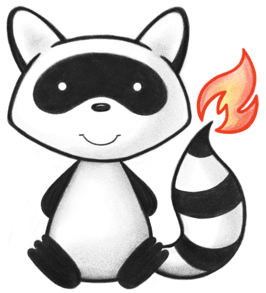
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * Specifies contact information for a person or organization. 047 */ 048@DatatypeDef(name="ContactDetail") 049public class ContactDetail extends Type implements ICompositeType { 050 051 /** 052 * The name of an individual to contact. 053 */ 054 @Child(name = "name", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 055 @Description(shortDefinition="Name of an individual to contact", formalDefinition="The name of an individual to contact." ) 056 protected StringType name; 057 058 /** 059 * The contact details for the individual (if a name was provided) or the organization. 060 */ 061 @Child(name = "telecom", type = {ContactPoint.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 062 @Description(shortDefinition="Contact details for individual or organization", formalDefinition="The contact details for the individual (if a name was provided) or the organization." ) 063 protected List<ContactPoint> telecom; 064 065 private static final long serialVersionUID = 816838773L; 066 067 /** 068 * Constructor 069 */ 070 public ContactDetail() { 071 super(); 072 } 073 074 /** 075 * @return {@link #name} (The name of an individual to contact.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 076 */ 077 public StringType getNameElement() { 078 if (this.name == null) 079 if (Configuration.errorOnAutoCreate()) 080 throw new Error("Attempt to auto-create ContactDetail.name"); 081 else if (Configuration.doAutoCreate()) 082 this.name = new StringType(); // bb 083 return this.name; 084 } 085 086 public boolean hasNameElement() { 087 return this.name != null && !this.name.isEmpty(); 088 } 089 090 public boolean hasName() { 091 return this.name != null && !this.name.isEmpty(); 092 } 093 094 /** 095 * @param value {@link #name} (The name of an individual to contact.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 096 */ 097 public ContactDetail setNameElement(StringType value) { 098 this.name = value; 099 return this; 100 } 101 102 /** 103 * @return The name of an individual to contact. 104 */ 105 public String getName() { 106 return this.name == null ? null : this.name.getValue(); 107 } 108 109 /** 110 * @param value The name of an individual to contact. 111 */ 112 public ContactDetail setName(String value) { 113 if (Utilities.noString(value)) 114 this.name = null; 115 else { 116 if (this.name == null) 117 this.name = new StringType(); 118 this.name.setValue(value); 119 } 120 return this; 121 } 122 123 /** 124 * @return {@link #telecom} (The contact details for the individual (if a name was provided) or the organization.) 125 */ 126 public List<ContactPoint> getTelecom() { 127 if (this.telecom == null) 128 this.telecom = new ArrayList<ContactPoint>(); 129 return this.telecom; 130 } 131 132 /** 133 * @return Returns a reference to <code>this</code> for easy method chaining 134 */ 135 public ContactDetail setTelecom(List<ContactPoint> theTelecom) { 136 this.telecom = theTelecom; 137 return this; 138 } 139 140 public boolean hasTelecom() { 141 if (this.telecom == null) 142 return false; 143 for (ContactPoint item : this.telecom) 144 if (!item.isEmpty()) 145 return true; 146 return false; 147 } 148 149 public ContactPoint addTelecom() { //3 150 ContactPoint t = new ContactPoint(); 151 if (this.telecom == null) 152 this.telecom = new ArrayList<ContactPoint>(); 153 this.telecom.add(t); 154 return t; 155 } 156 157 public ContactDetail addTelecom(ContactPoint t) { //3 158 if (t == null) 159 return this; 160 if (this.telecom == null) 161 this.telecom = new ArrayList<ContactPoint>(); 162 this.telecom.add(t); 163 return this; 164 } 165 166 /** 167 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 168 */ 169 public ContactPoint getTelecomFirstRep() { 170 if (getTelecom().isEmpty()) { 171 addTelecom(); 172 } 173 return getTelecom().get(0); 174 } 175 176 protected void listChildren(List<Property> children) { 177 super.listChildren(children); 178 children.add(new Property("name", "string", "The name of an individual to contact.", 0, 1, name)); 179 children.add(new Property("telecom", "ContactPoint", "The contact details for the individual (if a name was provided) or the organization.", 0, java.lang.Integer.MAX_VALUE, telecom)); 180 } 181 182 @Override 183 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 184 switch (_hash) { 185 case 3373707: /*name*/ return new Property("name", "string", "The name of an individual to contact.", 0, 1, name); 186 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "The contact details for the individual (if a name was provided) or the organization.", 0, java.lang.Integer.MAX_VALUE, telecom); 187 default: return super.getNamedProperty(_hash, _name, _checkValid); 188 } 189 190 } 191 192 @Override 193 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 194 switch (hash) { 195 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 196 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 197 default: return super.getProperty(hash, name, checkValid); 198 } 199 200 } 201 202 @Override 203 public Base setProperty(int hash, String name, Base value) throws FHIRException { 204 switch (hash) { 205 case 3373707: // name 206 this.name = castToString(value); // StringType 207 return value; 208 case -1429363305: // telecom 209 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 210 return value; 211 default: return super.setProperty(hash, name, value); 212 } 213 214 } 215 216 @Override 217 public Base setProperty(String name, Base value) throws FHIRException { 218 if (name.equals("name")) { 219 this.name = castToString(value); // StringType 220 } else if (name.equals("telecom")) { 221 this.getTelecom().add(castToContactPoint(value)); 222 } else 223 return super.setProperty(name, value); 224 return value; 225 } 226 227 @Override 228 public Base makeProperty(int hash, String name) throws FHIRException { 229 switch (hash) { 230 case 3373707: return getNameElement(); 231 case -1429363305: return addTelecom(); 232 default: return super.makeProperty(hash, name); 233 } 234 235 } 236 237 @Override 238 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 239 switch (hash) { 240 case 3373707: /*name*/ return new String[] {"string"}; 241 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 242 default: return super.getTypesForProperty(hash, name); 243 } 244 245 } 246 247 @Override 248 public Base addChild(String name) throws FHIRException { 249 if (name.equals("name")) { 250 throw new FHIRException("Cannot call addChild on a singleton property ContactDetail.name"); 251 } 252 else if (name.equals("telecom")) { 253 return addTelecom(); 254 } 255 else 256 return super.addChild(name); 257 } 258 259 public String fhirType() { 260 return "ContactDetail"; 261 262 } 263 264 public ContactDetail copy() { 265 ContactDetail dst = new ContactDetail(); 266 copyValues(dst); 267 dst.name = name == null ? null : name.copy(); 268 if (telecom != null) { 269 dst.telecom = new ArrayList<ContactPoint>(); 270 for (ContactPoint i : telecom) 271 dst.telecom.add(i.copy()); 272 }; 273 return dst; 274 } 275 276 protected ContactDetail typedCopy() { 277 return copy(); 278 } 279 280 @Override 281 public boolean equalsDeep(Base other_) { 282 if (!super.equalsDeep(other_)) 283 return false; 284 if (!(other_ instanceof ContactDetail)) 285 return false; 286 ContactDetail o = (ContactDetail) other_; 287 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 288 } 289 290 @Override 291 public boolean equalsShallow(Base other_) { 292 if (!super.equalsShallow(other_)) 293 return false; 294 if (!(other_ instanceof ContactDetail)) 295 return false; 296 ContactDetail o = (ContactDetail) other_; 297 return compareValues(name, o.name, true); 298 } 299 300 public boolean isEmpty() { 301 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, telecom); 302 } 303 304 305}