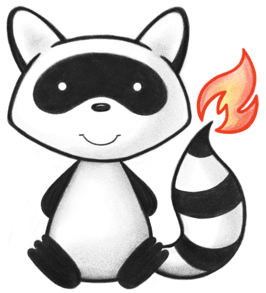
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 051 */ 052@ResourceDef(name="Composition", profile="http://hl7.org/fhir/Profile/Composition") 053public class Composition extends DomainResource { 054 055 public enum CompositionStatus { 056 /** 057 * This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified. 058 */ 059 PRELIMINARY, 060 /** 061 * This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition. 062 */ 063 FINAL, 064 /** 065 * The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as "final" and the composition is complete and verified by an authorized person. 066 */ 067 AMENDED, 068 /** 069 * The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static CompositionStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("preliminary".equals(codeString)) 080 return PRELIMINARY; 081 if ("final".equals(codeString)) 082 return FINAL; 083 if ("amended".equals(codeString)) 084 return AMENDED; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown CompositionStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case PRELIMINARY: return "preliminary"; 095 case FINAL: return "final"; 096 case AMENDED: return "amended"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case PRELIMINARY: return "http://hl7.org/fhir/composition-status"; 105 case FINAL: return "http://hl7.org/fhir/composition-status"; 106 case AMENDED: return "http://hl7.org/fhir/composition-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/composition-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case PRELIMINARY: return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 115 case FINAL: return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 116 case AMENDED: return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 117 case ENTEREDINERROR: return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case PRELIMINARY: return "Preliminary"; 125 case FINAL: return "Final"; 126 case AMENDED: return "Amended"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class CompositionStatusEnumFactory implements EnumFactory<CompositionStatus> { 135 public CompositionStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("preliminary".equals(codeString)) 140 return CompositionStatus.PRELIMINARY; 141 if ("final".equals(codeString)) 142 return CompositionStatus.FINAL; 143 if ("amended".equals(codeString)) 144 return CompositionStatus.AMENDED; 145 if ("entered-in-error".equals(codeString)) 146 return CompositionStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown CompositionStatus code '"+codeString+"'"); 148 } 149 public Enumeration<CompositionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<CompositionStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("preliminary".equals(codeString)) 158 return new Enumeration<CompositionStatus>(this, CompositionStatus.PRELIMINARY); 159 if ("final".equals(codeString)) 160 return new Enumeration<CompositionStatus>(this, CompositionStatus.FINAL); 161 if ("amended".equals(codeString)) 162 return new Enumeration<CompositionStatus>(this, CompositionStatus.AMENDED); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<CompositionStatus>(this, CompositionStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown CompositionStatus code '"+codeString+"'"); 166 } 167 public String toCode(CompositionStatus code) { 168 if (code == CompositionStatus.NULL) 169 return null; 170 if (code == CompositionStatus.PRELIMINARY) 171 return "preliminary"; 172 if (code == CompositionStatus.FINAL) 173 return "final"; 174 if (code == CompositionStatus.AMENDED) 175 return "amended"; 176 if (code == CompositionStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(CompositionStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 public enum DocumentConfidentiality { 186 /** 187 * null 188 */ 189 U, 190 /** 191 * null 192 */ 193 L, 194 /** 195 * null 196 */ 197 M, 198 /** 199 * null 200 */ 201 N, 202 /** 203 * null 204 */ 205 R, 206 /** 207 * null 208 */ 209 V, 210 /** 211 * added to help the parsers with the generic types 212 */ 213 NULL; 214 public static DocumentConfidentiality fromCode(String codeString) throws FHIRException { 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("U".equals(codeString)) 218 return U; 219 if ("L".equals(codeString)) 220 return L; 221 if ("M".equals(codeString)) 222 return M; 223 if ("N".equals(codeString)) 224 return N; 225 if ("R".equals(codeString)) 226 return R; 227 if ("V".equals(codeString)) 228 return V; 229 if (Configuration.isAcceptInvalidEnums()) 230 return null; 231 else 232 throw new FHIRException("Unknown DocumentConfidentiality code '"+codeString+"'"); 233 } 234 public String toCode() { 235 switch (this) { 236 case U: return "U"; 237 case L: return "L"; 238 case M: return "M"; 239 case N: return "N"; 240 case R: return "R"; 241 case V: return "V"; 242 case NULL: return null; 243 default: return "?"; 244 } 245 } 246 public String getSystem() { 247 switch (this) { 248 case U: return "http://hl7.org/fhir/v3/Confidentiality"; 249 case L: return "http://hl7.org/fhir/v3/Confidentiality"; 250 case M: return "http://hl7.org/fhir/v3/Confidentiality"; 251 case N: return "http://hl7.org/fhir/v3/Confidentiality"; 252 case R: return "http://hl7.org/fhir/v3/Confidentiality"; 253 case V: return "http://hl7.org/fhir/v3/Confidentiality"; 254 case NULL: return null; 255 default: return "?"; 256 } 257 } 258 public String getDefinition() { 259 switch (this) { 260 case U: return ""; 261 case L: return ""; 262 case M: return ""; 263 case N: return ""; 264 case R: return ""; 265 case V: return ""; 266 case NULL: return null; 267 default: return "?"; 268 } 269 } 270 public String getDisplay() { 271 switch (this) { 272 case U: return "U"; 273 case L: return "L"; 274 case M: return "M"; 275 case N: return "N"; 276 case R: return "R"; 277 case V: return "V"; 278 case NULL: return null; 279 default: return "?"; 280 } 281 } 282 } 283 284 public static class DocumentConfidentialityEnumFactory implements EnumFactory<DocumentConfidentiality> { 285 public DocumentConfidentiality fromCode(String codeString) throws IllegalArgumentException { 286 if (codeString == null || "".equals(codeString)) 287 if (codeString == null || "".equals(codeString)) 288 return null; 289 if ("U".equals(codeString)) 290 return DocumentConfidentiality.U; 291 if ("L".equals(codeString)) 292 return DocumentConfidentiality.L; 293 if ("M".equals(codeString)) 294 return DocumentConfidentiality.M; 295 if ("N".equals(codeString)) 296 return DocumentConfidentiality.N; 297 if ("R".equals(codeString)) 298 return DocumentConfidentiality.R; 299 if ("V".equals(codeString)) 300 return DocumentConfidentiality.V; 301 throw new IllegalArgumentException("Unknown DocumentConfidentiality code '"+codeString+"'"); 302 } 303 public Enumeration<DocumentConfidentiality> fromType(PrimitiveType<?> code) throws FHIRException { 304 if (code == null) 305 return null; 306 if (code.isEmpty()) 307 return new Enumeration<DocumentConfidentiality>(this); 308 String codeString = code.asStringValue(); 309 if (codeString == null || "".equals(codeString)) 310 return null; 311 if ("U".equals(codeString)) 312 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.U); 313 if ("L".equals(codeString)) 314 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.L); 315 if ("M".equals(codeString)) 316 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.M); 317 if ("N".equals(codeString)) 318 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.N); 319 if ("R".equals(codeString)) 320 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.R); 321 if ("V".equals(codeString)) 322 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.V); 323 throw new FHIRException("Unknown DocumentConfidentiality code '"+codeString+"'"); 324 } 325 public String toCode(DocumentConfidentiality code) { 326 if (code == DocumentConfidentiality.NULL) 327 return null; 328 if (code == DocumentConfidentiality.U) 329 return "U"; 330 if (code == DocumentConfidentiality.L) 331 return "L"; 332 if (code == DocumentConfidentiality.M) 333 return "M"; 334 if (code == DocumentConfidentiality.N) 335 return "N"; 336 if (code == DocumentConfidentiality.R) 337 return "R"; 338 if (code == DocumentConfidentiality.V) 339 return "V"; 340 return "?"; 341 } 342 public String toSystem(DocumentConfidentiality code) { 343 return code.getSystem(); 344 } 345 } 346 347 public enum CompositionAttestationMode { 348 /** 349 * The person authenticated the content in their personal capacity. 350 */ 351 PERSONAL, 352 /** 353 * The person authenticated the content in their professional capacity. 354 */ 355 PROFESSIONAL, 356 /** 357 * The person authenticated the content and accepted legal responsibility for its content. 358 */ 359 LEGAL, 360 /** 361 * The organization authenticated the content as consistent with their policies and procedures. 362 */ 363 OFFICIAL, 364 /** 365 * added to help the parsers with the generic types 366 */ 367 NULL; 368 public static CompositionAttestationMode fromCode(String codeString) throws FHIRException { 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("personal".equals(codeString)) 372 return PERSONAL; 373 if ("professional".equals(codeString)) 374 return PROFESSIONAL; 375 if ("legal".equals(codeString)) 376 return LEGAL; 377 if ("official".equals(codeString)) 378 return OFFICIAL; 379 if (Configuration.isAcceptInvalidEnums()) 380 return null; 381 else 382 throw new FHIRException("Unknown CompositionAttestationMode code '"+codeString+"'"); 383 } 384 public String toCode() { 385 switch (this) { 386 case PERSONAL: return "personal"; 387 case PROFESSIONAL: return "professional"; 388 case LEGAL: return "legal"; 389 case OFFICIAL: return "official"; 390 case NULL: return null; 391 default: return "?"; 392 } 393 } 394 public String getSystem() { 395 switch (this) { 396 case PERSONAL: return "http://hl7.org/fhir/composition-attestation-mode"; 397 case PROFESSIONAL: return "http://hl7.org/fhir/composition-attestation-mode"; 398 case LEGAL: return "http://hl7.org/fhir/composition-attestation-mode"; 399 case OFFICIAL: return "http://hl7.org/fhir/composition-attestation-mode"; 400 case NULL: return null; 401 default: return "?"; 402 } 403 } 404 public String getDefinition() { 405 switch (this) { 406 case PERSONAL: return "The person authenticated the content in their personal capacity."; 407 case PROFESSIONAL: return "The person authenticated the content in their professional capacity."; 408 case LEGAL: return "The person authenticated the content and accepted legal responsibility for its content."; 409 case OFFICIAL: return "The organization authenticated the content as consistent with their policies and procedures."; 410 case NULL: return null; 411 default: return "?"; 412 } 413 } 414 public String getDisplay() { 415 switch (this) { 416 case PERSONAL: return "Personal"; 417 case PROFESSIONAL: return "Professional"; 418 case LEGAL: return "Legal"; 419 case OFFICIAL: return "Official"; 420 case NULL: return null; 421 default: return "?"; 422 } 423 } 424 } 425 426 public static class CompositionAttestationModeEnumFactory implements EnumFactory<CompositionAttestationMode> { 427 public CompositionAttestationMode fromCode(String codeString) throws IllegalArgumentException { 428 if (codeString == null || "".equals(codeString)) 429 if (codeString == null || "".equals(codeString)) 430 return null; 431 if ("personal".equals(codeString)) 432 return CompositionAttestationMode.PERSONAL; 433 if ("professional".equals(codeString)) 434 return CompositionAttestationMode.PROFESSIONAL; 435 if ("legal".equals(codeString)) 436 return CompositionAttestationMode.LEGAL; 437 if ("official".equals(codeString)) 438 return CompositionAttestationMode.OFFICIAL; 439 throw new IllegalArgumentException("Unknown CompositionAttestationMode code '"+codeString+"'"); 440 } 441 public Enumeration<CompositionAttestationMode> fromType(PrimitiveType<?> code) throws FHIRException { 442 if (code == null) 443 return null; 444 if (code.isEmpty()) 445 return new Enumeration<CompositionAttestationMode>(this); 446 String codeString = code.asStringValue(); 447 if (codeString == null || "".equals(codeString)) 448 return null; 449 if ("personal".equals(codeString)) 450 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PERSONAL); 451 if ("professional".equals(codeString)) 452 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PROFESSIONAL); 453 if ("legal".equals(codeString)) 454 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.LEGAL); 455 if ("official".equals(codeString)) 456 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.OFFICIAL); 457 throw new FHIRException("Unknown CompositionAttestationMode code '"+codeString+"'"); 458 } 459 public String toCode(CompositionAttestationMode code) { 460 if (code == CompositionAttestationMode.NULL) 461 return null; 462 if (code == CompositionAttestationMode.PERSONAL) 463 return "personal"; 464 if (code == CompositionAttestationMode.PROFESSIONAL) 465 return "professional"; 466 if (code == CompositionAttestationMode.LEGAL) 467 return "legal"; 468 if (code == CompositionAttestationMode.OFFICIAL) 469 return "official"; 470 return "?"; 471 } 472 public String toSystem(CompositionAttestationMode code) { 473 return code.getSystem(); 474 } 475 } 476 477 public enum DocumentRelationshipType { 478 /** 479 * This document logically replaces or supersedes the target document. 480 */ 481 REPLACES, 482 /** 483 * This document was generated by transforming the target document (e.g. format or language conversion). 484 */ 485 TRANSFORMS, 486 /** 487 * This document is a signature of the target document. 488 */ 489 SIGNS, 490 /** 491 * This document adds additional information to the target document. 492 */ 493 APPENDS, 494 /** 495 * added to help the parsers with the generic types 496 */ 497 NULL; 498 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 499 if (codeString == null || "".equals(codeString)) 500 return null; 501 if ("replaces".equals(codeString)) 502 return REPLACES; 503 if ("transforms".equals(codeString)) 504 return TRANSFORMS; 505 if ("signs".equals(codeString)) 506 return SIGNS; 507 if ("appends".equals(codeString)) 508 return APPENDS; 509 if (Configuration.isAcceptInvalidEnums()) 510 return null; 511 else 512 throw new FHIRException("Unknown DocumentRelationshipType code '"+codeString+"'"); 513 } 514 public String toCode() { 515 switch (this) { 516 case REPLACES: return "replaces"; 517 case TRANSFORMS: return "transforms"; 518 case SIGNS: return "signs"; 519 case APPENDS: return "appends"; 520 case NULL: return null; 521 default: return "?"; 522 } 523 } 524 public String getSystem() { 525 switch (this) { 526 case REPLACES: return "http://hl7.org/fhir/document-relationship-type"; 527 case TRANSFORMS: return "http://hl7.org/fhir/document-relationship-type"; 528 case SIGNS: return "http://hl7.org/fhir/document-relationship-type"; 529 case APPENDS: return "http://hl7.org/fhir/document-relationship-type"; 530 case NULL: return null; 531 default: return "?"; 532 } 533 } 534 public String getDefinition() { 535 switch (this) { 536 case REPLACES: return "This document logically replaces or supersedes the target document."; 537 case TRANSFORMS: return "This document was generated by transforming the target document (e.g. format or language conversion)."; 538 case SIGNS: return "This document is a signature of the target document."; 539 case APPENDS: return "This document adds additional information to the target document."; 540 case NULL: return null; 541 default: return "?"; 542 } 543 } 544 public String getDisplay() { 545 switch (this) { 546 case REPLACES: return "Replaces"; 547 case TRANSFORMS: return "Transforms"; 548 case SIGNS: return "Signs"; 549 case APPENDS: return "Appends"; 550 case NULL: return null; 551 default: return "?"; 552 } 553 } 554 } 555 556 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 557 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 558 if (codeString == null || "".equals(codeString)) 559 if (codeString == null || "".equals(codeString)) 560 return null; 561 if ("replaces".equals(codeString)) 562 return DocumentRelationshipType.REPLACES; 563 if ("transforms".equals(codeString)) 564 return DocumentRelationshipType.TRANSFORMS; 565 if ("signs".equals(codeString)) 566 return DocumentRelationshipType.SIGNS; 567 if ("appends".equals(codeString)) 568 return DocumentRelationshipType.APPENDS; 569 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '"+codeString+"'"); 570 } 571 public Enumeration<DocumentRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 572 if (code == null) 573 return null; 574 if (code.isEmpty()) 575 return new Enumeration<DocumentRelationshipType>(this); 576 String codeString = code.asStringValue(); 577 if (codeString == null || "".equals(codeString)) 578 return null; 579 if ("replaces".equals(codeString)) 580 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES); 581 if ("transforms".equals(codeString)) 582 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS); 583 if ("signs".equals(codeString)) 584 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS); 585 if ("appends".equals(codeString)) 586 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS); 587 throw new FHIRException("Unknown DocumentRelationshipType code '"+codeString+"'"); 588 } 589 public String toCode(DocumentRelationshipType code) { 590 if (code == DocumentRelationshipType.NULL) 591 return null; 592 if (code == DocumentRelationshipType.REPLACES) 593 return "replaces"; 594 if (code == DocumentRelationshipType.TRANSFORMS) 595 return "transforms"; 596 if (code == DocumentRelationshipType.SIGNS) 597 return "signs"; 598 if (code == DocumentRelationshipType.APPENDS) 599 return "appends"; 600 return "?"; 601 } 602 public String toSystem(DocumentRelationshipType code) { 603 return code.getSystem(); 604 } 605 } 606 607 public enum SectionMode { 608 /** 609 * This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes 610 */ 611 WORKING, 612 /** 613 * This list was prepared as a snapshot. It should not be assumed to be current 614 */ 615 SNAPSHOT, 616 /** 617 * A list that indicates where changes have been made or recommended 618 */ 619 CHANGES, 620 /** 621 * added to help the parsers with the generic types 622 */ 623 NULL; 624 public static SectionMode fromCode(String codeString) throws FHIRException { 625 if (codeString == null || "".equals(codeString)) 626 return null; 627 if ("working".equals(codeString)) 628 return WORKING; 629 if ("snapshot".equals(codeString)) 630 return SNAPSHOT; 631 if ("changes".equals(codeString)) 632 return CHANGES; 633 if (Configuration.isAcceptInvalidEnums()) 634 return null; 635 else 636 throw new FHIRException("Unknown SectionMode code '"+codeString+"'"); 637 } 638 public String toCode() { 639 switch (this) { 640 case WORKING: return "working"; 641 case SNAPSHOT: return "snapshot"; 642 case CHANGES: return "changes"; 643 case NULL: return null; 644 default: return "?"; 645 } 646 } 647 public String getSystem() { 648 switch (this) { 649 case WORKING: return "http://hl7.org/fhir/list-mode"; 650 case SNAPSHOT: return "http://hl7.org/fhir/list-mode"; 651 case CHANGES: return "http://hl7.org/fhir/list-mode"; 652 case NULL: return null; 653 default: return "?"; 654 } 655 } 656 public String getDefinition() { 657 switch (this) { 658 case WORKING: return "This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes"; 659 case SNAPSHOT: return "This list was prepared as a snapshot. It should not be assumed to be current"; 660 case CHANGES: return "A list that indicates where changes have been made or recommended"; 661 case NULL: return null; 662 default: return "?"; 663 } 664 } 665 public String getDisplay() { 666 switch (this) { 667 case WORKING: return "Working List"; 668 case SNAPSHOT: return "Snapshot List"; 669 case CHANGES: return "Change List"; 670 case NULL: return null; 671 default: return "?"; 672 } 673 } 674 } 675 676 public static class SectionModeEnumFactory implements EnumFactory<SectionMode> { 677 public SectionMode fromCode(String codeString) throws IllegalArgumentException { 678 if (codeString == null || "".equals(codeString)) 679 if (codeString == null || "".equals(codeString)) 680 return null; 681 if ("working".equals(codeString)) 682 return SectionMode.WORKING; 683 if ("snapshot".equals(codeString)) 684 return SectionMode.SNAPSHOT; 685 if ("changes".equals(codeString)) 686 return SectionMode.CHANGES; 687 throw new IllegalArgumentException("Unknown SectionMode code '"+codeString+"'"); 688 } 689 public Enumeration<SectionMode> fromType(PrimitiveType<?> code) throws FHIRException { 690 if (code == null) 691 return null; 692 if (code.isEmpty()) 693 return new Enumeration<SectionMode>(this); 694 String codeString = code.asStringValue(); 695 if (codeString == null || "".equals(codeString)) 696 return null; 697 if ("working".equals(codeString)) 698 return new Enumeration<SectionMode>(this, SectionMode.WORKING); 699 if ("snapshot".equals(codeString)) 700 return new Enumeration<SectionMode>(this, SectionMode.SNAPSHOT); 701 if ("changes".equals(codeString)) 702 return new Enumeration<SectionMode>(this, SectionMode.CHANGES); 703 throw new FHIRException("Unknown SectionMode code '"+codeString+"'"); 704 } 705 public String toCode(SectionMode code) { 706 if (code == SectionMode.NULL) 707 return null; 708 if (code == SectionMode.WORKING) 709 return "working"; 710 if (code == SectionMode.SNAPSHOT) 711 return "snapshot"; 712 if (code == SectionMode.CHANGES) 713 return "changes"; 714 return "?"; 715 } 716 public String toSystem(SectionMode code) { 717 return code.getSystem(); 718 } 719 } 720 721 @Block() 722 public static class CompositionAttesterComponent extends BackboneElement implements IBaseBackboneElement { 723 /** 724 * The type of attestation the authenticator offers. 725 */ 726 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 727 @Description(shortDefinition="personal | professional | legal | official", formalDefinition="The type of attestation the authenticator offers." ) 728 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composition-attestation-mode") 729 protected List<Enumeration<CompositionAttestationMode>> mode; 730 731 /** 732 * When the composition was attested by the party. 733 */ 734 @Child(name = "time", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 735 @Description(shortDefinition="When the composition was attested", formalDefinition="When the composition was attested by the party." ) 736 protected DateTimeType time; 737 738 /** 739 * Who attested the composition in the specified way. 740 */ 741 @Child(name = "party", type = {Patient.class, Practitioner.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 742 @Description(shortDefinition="Who attested the composition", formalDefinition="Who attested the composition in the specified way." ) 743 protected Reference party; 744 745 /** 746 * The actual object that is the target of the reference (Who attested the composition in the specified way.) 747 */ 748 protected Resource partyTarget; 749 750 private static final long serialVersionUID = -436604745L; 751 752 /** 753 * Constructor 754 */ 755 public CompositionAttesterComponent() { 756 super(); 757 } 758 759 /** 760 * @return {@link #mode} (The type of attestation the authenticator offers.) 761 */ 762 public List<Enumeration<CompositionAttestationMode>> getMode() { 763 if (this.mode == null) 764 this.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 765 return this.mode; 766 } 767 768 /** 769 * @return Returns a reference to <code>this</code> for easy method chaining 770 */ 771 public CompositionAttesterComponent setMode(List<Enumeration<CompositionAttestationMode>> theMode) { 772 this.mode = theMode; 773 return this; 774 } 775 776 public boolean hasMode() { 777 if (this.mode == null) 778 return false; 779 for (Enumeration<CompositionAttestationMode> item : this.mode) 780 if (!item.isEmpty()) 781 return true; 782 return false; 783 } 784 785 /** 786 * @return {@link #mode} (The type of attestation the authenticator offers.) 787 */ 788 public Enumeration<CompositionAttestationMode> addModeElement() {//2 789 Enumeration<CompositionAttestationMode> t = new Enumeration<CompositionAttestationMode>(new CompositionAttestationModeEnumFactory()); 790 if (this.mode == null) 791 this.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 792 this.mode.add(t); 793 return t; 794 } 795 796 /** 797 * @param value {@link #mode} (The type of attestation the authenticator offers.) 798 */ 799 public CompositionAttesterComponent addMode(CompositionAttestationMode value) { //1 800 Enumeration<CompositionAttestationMode> t = new Enumeration<CompositionAttestationMode>(new CompositionAttestationModeEnumFactory()); 801 t.setValue(value); 802 if (this.mode == null) 803 this.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 804 this.mode.add(t); 805 return this; 806 } 807 808 /** 809 * @param value {@link #mode} (The type of attestation the authenticator offers.) 810 */ 811 public boolean hasMode(CompositionAttestationMode value) { 812 if (this.mode == null) 813 return false; 814 for (Enumeration<CompositionAttestationMode> v : this.mode) 815 if (v.getValue().equals(value)) // code 816 return true; 817 return false; 818 } 819 820 /** 821 * @return {@link #time} (When the composition was attested by the party.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 822 */ 823 public DateTimeType getTimeElement() { 824 if (this.time == null) 825 if (Configuration.errorOnAutoCreate()) 826 throw new Error("Attempt to auto-create CompositionAttesterComponent.time"); 827 else if (Configuration.doAutoCreate()) 828 this.time = new DateTimeType(); // bb 829 return this.time; 830 } 831 832 public boolean hasTimeElement() { 833 return this.time != null && !this.time.isEmpty(); 834 } 835 836 public boolean hasTime() { 837 return this.time != null && !this.time.isEmpty(); 838 } 839 840 /** 841 * @param value {@link #time} (When the composition was attested by the party.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 842 */ 843 public CompositionAttesterComponent setTimeElement(DateTimeType value) { 844 this.time = value; 845 return this; 846 } 847 848 /** 849 * @return When the composition was attested by the party. 850 */ 851 public Date getTime() { 852 return this.time == null ? null : this.time.getValue(); 853 } 854 855 /** 856 * @param value When the composition was attested by the party. 857 */ 858 public CompositionAttesterComponent setTime(Date value) { 859 if (value == null) 860 this.time = null; 861 else { 862 if (this.time == null) 863 this.time = new DateTimeType(); 864 this.time.setValue(value); 865 } 866 return this; 867 } 868 869 /** 870 * @return {@link #party} (Who attested the composition in the specified way.) 871 */ 872 public Reference getParty() { 873 if (this.party == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create CompositionAttesterComponent.party"); 876 else if (Configuration.doAutoCreate()) 877 this.party = new Reference(); // cc 878 return this.party; 879 } 880 881 public boolean hasParty() { 882 return this.party != null && !this.party.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #party} (Who attested the composition in the specified way.) 887 */ 888 public CompositionAttesterComponent setParty(Reference value) { 889 this.party = value; 890 return this; 891 } 892 893 /** 894 * @return {@link #party} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Who attested the composition in the specified way.) 895 */ 896 public Resource getPartyTarget() { 897 return this.partyTarget; 898 } 899 900 /** 901 * @param value {@link #party} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Who attested the composition in the specified way.) 902 */ 903 public CompositionAttesterComponent setPartyTarget(Resource value) { 904 this.partyTarget = value; 905 return this; 906 } 907 908 protected void listChildren(List<Property> children) { 909 super.listChildren(children); 910 children.add(new Property("mode", "code", "The type of attestation the authenticator offers.", 0, java.lang.Integer.MAX_VALUE, mode)); 911 children.add(new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, time)); 912 children.add(new Property("party", "Reference(Patient|Practitioner|Organization)", "Who attested the composition in the specified way.", 0, 1, party)); 913 } 914 915 @Override 916 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 917 switch (_hash) { 918 case 3357091: /*mode*/ return new Property("mode", "code", "The type of attestation the authenticator offers.", 0, java.lang.Integer.MAX_VALUE, mode); 919 case 3560141: /*time*/ return new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, time); 920 case 106437350: /*party*/ return new Property("party", "Reference(Patient|Practitioner|Organization)", "Who attested the composition in the specified way.", 0, 1, party); 921 default: return super.getNamedProperty(_hash, _name, _checkValid); 922 } 923 924 } 925 926 @Override 927 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 928 switch (hash) { 929 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : this.mode.toArray(new Base[this.mode.size()]); // Enumeration<CompositionAttestationMode> 930 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // DateTimeType 931 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 932 default: return super.getProperty(hash, name, checkValid); 933 } 934 935 } 936 937 @Override 938 public Base setProperty(int hash, String name, Base value) throws FHIRException { 939 switch (hash) { 940 case 3357091: // mode 941 value = new CompositionAttestationModeEnumFactory().fromType(castToCode(value)); 942 this.getMode().add((Enumeration) value); // Enumeration<CompositionAttestationMode> 943 return value; 944 case 3560141: // time 945 this.time = castToDateTime(value); // DateTimeType 946 return value; 947 case 106437350: // party 948 this.party = castToReference(value); // Reference 949 return value; 950 default: return super.setProperty(hash, name, value); 951 } 952 953 } 954 955 @Override 956 public Base setProperty(String name, Base value) throws FHIRException { 957 if (name.equals("mode")) { 958 value = new CompositionAttestationModeEnumFactory().fromType(castToCode(value)); 959 this.getMode().add((Enumeration) value); 960 } else if (name.equals("time")) { 961 this.time = castToDateTime(value); // DateTimeType 962 } else if (name.equals("party")) { 963 this.party = castToReference(value); // Reference 964 } else 965 return super.setProperty(name, value); 966 return value; 967 } 968 969 @Override 970 public Base makeProperty(int hash, String name) throws FHIRException { 971 switch (hash) { 972 case 3357091: return addModeElement(); 973 case 3560141: return getTimeElement(); 974 case 106437350: return getParty(); 975 default: return super.makeProperty(hash, name); 976 } 977 978 } 979 980 @Override 981 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 982 switch (hash) { 983 case 3357091: /*mode*/ return new String[] {"code"}; 984 case 3560141: /*time*/ return new String[] {"dateTime"}; 985 case 106437350: /*party*/ return new String[] {"Reference"}; 986 default: return super.getTypesForProperty(hash, name); 987 } 988 989 } 990 991 @Override 992 public Base addChild(String name) throws FHIRException { 993 if (name.equals("mode")) { 994 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 995 } 996 else if (name.equals("time")) { 997 throw new FHIRException("Cannot call addChild on a singleton property Composition.time"); 998 } 999 else if (name.equals("party")) { 1000 this.party = new Reference(); 1001 return this.party; 1002 } 1003 else 1004 return super.addChild(name); 1005 } 1006 1007 public CompositionAttesterComponent copy() { 1008 CompositionAttesterComponent dst = new CompositionAttesterComponent(); 1009 copyValues(dst); 1010 if (mode != null) { 1011 dst.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 1012 for (Enumeration<CompositionAttestationMode> i : mode) 1013 dst.mode.add(i.copy()); 1014 }; 1015 dst.time = time == null ? null : time.copy(); 1016 dst.party = party == null ? null : party.copy(); 1017 return dst; 1018 } 1019 1020 @Override 1021 public boolean equalsDeep(Base other_) { 1022 if (!super.equalsDeep(other_)) 1023 return false; 1024 if (!(other_ instanceof CompositionAttesterComponent)) 1025 return false; 1026 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 1027 return compareDeep(mode, o.mode, true) && compareDeep(time, o.time, true) && compareDeep(party, o.party, true) 1028 ; 1029 } 1030 1031 @Override 1032 public boolean equalsShallow(Base other_) { 1033 if (!super.equalsShallow(other_)) 1034 return false; 1035 if (!(other_ instanceof CompositionAttesterComponent)) 1036 return false; 1037 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 1038 return compareValues(mode, o.mode, true) && compareValues(time, o.time, true); 1039 } 1040 1041 public boolean isEmpty() { 1042 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, time, party); 1043 } 1044 1045 public String fhirType() { 1046 return "Composition.attester"; 1047 1048 } 1049 1050 } 1051 1052 @Block() 1053 public static class CompositionRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 1054 /** 1055 * The type of relationship that this composition has with anther composition or document. 1056 */ 1057 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1058 @Description(shortDefinition="replaces | transforms | signs | appends", formalDefinition="The type of relationship that this composition has with anther composition or document." ) 1059 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-relationship-type") 1060 protected Enumeration<DocumentRelationshipType> code; 1061 1062 /** 1063 * The target composition/document of this relationship. 1064 */ 1065 @Child(name = "target", type = {Identifier.class, Composition.class}, order=2, min=1, max=1, modifier=false, summary=true) 1066 @Description(shortDefinition="Target of the relationship", formalDefinition="The target composition/document of this relationship." ) 1067 protected Type target; 1068 1069 private static final long serialVersionUID = 1536930280L; 1070 1071 /** 1072 * Constructor 1073 */ 1074 public CompositionRelatesToComponent() { 1075 super(); 1076 } 1077 1078 /** 1079 * Constructor 1080 */ 1081 public CompositionRelatesToComponent(Enumeration<DocumentRelationshipType> code, Type target) { 1082 super(); 1083 this.code = code; 1084 this.target = target; 1085 } 1086 1087 /** 1088 * @return {@link #code} (The type of relationship that this composition has with anther composition or document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1089 */ 1090 public Enumeration<DocumentRelationshipType> getCodeElement() { 1091 if (this.code == null) 1092 if (Configuration.errorOnAutoCreate()) 1093 throw new Error("Attempt to auto-create CompositionRelatesToComponent.code"); 1094 else if (Configuration.doAutoCreate()) 1095 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 1096 return this.code; 1097 } 1098 1099 public boolean hasCodeElement() { 1100 return this.code != null && !this.code.isEmpty(); 1101 } 1102 1103 public boolean hasCode() { 1104 return this.code != null && !this.code.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #code} (The type of relationship that this composition has with anther composition or document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1109 */ 1110 public CompositionRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 1111 this.code = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return The type of relationship that this composition has with anther composition or document. 1117 */ 1118 public DocumentRelationshipType getCode() { 1119 return this.code == null ? null : this.code.getValue(); 1120 } 1121 1122 /** 1123 * @param value The type of relationship that this composition has with anther composition or document. 1124 */ 1125 public CompositionRelatesToComponent setCode(DocumentRelationshipType value) { 1126 if (this.code == null) 1127 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 1128 this.code.setValue(value); 1129 return this; 1130 } 1131 1132 /** 1133 * @return {@link #target} (The target composition/document of this relationship.) 1134 */ 1135 public Type getTarget() { 1136 return this.target; 1137 } 1138 1139 /** 1140 * @return {@link #target} (The target composition/document of this relationship.) 1141 */ 1142 public Identifier getTargetIdentifier() throws FHIRException { 1143 if (this.target == null) 1144 return null; 1145 if (!(this.target instanceof Identifier)) 1146 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.target.getClass().getName()+" was encountered"); 1147 return (Identifier) this.target; 1148 } 1149 1150 public boolean hasTargetIdentifier() { 1151 return this.target instanceof Identifier; 1152 } 1153 1154 /** 1155 * @return {@link #target} (The target composition/document of this relationship.) 1156 */ 1157 public Reference getTargetReference() throws FHIRException { 1158 if (this.target == null) 1159 return null; 1160 if (!(this.target instanceof Reference)) 1161 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.target.getClass().getName()+" was encountered"); 1162 return (Reference) this.target; 1163 } 1164 1165 public boolean hasTargetReference() { 1166 return this.target instanceof Reference; 1167 } 1168 1169 public boolean hasTarget() { 1170 return this.target != null && !this.target.isEmpty(); 1171 } 1172 1173 /** 1174 * @param value {@link #target} (The target composition/document of this relationship.) 1175 */ 1176 public CompositionRelatesToComponent setTarget(Type value) throws FHIRFormatError { 1177 if (value != null && !(value instanceof Identifier || value instanceof Reference)) 1178 throw new FHIRFormatError("Not the right type for Composition.relatesTo.target[x]: "+value.fhirType()); 1179 this.target = value; 1180 return this; 1181 } 1182 1183 protected void listChildren(List<Property> children) { 1184 super.listChildren(children); 1185 children.add(new Property("code", "code", "The type of relationship that this composition has with anther composition or document.", 0, 1, code)); 1186 children.add(new Property("target[x]", "Identifier|Reference(Composition)", "The target composition/document of this relationship.", 0, 1, target)); 1187 } 1188 1189 @Override 1190 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1191 switch (_hash) { 1192 case 3059181: /*code*/ return new Property("code", "code", "The type of relationship that this composition has with anther composition or document.", 0, 1, code); 1193 case -815579825: /*target[x]*/ return new Property("target[x]", "Identifier|Reference(Composition)", "The target composition/document of this relationship.", 0, 1, target); 1194 case -880905839: /*target*/ return new Property("target[x]", "Identifier|Reference(Composition)", "The target composition/document of this relationship.", 0, 1, target); 1195 case 1690892570: /*targetIdentifier*/ return new Property("target[x]", "Identifier|Reference(Composition)", "The target composition/document of this relationship.", 0, 1, target); 1196 case 1259806906: /*targetReference*/ return new Property("target[x]", "Identifier|Reference(Composition)", "The target composition/document of this relationship.", 0, 1, target); 1197 default: return super.getNamedProperty(_hash, _name, _checkValid); 1198 } 1199 1200 } 1201 1202 @Override 1203 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1204 switch (hash) { 1205 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<DocumentRelationshipType> 1206 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Type 1207 default: return super.getProperty(hash, name, checkValid); 1208 } 1209 1210 } 1211 1212 @Override 1213 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1214 switch (hash) { 1215 case 3059181: // code 1216 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 1217 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 1218 return value; 1219 case -880905839: // target 1220 this.target = castToType(value); // Type 1221 return value; 1222 default: return super.setProperty(hash, name, value); 1223 } 1224 1225 } 1226 1227 @Override 1228 public Base setProperty(String name, Base value) throws FHIRException { 1229 if (name.equals("code")) { 1230 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 1231 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 1232 } else if (name.equals("target[x]")) { 1233 this.target = castToType(value); // Type 1234 } else 1235 return super.setProperty(name, value); 1236 return value; 1237 } 1238 1239 @Override 1240 public Base makeProperty(int hash, String name) throws FHIRException { 1241 switch (hash) { 1242 case 3059181: return getCodeElement(); 1243 case -815579825: return getTarget(); 1244 case -880905839: return getTarget(); 1245 default: return super.makeProperty(hash, name); 1246 } 1247 1248 } 1249 1250 @Override 1251 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1252 switch (hash) { 1253 case 3059181: /*code*/ return new String[] {"code"}; 1254 case -880905839: /*target*/ return new String[] {"Identifier", "Reference"}; 1255 default: return super.getTypesForProperty(hash, name); 1256 } 1257 1258 } 1259 1260 @Override 1261 public Base addChild(String name) throws FHIRException { 1262 if (name.equals("code")) { 1263 throw new FHIRException("Cannot call addChild on a singleton property Composition.code"); 1264 } 1265 else if (name.equals("targetIdentifier")) { 1266 this.target = new Identifier(); 1267 return this.target; 1268 } 1269 else if (name.equals("targetReference")) { 1270 this.target = new Reference(); 1271 return this.target; 1272 } 1273 else 1274 return super.addChild(name); 1275 } 1276 1277 public CompositionRelatesToComponent copy() { 1278 CompositionRelatesToComponent dst = new CompositionRelatesToComponent(); 1279 copyValues(dst); 1280 dst.code = code == null ? null : code.copy(); 1281 dst.target = target == null ? null : target.copy(); 1282 return dst; 1283 } 1284 1285 @Override 1286 public boolean equalsDeep(Base other_) { 1287 if (!super.equalsDeep(other_)) 1288 return false; 1289 if (!(other_ instanceof CompositionRelatesToComponent)) 1290 return false; 1291 CompositionRelatesToComponent o = (CompositionRelatesToComponent) other_; 1292 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 1293 } 1294 1295 @Override 1296 public boolean equalsShallow(Base other_) { 1297 if (!super.equalsShallow(other_)) 1298 return false; 1299 if (!(other_ instanceof CompositionRelatesToComponent)) 1300 return false; 1301 CompositionRelatesToComponent o = (CompositionRelatesToComponent) other_; 1302 return compareValues(code, o.code, true); 1303 } 1304 1305 public boolean isEmpty() { 1306 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 1307 } 1308 1309 public String fhirType() { 1310 return "Composition.relatesTo"; 1311 1312 } 1313 1314 } 1315 1316 @Block() 1317 public static class CompositionEventComponent extends BackboneElement implements IBaseBackboneElement { 1318 /** 1319 * This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a "History and Physical Report" in which the procedure being documented is necessarily a "History and Physical" act. 1320 */ 1321 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1322 @Description(shortDefinition="Code(s) that apply to the event being documented", formalDefinition="This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act." ) 1323 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActCode") 1324 protected List<CodeableConcept> code; 1325 1326 /** 1327 * The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time. 1328 */ 1329 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 1330 @Description(shortDefinition="The period covered by the documentation", formalDefinition="The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time." ) 1331 protected Period period; 1332 1333 /** 1334 * The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy. 1335 */ 1336 @Child(name = "detail", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1337 @Description(shortDefinition="The event(s) being documented", formalDefinition="The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy." ) 1338 protected List<Reference> detail; 1339 /** 1340 * The actual objects that are the target of the reference (The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.) 1341 */ 1342 protected List<Resource> detailTarget; 1343 1344 1345 private static final long serialVersionUID = -1581379774L; 1346 1347 /** 1348 * Constructor 1349 */ 1350 public CompositionEventComponent() { 1351 super(); 1352 } 1353 1354 /** 1355 * @return {@link #code} (This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a "History and Physical Report" in which the procedure being documented is necessarily a "History and Physical" act.) 1356 */ 1357 public List<CodeableConcept> getCode() { 1358 if (this.code == null) 1359 this.code = new ArrayList<CodeableConcept>(); 1360 return this.code; 1361 } 1362 1363 /** 1364 * @return Returns a reference to <code>this</code> for easy method chaining 1365 */ 1366 public CompositionEventComponent setCode(List<CodeableConcept> theCode) { 1367 this.code = theCode; 1368 return this; 1369 } 1370 1371 public boolean hasCode() { 1372 if (this.code == null) 1373 return false; 1374 for (CodeableConcept item : this.code) 1375 if (!item.isEmpty()) 1376 return true; 1377 return false; 1378 } 1379 1380 public CodeableConcept addCode() { //3 1381 CodeableConcept t = new CodeableConcept(); 1382 if (this.code == null) 1383 this.code = new ArrayList<CodeableConcept>(); 1384 this.code.add(t); 1385 return t; 1386 } 1387 1388 public CompositionEventComponent addCode(CodeableConcept t) { //3 1389 if (t == null) 1390 return this; 1391 if (this.code == null) 1392 this.code = new ArrayList<CodeableConcept>(); 1393 this.code.add(t); 1394 return this; 1395 } 1396 1397 /** 1398 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 1399 */ 1400 public CodeableConcept getCodeFirstRep() { 1401 if (getCode().isEmpty()) { 1402 addCode(); 1403 } 1404 return getCode().get(0); 1405 } 1406 1407 /** 1408 * @return {@link #period} (The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.) 1409 */ 1410 public Period getPeriod() { 1411 if (this.period == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create CompositionEventComponent.period"); 1414 else if (Configuration.doAutoCreate()) 1415 this.period = new Period(); // cc 1416 return this.period; 1417 } 1418 1419 public boolean hasPeriod() { 1420 return this.period != null && !this.period.isEmpty(); 1421 } 1422 1423 /** 1424 * @param value {@link #period} (The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.) 1425 */ 1426 public CompositionEventComponent setPeriod(Period value) { 1427 this.period = value; 1428 return this; 1429 } 1430 1431 /** 1432 * @return {@link #detail} (The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.) 1433 */ 1434 public List<Reference> getDetail() { 1435 if (this.detail == null) 1436 this.detail = new ArrayList<Reference>(); 1437 return this.detail; 1438 } 1439 1440 /** 1441 * @return Returns a reference to <code>this</code> for easy method chaining 1442 */ 1443 public CompositionEventComponent setDetail(List<Reference> theDetail) { 1444 this.detail = theDetail; 1445 return this; 1446 } 1447 1448 public boolean hasDetail() { 1449 if (this.detail == null) 1450 return false; 1451 for (Reference item : this.detail) 1452 if (!item.isEmpty()) 1453 return true; 1454 return false; 1455 } 1456 1457 public Reference addDetail() { //3 1458 Reference t = new Reference(); 1459 if (this.detail == null) 1460 this.detail = new ArrayList<Reference>(); 1461 this.detail.add(t); 1462 return t; 1463 } 1464 1465 public CompositionEventComponent addDetail(Reference t) { //3 1466 if (t == null) 1467 return this; 1468 if (this.detail == null) 1469 this.detail = new ArrayList<Reference>(); 1470 this.detail.add(t); 1471 return this; 1472 } 1473 1474 /** 1475 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 1476 */ 1477 public Reference getDetailFirstRep() { 1478 if (getDetail().isEmpty()) { 1479 addDetail(); 1480 } 1481 return getDetail().get(0); 1482 } 1483 1484 /** 1485 * @deprecated Use Reference#setResource(IBaseResource) instead 1486 */ 1487 @Deprecated 1488 public List<Resource> getDetailTarget() { 1489 if (this.detailTarget == null) 1490 this.detailTarget = new ArrayList<Resource>(); 1491 return this.detailTarget; 1492 } 1493 1494 protected void listChildren(List<Property> children) { 1495 super.listChildren(children); 1496 children.add(new Property("code", "CodeableConcept", "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 0, java.lang.Integer.MAX_VALUE, code)); 1497 children.add(new Property("period", "Period", "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 0, 1, period)); 1498 children.add(new Property("detail", "Reference(Any)", "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.", 0, java.lang.Integer.MAX_VALUE, detail)); 1499 } 1500 1501 @Override 1502 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1503 switch (_hash) { 1504 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 0, java.lang.Integer.MAX_VALUE, code); 1505 case -991726143: /*period*/ return new Property("period", "Period", "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 0, 1, period); 1506 case -1335224239: /*detail*/ return new Property("detail", "Reference(Any)", "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.", 0, java.lang.Integer.MAX_VALUE, detail); 1507 default: return super.getNamedProperty(_hash, _name, _checkValid); 1508 } 1509 1510 } 1511 1512 @Override 1513 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1514 switch (hash) { 1515 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1516 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1517 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 1518 default: return super.getProperty(hash, name, checkValid); 1519 } 1520 1521 } 1522 1523 @Override 1524 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1525 switch (hash) { 1526 case 3059181: // code 1527 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 1528 return value; 1529 case -991726143: // period 1530 this.period = castToPeriod(value); // Period 1531 return value; 1532 case -1335224239: // detail 1533 this.getDetail().add(castToReference(value)); // Reference 1534 return value; 1535 default: return super.setProperty(hash, name, value); 1536 } 1537 1538 } 1539 1540 @Override 1541 public Base setProperty(String name, Base value) throws FHIRException { 1542 if (name.equals("code")) { 1543 this.getCode().add(castToCodeableConcept(value)); 1544 } else if (name.equals("period")) { 1545 this.period = castToPeriod(value); // Period 1546 } else if (name.equals("detail")) { 1547 this.getDetail().add(castToReference(value)); 1548 } else 1549 return super.setProperty(name, value); 1550 return value; 1551 } 1552 1553 @Override 1554 public Base makeProperty(int hash, String name) throws FHIRException { 1555 switch (hash) { 1556 case 3059181: return addCode(); 1557 case -991726143: return getPeriod(); 1558 case -1335224239: return addDetail(); 1559 default: return super.makeProperty(hash, name); 1560 } 1561 1562 } 1563 1564 @Override 1565 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1566 switch (hash) { 1567 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1568 case -991726143: /*period*/ return new String[] {"Period"}; 1569 case -1335224239: /*detail*/ return new String[] {"Reference"}; 1570 default: return super.getTypesForProperty(hash, name); 1571 } 1572 1573 } 1574 1575 @Override 1576 public Base addChild(String name) throws FHIRException { 1577 if (name.equals("code")) { 1578 return addCode(); 1579 } 1580 else if (name.equals("period")) { 1581 this.period = new Period(); 1582 return this.period; 1583 } 1584 else if (name.equals("detail")) { 1585 return addDetail(); 1586 } 1587 else 1588 return super.addChild(name); 1589 } 1590 1591 public CompositionEventComponent copy() { 1592 CompositionEventComponent dst = new CompositionEventComponent(); 1593 copyValues(dst); 1594 if (code != null) { 1595 dst.code = new ArrayList<CodeableConcept>(); 1596 for (CodeableConcept i : code) 1597 dst.code.add(i.copy()); 1598 }; 1599 dst.period = period == null ? null : period.copy(); 1600 if (detail != null) { 1601 dst.detail = new ArrayList<Reference>(); 1602 for (Reference i : detail) 1603 dst.detail.add(i.copy()); 1604 }; 1605 return dst; 1606 } 1607 1608 @Override 1609 public boolean equalsDeep(Base other_) { 1610 if (!super.equalsDeep(other_)) 1611 return false; 1612 if (!(other_ instanceof CompositionEventComponent)) 1613 return false; 1614 CompositionEventComponent o = (CompositionEventComponent) other_; 1615 return compareDeep(code, o.code, true) && compareDeep(period, o.period, true) && compareDeep(detail, o.detail, true) 1616 ; 1617 } 1618 1619 @Override 1620 public boolean equalsShallow(Base other_) { 1621 if (!super.equalsShallow(other_)) 1622 return false; 1623 if (!(other_ instanceof CompositionEventComponent)) 1624 return false; 1625 CompositionEventComponent o = (CompositionEventComponent) other_; 1626 return true; 1627 } 1628 1629 public boolean isEmpty() { 1630 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, period, detail); 1631 } 1632 1633 public String fhirType() { 1634 return "Composition.event"; 1635 1636 } 1637 1638 } 1639 1640 @Block() 1641 public static class SectionComponent extends BackboneElement implements IBaseBackboneElement { 1642 /** 1643 * The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1644 */ 1645 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1646 @Description(shortDefinition="Label for section (e.g. for ToC)", formalDefinition="The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents." ) 1647 protected StringType title; 1648 1649 /** 1650 * A code identifying the kind of content contained within the section. This must be consistent with the section title. 1651 */ 1652 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1653 @Description(shortDefinition="Classification of section (recommended)", formalDefinition="A code identifying the kind of content contained within the section. This must be consistent with the section title." ) 1654 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/doc-section-codes") 1655 protected CodeableConcept code; 1656 1657 /** 1658 * A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. 1659 */ 1660 @Child(name = "text", type = {Narrative.class}, order=3, min=0, max=1, modifier=false, summary=false) 1661 @Description(shortDefinition="Text summary of the section, for human interpretation", formalDefinition="A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative." ) 1662 protected Narrative text; 1663 1664 /** 1665 * How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1666 */ 1667 @Child(name = "mode", type = {CodeType.class}, order=4, min=0, max=1, modifier=true, summary=true) 1668 @Description(shortDefinition="working | snapshot | changes", formalDefinition="How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted." ) 1669 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-mode") 1670 protected Enumeration<SectionMode> mode; 1671 1672 /** 1673 * Specifies the order applied to the items in the section entries. 1674 */ 1675 @Child(name = "orderedBy", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1676 @Description(shortDefinition="Order of section entries", formalDefinition="Specifies the order applied to the items in the section entries." ) 1677 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-order") 1678 protected CodeableConcept orderedBy; 1679 1680 /** 1681 * A reference to the actual resource from which the narrative in the section is derived. 1682 */ 1683 @Child(name = "entry", type = {Reference.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1684 @Description(shortDefinition="A reference to data that supports this section", formalDefinition="A reference to the actual resource from which the narrative in the section is derived." ) 1685 protected List<Reference> entry; 1686 /** 1687 * The actual objects that are the target of the reference (A reference to the actual resource from which the narrative in the section is derived.) 1688 */ 1689 protected List<Resource> entryTarget; 1690 1691 1692 /** 1693 * If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason. 1694 */ 1695 @Child(name = "emptyReason", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1696 @Description(shortDefinition="Why the section is empty", formalDefinition="If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason." ) 1697 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-empty-reason") 1698 protected CodeableConcept emptyReason; 1699 1700 /** 1701 * A nested sub-section within this section. 1702 */ 1703 @Child(name = "section", type = {SectionComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1704 @Description(shortDefinition="Nested Section", formalDefinition="A nested sub-section within this section." ) 1705 protected List<SectionComponent> section; 1706 1707 private static final long serialVersionUID = -128426142L; 1708 1709 /** 1710 * Constructor 1711 */ 1712 public SectionComponent() { 1713 super(); 1714 } 1715 1716 /** 1717 * @return {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1718 */ 1719 public StringType getTitleElement() { 1720 if (this.title == null) 1721 if (Configuration.errorOnAutoCreate()) 1722 throw new Error("Attempt to auto-create SectionComponent.title"); 1723 else if (Configuration.doAutoCreate()) 1724 this.title = new StringType(); // bb 1725 return this.title; 1726 } 1727 1728 public boolean hasTitleElement() { 1729 return this.title != null && !this.title.isEmpty(); 1730 } 1731 1732 public boolean hasTitle() { 1733 return this.title != null && !this.title.isEmpty(); 1734 } 1735 1736 /** 1737 * @param value {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1738 */ 1739 public SectionComponent setTitleElement(StringType value) { 1740 this.title = value; 1741 return this; 1742 } 1743 1744 /** 1745 * @return The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1746 */ 1747 public String getTitle() { 1748 return this.title == null ? null : this.title.getValue(); 1749 } 1750 1751 /** 1752 * @param value The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1753 */ 1754 public SectionComponent setTitle(String value) { 1755 if (Utilities.noString(value)) 1756 this.title = null; 1757 else { 1758 if (this.title == null) 1759 this.title = new StringType(); 1760 this.title.setValue(value); 1761 } 1762 return this; 1763 } 1764 1765 /** 1766 * @return {@link #code} (A code identifying the kind of content contained within the section. This must be consistent with the section title.) 1767 */ 1768 public CodeableConcept getCode() { 1769 if (this.code == null) 1770 if (Configuration.errorOnAutoCreate()) 1771 throw new Error("Attempt to auto-create SectionComponent.code"); 1772 else if (Configuration.doAutoCreate()) 1773 this.code = new CodeableConcept(); // cc 1774 return this.code; 1775 } 1776 1777 public boolean hasCode() { 1778 return this.code != null && !this.code.isEmpty(); 1779 } 1780 1781 /** 1782 * @param value {@link #code} (A code identifying the kind of content contained within the section. This must be consistent with the section title.) 1783 */ 1784 public SectionComponent setCode(CodeableConcept value) { 1785 this.code = value; 1786 return this; 1787 } 1788 1789 /** 1790 * @return {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative.) 1791 */ 1792 public Narrative getText() { 1793 if (this.text == null) 1794 if (Configuration.errorOnAutoCreate()) 1795 throw new Error("Attempt to auto-create SectionComponent.text"); 1796 else if (Configuration.doAutoCreate()) 1797 this.text = new Narrative(); // cc 1798 return this.text; 1799 } 1800 1801 public boolean hasText() { 1802 return this.text != null && !this.text.isEmpty(); 1803 } 1804 1805 /** 1806 * @param value {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative.) 1807 */ 1808 public SectionComponent setText(Narrative value) { 1809 this.text = value; 1810 return this; 1811 } 1812 1813 /** 1814 * @return {@link #mode} (How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1815 */ 1816 public Enumeration<SectionMode> getModeElement() { 1817 if (this.mode == null) 1818 if (Configuration.errorOnAutoCreate()) 1819 throw new Error("Attempt to auto-create SectionComponent.mode"); 1820 else if (Configuration.doAutoCreate()) 1821 this.mode = new Enumeration<SectionMode>(new SectionModeEnumFactory()); // bb 1822 return this.mode; 1823 } 1824 1825 public boolean hasModeElement() { 1826 return this.mode != null && !this.mode.isEmpty(); 1827 } 1828 1829 public boolean hasMode() { 1830 return this.mode != null && !this.mode.isEmpty(); 1831 } 1832 1833 /** 1834 * @param value {@link #mode} (How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1835 */ 1836 public SectionComponent setModeElement(Enumeration<SectionMode> value) { 1837 this.mode = value; 1838 return this; 1839 } 1840 1841 /** 1842 * @return How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1843 */ 1844 public SectionMode getMode() { 1845 return this.mode == null ? null : this.mode.getValue(); 1846 } 1847 1848 /** 1849 * @param value How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1850 */ 1851 public SectionComponent setMode(SectionMode value) { 1852 if (value == null) 1853 this.mode = null; 1854 else { 1855 if (this.mode == null) 1856 this.mode = new Enumeration<SectionMode>(new SectionModeEnumFactory()); 1857 this.mode.setValue(value); 1858 } 1859 return this; 1860 } 1861 1862 /** 1863 * @return {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 1864 */ 1865 public CodeableConcept getOrderedBy() { 1866 if (this.orderedBy == null) 1867 if (Configuration.errorOnAutoCreate()) 1868 throw new Error("Attempt to auto-create SectionComponent.orderedBy"); 1869 else if (Configuration.doAutoCreate()) 1870 this.orderedBy = new CodeableConcept(); // cc 1871 return this.orderedBy; 1872 } 1873 1874 public boolean hasOrderedBy() { 1875 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1876 } 1877 1878 /** 1879 * @param value {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 1880 */ 1881 public SectionComponent setOrderedBy(CodeableConcept value) { 1882 this.orderedBy = value; 1883 return this; 1884 } 1885 1886 /** 1887 * @return {@link #entry} (A reference to the actual resource from which the narrative in the section is derived.) 1888 */ 1889 public List<Reference> getEntry() { 1890 if (this.entry == null) 1891 this.entry = new ArrayList<Reference>(); 1892 return this.entry; 1893 } 1894 1895 /** 1896 * @return Returns a reference to <code>this</code> for easy method chaining 1897 */ 1898 public SectionComponent setEntry(List<Reference> theEntry) { 1899 this.entry = theEntry; 1900 return this; 1901 } 1902 1903 public boolean hasEntry() { 1904 if (this.entry == null) 1905 return false; 1906 for (Reference item : this.entry) 1907 if (!item.isEmpty()) 1908 return true; 1909 return false; 1910 } 1911 1912 public Reference addEntry() { //3 1913 Reference t = new Reference(); 1914 if (this.entry == null) 1915 this.entry = new ArrayList<Reference>(); 1916 this.entry.add(t); 1917 return t; 1918 } 1919 1920 public SectionComponent addEntry(Reference t) { //3 1921 if (t == null) 1922 return this; 1923 if (this.entry == null) 1924 this.entry = new ArrayList<Reference>(); 1925 this.entry.add(t); 1926 return this; 1927 } 1928 1929 /** 1930 * @return The first repetition of repeating field {@link #entry}, creating it if it does not already exist 1931 */ 1932 public Reference getEntryFirstRep() { 1933 if (getEntry().isEmpty()) { 1934 addEntry(); 1935 } 1936 return getEntry().get(0); 1937 } 1938 1939 /** 1940 * @deprecated Use Reference#setResource(IBaseResource) instead 1941 */ 1942 @Deprecated 1943 public List<Resource> getEntryTarget() { 1944 if (this.entryTarget == null) 1945 this.entryTarget = new ArrayList<Resource>(); 1946 return this.entryTarget; 1947 } 1948 1949 /** 1950 * @return {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 1951 */ 1952 public CodeableConcept getEmptyReason() { 1953 if (this.emptyReason == null) 1954 if (Configuration.errorOnAutoCreate()) 1955 throw new Error("Attempt to auto-create SectionComponent.emptyReason"); 1956 else if (Configuration.doAutoCreate()) 1957 this.emptyReason = new CodeableConcept(); // cc 1958 return this.emptyReason; 1959 } 1960 1961 public boolean hasEmptyReason() { 1962 return this.emptyReason != null && !this.emptyReason.isEmpty(); 1963 } 1964 1965 /** 1966 * @param value {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 1967 */ 1968 public SectionComponent setEmptyReason(CodeableConcept value) { 1969 this.emptyReason = value; 1970 return this; 1971 } 1972 1973 /** 1974 * @return {@link #section} (A nested sub-section within this section.) 1975 */ 1976 public List<SectionComponent> getSection() { 1977 if (this.section == null) 1978 this.section = new ArrayList<SectionComponent>(); 1979 return this.section; 1980 } 1981 1982 /** 1983 * @return Returns a reference to <code>this</code> for easy method chaining 1984 */ 1985 public SectionComponent setSection(List<SectionComponent> theSection) { 1986 this.section = theSection; 1987 return this; 1988 } 1989 1990 public boolean hasSection() { 1991 if (this.section == null) 1992 return false; 1993 for (SectionComponent item : this.section) 1994 if (!item.isEmpty()) 1995 return true; 1996 return false; 1997 } 1998 1999 public SectionComponent addSection() { //3 2000 SectionComponent t = new SectionComponent(); 2001 if (this.section == null) 2002 this.section = new ArrayList<SectionComponent>(); 2003 this.section.add(t); 2004 return t; 2005 } 2006 2007 public SectionComponent addSection(SectionComponent t) { //3 2008 if (t == null) 2009 return this; 2010 if (this.section == null) 2011 this.section = new ArrayList<SectionComponent>(); 2012 this.section.add(t); 2013 return this; 2014 } 2015 2016 /** 2017 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist 2018 */ 2019 public SectionComponent getSectionFirstRep() { 2020 if (getSection().isEmpty()) { 2021 addSection(); 2022 } 2023 return getSection().get(0); 2024 } 2025 2026 protected void listChildren(List<Property> children) { 2027 super.listChildren(children); 2028 children.add(new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title)); 2029 children.add(new Property("code", "CodeableConcept", "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 0, 1, code)); 2030 children.add(new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 0, 1, text)); 2031 children.add(new Property("mode", "code", "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode)); 2032 children.add(new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy)); 2033 children.add(new Property("entry", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entry)); 2034 children.add(new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason)); 2035 children.add(new Property("section", "@Composition.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section)); 2036 } 2037 2038 @Override 2039 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2040 switch (_hash) { 2041 case 110371416: /*title*/ return new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title); 2042 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 0, 1, code); 2043 case 3556653: /*text*/ return new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 0, 1, text); 2044 case 3357091: /*mode*/ return new Property("mode", "code", "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode); 2045 case -391079516: /*orderedBy*/ return new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy); 2046 case 96667762: /*entry*/ return new Property("entry", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entry); 2047 case 1140135409: /*emptyReason*/ return new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason); 2048 case 1970241253: /*section*/ return new Property("section", "@Composition.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section); 2049 default: return super.getNamedProperty(_hash, _name, _checkValid); 2050 } 2051 2052 } 2053 2054 @Override 2055 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2056 switch (hash) { 2057 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2058 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2059 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // Narrative 2060 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<SectionMode> 2061 case -391079516: /*orderedBy*/ return this.orderedBy == null ? new Base[0] : new Base[] {this.orderedBy}; // CodeableConcept 2062 case 96667762: /*entry*/ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // Reference 2063 case 1140135409: /*emptyReason*/ return this.emptyReason == null ? new Base[0] : new Base[] {this.emptyReason}; // CodeableConcept 2064 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 2065 default: return super.getProperty(hash, name, checkValid); 2066 } 2067 2068 } 2069 2070 @Override 2071 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2072 switch (hash) { 2073 case 110371416: // title 2074 this.title = castToString(value); // StringType 2075 return value; 2076 case 3059181: // code 2077 this.code = castToCodeableConcept(value); // CodeableConcept 2078 return value; 2079 case 3556653: // text 2080 this.text = castToNarrative(value); // Narrative 2081 return value; 2082 case 3357091: // mode 2083 value = new SectionModeEnumFactory().fromType(castToCode(value)); 2084 this.mode = (Enumeration) value; // Enumeration<SectionMode> 2085 return value; 2086 case -391079516: // orderedBy 2087 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 2088 return value; 2089 case 96667762: // entry 2090 this.getEntry().add(castToReference(value)); // Reference 2091 return value; 2092 case 1140135409: // emptyReason 2093 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 2094 return value; 2095 case 1970241253: // section 2096 this.getSection().add((SectionComponent) value); // SectionComponent 2097 return value; 2098 default: return super.setProperty(hash, name, value); 2099 } 2100 2101 } 2102 2103 @Override 2104 public Base setProperty(String name, Base value) throws FHIRException { 2105 if (name.equals("title")) { 2106 this.title = castToString(value); // StringType 2107 } else if (name.equals("code")) { 2108 this.code = castToCodeableConcept(value); // CodeableConcept 2109 } else if (name.equals("text")) { 2110 this.text = castToNarrative(value); // Narrative 2111 } else if (name.equals("mode")) { 2112 value = new SectionModeEnumFactory().fromType(castToCode(value)); 2113 this.mode = (Enumeration) value; // Enumeration<SectionMode> 2114 } else if (name.equals("orderedBy")) { 2115 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 2116 } else if (name.equals("entry")) { 2117 this.getEntry().add(castToReference(value)); 2118 } else if (name.equals("emptyReason")) { 2119 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 2120 } else if (name.equals("section")) { 2121 this.getSection().add((SectionComponent) value); 2122 } else 2123 return super.setProperty(name, value); 2124 return value; 2125 } 2126 2127 @Override 2128 public Base makeProperty(int hash, String name) throws FHIRException { 2129 switch (hash) { 2130 case 110371416: return getTitleElement(); 2131 case 3059181: return getCode(); 2132 case 3556653: return getText(); 2133 case 3357091: return getModeElement(); 2134 case -391079516: return getOrderedBy(); 2135 case 96667762: return addEntry(); 2136 case 1140135409: return getEmptyReason(); 2137 case 1970241253: return addSection(); 2138 default: return super.makeProperty(hash, name); 2139 } 2140 2141 } 2142 2143 @Override 2144 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2145 switch (hash) { 2146 case 110371416: /*title*/ return new String[] {"string"}; 2147 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2148 case 3556653: /*text*/ return new String[] {"Narrative"}; 2149 case 3357091: /*mode*/ return new String[] {"code"}; 2150 case -391079516: /*orderedBy*/ return new String[] {"CodeableConcept"}; 2151 case 96667762: /*entry*/ return new String[] {"Reference"}; 2152 case 1140135409: /*emptyReason*/ return new String[] {"CodeableConcept"}; 2153 case 1970241253: /*section*/ return new String[] {"@Composition.section"}; 2154 default: return super.getTypesForProperty(hash, name); 2155 } 2156 2157 } 2158 2159 @Override 2160 public Base addChild(String name) throws FHIRException { 2161 if (name.equals("title")) { 2162 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 2163 } 2164 else if (name.equals("code")) { 2165 this.code = new CodeableConcept(); 2166 return this.code; 2167 } 2168 else if (name.equals("text")) { 2169 this.text = new Narrative(); 2170 return this.text; 2171 } 2172 else if (name.equals("mode")) { 2173 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 2174 } 2175 else if (name.equals("orderedBy")) { 2176 this.orderedBy = new CodeableConcept(); 2177 return this.orderedBy; 2178 } 2179 else if (name.equals("entry")) { 2180 return addEntry(); 2181 } 2182 else if (name.equals("emptyReason")) { 2183 this.emptyReason = new CodeableConcept(); 2184 return this.emptyReason; 2185 } 2186 else if (name.equals("section")) { 2187 return addSection(); 2188 } 2189 else 2190 return super.addChild(name); 2191 } 2192 2193 public SectionComponent copy() { 2194 SectionComponent dst = new SectionComponent(); 2195 copyValues(dst); 2196 dst.title = title == null ? null : title.copy(); 2197 dst.code = code == null ? null : code.copy(); 2198 dst.text = text == null ? null : text.copy(); 2199 dst.mode = mode == null ? null : mode.copy(); 2200 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 2201 if (entry != null) { 2202 dst.entry = new ArrayList<Reference>(); 2203 for (Reference i : entry) 2204 dst.entry.add(i.copy()); 2205 }; 2206 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 2207 if (section != null) { 2208 dst.section = new ArrayList<SectionComponent>(); 2209 for (SectionComponent i : section) 2210 dst.section.add(i.copy()); 2211 }; 2212 return dst; 2213 } 2214 2215 @Override 2216 public boolean equalsDeep(Base other_) { 2217 if (!super.equalsDeep(other_)) 2218 return false; 2219 if (!(other_ instanceof SectionComponent)) 2220 return false; 2221 SectionComponent o = (SectionComponent) other_; 2222 return compareDeep(title, o.title, true) && compareDeep(code, o.code, true) && compareDeep(text, o.text, true) 2223 && compareDeep(mode, o.mode, true) && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(entry, o.entry, true) 2224 && compareDeep(emptyReason, o.emptyReason, true) && compareDeep(section, o.section, true); 2225 } 2226 2227 @Override 2228 public boolean equalsShallow(Base other_) { 2229 if (!super.equalsShallow(other_)) 2230 return false; 2231 if (!(other_ instanceof SectionComponent)) 2232 return false; 2233 SectionComponent o = (SectionComponent) other_; 2234 return compareValues(title, o.title, true) && compareValues(mode, o.mode, true); 2235 } 2236 2237 public boolean isEmpty() { 2238 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, code, text, mode 2239 , orderedBy, entry, emptyReason, section); 2240 } 2241 2242 public String fhirType() { 2243 return "Composition.section"; 2244 2245 } 2246 2247 } 2248 2249 /** 2250 * Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time. 2251 */ 2252 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2253 @Description(shortDefinition="Logical identifier of composition (version-independent)", formalDefinition="Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time." ) 2254 protected Identifier identifier; 2255 2256 /** 2257 * The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document. 2258 */ 2259 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2260 @Description(shortDefinition="preliminary | final | amended | entered-in-error", formalDefinition="The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document." ) 2261 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composition-status") 2262 protected Enumeration<CompositionStatus> status; 2263 2264 /** 2265 * Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition. 2266 */ 2267 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 2268 @Description(shortDefinition="Kind of composition (LOINC if possible)", formalDefinition="Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition." ) 2269 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/doc-typecodes") 2270 protected CodeableConcept type; 2271 2272 /** 2273 * A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type. 2274 */ 2275 @Child(name = "class", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 2276 @Description(shortDefinition="Categorization of Composition", formalDefinition="A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type." ) 2277 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/doc-classcodes") 2278 protected CodeableConcept class_; 2279 2280 /** 2281 * Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure). 2282 */ 2283 @Child(name = "subject", type = {Reference.class}, order=4, min=1, max=1, modifier=false, summary=true) 2284 @Description(shortDefinition="Who and/or what the composition is about", formalDefinition="Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure)." ) 2285 protected Reference subject; 2286 2287 /** 2288 * The actual object that is the target of the reference (Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).) 2289 */ 2290 protected Resource subjectTarget; 2291 2292 /** 2293 * Describes the clinical encounter or type of care this documentation is associated with. 2294 */ 2295 @Child(name = "encounter", type = {Encounter.class}, order=5, min=0, max=1, modifier=false, summary=true) 2296 @Description(shortDefinition="Context of the Composition", formalDefinition="Describes the clinical encounter or type of care this documentation is associated with." ) 2297 protected Reference encounter; 2298 2299 /** 2300 * The actual object that is the target of the reference (Describes the clinical encounter or type of care this documentation is associated with.) 2301 */ 2302 protected Encounter encounterTarget; 2303 2304 /** 2305 * The composition editing time, when the composition was last logically changed by the author. 2306 */ 2307 @Child(name = "date", type = {DateTimeType.class}, order=6, min=1, max=1, modifier=false, summary=true) 2308 @Description(shortDefinition="Composition editing time", formalDefinition="The composition editing time, when the composition was last logically changed by the author." ) 2309 protected DateTimeType date; 2310 2311 /** 2312 * Identifies who is responsible for the information in the composition, not necessarily who typed it in. 2313 */ 2314 @Child(name = "author", type = {Practitioner.class, Device.class, Patient.class, RelatedPerson.class}, order=7, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2315 @Description(shortDefinition="Who and/or what authored the composition", formalDefinition="Identifies who is responsible for the information in the composition, not necessarily who typed it in." ) 2316 protected List<Reference> author; 2317 /** 2318 * The actual objects that are the target of the reference (Identifies who is responsible for the information in the composition, not necessarily who typed it in.) 2319 */ 2320 protected List<Resource> authorTarget; 2321 2322 2323 /** 2324 * Official human-readable label for the composition. 2325 */ 2326 @Child(name = "title", type = {StringType.class}, order=8, min=1, max=1, modifier=false, summary=true) 2327 @Description(shortDefinition="Human Readable name/title", formalDefinition="Official human-readable label for the composition." ) 2328 protected StringType title; 2329 2330 /** 2331 * The code specifying the level of confidentiality of the Composition. 2332 */ 2333 @Child(name = "confidentiality", type = {CodeType.class}, order=9, min=0, max=1, modifier=true, summary=true) 2334 @Description(shortDefinition="As defined by affinity domain", formalDefinition="The code specifying the level of confidentiality of the Composition." ) 2335 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ConfidentialityClassification") 2336 protected Enumeration<DocumentConfidentiality> confidentiality; 2337 2338 /** 2339 * A participant who has attested to the accuracy of the composition/document. 2340 */ 2341 @Child(name = "attester", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2342 @Description(shortDefinition="Attests to accuracy of composition", formalDefinition="A participant who has attested to the accuracy of the composition/document." ) 2343 protected List<CompositionAttesterComponent> attester; 2344 2345 /** 2346 * Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information. 2347 */ 2348 @Child(name = "custodian", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 2349 @Description(shortDefinition="Organization which maintains the composition", formalDefinition="Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information." ) 2350 protected Reference custodian; 2351 2352 /** 2353 * The actual object that is the target of the reference (Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.) 2354 */ 2355 protected Organization custodianTarget; 2356 2357 /** 2358 * Relationships that this composition has with other compositions or documents that already exist. 2359 */ 2360 @Child(name = "relatesTo", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2361 @Description(shortDefinition="Relationships to other compositions/documents", formalDefinition="Relationships that this composition has with other compositions or documents that already exist." ) 2362 protected List<CompositionRelatesToComponent> relatesTo; 2363 2364 /** 2365 * The clinical service, such as a colonoscopy or an appendectomy, being documented. 2366 */ 2367 @Child(name = "event", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2368 @Description(shortDefinition="The clinical service(s) being documented", formalDefinition="The clinical service, such as a colonoscopy or an appendectomy, being documented." ) 2369 protected List<CompositionEventComponent> event; 2370 2371 /** 2372 * The root of the sections that make up the composition. 2373 */ 2374 @Child(name = "section", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2375 @Description(shortDefinition="Composition is broken into sections", formalDefinition="The root of the sections that make up the composition." ) 2376 protected List<SectionComponent> section; 2377 2378 private static final long serialVersionUID = -1422555114L; 2379 2380 /** 2381 * Constructor 2382 */ 2383 public Composition() { 2384 super(); 2385 } 2386 2387 /** 2388 * Constructor 2389 */ 2390 public Composition(Enumeration<CompositionStatus> status, CodeableConcept type, Reference subject, DateTimeType date, StringType title) { 2391 super(); 2392 this.status = status; 2393 this.type = type; 2394 this.subject = subject; 2395 this.date = date; 2396 this.title = title; 2397 } 2398 2399 /** 2400 * @return {@link #identifier} (Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time.) 2401 */ 2402 public Identifier getIdentifier() { 2403 if (this.identifier == null) 2404 if (Configuration.errorOnAutoCreate()) 2405 throw new Error("Attempt to auto-create Composition.identifier"); 2406 else if (Configuration.doAutoCreate()) 2407 this.identifier = new Identifier(); // cc 2408 return this.identifier; 2409 } 2410 2411 public boolean hasIdentifier() { 2412 return this.identifier != null && !this.identifier.isEmpty(); 2413 } 2414 2415 /** 2416 * @param value {@link #identifier} (Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time.) 2417 */ 2418 public Composition setIdentifier(Identifier value) { 2419 this.identifier = value; 2420 return this; 2421 } 2422 2423 /** 2424 * @return {@link #status} (The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2425 */ 2426 public Enumeration<CompositionStatus> getStatusElement() { 2427 if (this.status == null) 2428 if (Configuration.errorOnAutoCreate()) 2429 throw new Error("Attempt to auto-create Composition.status"); 2430 else if (Configuration.doAutoCreate()) 2431 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); // bb 2432 return this.status; 2433 } 2434 2435 public boolean hasStatusElement() { 2436 return this.status != null && !this.status.isEmpty(); 2437 } 2438 2439 public boolean hasStatus() { 2440 return this.status != null && !this.status.isEmpty(); 2441 } 2442 2443 /** 2444 * @param value {@link #status} (The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2445 */ 2446 public Composition setStatusElement(Enumeration<CompositionStatus> value) { 2447 this.status = value; 2448 return this; 2449 } 2450 2451 /** 2452 * @return The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document. 2453 */ 2454 public CompositionStatus getStatus() { 2455 return this.status == null ? null : this.status.getValue(); 2456 } 2457 2458 /** 2459 * @param value The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document. 2460 */ 2461 public Composition setStatus(CompositionStatus value) { 2462 if (this.status == null) 2463 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); 2464 this.status.setValue(value); 2465 return this; 2466 } 2467 2468 /** 2469 * @return {@link #type} (Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.) 2470 */ 2471 public CodeableConcept getType() { 2472 if (this.type == null) 2473 if (Configuration.errorOnAutoCreate()) 2474 throw new Error("Attempt to auto-create Composition.type"); 2475 else if (Configuration.doAutoCreate()) 2476 this.type = new CodeableConcept(); // cc 2477 return this.type; 2478 } 2479 2480 public boolean hasType() { 2481 return this.type != null && !this.type.isEmpty(); 2482 } 2483 2484 /** 2485 * @param value {@link #type} (Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.) 2486 */ 2487 public Composition setType(CodeableConcept value) { 2488 this.type = value; 2489 return this; 2490 } 2491 2492 /** 2493 * @return {@link #class_} (A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.) 2494 */ 2495 public CodeableConcept getClass_() { 2496 if (this.class_ == null) 2497 if (Configuration.errorOnAutoCreate()) 2498 throw new Error("Attempt to auto-create Composition.class_"); 2499 else if (Configuration.doAutoCreate()) 2500 this.class_ = new CodeableConcept(); // cc 2501 return this.class_; 2502 } 2503 2504 public boolean hasClass_() { 2505 return this.class_ != null && !this.class_.isEmpty(); 2506 } 2507 2508 /** 2509 * @param value {@link #class_} (A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.) 2510 */ 2511 public Composition setClass_(CodeableConcept value) { 2512 this.class_ = value; 2513 return this; 2514 } 2515 2516 /** 2517 * @return {@link #subject} (Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).) 2518 */ 2519 public Reference getSubject() { 2520 if (this.subject == null) 2521 if (Configuration.errorOnAutoCreate()) 2522 throw new Error("Attempt to auto-create Composition.subject"); 2523 else if (Configuration.doAutoCreate()) 2524 this.subject = new Reference(); // cc 2525 return this.subject; 2526 } 2527 2528 public boolean hasSubject() { 2529 return this.subject != null && !this.subject.isEmpty(); 2530 } 2531 2532 /** 2533 * @param value {@link #subject} (Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).) 2534 */ 2535 public Composition setSubject(Reference value) { 2536 this.subject = value; 2537 return this; 2538 } 2539 2540 /** 2541 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).) 2542 */ 2543 public Resource getSubjectTarget() { 2544 return this.subjectTarget; 2545 } 2546 2547 /** 2548 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).) 2549 */ 2550 public Composition setSubjectTarget(Resource value) { 2551 this.subjectTarget = value; 2552 return this; 2553 } 2554 2555 /** 2556 * @return {@link #encounter} (Describes the clinical encounter or type of care this documentation is associated with.) 2557 */ 2558 public Reference getEncounter() { 2559 if (this.encounter == null) 2560 if (Configuration.errorOnAutoCreate()) 2561 throw new Error("Attempt to auto-create Composition.encounter"); 2562 else if (Configuration.doAutoCreate()) 2563 this.encounter = new Reference(); // cc 2564 return this.encounter; 2565 } 2566 2567 public boolean hasEncounter() { 2568 return this.encounter != null && !this.encounter.isEmpty(); 2569 } 2570 2571 /** 2572 * @param value {@link #encounter} (Describes the clinical encounter or type of care this documentation is associated with.) 2573 */ 2574 public Composition setEncounter(Reference value) { 2575 this.encounter = value; 2576 return this; 2577 } 2578 2579 /** 2580 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Describes the clinical encounter or type of care this documentation is associated with.) 2581 */ 2582 public Encounter getEncounterTarget() { 2583 if (this.encounterTarget == null) 2584 if (Configuration.errorOnAutoCreate()) 2585 throw new Error("Attempt to auto-create Composition.encounter"); 2586 else if (Configuration.doAutoCreate()) 2587 this.encounterTarget = new Encounter(); // aa 2588 return this.encounterTarget; 2589 } 2590 2591 /** 2592 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Describes the clinical encounter or type of care this documentation is associated with.) 2593 */ 2594 public Composition setEncounterTarget(Encounter value) { 2595 this.encounterTarget = value; 2596 return this; 2597 } 2598 2599 /** 2600 * @return {@link #date} (The composition editing time, when the composition was last logically changed by the author.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2601 */ 2602 public DateTimeType getDateElement() { 2603 if (this.date == null) 2604 if (Configuration.errorOnAutoCreate()) 2605 throw new Error("Attempt to auto-create Composition.date"); 2606 else if (Configuration.doAutoCreate()) 2607 this.date = new DateTimeType(); // bb 2608 return this.date; 2609 } 2610 2611 public boolean hasDateElement() { 2612 return this.date != null && !this.date.isEmpty(); 2613 } 2614 2615 public boolean hasDate() { 2616 return this.date != null && !this.date.isEmpty(); 2617 } 2618 2619 /** 2620 * @param value {@link #date} (The composition editing time, when the composition was last logically changed by the author.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2621 */ 2622 public Composition setDateElement(DateTimeType value) { 2623 this.date = value; 2624 return this; 2625 } 2626 2627 /** 2628 * @return The composition editing time, when the composition was last logically changed by the author. 2629 */ 2630 public Date getDate() { 2631 return this.date == null ? null : this.date.getValue(); 2632 } 2633 2634 /** 2635 * @param value The composition editing time, when the composition was last logically changed by the author. 2636 */ 2637 public Composition setDate(Date value) { 2638 if (this.date == null) 2639 this.date = new DateTimeType(); 2640 this.date.setValue(value); 2641 return this; 2642 } 2643 2644 /** 2645 * @return {@link #author} (Identifies who is responsible for the information in the composition, not necessarily who typed it in.) 2646 */ 2647 public List<Reference> getAuthor() { 2648 if (this.author == null) 2649 this.author = new ArrayList<Reference>(); 2650 return this.author; 2651 } 2652 2653 /** 2654 * @return Returns a reference to <code>this</code> for easy method chaining 2655 */ 2656 public Composition setAuthor(List<Reference> theAuthor) { 2657 this.author = theAuthor; 2658 return this; 2659 } 2660 2661 public boolean hasAuthor() { 2662 if (this.author == null) 2663 return false; 2664 for (Reference item : this.author) 2665 if (!item.isEmpty()) 2666 return true; 2667 return false; 2668 } 2669 2670 public Reference addAuthor() { //3 2671 Reference t = new Reference(); 2672 if (this.author == null) 2673 this.author = new ArrayList<Reference>(); 2674 this.author.add(t); 2675 return t; 2676 } 2677 2678 public Composition addAuthor(Reference t) { //3 2679 if (t == null) 2680 return this; 2681 if (this.author == null) 2682 this.author = new ArrayList<Reference>(); 2683 this.author.add(t); 2684 return this; 2685 } 2686 2687 /** 2688 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist 2689 */ 2690 public Reference getAuthorFirstRep() { 2691 if (getAuthor().isEmpty()) { 2692 addAuthor(); 2693 } 2694 return getAuthor().get(0); 2695 } 2696 2697 /** 2698 * @deprecated Use Reference#setResource(IBaseResource) instead 2699 */ 2700 @Deprecated 2701 public List<Resource> getAuthorTarget() { 2702 if (this.authorTarget == null) 2703 this.authorTarget = new ArrayList<Resource>(); 2704 return this.authorTarget; 2705 } 2706 2707 /** 2708 * @return {@link #title} (Official human-readable label for the composition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2709 */ 2710 public StringType getTitleElement() { 2711 if (this.title == null) 2712 if (Configuration.errorOnAutoCreate()) 2713 throw new Error("Attempt to auto-create Composition.title"); 2714 else if (Configuration.doAutoCreate()) 2715 this.title = new StringType(); // bb 2716 return this.title; 2717 } 2718 2719 public boolean hasTitleElement() { 2720 return this.title != null && !this.title.isEmpty(); 2721 } 2722 2723 public boolean hasTitle() { 2724 return this.title != null && !this.title.isEmpty(); 2725 } 2726 2727 /** 2728 * @param value {@link #title} (Official human-readable label for the composition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2729 */ 2730 public Composition setTitleElement(StringType value) { 2731 this.title = value; 2732 return this; 2733 } 2734 2735 /** 2736 * @return Official human-readable label for the composition. 2737 */ 2738 public String getTitle() { 2739 return this.title == null ? null : this.title.getValue(); 2740 } 2741 2742 /** 2743 * @param value Official human-readable label for the composition. 2744 */ 2745 public Composition setTitle(String value) { 2746 if (this.title == null) 2747 this.title = new StringType(); 2748 this.title.setValue(value); 2749 return this; 2750 } 2751 2752 /** 2753 * @return {@link #confidentiality} (The code specifying the level of confidentiality of the Composition.). This is the underlying object with id, value and extensions. The accessor "getConfidentiality" gives direct access to the value 2754 */ 2755 public Enumeration<DocumentConfidentiality> getConfidentialityElement() { 2756 if (this.confidentiality == null) 2757 if (Configuration.errorOnAutoCreate()) 2758 throw new Error("Attempt to auto-create Composition.confidentiality"); 2759 else if (Configuration.doAutoCreate()) 2760 this.confidentiality = new Enumeration<DocumentConfidentiality>(new DocumentConfidentialityEnumFactory()); // bb 2761 return this.confidentiality; 2762 } 2763 2764 public boolean hasConfidentialityElement() { 2765 return this.confidentiality != null && !this.confidentiality.isEmpty(); 2766 } 2767 2768 public boolean hasConfidentiality() { 2769 return this.confidentiality != null && !this.confidentiality.isEmpty(); 2770 } 2771 2772 /** 2773 * @param value {@link #confidentiality} (The code specifying the level of confidentiality of the Composition.). This is the underlying object with id, value and extensions. The accessor "getConfidentiality" gives direct access to the value 2774 */ 2775 public Composition setConfidentialityElement(Enumeration<DocumentConfidentiality> value) { 2776 this.confidentiality = value; 2777 return this; 2778 } 2779 2780 /** 2781 * @return The code specifying the level of confidentiality of the Composition. 2782 */ 2783 public DocumentConfidentiality getConfidentiality() { 2784 return this.confidentiality == null ? null : this.confidentiality.getValue(); 2785 } 2786 2787 /** 2788 * @param value The code specifying the level of confidentiality of the Composition. 2789 */ 2790 public Composition setConfidentiality(DocumentConfidentiality value) { 2791 if (value == null) 2792 this.confidentiality = null; 2793 else { 2794 if (this.confidentiality == null) 2795 this.confidentiality = new Enumeration<DocumentConfidentiality>(new DocumentConfidentialityEnumFactory()); 2796 this.confidentiality.setValue(value); 2797 } 2798 return this; 2799 } 2800 2801 /** 2802 * @return {@link #attester} (A participant who has attested to the accuracy of the composition/document.) 2803 */ 2804 public List<CompositionAttesterComponent> getAttester() { 2805 if (this.attester == null) 2806 this.attester = new ArrayList<CompositionAttesterComponent>(); 2807 return this.attester; 2808 } 2809 2810 /** 2811 * @return Returns a reference to <code>this</code> for easy method chaining 2812 */ 2813 public Composition setAttester(List<CompositionAttesterComponent> theAttester) { 2814 this.attester = theAttester; 2815 return this; 2816 } 2817 2818 public boolean hasAttester() { 2819 if (this.attester == null) 2820 return false; 2821 for (CompositionAttesterComponent item : this.attester) 2822 if (!item.isEmpty()) 2823 return true; 2824 return false; 2825 } 2826 2827 public CompositionAttesterComponent addAttester() { //3 2828 CompositionAttesterComponent t = new CompositionAttesterComponent(); 2829 if (this.attester == null) 2830 this.attester = new ArrayList<CompositionAttesterComponent>(); 2831 this.attester.add(t); 2832 return t; 2833 } 2834 2835 public Composition addAttester(CompositionAttesterComponent t) { //3 2836 if (t == null) 2837 return this; 2838 if (this.attester == null) 2839 this.attester = new ArrayList<CompositionAttesterComponent>(); 2840 this.attester.add(t); 2841 return this; 2842 } 2843 2844 /** 2845 * @return The first repetition of repeating field {@link #attester}, creating it if it does not already exist 2846 */ 2847 public CompositionAttesterComponent getAttesterFirstRep() { 2848 if (getAttester().isEmpty()) { 2849 addAttester(); 2850 } 2851 return getAttester().get(0); 2852 } 2853 2854 /** 2855 * @return {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.) 2856 */ 2857 public Reference getCustodian() { 2858 if (this.custodian == null) 2859 if (Configuration.errorOnAutoCreate()) 2860 throw new Error("Attempt to auto-create Composition.custodian"); 2861 else if (Configuration.doAutoCreate()) 2862 this.custodian = new Reference(); // cc 2863 return this.custodian; 2864 } 2865 2866 public boolean hasCustodian() { 2867 return this.custodian != null && !this.custodian.isEmpty(); 2868 } 2869 2870 /** 2871 * @param value {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.) 2872 */ 2873 public Composition setCustodian(Reference value) { 2874 this.custodian = value; 2875 return this; 2876 } 2877 2878 /** 2879 * @return {@link #custodian} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.) 2880 */ 2881 public Organization getCustodianTarget() { 2882 if (this.custodianTarget == null) 2883 if (Configuration.errorOnAutoCreate()) 2884 throw new Error("Attempt to auto-create Composition.custodian"); 2885 else if (Configuration.doAutoCreate()) 2886 this.custodianTarget = new Organization(); // aa 2887 return this.custodianTarget; 2888 } 2889 2890 /** 2891 * @param value {@link #custodian} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.) 2892 */ 2893 public Composition setCustodianTarget(Organization value) { 2894 this.custodianTarget = value; 2895 return this; 2896 } 2897 2898 /** 2899 * @return {@link #relatesTo} (Relationships that this composition has with other compositions or documents that already exist.) 2900 */ 2901 public List<CompositionRelatesToComponent> getRelatesTo() { 2902 if (this.relatesTo == null) 2903 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 2904 return this.relatesTo; 2905 } 2906 2907 /** 2908 * @return Returns a reference to <code>this</code> for easy method chaining 2909 */ 2910 public Composition setRelatesTo(List<CompositionRelatesToComponent> theRelatesTo) { 2911 this.relatesTo = theRelatesTo; 2912 return this; 2913 } 2914 2915 public boolean hasRelatesTo() { 2916 if (this.relatesTo == null) 2917 return false; 2918 for (CompositionRelatesToComponent item : this.relatesTo) 2919 if (!item.isEmpty()) 2920 return true; 2921 return false; 2922 } 2923 2924 public CompositionRelatesToComponent addRelatesTo() { //3 2925 CompositionRelatesToComponent t = new CompositionRelatesToComponent(); 2926 if (this.relatesTo == null) 2927 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 2928 this.relatesTo.add(t); 2929 return t; 2930 } 2931 2932 public Composition addRelatesTo(CompositionRelatesToComponent t) { //3 2933 if (t == null) 2934 return this; 2935 if (this.relatesTo == null) 2936 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 2937 this.relatesTo.add(t); 2938 return this; 2939 } 2940 2941 /** 2942 * @return The first repetition of repeating field {@link #relatesTo}, creating it if it does not already exist 2943 */ 2944 public CompositionRelatesToComponent getRelatesToFirstRep() { 2945 if (getRelatesTo().isEmpty()) { 2946 addRelatesTo(); 2947 } 2948 return getRelatesTo().get(0); 2949 } 2950 2951 /** 2952 * @return {@link #event} (The clinical service, such as a colonoscopy or an appendectomy, being documented.) 2953 */ 2954 public List<CompositionEventComponent> getEvent() { 2955 if (this.event == null) 2956 this.event = new ArrayList<CompositionEventComponent>(); 2957 return this.event; 2958 } 2959 2960 /** 2961 * @return Returns a reference to <code>this</code> for easy method chaining 2962 */ 2963 public Composition setEvent(List<CompositionEventComponent> theEvent) { 2964 this.event = theEvent; 2965 return this; 2966 } 2967 2968 public boolean hasEvent() { 2969 if (this.event == null) 2970 return false; 2971 for (CompositionEventComponent item : this.event) 2972 if (!item.isEmpty()) 2973 return true; 2974 return false; 2975 } 2976 2977 public CompositionEventComponent addEvent() { //3 2978 CompositionEventComponent t = new CompositionEventComponent(); 2979 if (this.event == null) 2980 this.event = new ArrayList<CompositionEventComponent>(); 2981 this.event.add(t); 2982 return t; 2983 } 2984 2985 public Composition addEvent(CompositionEventComponent t) { //3 2986 if (t == null) 2987 return this; 2988 if (this.event == null) 2989 this.event = new ArrayList<CompositionEventComponent>(); 2990 this.event.add(t); 2991 return this; 2992 } 2993 2994 /** 2995 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist 2996 */ 2997 public CompositionEventComponent getEventFirstRep() { 2998 if (getEvent().isEmpty()) { 2999 addEvent(); 3000 } 3001 return getEvent().get(0); 3002 } 3003 3004 /** 3005 * @return {@link #section} (The root of the sections that make up the composition.) 3006 */ 3007 public List<SectionComponent> getSection() { 3008 if (this.section == null) 3009 this.section = new ArrayList<SectionComponent>(); 3010 return this.section; 3011 } 3012 3013 /** 3014 * @return Returns a reference to <code>this</code> for easy method chaining 3015 */ 3016 public Composition setSection(List<SectionComponent> theSection) { 3017 this.section = theSection; 3018 return this; 3019 } 3020 3021 public boolean hasSection() { 3022 if (this.section == null) 3023 return false; 3024 for (SectionComponent item : this.section) 3025 if (!item.isEmpty()) 3026 return true; 3027 return false; 3028 } 3029 3030 public SectionComponent addSection() { //3 3031 SectionComponent t = new SectionComponent(); 3032 if (this.section == null) 3033 this.section = new ArrayList<SectionComponent>(); 3034 this.section.add(t); 3035 return t; 3036 } 3037 3038 public Composition addSection(SectionComponent t) { //3 3039 if (t == null) 3040 return this; 3041 if (this.section == null) 3042 this.section = new ArrayList<SectionComponent>(); 3043 this.section.add(t); 3044 return this; 3045 } 3046 3047 /** 3048 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist 3049 */ 3050 public SectionComponent getSectionFirstRep() { 3051 if (getSection().isEmpty()) { 3052 addSection(); 3053 } 3054 return getSection().get(0); 3055 } 3056 3057 protected void listChildren(List<Property> children) { 3058 super.listChildren(children); 3059 children.add(new Property("identifier", "Identifier", "Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time.", 0, 1, identifier)); 3060 children.add(new Property("status", "code", "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 0, 1, status)); 3061 children.add(new Property("type", "CodeableConcept", "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 0, 1, type)); 3062 children.add(new Property("class", "CodeableConcept", "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 0, 1, class_)); 3063 children.add(new Property("subject", "Reference(Any)", "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 0, 1, subject)); 3064 children.add(new Property("encounter", "Reference(Encounter)", "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter)); 3065 children.add(new Property("date", "dateTime", "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date)); 3066 children.add(new Property("author", "Reference(Practitioner|Device|Patient|RelatedPerson)", "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author)); 3067 children.add(new Property("title", "string", "Official human-readable label for the composition.", 0, 1, title)); 3068 children.add(new Property("confidentiality", "code", "The code specifying the level of confidentiality of the Composition.", 0, 1, confidentiality)); 3069 children.add(new Property("attester", "", "A participant who has attested to the accuracy of the composition/document.", 0, java.lang.Integer.MAX_VALUE, attester)); 3070 children.add(new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 0, 1, custodian)); 3071 children.add(new Property("relatesTo", "", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo)); 3072 children.add(new Property("event", "", "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 0, java.lang.Integer.MAX_VALUE, event)); 3073 children.add(new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section)); 3074 } 3075 3076 @Override 3077 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3078 switch (_hash) { 3079 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time.", 0, 1, identifier); 3080 case -892481550: /*status*/ return new Property("status", "code", "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 0, 1, status); 3081 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 0, 1, type); 3082 case 94742904: /*class*/ return new Property("class", "CodeableConcept", "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 0, 1, class_); 3083 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 0, 1, subject); 3084 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter); 3085 case 3076014: /*date*/ return new Property("date", "dateTime", "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date); 3086 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Device|Patient|RelatedPerson)", "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author); 3087 case 110371416: /*title*/ return new Property("title", "string", "Official human-readable label for the composition.", 0, 1, title); 3088 case -1923018202: /*confidentiality*/ return new Property("confidentiality", "code", "The code specifying the level of confidentiality of the Composition.", 0, 1, confidentiality); 3089 case 542920370: /*attester*/ return new Property("attester", "", "A participant who has attested to the accuracy of the composition/document.", 0, java.lang.Integer.MAX_VALUE, attester); 3090 case 1611297262: /*custodian*/ return new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 0, 1, custodian); 3091 case -7765931: /*relatesTo*/ return new Property("relatesTo", "", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo); 3092 case 96891546: /*event*/ return new Property("event", "", "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 0, java.lang.Integer.MAX_VALUE, event); 3093 case 1970241253: /*section*/ return new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section); 3094 default: return super.getNamedProperty(_hash, _name, _checkValid); 3095 } 3096 3097 } 3098 3099 @Override 3100 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3101 switch (hash) { 3102 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3103 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CompositionStatus> 3104 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3105 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // CodeableConcept 3106 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3107 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3108 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3109 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 3110 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3111 case -1923018202: /*confidentiality*/ return this.confidentiality == null ? new Base[0] : new Base[] {this.confidentiality}; // Enumeration<DocumentConfidentiality> 3112 case 542920370: /*attester*/ return this.attester == null ? new Base[0] : this.attester.toArray(new Base[this.attester.size()]); // CompositionAttesterComponent 3113 case 1611297262: /*custodian*/ return this.custodian == null ? new Base[0] : new Base[] {this.custodian}; // Reference 3114 case -7765931: /*relatesTo*/ return this.relatesTo == null ? new Base[0] : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // CompositionRelatesToComponent 3115 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CompositionEventComponent 3116 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 3117 default: return super.getProperty(hash, name, checkValid); 3118 } 3119 3120 } 3121 3122 @Override 3123 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3124 switch (hash) { 3125 case -1618432855: // identifier 3126 this.identifier = castToIdentifier(value); // Identifier 3127 return value; 3128 case -892481550: // status 3129 value = new CompositionStatusEnumFactory().fromType(castToCode(value)); 3130 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 3131 return value; 3132 case 3575610: // type 3133 this.type = castToCodeableConcept(value); // CodeableConcept 3134 return value; 3135 case 94742904: // class 3136 this.class_ = castToCodeableConcept(value); // CodeableConcept 3137 return value; 3138 case -1867885268: // subject 3139 this.subject = castToReference(value); // Reference 3140 return value; 3141 case 1524132147: // encounter 3142 this.encounter = castToReference(value); // Reference 3143 return value; 3144 case 3076014: // date 3145 this.date = castToDateTime(value); // DateTimeType 3146 return value; 3147 case -1406328437: // author 3148 this.getAuthor().add(castToReference(value)); // Reference 3149 return value; 3150 case 110371416: // title 3151 this.title = castToString(value); // StringType 3152 return value; 3153 case -1923018202: // confidentiality 3154 value = new DocumentConfidentialityEnumFactory().fromType(castToCode(value)); 3155 this.confidentiality = (Enumeration) value; // Enumeration<DocumentConfidentiality> 3156 return value; 3157 case 542920370: // attester 3158 this.getAttester().add((CompositionAttesterComponent) value); // CompositionAttesterComponent 3159 return value; 3160 case 1611297262: // custodian 3161 this.custodian = castToReference(value); // Reference 3162 return value; 3163 case -7765931: // relatesTo 3164 this.getRelatesTo().add((CompositionRelatesToComponent) value); // CompositionRelatesToComponent 3165 return value; 3166 case 96891546: // event 3167 this.getEvent().add((CompositionEventComponent) value); // CompositionEventComponent 3168 return value; 3169 case 1970241253: // section 3170 this.getSection().add((SectionComponent) value); // SectionComponent 3171 return value; 3172 default: return super.setProperty(hash, name, value); 3173 } 3174 3175 } 3176 3177 @Override 3178 public Base setProperty(String name, Base value) throws FHIRException { 3179 if (name.equals("identifier")) { 3180 this.identifier = castToIdentifier(value); // Identifier 3181 } else if (name.equals("status")) { 3182 value = new CompositionStatusEnumFactory().fromType(castToCode(value)); 3183 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 3184 } else if (name.equals("type")) { 3185 this.type = castToCodeableConcept(value); // CodeableConcept 3186 } else if (name.equals("class")) { 3187 this.class_ = castToCodeableConcept(value); // CodeableConcept 3188 } else if (name.equals("subject")) { 3189 this.subject = castToReference(value); // Reference 3190 } else if (name.equals("encounter")) { 3191 this.encounter = castToReference(value); // Reference 3192 } else if (name.equals("date")) { 3193 this.date = castToDateTime(value); // DateTimeType 3194 } else if (name.equals("author")) { 3195 this.getAuthor().add(castToReference(value)); 3196 } else if (name.equals("title")) { 3197 this.title = castToString(value); // StringType 3198 } else if (name.equals("confidentiality")) { 3199 value = new DocumentConfidentialityEnumFactory().fromType(castToCode(value)); 3200 this.confidentiality = (Enumeration) value; // Enumeration<DocumentConfidentiality> 3201 } else if (name.equals("attester")) { 3202 this.getAttester().add((CompositionAttesterComponent) value); 3203 } else if (name.equals("custodian")) { 3204 this.custodian = castToReference(value); // Reference 3205 } else if (name.equals("relatesTo")) { 3206 this.getRelatesTo().add((CompositionRelatesToComponent) value); 3207 } else if (name.equals("event")) { 3208 this.getEvent().add((CompositionEventComponent) value); 3209 } else if (name.equals("section")) { 3210 this.getSection().add((SectionComponent) value); 3211 } else 3212 return super.setProperty(name, value); 3213 return value; 3214 } 3215 3216 @Override 3217 public Base makeProperty(int hash, String name) throws FHIRException { 3218 switch (hash) { 3219 case -1618432855: return getIdentifier(); 3220 case -892481550: return getStatusElement(); 3221 case 3575610: return getType(); 3222 case 94742904: return getClass_(); 3223 case -1867885268: return getSubject(); 3224 case 1524132147: return getEncounter(); 3225 case 3076014: return getDateElement(); 3226 case -1406328437: return addAuthor(); 3227 case 110371416: return getTitleElement(); 3228 case -1923018202: return getConfidentialityElement(); 3229 case 542920370: return addAttester(); 3230 case 1611297262: return getCustodian(); 3231 case -7765931: return addRelatesTo(); 3232 case 96891546: return addEvent(); 3233 case 1970241253: return addSection(); 3234 default: return super.makeProperty(hash, name); 3235 } 3236 3237 } 3238 3239 @Override 3240 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3241 switch (hash) { 3242 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3243 case -892481550: /*status*/ return new String[] {"code"}; 3244 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3245 case 94742904: /*class*/ return new String[] {"CodeableConcept"}; 3246 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3247 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3248 case 3076014: /*date*/ return new String[] {"dateTime"}; 3249 case -1406328437: /*author*/ return new String[] {"Reference"}; 3250 case 110371416: /*title*/ return new String[] {"string"}; 3251 case -1923018202: /*confidentiality*/ return new String[] {"code"}; 3252 case 542920370: /*attester*/ return new String[] {}; 3253 case 1611297262: /*custodian*/ return new String[] {"Reference"}; 3254 case -7765931: /*relatesTo*/ return new String[] {}; 3255 case 96891546: /*event*/ return new String[] {}; 3256 case 1970241253: /*section*/ return new String[] {}; 3257 default: return super.getTypesForProperty(hash, name); 3258 } 3259 3260 } 3261 3262 @Override 3263 public Base addChild(String name) throws FHIRException { 3264 if (name.equals("identifier")) { 3265 this.identifier = new Identifier(); 3266 return this.identifier; 3267 } 3268 else if (name.equals("status")) { 3269 throw new FHIRException("Cannot call addChild on a singleton property Composition.status"); 3270 } 3271 else if (name.equals("type")) { 3272 this.type = new CodeableConcept(); 3273 return this.type; 3274 } 3275 else if (name.equals("class")) { 3276 this.class_ = new CodeableConcept(); 3277 return this.class_; 3278 } 3279 else if (name.equals("subject")) { 3280 this.subject = new Reference(); 3281 return this.subject; 3282 } 3283 else if (name.equals("encounter")) { 3284 this.encounter = new Reference(); 3285 return this.encounter; 3286 } 3287 else if (name.equals("date")) { 3288 throw new FHIRException("Cannot call addChild on a singleton property Composition.date"); 3289 } 3290 else if (name.equals("author")) { 3291 return addAuthor(); 3292 } 3293 else if (name.equals("title")) { 3294 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 3295 } 3296 else if (name.equals("confidentiality")) { 3297 throw new FHIRException("Cannot call addChild on a singleton property Composition.confidentiality"); 3298 } 3299 else if (name.equals("attester")) { 3300 return addAttester(); 3301 } 3302 else if (name.equals("custodian")) { 3303 this.custodian = new Reference(); 3304 return this.custodian; 3305 } 3306 else if (name.equals("relatesTo")) { 3307 return addRelatesTo(); 3308 } 3309 else if (name.equals("event")) { 3310 return addEvent(); 3311 } 3312 else if (name.equals("section")) { 3313 return addSection(); 3314 } 3315 else 3316 return super.addChild(name); 3317 } 3318 3319 public String fhirType() { 3320 return "Composition"; 3321 3322 } 3323 3324 public Composition copy() { 3325 Composition dst = new Composition(); 3326 copyValues(dst); 3327 dst.identifier = identifier == null ? null : identifier.copy(); 3328 dst.status = status == null ? null : status.copy(); 3329 dst.type = type == null ? null : type.copy(); 3330 dst.class_ = class_ == null ? null : class_.copy(); 3331 dst.subject = subject == null ? null : subject.copy(); 3332 dst.encounter = encounter == null ? null : encounter.copy(); 3333 dst.date = date == null ? null : date.copy(); 3334 if (author != null) { 3335 dst.author = new ArrayList<Reference>(); 3336 for (Reference i : author) 3337 dst.author.add(i.copy()); 3338 }; 3339 dst.title = title == null ? null : title.copy(); 3340 dst.confidentiality = confidentiality == null ? null : confidentiality.copy(); 3341 if (attester != null) { 3342 dst.attester = new ArrayList<CompositionAttesterComponent>(); 3343 for (CompositionAttesterComponent i : attester) 3344 dst.attester.add(i.copy()); 3345 }; 3346 dst.custodian = custodian == null ? null : custodian.copy(); 3347 if (relatesTo != null) { 3348 dst.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 3349 for (CompositionRelatesToComponent i : relatesTo) 3350 dst.relatesTo.add(i.copy()); 3351 }; 3352 if (event != null) { 3353 dst.event = new ArrayList<CompositionEventComponent>(); 3354 for (CompositionEventComponent i : event) 3355 dst.event.add(i.copy()); 3356 }; 3357 if (section != null) { 3358 dst.section = new ArrayList<SectionComponent>(); 3359 for (SectionComponent i : section) 3360 dst.section.add(i.copy()); 3361 }; 3362 return dst; 3363 } 3364 3365 protected Composition typedCopy() { 3366 return copy(); 3367 } 3368 3369 @Override 3370 public boolean equalsDeep(Base other_) { 3371 if (!super.equalsDeep(other_)) 3372 return false; 3373 if (!(other_ instanceof Composition)) 3374 return false; 3375 Composition o = (Composition) other_; 3376 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 3377 && compareDeep(class_, o.class_, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 3378 && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) && compareDeep(title, o.title, true) 3379 && compareDeep(confidentiality, o.confidentiality, true) && compareDeep(attester, o.attester, true) 3380 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) && compareDeep(event, o.event, true) 3381 && compareDeep(section, o.section, true); 3382 } 3383 3384 @Override 3385 public boolean equalsShallow(Base other_) { 3386 if (!super.equalsShallow(other_)) 3387 return false; 3388 if (!(other_ instanceof Composition)) 3389 return false; 3390 Composition o = (Composition) other_; 3391 return compareValues(status, o.status, true) && compareValues(date, o.date, true) && compareValues(title, o.title, true) 3392 && compareValues(confidentiality, o.confidentiality, true); 3393 } 3394 3395 public boolean isEmpty() { 3396 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 3397 , class_, subject, encounter, date, author, title, confidentiality, attester 3398 , custodian, relatesTo, event, section); 3399 } 3400 3401 @Override 3402 public ResourceType getResourceType() { 3403 return ResourceType.Composition; 3404 } 3405 3406 /** 3407 * Search parameter: <b>date</b> 3408 * <p> 3409 * Description: <b>Composition editing time</b><br> 3410 * Type: <b>date</b><br> 3411 * Path: <b>Composition.date</b><br> 3412 * </p> 3413 */ 3414 @SearchParamDefinition(name="date", path="Composition.date", description="Composition editing time", type="date" ) 3415 public static final String SP_DATE = "date"; 3416 /** 3417 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3418 * <p> 3419 * Description: <b>Composition editing time</b><br> 3420 * Type: <b>date</b><br> 3421 * Path: <b>Composition.date</b><br> 3422 * </p> 3423 */ 3424 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3425 3426 /** 3427 * Search parameter: <b>identifier</b> 3428 * <p> 3429 * Description: <b>Logical identifier of composition (version-independent)</b><br> 3430 * Type: <b>token</b><br> 3431 * Path: <b>Composition.identifier</b><br> 3432 * </p> 3433 */ 3434 @SearchParamDefinition(name="identifier", path="Composition.identifier", description="Logical identifier of composition (version-independent)", type="token" ) 3435 public static final String SP_IDENTIFIER = "identifier"; 3436 /** 3437 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3438 * <p> 3439 * Description: <b>Logical identifier of composition (version-independent)</b><br> 3440 * Type: <b>token</b><br> 3441 * Path: <b>Composition.identifier</b><br> 3442 * </p> 3443 */ 3444 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3445 3446 /** 3447 * Search parameter: <b>period</b> 3448 * <p> 3449 * Description: <b>The period covered by the documentation</b><br> 3450 * Type: <b>date</b><br> 3451 * Path: <b>Composition.event.period</b><br> 3452 * </p> 3453 */ 3454 @SearchParamDefinition(name="period", path="Composition.event.period", description="The period covered by the documentation", type="date" ) 3455 public static final String SP_PERIOD = "period"; 3456 /** 3457 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3458 * <p> 3459 * Description: <b>The period covered by the documentation</b><br> 3460 * Type: <b>date</b><br> 3461 * Path: <b>Composition.event.period</b><br> 3462 * </p> 3463 */ 3464 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 3465 3466 /** 3467 * Search parameter: <b>related-id</b> 3468 * <p> 3469 * Description: <b>Target of the relationship</b><br> 3470 * Type: <b>token</b><br> 3471 * Path: <b>Composition.relatesTo.targetIdentifier</b><br> 3472 * </p> 3473 */ 3474 @SearchParamDefinition(name="related-id", path="Composition.relatesTo.target.as(Identifier)", description="Target of the relationship", type="token" ) 3475 public static final String SP_RELATED_ID = "related-id"; 3476 /** 3477 * <b>Fluent Client</b> search parameter constant for <b>related-id</b> 3478 * <p> 3479 * Description: <b>Target of the relationship</b><br> 3480 * Type: <b>token</b><br> 3481 * Path: <b>Composition.relatesTo.targetIdentifier</b><br> 3482 * </p> 3483 */ 3484 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATED_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATED_ID); 3485 3486 /** 3487 * Search parameter: <b>subject</b> 3488 * <p> 3489 * Description: <b>Who and/or what the composition is about</b><br> 3490 * Type: <b>reference</b><br> 3491 * Path: <b>Composition.subject</b><br> 3492 * </p> 3493 */ 3494 @SearchParamDefinition(name="subject", path="Composition.subject", description="Who and/or what the composition is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") } ) 3495 public static final String SP_SUBJECT = "subject"; 3496 /** 3497 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3498 * <p> 3499 * Description: <b>Who and/or what the composition is about</b><br> 3500 * Type: <b>reference</b><br> 3501 * Path: <b>Composition.subject</b><br> 3502 * </p> 3503 */ 3504 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3505 3506/** 3507 * Constant for fluent queries to be used to add include statements. Specifies 3508 * the path value of "<b>Composition:subject</b>". 3509 */ 3510 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Composition:subject").toLocked(); 3511 3512 /** 3513 * Search parameter: <b>author</b> 3514 * <p> 3515 * Description: <b>Who and/or what authored the composition</b><br> 3516 * Type: <b>reference</b><br> 3517 * Path: <b>Composition.author</b><br> 3518 * </p> 3519 */ 3520 @SearchParamDefinition(name="author", path="Composition.author", description="Who and/or what authored the composition", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3521 public static final String SP_AUTHOR = "author"; 3522 /** 3523 * <b>Fluent Client</b> search parameter constant for <b>author</b> 3524 * <p> 3525 * Description: <b>Who and/or what authored the composition</b><br> 3526 * Type: <b>reference</b><br> 3527 * Path: <b>Composition.author</b><br> 3528 * </p> 3529 */ 3530 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 3531 3532/** 3533 * Constant for fluent queries to be used to add include statements. Specifies 3534 * the path value of "<b>Composition:author</b>". 3535 */ 3536 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Composition:author").toLocked(); 3537 3538 /** 3539 * Search parameter: <b>confidentiality</b> 3540 * <p> 3541 * Description: <b>As defined by affinity domain</b><br> 3542 * Type: <b>token</b><br> 3543 * Path: <b>Composition.confidentiality</b><br> 3544 * </p> 3545 */ 3546 @SearchParamDefinition(name="confidentiality", path="Composition.confidentiality", description="As defined by affinity domain", type="token" ) 3547 public static final String SP_CONFIDENTIALITY = "confidentiality"; 3548 /** 3549 * <b>Fluent Client</b> search parameter constant for <b>confidentiality</b> 3550 * <p> 3551 * Description: <b>As defined by affinity domain</b><br> 3552 * Type: <b>token</b><br> 3553 * Path: <b>Composition.confidentiality</b><br> 3554 * </p> 3555 */ 3556 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONFIDENTIALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONFIDENTIALITY); 3557 3558 /** 3559 * Search parameter: <b>section</b> 3560 * <p> 3561 * Description: <b>Classification of section (recommended)</b><br> 3562 * Type: <b>token</b><br> 3563 * Path: <b>Composition.section.code</b><br> 3564 * </p> 3565 */ 3566 @SearchParamDefinition(name="section", path="Composition.section.code", description="Classification of section (recommended)", type="token" ) 3567 public static final String SP_SECTION = "section"; 3568 /** 3569 * <b>Fluent Client</b> search parameter constant for <b>section</b> 3570 * <p> 3571 * Description: <b>Classification of section (recommended)</b><br> 3572 * Type: <b>token</b><br> 3573 * Path: <b>Composition.section.code</b><br> 3574 * </p> 3575 */ 3576 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECTION); 3577 3578 /** 3579 * Search parameter: <b>encounter</b> 3580 * <p> 3581 * Description: <b>Context of the Composition</b><br> 3582 * Type: <b>reference</b><br> 3583 * Path: <b>Composition.encounter</b><br> 3584 * </p> 3585 */ 3586 @SearchParamDefinition(name="encounter", path="Composition.encounter", description="Context of the Composition", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 3587 public static final String SP_ENCOUNTER = "encounter"; 3588 /** 3589 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3590 * <p> 3591 * Description: <b>Context of the Composition</b><br> 3592 * Type: <b>reference</b><br> 3593 * Path: <b>Composition.encounter</b><br> 3594 * </p> 3595 */ 3596 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3597 3598/** 3599 * Constant for fluent queries to be used to add include statements. Specifies 3600 * the path value of "<b>Composition:encounter</b>". 3601 */ 3602 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Composition:encounter").toLocked(); 3603 3604 /** 3605 * Search parameter: <b>type</b> 3606 * <p> 3607 * Description: <b>Kind of composition (LOINC if possible)</b><br> 3608 * Type: <b>token</b><br> 3609 * Path: <b>Composition.type</b><br> 3610 * </p> 3611 */ 3612 @SearchParamDefinition(name="type", path="Composition.type", description="Kind of composition (LOINC if possible)", type="token" ) 3613 public static final String SP_TYPE = "type"; 3614 /** 3615 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3616 * <p> 3617 * Description: <b>Kind of composition (LOINC if possible)</b><br> 3618 * Type: <b>token</b><br> 3619 * Path: <b>Composition.type</b><br> 3620 * </p> 3621 */ 3622 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3623 3624 /** 3625 * Search parameter: <b>title</b> 3626 * <p> 3627 * Description: <b>Human Readable name/title</b><br> 3628 * Type: <b>string</b><br> 3629 * Path: <b>Composition.title</b><br> 3630 * </p> 3631 */ 3632 @SearchParamDefinition(name="title", path="Composition.title", description="Human Readable name/title", type="string" ) 3633 public static final String SP_TITLE = "title"; 3634 /** 3635 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3636 * <p> 3637 * Description: <b>Human Readable name/title</b><br> 3638 * Type: <b>string</b><br> 3639 * Path: <b>Composition.title</b><br> 3640 * </p> 3641 */ 3642 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3643 3644 /** 3645 * Search parameter: <b>attester</b> 3646 * <p> 3647 * Description: <b>Who attested the composition</b><br> 3648 * Type: <b>reference</b><br> 3649 * Path: <b>Composition.attester.party</b><br> 3650 * </p> 3651 */ 3652 @SearchParamDefinition(name="attester", path="Composition.attester.party", description="Who attested the composition", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Patient.class, Practitioner.class } ) 3653 public static final String SP_ATTESTER = "attester"; 3654 /** 3655 * <b>Fluent Client</b> search parameter constant for <b>attester</b> 3656 * <p> 3657 * Description: <b>Who attested the composition</b><br> 3658 * Type: <b>reference</b><br> 3659 * Path: <b>Composition.attester.party</b><br> 3660 * </p> 3661 */ 3662 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ATTESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ATTESTER); 3663 3664/** 3665 * Constant for fluent queries to be used to add include statements. Specifies 3666 * the path value of "<b>Composition:attester</b>". 3667 */ 3668 public static final ca.uhn.fhir.model.api.Include INCLUDE_ATTESTER = new ca.uhn.fhir.model.api.Include("Composition:attester").toLocked(); 3669 3670 /** 3671 * Search parameter: <b>entry</b> 3672 * <p> 3673 * Description: <b>A reference to data that supports this section</b><br> 3674 * Type: <b>reference</b><br> 3675 * Path: <b>Composition.section.entry</b><br> 3676 * </p> 3677 */ 3678 @SearchParamDefinition(name="entry", path="Composition.section.entry", description="A reference to data that supports this section", type="reference" ) 3679 public static final String SP_ENTRY = "entry"; 3680 /** 3681 * <b>Fluent Client</b> search parameter constant for <b>entry</b> 3682 * <p> 3683 * Description: <b>A reference to data that supports this section</b><br> 3684 * Type: <b>reference</b><br> 3685 * Path: <b>Composition.section.entry</b><br> 3686 * </p> 3687 */ 3688 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTRY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTRY); 3689 3690/** 3691 * Constant for fluent queries to be used to add include statements. Specifies 3692 * the path value of "<b>Composition:entry</b>". 3693 */ 3694 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTRY = new ca.uhn.fhir.model.api.Include("Composition:entry").toLocked(); 3695 3696 /** 3697 * Search parameter: <b>related-ref</b> 3698 * <p> 3699 * Description: <b>Target of the relationship</b><br> 3700 * Type: <b>reference</b><br> 3701 * Path: <b>Composition.relatesTo.targetReference</b><br> 3702 * </p> 3703 */ 3704 @SearchParamDefinition(name="related-ref", path="Composition.relatesTo.target.as(Reference)", description="Target of the relationship", type="reference", target={Composition.class } ) 3705 public static final String SP_RELATED_REF = "related-ref"; 3706 /** 3707 * <b>Fluent Client</b> search parameter constant for <b>related-ref</b> 3708 * <p> 3709 * Description: <b>Target of the relationship</b><br> 3710 * Type: <b>reference</b><br> 3711 * Path: <b>Composition.relatesTo.targetReference</b><br> 3712 * </p> 3713 */ 3714 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATED_REF); 3715 3716/** 3717 * Constant for fluent queries to be used to add include statements. Specifies 3718 * the path value of "<b>Composition:related-ref</b>". 3719 */ 3720 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED_REF = new ca.uhn.fhir.model.api.Include("Composition:related-ref").toLocked(); 3721 3722 /** 3723 * Search parameter: <b>patient</b> 3724 * <p> 3725 * Description: <b>Who and/or what the composition is about</b><br> 3726 * Type: <b>reference</b><br> 3727 * Path: <b>Composition.subject</b><br> 3728 * </p> 3729 */ 3730 @SearchParamDefinition(name="patient", path="Composition.subject", description="Who and/or what the composition is about", type="reference", target={Patient.class } ) 3731 public static final String SP_PATIENT = "patient"; 3732 /** 3733 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3734 * <p> 3735 * Description: <b>Who and/or what the composition is about</b><br> 3736 * Type: <b>reference</b><br> 3737 * Path: <b>Composition.subject</b><br> 3738 * </p> 3739 */ 3740 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3741 3742/** 3743 * Constant for fluent queries to be used to add include statements. Specifies 3744 * the path value of "<b>Composition:patient</b>". 3745 */ 3746 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Composition:patient").toLocked(); 3747 3748 /** 3749 * Search parameter: <b>context</b> 3750 * <p> 3751 * Description: <b>Code(s) that apply to the event being documented</b><br> 3752 * Type: <b>token</b><br> 3753 * Path: <b>Composition.event.code</b><br> 3754 * </p> 3755 */ 3756 @SearchParamDefinition(name="context", path="Composition.event.code", description="Code(s) that apply to the event being documented", type="token" ) 3757 public static final String SP_CONTEXT = "context"; 3758 /** 3759 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3760 * <p> 3761 * Description: <b>Code(s) that apply to the event being documented</b><br> 3762 * Type: <b>token</b><br> 3763 * Path: <b>Composition.event.code</b><br> 3764 * </p> 3765 */ 3766 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3767 3768 /** 3769 * Search parameter: <b>class</b> 3770 * <p> 3771 * Description: <b>Categorization of Composition</b><br> 3772 * Type: <b>token</b><br> 3773 * Path: <b>Composition.class</b><br> 3774 * </p> 3775 */ 3776 @SearchParamDefinition(name="class", path="Composition.class", description="Categorization of Composition", type="token" ) 3777 public static final String SP_CLASS = "class"; 3778 /** 3779 * <b>Fluent Client</b> search parameter constant for <b>class</b> 3780 * <p> 3781 * Description: <b>Categorization of Composition</b><br> 3782 * Type: <b>token</b><br> 3783 * Path: <b>Composition.class</b><br> 3784 * </p> 3785 */ 3786 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASS); 3787 3788 /** 3789 * Search parameter: <b>status</b> 3790 * <p> 3791 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 3792 * Type: <b>token</b><br> 3793 * Path: <b>Composition.status</b><br> 3794 * </p> 3795 */ 3796 @SearchParamDefinition(name="status", path="Composition.status", description="preliminary | final | amended | entered-in-error", type="token" ) 3797 public static final String SP_STATUS = "status"; 3798 /** 3799 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3800 * <p> 3801 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 3802 * Type: <b>token</b><br> 3803 * Path: <b>Composition.status</b><br> 3804 * </p> 3805 */ 3806 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3807 3808 3809}