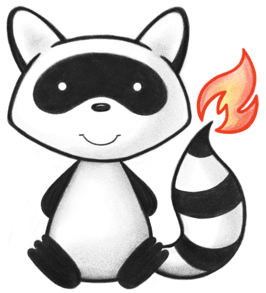
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044/** 045 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 046 */ 047@DatatypeDef(name="ContactPoint") 048public class ContactPoint extends Type implements ICompositeType { 049 050 public enum ContactPointSystem { 051 /** 052 * The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required. 053 */ 054 PHONE, 055 /** 056 * The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required. 057 */ 058 FAX, 059 /** 060 * The value is an email address. 061 */ 062 EMAIL, 063 /** 064 * The value is a pager number. These may be local pager numbers that are only usable on a particular pager system. 065 */ 066 PAGER, 067 /** 068 * A contact that is not a phone, fax, pager or email address and is expressed as a URL. This is intended for various personal contacts including blogs, Skype, Twitter, Facebook, etc. Do not use for email addresses. 069 */ 070 URL, 071 /** 072 * A contact that can be used for sending an sms message (e.g. mobide phones, some landlines) 073 */ 074 SMS, 075 /** 076 * A contact that is not a phone, fax, page or email address and is not expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) Extensions may be used to distinguish "other" contact types. 077 */ 078 OTHER, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 public static ContactPointSystem fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("phone".equals(codeString)) 087 return PHONE; 088 if ("fax".equals(codeString)) 089 return FAX; 090 if ("email".equals(codeString)) 091 return EMAIL; 092 if ("pager".equals(codeString)) 093 return PAGER; 094 if ("url".equals(codeString)) 095 return URL; 096 if ("sms".equals(codeString)) 097 return SMS; 098 if ("other".equals(codeString)) 099 return OTHER; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown ContactPointSystem code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case PHONE: return "phone"; 108 case FAX: return "fax"; 109 case EMAIL: return "email"; 110 case PAGER: return "pager"; 111 case URL: return "url"; 112 case SMS: return "sms"; 113 case OTHER: return "other"; 114 case NULL: return null; 115 default: return "?"; 116 } 117 } 118 public String getSystem() { 119 switch (this) { 120 case PHONE: return "http://hl7.org/fhir/contact-point-system"; 121 case FAX: return "http://hl7.org/fhir/contact-point-system"; 122 case EMAIL: return "http://hl7.org/fhir/contact-point-system"; 123 case PAGER: return "http://hl7.org/fhir/contact-point-system"; 124 case URL: return "http://hl7.org/fhir/contact-point-system"; 125 case SMS: return "http://hl7.org/fhir/contact-point-system"; 126 case OTHER: return "http://hl7.org/fhir/contact-point-system"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getDefinition() { 132 switch (this) { 133 case PHONE: return "The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 134 case FAX: return "The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 135 case EMAIL: return "The value is an email address."; 136 case PAGER: return "The value is a pager number. These may be local pager numbers that are only usable on a particular pager system."; 137 case URL: return "A contact that is not a phone, fax, pager or email address and is expressed as a URL. This is intended for various personal contacts including blogs, Skype, Twitter, Facebook, etc. Do not use for email addresses."; 138 case SMS: return "A contact that can be used for sending an sms message (e.g. mobide phones, some landlines)"; 139 case OTHER: return "A contact that is not a phone, fax, page or email address and is not expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) Extensions may be used to distinguish \"other\" contact types."; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 public String getDisplay() { 145 switch (this) { 146 case PHONE: return "Phone"; 147 case FAX: return "Fax"; 148 case EMAIL: return "Email"; 149 case PAGER: return "Pager"; 150 case URL: return "URL"; 151 case SMS: return "SMS"; 152 case OTHER: return "Other"; 153 case NULL: return null; 154 default: return "?"; 155 } 156 } 157 } 158 159 public static class ContactPointSystemEnumFactory implements EnumFactory<ContactPointSystem> { 160 public ContactPointSystem fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("phone".equals(codeString)) 165 return ContactPointSystem.PHONE; 166 if ("fax".equals(codeString)) 167 return ContactPointSystem.FAX; 168 if ("email".equals(codeString)) 169 return ContactPointSystem.EMAIL; 170 if ("pager".equals(codeString)) 171 return ContactPointSystem.PAGER; 172 if ("url".equals(codeString)) 173 return ContactPointSystem.URL; 174 if ("sms".equals(codeString)) 175 return ContactPointSystem.SMS; 176 if ("other".equals(codeString)) 177 return ContactPointSystem.OTHER; 178 throw new IllegalArgumentException("Unknown ContactPointSystem code '"+codeString+"'"); 179 } 180 public Enumeration<ContactPointSystem> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<ContactPointSystem>(this); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return null; 188 if ("phone".equals(codeString)) 189 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PHONE); 190 if ("fax".equals(codeString)) 191 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.FAX); 192 if ("email".equals(codeString)) 193 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.EMAIL); 194 if ("pager".equals(codeString)) 195 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PAGER); 196 if ("url".equals(codeString)) 197 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.URL); 198 if ("sms".equals(codeString)) 199 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.SMS); 200 if ("other".equals(codeString)) 201 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.OTHER); 202 throw new FHIRException("Unknown ContactPointSystem code '"+codeString+"'"); 203 } 204 public String toCode(ContactPointSystem code) { 205 if (code == ContactPointSystem.NULL) 206 return null; 207 if (code == ContactPointSystem.PHONE) 208 return "phone"; 209 if (code == ContactPointSystem.FAX) 210 return "fax"; 211 if (code == ContactPointSystem.EMAIL) 212 return "email"; 213 if (code == ContactPointSystem.PAGER) 214 return "pager"; 215 if (code == ContactPointSystem.URL) 216 return "url"; 217 if (code == ContactPointSystem.SMS) 218 return "sms"; 219 if (code == ContactPointSystem.OTHER) 220 return "other"; 221 return "?"; 222 } 223 public String toSystem(ContactPointSystem code) { 224 return code.getSystem(); 225 } 226 } 227 228 public enum ContactPointUse { 229 /** 230 * A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available. 231 */ 232 HOME, 233 /** 234 * An office contact point. First choice for business related contacts during business hours. 235 */ 236 WORK, 237 /** 238 * A temporary contact point. The period can provide more detailed information. 239 */ 240 TEMP, 241 /** 242 * This contact point is no longer in use (or was never correct, but retained for records). 243 */ 244 OLD, 245 /** 246 * A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business. 247 */ 248 MOBILE, 249 /** 250 * added to help the parsers with the generic types 251 */ 252 NULL; 253 public static ContactPointUse fromCode(String codeString) throws FHIRException { 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("home".equals(codeString)) 257 return HOME; 258 if ("work".equals(codeString)) 259 return WORK; 260 if ("temp".equals(codeString)) 261 return TEMP; 262 if ("old".equals(codeString)) 263 return OLD; 264 if ("mobile".equals(codeString)) 265 return MOBILE; 266 if (Configuration.isAcceptInvalidEnums()) 267 return null; 268 else 269 throw new FHIRException("Unknown ContactPointUse code '"+codeString+"'"); 270 } 271 public String toCode() { 272 switch (this) { 273 case HOME: return "home"; 274 case WORK: return "work"; 275 case TEMP: return "temp"; 276 case OLD: return "old"; 277 case MOBILE: return "mobile"; 278 case NULL: return null; 279 default: return "?"; 280 } 281 } 282 public String getSystem() { 283 switch (this) { 284 case HOME: return "http://hl7.org/fhir/contact-point-use"; 285 case WORK: return "http://hl7.org/fhir/contact-point-use"; 286 case TEMP: return "http://hl7.org/fhir/contact-point-use"; 287 case OLD: return "http://hl7.org/fhir/contact-point-use"; 288 case MOBILE: return "http://hl7.org/fhir/contact-point-use"; 289 case NULL: return null; 290 default: return "?"; 291 } 292 } 293 public String getDefinition() { 294 switch (this) { 295 case HOME: return "A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available."; 296 case WORK: return "An office contact point. First choice for business related contacts during business hours."; 297 case TEMP: return "A temporary contact point. The period can provide more detailed information."; 298 case OLD: return "This contact point is no longer in use (or was never correct, but retained for records)."; 299 case MOBILE: return "A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business."; 300 case NULL: return null; 301 default: return "?"; 302 } 303 } 304 public String getDisplay() { 305 switch (this) { 306 case HOME: return "Home"; 307 case WORK: return "Work"; 308 case TEMP: return "Temp"; 309 case OLD: return "Old"; 310 case MOBILE: return "Mobile"; 311 case NULL: return null; 312 default: return "?"; 313 } 314 } 315 } 316 317 public static class ContactPointUseEnumFactory implements EnumFactory<ContactPointUse> { 318 public ContactPointUse fromCode(String codeString) throws IllegalArgumentException { 319 if (codeString == null || "".equals(codeString)) 320 if (codeString == null || "".equals(codeString)) 321 return null; 322 if ("home".equals(codeString)) 323 return ContactPointUse.HOME; 324 if ("work".equals(codeString)) 325 return ContactPointUse.WORK; 326 if ("temp".equals(codeString)) 327 return ContactPointUse.TEMP; 328 if ("old".equals(codeString)) 329 return ContactPointUse.OLD; 330 if ("mobile".equals(codeString)) 331 return ContactPointUse.MOBILE; 332 throw new IllegalArgumentException("Unknown ContactPointUse code '"+codeString+"'"); 333 } 334 public Enumeration<ContactPointUse> fromType(PrimitiveType<?> code) throws FHIRException { 335 if (code == null) 336 return null; 337 if (code.isEmpty()) 338 return new Enumeration<ContactPointUse>(this); 339 String codeString = code.asStringValue(); 340 if (codeString == null || "".equals(codeString)) 341 return null; 342 if ("home".equals(codeString)) 343 return new Enumeration<ContactPointUse>(this, ContactPointUse.HOME); 344 if ("work".equals(codeString)) 345 return new Enumeration<ContactPointUse>(this, ContactPointUse.WORK); 346 if ("temp".equals(codeString)) 347 return new Enumeration<ContactPointUse>(this, ContactPointUse.TEMP); 348 if ("old".equals(codeString)) 349 return new Enumeration<ContactPointUse>(this, ContactPointUse.OLD); 350 if ("mobile".equals(codeString)) 351 return new Enumeration<ContactPointUse>(this, ContactPointUse.MOBILE); 352 throw new FHIRException("Unknown ContactPointUse code '"+codeString+"'"); 353 } 354 public String toCode(ContactPointUse code) { 355 if (code == ContactPointUse.NULL) 356 return null; 357 if (code == ContactPointUse.HOME) 358 return "home"; 359 if (code == ContactPointUse.WORK) 360 return "work"; 361 if (code == ContactPointUse.TEMP) 362 return "temp"; 363 if (code == ContactPointUse.OLD) 364 return "old"; 365 if (code == ContactPointUse.MOBILE) 366 return "mobile"; 367 return "?"; 368 } 369 public String toSystem(ContactPointUse code) { 370 return code.getSystem(); 371 } 372 } 373 374 /** 375 * Telecommunications form for contact point - what communications system is required to make use of the contact. 376 */ 377 @Child(name = "system", type = {CodeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 378 @Description(shortDefinition="phone | fax | email | pager | url | sms | other", formalDefinition="Telecommunications form for contact point - what communications system is required to make use of the contact." ) 379 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contact-point-system") 380 protected Enumeration<ContactPointSystem> system; 381 382 /** 383 * The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 384 */ 385 @Child(name = "value", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 386 @Description(shortDefinition="The actual contact point details", formalDefinition="The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address)." ) 387 protected StringType value; 388 389 /** 390 * Identifies the purpose for the contact point. 391 */ 392 @Child(name = "use", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 393 @Description(shortDefinition="home | work | temp | old | mobile - purpose of this contact point", formalDefinition="Identifies the purpose for the contact point." ) 394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contact-point-use") 395 protected Enumeration<ContactPointUse> use; 396 397 /** 398 * Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values. 399 */ 400 @Child(name = "rank", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=true) 401 @Description(shortDefinition="Specify preferred order of use (1 = highest)", formalDefinition="Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values." ) 402 protected PositiveIntType rank; 403 404 /** 405 * Time period when the contact point was/is in use. 406 */ 407 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 408 @Description(shortDefinition="Time period when the contact point was/is in use", formalDefinition="Time period when the contact point was/is in use." ) 409 protected Period period; 410 411 private static final long serialVersionUID = 1509610874L; 412 413 /** 414 * Constructor 415 */ 416 public ContactPoint() { 417 super(); 418 } 419 420 /** 421 * @return {@link #system} (Telecommunications form for contact point - what communications system is required to make use of the contact.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 422 */ 423 public Enumeration<ContactPointSystem> getSystemElement() { 424 if (this.system == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create ContactPoint.system"); 427 else if (Configuration.doAutoCreate()) 428 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); // bb 429 return this.system; 430 } 431 432 public boolean hasSystemElement() { 433 return this.system != null && !this.system.isEmpty(); 434 } 435 436 public boolean hasSystem() { 437 return this.system != null && !this.system.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #system} (Telecommunications form for contact point - what communications system is required to make use of the contact.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 442 */ 443 public ContactPoint setSystemElement(Enumeration<ContactPointSystem> value) { 444 this.system = value; 445 return this; 446 } 447 448 /** 449 * @return Telecommunications form for contact point - what communications system is required to make use of the contact. 450 */ 451 public ContactPointSystem getSystem() { 452 return this.system == null ? null : this.system.getValue(); 453 } 454 455 /** 456 * @param value Telecommunications form for contact point - what communications system is required to make use of the contact. 457 */ 458 public ContactPoint setSystem(ContactPointSystem value) { 459 if (value == null) 460 this.system = null; 461 else { 462 if (this.system == null) 463 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); 464 this.system.setValue(value); 465 } 466 return this; 467 } 468 469 /** 470 * @return {@link #value} (The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 471 */ 472 public StringType getValueElement() { 473 if (this.value == null) 474 if (Configuration.errorOnAutoCreate()) 475 throw new Error("Attempt to auto-create ContactPoint.value"); 476 else if (Configuration.doAutoCreate()) 477 this.value = new StringType(); // bb 478 return this.value; 479 } 480 481 public boolean hasValueElement() { 482 return this.value != null && !this.value.isEmpty(); 483 } 484 485 public boolean hasValue() { 486 return this.value != null && !this.value.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #value} (The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 491 */ 492 public ContactPoint setValueElement(StringType value) { 493 this.value = value; 494 return this; 495 } 496 497 /** 498 * @return The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 499 */ 500 public String getValue() { 501 return this.value == null ? null : this.value.getValue(); 502 } 503 504 /** 505 * @param value The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 506 */ 507 public ContactPoint setValue(String value) { 508 if (Utilities.noString(value)) 509 this.value = null; 510 else { 511 if (this.value == null) 512 this.value = new StringType(); 513 this.value.setValue(value); 514 } 515 return this; 516 } 517 518 /** 519 * @return {@link #use} (Identifies the purpose for the contact point.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 520 */ 521 public Enumeration<ContactPointUse> getUseElement() { 522 if (this.use == null) 523 if (Configuration.errorOnAutoCreate()) 524 throw new Error("Attempt to auto-create ContactPoint.use"); 525 else if (Configuration.doAutoCreate()) 526 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); // bb 527 return this.use; 528 } 529 530 public boolean hasUseElement() { 531 return this.use != null && !this.use.isEmpty(); 532 } 533 534 public boolean hasUse() { 535 return this.use != null && !this.use.isEmpty(); 536 } 537 538 /** 539 * @param value {@link #use} (Identifies the purpose for the contact point.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 540 */ 541 public ContactPoint setUseElement(Enumeration<ContactPointUse> value) { 542 this.use = value; 543 return this; 544 } 545 546 /** 547 * @return Identifies the purpose for the contact point. 548 */ 549 public ContactPointUse getUse() { 550 return this.use == null ? null : this.use.getValue(); 551 } 552 553 /** 554 * @param value Identifies the purpose for the contact point. 555 */ 556 public ContactPoint setUse(ContactPointUse value) { 557 if (value == null) 558 this.use = null; 559 else { 560 if (this.use == null) 561 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); 562 this.use.setValue(value); 563 } 564 return this; 565 } 566 567 /** 568 * @return {@link #rank} (Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values.). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 569 */ 570 public PositiveIntType getRankElement() { 571 if (this.rank == null) 572 if (Configuration.errorOnAutoCreate()) 573 throw new Error("Attempt to auto-create ContactPoint.rank"); 574 else if (Configuration.doAutoCreate()) 575 this.rank = new PositiveIntType(); // bb 576 return this.rank; 577 } 578 579 public boolean hasRankElement() { 580 return this.rank != null && !this.rank.isEmpty(); 581 } 582 583 public boolean hasRank() { 584 return this.rank != null && !this.rank.isEmpty(); 585 } 586 587 /** 588 * @param value {@link #rank} (Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values.). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 589 */ 590 public ContactPoint setRankElement(PositiveIntType value) { 591 this.rank = value; 592 return this; 593 } 594 595 /** 596 * @return Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values. 597 */ 598 public int getRank() { 599 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 600 } 601 602 /** 603 * @param value Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values. 604 */ 605 public ContactPoint setRank(int value) { 606 if (this.rank == null) 607 this.rank = new PositiveIntType(); 608 this.rank.setValue(value); 609 return this; 610 } 611 612 /** 613 * @return {@link #period} (Time period when the contact point was/is in use.) 614 */ 615 public Period getPeriod() { 616 if (this.period == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create ContactPoint.period"); 619 else if (Configuration.doAutoCreate()) 620 this.period = new Period(); // cc 621 return this.period; 622 } 623 624 public boolean hasPeriod() { 625 return this.period != null && !this.period.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #period} (Time period when the contact point was/is in use.) 630 */ 631 public ContactPoint setPeriod(Period value) { 632 this.period = value; 633 return this; 634 } 635 636 protected void listChildren(List<Property> children) { 637 super.listChildren(children); 638 children.add(new Property("system", "code", "Telecommunications form for contact point - what communications system is required to make use of the contact.", 0, 1, system)); 639 children.add(new Property("value", "string", "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 0, 1, value)); 640 children.add(new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use)); 641 children.add(new Property("rank", "positiveInt", "Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values.", 0, 1, rank)); 642 children.add(new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, period)); 643 } 644 645 @Override 646 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 647 switch (_hash) { 648 case -887328209: /*system*/ return new Property("system", "code", "Telecommunications form for contact point - what communications system is required to make use of the contact.", 0, 1, system); 649 case 111972721: /*value*/ return new Property("value", "string", "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 0, 1, value); 650 case 116103: /*use*/ return new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use); 651 case 3492908: /*rank*/ return new Property("rank", "positiveInt", "Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values.", 0, 1, rank); 652 case -991726143: /*period*/ return new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, period); 653 default: return super.getNamedProperty(_hash, _name, _checkValid); 654 } 655 656 } 657 658 @Override 659 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 660 switch (hash) { 661 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // Enumeration<ContactPointSystem> 662 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 663 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<ContactPointUse> 664 case 3492908: /*rank*/ return this.rank == null ? new Base[0] : new Base[] {this.rank}; // PositiveIntType 665 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 666 default: return super.getProperty(hash, name, checkValid); 667 } 668 669 } 670 671 @Override 672 public Base setProperty(int hash, String name, Base value) throws FHIRException { 673 switch (hash) { 674 case -887328209: // system 675 value = new ContactPointSystemEnumFactory().fromType(castToCode(value)); 676 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 677 return value; 678 case 111972721: // value 679 this.value = castToString(value); // StringType 680 return value; 681 case 116103: // use 682 value = new ContactPointUseEnumFactory().fromType(castToCode(value)); 683 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 684 return value; 685 case 3492908: // rank 686 this.rank = castToPositiveInt(value); // PositiveIntType 687 return value; 688 case -991726143: // period 689 this.period = castToPeriod(value); // Period 690 return value; 691 default: return super.setProperty(hash, name, value); 692 } 693 694 } 695 696 @Override 697 public Base setProperty(String name, Base value) throws FHIRException { 698 if (name.equals("system")) { 699 value = new ContactPointSystemEnumFactory().fromType(castToCode(value)); 700 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 701 } else if (name.equals("value")) { 702 this.value = castToString(value); // StringType 703 } else if (name.equals("use")) { 704 value = new ContactPointUseEnumFactory().fromType(castToCode(value)); 705 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 706 } else if (name.equals("rank")) { 707 this.rank = castToPositiveInt(value); // PositiveIntType 708 } else if (name.equals("period")) { 709 this.period = castToPeriod(value); // Period 710 } else 711 return super.setProperty(name, value); 712 return value; 713 } 714 715 @Override 716 public Base makeProperty(int hash, String name) throws FHIRException { 717 switch (hash) { 718 case -887328209: return getSystemElement(); 719 case 111972721: return getValueElement(); 720 case 116103: return getUseElement(); 721 case 3492908: return getRankElement(); 722 case -991726143: return getPeriod(); 723 default: return super.makeProperty(hash, name); 724 } 725 726 } 727 728 @Override 729 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 730 switch (hash) { 731 case -887328209: /*system*/ return new String[] {"code"}; 732 case 111972721: /*value*/ return new String[] {"string"}; 733 case 116103: /*use*/ return new String[] {"code"}; 734 case 3492908: /*rank*/ return new String[] {"positiveInt"}; 735 case -991726143: /*period*/ return new String[] {"Period"}; 736 default: return super.getTypesForProperty(hash, name); 737 } 738 739 } 740 741 @Override 742 public Base addChild(String name) throws FHIRException { 743 if (name.equals("system")) { 744 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.system"); 745 } 746 else if (name.equals("value")) { 747 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.value"); 748 } 749 else if (name.equals("use")) { 750 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.use"); 751 } 752 else if (name.equals("rank")) { 753 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.rank"); 754 } 755 else if (name.equals("period")) { 756 this.period = new Period(); 757 return this.period; 758 } 759 else 760 return super.addChild(name); 761 } 762 763 public String fhirType() { 764 return "ContactPoint"; 765 766 } 767 768 public ContactPoint copy() { 769 ContactPoint dst = new ContactPoint(); 770 copyValues(dst); 771 dst.system = system == null ? null : system.copy(); 772 dst.value = value == null ? null : value.copy(); 773 dst.use = use == null ? null : use.copy(); 774 dst.rank = rank == null ? null : rank.copy(); 775 dst.period = period == null ? null : period.copy(); 776 return dst; 777 } 778 779 protected ContactPoint typedCopy() { 780 return copy(); 781 } 782 783 @Override 784 public boolean equalsDeep(Base other_) { 785 if (!super.equalsDeep(other_)) 786 return false; 787 if (!(other_ instanceof ContactPoint)) 788 return false; 789 ContactPoint o = (ContactPoint) other_; 790 return compareDeep(system, o.system, true) && compareDeep(value, o.value, true) && compareDeep(use, o.use, true) 791 && compareDeep(rank, o.rank, true) && compareDeep(period, o.period, true); 792 } 793 794 @Override 795 public boolean equalsShallow(Base other_) { 796 if (!super.equalsShallow(other_)) 797 return false; 798 if (!(other_ instanceof ContactPoint)) 799 return false; 800 ContactPoint o = (ContactPoint) other_; 801 return compareValues(system, o.system, true) && compareValues(value, o.value, true) && compareValues(use, o.use, true) 802 && compareValues(rank, o.rank, true); 803 } 804 805 public boolean isEmpty() { 806 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, value, use, rank 807 , period); 808 } 809 810 811}