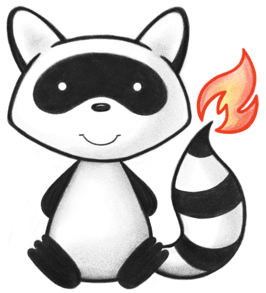
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence; 041import org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalenceEnumFactory; 042import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 043import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 044import org.hl7.fhir.exceptions.FHIRException; 045import org.hl7.fhir.exceptions.FHIRFormatError; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.utilities.Utilities; 048 049import ca.uhn.fhir.model.api.annotation.Block; 050import ca.uhn.fhir.model.api.annotation.Child; 051import ca.uhn.fhir.model.api.annotation.ChildOrder; 052import ca.uhn.fhir.model.api.annotation.Description; 053import ca.uhn.fhir.model.api.annotation.ResourceDef; 054import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 055/** 056 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 057 */ 058@ResourceDef(name="ConceptMap", profile="http://hl7.org/fhir/Profile/ConceptMap") 059@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "source[x]", "target[x]", "group"}) 060public class ConceptMap extends MetadataResource { 061 062 public enum ConceptMapGroupUnmappedMode { 063 /** 064 * Use the code as provided in the $translate request 065 */ 066 PROVIDED, 067 /** 068 * Use the code explicitly provided in the group.unmapped 069 */ 070 FIXED, 071 /** 072 * Use the map identified by the canonical URL in URL 073 */ 074 OTHERMAP, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static ConceptMapGroupUnmappedMode fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("provided".equals(codeString)) 083 return PROVIDED; 084 if ("fixed".equals(codeString)) 085 return FIXED; 086 if ("other-map".equals(codeString)) 087 return OTHERMAP; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case PROVIDED: return "provided"; 096 case FIXED: return "fixed"; 097 case OTHERMAP: return "other-map"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case PROVIDED: return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 105 case FIXED: return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 106 case OTHERMAP: return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case PROVIDED: return "Use the code as provided in the $translate request"; 114 case FIXED: return "Use the code explicitly provided in the group.unmapped"; 115 case OTHERMAP: return "Use the map identified by the canonical URL in URL"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDisplay() { 121 switch (this) { 122 case PROVIDED: return "Provided Code"; 123 case FIXED: return "Fixed Code"; 124 case OTHERMAP: return "Other Map"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 } 130 131 public static class ConceptMapGroupUnmappedModeEnumFactory implements EnumFactory<ConceptMapGroupUnmappedMode> { 132 public ConceptMapGroupUnmappedMode fromCode(String codeString) throws IllegalArgumentException { 133 if (codeString == null || "".equals(codeString)) 134 if (codeString == null || "".equals(codeString)) 135 return null; 136 if ("provided".equals(codeString)) 137 return ConceptMapGroupUnmappedMode.PROVIDED; 138 if ("fixed".equals(codeString)) 139 return ConceptMapGroupUnmappedMode.FIXED; 140 if ("other-map".equals(codeString)) 141 return ConceptMapGroupUnmappedMode.OTHERMAP; 142 throw new IllegalArgumentException("Unknown ConceptMapGroupUnmappedMode code '"+codeString+"'"); 143 } 144 public Enumeration<ConceptMapGroupUnmappedMode> fromType(PrimitiveType<?> code) throws FHIRException { 145 if (code == null) 146 return null; 147 if (code.isEmpty()) 148 return new Enumeration<ConceptMapGroupUnmappedMode>(this); 149 String codeString = code.asStringValue(); 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("provided".equals(codeString)) 153 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.PROVIDED); 154 if ("fixed".equals(codeString)) 155 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.FIXED); 156 if ("other-map".equals(codeString)) 157 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.OTHERMAP); 158 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '"+codeString+"'"); 159 } 160 public String toCode(ConceptMapGroupUnmappedMode code) { 161 if (code == ConceptMapGroupUnmappedMode.NULL) 162 return null; 163 if (code == ConceptMapGroupUnmappedMode.PROVIDED) 164 return "provided"; 165 if (code == ConceptMapGroupUnmappedMode.FIXED) 166 return "fixed"; 167 if (code == ConceptMapGroupUnmappedMode.OTHERMAP) 168 return "other-map"; 169 return "?"; 170 } 171 public String toSystem(ConceptMapGroupUnmappedMode code) { 172 return code.getSystem(); 173 } 174 } 175 176 @Block() 177 public static class ConceptMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 178 /** 179 * An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system). 180 */ 181 @Child(name = "source", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 182 @Description(shortDefinition="Code System (if value set crosses code systems)", formalDefinition="An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system)." ) 183 protected UriType source; 184 185 /** 186 * The specific version of the code system, as determined by the code system authority. 187 */ 188 @Child(name = "sourceVersion", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 189 @Description(shortDefinition="Specific version of the code system", formalDefinition="The specific version of the code system, as determined by the code system authority." ) 190 protected StringType sourceVersion; 191 192 /** 193 * An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems). 194 */ 195 @Child(name = "target", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=false) 196 @Description(shortDefinition="System of the target (if necessary)", formalDefinition="An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems)." ) 197 protected UriType target; 198 199 /** 200 * The specific version of the code system, as determined by the code system authority. 201 */ 202 @Child(name = "targetVersion", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 203 @Description(shortDefinition="Specific version of the code system", formalDefinition="The specific version of the code system, as determined by the code system authority." ) 204 protected StringType targetVersion; 205 206 /** 207 * Mappings for an individual concept in the source to one or more concepts in the target. 208 */ 209 @Child(name = "element", type = {}, order=5, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 210 @Description(shortDefinition="Mappings for a concept from the source set", formalDefinition="Mappings for an individual concept in the source to one or more concepts in the target." ) 211 protected List<SourceElementComponent> element; 212 213 /** 214 * What to do when there is no match in the mappings in the group. 215 */ 216 @Child(name = "unmapped", type = {}, order=6, min=0, max=1, modifier=false, summary=false) 217 @Description(shortDefinition="When no match in the mappings", formalDefinition="What to do when there is no match in the mappings in the group." ) 218 protected ConceptMapGroupUnmappedComponent unmapped; 219 220 private static final long serialVersionUID = 1606357508L; 221 222 /** 223 * Constructor 224 */ 225 public ConceptMapGroupComponent() { 226 super(); 227 } 228 229 /** 230 * @return {@link #source} (An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system).). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 231 */ 232 public UriType getSourceElement() { 233 if (this.source == null) 234 if (Configuration.errorOnAutoCreate()) 235 throw new Error("Attempt to auto-create ConceptMapGroupComponent.source"); 236 else if (Configuration.doAutoCreate()) 237 this.source = new UriType(); // bb 238 return this.source; 239 } 240 241 public boolean hasSourceElement() { 242 return this.source != null && !this.source.isEmpty(); 243 } 244 245 public boolean hasSource() { 246 return this.source != null && !this.source.isEmpty(); 247 } 248 249 /** 250 * @param value {@link #source} (An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system).). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 251 */ 252 public ConceptMapGroupComponent setSourceElement(UriType value) { 253 this.source = value; 254 return this; 255 } 256 257 /** 258 * @return An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system). 259 */ 260 public String getSource() { 261 return this.source == null ? null : this.source.getValue(); 262 } 263 264 /** 265 * @param value An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system). 266 */ 267 public ConceptMapGroupComponent setSource(String value) { 268 if (Utilities.noString(value)) 269 this.source = null; 270 else { 271 if (this.source == null) 272 this.source = new UriType(); 273 this.source.setValue(value); 274 } 275 return this; 276 } 277 278 /** 279 * @return {@link #sourceVersion} (The specific version of the code system, as determined by the code system authority.). This is the underlying object with id, value and extensions. The accessor "getSourceVersion" gives direct access to the value 280 */ 281 public StringType getSourceVersionElement() { 282 if (this.sourceVersion == null) 283 if (Configuration.errorOnAutoCreate()) 284 throw new Error("Attempt to auto-create ConceptMapGroupComponent.sourceVersion"); 285 else if (Configuration.doAutoCreate()) 286 this.sourceVersion = new StringType(); // bb 287 return this.sourceVersion; 288 } 289 290 public boolean hasSourceVersionElement() { 291 return this.sourceVersion != null && !this.sourceVersion.isEmpty(); 292 } 293 294 public boolean hasSourceVersion() { 295 return this.sourceVersion != null && !this.sourceVersion.isEmpty(); 296 } 297 298 /** 299 * @param value {@link #sourceVersion} (The specific version of the code system, as determined by the code system authority.). This is the underlying object with id, value and extensions. The accessor "getSourceVersion" gives direct access to the value 300 */ 301 public ConceptMapGroupComponent setSourceVersionElement(StringType value) { 302 this.sourceVersion = value; 303 return this; 304 } 305 306 /** 307 * @return The specific version of the code system, as determined by the code system authority. 308 */ 309 public String getSourceVersion() { 310 return this.sourceVersion == null ? null : this.sourceVersion.getValue(); 311 } 312 313 /** 314 * @param value The specific version of the code system, as determined by the code system authority. 315 */ 316 public ConceptMapGroupComponent setSourceVersion(String value) { 317 if (Utilities.noString(value)) 318 this.sourceVersion = null; 319 else { 320 if (this.sourceVersion == null) 321 this.sourceVersion = new StringType(); 322 this.sourceVersion.setValue(value); 323 } 324 return this; 325 } 326 327 /** 328 * @return {@link #target} (An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems).). This is the underlying object with id, value and extensions. The accessor "getTarget" gives direct access to the value 329 */ 330 public UriType getTargetElement() { 331 if (this.target == null) 332 if (Configuration.errorOnAutoCreate()) 333 throw new Error("Attempt to auto-create ConceptMapGroupComponent.target"); 334 else if (Configuration.doAutoCreate()) 335 this.target = new UriType(); // bb 336 return this.target; 337 } 338 339 public boolean hasTargetElement() { 340 return this.target != null && !this.target.isEmpty(); 341 } 342 343 public boolean hasTarget() { 344 return this.target != null && !this.target.isEmpty(); 345 } 346 347 /** 348 * @param value {@link #target} (An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems).). This is the underlying object with id, value and extensions. The accessor "getTarget" gives direct access to the value 349 */ 350 public ConceptMapGroupComponent setTargetElement(UriType value) { 351 this.target = value; 352 return this; 353 } 354 355 /** 356 * @return An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems). 357 */ 358 public String getTarget() { 359 return this.target == null ? null : this.target.getValue(); 360 } 361 362 /** 363 * @param value An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems). 364 */ 365 public ConceptMapGroupComponent setTarget(String value) { 366 if (Utilities.noString(value)) 367 this.target = null; 368 else { 369 if (this.target == null) 370 this.target = new UriType(); 371 this.target.setValue(value); 372 } 373 return this; 374 } 375 376 /** 377 * @return {@link #targetVersion} (The specific version of the code system, as determined by the code system authority.). This is the underlying object with id, value and extensions. The accessor "getTargetVersion" gives direct access to the value 378 */ 379 public StringType getTargetVersionElement() { 380 if (this.targetVersion == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create ConceptMapGroupComponent.targetVersion"); 383 else if (Configuration.doAutoCreate()) 384 this.targetVersion = new StringType(); // bb 385 return this.targetVersion; 386 } 387 388 public boolean hasTargetVersionElement() { 389 return this.targetVersion != null && !this.targetVersion.isEmpty(); 390 } 391 392 public boolean hasTargetVersion() { 393 return this.targetVersion != null && !this.targetVersion.isEmpty(); 394 } 395 396 /** 397 * @param value {@link #targetVersion} (The specific version of the code system, as determined by the code system authority.). This is the underlying object with id, value and extensions. The accessor "getTargetVersion" gives direct access to the value 398 */ 399 public ConceptMapGroupComponent setTargetVersionElement(StringType value) { 400 this.targetVersion = value; 401 return this; 402 } 403 404 /** 405 * @return The specific version of the code system, as determined by the code system authority. 406 */ 407 public String getTargetVersion() { 408 return this.targetVersion == null ? null : this.targetVersion.getValue(); 409 } 410 411 /** 412 * @param value The specific version of the code system, as determined by the code system authority. 413 */ 414 public ConceptMapGroupComponent setTargetVersion(String value) { 415 if (Utilities.noString(value)) 416 this.targetVersion = null; 417 else { 418 if (this.targetVersion == null) 419 this.targetVersion = new StringType(); 420 this.targetVersion.setValue(value); 421 } 422 return this; 423 } 424 425 /** 426 * @return {@link #element} (Mappings for an individual concept in the source to one or more concepts in the target.) 427 */ 428 public List<SourceElementComponent> getElement() { 429 if (this.element == null) 430 this.element = new ArrayList<SourceElementComponent>(); 431 return this.element; 432 } 433 434 /** 435 * @return Returns a reference to <code>this</code> for easy method chaining 436 */ 437 public ConceptMapGroupComponent setElement(List<SourceElementComponent> theElement) { 438 this.element = theElement; 439 return this; 440 } 441 442 public boolean hasElement() { 443 if (this.element == null) 444 return false; 445 for (SourceElementComponent item : this.element) 446 if (!item.isEmpty()) 447 return true; 448 return false; 449 } 450 451 public SourceElementComponent addElement() { //3 452 SourceElementComponent t = new SourceElementComponent(); 453 if (this.element == null) 454 this.element = new ArrayList<SourceElementComponent>(); 455 this.element.add(t); 456 return t; 457 } 458 459 public ConceptMapGroupComponent addElement(SourceElementComponent t) { //3 460 if (t == null) 461 return this; 462 if (this.element == null) 463 this.element = new ArrayList<SourceElementComponent>(); 464 this.element.add(t); 465 return this; 466 } 467 468 /** 469 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist 470 */ 471 public SourceElementComponent getElementFirstRep() { 472 if (getElement().isEmpty()) { 473 addElement(); 474 } 475 return getElement().get(0); 476 } 477 478 /** 479 * @return {@link #unmapped} (What to do when there is no match in the mappings in the group.) 480 */ 481 public ConceptMapGroupUnmappedComponent getUnmapped() { 482 if (this.unmapped == null) 483 if (Configuration.errorOnAutoCreate()) 484 throw new Error("Attempt to auto-create ConceptMapGroupComponent.unmapped"); 485 else if (Configuration.doAutoCreate()) 486 this.unmapped = new ConceptMapGroupUnmappedComponent(); // cc 487 return this.unmapped; 488 } 489 490 public boolean hasUnmapped() { 491 return this.unmapped != null && !this.unmapped.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #unmapped} (What to do when there is no match in the mappings in the group.) 496 */ 497 public ConceptMapGroupComponent setUnmapped(ConceptMapGroupUnmappedComponent value) { 498 this.unmapped = value; 499 return this; 500 } 501 502 protected void listChildren(List<Property> children) { 503 super.listChildren(children); 504 children.add(new Property("source", "uri", "An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system).", 0, 1, source)); 505 children.add(new Property("sourceVersion", "string", "The specific version of the code system, as determined by the code system authority.", 0, 1, sourceVersion)); 506 children.add(new Property("target", "uri", "An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems).", 0, 1, target)); 507 children.add(new Property("targetVersion", "string", "The specific version of the code system, as determined by the code system authority.", 0, 1, targetVersion)); 508 children.add(new Property("element", "", "Mappings for an individual concept in the source to one or more concepts in the target.", 0, java.lang.Integer.MAX_VALUE, element)); 509 children.add(new Property("unmapped", "", "What to do when there is no match in the mappings in the group.", 0, 1, unmapped)); 510 } 511 512 @Override 513 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 514 switch (_hash) { 515 case -896505829: /*source*/ return new Property("source", "uri", "An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system).", 0, 1, source); 516 case 446171197: /*sourceVersion*/ return new Property("sourceVersion", "string", "The specific version of the code system, as determined by the code system authority.", 0, 1, sourceVersion); 517 case -880905839: /*target*/ return new Property("target", "uri", "An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems).", 0, 1, target); 518 case -1639412217: /*targetVersion*/ return new Property("targetVersion", "string", "The specific version of the code system, as determined by the code system authority.", 0, 1, targetVersion); 519 case -1662836996: /*element*/ return new Property("element", "", "Mappings for an individual concept in the source to one or more concepts in the target.", 0, java.lang.Integer.MAX_VALUE, element); 520 case -194857460: /*unmapped*/ return new Property("unmapped", "", "What to do when there is no match in the mappings in the group.", 0, 1, unmapped); 521 default: return super.getNamedProperty(_hash, _name, _checkValid); 522 } 523 524 } 525 526 @Override 527 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 528 switch (hash) { 529 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // UriType 530 case 446171197: /*sourceVersion*/ return this.sourceVersion == null ? new Base[0] : new Base[] {this.sourceVersion}; // StringType 531 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // UriType 532 case -1639412217: /*targetVersion*/ return this.targetVersion == null ? new Base[0] : new Base[] {this.targetVersion}; // StringType 533 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // SourceElementComponent 534 case -194857460: /*unmapped*/ return this.unmapped == null ? new Base[0] : new Base[] {this.unmapped}; // ConceptMapGroupUnmappedComponent 535 default: return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case -896505829: // source 544 this.source = castToUri(value); // UriType 545 return value; 546 case 446171197: // sourceVersion 547 this.sourceVersion = castToString(value); // StringType 548 return value; 549 case -880905839: // target 550 this.target = castToUri(value); // UriType 551 return value; 552 case -1639412217: // targetVersion 553 this.targetVersion = castToString(value); // StringType 554 return value; 555 case -1662836996: // element 556 this.getElement().add((SourceElementComponent) value); // SourceElementComponent 557 return value; 558 case -194857460: // unmapped 559 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 560 return value; 561 default: return super.setProperty(hash, name, value); 562 } 563 564 } 565 566 @Override 567 public Base setProperty(String name, Base value) throws FHIRException { 568 if (name.equals("source")) { 569 this.source = castToUri(value); // UriType 570 } else if (name.equals("sourceVersion")) { 571 this.sourceVersion = castToString(value); // StringType 572 } else if (name.equals("target")) { 573 this.target = castToUri(value); // UriType 574 } else if (name.equals("targetVersion")) { 575 this.targetVersion = castToString(value); // StringType 576 } else if (name.equals("element")) { 577 this.getElement().add((SourceElementComponent) value); 578 } else if (name.equals("unmapped")) { 579 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 580 } else 581 return super.setProperty(name, value); 582 return value; 583 } 584 585 @Override 586 public Base makeProperty(int hash, String name) throws FHIRException { 587 switch (hash) { 588 case -896505829: return getSourceElement(); 589 case 446171197: return getSourceVersionElement(); 590 case -880905839: return getTargetElement(); 591 case -1639412217: return getTargetVersionElement(); 592 case -1662836996: return addElement(); 593 case -194857460: return getUnmapped(); 594 default: return super.makeProperty(hash, name); 595 } 596 597 } 598 599 @Override 600 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 601 switch (hash) { 602 case -896505829: /*source*/ return new String[] {"uri"}; 603 case 446171197: /*sourceVersion*/ return new String[] {"string"}; 604 case -880905839: /*target*/ return new String[] {"uri"}; 605 case -1639412217: /*targetVersion*/ return new String[] {"string"}; 606 case -1662836996: /*element*/ return new String[] {}; 607 case -194857460: /*unmapped*/ return new String[] {}; 608 default: return super.getTypesForProperty(hash, name); 609 } 610 611 } 612 613 @Override 614 public Base addChild(String name) throws FHIRException { 615 if (name.equals("source")) { 616 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.source"); 617 } 618 else if (name.equals("sourceVersion")) { 619 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.sourceVersion"); 620 } 621 else if (name.equals("target")) { 622 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.target"); 623 } 624 else if (name.equals("targetVersion")) { 625 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.targetVersion"); 626 } 627 else if (name.equals("element")) { 628 return addElement(); 629 } 630 else if (name.equals("unmapped")) { 631 this.unmapped = new ConceptMapGroupUnmappedComponent(); 632 return this.unmapped; 633 } 634 else 635 return super.addChild(name); 636 } 637 638 public ConceptMapGroupComponent copy() { 639 ConceptMapGroupComponent dst = new ConceptMapGroupComponent(); 640 copyValues(dst); 641 dst.source = source == null ? null : source.copy(); 642 dst.sourceVersion = sourceVersion == null ? null : sourceVersion.copy(); 643 dst.target = target == null ? null : target.copy(); 644 dst.targetVersion = targetVersion == null ? null : targetVersion.copy(); 645 if (element != null) { 646 dst.element = new ArrayList<SourceElementComponent>(); 647 for (SourceElementComponent i : element) 648 dst.element.add(i.copy()); 649 }; 650 dst.unmapped = unmapped == null ? null : unmapped.copy(); 651 return dst; 652 } 653 654 @Override 655 public boolean equalsDeep(Base other_) { 656 if (!super.equalsDeep(other_)) 657 return false; 658 if (!(other_ instanceof ConceptMapGroupComponent)) 659 return false; 660 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 661 return compareDeep(source, o.source, true) && compareDeep(sourceVersion, o.sourceVersion, true) 662 && compareDeep(target, o.target, true) && compareDeep(targetVersion, o.targetVersion, true) && compareDeep(element, o.element, true) 663 && compareDeep(unmapped, o.unmapped, true); 664 } 665 666 @Override 667 public boolean equalsShallow(Base other_) { 668 if (!super.equalsShallow(other_)) 669 return false; 670 if (!(other_ instanceof ConceptMapGroupComponent)) 671 return false; 672 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 673 return compareValues(source, o.source, true) && compareValues(sourceVersion, o.sourceVersion, true) 674 && compareValues(target, o.target, true) && compareValues(targetVersion, o.targetVersion, true); 675 } 676 677 public boolean isEmpty() { 678 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(source, sourceVersion, target 679 , targetVersion, element, unmapped); 680 } 681 682 public String fhirType() { 683 return "ConceptMap.group"; 684 685 } 686 687 } 688 689 @Block() 690 public static class SourceElementComponent extends BackboneElement implements IBaseBackboneElement { 691 /** 692 * Identity (code or path) or the element/item being mapped. 693 */ 694 @Child(name = "code", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 695 @Description(shortDefinition="Identifies element being mapped", formalDefinition="Identity (code or path) or the element/item being mapped." ) 696 protected CodeType code; 697 698 /** 699 * The display for the code. The display is only provided to help editors when editing the concept map. 700 */ 701 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 702 @Description(shortDefinition="Display for the code", formalDefinition="The display for the code. The display is only provided to help editors when editing the concept map." ) 703 protected StringType display; 704 705 /** 706 * A concept from the target value set that this concept maps to. 707 */ 708 @Child(name = "target", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 709 @Description(shortDefinition="Concept in target system for element", formalDefinition="A concept from the target value set that this concept maps to." ) 710 protected List<TargetElementComponent> target; 711 712 private static final long serialVersionUID = -1115258852L; 713 714 /** 715 * Constructor 716 */ 717 public SourceElementComponent() { 718 super(); 719 } 720 721 /** 722 * @return {@link #code} (Identity (code or path) or the element/item being mapped.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 723 */ 724 public CodeType getCodeElement() { 725 if (this.code == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create SourceElementComponent.code"); 728 else if (Configuration.doAutoCreate()) 729 this.code = new CodeType(); // bb 730 return this.code; 731 } 732 733 public boolean hasCodeElement() { 734 return this.code != null && !this.code.isEmpty(); 735 } 736 737 public boolean hasCode() { 738 return this.code != null && !this.code.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #code} (Identity (code or path) or the element/item being mapped.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 743 */ 744 public SourceElementComponent setCodeElement(CodeType value) { 745 this.code = value; 746 return this; 747 } 748 749 /** 750 * @return Identity (code or path) or the element/item being mapped. 751 */ 752 public String getCode() { 753 return this.code == null ? null : this.code.getValue(); 754 } 755 756 /** 757 * @param value Identity (code or path) or the element/item being mapped. 758 */ 759 public SourceElementComponent setCode(String value) { 760 if (Utilities.noString(value)) 761 this.code = null; 762 else { 763 if (this.code == null) 764 this.code = new CodeType(); 765 this.code.setValue(value); 766 } 767 return this; 768 } 769 770 /** 771 * @return {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 772 */ 773 public StringType getDisplayElement() { 774 if (this.display == null) 775 if (Configuration.errorOnAutoCreate()) 776 throw new Error("Attempt to auto-create SourceElementComponent.display"); 777 else if (Configuration.doAutoCreate()) 778 this.display = new StringType(); // bb 779 return this.display; 780 } 781 782 public boolean hasDisplayElement() { 783 return this.display != null && !this.display.isEmpty(); 784 } 785 786 public boolean hasDisplay() { 787 return this.display != null && !this.display.isEmpty(); 788 } 789 790 /** 791 * @param value {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 792 */ 793 public SourceElementComponent setDisplayElement(StringType value) { 794 this.display = value; 795 return this; 796 } 797 798 /** 799 * @return The display for the code. The display is only provided to help editors when editing the concept map. 800 */ 801 public String getDisplay() { 802 return this.display == null ? null : this.display.getValue(); 803 } 804 805 /** 806 * @param value The display for the code. The display is only provided to help editors when editing the concept map. 807 */ 808 public SourceElementComponent setDisplay(String value) { 809 if (Utilities.noString(value)) 810 this.display = null; 811 else { 812 if (this.display == null) 813 this.display = new StringType(); 814 this.display.setValue(value); 815 } 816 return this; 817 } 818 819 /** 820 * @return {@link #target} (A concept from the target value set that this concept maps to.) 821 */ 822 public List<TargetElementComponent> getTarget() { 823 if (this.target == null) 824 this.target = new ArrayList<TargetElementComponent>(); 825 return this.target; 826 } 827 828 /** 829 * @return Returns a reference to <code>this</code> for easy method chaining 830 */ 831 public SourceElementComponent setTarget(List<TargetElementComponent> theTarget) { 832 this.target = theTarget; 833 return this; 834 } 835 836 public boolean hasTarget() { 837 if (this.target == null) 838 return false; 839 for (TargetElementComponent item : this.target) 840 if (!item.isEmpty()) 841 return true; 842 return false; 843 } 844 845 public TargetElementComponent addTarget() { //3 846 TargetElementComponent t = new TargetElementComponent(); 847 if (this.target == null) 848 this.target = new ArrayList<TargetElementComponent>(); 849 this.target.add(t); 850 return t; 851 } 852 853 public SourceElementComponent addTarget(TargetElementComponent t) { //3 854 if (t == null) 855 return this; 856 if (this.target == null) 857 this.target = new ArrayList<TargetElementComponent>(); 858 this.target.add(t); 859 return this; 860 } 861 862 /** 863 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist 864 */ 865 public TargetElementComponent getTargetFirstRep() { 866 if (getTarget().isEmpty()) { 867 addTarget(); 868 } 869 return getTarget().get(0); 870 } 871 872 protected void listChildren(List<Property> children) { 873 super.listChildren(children); 874 children.add(new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1, code)); 875 children.add(new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display)); 876 children.add(new Property("target", "", "A concept from the target value set that this concept maps to.", 0, java.lang.Integer.MAX_VALUE, target)); 877 } 878 879 @Override 880 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 881 switch (_hash) { 882 case 3059181: /*code*/ return new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1, code); 883 case 1671764162: /*display*/ return new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display); 884 case -880905839: /*target*/ return new Property("target", "", "A concept from the target value set that this concept maps to.", 0, java.lang.Integer.MAX_VALUE, target); 885 default: return super.getNamedProperty(_hash, _name, _checkValid); 886 } 887 888 } 889 890 @Override 891 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 892 switch (hash) { 893 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 894 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 895 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // TargetElementComponent 896 default: return super.getProperty(hash, name, checkValid); 897 } 898 899 } 900 901 @Override 902 public Base setProperty(int hash, String name, Base value) throws FHIRException { 903 switch (hash) { 904 case 3059181: // code 905 this.code = castToCode(value); // CodeType 906 return value; 907 case 1671764162: // display 908 this.display = castToString(value); // StringType 909 return value; 910 case -880905839: // target 911 this.getTarget().add((TargetElementComponent) value); // TargetElementComponent 912 return value; 913 default: return super.setProperty(hash, name, value); 914 } 915 916 } 917 918 @Override 919 public Base setProperty(String name, Base value) throws FHIRException { 920 if (name.equals("code")) { 921 this.code = castToCode(value); // CodeType 922 } else if (name.equals("display")) { 923 this.display = castToString(value); // StringType 924 } else if (name.equals("target")) { 925 this.getTarget().add((TargetElementComponent) value); 926 } else 927 return super.setProperty(name, value); 928 return value; 929 } 930 931 @Override 932 public Base makeProperty(int hash, String name) throws FHIRException { 933 switch (hash) { 934 case 3059181: return getCodeElement(); 935 case 1671764162: return getDisplayElement(); 936 case -880905839: return addTarget(); 937 default: return super.makeProperty(hash, name); 938 } 939 940 } 941 942 @Override 943 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 944 switch (hash) { 945 case 3059181: /*code*/ return new String[] {"code"}; 946 case 1671764162: /*display*/ return new String[] {"string"}; 947 case -880905839: /*target*/ return new String[] {}; 948 default: return super.getTypesForProperty(hash, name); 949 } 950 951 } 952 953 @Override 954 public Base addChild(String name) throws FHIRException { 955 if (name.equals("code")) { 956 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 957 } 958 else if (name.equals("display")) { 959 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 960 } 961 else if (name.equals("target")) { 962 return addTarget(); 963 } 964 else 965 return super.addChild(name); 966 } 967 968 public SourceElementComponent copy() { 969 SourceElementComponent dst = new SourceElementComponent(); 970 copyValues(dst); 971 dst.code = code == null ? null : code.copy(); 972 dst.display = display == null ? null : display.copy(); 973 if (target != null) { 974 dst.target = new ArrayList<TargetElementComponent>(); 975 for (TargetElementComponent i : target) 976 dst.target.add(i.copy()); 977 }; 978 return dst; 979 } 980 981 @Override 982 public boolean equalsDeep(Base other_) { 983 if (!super.equalsDeep(other_)) 984 return false; 985 if (!(other_ instanceof SourceElementComponent)) 986 return false; 987 SourceElementComponent o = (SourceElementComponent) other_; 988 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(target, o.target, true) 989 ; 990 } 991 992 @Override 993 public boolean equalsShallow(Base other_) { 994 if (!super.equalsShallow(other_)) 995 return false; 996 if (!(other_ instanceof SourceElementComponent)) 997 return false; 998 SourceElementComponent o = (SourceElementComponent) other_; 999 return compareValues(code, o.code, true) && compareValues(display, o.display, true); 1000 } 1001 1002 public boolean isEmpty() { 1003 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, target); 1004 } 1005 1006 public String fhirType() { 1007 return "ConceptMap.group.element"; 1008 1009 } 1010 1011 } 1012 1013 @Block() 1014 public static class TargetElementComponent extends BackboneElement implements IBaseBackboneElement { 1015 /** 1016 * Identity (code or path) or the element/item that the map refers to. 1017 */ 1018 @Child(name = "code", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1019 @Description(shortDefinition="Code that identifies the target element", formalDefinition="Identity (code or path) or the element/item that the map refers to." ) 1020 protected CodeType code; 1021 1022 /** 1023 * The display for the code. The display is only provided to help editors when editing the concept map. 1024 */ 1025 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1026 @Description(shortDefinition="Display for the code", formalDefinition="The display for the code. The display is only provided to help editors when editing the concept map." ) 1027 protected StringType display; 1028 1029 /** 1030 * The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source). 1031 */ 1032 @Child(name = "equivalence", type = {CodeType.class}, order=3, min=0, max=1, modifier=true, summary=false) 1033 @Description(shortDefinition="relatedto | equivalent | equal | wider | subsumes | narrower | specializes | inexact | unmatched | disjoint", formalDefinition="The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source)." ) 1034 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/concept-map-equivalence") 1035 protected Enumeration<ConceptMapEquivalence> equivalence; 1036 1037 /** 1038 * A description of status/issues in mapping that conveys additional information not represented in the structured data. 1039 */ 1040 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1041 @Description(shortDefinition="Description of status/issues in mapping", formalDefinition="A description of status/issues in mapping that conveys additional information not represented in the structured data." ) 1042 protected StringType comment; 1043 1044 /** 1045 * A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value. 1046 */ 1047 @Child(name = "dependsOn", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1048 @Description(shortDefinition="Other elements required for this mapping (from context)", formalDefinition="A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value." ) 1049 protected List<OtherElementComponent> dependsOn; 1050 1051 /** 1052 * A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on. 1053 */ 1054 @Child(name = "product", type = {OtherElementComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1055 @Description(shortDefinition="Other concepts that this mapping also produces", formalDefinition="A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on." ) 1056 protected List<OtherElementComponent> product; 1057 1058 private static final long serialVersionUID = -2008997477L; 1059 1060 /** 1061 * Constructor 1062 */ 1063 public TargetElementComponent() { 1064 super(); 1065 } 1066 1067 /** 1068 * @return {@link #code} (Identity (code or path) or the element/item that the map refers to.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1069 */ 1070 public CodeType getCodeElement() { 1071 if (this.code == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error("Attempt to auto-create TargetElementComponent.code"); 1074 else if (Configuration.doAutoCreate()) 1075 this.code = new CodeType(); // bb 1076 return this.code; 1077 } 1078 1079 public boolean hasCodeElement() { 1080 return this.code != null && !this.code.isEmpty(); 1081 } 1082 1083 public boolean hasCode() { 1084 return this.code != null && !this.code.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #code} (Identity (code or path) or the element/item that the map refers to.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1089 */ 1090 public TargetElementComponent setCodeElement(CodeType value) { 1091 this.code = value; 1092 return this; 1093 } 1094 1095 /** 1096 * @return Identity (code or path) or the element/item that the map refers to. 1097 */ 1098 public String getCode() { 1099 return this.code == null ? null : this.code.getValue(); 1100 } 1101 1102 /** 1103 * @param value Identity (code or path) or the element/item that the map refers to. 1104 */ 1105 public TargetElementComponent setCode(String value) { 1106 if (Utilities.noString(value)) 1107 this.code = null; 1108 else { 1109 if (this.code == null) 1110 this.code = new CodeType(); 1111 this.code.setValue(value); 1112 } 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1118 */ 1119 public StringType getDisplayElement() { 1120 if (this.display == null) 1121 if (Configuration.errorOnAutoCreate()) 1122 throw new Error("Attempt to auto-create TargetElementComponent.display"); 1123 else if (Configuration.doAutoCreate()) 1124 this.display = new StringType(); // bb 1125 return this.display; 1126 } 1127 1128 public boolean hasDisplayElement() { 1129 return this.display != null && !this.display.isEmpty(); 1130 } 1131 1132 public boolean hasDisplay() { 1133 return this.display != null && !this.display.isEmpty(); 1134 } 1135 1136 /** 1137 * @param value {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1138 */ 1139 public TargetElementComponent setDisplayElement(StringType value) { 1140 this.display = value; 1141 return this; 1142 } 1143 1144 /** 1145 * @return The display for the code. The display is only provided to help editors when editing the concept map. 1146 */ 1147 public String getDisplay() { 1148 return this.display == null ? null : this.display.getValue(); 1149 } 1150 1151 /** 1152 * @param value The display for the code. The display is only provided to help editors when editing the concept map. 1153 */ 1154 public TargetElementComponent setDisplay(String value) { 1155 if (Utilities.noString(value)) 1156 this.display = null; 1157 else { 1158 if (this.display == null) 1159 this.display = new StringType(); 1160 this.display.setValue(value); 1161 } 1162 return this; 1163 } 1164 1165 /** 1166 * @return {@link #equivalence} (The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).). This is the underlying object with id, value and extensions. The accessor "getEquivalence" gives direct access to the value 1167 */ 1168 public Enumeration<ConceptMapEquivalence> getEquivalenceElement() { 1169 if (this.equivalence == null) 1170 if (Configuration.errorOnAutoCreate()) 1171 throw new Error("Attempt to auto-create TargetElementComponent.equivalence"); 1172 else if (Configuration.doAutoCreate()) 1173 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); // bb 1174 return this.equivalence; 1175 } 1176 1177 public boolean hasEquivalenceElement() { 1178 return this.equivalence != null && !this.equivalence.isEmpty(); 1179 } 1180 1181 public boolean hasEquivalence() { 1182 return this.equivalence != null && !this.equivalence.isEmpty(); 1183 } 1184 1185 /** 1186 * @param value {@link #equivalence} (The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).). This is the underlying object with id, value and extensions. The accessor "getEquivalence" gives direct access to the value 1187 */ 1188 public TargetElementComponent setEquivalenceElement(Enumeration<ConceptMapEquivalence> value) { 1189 this.equivalence = value; 1190 return this; 1191 } 1192 1193 /** 1194 * @return The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source). 1195 */ 1196 public ConceptMapEquivalence getEquivalence() { 1197 return this.equivalence == null ? null : this.equivalence.getValue(); 1198 } 1199 1200 /** 1201 * @param value The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source). 1202 */ 1203 public TargetElementComponent setEquivalence(ConceptMapEquivalence value) { 1204 if (value == null) 1205 this.equivalence = null; 1206 else { 1207 if (this.equivalence == null) 1208 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); 1209 this.equivalence.setValue(value); 1210 } 1211 return this; 1212 } 1213 1214 /** 1215 * @return {@link #comment} (A description of status/issues in mapping that conveys additional information not represented in the structured data.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1216 */ 1217 public StringType getCommentElement() { 1218 if (this.comment == null) 1219 if (Configuration.errorOnAutoCreate()) 1220 throw new Error("Attempt to auto-create TargetElementComponent.comment"); 1221 else if (Configuration.doAutoCreate()) 1222 this.comment = new StringType(); // bb 1223 return this.comment; 1224 } 1225 1226 public boolean hasCommentElement() { 1227 return this.comment != null && !this.comment.isEmpty(); 1228 } 1229 1230 public boolean hasComment() { 1231 return this.comment != null && !this.comment.isEmpty(); 1232 } 1233 1234 /** 1235 * @param value {@link #comment} (A description of status/issues in mapping that conveys additional information not represented in the structured data.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1236 */ 1237 public TargetElementComponent setCommentElement(StringType value) { 1238 this.comment = value; 1239 return this; 1240 } 1241 1242 /** 1243 * @return A description of status/issues in mapping that conveys additional information not represented in the structured data. 1244 */ 1245 public String getComment() { 1246 return this.comment == null ? null : this.comment.getValue(); 1247 } 1248 1249 /** 1250 * @param value A description of status/issues in mapping that conveys additional information not represented in the structured data. 1251 */ 1252 public TargetElementComponent setComment(String value) { 1253 if (Utilities.noString(value)) 1254 this.comment = null; 1255 else { 1256 if (this.comment == null) 1257 this.comment = new StringType(); 1258 this.comment.setValue(value); 1259 } 1260 return this; 1261 } 1262 1263 /** 1264 * @return {@link #dependsOn} (A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.) 1265 */ 1266 public List<OtherElementComponent> getDependsOn() { 1267 if (this.dependsOn == null) 1268 this.dependsOn = new ArrayList<OtherElementComponent>(); 1269 return this.dependsOn; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public TargetElementComponent setDependsOn(List<OtherElementComponent> theDependsOn) { 1276 this.dependsOn = theDependsOn; 1277 return this; 1278 } 1279 1280 public boolean hasDependsOn() { 1281 if (this.dependsOn == null) 1282 return false; 1283 for (OtherElementComponent item : this.dependsOn) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public OtherElementComponent addDependsOn() { //3 1290 OtherElementComponent t = new OtherElementComponent(); 1291 if (this.dependsOn == null) 1292 this.dependsOn = new ArrayList<OtherElementComponent>(); 1293 this.dependsOn.add(t); 1294 return t; 1295 } 1296 1297 public TargetElementComponent addDependsOn(OtherElementComponent t) { //3 1298 if (t == null) 1299 return this; 1300 if (this.dependsOn == null) 1301 this.dependsOn = new ArrayList<OtherElementComponent>(); 1302 this.dependsOn.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #dependsOn}, creating it if it does not already exist 1308 */ 1309 public OtherElementComponent getDependsOnFirstRep() { 1310 if (getDependsOn().isEmpty()) { 1311 addDependsOn(); 1312 } 1313 return getDependsOn().get(0); 1314 } 1315 1316 /** 1317 * @return {@link #product} (A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.) 1318 */ 1319 public List<OtherElementComponent> getProduct() { 1320 if (this.product == null) 1321 this.product = new ArrayList<OtherElementComponent>(); 1322 return this.product; 1323 } 1324 1325 /** 1326 * @return Returns a reference to <code>this</code> for easy method chaining 1327 */ 1328 public TargetElementComponent setProduct(List<OtherElementComponent> theProduct) { 1329 this.product = theProduct; 1330 return this; 1331 } 1332 1333 public boolean hasProduct() { 1334 if (this.product == null) 1335 return false; 1336 for (OtherElementComponent item : this.product) 1337 if (!item.isEmpty()) 1338 return true; 1339 return false; 1340 } 1341 1342 public OtherElementComponent addProduct() { //3 1343 OtherElementComponent t = new OtherElementComponent(); 1344 if (this.product == null) 1345 this.product = new ArrayList<OtherElementComponent>(); 1346 this.product.add(t); 1347 return t; 1348 } 1349 1350 public TargetElementComponent addProduct(OtherElementComponent t) { //3 1351 if (t == null) 1352 return this; 1353 if (this.product == null) 1354 this.product = new ArrayList<OtherElementComponent>(); 1355 this.product.add(t); 1356 return this; 1357 } 1358 1359 /** 1360 * @return The first repetition of repeating field {@link #product}, creating it if it does not already exist 1361 */ 1362 public OtherElementComponent getProductFirstRep() { 1363 if (getProduct().isEmpty()) { 1364 addProduct(); 1365 } 1366 return getProduct().get(0); 1367 } 1368 1369 protected void listChildren(List<Property> children) { 1370 super.listChildren(children); 1371 children.add(new Property("code", "code", "Identity (code or path) or the element/item that the map refers to.", 0, 1, code)); 1372 children.add(new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display)); 1373 children.add(new Property("equivalence", "code", "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).", 0, 1, equivalence)); 1374 children.add(new Property("comment", "string", "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 0, 1, comment)); 1375 children.add(new Property("dependsOn", "", "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.", 0, java.lang.Integer.MAX_VALUE, dependsOn)); 1376 children.add(new Property("product", "@ConceptMap.group.element.target.dependsOn", "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.", 0, java.lang.Integer.MAX_VALUE, product)); 1377 } 1378 1379 @Override 1380 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1381 switch (_hash) { 1382 case 3059181: /*code*/ return new Property("code", "code", "Identity (code or path) or the element/item that the map refers to.", 0, 1, code); 1383 case 1671764162: /*display*/ return new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display); 1384 case -15828692: /*equivalence*/ return new Property("equivalence", "code", "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).", 0, 1, equivalence); 1385 case 950398559: /*comment*/ return new Property("comment", "string", "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 0, 1, comment); 1386 case -1109214266: /*dependsOn*/ return new Property("dependsOn", "", "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.", 0, java.lang.Integer.MAX_VALUE, dependsOn); 1387 case -309474065: /*product*/ return new Property("product", "@ConceptMap.group.element.target.dependsOn", "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.", 0, java.lang.Integer.MAX_VALUE, product); 1388 default: return super.getNamedProperty(_hash, _name, _checkValid); 1389 } 1390 1391 } 1392 1393 @Override 1394 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1395 switch (hash) { 1396 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1397 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 1398 case -15828692: /*equivalence*/ return this.equivalence == null ? new Base[0] : new Base[] {this.equivalence}; // Enumeration<ConceptMapEquivalence> 1399 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 1400 case -1109214266: /*dependsOn*/ return this.dependsOn == null ? new Base[0] : this.dependsOn.toArray(new Base[this.dependsOn.size()]); // OtherElementComponent 1401 case -309474065: /*product*/ return this.product == null ? new Base[0] : this.product.toArray(new Base[this.product.size()]); // OtherElementComponent 1402 default: return super.getProperty(hash, name, checkValid); 1403 } 1404 1405 } 1406 1407 @Override 1408 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1409 switch (hash) { 1410 case 3059181: // code 1411 this.code = castToCode(value); // CodeType 1412 return value; 1413 case 1671764162: // display 1414 this.display = castToString(value); // StringType 1415 return value; 1416 case -15828692: // equivalence 1417 value = new ConceptMapEquivalenceEnumFactory().fromType(castToCode(value)); 1418 this.equivalence = (Enumeration) value; // Enumeration<ConceptMapEquivalence> 1419 return value; 1420 case 950398559: // comment 1421 this.comment = castToString(value); // StringType 1422 return value; 1423 case -1109214266: // dependsOn 1424 this.getDependsOn().add((OtherElementComponent) value); // OtherElementComponent 1425 return value; 1426 case -309474065: // product 1427 this.getProduct().add((OtherElementComponent) value); // OtherElementComponent 1428 return value; 1429 default: return super.setProperty(hash, name, value); 1430 } 1431 1432 } 1433 1434 @Override 1435 public Base setProperty(String name, Base value) throws FHIRException { 1436 if (name.equals("code")) { 1437 this.code = castToCode(value); // CodeType 1438 } else if (name.equals("display")) { 1439 this.display = castToString(value); // StringType 1440 } else if (name.equals("equivalence")) { 1441 value = new ConceptMapEquivalenceEnumFactory().fromType(castToCode(value)); 1442 this.equivalence = (Enumeration) value; // Enumeration<ConceptMapEquivalence> 1443 } else if (name.equals("comment")) { 1444 this.comment = castToString(value); // StringType 1445 } else if (name.equals("dependsOn")) { 1446 this.getDependsOn().add((OtherElementComponent) value); 1447 } else if (name.equals("product")) { 1448 this.getProduct().add((OtherElementComponent) value); 1449 } else 1450 return super.setProperty(name, value); 1451 return value; 1452 } 1453 1454 @Override 1455 public Base makeProperty(int hash, String name) throws FHIRException { 1456 switch (hash) { 1457 case 3059181: return getCodeElement(); 1458 case 1671764162: return getDisplayElement(); 1459 case -15828692: return getEquivalenceElement(); 1460 case 950398559: return getCommentElement(); 1461 case -1109214266: return addDependsOn(); 1462 case -309474065: return addProduct(); 1463 default: return super.makeProperty(hash, name); 1464 } 1465 1466 } 1467 1468 @Override 1469 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1470 switch (hash) { 1471 case 3059181: /*code*/ return new String[] {"code"}; 1472 case 1671764162: /*display*/ return new String[] {"string"}; 1473 case -15828692: /*equivalence*/ return new String[] {"code"}; 1474 case 950398559: /*comment*/ return new String[] {"string"}; 1475 case -1109214266: /*dependsOn*/ return new String[] {}; 1476 case -309474065: /*product*/ return new String[] {"@ConceptMap.group.element.target.dependsOn"}; 1477 default: return super.getTypesForProperty(hash, name); 1478 } 1479 1480 } 1481 1482 @Override 1483 public Base addChild(String name) throws FHIRException { 1484 if (name.equals("code")) { 1485 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 1486 } 1487 else if (name.equals("display")) { 1488 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 1489 } 1490 else if (name.equals("equivalence")) { 1491 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.equivalence"); 1492 } 1493 else if (name.equals("comment")) { 1494 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.comment"); 1495 } 1496 else if (name.equals("dependsOn")) { 1497 return addDependsOn(); 1498 } 1499 else if (name.equals("product")) { 1500 return addProduct(); 1501 } 1502 else 1503 return super.addChild(name); 1504 } 1505 1506 public TargetElementComponent copy() { 1507 TargetElementComponent dst = new TargetElementComponent(); 1508 copyValues(dst); 1509 dst.code = code == null ? null : code.copy(); 1510 dst.display = display == null ? null : display.copy(); 1511 dst.equivalence = equivalence == null ? null : equivalence.copy(); 1512 dst.comment = comment == null ? null : comment.copy(); 1513 if (dependsOn != null) { 1514 dst.dependsOn = new ArrayList<OtherElementComponent>(); 1515 for (OtherElementComponent i : dependsOn) 1516 dst.dependsOn.add(i.copy()); 1517 }; 1518 if (product != null) { 1519 dst.product = new ArrayList<OtherElementComponent>(); 1520 for (OtherElementComponent i : product) 1521 dst.product.add(i.copy()); 1522 }; 1523 return dst; 1524 } 1525 1526 @Override 1527 public boolean equalsDeep(Base other_) { 1528 if (!super.equalsDeep(other_)) 1529 return false; 1530 if (!(other_ instanceof TargetElementComponent)) 1531 return false; 1532 TargetElementComponent o = (TargetElementComponent) other_; 1533 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(equivalence, o.equivalence, true) 1534 && compareDeep(comment, o.comment, true) && compareDeep(dependsOn, o.dependsOn, true) && compareDeep(product, o.product, true) 1535 ; 1536 } 1537 1538 @Override 1539 public boolean equalsShallow(Base other_) { 1540 if (!super.equalsShallow(other_)) 1541 return false; 1542 if (!(other_ instanceof TargetElementComponent)) 1543 return false; 1544 TargetElementComponent o = (TargetElementComponent) other_; 1545 return compareValues(code, o.code, true) && compareValues(display, o.display, true) && compareValues(equivalence, o.equivalence, true) 1546 && compareValues(comment, o.comment, true); 1547 } 1548 1549 public boolean isEmpty() { 1550 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, equivalence 1551 , comment, dependsOn, product); 1552 } 1553 1554 public String fhirType() { 1555 return "ConceptMap.group.element.target"; 1556 1557 } 1558 1559 } 1560 1561 @Block() 1562 public static class OtherElementComponent extends BackboneElement implements IBaseBackboneElement { 1563 /** 1564 * A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property. 1565 */ 1566 @Child(name = "property", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1567 @Description(shortDefinition="Reference to property mapping depends on", formalDefinition="A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property." ) 1568 protected UriType property; 1569 1570 /** 1571 * An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems). 1572 */ 1573 @Child(name = "system", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1574 @Description(shortDefinition="Code System (if necessary)", formalDefinition="An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems)." ) 1575 protected UriType system; 1576 1577 /** 1578 * Identity (code or path) or the element/item/ValueSet that the map depends on / refers to. 1579 */ 1580 @Child(name = "code", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=false) 1581 @Description(shortDefinition="Value of the referenced element", formalDefinition="Identity (code or path) or the element/item/ValueSet that the map depends on / refers to." ) 1582 protected StringType code; 1583 1584 /** 1585 * The display for the code. The display is only provided to help editors when editing the concept map. 1586 */ 1587 @Child(name = "display", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1588 @Description(shortDefinition="Display for the code", formalDefinition="The display for the code. The display is only provided to help editors when editing the concept map." ) 1589 protected StringType display; 1590 1591 private static final long serialVersionUID = 678887659L; 1592 1593 /** 1594 * Constructor 1595 */ 1596 public OtherElementComponent() { 1597 super(); 1598 } 1599 1600 /** 1601 * Constructor 1602 */ 1603 public OtherElementComponent(UriType property, StringType code) { 1604 super(); 1605 this.property = property; 1606 this.code = code; 1607 } 1608 1609 /** 1610 * @return {@link #property} (A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property.). This is the underlying object with id, value and extensions. The accessor "getProperty" gives direct access to the value 1611 */ 1612 public UriType getPropertyElement() { 1613 if (this.property == null) 1614 if (Configuration.errorOnAutoCreate()) 1615 throw new Error("Attempt to auto-create OtherElementComponent.property"); 1616 else if (Configuration.doAutoCreate()) 1617 this.property = new UriType(); // bb 1618 return this.property; 1619 } 1620 1621 public boolean hasPropertyElement() { 1622 return this.property != null && !this.property.isEmpty(); 1623 } 1624 1625 public boolean hasProperty() { 1626 return this.property != null && !this.property.isEmpty(); 1627 } 1628 1629 /** 1630 * @param value {@link #property} (A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property.). This is the underlying object with id, value and extensions. The accessor "getProperty" gives direct access to the value 1631 */ 1632 public OtherElementComponent setPropertyElement(UriType value) { 1633 this.property = value; 1634 return this; 1635 } 1636 1637 /** 1638 * @return A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property. 1639 */ 1640 public String getProperty() { 1641 return this.property == null ? null : this.property.getValue(); 1642 } 1643 1644 /** 1645 * @param value A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property. 1646 */ 1647 public OtherElementComponent setProperty(String value) { 1648 if (this.property == null) 1649 this.property = new UriType(); 1650 this.property.setValue(value); 1651 return this; 1652 } 1653 1654 /** 1655 * @return {@link #system} (An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 1656 */ 1657 public UriType getSystemElement() { 1658 if (this.system == null) 1659 if (Configuration.errorOnAutoCreate()) 1660 throw new Error("Attempt to auto-create OtherElementComponent.system"); 1661 else if (Configuration.doAutoCreate()) 1662 this.system = new UriType(); // bb 1663 return this.system; 1664 } 1665 1666 public boolean hasSystemElement() { 1667 return this.system != null && !this.system.isEmpty(); 1668 } 1669 1670 public boolean hasSystem() { 1671 return this.system != null && !this.system.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #system} (An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 1676 */ 1677 public OtherElementComponent setSystemElement(UriType value) { 1678 this.system = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems). 1684 */ 1685 public String getSystem() { 1686 return this.system == null ? null : this.system.getValue(); 1687 } 1688 1689 /** 1690 * @param value An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems). 1691 */ 1692 public OtherElementComponent setSystem(String value) { 1693 if (Utilities.noString(value)) 1694 this.system = null; 1695 else { 1696 if (this.system == null) 1697 this.system = new UriType(); 1698 this.system.setValue(value); 1699 } 1700 return this; 1701 } 1702 1703 /** 1704 * @return {@link #code} (Identity (code or path) or the element/item/ValueSet that the map depends on / refers to.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1705 */ 1706 public StringType getCodeElement() { 1707 if (this.code == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create OtherElementComponent.code"); 1710 else if (Configuration.doAutoCreate()) 1711 this.code = new StringType(); // bb 1712 return this.code; 1713 } 1714 1715 public boolean hasCodeElement() { 1716 return this.code != null && !this.code.isEmpty(); 1717 } 1718 1719 public boolean hasCode() { 1720 return this.code != null && !this.code.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #code} (Identity (code or path) or the element/item/ValueSet that the map depends on / refers to.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1725 */ 1726 public OtherElementComponent setCodeElement(StringType value) { 1727 this.code = value; 1728 return this; 1729 } 1730 1731 /** 1732 * @return Identity (code or path) or the element/item/ValueSet that the map depends on / refers to. 1733 */ 1734 public String getCode() { 1735 return this.code == null ? null : this.code.getValue(); 1736 } 1737 1738 /** 1739 * @param value Identity (code or path) or the element/item/ValueSet that the map depends on / refers to. 1740 */ 1741 public OtherElementComponent setCode(String value) { 1742 if (this.code == null) 1743 this.code = new StringType(); 1744 this.code.setValue(value); 1745 return this; 1746 } 1747 1748 /** 1749 * @return {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1750 */ 1751 public StringType getDisplayElement() { 1752 if (this.display == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create OtherElementComponent.display"); 1755 else if (Configuration.doAutoCreate()) 1756 this.display = new StringType(); // bb 1757 return this.display; 1758 } 1759 1760 public boolean hasDisplayElement() { 1761 return this.display != null && !this.display.isEmpty(); 1762 } 1763 1764 public boolean hasDisplay() { 1765 return this.display != null && !this.display.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1770 */ 1771 public OtherElementComponent setDisplayElement(StringType value) { 1772 this.display = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return The display for the code. The display is only provided to help editors when editing the concept map. 1778 */ 1779 public String getDisplay() { 1780 return this.display == null ? null : this.display.getValue(); 1781 } 1782 1783 /** 1784 * @param value The display for the code. The display is only provided to help editors when editing the concept map. 1785 */ 1786 public OtherElementComponent setDisplay(String value) { 1787 if (Utilities.noString(value)) 1788 this.display = null; 1789 else { 1790 if (this.display == null) 1791 this.display = new StringType(); 1792 this.display.setValue(value); 1793 } 1794 return this; 1795 } 1796 1797 protected void listChildren(List<Property> children) { 1798 super.listChildren(children); 1799 children.add(new Property("property", "uri", "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property.", 0, 1, property)); 1800 children.add(new Property("system", "uri", "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).", 0, 1, system)); 1801 children.add(new Property("code", "string", "Identity (code or path) or the element/item/ValueSet that the map depends on / refers to.", 0, 1, code)); 1802 children.add(new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display)); 1803 } 1804 1805 @Override 1806 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1807 switch (_hash) { 1808 case -993141291: /*property*/ return new Property("property", "uri", "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somwhere that is labeled to correspond with a code system property.", 0, 1, property); 1809 case -887328209: /*system*/ return new Property("system", "uri", "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).", 0, 1, system); 1810 case 3059181: /*code*/ return new Property("code", "string", "Identity (code or path) or the element/item/ValueSet that the map depends on / refers to.", 0, 1, code); 1811 case 1671764162: /*display*/ return new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display); 1812 default: return super.getNamedProperty(_hash, _name, _checkValid); 1813 } 1814 1815 } 1816 1817 @Override 1818 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1819 switch (hash) { 1820 case -993141291: /*property*/ return this.property == null ? new Base[0] : new Base[] {this.property}; // UriType 1821 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 1822 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // StringType 1823 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 1824 default: return super.getProperty(hash, name, checkValid); 1825 } 1826 1827 } 1828 1829 @Override 1830 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1831 switch (hash) { 1832 case -993141291: // property 1833 this.property = castToUri(value); // UriType 1834 return value; 1835 case -887328209: // system 1836 this.system = castToUri(value); // UriType 1837 return value; 1838 case 3059181: // code 1839 this.code = castToString(value); // StringType 1840 return value; 1841 case 1671764162: // display 1842 this.display = castToString(value); // StringType 1843 return value; 1844 default: return super.setProperty(hash, name, value); 1845 } 1846 1847 } 1848 1849 @Override 1850 public Base setProperty(String name, Base value) throws FHIRException { 1851 if (name.equals("property")) { 1852 this.property = castToUri(value); // UriType 1853 } else if (name.equals("system")) { 1854 this.system = castToUri(value); // UriType 1855 } else if (name.equals("code")) { 1856 this.code = castToString(value); // StringType 1857 } else if (name.equals("display")) { 1858 this.display = castToString(value); // StringType 1859 } else 1860 return super.setProperty(name, value); 1861 return value; 1862 } 1863 1864 @Override 1865 public Base makeProperty(int hash, String name) throws FHIRException { 1866 switch (hash) { 1867 case -993141291: return getPropertyElement(); 1868 case -887328209: return getSystemElement(); 1869 case 3059181: return getCodeElement(); 1870 case 1671764162: return getDisplayElement(); 1871 default: return super.makeProperty(hash, name); 1872 } 1873 1874 } 1875 1876 @Override 1877 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1878 switch (hash) { 1879 case -993141291: /*property*/ return new String[] {"uri"}; 1880 case -887328209: /*system*/ return new String[] {"uri"}; 1881 case 3059181: /*code*/ return new String[] {"string"}; 1882 case 1671764162: /*display*/ return new String[] {"string"}; 1883 default: return super.getTypesForProperty(hash, name); 1884 } 1885 1886 } 1887 1888 @Override 1889 public Base addChild(String name) throws FHIRException { 1890 if (name.equals("property")) { 1891 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property"); 1892 } 1893 else if (name.equals("system")) { 1894 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.system"); 1895 } 1896 else if (name.equals("code")) { 1897 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 1898 } 1899 else if (name.equals("display")) { 1900 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 1901 } 1902 else 1903 return super.addChild(name); 1904 } 1905 1906 public OtherElementComponent copy() { 1907 OtherElementComponent dst = new OtherElementComponent(); 1908 copyValues(dst); 1909 dst.property = property == null ? null : property.copy(); 1910 dst.system = system == null ? null : system.copy(); 1911 dst.code = code == null ? null : code.copy(); 1912 dst.display = display == null ? null : display.copy(); 1913 return dst; 1914 } 1915 1916 @Override 1917 public boolean equalsDeep(Base other_) { 1918 if (!super.equalsDeep(other_)) 1919 return false; 1920 if (!(other_ instanceof OtherElementComponent)) 1921 return false; 1922 OtherElementComponent o = (OtherElementComponent) other_; 1923 return compareDeep(property, o.property, true) && compareDeep(system, o.system, true) && compareDeep(code, o.code, true) 1924 && compareDeep(display, o.display, true); 1925 } 1926 1927 @Override 1928 public boolean equalsShallow(Base other_) { 1929 if (!super.equalsShallow(other_)) 1930 return false; 1931 if (!(other_ instanceof OtherElementComponent)) 1932 return false; 1933 OtherElementComponent o = (OtherElementComponent) other_; 1934 return compareValues(property, o.property, true) && compareValues(system, o.system, true) && compareValues(code, o.code, true) 1935 && compareValues(display, o.display, true); 1936 } 1937 1938 public boolean isEmpty() { 1939 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(property, system, code, display 1940 ); 1941 } 1942 1943 public String fhirType() { 1944 return "ConceptMap.group.element.target.dependsOn"; 1945 1946 } 1947 1948 } 1949 1950 @Block() 1951 public static class ConceptMapGroupUnmappedComponent extends BackboneElement implements IBaseBackboneElement { 1952 /** 1953 * Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL). 1954 */ 1955 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1956 @Description(shortDefinition="provided | fixed | other-map", formalDefinition="Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL)." ) 1957 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conceptmap-unmapped-mode") 1958 protected Enumeration<ConceptMapGroupUnmappedMode> mode; 1959 1960 /** 1961 * The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code. 1962 */ 1963 @Child(name = "code", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1964 @Description(shortDefinition="Fixed code when mode = fixed", formalDefinition="The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code." ) 1965 protected CodeType code; 1966 1967 /** 1968 * The display for the code. The display is only provided to help editors when editing the concept map. 1969 */ 1970 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1971 @Description(shortDefinition="Display for the code", formalDefinition="The display for the code. The display is only provided to help editors when editing the concept map." ) 1972 protected StringType display; 1973 1974 /** 1975 * The canonical URL of the map to use if this map contains no mapping. 1976 */ 1977 @Child(name = "url", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1978 @Description(shortDefinition="Canonical URL for other concept map", formalDefinition="The canonical URL of the map to use if this map contains no mapping." ) 1979 protected UriType url; 1980 1981 private static final long serialVersionUID = -482446774L; 1982 1983 /** 1984 * Constructor 1985 */ 1986 public ConceptMapGroupUnmappedComponent() { 1987 super(); 1988 } 1989 1990 /** 1991 * Constructor 1992 */ 1993 public ConceptMapGroupUnmappedComponent(Enumeration<ConceptMapGroupUnmappedMode> mode) { 1994 super(); 1995 this.mode = mode; 1996 } 1997 1998 /** 1999 * @return {@link #mode} (Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2000 */ 2001 public Enumeration<ConceptMapGroupUnmappedMode> getModeElement() { 2002 if (this.mode == null) 2003 if (Configuration.errorOnAutoCreate()) 2004 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.mode"); 2005 else if (Configuration.doAutoCreate()) 2006 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); // bb 2007 return this.mode; 2008 } 2009 2010 public boolean hasModeElement() { 2011 return this.mode != null && !this.mode.isEmpty(); 2012 } 2013 2014 public boolean hasMode() { 2015 return this.mode != null && !this.mode.isEmpty(); 2016 } 2017 2018 /** 2019 * @param value {@link #mode} (Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2020 */ 2021 public ConceptMapGroupUnmappedComponent setModeElement(Enumeration<ConceptMapGroupUnmappedMode> value) { 2022 this.mode = value; 2023 return this; 2024 } 2025 2026 /** 2027 * @return Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL). 2028 */ 2029 public ConceptMapGroupUnmappedMode getMode() { 2030 return this.mode == null ? null : this.mode.getValue(); 2031 } 2032 2033 /** 2034 * @param value Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL). 2035 */ 2036 public ConceptMapGroupUnmappedComponent setMode(ConceptMapGroupUnmappedMode value) { 2037 if (this.mode == null) 2038 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); 2039 this.mode.setValue(value); 2040 return this; 2041 } 2042 2043 /** 2044 * @return {@link #code} (The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2045 */ 2046 public CodeType getCodeElement() { 2047 if (this.code == null) 2048 if (Configuration.errorOnAutoCreate()) 2049 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.code"); 2050 else if (Configuration.doAutoCreate()) 2051 this.code = new CodeType(); // bb 2052 return this.code; 2053 } 2054 2055 public boolean hasCodeElement() { 2056 return this.code != null && !this.code.isEmpty(); 2057 } 2058 2059 public boolean hasCode() { 2060 return this.code != null && !this.code.isEmpty(); 2061 } 2062 2063 /** 2064 * @param value {@link #code} (The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2065 */ 2066 public ConceptMapGroupUnmappedComponent setCodeElement(CodeType value) { 2067 this.code = value; 2068 return this; 2069 } 2070 2071 /** 2072 * @return The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code. 2073 */ 2074 public String getCode() { 2075 return this.code == null ? null : this.code.getValue(); 2076 } 2077 2078 /** 2079 * @param value The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code. 2080 */ 2081 public ConceptMapGroupUnmappedComponent setCode(String value) { 2082 if (Utilities.noString(value)) 2083 this.code = null; 2084 else { 2085 if (this.code == null) 2086 this.code = new CodeType(); 2087 this.code.setValue(value); 2088 } 2089 return this; 2090 } 2091 2092 /** 2093 * @return {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 2094 */ 2095 public StringType getDisplayElement() { 2096 if (this.display == null) 2097 if (Configuration.errorOnAutoCreate()) 2098 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.display"); 2099 else if (Configuration.doAutoCreate()) 2100 this.display = new StringType(); // bb 2101 return this.display; 2102 } 2103 2104 public boolean hasDisplayElement() { 2105 return this.display != null && !this.display.isEmpty(); 2106 } 2107 2108 public boolean hasDisplay() { 2109 return this.display != null && !this.display.isEmpty(); 2110 } 2111 2112 /** 2113 * @param value {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 2114 */ 2115 public ConceptMapGroupUnmappedComponent setDisplayElement(StringType value) { 2116 this.display = value; 2117 return this; 2118 } 2119 2120 /** 2121 * @return The display for the code. The display is only provided to help editors when editing the concept map. 2122 */ 2123 public String getDisplay() { 2124 return this.display == null ? null : this.display.getValue(); 2125 } 2126 2127 /** 2128 * @param value The display for the code. The display is only provided to help editors when editing the concept map. 2129 */ 2130 public ConceptMapGroupUnmappedComponent setDisplay(String value) { 2131 if (Utilities.noString(value)) 2132 this.display = null; 2133 else { 2134 if (this.display == null) 2135 this.display = new StringType(); 2136 this.display.setValue(value); 2137 } 2138 return this; 2139 } 2140 2141 /** 2142 * @return {@link #url} (The canonical URL of the map to use if this map contains no mapping.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2143 */ 2144 public UriType getUrlElement() { 2145 if (this.url == null) 2146 if (Configuration.errorOnAutoCreate()) 2147 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.url"); 2148 else if (Configuration.doAutoCreate()) 2149 this.url = new UriType(); // bb 2150 return this.url; 2151 } 2152 2153 public boolean hasUrlElement() { 2154 return this.url != null && !this.url.isEmpty(); 2155 } 2156 2157 public boolean hasUrl() { 2158 return this.url != null && !this.url.isEmpty(); 2159 } 2160 2161 /** 2162 * @param value {@link #url} (The canonical URL of the map to use if this map contains no mapping.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2163 */ 2164 public ConceptMapGroupUnmappedComponent setUrlElement(UriType value) { 2165 this.url = value; 2166 return this; 2167 } 2168 2169 /** 2170 * @return The canonical URL of the map to use if this map contains no mapping. 2171 */ 2172 public String getUrl() { 2173 return this.url == null ? null : this.url.getValue(); 2174 } 2175 2176 /** 2177 * @param value The canonical URL of the map to use if this map contains no mapping. 2178 */ 2179 public ConceptMapGroupUnmappedComponent setUrl(String value) { 2180 if (Utilities.noString(value)) 2181 this.url = null; 2182 else { 2183 if (this.url == null) 2184 this.url = new UriType(); 2185 this.url.setValue(value); 2186 } 2187 return this; 2188 } 2189 2190 protected void listChildren(List<Property> children) { 2191 super.listChildren(children); 2192 children.add(new Property("mode", "code", "Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 0, 1, mode)); 2193 children.add(new Property("code", "code", "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 1, code)); 2194 children.add(new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display)); 2195 children.add(new Property("url", "uri", "The canonical URL of the map to use if this map contains no mapping.", 0, 1, url)); 2196 } 2197 2198 @Override 2199 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2200 switch (_hash) { 2201 case 3357091: /*mode*/ return new Property("mode", "code", "Defines which action to take if there is no match in the group. One of 3 actions is possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 0, 1, mode); 2202 case 3059181: /*code*/ return new Property("code", "code", "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 1, code); 2203 case 1671764162: /*display*/ return new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display); 2204 case 116079: /*url*/ return new Property("url", "uri", "The canonical URL of the map to use if this map contains no mapping.", 0, 1, url); 2205 default: return super.getNamedProperty(_hash, _name, _checkValid); 2206 } 2207 2208 } 2209 2210 @Override 2211 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2212 switch (hash) { 2213 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<ConceptMapGroupUnmappedMode> 2214 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2215 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 2216 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2217 default: return super.getProperty(hash, name, checkValid); 2218 } 2219 2220 } 2221 2222 @Override 2223 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2224 switch (hash) { 2225 case 3357091: // mode 2226 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(castToCode(value)); 2227 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 2228 return value; 2229 case 3059181: // code 2230 this.code = castToCode(value); // CodeType 2231 return value; 2232 case 1671764162: // display 2233 this.display = castToString(value); // StringType 2234 return value; 2235 case 116079: // url 2236 this.url = castToUri(value); // UriType 2237 return value; 2238 default: return super.setProperty(hash, name, value); 2239 } 2240 2241 } 2242 2243 @Override 2244 public Base setProperty(String name, Base value) throws FHIRException { 2245 if (name.equals("mode")) { 2246 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(castToCode(value)); 2247 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 2248 } else if (name.equals("code")) { 2249 this.code = castToCode(value); // CodeType 2250 } else if (name.equals("display")) { 2251 this.display = castToString(value); // StringType 2252 } else if (name.equals("url")) { 2253 this.url = castToUri(value); // UriType 2254 } else 2255 return super.setProperty(name, value); 2256 return value; 2257 } 2258 2259 @Override 2260 public Base makeProperty(int hash, String name) throws FHIRException { 2261 switch (hash) { 2262 case 3357091: return getModeElement(); 2263 case 3059181: return getCodeElement(); 2264 case 1671764162: return getDisplayElement(); 2265 case 116079: return getUrlElement(); 2266 default: return super.makeProperty(hash, name); 2267 } 2268 2269 } 2270 2271 @Override 2272 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2273 switch (hash) { 2274 case 3357091: /*mode*/ return new String[] {"code"}; 2275 case 3059181: /*code*/ return new String[] {"code"}; 2276 case 1671764162: /*display*/ return new String[] {"string"}; 2277 case 116079: /*url*/ return new String[] {"uri"}; 2278 default: return super.getTypesForProperty(hash, name); 2279 } 2280 2281 } 2282 2283 @Override 2284 public Base addChild(String name) throws FHIRException { 2285 if (name.equals("mode")) { 2286 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.mode"); 2287 } 2288 else if (name.equals("code")) { 2289 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 2290 } 2291 else if (name.equals("display")) { 2292 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 2293 } 2294 else if (name.equals("url")) { 2295 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 2296 } 2297 else 2298 return super.addChild(name); 2299 } 2300 2301 public ConceptMapGroupUnmappedComponent copy() { 2302 ConceptMapGroupUnmappedComponent dst = new ConceptMapGroupUnmappedComponent(); 2303 copyValues(dst); 2304 dst.mode = mode == null ? null : mode.copy(); 2305 dst.code = code == null ? null : code.copy(); 2306 dst.display = display == null ? null : display.copy(); 2307 dst.url = url == null ? null : url.copy(); 2308 return dst; 2309 } 2310 2311 @Override 2312 public boolean equalsDeep(Base other_) { 2313 if (!super.equalsDeep(other_)) 2314 return false; 2315 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 2316 return false; 2317 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 2318 return compareDeep(mode, o.mode, true) && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 2319 && compareDeep(url, o.url, true); 2320 } 2321 2322 @Override 2323 public boolean equalsShallow(Base other_) { 2324 if (!super.equalsShallow(other_)) 2325 return false; 2326 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 2327 return false; 2328 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 2329 return compareValues(mode, o.mode, true) && compareValues(code, o.code, true) && compareValues(display, o.display, true) 2330 && compareValues(url, o.url, true); 2331 } 2332 2333 public boolean isEmpty() { 2334 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, code, display, url 2335 ); 2336 } 2337 2338 public String fhirType() { 2339 return "ConceptMap.group.unmapped"; 2340 2341 } 2342 2343 } 2344 2345 /** 2346 * A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance. 2347 */ 2348 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2349 @Description(shortDefinition="Additional identifier for the concept map", formalDefinition="A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2350 protected Identifier identifier; 2351 2352 /** 2353 * Explaination of why this concept map is needed and why it has been designed as it has. 2354 */ 2355 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2356 @Description(shortDefinition="Why this concept map is defined", formalDefinition="Explaination of why this concept map is needed and why it has been designed as it has." ) 2357 protected MarkdownType purpose; 2358 2359 /** 2360 * A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map. 2361 */ 2362 @Child(name = "copyright", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2363 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map." ) 2364 protected MarkdownType copyright; 2365 2366 /** 2367 * The source value set that specifies the concepts that are being mapped. 2368 */ 2369 @Child(name = "source", type = {UriType.class, ValueSet.class}, order=3, min=0, max=1, modifier=false, summary=true) 2370 @Description(shortDefinition="Identifies the source of the concepts which are being mapped", formalDefinition="The source value set that specifies the concepts that are being mapped." ) 2371 protected Type source; 2372 2373 /** 2374 * The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made. 2375 */ 2376 @Child(name = "target", type = {UriType.class, ValueSet.class}, order=4, min=0, max=1, modifier=false, summary=true) 2377 @Description(shortDefinition="Provides context to the mappings", formalDefinition="The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made." ) 2378 protected Type target; 2379 2380 /** 2381 * A group of mappings that all have the same source and target system. 2382 */ 2383 @Child(name = "group", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2384 @Description(shortDefinition="Same source and target systems", formalDefinition="A group of mappings that all have the same source and target system." ) 2385 protected List<ConceptMapGroupComponent> group; 2386 2387 private static final long serialVersionUID = -2081872580L; 2388 2389 /** 2390 * Constructor 2391 */ 2392 public ConceptMap() { 2393 super(); 2394 } 2395 2396 /** 2397 * Constructor 2398 */ 2399 public ConceptMap(Enumeration<PublicationStatus> status) { 2400 super(); 2401 this.status = status; 2402 } 2403 2404 /** 2405 * @return {@link #url} (An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published. The URL SHOULD include the major version of the concept map. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2406 */ 2407 public UriType getUrlElement() { 2408 if (this.url == null) 2409 if (Configuration.errorOnAutoCreate()) 2410 throw new Error("Attempt to auto-create ConceptMap.url"); 2411 else if (Configuration.doAutoCreate()) 2412 this.url = new UriType(); // bb 2413 return this.url; 2414 } 2415 2416 public boolean hasUrlElement() { 2417 return this.url != null && !this.url.isEmpty(); 2418 } 2419 2420 public boolean hasUrl() { 2421 return this.url != null && !this.url.isEmpty(); 2422 } 2423 2424 /** 2425 * @param value {@link #url} (An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published. The URL SHOULD include the major version of the concept map. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2426 */ 2427 public ConceptMap setUrlElement(UriType value) { 2428 this.url = value; 2429 return this; 2430 } 2431 2432 /** 2433 * @return An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published. The URL SHOULD include the major version of the concept map. For more information see [Technical and Business Versions](resource.html#versions). 2434 */ 2435 public String getUrl() { 2436 return this.url == null ? null : this.url.getValue(); 2437 } 2438 2439 /** 2440 * @param value An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published. The URL SHOULD include the major version of the concept map. For more information see [Technical and Business Versions](resource.html#versions). 2441 */ 2442 public ConceptMap setUrl(String value) { 2443 if (Utilities.noString(value)) 2444 this.url = null; 2445 else { 2446 if (this.url == null) 2447 this.url = new UriType(); 2448 this.url.setValue(value); 2449 } 2450 return this; 2451 } 2452 2453 /** 2454 * @return {@link #identifier} (A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2455 */ 2456 public Identifier getIdentifier() { 2457 if (this.identifier == null) 2458 if (Configuration.errorOnAutoCreate()) 2459 throw new Error("Attempt to auto-create ConceptMap.identifier"); 2460 else if (Configuration.doAutoCreate()) 2461 this.identifier = new Identifier(); // cc 2462 return this.identifier; 2463 } 2464 2465 public boolean hasIdentifier() { 2466 return this.identifier != null && !this.identifier.isEmpty(); 2467 } 2468 2469 /** 2470 * @param value {@link #identifier} (A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2471 */ 2472 public ConceptMap setIdentifier(Identifier value) { 2473 this.identifier = value; 2474 return this; 2475 } 2476 2477 /** 2478 * @return {@link #version} (The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2479 */ 2480 public StringType getVersionElement() { 2481 if (this.version == null) 2482 if (Configuration.errorOnAutoCreate()) 2483 throw new Error("Attempt to auto-create ConceptMap.version"); 2484 else if (Configuration.doAutoCreate()) 2485 this.version = new StringType(); // bb 2486 return this.version; 2487 } 2488 2489 public boolean hasVersionElement() { 2490 return this.version != null && !this.version.isEmpty(); 2491 } 2492 2493 public boolean hasVersion() { 2494 return this.version != null && !this.version.isEmpty(); 2495 } 2496 2497 /** 2498 * @param value {@link #version} (The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2499 */ 2500 public ConceptMap setVersionElement(StringType value) { 2501 this.version = value; 2502 return this; 2503 } 2504 2505 /** 2506 * @return The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2507 */ 2508 public String getVersion() { 2509 return this.version == null ? null : this.version.getValue(); 2510 } 2511 2512 /** 2513 * @param value The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2514 */ 2515 public ConceptMap setVersion(String value) { 2516 if (Utilities.noString(value)) 2517 this.version = null; 2518 else { 2519 if (this.version == null) 2520 this.version = new StringType(); 2521 this.version.setValue(value); 2522 } 2523 return this; 2524 } 2525 2526 /** 2527 * @return {@link #name} (A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2528 */ 2529 public StringType getNameElement() { 2530 if (this.name == null) 2531 if (Configuration.errorOnAutoCreate()) 2532 throw new Error("Attempt to auto-create ConceptMap.name"); 2533 else if (Configuration.doAutoCreate()) 2534 this.name = new StringType(); // bb 2535 return this.name; 2536 } 2537 2538 public boolean hasNameElement() { 2539 return this.name != null && !this.name.isEmpty(); 2540 } 2541 2542 public boolean hasName() { 2543 return this.name != null && !this.name.isEmpty(); 2544 } 2545 2546 /** 2547 * @param value {@link #name} (A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2548 */ 2549 public ConceptMap setNameElement(StringType value) { 2550 this.name = value; 2551 return this; 2552 } 2553 2554 /** 2555 * @return A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2556 */ 2557 public String getName() { 2558 return this.name == null ? null : this.name.getValue(); 2559 } 2560 2561 /** 2562 * @param value A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2563 */ 2564 public ConceptMap setName(String value) { 2565 if (Utilities.noString(value)) 2566 this.name = null; 2567 else { 2568 if (this.name == null) 2569 this.name = new StringType(); 2570 this.name.setValue(value); 2571 } 2572 return this; 2573 } 2574 2575 /** 2576 * @return {@link #title} (A short, descriptive, user-friendly title for the concept map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2577 */ 2578 public StringType getTitleElement() { 2579 if (this.title == null) 2580 if (Configuration.errorOnAutoCreate()) 2581 throw new Error("Attempt to auto-create ConceptMap.title"); 2582 else if (Configuration.doAutoCreate()) 2583 this.title = new StringType(); // bb 2584 return this.title; 2585 } 2586 2587 public boolean hasTitleElement() { 2588 return this.title != null && !this.title.isEmpty(); 2589 } 2590 2591 public boolean hasTitle() { 2592 return this.title != null && !this.title.isEmpty(); 2593 } 2594 2595 /** 2596 * @param value {@link #title} (A short, descriptive, user-friendly title for the concept map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2597 */ 2598 public ConceptMap setTitleElement(StringType value) { 2599 this.title = value; 2600 return this; 2601 } 2602 2603 /** 2604 * @return A short, descriptive, user-friendly title for the concept map. 2605 */ 2606 public String getTitle() { 2607 return this.title == null ? null : this.title.getValue(); 2608 } 2609 2610 /** 2611 * @param value A short, descriptive, user-friendly title for the concept map. 2612 */ 2613 public ConceptMap setTitle(String value) { 2614 if (Utilities.noString(value)) 2615 this.title = null; 2616 else { 2617 if (this.title == null) 2618 this.title = new StringType(); 2619 this.title.setValue(value); 2620 } 2621 return this; 2622 } 2623 2624 /** 2625 * @return {@link #status} (The status of this concept map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2626 */ 2627 public Enumeration<PublicationStatus> getStatusElement() { 2628 if (this.status == null) 2629 if (Configuration.errorOnAutoCreate()) 2630 throw new Error("Attempt to auto-create ConceptMap.status"); 2631 else if (Configuration.doAutoCreate()) 2632 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2633 return this.status; 2634 } 2635 2636 public boolean hasStatusElement() { 2637 return this.status != null && !this.status.isEmpty(); 2638 } 2639 2640 public boolean hasStatus() { 2641 return this.status != null && !this.status.isEmpty(); 2642 } 2643 2644 /** 2645 * @param value {@link #status} (The status of this concept map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2646 */ 2647 public ConceptMap setStatusElement(Enumeration<PublicationStatus> value) { 2648 this.status = value; 2649 return this; 2650 } 2651 2652 /** 2653 * @return The status of this concept map. Enables tracking the life-cycle of the content. 2654 */ 2655 public PublicationStatus getStatus() { 2656 return this.status == null ? null : this.status.getValue(); 2657 } 2658 2659 /** 2660 * @param value The status of this concept map. Enables tracking the life-cycle of the content. 2661 */ 2662 public ConceptMap setStatus(PublicationStatus value) { 2663 if (this.status == null) 2664 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2665 this.status.setValue(value); 2666 return this; 2667 } 2668 2669 /** 2670 * @return {@link #experimental} (A boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2671 */ 2672 public BooleanType getExperimentalElement() { 2673 if (this.experimental == null) 2674 if (Configuration.errorOnAutoCreate()) 2675 throw new Error("Attempt to auto-create ConceptMap.experimental"); 2676 else if (Configuration.doAutoCreate()) 2677 this.experimental = new BooleanType(); // bb 2678 return this.experimental; 2679 } 2680 2681 public boolean hasExperimentalElement() { 2682 return this.experimental != null && !this.experimental.isEmpty(); 2683 } 2684 2685 public boolean hasExperimental() { 2686 return this.experimental != null && !this.experimental.isEmpty(); 2687 } 2688 2689 /** 2690 * @param value {@link #experimental} (A boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2691 */ 2692 public ConceptMap setExperimentalElement(BooleanType value) { 2693 this.experimental = value; 2694 return this; 2695 } 2696 2697 /** 2698 * @return A boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2699 */ 2700 public boolean getExperimental() { 2701 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2702 } 2703 2704 /** 2705 * @param value A boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2706 */ 2707 public ConceptMap setExperimental(boolean value) { 2708 if (this.experimental == null) 2709 this.experimental = new BooleanType(); 2710 this.experimental.setValue(value); 2711 return this; 2712 } 2713 2714 /** 2715 * @return {@link #date} (The date (and optionally time) when the concept map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2716 */ 2717 public DateTimeType getDateElement() { 2718 if (this.date == null) 2719 if (Configuration.errorOnAutoCreate()) 2720 throw new Error("Attempt to auto-create ConceptMap.date"); 2721 else if (Configuration.doAutoCreate()) 2722 this.date = new DateTimeType(); // bb 2723 return this.date; 2724 } 2725 2726 public boolean hasDateElement() { 2727 return this.date != null && !this.date.isEmpty(); 2728 } 2729 2730 public boolean hasDate() { 2731 return this.date != null && !this.date.isEmpty(); 2732 } 2733 2734 /** 2735 * @param value {@link #date} (The date (and optionally time) when the concept map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2736 */ 2737 public ConceptMap setDateElement(DateTimeType value) { 2738 this.date = value; 2739 return this; 2740 } 2741 2742 /** 2743 * @return The date (and optionally time) when the concept map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes. 2744 */ 2745 public Date getDate() { 2746 return this.date == null ? null : this.date.getValue(); 2747 } 2748 2749 /** 2750 * @param value The date (and optionally time) when the concept map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes. 2751 */ 2752 public ConceptMap setDate(Date value) { 2753 if (value == null) 2754 this.date = null; 2755 else { 2756 if (this.date == null) 2757 this.date = new DateTimeType(); 2758 this.date.setValue(value); 2759 } 2760 return this; 2761 } 2762 2763 /** 2764 * @return {@link #publisher} (The name of the individual or organization that published the concept map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2765 */ 2766 public StringType getPublisherElement() { 2767 if (this.publisher == null) 2768 if (Configuration.errorOnAutoCreate()) 2769 throw new Error("Attempt to auto-create ConceptMap.publisher"); 2770 else if (Configuration.doAutoCreate()) 2771 this.publisher = new StringType(); // bb 2772 return this.publisher; 2773 } 2774 2775 public boolean hasPublisherElement() { 2776 return this.publisher != null && !this.publisher.isEmpty(); 2777 } 2778 2779 public boolean hasPublisher() { 2780 return this.publisher != null && !this.publisher.isEmpty(); 2781 } 2782 2783 /** 2784 * @param value {@link #publisher} (The name of the individual or organization that published the concept map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2785 */ 2786 public ConceptMap setPublisherElement(StringType value) { 2787 this.publisher = value; 2788 return this; 2789 } 2790 2791 /** 2792 * @return The name of the individual or organization that published the concept map. 2793 */ 2794 public String getPublisher() { 2795 return this.publisher == null ? null : this.publisher.getValue(); 2796 } 2797 2798 /** 2799 * @param value The name of the individual or organization that published the concept map. 2800 */ 2801 public ConceptMap setPublisher(String value) { 2802 if (Utilities.noString(value)) 2803 this.publisher = null; 2804 else { 2805 if (this.publisher == null) 2806 this.publisher = new StringType(); 2807 this.publisher.setValue(value); 2808 } 2809 return this; 2810 } 2811 2812 /** 2813 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2814 */ 2815 public List<ContactDetail> getContact() { 2816 if (this.contact == null) 2817 this.contact = new ArrayList<ContactDetail>(); 2818 return this.contact; 2819 } 2820 2821 /** 2822 * @return Returns a reference to <code>this</code> for easy method chaining 2823 */ 2824 public ConceptMap setContact(List<ContactDetail> theContact) { 2825 this.contact = theContact; 2826 return this; 2827 } 2828 2829 public boolean hasContact() { 2830 if (this.contact == null) 2831 return false; 2832 for (ContactDetail item : this.contact) 2833 if (!item.isEmpty()) 2834 return true; 2835 return false; 2836 } 2837 2838 public ContactDetail addContact() { //3 2839 ContactDetail t = new ContactDetail(); 2840 if (this.contact == null) 2841 this.contact = new ArrayList<ContactDetail>(); 2842 this.contact.add(t); 2843 return t; 2844 } 2845 2846 public ConceptMap addContact(ContactDetail t) { //3 2847 if (t == null) 2848 return this; 2849 if (this.contact == null) 2850 this.contact = new ArrayList<ContactDetail>(); 2851 this.contact.add(t); 2852 return this; 2853 } 2854 2855 /** 2856 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2857 */ 2858 public ContactDetail getContactFirstRep() { 2859 if (getContact().isEmpty()) { 2860 addContact(); 2861 } 2862 return getContact().get(0); 2863 } 2864 2865 /** 2866 * @return {@link #description} (A free text natural language description of the concept map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2867 */ 2868 public MarkdownType getDescriptionElement() { 2869 if (this.description == null) 2870 if (Configuration.errorOnAutoCreate()) 2871 throw new Error("Attempt to auto-create ConceptMap.description"); 2872 else if (Configuration.doAutoCreate()) 2873 this.description = new MarkdownType(); // bb 2874 return this.description; 2875 } 2876 2877 public boolean hasDescriptionElement() { 2878 return this.description != null && !this.description.isEmpty(); 2879 } 2880 2881 public boolean hasDescription() { 2882 return this.description != null && !this.description.isEmpty(); 2883 } 2884 2885 /** 2886 * @param value {@link #description} (A free text natural language description of the concept map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2887 */ 2888 public ConceptMap setDescriptionElement(MarkdownType value) { 2889 this.description = value; 2890 return this; 2891 } 2892 2893 /** 2894 * @return A free text natural language description of the concept map from a consumer's perspective. 2895 */ 2896 public String getDescription() { 2897 return this.description == null ? null : this.description.getValue(); 2898 } 2899 2900 /** 2901 * @param value A free text natural language description of the concept map from a consumer's perspective. 2902 */ 2903 public ConceptMap setDescription(String value) { 2904 if (value == null) 2905 this.description = null; 2906 else { 2907 if (this.description == null) 2908 this.description = new MarkdownType(); 2909 this.description.setValue(value); 2910 } 2911 return this; 2912 } 2913 2914 /** 2915 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate concept map instances.) 2916 */ 2917 public List<UsageContext> getUseContext() { 2918 if (this.useContext == null) 2919 this.useContext = new ArrayList<UsageContext>(); 2920 return this.useContext; 2921 } 2922 2923 /** 2924 * @return Returns a reference to <code>this</code> for easy method chaining 2925 */ 2926 public ConceptMap setUseContext(List<UsageContext> theUseContext) { 2927 this.useContext = theUseContext; 2928 return this; 2929 } 2930 2931 public boolean hasUseContext() { 2932 if (this.useContext == null) 2933 return false; 2934 for (UsageContext item : this.useContext) 2935 if (!item.isEmpty()) 2936 return true; 2937 return false; 2938 } 2939 2940 public UsageContext addUseContext() { //3 2941 UsageContext t = new UsageContext(); 2942 if (this.useContext == null) 2943 this.useContext = new ArrayList<UsageContext>(); 2944 this.useContext.add(t); 2945 return t; 2946 } 2947 2948 public ConceptMap addUseContext(UsageContext t) { //3 2949 if (t == null) 2950 return this; 2951 if (this.useContext == null) 2952 this.useContext = new ArrayList<UsageContext>(); 2953 this.useContext.add(t); 2954 return this; 2955 } 2956 2957 /** 2958 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 2959 */ 2960 public UsageContext getUseContextFirstRep() { 2961 if (getUseContext().isEmpty()) { 2962 addUseContext(); 2963 } 2964 return getUseContext().get(0); 2965 } 2966 2967 /** 2968 * @return {@link #jurisdiction} (A legal or geographic region in which the concept map is intended to be used.) 2969 */ 2970 public List<CodeableConcept> getJurisdiction() { 2971 if (this.jurisdiction == null) 2972 this.jurisdiction = new ArrayList<CodeableConcept>(); 2973 return this.jurisdiction; 2974 } 2975 2976 /** 2977 * @return Returns a reference to <code>this</code> for easy method chaining 2978 */ 2979 public ConceptMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 2980 this.jurisdiction = theJurisdiction; 2981 return this; 2982 } 2983 2984 public boolean hasJurisdiction() { 2985 if (this.jurisdiction == null) 2986 return false; 2987 for (CodeableConcept item : this.jurisdiction) 2988 if (!item.isEmpty()) 2989 return true; 2990 return false; 2991 } 2992 2993 public CodeableConcept addJurisdiction() { //3 2994 CodeableConcept t = new CodeableConcept(); 2995 if (this.jurisdiction == null) 2996 this.jurisdiction = new ArrayList<CodeableConcept>(); 2997 this.jurisdiction.add(t); 2998 return t; 2999 } 3000 3001 public ConceptMap addJurisdiction(CodeableConcept t) { //3 3002 if (t == null) 3003 return this; 3004 if (this.jurisdiction == null) 3005 this.jurisdiction = new ArrayList<CodeableConcept>(); 3006 this.jurisdiction.add(t); 3007 return this; 3008 } 3009 3010 /** 3011 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 3012 */ 3013 public CodeableConcept getJurisdictionFirstRep() { 3014 if (getJurisdiction().isEmpty()) { 3015 addJurisdiction(); 3016 } 3017 return getJurisdiction().get(0); 3018 } 3019 3020 /** 3021 * @return {@link #purpose} (Explaination of why this concept map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3022 */ 3023 public MarkdownType getPurposeElement() { 3024 if (this.purpose == null) 3025 if (Configuration.errorOnAutoCreate()) 3026 throw new Error("Attempt to auto-create ConceptMap.purpose"); 3027 else if (Configuration.doAutoCreate()) 3028 this.purpose = new MarkdownType(); // bb 3029 return this.purpose; 3030 } 3031 3032 public boolean hasPurposeElement() { 3033 return this.purpose != null && !this.purpose.isEmpty(); 3034 } 3035 3036 public boolean hasPurpose() { 3037 return this.purpose != null && !this.purpose.isEmpty(); 3038 } 3039 3040 /** 3041 * @param value {@link #purpose} (Explaination of why this concept map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3042 */ 3043 public ConceptMap setPurposeElement(MarkdownType value) { 3044 this.purpose = value; 3045 return this; 3046 } 3047 3048 /** 3049 * @return Explaination of why this concept map is needed and why it has been designed as it has. 3050 */ 3051 public String getPurpose() { 3052 return this.purpose == null ? null : this.purpose.getValue(); 3053 } 3054 3055 /** 3056 * @param value Explaination of why this concept map is needed and why it has been designed as it has. 3057 */ 3058 public ConceptMap setPurpose(String value) { 3059 if (value == null) 3060 this.purpose = null; 3061 else { 3062 if (this.purpose == null) 3063 this.purpose = new MarkdownType(); 3064 this.purpose.setValue(value); 3065 } 3066 return this; 3067 } 3068 3069 /** 3070 * @return {@link #copyright} (A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3071 */ 3072 public MarkdownType getCopyrightElement() { 3073 if (this.copyright == null) 3074 if (Configuration.errorOnAutoCreate()) 3075 throw new Error("Attempt to auto-create ConceptMap.copyright"); 3076 else if (Configuration.doAutoCreate()) 3077 this.copyright = new MarkdownType(); // bb 3078 return this.copyright; 3079 } 3080 3081 public boolean hasCopyrightElement() { 3082 return this.copyright != null && !this.copyright.isEmpty(); 3083 } 3084 3085 public boolean hasCopyright() { 3086 return this.copyright != null && !this.copyright.isEmpty(); 3087 } 3088 3089 /** 3090 * @param value {@link #copyright} (A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3091 */ 3092 public ConceptMap setCopyrightElement(MarkdownType value) { 3093 this.copyright = value; 3094 return this; 3095 } 3096 3097 /** 3098 * @return A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map. 3099 */ 3100 public String getCopyright() { 3101 return this.copyright == null ? null : this.copyright.getValue(); 3102 } 3103 3104 /** 3105 * @param value A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map. 3106 */ 3107 public ConceptMap setCopyright(String value) { 3108 if (value == null) 3109 this.copyright = null; 3110 else { 3111 if (this.copyright == null) 3112 this.copyright = new MarkdownType(); 3113 this.copyright.setValue(value); 3114 } 3115 return this; 3116 } 3117 3118 /** 3119 * @return {@link #source} (The source value set that specifies the concepts that are being mapped.) 3120 */ 3121 public Type getSource() { 3122 return this.source; 3123 } 3124 3125 /** 3126 * @return {@link #source} (The source value set that specifies the concepts that are being mapped.) 3127 */ 3128 public UriType getSourceUriType() throws FHIRException { 3129 if (this.source == null) 3130 return null; 3131 if (!(this.source instanceof UriType)) 3132 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.source.getClass().getName()+" was encountered"); 3133 return (UriType) this.source; 3134 } 3135 3136 public boolean hasSourceUriType() { 3137 return this.source instanceof UriType; 3138 } 3139 3140 /** 3141 * @return {@link #source} (The source value set that specifies the concepts that are being mapped.) 3142 */ 3143 public Reference getSourceReference() throws FHIRException { 3144 if (this.source == null) 3145 return null; 3146 if (!(this.source instanceof Reference)) 3147 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.source.getClass().getName()+" was encountered"); 3148 return (Reference) this.source; 3149 } 3150 3151 public boolean hasSourceReference() { 3152 return this.source instanceof Reference; 3153 } 3154 3155 public boolean hasSource() { 3156 return this.source != null && !this.source.isEmpty(); 3157 } 3158 3159 /** 3160 * @param value {@link #source} (The source value set that specifies the concepts that are being mapped.) 3161 */ 3162 public ConceptMap setSource(Type value) throws FHIRFormatError { 3163 if (value != null && !(value instanceof UriType || value instanceof Reference)) 3164 throw new FHIRFormatError("Not the right type for ConceptMap.source[x]: "+value.fhirType()); 3165 this.source = value; 3166 return this; 3167 } 3168 3169 /** 3170 * @return {@link #target} (The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.) 3171 */ 3172 public Type getTarget() { 3173 return this.target; 3174 } 3175 3176 /** 3177 * @return {@link #target} (The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.) 3178 */ 3179 public UriType getTargetUriType() throws FHIRException { 3180 if (this.target == null) 3181 return null; 3182 if (!(this.target instanceof UriType)) 3183 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.target.getClass().getName()+" was encountered"); 3184 return (UriType) this.target; 3185 } 3186 3187 public boolean hasTargetUriType() { 3188 return this.target instanceof UriType; 3189 } 3190 3191 /** 3192 * @return {@link #target} (The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.) 3193 */ 3194 public Reference getTargetReference() throws FHIRException { 3195 if (this.target == null) 3196 return null; 3197 if (!(this.target instanceof Reference)) 3198 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.target.getClass().getName()+" was encountered"); 3199 return (Reference) this.target; 3200 } 3201 3202 public boolean hasTargetReference() { 3203 return this.target instanceof Reference; 3204 } 3205 3206 public boolean hasTarget() { 3207 return this.target != null && !this.target.isEmpty(); 3208 } 3209 3210 /** 3211 * @param value {@link #target} (The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.) 3212 */ 3213 public ConceptMap setTarget(Type value) throws FHIRFormatError { 3214 if (value != null && !(value instanceof UriType || value instanceof Reference)) 3215 throw new FHIRFormatError("Not the right type for ConceptMap.target[x]: "+value.fhirType()); 3216 this.target = value; 3217 return this; 3218 } 3219 3220 /** 3221 * @return {@link #group} (A group of mappings that all have the same source and target system.) 3222 */ 3223 public List<ConceptMapGroupComponent> getGroup() { 3224 if (this.group == null) 3225 this.group = new ArrayList<ConceptMapGroupComponent>(); 3226 return this.group; 3227 } 3228 3229 /** 3230 * @return Returns a reference to <code>this</code> for easy method chaining 3231 */ 3232 public ConceptMap setGroup(List<ConceptMapGroupComponent> theGroup) { 3233 this.group = theGroup; 3234 return this; 3235 } 3236 3237 public boolean hasGroup() { 3238 if (this.group == null) 3239 return false; 3240 for (ConceptMapGroupComponent item : this.group) 3241 if (!item.isEmpty()) 3242 return true; 3243 return false; 3244 } 3245 3246 public ConceptMapGroupComponent addGroup() { //3 3247 ConceptMapGroupComponent t = new ConceptMapGroupComponent(); 3248 if (this.group == null) 3249 this.group = new ArrayList<ConceptMapGroupComponent>(); 3250 this.group.add(t); 3251 return t; 3252 } 3253 3254 public ConceptMap addGroup(ConceptMapGroupComponent t) { //3 3255 if (t == null) 3256 return this; 3257 if (this.group == null) 3258 this.group = new ArrayList<ConceptMapGroupComponent>(); 3259 this.group.add(t); 3260 return this; 3261 } 3262 3263 /** 3264 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist 3265 */ 3266 public ConceptMapGroupComponent getGroupFirstRep() { 3267 if (getGroup().isEmpty()) { 3268 addGroup(); 3269 } 3270 return getGroup().get(0); 3271 } 3272 3273 protected void listChildren(List<Property> children) { 3274 super.listChildren(children); 3275 children.add(new Property("url", "uri", "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published. The URL SHOULD include the major version of the concept map. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 3276 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier)); 3277 children.add(new Property("version", "string", "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3278 children.add(new Property("name", "string", "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3279 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the concept map.", 0, 1, title)); 3280 children.add(new Property("status", "code", "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status)); 3281 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3282 children.add(new Property("date", "dateTime", "The date (and optionally time) when the concept map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 0, 1, date)); 3283 children.add(new Property("publisher", "string", "The name of the individual or organization that published the concept map.", 0, 1, publisher)); 3284 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3285 children.add(new Property("description", "markdown", "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, description)); 3286 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate concept map instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3287 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the concept map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3288 children.add(new Property("purpose", "markdown", "Explaination of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose)); 3289 children.add(new Property("copyright", "markdown", "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 0, 1, copyright)); 3290 children.add(new Property("source[x]", "uri|Reference(ValueSet)", "The source value set that specifies the concepts that are being mapped.", 0, 1, source)); 3291 children.add(new Property("target[x]", "uri|Reference(ValueSet)", "The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 0, 1, target)); 3292 children.add(new Property("group", "", "A group of mappings that all have the same source and target system.", 0, java.lang.Integer.MAX_VALUE, group)); 3293 } 3294 3295 @Override 3296 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3297 switch (_hash) { 3298 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published. The URL SHOULD include the major version of the concept map. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 3299 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier); 3300 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3301 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3302 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the concept map.", 0, 1, title); 3303 case -892481550: /*status*/ return new Property("status", "code", "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status); 3304 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3305 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the concept map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 0, 1, date); 3306 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the concept map.", 0, 1, publisher); 3307 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3308 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, description); 3309 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate concept map instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3310 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the concept map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3311 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose); 3312 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 0, 1, copyright); 3313 case -1698413947: /*source[x]*/ return new Property("source[x]", "uri|Reference(ValueSet)", "The source value set that specifies the concepts that are being mapped.", 0, 1, source); 3314 case -896505829: /*source*/ return new Property("source[x]", "uri|Reference(ValueSet)", "The source value set that specifies the concepts that are being mapped.", 0, 1, source); 3315 case -1698419887: /*sourceUri*/ return new Property("source[x]", "uri|Reference(ValueSet)", "The source value set that specifies the concepts that are being mapped.", 0, 1, source); 3316 case -244259472: /*sourceReference*/ return new Property("source[x]", "uri|Reference(ValueSet)", "The source value set that specifies the concepts that are being mapped.", 0, 1, source); 3317 case -815579825: /*target[x]*/ return new Property("target[x]", "uri|Reference(ValueSet)", "The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 0, 1, target); 3318 case -880905839: /*target*/ return new Property("target[x]", "uri|Reference(ValueSet)", "The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 0, 1, target); 3319 case -815585765: /*targetUri*/ return new Property("target[x]", "uri|Reference(ValueSet)", "The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 0, 1, target); 3320 case 1259806906: /*targetReference*/ return new Property("target[x]", "uri|Reference(ValueSet)", "The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 0, 1, target); 3321 case 98629247: /*group*/ return new Property("group", "", "A group of mappings that all have the same source and target system.", 0, java.lang.Integer.MAX_VALUE, group); 3322 default: return super.getNamedProperty(_hash, _name, _checkValid); 3323 } 3324 3325 } 3326 3327 @Override 3328 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3329 switch (hash) { 3330 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3331 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3332 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3333 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3334 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3335 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3336 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3337 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3338 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3339 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3340 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3341 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3342 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3343 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3344 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3345 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Type 3346 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Type 3347 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // ConceptMapGroupComponent 3348 default: return super.getProperty(hash, name, checkValid); 3349 } 3350 3351 } 3352 3353 @Override 3354 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3355 switch (hash) { 3356 case 116079: // url 3357 this.url = castToUri(value); // UriType 3358 return value; 3359 case -1618432855: // identifier 3360 this.identifier = castToIdentifier(value); // Identifier 3361 return value; 3362 case 351608024: // version 3363 this.version = castToString(value); // StringType 3364 return value; 3365 case 3373707: // name 3366 this.name = castToString(value); // StringType 3367 return value; 3368 case 110371416: // title 3369 this.title = castToString(value); // StringType 3370 return value; 3371 case -892481550: // status 3372 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3373 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3374 return value; 3375 case -404562712: // experimental 3376 this.experimental = castToBoolean(value); // BooleanType 3377 return value; 3378 case 3076014: // date 3379 this.date = castToDateTime(value); // DateTimeType 3380 return value; 3381 case 1447404028: // publisher 3382 this.publisher = castToString(value); // StringType 3383 return value; 3384 case 951526432: // contact 3385 this.getContact().add(castToContactDetail(value)); // ContactDetail 3386 return value; 3387 case -1724546052: // description 3388 this.description = castToMarkdown(value); // MarkdownType 3389 return value; 3390 case -669707736: // useContext 3391 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3392 return value; 3393 case -507075711: // jurisdiction 3394 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3395 return value; 3396 case -220463842: // purpose 3397 this.purpose = castToMarkdown(value); // MarkdownType 3398 return value; 3399 case 1522889671: // copyright 3400 this.copyright = castToMarkdown(value); // MarkdownType 3401 return value; 3402 case -896505829: // source 3403 this.source = castToType(value); // Type 3404 return value; 3405 case -880905839: // target 3406 this.target = castToType(value); // Type 3407 return value; 3408 case 98629247: // group 3409 this.getGroup().add((ConceptMapGroupComponent) value); // ConceptMapGroupComponent 3410 return value; 3411 default: return super.setProperty(hash, name, value); 3412 } 3413 3414 } 3415 3416 @Override 3417 public Base setProperty(String name, Base value) throws FHIRException { 3418 if (name.equals("url")) { 3419 this.url = castToUri(value); // UriType 3420 } else if (name.equals("identifier")) { 3421 this.identifier = castToIdentifier(value); // Identifier 3422 } else if (name.equals("version")) { 3423 this.version = castToString(value); // StringType 3424 } else if (name.equals("name")) { 3425 this.name = castToString(value); // StringType 3426 } else if (name.equals("title")) { 3427 this.title = castToString(value); // StringType 3428 } else if (name.equals("status")) { 3429 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3430 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3431 } else if (name.equals("experimental")) { 3432 this.experimental = castToBoolean(value); // BooleanType 3433 } else if (name.equals("date")) { 3434 this.date = castToDateTime(value); // DateTimeType 3435 } else if (name.equals("publisher")) { 3436 this.publisher = castToString(value); // StringType 3437 } else if (name.equals("contact")) { 3438 this.getContact().add(castToContactDetail(value)); 3439 } else if (name.equals("description")) { 3440 this.description = castToMarkdown(value); // MarkdownType 3441 } else if (name.equals("useContext")) { 3442 this.getUseContext().add(castToUsageContext(value)); 3443 } else if (name.equals("jurisdiction")) { 3444 this.getJurisdiction().add(castToCodeableConcept(value)); 3445 } else if (name.equals("purpose")) { 3446 this.purpose = castToMarkdown(value); // MarkdownType 3447 } else if (name.equals("copyright")) { 3448 this.copyright = castToMarkdown(value); // MarkdownType 3449 } else if (name.equals("source[x]")) { 3450 this.source = castToType(value); // Type 3451 } else if (name.equals("target[x]")) { 3452 this.target = castToType(value); // Type 3453 } else if (name.equals("group")) { 3454 this.getGroup().add((ConceptMapGroupComponent) value); 3455 } else 3456 return super.setProperty(name, value); 3457 return value; 3458 } 3459 3460 @Override 3461 public Base makeProperty(int hash, String name) throws FHIRException { 3462 switch (hash) { 3463 case 116079: return getUrlElement(); 3464 case -1618432855: return getIdentifier(); 3465 case 351608024: return getVersionElement(); 3466 case 3373707: return getNameElement(); 3467 case 110371416: return getTitleElement(); 3468 case -892481550: return getStatusElement(); 3469 case -404562712: return getExperimentalElement(); 3470 case 3076014: return getDateElement(); 3471 case 1447404028: return getPublisherElement(); 3472 case 951526432: return addContact(); 3473 case -1724546052: return getDescriptionElement(); 3474 case -669707736: return addUseContext(); 3475 case -507075711: return addJurisdiction(); 3476 case -220463842: return getPurposeElement(); 3477 case 1522889671: return getCopyrightElement(); 3478 case -1698413947: return getSource(); 3479 case -896505829: return getSource(); 3480 case -815579825: return getTarget(); 3481 case -880905839: return getTarget(); 3482 case 98629247: return addGroup(); 3483 default: return super.makeProperty(hash, name); 3484 } 3485 3486 } 3487 3488 @Override 3489 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3490 switch (hash) { 3491 case 116079: /*url*/ return new String[] {"uri"}; 3492 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3493 case 351608024: /*version*/ return new String[] {"string"}; 3494 case 3373707: /*name*/ return new String[] {"string"}; 3495 case 110371416: /*title*/ return new String[] {"string"}; 3496 case -892481550: /*status*/ return new String[] {"code"}; 3497 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3498 case 3076014: /*date*/ return new String[] {"dateTime"}; 3499 case 1447404028: /*publisher*/ return new String[] {"string"}; 3500 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3501 case -1724546052: /*description*/ return new String[] {"markdown"}; 3502 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3503 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3504 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3505 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3506 case -896505829: /*source*/ return new String[] {"uri", "Reference"}; 3507 case -880905839: /*target*/ return new String[] {"uri", "Reference"}; 3508 case 98629247: /*group*/ return new String[] {}; 3509 default: return super.getTypesForProperty(hash, name); 3510 } 3511 3512 } 3513 3514 @Override 3515 public Base addChild(String name) throws FHIRException { 3516 if (name.equals("url")) { 3517 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 3518 } 3519 else if (name.equals("identifier")) { 3520 this.identifier = new Identifier(); 3521 return this.identifier; 3522 } 3523 else if (name.equals("version")) { 3524 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.version"); 3525 } 3526 else if (name.equals("name")) { 3527 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.name"); 3528 } 3529 else if (name.equals("title")) { 3530 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.title"); 3531 } 3532 else if (name.equals("status")) { 3533 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.status"); 3534 } 3535 else if (name.equals("experimental")) { 3536 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.experimental"); 3537 } 3538 else if (name.equals("date")) { 3539 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.date"); 3540 } 3541 else if (name.equals("publisher")) { 3542 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.publisher"); 3543 } 3544 else if (name.equals("contact")) { 3545 return addContact(); 3546 } 3547 else if (name.equals("description")) { 3548 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.description"); 3549 } 3550 else if (name.equals("useContext")) { 3551 return addUseContext(); 3552 } 3553 else if (name.equals("jurisdiction")) { 3554 return addJurisdiction(); 3555 } 3556 else if (name.equals("purpose")) { 3557 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.purpose"); 3558 } 3559 else if (name.equals("copyright")) { 3560 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.copyright"); 3561 } 3562 else if (name.equals("sourceUri")) { 3563 this.source = new UriType(); 3564 return this.source; 3565 } 3566 else if (name.equals("sourceReference")) { 3567 this.source = new Reference(); 3568 return this.source; 3569 } 3570 else if (name.equals("targetUri")) { 3571 this.target = new UriType(); 3572 return this.target; 3573 } 3574 else if (name.equals("targetReference")) { 3575 this.target = new Reference(); 3576 return this.target; 3577 } 3578 else if (name.equals("group")) { 3579 return addGroup(); 3580 } 3581 else 3582 return super.addChild(name); 3583 } 3584 3585 public String fhirType() { 3586 return "ConceptMap"; 3587 3588 } 3589 3590 public ConceptMap copy() { 3591 ConceptMap dst = new ConceptMap(); 3592 copyValues(dst); 3593 dst.url = url == null ? null : url.copy(); 3594 dst.identifier = identifier == null ? null : identifier.copy(); 3595 dst.version = version == null ? null : version.copy(); 3596 dst.name = name == null ? null : name.copy(); 3597 dst.title = title == null ? null : title.copy(); 3598 dst.status = status == null ? null : status.copy(); 3599 dst.experimental = experimental == null ? null : experimental.copy(); 3600 dst.date = date == null ? null : date.copy(); 3601 dst.publisher = publisher == null ? null : publisher.copy(); 3602 if (contact != null) { 3603 dst.contact = new ArrayList<ContactDetail>(); 3604 for (ContactDetail i : contact) 3605 dst.contact.add(i.copy()); 3606 }; 3607 dst.description = description == null ? null : description.copy(); 3608 if (useContext != null) { 3609 dst.useContext = new ArrayList<UsageContext>(); 3610 for (UsageContext i : useContext) 3611 dst.useContext.add(i.copy()); 3612 }; 3613 if (jurisdiction != null) { 3614 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3615 for (CodeableConcept i : jurisdiction) 3616 dst.jurisdiction.add(i.copy()); 3617 }; 3618 dst.purpose = purpose == null ? null : purpose.copy(); 3619 dst.copyright = copyright == null ? null : copyright.copy(); 3620 dst.source = source == null ? null : source.copy(); 3621 dst.target = target == null ? null : target.copy(); 3622 if (group != null) { 3623 dst.group = new ArrayList<ConceptMapGroupComponent>(); 3624 for (ConceptMapGroupComponent i : group) 3625 dst.group.add(i.copy()); 3626 }; 3627 return dst; 3628 } 3629 3630 protected ConceptMap typedCopy() { 3631 return copy(); 3632 } 3633 3634 @Override 3635 public boolean equalsDeep(Base other_) { 3636 if (!super.equalsDeep(other_)) 3637 return false; 3638 if (!(other_ instanceof ConceptMap)) 3639 return false; 3640 ConceptMap o = (ConceptMap) other_; 3641 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 3642 && compareDeep(source, o.source, true) && compareDeep(target, o.target, true) && compareDeep(group, o.group, true) 3643 ; 3644 } 3645 3646 @Override 3647 public boolean equalsShallow(Base other_) { 3648 if (!super.equalsShallow(other_)) 3649 return false; 3650 if (!(other_ instanceof ConceptMap)) 3651 return false; 3652 ConceptMap o = (ConceptMap) other_; 3653 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true); 3654 } 3655 3656 public boolean isEmpty() { 3657 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright 3658 , source, target, group); 3659 } 3660 3661 @Override 3662 public ResourceType getResourceType() { 3663 return ResourceType.ConceptMap; 3664 } 3665 3666 /** 3667 * Search parameter: <b>date</b> 3668 * <p> 3669 * Description: <b>The concept map publication date</b><br> 3670 * Type: <b>date</b><br> 3671 * Path: <b>ConceptMap.date</b><br> 3672 * </p> 3673 */ 3674 @SearchParamDefinition(name="date", path="ConceptMap.date", description="The concept map publication date", type="date" ) 3675 public static final String SP_DATE = "date"; 3676 /** 3677 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3678 * <p> 3679 * Description: <b>The concept map publication date</b><br> 3680 * Type: <b>date</b><br> 3681 * Path: <b>ConceptMap.date</b><br> 3682 * </p> 3683 */ 3684 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3685 3686 /** 3687 * Search parameter: <b>identifier</b> 3688 * <p> 3689 * Description: <b>External identifier for the concept map</b><br> 3690 * Type: <b>token</b><br> 3691 * Path: <b>ConceptMap.identifier</b><br> 3692 * </p> 3693 */ 3694 @SearchParamDefinition(name="identifier", path="ConceptMap.identifier", description="External identifier for the concept map", type="token" ) 3695 public static final String SP_IDENTIFIER = "identifier"; 3696 /** 3697 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3698 * <p> 3699 * Description: <b>External identifier for the concept map</b><br> 3700 * Type: <b>token</b><br> 3701 * Path: <b>ConceptMap.identifier</b><br> 3702 * </p> 3703 */ 3704 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3705 3706 /** 3707 * Search parameter: <b>product</b> 3708 * <p> 3709 * Description: <b>Reference to property mapping depends on</b><br> 3710 * Type: <b>uri</b><br> 3711 * Path: <b>ConceptMap.group.element.target.product.property</b><br> 3712 * </p> 3713 */ 3714 @SearchParamDefinition(name="product", path="ConceptMap.group.element.target.product.property", description="Reference to property mapping depends on", type="uri" ) 3715 public static final String SP_PRODUCT = "product"; 3716 /** 3717 * <b>Fluent Client</b> search parameter constant for <b>product</b> 3718 * <p> 3719 * Description: <b>Reference to property mapping depends on</b><br> 3720 * Type: <b>uri</b><br> 3721 * Path: <b>ConceptMap.group.element.target.product.property</b><br> 3722 * </p> 3723 */ 3724 public static final ca.uhn.fhir.rest.gclient.UriClientParam PRODUCT = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_PRODUCT); 3725 3726 /** 3727 * Search parameter: <b>other</b> 3728 * <p> 3729 * Description: <b>Canonical URL for other concept map</b><br> 3730 * Type: <b>uri</b><br> 3731 * Path: <b>ConceptMap.group.unmapped.url</b><br> 3732 * </p> 3733 */ 3734 @SearchParamDefinition(name="other", path="ConceptMap.group.unmapped.url", description="Canonical URL for other concept map", type="uri" ) 3735 public static final String SP_OTHER = "other"; 3736 /** 3737 * <b>Fluent Client</b> search parameter constant for <b>other</b> 3738 * <p> 3739 * Description: <b>Canonical URL for other concept map</b><br> 3740 * Type: <b>uri</b><br> 3741 * Path: <b>ConceptMap.group.unmapped.url</b><br> 3742 * </p> 3743 */ 3744 public static final ca.uhn.fhir.rest.gclient.UriClientParam OTHER = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_OTHER); 3745 3746 /** 3747 * Search parameter: <b>target-system</b> 3748 * <p> 3749 * Description: <b>System of the target (if necessary)</b><br> 3750 * Type: <b>uri</b><br> 3751 * Path: <b>ConceptMap.group.target</b><br> 3752 * </p> 3753 */ 3754 @SearchParamDefinition(name="target-system", path="ConceptMap.group.target", description="System of the target (if necessary)", type="uri" ) 3755 public static final String SP_TARGET_SYSTEM = "target-system"; 3756 /** 3757 * <b>Fluent Client</b> search parameter constant for <b>target-system</b> 3758 * <p> 3759 * Description: <b>System of the target (if necessary)</b><br> 3760 * Type: <b>uri</b><br> 3761 * Path: <b>ConceptMap.group.target</b><br> 3762 * </p> 3763 */ 3764 public static final ca.uhn.fhir.rest.gclient.UriClientParam TARGET_SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_TARGET_SYSTEM); 3765 3766 /** 3767 * Search parameter: <b>dependson</b> 3768 * <p> 3769 * Description: <b>Reference to property mapping depends on</b><br> 3770 * Type: <b>uri</b><br> 3771 * Path: <b>ConceptMap.group.element.target.dependsOn.property</b><br> 3772 * </p> 3773 */ 3774 @SearchParamDefinition(name="dependson", path="ConceptMap.group.element.target.dependsOn.property", description="Reference to property mapping depends on", type="uri" ) 3775 public static final String SP_DEPENDSON = "dependson"; 3776 /** 3777 * <b>Fluent Client</b> search parameter constant for <b>dependson</b> 3778 * <p> 3779 * Description: <b>Reference to property mapping depends on</b><br> 3780 * Type: <b>uri</b><br> 3781 * Path: <b>ConceptMap.group.element.target.dependsOn.property</b><br> 3782 * </p> 3783 */ 3784 public static final ca.uhn.fhir.rest.gclient.UriClientParam DEPENDSON = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DEPENDSON); 3785 3786 /** 3787 * Search parameter: <b>jurisdiction</b> 3788 * <p> 3789 * Description: <b>Intended jurisdiction for the concept map</b><br> 3790 * Type: <b>token</b><br> 3791 * Path: <b>ConceptMap.jurisdiction</b><br> 3792 * </p> 3793 */ 3794 @SearchParamDefinition(name="jurisdiction", path="ConceptMap.jurisdiction", description="Intended jurisdiction for the concept map", type="token" ) 3795 public static final String SP_JURISDICTION = "jurisdiction"; 3796 /** 3797 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3798 * <p> 3799 * Description: <b>Intended jurisdiction for the concept map</b><br> 3800 * Type: <b>token</b><br> 3801 * Path: <b>ConceptMap.jurisdiction</b><br> 3802 * </p> 3803 */ 3804 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3805 3806 /** 3807 * Search parameter: <b>description</b> 3808 * <p> 3809 * Description: <b>The description of the concept map</b><br> 3810 * Type: <b>string</b><br> 3811 * Path: <b>ConceptMap.description</b><br> 3812 * </p> 3813 */ 3814 @SearchParamDefinition(name="description", path="ConceptMap.description", description="The description of the concept map", type="string" ) 3815 public static final String SP_DESCRIPTION = "description"; 3816 /** 3817 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3818 * <p> 3819 * Description: <b>The description of the concept map</b><br> 3820 * Type: <b>string</b><br> 3821 * Path: <b>ConceptMap.description</b><br> 3822 * </p> 3823 */ 3824 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3825 3826 /** 3827 * Search parameter: <b>source</b> 3828 * <p> 3829 * Description: <b>Identifies the source of the concepts which are being mapped</b><br> 3830 * Type: <b>reference</b><br> 3831 * Path: <b>ConceptMap.sourceReference</b><br> 3832 * </p> 3833 */ 3834 @SearchParamDefinition(name="source", path="ConceptMap.source.as(Reference)", description="Identifies the source of the concepts which are being mapped", type="reference", target={ValueSet.class } ) 3835 public static final String SP_SOURCE = "source"; 3836 /** 3837 * <b>Fluent Client</b> search parameter constant for <b>source</b> 3838 * <p> 3839 * Description: <b>Identifies the source of the concepts which are being mapped</b><br> 3840 * Type: <b>reference</b><br> 3841 * Path: <b>ConceptMap.sourceReference</b><br> 3842 * </p> 3843 */ 3844 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 3845 3846/** 3847 * Constant for fluent queries to be used to add include statements. Specifies 3848 * the path value of "<b>ConceptMap:source</b>". 3849 */ 3850 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("ConceptMap:source").toLocked(); 3851 3852 /** 3853 * Search parameter: <b>title</b> 3854 * <p> 3855 * Description: <b>The human-friendly name of the concept map</b><br> 3856 * Type: <b>string</b><br> 3857 * Path: <b>ConceptMap.title</b><br> 3858 * </p> 3859 */ 3860 @SearchParamDefinition(name="title", path="ConceptMap.title", description="The human-friendly name of the concept map", type="string" ) 3861 public static final String SP_TITLE = "title"; 3862 /** 3863 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3864 * <p> 3865 * Description: <b>The human-friendly name of the concept map</b><br> 3866 * Type: <b>string</b><br> 3867 * Path: <b>ConceptMap.title</b><br> 3868 * </p> 3869 */ 3870 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3871 3872 /** 3873 * Search parameter: <b>version</b> 3874 * <p> 3875 * Description: <b>The business version of the concept map</b><br> 3876 * Type: <b>token</b><br> 3877 * Path: <b>ConceptMap.version</b><br> 3878 * </p> 3879 */ 3880 @SearchParamDefinition(name="version", path="ConceptMap.version", description="The business version of the concept map", type="token" ) 3881 public static final String SP_VERSION = "version"; 3882 /** 3883 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3884 * <p> 3885 * Description: <b>The business version of the concept map</b><br> 3886 * Type: <b>token</b><br> 3887 * Path: <b>ConceptMap.version</b><br> 3888 * </p> 3889 */ 3890 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3891 3892 /** 3893 * Search parameter: <b>url</b> 3894 * <p> 3895 * Description: <b>The uri that identifies the concept map</b><br> 3896 * Type: <b>uri</b><br> 3897 * Path: <b>ConceptMap.url</b><br> 3898 * </p> 3899 */ 3900 @SearchParamDefinition(name="url", path="ConceptMap.url", description="The uri that identifies the concept map", type="uri" ) 3901 public static final String SP_URL = "url"; 3902 /** 3903 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3904 * <p> 3905 * Description: <b>The uri that identifies the concept map</b><br> 3906 * Type: <b>uri</b><br> 3907 * Path: <b>ConceptMap.url</b><br> 3908 * </p> 3909 */ 3910 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3911 3912 /** 3913 * Search parameter: <b>target</b> 3914 * <p> 3915 * Description: <b>Provides context to the mappings</b><br> 3916 * Type: <b>reference</b><br> 3917 * Path: <b>ConceptMap.targetReference</b><br> 3918 * </p> 3919 */ 3920 @SearchParamDefinition(name="target", path="ConceptMap.target.as(Reference)", description="Provides context to the mappings", type="reference", target={ValueSet.class } ) 3921 public static final String SP_TARGET = "target"; 3922 /** 3923 * <b>Fluent Client</b> search parameter constant for <b>target</b> 3924 * <p> 3925 * Description: <b>Provides context to the mappings</b><br> 3926 * Type: <b>reference</b><br> 3927 * Path: <b>ConceptMap.targetReference</b><br> 3928 * </p> 3929 */ 3930 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET); 3931 3932/** 3933 * Constant for fluent queries to be used to add include statements. Specifies 3934 * the path value of "<b>ConceptMap:target</b>". 3935 */ 3936 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include("ConceptMap:target").toLocked(); 3937 3938 /** 3939 * Search parameter: <b>source-code</b> 3940 * <p> 3941 * Description: <b>Identifies element being mapped</b><br> 3942 * Type: <b>token</b><br> 3943 * Path: <b>ConceptMap.group.element.code</b><br> 3944 * </p> 3945 */ 3946 @SearchParamDefinition(name="source-code", path="ConceptMap.group.element.code", description="Identifies element being mapped", type="token" ) 3947 public static final String SP_SOURCE_CODE = "source-code"; 3948 /** 3949 * <b>Fluent Client</b> search parameter constant for <b>source-code</b> 3950 * <p> 3951 * Description: <b>Identifies element being mapped</b><br> 3952 * Type: <b>token</b><br> 3953 * Path: <b>ConceptMap.group.element.code</b><br> 3954 * </p> 3955 */ 3956 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SOURCE_CODE); 3957 3958 /** 3959 * Search parameter: <b>source-uri</b> 3960 * <p> 3961 * Description: <b>Identifies the source of the concepts which are being mapped</b><br> 3962 * Type: <b>reference</b><br> 3963 * Path: <b>ConceptMap.sourceUri</b><br> 3964 * </p> 3965 */ 3966 @SearchParamDefinition(name="source-uri", path="ConceptMap.source.as(Uri)", description="Identifies the source of the concepts which are being mapped", type="reference", target={ValueSet.class } ) 3967 public static final String SP_SOURCE_URI = "source-uri"; 3968 /** 3969 * <b>Fluent Client</b> search parameter constant for <b>source-uri</b> 3970 * <p> 3971 * Description: <b>Identifies the source of the concepts which are being mapped</b><br> 3972 * Type: <b>reference</b><br> 3973 * Path: <b>ConceptMap.sourceUri</b><br> 3974 * </p> 3975 */ 3976 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_URI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE_URI); 3977 3978/** 3979 * Constant for fluent queries to be used to add include statements. Specifies 3980 * the path value of "<b>ConceptMap:source-uri</b>". 3981 */ 3982 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_URI = new ca.uhn.fhir.model.api.Include("ConceptMap:source-uri").toLocked(); 3983 3984 /** 3985 * Search parameter: <b>name</b> 3986 * <p> 3987 * Description: <b>Computationally friendly name of the concept map</b><br> 3988 * Type: <b>string</b><br> 3989 * Path: <b>ConceptMap.name</b><br> 3990 * </p> 3991 */ 3992 @SearchParamDefinition(name="name", path="ConceptMap.name", description="Computationally friendly name of the concept map", type="string" ) 3993 public static final String SP_NAME = "name"; 3994 /** 3995 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3996 * <p> 3997 * Description: <b>Computationally friendly name of the concept map</b><br> 3998 * Type: <b>string</b><br> 3999 * Path: <b>ConceptMap.name</b><br> 4000 * </p> 4001 */ 4002 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4003 4004 /** 4005 * Search parameter: <b>publisher</b> 4006 * <p> 4007 * Description: <b>Name of the publisher of the concept map</b><br> 4008 * Type: <b>string</b><br> 4009 * Path: <b>ConceptMap.publisher</b><br> 4010 * </p> 4011 */ 4012 @SearchParamDefinition(name="publisher", path="ConceptMap.publisher", description="Name of the publisher of the concept map", type="string" ) 4013 public static final String SP_PUBLISHER = "publisher"; 4014 /** 4015 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4016 * <p> 4017 * Description: <b>Name of the publisher of the concept map</b><br> 4018 * Type: <b>string</b><br> 4019 * Path: <b>ConceptMap.publisher</b><br> 4020 * </p> 4021 */ 4022 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4023 4024 /** 4025 * Search parameter: <b>source-system</b> 4026 * <p> 4027 * Description: <b>Code System (if value set crosses code systems)</b><br> 4028 * Type: <b>uri</b><br> 4029 * Path: <b>ConceptMap.group.source</b><br> 4030 * </p> 4031 */ 4032 @SearchParamDefinition(name="source-system", path="ConceptMap.group.source", description="Code System (if value set crosses code systems)", type="uri" ) 4033 public static final String SP_SOURCE_SYSTEM = "source-system"; 4034 /** 4035 * <b>Fluent Client</b> search parameter constant for <b>source-system</b> 4036 * <p> 4037 * Description: <b>Code System (if value set crosses code systems)</b><br> 4038 * Type: <b>uri</b><br> 4039 * Path: <b>ConceptMap.group.source</b><br> 4040 * </p> 4041 */ 4042 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE_SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SOURCE_SYSTEM); 4043 4044 /** 4045 * Search parameter: <b>target-code</b> 4046 * <p> 4047 * Description: <b>Code that identifies the target element</b><br> 4048 * Type: <b>token</b><br> 4049 * Path: <b>ConceptMap.group.element.target.code</b><br> 4050 * </p> 4051 */ 4052 @SearchParamDefinition(name="target-code", path="ConceptMap.group.element.target.code", description="Code that identifies the target element", type="token" ) 4053 public static final String SP_TARGET_CODE = "target-code"; 4054 /** 4055 * <b>Fluent Client</b> search parameter constant for <b>target-code</b> 4056 * <p> 4057 * Description: <b>Code that identifies the target element</b><br> 4058 * Type: <b>token</b><br> 4059 * Path: <b>ConceptMap.group.element.target.code</b><br> 4060 * </p> 4061 */ 4062 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_CODE); 4063 4064 /** 4065 * Search parameter: <b>status</b> 4066 * <p> 4067 * Description: <b>The current status of the concept map</b><br> 4068 * Type: <b>token</b><br> 4069 * Path: <b>ConceptMap.status</b><br> 4070 * </p> 4071 */ 4072 @SearchParamDefinition(name="status", path="ConceptMap.status", description="The current status of the concept map", type="token" ) 4073 public static final String SP_STATUS = "status"; 4074 /** 4075 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4076 * <p> 4077 * Description: <b>The current status of the concept map</b><br> 4078 * Type: <b>token</b><br> 4079 * Path: <b>ConceptMap.status</b><br> 4080 * </p> 4081 */ 4082 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4083 4084 /** 4085 * Search parameter: <b>target-uri</b> 4086 * <p> 4087 * Description: <b>Provides context to the mappings</b><br> 4088 * Type: <b>reference</b><br> 4089 * Path: <b>ConceptMap.targetUri</b><br> 4090 * </p> 4091 */ 4092 @SearchParamDefinition(name="target-uri", path="ConceptMap.target.as(Uri)", description="Provides context to the mappings", type="reference", target={ValueSet.class } ) 4093 public static final String SP_TARGET_URI = "target-uri"; 4094 /** 4095 * <b>Fluent Client</b> search parameter constant for <b>target-uri</b> 4096 * <p> 4097 * Description: <b>Provides context to the mappings</b><br> 4098 * Type: <b>reference</b><br> 4099 * Path: <b>ConceptMap.targetUri</b><br> 4100 * </p> 4101 */ 4102 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET_URI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET_URI); 4103 4104/** 4105 * Constant for fluent queries to be used to add include statements. Specifies 4106 * the path value of "<b>ConceptMap:target-uri</b>". 4107 */ 4108 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET_URI = new ca.uhn.fhir.model.api.Include("ConceptMap:target-uri").toLocked(); 4109 4110 4111}