 public Communication setReceived(Date value) { 1357 if (value == null) 1358 this.received = null; 1359 else { 1360 if (this.received == null) 1361 this.received = new DateTimeType(); 1362 this.received.setValue(value); 1363 } 1364 return this; 1365 } 1366 1367 /** 1368 * @return {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1369 */ 1370 public Reference getSender() { 1371 if (this.sender == null) 1372 if (Configuration.errorOnAutoCreate()) 1373 throw new Error("Attempt to auto-create Communication.sender"); 1374 else if (Configuration.doAutoCreate()) 1375 this.sender = new Reference(); // cc 1376 return this.sender; 1377 } 1378 1379 public boolean hasSender() { 1380 return this.sender != null && !this.sender.isEmpty(); 1381 } 1382 1383 /** 1384 * @param value {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1385 */ 1386 public Communication setSender(Reference value) { 1387 this.sender = value; 1388 return this; 1389 } 1390 1391 /** 1392 * @return {@link #sender} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1393 */ 1394 public Resource getSenderTarget() { 1395 return this.senderTarget; 1396 } 1397 1398 /** 1399 * @param value {@link #sender} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1400 */ 1401 public Communication setSenderTarget(Resource value) { 1402 this.senderTarget = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #reasonCode} (The reason or justification for the communication.) 1408 */ 1409 public List<CodeableConcept> getReasonCode() { 1410 if (this.reasonCode == null) 1411 this.reasonCode = new ArrayList<CodeableConcept>(); 1412 return this.reasonCode; 1413 } 1414 1415 /** 1416 * @return Returns a reference to <code>this</code> for easy method chaining 1417 */ 1418 public Communication setReasonCode(List<CodeableConcept> theReasonCode) { 1419 this.reasonCode = theReasonCode; 1420 return this; 1421 } 1422 1423 public boolean hasReasonCode() { 1424 if (this.reasonCode == null) 1425 return false; 1426 for (CodeableConcept item : this.reasonCode) 1427 if (!item.isEmpty()) 1428 return true; 1429 return false; 1430 } 1431 1432 public CodeableConcept addReasonCode() { //3 1433 CodeableConcept t = new CodeableConcept(); 1434 if (this.reasonCode == null) 1435 this.reasonCode = new ArrayList<CodeableConcept>(); 1436 this.reasonCode.add(t); 1437 return t; 1438 } 1439 1440 public Communication addReasonCode(CodeableConcept t) { //3 1441 if (t == null) 1442 return this; 1443 if (this.reasonCode == null) 1444 this.reasonCode = new ArrayList<CodeableConcept>(); 1445 this.reasonCode.add(t); 1446 return this; 1447 } 1448 1449 /** 1450 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1451 */ 1452 public CodeableConcept getReasonCodeFirstRep() { 1453 if (getReasonCode().isEmpty()) { 1454 addReasonCode(); 1455 } 1456 return getReasonCode().get(0); 1457 } 1458 1459 /** 1460 * @return {@link #reasonReference} (Indicates another resource whose existence justifies this communication.) 1461 */ 1462 public List<Reference> getReasonReference() { 1463 if (this.reasonReference == null) 1464 this.reasonReference = new ArrayList<Reference>(); 1465 return this.reasonReference; 1466 } 1467 1468 /** 1469 * @return Returns a reference to <code>this</code> for easy method chaining 1470 */ 1471 public Communication setReasonReference(List<Reference> theReasonReference) { 1472 this.reasonReference = theReasonReference; 1473 return this; 1474 } 1475 1476 public boolean hasReasonReference() { 1477 if (this.reasonReference == null) 1478 return false; 1479 for (Reference item : this.reasonReference) 1480 if (!item.isEmpty()) 1481 return true; 1482 return false; 1483 } 1484 1485 public Reference addReasonReference() { //3 1486 Reference t = new Reference(); 1487 if (this.reasonReference == null) 1488 this.reasonReference = new ArrayList<Reference>(); 1489 this.reasonReference.add(t); 1490 return t; 1491 } 1492 1493 public Communication addReasonReference(Reference t) { //3 1494 if (t == null) 1495 return this; 1496 if (this.reasonReference == null) 1497 this.reasonReference = new ArrayList<Reference>(); 1498 this.reasonReference.add(t); 1499 return this; 1500 } 1501 1502 /** 1503 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1504 */ 1505 public Reference getReasonReferenceFirstRep() { 1506 if (getReasonReference().isEmpty()) { 1507 addReasonReference(); 1508 } 1509 return getReasonReference().get(0); 1510 } 1511 1512 /** 1513 * @return {@link #payload} (Text, attachment(s), or resource(s) that was communicated to the recipient.) 1514 */ 1515 public List<CommunicationPayloadComponent> getPayload() { 1516 if (this.payload == null) 1517 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1518 return this.payload; 1519 } 1520 1521 /** 1522 * @return Returns a reference to <code>this</code> for easy method chaining 1523 */ 1524 public Communication setPayload(List<CommunicationPayloadComponent> thePayload) { 1525 this.payload = thePayload; 1526 return this; 1527 } 1528 1529 public boolean hasPayload() { 1530 if (this.payload == null) 1531 return false; 1532 for (CommunicationPayloadComponent item : this.payload) 1533 if (!item.isEmpty()) 1534 return true; 1535 return false; 1536 } 1537 1538 public CommunicationPayloadComponent addPayload() { //3 1539 CommunicationPayloadComponent t = new CommunicationPayloadComponent(); 1540 if (this.payload == null) 1541 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1542 this.payload.add(t); 1543 return t; 1544 } 1545 1546 public Communication addPayload(CommunicationPayloadComponent t) { //3 1547 if (t == null) 1548 return this; 1549 if (this.payload == null) 1550 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1551 this.payload.add(t); 1552 return this; 1553 } 1554 1555 /** 1556 * @return The first repetition of repeating field {@link #payload}, creating it if it does not already exist 1557 */ 1558 public CommunicationPayloadComponent getPayloadFirstRep() { 1559 if (getPayload().isEmpty()) { 1560 addPayload(); 1561 } 1562 return getPayload().get(0); 1563 } 1564 1565 /** 1566 * @return {@link #note} (Additional notes or commentary about the communication by the sender, receiver or other interested parties.) 1567 */ 1568 public List<Annotation> getNote() { 1569 if (this.note == null) 1570 this.note = new ArrayList<Annotation>(); 1571 return this.note; 1572 } 1573 1574 /** 1575 * @return Returns a reference to <code>this</code> for easy method chaining 1576 */ 1577 public Communication setNote(List<Annotation> theNote) { 1578 this.note = theNote; 1579 return this; 1580 } 1581 1582 public boolean hasNote() { 1583 if (this.note == null) 1584 return false; 1585 for (Annotation item : this.note) 1586 if (!item.isEmpty()) 1587 return true; 1588 return false; 1589 } 1590 1591 public Annotation addNote() { //3 1592 Annotation t = new Annotation(); 1593 if (this.note == null) 1594 this.note = new ArrayList<Annotation>(); 1595 this.note.add(t); 1596 return t; 1597 } 1598 1599 public Communication addNote(Annotation t) { //3 1600 if (t == null) 1601 return this; 1602 if (this.note == null) 1603 this.note = new ArrayList<Annotation>(); 1604 this.note.add(t); 1605 return this; 1606 } 1607 1608 /** 1609 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1610 */ 1611 public Annotation getNoteFirstRep() { 1612 if (getNote().isEmpty()) { 1613 addNote(); 1614 } 1615 return getNote().get(0); 1616 } 1617 1618 protected void listChildren(List<Property> children) { 1619 super.listChildren(children); 1620 children.add(new Property("identifier", "Identifier", "Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 1621 children.add(new Property("definition", "Reference(PlanDefinition|ActivityDefinition)", "A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.", 0, java.lang.Integer.MAX_VALUE, definition)); 1622 children.add(new Property("basedOn", "Reference(Any)", "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1623 children.add(new Property("partOf", "Reference(Any)", "Part of this action.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1624 children.add(new Property("status", "code", "The status of the transmission.", 0, 1, status)); 1625 children.add(new Property("notDone", "boolean", "If true, indicates that the described communication event did not actually occur.", 0, 1, notDone)); 1626 children.add(new Property("notDoneReason", "CodeableConcept", "Describes why the communication event did not occur in coded and/or textual form.", 0, 1, notDoneReason)); 1627 children.add(new Property("category", "CodeableConcept", "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 1628 children.add(new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 1629 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group that was the focus of this communication.", 0, 1, subject)); 1630 children.add(new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson|Group)", "The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).", 0, java.lang.Integer.MAX_VALUE, recipient)); 1631 children.add(new Property("topic", "Reference(Any)", "The resources which were responsible for or related to producing this communication.", 0, java.lang.Integer.MAX_VALUE, topic)); 1632 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter within which the communication was sent.", 0, 1, context)); 1633 children.add(new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent)); 1634 children.add(new Property("received", "dateTime", "The time when this communication arrived at the destination.", 0, 1, received)); 1635 children.add(new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.", 0, 1, sender)); 1636 children.add(new Property("reasonCode", "CodeableConcept", "The reason or justification for the communication.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1637 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this communication.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1638 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload)); 1639 children.add(new Property("note", "Annotation", "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 0, java.lang.Integer.MAX_VALUE, note)); 1640 } 1641 1642 @Override 1643 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1644 switch (_hash) { 1645 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 1646 case -1014418093: /*definition*/ return new Property("definition", "Reference(PlanDefinition|ActivityDefinition)", "A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.", 0, java.lang.Integer.MAX_VALUE, definition); 1647 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1648 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Any)", "Part of this action.", 0, java.lang.Integer.MAX_VALUE, partOf); 1649 case -892481550: /*status*/ return new Property("status", "code", "The status of the transmission.", 0, 1, status); 1650 case 2128257269: /*notDone*/ return new Property("notDone", "boolean", "If true, indicates that the described communication event did not actually occur.", 0, 1, notDone); 1651 case -1973169255: /*notDoneReason*/ return new Property("notDoneReason", "CodeableConcept", "Describes why the communication event did not occur in coded and/or textual form.", 0, 1, notDoneReason); 1652 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category); 1653 case -1078030475: /*medium*/ return new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 1654 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group that was the focus of this communication.", 0, 1, subject); 1655 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson|Group)", "The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).", 0, java.lang.Integer.MAX_VALUE, recipient); 1656 case 110546223: /*topic*/ return new Property("topic", "Reference(Any)", "The resources which were responsible for or related to producing this communication.", 0, java.lang.Integer.MAX_VALUE, topic); 1657 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter within which the communication was sent.", 0, 1, context); 1658 case 3526552: /*sent*/ return new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent); 1659 case -808719903: /*received*/ return new Property("received", "dateTime", "The time when this communication arrived at the destination.", 0, 1, received); 1660 case -905962955: /*sender*/ return new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.", 0, 1, sender); 1661 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "The reason or justification for the communication.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1662 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this communication.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1663 case -786701938: /*payload*/ return new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload); 1664 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 0, java.lang.Integer.MAX_VALUE, note); 1665 default: return super.getNamedProperty(_hash, _name, _checkValid); 1666 } 1667 1668 } 1669 1670 @Override 1671 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1672 switch (hash) { 1673 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1674 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 1675 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1676 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1677 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CommunicationStatus> 1678 case 2128257269: /*notDone*/ return this.notDone == null ? new Base[0] : new Base[] {this.notDone}; // BooleanType 1679 case -1973169255: /*notDoneReason*/ return this.notDoneReason == null ? new Base[0] : new Base[] {this.notDoneReason}; // CodeableConcept 1680 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1681 case -1078030475: /*medium*/ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 1682 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1683 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1684 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // Reference 1685 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1686 case 3526552: /*sent*/ return this.sent == null ? new Base[0] : new Base[] {this.sent}; // DateTimeType 1687 case -808719903: /*received*/ return this.received == null ? new Base[0] : new Base[] {this.received}; // DateTimeType 1688 case -905962955: /*sender*/ return this.sender == null ? new Base[0] : new Base[] {this.sender}; // Reference 1689 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1690 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1691 case -786701938: /*payload*/ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationPayloadComponent 1692 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1693 default: return super.getProperty(hash, name, checkValid); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1700 switch (hash) { 1701 case -1618432855: // identifier 1702 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1703 return value; 1704 case -1014418093: // definition 1705 this.getDefinition().add(castToReference(value)); // Reference 1706 return value; 1707 case -332612366: // basedOn 1708 this.getBasedOn().add(castToReference(value)); // Reference 1709 return value; 1710 case -995410646: // partOf 1711 this.getPartOf().add(castToReference(value)); // Reference 1712 return value; 1713 case -892481550: // status 1714 value = new CommunicationStatusEnumFactory().fromType(castToCode(value)); 1715 this.status = (Enumeration) value; // Enumeration<CommunicationStatus> 1716 return value; 1717 case 2128257269: // notDone 1718 this.notDone = castToBoolean(value); // BooleanType 1719 return value; 1720 case -1973169255: // notDoneReason 1721 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 1722 return value; 1723 case 50511102: // category 1724 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1725 return value; 1726 case -1078030475: // medium 1727 this.getMedium().add(castToCodeableConcept(value)); // CodeableConcept 1728 return value; 1729 case -1867885268: // subject 1730 this.subject = castToReference(value); // Reference 1731 return value; 1732 case 820081177: // recipient 1733 this.getRecipient().add(castToReference(value)); // Reference 1734 return value; 1735 case 110546223: // topic 1736 this.getTopic().add(castToReference(value)); // Reference 1737 return value; 1738 case 951530927: // context 1739 this.context = castToReference(value); // Reference 1740 return value; 1741 case 3526552: // sent 1742 this.sent = castToDateTime(value); // DateTimeType 1743 return value; 1744 case -808719903: // received 1745 this.received = castToDateTime(value); // DateTimeType 1746 return value; 1747 case -905962955: // sender 1748 this.sender = castToReference(value); // Reference 1749 return value; 1750 case 722137681: // reasonCode 1751 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1752 return value; 1753 case -1146218137: // reasonReference 1754 this.getReasonReference().add(castToReference(value)); // Reference 1755 return value; 1756 case -786701938: // payload 1757 this.getPayload().add((CommunicationPayloadComponent) value); // CommunicationPayloadComponent 1758 return value; 1759 case 3387378: // note 1760 this.getNote().add(castToAnnotation(value)); // Annotation 1761 return value; 1762 default: return super.setProperty(hash, name, value); 1763 } 1764 1765 } 1766 1767 @Override 1768 public Base setProperty(String name, Base value) throws FHIRException { 1769 if (name.equals("identifier")) { 1770 this.getIdentifier().add(castToIdentifier(value)); 1771 } else if (name.equals("definition")) { 1772 this.getDefinition().add(castToReference(value)); 1773 } else if (name.equals("basedOn")) { 1774 this.getBasedOn().add(castToReference(value)); 1775 } else if (name.equals("partOf")) { 1776 this.getPartOf().add(castToReference(value)); 1777 } else if (name.equals("status")) { 1778 value = new CommunicationStatusEnumFactory().fromType(castToCode(value)); 1779 this.status = (Enumeration) value; // Enumeration<CommunicationStatus> 1780 } else if (name.equals("notDone")) { 1781 this.notDone = castToBoolean(value); // BooleanType 1782 } else if (name.equals("notDoneReason")) { 1783 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 1784 } else if (name.equals("category")) { 1785 this.getCategory().add(castToCodeableConcept(value)); 1786 } else if (name.equals("medium")) { 1787 this.getMedium().add(castToCodeableConcept(value)); 1788 } else if (name.equals("subject")) { 1789 this.subject = castToReference(value); // Reference 1790 } else if (name.equals("recipient")) { 1791 this.getRecipient().add(castToReference(value)); 1792 } else if (name.equals("topic")) { 1793 this.getTopic().add(castToReference(value)); 1794 } else if (name.equals("context")) { 1795 this.context = castToReference(value); // Reference 1796 } else if (name.equals("sent")) { 1797 this.sent = castToDateTime(value); // DateTimeType 1798 } else if (name.equals("received")) { 1799 this.received = castToDateTime(value); // DateTimeType 1800 } else if (name.equals("sender")) { 1801 this.sender = castToReference(value); // Reference 1802 } else if (name.equals("reasonCode")) { 1803 this.getReasonCode().add(castToCodeableConcept(value)); 1804 } else if (name.equals("reasonReference")) { 1805 this.getReasonReference().add(castToReference(value)); 1806 } else if (name.equals("payload")) { 1807 this.getPayload().add((CommunicationPayloadComponent) value); 1808 } else if (name.equals("note")) { 1809 this.getNote().add(castToAnnotation(value)); 1810 } else 1811 return super.setProperty(name, value); 1812 return value; 1813 } 1814 1815 @Override 1816 public Base makeProperty(int hash, String name) throws FHIRException { 1817 switch (hash) { 1818 case -1618432855: return addIdentifier(); 1819 case -1014418093: return addDefinition(); 1820 case -332612366: return addBasedOn(); 1821 case -995410646: return addPartOf(); 1822 case -892481550: return getStatusElement(); 1823 case 2128257269: return getNotDoneElement(); 1824 case -1973169255: return getNotDoneReason(); 1825 case 50511102: return addCategory(); 1826 case -1078030475: return addMedium(); 1827 case -1867885268: return getSubject(); 1828 case 820081177: return addRecipient(); 1829 case 110546223: return addTopic(); 1830 case 951530927: return getContext(); 1831 case 3526552: return getSentElement(); 1832 case -808719903: return getReceivedElement(); 1833 case -905962955: return getSender(); 1834 case 722137681: return addReasonCode(); 1835 case -1146218137: return addReasonReference(); 1836 case -786701938: return addPayload(); 1837 case 3387378: return addNote(); 1838 default: return super.makeProperty(hash, name); 1839 } 1840 1841 } 1842 1843 @Override 1844 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1845 switch (hash) { 1846 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1847 case -1014418093: /*definition*/ return new String[] {"Reference"}; 1848 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1849 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1850 case -892481550: /*status*/ return new String[] {"code"}; 1851 case 2128257269: /*notDone*/ return new String[] {"boolean"}; 1852 case -1973169255: /*notDoneReason*/ return new String[] {"CodeableConcept"}; 1853 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1854 case -1078030475: /*medium*/ return new String[] {"CodeableConcept"}; 1855 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1856 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1857 case 110546223: /*topic*/ return new String[] {"Reference"}; 1858 case 951530927: /*context*/ return new String[] {"Reference"}; 1859 case 3526552: /*sent*/ return new String[] {"dateTime"}; 1860 case -808719903: /*received*/ return new String[] {"dateTime"}; 1861 case -905962955: /*sender*/ return new String[] {"Reference"}; 1862 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1863 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 1864 case -786701938: /*payload*/ return new String[] {}; 1865 case 3387378: /*note*/ return new String[] {"Annotation"}; 1866 default: return super.getTypesForProperty(hash, name); 1867 } 1868 1869 } 1870 1871 @Override 1872 public Base addChild(String name) throws FHIRException { 1873 if (name.equals("identifier")) { 1874 return addIdentifier(); 1875 } 1876 else if (name.equals("definition")) { 1877 return addDefinition(); 1878 } 1879 else if (name.equals("basedOn")) { 1880 return addBasedOn(); 1881 } 1882 else if (name.equals("partOf")) { 1883 return addPartOf(); 1884 } 1885 else if (name.equals("status")) { 1886 throw new FHIRException("Cannot call addChild on a singleton property Communication.status"); 1887 } 1888 else if (name.equals("notDone")) { 1889 throw new FHIRException("Cannot call addChild on a singleton property Communication.notDone"); 1890 } 1891 else if (name.equals("notDoneReason")) { 1892 this.notDoneReason = new CodeableConcept(); 1893 return this.notDoneReason; 1894 } 1895 else if (name.equals("category")) { 1896 return addCategory(); 1897 } 1898 else if (name.equals("medium")) { 1899 return addMedium(); 1900 } 1901 else if (name.equals("subject")) { 1902 this.subject = new Reference(); 1903 return this.subject; 1904 } 1905 else if (name.equals("recipient")) { 1906 return addRecipient(); 1907 } 1908 else if (name.equals("topic")) { 1909 return addTopic(); 1910 } 1911 else if (name.equals("context")) { 1912 this.context = new Reference(); 1913 return this.context; 1914 } 1915 else if (name.equals("sent")) { 1916 throw new FHIRException("Cannot call addChild on a singleton property Communication.sent"); 1917 } 1918 else if (name.equals("received")) { 1919 throw new FHIRException("Cannot call addChild on a singleton property Communication.received"); 1920 } 1921 else if (name.equals("sender")) { 1922 this.sender = new Reference(); 1923 return this.sender; 1924 } 1925 else if (name.equals("reasonCode")) { 1926 return addReasonCode(); 1927 } 1928 else if (name.equals("reasonReference")) { 1929 return addReasonReference(); 1930 } 1931 else if (name.equals("payload")) { 1932 return addPayload(); 1933 } 1934 else if (name.equals("note")) { 1935 return addNote(); 1936 } 1937 else 1938 return super.addChild(name); 1939 } 1940 1941 public String fhirType() { 1942 return "Communication"; 1943 1944 } 1945 1946 public Communication copy() { 1947 Communication dst = new Communication(); 1948 copyValues(dst); 1949 if (identifier != null) { 1950 dst.identifier = new ArrayList<Identifier>(); 1951 for (Identifier i : identifier) 1952 dst.identifier.add(i.copy()); 1953 }; 1954 if (definition != null) { 1955 dst.definition = new ArrayList<Reference>(); 1956 for (Reference i : definition) 1957 dst.definition.add(i.copy()); 1958 }; 1959 if (basedOn != null) { 1960 dst.basedOn = new ArrayList<Reference>(); 1961 for (Reference i : basedOn) 1962 dst.basedOn.add(i.copy()); 1963 }; 1964 if (partOf != null) { 1965 dst.partOf = new ArrayList<Reference>(); 1966 for (Reference i : partOf) 1967 dst.partOf.add(i.copy()); 1968 }; 1969 dst.status = status == null ? null : status.copy(); 1970 dst.notDone = notDone == null ? null : notDone.copy(); 1971 dst.notDoneReason = notDoneReason == null ? null : notDoneReason.copy(); 1972 if (category != null) { 1973 dst.category = new ArrayList<CodeableConcept>(); 1974 for (CodeableConcept i : category) 1975 dst.category.add(i.copy()); 1976 }; 1977 if (medium != null) { 1978 dst.medium = new ArrayList<CodeableConcept>(); 1979 for (CodeableConcept i : medium) 1980 dst.medium.add(i.copy()); 1981 }; 1982 dst.subject = subject == null ? null : subject.copy(); 1983 if (recipient != null) { 1984 dst.recipient = new ArrayList<Reference>(); 1985 for (Reference i : recipient) 1986 dst.recipient.add(i.copy()); 1987 }; 1988 if (topic != null) { 1989 dst.topic = new ArrayList<Reference>(); 1990 for (Reference i : topic) 1991 dst.topic.add(i.copy()); 1992 }; 1993 dst.context = context == null ? null : context.copy(); 1994 dst.sent = sent == null ? null : sent.copy(); 1995 dst.received = received == null ? null : received.copy(); 1996 dst.sender = sender == null ? null : sender.copy(); 1997 if (reasonCode != null) { 1998 dst.reasonCode = new ArrayList<CodeableConcept>(); 1999 for (CodeableConcept i : reasonCode) 2000 dst.reasonCode.add(i.copy()); 2001 }; 2002 if (reasonReference != null) { 2003 dst.reasonReference = new ArrayList<Reference>(); 2004 for (Reference i : reasonReference) 2005 dst.reasonReference.add(i.copy()); 2006 }; 2007 if (payload != null) { 2008 dst.payload = new ArrayList<CommunicationPayloadComponent>(); 2009 for (CommunicationPayloadComponent i : payload) 2010 dst.payload.add(i.copy()); 2011 }; 2012 if (note != null) { 2013 dst.note = new ArrayList<Annotation>(); 2014 for (Annotation i : note) 2015 dst.note.add(i.copy()); 2016 }; 2017 return dst; 2018 } 2019 2020 protected Communication typedCopy() { 2021 return copy(); 2022 } 2023 2024 @Override 2025 public boolean equalsDeep(Base other_) { 2026 if (!super.equalsDeep(other_)) 2027 return false; 2028 if (!(other_ instanceof Communication)) 2029 return false; 2030 Communication o = (Communication) other_; 2031 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2032 && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 2033 && compareDeep(notDone, o.notDone, true) && compareDeep(notDoneReason, o.notDoneReason, true) && compareDeep(category, o.category, true) 2034 && compareDeep(medium, o.medium, true) && compareDeep(subject, o.subject, true) && compareDeep(recipient, o.recipient, true) 2035 && compareDeep(topic, o.topic, true) && compareDeep(context, o.context, true) && compareDeep(sent, o.sent, true) 2036 && compareDeep(received, o.received, true) && compareDeep(sender, o.sender, true) && compareDeep(reasonCode, o.reasonCode, true) 2037 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(payload, o.payload, true) 2038 && compareDeep(note, o.note, true); 2039 } 2040 2041 @Override 2042 public boolean equalsShallow(Base other_) { 2043 if (!super.equalsShallow(other_)) 2044 return false; 2045 if (!(other_ instanceof Communication)) 2046 return false; 2047 Communication o = (Communication) other_; 2048 return compareValues(status, o.status, true) && compareValues(notDone, o.notDone, true) && compareValues(sent, o.sent, true) 2049 && compareValues(received, o.received, true); 2050 } 2051 2052 public boolean isEmpty() { 2053 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2054 , partOf, status, notDone, notDoneReason, category, medium, subject, recipient 2055 , topic, context, sent, received, sender, reasonCode, reasonReference, payload 2056 , note); 2057 } 2058 2059 @Override 2060 public ResourceType getResourceType() { 2061 return ResourceType.Communication; 2062 } 2063 2064 /** 2065 * Search parameter: <b>identifier</b> 2066 * <p> 2067 * Description: <b>Unique identifier</b><br> 2068 * Type: <b>token</b><br> 2069 * Path: <b>Communication.identifier</b><br> 2070 * </p> 2071 */ 2072 @SearchParamDefinition(name="identifier", path="Communication.identifier", description="Unique identifier", type="token" ) 2073 public static final String SP_IDENTIFIER = "identifier"; 2074 /** 2075 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2076 * <p> 2077 * Description: <b>Unique identifier</b><br> 2078 * Type: <b>token</b><br> 2079 * Path: <b>Communication.identifier</b><br> 2080 * </p> 2081 */ 2082 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2083 2084 /** 2085 * Search parameter: <b>subject</b> 2086 * <p> 2087 * Description: <b>Focus of message</b><br> 2088 * Type: <b>reference</b><br> 2089 * Path: <b>Communication.subject</b><br> 2090 * </p> 2091 */ 2092 @SearchParamDefinition(name="subject", path="Communication.subject", description="Focus of message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2093 public static final String SP_SUBJECT = "subject"; 2094 /** 2095 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2096 * <p> 2097 * Description: <b>Focus of message</b><br> 2098 * Type: <b>reference</b><br> 2099 * Path: <b>Communication.subject</b><br> 2100 * </p> 2101 */ 2102 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2103 2104/** 2105 * Constant for fluent queries to be used to add include statements. Specifies 2106 * the path value of "<b>Communication:subject</b>". 2107 */ 2108 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Communication:subject").toLocked(); 2109 2110 /** 2111 * Search parameter: <b>received</b> 2112 * <p> 2113 * Description: <b>When received</b><br> 2114 * Type: <b>date</b><br> 2115 * Path: <b>Communication.received</b><br> 2116 * </p> 2117 */ 2118 @SearchParamDefinition(name="received", path="Communication.received", description="When received", type="date" ) 2119 public static final String SP_RECEIVED = "received"; 2120 /** 2121 * <b>Fluent Client</b> search parameter constant for <b>received</b> 2122 * <p> 2123 * Description: <b>When received</b><br> 2124 * Type: <b>date</b><br> 2125 * Path: <b>Communication.received</b><br> 2126 * </p> 2127 */ 2128 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECEIVED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_RECEIVED); 2129 2130 /** 2131 * Search parameter: <b>part-of</b> 2132 * <p> 2133 * Description: <b>Part of this action</b><br> 2134 * Type: <b>reference</b><br> 2135 * Path: <b>Communication.partOf</b><br> 2136 * </p> 2137 */ 2138 @SearchParamDefinition(name="part-of", path="Communication.partOf", description="Part of this action", type="reference" ) 2139 public static final String SP_PART_OF = "part-of"; 2140 /** 2141 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2142 * <p> 2143 * Description: <b>Part of this action</b><br> 2144 * Type: <b>reference</b><br> 2145 * Path: <b>Communication.partOf</b><br> 2146 * </p> 2147 */ 2148 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2149 2150/** 2151 * Constant for fluent queries to be used to add include statements. Specifies 2152 * the path value of "<b>Communication:part-of</b>". 2153 */ 2154 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Communication:part-of").toLocked(); 2155 2156 /** 2157 * Search parameter: <b>medium</b> 2158 * <p> 2159 * Description: <b>A channel of communication</b><br> 2160 * Type: <b>token</b><br> 2161 * Path: <b>Communication.medium</b><br> 2162 * </p> 2163 */ 2164 @SearchParamDefinition(name="medium", path="Communication.medium", description="A channel of communication", type="token" ) 2165 public static final String SP_MEDIUM = "medium"; 2166 /** 2167 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 2168 * <p> 2169 * Description: <b>A channel of communication</b><br> 2170 * Type: <b>token</b><br> 2171 * Path: <b>Communication.medium</b><br> 2172 * </p> 2173 */ 2174 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MEDIUM); 2175 2176 /** 2177 * Search parameter: <b>encounter</b> 2178 * <p> 2179 * Description: <b>Encounter leading to message</b><br> 2180 * Type: <b>reference</b><br> 2181 * Path: <b>Communication.context</b><br> 2182 * </p> 2183 */ 2184 @SearchParamDefinition(name="encounter", path="Communication.context", description="Encounter leading to message", type="reference", target={Encounter.class } ) 2185 public static final String SP_ENCOUNTER = "encounter"; 2186 /** 2187 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2188 * <p> 2189 * Description: <b>Encounter leading to message</b><br> 2190 * Type: <b>reference</b><br> 2191 * Path: <b>Communication.context</b><br> 2192 * </p> 2193 */ 2194 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2195 2196/** 2197 * Constant for fluent queries to be used to add include statements. Specifies 2198 * the path value of "<b>Communication:encounter</b>". 2199 */ 2200 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Communication:encounter").toLocked(); 2201 2202 /** 2203 * Search parameter: <b>sent</b> 2204 * <p> 2205 * Description: <b>When sent</b><br> 2206 * Type: <b>date</b><br> 2207 * Path: <b>Communication.sent</b><br> 2208 * </p> 2209 */ 2210 @SearchParamDefinition(name="sent", path="Communication.sent", description="When sent", type="date" ) 2211 public static final String SP_SENT = "sent"; 2212 /** 2213 * <b>Fluent Client</b> search parameter constant for <b>sent</b> 2214 * <p> 2215 * Description: <b>When sent</b><br> 2216 * Type: <b>date</b><br> 2217 * Path: <b>Communication.sent</b><br> 2218 * </p> 2219 */ 2220 public static final ca.uhn.fhir.rest.gclient.DateClientParam SENT = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_SENT); 2221 2222 /** 2223 * Search parameter: <b>based-on</b> 2224 * <p> 2225 * Description: <b>Request fulfilled by this communication</b><br> 2226 * Type: <b>reference</b><br> 2227 * Path: <b>Communication.basedOn</b><br> 2228 * </p> 2229 */ 2230 @SearchParamDefinition(name="based-on", path="Communication.basedOn", description="Request fulfilled by this communication", type="reference" ) 2231 public static final String SP_BASED_ON = "based-on"; 2232 /** 2233 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2234 * <p> 2235 * Description: <b>Request fulfilled by this communication</b><br> 2236 * Type: <b>reference</b><br> 2237 * Path: <b>Communication.basedOn</b><br> 2238 * </p> 2239 */ 2240 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2241 2242/** 2243 * Constant for fluent queries to be used to add include statements. Specifies 2244 * the path value of "<b>Communication:based-on</b>". 2245 */ 2246 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Communication:based-on").toLocked(); 2247 2248 /** 2249 * Search parameter: <b>sender</b> 2250 * <p> 2251 * Description: <b>Message sender</b><br> 2252 * Type: <b>reference</b><br> 2253 * Path: <b>Communication.sender</b><br> 2254 * </p> 2255 */ 2256 @SearchParamDefinition(name="sender", path="Communication.sender", description="Message sender", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2257 public static final String SP_SENDER = "sender"; 2258 /** 2259 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 2260 * <p> 2261 * Description: <b>Message sender</b><br> 2262 * Type: <b>reference</b><br> 2263 * Path: <b>Communication.sender</b><br> 2264 * </p> 2265 */ 2266 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SENDER); 2267 2268/** 2269 * Constant for fluent queries to be used to add include statements. Specifies 2270 * the path value of "<b>Communication:sender</b>". 2271 */ 2272 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include("Communication:sender").toLocked(); 2273 2274 /** 2275 * Search parameter: <b>patient</b> 2276 * <p> 2277 * Description: <b>Focus of message</b><br> 2278 * Type: <b>reference</b><br> 2279 * Path: <b>Communication.subject</b><br> 2280 * </p> 2281 */ 2282 @SearchParamDefinition(name="patient", path="Communication.subject", description="Focus of message", type="reference", target={Patient.class } ) 2283 public static final String SP_PATIENT = "patient"; 2284 /** 2285 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2286 * <p> 2287 * Description: <b>Focus of message</b><br> 2288 * Type: <b>reference</b><br> 2289 * Path: <b>Communication.subject</b><br> 2290 * </p> 2291 */ 2292 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2293 2294/** 2295 * Constant for fluent queries to be used to add include statements. Specifies 2296 * the path value of "<b>Communication:patient</b>". 2297 */ 2298 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Communication:patient").toLocked(); 2299 2300 /** 2301 * Search parameter: <b>recipient</b> 2302 * <p> 2303 * Description: <b>Message recipient</b><br> 2304 * Type: <b>reference</b><br> 2305 * Path: <b>Communication.recipient</b><br> 2306 * </p> 2307 */ 2308 @SearchParamDefinition(name="recipient", path="Communication.recipient", description="Message recipient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2309 public static final String SP_RECIPIENT = "recipient"; 2310 /** 2311 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 2312 * <p> 2313 * Description: <b>Message recipient</b><br> 2314 * Type: <b>reference</b><br> 2315 * Path: <b>Communication.recipient</b><br> 2316 * </p> 2317 */ 2318 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 2319 2320/** 2321 * Constant for fluent queries to be used to add include statements. Specifies 2322 * the path value of "<b>Communication:recipient</b>". 2323 */ 2324 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("Communication:recipient").toLocked(); 2325 2326 /** 2327 * Search parameter: <b>context</b> 2328 * <p> 2329 * Description: <b>Encounter or episode leading to message</b><br> 2330 * Type: <b>reference</b><br> 2331 * Path: <b>Communication.context</b><br> 2332 * </p> 2333 */ 2334 @SearchParamDefinition(name="context", path="Communication.context", description="Encounter or episode leading to message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2335 public static final String SP_CONTEXT = "context"; 2336 /** 2337 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2338 * <p> 2339 * Description: <b>Encounter or episode leading to message</b><br> 2340 * Type: <b>reference</b><br> 2341 * Path: <b>Communication.context</b><br> 2342 * </p> 2343 */ 2344 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2345 2346/** 2347 * Constant for fluent queries to be used to add include statements. Specifies 2348 * the path value of "<b>Communication:context</b>". 2349 */ 2350 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("Communication:context").toLocked(); 2351 2352 /** 2353 * Search parameter: <b>definition</b> 2354 * <p> 2355 * Description: <b>Instantiates protocol or definition</b><br> 2356 * Type: <b>reference</b><br> 2357 * Path: <b>Communication.definition</b><br> 2358 * </p> 2359 */ 2360 @SearchParamDefinition(name="definition", path="Communication.definition", description="Instantiates protocol or definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 2361 public static final String SP_DEFINITION = "definition"; 2362 /** 2363 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 2364 * <p> 2365 * Description: <b>Instantiates protocol or definition</b><br> 2366 * Type: <b>reference</b><br> 2367 * Path: <b>Communication.definition</b><br> 2368 * </p> 2369 */ 2370 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 2371 2372/** 2373 * Constant for fluent queries to be used to add include statements. Specifies 2374 * the path value of "<b>Communication:definition</b>". 2375 */ 2376 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("Communication:definition").toLocked(); 2377 2378 /** 2379 * Search parameter: <b>category</b> 2380 * <p> 2381 * Description: <b>Message category</b><br> 2382 * Type: <b>token</b><br> 2383 * Path: <b>Communication.category</b><br> 2384 * </p> 2385 */ 2386 @SearchParamDefinition(name="category", path="Communication.category", description="Message category", type="token" ) 2387 public static final String SP_CATEGORY = "category"; 2388 /** 2389 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2390 * <p> 2391 * Description: <b>Message category</b><br> 2392 * Type: <b>token</b><br> 2393 * Path: <b>Communication.category</b><br> 2394 * </p> 2395 */ 2396 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2397 2398 /** 2399 * Search parameter: <b>status</b> 2400 * <p> 2401 * Description: <b>preparation | in-progress | suspended | aborted | completed | entered-in-error</b><br> 2402 * Type: <b>token</b><br> 2403 * Path: <b>Communication.status</b><br> 2404 * </p> 2405 */ 2406 @SearchParamDefinition(name="status", path="Communication.status", description="preparation | in-progress | suspended | aborted | completed | entered-in-error", type="token" ) 2407 public static final String SP_STATUS = "status"; 2408 /** 2409 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2410 * <p> 2411 * Description: <b>preparation | in-progress | suspended | aborted | completed | entered-in-error</b><br> 2412 * Type: <b>token</b><br> 2413 * Path: <b>Communication.status</b><br> 2414 * </p> 2415 */ 2416 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2417 2418 2419}