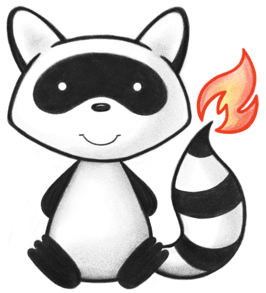
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 051 */ 052@ResourceDef(name="Consent", profile="http://hl7.org/fhir/Profile/Consent") 053public class Consent extends DomainResource { 054 055 public enum ConsentState { 056 /** 057 * The consent is in development or awaiting use but is not yet intended to be acted upon. 058 */ 059 DRAFT, 060 /** 061 * The consent has been proposed but not yet agreed to by all parties. The negotiation stage. 062 */ 063 PROPOSED, 064 /** 065 * The consent is to be followed and enforced. 066 */ 067 ACTIVE, 068 /** 069 * The consent has been rejected by one or more of the parties. 070 */ 071 REJECTED, 072 /** 073 * The consent is terminated or replaced. 074 */ 075 INACTIVE, 076 /** 077 * The consent was created wrongly (e.g. wrong patient) and should be ignored 078 */ 079 ENTEREDINERROR, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static ConsentState fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("draft".equals(codeString)) 088 return DRAFT; 089 if ("proposed".equals(codeString)) 090 return PROPOSED; 091 if ("active".equals(codeString)) 092 return ACTIVE; 093 if ("rejected".equals(codeString)) 094 return REJECTED; 095 if ("inactive".equals(codeString)) 096 return INACTIVE; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 103 } 104 public String toCode() { 105 switch (this) { 106 case DRAFT: return "draft"; 107 case PROPOSED: return "proposed"; 108 case ACTIVE: return "active"; 109 case REJECTED: return "rejected"; 110 case INACTIVE: return "inactive"; 111 case ENTEREDINERROR: return "entered-in-error"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getSystem() { 117 switch (this) { 118 case DRAFT: return "http://hl7.org/fhir/consent-state-codes"; 119 case PROPOSED: return "http://hl7.org/fhir/consent-state-codes"; 120 case ACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 121 case REJECTED: return "http://hl7.org/fhir/consent-state-codes"; 122 case INACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 123 case ENTEREDINERROR: return "http://hl7.org/fhir/consent-state-codes"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 public String getDefinition() { 129 switch (this) { 130 case DRAFT: return "The consent is in development or awaiting use but is not yet intended to be acted upon."; 131 case PROPOSED: return "The consent has been proposed but not yet agreed to by all parties. The negotiation stage."; 132 case ACTIVE: return "The consent is to be followed and enforced."; 133 case REJECTED: return "The consent has been rejected by one or more of the parties."; 134 case INACTIVE: return "The consent is terminated or replaced."; 135 case ENTEREDINERROR: return "The consent was created wrongly (e.g. wrong patient) and should be ignored"; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 public String getDisplay() { 141 switch (this) { 142 case DRAFT: return "Pending"; 143 case PROPOSED: return "Proposed"; 144 case ACTIVE: return "Active"; 145 case REJECTED: return "Rejected"; 146 case INACTIVE: return "Inactive"; 147 case ENTEREDINERROR: return "Entered in Error"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 } 153 154 public static class ConsentStateEnumFactory implements EnumFactory<ConsentState> { 155 public ConsentState fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("draft".equals(codeString)) 160 return ConsentState.DRAFT; 161 if ("proposed".equals(codeString)) 162 return ConsentState.PROPOSED; 163 if ("active".equals(codeString)) 164 return ConsentState.ACTIVE; 165 if ("rejected".equals(codeString)) 166 return ConsentState.REJECTED; 167 if ("inactive".equals(codeString)) 168 return ConsentState.INACTIVE; 169 if ("entered-in-error".equals(codeString)) 170 return ConsentState.ENTEREDINERROR; 171 throw new IllegalArgumentException("Unknown ConsentState code '"+codeString+"'"); 172 } 173 public Enumeration<ConsentState> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<ConsentState>(this); 178 String codeString = code.asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("draft".equals(codeString)) 182 return new Enumeration<ConsentState>(this, ConsentState.DRAFT); 183 if ("proposed".equals(codeString)) 184 return new Enumeration<ConsentState>(this, ConsentState.PROPOSED); 185 if ("active".equals(codeString)) 186 return new Enumeration<ConsentState>(this, ConsentState.ACTIVE); 187 if ("rejected".equals(codeString)) 188 return new Enumeration<ConsentState>(this, ConsentState.REJECTED); 189 if ("inactive".equals(codeString)) 190 return new Enumeration<ConsentState>(this, ConsentState.INACTIVE); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<ConsentState>(this, ConsentState.ENTEREDINERROR); 193 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 194 } 195 public String toCode(ConsentState code) { 196 if (code == ConsentState.NULL) 197 return null; 198 if (code == ConsentState.DRAFT) 199 return "draft"; 200 if (code == ConsentState.PROPOSED) 201 return "proposed"; 202 if (code == ConsentState.ACTIVE) 203 return "active"; 204 if (code == ConsentState.REJECTED) 205 return "rejected"; 206 if (code == ConsentState.INACTIVE) 207 return "inactive"; 208 if (code == ConsentState.ENTEREDINERROR) 209 return "entered-in-error"; 210 return "?"; 211 } 212 public String toSystem(ConsentState code) { 213 return code.getSystem(); 214 } 215 } 216 217 public enum ConsentDataMeaning { 218 /** 219 * The consent applies directly to the instance of the resource 220 */ 221 INSTANCE, 222 /** 223 * The consent applies directly to the instance of the resource and instances it refers to 224 */ 225 RELATED, 226 /** 227 * The consent applies directly to the instance of the resource and instances that refer to it 228 */ 229 DEPENDENTS, 230 /** 231 * The consent applies to instances of resources that are authored by 232 */ 233 AUTHOREDBY, 234 /** 235 * added to help the parsers with the generic types 236 */ 237 NULL; 238 public static ConsentDataMeaning fromCode(String codeString) throws FHIRException { 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("instance".equals(codeString)) 242 return INSTANCE; 243 if ("related".equals(codeString)) 244 return RELATED; 245 if ("dependents".equals(codeString)) 246 return DEPENDENTS; 247 if ("authoredby".equals(codeString)) 248 return AUTHOREDBY; 249 if (Configuration.isAcceptInvalidEnums()) 250 return null; 251 else 252 throw new FHIRException("Unknown ConsentDataMeaning code '"+codeString+"'"); 253 } 254 public String toCode() { 255 switch (this) { 256 case INSTANCE: return "instance"; 257 case RELATED: return "related"; 258 case DEPENDENTS: return "dependents"; 259 case AUTHOREDBY: return "authoredby"; 260 case NULL: return null; 261 default: return "?"; 262 } 263 } 264 public String getSystem() { 265 switch (this) { 266 case INSTANCE: return "http://hl7.org/fhir/consent-data-meaning"; 267 case RELATED: return "http://hl7.org/fhir/consent-data-meaning"; 268 case DEPENDENTS: return "http://hl7.org/fhir/consent-data-meaning"; 269 case AUTHOREDBY: return "http://hl7.org/fhir/consent-data-meaning"; 270 case NULL: return null; 271 default: return "?"; 272 } 273 } 274 public String getDefinition() { 275 switch (this) { 276 case INSTANCE: return "The consent applies directly to the instance of the resource"; 277 case RELATED: return "The consent applies directly to the instance of the resource and instances it refers to"; 278 case DEPENDENTS: return "The consent applies directly to the instance of the resource and instances that refer to it"; 279 case AUTHOREDBY: return "The consent applies to instances of resources that are authored by"; 280 case NULL: return null; 281 default: return "?"; 282 } 283 } 284 public String getDisplay() { 285 switch (this) { 286 case INSTANCE: return "Instance"; 287 case RELATED: return "Related"; 288 case DEPENDENTS: return "Dependents"; 289 case AUTHOREDBY: return "AuthoredBy"; 290 case NULL: return null; 291 default: return "?"; 292 } 293 } 294 } 295 296 public static class ConsentDataMeaningEnumFactory implements EnumFactory<ConsentDataMeaning> { 297 public ConsentDataMeaning fromCode(String codeString) throws IllegalArgumentException { 298 if (codeString == null || "".equals(codeString)) 299 if (codeString == null || "".equals(codeString)) 300 return null; 301 if ("instance".equals(codeString)) 302 return ConsentDataMeaning.INSTANCE; 303 if ("related".equals(codeString)) 304 return ConsentDataMeaning.RELATED; 305 if ("dependents".equals(codeString)) 306 return ConsentDataMeaning.DEPENDENTS; 307 if ("authoredby".equals(codeString)) 308 return ConsentDataMeaning.AUTHOREDBY; 309 throw new IllegalArgumentException("Unknown ConsentDataMeaning code '"+codeString+"'"); 310 } 311 public Enumeration<ConsentDataMeaning> fromType(PrimitiveType<?> code) throws FHIRException { 312 if (code == null) 313 return null; 314 if (code.isEmpty()) 315 return new Enumeration<ConsentDataMeaning>(this); 316 String codeString = code.asStringValue(); 317 if (codeString == null || "".equals(codeString)) 318 return null; 319 if ("instance".equals(codeString)) 320 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.INSTANCE); 321 if ("related".equals(codeString)) 322 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.RELATED); 323 if ("dependents".equals(codeString)) 324 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.DEPENDENTS); 325 if ("authoredby".equals(codeString)) 326 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.AUTHOREDBY); 327 throw new FHIRException("Unknown ConsentDataMeaning code '"+codeString+"'"); 328 } 329 public String toCode(ConsentDataMeaning code) { 330 if (code == ConsentDataMeaning.NULL) 331 return null; 332 if (code == ConsentDataMeaning.INSTANCE) 333 return "instance"; 334 if (code == ConsentDataMeaning.RELATED) 335 return "related"; 336 if (code == ConsentDataMeaning.DEPENDENTS) 337 return "dependents"; 338 if (code == ConsentDataMeaning.AUTHOREDBY) 339 return "authoredby"; 340 return "?"; 341 } 342 public String toSystem(ConsentDataMeaning code) { 343 return code.getSystem(); 344 } 345 } 346 347 public enum ConsentExceptType { 348 /** 349 * Consent is denied for actions meeting these rules 350 */ 351 DENY, 352 /** 353 * Consent is provided for actions meeting these rules 354 */ 355 PERMIT, 356 /** 357 * added to help the parsers with the generic types 358 */ 359 NULL; 360 public static ConsentExceptType fromCode(String codeString) throws FHIRException { 361 if (codeString == null || "".equals(codeString)) 362 return null; 363 if ("deny".equals(codeString)) 364 return DENY; 365 if ("permit".equals(codeString)) 366 return PERMIT; 367 if (Configuration.isAcceptInvalidEnums()) 368 return null; 369 else 370 throw new FHIRException("Unknown ConsentExceptType code '"+codeString+"'"); 371 } 372 public String toCode() { 373 switch (this) { 374 case DENY: return "deny"; 375 case PERMIT: return "permit"; 376 case NULL: return null; 377 default: return "?"; 378 } 379 } 380 public String getSystem() { 381 switch (this) { 382 case DENY: return "http://hl7.org/fhir/consent-except-type"; 383 case PERMIT: return "http://hl7.org/fhir/consent-except-type"; 384 case NULL: return null; 385 default: return "?"; 386 } 387 } 388 public String getDefinition() { 389 switch (this) { 390 case DENY: return "Consent is denied for actions meeting these rules"; 391 case PERMIT: return "Consent is provided for actions meeting these rules"; 392 case NULL: return null; 393 default: return "?"; 394 } 395 } 396 public String getDisplay() { 397 switch (this) { 398 case DENY: return "Opt Out"; 399 case PERMIT: return "Opt In"; 400 case NULL: return null; 401 default: return "?"; 402 } 403 } 404 } 405 406 public static class ConsentExceptTypeEnumFactory implements EnumFactory<ConsentExceptType> { 407 public ConsentExceptType fromCode(String codeString) throws IllegalArgumentException { 408 if (codeString == null || "".equals(codeString)) 409 if (codeString == null || "".equals(codeString)) 410 return null; 411 if ("deny".equals(codeString)) 412 return ConsentExceptType.DENY; 413 if ("permit".equals(codeString)) 414 return ConsentExceptType.PERMIT; 415 throw new IllegalArgumentException("Unknown ConsentExceptType code '"+codeString+"'"); 416 } 417 public Enumeration<ConsentExceptType> fromType(PrimitiveType<?> code) throws FHIRException { 418 if (code == null) 419 return null; 420 if (code.isEmpty()) 421 return new Enumeration<ConsentExceptType>(this); 422 String codeString = code.asStringValue(); 423 if (codeString == null || "".equals(codeString)) 424 return null; 425 if ("deny".equals(codeString)) 426 return new Enumeration<ConsentExceptType>(this, ConsentExceptType.DENY); 427 if ("permit".equals(codeString)) 428 return new Enumeration<ConsentExceptType>(this, ConsentExceptType.PERMIT); 429 throw new FHIRException("Unknown ConsentExceptType code '"+codeString+"'"); 430 } 431 public String toCode(ConsentExceptType code) { 432 if (code == ConsentExceptType.NULL) 433 return null; 434 if (code == ConsentExceptType.DENY) 435 return "deny"; 436 if (code == ConsentExceptType.PERMIT) 437 return "permit"; 438 return "?"; 439 } 440 public String toSystem(ConsentExceptType code) { 441 return code.getSystem(); 442 } 443 } 444 445 @Block() 446 public static class ConsentActorComponent extends BackboneElement implements IBaseBackboneElement { 447 /** 448 * How the individual is involved in the resources content that is described in the consent. 449 */ 450 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 451 @Description(shortDefinition="How the actor is involved", formalDefinition="How the individual is involved in the resources content that is described in the consent." ) 452 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 453 protected CodeableConcept role; 454 455 /** 456 * The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 457 */ 458 @Child(name = "reference", type = {Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 459 @Description(shortDefinition="Resource for the actor (or group, by role)", formalDefinition="The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 460 protected Reference reference; 461 462 /** 463 * The actual object that is the target of the reference (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 464 */ 465 protected Resource referenceTarget; 466 467 private static final long serialVersionUID = 1152919415L; 468 469 /** 470 * Constructor 471 */ 472 public ConsentActorComponent() { 473 super(); 474 } 475 476 /** 477 * Constructor 478 */ 479 public ConsentActorComponent(CodeableConcept role, Reference reference) { 480 super(); 481 this.role = role; 482 this.reference = reference; 483 } 484 485 /** 486 * @return {@link #role} (How the individual is involved in the resources content that is described in the consent.) 487 */ 488 public CodeableConcept getRole() { 489 if (this.role == null) 490 if (Configuration.errorOnAutoCreate()) 491 throw new Error("Attempt to auto-create ConsentActorComponent.role"); 492 else if (Configuration.doAutoCreate()) 493 this.role = new CodeableConcept(); // cc 494 return this.role; 495 } 496 497 public boolean hasRole() { 498 return this.role != null && !this.role.isEmpty(); 499 } 500 501 /** 502 * @param value {@link #role} (How the individual is involved in the resources content that is described in the consent.) 503 */ 504 public ConsentActorComponent setRole(CodeableConcept value) { 505 this.role = value; 506 return this; 507 } 508 509 /** 510 * @return {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 511 */ 512 public Reference getReference() { 513 if (this.reference == null) 514 if (Configuration.errorOnAutoCreate()) 515 throw new Error("Attempt to auto-create ConsentActorComponent.reference"); 516 else if (Configuration.doAutoCreate()) 517 this.reference = new Reference(); // cc 518 return this.reference; 519 } 520 521 public boolean hasReference() { 522 return this.reference != null && !this.reference.isEmpty(); 523 } 524 525 /** 526 * @param value {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 527 */ 528 public ConsentActorComponent setReference(Reference value) { 529 this.reference = value; 530 return this; 531 } 532 533 /** 534 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 535 */ 536 public Resource getReferenceTarget() { 537 return this.referenceTarget; 538 } 539 540 /** 541 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 542 */ 543 public ConsentActorComponent setReferenceTarget(Resource value) { 544 this.referenceTarget = value; 545 return this; 546 } 547 548 protected void listChildren(List<Property> children) { 549 super.listChildren(children); 550 children.add(new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the consent.", 0, 1, role)); 551 children.add(new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference)); 552 } 553 554 @Override 555 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 556 switch (_hash) { 557 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the consent.", 0, 1, role); 558 case -925155509: /*reference*/ return new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference); 559 default: return super.getNamedProperty(_hash, _name, _checkValid); 560 } 561 562 } 563 564 @Override 565 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 566 switch (hash) { 567 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 568 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 569 default: return super.getProperty(hash, name, checkValid); 570 } 571 572 } 573 574 @Override 575 public Base setProperty(int hash, String name, Base value) throws FHIRException { 576 switch (hash) { 577 case 3506294: // role 578 this.role = castToCodeableConcept(value); // CodeableConcept 579 return value; 580 case -925155509: // reference 581 this.reference = castToReference(value); // Reference 582 return value; 583 default: return super.setProperty(hash, name, value); 584 } 585 586 } 587 588 @Override 589 public Base setProperty(String name, Base value) throws FHIRException { 590 if (name.equals("role")) { 591 this.role = castToCodeableConcept(value); // CodeableConcept 592 } else if (name.equals("reference")) { 593 this.reference = castToReference(value); // Reference 594 } else 595 return super.setProperty(name, value); 596 return value; 597 } 598 599 @Override 600 public Base makeProperty(int hash, String name) throws FHIRException { 601 switch (hash) { 602 case 3506294: return getRole(); 603 case -925155509: return getReference(); 604 default: return super.makeProperty(hash, name); 605 } 606 607 } 608 609 @Override 610 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 611 switch (hash) { 612 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 613 case -925155509: /*reference*/ return new String[] {"Reference"}; 614 default: return super.getTypesForProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public Base addChild(String name) throws FHIRException { 621 if (name.equals("role")) { 622 this.role = new CodeableConcept(); 623 return this.role; 624 } 625 else if (name.equals("reference")) { 626 this.reference = new Reference(); 627 return this.reference; 628 } 629 else 630 return super.addChild(name); 631 } 632 633 public ConsentActorComponent copy() { 634 ConsentActorComponent dst = new ConsentActorComponent(); 635 copyValues(dst); 636 dst.role = role == null ? null : role.copy(); 637 dst.reference = reference == null ? null : reference.copy(); 638 return dst; 639 } 640 641 @Override 642 public boolean equalsDeep(Base other_) { 643 if (!super.equalsDeep(other_)) 644 return false; 645 if (!(other_ instanceof ConsentActorComponent)) 646 return false; 647 ConsentActorComponent o = (ConsentActorComponent) other_; 648 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 649 } 650 651 @Override 652 public boolean equalsShallow(Base other_) { 653 if (!super.equalsShallow(other_)) 654 return false; 655 if (!(other_ instanceof ConsentActorComponent)) 656 return false; 657 ConsentActorComponent o = (ConsentActorComponent) other_; 658 return true; 659 } 660 661 public boolean isEmpty() { 662 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 663 } 664 665 public String fhirType() { 666 return "Consent.actor"; 667 668 } 669 670 } 671 672 @Block() 673 public static class ConsentPolicyComponent extends BackboneElement implements IBaseBackboneElement { 674 /** 675 * Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives. 676 */ 677 @Child(name = "authority", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 678 @Description(shortDefinition="Enforcement source for policy", formalDefinition="Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives." ) 679 protected UriType authority; 680 681 /** 682 * The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 683 */ 684 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 685 @Description(shortDefinition="Specific policy covered by this consent", formalDefinition="The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law." ) 686 protected UriType uri; 687 688 private static final long serialVersionUID = 672275705L; 689 690 /** 691 * Constructor 692 */ 693 public ConsentPolicyComponent() { 694 super(); 695 } 696 697 /** 698 * @return {@link #authority} (Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.). This is the underlying object with id, value and extensions. The accessor "getAuthority" gives direct access to the value 699 */ 700 public UriType getAuthorityElement() { 701 if (this.authority == null) 702 if (Configuration.errorOnAutoCreate()) 703 throw new Error("Attempt to auto-create ConsentPolicyComponent.authority"); 704 else if (Configuration.doAutoCreate()) 705 this.authority = new UriType(); // bb 706 return this.authority; 707 } 708 709 public boolean hasAuthorityElement() { 710 return this.authority != null && !this.authority.isEmpty(); 711 } 712 713 public boolean hasAuthority() { 714 return this.authority != null && !this.authority.isEmpty(); 715 } 716 717 /** 718 * @param value {@link #authority} (Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.). This is the underlying object with id, value and extensions. The accessor "getAuthority" gives direct access to the value 719 */ 720 public ConsentPolicyComponent setAuthorityElement(UriType value) { 721 this.authority = value; 722 return this; 723 } 724 725 /** 726 * @return Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives. 727 */ 728 public String getAuthority() { 729 return this.authority == null ? null : this.authority.getValue(); 730 } 731 732 /** 733 * @param value Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives. 734 */ 735 public ConsentPolicyComponent setAuthority(String value) { 736 if (Utilities.noString(value)) 737 this.authority = null; 738 else { 739 if (this.authority == null) 740 this.authority = new UriType(); 741 this.authority.setValue(value); 742 } 743 return this; 744 } 745 746 /** 747 * @return {@link #uri} (The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 748 */ 749 public UriType getUriElement() { 750 if (this.uri == null) 751 if (Configuration.errorOnAutoCreate()) 752 throw new Error("Attempt to auto-create ConsentPolicyComponent.uri"); 753 else if (Configuration.doAutoCreate()) 754 this.uri = new UriType(); // bb 755 return this.uri; 756 } 757 758 public boolean hasUriElement() { 759 return this.uri != null && !this.uri.isEmpty(); 760 } 761 762 public boolean hasUri() { 763 return this.uri != null && !this.uri.isEmpty(); 764 } 765 766 /** 767 * @param value {@link #uri} (The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 768 */ 769 public ConsentPolicyComponent setUriElement(UriType value) { 770 this.uri = value; 771 return this; 772 } 773 774 /** 775 * @return The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 776 */ 777 public String getUri() { 778 return this.uri == null ? null : this.uri.getValue(); 779 } 780 781 /** 782 * @param value The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 783 */ 784 public ConsentPolicyComponent setUri(String value) { 785 if (Utilities.noString(value)) 786 this.uri = null; 787 else { 788 if (this.uri == null) 789 this.uri = new UriType(); 790 this.uri.setValue(value); 791 } 792 return this; 793 } 794 795 protected void listChildren(List<Property> children) { 796 super.listChildren(children); 797 children.add(new Property("authority", "uri", "Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.", 0, 1, authority)); 798 children.add(new Property("uri", "uri", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, 1, uri)); 799 } 800 801 @Override 802 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 803 switch (_hash) { 804 case 1475610435: /*authority*/ return new Property("authority", "uri", "Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.", 0, 1, authority); 805 case 116076: /*uri*/ return new Property("uri", "uri", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, 1, uri); 806 default: return super.getNamedProperty(_hash, _name, _checkValid); 807 } 808 809 } 810 811 @Override 812 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 813 switch (hash) { 814 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // UriType 815 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 816 default: return super.getProperty(hash, name, checkValid); 817 } 818 819 } 820 821 @Override 822 public Base setProperty(int hash, String name, Base value) throws FHIRException { 823 switch (hash) { 824 case 1475610435: // authority 825 this.authority = castToUri(value); // UriType 826 return value; 827 case 116076: // uri 828 this.uri = castToUri(value); // UriType 829 return value; 830 default: return super.setProperty(hash, name, value); 831 } 832 833 } 834 835 @Override 836 public Base setProperty(String name, Base value) throws FHIRException { 837 if (name.equals("authority")) { 838 this.authority = castToUri(value); // UriType 839 } else if (name.equals("uri")) { 840 this.uri = castToUri(value); // UriType 841 } else 842 return super.setProperty(name, value); 843 return value; 844 } 845 846 @Override 847 public Base makeProperty(int hash, String name) throws FHIRException { 848 switch (hash) { 849 case 1475610435: return getAuthorityElement(); 850 case 116076: return getUriElement(); 851 default: return super.makeProperty(hash, name); 852 } 853 854 } 855 856 @Override 857 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 858 switch (hash) { 859 case 1475610435: /*authority*/ return new String[] {"uri"}; 860 case 116076: /*uri*/ return new String[] {"uri"}; 861 default: return super.getTypesForProperty(hash, name); 862 } 863 864 } 865 866 @Override 867 public Base addChild(String name) throws FHIRException { 868 if (name.equals("authority")) { 869 throw new FHIRException("Cannot call addChild on a singleton property Consent.authority"); 870 } 871 else if (name.equals("uri")) { 872 throw new FHIRException("Cannot call addChild on a singleton property Consent.uri"); 873 } 874 else 875 return super.addChild(name); 876 } 877 878 public ConsentPolicyComponent copy() { 879 ConsentPolicyComponent dst = new ConsentPolicyComponent(); 880 copyValues(dst); 881 dst.authority = authority == null ? null : authority.copy(); 882 dst.uri = uri == null ? null : uri.copy(); 883 return dst; 884 } 885 886 @Override 887 public boolean equalsDeep(Base other_) { 888 if (!super.equalsDeep(other_)) 889 return false; 890 if (!(other_ instanceof ConsentPolicyComponent)) 891 return false; 892 ConsentPolicyComponent o = (ConsentPolicyComponent) other_; 893 return compareDeep(authority, o.authority, true) && compareDeep(uri, o.uri, true); 894 } 895 896 @Override 897 public boolean equalsShallow(Base other_) { 898 if (!super.equalsShallow(other_)) 899 return false; 900 if (!(other_ instanceof ConsentPolicyComponent)) 901 return false; 902 ConsentPolicyComponent o = (ConsentPolicyComponent) other_; 903 return compareValues(authority, o.authority, true) && compareValues(uri, o.uri, true); 904 } 905 906 public boolean isEmpty() { 907 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(authority, uri); 908 } 909 910 public String fhirType() { 911 return "Consent.policy"; 912 913 } 914 915 } 916 917 @Block() 918 public static class ConsentDataComponent extends BackboneElement implements IBaseBackboneElement { 919 /** 920 * How the resource reference is interpreted when testing consent restrictions. 921 */ 922 @Child(name = "meaning", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 923 @Description(shortDefinition="instance | related | dependents | authoredby", formalDefinition="How the resource reference is interpreted when testing consent restrictions." ) 924 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-data-meaning") 925 protected Enumeration<ConsentDataMeaning> meaning; 926 927 /** 928 * A reference to a specific resource that defines which resources are covered by this consent. 929 */ 930 @Child(name = "reference", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 931 @Description(shortDefinition="The actual data reference", formalDefinition="A reference to a specific resource that defines which resources are covered by this consent." ) 932 protected Reference reference; 933 934 /** 935 * The actual object that is the target of the reference (A reference to a specific resource that defines which resources are covered by this consent.) 936 */ 937 protected Resource referenceTarget; 938 939 private static final long serialVersionUID = -424898645L; 940 941 /** 942 * Constructor 943 */ 944 public ConsentDataComponent() { 945 super(); 946 } 947 948 /** 949 * Constructor 950 */ 951 public ConsentDataComponent(Enumeration<ConsentDataMeaning> meaning, Reference reference) { 952 super(); 953 this.meaning = meaning; 954 this.reference = reference; 955 } 956 957 /** 958 * @return {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 959 */ 960 public Enumeration<ConsentDataMeaning> getMeaningElement() { 961 if (this.meaning == null) 962 if (Configuration.errorOnAutoCreate()) 963 throw new Error("Attempt to auto-create ConsentDataComponent.meaning"); 964 else if (Configuration.doAutoCreate()) 965 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 966 return this.meaning; 967 } 968 969 public boolean hasMeaningElement() { 970 return this.meaning != null && !this.meaning.isEmpty(); 971 } 972 973 public boolean hasMeaning() { 974 return this.meaning != null && !this.meaning.isEmpty(); 975 } 976 977 /** 978 * @param value {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 979 */ 980 public ConsentDataComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 981 this.meaning = value; 982 return this; 983 } 984 985 /** 986 * @return How the resource reference is interpreted when testing consent restrictions. 987 */ 988 public ConsentDataMeaning getMeaning() { 989 return this.meaning == null ? null : this.meaning.getValue(); 990 } 991 992 /** 993 * @param value How the resource reference is interpreted when testing consent restrictions. 994 */ 995 public ConsentDataComponent setMeaning(ConsentDataMeaning value) { 996 if (this.meaning == null) 997 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 998 this.meaning.setValue(value); 999 return this; 1000 } 1001 1002 /** 1003 * @return {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 1004 */ 1005 public Reference getReference() { 1006 if (this.reference == null) 1007 if (Configuration.errorOnAutoCreate()) 1008 throw new Error("Attempt to auto-create ConsentDataComponent.reference"); 1009 else if (Configuration.doAutoCreate()) 1010 this.reference = new Reference(); // cc 1011 return this.reference; 1012 } 1013 1014 public boolean hasReference() { 1015 return this.reference != null && !this.reference.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 1020 */ 1021 public ConsentDataComponent setReference(Reference value) { 1022 this.reference = value; 1023 return this; 1024 } 1025 1026 /** 1027 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 1028 */ 1029 public Resource getReferenceTarget() { 1030 return this.referenceTarget; 1031 } 1032 1033 /** 1034 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 1035 */ 1036 public ConsentDataComponent setReferenceTarget(Resource value) { 1037 this.referenceTarget = value; 1038 return this; 1039 } 1040 1041 protected void listChildren(List<Property> children) { 1042 super.listChildren(children); 1043 children.add(new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 1044 children.add(new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference)); 1045 } 1046 1047 @Override 1048 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1049 switch (_hash) { 1050 case 938160637: /*meaning*/ return new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 1051 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference); 1052 default: return super.getNamedProperty(_hash, _name, _checkValid); 1053 } 1054 1055 } 1056 1057 @Override 1058 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1059 switch (hash) { 1060 case 938160637: /*meaning*/ return this.meaning == null ? new Base[0] : new Base[] {this.meaning}; // Enumeration<ConsentDataMeaning> 1061 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1062 default: return super.getProperty(hash, name, checkValid); 1063 } 1064 1065 } 1066 1067 @Override 1068 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1069 switch (hash) { 1070 case 938160637: // meaning 1071 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 1072 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 1073 return value; 1074 case -925155509: // reference 1075 this.reference = castToReference(value); // Reference 1076 return value; 1077 default: return super.setProperty(hash, name, value); 1078 } 1079 1080 } 1081 1082 @Override 1083 public Base setProperty(String name, Base value) throws FHIRException { 1084 if (name.equals("meaning")) { 1085 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 1086 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 1087 } else if (name.equals("reference")) { 1088 this.reference = castToReference(value); // Reference 1089 } else 1090 return super.setProperty(name, value); 1091 return value; 1092 } 1093 1094 @Override 1095 public Base makeProperty(int hash, String name) throws FHIRException { 1096 switch (hash) { 1097 case 938160637: return getMeaningElement(); 1098 case -925155509: return getReference(); 1099 default: return super.makeProperty(hash, name); 1100 } 1101 1102 } 1103 1104 @Override 1105 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1106 switch (hash) { 1107 case 938160637: /*meaning*/ return new String[] {"code"}; 1108 case -925155509: /*reference*/ return new String[] {"Reference"}; 1109 default: return super.getTypesForProperty(hash, name); 1110 } 1111 1112 } 1113 1114 @Override 1115 public Base addChild(String name) throws FHIRException { 1116 if (name.equals("meaning")) { 1117 throw new FHIRException("Cannot call addChild on a singleton property Consent.meaning"); 1118 } 1119 else if (name.equals("reference")) { 1120 this.reference = new Reference(); 1121 return this.reference; 1122 } 1123 else 1124 return super.addChild(name); 1125 } 1126 1127 public ConsentDataComponent copy() { 1128 ConsentDataComponent dst = new ConsentDataComponent(); 1129 copyValues(dst); 1130 dst.meaning = meaning == null ? null : meaning.copy(); 1131 dst.reference = reference == null ? null : reference.copy(); 1132 return dst; 1133 } 1134 1135 @Override 1136 public boolean equalsDeep(Base other_) { 1137 if (!super.equalsDeep(other_)) 1138 return false; 1139 if (!(other_ instanceof ConsentDataComponent)) 1140 return false; 1141 ConsentDataComponent o = (ConsentDataComponent) other_; 1142 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 1143 } 1144 1145 @Override 1146 public boolean equalsShallow(Base other_) { 1147 if (!super.equalsShallow(other_)) 1148 return false; 1149 if (!(other_ instanceof ConsentDataComponent)) 1150 return false; 1151 ConsentDataComponent o = (ConsentDataComponent) other_; 1152 return compareValues(meaning, o.meaning, true); 1153 } 1154 1155 public boolean isEmpty() { 1156 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 1157 } 1158 1159 public String fhirType() { 1160 return "Consent.data"; 1161 1162 } 1163 1164 } 1165 1166 @Block() 1167 public static class ExceptComponent extends BackboneElement implements IBaseBackboneElement { 1168 /** 1169 * Action to take - permit or deny - when the exception conditions are met. 1170 */ 1171 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1172 @Description(shortDefinition="deny | permit", formalDefinition="Action to take - permit or deny - when the exception conditions are met." ) 1173 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-except-type") 1174 protected Enumeration<ConsentExceptType> type; 1175 1176 /** 1177 * The timeframe in this exception is valid. 1178 */ 1179 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 1180 @Description(shortDefinition="Timeframe for this exception", formalDefinition="The timeframe in this exception is valid." ) 1181 protected Period period; 1182 1183 /** 1184 * Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 1185 */ 1186 @Child(name = "actor", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1187 @Description(shortDefinition="Who|what controlled by this exception (or group, by role)", formalDefinition="Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 1188 protected List<ExceptActorComponent> actor; 1189 1190 /** 1191 * Actions controlled by this Exception. 1192 */ 1193 @Child(name = "action", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1194 @Description(shortDefinition="Actions controlled by this exception", formalDefinition="Actions controlled by this Exception." ) 1195 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-action") 1196 protected List<CodeableConcept> action; 1197 1198 /** 1199 * A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels. 1200 */ 1201 @Child(name = "securityLabel", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1202 @Description(shortDefinition="Security Labels that define affected resources", formalDefinition="A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels." ) 1203 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 1204 protected List<Coding> securityLabel; 1205 1206 /** 1207 * The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception. 1208 */ 1209 @Child(name = "purpose", type = {Coding.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1210 @Description(shortDefinition="Context of activities covered by this exception", formalDefinition="The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception." ) 1211 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 1212 protected List<Coding> purpose; 1213 1214 /** 1215 * The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to. 1216 */ 1217 @Child(name = "class", type = {Coding.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1218 @Description(shortDefinition="e.g. Resource Type, Profile, or CDA etc", formalDefinition="The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to." ) 1219 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-class") 1220 protected List<Coding> class_; 1221 1222 /** 1223 * If this code is found in an instance, then the exception applies. 1224 */ 1225 @Child(name = "code", type = {Coding.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1226 @Description(shortDefinition="e.g. LOINC or SNOMED CT code, etc in the content", formalDefinition="If this code is found in an instance, then the exception applies." ) 1227 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-code") 1228 protected List<Coding> code; 1229 1230 /** 1231 * Clinical or Operational Relevant period of time that bounds the data controlled by this exception. 1232 */ 1233 @Child(name = "dataPeriod", type = {Period.class}, order=9, min=0, max=1, modifier=false, summary=true) 1234 @Description(shortDefinition="Timeframe for data controlled by this exception", formalDefinition="Clinical or Operational Relevant period of time that bounds the data controlled by this exception." ) 1235 protected Period dataPeriod; 1236 1237 /** 1238 * The resources controlled by this exception, if specific resources are referenced. 1239 */ 1240 @Child(name = "data", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1241 @Description(shortDefinition="Data controlled by this exception", formalDefinition="The resources controlled by this exception, if specific resources are referenced." ) 1242 protected List<ExceptDataComponent> data; 1243 1244 private static final long serialVersionUID = 1586636291L; 1245 1246 /** 1247 * Constructor 1248 */ 1249 public ExceptComponent() { 1250 super(); 1251 } 1252 1253 /** 1254 * Constructor 1255 */ 1256 public ExceptComponent(Enumeration<ConsentExceptType> type) { 1257 super(); 1258 this.type = type; 1259 } 1260 1261 /** 1262 * @return {@link #type} (Action to take - permit or deny - when the exception conditions are met.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1263 */ 1264 public Enumeration<ConsentExceptType> getTypeElement() { 1265 if (this.type == null) 1266 if (Configuration.errorOnAutoCreate()) 1267 throw new Error("Attempt to auto-create ExceptComponent.type"); 1268 else if (Configuration.doAutoCreate()) 1269 this.type = new Enumeration<ConsentExceptType>(new ConsentExceptTypeEnumFactory()); // bb 1270 return this.type; 1271 } 1272 1273 public boolean hasTypeElement() { 1274 return this.type != null && !this.type.isEmpty(); 1275 } 1276 1277 public boolean hasType() { 1278 return this.type != null && !this.type.isEmpty(); 1279 } 1280 1281 /** 1282 * @param value {@link #type} (Action to take - permit or deny - when the exception conditions are met.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1283 */ 1284 public ExceptComponent setTypeElement(Enumeration<ConsentExceptType> value) { 1285 this.type = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return Action to take - permit or deny - when the exception conditions are met. 1291 */ 1292 public ConsentExceptType getType() { 1293 return this.type == null ? null : this.type.getValue(); 1294 } 1295 1296 /** 1297 * @param value Action to take - permit or deny - when the exception conditions are met. 1298 */ 1299 public ExceptComponent setType(ConsentExceptType value) { 1300 if (this.type == null) 1301 this.type = new Enumeration<ConsentExceptType>(new ConsentExceptTypeEnumFactory()); 1302 this.type.setValue(value); 1303 return this; 1304 } 1305 1306 /** 1307 * @return {@link #period} (The timeframe in this exception is valid.) 1308 */ 1309 public Period getPeriod() { 1310 if (this.period == null) 1311 if (Configuration.errorOnAutoCreate()) 1312 throw new Error("Attempt to auto-create ExceptComponent.period"); 1313 else if (Configuration.doAutoCreate()) 1314 this.period = new Period(); // cc 1315 return this.period; 1316 } 1317 1318 public boolean hasPeriod() { 1319 return this.period != null && !this.period.isEmpty(); 1320 } 1321 1322 /** 1323 * @param value {@link #period} (The timeframe in this exception is valid.) 1324 */ 1325 public ExceptComponent setPeriod(Period value) { 1326 this.period = value; 1327 return this; 1328 } 1329 1330 /** 1331 * @return {@link #actor} (Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1332 */ 1333 public List<ExceptActorComponent> getActor() { 1334 if (this.actor == null) 1335 this.actor = new ArrayList<ExceptActorComponent>(); 1336 return this.actor; 1337 } 1338 1339 /** 1340 * @return Returns a reference to <code>this</code> for easy method chaining 1341 */ 1342 public ExceptComponent setActor(List<ExceptActorComponent> theActor) { 1343 this.actor = theActor; 1344 return this; 1345 } 1346 1347 public boolean hasActor() { 1348 if (this.actor == null) 1349 return false; 1350 for (ExceptActorComponent item : this.actor) 1351 if (!item.isEmpty()) 1352 return true; 1353 return false; 1354 } 1355 1356 public ExceptActorComponent addActor() { //3 1357 ExceptActorComponent t = new ExceptActorComponent(); 1358 if (this.actor == null) 1359 this.actor = new ArrayList<ExceptActorComponent>(); 1360 this.actor.add(t); 1361 return t; 1362 } 1363 1364 public ExceptComponent addActor(ExceptActorComponent t) { //3 1365 if (t == null) 1366 return this; 1367 if (this.actor == null) 1368 this.actor = new ArrayList<ExceptActorComponent>(); 1369 this.actor.add(t); 1370 return this; 1371 } 1372 1373 /** 1374 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist 1375 */ 1376 public ExceptActorComponent getActorFirstRep() { 1377 if (getActor().isEmpty()) { 1378 addActor(); 1379 } 1380 return getActor().get(0); 1381 } 1382 1383 /** 1384 * @return {@link #action} (Actions controlled by this Exception.) 1385 */ 1386 public List<CodeableConcept> getAction() { 1387 if (this.action == null) 1388 this.action = new ArrayList<CodeableConcept>(); 1389 return this.action; 1390 } 1391 1392 /** 1393 * @return Returns a reference to <code>this</code> for easy method chaining 1394 */ 1395 public ExceptComponent setAction(List<CodeableConcept> theAction) { 1396 this.action = theAction; 1397 return this; 1398 } 1399 1400 public boolean hasAction() { 1401 if (this.action == null) 1402 return false; 1403 for (CodeableConcept item : this.action) 1404 if (!item.isEmpty()) 1405 return true; 1406 return false; 1407 } 1408 1409 public CodeableConcept addAction() { //3 1410 CodeableConcept t = new CodeableConcept(); 1411 if (this.action == null) 1412 this.action = new ArrayList<CodeableConcept>(); 1413 this.action.add(t); 1414 return t; 1415 } 1416 1417 public ExceptComponent addAction(CodeableConcept t) { //3 1418 if (t == null) 1419 return this; 1420 if (this.action == null) 1421 this.action = new ArrayList<CodeableConcept>(); 1422 this.action.add(t); 1423 return this; 1424 } 1425 1426 /** 1427 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 1428 */ 1429 public CodeableConcept getActionFirstRep() { 1430 if (getAction().isEmpty()) { 1431 addAction(); 1432 } 1433 return getAction().get(0); 1434 } 1435 1436 /** 1437 * @return {@link #securityLabel} (A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels.) 1438 */ 1439 public List<Coding> getSecurityLabel() { 1440 if (this.securityLabel == null) 1441 this.securityLabel = new ArrayList<Coding>(); 1442 return this.securityLabel; 1443 } 1444 1445 /** 1446 * @return Returns a reference to <code>this</code> for easy method chaining 1447 */ 1448 public ExceptComponent setSecurityLabel(List<Coding> theSecurityLabel) { 1449 this.securityLabel = theSecurityLabel; 1450 return this; 1451 } 1452 1453 public boolean hasSecurityLabel() { 1454 if (this.securityLabel == null) 1455 return false; 1456 for (Coding item : this.securityLabel) 1457 if (!item.isEmpty()) 1458 return true; 1459 return false; 1460 } 1461 1462 public Coding addSecurityLabel() { //3 1463 Coding t = new Coding(); 1464 if (this.securityLabel == null) 1465 this.securityLabel = new ArrayList<Coding>(); 1466 this.securityLabel.add(t); 1467 return t; 1468 } 1469 1470 public ExceptComponent addSecurityLabel(Coding t) { //3 1471 if (t == null) 1472 return this; 1473 if (this.securityLabel == null) 1474 this.securityLabel = new ArrayList<Coding>(); 1475 this.securityLabel.add(t); 1476 return this; 1477 } 1478 1479 /** 1480 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 1481 */ 1482 public Coding getSecurityLabelFirstRep() { 1483 if (getSecurityLabel().isEmpty()) { 1484 addSecurityLabel(); 1485 } 1486 return getSecurityLabel().get(0); 1487 } 1488 1489 /** 1490 * @return {@link #purpose} (The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception.) 1491 */ 1492 public List<Coding> getPurpose() { 1493 if (this.purpose == null) 1494 this.purpose = new ArrayList<Coding>(); 1495 return this.purpose; 1496 } 1497 1498 /** 1499 * @return Returns a reference to <code>this</code> for easy method chaining 1500 */ 1501 public ExceptComponent setPurpose(List<Coding> thePurpose) { 1502 this.purpose = thePurpose; 1503 return this; 1504 } 1505 1506 public boolean hasPurpose() { 1507 if (this.purpose == null) 1508 return false; 1509 for (Coding item : this.purpose) 1510 if (!item.isEmpty()) 1511 return true; 1512 return false; 1513 } 1514 1515 public Coding addPurpose() { //3 1516 Coding t = new Coding(); 1517 if (this.purpose == null) 1518 this.purpose = new ArrayList<Coding>(); 1519 this.purpose.add(t); 1520 return t; 1521 } 1522 1523 public ExceptComponent addPurpose(Coding t) { //3 1524 if (t == null) 1525 return this; 1526 if (this.purpose == null) 1527 this.purpose = new ArrayList<Coding>(); 1528 this.purpose.add(t); 1529 return this; 1530 } 1531 1532 /** 1533 * @return The first repetition of repeating field {@link #purpose}, creating it if it does not already exist 1534 */ 1535 public Coding getPurposeFirstRep() { 1536 if (getPurpose().isEmpty()) { 1537 addPurpose(); 1538 } 1539 return getPurpose().get(0); 1540 } 1541 1542 /** 1543 * @return {@link #class_} (The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.) 1544 */ 1545 public List<Coding> getClass_() { 1546 if (this.class_ == null) 1547 this.class_ = new ArrayList<Coding>(); 1548 return this.class_; 1549 } 1550 1551 /** 1552 * @return Returns a reference to <code>this</code> for easy method chaining 1553 */ 1554 public ExceptComponent setClass_(List<Coding> theClass_) { 1555 this.class_ = theClass_; 1556 return this; 1557 } 1558 1559 public boolean hasClass_() { 1560 if (this.class_ == null) 1561 return false; 1562 for (Coding item : this.class_) 1563 if (!item.isEmpty()) 1564 return true; 1565 return false; 1566 } 1567 1568 public Coding addClass_() { //3 1569 Coding t = new Coding(); 1570 if (this.class_ == null) 1571 this.class_ = new ArrayList<Coding>(); 1572 this.class_.add(t); 1573 return t; 1574 } 1575 1576 public ExceptComponent addClass_(Coding t) { //3 1577 if (t == null) 1578 return this; 1579 if (this.class_ == null) 1580 this.class_ = new ArrayList<Coding>(); 1581 this.class_.add(t); 1582 return this; 1583 } 1584 1585 /** 1586 * @return The first repetition of repeating field {@link #class_}, creating it if it does not already exist 1587 */ 1588 public Coding getClass_FirstRep() { 1589 if (getClass_().isEmpty()) { 1590 addClass_(); 1591 } 1592 return getClass_().get(0); 1593 } 1594 1595 /** 1596 * @return {@link #code} (If this code is found in an instance, then the exception applies.) 1597 */ 1598 public List<Coding> getCode() { 1599 if (this.code == null) 1600 this.code = new ArrayList<Coding>(); 1601 return this.code; 1602 } 1603 1604 /** 1605 * @return Returns a reference to <code>this</code> for easy method chaining 1606 */ 1607 public ExceptComponent setCode(List<Coding> theCode) { 1608 this.code = theCode; 1609 return this; 1610 } 1611 1612 public boolean hasCode() { 1613 if (this.code == null) 1614 return false; 1615 for (Coding item : this.code) 1616 if (!item.isEmpty()) 1617 return true; 1618 return false; 1619 } 1620 1621 public Coding addCode() { //3 1622 Coding t = new Coding(); 1623 if (this.code == null) 1624 this.code = new ArrayList<Coding>(); 1625 this.code.add(t); 1626 return t; 1627 } 1628 1629 public ExceptComponent addCode(Coding t) { //3 1630 if (t == null) 1631 return this; 1632 if (this.code == null) 1633 this.code = new ArrayList<Coding>(); 1634 this.code.add(t); 1635 return this; 1636 } 1637 1638 /** 1639 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 1640 */ 1641 public Coding getCodeFirstRep() { 1642 if (getCode().isEmpty()) { 1643 addCode(); 1644 } 1645 return getCode().get(0); 1646 } 1647 1648 /** 1649 * @return {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this exception.) 1650 */ 1651 public Period getDataPeriod() { 1652 if (this.dataPeriod == null) 1653 if (Configuration.errorOnAutoCreate()) 1654 throw new Error("Attempt to auto-create ExceptComponent.dataPeriod"); 1655 else if (Configuration.doAutoCreate()) 1656 this.dataPeriod = new Period(); // cc 1657 return this.dataPeriod; 1658 } 1659 1660 public boolean hasDataPeriod() { 1661 return this.dataPeriod != null && !this.dataPeriod.isEmpty(); 1662 } 1663 1664 /** 1665 * @param value {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this exception.) 1666 */ 1667 public ExceptComponent setDataPeriod(Period value) { 1668 this.dataPeriod = value; 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #data} (The resources controlled by this exception, if specific resources are referenced.) 1674 */ 1675 public List<ExceptDataComponent> getData() { 1676 if (this.data == null) 1677 this.data = new ArrayList<ExceptDataComponent>(); 1678 return this.data; 1679 } 1680 1681 /** 1682 * @return Returns a reference to <code>this</code> for easy method chaining 1683 */ 1684 public ExceptComponent setData(List<ExceptDataComponent> theData) { 1685 this.data = theData; 1686 return this; 1687 } 1688 1689 public boolean hasData() { 1690 if (this.data == null) 1691 return false; 1692 for (ExceptDataComponent item : this.data) 1693 if (!item.isEmpty()) 1694 return true; 1695 return false; 1696 } 1697 1698 public ExceptDataComponent addData() { //3 1699 ExceptDataComponent t = new ExceptDataComponent(); 1700 if (this.data == null) 1701 this.data = new ArrayList<ExceptDataComponent>(); 1702 this.data.add(t); 1703 return t; 1704 } 1705 1706 public ExceptComponent addData(ExceptDataComponent t) { //3 1707 if (t == null) 1708 return this; 1709 if (this.data == null) 1710 this.data = new ArrayList<ExceptDataComponent>(); 1711 this.data.add(t); 1712 return this; 1713 } 1714 1715 /** 1716 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist 1717 */ 1718 public ExceptDataComponent getDataFirstRep() { 1719 if (getData().isEmpty()) { 1720 addData(); 1721 } 1722 return getData().get(0); 1723 } 1724 1725 protected void listChildren(List<Property> children) { 1726 super.listChildren(children); 1727 children.add(new Property("type", "code", "Action to take - permit or deny - when the exception conditions are met.", 0, 1, type)); 1728 children.add(new Property("period", "Period", "The timeframe in this exception is valid.", 0, 1, period)); 1729 children.add(new Property("actor", "", "Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor)); 1730 children.add(new Property("action", "CodeableConcept", "Actions controlled by this Exception.", 0, java.lang.Integer.MAX_VALUE, action)); 1731 children.add(new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 1732 children.add(new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, purpose)); 1733 children.add(new Property("class", "Coding", "The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, class_)); 1734 children.add(new Property("code", "Coding", "If this code is found in an instance, then the exception applies.", 0, java.lang.Integer.MAX_VALUE, code)); 1735 children.add(new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this exception.", 0, 1, dataPeriod)); 1736 children.add(new Property("data", "", "The resources controlled by this exception, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data)); 1737 } 1738 1739 @Override 1740 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1741 switch (_hash) { 1742 case 3575610: /*type*/ return new Property("type", "code", "Action to take - permit or deny - when the exception conditions are met.", 0, 1, type); 1743 case -991726143: /*period*/ return new Property("period", "Period", "The timeframe in this exception is valid.", 0, 1, period); 1744 case 92645877: /*actor*/ return new Property("actor", "", "Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor); 1745 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Actions controlled by this Exception.", 0, java.lang.Integer.MAX_VALUE, action); 1746 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 1747 case -220463842: /*purpose*/ return new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, purpose); 1748 case 94742904: /*class*/ return new Property("class", "Coding", "The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, class_); 1749 case 3059181: /*code*/ return new Property("code", "Coding", "If this code is found in an instance, then the exception applies.", 0, java.lang.Integer.MAX_VALUE, code); 1750 case 1177250315: /*dataPeriod*/ return new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this exception.", 0, 1, dataPeriod); 1751 case 3076010: /*data*/ return new Property("data", "", "The resources controlled by this exception, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data); 1752 default: return super.getNamedProperty(_hash, _name, _checkValid); 1753 } 1754 1755 } 1756 1757 @Override 1758 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1759 switch (hash) { 1760 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ConsentExceptType> 1761 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1762 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ExceptActorComponent 1763 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 1764 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 1765 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Coding 1766 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // Coding 1767 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 1768 case 1177250315: /*dataPeriod*/ return this.dataPeriod == null ? new Base[0] : new Base[] {this.dataPeriod}; // Period 1769 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // ExceptDataComponent 1770 default: return super.getProperty(hash, name, checkValid); 1771 } 1772 1773 } 1774 1775 @Override 1776 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1777 switch (hash) { 1778 case 3575610: // type 1779 value = new ConsentExceptTypeEnumFactory().fromType(castToCode(value)); 1780 this.type = (Enumeration) value; // Enumeration<ConsentExceptType> 1781 return value; 1782 case -991726143: // period 1783 this.period = castToPeriod(value); // Period 1784 return value; 1785 case 92645877: // actor 1786 this.getActor().add((ExceptActorComponent) value); // ExceptActorComponent 1787 return value; 1788 case -1422950858: // action 1789 this.getAction().add(castToCodeableConcept(value)); // CodeableConcept 1790 return value; 1791 case -722296940: // securityLabel 1792 this.getSecurityLabel().add(castToCoding(value)); // Coding 1793 return value; 1794 case -220463842: // purpose 1795 this.getPurpose().add(castToCoding(value)); // Coding 1796 return value; 1797 case 94742904: // class 1798 this.getClass_().add(castToCoding(value)); // Coding 1799 return value; 1800 case 3059181: // code 1801 this.getCode().add(castToCoding(value)); // Coding 1802 return value; 1803 case 1177250315: // dataPeriod 1804 this.dataPeriod = castToPeriod(value); // Period 1805 return value; 1806 case 3076010: // data 1807 this.getData().add((ExceptDataComponent) value); // ExceptDataComponent 1808 return value; 1809 default: return super.setProperty(hash, name, value); 1810 } 1811 1812 } 1813 1814 @Override 1815 public Base setProperty(String name, Base value) throws FHIRException { 1816 if (name.equals("type")) { 1817 value = new ConsentExceptTypeEnumFactory().fromType(castToCode(value)); 1818 this.type = (Enumeration) value; // Enumeration<ConsentExceptType> 1819 } else if (name.equals("period")) { 1820 this.period = castToPeriod(value); // Period 1821 } else if (name.equals("actor")) { 1822 this.getActor().add((ExceptActorComponent) value); 1823 } else if (name.equals("action")) { 1824 this.getAction().add(castToCodeableConcept(value)); 1825 } else if (name.equals("securityLabel")) { 1826 this.getSecurityLabel().add(castToCoding(value)); 1827 } else if (name.equals("purpose")) { 1828 this.getPurpose().add(castToCoding(value)); 1829 } else if (name.equals("class")) { 1830 this.getClass_().add(castToCoding(value)); 1831 } else if (name.equals("code")) { 1832 this.getCode().add(castToCoding(value)); 1833 } else if (name.equals("dataPeriod")) { 1834 this.dataPeriod = castToPeriod(value); // Period 1835 } else if (name.equals("data")) { 1836 this.getData().add((ExceptDataComponent) value); 1837 } else 1838 return super.setProperty(name, value); 1839 return value; 1840 } 1841 1842 @Override 1843 public Base makeProperty(int hash, String name) throws FHIRException { 1844 switch (hash) { 1845 case 3575610: return getTypeElement(); 1846 case -991726143: return getPeriod(); 1847 case 92645877: return addActor(); 1848 case -1422950858: return addAction(); 1849 case -722296940: return addSecurityLabel(); 1850 case -220463842: return addPurpose(); 1851 case 94742904: return addClass_(); 1852 case 3059181: return addCode(); 1853 case 1177250315: return getDataPeriod(); 1854 case 3076010: return addData(); 1855 default: return super.makeProperty(hash, name); 1856 } 1857 1858 } 1859 1860 @Override 1861 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1862 switch (hash) { 1863 case 3575610: /*type*/ return new String[] {"code"}; 1864 case -991726143: /*period*/ return new String[] {"Period"}; 1865 case 92645877: /*actor*/ return new String[] {}; 1866 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 1867 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 1868 case -220463842: /*purpose*/ return new String[] {"Coding"}; 1869 case 94742904: /*class*/ return new String[] {"Coding"}; 1870 case 3059181: /*code*/ return new String[] {"Coding"}; 1871 case 1177250315: /*dataPeriod*/ return new String[] {"Period"}; 1872 case 3076010: /*data*/ return new String[] {}; 1873 default: return super.getTypesForProperty(hash, name); 1874 } 1875 1876 } 1877 1878 @Override 1879 public Base addChild(String name) throws FHIRException { 1880 if (name.equals("type")) { 1881 throw new FHIRException("Cannot call addChild on a singleton property Consent.type"); 1882 } 1883 else if (name.equals("period")) { 1884 this.period = new Period(); 1885 return this.period; 1886 } 1887 else if (name.equals("actor")) { 1888 return addActor(); 1889 } 1890 else if (name.equals("action")) { 1891 return addAction(); 1892 } 1893 else if (name.equals("securityLabel")) { 1894 return addSecurityLabel(); 1895 } 1896 else if (name.equals("purpose")) { 1897 return addPurpose(); 1898 } 1899 else if (name.equals("class")) { 1900 return addClass_(); 1901 } 1902 else if (name.equals("code")) { 1903 return addCode(); 1904 } 1905 else if (name.equals("dataPeriod")) { 1906 this.dataPeriod = new Period(); 1907 return this.dataPeriod; 1908 } 1909 else if (name.equals("data")) { 1910 return addData(); 1911 } 1912 else 1913 return super.addChild(name); 1914 } 1915 1916 public ExceptComponent copy() { 1917 ExceptComponent dst = new ExceptComponent(); 1918 copyValues(dst); 1919 dst.type = type == null ? null : type.copy(); 1920 dst.period = period == null ? null : period.copy(); 1921 if (actor != null) { 1922 dst.actor = new ArrayList<ExceptActorComponent>(); 1923 for (ExceptActorComponent i : actor) 1924 dst.actor.add(i.copy()); 1925 }; 1926 if (action != null) { 1927 dst.action = new ArrayList<CodeableConcept>(); 1928 for (CodeableConcept i : action) 1929 dst.action.add(i.copy()); 1930 }; 1931 if (securityLabel != null) { 1932 dst.securityLabel = new ArrayList<Coding>(); 1933 for (Coding i : securityLabel) 1934 dst.securityLabel.add(i.copy()); 1935 }; 1936 if (purpose != null) { 1937 dst.purpose = new ArrayList<Coding>(); 1938 for (Coding i : purpose) 1939 dst.purpose.add(i.copy()); 1940 }; 1941 if (class_ != null) { 1942 dst.class_ = new ArrayList<Coding>(); 1943 for (Coding i : class_) 1944 dst.class_.add(i.copy()); 1945 }; 1946 if (code != null) { 1947 dst.code = new ArrayList<Coding>(); 1948 for (Coding i : code) 1949 dst.code.add(i.copy()); 1950 }; 1951 dst.dataPeriod = dataPeriod == null ? null : dataPeriod.copy(); 1952 if (data != null) { 1953 dst.data = new ArrayList<ExceptDataComponent>(); 1954 for (ExceptDataComponent i : data) 1955 dst.data.add(i.copy()); 1956 }; 1957 return dst; 1958 } 1959 1960 @Override 1961 public boolean equalsDeep(Base other_) { 1962 if (!super.equalsDeep(other_)) 1963 return false; 1964 if (!(other_ instanceof ExceptComponent)) 1965 return false; 1966 ExceptComponent o = (ExceptComponent) other_; 1967 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) && compareDeep(actor, o.actor, true) 1968 && compareDeep(action, o.action, true) && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(purpose, o.purpose, true) 1969 && compareDeep(class_, o.class_, true) && compareDeep(code, o.code, true) && compareDeep(dataPeriod, o.dataPeriod, true) 1970 && compareDeep(data, o.data, true); 1971 } 1972 1973 @Override 1974 public boolean equalsShallow(Base other_) { 1975 if (!super.equalsShallow(other_)) 1976 return false; 1977 if (!(other_ instanceof ExceptComponent)) 1978 return false; 1979 ExceptComponent o = (ExceptComponent) other_; 1980 return compareValues(type, o.type, true); 1981 } 1982 1983 public boolean isEmpty() { 1984 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, actor, action 1985 , securityLabel, purpose, class_, code, dataPeriod, data); 1986 } 1987 1988 public String fhirType() { 1989 return "Consent.except"; 1990 1991 } 1992 1993 } 1994 1995 @Block() 1996 public static class ExceptActorComponent extends BackboneElement implements IBaseBackboneElement { 1997 /** 1998 * How the individual is involved in the resources content that is described in the exception. 1999 */ 2000 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2001 @Description(shortDefinition="How the actor is involved", formalDefinition="How the individual is involved in the resources content that is described in the exception." ) 2002 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 2003 protected CodeableConcept role; 2004 2005 /** 2006 * The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 2007 */ 2008 @Child(name = "reference", type = {Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 2009 @Description(shortDefinition="Resource for the actor (or group, by role)", formalDefinition="The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 2010 protected Reference reference; 2011 2012 /** 2013 * The actual object that is the target of the reference (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2014 */ 2015 protected Resource referenceTarget; 2016 2017 private static final long serialVersionUID = 1152919415L; 2018 2019 /** 2020 * Constructor 2021 */ 2022 public ExceptActorComponent() { 2023 super(); 2024 } 2025 2026 /** 2027 * Constructor 2028 */ 2029 public ExceptActorComponent(CodeableConcept role, Reference reference) { 2030 super(); 2031 this.role = role; 2032 this.reference = reference; 2033 } 2034 2035 /** 2036 * @return {@link #role} (How the individual is involved in the resources content that is described in the exception.) 2037 */ 2038 public CodeableConcept getRole() { 2039 if (this.role == null) 2040 if (Configuration.errorOnAutoCreate()) 2041 throw new Error("Attempt to auto-create ExceptActorComponent.role"); 2042 else if (Configuration.doAutoCreate()) 2043 this.role = new CodeableConcept(); // cc 2044 return this.role; 2045 } 2046 2047 public boolean hasRole() { 2048 return this.role != null && !this.role.isEmpty(); 2049 } 2050 2051 /** 2052 * @param value {@link #role} (How the individual is involved in the resources content that is described in the exception.) 2053 */ 2054 public ExceptActorComponent setRole(CodeableConcept value) { 2055 this.role = value; 2056 return this; 2057 } 2058 2059 /** 2060 * @return {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2061 */ 2062 public Reference getReference() { 2063 if (this.reference == null) 2064 if (Configuration.errorOnAutoCreate()) 2065 throw new Error("Attempt to auto-create ExceptActorComponent.reference"); 2066 else if (Configuration.doAutoCreate()) 2067 this.reference = new Reference(); // cc 2068 return this.reference; 2069 } 2070 2071 public boolean hasReference() { 2072 return this.reference != null && !this.reference.isEmpty(); 2073 } 2074 2075 /** 2076 * @param value {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2077 */ 2078 public ExceptActorComponent setReference(Reference value) { 2079 this.reference = value; 2080 return this; 2081 } 2082 2083 /** 2084 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2085 */ 2086 public Resource getReferenceTarget() { 2087 return this.referenceTarget; 2088 } 2089 2090 /** 2091 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2092 */ 2093 public ExceptActorComponent setReferenceTarget(Resource value) { 2094 this.referenceTarget = value; 2095 return this; 2096 } 2097 2098 protected void listChildren(List<Property> children) { 2099 super.listChildren(children); 2100 children.add(new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role)); 2101 children.add(new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference)); 2102 } 2103 2104 @Override 2105 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2106 switch (_hash) { 2107 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role); 2108 case -925155509: /*reference*/ return new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference); 2109 default: return super.getNamedProperty(_hash, _name, _checkValid); 2110 } 2111 2112 } 2113 2114 @Override 2115 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2116 switch (hash) { 2117 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 2118 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2119 default: return super.getProperty(hash, name, checkValid); 2120 } 2121 2122 } 2123 2124 @Override 2125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2126 switch (hash) { 2127 case 3506294: // role 2128 this.role = castToCodeableConcept(value); // CodeableConcept 2129 return value; 2130 case -925155509: // reference 2131 this.reference = castToReference(value); // Reference 2132 return value; 2133 default: return super.setProperty(hash, name, value); 2134 } 2135 2136 } 2137 2138 @Override 2139 public Base setProperty(String name, Base value) throws FHIRException { 2140 if (name.equals("role")) { 2141 this.role = castToCodeableConcept(value); // CodeableConcept 2142 } else if (name.equals("reference")) { 2143 this.reference = castToReference(value); // Reference 2144 } else 2145 return super.setProperty(name, value); 2146 return value; 2147 } 2148 2149 @Override 2150 public Base makeProperty(int hash, String name) throws FHIRException { 2151 switch (hash) { 2152 case 3506294: return getRole(); 2153 case -925155509: return getReference(); 2154 default: return super.makeProperty(hash, name); 2155 } 2156 2157 } 2158 2159 @Override 2160 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2161 switch (hash) { 2162 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2163 case -925155509: /*reference*/ return new String[] {"Reference"}; 2164 default: return super.getTypesForProperty(hash, name); 2165 } 2166 2167 } 2168 2169 @Override 2170 public Base addChild(String name) throws FHIRException { 2171 if (name.equals("role")) { 2172 this.role = new CodeableConcept(); 2173 return this.role; 2174 } 2175 else if (name.equals("reference")) { 2176 this.reference = new Reference(); 2177 return this.reference; 2178 } 2179 else 2180 return super.addChild(name); 2181 } 2182 2183 public ExceptActorComponent copy() { 2184 ExceptActorComponent dst = new ExceptActorComponent(); 2185 copyValues(dst); 2186 dst.role = role == null ? null : role.copy(); 2187 dst.reference = reference == null ? null : reference.copy(); 2188 return dst; 2189 } 2190 2191 @Override 2192 public boolean equalsDeep(Base other_) { 2193 if (!super.equalsDeep(other_)) 2194 return false; 2195 if (!(other_ instanceof ExceptActorComponent)) 2196 return false; 2197 ExceptActorComponent o = (ExceptActorComponent) other_; 2198 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 2199 } 2200 2201 @Override 2202 public boolean equalsShallow(Base other_) { 2203 if (!super.equalsShallow(other_)) 2204 return false; 2205 if (!(other_ instanceof ExceptActorComponent)) 2206 return false; 2207 ExceptActorComponent o = (ExceptActorComponent) other_; 2208 return true; 2209 } 2210 2211 public boolean isEmpty() { 2212 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 2213 } 2214 2215 public String fhirType() { 2216 return "Consent.except.actor"; 2217 2218 } 2219 2220 } 2221 2222 @Block() 2223 public static class ExceptDataComponent extends BackboneElement implements IBaseBackboneElement { 2224 /** 2225 * How the resource reference is interpreted when testing consent restrictions. 2226 */ 2227 @Child(name = "meaning", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2228 @Description(shortDefinition="instance | related | dependents | authoredby", formalDefinition="How the resource reference is interpreted when testing consent restrictions." ) 2229 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-data-meaning") 2230 protected Enumeration<ConsentDataMeaning> meaning; 2231 2232 /** 2233 * A reference to a specific resource that defines which resources are covered by this consent. 2234 */ 2235 @Child(name = "reference", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 2236 @Description(shortDefinition="The actual data reference", formalDefinition="A reference to a specific resource that defines which resources are covered by this consent." ) 2237 protected Reference reference; 2238 2239 /** 2240 * The actual object that is the target of the reference (A reference to a specific resource that defines which resources are covered by this consent.) 2241 */ 2242 protected Resource referenceTarget; 2243 2244 private static final long serialVersionUID = -424898645L; 2245 2246 /** 2247 * Constructor 2248 */ 2249 public ExceptDataComponent() { 2250 super(); 2251 } 2252 2253 /** 2254 * Constructor 2255 */ 2256 public ExceptDataComponent(Enumeration<ConsentDataMeaning> meaning, Reference reference) { 2257 super(); 2258 this.meaning = meaning; 2259 this.reference = reference; 2260 } 2261 2262 /** 2263 * @return {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2264 */ 2265 public Enumeration<ConsentDataMeaning> getMeaningElement() { 2266 if (this.meaning == null) 2267 if (Configuration.errorOnAutoCreate()) 2268 throw new Error("Attempt to auto-create ExceptDataComponent.meaning"); 2269 else if (Configuration.doAutoCreate()) 2270 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 2271 return this.meaning; 2272 } 2273 2274 public boolean hasMeaningElement() { 2275 return this.meaning != null && !this.meaning.isEmpty(); 2276 } 2277 2278 public boolean hasMeaning() { 2279 return this.meaning != null && !this.meaning.isEmpty(); 2280 } 2281 2282 /** 2283 * @param value {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2284 */ 2285 public ExceptDataComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 2286 this.meaning = value; 2287 return this; 2288 } 2289 2290 /** 2291 * @return How the resource reference is interpreted when testing consent restrictions. 2292 */ 2293 public ConsentDataMeaning getMeaning() { 2294 return this.meaning == null ? null : this.meaning.getValue(); 2295 } 2296 2297 /** 2298 * @param value How the resource reference is interpreted when testing consent restrictions. 2299 */ 2300 public ExceptDataComponent setMeaning(ConsentDataMeaning value) { 2301 if (this.meaning == null) 2302 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 2303 this.meaning.setValue(value); 2304 return this; 2305 } 2306 2307 /** 2308 * @return {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2309 */ 2310 public Reference getReference() { 2311 if (this.reference == null) 2312 if (Configuration.errorOnAutoCreate()) 2313 throw new Error("Attempt to auto-create ExceptDataComponent.reference"); 2314 else if (Configuration.doAutoCreate()) 2315 this.reference = new Reference(); // cc 2316 return this.reference; 2317 } 2318 2319 public boolean hasReference() { 2320 return this.reference != null && !this.reference.isEmpty(); 2321 } 2322 2323 /** 2324 * @param value {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2325 */ 2326 public ExceptDataComponent setReference(Reference value) { 2327 this.reference = value; 2328 return this; 2329 } 2330 2331 /** 2332 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 2333 */ 2334 public Resource getReferenceTarget() { 2335 return this.referenceTarget; 2336 } 2337 2338 /** 2339 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 2340 */ 2341 public ExceptDataComponent setReferenceTarget(Resource value) { 2342 this.referenceTarget = value; 2343 return this; 2344 } 2345 2346 protected void listChildren(List<Property> children) { 2347 super.listChildren(children); 2348 children.add(new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 2349 children.add(new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference)); 2350 } 2351 2352 @Override 2353 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2354 switch (_hash) { 2355 case 938160637: /*meaning*/ return new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 2356 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference); 2357 default: return super.getNamedProperty(_hash, _name, _checkValid); 2358 } 2359 2360 } 2361 2362 @Override 2363 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2364 switch (hash) { 2365 case 938160637: /*meaning*/ return this.meaning == null ? new Base[0] : new Base[] {this.meaning}; // Enumeration<ConsentDataMeaning> 2366 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2367 default: return super.getProperty(hash, name, checkValid); 2368 } 2369 2370 } 2371 2372 @Override 2373 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2374 switch (hash) { 2375 case 938160637: // meaning 2376 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 2377 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2378 return value; 2379 case -925155509: // reference 2380 this.reference = castToReference(value); // Reference 2381 return value; 2382 default: return super.setProperty(hash, name, value); 2383 } 2384 2385 } 2386 2387 @Override 2388 public Base setProperty(String name, Base value) throws FHIRException { 2389 if (name.equals("meaning")) { 2390 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 2391 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2392 } else if (name.equals("reference")) { 2393 this.reference = castToReference(value); // Reference 2394 } else 2395 return super.setProperty(name, value); 2396 return value; 2397 } 2398 2399 @Override 2400 public Base makeProperty(int hash, String name) throws FHIRException { 2401 switch (hash) { 2402 case 938160637: return getMeaningElement(); 2403 case -925155509: return getReference(); 2404 default: return super.makeProperty(hash, name); 2405 } 2406 2407 } 2408 2409 @Override 2410 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2411 switch (hash) { 2412 case 938160637: /*meaning*/ return new String[] {"code"}; 2413 case -925155509: /*reference*/ return new String[] {"Reference"}; 2414 default: return super.getTypesForProperty(hash, name); 2415 } 2416 2417 } 2418 2419 @Override 2420 public Base addChild(String name) throws FHIRException { 2421 if (name.equals("meaning")) { 2422 throw new FHIRException("Cannot call addChild on a singleton property Consent.meaning"); 2423 } 2424 else if (name.equals("reference")) { 2425 this.reference = new Reference(); 2426 return this.reference; 2427 } 2428 else 2429 return super.addChild(name); 2430 } 2431 2432 public ExceptDataComponent copy() { 2433 ExceptDataComponent dst = new ExceptDataComponent(); 2434 copyValues(dst); 2435 dst.meaning = meaning == null ? null : meaning.copy(); 2436 dst.reference = reference == null ? null : reference.copy(); 2437 return dst; 2438 } 2439 2440 @Override 2441 public boolean equalsDeep(Base other_) { 2442 if (!super.equalsDeep(other_)) 2443 return false; 2444 if (!(other_ instanceof ExceptDataComponent)) 2445 return false; 2446 ExceptDataComponent o = (ExceptDataComponent) other_; 2447 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 2448 } 2449 2450 @Override 2451 public boolean equalsShallow(Base other_) { 2452 if (!super.equalsShallow(other_)) 2453 return false; 2454 if (!(other_ instanceof ExceptDataComponent)) 2455 return false; 2456 ExceptDataComponent o = (ExceptDataComponent) other_; 2457 return compareValues(meaning, o.meaning, true); 2458 } 2459 2460 public boolean isEmpty() { 2461 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 2462 } 2463 2464 public String fhirType() { 2465 return "Consent.except.data"; 2466 2467 } 2468 2469 } 2470 2471 /** 2472 * Unique identifier for this copy of the Consent Statement. 2473 */ 2474 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2475 @Description(shortDefinition="Identifier for this record (external references)", formalDefinition="Unique identifier for this copy of the Consent Statement." ) 2476 protected Identifier identifier; 2477 2478 /** 2479 * Indicates the current state of this consent. 2480 */ 2481 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2482 @Description(shortDefinition="draft | proposed | active | rejected | inactive | entered-in-error", formalDefinition="Indicates the current state of this consent." ) 2483 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-state-codes") 2484 protected Enumeration<ConsentState> status; 2485 2486 /** 2487 * A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements. 2488 */ 2489 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2490 @Description(shortDefinition="Classification of the consent statement - for indexing/retrieval", formalDefinition="A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements." ) 2491 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-category") 2492 protected List<CodeableConcept> category; 2493 2494 /** 2495 * The patient/healthcare consumer to whom this consent applies. 2496 */ 2497 @Child(name = "patient", type = {Patient.class}, order=3, min=1, max=1, modifier=false, summary=true) 2498 @Description(shortDefinition="Who the consent applies to", formalDefinition="The patient/healthcare consumer to whom this consent applies." ) 2499 protected Reference patient; 2500 2501 /** 2502 * The actual object that is the target of the reference (The patient/healthcare consumer to whom this consent applies.) 2503 */ 2504 protected Patient patientTarget; 2505 2506 /** 2507 * Relevant time or time-period when this Consent is applicable. 2508 */ 2509 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 2510 @Description(shortDefinition="Period that this consent applies", formalDefinition="Relevant time or time-period when this Consent is applicable." ) 2511 protected Period period; 2512 2513 /** 2514 * When this Consent was issued / created / indexed. 2515 */ 2516 @Child(name = "dateTime", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2517 @Description(shortDefinition="When this Consent was created or indexed", formalDefinition="When this Consent was issued / created / indexed." ) 2518 protected DateTimeType dateTime; 2519 2520 /** 2521 * Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions. 2522 */ 2523 @Child(name = "consentingParty", type = {Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2524 @Description(shortDefinition="Who is agreeing to the policy and exceptions", formalDefinition="Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions." ) 2525 protected List<Reference> consentingParty; 2526 /** 2527 * The actual objects that are the target of the reference (Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.) 2528 */ 2529 protected List<Resource> consentingPartyTarget; 2530 2531 2532 /** 2533 * Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 2534 */ 2535 @Child(name = "actor", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2536 @Description(shortDefinition="Who|what controlled by this consent (or group, by role)", formalDefinition="Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 2537 protected List<ConsentActorComponent> actor; 2538 2539 /** 2540 * Actions controlled by this consent. 2541 */ 2542 @Child(name = "action", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2543 @Description(shortDefinition="Actions controlled by this consent", formalDefinition="Actions controlled by this consent." ) 2544 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-action") 2545 protected List<CodeableConcept> action; 2546 2547 /** 2548 * The organization that manages the consent, and the framework within which it is executed. 2549 */ 2550 @Child(name = "organization", type = {Organization.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2551 @Description(shortDefinition="Custodian of the consent", formalDefinition="The organization that manages the consent, and the framework within which it is executed." ) 2552 protected List<Reference> organization; 2553 /** 2554 * The actual objects that are the target of the reference (The organization that manages the consent, and the framework within which it is executed.) 2555 */ 2556 protected List<Organization> organizationTarget; 2557 2558 2559 /** 2560 * The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document. 2561 */ 2562 @Child(name = "source", type = {Attachment.class, Identifier.class, Consent.class, DocumentReference.class, Contract.class, QuestionnaireResponse.class}, order=10, min=0, max=1, modifier=false, summary=true) 2563 @Description(shortDefinition="Source from which this consent is taken", formalDefinition="The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document." ) 2564 protected Type source; 2565 2566 /** 2567 * The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 2568 */ 2569 @Child(name = "policy", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2570 @Description(shortDefinition="Policies covered by this consent", formalDefinition="The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law." ) 2571 protected List<ConsentPolicyComponent> policy; 2572 2573 /** 2574 * A referece to the specific computable policy. 2575 */ 2576 @Child(name = "policyRule", type = {UriType.class}, order=12, min=0, max=1, modifier=false, summary=true) 2577 @Description(shortDefinition="Policy that this consents to", formalDefinition="A referece to the specific computable policy." ) 2578 protected UriType policyRule; 2579 2580 /** 2581 * A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels. 2582 */ 2583 @Child(name = "securityLabel", type = {Coding.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2584 @Description(shortDefinition="Security Labels that define affected resources", formalDefinition="A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels." ) 2585 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 2586 protected List<Coding> securityLabel; 2587 2588 /** 2589 * The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent. 2590 */ 2591 @Child(name = "purpose", type = {Coding.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2592 @Description(shortDefinition="Context of activities for which the agreement is made", formalDefinition="The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent." ) 2593 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 2594 protected List<Coding> purpose; 2595 2596 /** 2597 * Clinical or Operational Relevant period of time that bounds the data controlled by this consent. 2598 */ 2599 @Child(name = "dataPeriod", type = {Period.class}, order=15, min=0, max=1, modifier=false, summary=true) 2600 @Description(shortDefinition="Timeframe for data controlled by this consent", formalDefinition="Clinical or Operational Relevant period of time that bounds the data controlled by this consent." ) 2601 protected Period dataPeriod; 2602 2603 /** 2604 * The resources controlled by this consent, if specific resources are referenced. 2605 */ 2606 @Child(name = "data", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2607 @Description(shortDefinition="Data controlled by this consent", formalDefinition="The resources controlled by this consent, if specific resources are referenced." ) 2608 protected List<ConsentDataComponent> data; 2609 2610 /** 2611 * An exception to the base policy of this consent. An exception can be an addition or removal of access permissions. 2612 */ 2613 @Child(name = "except", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2614 @Description(shortDefinition="Additional rule - addition or removal of permissions", formalDefinition="An exception to the base policy of this consent. An exception can be an addition or removal of access permissions." ) 2615 protected List<ExceptComponent> except; 2616 2617 private static final long serialVersionUID = -345946739L; 2618 2619 /** 2620 * Constructor 2621 */ 2622 public Consent() { 2623 super(); 2624 } 2625 2626 /** 2627 * Constructor 2628 */ 2629 public Consent(Enumeration<ConsentState> status, Reference patient) { 2630 super(); 2631 this.status = status; 2632 this.patient = patient; 2633 } 2634 2635 /** 2636 * @return {@link #identifier} (Unique identifier for this copy of the Consent Statement.) 2637 */ 2638 public Identifier getIdentifier() { 2639 if (this.identifier == null) 2640 if (Configuration.errorOnAutoCreate()) 2641 throw new Error("Attempt to auto-create Consent.identifier"); 2642 else if (Configuration.doAutoCreate()) 2643 this.identifier = new Identifier(); // cc 2644 return this.identifier; 2645 } 2646 2647 public boolean hasIdentifier() { 2648 return this.identifier != null && !this.identifier.isEmpty(); 2649 } 2650 2651 /** 2652 * @param value {@link #identifier} (Unique identifier for this copy of the Consent Statement.) 2653 */ 2654 public Consent setIdentifier(Identifier value) { 2655 this.identifier = value; 2656 return this; 2657 } 2658 2659 /** 2660 * @return {@link #status} (Indicates the current state of this consent.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2661 */ 2662 public Enumeration<ConsentState> getStatusElement() { 2663 if (this.status == null) 2664 if (Configuration.errorOnAutoCreate()) 2665 throw new Error("Attempt to auto-create Consent.status"); 2666 else if (Configuration.doAutoCreate()) 2667 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); // bb 2668 return this.status; 2669 } 2670 2671 public boolean hasStatusElement() { 2672 return this.status != null && !this.status.isEmpty(); 2673 } 2674 2675 public boolean hasStatus() { 2676 return this.status != null && !this.status.isEmpty(); 2677 } 2678 2679 /** 2680 * @param value {@link #status} (Indicates the current state of this consent.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2681 */ 2682 public Consent setStatusElement(Enumeration<ConsentState> value) { 2683 this.status = value; 2684 return this; 2685 } 2686 2687 /** 2688 * @return Indicates the current state of this consent. 2689 */ 2690 public ConsentState getStatus() { 2691 return this.status == null ? null : this.status.getValue(); 2692 } 2693 2694 /** 2695 * @param value Indicates the current state of this consent. 2696 */ 2697 public Consent setStatus(ConsentState value) { 2698 if (this.status == null) 2699 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); 2700 this.status.setValue(value); 2701 return this; 2702 } 2703 2704 /** 2705 * @return {@link #category} (A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.) 2706 */ 2707 public List<CodeableConcept> getCategory() { 2708 if (this.category == null) 2709 this.category = new ArrayList<CodeableConcept>(); 2710 return this.category; 2711 } 2712 2713 /** 2714 * @return Returns a reference to <code>this</code> for easy method chaining 2715 */ 2716 public Consent setCategory(List<CodeableConcept> theCategory) { 2717 this.category = theCategory; 2718 return this; 2719 } 2720 2721 public boolean hasCategory() { 2722 if (this.category == null) 2723 return false; 2724 for (CodeableConcept item : this.category) 2725 if (!item.isEmpty()) 2726 return true; 2727 return false; 2728 } 2729 2730 public CodeableConcept addCategory() { //3 2731 CodeableConcept t = new CodeableConcept(); 2732 if (this.category == null) 2733 this.category = new ArrayList<CodeableConcept>(); 2734 this.category.add(t); 2735 return t; 2736 } 2737 2738 public Consent addCategory(CodeableConcept t) { //3 2739 if (t == null) 2740 return this; 2741 if (this.category == null) 2742 this.category = new ArrayList<CodeableConcept>(); 2743 this.category.add(t); 2744 return this; 2745 } 2746 2747 /** 2748 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 2749 */ 2750 public CodeableConcept getCategoryFirstRep() { 2751 if (getCategory().isEmpty()) { 2752 addCategory(); 2753 } 2754 return getCategory().get(0); 2755 } 2756 2757 /** 2758 * @return {@link #patient} (The patient/healthcare consumer to whom this consent applies.) 2759 */ 2760 public Reference getPatient() { 2761 if (this.patient == null) 2762 if (Configuration.errorOnAutoCreate()) 2763 throw new Error("Attempt to auto-create Consent.patient"); 2764 else if (Configuration.doAutoCreate()) 2765 this.patient = new Reference(); // cc 2766 return this.patient; 2767 } 2768 2769 public boolean hasPatient() { 2770 return this.patient != null && !this.patient.isEmpty(); 2771 } 2772 2773 /** 2774 * @param value {@link #patient} (The patient/healthcare consumer to whom this consent applies.) 2775 */ 2776 public Consent setPatient(Reference value) { 2777 this.patient = value; 2778 return this; 2779 } 2780 2781 /** 2782 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient/healthcare consumer to whom this consent applies.) 2783 */ 2784 public Patient getPatientTarget() { 2785 if (this.patientTarget == null) 2786 if (Configuration.errorOnAutoCreate()) 2787 throw new Error("Attempt to auto-create Consent.patient"); 2788 else if (Configuration.doAutoCreate()) 2789 this.patientTarget = new Patient(); // aa 2790 return this.patientTarget; 2791 } 2792 2793 /** 2794 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient/healthcare consumer to whom this consent applies.) 2795 */ 2796 public Consent setPatientTarget(Patient value) { 2797 this.patientTarget = value; 2798 return this; 2799 } 2800 2801 /** 2802 * @return {@link #period} (Relevant time or time-period when this Consent is applicable.) 2803 */ 2804 public Period getPeriod() { 2805 if (this.period == null) 2806 if (Configuration.errorOnAutoCreate()) 2807 throw new Error("Attempt to auto-create Consent.period"); 2808 else if (Configuration.doAutoCreate()) 2809 this.period = new Period(); // cc 2810 return this.period; 2811 } 2812 2813 public boolean hasPeriod() { 2814 return this.period != null && !this.period.isEmpty(); 2815 } 2816 2817 /** 2818 * @param value {@link #period} (Relevant time or time-period when this Consent is applicable.) 2819 */ 2820 public Consent setPeriod(Period value) { 2821 this.period = value; 2822 return this; 2823 } 2824 2825 /** 2826 * @return {@link #dateTime} (When this Consent was issued / created / indexed.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 2827 */ 2828 public DateTimeType getDateTimeElement() { 2829 if (this.dateTime == null) 2830 if (Configuration.errorOnAutoCreate()) 2831 throw new Error("Attempt to auto-create Consent.dateTime"); 2832 else if (Configuration.doAutoCreate()) 2833 this.dateTime = new DateTimeType(); // bb 2834 return this.dateTime; 2835 } 2836 2837 public boolean hasDateTimeElement() { 2838 return this.dateTime != null && !this.dateTime.isEmpty(); 2839 } 2840 2841 public boolean hasDateTime() { 2842 return this.dateTime != null && !this.dateTime.isEmpty(); 2843 } 2844 2845 /** 2846 * @param value {@link #dateTime} (When this Consent was issued / created / indexed.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 2847 */ 2848 public Consent setDateTimeElement(DateTimeType value) { 2849 this.dateTime = value; 2850 return this; 2851 } 2852 2853 /** 2854 * @return When this Consent was issued / created / indexed. 2855 */ 2856 public Date getDateTime() { 2857 return this.dateTime == null ? null : this.dateTime.getValue(); 2858 } 2859 2860 /** 2861 * @param value When this Consent was issued / created / indexed. 2862 */ 2863 public Consent setDateTime(Date value) { 2864 if (value == null) 2865 this.dateTime = null; 2866 else { 2867 if (this.dateTime == null) 2868 this.dateTime = new DateTimeType(); 2869 this.dateTime.setValue(value); 2870 } 2871 return this; 2872 } 2873 2874 /** 2875 * @return {@link #consentingParty} (Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.) 2876 */ 2877 public List<Reference> getConsentingParty() { 2878 if (this.consentingParty == null) 2879 this.consentingParty = new ArrayList<Reference>(); 2880 return this.consentingParty; 2881 } 2882 2883 /** 2884 * @return Returns a reference to <code>this</code> for easy method chaining 2885 */ 2886 public Consent setConsentingParty(List<Reference> theConsentingParty) { 2887 this.consentingParty = theConsentingParty; 2888 return this; 2889 } 2890 2891 public boolean hasConsentingParty() { 2892 if (this.consentingParty == null) 2893 return false; 2894 for (Reference item : this.consentingParty) 2895 if (!item.isEmpty()) 2896 return true; 2897 return false; 2898 } 2899 2900 public Reference addConsentingParty() { //3 2901 Reference t = new Reference(); 2902 if (this.consentingParty == null) 2903 this.consentingParty = new ArrayList<Reference>(); 2904 this.consentingParty.add(t); 2905 return t; 2906 } 2907 2908 public Consent addConsentingParty(Reference t) { //3 2909 if (t == null) 2910 return this; 2911 if (this.consentingParty == null) 2912 this.consentingParty = new ArrayList<Reference>(); 2913 this.consentingParty.add(t); 2914 return this; 2915 } 2916 2917 /** 2918 * @return The first repetition of repeating field {@link #consentingParty}, creating it if it does not already exist 2919 */ 2920 public Reference getConsentingPartyFirstRep() { 2921 if (getConsentingParty().isEmpty()) { 2922 addConsentingParty(); 2923 } 2924 return getConsentingParty().get(0); 2925 } 2926 2927 /** 2928 * @deprecated Use Reference#setResource(IBaseResource) instead 2929 */ 2930 @Deprecated 2931 public List<Resource> getConsentingPartyTarget() { 2932 if (this.consentingPartyTarget == null) 2933 this.consentingPartyTarget = new ArrayList<Resource>(); 2934 return this.consentingPartyTarget; 2935 } 2936 2937 /** 2938 * @return {@link #actor} (Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2939 */ 2940 public List<ConsentActorComponent> getActor() { 2941 if (this.actor == null) 2942 this.actor = new ArrayList<ConsentActorComponent>(); 2943 return this.actor; 2944 } 2945 2946 /** 2947 * @return Returns a reference to <code>this</code> for easy method chaining 2948 */ 2949 public Consent setActor(List<ConsentActorComponent> theActor) { 2950 this.actor = theActor; 2951 return this; 2952 } 2953 2954 public boolean hasActor() { 2955 if (this.actor == null) 2956 return false; 2957 for (ConsentActorComponent item : this.actor) 2958 if (!item.isEmpty()) 2959 return true; 2960 return false; 2961 } 2962 2963 public ConsentActorComponent addActor() { //3 2964 ConsentActorComponent t = new ConsentActorComponent(); 2965 if (this.actor == null) 2966 this.actor = new ArrayList<ConsentActorComponent>(); 2967 this.actor.add(t); 2968 return t; 2969 } 2970 2971 public Consent addActor(ConsentActorComponent t) { //3 2972 if (t == null) 2973 return this; 2974 if (this.actor == null) 2975 this.actor = new ArrayList<ConsentActorComponent>(); 2976 this.actor.add(t); 2977 return this; 2978 } 2979 2980 /** 2981 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist 2982 */ 2983 public ConsentActorComponent getActorFirstRep() { 2984 if (getActor().isEmpty()) { 2985 addActor(); 2986 } 2987 return getActor().get(0); 2988 } 2989 2990 /** 2991 * @return {@link #action} (Actions controlled by this consent.) 2992 */ 2993 public List<CodeableConcept> getAction() { 2994 if (this.action == null) 2995 this.action = new ArrayList<CodeableConcept>(); 2996 return this.action; 2997 } 2998 2999 /** 3000 * @return Returns a reference to <code>this</code> for easy method chaining 3001 */ 3002 public Consent setAction(List<CodeableConcept> theAction) { 3003 this.action = theAction; 3004 return this; 3005 } 3006 3007 public boolean hasAction() { 3008 if (this.action == null) 3009 return false; 3010 for (CodeableConcept item : this.action) 3011 if (!item.isEmpty()) 3012 return true; 3013 return false; 3014 } 3015 3016 public CodeableConcept addAction() { //3 3017 CodeableConcept t = new CodeableConcept(); 3018 if (this.action == null) 3019 this.action = new ArrayList<CodeableConcept>(); 3020 this.action.add(t); 3021 return t; 3022 } 3023 3024 public Consent addAction(CodeableConcept t) { //3 3025 if (t == null) 3026 return this; 3027 if (this.action == null) 3028 this.action = new ArrayList<CodeableConcept>(); 3029 this.action.add(t); 3030 return this; 3031 } 3032 3033 /** 3034 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 3035 */ 3036 public CodeableConcept getActionFirstRep() { 3037 if (getAction().isEmpty()) { 3038 addAction(); 3039 } 3040 return getAction().get(0); 3041 } 3042 3043 /** 3044 * @return {@link #organization} (The organization that manages the consent, and the framework within which it is executed.) 3045 */ 3046 public List<Reference> getOrganization() { 3047 if (this.organization == null) 3048 this.organization = new ArrayList<Reference>(); 3049 return this.organization; 3050 } 3051 3052 /** 3053 * @return Returns a reference to <code>this</code> for easy method chaining 3054 */ 3055 public Consent setOrganization(List<Reference> theOrganization) { 3056 this.organization = theOrganization; 3057 return this; 3058 } 3059 3060 public boolean hasOrganization() { 3061 if (this.organization == null) 3062 return false; 3063 for (Reference item : this.organization) 3064 if (!item.isEmpty()) 3065 return true; 3066 return false; 3067 } 3068 3069 public Reference addOrganization() { //3 3070 Reference t = new Reference(); 3071 if (this.organization == null) 3072 this.organization = new ArrayList<Reference>(); 3073 this.organization.add(t); 3074 return t; 3075 } 3076 3077 public Consent addOrganization(Reference t) { //3 3078 if (t == null) 3079 return this; 3080 if (this.organization == null) 3081 this.organization = new ArrayList<Reference>(); 3082 this.organization.add(t); 3083 return this; 3084 } 3085 3086 /** 3087 * @return The first repetition of repeating field {@link #organization}, creating it if it does not already exist 3088 */ 3089 public Reference getOrganizationFirstRep() { 3090 if (getOrganization().isEmpty()) { 3091 addOrganization(); 3092 } 3093 return getOrganization().get(0); 3094 } 3095 3096 /** 3097 * @deprecated Use Reference#setResource(IBaseResource) instead 3098 */ 3099 @Deprecated 3100 public List<Organization> getOrganizationTarget() { 3101 if (this.organizationTarget == null) 3102 this.organizationTarget = new ArrayList<Organization>(); 3103 return this.organizationTarget; 3104 } 3105 3106 /** 3107 * @deprecated Use Reference#setResource(IBaseResource) instead 3108 */ 3109 @Deprecated 3110 public Organization addOrganizationTarget() { 3111 Organization r = new Organization(); 3112 if (this.organizationTarget == null) 3113 this.organizationTarget = new ArrayList<Organization>(); 3114 this.organizationTarget.add(r); 3115 return r; 3116 } 3117 3118 /** 3119 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3120 */ 3121 public Type getSource() { 3122 return this.source; 3123 } 3124 3125 /** 3126 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3127 */ 3128 public Attachment getSourceAttachment() throws FHIRException { 3129 if (this.source == null) 3130 return null; 3131 if (!(this.source instanceof Attachment)) 3132 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.source.getClass().getName()+" was encountered"); 3133 return (Attachment) this.source; 3134 } 3135 3136 public boolean hasSourceAttachment() { 3137 return this != null && this.source instanceof Attachment; 3138 } 3139 3140 /** 3141 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3142 */ 3143 public Identifier getSourceIdentifier() throws FHIRException { 3144 if (this.source == null) 3145 return null; 3146 if (!(this.source instanceof Identifier)) 3147 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.source.getClass().getName()+" was encountered"); 3148 return (Identifier) this.source; 3149 } 3150 3151 public boolean hasSourceIdentifier() { 3152 return this != null && this.source instanceof Identifier; 3153 } 3154 3155 /** 3156 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3157 */ 3158 public Reference getSourceReference() throws FHIRException { 3159 if (this.source == null) 3160 return null; 3161 if (!(this.source instanceof Reference)) 3162 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.source.getClass().getName()+" was encountered"); 3163 return (Reference) this.source; 3164 } 3165 3166 public boolean hasSourceReference() { 3167 return this != null && this.source instanceof Reference; 3168 } 3169 3170 public boolean hasSource() { 3171 return this.source != null && !this.source.isEmpty(); 3172 } 3173 3174 /** 3175 * @param value {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3176 */ 3177 public Consent setSource(Type value) throws FHIRFormatError { 3178 if (value != null && !(value instanceof Attachment || value instanceof Identifier || value instanceof Reference)) 3179 throw new FHIRFormatError("Not the right type for Consent.source[x]: "+value.fhirType()); 3180 this.source = value; 3181 return this; 3182 } 3183 3184 /** 3185 * @return {@link #policy} (The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.) 3186 */ 3187 public List<ConsentPolicyComponent> getPolicy() { 3188 if (this.policy == null) 3189 this.policy = new ArrayList<ConsentPolicyComponent>(); 3190 return this.policy; 3191 } 3192 3193 /** 3194 * @return Returns a reference to <code>this</code> for easy method chaining 3195 */ 3196 public Consent setPolicy(List<ConsentPolicyComponent> thePolicy) { 3197 this.policy = thePolicy; 3198 return this; 3199 } 3200 3201 public boolean hasPolicy() { 3202 if (this.policy == null) 3203 return false; 3204 for (ConsentPolicyComponent item : this.policy) 3205 if (!item.isEmpty()) 3206 return true; 3207 return false; 3208 } 3209 3210 public ConsentPolicyComponent addPolicy() { //3 3211 ConsentPolicyComponent t = new ConsentPolicyComponent(); 3212 if (this.policy == null) 3213 this.policy = new ArrayList<ConsentPolicyComponent>(); 3214 this.policy.add(t); 3215 return t; 3216 } 3217 3218 public Consent addPolicy(ConsentPolicyComponent t) { //3 3219 if (t == null) 3220 return this; 3221 if (this.policy == null) 3222 this.policy = new ArrayList<ConsentPolicyComponent>(); 3223 this.policy.add(t); 3224 return this; 3225 } 3226 3227 /** 3228 * @return The first repetition of repeating field {@link #policy}, creating it if it does not already exist 3229 */ 3230 public ConsentPolicyComponent getPolicyFirstRep() { 3231 if (getPolicy().isEmpty()) { 3232 addPolicy(); 3233 } 3234 return getPolicy().get(0); 3235 } 3236 3237 /** 3238 * @return {@link #policyRule} (A referece to the specific computable policy.). This is the underlying object with id, value and extensions. The accessor "getPolicyRule" gives direct access to the value 3239 */ 3240 public UriType getPolicyRuleElement() { 3241 if (this.policyRule == null) 3242 if (Configuration.errorOnAutoCreate()) 3243 throw new Error("Attempt to auto-create Consent.policyRule"); 3244 else if (Configuration.doAutoCreate()) 3245 this.policyRule = new UriType(); // bb 3246 return this.policyRule; 3247 } 3248 3249 public boolean hasPolicyRuleElement() { 3250 return this.policyRule != null && !this.policyRule.isEmpty(); 3251 } 3252 3253 public boolean hasPolicyRule() { 3254 return this.policyRule != null && !this.policyRule.isEmpty(); 3255 } 3256 3257 /** 3258 * @param value {@link #policyRule} (A referece to the specific computable policy.). This is the underlying object with id, value and extensions. The accessor "getPolicyRule" gives direct access to the value 3259 */ 3260 public Consent setPolicyRuleElement(UriType value) { 3261 this.policyRule = value; 3262 return this; 3263 } 3264 3265 /** 3266 * @return A referece to the specific computable policy. 3267 */ 3268 public String getPolicyRule() { 3269 return this.policyRule == null ? null : this.policyRule.getValue(); 3270 } 3271 3272 /** 3273 * @param value A referece to the specific computable policy. 3274 */ 3275 public Consent setPolicyRule(String value) { 3276 if (Utilities.noString(value)) 3277 this.policyRule = null; 3278 else { 3279 if (this.policyRule == null) 3280 this.policyRule = new UriType(); 3281 this.policyRule.setValue(value); 3282 } 3283 return this; 3284 } 3285 3286 /** 3287 * @return {@link #securityLabel} (A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.) 3288 */ 3289 public List<Coding> getSecurityLabel() { 3290 if (this.securityLabel == null) 3291 this.securityLabel = new ArrayList<Coding>(); 3292 return this.securityLabel; 3293 } 3294 3295 /** 3296 * @return Returns a reference to <code>this</code> for easy method chaining 3297 */ 3298 public Consent setSecurityLabel(List<Coding> theSecurityLabel) { 3299 this.securityLabel = theSecurityLabel; 3300 return this; 3301 } 3302 3303 public boolean hasSecurityLabel() { 3304 if (this.securityLabel == null) 3305 return false; 3306 for (Coding item : this.securityLabel) 3307 if (!item.isEmpty()) 3308 return true; 3309 return false; 3310 } 3311 3312 public Coding addSecurityLabel() { //3 3313 Coding t = new Coding(); 3314 if (this.securityLabel == null) 3315 this.securityLabel = new ArrayList<Coding>(); 3316 this.securityLabel.add(t); 3317 return t; 3318 } 3319 3320 public Consent addSecurityLabel(Coding t) { //3 3321 if (t == null) 3322 return this; 3323 if (this.securityLabel == null) 3324 this.securityLabel = new ArrayList<Coding>(); 3325 this.securityLabel.add(t); 3326 return this; 3327 } 3328 3329 /** 3330 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 3331 */ 3332 public Coding getSecurityLabelFirstRep() { 3333 if (getSecurityLabel().isEmpty()) { 3334 addSecurityLabel(); 3335 } 3336 return getSecurityLabel().get(0); 3337 } 3338 3339 /** 3340 * @return {@link #purpose} (The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent.) 3341 */ 3342 public List<Coding> getPurpose() { 3343 if (this.purpose == null) 3344 this.purpose = new ArrayList<Coding>(); 3345 return this.purpose; 3346 } 3347 3348 /** 3349 * @return Returns a reference to <code>this</code> for easy method chaining 3350 */ 3351 public Consent setPurpose(List<Coding> thePurpose) { 3352 this.purpose = thePurpose; 3353 return this; 3354 } 3355 3356 public boolean hasPurpose() { 3357 if (this.purpose == null) 3358 return false; 3359 for (Coding item : this.purpose) 3360 if (!item.isEmpty()) 3361 return true; 3362 return false; 3363 } 3364 3365 public Coding addPurpose() { //3 3366 Coding t = new Coding(); 3367 if (this.purpose == null) 3368 this.purpose = new ArrayList<Coding>(); 3369 this.purpose.add(t); 3370 return t; 3371 } 3372 3373 public Consent addPurpose(Coding t) { //3 3374 if (t == null) 3375 return this; 3376 if (this.purpose == null) 3377 this.purpose = new ArrayList<Coding>(); 3378 this.purpose.add(t); 3379 return this; 3380 } 3381 3382 /** 3383 * @return The first repetition of repeating field {@link #purpose}, creating it if it does not already exist 3384 */ 3385 public Coding getPurposeFirstRep() { 3386 if (getPurpose().isEmpty()) { 3387 addPurpose(); 3388 } 3389 return getPurpose().get(0); 3390 } 3391 3392 /** 3393 * @return {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this consent.) 3394 */ 3395 public Period getDataPeriod() { 3396 if (this.dataPeriod == null) 3397 if (Configuration.errorOnAutoCreate()) 3398 throw new Error("Attempt to auto-create Consent.dataPeriod"); 3399 else if (Configuration.doAutoCreate()) 3400 this.dataPeriod = new Period(); // cc 3401 return this.dataPeriod; 3402 } 3403 3404 public boolean hasDataPeriod() { 3405 return this.dataPeriod != null && !this.dataPeriod.isEmpty(); 3406 } 3407 3408 /** 3409 * @param value {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this consent.) 3410 */ 3411 public Consent setDataPeriod(Period value) { 3412 this.dataPeriod = value; 3413 return this; 3414 } 3415 3416 /** 3417 * @return {@link #data} (The resources controlled by this consent, if specific resources are referenced.) 3418 */ 3419 public List<ConsentDataComponent> getData() { 3420 if (this.data == null) 3421 this.data = new ArrayList<ConsentDataComponent>(); 3422 return this.data; 3423 } 3424 3425 /** 3426 * @return Returns a reference to <code>this</code> for easy method chaining 3427 */ 3428 public Consent setData(List<ConsentDataComponent> theData) { 3429 this.data = theData; 3430 return this; 3431 } 3432 3433 public boolean hasData() { 3434 if (this.data == null) 3435 return false; 3436 for (ConsentDataComponent item : this.data) 3437 if (!item.isEmpty()) 3438 return true; 3439 return false; 3440 } 3441 3442 public ConsentDataComponent addData() { //3 3443 ConsentDataComponent t = new ConsentDataComponent(); 3444 if (this.data == null) 3445 this.data = new ArrayList<ConsentDataComponent>(); 3446 this.data.add(t); 3447 return t; 3448 } 3449 3450 public Consent addData(ConsentDataComponent t) { //3 3451 if (t == null) 3452 return this; 3453 if (this.data == null) 3454 this.data = new ArrayList<ConsentDataComponent>(); 3455 this.data.add(t); 3456 return this; 3457 } 3458 3459 /** 3460 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist 3461 */ 3462 public ConsentDataComponent getDataFirstRep() { 3463 if (getData().isEmpty()) { 3464 addData(); 3465 } 3466 return getData().get(0); 3467 } 3468 3469 /** 3470 * @return {@link #except} (An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.) 3471 */ 3472 public List<ExceptComponent> getExcept() { 3473 if (this.except == null) 3474 this.except = new ArrayList<ExceptComponent>(); 3475 return this.except; 3476 } 3477 3478 /** 3479 * @return Returns a reference to <code>this</code> for easy method chaining 3480 */ 3481 public Consent setExcept(List<ExceptComponent> theExcept) { 3482 this.except = theExcept; 3483 return this; 3484 } 3485 3486 public boolean hasExcept() { 3487 if (this.except == null) 3488 return false; 3489 for (ExceptComponent item : this.except) 3490 if (!item.isEmpty()) 3491 return true; 3492 return false; 3493 } 3494 3495 public ExceptComponent addExcept() { //3 3496 ExceptComponent t = new ExceptComponent(); 3497 if (this.except == null) 3498 this.except = new ArrayList<ExceptComponent>(); 3499 this.except.add(t); 3500 return t; 3501 } 3502 3503 public Consent addExcept(ExceptComponent t) { //3 3504 if (t == null) 3505 return this; 3506 if (this.except == null) 3507 this.except = new ArrayList<ExceptComponent>(); 3508 this.except.add(t); 3509 return this; 3510 } 3511 3512 /** 3513 * @return The first repetition of repeating field {@link #except}, creating it if it does not already exist 3514 */ 3515 public ExceptComponent getExceptFirstRep() { 3516 if (getExcept().isEmpty()) { 3517 addExcept(); 3518 } 3519 return getExcept().get(0); 3520 } 3521 3522 protected void listChildren(List<Property> children) { 3523 super.listChildren(children); 3524 children.add(new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, 1, identifier)); 3525 children.add(new Property("status", "code", "Indicates the current state of this consent.", 0, 1, status)); 3526 children.add(new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category)); 3527 children.add(new Property("patient", "Reference(Patient)", "The patient/healthcare consumer to whom this consent applies.", 0, 1, patient)); 3528 children.add(new Property("period", "Period", "Relevant time or time-period when this Consent is applicable.", 0, 1, period)); 3529 children.add(new Property("dateTime", "dateTime", "When this Consent was issued / created / indexed.", 0, 1, dateTime)); 3530 children.add(new Property("consentingParty", "Reference(Organization|Patient|Practitioner|RelatedPerson)", "Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, consentingParty)); 3531 children.add(new Property("actor", "", "Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor)); 3532 children.add(new Property("action", "CodeableConcept", "Actions controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, action)); 3533 children.add(new Property("organization", "Reference(Organization)", "The organization that manages the consent, and the framework within which it is executed.", 0, java.lang.Integer.MAX_VALUE, organization)); 3534 children.add(new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source)); 3535 children.add(new Property("policy", "", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, java.lang.Integer.MAX_VALUE, policy)); 3536 children.add(new Property("policyRule", "uri", "A referece to the specific computable policy.", 0, 1, policyRule)); 3537 children.add(new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 3538 children.add(new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, purpose)); 3539 children.add(new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this consent.", 0, 1, dataPeriod)); 3540 children.add(new Property("data", "", "The resources controlled by this consent, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data)); 3541 children.add(new Property("except", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, except)); 3542 } 3543 3544 @Override 3545 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3546 switch (_hash) { 3547 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, 1, identifier); 3548 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of this consent.", 0, 1, status); 3549 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category); 3550 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient/healthcare consumer to whom this consent applies.", 0, 1, patient); 3551 case -991726143: /*period*/ return new Property("period", "Period", "Relevant time or time-period when this Consent is applicable.", 0, 1, period); 3552 case 1792749467: /*dateTime*/ return new Property("dateTime", "dateTime", "When this Consent was issued / created / indexed.", 0, 1, dateTime); 3553 case -1886702018: /*consentingParty*/ return new Property("consentingParty", "Reference(Organization|Patient|Practitioner|RelatedPerson)", "Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, consentingParty); 3554 case 92645877: /*actor*/ return new Property("actor", "", "Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor); 3555 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Actions controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, action); 3556 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization that manages the consent, and the framework within which it is executed.", 0, java.lang.Integer.MAX_VALUE, organization); 3557 case -1698413947: /*source[x]*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3558 case -896505829: /*source*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3559 case 1964406686: /*sourceAttachment*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3560 case -1985492188: /*sourceIdentifier*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3561 case -244259472: /*sourceReference*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3562 case -982670030: /*policy*/ return new Property("policy", "", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, java.lang.Integer.MAX_VALUE, policy); 3563 case 1593493326: /*policyRule*/ return new Property("policyRule", "uri", "A referece to the specific computable policy.", 0, 1, policyRule); 3564 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 3565 case -220463842: /*purpose*/ return new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, purpose); 3566 case 1177250315: /*dataPeriod*/ return new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this consent.", 0, 1, dataPeriod); 3567 case 3076010: /*data*/ return new Property("data", "", "The resources controlled by this consent, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data); 3568 case -1289550567: /*except*/ return new Property("except", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, except); 3569 default: return super.getNamedProperty(_hash, _name, _checkValid); 3570 } 3571 3572 } 3573 3574 @Override 3575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3576 switch (hash) { 3577 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3578 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ConsentState> 3579 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3580 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 3581 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3582 case 1792749467: /*dateTime*/ return this.dateTime == null ? new Base[0] : new Base[] {this.dateTime}; // DateTimeType 3583 case -1886702018: /*consentingParty*/ return this.consentingParty == null ? new Base[0] : this.consentingParty.toArray(new Base[this.consentingParty.size()]); // Reference 3584 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ConsentActorComponent 3585 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 3586 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : this.organization.toArray(new Base[this.organization.size()]); // Reference 3587 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Type 3588 case -982670030: /*policy*/ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // ConsentPolicyComponent 3589 case 1593493326: /*policyRule*/ return this.policyRule == null ? new Base[0] : new Base[] {this.policyRule}; // UriType 3590 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 3591 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Coding 3592 case 1177250315: /*dataPeriod*/ return this.dataPeriod == null ? new Base[0] : new Base[] {this.dataPeriod}; // Period 3593 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // ConsentDataComponent 3594 case -1289550567: /*except*/ return this.except == null ? new Base[0] : this.except.toArray(new Base[this.except.size()]); // ExceptComponent 3595 default: return super.getProperty(hash, name, checkValid); 3596 } 3597 3598 } 3599 3600 @Override 3601 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3602 switch (hash) { 3603 case -1618432855: // identifier 3604 this.identifier = castToIdentifier(value); // Identifier 3605 return value; 3606 case -892481550: // status 3607 value = new ConsentStateEnumFactory().fromType(castToCode(value)); 3608 this.status = (Enumeration) value; // Enumeration<ConsentState> 3609 return value; 3610 case 50511102: // category 3611 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3612 return value; 3613 case -791418107: // patient 3614 this.patient = castToReference(value); // Reference 3615 return value; 3616 case -991726143: // period 3617 this.period = castToPeriod(value); // Period 3618 return value; 3619 case 1792749467: // dateTime 3620 this.dateTime = castToDateTime(value); // DateTimeType 3621 return value; 3622 case -1886702018: // consentingParty 3623 this.getConsentingParty().add(castToReference(value)); // Reference 3624 return value; 3625 case 92645877: // actor 3626 this.getActor().add((ConsentActorComponent) value); // ConsentActorComponent 3627 return value; 3628 case -1422950858: // action 3629 this.getAction().add(castToCodeableConcept(value)); // CodeableConcept 3630 return value; 3631 case 1178922291: // organization 3632 this.getOrganization().add(castToReference(value)); // Reference 3633 return value; 3634 case -896505829: // source 3635 this.source = castToType(value); // Type 3636 return value; 3637 case -982670030: // policy 3638 this.getPolicy().add((ConsentPolicyComponent) value); // ConsentPolicyComponent 3639 return value; 3640 case 1593493326: // policyRule 3641 this.policyRule = castToUri(value); // UriType 3642 return value; 3643 case -722296940: // securityLabel 3644 this.getSecurityLabel().add(castToCoding(value)); // Coding 3645 return value; 3646 case -220463842: // purpose 3647 this.getPurpose().add(castToCoding(value)); // Coding 3648 return value; 3649 case 1177250315: // dataPeriod 3650 this.dataPeriod = castToPeriod(value); // Period 3651 return value; 3652 case 3076010: // data 3653 this.getData().add((ConsentDataComponent) value); // ConsentDataComponent 3654 return value; 3655 case -1289550567: // except 3656 this.getExcept().add((ExceptComponent) value); // ExceptComponent 3657 return value; 3658 default: return super.setProperty(hash, name, value); 3659 } 3660 3661 } 3662 3663 @Override 3664 public Base setProperty(String name, Base value) throws FHIRException { 3665 if (name.equals("identifier")) { 3666 this.identifier = castToIdentifier(value); // Identifier 3667 } else if (name.equals("status")) { 3668 value = new ConsentStateEnumFactory().fromType(castToCode(value)); 3669 this.status = (Enumeration) value; // Enumeration<ConsentState> 3670 } else if (name.equals("category")) { 3671 this.getCategory().add(castToCodeableConcept(value)); 3672 } else if (name.equals("patient")) { 3673 this.patient = castToReference(value); // Reference 3674 } else if (name.equals("period")) { 3675 this.period = castToPeriod(value); // Period 3676 } else if (name.equals("dateTime")) { 3677 this.dateTime = castToDateTime(value); // DateTimeType 3678 } else if (name.equals("consentingParty")) { 3679 this.getConsentingParty().add(castToReference(value)); 3680 } else if (name.equals("actor")) { 3681 this.getActor().add((ConsentActorComponent) value); 3682 } else if (name.equals("action")) { 3683 this.getAction().add(castToCodeableConcept(value)); 3684 } else if (name.equals("organization")) { 3685 this.getOrganization().add(castToReference(value)); 3686 } else if (name.equals("source[x]")) { 3687 this.source = castToType(value); // Type 3688 } else if (name.equals("policy")) { 3689 this.getPolicy().add((ConsentPolicyComponent) value); 3690 } else if (name.equals("policyRule")) { 3691 this.policyRule = castToUri(value); // UriType 3692 } else if (name.equals("securityLabel")) { 3693 this.getSecurityLabel().add(castToCoding(value)); 3694 } else if (name.equals("purpose")) { 3695 this.getPurpose().add(castToCoding(value)); 3696 } else if (name.equals("dataPeriod")) { 3697 this.dataPeriod = castToPeriod(value); // Period 3698 } else if (name.equals("data")) { 3699 this.getData().add((ConsentDataComponent) value); 3700 } else if (name.equals("except")) { 3701 this.getExcept().add((ExceptComponent) value); 3702 } else 3703 return super.setProperty(name, value); 3704 return value; 3705 } 3706 3707 @Override 3708 public Base makeProperty(int hash, String name) throws FHIRException { 3709 switch (hash) { 3710 case -1618432855: return getIdentifier(); 3711 case -892481550: return getStatusElement(); 3712 case 50511102: return addCategory(); 3713 case -791418107: return getPatient(); 3714 case -991726143: return getPeriod(); 3715 case 1792749467: return getDateTimeElement(); 3716 case -1886702018: return addConsentingParty(); 3717 case 92645877: return addActor(); 3718 case -1422950858: return addAction(); 3719 case 1178922291: return addOrganization(); 3720 case -1698413947: return getSource(); 3721 case -896505829: return getSource(); 3722 case -982670030: return addPolicy(); 3723 case 1593493326: return getPolicyRuleElement(); 3724 case -722296940: return addSecurityLabel(); 3725 case -220463842: return addPurpose(); 3726 case 1177250315: return getDataPeriod(); 3727 case 3076010: return addData(); 3728 case -1289550567: return addExcept(); 3729 default: return super.makeProperty(hash, name); 3730 } 3731 3732 } 3733 3734 @Override 3735 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3736 switch (hash) { 3737 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3738 case -892481550: /*status*/ return new String[] {"code"}; 3739 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3740 case -791418107: /*patient*/ return new String[] {"Reference"}; 3741 case -991726143: /*period*/ return new String[] {"Period"}; 3742 case 1792749467: /*dateTime*/ return new String[] {"dateTime"}; 3743 case -1886702018: /*consentingParty*/ return new String[] {"Reference"}; 3744 case 92645877: /*actor*/ return new String[] {}; 3745 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 3746 case 1178922291: /*organization*/ return new String[] {"Reference"}; 3747 case -896505829: /*source*/ return new String[] {"Attachment", "Identifier", "Reference"}; 3748 case -982670030: /*policy*/ return new String[] {}; 3749 case 1593493326: /*policyRule*/ return new String[] {"uri"}; 3750 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 3751 case -220463842: /*purpose*/ return new String[] {"Coding"}; 3752 case 1177250315: /*dataPeriod*/ return new String[] {"Period"}; 3753 case 3076010: /*data*/ return new String[] {}; 3754 case -1289550567: /*except*/ return new String[] {}; 3755 default: return super.getTypesForProperty(hash, name); 3756 } 3757 3758 } 3759 3760 @Override 3761 public Base addChild(String name) throws FHIRException { 3762 if (name.equals("identifier")) { 3763 this.identifier = new Identifier(); 3764 return this.identifier; 3765 } 3766 else if (name.equals("status")) { 3767 throw new FHIRException("Cannot call addChild on a singleton property Consent.status"); 3768 } 3769 else if (name.equals("category")) { 3770 return addCategory(); 3771 } 3772 else if (name.equals("patient")) { 3773 this.patient = new Reference(); 3774 return this.patient; 3775 } 3776 else if (name.equals("period")) { 3777 this.period = new Period(); 3778 return this.period; 3779 } 3780 else if (name.equals("dateTime")) { 3781 throw new FHIRException("Cannot call addChild on a singleton property Consent.dateTime"); 3782 } 3783 else if (name.equals("consentingParty")) { 3784 return addConsentingParty(); 3785 } 3786 else if (name.equals("actor")) { 3787 return addActor(); 3788 } 3789 else if (name.equals("action")) { 3790 return addAction(); 3791 } 3792 else if (name.equals("organization")) { 3793 return addOrganization(); 3794 } 3795 else if (name.equals("sourceAttachment")) { 3796 this.source = new Attachment(); 3797 return this.source; 3798 } 3799 else if (name.equals("sourceIdentifier")) { 3800 this.source = new Identifier(); 3801 return this.source; 3802 } 3803 else if (name.equals("sourceReference")) { 3804 this.source = new Reference(); 3805 return this.source; 3806 } 3807 else if (name.equals("policy")) { 3808 return addPolicy(); 3809 } 3810 else if (name.equals("policyRule")) { 3811 throw new FHIRException("Cannot call addChild on a singleton property Consent.policyRule"); 3812 } 3813 else if (name.equals("securityLabel")) { 3814 return addSecurityLabel(); 3815 } 3816 else if (name.equals("purpose")) { 3817 return addPurpose(); 3818 } 3819 else if (name.equals("dataPeriod")) { 3820 this.dataPeriod = new Period(); 3821 return this.dataPeriod; 3822 } 3823 else if (name.equals("data")) { 3824 return addData(); 3825 } 3826 else if (name.equals("except")) { 3827 return addExcept(); 3828 } 3829 else 3830 return super.addChild(name); 3831 } 3832 3833 public String fhirType() { 3834 return "Consent"; 3835 3836 } 3837 3838 public Consent copy() { 3839 Consent dst = new Consent(); 3840 copyValues(dst); 3841 dst.identifier = identifier == null ? null : identifier.copy(); 3842 dst.status = status == null ? null : status.copy(); 3843 if (category != null) { 3844 dst.category = new ArrayList<CodeableConcept>(); 3845 for (CodeableConcept i : category) 3846 dst.category.add(i.copy()); 3847 }; 3848 dst.patient = patient == null ? null : patient.copy(); 3849 dst.period = period == null ? null : period.copy(); 3850 dst.dateTime = dateTime == null ? null : dateTime.copy(); 3851 if (consentingParty != null) { 3852 dst.consentingParty = new ArrayList<Reference>(); 3853 for (Reference i : consentingParty) 3854 dst.consentingParty.add(i.copy()); 3855 }; 3856 if (actor != null) { 3857 dst.actor = new ArrayList<ConsentActorComponent>(); 3858 for (ConsentActorComponent i : actor) 3859 dst.actor.add(i.copy()); 3860 }; 3861 if (action != null) { 3862 dst.action = new ArrayList<CodeableConcept>(); 3863 for (CodeableConcept i : action) 3864 dst.action.add(i.copy()); 3865 }; 3866 if (organization != null) { 3867 dst.organization = new ArrayList<Reference>(); 3868 for (Reference i : organization) 3869 dst.organization.add(i.copy()); 3870 }; 3871 dst.source = source == null ? null : source.copy(); 3872 if (policy != null) { 3873 dst.policy = new ArrayList<ConsentPolicyComponent>(); 3874 for (ConsentPolicyComponent i : policy) 3875 dst.policy.add(i.copy()); 3876 }; 3877 dst.policyRule = policyRule == null ? null : policyRule.copy(); 3878 if (securityLabel != null) { 3879 dst.securityLabel = new ArrayList<Coding>(); 3880 for (Coding i : securityLabel) 3881 dst.securityLabel.add(i.copy()); 3882 }; 3883 if (purpose != null) { 3884 dst.purpose = new ArrayList<Coding>(); 3885 for (Coding i : purpose) 3886 dst.purpose.add(i.copy()); 3887 }; 3888 dst.dataPeriod = dataPeriod == null ? null : dataPeriod.copy(); 3889 if (data != null) { 3890 dst.data = new ArrayList<ConsentDataComponent>(); 3891 for (ConsentDataComponent i : data) 3892 dst.data.add(i.copy()); 3893 }; 3894 if (except != null) { 3895 dst.except = new ArrayList<ExceptComponent>(); 3896 for (ExceptComponent i : except) 3897 dst.except.add(i.copy()); 3898 }; 3899 return dst; 3900 } 3901 3902 protected Consent typedCopy() { 3903 return copy(); 3904 } 3905 3906 @Override 3907 public boolean equalsDeep(Base other_) { 3908 if (!super.equalsDeep(other_)) 3909 return false; 3910 if (!(other_ instanceof Consent)) 3911 return false; 3912 Consent o = (Consent) other_; 3913 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 3914 && compareDeep(patient, o.patient, true) && compareDeep(period, o.period, true) && compareDeep(dateTime, o.dateTime, true) 3915 && compareDeep(consentingParty, o.consentingParty, true) && compareDeep(actor, o.actor, true) && compareDeep(action, o.action, true) 3916 && compareDeep(organization, o.organization, true) && compareDeep(source, o.source, true) && compareDeep(policy, o.policy, true) 3917 && compareDeep(policyRule, o.policyRule, true) && compareDeep(securityLabel, o.securityLabel, true) 3918 && compareDeep(purpose, o.purpose, true) && compareDeep(dataPeriod, o.dataPeriod, true) && compareDeep(data, o.data, true) 3919 && compareDeep(except, o.except, true); 3920 } 3921 3922 @Override 3923 public boolean equalsShallow(Base other_) { 3924 if (!super.equalsShallow(other_)) 3925 return false; 3926 if (!(other_ instanceof Consent)) 3927 return false; 3928 Consent o = (Consent) other_; 3929 return compareValues(status, o.status, true) && compareValues(dateTime, o.dateTime, true) && compareValues(policyRule, o.policyRule, true) 3930 ; 3931 } 3932 3933 public boolean isEmpty() { 3934 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 3935 , patient, period, dateTime, consentingParty, actor, action, organization, source 3936 , policy, policyRule, securityLabel, purpose, dataPeriod, data, except); 3937 } 3938 3939 @Override 3940 public ResourceType getResourceType() { 3941 return ResourceType.Consent; 3942 } 3943 3944 /** 3945 * Search parameter: <b>date</b> 3946 * <p> 3947 * Description: <b>When this Consent was created or indexed</b><br> 3948 * Type: <b>date</b><br> 3949 * Path: <b>Consent.dateTime</b><br> 3950 * </p> 3951 */ 3952 @SearchParamDefinition(name="date", path="Consent.dateTime", description="When this Consent was created or indexed", type="date" ) 3953 public static final String SP_DATE = "date"; 3954 /** 3955 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3956 * <p> 3957 * Description: <b>When this Consent was created or indexed</b><br> 3958 * Type: <b>date</b><br> 3959 * Path: <b>Consent.dateTime</b><br> 3960 * </p> 3961 */ 3962 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3963 3964 /** 3965 * Search parameter: <b>identifier</b> 3966 * <p> 3967 * Description: <b>Identifier for this record (external references)</b><br> 3968 * Type: <b>token</b><br> 3969 * Path: <b>Consent.identifier</b><br> 3970 * </p> 3971 */ 3972 @SearchParamDefinition(name="identifier", path="Consent.identifier", description="Identifier for this record (external references)", type="token" ) 3973 public static final String SP_IDENTIFIER = "identifier"; 3974 /** 3975 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3976 * <p> 3977 * Description: <b>Identifier for this record (external references)</b><br> 3978 * Type: <b>token</b><br> 3979 * Path: <b>Consent.identifier</b><br> 3980 * </p> 3981 */ 3982 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3983 3984 /** 3985 * Search parameter: <b>securitylabel</b> 3986 * <p> 3987 * Description: <b>Security Labels that define affected resources</b><br> 3988 * Type: <b>token</b><br> 3989 * Path: <b>Consent.securityLabel, Consent.except.securityLabel</b><br> 3990 * </p> 3991 */ 3992 @SearchParamDefinition(name="securitylabel", path="Consent.securityLabel | Consent.except.securityLabel", description="Security Labels that define affected resources", type="token" ) 3993 public static final String SP_SECURITYLABEL = "securitylabel"; 3994 /** 3995 * <b>Fluent Client</b> search parameter constant for <b>securitylabel</b> 3996 * <p> 3997 * Description: <b>Security Labels that define affected resources</b><br> 3998 * Type: <b>token</b><br> 3999 * Path: <b>Consent.securityLabel, Consent.except.securityLabel</b><br> 4000 * </p> 4001 */ 4002 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITYLABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITYLABEL); 4003 4004 /** 4005 * Search parameter: <b>period</b> 4006 * <p> 4007 * Description: <b>Period that this consent applies</b><br> 4008 * Type: <b>date</b><br> 4009 * Path: <b>Consent.period</b><br> 4010 * </p> 4011 */ 4012 @SearchParamDefinition(name="period", path="Consent.period", description="Period that this consent applies", type="date" ) 4013 public static final String SP_PERIOD = "period"; 4014 /** 4015 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4016 * <p> 4017 * Description: <b>Period that this consent applies</b><br> 4018 * Type: <b>date</b><br> 4019 * Path: <b>Consent.period</b><br> 4020 * </p> 4021 */ 4022 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 4023 4024 /** 4025 * Search parameter: <b>data</b> 4026 * <p> 4027 * Description: <b>The actual data reference</b><br> 4028 * Type: <b>reference</b><br> 4029 * Path: <b>Consent.data.reference, Consent.except.data.reference</b><br> 4030 * </p> 4031 */ 4032 @SearchParamDefinition(name="data", path="Consent.data.reference | Consent.except.data.reference", description="The actual data reference", type="reference" ) 4033 public static final String SP_DATA = "data"; 4034 /** 4035 * <b>Fluent Client</b> search parameter constant for <b>data</b> 4036 * <p> 4037 * Description: <b>The actual data reference</b><br> 4038 * Type: <b>reference</b><br> 4039 * Path: <b>Consent.data.reference, Consent.except.data.reference</b><br> 4040 * </p> 4041 */ 4042 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DATA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DATA); 4043 4044/** 4045 * Constant for fluent queries to be used to add include statements. Specifies 4046 * the path value of "<b>Consent:data</b>". 4047 */ 4048 public static final ca.uhn.fhir.model.api.Include INCLUDE_DATA = new ca.uhn.fhir.model.api.Include("Consent:data").toLocked(); 4049 4050 /** 4051 * Search parameter: <b>purpose</b> 4052 * <p> 4053 * Description: <b>Context of activities for which the agreement is made</b><br> 4054 * Type: <b>token</b><br> 4055 * Path: <b>Consent.purpose, Consent.except.purpose</b><br> 4056 * </p> 4057 */ 4058 @SearchParamDefinition(name="purpose", path="Consent.purpose | Consent.except.purpose", description="Context of activities for which the agreement is made", type="token" ) 4059 public static final String SP_PURPOSE = "purpose"; 4060 /** 4061 * <b>Fluent Client</b> search parameter constant for <b>purpose</b> 4062 * <p> 4063 * Description: <b>Context of activities for which the agreement is made</b><br> 4064 * Type: <b>token</b><br> 4065 * Path: <b>Consent.purpose, Consent.except.purpose</b><br> 4066 * </p> 4067 */ 4068 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PURPOSE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PURPOSE); 4069 4070 /** 4071 * Search parameter: <b>source</b> 4072 * <p> 4073 * Description: <b>Source from which this consent is taken</b><br> 4074 * Type: <b>reference</b><br> 4075 * Path: <b>Consent.source[x]</b><br> 4076 * </p> 4077 */ 4078 @SearchParamDefinition(name="source", path="Consent.source", description="Source from which this consent is taken", type="reference", target={Consent.class, Contract.class, DocumentReference.class, QuestionnaireResponse.class } ) 4079 public static final String SP_SOURCE = "source"; 4080 /** 4081 * <b>Fluent Client</b> search parameter constant for <b>source</b> 4082 * <p> 4083 * Description: <b>Source from which this consent is taken</b><br> 4084 * Type: <b>reference</b><br> 4085 * Path: <b>Consent.source[x]</b><br> 4086 * </p> 4087 */ 4088 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 4089 4090/** 4091 * Constant for fluent queries to be used to add include statements. Specifies 4092 * the path value of "<b>Consent:source</b>". 4093 */ 4094 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("Consent:source").toLocked(); 4095 4096 /** 4097 * Search parameter: <b>actor</b> 4098 * <p> 4099 * Description: <b>Resource for the actor (or group, by role)</b><br> 4100 * Type: <b>reference</b><br> 4101 * Path: <b>Consent.actor.reference, Consent.except.actor.reference</b><br> 4102 * </p> 4103 */ 4104 @SearchParamDefinition(name="actor", path="Consent.actor.reference | Consent.except.actor.reference", description="Resource for the actor (or group, by role)", type="reference", target={CareTeam.class, Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 4105 public static final String SP_ACTOR = "actor"; 4106 /** 4107 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 4108 * <p> 4109 * Description: <b>Resource for the actor (or group, by role)</b><br> 4110 * Type: <b>reference</b><br> 4111 * Path: <b>Consent.actor.reference, Consent.except.actor.reference</b><br> 4112 * </p> 4113 */ 4114 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 4115 4116/** 4117 * Constant for fluent queries to be used to add include statements. Specifies 4118 * the path value of "<b>Consent:actor</b>". 4119 */ 4120 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Consent:actor").toLocked(); 4121 4122 /** 4123 * Search parameter: <b>patient</b> 4124 * <p> 4125 * Description: <b>Who the consent applies to</b><br> 4126 * Type: <b>reference</b><br> 4127 * Path: <b>Consent.patient</b><br> 4128 * </p> 4129 */ 4130 @SearchParamDefinition(name="patient", path="Consent.patient", description="Who the consent applies to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 4131 public static final String SP_PATIENT = "patient"; 4132 /** 4133 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4134 * <p> 4135 * Description: <b>Who the consent applies to</b><br> 4136 * Type: <b>reference</b><br> 4137 * Path: <b>Consent.patient</b><br> 4138 * </p> 4139 */ 4140 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4141 4142/** 4143 * Constant for fluent queries to be used to add include statements. Specifies 4144 * the path value of "<b>Consent:patient</b>". 4145 */ 4146 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Consent:patient").toLocked(); 4147 4148 /** 4149 * Search parameter: <b>organization</b> 4150 * <p> 4151 * Description: <b>Custodian of the consent</b><br> 4152 * Type: <b>reference</b><br> 4153 * Path: <b>Consent.organization</b><br> 4154 * </p> 4155 */ 4156 @SearchParamDefinition(name="organization", path="Consent.organization", description="Custodian of the consent", type="reference", target={Organization.class } ) 4157 public static final String SP_ORGANIZATION = "organization"; 4158 /** 4159 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 4160 * <p> 4161 * Description: <b>Custodian of the consent</b><br> 4162 * Type: <b>reference</b><br> 4163 * Path: <b>Consent.organization</b><br> 4164 * </p> 4165 */ 4166 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 4167 4168/** 4169 * Constant for fluent queries to be used to add include statements. Specifies 4170 * the path value of "<b>Consent:organization</b>". 4171 */ 4172 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Consent:organization").toLocked(); 4173 4174 /** 4175 * Search parameter: <b>action</b> 4176 * <p> 4177 * Description: <b>Actions controlled by this consent</b><br> 4178 * Type: <b>token</b><br> 4179 * Path: <b>Consent.action, Consent.except.action</b><br> 4180 * </p> 4181 */ 4182 @SearchParamDefinition(name="action", path="Consent.action | Consent.except.action", description="Actions controlled by this consent", type="token" ) 4183 public static final String SP_ACTION = "action"; 4184 /** 4185 * <b>Fluent Client</b> search parameter constant for <b>action</b> 4186 * <p> 4187 * Description: <b>Actions controlled by this consent</b><br> 4188 * Type: <b>token</b><br> 4189 * Path: <b>Consent.action, Consent.except.action</b><br> 4190 * </p> 4191 */ 4192 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTION); 4193 4194 /** 4195 * Search parameter: <b>consentor</b> 4196 * <p> 4197 * Description: <b>Who is agreeing to the policy and exceptions</b><br> 4198 * Type: <b>reference</b><br> 4199 * Path: <b>Consent.consentingParty</b><br> 4200 * </p> 4201 */ 4202 @SearchParamDefinition(name="consentor", path="Consent.consentingParty", description="Who is agreeing to the policy and exceptions", type="reference", target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 4203 public static final String SP_CONSENTOR = "consentor"; 4204 /** 4205 * <b>Fluent Client</b> search parameter constant for <b>consentor</b> 4206 * <p> 4207 * Description: <b>Who is agreeing to the policy and exceptions</b><br> 4208 * Type: <b>reference</b><br> 4209 * Path: <b>Consent.consentingParty</b><br> 4210 * </p> 4211 */ 4212 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONSENTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONSENTOR); 4213 4214/** 4215 * Constant for fluent queries to be used to add include statements. Specifies 4216 * the path value of "<b>Consent:consentor</b>". 4217 */ 4218 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONSENTOR = new ca.uhn.fhir.model.api.Include("Consent:consentor").toLocked(); 4219 4220 /** 4221 * Search parameter: <b>category</b> 4222 * <p> 4223 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 4224 * Type: <b>token</b><br> 4225 * Path: <b>Consent.category</b><br> 4226 * </p> 4227 */ 4228 @SearchParamDefinition(name="category", path="Consent.category", description="Classification of the consent statement - for indexing/retrieval", type="token" ) 4229 public static final String SP_CATEGORY = "category"; 4230 /** 4231 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4232 * <p> 4233 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 4234 * Type: <b>token</b><br> 4235 * Path: <b>Consent.category</b><br> 4236 * </p> 4237 */ 4238 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4239 4240 /** 4241 * Search parameter: <b>status</b> 4242 * <p> 4243 * Description: <b>draft | proposed | active | rejected | inactive | entered-in-error</b><br> 4244 * Type: <b>token</b><br> 4245 * Path: <b>Consent.status</b><br> 4246 * </p> 4247 */ 4248 @SearchParamDefinition(name="status", path="Consent.status", description="draft | proposed | active | rejected | inactive | entered-in-error", type="token" ) 4249 public static final String SP_STATUS = "status"; 4250 /** 4251 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4252 * <p> 4253 * Description: <b>draft | proposed | active | rejected | inactive | entered-in-error</b><br> 4254 * Type: <b>token</b><br> 4255 * Path: <b>Consent.status</b><br> 4256 * </p> 4257 */ 4258 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4259 4260 4261}