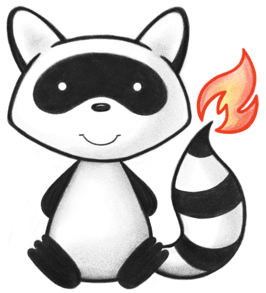
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 050 */ 051@ResourceDef(name="CommunicationRequest", profile="http://hl7.org/fhir/Profile/CommunicationRequest") 052public class CommunicationRequest extends DomainResource { 053 054 public enum CommunicationRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action 057 */ 058 DRAFT, 059 /** 060 * The request is ready to be acted upon 061 */ 062 ACTIVE, 063 /** 064 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 065 */ 066 SUSPENDED, 067 /** 068 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 069 */ 070 CANCELLED, 071 /** 072 * Activity against the request has been sufficiently completed to the satisfaction of the requester 073 */ 074 COMPLETED, 075 /** 076 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static CommunicationRequestStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("draft".equals(codeString)) 091 return DRAFT; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("cancelled".equals(codeString)) 097 return CANCELLED; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown CommunicationRequestStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case DRAFT: return "draft"; 112 case ACTIVE: return "active"; 113 case SUSPENDED: return "suspended"; 114 case CANCELLED: return "cancelled"; 115 case COMPLETED: return "completed"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case DRAFT: return "http://hl7.org/fhir/request-status"; 125 case ACTIVE: return "http://hl7.org/fhir/request-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 127 case CANCELLED: return "http://hl7.org/fhir/request-status"; 128 case COMPLETED: return "http://hl7.org/fhir/request-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 138 case ACTIVE: return "The request is ready to be acted upon"; 139 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 140 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 141 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 142 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 143 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case DRAFT: return "Draft"; 151 case ACTIVE: return "Active"; 152 case SUSPENDED: return "Suspended"; 153 case CANCELLED: return "Cancelled"; 154 case COMPLETED: return "Completed"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class CommunicationRequestStatusEnumFactory implements EnumFactory<CommunicationRequestStatus> { 164 public CommunicationRequestStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("draft".equals(codeString)) 169 return CommunicationRequestStatus.DRAFT; 170 if ("active".equals(codeString)) 171 return CommunicationRequestStatus.ACTIVE; 172 if ("suspended".equals(codeString)) 173 return CommunicationRequestStatus.SUSPENDED; 174 if ("cancelled".equals(codeString)) 175 return CommunicationRequestStatus.CANCELLED; 176 if ("completed".equals(codeString)) 177 return CommunicationRequestStatus.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return CommunicationRequestStatus.ENTEREDINERROR; 180 if ("unknown".equals(codeString)) 181 return CommunicationRequestStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown CommunicationRequestStatus code '"+codeString+"'"); 183 } 184 public Enumeration<CommunicationRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<CommunicationRequestStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("draft".equals(codeString)) 193 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.DRAFT); 194 if ("active".equals(codeString)) 195 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ACTIVE); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.SUSPENDED); 198 if ("cancelled".equals(codeString)) 199 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.CANCELLED); 200 if ("completed".equals(codeString)) 201 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.COMPLETED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ENTEREDINERROR); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.UNKNOWN); 206 throw new FHIRException("Unknown CommunicationRequestStatus code '"+codeString+"'"); 207 } 208 public String toCode(CommunicationRequestStatus code) { 209 if (code == CommunicationRequestStatus.NULL) 210 return null; 211 if (code == CommunicationRequestStatus.DRAFT) 212 return "draft"; 213 if (code == CommunicationRequestStatus.ACTIVE) 214 return "active"; 215 if (code == CommunicationRequestStatus.SUSPENDED) 216 return "suspended"; 217 if (code == CommunicationRequestStatus.CANCELLED) 218 return "cancelled"; 219 if (code == CommunicationRequestStatus.COMPLETED) 220 return "completed"; 221 if (code == CommunicationRequestStatus.ENTEREDINERROR) 222 return "entered-in-error"; 223 if (code == CommunicationRequestStatus.UNKNOWN) 224 return "unknown"; 225 return "?"; 226 } 227 public String toSystem(CommunicationRequestStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 public enum CommunicationPriority { 233 /** 234 * The request has normal priority 235 */ 236 ROUTINE, 237 /** 238 * The request should be actioned promptly - higher priority than routine 239 */ 240 URGENT, 241 /** 242 * The request should be actioned as soon as possible - higher priority than urgent 243 */ 244 ASAP, 245 /** 246 * The request should be actioned immediately - highest possible priority. E.g. an emergency 247 */ 248 STAT, 249 /** 250 * added to help the parsers with the generic types 251 */ 252 NULL; 253 public static CommunicationPriority fromCode(String codeString) throws FHIRException { 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("routine".equals(codeString)) 257 return ROUTINE; 258 if ("urgent".equals(codeString)) 259 return URGENT; 260 if ("asap".equals(codeString)) 261 return ASAP; 262 if ("stat".equals(codeString)) 263 return STAT; 264 if (Configuration.isAcceptInvalidEnums()) 265 return null; 266 else 267 throw new FHIRException("Unknown CommunicationPriority code '"+codeString+"'"); 268 } 269 public String toCode() { 270 switch (this) { 271 case ROUTINE: return "routine"; 272 case URGENT: return "urgent"; 273 case ASAP: return "asap"; 274 case STAT: return "stat"; 275 case NULL: return null; 276 default: return "?"; 277 } 278 } 279 public String getSystem() { 280 switch (this) { 281 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 282 case URGENT: return "http://hl7.org/fhir/request-priority"; 283 case ASAP: return "http://hl7.org/fhir/request-priority"; 284 case STAT: return "http://hl7.org/fhir/request-priority"; 285 case NULL: return null; 286 default: return "?"; 287 } 288 } 289 public String getDefinition() { 290 switch (this) { 291 case ROUTINE: return "The request has normal priority"; 292 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 293 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 294 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 295 case NULL: return null; 296 default: return "?"; 297 } 298 } 299 public String getDisplay() { 300 switch (this) { 301 case ROUTINE: return "Routine"; 302 case URGENT: return "Urgent"; 303 case ASAP: return "ASAP"; 304 case STAT: return "STAT"; 305 case NULL: return null; 306 default: return "?"; 307 } 308 } 309 } 310 311 public static class CommunicationPriorityEnumFactory implements EnumFactory<CommunicationPriority> { 312 public CommunicationPriority fromCode(String codeString) throws IllegalArgumentException { 313 if (codeString == null || "".equals(codeString)) 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("routine".equals(codeString)) 317 return CommunicationPriority.ROUTINE; 318 if ("urgent".equals(codeString)) 319 return CommunicationPriority.URGENT; 320 if ("asap".equals(codeString)) 321 return CommunicationPriority.ASAP; 322 if ("stat".equals(codeString)) 323 return CommunicationPriority.STAT; 324 throw new IllegalArgumentException("Unknown CommunicationPriority code '"+codeString+"'"); 325 } 326 public Enumeration<CommunicationPriority> fromType(PrimitiveType<?> code) throws FHIRException { 327 if (code == null) 328 return null; 329 if (code.isEmpty()) 330 return new Enumeration<CommunicationPriority>(this); 331 String codeString = code.asStringValue(); 332 if (codeString == null || "".equals(codeString)) 333 return null; 334 if ("routine".equals(codeString)) 335 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ROUTINE); 336 if ("urgent".equals(codeString)) 337 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.URGENT); 338 if ("asap".equals(codeString)) 339 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ASAP); 340 if ("stat".equals(codeString)) 341 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.STAT); 342 throw new FHIRException("Unknown CommunicationPriority code '"+codeString+"'"); 343 } 344 public String toCode(CommunicationPriority code) { 345 if (code == CommunicationPriority.NULL) 346 return null; 347 if (code == CommunicationPriority.ROUTINE) 348 return "routine"; 349 if (code == CommunicationPriority.URGENT) 350 return "urgent"; 351 if (code == CommunicationPriority.ASAP) 352 return "asap"; 353 if (code == CommunicationPriority.STAT) 354 return "stat"; 355 return "?"; 356 } 357 public String toSystem(CommunicationPriority code) { 358 return code.getSystem(); 359 } 360 } 361 362 @Block() 363 public static class CommunicationRequestPayloadComponent extends BackboneElement implements IBaseBackboneElement { 364 /** 365 * The communicated content (or for multi-part communications, one portion of the communication). 366 */ 367 @Child(name = "content", type = {StringType.class, Attachment.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 368 @Description(shortDefinition="Message part content", formalDefinition="The communicated content (or for multi-part communications, one portion of the communication)." ) 369 protected Type content; 370 371 private static final long serialVersionUID = -1763459053L; 372 373 /** 374 * Constructor 375 */ 376 public CommunicationRequestPayloadComponent() { 377 super(); 378 } 379 380 /** 381 * Constructor 382 */ 383 public CommunicationRequestPayloadComponent(Type content) { 384 super(); 385 this.content = content; 386 } 387 388 /** 389 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 390 */ 391 public Type getContent() { 392 return this.content; 393 } 394 395 /** 396 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 397 */ 398 public StringType getContentStringType() throws FHIRException { 399 if (this.content == null) 400 return null; 401 if (!(this.content instanceof StringType)) 402 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.content.getClass().getName()+" was encountered"); 403 return (StringType) this.content; 404 } 405 406 public boolean hasContentStringType() { 407 return this != null && this.content instanceof StringType; 408 } 409 410 /** 411 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 412 */ 413 public Attachment getContentAttachment() throws FHIRException { 414 if (this.content == null) 415 return null; 416 if (!(this.content instanceof Attachment)) 417 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 418 return (Attachment) this.content; 419 } 420 421 public boolean hasContentAttachment() { 422 return this != null && this.content instanceof Attachment; 423 } 424 425 /** 426 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 427 */ 428 public Reference getContentReference() throws FHIRException { 429 if (this.content == null) 430 return null; 431 if (!(this.content instanceof Reference)) 432 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 433 return (Reference) this.content; 434 } 435 436 public boolean hasContentReference() { 437 return this != null && this.content instanceof Reference; 438 } 439 440 public boolean hasContent() { 441 return this.content != null && !this.content.isEmpty(); 442 } 443 444 /** 445 * @param value {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 446 */ 447 public CommunicationRequestPayloadComponent setContent(Type value) throws FHIRFormatError { 448 if (value != null && !(value instanceof StringType || value instanceof Attachment || value instanceof Reference)) 449 throw new FHIRFormatError("Not the right type for CommunicationRequest.payload.content[x]: "+value.fhirType()); 450 this.content = value; 451 return this; 452 } 453 454 protected void listChildren(List<Property> children) { 455 super.listChildren(children); 456 children.add(new Property("content[x]", "string|Attachment|Reference(Any)", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content)); 457 } 458 459 @Override 460 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 461 switch (_hash) { 462 case 264548711: /*content[x]*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 463 case 951530617: /*content*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 464 case -326336022: /*contentString*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 465 case -702028164: /*contentAttachment*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 466 case 1193747154: /*contentReference*/ return new Property("content[x]", "string|Attachment|Reference(Any)", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 467 default: return super.getNamedProperty(_hash, _name, _checkValid); 468 } 469 470 } 471 472 @Override 473 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 474 switch (hash) { 475 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Type 476 default: return super.getProperty(hash, name, checkValid); 477 } 478 479 } 480 481 @Override 482 public Base setProperty(int hash, String name, Base value) throws FHIRException { 483 switch (hash) { 484 case 951530617: // content 485 this.content = castToType(value); // Type 486 return value; 487 default: return super.setProperty(hash, name, value); 488 } 489 490 } 491 492 @Override 493 public Base setProperty(String name, Base value) throws FHIRException { 494 if (name.equals("content[x]")) { 495 this.content = castToType(value); // Type 496 } else 497 return super.setProperty(name, value); 498 return value; 499 } 500 501 @Override 502 public Base makeProperty(int hash, String name) throws FHIRException { 503 switch (hash) { 504 case 264548711: return getContent(); 505 case 951530617: return getContent(); 506 default: return super.makeProperty(hash, name); 507 } 508 509 } 510 511 @Override 512 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 513 switch (hash) { 514 case 951530617: /*content*/ return new String[] {"string", "Attachment", "Reference"}; 515 default: return super.getTypesForProperty(hash, name); 516 } 517 518 } 519 520 @Override 521 public Base addChild(String name) throws FHIRException { 522 if (name.equals("contentString")) { 523 this.content = new StringType(); 524 return this.content; 525 } 526 else if (name.equals("contentAttachment")) { 527 this.content = new Attachment(); 528 return this.content; 529 } 530 else if (name.equals("contentReference")) { 531 this.content = new Reference(); 532 return this.content; 533 } 534 else 535 return super.addChild(name); 536 } 537 538 public CommunicationRequestPayloadComponent copy() { 539 CommunicationRequestPayloadComponent dst = new CommunicationRequestPayloadComponent(); 540 copyValues(dst); 541 dst.content = content == null ? null : content.copy(); 542 return dst; 543 } 544 545 @Override 546 public boolean equalsDeep(Base other_) { 547 if (!super.equalsDeep(other_)) 548 return false; 549 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 550 return false; 551 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 552 return compareDeep(content, o.content, true); 553 } 554 555 @Override 556 public boolean equalsShallow(Base other_) { 557 if (!super.equalsShallow(other_)) 558 return false; 559 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 560 return false; 561 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 562 return true; 563 } 564 565 public boolean isEmpty() { 566 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 567 } 568 569 public String fhirType() { 570 return "CommunicationRequest.payload"; 571 572 } 573 574 } 575 576 @Block() 577 public static class CommunicationRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 578 /** 579 * The device, practitioner, etc. who initiated the request. 580 */ 581 @Child(name = "agent", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 582 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner, etc. who initiated the request." ) 583 protected Reference agent; 584 585 /** 586 * The actual object that is the target of the reference (The device, practitioner, etc. who initiated the request.) 587 */ 588 protected Resource agentTarget; 589 590 /** 591 * The organization the device or practitioner was acting on behalf of. 592 */ 593 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 594 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 595 protected Reference onBehalfOf; 596 597 /** 598 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 599 */ 600 protected Organization onBehalfOfTarget; 601 602 private static final long serialVersionUID = -71453027L; 603 604 /** 605 * Constructor 606 */ 607 public CommunicationRequestRequesterComponent() { 608 super(); 609 } 610 611 /** 612 * Constructor 613 */ 614 public CommunicationRequestRequesterComponent(Reference agent) { 615 super(); 616 this.agent = agent; 617 } 618 619 /** 620 * @return {@link #agent} (The device, practitioner, etc. who initiated the request.) 621 */ 622 public Reference getAgent() { 623 if (this.agent == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create CommunicationRequestRequesterComponent.agent"); 626 else if (Configuration.doAutoCreate()) 627 this.agent = new Reference(); // cc 628 return this.agent; 629 } 630 631 public boolean hasAgent() { 632 return this.agent != null && !this.agent.isEmpty(); 633 } 634 635 /** 636 * @param value {@link #agent} (The device, practitioner, etc. who initiated the request.) 637 */ 638 public CommunicationRequestRequesterComponent setAgent(Reference value) { 639 this.agent = value; 640 return this; 641 } 642 643 /** 644 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 645 */ 646 public Resource getAgentTarget() { 647 return this.agentTarget; 648 } 649 650 /** 651 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 652 */ 653 public CommunicationRequestRequesterComponent setAgentTarget(Resource value) { 654 this.agentTarget = value; 655 return this; 656 } 657 658 /** 659 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 660 */ 661 public Reference getOnBehalfOf() { 662 if (this.onBehalfOf == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create CommunicationRequestRequesterComponent.onBehalfOf"); 665 else if (Configuration.doAutoCreate()) 666 this.onBehalfOf = new Reference(); // cc 667 return this.onBehalfOf; 668 } 669 670 public boolean hasOnBehalfOf() { 671 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 672 } 673 674 /** 675 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 676 */ 677 public CommunicationRequestRequesterComponent setOnBehalfOf(Reference value) { 678 this.onBehalfOf = value; 679 return this; 680 } 681 682 /** 683 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 684 */ 685 public Organization getOnBehalfOfTarget() { 686 if (this.onBehalfOfTarget == null) 687 if (Configuration.errorOnAutoCreate()) 688 throw new Error("Attempt to auto-create CommunicationRequestRequesterComponent.onBehalfOf"); 689 else if (Configuration.doAutoCreate()) 690 this.onBehalfOfTarget = new Organization(); // aa 691 return this.onBehalfOfTarget; 692 } 693 694 /** 695 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 696 */ 697 public CommunicationRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 698 this.onBehalfOfTarget = value; 699 return this; 700 } 701 702 protected void listChildren(List<Property> children) { 703 super.listChildren(children); 704 children.add(new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent)); 705 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 706 } 707 708 @Override 709 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 710 switch (_hash) { 711 case 92750597: /*agent*/ return new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent); 712 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 713 default: return super.getNamedProperty(_hash, _name, _checkValid); 714 } 715 716 } 717 718 @Override 719 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 720 switch (hash) { 721 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 722 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 723 default: return super.getProperty(hash, name, checkValid); 724 } 725 726 } 727 728 @Override 729 public Base setProperty(int hash, String name, Base value) throws FHIRException { 730 switch (hash) { 731 case 92750597: // agent 732 this.agent = castToReference(value); // Reference 733 return value; 734 case -14402964: // onBehalfOf 735 this.onBehalfOf = castToReference(value); // Reference 736 return value; 737 default: return super.setProperty(hash, name, value); 738 } 739 740 } 741 742 @Override 743 public Base setProperty(String name, Base value) throws FHIRException { 744 if (name.equals("agent")) { 745 this.agent = castToReference(value); // Reference 746 } else if (name.equals("onBehalfOf")) { 747 this.onBehalfOf = castToReference(value); // Reference 748 } else 749 return super.setProperty(name, value); 750 return value; 751 } 752 753 @Override 754 public Base makeProperty(int hash, String name) throws FHIRException { 755 switch (hash) { 756 case 92750597: return getAgent(); 757 case -14402964: return getOnBehalfOf(); 758 default: return super.makeProperty(hash, name); 759 } 760 761 } 762 763 @Override 764 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 765 switch (hash) { 766 case 92750597: /*agent*/ return new String[] {"Reference"}; 767 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 768 default: return super.getTypesForProperty(hash, name); 769 } 770 771 } 772 773 @Override 774 public Base addChild(String name) throws FHIRException { 775 if (name.equals("agent")) { 776 this.agent = new Reference(); 777 return this.agent; 778 } 779 else if (name.equals("onBehalfOf")) { 780 this.onBehalfOf = new Reference(); 781 return this.onBehalfOf; 782 } 783 else 784 return super.addChild(name); 785 } 786 787 public CommunicationRequestRequesterComponent copy() { 788 CommunicationRequestRequesterComponent dst = new CommunicationRequestRequesterComponent(); 789 copyValues(dst); 790 dst.agent = agent == null ? null : agent.copy(); 791 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 792 return dst; 793 } 794 795 @Override 796 public boolean equalsDeep(Base other_) { 797 if (!super.equalsDeep(other_)) 798 return false; 799 if (!(other_ instanceof CommunicationRequestRequesterComponent)) 800 return false; 801 CommunicationRequestRequesterComponent o = (CommunicationRequestRequesterComponent) other_; 802 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 803 } 804 805 @Override 806 public boolean equalsShallow(Base other_) { 807 if (!super.equalsShallow(other_)) 808 return false; 809 if (!(other_ instanceof CommunicationRequestRequesterComponent)) 810 return false; 811 CommunicationRequestRequesterComponent o = (CommunicationRequestRequesterComponent) other_; 812 return true; 813 } 814 815 public boolean isEmpty() { 816 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 817 } 818 819 public String fhirType() { 820 return "CommunicationRequest.requester"; 821 822 } 823 824 } 825 826 /** 827 * A unique ID of this request for reference purposes. It must be provided if user wants it returned as part of any output, otherwise it will be autogenerated, if needed, by CDS system. Does not need to be the actual ID of the source system. 828 */ 829 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 830 @Description(shortDefinition="Unique identifier", formalDefinition="A unique ID of this request for reference purposes. It must be provided if user wants it returned as part of any output, otherwise it will be autogenerated, if needed, by CDS system. Does not need to be the actual ID of the source system." ) 831 protected List<Identifier> identifier; 832 833 /** 834 * A plan or proposal that is fulfilled in whole or in part by this request. 835 */ 836 @Child(name = "basedOn", type = {Reference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 837 @Description(shortDefinition="Fulfills plan or proposal", formalDefinition="A plan or proposal that is fulfilled in whole or in part by this request." ) 838 protected List<Reference> basedOn; 839 /** 840 * The actual objects that are the target of the reference (A plan or proposal that is fulfilled in whole or in part by this request.) 841 */ 842 protected List<Resource> basedOnTarget; 843 844 845 /** 846 * Completed or terminated request(s) whose function is taken by this new request. 847 */ 848 @Child(name = "replaces", type = {CommunicationRequest.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 849 @Description(shortDefinition="Request(s) replaced by this request", formalDefinition="Completed or terminated request(s) whose function is taken by this new request." ) 850 protected List<Reference> replaces; 851 /** 852 * The actual objects that are the target of the reference (Completed or terminated request(s) whose function is taken by this new request.) 853 */ 854 protected List<CommunicationRequest> replacesTarget; 855 856 857 /** 858 * A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form. 859 */ 860 @Child(name = "groupIdentifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 861 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form." ) 862 protected Identifier groupIdentifier; 863 864 /** 865 * The status of the proposal or order. 866 */ 867 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 868 @Description(shortDefinition="draft | active | suspended | cancelled | completed | entered-in-error | unknown", formalDefinition="The status of the proposal or order." ) 869 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 870 protected Enumeration<CommunicationRequestStatus> status; 871 872 /** 873 * The type of message to be sent such as alert, notification, reminder, instruction, etc. 874 */ 875 @Child(name = "category", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 876 @Description(shortDefinition="Message category", formalDefinition="The type of message to be sent such as alert, notification, reminder, instruction, etc." ) 877 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-category") 878 protected List<CodeableConcept> category; 879 880 /** 881 * Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine. 882 */ 883 @Child(name = "priority", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 884 @Description(shortDefinition="Message urgency", formalDefinition="Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine." ) 885 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 886 protected Enumeration<CommunicationPriority> priority; 887 888 /** 889 * A channel that was used for this communication (e.g. email, fax). 890 */ 891 @Child(name = "medium", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 892 @Description(shortDefinition="A channel of communication", formalDefinition="A channel that was used for this communication (e.g. email, fax)." ) 893 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ParticipationMode") 894 protected List<CodeableConcept> medium; 895 896 /** 897 * The patient or group that is the focus of this communication request. 898 */ 899 @Child(name = "subject", type = {Patient.class, Group.class}, order=8, min=0, max=1, modifier=false, summary=false) 900 @Description(shortDefinition="Focus of message", formalDefinition="The patient or group that is the focus of this communication request." ) 901 protected Reference subject; 902 903 /** 904 * The actual object that is the target of the reference (The patient or group that is the focus of this communication request.) 905 */ 906 protected Resource subjectTarget; 907 908 /** 909 * The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication. 910 */ 911 @Child(name = "recipient", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, Group.class, CareTeam.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 912 @Description(shortDefinition="Message recipient", formalDefinition="The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication." ) 913 protected List<Reference> recipient; 914 /** 915 * The actual objects that are the target of the reference (The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.) 916 */ 917 protected List<Resource> recipientTarget; 918 919 920 /** 921 * The resources which were related to producing this communication request. 922 */ 923 @Child(name = "topic", type = {Reference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 924 @Description(shortDefinition="Focal resources", formalDefinition="The resources which were related to producing this communication request." ) 925 protected List<Reference> topic; 926 /** 927 * The actual objects that are the target of the reference (The resources which were related to producing this communication request.) 928 */ 929 protected List<Resource> topicTarget; 930 931 932 /** 933 * The encounter or episode of care within which the communication request was created. 934 */ 935 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=11, min=0, max=1, modifier=false, summary=true) 936 @Description(shortDefinition="Encounter or episode leading to message", formalDefinition="The encounter or episode of care within which the communication request was created." ) 937 protected Reference context; 938 939 /** 940 * The actual object that is the target of the reference (The encounter or episode of care within which the communication request was created.) 941 */ 942 protected Resource contextTarget; 943 944 /** 945 * Text, attachment(s), or resource(s) to be communicated to the recipient. 946 */ 947 @Child(name = "payload", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 948 @Description(shortDefinition="Message payload", formalDefinition="Text, attachment(s), or resource(s) to be communicated to the recipient." ) 949 protected List<CommunicationRequestPayloadComponent> payload; 950 951 /** 952 * The time when this communication is to occur. 953 */ 954 @Child(name = "occurrence", type = {DateTimeType.class, Period.class}, order=13, min=0, max=1, modifier=false, summary=true) 955 @Description(shortDefinition="When scheduled", formalDefinition="The time when this communication is to occur." ) 956 protected Type occurrence; 957 958 /** 959 * For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 960 */ 961 @Child(name = "authoredOn", type = {DateTimeType.class}, order=14, min=0, max=1, modifier=false, summary=true) 962 @Description(shortDefinition="When request transitioned to being actionable", formalDefinition="For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation." ) 963 protected DateTimeType authoredOn; 964 965 /** 966 * The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication. 967 */ 968 @Child(name = "sender", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=15, min=0, max=1, modifier=false, summary=false) 969 @Description(shortDefinition="Message sender", formalDefinition="The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication." ) 970 protected Reference sender; 971 972 /** 973 * The actual object that is the target of the reference (The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.) 974 */ 975 protected Resource senderTarget; 976 977 /** 978 * The individual who initiated the request and has responsibility for its activation. 979 */ 980 @Child(name = "requester", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 981 @Description(shortDefinition="Who/what is requesting service", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 982 protected CommunicationRequestRequesterComponent requester; 983 984 /** 985 * Describes why the request is being made in coded or textual form. 986 */ 987 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 988 @Description(shortDefinition="Why is communication needed?", formalDefinition="Describes why the request is being made in coded or textual form." ) 989 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActReason") 990 protected List<CodeableConcept> reasonCode; 991 992 /** 993 * Indicates another resource whose existence justifies this request. 994 */ 995 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 996 @Description(shortDefinition="Why is communication needed?", formalDefinition="Indicates another resource whose existence justifies this request." ) 997 protected List<Reference> reasonReference; 998 /** 999 * The actual objects that are the target of the reference (Indicates another resource whose existence justifies this request.) 1000 */ 1001 protected List<Resource> reasonReferenceTarget; 1002 1003 1004 /** 1005 * Comments made about the request by the requester, sender, recipient, subject or other participants. 1006 */ 1007 @Child(name = "note", type = {Annotation.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1008 @Description(shortDefinition="Comments made about communication request", formalDefinition="Comments made about the request by the requester, sender, recipient, subject or other participants." ) 1009 protected List<Annotation> note; 1010 1011 private static final long serialVersionUID = -722867744L; 1012 1013 /** 1014 * Constructor 1015 */ 1016 public CommunicationRequest() { 1017 super(); 1018 } 1019 1020 /** 1021 * Constructor 1022 */ 1023 public CommunicationRequest(Enumeration<CommunicationRequestStatus> status) { 1024 super(); 1025 this.status = status; 1026 } 1027 1028 /** 1029 * @return {@link #identifier} (A unique ID of this request for reference purposes. It must be provided if user wants it returned as part of any output, otherwise it will be autogenerated, if needed, by CDS system. Does not need to be the actual ID of the source system.) 1030 */ 1031 public List<Identifier> getIdentifier() { 1032 if (this.identifier == null) 1033 this.identifier = new ArrayList<Identifier>(); 1034 return this.identifier; 1035 } 1036 1037 /** 1038 * @return Returns a reference to <code>this</code> for easy method chaining 1039 */ 1040 public CommunicationRequest setIdentifier(List<Identifier> theIdentifier) { 1041 this.identifier = theIdentifier; 1042 return this; 1043 } 1044 1045 public boolean hasIdentifier() { 1046 if (this.identifier == null) 1047 return false; 1048 for (Identifier item : this.identifier) 1049 if (!item.isEmpty()) 1050 return true; 1051 return false; 1052 } 1053 1054 public Identifier addIdentifier() { //3 1055 Identifier t = new Identifier(); 1056 if (this.identifier == null) 1057 this.identifier = new ArrayList<Identifier>(); 1058 this.identifier.add(t); 1059 return t; 1060 } 1061 1062 public CommunicationRequest addIdentifier(Identifier t) { //3 1063 if (t == null) 1064 return this; 1065 if (this.identifier == null) 1066 this.identifier = new ArrayList<Identifier>(); 1067 this.identifier.add(t); 1068 return this; 1069 } 1070 1071 /** 1072 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1073 */ 1074 public Identifier getIdentifierFirstRep() { 1075 if (getIdentifier().isEmpty()) { 1076 addIdentifier(); 1077 } 1078 return getIdentifier().get(0); 1079 } 1080 1081 /** 1082 * @return {@link #basedOn} (A plan or proposal that is fulfilled in whole or in part by this request.) 1083 */ 1084 public List<Reference> getBasedOn() { 1085 if (this.basedOn == null) 1086 this.basedOn = new ArrayList<Reference>(); 1087 return this.basedOn; 1088 } 1089 1090 /** 1091 * @return Returns a reference to <code>this</code> for easy method chaining 1092 */ 1093 public CommunicationRequest setBasedOn(List<Reference> theBasedOn) { 1094 this.basedOn = theBasedOn; 1095 return this; 1096 } 1097 1098 public boolean hasBasedOn() { 1099 if (this.basedOn == null) 1100 return false; 1101 for (Reference item : this.basedOn) 1102 if (!item.isEmpty()) 1103 return true; 1104 return false; 1105 } 1106 1107 public Reference addBasedOn() { //3 1108 Reference t = new Reference(); 1109 if (this.basedOn == null) 1110 this.basedOn = new ArrayList<Reference>(); 1111 this.basedOn.add(t); 1112 return t; 1113 } 1114 1115 public CommunicationRequest addBasedOn(Reference t) { //3 1116 if (t == null) 1117 return this; 1118 if (this.basedOn == null) 1119 this.basedOn = new ArrayList<Reference>(); 1120 this.basedOn.add(t); 1121 return this; 1122 } 1123 1124 /** 1125 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1126 */ 1127 public Reference getBasedOnFirstRep() { 1128 if (getBasedOn().isEmpty()) { 1129 addBasedOn(); 1130 } 1131 return getBasedOn().get(0); 1132 } 1133 1134 /** 1135 * @deprecated Use Reference#setResource(IBaseResource) instead 1136 */ 1137 @Deprecated 1138 public List<Resource> getBasedOnTarget() { 1139 if (this.basedOnTarget == null) 1140 this.basedOnTarget = new ArrayList<Resource>(); 1141 return this.basedOnTarget; 1142 } 1143 1144 /** 1145 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 1146 */ 1147 public List<Reference> getReplaces() { 1148 if (this.replaces == null) 1149 this.replaces = new ArrayList<Reference>(); 1150 return this.replaces; 1151 } 1152 1153 /** 1154 * @return Returns a reference to <code>this</code> for easy method chaining 1155 */ 1156 public CommunicationRequest setReplaces(List<Reference> theReplaces) { 1157 this.replaces = theReplaces; 1158 return this; 1159 } 1160 1161 public boolean hasReplaces() { 1162 if (this.replaces == null) 1163 return false; 1164 for (Reference item : this.replaces) 1165 if (!item.isEmpty()) 1166 return true; 1167 return false; 1168 } 1169 1170 public Reference addReplaces() { //3 1171 Reference t = new Reference(); 1172 if (this.replaces == null) 1173 this.replaces = new ArrayList<Reference>(); 1174 this.replaces.add(t); 1175 return t; 1176 } 1177 1178 public CommunicationRequest addReplaces(Reference t) { //3 1179 if (t == null) 1180 return this; 1181 if (this.replaces == null) 1182 this.replaces = new ArrayList<Reference>(); 1183 this.replaces.add(t); 1184 return this; 1185 } 1186 1187 /** 1188 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 1189 */ 1190 public Reference getReplacesFirstRep() { 1191 if (getReplaces().isEmpty()) { 1192 addReplaces(); 1193 } 1194 return getReplaces().get(0); 1195 } 1196 1197 /** 1198 * @deprecated Use Reference#setResource(IBaseResource) instead 1199 */ 1200 @Deprecated 1201 public List<CommunicationRequest> getReplacesTarget() { 1202 if (this.replacesTarget == null) 1203 this.replacesTarget = new ArrayList<CommunicationRequest>(); 1204 return this.replacesTarget; 1205 } 1206 1207 /** 1208 * @deprecated Use Reference#setResource(IBaseResource) instead 1209 */ 1210 @Deprecated 1211 public CommunicationRequest addReplacesTarget() { 1212 CommunicationRequest r = new CommunicationRequest(); 1213 if (this.replacesTarget == null) 1214 this.replacesTarget = new ArrayList<CommunicationRequest>(); 1215 this.replacesTarget.add(r); 1216 return r; 1217 } 1218 1219 /** 1220 * @return {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 1221 */ 1222 public Identifier getGroupIdentifier() { 1223 if (this.groupIdentifier == null) 1224 if (Configuration.errorOnAutoCreate()) 1225 throw new Error("Attempt to auto-create CommunicationRequest.groupIdentifier"); 1226 else if (Configuration.doAutoCreate()) 1227 this.groupIdentifier = new Identifier(); // cc 1228 return this.groupIdentifier; 1229 } 1230 1231 public boolean hasGroupIdentifier() { 1232 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1233 } 1234 1235 /** 1236 * @param value {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 1237 */ 1238 public CommunicationRequest setGroupIdentifier(Identifier value) { 1239 this.groupIdentifier = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return {@link #status} (The status of the proposal or order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1245 */ 1246 public Enumeration<CommunicationRequestStatus> getStatusElement() { 1247 if (this.status == null) 1248 if (Configuration.errorOnAutoCreate()) 1249 throw new Error("Attempt to auto-create CommunicationRequest.status"); 1250 else if (Configuration.doAutoCreate()) 1251 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); // bb 1252 return this.status; 1253 } 1254 1255 public boolean hasStatusElement() { 1256 return this.status != null && !this.status.isEmpty(); 1257 } 1258 1259 public boolean hasStatus() { 1260 return this.status != null && !this.status.isEmpty(); 1261 } 1262 1263 /** 1264 * @param value {@link #status} (The status of the proposal or order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1265 */ 1266 public CommunicationRequest setStatusElement(Enumeration<CommunicationRequestStatus> value) { 1267 this.status = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return The status of the proposal or order. 1273 */ 1274 public CommunicationRequestStatus getStatus() { 1275 return this.status == null ? null : this.status.getValue(); 1276 } 1277 1278 /** 1279 * @param value The status of the proposal or order. 1280 */ 1281 public CommunicationRequest setStatus(CommunicationRequestStatus value) { 1282 if (this.status == null) 1283 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); 1284 this.status.setValue(value); 1285 return this; 1286 } 1287 1288 /** 1289 * @return {@link #category} (The type of message to be sent such as alert, notification, reminder, instruction, etc.) 1290 */ 1291 public List<CodeableConcept> getCategory() { 1292 if (this.category == null) 1293 this.category = new ArrayList<CodeableConcept>(); 1294 return this.category; 1295 } 1296 1297 /** 1298 * @return Returns a reference to <code>this</code> for easy method chaining 1299 */ 1300 public CommunicationRequest setCategory(List<CodeableConcept> theCategory) { 1301 this.category = theCategory; 1302 return this; 1303 } 1304 1305 public boolean hasCategory() { 1306 if (this.category == null) 1307 return false; 1308 for (CodeableConcept item : this.category) 1309 if (!item.isEmpty()) 1310 return true; 1311 return false; 1312 } 1313 1314 public CodeableConcept addCategory() { //3 1315 CodeableConcept t = new CodeableConcept(); 1316 if (this.category == null) 1317 this.category = new ArrayList<CodeableConcept>(); 1318 this.category.add(t); 1319 return t; 1320 } 1321 1322 public CommunicationRequest addCategory(CodeableConcept t) { //3 1323 if (t == null) 1324 return this; 1325 if (this.category == null) 1326 this.category = new ArrayList<CodeableConcept>(); 1327 this.category.add(t); 1328 return this; 1329 } 1330 1331 /** 1332 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 1333 */ 1334 public CodeableConcept getCategoryFirstRep() { 1335 if (getCategory().isEmpty()) { 1336 addCategory(); 1337 } 1338 return getCategory().get(0); 1339 } 1340 1341 /** 1342 * @return {@link #priority} (Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1343 */ 1344 public Enumeration<CommunicationPriority> getPriorityElement() { 1345 if (this.priority == null) 1346 if (Configuration.errorOnAutoCreate()) 1347 throw new Error("Attempt to auto-create CommunicationRequest.priority"); 1348 else if (Configuration.doAutoCreate()) 1349 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); // bb 1350 return this.priority; 1351 } 1352 1353 public boolean hasPriorityElement() { 1354 return this.priority != null && !this.priority.isEmpty(); 1355 } 1356 1357 public boolean hasPriority() { 1358 return this.priority != null && !this.priority.isEmpty(); 1359 } 1360 1361 /** 1362 * @param value {@link #priority} (Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1363 */ 1364 public CommunicationRequest setPriorityElement(Enumeration<CommunicationPriority> value) { 1365 this.priority = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine. 1371 */ 1372 public CommunicationPriority getPriority() { 1373 return this.priority == null ? null : this.priority.getValue(); 1374 } 1375 1376 /** 1377 * @param value Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine. 1378 */ 1379 public CommunicationRequest setPriority(CommunicationPriority value) { 1380 if (value == null) 1381 this.priority = null; 1382 else { 1383 if (this.priority == null) 1384 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); 1385 this.priority.setValue(value); 1386 } 1387 return this; 1388 } 1389 1390 /** 1391 * @return {@link #medium} (A channel that was used for this communication (e.g. email, fax).) 1392 */ 1393 public List<CodeableConcept> getMedium() { 1394 if (this.medium == null) 1395 this.medium = new ArrayList<CodeableConcept>(); 1396 return this.medium; 1397 } 1398 1399 /** 1400 * @return Returns a reference to <code>this</code> for easy method chaining 1401 */ 1402 public CommunicationRequest setMedium(List<CodeableConcept> theMedium) { 1403 this.medium = theMedium; 1404 return this; 1405 } 1406 1407 public boolean hasMedium() { 1408 if (this.medium == null) 1409 return false; 1410 for (CodeableConcept item : this.medium) 1411 if (!item.isEmpty()) 1412 return true; 1413 return false; 1414 } 1415 1416 public CodeableConcept addMedium() { //3 1417 CodeableConcept t = new CodeableConcept(); 1418 if (this.medium == null) 1419 this.medium = new ArrayList<CodeableConcept>(); 1420 this.medium.add(t); 1421 return t; 1422 } 1423 1424 public CommunicationRequest addMedium(CodeableConcept t) { //3 1425 if (t == null) 1426 return this; 1427 if (this.medium == null) 1428 this.medium = new ArrayList<CodeableConcept>(); 1429 this.medium.add(t); 1430 return this; 1431 } 1432 1433 /** 1434 * @return The first repetition of repeating field {@link #medium}, creating it if it does not already exist 1435 */ 1436 public CodeableConcept getMediumFirstRep() { 1437 if (getMedium().isEmpty()) { 1438 addMedium(); 1439 } 1440 return getMedium().get(0); 1441 } 1442 1443 /** 1444 * @return {@link #subject} (The patient or group that is the focus of this communication request.) 1445 */ 1446 public Reference getSubject() { 1447 if (this.subject == null) 1448 if (Configuration.errorOnAutoCreate()) 1449 throw new Error("Attempt to auto-create CommunicationRequest.subject"); 1450 else if (Configuration.doAutoCreate()) 1451 this.subject = new Reference(); // cc 1452 return this.subject; 1453 } 1454 1455 public boolean hasSubject() { 1456 return this.subject != null && !this.subject.isEmpty(); 1457 } 1458 1459 /** 1460 * @param value {@link #subject} (The patient or group that is the focus of this communication request.) 1461 */ 1462 public CommunicationRequest setSubject(Reference value) { 1463 this.subject = value; 1464 return this; 1465 } 1466 1467 /** 1468 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient or group that is the focus of this communication request.) 1469 */ 1470 public Resource getSubjectTarget() { 1471 return this.subjectTarget; 1472 } 1473 1474 /** 1475 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient or group that is the focus of this communication request.) 1476 */ 1477 public CommunicationRequest setSubjectTarget(Resource value) { 1478 this.subjectTarget = value; 1479 return this; 1480 } 1481 1482 /** 1483 * @return {@link #recipient} (The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.) 1484 */ 1485 public List<Reference> getRecipient() { 1486 if (this.recipient == null) 1487 this.recipient = new ArrayList<Reference>(); 1488 return this.recipient; 1489 } 1490 1491 /** 1492 * @return Returns a reference to <code>this</code> for easy method chaining 1493 */ 1494 public CommunicationRequest setRecipient(List<Reference> theRecipient) { 1495 this.recipient = theRecipient; 1496 return this; 1497 } 1498 1499 public boolean hasRecipient() { 1500 if (this.recipient == null) 1501 return false; 1502 for (Reference item : this.recipient) 1503 if (!item.isEmpty()) 1504 return true; 1505 return false; 1506 } 1507 1508 public Reference addRecipient() { //3 1509 Reference t = new Reference(); 1510 if (this.recipient == null) 1511 this.recipient = new ArrayList<Reference>(); 1512 this.recipient.add(t); 1513 return t; 1514 } 1515 1516 public CommunicationRequest addRecipient(Reference t) { //3 1517 if (t == null) 1518 return this; 1519 if (this.recipient == null) 1520 this.recipient = new ArrayList<Reference>(); 1521 this.recipient.add(t); 1522 return this; 1523 } 1524 1525 /** 1526 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist 1527 */ 1528 public Reference getRecipientFirstRep() { 1529 if (getRecipient().isEmpty()) { 1530 addRecipient(); 1531 } 1532 return getRecipient().get(0); 1533 } 1534 1535 /** 1536 * @deprecated Use Reference#setResource(IBaseResource) instead 1537 */ 1538 @Deprecated 1539 public List<Resource> getRecipientTarget() { 1540 if (this.recipientTarget == null) 1541 this.recipientTarget = new ArrayList<Resource>(); 1542 return this.recipientTarget; 1543 } 1544 1545 /** 1546 * @return {@link #topic} (The resources which were related to producing this communication request.) 1547 */ 1548 public List<Reference> getTopic() { 1549 if (this.topic == null) 1550 this.topic = new ArrayList<Reference>(); 1551 return this.topic; 1552 } 1553 1554 /** 1555 * @return Returns a reference to <code>this</code> for easy method chaining 1556 */ 1557 public CommunicationRequest setTopic(List<Reference> theTopic) { 1558 this.topic = theTopic; 1559 return this; 1560 } 1561 1562 public boolean hasTopic() { 1563 if (this.topic == null) 1564 return false; 1565 for (Reference item : this.topic) 1566 if (!item.isEmpty()) 1567 return true; 1568 return false; 1569 } 1570 1571 public Reference addTopic() { //3 1572 Reference t = new Reference(); 1573 if (this.topic == null) 1574 this.topic = new ArrayList<Reference>(); 1575 this.topic.add(t); 1576 return t; 1577 } 1578 1579 public CommunicationRequest addTopic(Reference t) { //3 1580 if (t == null) 1581 return this; 1582 if (this.topic == null) 1583 this.topic = new ArrayList<Reference>(); 1584 this.topic.add(t); 1585 return this; 1586 } 1587 1588 /** 1589 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 1590 */ 1591 public Reference getTopicFirstRep() { 1592 if (getTopic().isEmpty()) { 1593 addTopic(); 1594 } 1595 return getTopic().get(0); 1596 } 1597 1598 /** 1599 * @deprecated Use Reference#setResource(IBaseResource) instead 1600 */ 1601 @Deprecated 1602 public List<Resource> getTopicTarget() { 1603 if (this.topicTarget == null) 1604 this.topicTarget = new ArrayList<Resource>(); 1605 return this.topicTarget; 1606 } 1607 1608 /** 1609 * @return {@link #context} (The encounter or episode of care within which the communication request was created.) 1610 */ 1611 public Reference getContext() { 1612 if (this.context == null) 1613 if (Configuration.errorOnAutoCreate()) 1614 throw new Error("Attempt to auto-create CommunicationRequest.context"); 1615 else if (Configuration.doAutoCreate()) 1616 this.context = new Reference(); // cc 1617 return this.context; 1618 } 1619 1620 public boolean hasContext() { 1621 return this.context != null && !this.context.isEmpty(); 1622 } 1623 1624 /** 1625 * @param value {@link #context} (The encounter or episode of care within which the communication request was created.) 1626 */ 1627 public CommunicationRequest setContext(Reference value) { 1628 this.context = value; 1629 return this; 1630 } 1631 1632 /** 1633 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care within which the communication request was created.) 1634 */ 1635 public Resource getContextTarget() { 1636 return this.contextTarget; 1637 } 1638 1639 /** 1640 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care within which the communication request was created.) 1641 */ 1642 public CommunicationRequest setContextTarget(Resource value) { 1643 this.contextTarget = value; 1644 return this; 1645 } 1646 1647 /** 1648 * @return {@link #payload} (Text, attachment(s), or resource(s) to be communicated to the recipient.) 1649 */ 1650 public List<CommunicationRequestPayloadComponent> getPayload() { 1651 if (this.payload == null) 1652 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1653 return this.payload; 1654 } 1655 1656 /** 1657 * @return Returns a reference to <code>this</code> for easy method chaining 1658 */ 1659 public CommunicationRequest setPayload(List<CommunicationRequestPayloadComponent> thePayload) { 1660 this.payload = thePayload; 1661 return this; 1662 } 1663 1664 public boolean hasPayload() { 1665 if (this.payload == null) 1666 return false; 1667 for (CommunicationRequestPayloadComponent item : this.payload) 1668 if (!item.isEmpty()) 1669 return true; 1670 return false; 1671 } 1672 1673 public CommunicationRequestPayloadComponent addPayload() { //3 1674 CommunicationRequestPayloadComponent t = new CommunicationRequestPayloadComponent(); 1675 if (this.payload == null) 1676 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1677 this.payload.add(t); 1678 return t; 1679 } 1680 1681 public CommunicationRequest addPayload(CommunicationRequestPayloadComponent t) { //3 1682 if (t == null) 1683 return this; 1684 if (this.payload == null) 1685 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1686 this.payload.add(t); 1687 return this; 1688 } 1689 1690 /** 1691 * @return The first repetition of repeating field {@link #payload}, creating it if it does not already exist 1692 */ 1693 public CommunicationRequestPayloadComponent getPayloadFirstRep() { 1694 if (getPayload().isEmpty()) { 1695 addPayload(); 1696 } 1697 return getPayload().get(0); 1698 } 1699 1700 /** 1701 * @return {@link #occurrence} (The time when this communication is to occur.) 1702 */ 1703 public Type getOccurrence() { 1704 return this.occurrence; 1705 } 1706 1707 /** 1708 * @return {@link #occurrence} (The time when this communication is to occur.) 1709 */ 1710 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1711 if (this.occurrence == null) 1712 return null; 1713 if (!(this.occurrence instanceof DateTimeType)) 1714 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1715 return (DateTimeType) this.occurrence; 1716 } 1717 1718 public boolean hasOccurrenceDateTimeType() { 1719 return this != null && this.occurrence instanceof DateTimeType; 1720 } 1721 1722 /** 1723 * @return {@link #occurrence} (The time when this communication is to occur.) 1724 */ 1725 public Period getOccurrencePeriod() throws FHIRException { 1726 if (this.occurrence == null) 1727 return null; 1728 if (!(this.occurrence instanceof Period)) 1729 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1730 return (Period) this.occurrence; 1731 } 1732 1733 public boolean hasOccurrencePeriod() { 1734 return this != null && this.occurrence instanceof Period; 1735 } 1736 1737 public boolean hasOccurrence() { 1738 return this.occurrence != null && !this.occurrence.isEmpty(); 1739 } 1740 1741 /** 1742 * @param value {@link #occurrence} (The time when this communication is to occur.) 1743 */ 1744 public CommunicationRequest setOccurrence(Type value) throws FHIRFormatError { 1745 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1746 throw new FHIRFormatError("Not the right type for CommunicationRequest.occurrence[x]: "+value.fhirType()); 1747 this.occurrence = value; 1748 return this; 1749 } 1750 1751 /** 1752 * @return {@link #authoredOn} (For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1753 */ 1754 public DateTimeType getAuthoredOnElement() { 1755 if (this.authoredOn == null) 1756 if (Configuration.errorOnAutoCreate()) 1757 throw new Error("Attempt to auto-create CommunicationRequest.authoredOn"); 1758 else if (Configuration.doAutoCreate()) 1759 this.authoredOn = new DateTimeType(); // bb 1760 return this.authoredOn; 1761 } 1762 1763 public boolean hasAuthoredOnElement() { 1764 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1765 } 1766 1767 public boolean hasAuthoredOn() { 1768 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1769 } 1770 1771 /** 1772 * @param value {@link #authoredOn} (For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1773 */ 1774 public CommunicationRequest setAuthoredOnElement(DateTimeType value) { 1775 this.authoredOn = value; 1776 return this; 1777 } 1778 1779 /** 1780 * @return For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1781 */ 1782 public Date getAuthoredOn() { 1783 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1784 } 1785 1786 /** 1787 * @param value For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1788 */ 1789 public CommunicationRequest setAuthoredOn(Date value) { 1790 if (value == null) 1791 this.authoredOn = null; 1792 else { 1793 if (this.authoredOn == null) 1794 this.authoredOn = new DateTimeType(); 1795 this.authoredOn.setValue(value); 1796 } 1797 return this; 1798 } 1799 1800 /** 1801 * @return {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.) 1802 */ 1803 public Reference getSender() { 1804 if (this.sender == null) 1805 if (Configuration.errorOnAutoCreate()) 1806 throw new Error("Attempt to auto-create CommunicationRequest.sender"); 1807 else if (Configuration.doAutoCreate()) 1808 this.sender = new Reference(); // cc 1809 return this.sender; 1810 } 1811 1812 public boolean hasSender() { 1813 return this.sender != null && !this.sender.isEmpty(); 1814 } 1815 1816 /** 1817 * @param value {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.) 1818 */ 1819 public CommunicationRequest setSender(Reference value) { 1820 this.sender = value; 1821 return this; 1822 } 1823 1824 /** 1825 * @return {@link #sender} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.) 1826 */ 1827 public Resource getSenderTarget() { 1828 return this.senderTarget; 1829 } 1830 1831 /** 1832 * @param value {@link #sender} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.) 1833 */ 1834 public CommunicationRequest setSenderTarget(Resource value) { 1835 this.senderTarget = value; 1836 return this; 1837 } 1838 1839 /** 1840 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1841 */ 1842 public CommunicationRequestRequesterComponent getRequester() { 1843 if (this.requester == null) 1844 if (Configuration.errorOnAutoCreate()) 1845 throw new Error("Attempt to auto-create CommunicationRequest.requester"); 1846 else if (Configuration.doAutoCreate()) 1847 this.requester = new CommunicationRequestRequesterComponent(); // cc 1848 return this.requester; 1849 } 1850 1851 public boolean hasRequester() { 1852 return this.requester != null && !this.requester.isEmpty(); 1853 } 1854 1855 /** 1856 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1857 */ 1858 public CommunicationRequest setRequester(CommunicationRequestRequesterComponent value) { 1859 this.requester = value; 1860 return this; 1861 } 1862 1863 /** 1864 * @return {@link #reasonCode} (Describes why the request is being made in coded or textual form.) 1865 */ 1866 public List<CodeableConcept> getReasonCode() { 1867 if (this.reasonCode == null) 1868 this.reasonCode = new ArrayList<CodeableConcept>(); 1869 return this.reasonCode; 1870 } 1871 1872 /** 1873 * @return Returns a reference to <code>this</code> for easy method chaining 1874 */ 1875 public CommunicationRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1876 this.reasonCode = theReasonCode; 1877 return this; 1878 } 1879 1880 public boolean hasReasonCode() { 1881 if (this.reasonCode == null) 1882 return false; 1883 for (CodeableConcept item : this.reasonCode) 1884 if (!item.isEmpty()) 1885 return true; 1886 return false; 1887 } 1888 1889 public CodeableConcept addReasonCode() { //3 1890 CodeableConcept t = new CodeableConcept(); 1891 if (this.reasonCode == null) 1892 this.reasonCode = new ArrayList<CodeableConcept>(); 1893 this.reasonCode.add(t); 1894 return t; 1895 } 1896 1897 public CommunicationRequest addReasonCode(CodeableConcept t) { //3 1898 if (t == null) 1899 return this; 1900 if (this.reasonCode == null) 1901 this.reasonCode = new ArrayList<CodeableConcept>(); 1902 this.reasonCode.add(t); 1903 return this; 1904 } 1905 1906 /** 1907 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1908 */ 1909 public CodeableConcept getReasonCodeFirstRep() { 1910 if (getReasonCode().isEmpty()) { 1911 addReasonCode(); 1912 } 1913 return getReasonCode().get(0); 1914 } 1915 1916 /** 1917 * @return {@link #reasonReference} (Indicates another resource whose existence justifies this request.) 1918 */ 1919 public List<Reference> getReasonReference() { 1920 if (this.reasonReference == null) 1921 this.reasonReference = new ArrayList<Reference>(); 1922 return this.reasonReference; 1923 } 1924 1925 /** 1926 * @return Returns a reference to <code>this</code> for easy method chaining 1927 */ 1928 public CommunicationRequest setReasonReference(List<Reference> theReasonReference) { 1929 this.reasonReference = theReasonReference; 1930 return this; 1931 } 1932 1933 public boolean hasReasonReference() { 1934 if (this.reasonReference == null) 1935 return false; 1936 for (Reference item : this.reasonReference) 1937 if (!item.isEmpty()) 1938 return true; 1939 return false; 1940 } 1941 1942 public Reference addReasonReference() { //3 1943 Reference t = new Reference(); 1944 if (this.reasonReference == null) 1945 this.reasonReference = new ArrayList<Reference>(); 1946 this.reasonReference.add(t); 1947 return t; 1948 } 1949 1950 public CommunicationRequest addReasonReference(Reference t) { //3 1951 if (t == null) 1952 return this; 1953 if (this.reasonReference == null) 1954 this.reasonReference = new ArrayList<Reference>(); 1955 this.reasonReference.add(t); 1956 return this; 1957 } 1958 1959 /** 1960 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1961 */ 1962 public Reference getReasonReferenceFirstRep() { 1963 if (getReasonReference().isEmpty()) { 1964 addReasonReference(); 1965 } 1966 return getReasonReference().get(0); 1967 } 1968 1969 /** 1970 * @deprecated Use Reference#setResource(IBaseResource) instead 1971 */ 1972 @Deprecated 1973 public List<Resource> getReasonReferenceTarget() { 1974 if (this.reasonReferenceTarget == null) 1975 this.reasonReferenceTarget = new ArrayList<Resource>(); 1976 return this.reasonReferenceTarget; 1977 } 1978 1979 /** 1980 * @return {@link #note} (Comments made about the request by the requester, sender, recipient, subject or other participants.) 1981 */ 1982 public List<Annotation> getNote() { 1983 if (this.note == null) 1984 this.note = new ArrayList<Annotation>(); 1985 return this.note; 1986 } 1987 1988 /** 1989 * @return Returns a reference to <code>this</code> for easy method chaining 1990 */ 1991 public CommunicationRequest setNote(List<Annotation> theNote) { 1992 this.note = theNote; 1993 return this; 1994 } 1995 1996 public boolean hasNote() { 1997 if (this.note == null) 1998 return false; 1999 for (Annotation item : this.note) 2000 if (!item.isEmpty()) 2001 return true; 2002 return false; 2003 } 2004 2005 public Annotation addNote() { //3 2006 Annotation t = new Annotation(); 2007 if (this.note == null) 2008 this.note = new ArrayList<Annotation>(); 2009 this.note.add(t); 2010 return t; 2011 } 2012 2013 public CommunicationRequest addNote(Annotation t) { //3 2014 if (t == null) 2015 return this; 2016 if (this.note == null) 2017 this.note = new ArrayList<Annotation>(); 2018 this.note.add(t); 2019 return this; 2020 } 2021 2022 /** 2023 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2024 */ 2025 public Annotation getNoteFirstRep() { 2026 if (getNote().isEmpty()) { 2027 addNote(); 2028 } 2029 return getNote().get(0); 2030 } 2031 2032 protected void listChildren(List<Property> children) { 2033 super.listChildren(children); 2034 children.add(new Property("identifier", "Identifier", "A unique ID of this request for reference purposes. It must be provided if user wants it returned as part of any output, otherwise it will be autogenerated, if needed, by CDS system. Does not need to be the actual ID of the source system.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2035 children.add(new Property("basedOn", "Reference(Any)", "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2036 children.add(new Property("replaces", "Reference(CommunicationRequest)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2037 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 0, 1, groupIdentifier)); 2038 children.add(new Property("status", "code", "The status of the proposal or order.", 0, 1, status)); 2039 children.add(new Property("category", "CodeableConcept", "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 2040 children.add(new Property("priority", "code", "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 0, 1, priority)); 2041 children.add(new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 2042 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group that is the focus of this communication request.", 0, 1, subject)); 2043 children.add(new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson|Group|CareTeam)", "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 0, java.lang.Integer.MAX_VALUE, recipient)); 2044 children.add(new Property("topic", "Reference(Any)", "The resources which were related to producing this communication request.", 0, java.lang.Integer.MAX_VALUE, topic)); 2045 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care within which the communication request was created.", 0, 1, context)); 2046 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload)); 2047 children.add(new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence)); 2048 children.add(new Property("authoredOn", "dateTime", "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 0, 1, authoredOn)); 2049 children.add(new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 0, 1, sender)); 2050 children.add(new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 2051 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2052 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2053 children.add(new Property("note", "Annotation", "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 2054 } 2055 2056 @Override 2057 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2058 switch (_hash) { 2059 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique ID of this request for reference purposes. It must be provided if user wants it returned as part of any output, otherwise it will be autogenerated, if needed, by CDS system. Does not need to be the actual ID of the source system.", 0, java.lang.Integer.MAX_VALUE, identifier); 2060 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2061 case -430332865: /*replaces*/ return new Property("replaces", "Reference(CommunicationRequest)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces); 2062 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 0, 1, groupIdentifier); 2063 case -892481550: /*status*/ return new Property("status", "code", "The status of the proposal or order.", 0, 1, status); 2064 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category); 2065 case -1165461084: /*priority*/ return new Property("priority", "code", "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 0, 1, priority); 2066 case -1078030475: /*medium*/ return new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 2067 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group that is the focus of this communication request.", 0, 1, subject); 2068 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson|Group|CareTeam)", "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 0, java.lang.Integer.MAX_VALUE, recipient); 2069 case 110546223: /*topic*/ return new Property("topic", "Reference(Any)", "The resources which were related to producing this communication request.", 0, java.lang.Integer.MAX_VALUE, topic); 2070 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care within which the communication request was created.", 0, 1, context); 2071 case -786701938: /*payload*/ return new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload); 2072 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence); 2073 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence); 2074 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence); 2075 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence); 2076 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 0, 1, authoredOn); 2077 case -905962955: /*sender*/ return new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 0, 1, sender); 2078 case 693933948: /*requester*/ return new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 2079 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2080 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2081 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 2082 default: return super.getNamedProperty(_hash, _name, _checkValid); 2083 } 2084 2085 } 2086 2087 @Override 2088 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2089 switch (hash) { 2090 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2091 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2092 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2093 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 2094 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CommunicationRequestStatus> 2095 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2096 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<CommunicationPriority> 2097 case -1078030475: /*medium*/ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 2098 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2099 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 2100 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // Reference 2101 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2102 case -786701938: /*payload*/ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationRequestPayloadComponent 2103 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 2104 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 2105 case -905962955: /*sender*/ return this.sender == null ? new Base[0] : new Base[] {this.sender}; // Reference 2106 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // CommunicationRequestRequesterComponent 2107 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2108 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2109 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2110 default: return super.getProperty(hash, name, checkValid); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2117 switch (hash) { 2118 case -1618432855: // identifier 2119 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2120 return value; 2121 case -332612366: // basedOn 2122 this.getBasedOn().add(castToReference(value)); // Reference 2123 return value; 2124 case -430332865: // replaces 2125 this.getReplaces().add(castToReference(value)); // Reference 2126 return value; 2127 case -445338488: // groupIdentifier 2128 this.groupIdentifier = castToIdentifier(value); // Identifier 2129 return value; 2130 case -892481550: // status 2131 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2132 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2133 return value; 2134 case 50511102: // category 2135 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2136 return value; 2137 case -1165461084: // priority 2138 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2139 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2140 return value; 2141 case -1078030475: // medium 2142 this.getMedium().add(castToCodeableConcept(value)); // CodeableConcept 2143 return value; 2144 case -1867885268: // subject 2145 this.subject = castToReference(value); // Reference 2146 return value; 2147 case 820081177: // recipient 2148 this.getRecipient().add(castToReference(value)); // Reference 2149 return value; 2150 case 110546223: // topic 2151 this.getTopic().add(castToReference(value)); // Reference 2152 return value; 2153 case 951530927: // context 2154 this.context = castToReference(value); // Reference 2155 return value; 2156 case -786701938: // payload 2157 this.getPayload().add((CommunicationRequestPayloadComponent) value); // CommunicationRequestPayloadComponent 2158 return value; 2159 case 1687874001: // occurrence 2160 this.occurrence = castToType(value); // Type 2161 return value; 2162 case -1500852503: // authoredOn 2163 this.authoredOn = castToDateTime(value); // DateTimeType 2164 return value; 2165 case -905962955: // sender 2166 this.sender = castToReference(value); // Reference 2167 return value; 2168 case 693933948: // requester 2169 this.requester = (CommunicationRequestRequesterComponent) value; // CommunicationRequestRequesterComponent 2170 return value; 2171 case 722137681: // reasonCode 2172 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2173 return value; 2174 case -1146218137: // reasonReference 2175 this.getReasonReference().add(castToReference(value)); // Reference 2176 return value; 2177 case 3387378: // note 2178 this.getNote().add(castToAnnotation(value)); // Annotation 2179 return value; 2180 default: return super.setProperty(hash, name, value); 2181 } 2182 2183 } 2184 2185 @Override 2186 public Base setProperty(String name, Base value) throws FHIRException { 2187 if (name.equals("identifier")) { 2188 this.getIdentifier().add(castToIdentifier(value)); 2189 } else if (name.equals("basedOn")) { 2190 this.getBasedOn().add(castToReference(value)); 2191 } else if (name.equals("replaces")) { 2192 this.getReplaces().add(castToReference(value)); 2193 } else if (name.equals("groupIdentifier")) { 2194 this.groupIdentifier = castToIdentifier(value); // Identifier 2195 } else if (name.equals("status")) { 2196 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2197 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2198 } else if (name.equals("category")) { 2199 this.getCategory().add(castToCodeableConcept(value)); 2200 } else if (name.equals("priority")) { 2201 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2202 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2203 } else if (name.equals("medium")) { 2204 this.getMedium().add(castToCodeableConcept(value)); 2205 } else if (name.equals("subject")) { 2206 this.subject = castToReference(value); // Reference 2207 } else if (name.equals("recipient")) { 2208 this.getRecipient().add(castToReference(value)); 2209 } else if (name.equals("topic")) { 2210 this.getTopic().add(castToReference(value)); 2211 } else if (name.equals("context")) { 2212 this.context = castToReference(value); // Reference 2213 } else if (name.equals("payload")) { 2214 this.getPayload().add((CommunicationRequestPayloadComponent) value); 2215 } else if (name.equals("occurrence[x]")) { 2216 this.occurrence = castToType(value); // Type 2217 } else if (name.equals("authoredOn")) { 2218 this.authoredOn = castToDateTime(value); // DateTimeType 2219 } else if (name.equals("sender")) { 2220 this.sender = castToReference(value); // Reference 2221 } else if (name.equals("requester")) { 2222 this.requester = (CommunicationRequestRequesterComponent) value; // CommunicationRequestRequesterComponent 2223 } else if (name.equals("reasonCode")) { 2224 this.getReasonCode().add(castToCodeableConcept(value)); 2225 } else if (name.equals("reasonReference")) { 2226 this.getReasonReference().add(castToReference(value)); 2227 } else if (name.equals("note")) { 2228 this.getNote().add(castToAnnotation(value)); 2229 } else 2230 return super.setProperty(name, value); 2231 return value; 2232 } 2233 2234 @Override 2235 public Base makeProperty(int hash, String name) throws FHIRException { 2236 switch (hash) { 2237 case -1618432855: return addIdentifier(); 2238 case -332612366: return addBasedOn(); 2239 case -430332865: return addReplaces(); 2240 case -445338488: return getGroupIdentifier(); 2241 case -892481550: return getStatusElement(); 2242 case 50511102: return addCategory(); 2243 case -1165461084: return getPriorityElement(); 2244 case -1078030475: return addMedium(); 2245 case -1867885268: return getSubject(); 2246 case 820081177: return addRecipient(); 2247 case 110546223: return addTopic(); 2248 case 951530927: return getContext(); 2249 case -786701938: return addPayload(); 2250 case -2022646513: return getOccurrence(); 2251 case 1687874001: return getOccurrence(); 2252 case -1500852503: return getAuthoredOnElement(); 2253 case -905962955: return getSender(); 2254 case 693933948: return getRequester(); 2255 case 722137681: return addReasonCode(); 2256 case -1146218137: return addReasonReference(); 2257 case 3387378: return addNote(); 2258 default: return super.makeProperty(hash, name); 2259 } 2260 2261 } 2262 2263 @Override 2264 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2265 switch (hash) { 2266 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2267 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2268 case -430332865: /*replaces*/ return new String[] {"Reference"}; 2269 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 2270 case -892481550: /*status*/ return new String[] {"code"}; 2271 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2272 case -1165461084: /*priority*/ return new String[] {"code"}; 2273 case -1078030475: /*medium*/ return new String[] {"CodeableConcept"}; 2274 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2275 case 820081177: /*recipient*/ return new String[] {"Reference"}; 2276 case 110546223: /*topic*/ return new String[] {"Reference"}; 2277 case 951530927: /*context*/ return new String[] {"Reference"}; 2278 case -786701938: /*payload*/ return new String[] {}; 2279 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period"}; 2280 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 2281 case -905962955: /*sender*/ return new String[] {"Reference"}; 2282 case 693933948: /*requester*/ return new String[] {}; 2283 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2284 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2285 case 3387378: /*note*/ return new String[] {"Annotation"}; 2286 default: return super.getTypesForProperty(hash, name); 2287 } 2288 2289 } 2290 2291 @Override 2292 public Base addChild(String name) throws FHIRException { 2293 if (name.equals("identifier")) { 2294 return addIdentifier(); 2295 } 2296 else if (name.equals("basedOn")) { 2297 return addBasedOn(); 2298 } 2299 else if (name.equals("replaces")) { 2300 return addReplaces(); 2301 } 2302 else if (name.equals("groupIdentifier")) { 2303 this.groupIdentifier = new Identifier(); 2304 return this.groupIdentifier; 2305 } 2306 else if (name.equals("status")) { 2307 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.status"); 2308 } 2309 else if (name.equals("category")) { 2310 return addCategory(); 2311 } 2312 else if (name.equals("priority")) { 2313 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.priority"); 2314 } 2315 else if (name.equals("medium")) { 2316 return addMedium(); 2317 } 2318 else if (name.equals("subject")) { 2319 this.subject = new Reference(); 2320 return this.subject; 2321 } 2322 else if (name.equals("recipient")) { 2323 return addRecipient(); 2324 } 2325 else if (name.equals("topic")) { 2326 return addTopic(); 2327 } 2328 else if (name.equals("context")) { 2329 this.context = new Reference(); 2330 return this.context; 2331 } 2332 else if (name.equals("payload")) { 2333 return addPayload(); 2334 } 2335 else if (name.equals("occurrenceDateTime")) { 2336 this.occurrence = new DateTimeType(); 2337 return this.occurrence; 2338 } 2339 else if (name.equals("occurrencePeriod")) { 2340 this.occurrence = new Period(); 2341 return this.occurrence; 2342 } 2343 else if (name.equals("authoredOn")) { 2344 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.authoredOn"); 2345 } 2346 else if (name.equals("sender")) { 2347 this.sender = new Reference(); 2348 return this.sender; 2349 } 2350 else if (name.equals("requester")) { 2351 this.requester = new CommunicationRequestRequesterComponent(); 2352 return this.requester; 2353 } 2354 else if (name.equals("reasonCode")) { 2355 return addReasonCode(); 2356 } 2357 else if (name.equals("reasonReference")) { 2358 return addReasonReference(); 2359 } 2360 else if (name.equals("note")) { 2361 return addNote(); 2362 } 2363 else 2364 return super.addChild(name); 2365 } 2366 2367 public String fhirType() { 2368 return "CommunicationRequest"; 2369 2370 } 2371 2372 public CommunicationRequest copy() { 2373 CommunicationRequest dst = new CommunicationRequest(); 2374 copyValues(dst); 2375 if (identifier != null) { 2376 dst.identifier = new ArrayList<Identifier>(); 2377 for (Identifier i : identifier) 2378 dst.identifier.add(i.copy()); 2379 }; 2380 if (basedOn != null) { 2381 dst.basedOn = new ArrayList<Reference>(); 2382 for (Reference i : basedOn) 2383 dst.basedOn.add(i.copy()); 2384 }; 2385 if (replaces != null) { 2386 dst.replaces = new ArrayList<Reference>(); 2387 for (Reference i : replaces) 2388 dst.replaces.add(i.copy()); 2389 }; 2390 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2391 dst.status = status == null ? null : status.copy(); 2392 if (category != null) { 2393 dst.category = new ArrayList<CodeableConcept>(); 2394 for (CodeableConcept i : category) 2395 dst.category.add(i.copy()); 2396 }; 2397 dst.priority = priority == null ? null : priority.copy(); 2398 if (medium != null) { 2399 dst.medium = new ArrayList<CodeableConcept>(); 2400 for (CodeableConcept i : medium) 2401 dst.medium.add(i.copy()); 2402 }; 2403 dst.subject = subject == null ? null : subject.copy(); 2404 if (recipient != null) { 2405 dst.recipient = new ArrayList<Reference>(); 2406 for (Reference i : recipient) 2407 dst.recipient.add(i.copy()); 2408 }; 2409 if (topic != null) { 2410 dst.topic = new ArrayList<Reference>(); 2411 for (Reference i : topic) 2412 dst.topic.add(i.copy()); 2413 }; 2414 dst.context = context == null ? null : context.copy(); 2415 if (payload != null) { 2416 dst.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 2417 for (CommunicationRequestPayloadComponent i : payload) 2418 dst.payload.add(i.copy()); 2419 }; 2420 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2421 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2422 dst.sender = sender == null ? null : sender.copy(); 2423 dst.requester = requester == null ? null : requester.copy(); 2424 if (reasonCode != null) { 2425 dst.reasonCode = new ArrayList<CodeableConcept>(); 2426 for (CodeableConcept i : reasonCode) 2427 dst.reasonCode.add(i.copy()); 2428 }; 2429 if (reasonReference != null) { 2430 dst.reasonReference = new ArrayList<Reference>(); 2431 for (Reference i : reasonReference) 2432 dst.reasonReference.add(i.copy()); 2433 }; 2434 if (note != null) { 2435 dst.note = new ArrayList<Annotation>(); 2436 for (Annotation i : note) 2437 dst.note.add(i.copy()); 2438 }; 2439 return dst; 2440 } 2441 2442 protected CommunicationRequest typedCopy() { 2443 return copy(); 2444 } 2445 2446 @Override 2447 public boolean equalsDeep(Base other_) { 2448 if (!super.equalsDeep(other_)) 2449 return false; 2450 if (!(other_ instanceof CommunicationRequest)) 2451 return false; 2452 CommunicationRequest o = (CommunicationRequest) other_; 2453 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) 2454 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(status, o.status, true) 2455 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) && compareDeep(medium, o.medium, true) 2456 && compareDeep(subject, o.subject, true) && compareDeep(recipient, o.recipient, true) && compareDeep(topic, o.topic, true) 2457 && compareDeep(context, o.context, true) && compareDeep(payload, o.payload, true) && compareDeep(occurrence, o.occurrence, true) 2458 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(sender, o.sender, true) && compareDeep(requester, o.requester, true) 2459 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2460 && compareDeep(note, o.note, true); 2461 } 2462 2463 @Override 2464 public boolean equalsShallow(Base other_) { 2465 if (!super.equalsShallow(other_)) 2466 return false; 2467 if (!(other_ instanceof CommunicationRequest)) 2468 return false; 2469 CommunicationRequest o = (CommunicationRequest) other_; 2470 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) && compareValues(authoredOn, o.authoredOn, true) 2471 ; 2472 } 2473 2474 public boolean isEmpty() { 2475 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, replaces 2476 , groupIdentifier, status, category, priority, medium, subject, recipient, topic 2477 , context, payload, occurrence, authoredOn, sender, requester, reasonCode, reasonReference 2478 , note); 2479 } 2480 2481 @Override 2482 public ResourceType getResourceType() { 2483 return ResourceType.CommunicationRequest; 2484 } 2485 2486 /** 2487 * Search parameter: <b>requester</b> 2488 * <p> 2489 * Description: <b>Individual making the request</b><br> 2490 * Type: <b>reference</b><br> 2491 * Path: <b>CommunicationRequest.requester.agent</b><br> 2492 * </p> 2493 */ 2494 @SearchParamDefinition(name="requester", path="CommunicationRequest.requester.agent", description="Individual making the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2495 public static final String SP_REQUESTER = "requester"; 2496 /** 2497 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2498 * <p> 2499 * Description: <b>Individual making the request</b><br> 2500 * Type: <b>reference</b><br> 2501 * Path: <b>CommunicationRequest.requester.agent</b><br> 2502 * </p> 2503 */ 2504 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2505 2506/** 2507 * Constant for fluent queries to be used to add include statements. Specifies 2508 * the path value of "<b>CommunicationRequest:requester</b>". 2509 */ 2510 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("CommunicationRequest:requester").toLocked(); 2511 2512 /** 2513 * Search parameter: <b>authored</b> 2514 * <p> 2515 * Description: <b>When request transitioned to being actionable</b><br> 2516 * Type: <b>date</b><br> 2517 * Path: <b>CommunicationRequest.authoredOn</b><br> 2518 * </p> 2519 */ 2520 @SearchParamDefinition(name="authored", path="CommunicationRequest.authoredOn", description="When request transitioned to being actionable", type="date" ) 2521 public static final String SP_AUTHORED = "authored"; 2522 /** 2523 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2524 * <p> 2525 * Description: <b>When request transitioned to being actionable</b><br> 2526 * Type: <b>date</b><br> 2527 * Path: <b>CommunicationRequest.authoredOn</b><br> 2528 * </p> 2529 */ 2530 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 2531 2532 /** 2533 * Search parameter: <b>identifier</b> 2534 * <p> 2535 * Description: <b>Unique identifier</b><br> 2536 * Type: <b>token</b><br> 2537 * Path: <b>CommunicationRequest.identifier</b><br> 2538 * </p> 2539 */ 2540 @SearchParamDefinition(name="identifier", path="CommunicationRequest.identifier", description="Unique identifier", type="token" ) 2541 public static final String SP_IDENTIFIER = "identifier"; 2542 /** 2543 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2544 * <p> 2545 * Description: <b>Unique identifier</b><br> 2546 * Type: <b>token</b><br> 2547 * Path: <b>CommunicationRequest.identifier</b><br> 2548 * </p> 2549 */ 2550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2551 2552 /** 2553 * Search parameter: <b>subject</b> 2554 * <p> 2555 * Description: <b>Focus of message</b><br> 2556 * Type: <b>reference</b><br> 2557 * Path: <b>CommunicationRequest.subject</b><br> 2558 * </p> 2559 */ 2560 @SearchParamDefinition(name="subject", path="CommunicationRequest.subject", description="Focus of message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2561 public static final String SP_SUBJECT = "subject"; 2562 /** 2563 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2564 * <p> 2565 * Description: <b>Focus of message</b><br> 2566 * Type: <b>reference</b><br> 2567 * Path: <b>CommunicationRequest.subject</b><br> 2568 * </p> 2569 */ 2570 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2571 2572/** 2573 * Constant for fluent queries to be used to add include statements. Specifies 2574 * the path value of "<b>CommunicationRequest:subject</b>". 2575 */ 2576 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("CommunicationRequest:subject").toLocked(); 2577 2578 /** 2579 * Search parameter: <b>replaces</b> 2580 * <p> 2581 * Description: <b>Request(s) replaced by this request</b><br> 2582 * Type: <b>reference</b><br> 2583 * Path: <b>CommunicationRequest.replaces</b><br> 2584 * </p> 2585 */ 2586 @SearchParamDefinition(name="replaces", path="CommunicationRequest.replaces", description="Request(s) replaced by this request", type="reference", target={CommunicationRequest.class } ) 2587 public static final String SP_REPLACES = "replaces"; 2588 /** 2589 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 2590 * <p> 2591 * Description: <b>Request(s) replaced by this request</b><br> 2592 * Type: <b>reference</b><br> 2593 * Path: <b>CommunicationRequest.replaces</b><br> 2594 * </p> 2595 */ 2596 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 2597 2598/** 2599 * Constant for fluent queries to be used to add include statements. Specifies 2600 * the path value of "<b>CommunicationRequest:replaces</b>". 2601 */ 2602 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("CommunicationRequest:replaces").toLocked(); 2603 2604 /** 2605 * Search parameter: <b>medium</b> 2606 * <p> 2607 * Description: <b>A channel of communication</b><br> 2608 * Type: <b>token</b><br> 2609 * Path: <b>CommunicationRequest.medium</b><br> 2610 * </p> 2611 */ 2612 @SearchParamDefinition(name="medium", path="CommunicationRequest.medium", description="A channel of communication", type="token" ) 2613 public static final String SP_MEDIUM = "medium"; 2614 /** 2615 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 2616 * <p> 2617 * Description: <b>A channel of communication</b><br> 2618 * Type: <b>token</b><br> 2619 * Path: <b>CommunicationRequest.medium</b><br> 2620 * </p> 2621 */ 2622 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MEDIUM); 2623 2624 /** 2625 * Search parameter: <b>occurrence</b> 2626 * <p> 2627 * Description: <b>When scheduled</b><br> 2628 * Type: <b>date</b><br> 2629 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 2630 * </p> 2631 */ 2632 @SearchParamDefinition(name="occurrence", path="CommunicationRequest.occurrence.as(DateTime)", description="When scheduled", type="date" ) 2633 public static final String SP_OCCURRENCE = "occurrence"; 2634 /** 2635 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 2636 * <p> 2637 * Description: <b>When scheduled</b><br> 2638 * Type: <b>date</b><br> 2639 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 2640 * </p> 2641 */ 2642 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE); 2643 2644 /** 2645 * Search parameter: <b>encounter</b> 2646 * <p> 2647 * Description: <b>Encounter leading to message</b><br> 2648 * Type: <b>reference</b><br> 2649 * Path: <b>CommunicationRequest.context</b><br> 2650 * </p> 2651 */ 2652 @SearchParamDefinition(name="encounter", path="CommunicationRequest.context", description="Encounter leading to message", type="reference", target={Encounter.class } ) 2653 public static final String SP_ENCOUNTER = "encounter"; 2654 /** 2655 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2656 * <p> 2657 * Description: <b>Encounter leading to message</b><br> 2658 * Type: <b>reference</b><br> 2659 * Path: <b>CommunicationRequest.context</b><br> 2660 * </p> 2661 */ 2662 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2663 2664/** 2665 * Constant for fluent queries to be used to add include statements. Specifies 2666 * the path value of "<b>CommunicationRequest:encounter</b>". 2667 */ 2668 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("CommunicationRequest:encounter").toLocked(); 2669 2670 /** 2671 * Search parameter: <b>priority</b> 2672 * <p> 2673 * Description: <b>Message urgency</b><br> 2674 * Type: <b>token</b><br> 2675 * Path: <b>CommunicationRequest.priority</b><br> 2676 * </p> 2677 */ 2678 @SearchParamDefinition(name="priority", path="CommunicationRequest.priority", description="Message urgency", type="token" ) 2679 public static final String SP_PRIORITY = "priority"; 2680 /** 2681 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 2682 * <p> 2683 * Description: <b>Message urgency</b><br> 2684 * Type: <b>token</b><br> 2685 * Path: <b>CommunicationRequest.priority</b><br> 2686 * </p> 2687 */ 2688 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 2689 2690 /** 2691 * Search parameter: <b>group-identifier</b> 2692 * <p> 2693 * Description: <b>Composite request this is part of</b><br> 2694 * Type: <b>token</b><br> 2695 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 2696 * </p> 2697 */ 2698 @SearchParamDefinition(name="group-identifier", path="CommunicationRequest.groupIdentifier", description="Composite request this is part of", type="token" ) 2699 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 2700 /** 2701 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 2702 * <p> 2703 * Description: <b>Composite request this is part of</b><br> 2704 * Type: <b>token</b><br> 2705 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 2706 * </p> 2707 */ 2708 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 2709 2710 /** 2711 * Search parameter: <b>based-on</b> 2712 * <p> 2713 * Description: <b>Fulfills plan or proposal</b><br> 2714 * Type: <b>reference</b><br> 2715 * Path: <b>CommunicationRequest.basedOn</b><br> 2716 * </p> 2717 */ 2718 @SearchParamDefinition(name="based-on", path="CommunicationRequest.basedOn", description="Fulfills plan or proposal", type="reference" ) 2719 public static final String SP_BASED_ON = "based-on"; 2720 /** 2721 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2722 * <p> 2723 * Description: <b>Fulfills plan or proposal</b><br> 2724 * Type: <b>reference</b><br> 2725 * Path: <b>CommunicationRequest.basedOn</b><br> 2726 * </p> 2727 */ 2728 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2729 2730/** 2731 * Constant for fluent queries to be used to add include statements. Specifies 2732 * the path value of "<b>CommunicationRequest:based-on</b>". 2733 */ 2734 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("CommunicationRequest:based-on").toLocked(); 2735 2736 /** 2737 * Search parameter: <b>sender</b> 2738 * <p> 2739 * Description: <b>Message sender</b><br> 2740 * Type: <b>reference</b><br> 2741 * Path: <b>CommunicationRequest.sender</b><br> 2742 * </p> 2743 */ 2744 @SearchParamDefinition(name="sender", path="CommunicationRequest.sender", description="Message sender", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2745 public static final String SP_SENDER = "sender"; 2746 /** 2747 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 2748 * <p> 2749 * Description: <b>Message sender</b><br> 2750 * Type: <b>reference</b><br> 2751 * Path: <b>CommunicationRequest.sender</b><br> 2752 * </p> 2753 */ 2754 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SENDER); 2755 2756/** 2757 * Constant for fluent queries to be used to add include statements. Specifies 2758 * the path value of "<b>CommunicationRequest:sender</b>". 2759 */ 2760 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include("CommunicationRequest:sender").toLocked(); 2761 2762 /** 2763 * Search parameter: <b>patient</b> 2764 * <p> 2765 * Description: <b>Focus of message</b><br> 2766 * Type: <b>reference</b><br> 2767 * Path: <b>CommunicationRequest.subject</b><br> 2768 * </p> 2769 */ 2770 @SearchParamDefinition(name="patient", path="CommunicationRequest.subject", description="Focus of message", type="reference", target={Patient.class } ) 2771 public static final String SP_PATIENT = "patient"; 2772 /** 2773 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2774 * <p> 2775 * Description: <b>Focus of message</b><br> 2776 * Type: <b>reference</b><br> 2777 * Path: <b>CommunicationRequest.subject</b><br> 2778 * </p> 2779 */ 2780 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2781 2782/** 2783 * Constant for fluent queries to be used to add include statements. Specifies 2784 * the path value of "<b>CommunicationRequest:patient</b>". 2785 */ 2786 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CommunicationRequest:patient").toLocked(); 2787 2788 /** 2789 * Search parameter: <b>recipient</b> 2790 * <p> 2791 * Description: <b>Message recipient</b><br> 2792 * Type: <b>reference</b><br> 2793 * Path: <b>CommunicationRequest.recipient</b><br> 2794 * </p> 2795 */ 2796 @SearchParamDefinition(name="recipient", path="CommunicationRequest.recipient", description="Message recipient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={CareTeam.class, Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2797 public static final String SP_RECIPIENT = "recipient"; 2798 /** 2799 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 2800 * <p> 2801 * Description: <b>Message recipient</b><br> 2802 * Type: <b>reference</b><br> 2803 * Path: <b>CommunicationRequest.recipient</b><br> 2804 * </p> 2805 */ 2806 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 2807 2808/** 2809 * Constant for fluent queries to be used to add include statements. Specifies 2810 * the path value of "<b>CommunicationRequest:recipient</b>". 2811 */ 2812 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("CommunicationRequest:recipient").toLocked(); 2813 2814 /** 2815 * Search parameter: <b>context</b> 2816 * <p> 2817 * Description: <b>Encounter or episode leading to message</b><br> 2818 * Type: <b>reference</b><br> 2819 * Path: <b>CommunicationRequest.context</b><br> 2820 * </p> 2821 */ 2822 @SearchParamDefinition(name="context", path="CommunicationRequest.context", description="Encounter or episode leading to message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2823 public static final String SP_CONTEXT = "context"; 2824 /** 2825 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2826 * <p> 2827 * Description: <b>Encounter or episode leading to message</b><br> 2828 * Type: <b>reference</b><br> 2829 * Path: <b>CommunicationRequest.context</b><br> 2830 * </p> 2831 */ 2832 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2833 2834/** 2835 * Constant for fluent queries to be used to add include statements. Specifies 2836 * the path value of "<b>CommunicationRequest:context</b>". 2837 */ 2838 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("CommunicationRequest:context").toLocked(); 2839 2840 /** 2841 * Search parameter: <b>category</b> 2842 * <p> 2843 * Description: <b>Message category</b><br> 2844 * Type: <b>token</b><br> 2845 * Path: <b>CommunicationRequest.category</b><br> 2846 * </p> 2847 */ 2848 @SearchParamDefinition(name="category", path="CommunicationRequest.category", description="Message category", type="token" ) 2849 public static final String SP_CATEGORY = "category"; 2850 /** 2851 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2852 * <p> 2853 * Description: <b>Message category</b><br> 2854 * Type: <b>token</b><br> 2855 * Path: <b>CommunicationRequest.category</b><br> 2856 * </p> 2857 */ 2858 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2859 2860 /** 2861 * Search parameter: <b>status</b> 2862 * <p> 2863 * Description: <b>draft | active | suspended | cancelled | completed | entered-in-error | unknown</b><br> 2864 * Type: <b>token</b><br> 2865 * Path: <b>CommunicationRequest.status</b><br> 2866 * </p> 2867 */ 2868 @SearchParamDefinition(name="status", path="CommunicationRequest.status", description="draft | active | suspended | cancelled | completed | entered-in-error | unknown", type="token" ) 2869 public static final String SP_STATUS = "status"; 2870 /** 2871 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2872 * <p> 2873 * Description: <b>draft | active | suspended | cancelled | completed | entered-in-error | unknown</b><br> 2874 * Type: <b>token</b><br> 2875 * Path: <b>CommunicationRequest.status</b><br> 2876 * </p> 2877 */ 2878 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2879 2880 2881}