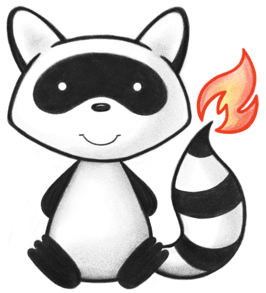
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 051 */ 052@ResourceDef(name="Consent", profile="http://hl7.org/fhir/Profile/Consent") 053public class Consent extends DomainResource { 054 055 public enum ConsentState { 056 /** 057 * The consent is in development or awaiting use but is not yet intended to be acted upon. 058 */ 059 DRAFT, 060 /** 061 * The consent has been proposed but not yet agreed to by all parties. The negotiation stage. 062 */ 063 PROPOSED, 064 /** 065 * The consent is to be followed and enforced. 066 */ 067 ACTIVE, 068 /** 069 * The consent has been rejected by one or more of the parties. 070 */ 071 REJECTED, 072 /** 073 * The consent is terminated or replaced. 074 */ 075 INACTIVE, 076 /** 077 * The consent was created wrongly (e.g. wrong patient) and should be ignored 078 */ 079 ENTEREDINERROR, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static ConsentState fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("draft".equals(codeString)) 088 return DRAFT; 089 if ("proposed".equals(codeString)) 090 return PROPOSED; 091 if ("active".equals(codeString)) 092 return ACTIVE; 093 if ("rejected".equals(codeString)) 094 return REJECTED; 095 if ("inactive".equals(codeString)) 096 return INACTIVE; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 103 } 104 public String toCode() { 105 switch (this) { 106 case DRAFT: return "draft"; 107 case PROPOSED: return "proposed"; 108 case ACTIVE: return "active"; 109 case REJECTED: return "rejected"; 110 case INACTIVE: return "inactive"; 111 case ENTEREDINERROR: return "entered-in-error"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getSystem() { 117 switch (this) { 118 case DRAFT: return "http://hl7.org/fhir/consent-state-codes"; 119 case PROPOSED: return "http://hl7.org/fhir/consent-state-codes"; 120 case ACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 121 case REJECTED: return "http://hl7.org/fhir/consent-state-codes"; 122 case INACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 123 case ENTEREDINERROR: return "http://hl7.org/fhir/consent-state-codes"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 public String getDefinition() { 129 switch (this) { 130 case DRAFT: return "The consent is in development or awaiting use but is not yet intended to be acted upon."; 131 case PROPOSED: return "The consent has been proposed but not yet agreed to by all parties. The negotiation stage."; 132 case ACTIVE: return "The consent is to be followed and enforced."; 133 case REJECTED: return "The consent has been rejected by one or more of the parties."; 134 case INACTIVE: return "The consent is terminated or replaced."; 135 case ENTEREDINERROR: return "The consent was created wrongly (e.g. wrong patient) and should be ignored"; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 public String getDisplay() { 141 switch (this) { 142 case DRAFT: return "Pending"; 143 case PROPOSED: return "Proposed"; 144 case ACTIVE: return "Active"; 145 case REJECTED: return "Rejected"; 146 case INACTIVE: return "Inactive"; 147 case ENTEREDINERROR: return "Entered in Error"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 } 153 154 public static class ConsentStateEnumFactory implements EnumFactory<ConsentState> { 155 public ConsentState fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("draft".equals(codeString)) 160 return ConsentState.DRAFT; 161 if ("proposed".equals(codeString)) 162 return ConsentState.PROPOSED; 163 if ("active".equals(codeString)) 164 return ConsentState.ACTIVE; 165 if ("rejected".equals(codeString)) 166 return ConsentState.REJECTED; 167 if ("inactive".equals(codeString)) 168 return ConsentState.INACTIVE; 169 if ("entered-in-error".equals(codeString)) 170 return ConsentState.ENTEREDINERROR; 171 throw new IllegalArgumentException("Unknown ConsentState code '"+codeString+"'"); 172 } 173 public Enumeration<ConsentState> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<ConsentState>(this); 178 String codeString = code.asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("draft".equals(codeString)) 182 return new Enumeration<ConsentState>(this, ConsentState.DRAFT); 183 if ("proposed".equals(codeString)) 184 return new Enumeration<ConsentState>(this, ConsentState.PROPOSED); 185 if ("active".equals(codeString)) 186 return new Enumeration<ConsentState>(this, ConsentState.ACTIVE); 187 if ("rejected".equals(codeString)) 188 return new Enumeration<ConsentState>(this, ConsentState.REJECTED); 189 if ("inactive".equals(codeString)) 190 return new Enumeration<ConsentState>(this, ConsentState.INACTIVE); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<ConsentState>(this, ConsentState.ENTEREDINERROR); 193 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 194 } 195 public String toCode(ConsentState code) { 196 if (code == ConsentState.DRAFT) 197 return "draft"; 198 if (code == ConsentState.PROPOSED) 199 return "proposed"; 200 if (code == ConsentState.ACTIVE) 201 return "active"; 202 if (code == ConsentState.REJECTED) 203 return "rejected"; 204 if (code == ConsentState.INACTIVE) 205 return "inactive"; 206 if (code == ConsentState.ENTEREDINERROR) 207 return "entered-in-error"; 208 return "?"; 209 } 210 public String toSystem(ConsentState code) { 211 return code.getSystem(); 212 } 213 } 214 215 public enum ConsentDataMeaning { 216 /** 217 * The consent applies directly to the instance of the resource 218 */ 219 INSTANCE, 220 /** 221 * The consent applies directly to the instance of the resource and instances it refers to 222 */ 223 RELATED, 224 /** 225 * The consent applies directly to the instance of the resource and instances that refer to it 226 */ 227 DEPENDENTS, 228 /** 229 * The consent applies to instances of resources that are authored by 230 */ 231 AUTHOREDBY, 232 /** 233 * added to help the parsers with the generic types 234 */ 235 NULL; 236 public static ConsentDataMeaning fromCode(String codeString) throws FHIRException { 237 if (codeString == null || "".equals(codeString)) 238 return null; 239 if ("instance".equals(codeString)) 240 return INSTANCE; 241 if ("related".equals(codeString)) 242 return RELATED; 243 if ("dependents".equals(codeString)) 244 return DEPENDENTS; 245 if ("authoredby".equals(codeString)) 246 return AUTHOREDBY; 247 if (Configuration.isAcceptInvalidEnums()) 248 return null; 249 else 250 throw new FHIRException("Unknown ConsentDataMeaning code '"+codeString+"'"); 251 } 252 public String toCode() { 253 switch (this) { 254 case INSTANCE: return "instance"; 255 case RELATED: return "related"; 256 case DEPENDENTS: return "dependents"; 257 case AUTHOREDBY: return "authoredby"; 258 case NULL: return null; 259 default: return "?"; 260 } 261 } 262 public String getSystem() { 263 switch (this) { 264 case INSTANCE: return "http://hl7.org/fhir/consent-data-meaning"; 265 case RELATED: return "http://hl7.org/fhir/consent-data-meaning"; 266 case DEPENDENTS: return "http://hl7.org/fhir/consent-data-meaning"; 267 case AUTHOREDBY: return "http://hl7.org/fhir/consent-data-meaning"; 268 case NULL: return null; 269 default: return "?"; 270 } 271 } 272 public String getDefinition() { 273 switch (this) { 274 case INSTANCE: return "The consent applies directly to the instance of the resource"; 275 case RELATED: return "The consent applies directly to the instance of the resource and instances it refers to"; 276 case DEPENDENTS: return "The consent applies directly to the instance of the resource and instances that refer to it"; 277 case AUTHOREDBY: return "The consent applies to instances of resources that are authored by"; 278 case NULL: return null; 279 default: return "?"; 280 } 281 } 282 public String getDisplay() { 283 switch (this) { 284 case INSTANCE: return "Instance"; 285 case RELATED: return "Related"; 286 case DEPENDENTS: return "Dependents"; 287 case AUTHOREDBY: return "AuthoredBy"; 288 case NULL: return null; 289 default: return "?"; 290 } 291 } 292 } 293 294 public static class ConsentDataMeaningEnumFactory implements EnumFactory<ConsentDataMeaning> { 295 public ConsentDataMeaning fromCode(String codeString) throws IllegalArgumentException { 296 if (codeString == null || "".equals(codeString)) 297 if (codeString == null || "".equals(codeString)) 298 return null; 299 if ("instance".equals(codeString)) 300 return ConsentDataMeaning.INSTANCE; 301 if ("related".equals(codeString)) 302 return ConsentDataMeaning.RELATED; 303 if ("dependents".equals(codeString)) 304 return ConsentDataMeaning.DEPENDENTS; 305 if ("authoredby".equals(codeString)) 306 return ConsentDataMeaning.AUTHOREDBY; 307 throw new IllegalArgumentException("Unknown ConsentDataMeaning code '"+codeString+"'"); 308 } 309 public Enumeration<ConsentDataMeaning> fromType(PrimitiveType<?> code) throws FHIRException { 310 if (code == null) 311 return null; 312 if (code.isEmpty()) 313 return new Enumeration<ConsentDataMeaning>(this); 314 String codeString = code.asStringValue(); 315 if (codeString == null || "".equals(codeString)) 316 return null; 317 if ("instance".equals(codeString)) 318 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.INSTANCE); 319 if ("related".equals(codeString)) 320 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.RELATED); 321 if ("dependents".equals(codeString)) 322 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.DEPENDENTS); 323 if ("authoredby".equals(codeString)) 324 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.AUTHOREDBY); 325 throw new FHIRException("Unknown ConsentDataMeaning code '"+codeString+"'"); 326 } 327 public String toCode(ConsentDataMeaning code) { 328 if (code == ConsentDataMeaning.INSTANCE) 329 return "instance"; 330 if (code == ConsentDataMeaning.RELATED) 331 return "related"; 332 if (code == ConsentDataMeaning.DEPENDENTS) 333 return "dependents"; 334 if (code == ConsentDataMeaning.AUTHOREDBY) 335 return "authoredby"; 336 return "?"; 337 } 338 public String toSystem(ConsentDataMeaning code) { 339 return code.getSystem(); 340 } 341 } 342 343 public enum ConsentExceptType { 344 /** 345 * Consent is denied for actions meeting these rules 346 */ 347 DENY, 348 /** 349 * Consent is provided for actions meeting these rules 350 */ 351 PERMIT, 352 /** 353 * added to help the parsers with the generic types 354 */ 355 NULL; 356 public static ConsentExceptType fromCode(String codeString) throws FHIRException { 357 if (codeString == null || "".equals(codeString)) 358 return null; 359 if ("deny".equals(codeString)) 360 return DENY; 361 if ("permit".equals(codeString)) 362 return PERMIT; 363 if (Configuration.isAcceptInvalidEnums()) 364 return null; 365 else 366 throw new FHIRException("Unknown ConsentExceptType code '"+codeString+"'"); 367 } 368 public String toCode() { 369 switch (this) { 370 case DENY: return "deny"; 371 case PERMIT: return "permit"; 372 case NULL: return null; 373 default: return "?"; 374 } 375 } 376 public String getSystem() { 377 switch (this) { 378 case DENY: return "http://hl7.org/fhir/consent-except-type"; 379 case PERMIT: return "http://hl7.org/fhir/consent-except-type"; 380 case NULL: return null; 381 default: return "?"; 382 } 383 } 384 public String getDefinition() { 385 switch (this) { 386 case DENY: return "Consent is denied for actions meeting these rules"; 387 case PERMIT: return "Consent is provided for actions meeting these rules"; 388 case NULL: return null; 389 default: return "?"; 390 } 391 } 392 public String getDisplay() { 393 switch (this) { 394 case DENY: return "Opt Out"; 395 case PERMIT: return "Opt In"; 396 case NULL: return null; 397 default: return "?"; 398 } 399 } 400 } 401 402 public static class ConsentExceptTypeEnumFactory implements EnumFactory<ConsentExceptType> { 403 public ConsentExceptType fromCode(String codeString) throws IllegalArgumentException { 404 if (codeString == null || "".equals(codeString)) 405 if (codeString == null || "".equals(codeString)) 406 return null; 407 if ("deny".equals(codeString)) 408 return ConsentExceptType.DENY; 409 if ("permit".equals(codeString)) 410 return ConsentExceptType.PERMIT; 411 throw new IllegalArgumentException("Unknown ConsentExceptType code '"+codeString+"'"); 412 } 413 public Enumeration<ConsentExceptType> fromType(PrimitiveType<?> code) throws FHIRException { 414 if (code == null) 415 return null; 416 if (code.isEmpty()) 417 return new Enumeration<ConsentExceptType>(this); 418 String codeString = code.asStringValue(); 419 if (codeString == null || "".equals(codeString)) 420 return null; 421 if ("deny".equals(codeString)) 422 return new Enumeration<ConsentExceptType>(this, ConsentExceptType.DENY); 423 if ("permit".equals(codeString)) 424 return new Enumeration<ConsentExceptType>(this, ConsentExceptType.PERMIT); 425 throw new FHIRException("Unknown ConsentExceptType code '"+codeString+"'"); 426 } 427 public String toCode(ConsentExceptType code) { 428 if (code == ConsentExceptType.DENY) 429 return "deny"; 430 if (code == ConsentExceptType.PERMIT) 431 return "permit"; 432 return "?"; 433 } 434 public String toSystem(ConsentExceptType code) { 435 return code.getSystem(); 436 } 437 } 438 439 @Block() 440 public static class ConsentActorComponent extends BackboneElement implements IBaseBackboneElement { 441 /** 442 * How the individual is involved in the resources content that is described in the consent. 443 */ 444 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 445 @Description(shortDefinition="How the actor is involved", formalDefinition="How the individual is involved in the resources content that is described in the consent." ) 446 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 447 protected CodeableConcept role; 448 449 /** 450 * The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 451 */ 452 @Child(name = "reference", type = {Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 453 @Description(shortDefinition="Resource for the actor (or group, by role)", formalDefinition="The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 454 protected Reference reference; 455 456 /** 457 * The actual object that is the target of the reference (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 458 */ 459 protected Resource referenceTarget; 460 461 private static final long serialVersionUID = 1152919415L; 462 463 /** 464 * Constructor 465 */ 466 public ConsentActorComponent() { 467 super(); 468 } 469 470 /** 471 * Constructor 472 */ 473 public ConsentActorComponent(CodeableConcept role, Reference reference) { 474 super(); 475 this.role = role; 476 this.reference = reference; 477 } 478 479 /** 480 * @return {@link #role} (How the individual is involved in the resources content that is described in the consent.) 481 */ 482 public CodeableConcept getRole() { 483 if (this.role == null) 484 if (Configuration.errorOnAutoCreate()) 485 throw new Error("Attempt to auto-create ConsentActorComponent.role"); 486 else if (Configuration.doAutoCreate()) 487 this.role = new CodeableConcept(); // cc 488 return this.role; 489 } 490 491 public boolean hasRole() { 492 return this.role != null && !this.role.isEmpty(); 493 } 494 495 /** 496 * @param value {@link #role} (How the individual is involved in the resources content that is described in the consent.) 497 */ 498 public ConsentActorComponent setRole(CodeableConcept value) { 499 this.role = value; 500 return this; 501 } 502 503 /** 504 * @return {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 505 */ 506 public Reference getReference() { 507 if (this.reference == null) 508 if (Configuration.errorOnAutoCreate()) 509 throw new Error("Attempt to auto-create ConsentActorComponent.reference"); 510 else if (Configuration.doAutoCreate()) 511 this.reference = new Reference(); // cc 512 return this.reference; 513 } 514 515 public boolean hasReference() { 516 return this.reference != null && !this.reference.isEmpty(); 517 } 518 519 /** 520 * @param value {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 521 */ 522 public ConsentActorComponent setReference(Reference value) { 523 this.reference = value; 524 return this; 525 } 526 527 /** 528 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 529 */ 530 public Resource getReferenceTarget() { 531 return this.referenceTarget; 532 } 533 534 /** 535 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 536 */ 537 public ConsentActorComponent setReferenceTarget(Resource value) { 538 this.referenceTarget = value; 539 return this; 540 } 541 542 protected void listChildren(List<Property> children) { 543 super.listChildren(children); 544 children.add(new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the consent.", 0, 1, role)); 545 children.add(new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference)); 546 } 547 548 @Override 549 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 550 switch (_hash) { 551 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the consent.", 0, 1, role); 552 case -925155509: /*reference*/ return new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference); 553 default: return super.getNamedProperty(_hash, _name, _checkValid); 554 } 555 556 } 557 558 @Override 559 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 560 switch (hash) { 561 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 562 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 563 default: return super.getProperty(hash, name, checkValid); 564 } 565 566 } 567 568 @Override 569 public Base setProperty(int hash, String name, Base value) throws FHIRException { 570 switch (hash) { 571 case 3506294: // role 572 this.role = castToCodeableConcept(value); // CodeableConcept 573 return value; 574 case -925155509: // reference 575 this.reference = castToReference(value); // Reference 576 return value; 577 default: return super.setProperty(hash, name, value); 578 } 579 580 } 581 582 @Override 583 public Base setProperty(String name, Base value) throws FHIRException { 584 if (name.equals("role")) { 585 this.role = castToCodeableConcept(value); // CodeableConcept 586 } else if (name.equals("reference")) { 587 this.reference = castToReference(value); // Reference 588 } else 589 return super.setProperty(name, value); 590 return value; 591 } 592 593 @Override 594 public Base makeProperty(int hash, String name) throws FHIRException { 595 switch (hash) { 596 case 3506294: return getRole(); 597 case -925155509: return getReference(); 598 default: return super.makeProperty(hash, name); 599 } 600 601 } 602 603 @Override 604 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 605 switch (hash) { 606 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 607 case -925155509: /*reference*/ return new String[] {"Reference"}; 608 default: return super.getTypesForProperty(hash, name); 609 } 610 611 } 612 613 @Override 614 public Base addChild(String name) throws FHIRException { 615 if (name.equals("role")) { 616 this.role = new CodeableConcept(); 617 return this.role; 618 } 619 else if (name.equals("reference")) { 620 this.reference = new Reference(); 621 return this.reference; 622 } 623 else 624 return super.addChild(name); 625 } 626 627 public ConsentActorComponent copy() { 628 ConsentActorComponent dst = new ConsentActorComponent(); 629 copyValues(dst); 630 dst.role = role == null ? null : role.copy(); 631 dst.reference = reference == null ? null : reference.copy(); 632 return dst; 633 } 634 635 @Override 636 public boolean equalsDeep(Base other_) { 637 if (!super.equalsDeep(other_)) 638 return false; 639 if (!(other_ instanceof ConsentActorComponent)) 640 return false; 641 ConsentActorComponent o = (ConsentActorComponent) other_; 642 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 643 } 644 645 @Override 646 public boolean equalsShallow(Base other_) { 647 if (!super.equalsShallow(other_)) 648 return false; 649 if (!(other_ instanceof ConsentActorComponent)) 650 return false; 651 ConsentActorComponent o = (ConsentActorComponent) other_; 652 return true; 653 } 654 655 public boolean isEmpty() { 656 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 657 } 658 659 public String fhirType() { 660 return "Consent.actor"; 661 662 } 663 664 } 665 666 @Block() 667 public static class ConsentPolicyComponent extends BackboneElement implements IBaseBackboneElement { 668 /** 669 * Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives. 670 */ 671 @Child(name = "authority", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 672 @Description(shortDefinition="Enforcement source for policy", formalDefinition="Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives." ) 673 protected UriType authority; 674 675 /** 676 * The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 677 */ 678 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 679 @Description(shortDefinition="Specific policy covered by this consent", formalDefinition="The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law." ) 680 protected UriType uri; 681 682 private static final long serialVersionUID = 672275705L; 683 684 /** 685 * Constructor 686 */ 687 public ConsentPolicyComponent() { 688 super(); 689 } 690 691 /** 692 * @return {@link #authority} (Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.). This is the underlying object with id, value and extensions. The accessor "getAuthority" gives direct access to the value 693 */ 694 public UriType getAuthorityElement() { 695 if (this.authority == null) 696 if (Configuration.errorOnAutoCreate()) 697 throw new Error("Attempt to auto-create ConsentPolicyComponent.authority"); 698 else if (Configuration.doAutoCreate()) 699 this.authority = new UriType(); // bb 700 return this.authority; 701 } 702 703 public boolean hasAuthorityElement() { 704 return this.authority != null && !this.authority.isEmpty(); 705 } 706 707 public boolean hasAuthority() { 708 return this.authority != null && !this.authority.isEmpty(); 709 } 710 711 /** 712 * @param value {@link #authority} (Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.). This is the underlying object with id, value and extensions. The accessor "getAuthority" gives direct access to the value 713 */ 714 public ConsentPolicyComponent setAuthorityElement(UriType value) { 715 this.authority = value; 716 return this; 717 } 718 719 /** 720 * @return Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives. 721 */ 722 public String getAuthority() { 723 return this.authority == null ? null : this.authority.getValue(); 724 } 725 726 /** 727 * @param value Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives. 728 */ 729 public ConsentPolicyComponent setAuthority(String value) { 730 if (Utilities.noString(value)) 731 this.authority = null; 732 else { 733 if (this.authority == null) 734 this.authority = new UriType(); 735 this.authority.setValue(value); 736 } 737 return this; 738 } 739 740 /** 741 * @return {@link #uri} (The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 742 */ 743 public UriType getUriElement() { 744 if (this.uri == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create ConsentPolicyComponent.uri"); 747 else if (Configuration.doAutoCreate()) 748 this.uri = new UriType(); // bb 749 return this.uri; 750 } 751 752 public boolean hasUriElement() { 753 return this.uri != null && !this.uri.isEmpty(); 754 } 755 756 public boolean hasUri() { 757 return this.uri != null && !this.uri.isEmpty(); 758 } 759 760 /** 761 * @param value {@link #uri} (The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 762 */ 763 public ConsentPolicyComponent setUriElement(UriType value) { 764 this.uri = value; 765 return this; 766 } 767 768 /** 769 * @return The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 770 */ 771 public String getUri() { 772 return this.uri == null ? null : this.uri.getValue(); 773 } 774 775 /** 776 * @param value The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 777 */ 778 public ConsentPolicyComponent setUri(String value) { 779 if (Utilities.noString(value)) 780 this.uri = null; 781 else { 782 if (this.uri == null) 783 this.uri = new UriType(); 784 this.uri.setValue(value); 785 } 786 return this; 787 } 788 789 protected void listChildren(List<Property> children) { 790 super.listChildren(children); 791 children.add(new Property("authority", "uri", "Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.", 0, 1, authority)); 792 children.add(new Property("uri", "uri", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, 1, uri)); 793 } 794 795 @Override 796 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 797 switch (_hash) { 798 case 1475610435: /*authority*/ return new Property("authority", "uri", "Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.", 0, 1, authority); 799 case 116076: /*uri*/ return new Property("uri", "uri", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, 1, uri); 800 default: return super.getNamedProperty(_hash, _name, _checkValid); 801 } 802 803 } 804 805 @Override 806 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 807 switch (hash) { 808 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // UriType 809 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 810 default: return super.getProperty(hash, name, checkValid); 811 } 812 813 } 814 815 @Override 816 public Base setProperty(int hash, String name, Base value) throws FHIRException { 817 switch (hash) { 818 case 1475610435: // authority 819 this.authority = castToUri(value); // UriType 820 return value; 821 case 116076: // uri 822 this.uri = castToUri(value); // UriType 823 return value; 824 default: return super.setProperty(hash, name, value); 825 } 826 827 } 828 829 @Override 830 public Base setProperty(String name, Base value) throws FHIRException { 831 if (name.equals("authority")) { 832 this.authority = castToUri(value); // UriType 833 } else if (name.equals("uri")) { 834 this.uri = castToUri(value); // UriType 835 } else 836 return super.setProperty(name, value); 837 return value; 838 } 839 840 @Override 841 public Base makeProperty(int hash, String name) throws FHIRException { 842 switch (hash) { 843 case 1475610435: return getAuthorityElement(); 844 case 116076: return getUriElement(); 845 default: return super.makeProperty(hash, name); 846 } 847 848 } 849 850 @Override 851 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 852 switch (hash) { 853 case 1475610435: /*authority*/ return new String[] {"uri"}; 854 case 116076: /*uri*/ return new String[] {"uri"}; 855 default: return super.getTypesForProperty(hash, name); 856 } 857 858 } 859 860 @Override 861 public Base addChild(String name) throws FHIRException { 862 if (name.equals("authority")) { 863 throw new FHIRException("Cannot call addChild on a singleton property Consent.authority"); 864 } 865 else if (name.equals("uri")) { 866 throw new FHIRException("Cannot call addChild on a singleton property Consent.uri"); 867 } 868 else 869 return super.addChild(name); 870 } 871 872 public ConsentPolicyComponent copy() { 873 ConsentPolicyComponent dst = new ConsentPolicyComponent(); 874 copyValues(dst); 875 dst.authority = authority == null ? null : authority.copy(); 876 dst.uri = uri == null ? null : uri.copy(); 877 return dst; 878 } 879 880 @Override 881 public boolean equalsDeep(Base other_) { 882 if (!super.equalsDeep(other_)) 883 return false; 884 if (!(other_ instanceof ConsentPolicyComponent)) 885 return false; 886 ConsentPolicyComponent o = (ConsentPolicyComponent) other_; 887 return compareDeep(authority, o.authority, true) && compareDeep(uri, o.uri, true); 888 } 889 890 @Override 891 public boolean equalsShallow(Base other_) { 892 if (!super.equalsShallow(other_)) 893 return false; 894 if (!(other_ instanceof ConsentPolicyComponent)) 895 return false; 896 ConsentPolicyComponent o = (ConsentPolicyComponent) other_; 897 return compareValues(authority, o.authority, true) && compareValues(uri, o.uri, true); 898 } 899 900 public boolean isEmpty() { 901 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(authority, uri); 902 } 903 904 public String fhirType() { 905 return "Consent.policy"; 906 907 } 908 909 } 910 911 @Block() 912 public static class ConsentDataComponent extends BackboneElement implements IBaseBackboneElement { 913 /** 914 * How the resource reference is interpreted when testing consent restrictions. 915 */ 916 @Child(name = "meaning", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 917 @Description(shortDefinition="instance | related | dependents | authoredby", formalDefinition="How the resource reference is interpreted when testing consent restrictions." ) 918 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-data-meaning") 919 protected Enumeration<ConsentDataMeaning> meaning; 920 921 /** 922 * A reference to a specific resource that defines which resources are covered by this consent. 923 */ 924 @Child(name = "reference", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 925 @Description(shortDefinition="The actual data reference", formalDefinition="A reference to a specific resource that defines which resources are covered by this consent." ) 926 protected Reference reference; 927 928 /** 929 * The actual object that is the target of the reference (A reference to a specific resource that defines which resources are covered by this consent.) 930 */ 931 protected Resource referenceTarget; 932 933 private static final long serialVersionUID = -424898645L; 934 935 /** 936 * Constructor 937 */ 938 public ConsentDataComponent() { 939 super(); 940 } 941 942 /** 943 * Constructor 944 */ 945 public ConsentDataComponent(Enumeration<ConsentDataMeaning> meaning, Reference reference) { 946 super(); 947 this.meaning = meaning; 948 this.reference = reference; 949 } 950 951 /** 952 * @return {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 953 */ 954 public Enumeration<ConsentDataMeaning> getMeaningElement() { 955 if (this.meaning == null) 956 if (Configuration.errorOnAutoCreate()) 957 throw new Error("Attempt to auto-create ConsentDataComponent.meaning"); 958 else if (Configuration.doAutoCreate()) 959 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 960 return this.meaning; 961 } 962 963 public boolean hasMeaningElement() { 964 return this.meaning != null && !this.meaning.isEmpty(); 965 } 966 967 public boolean hasMeaning() { 968 return this.meaning != null && !this.meaning.isEmpty(); 969 } 970 971 /** 972 * @param value {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 973 */ 974 public ConsentDataComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 975 this.meaning = value; 976 return this; 977 } 978 979 /** 980 * @return How the resource reference is interpreted when testing consent restrictions. 981 */ 982 public ConsentDataMeaning getMeaning() { 983 return this.meaning == null ? null : this.meaning.getValue(); 984 } 985 986 /** 987 * @param value How the resource reference is interpreted when testing consent restrictions. 988 */ 989 public ConsentDataComponent setMeaning(ConsentDataMeaning value) { 990 if (this.meaning == null) 991 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 992 this.meaning.setValue(value); 993 return this; 994 } 995 996 /** 997 * @return {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 998 */ 999 public Reference getReference() { 1000 if (this.reference == null) 1001 if (Configuration.errorOnAutoCreate()) 1002 throw new Error("Attempt to auto-create ConsentDataComponent.reference"); 1003 else if (Configuration.doAutoCreate()) 1004 this.reference = new Reference(); // cc 1005 return this.reference; 1006 } 1007 1008 public boolean hasReference() { 1009 return this.reference != null && !this.reference.isEmpty(); 1010 } 1011 1012 /** 1013 * @param value {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 1014 */ 1015 public ConsentDataComponent setReference(Reference value) { 1016 this.reference = value; 1017 return this; 1018 } 1019 1020 /** 1021 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 1022 */ 1023 public Resource getReferenceTarget() { 1024 return this.referenceTarget; 1025 } 1026 1027 /** 1028 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 1029 */ 1030 public ConsentDataComponent setReferenceTarget(Resource value) { 1031 this.referenceTarget = value; 1032 return this; 1033 } 1034 1035 protected void listChildren(List<Property> children) { 1036 super.listChildren(children); 1037 children.add(new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 1038 children.add(new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference)); 1039 } 1040 1041 @Override 1042 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1043 switch (_hash) { 1044 case 938160637: /*meaning*/ return new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 1045 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference); 1046 default: return super.getNamedProperty(_hash, _name, _checkValid); 1047 } 1048 1049 } 1050 1051 @Override 1052 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1053 switch (hash) { 1054 case 938160637: /*meaning*/ return this.meaning == null ? new Base[0] : new Base[] {this.meaning}; // Enumeration<ConsentDataMeaning> 1055 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1056 default: return super.getProperty(hash, name, checkValid); 1057 } 1058 1059 } 1060 1061 @Override 1062 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1063 switch (hash) { 1064 case 938160637: // meaning 1065 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 1066 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 1067 return value; 1068 case -925155509: // reference 1069 this.reference = castToReference(value); // Reference 1070 return value; 1071 default: return super.setProperty(hash, name, value); 1072 } 1073 1074 } 1075 1076 @Override 1077 public Base setProperty(String name, Base value) throws FHIRException { 1078 if (name.equals("meaning")) { 1079 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 1080 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 1081 } else if (name.equals("reference")) { 1082 this.reference = castToReference(value); // Reference 1083 } else 1084 return super.setProperty(name, value); 1085 return value; 1086 } 1087 1088 @Override 1089 public Base makeProperty(int hash, String name) throws FHIRException { 1090 switch (hash) { 1091 case 938160637: return getMeaningElement(); 1092 case -925155509: return getReference(); 1093 default: return super.makeProperty(hash, name); 1094 } 1095 1096 } 1097 1098 @Override 1099 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1100 switch (hash) { 1101 case 938160637: /*meaning*/ return new String[] {"code"}; 1102 case -925155509: /*reference*/ return new String[] {"Reference"}; 1103 default: return super.getTypesForProperty(hash, name); 1104 } 1105 1106 } 1107 1108 @Override 1109 public Base addChild(String name) throws FHIRException { 1110 if (name.equals("meaning")) { 1111 throw new FHIRException("Cannot call addChild on a singleton property Consent.meaning"); 1112 } 1113 else if (name.equals("reference")) { 1114 this.reference = new Reference(); 1115 return this.reference; 1116 } 1117 else 1118 return super.addChild(name); 1119 } 1120 1121 public ConsentDataComponent copy() { 1122 ConsentDataComponent dst = new ConsentDataComponent(); 1123 copyValues(dst); 1124 dst.meaning = meaning == null ? null : meaning.copy(); 1125 dst.reference = reference == null ? null : reference.copy(); 1126 return dst; 1127 } 1128 1129 @Override 1130 public boolean equalsDeep(Base other_) { 1131 if (!super.equalsDeep(other_)) 1132 return false; 1133 if (!(other_ instanceof ConsentDataComponent)) 1134 return false; 1135 ConsentDataComponent o = (ConsentDataComponent) other_; 1136 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 1137 } 1138 1139 @Override 1140 public boolean equalsShallow(Base other_) { 1141 if (!super.equalsShallow(other_)) 1142 return false; 1143 if (!(other_ instanceof ConsentDataComponent)) 1144 return false; 1145 ConsentDataComponent o = (ConsentDataComponent) other_; 1146 return compareValues(meaning, o.meaning, true); 1147 } 1148 1149 public boolean isEmpty() { 1150 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 1151 } 1152 1153 public String fhirType() { 1154 return "Consent.data"; 1155 1156 } 1157 1158 } 1159 1160 @Block() 1161 public static class ExceptComponent extends BackboneElement implements IBaseBackboneElement { 1162 /** 1163 * Action to take - permit or deny - when the exception conditions are met. 1164 */ 1165 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1166 @Description(shortDefinition="deny | permit", formalDefinition="Action to take - permit or deny - when the exception conditions are met." ) 1167 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-except-type") 1168 protected Enumeration<ConsentExceptType> type; 1169 1170 /** 1171 * The timeframe in this exception is valid. 1172 */ 1173 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 1174 @Description(shortDefinition="Timeframe for this exception", formalDefinition="The timeframe in this exception is valid." ) 1175 protected Period period; 1176 1177 /** 1178 * Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 1179 */ 1180 @Child(name = "actor", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1181 @Description(shortDefinition="Who|what controlled by this exception (or group, by role)", formalDefinition="Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 1182 protected List<ExceptActorComponent> actor; 1183 1184 /** 1185 * Actions controlled by this Exception. 1186 */ 1187 @Child(name = "action", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1188 @Description(shortDefinition="Actions controlled by this exception", formalDefinition="Actions controlled by this Exception." ) 1189 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-action") 1190 protected List<CodeableConcept> action; 1191 1192 /** 1193 * A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels. 1194 */ 1195 @Child(name = "securityLabel", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1196 @Description(shortDefinition="Security Labels that define affected resources", formalDefinition="A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels." ) 1197 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 1198 protected List<Coding> securityLabel; 1199 1200 /** 1201 * The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception. 1202 */ 1203 @Child(name = "purpose", type = {Coding.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1204 @Description(shortDefinition="Context of activities covered by this exception", formalDefinition="The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception." ) 1205 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 1206 protected List<Coding> purpose; 1207 1208 /** 1209 * The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to. 1210 */ 1211 @Child(name = "class", type = {Coding.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1212 @Description(shortDefinition="e.g. Resource Type, Profile, or CDA etc", formalDefinition="The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to." ) 1213 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-class") 1214 protected List<Coding> class_; 1215 1216 /** 1217 * If this code is found in an instance, then the exception applies. 1218 */ 1219 @Child(name = "code", type = {Coding.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1220 @Description(shortDefinition="e.g. LOINC or SNOMED CT code, etc in the content", formalDefinition="If this code is found in an instance, then the exception applies." ) 1221 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-code") 1222 protected List<Coding> code; 1223 1224 /** 1225 * Clinical or Operational Relevant period of time that bounds the data controlled by this exception. 1226 */ 1227 @Child(name = "dataPeriod", type = {Period.class}, order=9, min=0, max=1, modifier=false, summary=true) 1228 @Description(shortDefinition="Timeframe for data controlled by this exception", formalDefinition="Clinical or Operational Relevant period of time that bounds the data controlled by this exception." ) 1229 protected Period dataPeriod; 1230 1231 /** 1232 * The resources controlled by this exception, if specific resources are referenced. 1233 */ 1234 @Child(name = "data", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1235 @Description(shortDefinition="Data controlled by this exception", formalDefinition="The resources controlled by this exception, if specific resources are referenced." ) 1236 protected List<ExceptDataComponent> data; 1237 1238 private static final long serialVersionUID = 1586636291L; 1239 1240 /** 1241 * Constructor 1242 */ 1243 public ExceptComponent() { 1244 super(); 1245 } 1246 1247 /** 1248 * Constructor 1249 */ 1250 public ExceptComponent(Enumeration<ConsentExceptType> type) { 1251 super(); 1252 this.type = type; 1253 } 1254 1255 /** 1256 * @return {@link #type} (Action to take - permit or deny - when the exception conditions are met.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1257 */ 1258 public Enumeration<ConsentExceptType> getTypeElement() { 1259 if (this.type == null) 1260 if (Configuration.errorOnAutoCreate()) 1261 throw new Error("Attempt to auto-create ExceptComponent.type"); 1262 else if (Configuration.doAutoCreate()) 1263 this.type = new Enumeration<ConsentExceptType>(new ConsentExceptTypeEnumFactory()); // bb 1264 return this.type; 1265 } 1266 1267 public boolean hasTypeElement() { 1268 return this.type != null && !this.type.isEmpty(); 1269 } 1270 1271 public boolean hasType() { 1272 return this.type != null && !this.type.isEmpty(); 1273 } 1274 1275 /** 1276 * @param value {@link #type} (Action to take - permit or deny - when the exception conditions are met.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1277 */ 1278 public ExceptComponent setTypeElement(Enumeration<ConsentExceptType> value) { 1279 this.type = value; 1280 return this; 1281 } 1282 1283 /** 1284 * @return Action to take - permit or deny - when the exception conditions are met. 1285 */ 1286 public ConsentExceptType getType() { 1287 return this.type == null ? null : this.type.getValue(); 1288 } 1289 1290 /** 1291 * @param value Action to take - permit or deny - when the exception conditions are met. 1292 */ 1293 public ExceptComponent setType(ConsentExceptType value) { 1294 if (this.type == null) 1295 this.type = new Enumeration<ConsentExceptType>(new ConsentExceptTypeEnumFactory()); 1296 this.type.setValue(value); 1297 return this; 1298 } 1299 1300 /** 1301 * @return {@link #period} (The timeframe in this exception is valid.) 1302 */ 1303 public Period getPeriod() { 1304 if (this.period == null) 1305 if (Configuration.errorOnAutoCreate()) 1306 throw new Error("Attempt to auto-create ExceptComponent.period"); 1307 else if (Configuration.doAutoCreate()) 1308 this.period = new Period(); // cc 1309 return this.period; 1310 } 1311 1312 public boolean hasPeriod() { 1313 return this.period != null && !this.period.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #period} (The timeframe in this exception is valid.) 1318 */ 1319 public ExceptComponent setPeriod(Period value) { 1320 this.period = value; 1321 return this; 1322 } 1323 1324 /** 1325 * @return {@link #actor} (Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1326 */ 1327 public List<ExceptActorComponent> getActor() { 1328 if (this.actor == null) 1329 this.actor = new ArrayList<ExceptActorComponent>(); 1330 return this.actor; 1331 } 1332 1333 /** 1334 * @return Returns a reference to <code>this</code> for easy method chaining 1335 */ 1336 public ExceptComponent setActor(List<ExceptActorComponent> theActor) { 1337 this.actor = theActor; 1338 return this; 1339 } 1340 1341 public boolean hasActor() { 1342 if (this.actor == null) 1343 return false; 1344 for (ExceptActorComponent item : this.actor) 1345 if (!item.isEmpty()) 1346 return true; 1347 return false; 1348 } 1349 1350 public ExceptActorComponent addActor() { //3 1351 ExceptActorComponent t = new ExceptActorComponent(); 1352 if (this.actor == null) 1353 this.actor = new ArrayList<ExceptActorComponent>(); 1354 this.actor.add(t); 1355 return t; 1356 } 1357 1358 public ExceptComponent addActor(ExceptActorComponent t) { //3 1359 if (t == null) 1360 return this; 1361 if (this.actor == null) 1362 this.actor = new ArrayList<ExceptActorComponent>(); 1363 this.actor.add(t); 1364 return this; 1365 } 1366 1367 /** 1368 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist 1369 */ 1370 public ExceptActorComponent getActorFirstRep() { 1371 if (getActor().isEmpty()) { 1372 addActor(); 1373 } 1374 return getActor().get(0); 1375 } 1376 1377 /** 1378 * @return {@link #action} (Actions controlled by this Exception.) 1379 */ 1380 public List<CodeableConcept> getAction() { 1381 if (this.action == null) 1382 this.action = new ArrayList<CodeableConcept>(); 1383 return this.action; 1384 } 1385 1386 /** 1387 * @return Returns a reference to <code>this</code> for easy method chaining 1388 */ 1389 public ExceptComponent setAction(List<CodeableConcept> theAction) { 1390 this.action = theAction; 1391 return this; 1392 } 1393 1394 public boolean hasAction() { 1395 if (this.action == null) 1396 return false; 1397 for (CodeableConcept item : this.action) 1398 if (!item.isEmpty()) 1399 return true; 1400 return false; 1401 } 1402 1403 public CodeableConcept addAction() { //3 1404 CodeableConcept t = new CodeableConcept(); 1405 if (this.action == null) 1406 this.action = new ArrayList<CodeableConcept>(); 1407 this.action.add(t); 1408 return t; 1409 } 1410 1411 public ExceptComponent addAction(CodeableConcept t) { //3 1412 if (t == null) 1413 return this; 1414 if (this.action == null) 1415 this.action = new ArrayList<CodeableConcept>(); 1416 this.action.add(t); 1417 return this; 1418 } 1419 1420 /** 1421 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 1422 */ 1423 public CodeableConcept getActionFirstRep() { 1424 if (getAction().isEmpty()) { 1425 addAction(); 1426 } 1427 return getAction().get(0); 1428 } 1429 1430 /** 1431 * @return {@link #securityLabel} (A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels.) 1432 */ 1433 public List<Coding> getSecurityLabel() { 1434 if (this.securityLabel == null) 1435 this.securityLabel = new ArrayList<Coding>(); 1436 return this.securityLabel; 1437 } 1438 1439 /** 1440 * @return Returns a reference to <code>this</code> for easy method chaining 1441 */ 1442 public ExceptComponent setSecurityLabel(List<Coding> theSecurityLabel) { 1443 this.securityLabel = theSecurityLabel; 1444 return this; 1445 } 1446 1447 public boolean hasSecurityLabel() { 1448 if (this.securityLabel == null) 1449 return false; 1450 for (Coding item : this.securityLabel) 1451 if (!item.isEmpty()) 1452 return true; 1453 return false; 1454 } 1455 1456 public Coding addSecurityLabel() { //3 1457 Coding t = new Coding(); 1458 if (this.securityLabel == null) 1459 this.securityLabel = new ArrayList<Coding>(); 1460 this.securityLabel.add(t); 1461 return t; 1462 } 1463 1464 public ExceptComponent addSecurityLabel(Coding t) { //3 1465 if (t == null) 1466 return this; 1467 if (this.securityLabel == null) 1468 this.securityLabel = new ArrayList<Coding>(); 1469 this.securityLabel.add(t); 1470 return this; 1471 } 1472 1473 /** 1474 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 1475 */ 1476 public Coding getSecurityLabelFirstRep() { 1477 if (getSecurityLabel().isEmpty()) { 1478 addSecurityLabel(); 1479 } 1480 return getSecurityLabel().get(0); 1481 } 1482 1483 /** 1484 * @return {@link #purpose} (The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception.) 1485 */ 1486 public List<Coding> getPurpose() { 1487 if (this.purpose == null) 1488 this.purpose = new ArrayList<Coding>(); 1489 return this.purpose; 1490 } 1491 1492 /** 1493 * @return Returns a reference to <code>this</code> for easy method chaining 1494 */ 1495 public ExceptComponent setPurpose(List<Coding> thePurpose) { 1496 this.purpose = thePurpose; 1497 return this; 1498 } 1499 1500 public boolean hasPurpose() { 1501 if (this.purpose == null) 1502 return false; 1503 for (Coding item : this.purpose) 1504 if (!item.isEmpty()) 1505 return true; 1506 return false; 1507 } 1508 1509 public Coding addPurpose() { //3 1510 Coding t = new Coding(); 1511 if (this.purpose == null) 1512 this.purpose = new ArrayList<Coding>(); 1513 this.purpose.add(t); 1514 return t; 1515 } 1516 1517 public ExceptComponent addPurpose(Coding t) { //3 1518 if (t == null) 1519 return this; 1520 if (this.purpose == null) 1521 this.purpose = new ArrayList<Coding>(); 1522 this.purpose.add(t); 1523 return this; 1524 } 1525 1526 /** 1527 * @return The first repetition of repeating field {@link #purpose}, creating it if it does not already exist 1528 */ 1529 public Coding getPurposeFirstRep() { 1530 if (getPurpose().isEmpty()) { 1531 addPurpose(); 1532 } 1533 return getPurpose().get(0); 1534 } 1535 1536 /** 1537 * @return {@link #class_} (The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.) 1538 */ 1539 public List<Coding> getClass_() { 1540 if (this.class_ == null) 1541 this.class_ = new ArrayList<Coding>(); 1542 return this.class_; 1543 } 1544 1545 /** 1546 * @return Returns a reference to <code>this</code> for easy method chaining 1547 */ 1548 public ExceptComponent setClass_(List<Coding> theClass_) { 1549 this.class_ = theClass_; 1550 return this; 1551 } 1552 1553 public boolean hasClass_() { 1554 if (this.class_ == null) 1555 return false; 1556 for (Coding item : this.class_) 1557 if (!item.isEmpty()) 1558 return true; 1559 return false; 1560 } 1561 1562 public Coding addClass_() { //3 1563 Coding t = new Coding(); 1564 if (this.class_ == null) 1565 this.class_ = new ArrayList<Coding>(); 1566 this.class_.add(t); 1567 return t; 1568 } 1569 1570 public ExceptComponent addClass_(Coding t) { //3 1571 if (t == null) 1572 return this; 1573 if (this.class_ == null) 1574 this.class_ = new ArrayList<Coding>(); 1575 this.class_.add(t); 1576 return this; 1577 } 1578 1579 /** 1580 * @return The first repetition of repeating field {@link #class_}, creating it if it does not already exist 1581 */ 1582 public Coding getClass_FirstRep() { 1583 if (getClass_().isEmpty()) { 1584 addClass_(); 1585 } 1586 return getClass_().get(0); 1587 } 1588 1589 /** 1590 * @return {@link #code} (If this code is found in an instance, then the exception applies.) 1591 */ 1592 public List<Coding> getCode() { 1593 if (this.code == null) 1594 this.code = new ArrayList<Coding>(); 1595 return this.code; 1596 } 1597 1598 /** 1599 * @return Returns a reference to <code>this</code> for easy method chaining 1600 */ 1601 public ExceptComponent setCode(List<Coding> theCode) { 1602 this.code = theCode; 1603 return this; 1604 } 1605 1606 public boolean hasCode() { 1607 if (this.code == null) 1608 return false; 1609 for (Coding item : this.code) 1610 if (!item.isEmpty()) 1611 return true; 1612 return false; 1613 } 1614 1615 public Coding addCode() { //3 1616 Coding t = new Coding(); 1617 if (this.code == null) 1618 this.code = new ArrayList<Coding>(); 1619 this.code.add(t); 1620 return t; 1621 } 1622 1623 public ExceptComponent addCode(Coding t) { //3 1624 if (t == null) 1625 return this; 1626 if (this.code == null) 1627 this.code = new ArrayList<Coding>(); 1628 this.code.add(t); 1629 return this; 1630 } 1631 1632 /** 1633 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 1634 */ 1635 public Coding getCodeFirstRep() { 1636 if (getCode().isEmpty()) { 1637 addCode(); 1638 } 1639 return getCode().get(0); 1640 } 1641 1642 /** 1643 * @return {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this exception.) 1644 */ 1645 public Period getDataPeriod() { 1646 if (this.dataPeriod == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create ExceptComponent.dataPeriod"); 1649 else if (Configuration.doAutoCreate()) 1650 this.dataPeriod = new Period(); // cc 1651 return this.dataPeriod; 1652 } 1653 1654 public boolean hasDataPeriod() { 1655 return this.dataPeriod != null && !this.dataPeriod.isEmpty(); 1656 } 1657 1658 /** 1659 * @param value {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this exception.) 1660 */ 1661 public ExceptComponent setDataPeriod(Period value) { 1662 this.dataPeriod = value; 1663 return this; 1664 } 1665 1666 /** 1667 * @return {@link #data} (The resources controlled by this exception, if specific resources are referenced.) 1668 */ 1669 public List<ExceptDataComponent> getData() { 1670 if (this.data == null) 1671 this.data = new ArrayList<ExceptDataComponent>(); 1672 return this.data; 1673 } 1674 1675 /** 1676 * @return Returns a reference to <code>this</code> for easy method chaining 1677 */ 1678 public ExceptComponent setData(List<ExceptDataComponent> theData) { 1679 this.data = theData; 1680 return this; 1681 } 1682 1683 public boolean hasData() { 1684 if (this.data == null) 1685 return false; 1686 for (ExceptDataComponent item : this.data) 1687 if (!item.isEmpty()) 1688 return true; 1689 return false; 1690 } 1691 1692 public ExceptDataComponent addData() { //3 1693 ExceptDataComponent t = new ExceptDataComponent(); 1694 if (this.data == null) 1695 this.data = new ArrayList<ExceptDataComponent>(); 1696 this.data.add(t); 1697 return t; 1698 } 1699 1700 public ExceptComponent addData(ExceptDataComponent t) { //3 1701 if (t == null) 1702 return this; 1703 if (this.data == null) 1704 this.data = new ArrayList<ExceptDataComponent>(); 1705 this.data.add(t); 1706 return this; 1707 } 1708 1709 /** 1710 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist 1711 */ 1712 public ExceptDataComponent getDataFirstRep() { 1713 if (getData().isEmpty()) { 1714 addData(); 1715 } 1716 return getData().get(0); 1717 } 1718 1719 protected void listChildren(List<Property> children) { 1720 super.listChildren(children); 1721 children.add(new Property("type", "code", "Action to take - permit or deny - when the exception conditions are met.", 0, 1, type)); 1722 children.add(new Property("period", "Period", "The timeframe in this exception is valid.", 0, 1, period)); 1723 children.add(new Property("actor", "", "Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor)); 1724 children.add(new Property("action", "CodeableConcept", "Actions controlled by this Exception.", 0, java.lang.Integer.MAX_VALUE, action)); 1725 children.add(new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 1726 children.add(new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, purpose)); 1727 children.add(new Property("class", "Coding", "The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, class_)); 1728 children.add(new Property("code", "Coding", "If this code is found in an instance, then the exception applies.", 0, java.lang.Integer.MAX_VALUE, code)); 1729 children.add(new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this exception.", 0, 1, dataPeriod)); 1730 children.add(new Property("data", "", "The resources controlled by this exception, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data)); 1731 } 1732 1733 @Override 1734 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1735 switch (_hash) { 1736 case 3575610: /*type*/ return new Property("type", "code", "Action to take - permit or deny - when the exception conditions are met.", 0, 1, type); 1737 case -991726143: /*period*/ return new Property("period", "Period", "The timeframe in this exception is valid.", 0, 1, period); 1738 case 92645877: /*actor*/ return new Property("actor", "", "Who or what is controlled by this Exception. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor); 1739 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Actions controlled by this Exception.", 0, java.lang.Integer.MAX_VALUE, action); 1740 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this exception. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 1741 case -220463842: /*purpose*/ return new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, purpose); 1742 case 94742904: /*class*/ return new Property("class", "Coding", "The class of information covered by this exception. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, class_); 1743 case 3059181: /*code*/ return new Property("code", "Coding", "If this code is found in an instance, then the exception applies.", 0, java.lang.Integer.MAX_VALUE, code); 1744 case 1177250315: /*dataPeriod*/ return new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this exception.", 0, 1, dataPeriod); 1745 case 3076010: /*data*/ return new Property("data", "", "The resources controlled by this exception, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data); 1746 default: return super.getNamedProperty(_hash, _name, _checkValid); 1747 } 1748 1749 } 1750 1751 @Override 1752 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1753 switch (hash) { 1754 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ConsentExceptType> 1755 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1756 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ExceptActorComponent 1757 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 1758 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 1759 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Coding 1760 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // Coding 1761 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 1762 case 1177250315: /*dataPeriod*/ return this.dataPeriod == null ? new Base[0] : new Base[] {this.dataPeriod}; // Period 1763 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // ExceptDataComponent 1764 default: return super.getProperty(hash, name, checkValid); 1765 } 1766 1767 } 1768 1769 @Override 1770 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1771 switch (hash) { 1772 case 3575610: // type 1773 value = new ConsentExceptTypeEnumFactory().fromType(castToCode(value)); 1774 this.type = (Enumeration) value; // Enumeration<ConsentExceptType> 1775 return value; 1776 case -991726143: // period 1777 this.period = castToPeriod(value); // Period 1778 return value; 1779 case 92645877: // actor 1780 this.getActor().add((ExceptActorComponent) value); // ExceptActorComponent 1781 return value; 1782 case -1422950858: // action 1783 this.getAction().add(castToCodeableConcept(value)); // CodeableConcept 1784 return value; 1785 case -722296940: // securityLabel 1786 this.getSecurityLabel().add(castToCoding(value)); // Coding 1787 return value; 1788 case -220463842: // purpose 1789 this.getPurpose().add(castToCoding(value)); // Coding 1790 return value; 1791 case 94742904: // class 1792 this.getClass_().add(castToCoding(value)); // Coding 1793 return value; 1794 case 3059181: // code 1795 this.getCode().add(castToCoding(value)); // Coding 1796 return value; 1797 case 1177250315: // dataPeriod 1798 this.dataPeriod = castToPeriod(value); // Period 1799 return value; 1800 case 3076010: // data 1801 this.getData().add((ExceptDataComponent) value); // ExceptDataComponent 1802 return value; 1803 default: return super.setProperty(hash, name, value); 1804 } 1805 1806 } 1807 1808 @Override 1809 public Base setProperty(String name, Base value) throws FHIRException { 1810 if (name.equals("type")) { 1811 value = new ConsentExceptTypeEnumFactory().fromType(castToCode(value)); 1812 this.type = (Enumeration) value; // Enumeration<ConsentExceptType> 1813 } else if (name.equals("period")) { 1814 this.period = castToPeriod(value); // Period 1815 } else if (name.equals("actor")) { 1816 this.getActor().add((ExceptActorComponent) value); 1817 } else if (name.equals("action")) { 1818 this.getAction().add(castToCodeableConcept(value)); 1819 } else if (name.equals("securityLabel")) { 1820 this.getSecurityLabel().add(castToCoding(value)); 1821 } else if (name.equals("purpose")) { 1822 this.getPurpose().add(castToCoding(value)); 1823 } else if (name.equals("class")) { 1824 this.getClass_().add(castToCoding(value)); 1825 } else if (name.equals("code")) { 1826 this.getCode().add(castToCoding(value)); 1827 } else if (name.equals("dataPeriod")) { 1828 this.dataPeriod = castToPeriod(value); // Period 1829 } else if (name.equals("data")) { 1830 this.getData().add((ExceptDataComponent) value); 1831 } else 1832 return super.setProperty(name, value); 1833 return value; 1834 } 1835 1836 @Override 1837 public Base makeProperty(int hash, String name) throws FHIRException { 1838 switch (hash) { 1839 case 3575610: return getTypeElement(); 1840 case -991726143: return getPeriod(); 1841 case 92645877: return addActor(); 1842 case -1422950858: return addAction(); 1843 case -722296940: return addSecurityLabel(); 1844 case -220463842: return addPurpose(); 1845 case 94742904: return addClass_(); 1846 case 3059181: return addCode(); 1847 case 1177250315: return getDataPeriod(); 1848 case 3076010: return addData(); 1849 default: return super.makeProperty(hash, name); 1850 } 1851 1852 } 1853 1854 @Override 1855 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1856 switch (hash) { 1857 case 3575610: /*type*/ return new String[] {"code"}; 1858 case -991726143: /*period*/ return new String[] {"Period"}; 1859 case 92645877: /*actor*/ return new String[] {}; 1860 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 1861 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 1862 case -220463842: /*purpose*/ return new String[] {"Coding"}; 1863 case 94742904: /*class*/ return new String[] {"Coding"}; 1864 case 3059181: /*code*/ return new String[] {"Coding"}; 1865 case 1177250315: /*dataPeriod*/ return new String[] {"Period"}; 1866 case 3076010: /*data*/ return new String[] {}; 1867 default: return super.getTypesForProperty(hash, name); 1868 } 1869 1870 } 1871 1872 @Override 1873 public Base addChild(String name) throws FHIRException { 1874 if (name.equals("type")) { 1875 throw new FHIRException("Cannot call addChild on a singleton property Consent.type"); 1876 } 1877 else if (name.equals("period")) { 1878 this.period = new Period(); 1879 return this.period; 1880 } 1881 else if (name.equals("actor")) { 1882 return addActor(); 1883 } 1884 else if (name.equals("action")) { 1885 return addAction(); 1886 } 1887 else if (name.equals("securityLabel")) { 1888 return addSecurityLabel(); 1889 } 1890 else if (name.equals("purpose")) { 1891 return addPurpose(); 1892 } 1893 else if (name.equals("class")) { 1894 return addClass_(); 1895 } 1896 else if (name.equals("code")) { 1897 return addCode(); 1898 } 1899 else if (name.equals("dataPeriod")) { 1900 this.dataPeriod = new Period(); 1901 return this.dataPeriod; 1902 } 1903 else if (name.equals("data")) { 1904 return addData(); 1905 } 1906 else 1907 return super.addChild(name); 1908 } 1909 1910 public ExceptComponent copy() { 1911 ExceptComponent dst = new ExceptComponent(); 1912 copyValues(dst); 1913 dst.type = type == null ? null : type.copy(); 1914 dst.period = period == null ? null : period.copy(); 1915 if (actor != null) { 1916 dst.actor = new ArrayList<ExceptActorComponent>(); 1917 for (ExceptActorComponent i : actor) 1918 dst.actor.add(i.copy()); 1919 }; 1920 if (action != null) { 1921 dst.action = new ArrayList<CodeableConcept>(); 1922 for (CodeableConcept i : action) 1923 dst.action.add(i.copy()); 1924 }; 1925 if (securityLabel != null) { 1926 dst.securityLabel = new ArrayList<Coding>(); 1927 for (Coding i : securityLabel) 1928 dst.securityLabel.add(i.copy()); 1929 }; 1930 if (purpose != null) { 1931 dst.purpose = new ArrayList<Coding>(); 1932 for (Coding i : purpose) 1933 dst.purpose.add(i.copy()); 1934 }; 1935 if (class_ != null) { 1936 dst.class_ = new ArrayList<Coding>(); 1937 for (Coding i : class_) 1938 dst.class_.add(i.copy()); 1939 }; 1940 if (code != null) { 1941 dst.code = new ArrayList<Coding>(); 1942 for (Coding i : code) 1943 dst.code.add(i.copy()); 1944 }; 1945 dst.dataPeriod = dataPeriod == null ? null : dataPeriod.copy(); 1946 if (data != null) { 1947 dst.data = new ArrayList<ExceptDataComponent>(); 1948 for (ExceptDataComponent i : data) 1949 dst.data.add(i.copy()); 1950 }; 1951 return dst; 1952 } 1953 1954 @Override 1955 public boolean equalsDeep(Base other_) { 1956 if (!super.equalsDeep(other_)) 1957 return false; 1958 if (!(other_ instanceof ExceptComponent)) 1959 return false; 1960 ExceptComponent o = (ExceptComponent) other_; 1961 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) && compareDeep(actor, o.actor, true) 1962 && compareDeep(action, o.action, true) && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(purpose, o.purpose, true) 1963 && compareDeep(class_, o.class_, true) && compareDeep(code, o.code, true) && compareDeep(dataPeriod, o.dataPeriod, true) 1964 && compareDeep(data, o.data, true); 1965 } 1966 1967 @Override 1968 public boolean equalsShallow(Base other_) { 1969 if (!super.equalsShallow(other_)) 1970 return false; 1971 if (!(other_ instanceof ExceptComponent)) 1972 return false; 1973 ExceptComponent o = (ExceptComponent) other_; 1974 return compareValues(type, o.type, true); 1975 } 1976 1977 public boolean isEmpty() { 1978 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, actor, action 1979 , securityLabel, purpose, class_, code, dataPeriod, data); 1980 } 1981 1982 public String fhirType() { 1983 return "Consent.except"; 1984 1985 } 1986 1987 } 1988 1989 @Block() 1990 public static class ExceptActorComponent extends BackboneElement implements IBaseBackboneElement { 1991 /** 1992 * How the individual is involved in the resources content that is described in the exception. 1993 */ 1994 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1995 @Description(shortDefinition="How the actor is involved", formalDefinition="How the individual is involved in the resources content that is described in the exception." ) 1996 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 1997 protected CodeableConcept role; 1998 1999 /** 2000 * The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 2001 */ 2002 @Child(name = "reference", type = {Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 2003 @Description(shortDefinition="Resource for the actor (or group, by role)", formalDefinition="The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 2004 protected Reference reference; 2005 2006 /** 2007 * The actual object that is the target of the reference (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2008 */ 2009 protected Resource referenceTarget; 2010 2011 private static final long serialVersionUID = 1152919415L; 2012 2013 /** 2014 * Constructor 2015 */ 2016 public ExceptActorComponent() { 2017 super(); 2018 } 2019 2020 /** 2021 * Constructor 2022 */ 2023 public ExceptActorComponent(CodeableConcept role, Reference reference) { 2024 super(); 2025 this.role = role; 2026 this.reference = reference; 2027 } 2028 2029 /** 2030 * @return {@link #role} (How the individual is involved in the resources content that is described in the exception.) 2031 */ 2032 public CodeableConcept getRole() { 2033 if (this.role == null) 2034 if (Configuration.errorOnAutoCreate()) 2035 throw new Error("Attempt to auto-create ExceptActorComponent.role"); 2036 else if (Configuration.doAutoCreate()) 2037 this.role = new CodeableConcept(); // cc 2038 return this.role; 2039 } 2040 2041 public boolean hasRole() { 2042 return this.role != null && !this.role.isEmpty(); 2043 } 2044 2045 /** 2046 * @param value {@link #role} (How the individual is involved in the resources content that is described in the exception.) 2047 */ 2048 public ExceptActorComponent setRole(CodeableConcept value) { 2049 this.role = value; 2050 return this; 2051 } 2052 2053 /** 2054 * @return {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2055 */ 2056 public Reference getReference() { 2057 if (this.reference == null) 2058 if (Configuration.errorOnAutoCreate()) 2059 throw new Error("Attempt to auto-create ExceptActorComponent.reference"); 2060 else if (Configuration.doAutoCreate()) 2061 this.reference = new Reference(); // cc 2062 return this.reference; 2063 } 2064 2065 public boolean hasReference() { 2066 return this.reference != null && !this.reference.isEmpty(); 2067 } 2068 2069 /** 2070 * @param value {@link #reference} (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2071 */ 2072 public ExceptActorComponent setReference(Reference value) { 2073 this.reference = value; 2074 return this; 2075 } 2076 2077 /** 2078 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2079 */ 2080 public Resource getReferenceTarget() { 2081 return this.referenceTarget; 2082 } 2083 2084 /** 2085 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2086 */ 2087 public ExceptActorComponent setReferenceTarget(Resource value) { 2088 this.referenceTarget = value; 2089 return this; 2090 } 2091 2092 protected void listChildren(List<Property> children) { 2093 super.listChildren(children); 2094 children.add(new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role)); 2095 children.add(new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference)); 2096 } 2097 2098 @Override 2099 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2100 switch (_hash) { 2101 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role); 2102 case -925155509: /*reference*/ return new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson)", "The resource that identifies the actor. To identify a actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference); 2103 default: return super.getNamedProperty(_hash, _name, _checkValid); 2104 } 2105 2106 } 2107 2108 @Override 2109 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2110 switch (hash) { 2111 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 2112 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2113 default: return super.getProperty(hash, name, checkValid); 2114 } 2115 2116 } 2117 2118 @Override 2119 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2120 switch (hash) { 2121 case 3506294: // role 2122 this.role = castToCodeableConcept(value); // CodeableConcept 2123 return value; 2124 case -925155509: // reference 2125 this.reference = castToReference(value); // Reference 2126 return value; 2127 default: return super.setProperty(hash, name, value); 2128 } 2129 2130 } 2131 2132 @Override 2133 public Base setProperty(String name, Base value) throws FHIRException { 2134 if (name.equals("role")) { 2135 this.role = castToCodeableConcept(value); // CodeableConcept 2136 } else if (name.equals("reference")) { 2137 this.reference = castToReference(value); // Reference 2138 } else 2139 return super.setProperty(name, value); 2140 return value; 2141 } 2142 2143 @Override 2144 public Base makeProperty(int hash, String name) throws FHIRException { 2145 switch (hash) { 2146 case 3506294: return getRole(); 2147 case -925155509: return getReference(); 2148 default: return super.makeProperty(hash, name); 2149 } 2150 2151 } 2152 2153 @Override 2154 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2155 switch (hash) { 2156 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2157 case -925155509: /*reference*/ return new String[] {"Reference"}; 2158 default: return super.getTypesForProperty(hash, name); 2159 } 2160 2161 } 2162 2163 @Override 2164 public Base addChild(String name) throws FHIRException { 2165 if (name.equals("role")) { 2166 this.role = new CodeableConcept(); 2167 return this.role; 2168 } 2169 else if (name.equals("reference")) { 2170 this.reference = new Reference(); 2171 return this.reference; 2172 } 2173 else 2174 return super.addChild(name); 2175 } 2176 2177 public ExceptActorComponent copy() { 2178 ExceptActorComponent dst = new ExceptActorComponent(); 2179 copyValues(dst); 2180 dst.role = role == null ? null : role.copy(); 2181 dst.reference = reference == null ? null : reference.copy(); 2182 return dst; 2183 } 2184 2185 @Override 2186 public boolean equalsDeep(Base other_) { 2187 if (!super.equalsDeep(other_)) 2188 return false; 2189 if (!(other_ instanceof ExceptActorComponent)) 2190 return false; 2191 ExceptActorComponent o = (ExceptActorComponent) other_; 2192 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 2193 } 2194 2195 @Override 2196 public boolean equalsShallow(Base other_) { 2197 if (!super.equalsShallow(other_)) 2198 return false; 2199 if (!(other_ instanceof ExceptActorComponent)) 2200 return false; 2201 ExceptActorComponent o = (ExceptActorComponent) other_; 2202 return true; 2203 } 2204 2205 public boolean isEmpty() { 2206 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 2207 } 2208 2209 public String fhirType() { 2210 return "Consent.except.actor"; 2211 2212 } 2213 2214 } 2215 2216 @Block() 2217 public static class ExceptDataComponent extends BackboneElement implements IBaseBackboneElement { 2218 /** 2219 * How the resource reference is interpreted when testing consent restrictions. 2220 */ 2221 @Child(name = "meaning", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2222 @Description(shortDefinition="instance | related | dependents | authoredby", formalDefinition="How the resource reference is interpreted when testing consent restrictions." ) 2223 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-data-meaning") 2224 protected Enumeration<ConsentDataMeaning> meaning; 2225 2226 /** 2227 * A reference to a specific resource that defines which resources are covered by this consent. 2228 */ 2229 @Child(name = "reference", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 2230 @Description(shortDefinition="The actual data reference", formalDefinition="A reference to a specific resource that defines which resources are covered by this consent." ) 2231 protected Reference reference; 2232 2233 /** 2234 * The actual object that is the target of the reference (A reference to a specific resource that defines which resources are covered by this consent.) 2235 */ 2236 protected Resource referenceTarget; 2237 2238 private static final long serialVersionUID = -424898645L; 2239 2240 /** 2241 * Constructor 2242 */ 2243 public ExceptDataComponent() { 2244 super(); 2245 } 2246 2247 /** 2248 * Constructor 2249 */ 2250 public ExceptDataComponent(Enumeration<ConsentDataMeaning> meaning, Reference reference) { 2251 super(); 2252 this.meaning = meaning; 2253 this.reference = reference; 2254 } 2255 2256 /** 2257 * @return {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2258 */ 2259 public Enumeration<ConsentDataMeaning> getMeaningElement() { 2260 if (this.meaning == null) 2261 if (Configuration.errorOnAutoCreate()) 2262 throw new Error("Attempt to auto-create ExceptDataComponent.meaning"); 2263 else if (Configuration.doAutoCreate()) 2264 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 2265 return this.meaning; 2266 } 2267 2268 public boolean hasMeaningElement() { 2269 return this.meaning != null && !this.meaning.isEmpty(); 2270 } 2271 2272 public boolean hasMeaning() { 2273 return this.meaning != null && !this.meaning.isEmpty(); 2274 } 2275 2276 /** 2277 * @param value {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2278 */ 2279 public ExceptDataComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 2280 this.meaning = value; 2281 return this; 2282 } 2283 2284 /** 2285 * @return How the resource reference is interpreted when testing consent restrictions. 2286 */ 2287 public ConsentDataMeaning getMeaning() { 2288 return this.meaning == null ? null : this.meaning.getValue(); 2289 } 2290 2291 /** 2292 * @param value How the resource reference is interpreted when testing consent restrictions. 2293 */ 2294 public ExceptDataComponent setMeaning(ConsentDataMeaning value) { 2295 if (this.meaning == null) 2296 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 2297 this.meaning.setValue(value); 2298 return this; 2299 } 2300 2301 /** 2302 * @return {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2303 */ 2304 public Reference getReference() { 2305 if (this.reference == null) 2306 if (Configuration.errorOnAutoCreate()) 2307 throw new Error("Attempt to auto-create ExceptDataComponent.reference"); 2308 else if (Configuration.doAutoCreate()) 2309 this.reference = new Reference(); // cc 2310 return this.reference; 2311 } 2312 2313 public boolean hasReference() { 2314 return this.reference != null && !this.reference.isEmpty(); 2315 } 2316 2317 /** 2318 * @param value {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2319 */ 2320 public ExceptDataComponent setReference(Reference value) { 2321 this.reference = value; 2322 return this; 2323 } 2324 2325 /** 2326 * @return {@link #reference} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 2327 */ 2328 public Resource getReferenceTarget() { 2329 return this.referenceTarget; 2330 } 2331 2332 /** 2333 * @param value {@link #reference} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a specific resource that defines which resources are covered by this consent.) 2334 */ 2335 public ExceptDataComponent setReferenceTarget(Resource value) { 2336 this.referenceTarget = value; 2337 return this; 2338 } 2339 2340 protected void listChildren(List<Property> children) { 2341 super.listChildren(children); 2342 children.add(new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 2343 children.add(new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference)); 2344 } 2345 2346 @Override 2347 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2348 switch (_hash) { 2349 case 938160637: /*meaning*/ return new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 2350 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference); 2351 default: return super.getNamedProperty(_hash, _name, _checkValid); 2352 } 2353 2354 } 2355 2356 @Override 2357 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2358 switch (hash) { 2359 case 938160637: /*meaning*/ return this.meaning == null ? new Base[0] : new Base[] {this.meaning}; // Enumeration<ConsentDataMeaning> 2360 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2361 default: return super.getProperty(hash, name, checkValid); 2362 } 2363 2364 } 2365 2366 @Override 2367 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2368 switch (hash) { 2369 case 938160637: // meaning 2370 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 2371 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2372 return value; 2373 case -925155509: // reference 2374 this.reference = castToReference(value); // Reference 2375 return value; 2376 default: return super.setProperty(hash, name, value); 2377 } 2378 2379 } 2380 2381 @Override 2382 public Base setProperty(String name, Base value) throws FHIRException { 2383 if (name.equals("meaning")) { 2384 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 2385 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2386 } else if (name.equals("reference")) { 2387 this.reference = castToReference(value); // Reference 2388 } else 2389 return super.setProperty(name, value); 2390 return value; 2391 } 2392 2393 @Override 2394 public Base makeProperty(int hash, String name) throws FHIRException { 2395 switch (hash) { 2396 case 938160637: return getMeaningElement(); 2397 case -925155509: return getReference(); 2398 default: return super.makeProperty(hash, name); 2399 } 2400 2401 } 2402 2403 @Override 2404 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2405 switch (hash) { 2406 case 938160637: /*meaning*/ return new String[] {"code"}; 2407 case -925155509: /*reference*/ return new String[] {"Reference"}; 2408 default: return super.getTypesForProperty(hash, name); 2409 } 2410 2411 } 2412 2413 @Override 2414 public Base addChild(String name) throws FHIRException { 2415 if (name.equals("meaning")) { 2416 throw new FHIRException("Cannot call addChild on a singleton property Consent.meaning"); 2417 } 2418 else if (name.equals("reference")) { 2419 this.reference = new Reference(); 2420 return this.reference; 2421 } 2422 else 2423 return super.addChild(name); 2424 } 2425 2426 public ExceptDataComponent copy() { 2427 ExceptDataComponent dst = new ExceptDataComponent(); 2428 copyValues(dst); 2429 dst.meaning = meaning == null ? null : meaning.copy(); 2430 dst.reference = reference == null ? null : reference.copy(); 2431 return dst; 2432 } 2433 2434 @Override 2435 public boolean equalsDeep(Base other_) { 2436 if (!super.equalsDeep(other_)) 2437 return false; 2438 if (!(other_ instanceof ExceptDataComponent)) 2439 return false; 2440 ExceptDataComponent o = (ExceptDataComponent) other_; 2441 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 2442 } 2443 2444 @Override 2445 public boolean equalsShallow(Base other_) { 2446 if (!super.equalsShallow(other_)) 2447 return false; 2448 if (!(other_ instanceof ExceptDataComponent)) 2449 return false; 2450 ExceptDataComponent o = (ExceptDataComponent) other_; 2451 return compareValues(meaning, o.meaning, true); 2452 } 2453 2454 public boolean isEmpty() { 2455 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 2456 } 2457 2458 public String fhirType() { 2459 return "Consent.except.data"; 2460 2461 } 2462 2463 } 2464 2465 /** 2466 * Unique identifier for this copy of the Consent Statement. 2467 */ 2468 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2469 @Description(shortDefinition="Identifier for this record (external references)", formalDefinition="Unique identifier for this copy of the Consent Statement." ) 2470 protected Identifier identifier; 2471 2472 /** 2473 * Indicates the current state of this consent. 2474 */ 2475 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2476 @Description(shortDefinition="draft | proposed | active | rejected | inactive | entered-in-error", formalDefinition="Indicates the current state of this consent." ) 2477 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-state-codes") 2478 protected Enumeration<ConsentState> status; 2479 2480 /** 2481 * A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements. 2482 */ 2483 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2484 @Description(shortDefinition="Classification of the consent statement - for indexing/retrieval", formalDefinition="A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements." ) 2485 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-category") 2486 protected List<CodeableConcept> category; 2487 2488 /** 2489 * The patient/healthcare consumer to whom this consent applies. 2490 */ 2491 @Child(name = "patient", type = {Patient.class}, order=3, min=1, max=1, modifier=false, summary=true) 2492 @Description(shortDefinition="Who the consent applies to", formalDefinition="The patient/healthcare consumer to whom this consent applies." ) 2493 protected Reference patient; 2494 2495 /** 2496 * The actual object that is the target of the reference (The patient/healthcare consumer to whom this consent applies.) 2497 */ 2498 protected Patient patientTarget; 2499 2500 /** 2501 * Relevant time or time-period when this Consent is applicable. 2502 */ 2503 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 2504 @Description(shortDefinition="Period that this consent applies", formalDefinition="Relevant time or time-period when this Consent is applicable." ) 2505 protected Period period; 2506 2507 /** 2508 * When this Consent was issued / created / indexed. 2509 */ 2510 @Child(name = "dateTime", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2511 @Description(shortDefinition="When this Consent was created or indexed", formalDefinition="When this Consent was issued / created / indexed." ) 2512 protected DateTimeType dateTime; 2513 2514 /** 2515 * Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions. 2516 */ 2517 @Child(name = "consentingParty", type = {Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2518 @Description(shortDefinition="Who is agreeing to the policy and exceptions", formalDefinition="Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions." ) 2519 protected List<Reference> consentingParty; 2520 /** 2521 * The actual objects that are the target of the reference (Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.) 2522 */ 2523 protected List<Resource> consentingPartyTarget; 2524 2525 2526 /** 2527 * Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 2528 */ 2529 @Child(name = "actor", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2530 @Description(shortDefinition="Who|what controlled by this consent (or group, by role)", formalDefinition="Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 2531 protected List<ConsentActorComponent> actor; 2532 2533 /** 2534 * Actions controlled by this consent. 2535 */ 2536 @Child(name = "action", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2537 @Description(shortDefinition="Actions controlled by this consent", formalDefinition="Actions controlled by this consent." ) 2538 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-action") 2539 protected List<CodeableConcept> action; 2540 2541 /** 2542 * The organization that manages the consent, and the framework within which it is executed. 2543 */ 2544 @Child(name = "organization", type = {Organization.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2545 @Description(shortDefinition="Custodian of the consent", formalDefinition="The organization that manages the consent, and the framework within which it is executed." ) 2546 protected List<Reference> organization; 2547 /** 2548 * The actual objects that are the target of the reference (The organization that manages the consent, and the framework within which it is executed.) 2549 */ 2550 protected List<Organization> organizationTarget; 2551 2552 2553 /** 2554 * The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document. 2555 */ 2556 @Child(name = "source", type = {Attachment.class, Identifier.class, Consent.class, DocumentReference.class, Contract.class, QuestionnaireResponse.class}, order=10, min=0, max=1, modifier=false, summary=true) 2557 @Description(shortDefinition="Source from which this consent is taken", formalDefinition="The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document." ) 2558 protected Type source; 2559 2560 /** 2561 * The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law. 2562 */ 2563 @Child(name = "policy", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2564 @Description(shortDefinition="Policies covered by this consent", formalDefinition="The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law." ) 2565 protected List<ConsentPolicyComponent> policy; 2566 2567 /** 2568 * A referece to the specific computable policy. 2569 */ 2570 @Child(name = "policyRule", type = {UriType.class}, order=12, min=0, max=1, modifier=false, summary=true) 2571 @Description(shortDefinition="Policy that this consents to", formalDefinition="A referece to the specific computable policy." ) 2572 protected UriType policyRule; 2573 2574 /** 2575 * A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels. 2576 */ 2577 @Child(name = "securityLabel", type = {Coding.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2578 @Description(shortDefinition="Security Labels that define affected resources", formalDefinition="A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels." ) 2579 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 2580 protected List<Coding> securityLabel; 2581 2582 /** 2583 * The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent. 2584 */ 2585 @Child(name = "purpose", type = {Coding.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2586 @Description(shortDefinition="Context of activities for which the agreement is made", formalDefinition="The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent." ) 2587 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 2588 protected List<Coding> purpose; 2589 2590 /** 2591 * Clinical or Operational Relevant period of time that bounds the data controlled by this consent. 2592 */ 2593 @Child(name = "dataPeriod", type = {Period.class}, order=15, min=0, max=1, modifier=false, summary=true) 2594 @Description(shortDefinition="Timeframe for data controlled by this consent", formalDefinition="Clinical or Operational Relevant period of time that bounds the data controlled by this consent." ) 2595 protected Period dataPeriod; 2596 2597 /** 2598 * The resources controlled by this consent, if specific resources are referenced. 2599 */ 2600 @Child(name = "data", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2601 @Description(shortDefinition="Data controlled by this consent", formalDefinition="The resources controlled by this consent, if specific resources are referenced." ) 2602 protected List<ConsentDataComponent> data; 2603 2604 /** 2605 * An exception to the base policy of this consent. An exception can be an addition or removal of access permissions. 2606 */ 2607 @Child(name = "except", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2608 @Description(shortDefinition="Additional rule - addition or removal of permissions", formalDefinition="An exception to the base policy of this consent. An exception can be an addition or removal of access permissions." ) 2609 protected List<ExceptComponent> except; 2610 2611 private static final long serialVersionUID = -345946739L; 2612 2613 /** 2614 * Constructor 2615 */ 2616 public Consent() { 2617 super(); 2618 } 2619 2620 /** 2621 * Constructor 2622 */ 2623 public Consent(Enumeration<ConsentState> status, Reference patient) { 2624 super(); 2625 this.status = status; 2626 this.patient = patient; 2627 } 2628 2629 /** 2630 * @return {@link #identifier} (Unique identifier for this copy of the Consent Statement.) 2631 */ 2632 public Identifier getIdentifier() { 2633 if (this.identifier == null) 2634 if (Configuration.errorOnAutoCreate()) 2635 throw new Error("Attempt to auto-create Consent.identifier"); 2636 else if (Configuration.doAutoCreate()) 2637 this.identifier = new Identifier(); // cc 2638 return this.identifier; 2639 } 2640 2641 public boolean hasIdentifier() { 2642 return this.identifier != null && !this.identifier.isEmpty(); 2643 } 2644 2645 /** 2646 * @param value {@link #identifier} (Unique identifier for this copy of the Consent Statement.) 2647 */ 2648 public Consent setIdentifier(Identifier value) { 2649 this.identifier = value; 2650 return this; 2651 } 2652 2653 /** 2654 * @return {@link #status} (Indicates the current state of this consent.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2655 */ 2656 public Enumeration<ConsentState> getStatusElement() { 2657 if (this.status == null) 2658 if (Configuration.errorOnAutoCreate()) 2659 throw new Error("Attempt to auto-create Consent.status"); 2660 else if (Configuration.doAutoCreate()) 2661 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); // bb 2662 return this.status; 2663 } 2664 2665 public boolean hasStatusElement() { 2666 return this.status != null && !this.status.isEmpty(); 2667 } 2668 2669 public boolean hasStatus() { 2670 return this.status != null && !this.status.isEmpty(); 2671 } 2672 2673 /** 2674 * @param value {@link #status} (Indicates the current state of this consent.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2675 */ 2676 public Consent setStatusElement(Enumeration<ConsentState> value) { 2677 this.status = value; 2678 return this; 2679 } 2680 2681 /** 2682 * @return Indicates the current state of this consent. 2683 */ 2684 public ConsentState getStatus() { 2685 return this.status == null ? null : this.status.getValue(); 2686 } 2687 2688 /** 2689 * @param value Indicates the current state of this consent. 2690 */ 2691 public Consent setStatus(ConsentState value) { 2692 if (this.status == null) 2693 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); 2694 this.status.setValue(value); 2695 return this; 2696 } 2697 2698 /** 2699 * @return {@link #category} (A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.) 2700 */ 2701 public List<CodeableConcept> getCategory() { 2702 if (this.category == null) 2703 this.category = new ArrayList<CodeableConcept>(); 2704 return this.category; 2705 } 2706 2707 /** 2708 * @return Returns a reference to <code>this</code> for easy method chaining 2709 */ 2710 public Consent setCategory(List<CodeableConcept> theCategory) { 2711 this.category = theCategory; 2712 return this; 2713 } 2714 2715 public boolean hasCategory() { 2716 if (this.category == null) 2717 return false; 2718 for (CodeableConcept item : this.category) 2719 if (!item.isEmpty()) 2720 return true; 2721 return false; 2722 } 2723 2724 public CodeableConcept addCategory() { //3 2725 CodeableConcept t = new CodeableConcept(); 2726 if (this.category == null) 2727 this.category = new ArrayList<CodeableConcept>(); 2728 this.category.add(t); 2729 return t; 2730 } 2731 2732 public Consent addCategory(CodeableConcept t) { //3 2733 if (t == null) 2734 return this; 2735 if (this.category == null) 2736 this.category = new ArrayList<CodeableConcept>(); 2737 this.category.add(t); 2738 return this; 2739 } 2740 2741 /** 2742 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 2743 */ 2744 public CodeableConcept getCategoryFirstRep() { 2745 if (getCategory().isEmpty()) { 2746 addCategory(); 2747 } 2748 return getCategory().get(0); 2749 } 2750 2751 /** 2752 * @return {@link #patient} (The patient/healthcare consumer to whom this consent applies.) 2753 */ 2754 public Reference getPatient() { 2755 if (this.patient == null) 2756 if (Configuration.errorOnAutoCreate()) 2757 throw new Error("Attempt to auto-create Consent.patient"); 2758 else if (Configuration.doAutoCreate()) 2759 this.patient = new Reference(); // cc 2760 return this.patient; 2761 } 2762 2763 public boolean hasPatient() { 2764 return this.patient != null && !this.patient.isEmpty(); 2765 } 2766 2767 /** 2768 * @param value {@link #patient} (The patient/healthcare consumer to whom this consent applies.) 2769 */ 2770 public Consent setPatient(Reference value) { 2771 this.patient = value; 2772 return this; 2773 } 2774 2775 /** 2776 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient/healthcare consumer to whom this consent applies.) 2777 */ 2778 public Patient getPatientTarget() { 2779 if (this.patientTarget == null) 2780 if (Configuration.errorOnAutoCreate()) 2781 throw new Error("Attempt to auto-create Consent.patient"); 2782 else if (Configuration.doAutoCreate()) 2783 this.patientTarget = new Patient(); // aa 2784 return this.patientTarget; 2785 } 2786 2787 /** 2788 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient/healthcare consumer to whom this consent applies.) 2789 */ 2790 public Consent setPatientTarget(Patient value) { 2791 this.patientTarget = value; 2792 return this; 2793 } 2794 2795 /** 2796 * @return {@link #period} (Relevant time or time-period when this Consent is applicable.) 2797 */ 2798 public Period getPeriod() { 2799 if (this.period == null) 2800 if (Configuration.errorOnAutoCreate()) 2801 throw new Error("Attempt to auto-create Consent.period"); 2802 else if (Configuration.doAutoCreate()) 2803 this.period = new Period(); // cc 2804 return this.period; 2805 } 2806 2807 public boolean hasPeriod() { 2808 return this.period != null && !this.period.isEmpty(); 2809 } 2810 2811 /** 2812 * @param value {@link #period} (Relevant time or time-period when this Consent is applicable.) 2813 */ 2814 public Consent setPeriod(Period value) { 2815 this.period = value; 2816 return this; 2817 } 2818 2819 /** 2820 * @return {@link #dateTime} (When this Consent was issued / created / indexed.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 2821 */ 2822 public DateTimeType getDateTimeElement() { 2823 if (this.dateTime == null) 2824 if (Configuration.errorOnAutoCreate()) 2825 throw new Error("Attempt to auto-create Consent.dateTime"); 2826 else if (Configuration.doAutoCreate()) 2827 this.dateTime = new DateTimeType(); // bb 2828 return this.dateTime; 2829 } 2830 2831 public boolean hasDateTimeElement() { 2832 return this.dateTime != null && !this.dateTime.isEmpty(); 2833 } 2834 2835 public boolean hasDateTime() { 2836 return this.dateTime != null && !this.dateTime.isEmpty(); 2837 } 2838 2839 /** 2840 * @param value {@link #dateTime} (When this Consent was issued / created / indexed.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 2841 */ 2842 public Consent setDateTimeElement(DateTimeType value) { 2843 this.dateTime = value; 2844 return this; 2845 } 2846 2847 /** 2848 * @return When this Consent was issued / created / indexed. 2849 */ 2850 public Date getDateTime() { 2851 return this.dateTime == null ? null : this.dateTime.getValue(); 2852 } 2853 2854 /** 2855 * @param value When this Consent was issued / created / indexed. 2856 */ 2857 public Consent setDateTime(Date value) { 2858 if (value == null) 2859 this.dateTime = null; 2860 else { 2861 if (this.dateTime == null) 2862 this.dateTime = new DateTimeType(); 2863 this.dateTime.setValue(value); 2864 } 2865 return this; 2866 } 2867 2868 /** 2869 * @return {@link #consentingParty} (Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.) 2870 */ 2871 public List<Reference> getConsentingParty() { 2872 if (this.consentingParty == null) 2873 this.consentingParty = new ArrayList<Reference>(); 2874 return this.consentingParty; 2875 } 2876 2877 /** 2878 * @return Returns a reference to <code>this</code> for easy method chaining 2879 */ 2880 public Consent setConsentingParty(List<Reference> theConsentingParty) { 2881 this.consentingParty = theConsentingParty; 2882 return this; 2883 } 2884 2885 public boolean hasConsentingParty() { 2886 if (this.consentingParty == null) 2887 return false; 2888 for (Reference item : this.consentingParty) 2889 if (!item.isEmpty()) 2890 return true; 2891 return false; 2892 } 2893 2894 public Reference addConsentingParty() { //3 2895 Reference t = new Reference(); 2896 if (this.consentingParty == null) 2897 this.consentingParty = new ArrayList<Reference>(); 2898 this.consentingParty.add(t); 2899 return t; 2900 } 2901 2902 public Consent addConsentingParty(Reference t) { //3 2903 if (t == null) 2904 return this; 2905 if (this.consentingParty == null) 2906 this.consentingParty = new ArrayList<Reference>(); 2907 this.consentingParty.add(t); 2908 return this; 2909 } 2910 2911 /** 2912 * @return The first repetition of repeating field {@link #consentingParty}, creating it if it does not already exist 2913 */ 2914 public Reference getConsentingPartyFirstRep() { 2915 if (getConsentingParty().isEmpty()) { 2916 addConsentingParty(); 2917 } 2918 return getConsentingParty().get(0); 2919 } 2920 2921 /** 2922 * @deprecated Use Reference#setResource(IBaseResource) instead 2923 */ 2924 @Deprecated 2925 public List<Resource> getConsentingPartyTarget() { 2926 if (this.consentingPartyTarget == null) 2927 this.consentingPartyTarget = new ArrayList<Resource>(); 2928 return this.consentingPartyTarget; 2929 } 2930 2931 /** 2932 * @return {@link #actor} (Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 2933 */ 2934 public List<ConsentActorComponent> getActor() { 2935 if (this.actor == null) 2936 this.actor = new ArrayList<ConsentActorComponent>(); 2937 return this.actor; 2938 } 2939 2940 /** 2941 * @return Returns a reference to <code>this</code> for easy method chaining 2942 */ 2943 public Consent setActor(List<ConsentActorComponent> theActor) { 2944 this.actor = theActor; 2945 return this; 2946 } 2947 2948 public boolean hasActor() { 2949 if (this.actor == null) 2950 return false; 2951 for (ConsentActorComponent item : this.actor) 2952 if (!item.isEmpty()) 2953 return true; 2954 return false; 2955 } 2956 2957 public ConsentActorComponent addActor() { //3 2958 ConsentActorComponent t = new ConsentActorComponent(); 2959 if (this.actor == null) 2960 this.actor = new ArrayList<ConsentActorComponent>(); 2961 this.actor.add(t); 2962 return t; 2963 } 2964 2965 public Consent addActor(ConsentActorComponent t) { //3 2966 if (t == null) 2967 return this; 2968 if (this.actor == null) 2969 this.actor = new ArrayList<ConsentActorComponent>(); 2970 this.actor.add(t); 2971 return this; 2972 } 2973 2974 /** 2975 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist 2976 */ 2977 public ConsentActorComponent getActorFirstRep() { 2978 if (getActor().isEmpty()) { 2979 addActor(); 2980 } 2981 return getActor().get(0); 2982 } 2983 2984 /** 2985 * @return {@link #action} (Actions controlled by this consent.) 2986 */ 2987 public List<CodeableConcept> getAction() { 2988 if (this.action == null) 2989 this.action = new ArrayList<CodeableConcept>(); 2990 return this.action; 2991 } 2992 2993 /** 2994 * @return Returns a reference to <code>this</code> for easy method chaining 2995 */ 2996 public Consent setAction(List<CodeableConcept> theAction) { 2997 this.action = theAction; 2998 return this; 2999 } 3000 3001 public boolean hasAction() { 3002 if (this.action == null) 3003 return false; 3004 for (CodeableConcept item : this.action) 3005 if (!item.isEmpty()) 3006 return true; 3007 return false; 3008 } 3009 3010 public CodeableConcept addAction() { //3 3011 CodeableConcept t = new CodeableConcept(); 3012 if (this.action == null) 3013 this.action = new ArrayList<CodeableConcept>(); 3014 this.action.add(t); 3015 return t; 3016 } 3017 3018 public Consent addAction(CodeableConcept t) { //3 3019 if (t == null) 3020 return this; 3021 if (this.action == null) 3022 this.action = new ArrayList<CodeableConcept>(); 3023 this.action.add(t); 3024 return this; 3025 } 3026 3027 /** 3028 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 3029 */ 3030 public CodeableConcept getActionFirstRep() { 3031 if (getAction().isEmpty()) { 3032 addAction(); 3033 } 3034 return getAction().get(0); 3035 } 3036 3037 /** 3038 * @return {@link #organization} (The organization that manages the consent, and the framework within which it is executed.) 3039 */ 3040 public List<Reference> getOrganization() { 3041 if (this.organization == null) 3042 this.organization = new ArrayList<Reference>(); 3043 return this.organization; 3044 } 3045 3046 /** 3047 * @return Returns a reference to <code>this</code> for easy method chaining 3048 */ 3049 public Consent setOrganization(List<Reference> theOrganization) { 3050 this.organization = theOrganization; 3051 return this; 3052 } 3053 3054 public boolean hasOrganization() { 3055 if (this.organization == null) 3056 return false; 3057 for (Reference item : this.organization) 3058 if (!item.isEmpty()) 3059 return true; 3060 return false; 3061 } 3062 3063 public Reference addOrganization() { //3 3064 Reference t = new Reference(); 3065 if (this.organization == null) 3066 this.organization = new ArrayList<Reference>(); 3067 this.organization.add(t); 3068 return t; 3069 } 3070 3071 public Consent addOrganization(Reference t) { //3 3072 if (t == null) 3073 return this; 3074 if (this.organization == null) 3075 this.organization = new ArrayList<Reference>(); 3076 this.organization.add(t); 3077 return this; 3078 } 3079 3080 /** 3081 * @return The first repetition of repeating field {@link #organization}, creating it if it does not already exist 3082 */ 3083 public Reference getOrganizationFirstRep() { 3084 if (getOrganization().isEmpty()) { 3085 addOrganization(); 3086 } 3087 return getOrganization().get(0); 3088 } 3089 3090 /** 3091 * @deprecated Use Reference#setResource(IBaseResource) instead 3092 */ 3093 @Deprecated 3094 public List<Organization> getOrganizationTarget() { 3095 if (this.organizationTarget == null) 3096 this.organizationTarget = new ArrayList<Organization>(); 3097 return this.organizationTarget; 3098 } 3099 3100 /** 3101 * @deprecated Use Reference#setResource(IBaseResource) instead 3102 */ 3103 @Deprecated 3104 public Organization addOrganizationTarget() { 3105 Organization r = new Organization(); 3106 if (this.organizationTarget == null) 3107 this.organizationTarget = new ArrayList<Organization>(); 3108 this.organizationTarget.add(r); 3109 return r; 3110 } 3111 3112 /** 3113 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3114 */ 3115 public Type getSource() { 3116 return this.source; 3117 } 3118 3119 /** 3120 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3121 */ 3122 public Attachment getSourceAttachment() throws FHIRException { 3123 if (this.source == null) 3124 return null; 3125 if (!(this.source instanceof Attachment)) 3126 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.source.getClass().getName()+" was encountered"); 3127 return (Attachment) this.source; 3128 } 3129 3130 public boolean hasSourceAttachment() { 3131 return this != null && this.source instanceof Attachment; 3132 } 3133 3134 /** 3135 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3136 */ 3137 public Identifier getSourceIdentifier() throws FHIRException { 3138 if (this.source == null) 3139 return null; 3140 if (!(this.source instanceof Identifier)) 3141 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.source.getClass().getName()+" was encountered"); 3142 return (Identifier) this.source; 3143 } 3144 3145 public boolean hasSourceIdentifier() { 3146 return this != null && this.source instanceof Identifier; 3147 } 3148 3149 /** 3150 * @return {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3151 */ 3152 public Reference getSourceReference() throws FHIRException { 3153 if (this.source == null) 3154 return null; 3155 if (!(this.source instanceof Reference)) 3156 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.source.getClass().getName()+" was encountered"); 3157 return (Reference) this.source; 3158 } 3159 3160 public boolean hasSourceReference() { 3161 return this != null && this.source instanceof Reference; 3162 } 3163 3164 public boolean hasSource() { 3165 return this.source != null && !this.source.isEmpty(); 3166 } 3167 3168 /** 3169 * @param value {@link #source} (The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 3170 */ 3171 public Consent setSource(Type value) throws FHIRFormatError { 3172 if (value != null && !(value instanceof Attachment || value instanceof Identifier || value instanceof Reference)) 3173 throw new FHIRFormatError("Not the right type for Consent.source[x]: "+value.fhirType()); 3174 this.source = value; 3175 return this; 3176 } 3177 3178 /** 3179 * @return {@link #policy} (The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.) 3180 */ 3181 public List<ConsentPolicyComponent> getPolicy() { 3182 if (this.policy == null) 3183 this.policy = new ArrayList<ConsentPolicyComponent>(); 3184 return this.policy; 3185 } 3186 3187 /** 3188 * @return Returns a reference to <code>this</code> for easy method chaining 3189 */ 3190 public Consent setPolicy(List<ConsentPolicyComponent> thePolicy) { 3191 this.policy = thePolicy; 3192 return this; 3193 } 3194 3195 public boolean hasPolicy() { 3196 if (this.policy == null) 3197 return false; 3198 for (ConsentPolicyComponent item : this.policy) 3199 if (!item.isEmpty()) 3200 return true; 3201 return false; 3202 } 3203 3204 public ConsentPolicyComponent addPolicy() { //3 3205 ConsentPolicyComponent t = new ConsentPolicyComponent(); 3206 if (this.policy == null) 3207 this.policy = new ArrayList<ConsentPolicyComponent>(); 3208 this.policy.add(t); 3209 return t; 3210 } 3211 3212 public Consent addPolicy(ConsentPolicyComponent t) { //3 3213 if (t == null) 3214 return this; 3215 if (this.policy == null) 3216 this.policy = new ArrayList<ConsentPolicyComponent>(); 3217 this.policy.add(t); 3218 return this; 3219 } 3220 3221 /** 3222 * @return The first repetition of repeating field {@link #policy}, creating it if it does not already exist 3223 */ 3224 public ConsentPolicyComponent getPolicyFirstRep() { 3225 if (getPolicy().isEmpty()) { 3226 addPolicy(); 3227 } 3228 return getPolicy().get(0); 3229 } 3230 3231 /** 3232 * @return {@link #policyRule} (A referece to the specific computable policy.). This is the underlying object with id, value and extensions. The accessor "getPolicyRule" gives direct access to the value 3233 */ 3234 public UriType getPolicyRuleElement() { 3235 if (this.policyRule == null) 3236 if (Configuration.errorOnAutoCreate()) 3237 throw new Error("Attempt to auto-create Consent.policyRule"); 3238 else if (Configuration.doAutoCreate()) 3239 this.policyRule = new UriType(); // bb 3240 return this.policyRule; 3241 } 3242 3243 public boolean hasPolicyRuleElement() { 3244 return this.policyRule != null && !this.policyRule.isEmpty(); 3245 } 3246 3247 public boolean hasPolicyRule() { 3248 return this.policyRule != null && !this.policyRule.isEmpty(); 3249 } 3250 3251 /** 3252 * @param value {@link #policyRule} (A referece to the specific computable policy.). This is the underlying object with id, value and extensions. The accessor "getPolicyRule" gives direct access to the value 3253 */ 3254 public Consent setPolicyRuleElement(UriType value) { 3255 this.policyRule = value; 3256 return this; 3257 } 3258 3259 /** 3260 * @return A referece to the specific computable policy. 3261 */ 3262 public String getPolicyRule() { 3263 return this.policyRule == null ? null : this.policyRule.getValue(); 3264 } 3265 3266 /** 3267 * @param value A referece to the specific computable policy. 3268 */ 3269 public Consent setPolicyRule(String value) { 3270 if (Utilities.noString(value)) 3271 this.policyRule = null; 3272 else { 3273 if (this.policyRule == null) 3274 this.policyRule = new UriType(); 3275 this.policyRule.setValue(value); 3276 } 3277 return this; 3278 } 3279 3280 /** 3281 * @return {@link #securityLabel} (A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.) 3282 */ 3283 public List<Coding> getSecurityLabel() { 3284 if (this.securityLabel == null) 3285 this.securityLabel = new ArrayList<Coding>(); 3286 return this.securityLabel; 3287 } 3288 3289 /** 3290 * @return Returns a reference to <code>this</code> for easy method chaining 3291 */ 3292 public Consent setSecurityLabel(List<Coding> theSecurityLabel) { 3293 this.securityLabel = theSecurityLabel; 3294 return this; 3295 } 3296 3297 public boolean hasSecurityLabel() { 3298 if (this.securityLabel == null) 3299 return false; 3300 for (Coding item : this.securityLabel) 3301 if (!item.isEmpty()) 3302 return true; 3303 return false; 3304 } 3305 3306 public Coding addSecurityLabel() { //3 3307 Coding t = new Coding(); 3308 if (this.securityLabel == null) 3309 this.securityLabel = new ArrayList<Coding>(); 3310 this.securityLabel.add(t); 3311 return t; 3312 } 3313 3314 public Consent addSecurityLabel(Coding t) { //3 3315 if (t == null) 3316 return this; 3317 if (this.securityLabel == null) 3318 this.securityLabel = new ArrayList<Coding>(); 3319 this.securityLabel.add(t); 3320 return this; 3321 } 3322 3323 /** 3324 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 3325 */ 3326 public Coding getSecurityLabelFirstRep() { 3327 if (getSecurityLabel().isEmpty()) { 3328 addSecurityLabel(); 3329 } 3330 return getSecurityLabel().get(0); 3331 } 3332 3333 /** 3334 * @return {@link #purpose} (The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent.) 3335 */ 3336 public List<Coding> getPurpose() { 3337 if (this.purpose == null) 3338 this.purpose = new ArrayList<Coding>(); 3339 return this.purpose; 3340 } 3341 3342 /** 3343 * @return Returns a reference to <code>this</code> for easy method chaining 3344 */ 3345 public Consent setPurpose(List<Coding> thePurpose) { 3346 this.purpose = thePurpose; 3347 return this; 3348 } 3349 3350 public boolean hasPurpose() { 3351 if (this.purpose == null) 3352 return false; 3353 for (Coding item : this.purpose) 3354 if (!item.isEmpty()) 3355 return true; 3356 return false; 3357 } 3358 3359 public Coding addPurpose() { //3 3360 Coding t = new Coding(); 3361 if (this.purpose == null) 3362 this.purpose = new ArrayList<Coding>(); 3363 this.purpose.add(t); 3364 return t; 3365 } 3366 3367 public Consent addPurpose(Coding t) { //3 3368 if (t == null) 3369 return this; 3370 if (this.purpose == null) 3371 this.purpose = new ArrayList<Coding>(); 3372 this.purpose.add(t); 3373 return this; 3374 } 3375 3376 /** 3377 * @return The first repetition of repeating field {@link #purpose}, creating it if it does not already exist 3378 */ 3379 public Coding getPurposeFirstRep() { 3380 if (getPurpose().isEmpty()) { 3381 addPurpose(); 3382 } 3383 return getPurpose().get(0); 3384 } 3385 3386 /** 3387 * @return {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this consent.) 3388 */ 3389 public Period getDataPeriod() { 3390 if (this.dataPeriod == null) 3391 if (Configuration.errorOnAutoCreate()) 3392 throw new Error("Attempt to auto-create Consent.dataPeriod"); 3393 else if (Configuration.doAutoCreate()) 3394 this.dataPeriod = new Period(); // cc 3395 return this.dataPeriod; 3396 } 3397 3398 public boolean hasDataPeriod() { 3399 return this.dataPeriod != null && !this.dataPeriod.isEmpty(); 3400 } 3401 3402 /** 3403 * @param value {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this consent.) 3404 */ 3405 public Consent setDataPeriod(Period value) { 3406 this.dataPeriod = value; 3407 return this; 3408 } 3409 3410 /** 3411 * @return {@link #data} (The resources controlled by this consent, if specific resources are referenced.) 3412 */ 3413 public List<ConsentDataComponent> getData() { 3414 if (this.data == null) 3415 this.data = new ArrayList<ConsentDataComponent>(); 3416 return this.data; 3417 } 3418 3419 /** 3420 * @return Returns a reference to <code>this</code> for easy method chaining 3421 */ 3422 public Consent setData(List<ConsentDataComponent> theData) { 3423 this.data = theData; 3424 return this; 3425 } 3426 3427 public boolean hasData() { 3428 if (this.data == null) 3429 return false; 3430 for (ConsentDataComponent item : this.data) 3431 if (!item.isEmpty()) 3432 return true; 3433 return false; 3434 } 3435 3436 public ConsentDataComponent addData() { //3 3437 ConsentDataComponent t = new ConsentDataComponent(); 3438 if (this.data == null) 3439 this.data = new ArrayList<ConsentDataComponent>(); 3440 this.data.add(t); 3441 return t; 3442 } 3443 3444 public Consent addData(ConsentDataComponent t) { //3 3445 if (t == null) 3446 return this; 3447 if (this.data == null) 3448 this.data = new ArrayList<ConsentDataComponent>(); 3449 this.data.add(t); 3450 return this; 3451 } 3452 3453 /** 3454 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist 3455 */ 3456 public ConsentDataComponent getDataFirstRep() { 3457 if (getData().isEmpty()) { 3458 addData(); 3459 } 3460 return getData().get(0); 3461 } 3462 3463 /** 3464 * @return {@link #except} (An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.) 3465 */ 3466 public List<ExceptComponent> getExcept() { 3467 if (this.except == null) 3468 this.except = new ArrayList<ExceptComponent>(); 3469 return this.except; 3470 } 3471 3472 /** 3473 * @return Returns a reference to <code>this</code> for easy method chaining 3474 */ 3475 public Consent setExcept(List<ExceptComponent> theExcept) { 3476 this.except = theExcept; 3477 return this; 3478 } 3479 3480 public boolean hasExcept() { 3481 if (this.except == null) 3482 return false; 3483 for (ExceptComponent item : this.except) 3484 if (!item.isEmpty()) 3485 return true; 3486 return false; 3487 } 3488 3489 public ExceptComponent addExcept() { //3 3490 ExceptComponent t = new ExceptComponent(); 3491 if (this.except == null) 3492 this.except = new ArrayList<ExceptComponent>(); 3493 this.except.add(t); 3494 return t; 3495 } 3496 3497 public Consent addExcept(ExceptComponent t) { //3 3498 if (t == null) 3499 return this; 3500 if (this.except == null) 3501 this.except = new ArrayList<ExceptComponent>(); 3502 this.except.add(t); 3503 return this; 3504 } 3505 3506 /** 3507 * @return The first repetition of repeating field {@link #except}, creating it if it does not already exist 3508 */ 3509 public ExceptComponent getExceptFirstRep() { 3510 if (getExcept().isEmpty()) { 3511 addExcept(); 3512 } 3513 return getExcept().get(0); 3514 } 3515 3516 protected void listChildren(List<Property> children) { 3517 super.listChildren(children); 3518 children.add(new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, 1, identifier)); 3519 children.add(new Property("status", "code", "Indicates the current state of this consent.", 0, 1, status)); 3520 children.add(new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category)); 3521 children.add(new Property("patient", "Reference(Patient)", "The patient/healthcare consumer to whom this consent applies.", 0, 1, patient)); 3522 children.add(new Property("period", "Period", "Relevant time or time-period when this Consent is applicable.", 0, 1, period)); 3523 children.add(new Property("dateTime", "dateTime", "When this Consent was issued / created / indexed.", 0, 1, dateTime)); 3524 children.add(new Property("consentingParty", "Reference(Organization|Patient|Practitioner|RelatedPerson)", "Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, consentingParty)); 3525 children.add(new Property("actor", "", "Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor)); 3526 children.add(new Property("action", "CodeableConcept", "Actions controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, action)); 3527 children.add(new Property("organization", "Reference(Organization)", "The organization that manages the consent, and the framework within which it is executed.", 0, java.lang.Integer.MAX_VALUE, organization)); 3528 children.add(new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source)); 3529 children.add(new Property("policy", "", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, java.lang.Integer.MAX_VALUE, policy)); 3530 children.add(new Property("policyRule", "uri", "A referece to the specific computable policy.", 0, 1, policyRule)); 3531 children.add(new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 3532 children.add(new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, purpose)); 3533 children.add(new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this consent.", 0, 1, dataPeriod)); 3534 children.add(new Property("data", "", "The resources controlled by this consent, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data)); 3535 children.add(new Property("except", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, except)); 3536 } 3537 3538 @Override 3539 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3540 switch (_hash) { 3541 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, 1, identifier); 3542 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of this consent.", 0, 1, status); 3543 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category); 3544 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient/healthcare consumer to whom this consent applies.", 0, 1, patient); 3545 case -991726143: /*period*/ return new Property("period", "Period", "Relevant time or time-period when this Consent is applicable.", 0, 1, period); 3546 case 1792749467: /*dateTime*/ return new Property("dateTime", "dateTime", "When this Consent was issued / created / indexed.", 0, 1, dateTime); 3547 case -1886702018: /*consentingParty*/ return new Property("consentingParty", "Reference(Organization|Patient|Practitioner|RelatedPerson)", "Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, consentingParty); 3548 case 92645877: /*actor*/ return new Property("actor", "", "Who or what is controlled by this consent. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor); 3549 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Actions controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, action); 3550 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization that manages the consent, and the framework within which it is executed.", 0, java.lang.Integer.MAX_VALUE, organization); 3551 case -1698413947: /*source[x]*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3552 case -896505829: /*source*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3553 case 1964406686: /*sourceAttachment*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3554 case -1985492188: /*sourceIdentifier*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3555 case -244259472: /*sourceReference*/ return new Property("source[x]", "Attachment|Identifier|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, 1, source); 3556 case -982670030: /*policy*/ return new Property("policy", "", "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 0, java.lang.Integer.MAX_VALUE, policy); 3557 case 1593493326: /*policyRule*/ return new Property("policyRule", "uri", "A referece to the specific computable policy.", 0, 1, policyRule); 3558 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 3559 case -220463842: /*purpose*/ return new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this consent.", 0, java.lang.Integer.MAX_VALUE, purpose); 3560 case 1177250315: /*dataPeriod*/ return new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this consent.", 0, 1, dataPeriod); 3561 case 3076010: /*data*/ return new Property("data", "", "The resources controlled by this consent, if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data); 3562 case -1289550567: /*except*/ return new Property("except", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, except); 3563 default: return super.getNamedProperty(_hash, _name, _checkValid); 3564 } 3565 3566 } 3567 3568 @Override 3569 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3570 switch (hash) { 3571 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3572 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ConsentState> 3573 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3574 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 3575 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3576 case 1792749467: /*dateTime*/ return this.dateTime == null ? new Base[0] : new Base[] {this.dateTime}; // DateTimeType 3577 case -1886702018: /*consentingParty*/ return this.consentingParty == null ? new Base[0] : this.consentingParty.toArray(new Base[this.consentingParty.size()]); // Reference 3578 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ConsentActorComponent 3579 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 3580 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : this.organization.toArray(new Base[this.organization.size()]); // Reference 3581 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Type 3582 case -982670030: /*policy*/ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // ConsentPolicyComponent 3583 case 1593493326: /*policyRule*/ return this.policyRule == null ? new Base[0] : new Base[] {this.policyRule}; // UriType 3584 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 3585 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Coding 3586 case 1177250315: /*dataPeriod*/ return this.dataPeriod == null ? new Base[0] : new Base[] {this.dataPeriod}; // Period 3587 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // ConsentDataComponent 3588 case -1289550567: /*except*/ return this.except == null ? new Base[0] : this.except.toArray(new Base[this.except.size()]); // ExceptComponent 3589 default: return super.getProperty(hash, name, checkValid); 3590 } 3591 3592 } 3593 3594 @Override 3595 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3596 switch (hash) { 3597 case -1618432855: // identifier 3598 this.identifier = castToIdentifier(value); // Identifier 3599 return value; 3600 case -892481550: // status 3601 value = new ConsentStateEnumFactory().fromType(castToCode(value)); 3602 this.status = (Enumeration) value; // Enumeration<ConsentState> 3603 return value; 3604 case 50511102: // category 3605 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3606 return value; 3607 case -791418107: // patient 3608 this.patient = castToReference(value); // Reference 3609 return value; 3610 case -991726143: // period 3611 this.period = castToPeriod(value); // Period 3612 return value; 3613 case 1792749467: // dateTime 3614 this.dateTime = castToDateTime(value); // DateTimeType 3615 return value; 3616 case -1886702018: // consentingParty 3617 this.getConsentingParty().add(castToReference(value)); // Reference 3618 return value; 3619 case 92645877: // actor 3620 this.getActor().add((ConsentActorComponent) value); // ConsentActorComponent 3621 return value; 3622 case -1422950858: // action 3623 this.getAction().add(castToCodeableConcept(value)); // CodeableConcept 3624 return value; 3625 case 1178922291: // organization 3626 this.getOrganization().add(castToReference(value)); // Reference 3627 return value; 3628 case -896505829: // source 3629 this.source = castToType(value); // Type 3630 return value; 3631 case -982670030: // policy 3632 this.getPolicy().add((ConsentPolicyComponent) value); // ConsentPolicyComponent 3633 return value; 3634 case 1593493326: // policyRule 3635 this.policyRule = castToUri(value); // UriType 3636 return value; 3637 case -722296940: // securityLabel 3638 this.getSecurityLabel().add(castToCoding(value)); // Coding 3639 return value; 3640 case -220463842: // purpose 3641 this.getPurpose().add(castToCoding(value)); // Coding 3642 return value; 3643 case 1177250315: // dataPeriod 3644 this.dataPeriod = castToPeriod(value); // Period 3645 return value; 3646 case 3076010: // data 3647 this.getData().add((ConsentDataComponent) value); // ConsentDataComponent 3648 return value; 3649 case -1289550567: // except 3650 this.getExcept().add((ExceptComponent) value); // ExceptComponent 3651 return value; 3652 default: return super.setProperty(hash, name, value); 3653 } 3654 3655 } 3656 3657 @Override 3658 public Base setProperty(String name, Base value) throws FHIRException { 3659 if (name.equals("identifier")) { 3660 this.identifier = castToIdentifier(value); // Identifier 3661 } else if (name.equals("status")) { 3662 value = new ConsentStateEnumFactory().fromType(castToCode(value)); 3663 this.status = (Enumeration) value; // Enumeration<ConsentState> 3664 } else if (name.equals("category")) { 3665 this.getCategory().add(castToCodeableConcept(value)); 3666 } else if (name.equals("patient")) { 3667 this.patient = castToReference(value); // Reference 3668 } else if (name.equals("period")) { 3669 this.period = castToPeriod(value); // Period 3670 } else if (name.equals("dateTime")) { 3671 this.dateTime = castToDateTime(value); // DateTimeType 3672 } else if (name.equals("consentingParty")) { 3673 this.getConsentingParty().add(castToReference(value)); 3674 } else if (name.equals("actor")) { 3675 this.getActor().add((ConsentActorComponent) value); 3676 } else if (name.equals("action")) { 3677 this.getAction().add(castToCodeableConcept(value)); 3678 } else if (name.equals("organization")) { 3679 this.getOrganization().add(castToReference(value)); 3680 } else if (name.equals("source[x]")) { 3681 this.source = castToType(value); // Type 3682 } else if (name.equals("policy")) { 3683 this.getPolicy().add((ConsentPolicyComponent) value); 3684 } else if (name.equals("policyRule")) { 3685 this.policyRule = castToUri(value); // UriType 3686 } else if (name.equals("securityLabel")) { 3687 this.getSecurityLabel().add(castToCoding(value)); 3688 } else if (name.equals("purpose")) { 3689 this.getPurpose().add(castToCoding(value)); 3690 } else if (name.equals("dataPeriod")) { 3691 this.dataPeriod = castToPeriod(value); // Period 3692 } else if (name.equals("data")) { 3693 this.getData().add((ConsentDataComponent) value); 3694 } else if (name.equals("except")) { 3695 this.getExcept().add((ExceptComponent) value); 3696 } else 3697 return super.setProperty(name, value); 3698 return value; 3699 } 3700 3701 @Override 3702 public Base makeProperty(int hash, String name) throws FHIRException { 3703 switch (hash) { 3704 case -1618432855: return getIdentifier(); 3705 case -892481550: return getStatusElement(); 3706 case 50511102: return addCategory(); 3707 case -791418107: return getPatient(); 3708 case -991726143: return getPeriod(); 3709 case 1792749467: return getDateTimeElement(); 3710 case -1886702018: return addConsentingParty(); 3711 case 92645877: return addActor(); 3712 case -1422950858: return addAction(); 3713 case 1178922291: return addOrganization(); 3714 case -1698413947: return getSource(); 3715 case -896505829: return getSource(); 3716 case -982670030: return addPolicy(); 3717 case 1593493326: return getPolicyRuleElement(); 3718 case -722296940: return addSecurityLabel(); 3719 case -220463842: return addPurpose(); 3720 case 1177250315: return getDataPeriod(); 3721 case 3076010: return addData(); 3722 case -1289550567: return addExcept(); 3723 default: return super.makeProperty(hash, name); 3724 } 3725 3726 } 3727 3728 @Override 3729 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3730 switch (hash) { 3731 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3732 case -892481550: /*status*/ return new String[] {"code"}; 3733 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3734 case -791418107: /*patient*/ return new String[] {"Reference"}; 3735 case -991726143: /*period*/ return new String[] {"Period"}; 3736 case 1792749467: /*dateTime*/ return new String[] {"dateTime"}; 3737 case -1886702018: /*consentingParty*/ return new String[] {"Reference"}; 3738 case 92645877: /*actor*/ return new String[] {}; 3739 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 3740 case 1178922291: /*organization*/ return new String[] {"Reference"}; 3741 case -896505829: /*source*/ return new String[] {"Attachment", "Identifier", "Reference"}; 3742 case -982670030: /*policy*/ return new String[] {}; 3743 case 1593493326: /*policyRule*/ return new String[] {"uri"}; 3744 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 3745 case -220463842: /*purpose*/ return new String[] {"Coding"}; 3746 case 1177250315: /*dataPeriod*/ return new String[] {"Period"}; 3747 case 3076010: /*data*/ return new String[] {}; 3748 case -1289550567: /*except*/ return new String[] {}; 3749 default: return super.getTypesForProperty(hash, name); 3750 } 3751 3752 } 3753 3754 @Override 3755 public Base addChild(String name) throws FHIRException { 3756 if (name.equals("identifier")) { 3757 this.identifier = new Identifier(); 3758 return this.identifier; 3759 } 3760 else if (name.equals("status")) { 3761 throw new FHIRException("Cannot call addChild on a singleton property Consent.status"); 3762 } 3763 else if (name.equals("category")) { 3764 return addCategory(); 3765 } 3766 else if (name.equals("patient")) { 3767 this.patient = new Reference(); 3768 return this.patient; 3769 } 3770 else if (name.equals("period")) { 3771 this.period = new Period(); 3772 return this.period; 3773 } 3774 else if (name.equals("dateTime")) { 3775 throw new FHIRException("Cannot call addChild on a singleton property Consent.dateTime"); 3776 } 3777 else if (name.equals("consentingParty")) { 3778 return addConsentingParty(); 3779 } 3780 else if (name.equals("actor")) { 3781 return addActor(); 3782 } 3783 else if (name.equals("action")) { 3784 return addAction(); 3785 } 3786 else if (name.equals("organization")) { 3787 return addOrganization(); 3788 } 3789 else if (name.equals("sourceAttachment")) { 3790 this.source = new Attachment(); 3791 return this.source; 3792 } 3793 else if (name.equals("sourceIdentifier")) { 3794 this.source = new Identifier(); 3795 return this.source; 3796 } 3797 else if (name.equals("sourceReference")) { 3798 this.source = new Reference(); 3799 return this.source; 3800 } 3801 else if (name.equals("policy")) { 3802 return addPolicy(); 3803 } 3804 else if (name.equals("policyRule")) { 3805 throw new FHIRException("Cannot call addChild on a singleton property Consent.policyRule"); 3806 } 3807 else if (name.equals("securityLabel")) { 3808 return addSecurityLabel(); 3809 } 3810 else if (name.equals("purpose")) { 3811 return addPurpose(); 3812 } 3813 else if (name.equals("dataPeriod")) { 3814 this.dataPeriod = new Period(); 3815 return this.dataPeriod; 3816 } 3817 else if (name.equals("data")) { 3818 return addData(); 3819 } 3820 else if (name.equals("except")) { 3821 return addExcept(); 3822 } 3823 else 3824 return super.addChild(name); 3825 } 3826 3827 public String fhirType() { 3828 return "Consent"; 3829 3830 } 3831 3832 public Consent copy() { 3833 Consent dst = new Consent(); 3834 copyValues(dst); 3835 dst.identifier = identifier == null ? null : identifier.copy(); 3836 dst.status = status == null ? null : status.copy(); 3837 if (category != null) { 3838 dst.category = new ArrayList<CodeableConcept>(); 3839 for (CodeableConcept i : category) 3840 dst.category.add(i.copy()); 3841 }; 3842 dst.patient = patient == null ? null : patient.copy(); 3843 dst.period = period == null ? null : period.copy(); 3844 dst.dateTime = dateTime == null ? null : dateTime.copy(); 3845 if (consentingParty != null) { 3846 dst.consentingParty = new ArrayList<Reference>(); 3847 for (Reference i : consentingParty) 3848 dst.consentingParty.add(i.copy()); 3849 }; 3850 if (actor != null) { 3851 dst.actor = new ArrayList<ConsentActorComponent>(); 3852 for (ConsentActorComponent i : actor) 3853 dst.actor.add(i.copy()); 3854 }; 3855 if (action != null) { 3856 dst.action = new ArrayList<CodeableConcept>(); 3857 for (CodeableConcept i : action) 3858 dst.action.add(i.copy()); 3859 }; 3860 if (organization != null) { 3861 dst.organization = new ArrayList<Reference>(); 3862 for (Reference i : organization) 3863 dst.organization.add(i.copy()); 3864 }; 3865 dst.source = source == null ? null : source.copy(); 3866 if (policy != null) { 3867 dst.policy = new ArrayList<ConsentPolicyComponent>(); 3868 for (ConsentPolicyComponent i : policy) 3869 dst.policy.add(i.copy()); 3870 }; 3871 dst.policyRule = policyRule == null ? null : policyRule.copy(); 3872 if (securityLabel != null) { 3873 dst.securityLabel = new ArrayList<Coding>(); 3874 for (Coding i : securityLabel) 3875 dst.securityLabel.add(i.copy()); 3876 }; 3877 if (purpose != null) { 3878 dst.purpose = new ArrayList<Coding>(); 3879 for (Coding i : purpose) 3880 dst.purpose.add(i.copy()); 3881 }; 3882 dst.dataPeriod = dataPeriod == null ? null : dataPeriod.copy(); 3883 if (data != null) { 3884 dst.data = new ArrayList<ConsentDataComponent>(); 3885 for (ConsentDataComponent i : data) 3886 dst.data.add(i.copy()); 3887 }; 3888 if (except != null) { 3889 dst.except = new ArrayList<ExceptComponent>(); 3890 for (ExceptComponent i : except) 3891 dst.except.add(i.copy()); 3892 }; 3893 return dst; 3894 } 3895 3896 protected Consent typedCopy() { 3897 return copy(); 3898 } 3899 3900 @Override 3901 public boolean equalsDeep(Base other_) { 3902 if (!super.equalsDeep(other_)) 3903 return false; 3904 if (!(other_ instanceof Consent)) 3905 return false; 3906 Consent o = (Consent) other_; 3907 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 3908 && compareDeep(patient, o.patient, true) && compareDeep(period, o.period, true) && compareDeep(dateTime, o.dateTime, true) 3909 && compareDeep(consentingParty, o.consentingParty, true) && compareDeep(actor, o.actor, true) && compareDeep(action, o.action, true) 3910 && compareDeep(organization, o.organization, true) && compareDeep(source, o.source, true) && compareDeep(policy, o.policy, true) 3911 && compareDeep(policyRule, o.policyRule, true) && compareDeep(securityLabel, o.securityLabel, true) 3912 && compareDeep(purpose, o.purpose, true) && compareDeep(dataPeriod, o.dataPeriod, true) && compareDeep(data, o.data, true) 3913 && compareDeep(except, o.except, true); 3914 } 3915 3916 @Override 3917 public boolean equalsShallow(Base other_) { 3918 if (!super.equalsShallow(other_)) 3919 return false; 3920 if (!(other_ instanceof Consent)) 3921 return false; 3922 Consent o = (Consent) other_; 3923 return compareValues(status, o.status, true) && compareValues(dateTime, o.dateTime, true) && compareValues(policyRule, o.policyRule, true) 3924 ; 3925 } 3926 3927 public boolean isEmpty() { 3928 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 3929 , patient, period, dateTime, consentingParty, actor, action, organization, source 3930 , policy, policyRule, securityLabel, purpose, dataPeriod, data, except); 3931 } 3932 3933 @Override 3934 public ResourceType getResourceType() { 3935 return ResourceType.Consent; 3936 } 3937 3938 /** 3939 * Search parameter: <b>date</b> 3940 * <p> 3941 * Description: <b>When this Consent was created or indexed</b><br> 3942 * Type: <b>date</b><br> 3943 * Path: <b>Consent.dateTime</b><br> 3944 * </p> 3945 */ 3946 @SearchParamDefinition(name="date", path="Consent.dateTime", description="When this Consent was created or indexed", type="date" ) 3947 public static final String SP_DATE = "date"; 3948 /** 3949 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3950 * <p> 3951 * Description: <b>When this Consent was created or indexed</b><br> 3952 * Type: <b>date</b><br> 3953 * Path: <b>Consent.dateTime</b><br> 3954 * </p> 3955 */ 3956 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3957 3958 /** 3959 * Search parameter: <b>identifier</b> 3960 * <p> 3961 * Description: <b>Identifier for this record (external references)</b><br> 3962 * Type: <b>token</b><br> 3963 * Path: <b>Consent.identifier</b><br> 3964 * </p> 3965 */ 3966 @SearchParamDefinition(name="identifier", path="Consent.identifier", description="Identifier for this record (external references)", type="token" ) 3967 public static final String SP_IDENTIFIER = "identifier"; 3968 /** 3969 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3970 * <p> 3971 * Description: <b>Identifier for this record (external references)</b><br> 3972 * Type: <b>token</b><br> 3973 * Path: <b>Consent.identifier</b><br> 3974 * </p> 3975 */ 3976 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3977 3978 /** 3979 * Search parameter: <b>securitylabel</b> 3980 * <p> 3981 * Description: <b>Security Labels that define affected resources</b><br> 3982 * Type: <b>token</b><br> 3983 * Path: <b>Consent.securityLabel, Consent.except.securityLabel</b><br> 3984 * </p> 3985 */ 3986 @SearchParamDefinition(name="securitylabel", path="Consent.securityLabel | Consent.except.securityLabel", description="Security Labels that define affected resources", type="token" ) 3987 public static final String SP_SECURITYLABEL = "securitylabel"; 3988 /** 3989 * <b>Fluent Client</b> search parameter constant for <b>securitylabel</b> 3990 * <p> 3991 * Description: <b>Security Labels that define affected resources</b><br> 3992 * Type: <b>token</b><br> 3993 * Path: <b>Consent.securityLabel, Consent.except.securityLabel</b><br> 3994 * </p> 3995 */ 3996 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITYLABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITYLABEL); 3997 3998 /** 3999 * Search parameter: <b>period</b> 4000 * <p> 4001 * Description: <b>Period that this consent applies</b><br> 4002 * Type: <b>date</b><br> 4003 * Path: <b>Consent.period</b><br> 4004 * </p> 4005 */ 4006 @SearchParamDefinition(name="period", path="Consent.period", description="Period that this consent applies", type="date" ) 4007 public static final String SP_PERIOD = "period"; 4008 /** 4009 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4010 * <p> 4011 * Description: <b>Period that this consent applies</b><br> 4012 * Type: <b>date</b><br> 4013 * Path: <b>Consent.period</b><br> 4014 * </p> 4015 */ 4016 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 4017 4018 /** 4019 * Search parameter: <b>data</b> 4020 * <p> 4021 * Description: <b>The actual data reference</b><br> 4022 * Type: <b>reference</b><br> 4023 * Path: <b>Consent.data.reference, Consent.except.data.reference</b><br> 4024 * </p> 4025 */ 4026 @SearchParamDefinition(name="data", path="Consent.data.reference | Consent.except.data.reference", description="The actual data reference", type="reference" ) 4027 public static final String SP_DATA = "data"; 4028 /** 4029 * <b>Fluent Client</b> search parameter constant for <b>data</b> 4030 * <p> 4031 * Description: <b>The actual data reference</b><br> 4032 * Type: <b>reference</b><br> 4033 * Path: <b>Consent.data.reference, Consent.except.data.reference</b><br> 4034 * </p> 4035 */ 4036 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DATA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DATA); 4037 4038/** 4039 * Constant for fluent queries to be used to add include statements. Specifies 4040 * the path value of "<b>Consent:data</b>". 4041 */ 4042 public static final ca.uhn.fhir.model.api.Include INCLUDE_DATA = new ca.uhn.fhir.model.api.Include("Consent:data").toLocked(); 4043 4044 /** 4045 * Search parameter: <b>purpose</b> 4046 * <p> 4047 * Description: <b>Context of activities for which the agreement is made</b><br> 4048 * Type: <b>token</b><br> 4049 * Path: <b>Consent.purpose, Consent.except.purpose</b><br> 4050 * </p> 4051 */ 4052 @SearchParamDefinition(name="purpose", path="Consent.purpose | Consent.except.purpose", description="Context of activities for which the agreement is made", type="token" ) 4053 public static final String SP_PURPOSE = "purpose"; 4054 /** 4055 * <b>Fluent Client</b> search parameter constant for <b>purpose</b> 4056 * <p> 4057 * Description: <b>Context of activities for which the agreement is made</b><br> 4058 * Type: <b>token</b><br> 4059 * Path: <b>Consent.purpose, Consent.except.purpose</b><br> 4060 * </p> 4061 */ 4062 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PURPOSE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PURPOSE); 4063 4064 /** 4065 * Search parameter: <b>source</b> 4066 * <p> 4067 * Description: <b>Source from which this consent is taken</b><br> 4068 * Type: <b>reference</b><br> 4069 * Path: <b>Consent.source[x]</b><br> 4070 * </p> 4071 */ 4072 @SearchParamDefinition(name="source", path="Consent.source", description="Source from which this consent is taken", type="reference", target={Consent.class, Contract.class, DocumentReference.class, QuestionnaireResponse.class } ) 4073 public static final String SP_SOURCE = "source"; 4074 /** 4075 * <b>Fluent Client</b> search parameter constant for <b>source</b> 4076 * <p> 4077 * Description: <b>Source from which this consent is taken</b><br> 4078 * Type: <b>reference</b><br> 4079 * Path: <b>Consent.source[x]</b><br> 4080 * </p> 4081 */ 4082 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 4083 4084/** 4085 * Constant for fluent queries to be used to add include statements. Specifies 4086 * the path value of "<b>Consent:source</b>". 4087 */ 4088 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("Consent:source").toLocked(); 4089 4090 /** 4091 * Search parameter: <b>actor</b> 4092 * <p> 4093 * Description: <b>Resource for the actor (or group, by role)</b><br> 4094 * Type: <b>reference</b><br> 4095 * Path: <b>Consent.actor.reference, Consent.except.actor.reference</b><br> 4096 * </p> 4097 */ 4098 @SearchParamDefinition(name="actor", path="Consent.actor.reference | Consent.except.actor.reference", description="Resource for the actor (or group, by role)", type="reference", target={CareTeam.class, Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 4099 public static final String SP_ACTOR = "actor"; 4100 /** 4101 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 4102 * <p> 4103 * Description: <b>Resource for the actor (or group, by role)</b><br> 4104 * Type: <b>reference</b><br> 4105 * Path: <b>Consent.actor.reference, Consent.except.actor.reference</b><br> 4106 * </p> 4107 */ 4108 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 4109 4110/** 4111 * Constant for fluent queries to be used to add include statements. Specifies 4112 * the path value of "<b>Consent:actor</b>". 4113 */ 4114 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Consent:actor").toLocked(); 4115 4116 /** 4117 * Search parameter: <b>patient</b> 4118 * <p> 4119 * Description: <b>Who the consent applies to</b><br> 4120 * Type: <b>reference</b><br> 4121 * Path: <b>Consent.patient</b><br> 4122 * </p> 4123 */ 4124 @SearchParamDefinition(name="patient", path="Consent.patient", description="Who the consent applies to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 4125 public static final String SP_PATIENT = "patient"; 4126 /** 4127 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4128 * <p> 4129 * Description: <b>Who the consent applies to</b><br> 4130 * Type: <b>reference</b><br> 4131 * Path: <b>Consent.patient</b><br> 4132 * </p> 4133 */ 4134 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4135 4136/** 4137 * Constant for fluent queries to be used to add include statements. Specifies 4138 * the path value of "<b>Consent:patient</b>". 4139 */ 4140 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Consent:patient").toLocked(); 4141 4142 /** 4143 * Search parameter: <b>organization</b> 4144 * <p> 4145 * Description: <b>Custodian of the consent</b><br> 4146 * Type: <b>reference</b><br> 4147 * Path: <b>Consent.organization</b><br> 4148 * </p> 4149 */ 4150 @SearchParamDefinition(name="organization", path="Consent.organization", description="Custodian of the consent", type="reference", target={Organization.class } ) 4151 public static final String SP_ORGANIZATION = "organization"; 4152 /** 4153 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 4154 * <p> 4155 * Description: <b>Custodian of the consent</b><br> 4156 * Type: <b>reference</b><br> 4157 * Path: <b>Consent.organization</b><br> 4158 * </p> 4159 */ 4160 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 4161 4162/** 4163 * Constant for fluent queries to be used to add include statements. Specifies 4164 * the path value of "<b>Consent:organization</b>". 4165 */ 4166 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Consent:organization").toLocked(); 4167 4168 /** 4169 * Search parameter: <b>action</b> 4170 * <p> 4171 * Description: <b>Actions controlled by this consent</b><br> 4172 * Type: <b>token</b><br> 4173 * Path: <b>Consent.action, Consent.except.action</b><br> 4174 * </p> 4175 */ 4176 @SearchParamDefinition(name="action", path="Consent.action | Consent.except.action", description="Actions controlled by this consent", type="token" ) 4177 public static final String SP_ACTION = "action"; 4178 /** 4179 * <b>Fluent Client</b> search parameter constant for <b>action</b> 4180 * <p> 4181 * Description: <b>Actions controlled by this consent</b><br> 4182 * Type: <b>token</b><br> 4183 * Path: <b>Consent.action, Consent.except.action</b><br> 4184 * </p> 4185 */ 4186 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTION); 4187 4188 /** 4189 * Search parameter: <b>consentor</b> 4190 * <p> 4191 * Description: <b>Who is agreeing to the policy and exceptions</b><br> 4192 * Type: <b>reference</b><br> 4193 * Path: <b>Consent.consentingParty</b><br> 4194 * </p> 4195 */ 4196 @SearchParamDefinition(name="consentor", path="Consent.consentingParty", description="Who is agreeing to the policy and exceptions", type="reference", target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 4197 public static final String SP_CONSENTOR = "consentor"; 4198 /** 4199 * <b>Fluent Client</b> search parameter constant for <b>consentor</b> 4200 * <p> 4201 * Description: <b>Who is agreeing to the policy and exceptions</b><br> 4202 * Type: <b>reference</b><br> 4203 * Path: <b>Consent.consentingParty</b><br> 4204 * </p> 4205 */ 4206 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONSENTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONSENTOR); 4207 4208/** 4209 * Constant for fluent queries to be used to add include statements. Specifies 4210 * the path value of "<b>Consent:consentor</b>". 4211 */ 4212 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONSENTOR = new ca.uhn.fhir.model.api.Include("Consent:consentor").toLocked(); 4213 4214 /** 4215 * Search parameter: <b>category</b> 4216 * <p> 4217 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 4218 * Type: <b>token</b><br> 4219 * Path: <b>Consent.category</b><br> 4220 * </p> 4221 */ 4222 @SearchParamDefinition(name="category", path="Consent.category", description="Classification of the consent statement - for indexing/retrieval", type="token" ) 4223 public static final String SP_CATEGORY = "category"; 4224 /** 4225 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4226 * <p> 4227 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 4228 * Type: <b>token</b><br> 4229 * Path: <b>Consent.category</b><br> 4230 * </p> 4231 */ 4232 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4233 4234 /** 4235 * Search parameter: <b>status</b> 4236 * <p> 4237 * Description: <b>draft | proposed | active | rejected | inactive | entered-in-error</b><br> 4238 * Type: <b>token</b><br> 4239 * Path: <b>Consent.status</b><br> 4240 * </p> 4241 */ 4242 @SearchParamDefinition(name="status", path="Consent.status", description="draft | proposed | active | rejected | inactive | entered-in-error", type="token" ) 4243 public static final String SP_STATUS = "status"; 4244 /** 4245 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4246 * <p> 4247 * Description: <b>draft | proposed | active | rejected | inactive | entered-in-error</b><br> 4248 * Type: <b>token</b><br> 4249 * Path: <b>Consent.status</b><br> 4250 * </p> 4251 */ 4252 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4253 4254 4255}