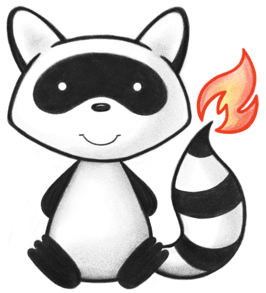
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 050 */ 051@ResourceDef(name="Communication", profile="http://hl7.org/fhir/Profile/Communication") 052public class Communication extends DomainResource { 053 054 public enum CommunicationStatus { 055 /** 056 * The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes. 057 */ 058 PREPARATION, 059 /** 060 * The event is currently occurring 061 */ 062 INPROGRESS, 063 /** 064 * The event has been temporarily stopped but is expected to resume in the future 065 */ 066 SUSPENDED, 067 /** 068 * The event was prior to the full completion of the intended actions 069 */ 070 ABORTED, 071 /** 072 * The event has now concluded 073 */ 074 COMPLETED, 075 /** 076 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static CommunicationStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("preparation".equals(codeString)) 091 return PREPARATION; 092 if ("in-progress".equals(codeString)) 093 return INPROGRESS; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("aborted".equals(codeString)) 097 return ABORTED; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown CommunicationStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case PREPARATION: return "preparation"; 112 case INPROGRESS: return "in-progress"; 113 case SUSPENDED: return "suspended"; 114 case ABORTED: return "aborted"; 115 case COMPLETED: return "completed"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case PREPARATION: return "http://hl7.org/fhir/event-status"; 125 case INPROGRESS: return "http://hl7.org/fhir/event-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/event-status"; 127 case ABORTED: return "http://hl7.org/fhir/event-status"; 128 case COMPLETED: return "http://hl7.org/fhir/event-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/event-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/event-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case PREPARATION: return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 138 case INPROGRESS: return "The event is currently occurring"; 139 case SUSPENDED: return "The event has been temporarily stopped but is expected to resume in the future"; 140 case ABORTED: return "The event was prior to the full completion of the intended actions"; 141 case COMPLETED: return "The event has now concluded"; 142 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 143 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case PREPARATION: return "Preparation"; 151 case INPROGRESS: return "In Progress"; 152 case SUSPENDED: return "Suspended"; 153 case ABORTED: return "Aborted"; 154 case COMPLETED: return "Completed"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class CommunicationStatusEnumFactory implements EnumFactory<CommunicationStatus> { 164 public CommunicationStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("preparation".equals(codeString)) 169 return CommunicationStatus.PREPARATION; 170 if ("in-progress".equals(codeString)) 171 return CommunicationStatus.INPROGRESS; 172 if ("suspended".equals(codeString)) 173 return CommunicationStatus.SUSPENDED; 174 if ("aborted".equals(codeString)) 175 return CommunicationStatus.ABORTED; 176 if ("completed".equals(codeString)) 177 return CommunicationStatus.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return CommunicationStatus.ENTEREDINERROR; 180 if ("unknown".equals(codeString)) 181 return CommunicationStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown CommunicationStatus code '"+codeString+"'"); 183 } 184 public Enumeration<CommunicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<CommunicationStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("preparation".equals(codeString)) 193 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.PREPARATION); 194 if ("in-progress".equals(codeString)) 195 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.INPROGRESS); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.SUSPENDED); 198 if ("aborted".equals(codeString)) 199 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.ABORTED); 200 if ("completed".equals(codeString)) 201 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.COMPLETED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.ENTEREDINERROR); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.UNKNOWN); 206 throw new FHIRException("Unknown CommunicationStatus code '"+codeString+"'"); 207 } 208 public String toCode(CommunicationStatus code) { 209 if (code == CommunicationStatus.NULL) 210 return null; 211 if (code == CommunicationStatus.PREPARATION) 212 return "preparation"; 213 if (code == CommunicationStatus.INPROGRESS) 214 return "in-progress"; 215 if (code == CommunicationStatus.SUSPENDED) 216 return "suspended"; 217 if (code == CommunicationStatus.ABORTED) 218 return "aborted"; 219 if (code == CommunicationStatus.COMPLETED) 220 return "completed"; 221 if (code == CommunicationStatus.ENTEREDINERROR) 222 return "entered-in-error"; 223 if (code == CommunicationStatus.UNKNOWN) 224 return "unknown"; 225 return "?"; 226 } 227 public String toSystem(CommunicationStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 @Block() 233 public static class CommunicationPayloadComponent extends BackboneElement implements IBaseBackboneElement { 234 /** 235 * A communicated content (or for multi-part communications, one portion of the communication). 236 */ 237 @Child(name = "content", type = {StringType.class, Attachment.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 238 @Description(shortDefinition="Message part content", formalDefinition="A communicated content (or for multi-part communications, one portion of the communication)." ) 239 protected Type content; 240 241 private static final long serialVersionUID = -1763459053L; 242 243 /** 244 * Constructor 245 */ 246 public CommunicationPayloadComponent() { 247 super(); 248 } 249 250 /** 251 * Constructor 252 */ 253 public CommunicationPayloadComponent(Type content) { 254 super(); 255 this.content = content; 256 } 257 258 /** 259 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 260 */ 261 public Type getContent() { 262 return this.content; 263 } 264 265 /** 266 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 267 */ 268 public StringType getContentStringType() throws FHIRException { 269 if (this.content == null) 270 return null; 271 if (!(this.content instanceof StringType)) 272 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.content.getClass().getName()+" was encountered"); 273 return (StringType) this.content; 274 } 275 276 public boolean hasContentStringType() { 277 return this != null && this.content instanceof StringType; 278 } 279 280 /** 281 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 282 */ 283 public Attachment getContentAttachment() throws FHIRException { 284 if (this.content == null) 285 return null; 286 if (!(this.content instanceof Attachment)) 287 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 288 return (Attachment) this.content; 289 } 290 291 public boolean hasContentAttachment() { 292 return this != null && this.content instanceof Attachment; 293 } 294 295 /** 296 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 297 */ 298 public Reference getContentReference() throws FHIRException { 299 if (this.content == null) 300 return null; 301 if (!(this.content instanceof Reference)) 302 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 303 return (Reference) this.content; 304 } 305 306 public boolean hasContentReference() { 307 return this != null && this.content instanceof Reference; 308 } 309 310 public boolean hasContent() { 311 return this.content != null && !this.content.isEmpty(); 312 } 313 314 /** 315 * @param value {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 316 */ 317 public CommunicationPayloadComponent setContent(Type value) throws FHIRFormatError { 318 if (value != null && !(value instanceof StringType || value instanceof Attachment || value instanceof Reference)) 319 throw new FHIRFormatError("Not the right type for Communication.payload.content[x]: "+value.fhirType()); 320 this.content = value; 321 return this; 322 } 323 324 protected void listChildren(List<Property> children) { 325 super.listChildren(children); 326 children.add(new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content)); 327 } 328 329 @Override 330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 331 switch (_hash) { 332 case 264548711: /*content[x]*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 333 case 951530617: /*content*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 334 case -326336022: /*contentString*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 335 case -702028164: /*contentAttachment*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 336 case 1193747154: /*contentReference*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 337 default: return super.getNamedProperty(_hash, _name, _checkValid); 338 } 339 340 } 341 342 @Override 343 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 344 switch (hash) { 345 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Type 346 default: return super.getProperty(hash, name, checkValid); 347 } 348 349 } 350 351 @Override 352 public Base setProperty(int hash, String name, Base value) throws FHIRException { 353 switch (hash) { 354 case 951530617: // content 355 this.content = castToType(value); // Type 356 return value; 357 default: return super.setProperty(hash, name, value); 358 } 359 360 } 361 362 @Override 363 public Base setProperty(String name, Base value) throws FHIRException { 364 if (name.equals("content[x]")) { 365 this.content = castToType(value); // Type 366 } else 367 return super.setProperty(name, value); 368 return value; 369 } 370 371 @Override 372 public Base makeProperty(int hash, String name) throws FHIRException { 373 switch (hash) { 374 case 264548711: return getContent(); 375 case 951530617: return getContent(); 376 default: return super.makeProperty(hash, name); 377 } 378 379 } 380 381 @Override 382 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 383 switch (hash) { 384 case 951530617: /*content*/ return new String[] {"string", "Attachment", "Reference"}; 385 default: return super.getTypesForProperty(hash, name); 386 } 387 388 } 389 390 @Override 391 public Base addChild(String name) throws FHIRException { 392 if (name.equals("contentString")) { 393 this.content = new StringType(); 394 return this.content; 395 } 396 else if (name.equals("contentAttachment")) { 397 this.content = new Attachment(); 398 return this.content; 399 } 400 else if (name.equals("contentReference")) { 401 this.content = new Reference(); 402 return this.content; 403 } 404 else 405 return super.addChild(name); 406 } 407 408 public CommunicationPayloadComponent copy() { 409 CommunicationPayloadComponent dst = new CommunicationPayloadComponent(); 410 copyValues(dst); 411 dst.content = content == null ? null : content.copy(); 412 return dst; 413 } 414 415 @Override 416 public boolean equalsDeep(Base other_) { 417 if (!super.equalsDeep(other_)) 418 return false; 419 if (!(other_ instanceof CommunicationPayloadComponent)) 420 return false; 421 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 422 return compareDeep(content, o.content, true); 423 } 424 425 @Override 426 public boolean equalsShallow(Base other_) { 427 if (!super.equalsShallow(other_)) 428 return false; 429 if (!(other_ instanceof CommunicationPayloadComponent)) 430 return false; 431 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 432 return true; 433 } 434 435 public boolean isEmpty() { 436 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 437 } 438 439 public String fhirType() { 440 return "Communication.payload"; 441 442 } 443 444 } 445 446 /** 447 * Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 448 */ 449 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 450 @Description(shortDefinition="Unique identifier", formalDefinition="Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 451 protected List<Identifier> identifier; 452 453 /** 454 * A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event. 455 */ 456 @Child(name = "definition", type = {PlanDefinition.class, ActivityDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 457 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event." ) 458 protected List<Reference> definition; 459 /** 460 * The actual objects that are the target of the reference (A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.) 461 */ 462 protected List<Resource> definitionTarget; 463 464 465 /** 466 * An order, proposal or plan fulfilled in whole or in part by this Communication. 467 */ 468 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 469 @Description(shortDefinition="Request fulfilled by this communication", formalDefinition="An order, proposal or plan fulfilled in whole or in part by this Communication." ) 470 protected List<Reference> basedOn; 471 /** 472 * The actual objects that are the target of the reference (An order, proposal or plan fulfilled in whole or in part by this Communication.) 473 */ 474 protected List<Resource> basedOnTarget; 475 476 477 /** 478 * Part of this action. 479 */ 480 @Child(name = "partOf", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 481 @Description(shortDefinition="Part of this action", formalDefinition="Part of this action." ) 482 protected List<Reference> partOf; 483 /** 484 * The actual objects that are the target of the reference (Part of this action.) 485 */ 486 protected List<Resource> partOfTarget; 487 488 489 /** 490 * The status of the transmission. 491 */ 492 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 493 @Description(shortDefinition="preparation | in-progress | suspended | aborted | completed | entered-in-error", formalDefinition="The status of the transmission." ) 494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-status") 495 protected Enumeration<CommunicationStatus> status; 496 497 /** 498 * If true, indicates that the described communication event did not actually occur. 499 */ 500 @Child(name = "notDone", type = {BooleanType.class}, order=5, min=0, max=1, modifier=true, summary=true) 501 @Description(shortDefinition="Communication did not occur", formalDefinition="If true, indicates that the described communication event did not actually occur." ) 502 protected BooleanType notDone; 503 504 /** 505 * Describes why the communication event did not occur in coded and/or textual form. 506 */ 507 @Child(name = "notDoneReason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 508 @Description(shortDefinition="Why communication did not occur", formalDefinition="Describes why the communication event did not occur in coded and/or textual form." ) 509 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-not-done-reason") 510 protected CodeableConcept notDoneReason; 511 512 /** 513 * The type of message conveyed such as alert, notification, reminder, instruction, etc. 514 */ 515 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 516 @Description(shortDefinition="Message category", formalDefinition="The type of message conveyed such as alert, notification, reminder, instruction, etc." ) 517 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-category") 518 protected List<CodeableConcept> category; 519 520 /** 521 * A channel that was used for this communication (e.g. email, fax). 522 */ 523 @Child(name = "medium", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 524 @Description(shortDefinition="A channel of communication", formalDefinition="A channel that was used for this communication (e.g. email, fax)." ) 525 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ParticipationMode") 526 protected List<CodeableConcept> medium; 527 528 /** 529 * The patient or group that was the focus of this communication. 530 */ 531 @Child(name = "subject", type = {Patient.class, Group.class}, order=9, min=0, max=1, modifier=false, summary=true) 532 @Description(shortDefinition="Focus of message", formalDefinition="The patient or group that was the focus of this communication." ) 533 protected Reference subject; 534 535 /** 536 * The actual object that is the target of the reference (The patient or group that was the focus of this communication.) 537 */ 538 protected Resource subjectTarget; 539 540 /** 541 * The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time). 542 */ 543 @Child(name = "recipient", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, Group.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 544 @Description(shortDefinition="Message recipient", formalDefinition="The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time)." ) 545 protected List<Reference> recipient; 546 /** 547 * The actual objects that are the target of the reference (The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).) 548 */ 549 protected List<Resource> recipientTarget; 550 551 552 /** 553 * The resources which were responsible for or related to producing this communication. 554 */ 555 @Child(name = "topic", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 556 @Description(shortDefinition="Focal resources", formalDefinition="The resources which were responsible for or related to producing this communication." ) 557 protected List<Reference> topic; 558 /** 559 * The actual objects that are the target of the reference (The resources which were responsible for or related to producing this communication.) 560 */ 561 protected List<Resource> topicTarget; 562 563 564 /** 565 * The encounter within which the communication was sent. 566 */ 567 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=12, min=0, max=1, modifier=false, summary=true) 568 @Description(shortDefinition="Encounter or episode leading to message", formalDefinition="The encounter within which the communication was sent." ) 569 protected Reference context; 570 571 /** 572 * The actual object that is the target of the reference (The encounter within which the communication was sent.) 573 */ 574 protected Resource contextTarget; 575 576 /** 577 * The time when this communication was sent. 578 */ 579 @Child(name = "sent", type = {DateTimeType.class}, order=13, min=0, max=1, modifier=false, summary=false) 580 @Description(shortDefinition="When sent", formalDefinition="The time when this communication was sent." ) 581 protected DateTimeType sent; 582 583 /** 584 * The time when this communication arrived at the destination. 585 */ 586 @Child(name = "received", type = {DateTimeType.class}, order=14, min=0, max=1, modifier=false, summary=false) 587 @Description(shortDefinition="When received", formalDefinition="The time when this communication arrived at the destination." ) 588 protected DateTimeType received; 589 590 /** 591 * The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication. 592 */ 593 @Child(name = "sender", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=15, min=0, max=1, modifier=false, summary=false) 594 @Description(shortDefinition="Message sender", formalDefinition="The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication." ) 595 protected Reference sender; 596 597 /** 598 * The actual object that is the target of the reference (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 599 */ 600 protected Resource senderTarget; 601 602 /** 603 * The reason or justification for the communication. 604 */ 605 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 606 @Description(shortDefinition="Indication for message", formalDefinition="The reason or justification for the communication." ) 607 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 608 protected List<CodeableConcept> reasonCode; 609 610 /** 611 * Indicates another resource whose existence justifies this communication. 612 */ 613 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 614 @Description(shortDefinition="Why was communication done?", formalDefinition="Indicates another resource whose existence justifies this communication." ) 615 protected List<Reference> reasonReference; 616 /** 617 * The actual objects that are the target of the reference (Indicates another resource whose existence justifies this communication.) 618 */ 619 protected List<Resource> reasonReferenceTarget; 620 621 622 /** 623 * Text, attachment(s), or resource(s) that was communicated to the recipient. 624 */ 625 @Child(name = "payload", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 626 @Description(shortDefinition="Message payload", formalDefinition="Text, attachment(s), or resource(s) that was communicated to the recipient." ) 627 protected List<CommunicationPayloadComponent> payload; 628 629 /** 630 * Additional notes or commentary about the communication by the sender, receiver or other interested parties. 631 */ 632 @Child(name = "note", type = {Annotation.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 633 @Description(shortDefinition="Comments made about the communication", formalDefinition="Additional notes or commentary about the communication by the sender, receiver or other interested parties." ) 634 protected List<Annotation> note; 635 636 private static final long serialVersionUID = -1362735590L; 637 638 /** 639 * Constructor 640 */ 641 public Communication() { 642 super(); 643 } 644 645 /** 646 * Constructor 647 */ 648 public Communication(Enumeration<CommunicationStatus> status) { 649 super(); 650 this.status = status; 651 } 652 653 /** 654 * @return {@link #identifier} (Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 655 */ 656 public List<Identifier> getIdentifier() { 657 if (this.identifier == null) 658 this.identifier = new ArrayList<Identifier>(); 659 return this.identifier; 660 } 661 662 /** 663 * @return Returns a reference to <code>this</code> for easy method chaining 664 */ 665 public Communication setIdentifier(List<Identifier> theIdentifier) { 666 this.identifier = theIdentifier; 667 return this; 668 } 669 670 public boolean hasIdentifier() { 671 if (this.identifier == null) 672 return false; 673 for (Identifier item : this.identifier) 674 if (!item.isEmpty()) 675 return true; 676 return false; 677 } 678 679 public Identifier addIdentifier() { //3 680 Identifier t = new Identifier(); 681 if (this.identifier == null) 682 this.identifier = new ArrayList<Identifier>(); 683 this.identifier.add(t); 684 return t; 685 } 686 687 public Communication addIdentifier(Identifier t) { //3 688 if (t == null) 689 return this; 690 if (this.identifier == null) 691 this.identifier = new ArrayList<Identifier>(); 692 this.identifier.add(t); 693 return this; 694 } 695 696 /** 697 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 698 */ 699 public Identifier getIdentifierFirstRep() { 700 if (getIdentifier().isEmpty()) { 701 addIdentifier(); 702 } 703 return getIdentifier().get(0); 704 } 705 706 /** 707 * @return {@link #definition} (A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.) 708 */ 709 public List<Reference> getDefinition() { 710 if (this.definition == null) 711 this.definition = new ArrayList<Reference>(); 712 return this.definition; 713 } 714 715 /** 716 * @return Returns a reference to <code>this</code> for easy method chaining 717 */ 718 public Communication setDefinition(List<Reference> theDefinition) { 719 this.definition = theDefinition; 720 return this; 721 } 722 723 public boolean hasDefinition() { 724 if (this.definition == null) 725 return false; 726 for (Reference item : this.definition) 727 if (!item.isEmpty()) 728 return true; 729 return false; 730 } 731 732 public Reference addDefinition() { //3 733 Reference t = new Reference(); 734 if (this.definition == null) 735 this.definition = new ArrayList<Reference>(); 736 this.definition.add(t); 737 return t; 738 } 739 740 public Communication addDefinition(Reference t) { //3 741 if (t == null) 742 return this; 743 if (this.definition == null) 744 this.definition = new ArrayList<Reference>(); 745 this.definition.add(t); 746 return this; 747 } 748 749 /** 750 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 751 */ 752 public Reference getDefinitionFirstRep() { 753 if (getDefinition().isEmpty()) { 754 addDefinition(); 755 } 756 return getDefinition().get(0); 757 } 758 759 /** 760 * @deprecated Use Reference#setResource(IBaseResource) instead 761 */ 762 @Deprecated 763 public List<Resource> getDefinitionTarget() { 764 if (this.definitionTarget == null) 765 this.definitionTarget = new ArrayList<Resource>(); 766 return this.definitionTarget; 767 } 768 769 /** 770 * @return {@link #basedOn} (An order, proposal or plan fulfilled in whole or in part by this Communication.) 771 */ 772 public List<Reference> getBasedOn() { 773 if (this.basedOn == null) 774 this.basedOn = new ArrayList<Reference>(); 775 return this.basedOn; 776 } 777 778 /** 779 * @return Returns a reference to <code>this</code> for easy method chaining 780 */ 781 public Communication setBasedOn(List<Reference> theBasedOn) { 782 this.basedOn = theBasedOn; 783 return this; 784 } 785 786 public boolean hasBasedOn() { 787 if (this.basedOn == null) 788 return false; 789 for (Reference item : this.basedOn) 790 if (!item.isEmpty()) 791 return true; 792 return false; 793 } 794 795 public Reference addBasedOn() { //3 796 Reference t = new Reference(); 797 if (this.basedOn == null) 798 this.basedOn = new ArrayList<Reference>(); 799 this.basedOn.add(t); 800 return t; 801 } 802 803 public Communication addBasedOn(Reference t) { //3 804 if (t == null) 805 return this; 806 if (this.basedOn == null) 807 this.basedOn = new ArrayList<Reference>(); 808 this.basedOn.add(t); 809 return this; 810 } 811 812 /** 813 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 814 */ 815 public Reference getBasedOnFirstRep() { 816 if (getBasedOn().isEmpty()) { 817 addBasedOn(); 818 } 819 return getBasedOn().get(0); 820 } 821 822 /** 823 * @deprecated Use Reference#setResource(IBaseResource) instead 824 */ 825 @Deprecated 826 public List<Resource> getBasedOnTarget() { 827 if (this.basedOnTarget == null) 828 this.basedOnTarget = new ArrayList<Resource>(); 829 return this.basedOnTarget; 830 } 831 832 /** 833 * @return {@link #partOf} (Part of this action.) 834 */ 835 public List<Reference> getPartOf() { 836 if (this.partOf == null) 837 this.partOf = new ArrayList<Reference>(); 838 return this.partOf; 839 } 840 841 /** 842 * @return Returns a reference to <code>this</code> for easy method chaining 843 */ 844 public Communication setPartOf(List<Reference> thePartOf) { 845 this.partOf = thePartOf; 846 return this; 847 } 848 849 public boolean hasPartOf() { 850 if (this.partOf == null) 851 return false; 852 for (Reference item : this.partOf) 853 if (!item.isEmpty()) 854 return true; 855 return false; 856 } 857 858 public Reference addPartOf() { //3 859 Reference t = new Reference(); 860 if (this.partOf == null) 861 this.partOf = new ArrayList<Reference>(); 862 this.partOf.add(t); 863 return t; 864 } 865 866 public Communication addPartOf(Reference t) { //3 867 if (t == null) 868 return this; 869 if (this.partOf == null) 870 this.partOf = new ArrayList<Reference>(); 871 this.partOf.add(t); 872 return this; 873 } 874 875 /** 876 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 877 */ 878 public Reference getPartOfFirstRep() { 879 if (getPartOf().isEmpty()) { 880 addPartOf(); 881 } 882 return getPartOf().get(0); 883 } 884 885 /** 886 * @deprecated Use Reference#setResource(IBaseResource) instead 887 */ 888 @Deprecated 889 public List<Resource> getPartOfTarget() { 890 if (this.partOfTarget == null) 891 this.partOfTarget = new ArrayList<Resource>(); 892 return this.partOfTarget; 893 } 894 895 /** 896 * @return {@link #status} (The status of the transmission.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 897 */ 898 public Enumeration<CommunicationStatus> getStatusElement() { 899 if (this.status == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create Communication.status"); 902 else if (Configuration.doAutoCreate()) 903 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); // bb 904 return this.status; 905 } 906 907 public boolean hasStatusElement() { 908 return this.status != null && !this.status.isEmpty(); 909 } 910 911 public boolean hasStatus() { 912 return this.status != null && !this.status.isEmpty(); 913 } 914 915 /** 916 * @param value {@link #status} (The status of the transmission.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 917 */ 918 public Communication setStatusElement(Enumeration<CommunicationStatus> value) { 919 this.status = value; 920 return this; 921 } 922 923 /** 924 * @return The status of the transmission. 925 */ 926 public CommunicationStatus getStatus() { 927 return this.status == null ? null : this.status.getValue(); 928 } 929 930 /** 931 * @param value The status of the transmission. 932 */ 933 public Communication setStatus(CommunicationStatus value) { 934 if (this.status == null) 935 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); 936 this.status.setValue(value); 937 return this; 938 } 939 940 /** 941 * @return {@link #notDone} (If true, indicates that the described communication event did not actually occur.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 942 */ 943 public BooleanType getNotDoneElement() { 944 if (this.notDone == null) 945 if (Configuration.errorOnAutoCreate()) 946 throw new Error("Attempt to auto-create Communication.notDone"); 947 else if (Configuration.doAutoCreate()) 948 this.notDone = new BooleanType(); // bb 949 return this.notDone; 950 } 951 952 public boolean hasNotDoneElement() { 953 return this.notDone != null && !this.notDone.isEmpty(); 954 } 955 956 public boolean hasNotDone() { 957 return this.notDone != null && !this.notDone.isEmpty(); 958 } 959 960 /** 961 * @param value {@link #notDone} (If true, indicates that the described communication event did not actually occur.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 962 */ 963 public Communication setNotDoneElement(BooleanType value) { 964 this.notDone = value; 965 return this; 966 } 967 968 /** 969 * @return If true, indicates that the described communication event did not actually occur. 970 */ 971 public boolean getNotDone() { 972 return this.notDone == null || this.notDone.isEmpty() ? false : this.notDone.getValue(); 973 } 974 975 /** 976 * @param value If true, indicates that the described communication event did not actually occur. 977 */ 978 public Communication setNotDone(boolean value) { 979 if (this.notDone == null) 980 this.notDone = new BooleanType(); 981 this.notDone.setValue(value); 982 return this; 983 } 984 985 /** 986 * @return {@link #notDoneReason} (Describes why the communication event did not occur in coded and/or textual form.) 987 */ 988 public CodeableConcept getNotDoneReason() { 989 if (this.notDoneReason == null) 990 if (Configuration.errorOnAutoCreate()) 991 throw new Error("Attempt to auto-create Communication.notDoneReason"); 992 else if (Configuration.doAutoCreate()) 993 this.notDoneReason = new CodeableConcept(); // cc 994 return this.notDoneReason; 995 } 996 997 public boolean hasNotDoneReason() { 998 return this.notDoneReason != null && !this.notDoneReason.isEmpty(); 999 } 1000 1001 /** 1002 * @param value {@link #notDoneReason} (Describes why the communication event did not occur in coded and/or textual form.) 1003 */ 1004 public Communication setNotDoneReason(CodeableConcept value) { 1005 this.notDoneReason = value; 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #category} (The type of message conveyed such as alert, notification, reminder, instruction, etc.) 1011 */ 1012 public List<CodeableConcept> getCategory() { 1013 if (this.category == null) 1014 this.category = new ArrayList<CodeableConcept>(); 1015 return this.category; 1016 } 1017 1018 /** 1019 * @return Returns a reference to <code>this</code> for easy method chaining 1020 */ 1021 public Communication setCategory(List<CodeableConcept> theCategory) { 1022 this.category = theCategory; 1023 return this; 1024 } 1025 1026 public boolean hasCategory() { 1027 if (this.category == null) 1028 return false; 1029 for (CodeableConcept item : this.category) 1030 if (!item.isEmpty()) 1031 return true; 1032 return false; 1033 } 1034 1035 public CodeableConcept addCategory() { //3 1036 CodeableConcept t = new CodeableConcept(); 1037 if (this.category == null) 1038 this.category = new ArrayList<CodeableConcept>(); 1039 this.category.add(t); 1040 return t; 1041 } 1042 1043 public Communication addCategory(CodeableConcept t) { //3 1044 if (t == null) 1045 return this; 1046 if (this.category == null) 1047 this.category = new ArrayList<CodeableConcept>(); 1048 this.category.add(t); 1049 return this; 1050 } 1051 1052 /** 1053 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 1054 */ 1055 public CodeableConcept getCategoryFirstRep() { 1056 if (getCategory().isEmpty()) { 1057 addCategory(); 1058 } 1059 return getCategory().get(0); 1060 } 1061 1062 /** 1063 * @return {@link #medium} (A channel that was used for this communication (e.g. email, fax).) 1064 */ 1065 public List<CodeableConcept> getMedium() { 1066 if (this.medium == null) 1067 this.medium = new ArrayList<CodeableConcept>(); 1068 return this.medium; 1069 } 1070 1071 /** 1072 * @return Returns a reference to <code>this</code> for easy method chaining 1073 */ 1074 public Communication setMedium(List<CodeableConcept> theMedium) { 1075 this.medium = theMedium; 1076 return this; 1077 } 1078 1079 public boolean hasMedium() { 1080 if (this.medium == null) 1081 return false; 1082 for (CodeableConcept item : this.medium) 1083 if (!item.isEmpty()) 1084 return true; 1085 return false; 1086 } 1087 1088 public CodeableConcept addMedium() { //3 1089 CodeableConcept t = new CodeableConcept(); 1090 if (this.medium == null) 1091 this.medium = new ArrayList<CodeableConcept>(); 1092 this.medium.add(t); 1093 return t; 1094 } 1095 1096 public Communication addMedium(CodeableConcept t) { //3 1097 if (t == null) 1098 return this; 1099 if (this.medium == null) 1100 this.medium = new ArrayList<CodeableConcept>(); 1101 this.medium.add(t); 1102 return this; 1103 } 1104 1105 /** 1106 * @return The first repetition of repeating field {@link #medium}, creating it if it does not already exist 1107 */ 1108 public CodeableConcept getMediumFirstRep() { 1109 if (getMedium().isEmpty()) { 1110 addMedium(); 1111 } 1112 return getMedium().get(0); 1113 } 1114 1115 /** 1116 * @return {@link #subject} (The patient or group that was the focus of this communication.) 1117 */ 1118 public Reference getSubject() { 1119 if (this.subject == null) 1120 if (Configuration.errorOnAutoCreate()) 1121 throw new Error("Attempt to auto-create Communication.subject"); 1122 else if (Configuration.doAutoCreate()) 1123 this.subject = new Reference(); // cc 1124 return this.subject; 1125 } 1126 1127 public boolean hasSubject() { 1128 return this.subject != null && !this.subject.isEmpty(); 1129 } 1130 1131 /** 1132 * @param value {@link #subject} (The patient or group that was the focus of this communication.) 1133 */ 1134 public Communication setSubject(Reference value) { 1135 this.subject = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient or group that was the focus of this communication.) 1141 */ 1142 public Resource getSubjectTarget() { 1143 return this.subjectTarget; 1144 } 1145 1146 /** 1147 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient or group that was the focus of this communication.) 1148 */ 1149 public Communication setSubjectTarget(Resource value) { 1150 this.subjectTarget = value; 1151 return this; 1152 } 1153 1154 /** 1155 * @return {@link #recipient} (The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).) 1156 */ 1157 public List<Reference> getRecipient() { 1158 if (this.recipient == null) 1159 this.recipient = new ArrayList<Reference>(); 1160 return this.recipient; 1161 } 1162 1163 /** 1164 * @return Returns a reference to <code>this</code> for easy method chaining 1165 */ 1166 public Communication setRecipient(List<Reference> theRecipient) { 1167 this.recipient = theRecipient; 1168 return this; 1169 } 1170 1171 public boolean hasRecipient() { 1172 if (this.recipient == null) 1173 return false; 1174 for (Reference item : this.recipient) 1175 if (!item.isEmpty()) 1176 return true; 1177 return false; 1178 } 1179 1180 public Reference addRecipient() { //3 1181 Reference t = new Reference(); 1182 if (this.recipient == null) 1183 this.recipient = new ArrayList<Reference>(); 1184 this.recipient.add(t); 1185 return t; 1186 } 1187 1188 public Communication addRecipient(Reference t) { //3 1189 if (t == null) 1190 return this; 1191 if (this.recipient == null) 1192 this.recipient = new ArrayList<Reference>(); 1193 this.recipient.add(t); 1194 return this; 1195 } 1196 1197 /** 1198 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist 1199 */ 1200 public Reference getRecipientFirstRep() { 1201 if (getRecipient().isEmpty()) { 1202 addRecipient(); 1203 } 1204 return getRecipient().get(0); 1205 } 1206 1207 /** 1208 * @deprecated Use Reference#setResource(IBaseResource) instead 1209 */ 1210 @Deprecated 1211 public List<Resource> getRecipientTarget() { 1212 if (this.recipientTarget == null) 1213 this.recipientTarget = new ArrayList<Resource>(); 1214 return this.recipientTarget; 1215 } 1216 1217 /** 1218 * @return {@link #topic} (The resources which were responsible for or related to producing this communication.) 1219 */ 1220 public List<Reference> getTopic() { 1221 if (this.topic == null) 1222 this.topic = new ArrayList<Reference>(); 1223 return this.topic; 1224 } 1225 1226 /** 1227 * @return Returns a reference to <code>this</code> for easy method chaining 1228 */ 1229 public Communication setTopic(List<Reference> theTopic) { 1230 this.topic = theTopic; 1231 return this; 1232 } 1233 1234 public boolean hasTopic() { 1235 if (this.topic == null) 1236 return false; 1237 for (Reference item : this.topic) 1238 if (!item.isEmpty()) 1239 return true; 1240 return false; 1241 } 1242 1243 public Reference addTopic() { //3 1244 Reference t = new Reference(); 1245 if (this.topic == null) 1246 this.topic = new ArrayList<Reference>(); 1247 this.topic.add(t); 1248 return t; 1249 } 1250 1251 public Communication addTopic(Reference t) { //3 1252 if (t == null) 1253 return this; 1254 if (this.topic == null) 1255 this.topic = new ArrayList<Reference>(); 1256 this.topic.add(t); 1257 return this; 1258 } 1259 1260 /** 1261 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 1262 */ 1263 public Reference getTopicFirstRep() { 1264 if (getTopic().isEmpty()) { 1265 addTopic(); 1266 } 1267 return getTopic().get(0); 1268 } 1269 1270 /** 1271 * @deprecated Use Reference#setResource(IBaseResource) instead 1272 */ 1273 @Deprecated 1274 public List<Resource> getTopicTarget() { 1275 if (this.topicTarget == null) 1276 this.topicTarget = new ArrayList<Resource>(); 1277 return this.topicTarget; 1278 } 1279 1280 /** 1281 * @return {@link #context} (The encounter within which the communication was sent.) 1282 */ 1283 public Reference getContext() { 1284 if (this.context == null) 1285 if (Configuration.errorOnAutoCreate()) 1286 throw new Error("Attempt to auto-create Communication.context"); 1287 else if (Configuration.doAutoCreate()) 1288 this.context = new Reference(); // cc 1289 return this.context; 1290 } 1291 1292 public boolean hasContext() { 1293 return this.context != null && !this.context.isEmpty(); 1294 } 1295 1296 /** 1297 * @param value {@link #context} (The encounter within which the communication was sent.) 1298 */ 1299 public Communication setContext(Reference value) { 1300 this.context = value; 1301 return this; 1302 } 1303 1304 /** 1305 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter within which the communication was sent.) 1306 */ 1307 public Resource getContextTarget() { 1308 return this.contextTarget; 1309 } 1310 1311 /** 1312 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter within which the communication was sent.) 1313 */ 1314 public Communication setContextTarget(Resource value) { 1315 this.contextTarget = value; 1316 return this; 1317 } 1318 1319 /** 1320 * @return {@link #sent} (The time when this communication was sent.). This is the underlying object with id, value and extensions. The accessor "getSent" gives direct access to the value 1321 */ 1322 public DateTimeType getSentElement() { 1323 if (this.sent == null) 1324 if (Configuration.errorOnAutoCreate()) 1325 throw new Error("Attempt to auto-create Communication.sent"); 1326 else if (Configuration.doAutoCreate()) 1327 this.sent = new DateTimeType(); // bb 1328 return this.sent; 1329 } 1330 1331 public boolean hasSentElement() { 1332 return this.sent != null && !this.sent.isEmpty(); 1333 } 1334 1335 public boolean hasSent() { 1336 return this.sent != null && !this.sent.isEmpty(); 1337 } 1338 1339 /** 1340 * @param value {@link #sent} (The time when this communication was sent.). This is the underlying object with id, value and extensions. The accessor "getSent" gives direct access to the value 1341 */ 1342 public Communication setSentElement(DateTimeType value) { 1343 this.sent = value; 1344 return this; 1345 } 1346 1347 /** 1348 * @return The time when this communication was sent. 1349 */ 1350 public Date getSent() { 1351 return this.sent == null ? null : this.sent.getValue(); 1352 } 1353 1354 /** 1355 * @param value The time when this communication was sent. 1356 */ 1357 public Communication setSent(Date value) { 1358 if (value == null) 1359 this.sent = null; 1360 else { 1361 if (this.sent == null) 1362 this.sent = new DateTimeType(); 1363 this.sent.setValue(value); 1364 } 1365 return this; 1366 } 1367 1368 /** 1369 * @return {@link #received} (The time when this communication arrived at the destination.). This is the underlying object with id, value and extensions. The accessor "getReceived" gives direct access to the value 1370 */ 1371 public DateTimeType getReceivedElement() { 1372 if (this.received == null) 1373 if (Configuration.errorOnAutoCreate()) 1374 throw new Error("Attempt to auto-create Communication.received"); 1375 else if (Configuration.doAutoCreate()) 1376 this.received = new DateTimeType(); // bb 1377 return this.received; 1378 } 1379 1380 public boolean hasReceivedElement() { 1381 return this.received != null && !this.received.isEmpty(); 1382 } 1383 1384 public boolean hasReceived() { 1385 return this.received != null && !this.received.isEmpty(); 1386 } 1387 1388 /** 1389 * @param value {@link #received} (The time when this communication arrived at the destination.). This is the underlying object with id, value and extensions. The accessor "getReceived" gives direct access to the value 1390 */ 1391 public Communication setReceivedElement(DateTimeType value) { 1392 this.received = value; 1393 return this; 1394 } 1395 1396 /** 1397 * @return The time when this communication arrived at the destination. 1398 */ 1399 public Date getReceived() { 1400 return this.received == null ? null : this.received.getValue(); 1401 } 1402 1403 /** 1404 * @param value The time when this communication arrived at the destination. 1405 */ 1406 public Communication setReceived(Date value) { 1407 if (value == null) 1408 this.received = null; 1409 else { 1410 if (this.received == null) 1411 this.received = new DateTimeType(); 1412 this.received.setValue(value); 1413 } 1414 return this; 1415 } 1416 1417 /** 1418 * @return {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1419 */ 1420 public Reference getSender() { 1421 if (this.sender == null) 1422 if (Configuration.errorOnAutoCreate()) 1423 throw new Error("Attempt to auto-create Communication.sender"); 1424 else if (Configuration.doAutoCreate()) 1425 this.sender = new Reference(); // cc 1426 return this.sender; 1427 } 1428 1429 public boolean hasSender() { 1430 return this.sender != null && !this.sender.isEmpty(); 1431 } 1432 1433 /** 1434 * @param value {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1435 */ 1436 public Communication setSender(Reference value) { 1437 this.sender = value; 1438 return this; 1439 } 1440 1441 /** 1442 * @return {@link #sender} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1443 */ 1444 public Resource getSenderTarget() { 1445 return this.senderTarget; 1446 } 1447 1448 /** 1449 * @param value {@link #sender} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.) 1450 */ 1451 public Communication setSenderTarget(Resource value) { 1452 this.senderTarget = value; 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #reasonCode} (The reason or justification for the communication.) 1458 */ 1459 public List<CodeableConcept> getReasonCode() { 1460 if (this.reasonCode == null) 1461 this.reasonCode = new ArrayList<CodeableConcept>(); 1462 return this.reasonCode; 1463 } 1464 1465 /** 1466 * @return Returns a reference to <code>this</code> for easy method chaining 1467 */ 1468 public Communication setReasonCode(List<CodeableConcept> theReasonCode) { 1469 this.reasonCode = theReasonCode; 1470 return this; 1471 } 1472 1473 public boolean hasReasonCode() { 1474 if (this.reasonCode == null) 1475 return false; 1476 for (CodeableConcept item : this.reasonCode) 1477 if (!item.isEmpty()) 1478 return true; 1479 return false; 1480 } 1481 1482 public CodeableConcept addReasonCode() { //3 1483 CodeableConcept t = new CodeableConcept(); 1484 if (this.reasonCode == null) 1485 this.reasonCode = new ArrayList<CodeableConcept>(); 1486 this.reasonCode.add(t); 1487 return t; 1488 } 1489 1490 public Communication addReasonCode(CodeableConcept t) { //3 1491 if (t == null) 1492 return this; 1493 if (this.reasonCode == null) 1494 this.reasonCode = new ArrayList<CodeableConcept>(); 1495 this.reasonCode.add(t); 1496 return this; 1497 } 1498 1499 /** 1500 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1501 */ 1502 public CodeableConcept getReasonCodeFirstRep() { 1503 if (getReasonCode().isEmpty()) { 1504 addReasonCode(); 1505 } 1506 return getReasonCode().get(0); 1507 } 1508 1509 /** 1510 * @return {@link #reasonReference} (Indicates another resource whose existence justifies this communication.) 1511 */ 1512 public List<Reference> getReasonReference() { 1513 if (this.reasonReference == null) 1514 this.reasonReference = new ArrayList<Reference>(); 1515 return this.reasonReference; 1516 } 1517 1518 /** 1519 * @return Returns a reference to <code>this</code> for easy method chaining 1520 */ 1521 public Communication setReasonReference(List<Reference> theReasonReference) { 1522 this.reasonReference = theReasonReference; 1523 return this; 1524 } 1525 1526 public boolean hasReasonReference() { 1527 if (this.reasonReference == null) 1528 return false; 1529 for (Reference item : this.reasonReference) 1530 if (!item.isEmpty()) 1531 return true; 1532 return false; 1533 } 1534 1535 public Reference addReasonReference() { //3 1536 Reference t = new Reference(); 1537 if (this.reasonReference == null) 1538 this.reasonReference = new ArrayList<Reference>(); 1539 this.reasonReference.add(t); 1540 return t; 1541 } 1542 1543 public Communication addReasonReference(Reference t) { //3 1544 if (t == null) 1545 return this; 1546 if (this.reasonReference == null) 1547 this.reasonReference = new ArrayList<Reference>(); 1548 this.reasonReference.add(t); 1549 return this; 1550 } 1551 1552 /** 1553 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1554 */ 1555 public Reference getReasonReferenceFirstRep() { 1556 if (getReasonReference().isEmpty()) { 1557 addReasonReference(); 1558 } 1559 return getReasonReference().get(0); 1560 } 1561 1562 /** 1563 * @deprecated Use Reference#setResource(IBaseResource) instead 1564 */ 1565 @Deprecated 1566 public List<Resource> getReasonReferenceTarget() { 1567 if (this.reasonReferenceTarget == null) 1568 this.reasonReferenceTarget = new ArrayList<Resource>(); 1569 return this.reasonReferenceTarget; 1570 } 1571 1572 /** 1573 * @return {@link #payload} (Text, attachment(s), or resource(s) that was communicated to the recipient.) 1574 */ 1575 public List<CommunicationPayloadComponent> getPayload() { 1576 if (this.payload == null) 1577 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1578 return this.payload; 1579 } 1580 1581 /** 1582 * @return Returns a reference to <code>this</code> for easy method chaining 1583 */ 1584 public Communication setPayload(List<CommunicationPayloadComponent> thePayload) { 1585 this.payload = thePayload; 1586 return this; 1587 } 1588 1589 public boolean hasPayload() { 1590 if (this.payload == null) 1591 return false; 1592 for (CommunicationPayloadComponent item : this.payload) 1593 if (!item.isEmpty()) 1594 return true; 1595 return false; 1596 } 1597 1598 public CommunicationPayloadComponent addPayload() { //3 1599 CommunicationPayloadComponent t = new CommunicationPayloadComponent(); 1600 if (this.payload == null) 1601 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1602 this.payload.add(t); 1603 return t; 1604 } 1605 1606 public Communication addPayload(CommunicationPayloadComponent t) { //3 1607 if (t == null) 1608 return this; 1609 if (this.payload == null) 1610 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1611 this.payload.add(t); 1612 return this; 1613 } 1614 1615 /** 1616 * @return The first repetition of repeating field {@link #payload}, creating it if it does not already exist 1617 */ 1618 public CommunicationPayloadComponent getPayloadFirstRep() { 1619 if (getPayload().isEmpty()) { 1620 addPayload(); 1621 } 1622 return getPayload().get(0); 1623 } 1624 1625 /** 1626 * @return {@link #note} (Additional notes or commentary about the communication by the sender, receiver or other interested parties.) 1627 */ 1628 public List<Annotation> getNote() { 1629 if (this.note == null) 1630 this.note = new ArrayList<Annotation>(); 1631 return this.note; 1632 } 1633 1634 /** 1635 * @return Returns a reference to <code>this</code> for easy method chaining 1636 */ 1637 public Communication setNote(List<Annotation> theNote) { 1638 this.note = theNote; 1639 return this; 1640 } 1641 1642 public boolean hasNote() { 1643 if (this.note == null) 1644 return false; 1645 for (Annotation item : this.note) 1646 if (!item.isEmpty()) 1647 return true; 1648 return false; 1649 } 1650 1651 public Annotation addNote() { //3 1652 Annotation t = new Annotation(); 1653 if (this.note == null) 1654 this.note = new ArrayList<Annotation>(); 1655 this.note.add(t); 1656 return t; 1657 } 1658 1659 public Communication addNote(Annotation t) { //3 1660 if (t == null) 1661 return this; 1662 if (this.note == null) 1663 this.note = new ArrayList<Annotation>(); 1664 this.note.add(t); 1665 return this; 1666 } 1667 1668 /** 1669 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1670 */ 1671 public Annotation getNoteFirstRep() { 1672 if (getNote().isEmpty()) { 1673 addNote(); 1674 } 1675 return getNote().get(0); 1676 } 1677 1678 protected void listChildren(List<Property> children) { 1679 super.listChildren(children); 1680 children.add(new Property("identifier", "Identifier", "Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 1681 children.add(new Property("definition", "Reference(PlanDefinition|ActivityDefinition)", "A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.", 0, java.lang.Integer.MAX_VALUE, definition)); 1682 children.add(new Property("basedOn", "Reference(Any)", "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1683 children.add(new Property("partOf", "Reference(Any)", "Part of this action.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1684 children.add(new Property("status", "code", "The status of the transmission.", 0, 1, status)); 1685 children.add(new Property("notDone", "boolean", "If true, indicates that the described communication event did not actually occur.", 0, 1, notDone)); 1686 children.add(new Property("notDoneReason", "CodeableConcept", "Describes why the communication event did not occur in coded and/or textual form.", 0, 1, notDoneReason)); 1687 children.add(new Property("category", "CodeableConcept", "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 1688 children.add(new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 1689 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group that was the focus of this communication.", 0, 1, subject)); 1690 children.add(new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson|Group)", "The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).", 0, java.lang.Integer.MAX_VALUE, recipient)); 1691 children.add(new Property("topic", "Reference(Any)", "The resources which were responsible for or related to producing this communication.", 0, java.lang.Integer.MAX_VALUE, topic)); 1692 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter within which the communication was sent.", 0, 1, context)); 1693 children.add(new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent)); 1694 children.add(new Property("received", "dateTime", "The time when this communication arrived at the destination.", 0, 1, received)); 1695 children.add(new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.", 0, 1, sender)); 1696 children.add(new Property("reasonCode", "CodeableConcept", "The reason or justification for the communication.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1697 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this communication.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1698 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload)); 1699 children.add(new Property("note", "Annotation", "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 0, java.lang.Integer.MAX_VALUE, note)); 1700 } 1701 1702 @Override 1703 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1704 switch (_hash) { 1705 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 1706 case -1014418093: /*definition*/ return new Property("definition", "Reference(PlanDefinition|ActivityDefinition)", "A protocol, guideline, or other definition that was adhered to in whole or in part by this communication event.", 0, java.lang.Integer.MAX_VALUE, definition); 1707 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1708 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Any)", "Part of this action.", 0, java.lang.Integer.MAX_VALUE, partOf); 1709 case -892481550: /*status*/ return new Property("status", "code", "The status of the transmission.", 0, 1, status); 1710 case 2128257269: /*notDone*/ return new Property("notDone", "boolean", "If true, indicates that the described communication event did not actually occur.", 0, 1, notDone); 1711 case -1973169255: /*notDoneReason*/ return new Property("notDoneReason", "CodeableConcept", "Describes why the communication event did not occur in coded and/or textual form.", 0, 1, notDoneReason); 1712 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category); 1713 case -1078030475: /*medium*/ return new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 1714 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group that was the focus of this communication.", 0, 1, subject); 1715 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson|Group)", "The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).", 0, java.lang.Integer.MAX_VALUE, recipient); 1716 case 110546223: /*topic*/ return new Property("topic", "Reference(Any)", "The resources which were responsible for or related to producing this communication.", 0, java.lang.Integer.MAX_VALUE, topic); 1717 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter within which the communication was sent.", 0, 1, context); 1718 case 3526552: /*sent*/ return new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent); 1719 case -808719903: /*received*/ return new Property("received", "dateTime", "The time when this communication arrived at the destination.", 0, 1, received); 1720 case -905962955: /*sender*/ return new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.", 0, 1, sender); 1721 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "The reason or justification for the communication.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1722 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this communication.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1723 case -786701938: /*payload*/ return new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload); 1724 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 0, java.lang.Integer.MAX_VALUE, note); 1725 default: return super.getNamedProperty(_hash, _name, _checkValid); 1726 } 1727 1728 } 1729 1730 @Override 1731 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1732 switch (hash) { 1733 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1734 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 1735 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1736 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1737 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CommunicationStatus> 1738 case 2128257269: /*notDone*/ return this.notDone == null ? new Base[0] : new Base[] {this.notDone}; // BooleanType 1739 case -1973169255: /*notDoneReason*/ return this.notDoneReason == null ? new Base[0] : new Base[] {this.notDoneReason}; // CodeableConcept 1740 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1741 case -1078030475: /*medium*/ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 1742 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1743 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1744 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // Reference 1745 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1746 case 3526552: /*sent*/ return this.sent == null ? new Base[0] : new Base[] {this.sent}; // DateTimeType 1747 case -808719903: /*received*/ return this.received == null ? new Base[0] : new Base[] {this.received}; // DateTimeType 1748 case -905962955: /*sender*/ return this.sender == null ? new Base[0] : new Base[] {this.sender}; // Reference 1749 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1750 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1751 case -786701938: /*payload*/ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationPayloadComponent 1752 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1753 default: return super.getProperty(hash, name, checkValid); 1754 } 1755 1756 } 1757 1758 @Override 1759 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1760 switch (hash) { 1761 case -1618432855: // identifier 1762 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1763 return value; 1764 case -1014418093: // definition 1765 this.getDefinition().add(castToReference(value)); // Reference 1766 return value; 1767 case -332612366: // basedOn 1768 this.getBasedOn().add(castToReference(value)); // Reference 1769 return value; 1770 case -995410646: // partOf 1771 this.getPartOf().add(castToReference(value)); // Reference 1772 return value; 1773 case -892481550: // status 1774 value = new CommunicationStatusEnumFactory().fromType(castToCode(value)); 1775 this.status = (Enumeration) value; // Enumeration<CommunicationStatus> 1776 return value; 1777 case 2128257269: // notDone 1778 this.notDone = castToBoolean(value); // BooleanType 1779 return value; 1780 case -1973169255: // notDoneReason 1781 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 1782 return value; 1783 case 50511102: // category 1784 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1785 return value; 1786 case -1078030475: // medium 1787 this.getMedium().add(castToCodeableConcept(value)); // CodeableConcept 1788 return value; 1789 case -1867885268: // subject 1790 this.subject = castToReference(value); // Reference 1791 return value; 1792 case 820081177: // recipient 1793 this.getRecipient().add(castToReference(value)); // Reference 1794 return value; 1795 case 110546223: // topic 1796 this.getTopic().add(castToReference(value)); // Reference 1797 return value; 1798 case 951530927: // context 1799 this.context = castToReference(value); // Reference 1800 return value; 1801 case 3526552: // sent 1802 this.sent = castToDateTime(value); // DateTimeType 1803 return value; 1804 case -808719903: // received 1805 this.received = castToDateTime(value); // DateTimeType 1806 return value; 1807 case -905962955: // sender 1808 this.sender = castToReference(value); // Reference 1809 return value; 1810 case 722137681: // reasonCode 1811 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1812 return value; 1813 case -1146218137: // reasonReference 1814 this.getReasonReference().add(castToReference(value)); // Reference 1815 return value; 1816 case -786701938: // payload 1817 this.getPayload().add((CommunicationPayloadComponent) value); // CommunicationPayloadComponent 1818 return value; 1819 case 3387378: // note 1820 this.getNote().add(castToAnnotation(value)); // Annotation 1821 return value; 1822 default: return super.setProperty(hash, name, value); 1823 } 1824 1825 } 1826 1827 @Override 1828 public Base setProperty(String name, Base value) throws FHIRException { 1829 if (name.equals("identifier")) { 1830 this.getIdentifier().add(castToIdentifier(value)); 1831 } else if (name.equals("definition")) { 1832 this.getDefinition().add(castToReference(value)); 1833 } else if (name.equals("basedOn")) { 1834 this.getBasedOn().add(castToReference(value)); 1835 } else if (name.equals("partOf")) { 1836 this.getPartOf().add(castToReference(value)); 1837 } else if (name.equals("status")) { 1838 value = new CommunicationStatusEnumFactory().fromType(castToCode(value)); 1839 this.status = (Enumeration) value; // Enumeration<CommunicationStatus> 1840 } else if (name.equals("notDone")) { 1841 this.notDone = castToBoolean(value); // BooleanType 1842 } else if (name.equals("notDoneReason")) { 1843 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 1844 } else if (name.equals("category")) { 1845 this.getCategory().add(castToCodeableConcept(value)); 1846 } else if (name.equals("medium")) { 1847 this.getMedium().add(castToCodeableConcept(value)); 1848 } else if (name.equals("subject")) { 1849 this.subject = castToReference(value); // Reference 1850 } else if (name.equals("recipient")) { 1851 this.getRecipient().add(castToReference(value)); 1852 } else if (name.equals("topic")) { 1853 this.getTopic().add(castToReference(value)); 1854 } else if (name.equals("context")) { 1855 this.context = castToReference(value); // Reference 1856 } else if (name.equals("sent")) { 1857 this.sent = castToDateTime(value); // DateTimeType 1858 } else if (name.equals("received")) { 1859 this.received = castToDateTime(value); // DateTimeType 1860 } else if (name.equals("sender")) { 1861 this.sender = castToReference(value); // Reference 1862 } else if (name.equals("reasonCode")) { 1863 this.getReasonCode().add(castToCodeableConcept(value)); 1864 } else if (name.equals("reasonReference")) { 1865 this.getReasonReference().add(castToReference(value)); 1866 } else if (name.equals("payload")) { 1867 this.getPayload().add((CommunicationPayloadComponent) value); 1868 } else if (name.equals("note")) { 1869 this.getNote().add(castToAnnotation(value)); 1870 } else 1871 return super.setProperty(name, value); 1872 return value; 1873 } 1874 1875 @Override 1876 public Base makeProperty(int hash, String name) throws FHIRException { 1877 switch (hash) { 1878 case -1618432855: return addIdentifier(); 1879 case -1014418093: return addDefinition(); 1880 case -332612366: return addBasedOn(); 1881 case -995410646: return addPartOf(); 1882 case -892481550: return getStatusElement(); 1883 case 2128257269: return getNotDoneElement(); 1884 case -1973169255: return getNotDoneReason(); 1885 case 50511102: return addCategory(); 1886 case -1078030475: return addMedium(); 1887 case -1867885268: return getSubject(); 1888 case 820081177: return addRecipient(); 1889 case 110546223: return addTopic(); 1890 case 951530927: return getContext(); 1891 case 3526552: return getSentElement(); 1892 case -808719903: return getReceivedElement(); 1893 case -905962955: return getSender(); 1894 case 722137681: return addReasonCode(); 1895 case -1146218137: return addReasonReference(); 1896 case -786701938: return addPayload(); 1897 case 3387378: return addNote(); 1898 default: return super.makeProperty(hash, name); 1899 } 1900 1901 } 1902 1903 @Override 1904 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1905 switch (hash) { 1906 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1907 case -1014418093: /*definition*/ return new String[] {"Reference"}; 1908 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1909 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1910 case -892481550: /*status*/ return new String[] {"code"}; 1911 case 2128257269: /*notDone*/ return new String[] {"boolean"}; 1912 case -1973169255: /*notDoneReason*/ return new String[] {"CodeableConcept"}; 1913 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1914 case -1078030475: /*medium*/ return new String[] {"CodeableConcept"}; 1915 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1916 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1917 case 110546223: /*topic*/ return new String[] {"Reference"}; 1918 case 951530927: /*context*/ return new String[] {"Reference"}; 1919 case 3526552: /*sent*/ return new String[] {"dateTime"}; 1920 case -808719903: /*received*/ return new String[] {"dateTime"}; 1921 case -905962955: /*sender*/ return new String[] {"Reference"}; 1922 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1923 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 1924 case -786701938: /*payload*/ return new String[] {}; 1925 case 3387378: /*note*/ return new String[] {"Annotation"}; 1926 default: return super.getTypesForProperty(hash, name); 1927 } 1928 1929 } 1930 1931 @Override 1932 public Base addChild(String name) throws FHIRException { 1933 if (name.equals("identifier")) { 1934 return addIdentifier(); 1935 } 1936 else if (name.equals("definition")) { 1937 return addDefinition(); 1938 } 1939 else if (name.equals("basedOn")) { 1940 return addBasedOn(); 1941 } 1942 else if (name.equals("partOf")) { 1943 return addPartOf(); 1944 } 1945 else if (name.equals("status")) { 1946 throw new FHIRException("Cannot call addChild on a singleton property Communication.status"); 1947 } 1948 else if (name.equals("notDone")) { 1949 throw new FHIRException("Cannot call addChild on a singleton property Communication.notDone"); 1950 } 1951 else if (name.equals("notDoneReason")) { 1952 this.notDoneReason = new CodeableConcept(); 1953 return this.notDoneReason; 1954 } 1955 else if (name.equals("category")) { 1956 return addCategory(); 1957 } 1958 else if (name.equals("medium")) { 1959 return addMedium(); 1960 } 1961 else if (name.equals("subject")) { 1962 this.subject = new Reference(); 1963 return this.subject; 1964 } 1965 else if (name.equals("recipient")) { 1966 return addRecipient(); 1967 } 1968 else if (name.equals("topic")) { 1969 return addTopic(); 1970 } 1971 else if (name.equals("context")) { 1972 this.context = new Reference(); 1973 return this.context; 1974 } 1975 else if (name.equals("sent")) { 1976 throw new FHIRException("Cannot call addChild on a singleton property Communication.sent"); 1977 } 1978 else if (name.equals("received")) { 1979 throw new FHIRException("Cannot call addChild on a singleton property Communication.received"); 1980 } 1981 else if (name.equals("sender")) { 1982 this.sender = new Reference(); 1983 return this.sender; 1984 } 1985 else if (name.equals("reasonCode")) { 1986 return addReasonCode(); 1987 } 1988 else if (name.equals("reasonReference")) { 1989 return addReasonReference(); 1990 } 1991 else if (name.equals("payload")) { 1992 return addPayload(); 1993 } 1994 else if (name.equals("note")) { 1995 return addNote(); 1996 } 1997 else 1998 return super.addChild(name); 1999 } 2000 2001 public String fhirType() { 2002 return "Communication"; 2003 2004 } 2005 2006 public Communication copy() { 2007 Communication dst = new Communication(); 2008 copyValues(dst); 2009 if (identifier != null) { 2010 dst.identifier = new ArrayList<Identifier>(); 2011 for (Identifier i : identifier) 2012 dst.identifier.add(i.copy()); 2013 }; 2014 if (definition != null) { 2015 dst.definition = new ArrayList<Reference>(); 2016 for (Reference i : definition) 2017 dst.definition.add(i.copy()); 2018 }; 2019 if (basedOn != null) { 2020 dst.basedOn = new ArrayList<Reference>(); 2021 for (Reference i : basedOn) 2022 dst.basedOn.add(i.copy()); 2023 }; 2024 if (partOf != null) { 2025 dst.partOf = new ArrayList<Reference>(); 2026 for (Reference i : partOf) 2027 dst.partOf.add(i.copy()); 2028 }; 2029 dst.status = status == null ? null : status.copy(); 2030 dst.notDone = notDone == null ? null : notDone.copy(); 2031 dst.notDoneReason = notDoneReason == null ? null : notDoneReason.copy(); 2032 if (category != null) { 2033 dst.category = new ArrayList<CodeableConcept>(); 2034 for (CodeableConcept i : category) 2035 dst.category.add(i.copy()); 2036 }; 2037 if (medium != null) { 2038 dst.medium = new ArrayList<CodeableConcept>(); 2039 for (CodeableConcept i : medium) 2040 dst.medium.add(i.copy()); 2041 }; 2042 dst.subject = subject == null ? null : subject.copy(); 2043 if (recipient != null) { 2044 dst.recipient = new ArrayList<Reference>(); 2045 for (Reference i : recipient) 2046 dst.recipient.add(i.copy()); 2047 }; 2048 if (topic != null) { 2049 dst.topic = new ArrayList<Reference>(); 2050 for (Reference i : topic) 2051 dst.topic.add(i.copy()); 2052 }; 2053 dst.context = context == null ? null : context.copy(); 2054 dst.sent = sent == null ? null : sent.copy(); 2055 dst.received = received == null ? null : received.copy(); 2056 dst.sender = sender == null ? null : sender.copy(); 2057 if (reasonCode != null) { 2058 dst.reasonCode = new ArrayList<CodeableConcept>(); 2059 for (CodeableConcept i : reasonCode) 2060 dst.reasonCode.add(i.copy()); 2061 }; 2062 if (reasonReference != null) { 2063 dst.reasonReference = new ArrayList<Reference>(); 2064 for (Reference i : reasonReference) 2065 dst.reasonReference.add(i.copy()); 2066 }; 2067 if (payload != null) { 2068 dst.payload = new ArrayList<CommunicationPayloadComponent>(); 2069 for (CommunicationPayloadComponent i : payload) 2070 dst.payload.add(i.copy()); 2071 }; 2072 if (note != null) { 2073 dst.note = new ArrayList<Annotation>(); 2074 for (Annotation i : note) 2075 dst.note.add(i.copy()); 2076 }; 2077 return dst; 2078 } 2079 2080 protected Communication typedCopy() { 2081 return copy(); 2082 } 2083 2084 @Override 2085 public boolean equalsDeep(Base other_) { 2086 if (!super.equalsDeep(other_)) 2087 return false; 2088 if (!(other_ instanceof Communication)) 2089 return false; 2090 Communication o = (Communication) other_; 2091 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2092 && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 2093 && compareDeep(notDone, o.notDone, true) && compareDeep(notDoneReason, o.notDoneReason, true) && compareDeep(category, o.category, true) 2094 && compareDeep(medium, o.medium, true) && compareDeep(subject, o.subject, true) && compareDeep(recipient, o.recipient, true) 2095 && compareDeep(topic, o.topic, true) && compareDeep(context, o.context, true) && compareDeep(sent, o.sent, true) 2096 && compareDeep(received, o.received, true) && compareDeep(sender, o.sender, true) && compareDeep(reasonCode, o.reasonCode, true) 2097 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(payload, o.payload, true) 2098 && compareDeep(note, o.note, true); 2099 } 2100 2101 @Override 2102 public boolean equalsShallow(Base other_) { 2103 if (!super.equalsShallow(other_)) 2104 return false; 2105 if (!(other_ instanceof Communication)) 2106 return false; 2107 Communication o = (Communication) other_; 2108 return compareValues(status, o.status, true) && compareValues(notDone, o.notDone, true) && compareValues(sent, o.sent, true) 2109 && compareValues(received, o.received, true); 2110 } 2111 2112 public boolean isEmpty() { 2113 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2114 , partOf, status, notDone, notDoneReason, category, medium, subject, recipient 2115 , topic, context, sent, received, sender, reasonCode, reasonReference, payload 2116 , note); 2117 } 2118 2119 @Override 2120 public ResourceType getResourceType() { 2121 return ResourceType.Communication; 2122 } 2123 2124 /** 2125 * Search parameter: <b>identifier</b> 2126 * <p> 2127 * Description: <b>Unique identifier</b><br> 2128 * Type: <b>token</b><br> 2129 * Path: <b>Communication.identifier</b><br> 2130 * </p> 2131 */ 2132 @SearchParamDefinition(name="identifier", path="Communication.identifier", description="Unique identifier", type="token" ) 2133 public static final String SP_IDENTIFIER = "identifier"; 2134 /** 2135 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2136 * <p> 2137 * Description: <b>Unique identifier</b><br> 2138 * Type: <b>token</b><br> 2139 * Path: <b>Communication.identifier</b><br> 2140 * </p> 2141 */ 2142 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2143 2144 /** 2145 * Search parameter: <b>subject</b> 2146 * <p> 2147 * Description: <b>Focus of message</b><br> 2148 * Type: <b>reference</b><br> 2149 * Path: <b>Communication.subject</b><br> 2150 * </p> 2151 */ 2152 @SearchParamDefinition(name="subject", path="Communication.subject", description="Focus of message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2153 public static final String SP_SUBJECT = "subject"; 2154 /** 2155 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2156 * <p> 2157 * Description: <b>Focus of message</b><br> 2158 * Type: <b>reference</b><br> 2159 * Path: <b>Communication.subject</b><br> 2160 * </p> 2161 */ 2162 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2163 2164/** 2165 * Constant for fluent queries to be used to add include statements. Specifies 2166 * the path value of "<b>Communication:subject</b>". 2167 */ 2168 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Communication:subject").toLocked(); 2169 2170 /** 2171 * Search parameter: <b>received</b> 2172 * <p> 2173 * Description: <b>When received</b><br> 2174 * Type: <b>date</b><br> 2175 * Path: <b>Communication.received</b><br> 2176 * </p> 2177 */ 2178 @SearchParamDefinition(name="received", path="Communication.received", description="When received", type="date" ) 2179 public static final String SP_RECEIVED = "received"; 2180 /** 2181 * <b>Fluent Client</b> search parameter constant for <b>received</b> 2182 * <p> 2183 * Description: <b>When received</b><br> 2184 * Type: <b>date</b><br> 2185 * Path: <b>Communication.received</b><br> 2186 * </p> 2187 */ 2188 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECEIVED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_RECEIVED); 2189 2190 /** 2191 * Search parameter: <b>part-of</b> 2192 * <p> 2193 * Description: <b>Part of this action</b><br> 2194 * Type: <b>reference</b><br> 2195 * Path: <b>Communication.partOf</b><br> 2196 * </p> 2197 */ 2198 @SearchParamDefinition(name="part-of", path="Communication.partOf", description="Part of this action", type="reference" ) 2199 public static final String SP_PART_OF = "part-of"; 2200 /** 2201 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2202 * <p> 2203 * Description: <b>Part of this action</b><br> 2204 * Type: <b>reference</b><br> 2205 * Path: <b>Communication.partOf</b><br> 2206 * </p> 2207 */ 2208 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2209 2210/** 2211 * Constant for fluent queries to be used to add include statements. Specifies 2212 * the path value of "<b>Communication:part-of</b>". 2213 */ 2214 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Communication:part-of").toLocked(); 2215 2216 /** 2217 * Search parameter: <b>medium</b> 2218 * <p> 2219 * Description: <b>A channel of communication</b><br> 2220 * Type: <b>token</b><br> 2221 * Path: <b>Communication.medium</b><br> 2222 * </p> 2223 */ 2224 @SearchParamDefinition(name="medium", path="Communication.medium", description="A channel of communication", type="token" ) 2225 public static final String SP_MEDIUM = "medium"; 2226 /** 2227 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 2228 * <p> 2229 * Description: <b>A channel of communication</b><br> 2230 * Type: <b>token</b><br> 2231 * Path: <b>Communication.medium</b><br> 2232 * </p> 2233 */ 2234 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MEDIUM); 2235 2236 /** 2237 * Search parameter: <b>encounter</b> 2238 * <p> 2239 * Description: <b>Encounter leading to message</b><br> 2240 * Type: <b>reference</b><br> 2241 * Path: <b>Communication.context</b><br> 2242 * </p> 2243 */ 2244 @SearchParamDefinition(name="encounter", path="Communication.context", description="Encounter leading to message", type="reference", target={Encounter.class } ) 2245 public static final String SP_ENCOUNTER = "encounter"; 2246 /** 2247 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2248 * <p> 2249 * Description: <b>Encounter leading to message</b><br> 2250 * Type: <b>reference</b><br> 2251 * Path: <b>Communication.context</b><br> 2252 * </p> 2253 */ 2254 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2255 2256/** 2257 * Constant for fluent queries to be used to add include statements. Specifies 2258 * the path value of "<b>Communication:encounter</b>". 2259 */ 2260 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Communication:encounter").toLocked(); 2261 2262 /** 2263 * Search parameter: <b>sent</b> 2264 * <p> 2265 * Description: <b>When sent</b><br> 2266 * Type: <b>date</b><br> 2267 * Path: <b>Communication.sent</b><br> 2268 * </p> 2269 */ 2270 @SearchParamDefinition(name="sent", path="Communication.sent", description="When sent", type="date" ) 2271 public static final String SP_SENT = "sent"; 2272 /** 2273 * <b>Fluent Client</b> search parameter constant for <b>sent</b> 2274 * <p> 2275 * Description: <b>When sent</b><br> 2276 * Type: <b>date</b><br> 2277 * Path: <b>Communication.sent</b><br> 2278 * </p> 2279 */ 2280 public static final ca.uhn.fhir.rest.gclient.DateClientParam SENT = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_SENT); 2281 2282 /** 2283 * Search parameter: <b>based-on</b> 2284 * <p> 2285 * Description: <b>Request fulfilled by this communication</b><br> 2286 * Type: <b>reference</b><br> 2287 * Path: <b>Communication.basedOn</b><br> 2288 * </p> 2289 */ 2290 @SearchParamDefinition(name="based-on", path="Communication.basedOn", description="Request fulfilled by this communication", type="reference" ) 2291 public static final String SP_BASED_ON = "based-on"; 2292 /** 2293 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2294 * <p> 2295 * Description: <b>Request fulfilled by this communication</b><br> 2296 * Type: <b>reference</b><br> 2297 * Path: <b>Communication.basedOn</b><br> 2298 * </p> 2299 */ 2300 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2301 2302/** 2303 * Constant for fluent queries to be used to add include statements. Specifies 2304 * the path value of "<b>Communication:based-on</b>". 2305 */ 2306 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Communication:based-on").toLocked(); 2307 2308 /** 2309 * Search parameter: <b>sender</b> 2310 * <p> 2311 * Description: <b>Message sender</b><br> 2312 * Type: <b>reference</b><br> 2313 * Path: <b>Communication.sender</b><br> 2314 * </p> 2315 */ 2316 @SearchParamDefinition(name="sender", path="Communication.sender", description="Message sender", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2317 public static final String SP_SENDER = "sender"; 2318 /** 2319 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 2320 * <p> 2321 * Description: <b>Message sender</b><br> 2322 * Type: <b>reference</b><br> 2323 * Path: <b>Communication.sender</b><br> 2324 * </p> 2325 */ 2326 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SENDER); 2327 2328/** 2329 * Constant for fluent queries to be used to add include statements. Specifies 2330 * the path value of "<b>Communication:sender</b>". 2331 */ 2332 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include("Communication:sender").toLocked(); 2333 2334 /** 2335 * Search parameter: <b>patient</b> 2336 * <p> 2337 * Description: <b>Focus of message</b><br> 2338 * Type: <b>reference</b><br> 2339 * Path: <b>Communication.subject</b><br> 2340 * </p> 2341 */ 2342 @SearchParamDefinition(name="patient", path="Communication.subject", description="Focus of message", type="reference", target={Patient.class } ) 2343 public static final String SP_PATIENT = "patient"; 2344 /** 2345 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2346 * <p> 2347 * Description: <b>Focus of message</b><br> 2348 * Type: <b>reference</b><br> 2349 * Path: <b>Communication.subject</b><br> 2350 * </p> 2351 */ 2352 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2353 2354/** 2355 * Constant for fluent queries to be used to add include statements. Specifies 2356 * the path value of "<b>Communication:patient</b>". 2357 */ 2358 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Communication:patient").toLocked(); 2359 2360 /** 2361 * Search parameter: <b>recipient</b> 2362 * <p> 2363 * Description: <b>Message recipient</b><br> 2364 * Type: <b>reference</b><br> 2365 * Path: <b>Communication.recipient</b><br> 2366 * </p> 2367 */ 2368 @SearchParamDefinition(name="recipient", path="Communication.recipient", description="Message recipient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2369 public static final String SP_RECIPIENT = "recipient"; 2370 /** 2371 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 2372 * <p> 2373 * Description: <b>Message recipient</b><br> 2374 * Type: <b>reference</b><br> 2375 * Path: <b>Communication.recipient</b><br> 2376 * </p> 2377 */ 2378 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 2379 2380/** 2381 * Constant for fluent queries to be used to add include statements. Specifies 2382 * the path value of "<b>Communication:recipient</b>". 2383 */ 2384 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("Communication:recipient").toLocked(); 2385 2386 /** 2387 * Search parameter: <b>context</b> 2388 * <p> 2389 * Description: <b>Encounter or episode leading to message</b><br> 2390 * Type: <b>reference</b><br> 2391 * Path: <b>Communication.context</b><br> 2392 * </p> 2393 */ 2394 @SearchParamDefinition(name="context", path="Communication.context", description="Encounter or episode leading to message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2395 public static final String SP_CONTEXT = "context"; 2396 /** 2397 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2398 * <p> 2399 * Description: <b>Encounter or episode leading to message</b><br> 2400 * Type: <b>reference</b><br> 2401 * Path: <b>Communication.context</b><br> 2402 * </p> 2403 */ 2404 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2405 2406/** 2407 * Constant for fluent queries to be used to add include statements. Specifies 2408 * the path value of "<b>Communication:context</b>". 2409 */ 2410 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("Communication:context").toLocked(); 2411 2412 /** 2413 * Search parameter: <b>definition</b> 2414 * <p> 2415 * Description: <b>Instantiates protocol or definition</b><br> 2416 * Type: <b>reference</b><br> 2417 * Path: <b>Communication.definition</b><br> 2418 * </p> 2419 */ 2420 @SearchParamDefinition(name="definition", path="Communication.definition", description="Instantiates protocol or definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 2421 public static final String SP_DEFINITION = "definition"; 2422 /** 2423 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 2424 * <p> 2425 * Description: <b>Instantiates protocol or definition</b><br> 2426 * Type: <b>reference</b><br> 2427 * Path: <b>Communication.definition</b><br> 2428 * </p> 2429 */ 2430 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 2431 2432/** 2433 * Constant for fluent queries to be used to add include statements. Specifies 2434 * the path value of "<b>Communication:definition</b>". 2435 */ 2436 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("Communication:definition").toLocked(); 2437 2438 /** 2439 * Search parameter: <b>category</b> 2440 * <p> 2441 * Description: <b>Message category</b><br> 2442 * Type: <b>token</b><br> 2443 * Path: <b>Communication.category</b><br> 2444 * </p> 2445 */ 2446 @SearchParamDefinition(name="category", path="Communication.category", description="Message category", type="token" ) 2447 public static final String SP_CATEGORY = "category"; 2448 /** 2449 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2450 * <p> 2451 * Description: <b>Message category</b><br> 2452 * Type: <b>token</b><br> 2453 * Path: <b>Communication.category</b><br> 2454 * </p> 2455 */ 2456 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2457 2458 /** 2459 * Search parameter: <b>status</b> 2460 * <p> 2461 * Description: <b>preparation | in-progress | suspended | aborted | completed | entered-in-error</b><br> 2462 * Type: <b>token</b><br> 2463 * Path: <b>Communication.status</b><br> 2464 * </p> 2465 */ 2466 @SearchParamDefinition(name="status", path="Communication.status", description="preparation | in-progress | suspended | aborted | completed | entered-in-error", type="token" ) 2467 public static final String SP_STATUS = "status"; 2468 /** 2469 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2470 * <p> 2471 * Description: <b>preparation | in-progress | suspended | aborted | completed | entered-in-error</b><br> 2472 * Type: <b>token</b><br> 2473 * Path: <b>Communication.status</b><br> 2474 * </p> 2475 */ 2476 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2477 2478 2479}