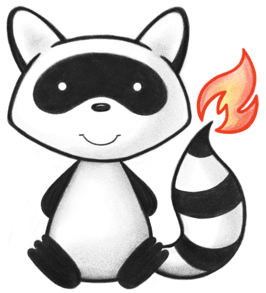
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 050 */ 051@ResourceDef(name="Condition", profile="http://hl7.org/fhir/Profile/Condition") 052public class Condition extends DomainResource { 053 054 public enum ConditionClinicalStatus { 055 /** 056 * The subject is currently experiencing the symptoms of the condition or there is evidence of the condition. 057 */ 058 ACTIVE, 059 /** 060 * The subject is having a relapse or re-experiencing the condition after a period of remission or presumed resolution. 061 */ 062 RECURRENCE, 063 /** 064 * The subject is no longer experiencing the symptoms of the condition or there is no longer evidence of the condition. 065 */ 066 INACTIVE, 067 /** 068 * The subject is no longer experiencing the symptoms of the condition, but there is a risk of the symptoms returning. 069 */ 070 REMISSION, 071 /** 072 * The subject is no longer experiencing the symptoms of the condition and there is a negligible perceived risk of the symptoms returning. 073 */ 074 RESOLVED, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static ConditionClinicalStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("active".equals(codeString)) 083 return ACTIVE; 084 if ("recurrence".equals(codeString)) 085 return RECURRENCE; 086 if ("inactive".equals(codeString)) 087 return INACTIVE; 088 if ("remission".equals(codeString)) 089 return REMISSION; 090 if ("resolved".equals(codeString)) 091 return RESOLVED; 092 if (Configuration.isAcceptInvalidEnums()) 093 return null; 094 else 095 throw new FHIRException("Unknown ConditionClinicalStatus code '"+codeString+"'"); 096 } 097 public String toCode() { 098 switch (this) { 099 case ACTIVE: return "active"; 100 case RECURRENCE: return "recurrence"; 101 case INACTIVE: return "inactive"; 102 case REMISSION: return "remission"; 103 case RESOLVED: return "resolved"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getSystem() { 109 switch (this) { 110 case ACTIVE: return "http://hl7.org/fhir/condition-clinical"; 111 case RECURRENCE: return "http://hl7.org/fhir/condition-clinical"; 112 case INACTIVE: return "http://hl7.org/fhir/condition-clinical"; 113 case REMISSION: return "http://hl7.org/fhir/condition-clinical"; 114 case RESOLVED: return "http://hl7.org/fhir/condition-clinical"; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getDefinition() { 120 switch (this) { 121 case ACTIVE: return "The subject is currently experiencing the symptoms of the condition or there is evidence of the condition."; 122 case RECURRENCE: return "The subject is having a relapse or re-experiencing the condition after a period of remission or presumed resolution."; 123 case INACTIVE: return "The subject is no longer experiencing the symptoms of the condition or there is no longer evidence of the condition."; 124 case REMISSION: return "The subject is no longer experiencing the symptoms of the condition, but there is a risk of the symptoms returning."; 125 case RESOLVED: return "The subject is no longer experiencing the symptoms of the condition and there is a negligible perceived risk of the symptoms returning."; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case ACTIVE: return "Active"; 133 case RECURRENCE: return "Recurrence"; 134 case INACTIVE: return "Inactive"; 135 case REMISSION: return "Remission"; 136 case RESOLVED: return "Resolved"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 } 142 143 public static class ConditionClinicalStatusEnumFactory implements EnumFactory<ConditionClinicalStatus> { 144 public ConditionClinicalStatus fromCode(String codeString) throws IllegalArgumentException { 145 if (codeString == null || "".equals(codeString)) 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("active".equals(codeString)) 149 return ConditionClinicalStatus.ACTIVE; 150 if ("recurrence".equals(codeString)) 151 return ConditionClinicalStatus.RECURRENCE; 152 if ("inactive".equals(codeString)) 153 return ConditionClinicalStatus.INACTIVE; 154 if ("remission".equals(codeString)) 155 return ConditionClinicalStatus.REMISSION; 156 if ("resolved".equals(codeString)) 157 return ConditionClinicalStatus.RESOLVED; 158 throw new IllegalArgumentException("Unknown ConditionClinicalStatus code '"+codeString+"'"); 159 } 160 public Enumeration<ConditionClinicalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 161 if (code == null) 162 return null; 163 if (code.isEmpty()) 164 return new Enumeration<ConditionClinicalStatus>(this); 165 String codeString = code.asStringValue(); 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("active".equals(codeString)) 169 return new Enumeration<ConditionClinicalStatus>(this, ConditionClinicalStatus.ACTIVE); 170 if ("recurrence".equals(codeString)) 171 return new Enumeration<ConditionClinicalStatus>(this, ConditionClinicalStatus.RECURRENCE); 172 if ("inactive".equals(codeString)) 173 return new Enumeration<ConditionClinicalStatus>(this, ConditionClinicalStatus.INACTIVE); 174 if ("remission".equals(codeString)) 175 return new Enumeration<ConditionClinicalStatus>(this, ConditionClinicalStatus.REMISSION); 176 if ("resolved".equals(codeString)) 177 return new Enumeration<ConditionClinicalStatus>(this, ConditionClinicalStatus.RESOLVED); 178 throw new FHIRException("Unknown ConditionClinicalStatus code '"+codeString+"'"); 179 } 180 public String toCode(ConditionClinicalStatus code) { 181 if (code == ConditionClinicalStatus.NULL) 182 return null; 183 if (code == ConditionClinicalStatus.ACTIVE) 184 return "active"; 185 if (code == ConditionClinicalStatus.RECURRENCE) 186 return "recurrence"; 187 if (code == ConditionClinicalStatus.INACTIVE) 188 return "inactive"; 189 if (code == ConditionClinicalStatus.REMISSION) 190 return "remission"; 191 if (code == ConditionClinicalStatus.RESOLVED) 192 return "resolved"; 193 return "?"; 194 } 195 public String toSystem(ConditionClinicalStatus code) { 196 return code.getSystem(); 197 } 198 } 199 200 public enum ConditionVerificationStatus { 201 /** 202 * This is a tentative diagnosis - still a candidate that is under consideration. 203 */ 204 PROVISIONAL, 205 /** 206 * One of a set of potential (and typically mutually exclusive) diagnoses asserted to further guide the diagnostic process and preliminary treatment. 207 */ 208 DIFFERENTIAL, 209 /** 210 * There is sufficient diagnostic and/or clinical evidence to treat this as a confirmed condition. 211 */ 212 CONFIRMED, 213 /** 214 * This condition has been ruled out by diagnostic and clinical evidence. 215 */ 216 REFUTED, 217 /** 218 * The statement was entered in error and is not valid. 219 */ 220 ENTEREDINERROR, 221 /** 222 * The condition status is unknown. Note that "unknown" is a value of last resort and every attempt should be made to provide a meaningful value other than "unknown". 223 */ 224 UNKNOWN, 225 /** 226 * added to help the parsers with the generic types 227 */ 228 NULL; 229 public static ConditionVerificationStatus fromCode(String codeString) throws FHIRException { 230 if (codeString == null || "".equals(codeString)) 231 return null; 232 if ("provisional".equals(codeString)) 233 return PROVISIONAL; 234 if ("differential".equals(codeString)) 235 return DIFFERENTIAL; 236 if ("confirmed".equals(codeString)) 237 return CONFIRMED; 238 if ("refuted".equals(codeString)) 239 return REFUTED; 240 if ("entered-in-error".equals(codeString)) 241 return ENTEREDINERROR; 242 if ("unknown".equals(codeString)) 243 return UNKNOWN; 244 if (Configuration.isAcceptInvalidEnums()) 245 return null; 246 else 247 throw new FHIRException("Unknown ConditionVerificationStatus code '"+codeString+"'"); 248 } 249 public String toCode() { 250 switch (this) { 251 case PROVISIONAL: return "provisional"; 252 case DIFFERENTIAL: return "differential"; 253 case CONFIRMED: return "confirmed"; 254 case REFUTED: return "refuted"; 255 case ENTEREDINERROR: return "entered-in-error"; 256 case UNKNOWN: return "unknown"; 257 case NULL: return null; 258 default: return "?"; 259 } 260 } 261 public String getSystem() { 262 switch (this) { 263 case PROVISIONAL: return "http://hl7.org/fhir/condition-ver-status"; 264 case DIFFERENTIAL: return "http://hl7.org/fhir/condition-ver-status"; 265 case CONFIRMED: return "http://hl7.org/fhir/condition-ver-status"; 266 case REFUTED: return "http://hl7.org/fhir/condition-ver-status"; 267 case ENTEREDINERROR: return "http://hl7.org/fhir/condition-ver-status"; 268 case UNKNOWN: return "http://hl7.org/fhir/condition-ver-status"; 269 case NULL: return null; 270 default: return "?"; 271 } 272 } 273 public String getDefinition() { 274 switch (this) { 275 case PROVISIONAL: return "This is a tentative diagnosis - still a candidate that is under consideration."; 276 case DIFFERENTIAL: return "One of a set of potential (and typically mutually exclusive) diagnoses asserted to further guide the diagnostic process and preliminary treatment."; 277 case CONFIRMED: return "There is sufficient diagnostic and/or clinical evidence to treat this as a confirmed condition."; 278 case REFUTED: return "This condition has been ruled out by diagnostic and clinical evidence."; 279 case ENTEREDINERROR: return "The statement was entered in error and is not valid."; 280 case UNKNOWN: return "The condition status is unknown. Note that \"unknown\" is a value of last resort and every attempt should be made to provide a meaningful value other than \"unknown\"."; 281 case NULL: return null; 282 default: return "?"; 283 } 284 } 285 public String getDisplay() { 286 switch (this) { 287 case PROVISIONAL: return "Provisional"; 288 case DIFFERENTIAL: return "Differential"; 289 case CONFIRMED: return "Confirmed"; 290 case REFUTED: return "Refuted"; 291 case ENTEREDINERROR: return "Entered In Error"; 292 case UNKNOWN: return "Unknown"; 293 case NULL: return null; 294 default: return "?"; 295 } 296 } 297 } 298 299 public static class ConditionVerificationStatusEnumFactory implements EnumFactory<ConditionVerificationStatus> { 300 public ConditionVerificationStatus fromCode(String codeString) throws IllegalArgumentException { 301 if (codeString == null || "".equals(codeString)) 302 if (codeString == null || "".equals(codeString)) 303 return null; 304 if ("provisional".equals(codeString)) 305 return ConditionVerificationStatus.PROVISIONAL; 306 if ("differential".equals(codeString)) 307 return ConditionVerificationStatus.DIFFERENTIAL; 308 if ("confirmed".equals(codeString)) 309 return ConditionVerificationStatus.CONFIRMED; 310 if ("refuted".equals(codeString)) 311 return ConditionVerificationStatus.REFUTED; 312 if ("entered-in-error".equals(codeString)) 313 return ConditionVerificationStatus.ENTEREDINERROR; 314 if ("unknown".equals(codeString)) 315 return ConditionVerificationStatus.UNKNOWN; 316 throw new IllegalArgumentException("Unknown ConditionVerificationStatus code '"+codeString+"'"); 317 } 318 public Enumeration<ConditionVerificationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 319 if (code == null) 320 return null; 321 if (code.isEmpty()) 322 return new Enumeration<ConditionVerificationStatus>(this); 323 String codeString = code.asStringValue(); 324 if (codeString == null || "".equals(codeString)) 325 return null; 326 if ("provisional".equals(codeString)) 327 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.PROVISIONAL); 328 if ("differential".equals(codeString)) 329 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.DIFFERENTIAL); 330 if ("confirmed".equals(codeString)) 331 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.CONFIRMED); 332 if ("refuted".equals(codeString)) 333 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.REFUTED); 334 if ("entered-in-error".equals(codeString)) 335 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.ENTEREDINERROR); 336 if ("unknown".equals(codeString)) 337 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.UNKNOWN); 338 throw new FHIRException("Unknown ConditionVerificationStatus code '"+codeString+"'"); 339 } 340 public String toCode(ConditionVerificationStatus code) { 341 if (code == ConditionVerificationStatus.NULL) 342 return null; 343 if (code == ConditionVerificationStatus.PROVISIONAL) 344 return "provisional"; 345 if (code == ConditionVerificationStatus.DIFFERENTIAL) 346 return "differential"; 347 if (code == ConditionVerificationStatus.CONFIRMED) 348 return "confirmed"; 349 if (code == ConditionVerificationStatus.REFUTED) 350 return "refuted"; 351 if (code == ConditionVerificationStatus.ENTEREDINERROR) 352 return "entered-in-error"; 353 if (code == ConditionVerificationStatus.UNKNOWN) 354 return "unknown"; 355 return "?"; 356 } 357 public String toSystem(ConditionVerificationStatus code) { 358 return code.getSystem(); 359 } 360 } 361 362 @Block() 363 public static class ConditionStageComponent extends BackboneElement implements IBaseBackboneElement { 364 /** 365 * A simple summary of the stage such as "Stage 3". The determination of the stage is disease-specific. 366 */ 367 @Child(name = "summary", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 368 @Description(shortDefinition="Simple summary (disease specific)", formalDefinition="A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific." ) 369 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-stage") 370 protected CodeableConcept summary; 371 372 /** 373 * Reference to a formal record of the evidence on which the staging assessment is based. 374 */ 375 @Child(name = "assessment", type = {ClinicalImpression.class, DiagnosticReport.class, Observation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 376 @Description(shortDefinition="Formal record of assessment", formalDefinition="Reference to a formal record of the evidence on which the staging assessment is based." ) 377 protected List<Reference> assessment; 378 /** 379 * The actual objects that are the target of the reference (Reference to a formal record of the evidence on which the staging assessment is based.) 380 */ 381 protected List<Resource> assessmentTarget; 382 383 384 private static final long serialVersionUID = -1961530405L; 385 386 /** 387 * Constructor 388 */ 389 public ConditionStageComponent() { 390 super(); 391 } 392 393 /** 394 * @return {@link #summary} (A simple summary of the stage such as "Stage 3". The determination of the stage is disease-specific.) 395 */ 396 public CodeableConcept getSummary() { 397 if (this.summary == null) 398 if (Configuration.errorOnAutoCreate()) 399 throw new Error("Attempt to auto-create ConditionStageComponent.summary"); 400 else if (Configuration.doAutoCreate()) 401 this.summary = new CodeableConcept(); // cc 402 return this.summary; 403 } 404 405 public boolean hasSummary() { 406 return this.summary != null && !this.summary.isEmpty(); 407 } 408 409 /** 410 * @param value {@link #summary} (A simple summary of the stage such as "Stage 3". The determination of the stage is disease-specific.) 411 */ 412 public ConditionStageComponent setSummary(CodeableConcept value) { 413 this.summary = value; 414 return this; 415 } 416 417 /** 418 * @return {@link #assessment} (Reference to a formal record of the evidence on which the staging assessment is based.) 419 */ 420 public List<Reference> getAssessment() { 421 if (this.assessment == null) 422 this.assessment = new ArrayList<Reference>(); 423 return this.assessment; 424 } 425 426 /** 427 * @return Returns a reference to <code>this</code> for easy method chaining 428 */ 429 public ConditionStageComponent setAssessment(List<Reference> theAssessment) { 430 this.assessment = theAssessment; 431 return this; 432 } 433 434 public boolean hasAssessment() { 435 if (this.assessment == null) 436 return false; 437 for (Reference item : this.assessment) 438 if (!item.isEmpty()) 439 return true; 440 return false; 441 } 442 443 public Reference addAssessment() { //3 444 Reference t = new Reference(); 445 if (this.assessment == null) 446 this.assessment = new ArrayList<Reference>(); 447 this.assessment.add(t); 448 return t; 449 } 450 451 public ConditionStageComponent addAssessment(Reference t) { //3 452 if (t == null) 453 return this; 454 if (this.assessment == null) 455 this.assessment = new ArrayList<Reference>(); 456 this.assessment.add(t); 457 return this; 458 } 459 460 /** 461 * @return The first repetition of repeating field {@link #assessment}, creating it if it does not already exist 462 */ 463 public Reference getAssessmentFirstRep() { 464 if (getAssessment().isEmpty()) { 465 addAssessment(); 466 } 467 return getAssessment().get(0); 468 } 469 470 /** 471 * @deprecated Use Reference#setResource(IBaseResource) instead 472 */ 473 @Deprecated 474 public List<Resource> getAssessmentTarget() { 475 if (this.assessmentTarget == null) 476 this.assessmentTarget = new ArrayList<Resource>(); 477 return this.assessmentTarget; 478 } 479 480 protected void listChildren(List<Property> children) { 481 super.listChildren(children); 482 children.add(new Property("summary", "CodeableConcept", "A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific.", 0, 1, summary)); 483 children.add(new Property("assessment", "Reference(ClinicalImpression|DiagnosticReport|Observation)", "Reference to a formal record of the evidence on which the staging assessment is based.", 0, java.lang.Integer.MAX_VALUE, assessment)); 484 } 485 486 @Override 487 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 488 switch (_hash) { 489 case -1857640538: /*summary*/ return new Property("summary", "CodeableConcept", "A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific.", 0, 1, summary); 490 case 2119382722: /*assessment*/ return new Property("assessment", "Reference(ClinicalImpression|DiagnosticReport|Observation)", "Reference to a formal record of the evidence on which the staging assessment is based.", 0, java.lang.Integer.MAX_VALUE, assessment); 491 default: return super.getNamedProperty(_hash, _name, _checkValid); 492 } 493 494 } 495 496 @Override 497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 498 switch (hash) { 499 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : new Base[] {this.summary}; // CodeableConcept 500 case 2119382722: /*assessment*/ return this.assessment == null ? new Base[0] : this.assessment.toArray(new Base[this.assessment.size()]); // Reference 501 default: return super.getProperty(hash, name, checkValid); 502 } 503 504 } 505 506 @Override 507 public Base setProperty(int hash, String name, Base value) throws FHIRException { 508 switch (hash) { 509 case -1857640538: // summary 510 this.summary = castToCodeableConcept(value); // CodeableConcept 511 return value; 512 case 2119382722: // assessment 513 this.getAssessment().add(castToReference(value)); // Reference 514 return value; 515 default: return super.setProperty(hash, name, value); 516 } 517 518 } 519 520 @Override 521 public Base setProperty(String name, Base value) throws FHIRException { 522 if (name.equals("summary")) { 523 this.summary = castToCodeableConcept(value); // CodeableConcept 524 } else if (name.equals("assessment")) { 525 this.getAssessment().add(castToReference(value)); 526 } else 527 return super.setProperty(name, value); 528 return value; 529 } 530 531 @Override 532 public Base makeProperty(int hash, String name) throws FHIRException { 533 switch (hash) { 534 case -1857640538: return getSummary(); 535 case 2119382722: return addAssessment(); 536 default: return super.makeProperty(hash, name); 537 } 538 539 } 540 541 @Override 542 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 543 switch (hash) { 544 case -1857640538: /*summary*/ return new String[] {"CodeableConcept"}; 545 case 2119382722: /*assessment*/ return new String[] {"Reference"}; 546 default: return super.getTypesForProperty(hash, name); 547 } 548 549 } 550 551 @Override 552 public Base addChild(String name) throws FHIRException { 553 if (name.equals("summary")) { 554 this.summary = new CodeableConcept(); 555 return this.summary; 556 } 557 else if (name.equals("assessment")) { 558 return addAssessment(); 559 } 560 else 561 return super.addChild(name); 562 } 563 564 public ConditionStageComponent copy() { 565 ConditionStageComponent dst = new ConditionStageComponent(); 566 copyValues(dst); 567 dst.summary = summary == null ? null : summary.copy(); 568 if (assessment != null) { 569 dst.assessment = new ArrayList<Reference>(); 570 for (Reference i : assessment) 571 dst.assessment.add(i.copy()); 572 }; 573 return dst; 574 } 575 576 @Override 577 public boolean equalsDeep(Base other_) { 578 if (!super.equalsDeep(other_)) 579 return false; 580 if (!(other_ instanceof ConditionStageComponent)) 581 return false; 582 ConditionStageComponent o = (ConditionStageComponent) other_; 583 return compareDeep(summary, o.summary, true) && compareDeep(assessment, o.assessment, true); 584 } 585 586 @Override 587 public boolean equalsShallow(Base other_) { 588 if (!super.equalsShallow(other_)) 589 return false; 590 if (!(other_ instanceof ConditionStageComponent)) 591 return false; 592 ConditionStageComponent o = (ConditionStageComponent) other_; 593 return true; 594 } 595 596 public boolean isEmpty() { 597 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(summary, assessment); 598 } 599 600 public String fhirType() { 601 return "Condition.stage"; 602 603 } 604 605 } 606 607 @Block() 608 public static class ConditionEvidenceComponent extends BackboneElement implements IBaseBackboneElement { 609 /** 610 * A manifestation or symptom that led to the recording of this condition. 611 */ 612 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 613 @Description(shortDefinition="Manifestation/symptom", formalDefinition="A manifestation or symptom that led to the recording of this condition." ) 614 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/manifestation-or-symptom") 615 protected List<CodeableConcept> code; 616 617 /** 618 * Links to other relevant information, including pathology reports. 619 */ 620 @Child(name = "detail", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 621 @Description(shortDefinition="Supporting information found elsewhere", formalDefinition="Links to other relevant information, including pathology reports." ) 622 protected List<Reference> detail; 623 /** 624 * The actual objects that are the target of the reference (Links to other relevant information, including pathology reports.) 625 */ 626 protected List<Resource> detailTarget; 627 628 629 private static final long serialVersionUID = 1135831276L; 630 631 /** 632 * Constructor 633 */ 634 public ConditionEvidenceComponent() { 635 super(); 636 } 637 638 /** 639 * @return {@link #code} (A manifestation or symptom that led to the recording of this condition.) 640 */ 641 public List<CodeableConcept> getCode() { 642 if (this.code == null) 643 this.code = new ArrayList<CodeableConcept>(); 644 return this.code; 645 } 646 647 /** 648 * @return Returns a reference to <code>this</code> for easy method chaining 649 */ 650 public ConditionEvidenceComponent setCode(List<CodeableConcept> theCode) { 651 this.code = theCode; 652 return this; 653 } 654 655 public boolean hasCode() { 656 if (this.code == null) 657 return false; 658 for (CodeableConcept item : this.code) 659 if (!item.isEmpty()) 660 return true; 661 return false; 662 } 663 664 public CodeableConcept addCode() { //3 665 CodeableConcept t = new CodeableConcept(); 666 if (this.code == null) 667 this.code = new ArrayList<CodeableConcept>(); 668 this.code.add(t); 669 return t; 670 } 671 672 public ConditionEvidenceComponent addCode(CodeableConcept t) { //3 673 if (t == null) 674 return this; 675 if (this.code == null) 676 this.code = new ArrayList<CodeableConcept>(); 677 this.code.add(t); 678 return this; 679 } 680 681 /** 682 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 683 */ 684 public CodeableConcept getCodeFirstRep() { 685 if (getCode().isEmpty()) { 686 addCode(); 687 } 688 return getCode().get(0); 689 } 690 691 /** 692 * @return {@link #detail} (Links to other relevant information, including pathology reports.) 693 */ 694 public List<Reference> getDetail() { 695 if (this.detail == null) 696 this.detail = new ArrayList<Reference>(); 697 return this.detail; 698 } 699 700 /** 701 * @return Returns a reference to <code>this</code> for easy method chaining 702 */ 703 public ConditionEvidenceComponent setDetail(List<Reference> theDetail) { 704 this.detail = theDetail; 705 return this; 706 } 707 708 public boolean hasDetail() { 709 if (this.detail == null) 710 return false; 711 for (Reference item : this.detail) 712 if (!item.isEmpty()) 713 return true; 714 return false; 715 } 716 717 public Reference addDetail() { //3 718 Reference t = new Reference(); 719 if (this.detail == null) 720 this.detail = new ArrayList<Reference>(); 721 this.detail.add(t); 722 return t; 723 } 724 725 public ConditionEvidenceComponent addDetail(Reference t) { //3 726 if (t == null) 727 return this; 728 if (this.detail == null) 729 this.detail = new ArrayList<Reference>(); 730 this.detail.add(t); 731 return this; 732 } 733 734 /** 735 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 736 */ 737 public Reference getDetailFirstRep() { 738 if (getDetail().isEmpty()) { 739 addDetail(); 740 } 741 return getDetail().get(0); 742 } 743 744 /** 745 * @deprecated Use Reference#setResource(IBaseResource) instead 746 */ 747 @Deprecated 748 public List<Resource> getDetailTarget() { 749 if (this.detailTarget == null) 750 this.detailTarget = new ArrayList<Resource>(); 751 return this.detailTarget; 752 } 753 754 protected void listChildren(List<Property> children) { 755 super.listChildren(children); 756 children.add(new Property("code", "CodeableConcept", "A manifestation or symptom that led to the recording of this condition.", 0, java.lang.Integer.MAX_VALUE, code)); 757 children.add(new Property("detail", "Reference(Any)", "Links to other relevant information, including pathology reports.", 0, java.lang.Integer.MAX_VALUE, detail)); 758 } 759 760 @Override 761 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 762 switch (_hash) { 763 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A manifestation or symptom that led to the recording of this condition.", 0, java.lang.Integer.MAX_VALUE, code); 764 case -1335224239: /*detail*/ return new Property("detail", "Reference(Any)", "Links to other relevant information, including pathology reports.", 0, java.lang.Integer.MAX_VALUE, detail); 765 default: return super.getNamedProperty(_hash, _name, _checkValid); 766 } 767 768 } 769 770 @Override 771 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 772 switch (hash) { 773 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 774 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 775 default: return super.getProperty(hash, name, checkValid); 776 } 777 778 } 779 780 @Override 781 public Base setProperty(int hash, String name, Base value) throws FHIRException { 782 switch (hash) { 783 case 3059181: // code 784 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 785 return value; 786 case -1335224239: // detail 787 this.getDetail().add(castToReference(value)); // Reference 788 return value; 789 default: return super.setProperty(hash, name, value); 790 } 791 792 } 793 794 @Override 795 public Base setProperty(String name, Base value) throws FHIRException { 796 if (name.equals("code")) { 797 this.getCode().add(castToCodeableConcept(value)); 798 } else if (name.equals("detail")) { 799 this.getDetail().add(castToReference(value)); 800 } else 801 return super.setProperty(name, value); 802 return value; 803 } 804 805 @Override 806 public Base makeProperty(int hash, String name) throws FHIRException { 807 switch (hash) { 808 case 3059181: return addCode(); 809 case -1335224239: return addDetail(); 810 default: return super.makeProperty(hash, name); 811 } 812 813 } 814 815 @Override 816 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 817 switch (hash) { 818 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 819 case -1335224239: /*detail*/ return new String[] {"Reference"}; 820 default: return super.getTypesForProperty(hash, name); 821 } 822 823 } 824 825 @Override 826 public Base addChild(String name) throws FHIRException { 827 if (name.equals("code")) { 828 return addCode(); 829 } 830 else if (name.equals("detail")) { 831 return addDetail(); 832 } 833 else 834 return super.addChild(name); 835 } 836 837 public ConditionEvidenceComponent copy() { 838 ConditionEvidenceComponent dst = new ConditionEvidenceComponent(); 839 copyValues(dst); 840 if (code != null) { 841 dst.code = new ArrayList<CodeableConcept>(); 842 for (CodeableConcept i : code) 843 dst.code.add(i.copy()); 844 }; 845 if (detail != null) { 846 dst.detail = new ArrayList<Reference>(); 847 for (Reference i : detail) 848 dst.detail.add(i.copy()); 849 }; 850 return dst; 851 } 852 853 @Override 854 public boolean equalsDeep(Base other_) { 855 if (!super.equalsDeep(other_)) 856 return false; 857 if (!(other_ instanceof ConditionEvidenceComponent)) 858 return false; 859 ConditionEvidenceComponent o = (ConditionEvidenceComponent) other_; 860 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 861 } 862 863 @Override 864 public boolean equalsShallow(Base other_) { 865 if (!super.equalsShallow(other_)) 866 return false; 867 if (!(other_ instanceof ConditionEvidenceComponent)) 868 return false; 869 ConditionEvidenceComponent o = (ConditionEvidenceComponent) other_; 870 return true; 871 } 872 873 public boolean isEmpty() { 874 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, detail); 875 } 876 877 public String fhirType() { 878 return "Condition.evidence"; 879 880 } 881 882 } 883 884 /** 885 * This records identifiers associated with this condition that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 886 */ 887 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 888 @Description(shortDefinition="External Ids for this condition", formalDefinition="This records identifiers associated with this condition that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 889 protected List<Identifier> identifier; 890 891 /** 892 * The clinical status of the condition. 893 */ 894 @Child(name = "clinicalStatus", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 895 @Description(shortDefinition="active | recurrence | inactive | remission | resolved", formalDefinition="The clinical status of the condition." ) 896 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-clinical") 897 protected Enumeration<ConditionClinicalStatus> clinicalStatus; 898 899 /** 900 * The verification status to support the clinical status of the condition. 901 */ 902 @Child(name = "verificationStatus", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 903 @Description(shortDefinition="provisional | differential | confirmed | refuted | entered-in-error | unknown", formalDefinition="The verification status to support the clinical status of the condition." ) 904 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-ver-status") 905 protected Enumeration<ConditionVerificationStatus> verificationStatus; 906 907 /** 908 * A category assigned to the condition. 909 */ 910 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 911 @Description(shortDefinition="problem-list-item | encounter-diagnosis", formalDefinition="A category assigned to the condition." ) 912 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-category") 913 protected List<CodeableConcept> category; 914 915 /** 916 * A subjective assessment of the severity of the condition as evaluated by the clinician. 917 */ 918 @Child(name = "severity", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 919 @Description(shortDefinition="Subjective severity of condition", formalDefinition="A subjective assessment of the severity of the condition as evaluated by the clinician." ) 920 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-severity") 921 protected CodeableConcept severity; 922 923 /** 924 * Identification of the condition, problem or diagnosis. 925 */ 926 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 927 @Description(shortDefinition="Identification of the condition, problem or diagnosis", formalDefinition="Identification of the condition, problem or diagnosis." ) 928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 929 protected CodeableConcept code; 930 931 /** 932 * The anatomical location where this condition manifests itself. 933 */ 934 @Child(name = "bodySite", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 935 @Description(shortDefinition="Anatomical location, if relevant", formalDefinition="The anatomical location where this condition manifests itself." ) 936 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 937 protected List<CodeableConcept> bodySite; 938 939 /** 940 * Indicates the patient or group who the condition record is associated with. 941 */ 942 @Child(name = "subject", type = {Patient.class, Group.class}, order=7, min=1, max=1, modifier=false, summary=true) 943 @Description(shortDefinition="Who has the condition?", formalDefinition="Indicates the patient or group who the condition record is associated with." ) 944 protected Reference subject; 945 946 /** 947 * The actual object that is the target of the reference (Indicates the patient or group who the condition record is associated with.) 948 */ 949 protected Resource subjectTarget; 950 951 /** 952 * Encounter during which the condition was first asserted. 953 */ 954 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=8, min=0, max=1, modifier=false, summary=true) 955 @Description(shortDefinition="Encounter or episode when condition first asserted", formalDefinition="Encounter during which the condition was first asserted." ) 956 protected Reference context; 957 958 /** 959 * The actual object that is the target of the reference (Encounter during which the condition was first asserted.) 960 */ 961 protected Resource contextTarget; 962 963 /** 964 * Estimated or actual date or date-time the condition began, in the opinion of the clinician. 965 */ 966 @Child(name = "onset", type = {DateTimeType.class, Age.class, Period.class, Range.class, StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 967 @Description(shortDefinition="Estimated or actual date, date-time, or age", formalDefinition="Estimated or actual date or date-time the condition began, in the opinion of the clinician." ) 968 protected Type onset; 969 970 /** 971 * The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate. 972 */ 973 @Child(name = "abatement", type = {DateTimeType.class, Age.class, BooleanType.class, Period.class, Range.class, StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 974 @Description(shortDefinition="If/when in resolution/remission", formalDefinition="The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate." ) 975 protected Type abatement; 976 977 /** 978 * The date on which the existance of the Condition was first asserted or acknowledged. 979 */ 980 @Child(name = "assertedDate", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 981 @Description(shortDefinition="Date record was believed accurate", formalDefinition="The date on which the existance of the Condition was first asserted or acknowledged." ) 982 protected DateTimeType assertedDate; 983 984 /** 985 * Individual who is making the condition statement. 986 */ 987 @Child(name = "asserter", type = {Practitioner.class, Patient.class, RelatedPerson.class}, order=12, min=0, max=1, modifier=false, summary=true) 988 @Description(shortDefinition="Person who asserts this condition", formalDefinition="Individual who is making the condition statement." ) 989 protected Reference asserter; 990 991 /** 992 * The actual object that is the target of the reference (Individual who is making the condition statement.) 993 */ 994 protected Resource asserterTarget; 995 996 /** 997 * Clinical stage or grade of a condition. May include formal severity assessments. 998 */ 999 @Child(name = "stage", type = {}, order=13, min=0, max=1, modifier=false, summary=false) 1000 @Description(shortDefinition="Stage/grade, usually assessed formally", formalDefinition="Clinical stage or grade of a condition. May include formal severity assessments." ) 1001 protected ConditionStageComponent stage; 1002 1003 /** 1004 * Supporting Evidence / manifestations that are the basis on which this condition is suspected or confirmed. 1005 */ 1006 @Child(name = "evidence", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1007 @Description(shortDefinition="Supporting evidence", formalDefinition="Supporting Evidence / manifestations that are the basis on which this condition is suspected or confirmed." ) 1008 protected List<ConditionEvidenceComponent> evidence; 1009 1010 /** 1011 * Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis. 1012 */ 1013 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1014 @Description(shortDefinition="Additional information about the Condition", formalDefinition="Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis." ) 1015 protected List<Annotation> note; 1016 1017 private static final long serialVersionUID = -585250376L; 1018 1019 /** 1020 * Constructor 1021 */ 1022 public Condition() { 1023 super(); 1024 } 1025 1026 /** 1027 * Constructor 1028 */ 1029 public Condition(Reference subject) { 1030 super(); 1031 this.subject = subject; 1032 } 1033 1034 /** 1035 * @return {@link #identifier} (This records identifiers associated with this condition that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 1036 */ 1037 public List<Identifier> getIdentifier() { 1038 if (this.identifier == null) 1039 this.identifier = new ArrayList<Identifier>(); 1040 return this.identifier; 1041 } 1042 1043 /** 1044 * @return Returns a reference to <code>this</code> for easy method chaining 1045 */ 1046 public Condition setIdentifier(List<Identifier> theIdentifier) { 1047 this.identifier = theIdentifier; 1048 return this; 1049 } 1050 1051 public boolean hasIdentifier() { 1052 if (this.identifier == null) 1053 return false; 1054 for (Identifier item : this.identifier) 1055 if (!item.isEmpty()) 1056 return true; 1057 return false; 1058 } 1059 1060 public Identifier addIdentifier() { //3 1061 Identifier t = new Identifier(); 1062 if (this.identifier == null) 1063 this.identifier = new ArrayList<Identifier>(); 1064 this.identifier.add(t); 1065 return t; 1066 } 1067 1068 public Condition addIdentifier(Identifier t) { //3 1069 if (t == null) 1070 return this; 1071 if (this.identifier == null) 1072 this.identifier = new ArrayList<Identifier>(); 1073 this.identifier.add(t); 1074 return this; 1075 } 1076 1077 /** 1078 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1079 */ 1080 public Identifier getIdentifierFirstRep() { 1081 if (getIdentifier().isEmpty()) { 1082 addIdentifier(); 1083 } 1084 return getIdentifier().get(0); 1085 } 1086 1087 /** 1088 * @return {@link #clinicalStatus} (The clinical status of the condition.). This is the underlying object with id, value and extensions. The accessor "getClinicalStatus" gives direct access to the value 1089 */ 1090 public Enumeration<ConditionClinicalStatus> getClinicalStatusElement() { 1091 if (this.clinicalStatus == null) 1092 if (Configuration.errorOnAutoCreate()) 1093 throw new Error("Attempt to auto-create Condition.clinicalStatus"); 1094 else if (Configuration.doAutoCreate()) 1095 this.clinicalStatus = new Enumeration<ConditionClinicalStatus>(new ConditionClinicalStatusEnumFactory()); // bb 1096 return this.clinicalStatus; 1097 } 1098 1099 public boolean hasClinicalStatusElement() { 1100 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1101 } 1102 1103 public boolean hasClinicalStatus() { 1104 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #clinicalStatus} (The clinical status of the condition.). This is the underlying object with id, value and extensions. The accessor "getClinicalStatus" gives direct access to the value 1109 */ 1110 public Condition setClinicalStatusElement(Enumeration<ConditionClinicalStatus> value) { 1111 this.clinicalStatus = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return The clinical status of the condition. 1117 */ 1118 public ConditionClinicalStatus getClinicalStatus() { 1119 return this.clinicalStatus == null ? null : this.clinicalStatus.getValue(); 1120 } 1121 1122 /** 1123 * @param value The clinical status of the condition. 1124 */ 1125 public Condition setClinicalStatus(ConditionClinicalStatus value) { 1126 if (value == null) 1127 this.clinicalStatus = null; 1128 else { 1129 if (this.clinicalStatus == null) 1130 this.clinicalStatus = new Enumeration<ConditionClinicalStatus>(new ConditionClinicalStatusEnumFactory()); 1131 this.clinicalStatus.setValue(value); 1132 } 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #verificationStatus} (The verification status to support the clinical status of the condition.). This is the underlying object with id, value and extensions. The accessor "getVerificationStatus" gives direct access to the value 1138 */ 1139 public Enumeration<ConditionVerificationStatus> getVerificationStatusElement() { 1140 if (this.verificationStatus == null) 1141 if (Configuration.errorOnAutoCreate()) 1142 throw new Error("Attempt to auto-create Condition.verificationStatus"); 1143 else if (Configuration.doAutoCreate()) 1144 this.verificationStatus = new Enumeration<ConditionVerificationStatus>(new ConditionVerificationStatusEnumFactory()); // bb 1145 return this.verificationStatus; 1146 } 1147 1148 public boolean hasVerificationStatusElement() { 1149 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1150 } 1151 1152 public boolean hasVerificationStatus() { 1153 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1154 } 1155 1156 /** 1157 * @param value {@link #verificationStatus} (The verification status to support the clinical status of the condition.). This is the underlying object with id, value and extensions. The accessor "getVerificationStatus" gives direct access to the value 1158 */ 1159 public Condition setVerificationStatusElement(Enumeration<ConditionVerificationStatus> value) { 1160 this.verificationStatus = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return The verification status to support the clinical status of the condition. 1166 */ 1167 public ConditionVerificationStatus getVerificationStatus() { 1168 return this.verificationStatus == null ? null : this.verificationStatus.getValue(); 1169 } 1170 1171 /** 1172 * @param value The verification status to support the clinical status of the condition. 1173 */ 1174 public Condition setVerificationStatus(ConditionVerificationStatus value) { 1175 if (value == null) 1176 this.verificationStatus = null; 1177 else { 1178 if (this.verificationStatus == null) 1179 this.verificationStatus = new Enumeration<ConditionVerificationStatus>(new ConditionVerificationStatusEnumFactory()); 1180 this.verificationStatus.setValue(value); 1181 } 1182 return this; 1183 } 1184 1185 /** 1186 * @return {@link #category} (A category assigned to the condition.) 1187 */ 1188 public List<CodeableConcept> getCategory() { 1189 if (this.category == null) 1190 this.category = new ArrayList<CodeableConcept>(); 1191 return this.category; 1192 } 1193 1194 /** 1195 * @return Returns a reference to <code>this</code> for easy method chaining 1196 */ 1197 public Condition setCategory(List<CodeableConcept> theCategory) { 1198 this.category = theCategory; 1199 return this; 1200 } 1201 1202 public boolean hasCategory() { 1203 if (this.category == null) 1204 return false; 1205 for (CodeableConcept item : this.category) 1206 if (!item.isEmpty()) 1207 return true; 1208 return false; 1209 } 1210 1211 public CodeableConcept addCategory() { //3 1212 CodeableConcept t = new CodeableConcept(); 1213 if (this.category == null) 1214 this.category = new ArrayList<CodeableConcept>(); 1215 this.category.add(t); 1216 return t; 1217 } 1218 1219 public Condition addCategory(CodeableConcept t) { //3 1220 if (t == null) 1221 return this; 1222 if (this.category == null) 1223 this.category = new ArrayList<CodeableConcept>(); 1224 this.category.add(t); 1225 return this; 1226 } 1227 1228 /** 1229 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 1230 */ 1231 public CodeableConcept getCategoryFirstRep() { 1232 if (getCategory().isEmpty()) { 1233 addCategory(); 1234 } 1235 return getCategory().get(0); 1236 } 1237 1238 /** 1239 * @return {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 1240 */ 1241 public CodeableConcept getSeverity() { 1242 if (this.severity == null) 1243 if (Configuration.errorOnAutoCreate()) 1244 throw new Error("Attempt to auto-create Condition.severity"); 1245 else if (Configuration.doAutoCreate()) 1246 this.severity = new CodeableConcept(); // cc 1247 return this.severity; 1248 } 1249 1250 public boolean hasSeverity() { 1251 return this.severity != null && !this.severity.isEmpty(); 1252 } 1253 1254 /** 1255 * @param value {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 1256 */ 1257 public Condition setSeverity(CodeableConcept value) { 1258 this.severity = value; 1259 return this; 1260 } 1261 1262 /** 1263 * @return {@link #code} (Identification of the condition, problem or diagnosis.) 1264 */ 1265 public CodeableConcept getCode() { 1266 if (this.code == null) 1267 if (Configuration.errorOnAutoCreate()) 1268 throw new Error("Attempt to auto-create Condition.code"); 1269 else if (Configuration.doAutoCreate()) 1270 this.code = new CodeableConcept(); // cc 1271 return this.code; 1272 } 1273 1274 public boolean hasCode() { 1275 return this.code != null && !this.code.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #code} (Identification of the condition, problem or diagnosis.) 1280 */ 1281 public Condition setCode(CodeableConcept value) { 1282 this.code = value; 1283 return this; 1284 } 1285 1286 /** 1287 * @return {@link #bodySite} (The anatomical location where this condition manifests itself.) 1288 */ 1289 public List<CodeableConcept> getBodySite() { 1290 if (this.bodySite == null) 1291 this.bodySite = new ArrayList<CodeableConcept>(); 1292 return this.bodySite; 1293 } 1294 1295 /** 1296 * @return Returns a reference to <code>this</code> for easy method chaining 1297 */ 1298 public Condition setBodySite(List<CodeableConcept> theBodySite) { 1299 this.bodySite = theBodySite; 1300 return this; 1301 } 1302 1303 public boolean hasBodySite() { 1304 if (this.bodySite == null) 1305 return false; 1306 for (CodeableConcept item : this.bodySite) 1307 if (!item.isEmpty()) 1308 return true; 1309 return false; 1310 } 1311 1312 public CodeableConcept addBodySite() { //3 1313 CodeableConcept t = new CodeableConcept(); 1314 if (this.bodySite == null) 1315 this.bodySite = new ArrayList<CodeableConcept>(); 1316 this.bodySite.add(t); 1317 return t; 1318 } 1319 1320 public Condition addBodySite(CodeableConcept t) { //3 1321 if (t == null) 1322 return this; 1323 if (this.bodySite == null) 1324 this.bodySite = new ArrayList<CodeableConcept>(); 1325 this.bodySite.add(t); 1326 return this; 1327 } 1328 1329 /** 1330 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist 1331 */ 1332 public CodeableConcept getBodySiteFirstRep() { 1333 if (getBodySite().isEmpty()) { 1334 addBodySite(); 1335 } 1336 return getBodySite().get(0); 1337 } 1338 1339 /** 1340 * @return {@link #subject} (Indicates the patient or group who the condition record is associated with.) 1341 */ 1342 public Reference getSubject() { 1343 if (this.subject == null) 1344 if (Configuration.errorOnAutoCreate()) 1345 throw new Error("Attempt to auto-create Condition.subject"); 1346 else if (Configuration.doAutoCreate()) 1347 this.subject = new Reference(); // cc 1348 return this.subject; 1349 } 1350 1351 public boolean hasSubject() { 1352 return this.subject != null && !this.subject.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #subject} (Indicates the patient or group who the condition record is associated with.) 1357 */ 1358 public Condition setSubject(Reference value) { 1359 this.subject = value; 1360 return this; 1361 } 1362 1363 /** 1364 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the patient or group who the condition record is associated with.) 1365 */ 1366 public Resource getSubjectTarget() { 1367 return this.subjectTarget; 1368 } 1369 1370 /** 1371 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the patient or group who the condition record is associated with.) 1372 */ 1373 public Condition setSubjectTarget(Resource value) { 1374 this.subjectTarget = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #context} (Encounter during which the condition was first asserted.) 1380 */ 1381 public Reference getContext() { 1382 if (this.context == null) 1383 if (Configuration.errorOnAutoCreate()) 1384 throw new Error("Attempt to auto-create Condition.context"); 1385 else if (Configuration.doAutoCreate()) 1386 this.context = new Reference(); // cc 1387 return this.context; 1388 } 1389 1390 public boolean hasContext() { 1391 return this.context != null && !this.context.isEmpty(); 1392 } 1393 1394 /** 1395 * @param value {@link #context} (Encounter during which the condition was first asserted.) 1396 */ 1397 public Condition setContext(Reference value) { 1398 this.context = value; 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Encounter during which the condition was first asserted.) 1404 */ 1405 public Resource getContextTarget() { 1406 return this.contextTarget; 1407 } 1408 1409 /** 1410 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Encounter during which the condition was first asserted.) 1411 */ 1412 public Condition setContextTarget(Resource value) { 1413 this.contextTarget = value; 1414 return this; 1415 } 1416 1417 /** 1418 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1419 */ 1420 public Type getOnset() { 1421 return this.onset; 1422 } 1423 1424 /** 1425 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1426 */ 1427 public DateTimeType getOnsetDateTimeType() throws FHIRException { 1428 if (this.onset == null) 1429 return null; 1430 if (!(this.onset instanceof DateTimeType)) 1431 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1432 return (DateTimeType) this.onset; 1433 } 1434 1435 public boolean hasOnsetDateTimeType() { 1436 return this != null && this.onset instanceof DateTimeType; 1437 } 1438 1439 /** 1440 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1441 */ 1442 public Age getOnsetAge() throws FHIRException { 1443 if (this.onset == null) 1444 return null; 1445 if (!(this.onset instanceof Age)) 1446 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.onset.getClass().getName()+" was encountered"); 1447 return (Age) this.onset; 1448 } 1449 1450 public boolean hasOnsetAge() { 1451 return this != null && this.onset instanceof Age; 1452 } 1453 1454 /** 1455 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1456 */ 1457 public Period getOnsetPeriod() throws FHIRException { 1458 if (this.onset == null) 1459 return null; 1460 if (!(this.onset instanceof Period)) 1461 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.onset.getClass().getName()+" was encountered"); 1462 return (Period) this.onset; 1463 } 1464 1465 public boolean hasOnsetPeriod() { 1466 return this != null && this.onset instanceof Period; 1467 } 1468 1469 /** 1470 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1471 */ 1472 public Range getOnsetRange() throws FHIRException { 1473 if (this.onset == null) 1474 return null; 1475 if (!(this.onset instanceof Range)) 1476 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.onset.getClass().getName()+" was encountered"); 1477 return (Range) this.onset; 1478 } 1479 1480 public boolean hasOnsetRange() { 1481 return this != null && this.onset instanceof Range; 1482 } 1483 1484 /** 1485 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1486 */ 1487 public StringType getOnsetStringType() throws FHIRException { 1488 if (this.onset == null) 1489 return null; 1490 if (!(this.onset instanceof StringType)) 1491 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1492 return (StringType) this.onset; 1493 } 1494 1495 public boolean hasOnsetStringType() { 1496 return this != null && this.onset instanceof StringType; 1497 } 1498 1499 public boolean hasOnset() { 1500 return this.onset != null && !this.onset.isEmpty(); 1501 } 1502 1503 /** 1504 * @param value {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1505 */ 1506 public Condition setOnset(Type value) throws FHIRFormatError { 1507 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period || value instanceof Range || value instanceof StringType)) 1508 throw new FHIRFormatError("Not the right type for Condition.onset[x]: "+value.fhirType()); 1509 this.onset = value; 1510 return this; 1511 } 1512 1513 /** 1514 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1515 */ 1516 public Type getAbatement() { 1517 return this.abatement; 1518 } 1519 1520 /** 1521 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1522 */ 1523 public DateTimeType getAbatementDateTimeType() throws FHIRException { 1524 if (this.abatement == null) 1525 return null; 1526 if (!(this.abatement instanceof DateTimeType)) 1527 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1528 return (DateTimeType) this.abatement; 1529 } 1530 1531 public boolean hasAbatementDateTimeType() { 1532 return this != null && this.abatement instanceof DateTimeType; 1533 } 1534 1535 /** 1536 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1537 */ 1538 public Age getAbatementAge() throws FHIRException { 1539 if (this.abatement == null) 1540 return null; 1541 if (!(this.abatement instanceof Age)) 1542 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1543 return (Age) this.abatement; 1544 } 1545 1546 public boolean hasAbatementAge() { 1547 return this != null && this.abatement instanceof Age; 1548 } 1549 1550 /** 1551 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1552 */ 1553 public BooleanType getAbatementBooleanType() throws FHIRException { 1554 if (this.abatement == null) 1555 return null; 1556 if (!(this.abatement instanceof BooleanType)) 1557 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1558 return (BooleanType) this.abatement; 1559 } 1560 1561 public boolean hasAbatementBooleanType() { 1562 return this != null && this.abatement instanceof BooleanType; 1563 } 1564 1565 /** 1566 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1567 */ 1568 public Period getAbatementPeriod() throws FHIRException { 1569 if (this.abatement == null) 1570 return null; 1571 if (!(this.abatement instanceof Period)) 1572 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1573 return (Period) this.abatement; 1574 } 1575 1576 public boolean hasAbatementPeriod() { 1577 return this != null && this.abatement instanceof Period; 1578 } 1579 1580 /** 1581 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1582 */ 1583 public Range getAbatementRange() throws FHIRException { 1584 if (this.abatement == null) 1585 return null; 1586 if (!(this.abatement instanceof Range)) 1587 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1588 return (Range) this.abatement; 1589 } 1590 1591 public boolean hasAbatementRange() { 1592 return this != null && this.abatement instanceof Range; 1593 } 1594 1595 /** 1596 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1597 */ 1598 public StringType getAbatementStringType() throws FHIRException { 1599 if (this.abatement == null) 1600 return null; 1601 if (!(this.abatement instanceof StringType)) 1602 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1603 return (StringType) this.abatement; 1604 } 1605 1606 public boolean hasAbatementStringType() { 1607 return this != null && this.abatement instanceof StringType; 1608 } 1609 1610 public boolean hasAbatement() { 1611 return this.abatement != null && !this.abatement.isEmpty(); 1612 } 1613 1614 /** 1615 * @param value {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Conditions are never really resolved, but they can abate.) 1616 */ 1617 public Condition setAbatement(Type value) throws FHIRFormatError { 1618 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof BooleanType || value instanceof Period || value instanceof Range || value instanceof StringType)) 1619 throw new FHIRFormatError("Not the right type for Condition.abatement[x]: "+value.fhirType()); 1620 this.abatement = value; 1621 return this; 1622 } 1623 1624 /** 1625 * @return {@link #assertedDate} (The date on which the existance of the Condition was first asserted or acknowledged.). This is the underlying object with id, value and extensions. The accessor "getAssertedDate" gives direct access to the value 1626 */ 1627 public DateTimeType getAssertedDateElement() { 1628 if (this.assertedDate == null) 1629 if (Configuration.errorOnAutoCreate()) 1630 throw new Error("Attempt to auto-create Condition.assertedDate"); 1631 else if (Configuration.doAutoCreate()) 1632 this.assertedDate = new DateTimeType(); // bb 1633 return this.assertedDate; 1634 } 1635 1636 public boolean hasAssertedDateElement() { 1637 return this.assertedDate != null && !this.assertedDate.isEmpty(); 1638 } 1639 1640 public boolean hasAssertedDate() { 1641 return this.assertedDate != null && !this.assertedDate.isEmpty(); 1642 } 1643 1644 /** 1645 * @param value {@link #assertedDate} (The date on which the existance of the Condition was first asserted or acknowledged.). This is the underlying object with id, value and extensions. The accessor "getAssertedDate" gives direct access to the value 1646 */ 1647 public Condition setAssertedDateElement(DateTimeType value) { 1648 this.assertedDate = value; 1649 return this; 1650 } 1651 1652 /** 1653 * @return The date on which the existance of the Condition was first asserted or acknowledged. 1654 */ 1655 public Date getAssertedDate() { 1656 return this.assertedDate == null ? null : this.assertedDate.getValue(); 1657 } 1658 1659 /** 1660 * @param value The date on which the existance of the Condition was first asserted or acknowledged. 1661 */ 1662 public Condition setAssertedDate(Date value) { 1663 if (value == null) 1664 this.assertedDate = null; 1665 else { 1666 if (this.assertedDate == null) 1667 this.assertedDate = new DateTimeType(); 1668 this.assertedDate.setValue(value); 1669 } 1670 return this; 1671 } 1672 1673 /** 1674 * @return {@link #asserter} (Individual who is making the condition statement.) 1675 */ 1676 public Reference getAsserter() { 1677 if (this.asserter == null) 1678 if (Configuration.errorOnAutoCreate()) 1679 throw new Error("Attempt to auto-create Condition.asserter"); 1680 else if (Configuration.doAutoCreate()) 1681 this.asserter = new Reference(); // cc 1682 return this.asserter; 1683 } 1684 1685 public boolean hasAsserter() { 1686 return this.asserter != null && !this.asserter.isEmpty(); 1687 } 1688 1689 /** 1690 * @param value {@link #asserter} (Individual who is making the condition statement.) 1691 */ 1692 public Condition setAsserter(Reference value) { 1693 this.asserter = value; 1694 return this; 1695 } 1696 1697 /** 1698 * @return {@link #asserter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Individual who is making the condition statement.) 1699 */ 1700 public Resource getAsserterTarget() { 1701 return this.asserterTarget; 1702 } 1703 1704 /** 1705 * @param value {@link #asserter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Individual who is making the condition statement.) 1706 */ 1707 public Condition setAsserterTarget(Resource value) { 1708 this.asserterTarget = value; 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #stage} (Clinical stage or grade of a condition. May include formal severity assessments.) 1714 */ 1715 public ConditionStageComponent getStage() { 1716 if (this.stage == null) 1717 if (Configuration.errorOnAutoCreate()) 1718 throw new Error("Attempt to auto-create Condition.stage"); 1719 else if (Configuration.doAutoCreate()) 1720 this.stage = new ConditionStageComponent(); // cc 1721 return this.stage; 1722 } 1723 1724 public boolean hasStage() { 1725 return this.stage != null && !this.stage.isEmpty(); 1726 } 1727 1728 /** 1729 * @param value {@link #stage} (Clinical stage or grade of a condition. May include formal severity assessments.) 1730 */ 1731 public Condition setStage(ConditionStageComponent value) { 1732 this.stage = value; 1733 return this; 1734 } 1735 1736 /** 1737 * @return {@link #evidence} (Supporting Evidence / manifestations that are the basis on which this condition is suspected or confirmed.) 1738 */ 1739 public List<ConditionEvidenceComponent> getEvidence() { 1740 if (this.evidence == null) 1741 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1742 return this.evidence; 1743 } 1744 1745 /** 1746 * @return Returns a reference to <code>this</code> for easy method chaining 1747 */ 1748 public Condition setEvidence(List<ConditionEvidenceComponent> theEvidence) { 1749 this.evidence = theEvidence; 1750 return this; 1751 } 1752 1753 public boolean hasEvidence() { 1754 if (this.evidence == null) 1755 return false; 1756 for (ConditionEvidenceComponent item : this.evidence) 1757 if (!item.isEmpty()) 1758 return true; 1759 return false; 1760 } 1761 1762 public ConditionEvidenceComponent addEvidence() { //3 1763 ConditionEvidenceComponent t = new ConditionEvidenceComponent(); 1764 if (this.evidence == null) 1765 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1766 this.evidence.add(t); 1767 return t; 1768 } 1769 1770 public Condition addEvidence(ConditionEvidenceComponent t) { //3 1771 if (t == null) 1772 return this; 1773 if (this.evidence == null) 1774 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1775 this.evidence.add(t); 1776 return this; 1777 } 1778 1779 /** 1780 * @return The first repetition of repeating field {@link #evidence}, creating it if it does not already exist 1781 */ 1782 public ConditionEvidenceComponent getEvidenceFirstRep() { 1783 if (getEvidence().isEmpty()) { 1784 addEvidence(); 1785 } 1786 return getEvidence().get(0); 1787 } 1788 1789 /** 1790 * @return {@link #note} (Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.) 1791 */ 1792 public List<Annotation> getNote() { 1793 if (this.note == null) 1794 this.note = new ArrayList<Annotation>(); 1795 return this.note; 1796 } 1797 1798 /** 1799 * @return Returns a reference to <code>this</code> for easy method chaining 1800 */ 1801 public Condition setNote(List<Annotation> theNote) { 1802 this.note = theNote; 1803 return this; 1804 } 1805 1806 public boolean hasNote() { 1807 if (this.note == null) 1808 return false; 1809 for (Annotation item : this.note) 1810 if (!item.isEmpty()) 1811 return true; 1812 return false; 1813 } 1814 1815 public Annotation addNote() { //3 1816 Annotation t = new Annotation(); 1817 if (this.note == null) 1818 this.note = new ArrayList<Annotation>(); 1819 this.note.add(t); 1820 return t; 1821 } 1822 1823 public Condition addNote(Annotation t) { //3 1824 if (t == null) 1825 return this; 1826 if (this.note == null) 1827 this.note = new ArrayList<Annotation>(); 1828 this.note.add(t); 1829 return this; 1830 } 1831 1832 /** 1833 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1834 */ 1835 public Annotation getNoteFirstRep() { 1836 if (getNote().isEmpty()) { 1837 addNote(); 1838 } 1839 return getNote().get(0); 1840 } 1841 1842 protected void listChildren(List<Property> children) { 1843 super.listChildren(children); 1844 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this condition that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 1845 children.add(new Property("clinicalStatus", "code", "The clinical status of the condition.", 0, 1, clinicalStatus)); 1846 children.add(new Property("verificationStatus", "code", "The verification status to support the clinical status of the condition.", 0, 1, verificationStatus)); 1847 children.add(new Property("category", "CodeableConcept", "A category assigned to the condition.", 0, java.lang.Integer.MAX_VALUE, category)); 1848 children.add(new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity)); 1849 children.add(new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code)); 1850 children.add(new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 1851 children.add(new Property("subject", "Reference(Patient|Group)", "Indicates the patient or group who the condition record is associated with.", 0, 1, subject)); 1852 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "Encounter during which the condition was first asserted.", 0, 1, context)); 1853 children.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset)); 1854 children.add(new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement)); 1855 children.add(new Property("assertedDate", "dateTime", "The date on which the existance of the Condition was first asserted or acknowledged.", 0, 1, assertedDate)); 1856 children.add(new Property("asserter", "Reference(Practitioner|Patient|RelatedPerson)", "Individual who is making the condition statement.", 0, 1, asserter)); 1857 children.add(new Property("stage", "", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1, stage)); 1858 children.add(new Property("evidence", "", "Supporting Evidence / manifestations that are the basis on which this condition is suspected or confirmed.", 0, java.lang.Integer.MAX_VALUE, evidence)); 1859 children.add(new Property("note", "Annotation", "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.", 0, java.lang.Integer.MAX_VALUE, note)); 1860 } 1861 1862 @Override 1863 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1864 switch (_hash) { 1865 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this condition that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 1866 case -462853915: /*clinicalStatus*/ return new Property("clinicalStatus", "code", "The clinical status of the condition.", 0, 1, clinicalStatus); 1867 case -842509843: /*verificationStatus*/ return new Property("verificationStatus", "code", "The verification status to support the clinical status of the condition.", 0, 1, verificationStatus); 1868 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A category assigned to the condition.", 0, java.lang.Integer.MAX_VALUE, category); 1869 case 1478300413: /*severity*/ return new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity); 1870 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code); 1871 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, java.lang.Integer.MAX_VALUE, bodySite); 1872 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "Indicates the patient or group who the condition record is associated with.", 0, 1, subject); 1873 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "Encounter during which the condition was first asserted.", 0, 1, context); 1874 case -1886216323: /*onset[x]*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1875 case 105901603: /*onset*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1876 case -1701663010: /*onsetDateTime*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1877 case -1886241828: /*onsetAge*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1878 case -1545082428: /*onsetPeriod*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1879 case -186664742: /*onsetRange*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1880 case -1445342188: /*onsetString*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1881 case -584196495: /*abatement[x]*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1882 case -921554001: /*abatement*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1883 case 44869738: /*abatementDateTime*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1884 case -584222000: /*abatementAge*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1885 case -2069881479: /*abatementBoolean*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1886 case -922036656: /*abatementPeriod*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1887 case 1218906830: /*abatementRange*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1888 case -822296416: /*abatementString*/ return new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 0, 1, abatement); 1889 case -174231629: /*assertedDate*/ return new Property("assertedDate", "dateTime", "The date on which the existance of the Condition was first asserted or acknowledged.", 0, 1, assertedDate); 1890 case -373242253: /*asserter*/ return new Property("asserter", "Reference(Practitioner|Patient|RelatedPerson)", "Individual who is making the condition statement.", 0, 1, asserter); 1891 case 109757182: /*stage*/ return new Property("stage", "", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1, stage); 1892 case 382967383: /*evidence*/ return new Property("evidence", "", "Supporting Evidence / manifestations that are the basis on which this condition is suspected or confirmed.", 0, java.lang.Integer.MAX_VALUE, evidence); 1893 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.", 0, java.lang.Integer.MAX_VALUE, note); 1894 default: return super.getNamedProperty(_hash, _name, _checkValid); 1895 } 1896 1897 } 1898 1899 @Override 1900 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1901 switch (hash) { 1902 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1903 case -462853915: /*clinicalStatus*/ return this.clinicalStatus == null ? new Base[0] : new Base[] {this.clinicalStatus}; // Enumeration<ConditionClinicalStatus> 1904 case -842509843: /*verificationStatus*/ return this.verificationStatus == null ? new Base[0] : new Base[] {this.verificationStatus}; // Enumeration<ConditionVerificationStatus> 1905 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1906 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // CodeableConcept 1907 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1908 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 1909 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1910 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1911 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // Type 1912 case -921554001: /*abatement*/ return this.abatement == null ? new Base[0] : new Base[] {this.abatement}; // Type 1913 case -174231629: /*assertedDate*/ return this.assertedDate == null ? new Base[0] : new Base[] {this.assertedDate}; // DateTimeType 1914 case -373242253: /*asserter*/ return this.asserter == null ? new Base[0] : new Base[] {this.asserter}; // Reference 1915 case 109757182: /*stage*/ return this.stage == null ? new Base[0] : new Base[] {this.stage}; // ConditionStageComponent 1916 case 382967383: /*evidence*/ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // ConditionEvidenceComponent 1917 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1918 default: return super.getProperty(hash, name, checkValid); 1919 } 1920 1921 } 1922 1923 @Override 1924 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1925 switch (hash) { 1926 case -1618432855: // identifier 1927 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1928 return value; 1929 case -462853915: // clinicalStatus 1930 value = new ConditionClinicalStatusEnumFactory().fromType(castToCode(value)); 1931 this.clinicalStatus = (Enumeration) value; // Enumeration<ConditionClinicalStatus> 1932 return value; 1933 case -842509843: // verificationStatus 1934 value = new ConditionVerificationStatusEnumFactory().fromType(castToCode(value)); 1935 this.verificationStatus = (Enumeration) value; // Enumeration<ConditionVerificationStatus> 1936 return value; 1937 case 50511102: // category 1938 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1939 return value; 1940 case 1478300413: // severity 1941 this.severity = castToCodeableConcept(value); // CodeableConcept 1942 return value; 1943 case 3059181: // code 1944 this.code = castToCodeableConcept(value); // CodeableConcept 1945 return value; 1946 case 1702620169: // bodySite 1947 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 1948 return value; 1949 case -1867885268: // subject 1950 this.subject = castToReference(value); // Reference 1951 return value; 1952 case 951530927: // context 1953 this.context = castToReference(value); // Reference 1954 return value; 1955 case 105901603: // onset 1956 this.onset = castToType(value); // Type 1957 return value; 1958 case -921554001: // abatement 1959 this.abatement = castToType(value); // Type 1960 return value; 1961 case -174231629: // assertedDate 1962 this.assertedDate = castToDateTime(value); // DateTimeType 1963 return value; 1964 case -373242253: // asserter 1965 this.asserter = castToReference(value); // Reference 1966 return value; 1967 case 109757182: // stage 1968 this.stage = (ConditionStageComponent) value; // ConditionStageComponent 1969 return value; 1970 case 382967383: // evidence 1971 this.getEvidence().add((ConditionEvidenceComponent) value); // ConditionEvidenceComponent 1972 return value; 1973 case 3387378: // note 1974 this.getNote().add(castToAnnotation(value)); // Annotation 1975 return value; 1976 default: return super.setProperty(hash, name, value); 1977 } 1978 1979 } 1980 1981 @Override 1982 public Base setProperty(String name, Base value) throws FHIRException { 1983 if (name.equals("identifier")) { 1984 this.getIdentifier().add(castToIdentifier(value)); 1985 } else if (name.equals("clinicalStatus")) { 1986 value = new ConditionClinicalStatusEnumFactory().fromType(castToCode(value)); 1987 this.clinicalStatus = (Enumeration) value; // Enumeration<ConditionClinicalStatus> 1988 } else if (name.equals("verificationStatus")) { 1989 value = new ConditionVerificationStatusEnumFactory().fromType(castToCode(value)); 1990 this.verificationStatus = (Enumeration) value; // Enumeration<ConditionVerificationStatus> 1991 } else if (name.equals("category")) { 1992 this.getCategory().add(castToCodeableConcept(value)); 1993 } else if (name.equals("severity")) { 1994 this.severity = castToCodeableConcept(value); // CodeableConcept 1995 } else if (name.equals("code")) { 1996 this.code = castToCodeableConcept(value); // CodeableConcept 1997 } else if (name.equals("bodySite")) { 1998 this.getBodySite().add(castToCodeableConcept(value)); 1999 } else if (name.equals("subject")) { 2000 this.subject = castToReference(value); // Reference 2001 } else if (name.equals("context")) { 2002 this.context = castToReference(value); // Reference 2003 } else if (name.equals("onset[x]")) { 2004 this.onset = castToType(value); // Type 2005 } else if (name.equals("abatement[x]")) { 2006 this.abatement = castToType(value); // Type 2007 } else if (name.equals("assertedDate")) { 2008 this.assertedDate = castToDateTime(value); // DateTimeType 2009 } else if (name.equals("asserter")) { 2010 this.asserter = castToReference(value); // Reference 2011 } else if (name.equals("stage")) { 2012 this.stage = (ConditionStageComponent) value; // ConditionStageComponent 2013 } else if (name.equals("evidence")) { 2014 this.getEvidence().add((ConditionEvidenceComponent) value); 2015 } else if (name.equals("note")) { 2016 this.getNote().add(castToAnnotation(value)); 2017 } else 2018 return super.setProperty(name, value); 2019 return value; 2020 } 2021 2022 @Override 2023 public Base makeProperty(int hash, String name) throws FHIRException { 2024 switch (hash) { 2025 case -1618432855: return addIdentifier(); 2026 case -462853915: return getClinicalStatusElement(); 2027 case -842509843: return getVerificationStatusElement(); 2028 case 50511102: return addCategory(); 2029 case 1478300413: return getSeverity(); 2030 case 3059181: return getCode(); 2031 case 1702620169: return addBodySite(); 2032 case -1867885268: return getSubject(); 2033 case 951530927: return getContext(); 2034 case -1886216323: return getOnset(); 2035 case 105901603: return getOnset(); 2036 case -584196495: return getAbatement(); 2037 case -921554001: return getAbatement(); 2038 case -174231629: return getAssertedDateElement(); 2039 case -373242253: return getAsserter(); 2040 case 109757182: return getStage(); 2041 case 382967383: return addEvidence(); 2042 case 3387378: return addNote(); 2043 default: return super.makeProperty(hash, name); 2044 } 2045 2046 } 2047 2048 @Override 2049 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2050 switch (hash) { 2051 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2052 case -462853915: /*clinicalStatus*/ return new String[] {"code"}; 2053 case -842509843: /*verificationStatus*/ return new String[] {"code"}; 2054 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2055 case 1478300413: /*severity*/ return new String[] {"CodeableConcept"}; 2056 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2057 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 2058 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2059 case 951530927: /*context*/ return new String[] {"Reference"}; 2060 case 105901603: /*onset*/ return new String[] {"dateTime", "Age", "Period", "Range", "string"}; 2061 case -921554001: /*abatement*/ return new String[] {"dateTime", "Age", "boolean", "Period", "Range", "string"}; 2062 case -174231629: /*assertedDate*/ return new String[] {"dateTime"}; 2063 case -373242253: /*asserter*/ return new String[] {"Reference"}; 2064 case 109757182: /*stage*/ return new String[] {}; 2065 case 382967383: /*evidence*/ return new String[] {}; 2066 case 3387378: /*note*/ return new String[] {"Annotation"}; 2067 default: return super.getTypesForProperty(hash, name); 2068 } 2069 2070 } 2071 2072 @Override 2073 public Base addChild(String name) throws FHIRException { 2074 if (name.equals("identifier")) { 2075 return addIdentifier(); 2076 } 2077 else if (name.equals("clinicalStatus")) { 2078 throw new FHIRException("Cannot call addChild on a singleton property Condition.clinicalStatus"); 2079 } 2080 else if (name.equals("verificationStatus")) { 2081 throw new FHIRException("Cannot call addChild on a singleton property Condition.verificationStatus"); 2082 } 2083 else if (name.equals("category")) { 2084 return addCategory(); 2085 } 2086 else if (name.equals("severity")) { 2087 this.severity = new CodeableConcept(); 2088 return this.severity; 2089 } 2090 else if (name.equals("code")) { 2091 this.code = new CodeableConcept(); 2092 return this.code; 2093 } 2094 else if (name.equals("bodySite")) { 2095 return addBodySite(); 2096 } 2097 else if (name.equals("subject")) { 2098 this.subject = new Reference(); 2099 return this.subject; 2100 } 2101 else if (name.equals("context")) { 2102 this.context = new Reference(); 2103 return this.context; 2104 } 2105 else if (name.equals("onsetDateTime")) { 2106 this.onset = new DateTimeType(); 2107 return this.onset; 2108 } 2109 else if (name.equals("onsetAge")) { 2110 this.onset = new Age(); 2111 return this.onset; 2112 } 2113 else if (name.equals("onsetPeriod")) { 2114 this.onset = new Period(); 2115 return this.onset; 2116 } 2117 else if (name.equals("onsetRange")) { 2118 this.onset = new Range(); 2119 return this.onset; 2120 } 2121 else if (name.equals("onsetString")) { 2122 this.onset = new StringType(); 2123 return this.onset; 2124 } 2125 else if (name.equals("abatementDateTime")) { 2126 this.abatement = new DateTimeType(); 2127 return this.abatement; 2128 } 2129 else if (name.equals("abatementAge")) { 2130 this.abatement = new Age(); 2131 return this.abatement; 2132 } 2133 else if (name.equals("abatementBoolean")) { 2134 this.abatement = new BooleanType(); 2135 return this.abatement; 2136 } 2137 else if (name.equals("abatementPeriod")) { 2138 this.abatement = new Period(); 2139 return this.abatement; 2140 } 2141 else if (name.equals("abatementRange")) { 2142 this.abatement = new Range(); 2143 return this.abatement; 2144 } 2145 else if (name.equals("abatementString")) { 2146 this.abatement = new StringType(); 2147 return this.abatement; 2148 } 2149 else if (name.equals("assertedDate")) { 2150 throw new FHIRException("Cannot call addChild on a singleton property Condition.assertedDate"); 2151 } 2152 else if (name.equals("asserter")) { 2153 this.asserter = new Reference(); 2154 return this.asserter; 2155 } 2156 else if (name.equals("stage")) { 2157 this.stage = new ConditionStageComponent(); 2158 return this.stage; 2159 } 2160 else if (name.equals("evidence")) { 2161 return addEvidence(); 2162 } 2163 else if (name.equals("note")) { 2164 return addNote(); 2165 } 2166 else 2167 return super.addChild(name); 2168 } 2169 2170 public String fhirType() { 2171 return "Condition"; 2172 2173 } 2174 2175 public Condition copy() { 2176 Condition dst = new Condition(); 2177 copyValues(dst); 2178 if (identifier != null) { 2179 dst.identifier = new ArrayList<Identifier>(); 2180 for (Identifier i : identifier) 2181 dst.identifier.add(i.copy()); 2182 }; 2183 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 2184 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 2185 if (category != null) { 2186 dst.category = new ArrayList<CodeableConcept>(); 2187 for (CodeableConcept i : category) 2188 dst.category.add(i.copy()); 2189 }; 2190 dst.severity = severity == null ? null : severity.copy(); 2191 dst.code = code == null ? null : code.copy(); 2192 if (bodySite != null) { 2193 dst.bodySite = new ArrayList<CodeableConcept>(); 2194 for (CodeableConcept i : bodySite) 2195 dst.bodySite.add(i.copy()); 2196 }; 2197 dst.subject = subject == null ? null : subject.copy(); 2198 dst.context = context == null ? null : context.copy(); 2199 dst.onset = onset == null ? null : onset.copy(); 2200 dst.abatement = abatement == null ? null : abatement.copy(); 2201 dst.assertedDate = assertedDate == null ? null : assertedDate.copy(); 2202 dst.asserter = asserter == null ? null : asserter.copy(); 2203 dst.stage = stage == null ? null : stage.copy(); 2204 if (evidence != null) { 2205 dst.evidence = new ArrayList<ConditionEvidenceComponent>(); 2206 for (ConditionEvidenceComponent i : evidence) 2207 dst.evidence.add(i.copy()); 2208 }; 2209 if (note != null) { 2210 dst.note = new ArrayList<Annotation>(); 2211 for (Annotation i : note) 2212 dst.note.add(i.copy()); 2213 }; 2214 return dst; 2215 } 2216 2217 protected Condition typedCopy() { 2218 return copy(); 2219 } 2220 2221 @Override 2222 public boolean equalsDeep(Base other_) { 2223 if (!super.equalsDeep(other_)) 2224 return false; 2225 if (!(other_ instanceof Condition)) 2226 return false; 2227 Condition o = (Condition) other_; 2228 return compareDeep(identifier, o.identifier, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 2229 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(category, o.category, true) 2230 && compareDeep(severity, o.severity, true) && compareDeep(code, o.code, true) && compareDeep(bodySite, o.bodySite, true) 2231 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(onset, o.onset, true) 2232 && compareDeep(abatement, o.abatement, true) && compareDeep(assertedDate, o.assertedDate, true) 2233 && compareDeep(asserter, o.asserter, true) && compareDeep(stage, o.stage, true) && compareDeep(evidence, o.evidence, true) 2234 && compareDeep(note, o.note, true); 2235 } 2236 2237 @Override 2238 public boolean equalsShallow(Base other_) { 2239 if (!super.equalsShallow(other_)) 2240 return false; 2241 if (!(other_ instanceof Condition)) 2242 return false; 2243 Condition o = (Condition) other_; 2244 return compareValues(clinicalStatus, o.clinicalStatus, true) && compareValues(verificationStatus, o.verificationStatus, true) 2245 && compareValues(assertedDate, o.assertedDate, true); 2246 } 2247 2248 public boolean isEmpty() { 2249 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, clinicalStatus 2250 , verificationStatus, category, severity, code, bodySite, subject, context, onset 2251 , abatement, assertedDate, asserter, stage, evidence, note); 2252 } 2253 2254 @Override 2255 public ResourceType getResourceType() { 2256 return ResourceType.Condition; 2257 } 2258 2259 /** 2260 * Search parameter: <b>severity</b> 2261 * <p> 2262 * Description: <b>The severity of the condition</b><br> 2263 * Type: <b>token</b><br> 2264 * Path: <b>Condition.severity</b><br> 2265 * </p> 2266 */ 2267 @SearchParamDefinition(name="severity", path="Condition.severity", description="The severity of the condition", type="token" ) 2268 public static final String SP_SEVERITY = "severity"; 2269 /** 2270 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2271 * <p> 2272 * Description: <b>The severity of the condition</b><br> 2273 * Type: <b>token</b><br> 2274 * Path: <b>Condition.severity</b><br> 2275 * </p> 2276 */ 2277 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SEVERITY); 2278 2279 /** 2280 * Search parameter: <b>evidence-detail</b> 2281 * <p> 2282 * Description: <b>Supporting information found elsewhere</b><br> 2283 * Type: <b>reference</b><br> 2284 * Path: <b>Condition.evidence.detail</b><br> 2285 * </p> 2286 */ 2287 @SearchParamDefinition(name="evidence-detail", path="Condition.evidence.detail", description="Supporting information found elsewhere", type="reference" ) 2288 public static final String SP_EVIDENCE_DETAIL = "evidence-detail"; 2289 /** 2290 * <b>Fluent Client</b> search parameter constant for <b>evidence-detail</b> 2291 * <p> 2292 * Description: <b>Supporting information found elsewhere</b><br> 2293 * Type: <b>reference</b><br> 2294 * Path: <b>Condition.evidence.detail</b><br> 2295 * </p> 2296 */ 2297 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EVIDENCE_DETAIL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EVIDENCE_DETAIL); 2298 2299/** 2300 * Constant for fluent queries to be used to add include statements. Specifies 2301 * the path value of "<b>Condition:evidence-detail</b>". 2302 */ 2303 public static final ca.uhn.fhir.model.api.Include INCLUDE_EVIDENCE_DETAIL = new ca.uhn.fhir.model.api.Include("Condition:evidence-detail").toLocked(); 2304 2305 /** 2306 * Search parameter: <b>identifier</b> 2307 * <p> 2308 * Description: <b>A unique identifier of the condition record</b><br> 2309 * Type: <b>token</b><br> 2310 * Path: <b>Condition.identifier</b><br> 2311 * </p> 2312 */ 2313 @SearchParamDefinition(name="identifier", path="Condition.identifier", description="A unique identifier of the condition record", type="token" ) 2314 public static final String SP_IDENTIFIER = "identifier"; 2315 /** 2316 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2317 * <p> 2318 * Description: <b>A unique identifier of the condition record</b><br> 2319 * Type: <b>token</b><br> 2320 * Path: <b>Condition.identifier</b><br> 2321 * </p> 2322 */ 2323 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2324 2325 /** 2326 * Search parameter: <b>onset-info</b> 2327 * <p> 2328 * Description: <b>Onsets as a string</b><br> 2329 * Type: <b>string</b><br> 2330 * Path: <b>Condition.onset[x]</b><br> 2331 * </p> 2332 */ 2333 @SearchParamDefinition(name="onset-info", path="Condition.onset.as(string)", description="Onsets as a string", type="string" ) 2334 public static final String SP_ONSET_INFO = "onset-info"; 2335 /** 2336 * <b>Fluent Client</b> search parameter constant for <b>onset-info</b> 2337 * <p> 2338 * Description: <b>Onsets as a string</b><br> 2339 * Type: <b>string</b><br> 2340 * Path: <b>Condition.onset[x]</b><br> 2341 * </p> 2342 */ 2343 public static final ca.uhn.fhir.rest.gclient.StringClientParam ONSET_INFO = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ONSET_INFO); 2344 2345 /** 2346 * Search parameter: <b>code</b> 2347 * <p> 2348 * Description: <b>Code for the condition</b><br> 2349 * Type: <b>token</b><br> 2350 * Path: <b>Condition.code</b><br> 2351 * </p> 2352 */ 2353 @SearchParamDefinition(name="code", path="Condition.code", description="Code for the condition", type="token" ) 2354 public static final String SP_CODE = "code"; 2355 /** 2356 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2357 * <p> 2358 * Description: <b>Code for the condition</b><br> 2359 * Type: <b>token</b><br> 2360 * Path: <b>Condition.code</b><br> 2361 * </p> 2362 */ 2363 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2364 2365 /** 2366 * Search parameter: <b>evidence</b> 2367 * <p> 2368 * Description: <b>Manifestation/symptom</b><br> 2369 * Type: <b>token</b><br> 2370 * Path: <b>Condition.evidence.code</b><br> 2371 * </p> 2372 */ 2373 @SearchParamDefinition(name="evidence", path="Condition.evidence.code", description="Manifestation/symptom", type="token" ) 2374 public static final String SP_EVIDENCE = "evidence"; 2375 /** 2376 * <b>Fluent Client</b> search parameter constant for <b>evidence</b> 2377 * <p> 2378 * Description: <b>Manifestation/symptom</b><br> 2379 * Type: <b>token</b><br> 2380 * Path: <b>Condition.evidence.code</b><br> 2381 * </p> 2382 */ 2383 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVIDENCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVIDENCE); 2384 2385 /** 2386 * Search parameter: <b>subject</b> 2387 * <p> 2388 * Description: <b>Who has the condition?</b><br> 2389 * Type: <b>reference</b><br> 2390 * Path: <b>Condition.subject</b><br> 2391 * </p> 2392 */ 2393 @SearchParamDefinition(name="subject", path="Condition.subject", description="Who has the condition?", type="reference", target={Group.class, Patient.class } ) 2394 public static final String SP_SUBJECT = "subject"; 2395 /** 2396 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2397 * <p> 2398 * Description: <b>Who has the condition?</b><br> 2399 * Type: <b>reference</b><br> 2400 * Path: <b>Condition.subject</b><br> 2401 * </p> 2402 */ 2403 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2404 2405/** 2406 * Constant for fluent queries to be used to add include statements. Specifies 2407 * the path value of "<b>Condition:subject</b>". 2408 */ 2409 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Condition:subject").toLocked(); 2410 2411 /** 2412 * Search parameter: <b>verification-status</b> 2413 * <p> 2414 * Description: <b>provisional | differential | confirmed | refuted | entered-in-error | unknown</b><br> 2415 * Type: <b>token</b><br> 2416 * Path: <b>Condition.verificationStatus</b><br> 2417 * </p> 2418 */ 2419 @SearchParamDefinition(name="verification-status", path="Condition.verificationStatus", description="provisional | differential | confirmed | refuted | entered-in-error | unknown", type="token" ) 2420 public static final String SP_VERIFICATION_STATUS = "verification-status"; 2421 /** 2422 * <b>Fluent Client</b> search parameter constant for <b>verification-status</b> 2423 * <p> 2424 * Description: <b>provisional | differential | confirmed | refuted | entered-in-error | unknown</b><br> 2425 * Type: <b>token</b><br> 2426 * Path: <b>Condition.verificationStatus</b><br> 2427 * </p> 2428 */ 2429 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFICATION_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERIFICATION_STATUS); 2430 2431 /** 2432 * Search parameter: <b>clinical-status</b> 2433 * <p> 2434 * Description: <b>The clinical status of the condition</b><br> 2435 * Type: <b>token</b><br> 2436 * Path: <b>Condition.clinicalStatus</b><br> 2437 * </p> 2438 */ 2439 @SearchParamDefinition(name="clinical-status", path="Condition.clinicalStatus", description="The clinical status of the condition", type="token" ) 2440 public static final String SP_CLINICAL_STATUS = "clinical-status"; 2441 /** 2442 * <b>Fluent Client</b> search parameter constant for <b>clinical-status</b> 2443 * <p> 2444 * Description: <b>The clinical status of the condition</b><br> 2445 * Type: <b>token</b><br> 2446 * Path: <b>Condition.clinicalStatus</b><br> 2447 * </p> 2448 */ 2449 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLINICAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLINICAL_STATUS); 2450 2451 /** 2452 * Search parameter: <b>encounter</b> 2453 * <p> 2454 * Description: <b>Encounter when condition first asserted</b><br> 2455 * Type: <b>reference</b><br> 2456 * Path: <b>Condition.context</b><br> 2457 * </p> 2458 */ 2459 @SearchParamDefinition(name="encounter", path="Condition.context", description="Encounter when condition first asserted", type="reference", target={Encounter.class } ) 2460 public static final String SP_ENCOUNTER = "encounter"; 2461 /** 2462 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2463 * <p> 2464 * Description: <b>Encounter when condition first asserted</b><br> 2465 * Type: <b>reference</b><br> 2466 * Path: <b>Condition.context</b><br> 2467 * </p> 2468 */ 2469 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2470 2471/** 2472 * Constant for fluent queries to be used to add include statements. Specifies 2473 * the path value of "<b>Condition:encounter</b>". 2474 */ 2475 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Condition:encounter").toLocked(); 2476 2477 /** 2478 * Search parameter: <b>abatement-boolean</b> 2479 * <p> 2480 * Description: <b>Abatement boolean (boolean is true or non-boolean values are present)</b><br> 2481 * Type: <b>token</b><br> 2482 * Path: <b>Condition.abatement[x]</b><br> 2483 * </p> 2484 */ 2485 @SearchParamDefinition(name="abatement-boolean", path="Condition.abatement.as(boolean) | Condition.abatement.is(dateTime) | Condition.abatement.is(Age) | Condition.abatement.is(Period) | Condition.abatement.is(Range) | Condition.abatement.is(string)", description="Abatement boolean (boolean is true or non-boolean values are present)", type="token" ) 2486 public static final String SP_ABATEMENT_BOOLEAN = "abatement-boolean"; 2487 /** 2488 * <b>Fluent Client</b> search parameter constant for <b>abatement-boolean</b> 2489 * <p> 2490 * Description: <b>Abatement boolean (boolean is true or non-boolean values are present)</b><br> 2491 * Type: <b>token</b><br> 2492 * Path: <b>Condition.abatement[x]</b><br> 2493 * </p> 2494 */ 2495 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ABATEMENT_BOOLEAN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ABATEMENT_BOOLEAN); 2496 2497 /** 2498 * Search parameter: <b>onset-date</b> 2499 * <p> 2500 * Description: <b>Date related onsets (dateTime and Period)</b><br> 2501 * Type: <b>date</b><br> 2502 * Path: <b>Condition.onset[x]</b><br> 2503 * </p> 2504 */ 2505 @SearchParamDefinition(name="onset-date", path="Condition.onset.as(dateTime) | Condition.onset.as(Period)", description="Date related onsets (dateTime and Period)", type="date" ) 2506 public static final String SP_ONSET_DATE = "onset-date"; 2507 /** 2508 * <b>Fluent Client</b> search parameter constant for <b>onset-date</b> 2509 * <p> 2510 * Description: <b>Date related onsets (dateTime and Period)</b><br> 2511 * Type: <b>date</b><br> 2512 * Path: <b>Condition.onset[x]</b><br> 2513 * </p> 2514 */ 2515 public static final ca.uhn.fhir.rest.gclient.DateClientParam ONSET_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ONSET_DATE); 2516 2517 /** 2518 * Search parameter: <b>abatement-date</b> 2519 * <p> 2520 * Description: <b>Date-related abatements (dateTime and period)</b><br> 2521 * Type: <b>date</b><br> 2522 * Path: <b>Condition.abatement[x]</b><br> 2523 * </p> 2524 */ 2525 @SearchParamDefinition(name="abatement-date", path="Condition.abatement.as(dateTime) | Condition.abatement.as(Period)", description="Date-related abatements (dateTime and period)", type="date" ) 2526 public static final String SP_ABATEMENT_DATE = "abatement-date"; 2527 /** 2528 * <b>Fluent Client</b> search parameter constant for <b>abatement-date</b> 2529 * <p> 2530 * Description: <b>Date-related abatements (dateTime and period)</b><br> 2531 * Type: <b>date</b><br> 2532 * Path: <b>Condition.abatement[x]</b><br> 2533 * </p> 2534 */ 2535 public static final ca.uhn.fhir.rest.gclient.DateClientParam ABATEMENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ABATEMENT_DATE); 2536 2537 /** 2538 * Search parameter: <b>asserter</b> 2539 * <p> 2540 * Description: <b>Person who asserts this condition</b><br> 2541 * Type: <b>reference</b><br> 2542 * Path: <b>Condition.asserter</b><br> 2543 * </p> 2544 */ 2545 @SearchParamDefinition(name="asserter", path="Condition.asserter", description="Person who asserts this condition", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Practitioner.class, RelatedPerson.class } ) 2546 public static final String SP_ASSERTER = "asserter"; 2547 /** 2548 * <b>Fluent Client</b> search parameter constant for <b>asserter</b> 2549 * <p> 2550 * Description: <b>Person who asserts this condition</b><br> 2551 * Type: <b>reference</b><br> 2552 * Path: <b>Condition.asserter</b><br> 2553 * </p> 2554 */ 2555 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSERTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ASSERTER); 2556 2557/** 2558 * Constant for fluent queries to be used to add include statements. Specifies 2559 * the path value of "<b>Condition:asserter</b>". 2560 */ 2561 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSERTER = new ca.uhn.fhir.model.api.Include("Condition:asserter").toLocked(); 2562 2563 /** 2564 * Search parameter: <b>stage</b> 2565 * <p> 2566 * Description: <b>Simple summary (disease specific)</b><br> 2567 * Type: <b>token</b><br> 2568 * Path: <b>Condition.stage.summary</b><br> 2569 * </p> 2570 */ 2571 @SearchParamDefinition(name="stage", path="Condition.stage.summary", description="Simple summary (disease specific)", type="token" ) 2572 public static final String SP_STAGE = "stage"; 2573 /** 2574 * <b>Fluent Client</b> search parameter constant for <b>stage</b> 2575 * <p> 2576 * Description: <b>Simple summary (disease specific)</b><br> 2577 * Type: <b>token</b><br> 2578 * Path: <b>Condition.stage.summary</b><br> 2579 * </p> 2580 */ 2581 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STAGE); 2582 2583 /** 2584 * Search parameter: <b>abatement-string</b> 2585 * <p> 2586 * Description: <b>Abatement as a string</b><br> 2587 * Type: <b>string</b><br> 2588 * Path: <b>Condition.abatement[x]</b><br> 2589 * </p> 2590 */ 2591 @SearchParamDefinition(name="abatement-string", path="Condition.abatement.as(string)", description="Abatement as a string", type="string" ) 2592 public static final String SP_ABATEMENT_STRING = "abatement-string"; 2593 /** 2594 * <b>Fluent Client</b> search parameter constant for <b>abatement-string</b> 2595 * <p> 2596 * Description: <b>Abatement as a string</b><br> 2597 * Type: <b>string</b><br> 2598 * Path: <b>Condition.abatement[x]</b><br> 2599 * </p> 2600 */ 2601 public static final ca.uhn.fhir.rest.gclient.StringClientParam ABATEMENT_STRING = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ABATEMENT_STRING); 2602 2603 /** 2604 * Search parameter: <b>patient</b> 2605 * <p> 2606 * Description: <b>Who has the condition?</b><br> 2607 * Type: <b>reference</b><br> 2608 * Path: <b>Condition.subject</b><br> 2609 * </p> 2610 */ 2611 @SearchParamDefinition(name="patient", path="Condition.subject", description="Who has the condition?", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2612 public static final String SP_PATIENT = "patient"; 2613 /** 2614 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2615 * <p> 2616 * Description: <b>Who has the condition?</b><br> 2617 * Type: <b>reference</b><br> 2618 * Path: <b>Condition.subject</b><br> 2619 * </p> 2620 */ 2621 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2622 2623/** 2624 * Constant for fluent queries to be used to add include statements. Specifies 2625 * the path value of "<b>Condition:patient</b>". 2626 */ 2627 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Condition:patient").toLocked(); 2628 2629 /** 2630 * Search parameter: <b>context</b> 2631 * <p> 2632 * Description: <b>Encounter or episode when condition first asserted</b><br> 2633 * Type: <b>reference</b><br> 2634 * Path: <b>Condition.context</b><br> 2635 * </p> 2636 */ 2637 @SearchParamDefinition(name="context", path="Condition.context", description="Encounter or episode when condition first asserted", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2638 public static final String SP_CONTEXT = "context"; 2639 /** 2640 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2641 * <p> 2642 * Description: <b>Encounter or episode when condition first asserted</b><br> 2643 * Type: <b>reference</b><br> 2644 * Path: <b>Condition.context</b><br> 2645 * </p> 2646 */ 2647 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2648 2649/** 2650 * Constant for fluent queries to be used to add include statements. Specifies 2651 * the path value of "<b>Condition:context</b>". 2652 */ 2653 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("Condition:context").toLocked(); 2654 2655 /** 2656 * Search parameter: <b>onset-age</b> 2657 * <p> 2658 * Description: <b>Onsets as age or age range</b><br> 2659 * Type: <b>quantity</b><br> 2660 * Path: <b>Condition.onset[x]</b><br> 2661 * </p> 2662 */ 2663 @SearchParamDefinition(name="onset-age", path="Condition.onset.as(Age) | Condition.onset.as(Range)", description="Onsets as age or age range", type="quantity" ) 2664 public static final String SP_ONSET_AGE = "onset-age"; 2665 /** 2666 * <b>Fluent Client</b> search parameter constant for <b>onset-age</b> 2667 * <p> 2668 * Description: <b>Onsets as age or age range</b><br> 2669 * Type: <b>quantity</b><br> 2670 * Path: <b>Condition.onset[x]</b><br> 2671 * </p> 2672 */ 2673 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam ONSET_AGE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_ONSET_AGE); 2674 2675 /** 2676 * Search parameter: <b>abatement-age</b> 2677 * <p> 2678 * Description: <b>Abatement as age or age range</b><br> 2679 * Type: <b>quantity</b><br> 2680 * Path: <b>Condition.abatement[x]</b><br> 2681 * </p> 2682 */ 2683 @SearchParamDefinition(name="abatement-age", path="Condition.abatement.as(Age) | Condition.abatement.as(Range) | Condition.abatement.as(Age)", description="Abatement as age or age range", type="quantity" ) 2684 public static final String SP_ABATEMENT_AGE = "abatement-age"; 2685 /** 2686 * <b>Fluent Client</b> search parameter constant for <b>abatement-age</b> 2687 * <p> 2688 * Description: <b>Abatement as age or age range</b><br> 2689 * Type: <b>quantity</b><br> 2690 * Path: <b>Condition.abatement[x]</b><br> 2691 * </p> 2692 */ 2693 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam ABATEMENT_AGE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_ABATEMENT_AGE); 2694 2695 /** 2696 * Search parameter: <b>asserted-date</b> 2697 * <p> 2698 * Description: <b>Date record was believed accurate</b><br> 2699 * Type: <b>date</b><br> 2700 * Path: <b>Condition.assertedDate</b><br> 2701 * </p> 2702 */ 2703 @SearchParamDefinition(name="asserted-date", path="Condition.assertedDate", description="Date record was believed accurate", type="date" ) 2704 public static final String SP_ASSERTED_DATE = "asserted-date"; 2705 /** 2706 * <b>Fluent Client</b> search parameter constant for <b>asserted-date</b> 2707 * <p> 2708 * Description: <b>Date record was believed accurate</b><br> 2709 * Type: <b>date</b><br> 2710 * Path: <b>Condition.assertedDate</b><br> 2711 * </p> 2712 */ 2713 public static final ca.uhn.fhir.rest.gclient.DateClientParam ASSERTED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ASSERTED_DATE); 2714 2715 /** 2716 * Search parameter: <b>category</b> 2717 * <p> 2718 * Description: <b>The category of the condition</b><br> 2719 * Type: <b>token</b><br> 2720 * Path: <b>Condition.category</b><br> 2721 * </p> 2722 */ 2723 @SearchParamDefinition(name="category", path="Condition.category", description="The category of the condition", type="token" ) 2724 public static final String SP_CATEGORY = "category"; 2725 /** 2726 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2727 * <p> 2728 * Description: <b>The category of the condition</b><br> 2729 * Type: <b>token</b><br> 2730 * Path: <b>Condition.category</b><br> 2731 * </p> 2732 */ 2733 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2734 2735 /** 2736 * Search parameter: <b>body-site</b> 2737 * <p> 2738 * Description: <b>Anatomical location, if relevant</b><br> 2739 * Type: <b>token</b><br> 2740 * Path: <b>Condition.bodySite</b><br> 2741 * </p> 2742 */ 2743 @SearchParamDefinition(name="body-site", path="Condition.bodySite", description="Anatomical location, if relevant", type="token" ) 2744 public static final String SP_BODY_SITE = "body-site"; 2745 /** 2746 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 2747 * <p> 2748 * Description: <b>Anatomical location, if relevant</b><br> 2749 * Type: <b>token</b><br> 2750 * Path: <b>Condition.bodySite</b><br> 2751 * </p> 2752 */ 2753 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 2754 2755 2756}