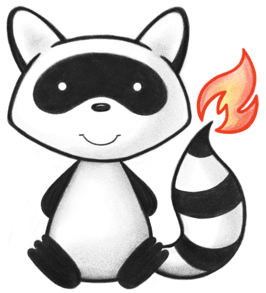
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * A compartment definition that defines how resources are accessed on a server. 053 */ 054@ResourceDef(name="CompartmentDefinition", profile="http://hl7.org/fhir/Profile/CompartmentDefinition") 055@ChildOrder(names={"url", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "purpose", "useContext", "jurisdiction", "code", "search", "resource"}) 056public class CompartmentDefinition extends MetadataResource { 057 058 public enum CompartmentType { 059 /** 060 * The compartment definition is for the patient compartment 061 */ 062 PATIENT, 063 /** 064 * The compartment definition is for the encounter compartment 065 */ 066 ENCOUNTER, 067 /** 068 * The compartment definition is for the related-person compartment 069 */ 070 RELATEDPERSON, 071 /** 072 * The compartment definition is for the practitioner compartment 073 */ 074 PRACTITIONER, 075 /** 076 * The compartment definition is for the device compartment 077 */ 078 DEVICE, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 public static CompartmentType fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("Patient".equals(codeString)) 087 return PATIENT; 088 if ("Encounter".equals(codeString)) 089 return ENCOUNTER; 090 if ("RelatedPerson".equals(codeString)) 091 return RELATEDPERSON; 092 if ("Practitioner".equals(codeString)) 093 return PRACTITIONER; 094 if ("Device".equals(codeString)) 095 return DEVICE; 096 if (Configuration.isAcceptInvalidEnums()) 097 return null; 098 else 099 throw new FHIRException("Unknown CompartmentType code '"+codeString+"'"); 100 } 101 public String toCode() { 102 switch (this) { 103 case PATIENT: return "Patient"; 104 case ENCOUNTER: return "Encounter"; 105 case RELATEDPERSON: return "RelatedPerson"; 106 case PRACTITIONER: return "Practitioner"; 107 case DEVICE: return "Device"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getSystem() { 113 switch (this) { 114 case PATIENT: return "http://hl7.org/fhir/compartment-type"; 115 case ENCOUNTER: return "http://hl7.org/fhir/compartment-type"; 116 case RELATEDPERSON: return "http://hl7.org/fhir/compartment-type"; 117 case PRACTITIONER: return "http://hl7.org/fhir/compartment-type"; 118 case DEVICE: return "http://hl7.org/fhir/compartment-type"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDefinition() { 124 switch (this) { 125 case PATIENT: return "The compartment definition is for the patient compartment"; 126 case ENCOUNTER: return "The compartment definition is for the encounter compartment"; 127 case RELATEDPERSON: return "The compartment definition is for the related-person compartment"; 128 case PRACTITIONER: return "The compartment definition is for the practitioner compartment"; 129 case DEVICE: return "The compartment definition is for the device compartment"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getDisplay() { 135 switch (this) { 136 case PATIENT: return "Patient"; 137 case ENCOUNTER: return "Encounter"; 138 case RELATEDPERSON: return "RelatedPerson"; 139 case PRACTITIONER: return "Practitioner"; 140 case DEVICE: return "Device"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 } 146 147 public static class CompartmentTypeEnumFactory implements EnumFactory<CompartmentType> { 148 public CompartmentType fromCode(String codeString) throws IllegalArgumentException { 149 if (codeString == null || "".equals(codeString)) 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("Patient".equals(codeString)) 153 return CompartmentType.PATIENT; 154 if ("Encounter".equals(codeString)) 155 return CompartmentType.ENCOUNTER; 156 if ("RelatedPerson".equals(codeString)) 157 return CompartmentType.RELATEDPERSON; 158 if ("Practitioner".equals(codeString)) 159 return CompartmentType.PRACTITIONER; 160 if ("Device".equals(codeString)) 161 return CompartmentType.DEVICE; 162 throw new IllegalArgumentException("Unknown CompartmentType code '"+codeString+"'"); 163 } 164 public Enumeration<CompartmentType> fromType(PrimitiveType<?> code) throws FHIRException { 165 if (code == null) 166 return null; 167 if (code.isEmpty()) 168 return new Enumeration<CompartmentType>(this); 169 String codeString = code.asStringValue(); 170 if (codeString == null || "".equals(codeString)) 171 return null; 172 if ("Patient".equals(codeString)) 173 return new Enumeration<CompartmentType>(this, CompartmentType.PATIENT); 174 if ("Encounter".equals(codeString)) 175 return new Enumeration<CompartmentType>(this, CompartmentType.ENCOUNTER); 176 if ("RelatedPerson".equals(codeString)) 177 return new Enumeration<CompartmentType>(this, CompartmentType.RELATEDPERSON); 178 if ("Practitioner".equals(codeString)) 179 return new Enumeration<CompartmentType>(this, CompartmentType.PRACTITIONER); 180 if ("Device".equals(codeString)) 181 return new Enumeration<CompartmentType>(this, CompartmentType.DEVICE); 182 throw new FHIRException("Unknown CompartmentType code '"+codeString+"'"); 183 } 184 public String toCode(CompartmentType code) { 185 if (code == CompartmentType.NULL) 186 return null; 187 if (code == CompartmentType.PATIENT) 188 return "Patient"; 189 if (code == CompartmentType.ENCOUNTER) 190 return "Encounter"; 191 if (code == CompartmentType.RELATEDPERSON) 192 return "RelatedPerson"; 193 if (code == CompartmentType.PRACTITIONER) 194 return "Practitioner"; 195 if (code == CompartmentType.DEVICE) 196 return "Device"; 197 return "?"; 198 } 199 public String toSystem(CompartmentType code) { 200 return code.getSystem(); 201 } 202 } 203 204 @Block() 205 public static class CompartmentDefinitionResourceComponent extends BackboneElement implements IBaseBackboneElement { 206 /** 207 * The name of a resource supported by the server. 208 */ 209 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 210 @Description(shortDefinition="Name of resource type", formalDefinition="The name of a resource supported by the server." ) 211 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 212 protected CodeType code; 213 214 /** 215 * The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,. 216 */ 217 @Child(name = "param", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 218 @Description(shortDefinition="Search Parameter Name, or chained parameters", formalDefinition="The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,." ) 219 protected List<StringType> param; 220 221 /** 222 * Additional documentation about the resource and compartment. 223 */ 224 @Child(name = "documentation", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 225 @Description(shortDefinition="Additional documentation about the resource and compartment", formalDefinition="Additional documentation about the resource and compartment." ) 226 protected StringType documentation; 227 228 private static final long serialVersionUID = 988080897L; 229 230 /** 231 * Constructor 232 */ 233 public CompartmentDefinitionResourceComponent() { 234 super(); 235 } 236 237 /** 238 * Constructor 239 */ 240 public CompartmentDefinitionResourceComponent(CodeType code) { 241 super(); 242 this.code = code; 243 } 244 245 /** 246 * @return {@link #code} (The name of a resource supported by the server.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 247 */ 248 public CodeType getCodeElement() { 249 if (this.code == null) 250 if (Configuration.errorOnAutoCreate()) 251 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.code"); 252 else if (Configuration.doAutoCreate()) 253 this.code = new CodeType(); // bb 254 return this.code; 255 } 256 257 public boolean hasCodeElement() { 258 return this.code != null && !this.code.isEmpty(); 259 } 260 261 public boolean hasCode() { 262 return this.code != null && !this.code.isEmpty(); 263 } 264 265 /** 266 * @param value {@link #code} (The name of a resource supported by the server.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 267 */ 268 public CompartmentDefinitionResourceComponent setCodeElement(CodeType value) { 269 this.code = value; 270 return this; 271 } 272 273 /** 274 * @return The name of a resource supported by the server. 275 */ 276 public String getCode() { 277 return this.code == null ? null : this.code.getValue(); 278 } 279 280 /** 281 * @param value The name of a resource supported by the server. 282 */ 283 public CompartmentDefinitionResourceComponent setCode(String value) { 284 if (this.code == null) 285 this.code = new CodeType(); 286 this.code.setValue(value); 287 return this; 288 } 289 290 /** 291 * @return {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 292 */ 293 public List<StringType> getParam() { 294 if (this.param == null) 295 this.param = new ArrayList<StringType>(); 296 return this.param; 297 } 298 299 /** 300 * @return Returns a reference to <code>this</code> for easy method chaining 301 */ 302 public CompartmentDefinitionResourceComponent setParam(List<StringType> theParam) { 303 this.param = theParam; 304 return this; 305 } 306 307 public boolean hasParam() { 308 if (this.param == null) 309 return false; 310 for (StringType item : this.param) 311 if (!item.isEmpty()) 312 return true; 313 return false; 314 } 315 316 /** 317 * @return {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 318 */ 319 public StringType addParamElement() {//2 320 StringType t = new StringType(); 321 if (this.param == null) 322 this.param = new ArrayList<StringType>(); 323 this.param.add(t); 324 return t; 325 } 326 327 /** 328 * @param value {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 329 */ 330 public CompartmentDefinitionResourceComponent addParam(String value) { //1 331 StringType t = new StringType(); 332 t.setValue(value); 333 if (this.param == null) 334 this.param = new ArrayList<StringType>(); 335 this.param.add(t); 336 return this; 337 } 338 339 /** 340 * @param value {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 341 */ 342 public boolean hasParam(String value) { 343 if (this.param == null) 344 return false; 345 for (StringType v : this.param) 346 if (v.getValue().equals(value)) // string 347 return true; 348 return false; 349 } 350 351 /** 352 * @return {@link #documentation} (Additional documentation about the resource and compartment.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 353 */ 354 public StringType getDocumentationElement() { 355 if (this.documentation == null) 356 if (Configuration.errorOnAutoCreate()) 357 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.documentation"); 358 else if (Configuration.doAutoCreate()) 359 this.documentation = new StringType(); // bb 360 return this.documentation; 361 } 362 363 public boolean hasDocumentationElement() { 364 return this.documentation != null && !this.documentation.isEmpty(); 365 } 366 367 public boolean hasDocumentation() { 368 return this.documentation != null && !this.documentation.isEmpty(); 369 } 370 371 /** 372 * @param value {@link #documentation} (Additional documentation about the resource and compartment.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 373 */ 374 public CompartmentDefinitionResourceComponent setDocumentationElement(StringType value) { 375 this.documentation = value; 376 return this; 377 } 378 379 /** 380 * @return Additional documentation about the resource and compartment. 381 */ 382 public String getDocumentation() { 383 return this.documentation == null ? null : this.documentation.getValue(); 384 } 385 386 /** 387 * @param value Additional documentation about the resource and compartment. 388 */ 389 public CompartmentDefinitionResourceComponent setDocumentation(String value) { 390 if (Utilities.noString(value)) 391 this.documentation = null; 392 else { 393 if (this.documentation == null) 394 this.documentation = new StringType(); 395 this.documentation.setValue(value); 396 } 397 return this; 398 } 399 400 protected void listChildren(List<Property> children) { 401 super.listChildren(children); 402 children.add(new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code)); 403 children.add(new Property("param", "string", "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 0, java.lang.Integer.MAX_VALUE, param)); 404 children.add(new Property("documentation", "string", "Additional documentation about the resource and compartment.", 0, 1, documentation)); 405 } 406 407 @Override 408 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 409 switch (_hash) { 410 case 3059181: /*code*/ return new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code); 411 case 106436749: /*param*/ return new Property("param", "string", "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 0, java.lang.Integer.MAX_VALUE, param); 412 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Additional documentation about the resource and compartment.", 0, 1, documentation); 413 default: return super.getNamedProperty(_hash, _name, _checkValid); 414 } 415 416 } 417 418 @Override 419 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 420 switch (hash) { 421 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 422 case 106436749: /*param*/ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // StringType 423 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 424 default: return super.getProperty(hash, name, checkValid); 425 } 426 427 } 428 429 @Override 430 public Base setProperty(int hash, String name, Base value) throws FHIRException { 431 switch (hash) { 432 case 3059181: // code 433 this.code = castToCode(value); // CodeType 434 return value; 435 case 106436749: // param 436 this.getParam().add(castToString(value)); // StringType 437 return value; 438 case 1587405498: // documentation 439 this.documentation = castToString(value); // StringType 440 return value; 441 default: return super.setProperty(hash, name, value); 442 } 443 444 } 445 446 @Override 447 public Base setProperty(String name, Base value) throws FHIRException { 448 if (name.equals("code")) { 449 this.code = castToCode(value); // CodeType 450 } else if (name.equals("param")) { 451 this.getParam().add(castToString(value)); 452 } else if (name.equals("documentation")) { 453 this.documentation = castToString(value); // StringType 454 } else 455 return super.setProperty(name, value); 456 return value; 457 } 458 459 @Override 460 public Base makeProperty(int hash, String name) throws FHIRException { 461 switch (hash) { 462 case 3059181: return getCodeElement(); 463 case 106436749: return addParamElement(); 464 case 1587405498: return getDocumentationElement(); 465 default: return super.makeProperty(hash, name); 466 } 467 468 } 469 470 @Override 471 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 472 switch (hash) { 473 case 3059181: /*code*/ return new String[] {"code"}; 474 case 106436749: /*param*/ return new String[] {"string"}; 475 case 1587405498: /*documentation*/ return new String[] {"string"}; 476 default: return super.getTypesForProperty(hash, name); 477 } 478 479 } 480 481 @Override 482 public Base addChild(String name) throws FHIRException { 483 if (name.equals("code")) { 484 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.code"); 485 } 486 else if (name.equals("param")) { 487 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.param"); 488 } 489 else if (name.equals("documentation")) { 490 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.documentation"); 491 } 492 else 493 return super.addChild(name); 494 } 495 496 public CompartmentDefinitionResourceComponent copy() { 497 CompartmentDefinitionResourceComponent dst = new CompartmentDefinitionResourceComponent(); 498 copyValues(dst); 499 dst.code = code == null ? null : code.copy(); 500 if (param != null) { 501 dst.param = new ArrayList<StringType>(); 502 for (StringType i : param) 503 dst.param.add(i.copy()); 504 }; 505 dst.documentation = documentation == null ? null : documentation.copy(); 506 return dst; 507 } 508 509 @Override 510 public boolean equalsDeep(Base other_) { 511 if (!super.equalsDeep(other_)) 512 return false; 513 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 514 return false; 515 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 516 return compareDeep(code, o.code, true) && compareDeep(param, o.param, true) && compareDeep(documentation, o.documentation, true) 517 ; 518 } 519 520 @Override 521 public boolean equalsShallow(Base other_) { 522 if (!super.equalsShallow(other_)) 523 return false; 524 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 525 return false; 526 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 527 return compareValues(code, o.code, true) && compareValues(param, o.param, true) && compareValues(documentation, o.documentation, true) 528 ; 529 } 530 531 public boolean isEmpty() { 532 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, param, documentation 533 ); 534 } 535 536 public String fhirType() { 537 return "CompartmentDefinition.resource"; 538 539 } 540 541 } 542 543 /** 544 * Explaination of why this compartment definition is needed and why it has been designed as it has. 545 */ 546 @Child(name = "purpose", type = {MarkdownType.class}, order=0, min=0, max=1, modifier=false, summary=false) 547 @Description(shortDefinition="Why this compartment definition is defined", formalDefinition="Explaination of why this compartment definition is needed and why it has been designed as it has." ) 548 protected MarkdownType purpose; 549 550 /** 551 * Which compartment this definition describes. 552 */ 553 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 554 @Description(shortDefinition="Patient | Encounter | RelatedPerson | Practitioner | Device", formalDefinition="Which compartment this definition describes." ) 555 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/compartment-type") 556 protected Enumeration<CompartmentType> code; 557 558 /** 559 * Whether the search syntax is supported,. 560 */ 561 @Child(name = "search", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=true) 562 @Description(shortDefinition="Whether the search syntax is supported", formalDefinition="Whether the search syntax is supported,." ) 563 protected BooleanType search; 564 565 /** 566 * Information about how a resource is related to the compartment. 567 */ 568 @Child(name = "resource", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 569 @Description(shortDefinition="How a resource is related to the compartment", formalDefinition="Information about how a resource is related to the compartment." ) 570 protected List<CompartmentDefinitionResourceComponent> resource; 571 572 private static final long serialVersionUID = -1159172945L; 573 574 /** 575 * Constructor 576 */ 577 public CompartmentDefinition() { 578 super(); 579 } 580 581 /** 582 * Constructor 583 */ 584 public CompartmentDefinition(UriType url, StringType name, Enumeration<PublicationStatus> status, Enumeration<CompartmentType> code, BooleanType search) { 585 super(); 586 this.url = url; 587 this.name = name; 588 this.status = status; 589 this.code = code; 590 this.search = search; 591 } 592 593 /** 594 * @return {@link #url} (An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this compartment definition is (or will be) published. The URL SHOULD include the major version of the compartment definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 595 */ 596 public UriType getUrlElement() { 597 if (this.url == null) 598 if (Configuration.errorOnAutoCreate()) 599 throw new Error("Attempt to auto-create CompartmentDefinition.url"); 600 else if (Configuration.doAutoCreate()) 601 this.url = new UriType(); // bb 602 return this.url; 603 } 604 605 public boolean hasUrlElement() { 606 return this.url != null && !this.url.isEmpty(); 607 } 608 609 public boolean hasUrl() { 610 return this.url != null && !this.url.isEmpty(); 611 } 612 613 /** 614 * @param value {@link #url} (An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this compartment definition is (or will be) published. The URL SHOULD include the major version of the compartment definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 615 */ 616 public CompartmentDefinition setUrlElement(UriType value) { 617 this.url = value; 618 return this; 619 } 620 621 /** 622 * @return An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this compartment definition is (or will be) published. The URL SHOULD include the major version of the compartment definition. For more information see [Technical and Business Versions](resource.html#versions). 623 */ 624 public String getUrl() { 625 return this.url == null ? null : this.url.getValue(); 626 } 627 628 /** 629 * @param value An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this compartment definition is (or will be) published. The URL SHOULD include the major version of the compartment definition. For more information see [Technical and Business Versions](resource.html#versions). 630 */ 631 public CompartmentDefinition setUrl(String value) { 632 if (this.url == null) 633 this.url = new UriType(); 634 this.url.setValue(value); 635 return this; 636 } 637 638 /** 639 * @return {@link #name} (A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 640 */ 641 public StringType getNameElement() { 642 if (this.name == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create CompartmentDefinition.name"); 645 else if (Configuration.doAutoCreate()) 646 this.name = new StringType(); // bb 647 return this.name; 648 } 649 650 public boolean hasNameElement() { 651 return this.name != null && !this.name.isEmpty(); 652 } 653 654 public boolean hasName() { 655 return this.name != null && !this.name.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #name} (A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 660 */ 661 public CompartmentDefinition setNameElement(StringType value) { 662 this.name = value; 663 return this; 664 } 665 666 /** 667 * @return A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 668 */ 669 public String getName() { 670 return this.name == null ? null : this.name.getValue(); 671 } 672 673 /** 674 * @param value A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 675 */ 676 public CompartmentDefinition setName(String value) { 677 if (this.name == null) 678 this.name = new StringType(); 679 this.name.setValue(value); 680 return this; 681 } 682 683 /** 684 * @return {@link #title} (A short, descriptive, user-friendly title for the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 685 */ 686 public StringType getTitleElement() { 687 if (this.title == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create CompartmentDefinition.title"); 690 else if (Configuration.doAutoCreate()) 691 this.title = new StringType(); // bb 692 return this.title; 693 } 694 695 public boolean hasTitleElement() { 696 return this.title != null && !this.title.isEmpty(); 697 } 698 699 public boolean hasTitle() { 700 return this.title != null && !this.title.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #title} (A short, descriptive, user-friendly title for the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 705 */ 706 public CompartmentDefinition setTitleElement(StringType value) { 707 this.title = value; 708 return this; 709 } 710 711 /** 712 * @return A short, descriptive, user-friendly title for the compartment definition. 713 */ 714 public String getTitle() { 715 return this.title == null ? null : this.title.getValue(); 716 } 717 718 /** 719 * @param value A short, descriptive, user-friendly title for the compartment definition. 720 */ 721 public CompartmentDefinition setTitle(String value) { 722 if (Utilities.noString(value)) 723 this.title = null; 724 else { 725 if (this.title == null) 726 this.title = new StringType(); 727 this.title.setValue(value); 728 } 729 return this; 730 } 731 732 /** 733 * @return {@link #status} (The status of this compartment definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 734 */ 735 public Enumeration<PublicationStatus> getStatusElement() { 736 if (this.status == null) 737 if (Configuration.errorOnAutoCreate()) 738 throw new Error("Attempt to auto-create CompartmentDefinition.status"); 739 else if (Configuration.doAutoCreate()) 740 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 741 return this.status; 742 } 743 744 public boolean hasStatusElement() { 745 return this.status != null && !this.status.isEmpty(); 746 } 747 748 public boolean hasStatus() { 749 return this.status != null && !this.status.isEmpty(); 750 } 751 752 /** 753 * @param value {@link #status} (The status of this compartment definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 754 */ 755 public CompartmentDefinition setStatusElement(Enumeration<PublicationStatus> value) { 756 this.status = value; 757 return this; 758 } 759 760 /** 761 * @return The status of this compartment definition. Enables tracking the life-cycle of the content. 762 */ 763 public PublicationStatus getStatus() { 764 return this.status == null ? null : this.status.getValue(); 765 } 766 767 /** 768 * @param value The status of this compartment definition. Enables tracking the life-cycle of the content. 769 */ 770 public CompartmentDefinition setStatus(PublicationStatus value) { 771 if (this.status == null) 772 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 773 this.status.setValue(value); 774 return this; 775 } 776 777 /** 778 * @return {@link #experimental} (A boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 779 */ 780 public BooleanType getExperimentalElement() { 781 if (this.experimental == null) 782 if (Configuration.errorOnAutoCreate()) 783 throw new Error("Attempt to auto-create CompartmentDefinition.experimental"); 784 else if (Configuration.doAutoCreate()) 785 this.experimental = new BooleanType(); // bb 786 return this.experimental; 787 } 788 789 public boolean hasExperimentalElement() { 790 return this.experimental != null && !this.experimental.isEmpty(); 791 } 792 793 public boolean hasExperimental() { 794 return this.experimental != null && !this.experimental.isEmpty(); 795 } 796 797 /** 798 * @param value {@link #experimental} (A boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 799 */ 800 public CompartmentDefinition setExperimentalElement(BooleanType value) { 801 this.experimental = value; 802 return this; 803 } 804 805 /** 806 * @return A boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 807 */ 808 public boolean getExperimental() { 809 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 810 } 811 812 /** 813 * @param value A boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 814 */ 815 public CompartmentDefinition setExperimental(boolean value) { 816 if (this.experimental == null) 817 this.experimental = new BooleanType(); 818 this.experimental.setValue(value); 819 return this; 820 } 821 822 /** 823 * @return {@link #date} (The date (and optionally time) when the compartment definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 824 */ 825 public DateTimeType getDateElement() { 826 if (this.date == null) 827 if (Configuration.errorOnAutoCreate()) 828 throw new Error("Attempt to auto-create CompartmentDefinition.date"); 829 else if (Configuration.doAutoCreate()) 830 this.date = new DateTimeType(); // bb 831 return this.date; 832 } 833 834 public boolean hasDateElement() { 835 return this.date != null && !this.date.isEmpty(); 836 } 837 838 public boolean hasDate() { 839 return this.date != null && !this.date.isEmpty(); 840 } 841 842 /** 843 * @param value {@link #date} (The date (and optionally time) when the compartment definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 844 */ 845 public CompartmentDefinition setDateElement(DateTimeType value) { 846 this.date = value; 847 return this; 848 } 849 850 /** 851 * @return The date (and optionally time) when the compartment definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes. 852 */ 853 public Date getDate() { 854 return this.date == null ? null : this.date.getValue(); 855 } 856 857 /** 858 * @param value The date (and optionally time) when the compartment definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes. 859 */ 860 public CompartmentDefinition setDate(Date value) { 861 if (value == null) 862 this.date = null; 863 else { 864 if (this.date == null) 865 this.date = new DateTimeType(); 866 this.date.setValue(value); 867 } 868 return this; 869 } 870 871 /** 872 * @return {@link #publisher} (The name of the individual or organization that published the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 873 */ 874 public StringType getPublisherElement() { 875 if (this.publisher == null) 876 if (Configuration.errorOnAutoCreate()) 877 throw new Error("Attempt to auto-create CompartmentDefinition.publisher"); 878 else if (Configuration.doAutoCreate()) 879 this.publisher = new StringType(); // bb 880 return this.publisher; 881 } 882 883 public boolean hasPublisherElement() { 884 return this.publisher != null && !this.publisher.isEmpty(); 885 } 886 887 public boolean hasPublisher() { 888 return this.publisher != null && !this.publisher.isEmpty(); 889 } 890 891 /** 892 * @param value {@link #publisher} (The name of the individual or organization that published the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 893 */ 894 public CompartmentDefinition setPublisherElement(StringType value) { 895 this.publisher = value; 896 return this; 897 } 898 899 /** 900 * @return The name of the individual or organization that published the compartment definition. 901 */ 902 public String getPublisher() { 903 return this.publisher == null ? null : this.publisher.getValue(); 904 } 905 906 /** 907 * @param value The name of the individual or organization that published the compartment definition. 908 */ 909 public CompartmentDefinition setPublisher(String value) { 910 if (Utilities.noString(value)) 911 this.publisher = null; 912 else { 913 if (this.publisher == null) 914 this.publisher = new StringType(); 915 this.publisher.setValue(value); 916 } 917 return this; 918 } 919 920 /** 921 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 922 */ 923 public List<ContactDetail> getContact() { 924 if (this.contact == null) 925 this.contact = new ArrayList<ContactDetail>(); 926 return this.contact; 927 } 928 929 /** 930 * @return Returns a reference to <code>this</code> for easy method chaining 931 */ 932 public CompartmentDefinition setContact(List<ContactDetail> theContact) { 933 this.contact = theContact; 934 return this; 935 } 936 937 public boolean hasContact() { 938 if (this.contact == null) 939 return false; 940 for (ContactDetail item : this.contact) 941 if (!item.isEmpty()) 942 return true; 943 return false; 944 } 945 946 public ContactDetail addContact() { //3 947 ContactDetail t = new ContactDetail(); 948 if (this.contact == null) 949 this.contact = new ArrayList<ContactDetail>(); 950 this.contact.add(t); 951 return t; 952 } 953 954 public CompartmentDefinition addContact(ContactDetail t) { //3 955 if (t == null) 956 return this; 957 if (this.contact == null) 958 this.contact = new ArrayList<ContactDetail>(); 959 this.contact.add(t); 960 return this; 961 } 962 963 /** 964 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 965 */ 966 public ContactDetail getContactFirstRep() { 967 if (getContact().isEmpty()) { 968 addContact(); 969 } 970 return getContact().get(0); 971 } 972 973 /** 974 * @return {@link #description} (A free text natural language description of the compartment definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 975 */ 976 public MarkdownType getDescriptionElement() { 977 if (this.description == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create CompartmentDefinition.description"); 980 else if (Configuration.doAutoCreate()) 981 this.description = new MarkdownType(); // bb 982 return this.description; 983 } 984 985 public boolean hasDescriptionElement() { 986 return this.description != null && !this.description.isEmpty(); 987 } 988 989 public boolean hasDescription() { 990 return this.description != null && !this.description.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #description} (A free text natural language description of the compartment definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 995 */ 996 public CompartmentDefinition setDescriptionElement(MarkdownType value) { 997 this.description = value; 998 return this; 999 } 1000 1001 /** 1002 * @return A free text natural language description of the compartment definition from a consumer's perspective. 1003 */ 1004 public String getDescription() { 1005 return this.description == null ? null : this.description.getValue(); 1006 } 1007 1008 /** 1009 * @param value A free text natural language description of the compartment definition from a consumer's perspective. 1010 */ 1011 public CompartmentDefinition setDescription(String value) { 1012 if (value == null) 1013 this.description = null; 1014 else { 1015 if (this.description == null) 1016 this.description = new MarkdownType(); 1017 this.description.setValue(value); 1018 } 1019 return this; 1020 } 1021 1022 /** 1023 * @return {@link #purpose} (Explaination of why this compartment definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1024 */ 1025 public MarkdownType getPurposeElement() { 1026 if (this.purpose == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create CompartmentDefinition.purpose"); 1029 else if (Configuration.doAutoCreate()) 1030 this.purpose = new MarkdownType(); // bb 1031 return this.purpose; 1032 } 1033 1034 public boolean hasPurposeElement() { 1035 return this.purpose != null && !this.purpose.isEmpty(); 1036 } 1037 1038 public boolean hasPurpose() { 1039 return this.purpose != null && !this.purpose.isEmpty(); 1040 } 1041 1042 /** 1043 * @param value {@link #purpose} (Explaination of why this compartment definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1044 */ 1045 public CompartmentDefinition setPurposeElement(MarkdownType value) { 1046 this.purpose = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return Explaination of why this compartment definition is needed and why it has been designed as it has. 1052 */ 1053 public String getPurpose() { 1054 return this.purpose == null ? null : this.purpose.getValue(); 1055 } 1056 1057 /** 1058 * @param value Explaination of why this compartment definition is needed and why it has been designed as it has. 1059 */ 1060 public CompartmentDefinition setPurpose(String value) { 1061 if (value == null) 1062 this.purpose = null; 1063 else { 1064 if (this.purpose == null) 1065 this.purpose = new MarkdownType(); 1066 this.purpose.setValue(value); 1067 } 1068 return this; 1069 } 1070 1071 /** 1072 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate compartment definition instances.) 1073 */ 1074 public List<UsageContext> getUseContext() { 1075 if (this.useContext == null) 1076 this.useContext = new ArrayList<UsageContext>(); 1077 return this.useContext; 1078 } 1079 1080 /** 1081 * @return Returns a reference to <code>this</code> for easy method chaining 1082 */ 1083 public CompartmentDefinition setUseContext(List<UsageContext> theUseContext) { 1084 this.useContext = theUseContext; 1085 return this; 1086 } 1087 1088 public boolean hasUseContext() { 1089 if (this.useContext == null) 1090 return false; 1091 for (UsageContext item : this.useContext) 1092 if (!item.isEmpty()) 1093 return true; 1094 return false; 1095 } 1096 1097 public UsageContext addUseContext() { //3 1098 UsageContext t = new UsageContext(); 1099 if (this.useContext == null) 1100 this.useContext = new ArrayList<UsageContext>(); 1101 this.useContext.add(t); 1102 return t; 1103 } 1104 1105 public CompartmentDefinition addUseContext(UsageContext t) { //3 1106 if (t == null) 1107 return this; 1108 if (this.useContext == null) 1109 this.useContext = new ArrayList<UsageContext>(); 1110 this.useContext.add(t); 1111 return this; 1112 } 1113 1114 /** 1115 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 1116 */ 1117 public UsageContext getUseContextFirstRep() { 1118 if (getUseContext().isEmpty()) { 1119 addUseContext(); 1120 } 1121 return getUseContext().get(0); 1122 } 1123 1124 /** 1125 * @return {@link #jurisdiction} (A legal or geographic region in which the compartment definition is intended to be used.) 1126 */ 1127 public List<CodeableConcept> getJurisdiction() { 1128 if (this.jurisdiction == null) 1129 this.jurisdiction = new ArrayList<CodeableConcept>(); 1130 return this.jurisdiction; 1131 } 1132 1133 /** 1134 * @return Returns a reference to <code>this</code> for easy method chaining 1135 */ 1136 public CompartmentDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1137 this.jurisdiction = theJurisdiction; 1138 return this; 1139 } 1140 1141 public boolean hasJurisdiction() { 1142 if (this.jurisdiction == null) 1143 return false; 1144 for (CodeableConcept item : this.jurisdiction) 1145 if (!item.isEmpty()) 1146 return true; 1147 return false; 1148 } 1149 1150 public CodeableConcept addJurisdiction() { //3 1151 CodeableConcept t = new CodeableConcept(); 1152 if (this.jurisdiction == null) 1153 this.jurisdiction = new ArrayList<CodeableConcept>(); 1154 this.jurisdiction.add(t); 1155 return t; 1156 } 1157 1158 public CompartmentDefinition addJurisdiction(CodeableConcept t) { //3 1159 if (t == null) 1160 return this; 1161 if (this.jurisdiction == null) 1162 this.jurisdiction = new ArrayList<CodeableConcept>(); 1163 this.jurisdiction.add(t); 1164 return this; 1165 } 1166 1167 /** 1168 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1169 */ 1170 public CodeableConcept getJurisdictionFirstRep() { 1171 if (getJurisdiction().isEmpty()) { 1172 addJurisdiction(); 1173 } 1174 return getJurisdiction().get(0); 1175 } 1176 1177 /** 1178 * @return {@link #code} (Which compartment this definition describes.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1179 */ 1180 public Enumeration<CompartmentType> getCodeElement() { 1181 if (this.code == null) 1182 if (Configuration.errorOnAutoCreate()) 1183 throw new Error("Attempt to auto-create CompartmentDefinition.code"); 1184 else if (Configuration.doAutoCreate()) 1185 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); // bb 1186 return this.code; 1187 } 1188 1189 public boolean hasCodeElement() { 1190 return this.code != null && !this.code.isEmpty(); 1191 } 1192 1193 public boolean hasCode() { 1194 return this.code != null && !this.code.isEmpty(); 1195 } 1196 1197 /** 1198 * @param value {@link #code} (Which compartment this definition describes.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1199 */ 1200 public CompartmentDefinition setCodeElement(Enumeration<CompartmentType> value) { 1201 this.code = value; 1202 return this; 1203 } 1204 1205 /** 1206 * @return Which compartment this definition describes. 1207 */ 1208 public CompartmentType getCode() { 1209 return this.code == null ? null : this.code.getValue(); 1210 } 1211 1212 /** 1213 * @param value Which compartment this definition describes. 1214 */ 1215 public CompartmentDefinition setCode(CompartmentType value) { 1216 if (this.code == null) 1217 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); 1218 this.code.setValue(value); 1219 return this; 1220 } 1221 1222 /** 1223 * @return {@link #search} (Whether the search syntax is supported,.). This is the underlying object with id, value and extensions. The accessor "getSearch" gives direct access to the value 1224 */ 1225 public BooleanType getSearchElement() { 1226 if (this.search == null) 1227 if (Configuration.errorOnAutoCreate()) 1228 throw new Error("Attempt to auto-create CompartmentDefinition.search"); 1229 else if (Configuration.doAutoCreate()) 1230 this.search = new BooleanType(); // bb 1231 return this.search; 1232 } 1233 1234 public boolean hasSearchElement() { 1235 return this.search != null && !this.search.isEmpty(); 1236 } 1237 1238 public boolean hasSearch() { 1239 return this.search != null && !this.search.isEmpty(); 1240 } 1241 1242 /** 1243 * @param value {@link #search} (Whether the search syntax is supported,.). This is the underlying object with id, value and extensions. The accessor "getSearch" gives direct access to the value 1244 */ 1245 public CompartmentDefinition setSearchElement(BooleanType value) { 1246 this.search = value; 1247 return this; 1248 } 1249 1250 /** 1251 * @return Whether the search syntax is supported,. 1252 */ 1253 public boolean getSearch() { 1254 return this.search == null || this.search.isEmpty() ? false : this.search.getValue(); 1255 } 1256 1257 /** 1258 * @param value Whether the search syntax is supported,. 1259 */ 1260 public CompartmentDefinition setSearch(boolean value) { 1261 if (this.search == null) 1262 this.search = new BooleanType(); 1263 this.search.setValue(value); 1264 return this; 1265 } 1266 1267 /** 1268 * @return {@link #resource} (Information about how a resource is related to the compartment.) 1269 */ 1270 public List<CompartmentDefinitionResourceComponent> getResource() { 1271 if (this.resource == null) 1272 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1273 return this.resource; 1274 } 1275 1276 /** 1277 * @return Returns a reference to <code>this</code> for easy method chaining 1278 */ 1279 public CompartmentDefinition setResource(List<CompartmentDefinitionResourceComponent> theResource) { 1280 this.resource = theResource; 1281 return this; 1282 } 1283 1284 public boolean hasResource() { 1285 if (this.resource == null) 1286 return false; 1287 for (CompartmentDefinitionResourceComponent item : this.resource) 1288 if (!item.isEmpty()) 1289 return true; 1290 return false; 1291 } 1292 1293 public CompartmentDefinitionResourceComponent addResource() { //3 1294 CompartmentDefinitionResourceComponent t = new CompartmentDefinitionResourceComponent(); 1295 if (this.resource == null) 1296 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1297 this.resource.add(t); 1298 return t; 1299 } 1300 1301 public CompartmentDefinition addResource(CompartmentDefinitionResourceComponent t) { //3 1302 if (t == null) 1303 return this; 1304 if (this.resource == null) 1305 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1306 this.resource.add(t); 1307 return this; 1308 } 1309 1310 /** 1311 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist 1312 */ 1313 public CompartmentDefinitionResourceComponent getResourceFirstRep() { 1314 if (getResource().isEmpty()) { 1315 addResource(); 1316 } 1317 return getResource().get(0); 1318 } 1319 1320 protected void listChildren(List<Property> children) { 1321 super.listChildren(children); 1322 children.add(new Property("url", "uri", "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this compartment definition is (or will be) published. The URL SHOULD include the major version of the compartment definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 1323 children.add(new Property("name", "string", "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1324 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the compartment definition.", 0, 1, title)); 1325 children.add(new Property("status", "code", "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1326 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 1327 children.add(new Property("date", "dateTime", "The date (and optionally time) when the compartment definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 0, 1, date)); 1328 children.add(new Property("publisher", "string", "The name of the individual or organization that published the compartment definition.", 0, 1, publisher)); 1329 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1330 children.add(new Property("description", "markdown", "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, description)); 1331 children.add(new Property("purpose", "markdown", "Explaination of why this compartment definition is needed and why it has been designed as it has.", 0, 1, purpose)); 1332 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate compartment definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1333 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the compartment definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1334 children.add(new Property("code", "code", "Which compartment this definition describes.", 0, 1, code)); 1335 children.add(new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search)); 1336 children.add(new Property("resource", "", "Information about how a resource is related to the compartment.", 0, java.lang.Integer.MAX_VALUE, resource)); 1337 } 1338 1339 @Override 1340 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1341 switch (_hash) { 1342 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this compartment definition is (or will be) published. The URL SHOULD include the major version of the compartment definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 1343 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1344 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the compartment definition.", 0, 1, title); 1345 case -892481550: /*status*/ return new Property("status", "code", "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1346 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 1347 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the compartment definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 0, 1, date); 1348 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the compartment definition.", 0, 1, publisher); 1349 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1350 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, description); 1351 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this compartment definition is needed and why it has been designed as it has.", 0, 1, purpose); 1352 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate compartment definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1353 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the compartment definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1354 case 3059181: /*code*/ return new Property("code", "code", "Which compartment this definition describes.", 0, 1, code); 1355 case -906336856: /*search*/ return new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search); 1356 case -341064690: /*resource*/ return new Property("resource", "", "Information about how a resource is related to the compartment.", 0, java.lang.Integer.MAX_VALUE, resource); 1357 default: return super.getNamedProperty(_hash, _name, _checkValid); 1358 } 1359 1360 } 1361 1362 @Override 1363 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1364 switch (hash) { 1365 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1366 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1367 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1368 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1369 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 1370 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1371 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1372 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1373 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1374 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 1375 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1376 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1377 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<CompartmentType> 1378 case -906336856: /*search*/ return this.search == null ? new Base[0] : new Base[] {this.search}; // BooleanType 1379 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CompartmentDefinitionResourceComponent 1380 default: return super.getProperty(hash, name, checkValid); 1381 } 1382 1383 } 1384 1385 @Override 1386 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1387 switch (hash) { 1388 case 116079: // url 1389 this.url = castToUri(value); // UriType 1390 return value; 1391 case 3373707: // name 1392 this.name = castToString(value); // StringType 1393 return value; 1394 case 110371416: // title 1395 this.title = castToString(value); // StringType 1396 return value; 1397 case -892481550: // status 1398 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1399 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1400 return value; 1401 case -404562712: // experimental 1402 this.experimental = castToBoolean(value); // BooleanType 1403 return value; 1404 case 3076014: // date 1405 this.date = castToDateTime(value); // DateTimeType 1406 return value; 1407 case 1447404028: // publisher 1408 this.publisher = castToString(value); // StringType 1409 return value; 1410 case 951526432: // contact 1411 this.getContact().add(castToContactDetail(value)); // ContactDetail 1412 return value; 1413 case -1724546052: // description 1414 this.description = castToMarkdown(value); // MarkdownType 1415 return value; 1416 case -220463842: // purpose 1417 this.purpose = castToMarkdown(value); // MarkdownType 1418 return value; 1419 case -669707736: // useContext 1420 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1421 return value; 1422 case -507075711: // jurisdiction 1423 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1424 return value; 1425 case 3059181: // code 1426 value = new CompartmentTypeEnumFactory().fromType(castToCode(value)); 1427 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1428 return value; 1429 case -906336856: // search 1430 this.search = castToBoolean(value); // BooleanType 1431 return value; 1432 case -341064690: // resource 1433 this.getResource().add((CompartmentDefinitionResourceComponent) value); // CompartmentDefinitionResourceComponent 1434 return value; 1435 default: return super.setProperty(hash, name, value); 1436 } 1437 1438 } 1439 1440 @Override 1441 public Base setProperty(String name, Base value) throws FHIRException { 1442 if (name.equals("url")) { 1443 this.url = castToUri(value); // UriType 1444 } else if (name.equals("name")) { 1445 this.name = castToString(value); // StringType 1446 } else if (name.equals("title")) { 1447 this.title = castToString(value); // StringType 1448 } else if (name.equals("status")) { 1449 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1450 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1451 } else if (name.equals("experimental")) { 1452 this.experimental = castToBoolean(value); // BooleanType 1453 } else if (name.equals("date")) { 1454 this.date = castToDateTime(value); // DateTimeType 1455 } else if (name.equals("publisher")) { 1456 this.publisher = castToString(value); // StringType 1457 } else if (name.equals("contact")) { 1458 this.getContact().add(castToContactDetail(value)); 1459 } else if (name.equals("description")) { 1460 this.description = castToMarkdown(value); // MarkdownType 1461 } else if (name.equals("purpose")) { 1462 this.purpose = castToMarkdown(value); // MarkdownType 1463 } else if (name.equals("useContext")) { 1464 this.getUseContext().add(castToUsageContext(value)); 1465 } else if (name.equals("jurisdiction")) { 1466 this.getJurisdiction().add(castToCodeableConcept(value)); 1467 } else if (name.equals("code")) { 1468 value = new CompartmentTypeEnumFactory().fromType(castToCode(value)); 1469 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1470 } else if (name.equals("search")) { 1471 this.search = castToBoolean(value); // BooleanType 1472 } else if (name.equals("resource")) { 1473 this.getResource().add((CompartmentDefinitionResourceComponent) value); 1474 } else 1475 return super.setProperty(name, value); 1476 return value; 1477 } 1478 1479 @Override 1480 public Base makeProperty(int hash, String name) throws FHIRException { 1481 switch (hash) { 1482 case 116079: return getUrlElement(); 1483 case 3373707: return getNameElement(); 1484 case 110371416: return getTitleElement(); 1485 case -892481550: return getStatusElement(); 1486 case -404562712: return getExperimentalElement(); 1487 case 3076014: return getDateElement(); 1488 case 1447404028: return getPublisherElement(); 1489 case 951526432: return addContact(); 1490 case -1724546052: return getDescriptionElement(); 1491 case -220463842: return getPurposeElement(); 1492 case -669707736: return addUseContext(); 1493 case -507075711: return addJurisdiction(); 1494 case 3059181: return getCodeElement(); 1495 case -906336856: return getSearchElement(); 1496 case -341064690: return addResource(); 1497 default: return super.makeProperty(hash, name); 1498 } 1499 1500 } 1501 1502 @Override 1503 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1504 switch (hash) { 1505 case 116079: /*url*/ return new String[] {"uri"}; 1506 case 3373707: /*name*/ return new String[] {"string"}; 1507 case 110371416: /*title*/ return new String[] {"string"}; 1508 case -892481550: /*status*/ return new String[] {"code"}; 1509 case -404562712: /*experimental*/ return new String[] {"boolean"}; 1510 case 3076014: /*date*/ return new String[] {"dateTime"}; 1511 case 1447404028: /*publisher*/ return new String[] {"string"}; 1512 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1513 case -1724546052: /*description*/ return new String[] {"markdown"}; 1514 case -220463842: /*purpose*/ return new String[] {"markdown"}; 1515 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 1516 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1517 case 3059181: /*code*/ return new String[] {"code"}; 1518 case -906336856: /*search*/ return new String[] {"boolean"}; 1519 case -341064690: /*resource*/ return new String[] {}; 1520 default: return super.getTypesForProperty(hash, name); 1521 } 1522 1523 } 1524 1525 @Override 1526 public Base addChild(String name) throws FHIRException { 1527 if (name.equals("url")) { 1528 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.url"); 1529 } 1530 else if (name.equals("name")) { 1531 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.name"); 1532 } 1533 else if (name.equals("title")) { 1534 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.title"); 1535 } 1536 else if (name.equals("status")) { 1537 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.status"); 1538 } 1539 else if (name.equals("experimental")) { 1540 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.experimental"); 1541 } 1542 else if (name.equals("date")) { 1543 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.date"); 1544 } 1545 else if (name.equals("publisher")) { 1546 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.publisher"); 1547 } 1548 else if (name.equals("contact")) { 1549 return addContact(); 1550 } 1551 else if (name.equals("description")) { 1552 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.description"); 1553 } 1554 else if (name.equals("purpose")) { 1555 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.purpose"); 1556 } 1557 else if (name.equals("useContext")) { 1558 return addUseContext(); 1559 } 1560 else if (name.equals("jurisdiction")) { 1561 return addJurisdiction(); 1562 } 1563 else if (name.equals("code")) { 1564 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.code"); 1565 } 1566 else if (name.equals("search")) { 1567 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.search"); 1568 } 1569 else if (name.equals("resource")) { 1570 return addResource(); 1571 } 1572 else 1573 return super.addChild(name); 1574 } 1575 1576 public String fhirType() { 1577 return "CompartmentDefinition"; 1578 1579 } 1580 1581 public CompartmentDefinition copy() { 1582 CompartmentDefinition dst = new CompartmentDefinition(); 1583 copyValues(dst); 1584 dst.url = url == null ? null : url.copy(); 1585 dst.name = name == null ? null : name.copy(); 1586 dst.title = title == null ? null : title.copy(); 1587 dst.status = status == null ? null : status.copy(); 1588 dst.experimental = experimental == null ? null : experimental.copy(); 1589 dst.date = date == null ? null : date.copy(); 1590 dst.publisher = publisher == null ? null : publisher.copy(); 1591 if (contact != null) { 1592 dst.contact = new ArrayList<ContactDetail>(); 1593 for (ContactDetail i : contact) 1594 dst.contact.add(i.copy()); 1595 }; 1596 dst.description = description == null ? null : description.copy(); 1597 dst.purpose = purpose == null ? null : purpose.copy(); 1598 if (useContext != null) { 1599 dst.useContext = new ArrayList<UsageContext>(); 1600 for (UsageContext i : useContext) 1601 dst.useContext.add(i.copy()); 1602 }; 1603 if (jurisdiction != null) { 1604 dst.jurisdiction = new ArrayList<CodeableConcept>(); 1605 for (CodeableConcept i : jurisdiction) 1606 dst.jurisdiction.add(i.copy()); 1607 }; 1608 dst.code = code == null ? null : code.copy(); 1609 dst.search = search == null ? null : search.copy(); 1610 if (resource != null) { 1611 dst.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1612 for (CompartmentDefinitionResourceComponent i : resource) 1613 dst.resource.add(i.copy()); 1614 }; 1615 return dst; 1616 } 1617 1618 protected CompartmentDefinition typedCopy() { 1619 return copy(); 1620 } 1621 1622 @Override 1623 public boolean equalsDeep(Base other_) { 1624 if (!super.equalsDeep(other_)) 1625 return false; 1626 if (!(other_ instanceof CompartmentDefinition)) 1627 return false; 1628 CompartmentDefinition o = (CompartmentDefinition) other_; 1629 return compareDeep(purpose, o.purpose, true) && compareDeep(code, o.code, true) && compareDeep(search, o.search, true) 1630 && compareDeep(resource, o.resource, true); 1631 } 1632 1633 @Override 1634 public boolean equalsShallow(Base other_) { 1635 if (!super.equalsShallow(other_)) 1636 return false; 1637 if (!(other_ instanceof CompartmentDefinition)) 1638 return false; 1639 CompartmentDefinition o = (CompartmentDefinition) other_; 1640 return compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) && compareValues(search, o.search, true) 1641 ; 1642 } 1643 1644 public boolean isEmpty() { 1645 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, code, search, resource 1646 ); 1647 } 1648 1649 @Override 1650 public ResourceType getResourceType() { 1651 return ResourceType.CompartmentDefinition; 1652 } 1653 1654 /** 1655 * Search parameter: <b>date</b> 1656 * <p> 1657 * Description: <b>The compartment definition publication date</b><br> 1658 * Type: <b>date</b><br> 1659 * Path: <b>CompartmentDefinition.date</b><br> 1660 * </p> 1661 */ 1662 @SearchParamDefinition(name="date", path="CompartmentDefinition.date", description="The compartment definition publication date", type="date" ) 1663 public static final String SP_DATE = "date"; 1664 /** 1665 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1666 * <p> 1667 * Description: <b>The compartment definition publication date</b><br> 1668 * Type: <b>date</b><br> 1669 * Path: <b>CompartmentDefinition.date</b><br> 1670 * </p> 1671 */ 1672 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1673 1674 /** 1675 * Search parameter: <b>code</b> 1676 * <p> 1677 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | Device</b><br> 1678 * Type: <b>token</b><br> 1679 * Path: <b>CompartmentDefinition.code</b><br> 1680 * </p> 1681 */ 1682 @SearchParamDefinition(name="code", path="CompartmentDefinition.code", description="Patient | Encounter | RelatedPerson | Practitioner | Device", type="token" ) 1683 public static final String SP_CODE = "code"; 1684 /** 1685 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1686 * <p> 1687 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | Device</b><br> 1688 * Type: <b>token</b><br> 1689 * Path: <b>CompartmentDefinition.code</b><br> 1690 * </p> 1691 */ 1692 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1693 1694 /** 1695 * Search parameter: <b>resource</b> 1696 * <p> 1697 * Description: <b>Name of resource type</b><br> 1698 * Type: <b>token</b><br> 1699 * Path: <b>CompartmentDefinition.resource.code</b><br> 1700 * </p> 1701 */ 1702 @SearchParamDefinition(name="resource", path="CompartmentDefinition.resource.code", description="Name of resource type", type="token" ) 1703 public static final String SP_RESOURCE = "resource"; 1704 /** 1705 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 1706 * <p> 1707 * Description: <b>Name of resource type</b><br> 1708 * Type: <b>token</b><br> 1709 * Path: <b>CompartmentDefinition.resource.code</b><br> 1710 * </p> 1711 */ 1712 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESOURCE); 1713 1714 /** 1715 * Search parameter: <b>jurisdiction</b> 1716 * <p> 1717 * Description: <b>Intended jurisdiction for the compartment definition</b><br> 1718 * Type: <b>token</b><br> 1719 * Path: <b>CompartmentDefinition.jurisdiction</b><br> 1720 * </p> 1721 */ 1722 @SearchParamDefinition(name="jurisdiction", path="CompartmentDefinition.jurisdiction", description="Intended jurisdiction for the compartment definition", type="token" ) 1723 public static final String SP_JURISDICTION = "jurisdiction"; 1724 /** 1725 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 1726 * <p> 1727 * Description: <b>Intended jurisdiction for the compartment definition</b><br> 1728 * Type: <b>token</b><br> 1729 * Path: <b>CompartmentDefinition.jurisdiction</b><br> 1730 * </p> 1731 */ 1732 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 1733 1734 /** 1735 * Search parameter: <b>name</b> 1736 * <p> 1737 * Description: <b>Computationally friendly name of the compartment definition</b><br> 1738 * Type: <b>string</b><br> 1739 * Path: <b>CompartmentDefinition.name</b><br> 1740 * </p> 1741 */ 1742 @SearchParamDefinition(name="name", path="CompartmentDefinition.name", description="Computationally friendly name of the compartment definition", type="string" ) 1743 public static final String SP_NAME = "name"; 1744 /** 1745 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1746 * <p> 1747 * Description: <b>Computationally friendly name of the compartment definition</b><br> 1748 * Type: <b>string</b><br> 1749 * Path: <b>CompartmentDefinition.name</b><br> 1750 * </p> 1751 */ 1752 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1753 1754 /** 1755 * Search parameter: <b>description</b> 1756 * <p> 1757 * Description: <b>The description of the compartment definition</b><br> 1758 * Type: <b>string</b><br> 1759 * Path: <b>CompartmentDefinition.description</b><br> 1760 * </p> 1761 */ 1762 @SearchParamDefinition(name="description", path="CompartmentDefinition.description", description="The description of the compartment definition", type="string" ) 1763 public static final String SP_DESCRIPTION = "description"; 1764 /** 1765 * <b>Fluent Client</b> search parameter constant for <b>description</b> 1766 * <p> 1767 * Description: <b>The description of the compartment definition</b><br> 1768 * Type: <b>string</b><br> 1769 * Path: <b>CompartmentDefinition.description</b><br> 1770 * </p> 1771 */ 1772 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 1773 1774 /** 1775 * Search parameter: <b>publisher</b> 1776 * <p> 1777 * Description: <b>Name of the publisher of the compartment definition</b><br> 1778 * Type: <b>string</b><br> 1779 * Path: <b>CompartmentDefinition.publisher</b><br> 1780 * </p> 1781 */ 1782 @SearchParamDefinition(name="publisher", path="CompartmentDefinition.publisher", description="Name of the publisher of the compartment definition", type="string" ) 1783 public static final String SP_PUBLISHER = "publisher"; 1784 /** 1785 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 1786 * <p> 1787 * Description: <b>Name of the publisher of the compartment definition</b><br> 1788 * Type: <b>string</b><br> 1789 * Path: <b>CompartmentDefinition.publisher</b><br> 1790 * </p> 1791 */ 1792 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 1793 1794 /** 1795 * Search parameter: <b>title</b> 1796 * <p> 1797 * Description: <b>The human-friendly name of the compartment definition</b><br> 1798 * Type: <b>string</b><br> 1799 * Path: <b>CompartmentDefinition.title</b><br> 1800 * </p> 1801 */ 1802 @SearchParamDefinition(name="title", path="CompartmentDefinition.title", description="The human-friendly name of the compartment definition", type="string" ) 1803 public static final String SP_TITLE = "title"; 1804 /** 1805 * <b>Fluent Client</b> search parameter constant for <b>title</b> 1806 * <p> 1807 * Description: <b>The human-friendly name of the compartment definition</b><br> 1808 * Type: <b>string</b><br> 1809 * Path: <b>CompartmentDefinition.title</b><br> 1810 * </p> 1811 */ 1812 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 1813 1814 /** 1815 * Search parameter: <b>url</b> 1816 * <p> 1817 * Description: <b>The uri that identifies the compartment definition</b><br> 1818 * Type: <b>uri</b><br> 1819 * Path: <b>CompartmentDefinition.url</b><br> 1820 * </p> 1821 */ 1822 @SearchParamDefinition(name="url", path="CompartmentDefinition.url", description="The uri that identifies the compartment definition", type="uri" ) 1823 public static final String SP_URL = "url"; 1824 /** 1825 * <b>Fluent Client</b> search parameter constant for <b>url</b> 1826 * <p> 1827 * Description: <b>The uri that identifies the compartment definition</b><br> 1828 * Type: <b>uri</b><br> 1829 * Path: <b>CompartmentDefinition.url</b><br> 1830 * </p> 1831 */ 1832 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 1833 1834 /** 1835 * Search parameter: <b>status</b> 1836 * <p> 1837 * Description: <b>The current status of the compartment definition</b><br> 1838 * Type: <b>token</b><br> 1839 * Path: <b>CompartmentDefinition.status</b><br> 1840 * </p> 1841 */ 1842 @SearchParamDefinition(name="status", path="CompartmentDefinition.status", description="The current status of the compartment definition", type="token" ) 1843 public static final String SP_STATUS = "status"; 1844 /** 1845 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1846 * <p> 1847 * Description: <b>The current status of the compartment definition</b><br> 1848 * Type: <b>token</b><br> 1849 * Path: <b>CompartmentDefinition.status</b><br> 1850 * </p> 1851 */ 1852 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1853 1854 1855}