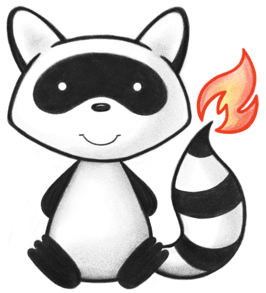
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006import java.math.BigDecimal; 007 008/* 009 Copyright (c) 2011+, HL7, Inc. 010 All rights reserved. 011 012 Redistribution and use in source and binary forms, with or without modification, 013 are permitted provided that the following conditions are met: 014 015 * Redistributions of source code must retain the above copyright notice, this 016 list of conditions and the following disclaimer. 017 * Redistributions in binary form must reproduce the above copyright notice, 018 this list of conditions and the following disclaimer in the documentation 019 and/or other materials provided with the distribution. 020 * Neither the name of HL7 nor the names of its contributors may be used to 021 endorse or promote products derived from this software without specific 022 prior written permission. 023 024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 025 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 027 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 028 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 029 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 030 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 031 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 032 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 033 POSSIBILITY OF SUCH DAMAGE. 034 035*/ 036 037// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 038import java.util.ArrayList; 039import java.util.Date; 040import java.util.List; 041 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.exceptions.FHIRFormatError; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 054 */ 055@ResourceDef(name="Contract", profile="http://hl7.org/fhir/Profile/Contract") 056public class Contract extends DomainResource { 057 058 public enum ContractStatus { 059 /** 060 * Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced. 061 */ 062 AMENDED, 063 /** 064 * Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced. 065 */ 066 APPENDED, 067 /** 068 * Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted. 069 */ 070 CANCELLED, 071 /** 072 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7.Comparable FHIR and v.3 status codes: on hold; pended; suspended. 073 */ 074 DISPUTED, 075 /** 076 * Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status. 077 */ 078 ENTEREDINERROR, 079 /** 080 * Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active. 081 */ 082 EXECUTABLE, 083 /** 084 * Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed. 085 */ 086 EXECUTED, 087 /** 088 * Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 089 */ 090 NEGOTIABLE, 091 /** 092 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template','form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new. 093 */ 094 OFFERED, 095 /** 096 * Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended. 097 */ 098 POLICY, 099 /** 100 * Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled. 101 */ 102 REJECTED, 103 /** 104 * Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded. 105 */ 106 RENEWED, 107 /** 108 * A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified. 109 */ 110 REVOKED, 111 /** 112 * Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated. 113 */ 114 RESOLVED, 115 /** 116 * Contract reaches its expiry date. It may or may not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted. 117 */ 118 TERMINATED, 119 /** 120 * added to help the parsers with the generic types 121 */ 122 NULL; 123 public static ContractStatus fromCode(String codeString) throws FHIRException { 124 if (codeString == null || "".equals(codeString)) 125 return null; 126 if ("amended".equals(codeString)) 127 return AMENDED; 128 if ("appended".equals(codeString)) 129 return APPENDED; 130 if ("cancelled".equals(codeString)) 131 return CANCELLED; 132 if ("disputed".equals(codeString)) 133 return DISPUTED; 134 if ("entered-in-error".equals(codeString)) 135 return ENTEREDINERROR; 136 if ("executable".equals(codeString)) 137 return EXECUTABLE; 138 if ("executed".equals(codeString)) 139 return EXECUTED; 140 if ("negotiable".equals(codeString)) 141 return NEGOTIABLE; 142 if ("offered".equals(codeString)) 143 return OFFERED; 144 if ("policy".equals(codeString)) 145 return POLICY; 146 if ("rejected".equals(codeString)) 147 return REJECTED; 148 if ("renewed".equals(codeString)) 149 return RENEWED; 150 if ("revoked".equals(codeString)) 151 return REVOKED; 152 if ("resolved".equals(codeString)) 153 return RESOLVED; 154 if ("terminated".equals(codeString)) 155 return TERMINATED; 156 if (Configuration.isAcceptInvalidEnums()) 157 return null; 158 else 159 throw new FHIRException("Unknown ContractStatus code '"+codeString+"'"); 160 } 161 public String toCode() { 162 switch (this) { 163 case AMENDED: return "amended"; 164 case APPENDED: return "appended"; 165 case CANCELLED: return "cancelled"; 166 case DISPUTED: return "disputed"; 167 case ENTEREDINERROR: return "entered-in-error"; 168 case EXECUTABLE: return "executable"; 169 case EXECUTED: return "executed"; 170 case NEGOTIABLE: return "negotiable"; 171 case OFFERED: return "offered"; 172 case POLICY: return "policy"; 173 case REJECTED: return "rejected"; 174 case RENEWED: return "renewed"; 175 case REVOKED: return "revoked"; 176 case RESOLVED: return "resolved"; 177 case TERMINATED: return "terminated"; 178 case NULL: return null; 179 default: return "?"; 180 } 181 } 182 public String getSystem() { 183 switch (this) { 184 case AMENDED: return "http://hl7.org/fhir/contract-status"; 185 case APPENDED: return "http://hl7.org/fhir/contract-status"; 186 case CANCELLED: return "http://hl7.org/fhir/contract-status"; 187 case DISPUTED: return "http://hl7.org/fhir/contract-status"; 188 case ENTEREDINERROR: return "http://hl7.org/fhir/contract-status"; 189 case EXECUTABLE: return "http://hl7.org/fhir/contract-status"; 190 case EXECUTED: return "http://hl7.org/fhir/contract-status"; 191 case NEGOTIABLE: return "http://hl7.org/fhir/contract-status"; 192 case OFFERED: return "http://hl7.org/fhir/contract-status"; 193 case POLICY: return "http://hl7.org/fhir/contract-status"; 194 case REJECTED: return "http://hl7.org/fhir/contract-status"; 195 case RENEWED: return "http://hl7.org/fhir/contract-status"; 196 case REVOKED: return "http://hl7.org/fhir/contract-status"; 197 case RESOLVED: return "http://hl7.org/fhir/contract-status"; 198 case TERMINATED: return "http://hl7.org/fhir/contract-status"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getDefinition() { 204 switch (this) { 205 case AMENDED: return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 206 case APPENDED: return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 207 case CANCELLED: return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 208 case DISPUTED: return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7.Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 209 case ENTEREDINERROR: return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 210 case EXECUTABLE: return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 211 case EXECUTED: return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 212 case NEGOTIABLE: return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 213 case OFFERED: return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template','form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 214 case POLICY: return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 215 case REJECTED: return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 216 case RENEWED: return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 217 case REVOKED: return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 218 case RESOLVED: return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 219 case TERMINATED: return "Contract reaches its expiry date. It may or may not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 220 case NULL: return null; 221 default: return "?"; 222 } 223 } 224 public String getDisplay() { 225 switch (this) { 226 case AMENDED: return "Amended"; 227 case APPENDED: return "Appended"; 228 case CANCELLED: return "Cancelled"; 229 case DISPUTED: return "Disputed"; 230 case ENTEREDINERROR: return "Entered in Error"; 231 case EXECUTABLE: return "Executable"; 232 case EXECUTED: return "Executed"; 233 case NEGOTIABLE: return "Negotiable"; 234 case OFFERED: return "Offered"; 235 case POLICY: return "Policy"; 236 case REJECTED: return "Rejected"; 237 case RENEWED: return "Renewed"; 238 case REVOKED: return "Revoked"; 239 case RESOLVED: return "Resolved"; 240 case TERMINATED: return "Terminated"; 241 case NULL: return null; 242 default: return "?"; 243 } 244 } 245 } 246 247 public static class ContractStatusEnumFactory implements EnumFactory<ContractStatus> { 248 public ContractStatus fromCode(String codeString) throws IllegalArgumentException { 249 if (codeString == null || "".equals(codeString)) 250 if (codeString == null || "".equals(codeString)) 251 return null; 252 if ("amended".equals(codeString)) 253 return ContractStatus.AMENDED; 254 if ("appended".equals(codeString)) 255 return ContractStatus.APPENDED; 256 if ("cancelled".equals(codeString)) 257 return ContractStatus.CANCELLED; 258 if ("disputed".equals(codeString)) 259 return ContractStatus.DISPUTED; 260 if ("entered-in-error".equals(codeString)) 261 return ContractStatus.ENTEREDINERROR; 262 if ("executable".equals(codeString)) 263 return ContractStatus.EXECUTABLE; 264 if ("executed".equals(codeString)) 265 return ContractStatus.EXECUTED; 266 if ("negotiable".equals(codeString)) 267 return ContractStatus.NEGOTIABLE; 268 if ("offered".equals(codeString)) 269 return ContractStatus.OFFERED; 270 if ("policy".equals(codeString)) 271 return ContractStatus.POLICY; 272 if ("rejected".equals(codeString)) 273 return ContractStatus.REJECTED; 274 if ("renewed".equals(codeString)) 275 return ContractStatus.RENEWED; 276 if ("revoked".equals(codeString)) 277 return ContractStatus.REVOKED; 278 if ("resolved".equals(codeString)) 279 return ContractStatus.RESOLVED; 280 if ("terminated".equals(codeString)) 281 return ContractStatus.TERMINATED; 282 throw new IllegalArgumentException("Unknown ContractStatus code '"+codeString+"'"); 283 } 284 public Enumeration<ContractStatus> fromType(PrimitiveType<?> code) throws FHIRException { 285 if (code == null) 286 return null; 287 if (code.isEmpty()) 288 return new Enumeration<ContractStatus>(this); 289 String codeString = code.asStringValue(); 290 if (codeString == null || "".equals(codeString)) 291 return null; 292 if ("amended".equals(codeString)) 293 return new Enumeration<ContractStatus>(this, ContractStatus.AMENDED); 294 if ("appended".equals(codeString)) 295 return new Enumeration<ContractStatus>(this, ContractStatus.APPENDED); 296 if ("cancelled".equals(codeString)) 297 return new Enumeration<ContractStatus>(this, ContractStatus.CANCELLED); 298 if ("disputed".equals(codeString)) 299 return new Enumeration<ContractStatus>(this, ContractStatus.DISPUTED); 300 if ("entered-in-error".equals(codeString)) 301 return new Enumeration<ContractStatus>(this, ContractStatus.ENTEREDINERROR); 302 if ("executable".equals(codeString)) 303 return new Enumeration<ContractStatus>(this, ContractStatus.EXECUTABLE); 304 if ("executed".equals(codeString)) 305 return new Enumeration<ContractStatus>(this, ContractStatus.EXECUTED); 306 if ("negotiable".equals(codeString)) 307 return new Enumeration<ContractStatus>(this, ContractStatus.NEGOTIABLE); 308 if ("offered".equals(codeString)) 309 return new Enumeration<ContractStatus>(this, ContractStatus.OFFERED); 310 if ("policy".equals(codeString)) 311 return new Enumeration<ContractStatus>(this, ContractStatus.POLICY); 312 if ("rejected".equals(codeString)) 313 return new Enumeration<ContractStatus>(this, ContractStatus.REJECTED); 314 if ("renewed".equals(codeString)) 315 return new Enumeration<ContractStatus>(this, ContractStatus.RENEWED); 316 if ("revoked".equals(codeString)) 317 return new Enumeration<ContractStatus>(this, ContractStatus.REVOKED); 318 if ("resolved".equals(codeString)) 319 return new Enumeration<ContractStatus>(this, ContractStatus.RESOLVED); 320 if ("terminated".equals(codeString)) 321 return new Enumeration<ContractStatus>(this, ContractStatus.TERMINATED); 322 throw new FHIRException("Unknown ContractStatus code '"+codeString+"'"); 323 } 324 public String toCode(ContractStatus code) { 325 if (code == ContractStatus.NULL) 326 return null; 327 if (code == ContractStatus.AMENDED) 328 return "amended"; 329 if (code == ContractStatus.APPENDED) 330 return "appended"; 331 if (code == ContractStatus.CANCELLED) 332 return "cancelled"; 333 if (code == ContractStatus.DISPUTED) 334 return "disputed"; 335 if (code == ContractStatus.ENTEREDINERROR) 336 return "entered-in-error"; 337 if (code == ContractStatus.EXECUTABLE) 338 return "executable"; 339 if (code == ContractStatus.EXECUTED) 340 return "executed"; 341 if (code == ContractStatus.NEGOTIABLE) 342 return "negotiable"; 343 if (code == ContractStatus.OFFERED) 344 return "offered"; 345 if (code == ContractStatus.POLICY) 346 return "policy"; 347 if (code == ContractStatus.REJECTED) 348 return "rejected"; 349 if (code == ContractStatus.RENEWED) 350 return "renewed"; 351 if (code == ContractStatus.REVOKED) 352 return "revoked"; 353 if (code == ContractStatus.RESOLVED) 354 return "resolved"; 355 if (code == ContractStatus.TERMINATED) 356 return "terminated"; 357 return "?"; 358 } 359 public String toSystem(ContractStatus code) { 360 return code.getSystem(); 361 } 362 } 363 364 @Block() 365 public static class AgentComponent extends BackboneElement implements IBaseBackboneElement { 366 /** 367 * Who or what parties are assigned roles in this Contract. 368 */ 369 @Child(name = "actor", type = {Contract.class, Device.class, Group.class, Location.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, Substance.class}, order=1, min=1, max=1, modifier=false, summary=false) 370 @Description(shortDefinition="Contract Agent Type", formalDefinition="Who or what parties are assigned roles in this Contract." ) 371 protected Reference actor; 372 373 /** 374 * The actual object that is the target of the reference (Who or what parties are assigned roles in this Contract.) 375 */ 376 protected Resource actorTarget; 377 378 /** 379 * Role type of agent assigned roles in this Contract. 380 */ 381 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 382 @Description(shortDefinition="Role type of the agent", formalDefinition="Role type of agent assigned roles in this Contract." ) 383 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-actorrole") 384 protected List<CodeableConcept> role; 385 386 private static final long serialVersionUID = -454551165L; 387 388 /** 389 * Constructor 390 */ 391 public AgentComponent() { 392 super(); 393 } 394 395 /** 396 * Constructor 397 */ 398 public AgentComponent(Reference actor) { 399 super(); 400 this.actor = actor; 401 } 402 403 /** 404 * @return {@link #actor} (Who or what parties are assigned roles in this Contract.) 405 */ 406 public Reference getActor() { 407 if (this.actor == null) 408 if (Configuration.errorOnAutoCreate()) 409 throw new Error("Attempt to auto-create AgentComponent.actor"); 410 else if (Configuration.doAutoCreate()) 411 this.actor = new Reference(); // cc 412 return this.actor; 413 } 414 415 public boolean hasActor() { 416 return this.actor != null && !this.actor.isEmpty(); 417 } 418 419 /** 420 * @param value {@link #actor} (Who or what parties are assigned roles in this Contract.) 421 */ 422 public AgentComponent setActor(Reference value) { 423 this.actor = value; 424 return this; 425 } 426 427 /** 428 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Who or what parties are assigned roles in this Contract.) 429 */ 430 public Resource getActorTarget() { 431 return this.actorTarget; 432 } 433 434 /** 435 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Who or what parties are assigned roles in this Contract.) 436 */ 437 public AgentComponent setActorTarget(Resource value) { 438 this.actorTarget = value; 439 return this; 440 } 441 442 /** 443 * @return {@link #role} (Role type of agent assigned roles in this Contract.) 444 */ 445 public List<CodeableConcept> getRole() { 446 if (this.role == null) 447 this.role = new ArrayList<CodeableConcept>(); 448 return this.role; 449 } 450 451 /** 452 * @return Returns a reference to <code>this</code> for easy method chaining 453 */ 454 public AgentComponent setRole(List<CodeableConcept> theRole) { 455 this.role = theRole; 456 return this; 457 } 458 459 public boolean hasRole() { 460 if (this.role == null) 461 return false; 462 for (CodeableConcept item : this.role) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 public CodeableConcept addRole() { //3 469 CodeableConcept t = new CodeableConcept(); 470 if (this.role == null) 471 this.role = new ArrayList<CodeableConcept>(); 472 this.role.add(t); 473 return t; 474 } 475 476 public AgentComponent addRole(CodeableConcept t) { //3 477 if (t == null) 478 return this; 479 if (this.role == null) 480 this.role = new ArrayList<CodeableConcept>(); 481 this.role.add(t); 482 return this; 483 } 484 485 /** 486 * @return The first repetition of repeating field {@link #role}, creating it if it does not already exist 487 */ 488 public CodeableConcept getRoleFirstRep() { 489 if (getRole().isEmpty()) { 490 addRole(); 491 } 492 return getRole().get(0); 493 } 494 495 protected void listChildren(List<Property> children) { 496 super.listChildren(children); 497 children.add(new Property("actor", "Reference(Contract|Device|Group|Location|Organization|Patient|Practitioner|RelatedPerson|Substance)", "Who or what parties are assigned roles in this Contract.", 0, 1, actor)); 498 children.add(new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 0, java.lang.Integer.MAX_VALUE, role)); 499 } 500 501 @Override 502 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 503 switch (_hash) { 504 case 92645877: /*actor*/ return new Property("actor", "Reference(Contract|Device|Group|Location|Organization|Patient|Practitioner|RelatedPerson|Substance)", "Who or what parties are assigned roles in this Contract.", 0, 1, actor); 505 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 0, java.lang.Integer.MAX_VALUE, role); 506 default: return super.getNamedProperty(_hash, _name, _checkValid); 507 } 508 509 } 510 511 @Override 512 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 513 switch (hash) { 514 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 515 case 3506294: /*role*/ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 516 default: return super.getProperty(hash, name, checkValid); 517 } 518 519 } 520 521 @Override 522 public Base setProperty(int hash, String name, Base value) throws FHIRException { 523 switch (hash) { 524 case 92645877: // actor 525 this.actor = castToReference(value); // Reference 526 return value; 527 case 3506294: // role 528 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 529 return value; 530 default: return super.setProperty(hash, name, value); 531 } 532 533 } 534 535 @Override 536 public Base setProperty(String name, Base value) throws FHIRException { 537 if (name.equals("actor")) { 538 this.actor = castToReference(value); // Reference 539 } else if (name.equals("role")) { 540 this.getRole().add(castToCodeableConcept(value)); 541 } else 542 return super.setProperty(name, value); 543 return value; 544 } 545 546 @Override 547 public Base makeProperty(int hash, String name) throws FHIRException { 548 switch (hash) { 549 case 92645877: return getActor(); 550 case 3506294: return addRole(); 551 default: return super.makeProperty(hash, name); 552 } 553 554 } 555 556 @Override 557 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 558 switch (hash) { 559 case 92645877: /*actor*/ return new String[] {"Reference"}; 560 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 561 default: return super.getTypesForProperty(hash, name); 562 } 563 564 } 565 566 @Override 567 public Base addChild(String name) throws FHIRException { 568 if (name.equals("actor")) { 569 this.actor = new Reference(); 570 return this.actor; 571 } 572 else if (name.equals("role")) { 573 return addRole(); 574 } 575 else 576 return super.addChild(name); 577 } 578 579 public AgentComponent copy() { 580 AgentComponent dst = new AgentComponent(); 581 copyValues(dst); 582 dst.actor = actor == null ? null : actor.copy(); 583 if (role != null) { 584 dst.role = new ArrayList<CodeableConcept>(); 585 for (CodeableConcept i : role) 586 dst.role.add(i.copy()); 587 }; 588 return dst; 589 } 590 591 @Override 592 public boolean equalsDeep(Base other_) { 593 if (!super.equalsDeep(other_)) 594 return false; 595 if (!(other_ instanceof AgentComponent)) 596 return false; 597 AgentComponent o = (AgentComponent) other_; 598 return compareDeep(actor, o.actor, true) && compareDeep(role, o.role, true); 599 } 600 601 @Override 602 public boolean equalsShallow(Base other_) { 603 if (!super.equalsShallow(other_)) 604 return false; 605 if (!(other_ instanceof AgentComponent)) 606 return false; 607 AgentComponent o = (AgentComponent) other_; 608 return true; 609 } 610 611 public boolean isEmpty() { 612 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actor, role); 613 } 614 615 public String fhirType() { 616 return "Contract.agent"; 617 618 } 619 620 } 621 622 @Block() 623 public static class SignatoryComponent extends BackboneElement implements IBaseBackboneElement { 624 /** 625 * Role of this Contract signer, e.g. notary, grantee. 626 */ 627 @Child(name = "type", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 628 @Description(shortDefinition="Contract Signatory Role", formalDefinition="Role of this Contract signer, e.g. notary, grantee." ) 629 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-signer-type") 630 protected Coding type; 631 632 /** 633 * Party which is a signator to this Contract. 634 */ 635 @Child(name = "party", type = {Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 636 @Description(shortDefinition="Contract Signatory Party", formalDefinition="Party which is a signator to this Contract." ) 637 protected Reference party; 638 639 /** 640 * The actual object that is the target of the reference (Party which is a signator to this Contract.) 641 */ 642 protected Resource partyTarget; 643 644 /** 645 * Legally binding Contract DSIG signature contents in Base64. 646 */ 647 @Child(name = "signature", type = {Signature.class}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 648 @Description(shortDefinition="Contract Documentation Signature", formalDefinition="Legally binding Contract DSIG signature contents in Base64." ) 649 protected List<Signature> signature; 650 651 private static final long serialVersionUID = 1948139228L; 652 653 /** 654 * Constructor 655 */ 656 public SignatoryComponent() { 657 super(); 658 } 659 660 /** 661 * Constructor 662 */ 663 public SignatoryComponent(Coding type, Reference party) { 664 super(); 665 this.type = type; 666 this.party = party; 667 } 668 669 /** 670 * @return {@link #type} (Role of this Contract signer, e.g. notary, grantee.) 671 */ 672 public Coding getType() { 673 if (this.type == null) 674 if (Configuration.errorOnAutoCreate()) 675 throw new Error("Attempt to auto-create SignatoryComponent.type"); 676 else if (Configuration.doAutoCreate()) 677 this.type = new Coding(); // cc 678 return this.type; 679 } 680 681 public boolean hasType() { 682 return this.type != null && !this.type.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #type} (Role of this Contract signer, e.g. notary, grantee.) 687 */ 688 public SignatoryComponent setType(Coding value) { 689 this.type = value; 690 return this; 691 } 692 693 /** 694 * @return {@link #party} (Party which is a signator to this Contract.) 695 */ 696 public Reference getParty() { 697 if (this.party == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create SignatoryComponent.party"); 700 else if (Configuration.doAutoCreate()) 701 this.party = new Reference(); // cc 702 return this.party; 703 } 704 705 public boolean hasParty() { 706 return this.party != null && !this.party.isEmpty(); 707 } 708 709 /** 710 * @param value {@link #party} (Party which is a signator to this Contract.) 711 */ 712 public SignatoryComponent setParty(Reference value) { 713 this.party = value; 714 return this; 715 } 716 717 /** 718 * @return {@link #party} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Party which is a signator to this Contract.) 719 */ 720 public Resource getPartyTarget() { 721 return this.partyTarget; 722 } 723 724 /** 725 * @param value {@link #party} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Party which is a signator to this Contract.) 726 */ 727 public SignatoryComponent setPartyTarget(Resource value) { 728 this.partyTarget = value; 729 return this; 730 } 731 732 /** 733 * @return {@link #signature} (Legally binding Contract DSIG signature contents in Base64.) 734 */ 735 public List<Signature> getSignature() { 736 if (this.signature == null) 737 this.signature = new ArrayList<Signature>(); 738 return this.signature; 739 } 740 741 /** 742 * @return Returns a reference to <code>this</code> for easy method chaining 743 */ 744 public SignatoryComponent setSignature(List<Signature> theSignature) { 745 this.signature = theSignature; 746 return this; 747 } 748 749 public boolean hasSignature() { 750 if (this.signature == null) 751 return false; 752 for (Signature item : this.signature) 753 if (!item.isEmpty()) 754 return true; 755 return false; 756 } 757 758 public Signature addSignature() { //3 759 Signature t = new Signature(); 760 if (this.signature == null) 761 this.signature = new ArrayList<Signature>(); 762 this.signature.add(t); 763 return t; 764 } 765 766 public SignatoryComponent addSignature(Signature t) { //3 767 if (t == null) 768 return this; 769 if (this.signature == null) 770 this.signature = new ArrayList<Signature>(); 771 this.signature.add(t); 772 return this; 773 } 774 775 /** 776 * @return The first repetition of repeating field {@link #signature}, creating it if it does not already exist 777 */ 778 public Signature getSignatureFirstRep() { 779 if (getSignature().isEmpty()) { 780 addSignature(); 781 } 782 return getSignature().get(0); 783 } 784 785 protected void listChildren(List<Property> children) { 786 super.listChildren(children); 787 children.add(new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, type)); 788 children.add(new Property("party", "Reference(Organization|Patient|Practitioner|RelatedPerson)", "Party which is a signator to this Contract.", 0, 1, party)); 789 children.add(new Property("signature", "Signature", "Legally binding Contract DSIG signature contents in Base64.", 0, java.lang.Integer.MAX_VALUE, signature)); 790 } 791 792 @Override 793 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 794 switch (_hash) { 795 case 3575610: /*type*/ return new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, type); 796 case 106437350: /*party*/ return new Property("party", "Reference(Organization|Patient|Practitioner|RelatedPerson)", "Party which is a signator to this Contract.", 0, 1, party); 797 case 1073584312: /*signature*/ return new Property("signature", "Signature", "Legally binding Contract DSIG signature contents in Base64.", 0, java.lang.Integer.MAX_VALUE, signature); 798 default: return super.getNamedProperty(_hash, _name, _checkValid); 799 } 800 801 } 802 803 @Override 804 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 805 switch (hash) { 806 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 807 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 808 case 1073584312: /*signature*/ return this.signature == null ? new Base[0] : this.signature.toArray(new Base[this.signature.size()]); // Signature 809 default: return super.getProperty(hash, name, checkValid); 810 } 811 812 } 813 814 @Override 815 public Base setProperty(int hash, String name, Base value) throws FHIRException { 816 switch (hash) { 817 case 3575610: // type 818 this.type = castToCoding(value); // Coding 819 return value; 820 case 106437350: // party 821 this.party = castToReference(value); // Reference 822 return value; 823 case 1073584312: // signature 824 this.getSignature().add(castToSignature(value)); // Signature 825 return value; 826 default: return super.setProperty(hash, name, value); 827 } 828 829 } 830 831 @Override 832 public Base setProperty(String name, Base value) throws FHIRException { 833 if (name.equals("type")) { 834 this.type = castToCoding(value); // Coding 835 } else if (name.equals("party")) { 836 this.party = castToReference(value); // Reference 837 } else if (name.equals("signature")) { 838 this.getSignature().add(castToSignature(value)); 839 } else 840 return super.setProperty(name, value); 841 return value; 842 } 843 844 @Override 845 public Base makeProperty(int hash, String name) throws FHIRException { 846 switch (hash) { 847 case 3575610: return getType(); 848 case 106437350: return getParty(); 849 case 1073584312: return addSignature(); 850 default: return super.makeProperty(hash, name); 851 } 852 853 } 854 855 @Override 856 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 857 switch (hash) { 858 case 3575610: /*type*/ return new String[] {"Coding"}; 859 case 106437350: /*party*/ return new String[] {"Reference"}; 860 case 1073584312: /*signature*/ return new String[] {"Signature"}; 861 default: return super.getTypesForProperty(hash, name); 862 } 863 864 } 865 866 @Override 867 public Base addChild(String name) throws FHIRException { 868 if (name.equals("type")) { 869 this.type = new Coding(); 870 return this.type; 871 } 872 else if (name.equals("party")) { 873 this.party = new Reference(); 874 return this.party; 875 } 876 else if (name.equals("signature")) { 877 return addSignature(); 878 } 879 else 880 return super.addChild(name); 881 } 882 883 public SignatoryComponent copy() { 884 SignatoryComponent dst = new SignatoryComponent(); 885 copyValues(dst); 886 dst.type = type == null ? null : type.copy(); 887 dst.party = party == null ? null : party.copy(); 888 if (signature != null) { 889 dst.signature = new ArrayList<Signature>(); 890 for (Signature i : signature) 891 dst.signature.add(i.copy()); 892 }; 893 return dst; 894 } 895 896 @Override 897 public boolean equalsDeep(Base other_) { 898 if (!super.equalsDeep(other_)) 899 return false; 900 if (!(other_ instanceof SignatoryComponent)) 901 return false; 902 SignatoryComponent o = (SignatoryComponent) other_; 903 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true) && compareDeep(signature, o.signature, true) 904 ; 905 } 906 907 @Override 908 public boolean equalsShallow(Base other_) { 909 if (!super.equalsShallow(other_)) 910 return false; 911 if (!(other_ instanceof SignatoryComponent)) 912 return false; 913 SignatoryComponent o = (SignatoryComponent) other_; 914 return true; 915 } 916 917 public boolean isEmpty() { 918 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party, signature); 919 } 920 921 public String fhirType() { 922 return "Contract.signer"; 923 924 } 925 926 } 927 928 @Block() 929 public static class ValuedItemComponent extends BackboneElement implements IBaseBackboneElement { 930 /** 931 * Specific type of Contract Valued Item that may be priced. 932 */ 933 @Child(name = "entity", type = {CodeableConcept.class, Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 934 @Description(shortDefinition="Contract Valued Item Type", formalDefinition="Specific type of Contract Valued Item that may be priced." ) 935 protected Type entity; 936 937 /** 938 * Identifies a Contract Valued Item instance. 939 */ 940 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 941 @Description(shortDefinition="Contract Valued Item Number", formalDefinition="Identifies a Contract Valued Item instance." ) 942 protected Identifier identifier; 943 944 /** 945 * Indicates the time during which this Contract ValuedItem information is effective. 946 */ 947 @Child(name = "effectiveTime", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 948 @Description(shortDefinition="Contract Valued Item Effective Tiem", formalDefinition="Indicates the time during which this Contract ValuedItem information is effective." ) 949 protected DateTimeType effectiveTime; 950 951 /** 952 * Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances. 953 */ 954 @Child(name = "quantity", type = {SimpleQuantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 955 @Description(shortDefinition="Count of Contract Valued Items", formalDefinition="Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances." ) 956 protected SimpleQuantity quantity; 957 958 /** 959 * A Contract Valued Item unit valuation measure. 960 */ 961 @Child(name = "unitPrice", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 962 @Description(shortDefinition="Contract Valued Item fee, charge, or cost", formalDefinition="A Contract Valued Item unit valuation measure." ) 963 protected Money unitPrice; 964 965 /** 966 * A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 967 */ 968 @Child(name = "factor", type = {DecimalType.class}, order=6, min=0, max=1, modifier=false, summary=false) 969 @Description(shortDefinition="Contract Valued Item Price Scaling Factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 970 protected DecimalType factor; 971 972 /** 973 * An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 974 */ 975 @Child(name = "points", type = {DecimalType.class}, order=7, min=0, max=1, modifier=false, summary=false) 976 @Description(shortDefinition="Contract Valued Item Difficulty Scaling Factor", formalDefinition="An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point." ) 977 protected DecimalType points; 978 979 /** 980 * Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 981 */ 982 @Child(name = "net", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 983 @Description(shortDefinition="Total Contract Valued Item Value", formalDefinition="Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 984 protected Money net; 985 986 private static final long serialVersionUID = 1782449516L; 987 988 /** 989 * Constructor 990 */ 991 public ValuedItemComponent() { 992 super(); 993 } 994 995 /** 996 * @return {@link #entity} (Specific type of Contract Valued Item that may be priced.) 997 */ 998 public Type getEntity() { 999 return this.entity; 1000 } 1001 1002 /** 1003 * @return {@link #entity} (Specific type of Contract Valued Item that may be priced.) 1004 */ 1005 public CodeableConcept getEntityCodeableConcept() throws FHIRException { 1006 if (this.entity == null) 1007 return null; 1008 if (!(this.entity instanceof CodeableConcept)) 1009 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.entity.getClass().getName()+" was encountered"); 1010 return (CodeableConcept) this.entity; 1011 } 1012 1013 public boolean hasEntityCodeableConcept() { 1014 return this != null && this.entity instanceof CodeableConcept; 1015 } 1016 1017 /** 1018 * @return {@link #entity} (Specific type of Contract Valued Item that may be priced.) 1019 */ 1020 public Reference getEntityReference() throws FHIRException { 1021 if (this.entity == null) 1022 return null; 1023 if (!(this.entity instanceof Reference)) 1024 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.entity.getClass().getName()+" was encountered"); 1025 return (Reference) this.entity; 1026 } 1027 1028 public boolean hasEntityReference() { 1029 return this != null && this.entity instanceof Reference; 1030 } 1031 1032 public boolean hasEntity() { 1033 return this.entity != null && !this.entity.isEmpty(); 1034 } 1035 1036 /** 1037 * @param value {@link #entity} (Specific type of Contract Valued Item that may be priced.) 1038 */ 1039 public ValuedItemComponent setEntity(Type value) throws FHIRFormatError { 1040 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1041 throw new FHIRFormatError("Not the right type for Contract.valuedItem.entity[x]: "+value.fhirType()); 1042 this.entity = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #identifier} (Identifies a Contract Valued Item instance.) 1048 */ 1049 public Identifier getIdentifier() { 1050 if (this.identifier == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create ValuedItemComponent.identifier"); 1053 else if (Configuration.doAutoCreate()) 1054 this.identifier = new Identifier(); // cc 1055 return this.identifier; 1056 } 1057 1058 public boolean hasIdentifier() { 1059 return this.identifier != null && !this.identifier.isEmpty(); 1060 } 1061 1062 /** 1063 * @param value {@link #identifier} (Identifies a Contract Valued Item instance.) 1064 */ 1065 public ValuedItemComponent setIdentifier(Identifier value) { 1066 this.identifier = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #effectiveTime} (Indicates the time during which this Contract ValuedItem information is effective.). This is the underlying object with id, value and extensions. The accessor "getEffectiveTime" gives direct access to the value 1072 */ 1073 public DateTimeType getEffectiveTimeElement() { 1074 if (this.effectiveTime == null) 1075 if (Configuration.errorOnAutoCreate()) 1076 throw new Error("Attempt to auto-create ValuedItemComponent.effectiveTime"); 1077 else if (Configuration.doAutoCreate()) 1078 this.effectiveTime = new DateTimeType(); // bb 1079 return this.effectiveTime; 1080 } 1081 1082 public boolean hasEffectiveTimeElement() { 1083 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 1084 } 1085 1086 public boolean hasEffectiveTime() { 1087 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 1088 } 1089 1090 /** 1091 * @param value {@link #effectiveTime} (Indicates the time during which this Contract ValuedItem information is effective.). This is the underlying object with id, value and extensions. The accessor "getEffectiveTime" gives direct access to the value 1092 */ 1093 public ValuedItemComponent setEffectiveTimeElement(DateTimeType value) { 1094 this.effectiveTime = value; 1095 return this; 1096 } 1097 1098 /** 1099 * @return Indicates the time during which this Contract ValuedItem information is effective. 1100 */ 1101 public Date getEffectiveTime() { 1102 return this.effectiveTime == null ? null : this.effectiveTime.getValue(); 1103 } 1104 1105 /** 1106 * @param value Indicates the time during which this Contract ValuedItem information is effective. 1107 */ 1108 public ValuedItemComponent setEffectiveTime(Date value) { 1109 if (value == null) 1110 this.effectiveTime = null; 1111 else { 1112 if (this.effectiveTime == null) 1113 this.effectiveTime = new DateTimeType(); 1114 this.effectiveTime.setValue(value); 1115 } 1116 return this; 1117 } 1118 1119 /** 1120 * @return {@link #quantity} (Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.) 1121 */ 1122 public SimpleQuantity getQuantity() { 1123 if (this.quantity == null) 1124 if (Configuration.errorOnAutoCreate()) 1125 throw new Error("Attempt to auto-create ValuedItemComponent.quantity"); 1126 else if (Configuration.doAutoCreate()) 1127 this.quantity = new SimpleQuantity(); // cc 1128 return this.quantity; 1129 } 1130 1131 public boolean hasQuantity() { 1132 return this.quantity != null && !this.quantity.isEmpty(); 1133 } 1134 1135 /** 1136 * @param value {@link #quantity} (Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.) 1137 */ 1138 public ValuedItemComponent setQuantity(SimpleQuantity value) { 1139 this.quantity = value; 1140 return this; 1141 } 1142 1143 /** 1144 * @return {@link #unitPrice} (A Contract Valued Item unit valuation measure.) 1145 */ 1146 public Money getUnitPrice() { 1147 if (this.unitPrice == null) 1148 if (Configuration.errorOnAutoCreate()) 1149 throw new Error("Attempt to auto-create ValuedItemComponent.unitPrice"); 1150 else if (Configuration.doAutoCreate()) 1151 this.unitPrice = new Money(); // cc 1152 return this.unitPrice; 1153 } 1154 1155 public boolean hasUnitPrice() { 1156 return this.unitPrice != null && !this.unitPrice.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #unitPrice} (A Contract Valued Item unit valuation measure.) 1161 */ 1162 public ValuedItemComponent setUnitPrice(Money value) { 1163 this.unitPrice = value; 1164 return this; 1165 } 1166 1167 /** 1168 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 1169 */ 1170 public DecimalType getFactorElement() { 1171 if (this.factor == null) 1172 if (Configuration.errorOnAutoCreate()) 1173 throw new Error("Attempt to auto-create ValuedItemComponent.factor"); 1174 else if (Configuration.doAutoCreate()) 1175 this.factor = new DecimalType(); // bb 1176 return this.factor; 1177 } 1178 1179 public boolean hasFactorElement() { 1180 return this.factor != null && !this.factor.isEmpty(); 1181 } 1182 1183 public boolean hasFactor() { 1184 return this.factor != null && !this.factor.isEmpty(); 1185 } 1186 1187 /** 1188 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 1189 */ 1190 public ValuedItemComponent setFactorElement(DecimalType value) { 1191 this.factor = value; 1192 return this; 1193 } 1194 1195 /** 1196 * @return A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 1197 */ 1198 public BigDecimal getFactor() { 1199 return this.factor == null ? null : this.factor.getValue(); 1200 } 1201 1202 /** 1203 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 1204 */ 1205 public ValuedItemComponent setFactor(BigDecimal value) { 1206 if (value == null) 1207 this.factor = null; 1208 else { 1209 if (this.factor == null) 1210 this.factor = new DecimalType(); 1211 this.factor.setValue(value); 1212 } 1213 return this; 1214 } 1215 1216 /** 1217 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 1218 */ 1219 public ValuedItemComponent setFactor(long value) { 1220 this.factor = new DecimalType(); 1221 this.factor.setValue(value); 1222 return this; 1223 } 1224 1225 /** 1226 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 1227 */ 1228 public ValuedItemComponent setFactor(double value) { 1229 this.factor = new DecimalType(); 1230 this.factor.setValue(value); 1231 return this; 1232 } 1233 1234 /** 1235 * @return {@link #points} (An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.). This is the underlying object with id, value and extensions. The accessor "getPoints" gives direct access to the value 1236 */ 1237 public DecimalType getPointsElement() { 1238 if (this.points == null) 1239 if (Configuration.errorOnAutoCreate()) 1240 throw new Error("Attempt to auto-create ValuedItemComponent.points"); 1241 else if (Configuration.doAutoCreate()) 1242 this.points = new DecimalType(); // bb 1243 return this.points; 1244 } 1245 1246 public boolean hasPointsElement() { 1247 return this.points != null && !this.points.isEmpty(); 1248 } 1249 1250 public boolean hasPoints() { 1251 return this.points != null && !this.points.isEmpty(); 1252 } 1253 1254 /** 1255 * @param value {@link #points} (An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.). This is the underlying object with id, value and extensions. The accessor "getPoints" gives direct access to the value 1256 */ 1257 public ValuedItemComponent setPointsElement(DecimalType value) { 1258 this.points = value; 1259 return this; 1260 } 1261 1262 /** 1263 * @return An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 1264 */ 1265 public BigDecimal getPoints() { 1266 return this.points == null ? null : this.points.getValue(); 1267 } 1268 1269 /** 1270 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 1271 */ 1272 public ValuedItemComponent setPoints(BigDecimal value) { 1273 if (value == null) 1274 this.points = null; 1275 else { 1276 if (this.points == null) 1277 this.points = new DecimalType(); 1278 this.points.setValue(value); 1279 } 1280 return this; 1281 } 1282 1283 /** 1284 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 1285 */ 1286 public ValuedItemComponent setPoints(long value) { 1287 this.points = new DecimalType(); 1288 this.points.setValue(value); 1289 return this; 1290 } 1291 1292 /** 1293 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 1294 */ 1295 public ValuedItemComponent setPoints(double value) { 1296 this.points = new DecimalType(); 1297 this.points.setValue(value); 1298 return this; 1299 } 1300 1301 /** 1302 * @return {@link #net} (Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 1303 */ 1304 public Money getNet() { 1305 if (this.net == null) 1306 if (Configuration.errorOnAutoCreate()) 1307 throw new Error("Attempt to auto-create ValuedItemComponent.net"); 1308 else if (Configuration.doAutoCreate()) 1309 this.net = new Money(); // cc 1310 return this.net; 1311 } 1312 1313 public boolean hasNet() { 1314 return this.net != null && !this.net.isEmpty(); 1315 } 1316 1317 /** 1318 * @param value {@link #net} (Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 1319 */ 1320 public ValuedItemComponent setNet(Money value) { 1321 this.net = value; 1322 return this; 1323 } 1324 1325 protected void listChildren(List<Property> children) { 1326 super.listChildren(children); 1327 children.add(new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity)); 1328 children.add(new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 0, 1, identifier)); 1329 children.add(new Property("effectiveTime", "dateTime", "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime)); 1330 children.add(new Property("quantity", "SimpleQuantity", "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 0, 1, quantity)); 1331 children.add(new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 1, unitPrice)); 1332 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 1333 children.add(new Property("points", "decimal", "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 0, 1, points)); 1334 children.add(new Property("net", "Money", "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 1335 } 1336 1337 @Override 1338 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1339 switch (_hash) { 1340 case -740568643: /*entity[x]*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 1341 case -1298275357: /*entity*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 1342 case 924197182: /*entityCodeableConcept*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 1343 case -356635992: /*entityReference*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 1344 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 0, 1, identifier); 1345 case -929905388: /*effectiveTime*/ return new Property("effectiveTime", "dateTime", "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime); 1346 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 0, 1, quantity); 1347 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 1, unitPrice); 1348 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 1349 case -982754077: /*points*/ return new Property("points", "decimal", "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 0, 1, points); 1350 case 108957: /*net*/ return new Property("net", "Money", "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 1351 default: return super.getNamedProperty(_hash, _name, _checkValid); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1358 switch (hash) { 1359 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : new Base[] {this.entity}; // Type 1360 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1361 case -929905388: /*effectiveTime*/ return this.effectiveTime == null ? new Base[0] : new Base[] {this.effectiveTime}; // DateTimeType 1362 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 1363 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 1364 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 1365 case -982754077: /*points*/ return this.points == null ? new Base[0] : new Base[] {this.points}; // DecimalType 1366 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 1367 default: return super.getProperty(hash, name, checkValid); 1368 } 1369 1370 } 1371 1372 @Override 1373 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1374 switch (hash) { 1375 case -1298275357: // entity 1376 this.entity = castToType(value); // Type 1377 return value; 1378 case -1618432855: // identifier 1379 this.identifier = castToIdentifier(value); // Identifier 1380 return value; 1381 case -929905388: // effectiveTime 1382 this.effectiveTime = castToDateTime(value); // DateTimeType 1383 return value; 1384 case -1285004149: // quantity 1385 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1386 return value; 1387 case -486196699: // unitPrice 1388 this.unitPrice = castToMoney(value); // Money 1389 return value; 1390 case -1282148017: // factor 1391 this.factor = castToDecimal(value); // DecimalType 1392 return value; 1393 case -982754077: // points 1394 this.points = castToDecimal(value); // DecimalType 1395 return value; 1396 case 108957: // net 1397 this.net = castToMoney(value); // Money 1398 return value; 1399 default: return super.setProperty(hash, name, value); 1400 } 1401 1402 } 1403 1404 @Override 1405 public Base setProperty(String name, Base value) throws FHIRException { 1406 if (name.equals("entity[x]")) { 1407 this.entity = castToType(value); // Type 1408 } else if (name.equals("identifier")) { 1409 this.identifier = castToIdentifier(value); // Identifier 1410 } else if (name.equals("effectiveTime")) { 1411 this.effectiveTime = castToDateTime(value); // DateTimeType 1412 } else if (name.equals("quantity")) { 1413 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1414 } else if (name.equals("unitPrice")) { 1415 this.unitPrice = castToMoney(value); // Money 1416 } else if (name.equals("factor")) { 1417 this.factor = castToDecimal(value); // DecimalType 1418 } else if (name.equals("points")) { 1419 this.points = castToDecimal(value); // DecimalType 1420 } else if (name.equals("net")) { 1421 this.net = castToMoney(value); // Money 1422 } else 1423 return super.setProperty(name, value); 1424 return value; 1425 } 1426 1427 @Override 1428 public Base makeProperty(int hash, String name) throws FHIRException { 1429 switch (hash) { 1430 case -740568643: return getEntity(); 1431 case -1298275357: return getEntity(); 1432 case -1618432855: return getIdentifier(); 1433 case -929905388: return getEffectiveTimeElement(); 1434 case -1285004149: return getQuantity(); 1435 case -486196699: return getUnitPrice(); 1436 case -1282148017: return getFactorElement(); 1437 case -982754077: return getPointsElement(); 1438 case 108957: return getNet(); 1439 default: return super.makeProperty(hash, name); 1440 } 1441 1442 } 1443 1444 @Override 1445 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1446 switch (hash) { 1447 case -1298275357: /*entity*/ return new String[] {"CodeableConcept", "Reference"}; 1448 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1449 case -929905388: /*effectiveTime*/ return new String[] {"dateTime"}; 1450 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 1451 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 1452 case -1282148017: /*factor*/ return new String[] {"decimal"}; 1453 case -982754077: /*points*/ return new String[] {"decimal"}; 1454 case 108957: /*net*/ return new String[] {"Money"}; 1455 default: return super.getTypesForProperty(hash, name); 1456 } 1457 1458 } 1459 1460 @Override 1461 public Base addChild(String name) throws FHIRException { 1462 if (name.equals("entityCodeableConcept")) { 1463 this.entity = new CodeableConcept(); 1464 return this.entity; 1465 } 1466 else if (name.equals("entityReference")) { 1467 this.entity = new Reference(); 1468 return this.entity; 1469 } 1470 else if (name.equals("identifier")) { 1471 this.identifier = new Identifier(); 1472 return this.identifier; 1473 } 1474 else if (name.equals("effectiveTime")) { 1475 throw new FHIRException("Cannot call addChild on a singleton property Contract.effectiveTime"); 1476 } 1477 else if (name.equals("quantity")) { 1478 this.quantity = new SimpleQuantity(); 1479 return this.quantity; 1480 } 1481 else if (name.equals("unitPrice")) { 1482 this.unitPrice = new Money(); 1483 return this.unitPrice; 1484 } 1485 else if (name.equals("factor")) { 1486 throw new FHIRException("Cannot call addChild on a singleton property Contract.factor"); 1487 } 1488 else if (name.equals("points")) { 1489 throw new FHIRException("Cannot call addChild on a singleton property Contract.points"); 1490 } 1491 else if (name.equals("net")) { 1492 this.net = new Money(); 1493 return this.net; 1494 } 1495 else 1496 return super.addChild(name); 1497 } 1498 1499 public ValuedItemComponent copy() { 1500 ValuedItemComponent dst = new ValuedItemComponent(); 1501 copyValues(dst); 1502 dst.entity = entity == null ? null : entity.copy(); 1503 dst.identifier = identifier == null ? null : identifier.copy(); 1504 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 1505 dst.quantity = quantity == null ? null : quantity.copy(); 1506 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 1507 dst.factor = factor == null ? null : factor.copy(); 1508 dst.points = points == null ? null : points.copy(); 1509 dst.net = net == null ? null : net.copy(); 1510 return dst; 1511 } 1512 1513 @Override 1514 public boolean equalsDeep(Base other_) { 1515 if (!super.equalsDeep(other_)) 1516 return false; 1517 if (!(other_ instanceof ValuedItemComponent)) 1518 return false; 1519 ValuedItemComponent o = (ValuedItemComponent) other_; 1520 return compareDeep(entity, o.entity, true) && compareDeep(identifier, o.identifier, true) && compareDeep(effectiveTime, o.effectiveTime, true) 1521 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 1522 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true); 1523 } 1524 1525 @Override 1526 public boolean equalsShallow(Base other_) { 1527 if (!super.equalsShallow(other_)) 1528 return false; 1529 if (!(other_ instanceof ValuedItemComponent)) 1530 return false; 1531 ValuedItemComponent o = (ValuedItemComponent) other_; 1532 return compareValues(effectiveTime, o.effectiveTime, true) && compareValues(factor, o.factor, true) 1533 && compareValues(points, o.points, true); 1534 } 1535 1536 public boolean isEmpty() { 1537 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, identifier, effectiveTime 1538 , quantity, unitPrice, factor, points, net); 1539 } 1540 1541 public String fhirType() { 1542 return "Contract.valuedItem"; 1543 1544 } 1545 1546 } 1547 1548 @Block() 1549 public static class TermComponent extends BackboneElement implements IBaseBackboneElement { 1550 /** 1551 * Unique identifier for this particular Contract Provision. 1552 */ 1553 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1554 @Description(shortDefinition="Contract Term Number", formalDefinition="Unique identifier for this particular Contract Provision." ) 1555 protected Identifier identifier; 1556 1557 /** 1558 * When this Contract Provision was issued. 1559 */ 1560 @Child(name = "issued", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1561 @Description(shortDefinition="Contract Term Issue Date Time", formalDefinition="When this Contract Provision was issued." ) 1562 protected DateTimeType issued; 1563 1564 /** 1565 * Relevant time or time-period when this Contract Provision is applicable. 1566 */ 1567 @Child(name = "applies", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 1568 @Description(shortDefinition="Contract Term Effective Time", formalDefinition="Relevant time or time-period when this Contract Provision is applicable." ) 1569 protected Period applies; 1570 1571 /** 1572 * Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit. 1573 */ 1574 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1575 @Description(shortDefinition="Contract Term Type or Form", formalDefinition="Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit." ) 1576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-term-type") 1577 protected CodeableConcept type; 1578 1579 /** 1580 * Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment. 1581 */ 1582 @Child(name = "subType", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1583 @Description(shortDefinition="Contract Term Type specific classification", formalDefinition="Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment." ) 1584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-term-subtype") 1585 protected CodeableConcept subType; 1586 1587 /** 1588 * The matter of concern in the context of this provision of the agrement. 1589 */ 1590 @Child(name = "topic", type = {Reference.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1591 @Description(shortDefinition="Context of the Contract term", formalDefinition="The matter of concern in the context of this provision of the agrement." ) 1592 protected List<Reference> topic; 1593 /** 1594 * The actual objects that are the target of the reference (The matter of concern in the context of this provision of the agrement.) 1595 */ 1596 protected List<Resource> topicTarget; 1597 1598 1599 /** 1600 * Action stipulated by this Contract Provision. 1601 */ 1602 @Child(name = "action", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1603 @Description(shortDefinition="Contract Term Activity", formalDefinition="Action stipulated by this Contract Provision." ) 1604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-action") 1605 protected List<CodeableConcept> action; 1606 1607 /** 1608 * Reason or purpose for the action stipulated by this Contract Provision. 1609 */ 1610 @Child(name = "actionReason", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1611 @Description(shortDefinition="Purpose for the Contract Term Action", formalDefinition="Reason or purpose for the action stipulated by this Contract Provision." ) 1612 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 1613 protected List<CodeableConcept> actionReason; 1614 1615 /** 1616 * A set of security labels that define which terms are controlled by this condition. 1617 */ 1618 @Child(name = "securityLabel", type = {Coding.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1619 @Description(shortDefinition="Security Labels that define affected terms", formalDefinition="A set of security labels that define which terms are controlled by this condition." ) 1620 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 1621 protected List<Coding> securityLabel; 1622 1623 /** 1624 * An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place. 1625 */ 1626 @Child(name = "agent", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1627 @Description(shortDefinition="Contract Term Agent List", formalDefinition="An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place." ) 1628 protected List<TermAgentComponent> agent; 1629 1630 /** 1631 * Human readable form of this Contract Provision. 1632 */ 1633 @Child(name = "text", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1634 @Description(shortDefinition="Human readable Contract term text", formalDefinition="Human readable form of this Contract Provision." ) 1635 protected StringType text; 1636 1637 /** 1638 * Contract Provision Valued Item List. 1639 */ 1640 @Child(name = "valuedItem", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1641 @Description(shortDefinition="Contract Term Valued Item List", formalDefinition="Contract Provision Valued Item List." ) 1642 protected List<TermValuedItemComponent> valuedItem; 1643 1644 /** 1645 * Nested group of Contract Provisions. 1646 */ 1647 @Child(name = "group", type = {TermComponent.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1648 @Description(shortDefinition="Nested Contract Term Group", formalDefinition="Nested group of Contract Provisions." ) 1649 protected List<TermComponent> group; 1650 1651 private static final long serialVersionUID = 812661371L; 1652 1653 /** 1654 * Constructor 1655 */ 1656 public TermComponent() { 1657 super(); 1658 } 1659 1660 /** 1661 * @return {@link #identifier} (Unique identifier for this particular Contract Provision.) 1662 */ 1663 public Identifier getIdentifier() { 1664 if (this.identifier == null) 1665 if (Configuration.errorOnAutoCreate()) 1666 throw new Error("Attempt to auto-create TermComponent.identifier"); 1667 else if (Configuration.doAutoCreate()) 1668 this.identifier = new Identifier(); // cc 1669 return this.identifier; 1670 } 1671 1672 public boolean hasIdentifier() { 1673 return this.identifier != null && !this.identifier.isEmpty(); 1674 } 1675 1676 /** 1677 * @param value {@link #identifier} (Unique identifier for this particular Contract Provision.) 1678 */ 1679 public TermComponent setIdentifier(Identifier value) { 1680 this.identifier = value; 1681 return this; 1682 } 1683 1684 /** 1685 * @return {@link #issued} (When this Contract Provision was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1686 */ 1687 public DateTimeType getIssuedElement() { 1688 if (this.issued == null) 1689 if (Configuration.errorOnAutoCreate()) 1690 throw new Error("Attempt to auto-create TermComponent.issued"); 1691 else if (Configuration.doAutoCreate()) 1692 this.issued = new DateTimeType(); // bb 1693 return this.issued; 1694 } 1695 1696 public boolean hasIssuedElement() { 1697 return this.issued != null && !this.issued.isEmpty(); 1698 } 1699 1700 public boolean hasIssued() { 1701 return this.issued != null && !this.issued.isEmpty(); 1702 } 1703 1704 /** 1705 * @param value {@link #issued} (When this Contract Provision was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1706 */ 1707 public TermComponent setIssuedElement(DateTimeType value) { 1708 this.issued = value; 1709 return this; 1710 } 1711 1712 /** 1713 * @return When this Contract Provision was issued. 1714 */ 1715 public Date getIssued() { 1716 return this.issued == null ? null : this.issued.getValue(); 1717 } 1718 1719 /** 1720 * @param value When this Contract Provision was issued. 1721 */ 1722 public TermComponent setIssued(Date value) { 1723 if (value == null) 1724 this.issued = null; 1725 else { 1726 if (this.issued == null) 1727 this.issued = new DateTimeType(); 1728 this.issued.setValue(value); 1729 } 1730 return this; 1731 } 1732 1733 /** 1734 * @return {@link #applies} (Relevant time or time-period when this Contract Provision is applicable.) 1735 */ 1736 public Period getApplies() { 1737 if (this.applies == null) 1738 if (Configuration.errorOnAutoCreate()) 1739 throw new Error("Attempt to auto-create TermComponent.applies"); 1740 else if (Configuration.doAutoCreate()) 1741 this.applies = new Period(); // cc 1742 return this.applies; 1743 } 1744 1745 public boolean hasApplies() { 1746 return this.applies != null && !this.applies.isEmpty(); 1747 } 1748 1749 /** 1750 * @param value {@link #applies} (Relevant time or time-period when this Contract Provision is applicable.) 1751 */ 1752 public TermComponent setApplies(Period value) { 1753 this.applies = value; 1754 return this; 1755 } 1756 1757 /** 1758 * @return {@link #type} (Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.) 1759 */ 1760 public CodeableConcept getType() { 1761 if (this.type == null) 1762 if (Configuration.errorOnAutoCreate()) 1763 throw new Error("Attempt to auto-create TermComponent.type"); 1764 else if (Configuration.doAutoCreate()) 1765 this.type = new CodeableConcept(); // cc 1766 return this.type; 1767 } 1768 1769 public boolean hasType() { 1770 return this.type != null && !this.type.isEmpty(); 1771 } 1772 1773 /** 1774 * @param value {@link #type} (Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.) 1775 */ 1776 public TermComponent setType(CodeableConcept value) { 1777 this.type = value; 1778 return this; 1779 } 1780 1781 /** 1782 * @return {@link #subType} (Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment.) 1783 */ 1784 public CodeableConcept getSubType() { 1785 if (this.subType == null) 1786 if (Configuration.errorOnAutoCreate()) 1787 throw new Error("Attempt to auto-create TermComponent.subType"); 1788 else if (Configuration.doAutoCreate()) 1789 this.subType = new CodeableConcept(); // cc 1790 return this.subType; 1791 } 1792 1793 public boolean hasSubType() { 1794 return this.subType != null && !this.subType.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #subType} (Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment.) 1799 */ 1800 public TermComponent setSubType(CodeableConcept value) { 1801 this.subType = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return {@link #topic} (The matter of concern in the context of this provision of the agrement.) 1807 */ 1808 public List<Reference> getTopic() { 1809 if (this.topic == null) 1810 this.topic = new ArrayList<Reference>(); 1811 return this.topic; 1812 } 1813 1814 /** 1815 * @return Returns a reference to <code>this</code> for easy method chaining 1816 */ 1817 public TermComponent setTopic(List<Reference> theTopic) { 1818 this.topic = theTopic; 1819 return this; 1820 } 1821 1822 public boolean hasTopic() { 1823 if (this.topic == null) 1824 return false; 1825 for (Reference item : this.topic) 1826 if (!item.isEmpty()) 1827 return true; 1828 return false; 1829 } 1830 1831 public Reference addTopic() { //3 1832 Reference t = new Reference(); 1833 if (this.topic == null) 1834 this.topic = new ArrayList<Reference>(); 1835 this.topic.add(t); 1836 return t; 1837 } 1838 1839 public TermComponent addTopic(Reference t) { //3 1840 if (t == null) 1841 return this; 1842 if (this.topic == null) 1843 this.topic = new ArrayList<Reference>(); 1844 this.topic.add(t); 1845 return this; 1846 } 1847 1848 /** 1849 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 1850 */ 1851 public Reference getTopicFirstRep() { 1852 if (getTopic().isEmpty()) { 1853 addTopic(); 1854 } 1855 return getTopic().get(0); 1856 } 1857 1858 /** 1859 * @deprecated Use Reference#setResource(IBaseResource) instead 1860 */ 1861 @Deprecated 1862 public List<Resource> getTopicTarget() { 1863 if (this.topicTarget == null) 1864 this.topicTarget = new ArrayList<Resource>(); 1865 return this.topicTarget; 1866 } 1867 1868 /** 1869 * @return {@link #action} (Action stipulated by this Contract Provision.) 1870 */ 1871 public List<CodeableConcept> getAction() { 1872 if (this.action == null) 1873 this.action = new ArrayList<CodeableConcept>(); 1874 return this.action; 1875 } 1876 1877 /** 1878 * @return Returns a reference to <code>this</code> for easy method chaining 1879 */ 1880 public TermComponent setAction(List<CodeableConcept> theAction) { 1881 this.action = theAction; 1882 return this; 1883 } 1884 1885 public boolean hasAction() { 1886 if (this.action == null) 1887 return false; 1888 for (CodeableConcept item : this.action) 1889 if (!item.isEmpty()) 1890 return true; 1891 return false; 1892 } 1893 1894 public CodeableConcept addAction() { //3 1895 CodeableConcept t = new CodeableConcept(); 1896 if (this.action == null) 1897 this.action = new ArrayList<CodeableConcept>(); 1898 this.action.add(t); 1899 return t; 1900 } 1901 1902 public TermComponent addAction(CodeableConcept t) { //3 1903 if (t == null) 1904 return this; 1905 if (this.action == null) 1906 this.action = new ArrayList<CodeableConcept>(); 1907 this.action.add(t); 1908 return this; 1909 } 1910 1911 /** 1912 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 1913 */ 1914 public CodeableConcept getActionFirstRep() { 1915 if (getAction().isEmpty()) { 1916 addAction(); 1917 } 1918 return getAction().get(0); 1919 } 1920 1921 /** 1922 * @return {@link #actionReason} (Reason or purpose for the action stipulated by this Contract Provision.) 1923 */ 1924 public List<CodeableConcept> getActionReason() { 1925 if (this.actionReason == null) 1926 this.actionReason = new ArrayList<CodeableConcept>(); 1927 return this.actionReason; 1928 } 1929 1930 /** 1931 * @return Returns a reference to <code>this</code> for easy method chaining 1932 */ 1933 public TermComponent setActionReason(List<CodeableConcept> theActionReason) { 1934 this.actionReason = theActionReason; 1935 return this; 1936 } 1937 1938 public boolean hasActionReason() { 1939 if (this.actionReason == null) 1940 return false; 1941 for (CodeableConcept item : this.actionReason) 1942 if (!item.isEmpty()) 1943 return true; 1944 return false; 1945 } 1946 1947 public CodeableConcept addActionReason() { //3 1948 CodeableConcept t = new CodeableConcept(); 1949 if (this.actionReason == null) 1950 this.actionReason = new ArrayList<CodeableConcept>(); 1951 this.actionReason.add(t); 1952 return t; 1953 } 1954 1955 public TermComponent addActionReason(CodeableConcept t) { //3 1956 if (t == null) 1957 return this; 1958 if (this.actionReason == null) 1959 this.actionReason = new ArrayList<CodeableConcept>(); 1960 this.actionReason.add(t); 1961 return this; 1962 } 1963 1964 /** 1965 * @return The first repetition of repeating field {@link #actionReason}, creating it if it does not already exist 1966 */ 1967 public CodeableConcept getActionReasonFirstRep() { 1968 if (getActionReason().isEmpty()) { 1969 addActionReason(); 1970 } 1971 return getActionReason().get(0); 1972 } 1973 1974 /** 1975 * @return {@link #securityLabel} (A set of security labels that define which terms are controlled by this condition.) 1976 */ 1977 public List<Coding> getSecurityLabel() { 1978 if (this.securityLabel == null) 1979 this.securityLabel = new ArrayList<Coding>(); 1980 return this.securityLabel; 1981 } 1982 1983 /** 1984 * @return Returns a reference to <code>this</code> for easy method chaining 1985 */ 1986 public TermComponent setSecurityLabel(List<Coding> theSecurityLabel) { 1987 this.securityLabel = theSecurityLabel; 1988 return this; 1989 } 1990 1991 public boolean hasSecurityLabel() { 1992 if (this.securityLabel == null) 1993 return false; 1994 for (Coding item : this.securityLabel) 1995 if (!item.isEmpty()) 1996 return true; 1997 return false; 1998 } 1999 2000 public Coding addSecurityLabel() { //3 2001 Coding t = new Coding(); 2002 if (this.securityLabel == null) 2003 this.securityLabel = new ArrayList<Coding>(); 2004 this.securityLabel.add(t); 2005 return t; 2006 } 2007 2008 public TermComponent addSecurityLabel(Coding t) { //3 2009 if (t == null) 2010 return this; 2011 if (this.securityLabel == null) 2012 this.securityLabel = new ArrayList<Coding>(); 2013 this.securityLabel.add(t); 2014 return this; 2015 } 2016 2017 /** 2018 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 2019 */ 2020 public Coding getSecurityLabelFirstRep() { 2021 if (getSecurityLabel().isEmpty()) { 2022 addSecurityLabel(); 2023 } 2024 return getSecurityLabel().get(0); 2025 } 2026 2027 /** 2028 * @return {@link #agent} (An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.) 2029 */ 2030 public List<TermAgentComponent> getAgent() { 2031 if (this.agent == null) 2032 this.agent = new ArrayList<TermAgentComponent>(); 2033 return this.agent; 2034 } 2035 2036 /** 2037 * @return Returns a reference to <code>this</code> for easy method chaining 2038 */ 2039 public TermComponent setAgent(List<TermAgentComponent> theAgent) { 2040 this.agent = theAgent; 2041 return this; 2042 } 2043 2044 public boolean hasAgent() { 2045 if (this.agent == null) 2046 return false; 2047 for (TermAgentComponent item : this.agent) 2048 if (!item.isEmpty()) 2049 return true; 2050 return false; 2051 } 2052 2053 public TermAgentComponent addAgent() { //3 2054 TermAgentComponent t = new TermAgentComponent(); 2055 if (this.agent == null) 2056 this.agent = new ArrayList<TermAgentComponent>(); 2057 this.agent.add(t); 2058 return t; 2059 } 2060 2061 public TermComponent addAgent(TermAgentComponent t) { //3 2062 if (t == null) 2063 return this; 2064 if (this.agent == null) 2065 this.agent = new ArrayList<TermAgentComponent>(); 2066 this.agent.add(t); 2067 return this; 2068 } 2069 2070 /** 2071 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist 2072 */ 2073 public TermAgentComponent getAgentFirstRep() { 2074 if (getAgent().isEmpty()) { 2075 addAgent(); 2076 } 2077 return getAgent().get(0); 2078 } 2079 2080 /** 2081 * @return {@link #text} (Human readable form of this Contract Provision.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 2082 */ 2083 public StringType getTextElement() { 2084 if (this.text == null) 2085 if (Configuration.errorOnAutoCreate()) 2086 throw new Error("Attempt to auto-create TermComponent.text"); 2087 else if (Configuration.doAutoCreate()) 2088 this.text = new StringType(); // bb 2089 return this.text; 2090 } 2091 2092 public boolean hasTextElement() { 2093 return this.text != null && !this.text.isEmpty(); 2094 } 2095 2096 public boolean hasText() { 2097 return this.text != null && !this.text.isEmpty(); 2098 } 2099 2100 /** 2101 * @param value {@link #text} (Human readable form of this Contract Provision.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 2102 */ 2103 public TermComponent setTextElement(StringType value) { 2104 this.text = value; 2105 return this; 2106 } 2107 2108 /** 2109 * @return Human readable form of this Contract Provision. 2110 */ 2111 public String getText() { 2112 return this.text == null ? null : this.text.getValue(); 2113 } 2114 2115 /** 2116 * @param value Human readable form of this Contract Provision. 2117 */ 2118 public TermComponent setText(String value) { 2119 if (Utilities.noString(value)) 2120 this.text = null; 2121 else { 2122 if (this.text == null) 2123 this.text = new StringType(); 2124 this.text.setValue(value); 2125 } 2126 return this; 2127 } 2128 2129 /** 2130 * @return {@link #valuedItem} (Contract Provision Valued Item List.) 2131 */ 2132 public List<TermValuedItemComponent> getValuedItem() { 2133 if (this.valuedItem == null) 2134 this.valuedItem = new ArrayList<TermValuedItemComponent>(); 2135 return this.valuedItem; 2136 } 2137 2138 /** 2139 * @return Returns a reference to <code>this</code> for easy method chaining 2140 */ 2141 public TermComponent setValuedItem(List<TermValuedItemComponent> theValuedItem) { 2142 this.valuedItem = theValuedItem; 2143 return this; 2144 } 2145 2146 public boolean hasValuedItem() { 2147 if (this.valuedItem == null) 2148 return false; 2149 for (TermValuedItemComponent item : this.valuedItem) 2150 if (!item.isEmpty()) 2151 return true; 2152 return false; 2153 } 2154 2155 public TermValuedItemComponent addValuedItem() { //3 2156 TermValuedItemComponent t = new TermValuedItemComponent(); 2157 if (this.valuedItem == null) 2158 this.valuedItem = new ArrayList<TermValuedItemComponent>(); 2159 this.valuedItem.add(t); 2160 return t; 2161 } 2162 2163 public TermComponent addValuedItem(TermValuedItemComponent t) { //3 2164 if (t == null) 2165 return this; 2166 if (this.valuedItem == null) 2167 this.valuedItem = new ArrayList<TermValuedItemComponent>(); 2168 this.valuedItem.add(t); 2169 return this; 2170 } 2171 2172 /** 2173 * @return The first repetition of repeating field {@link #valuedItem}, creating it if it does not already exist 2174 */ 2175 public TermValuedItemComponent getValuedItemFirstRep() { 2176 if (getValuedItem().isEmpty()) { 2177 addValuedItem(); 2178 } 2179 return getValuedItem().get(0); 2180 } 2181 2182 /** 2183 * @return {@link #group} (Nested group of Contract Provisions.) 2184 */ 2185 public List<TermComponent> getGroup() { 2186 if (this.group == null) 2187 this.group = new ArrayList<TermComponent>(); 2188 return this.group; 2189 } 2190 2191 /** 2192 * @return Returns a reference to <code>this</code> for easy method chaining 2193 */ 2194 public TermComponent setGroup(List<TermComponent> theGroup) { 2195 this.group = theGroup; 2196 return this; 2197 } 2198 2199 public boolean hasGroup() { 2200 if (this.group == null) 2201 return false; 2202 for (TermComponent item : this.group) 2203 if (!item.isEmpty()) 2204 return true; 2205 return false; 2206 } 2207 2208 public TermComponent addGroup() { //3 2209 TermComponent t = new TermComponent(); 2210 if (this.group == null) 2211 this.group = new ArrayList<TermComponent>(); 2212 this.group.add(t); 2213 return t; 2214 } 2215 2216 public TermComponent addGroup(TermComponent t) { //3 2217 if (t == null) 2218 return this; 2219 if (this.group == null) 2220 this.group = new ArrayList<TermComponent>(); 2221 this.group.add(t); 2222 return this; 2223 } 2224 2225 /** 2226 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist 2227 */ 2228 public TermComponent getGroupFirstRep() { 2229 if (getGroup().isEmpty()) { 2230 addGroup(); 2231 } 2232 return getGroup().get(0); 2233 } 2234 2235 protected void listChildren(List<Property> children) { 2236 super.listChildren(children); 2237 children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 0, 1, identifier)); 2238 children.add(new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, issued)); 2239 children.add(new Property("applies", "Period", "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies)); 2240 children.add(new Property("type", "CodeableConcept", "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 0, 1, type)); 2241 children.add(new Property("subType", "CodeableConcept", "Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment.", 0, 1, subType)); 2242 children.add(new Property("topic", "Reference(Any)", "The matter of concern in the context of this provision of the agrement.", 0, java.lang.Integer.MAX_VALUE, topic)); 2243 children.add(new Property("action", "CodeableConcept", "Action stipulated by this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, action)); 2244 children.add(new Property("actionReason", "CodeableConcept", "Reason or purpose for the action stipulated by this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, actionReason)); 2245 children.add(new Property("securityLabel", "Coding", "A set of security labels that define which terms are controlled by this condition.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2246 children.add(new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent)); 2247 children.add(new Property("text", "string", "Human readable form of this Contract Provision.", 0, 1, text)); 2248 children.add(new Property("valuedItem", "", "Contract Provision Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem)); 2249 children.add(new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, java.lang.Integer.MAX_VALUE, group)); 2250 } 2251 2252 @Override 2253 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2254 switch (_hash) { 2255 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 0, 1, identifier); 2256 case -1179159893: /*issued*/ return new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, issued); 2257 case -793235316: /*applies*/ return new Property("applies", "Period", "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies); 2258 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 0, 1, type); 2259 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment.", 0, 1, subType); 2260 case 110546223: /*topic*/ return new Property("topic", "Reference(Any)", "The matter of concern in the context of this provision of the agrement.", 0, java.lang.Integer.MAX_VALUE, topic); 2261 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Action stipulated by this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, action); 2262 case 1465121818: /*actionReason*/ return new Property("actionReason", "CodeableConcept", "Reason or purpose for the action stipulated by this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, actionReason); 2263 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A set of security labels that define which terms are controlled by this condition.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 2264 case 92750597: /*agent*/ return new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent); 2265 case 3556653: /*text*/ return new Property("text", "string", "Human readable form of this Contract Provision.", 0, 1, text); 2266 case 2046675654: /*valuedItem*/ return new Property("valuedItem", "", "Contract Provision Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem); 2267 case 98629247: /*group*/ return new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, java.lang.Integer.MAX_VALUE, group); 2268 default: return super.getNamedProperty(_hash, _name, _checkValid); 2269 } 2270 2271 } 2272 2273 @Override 2274 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2275 switch (hash) { 2276 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2277 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // DateTimeType 2278 case -793235316: /*applies*/ return this.applies == null ? new Base[0] : new Base[] {this.applies}; // Period 2279 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2280 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 2281 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // Reference 2282 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 2283 case 1465121818: /*actionReason*/ return this.actionReason == null ? new Base[0] : this.actionReason.toArray(new Base[this.actionReason.size()]); // CodeableConcept 2284 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 2285 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // TermAgentComponent 2286 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 2287 case 2046675654: /*valuedItem*/ return this.valuedItem == null ? new Base[0] : this.valuedItem.toArray(new Base[this.valuedItem.size()]); // TermValuedItemComponent 2288 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // TermComponent 2289 default: return super.getProperty(hash, name, checkValid); 2290 } 2291 2292 } 2293 2294 @Override 2295 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2296 switch (hash) { 2297 case -1618432855: // identifier 2298 this.identifier = castToIdentifier(value); // Identifier 2299 return value; 2300 case -1179159893: // issued 2301 this.issued = castToDateTime(value); // DateTimeType 2302 return value; 2303 case -793235316: // applies 2304 this.applies = castToPeriod(value); // Period 2305 return value; 2306 case 3575610: // type 2307 this.type = castToCodeableConcept(value); // CodeableConcept 2308 return value; 2309 case -1868521062: // subType 2310 this.subType = castToCodeableConcept(value); // CodeableConcept 2311 return value; 2312 case 110546223: // topic 2313 this.getTopic().add(castToReference(value)); // Reference 2314 return value; 2315 case -1422950858: // action 2316 this.getAction().add(castToCodeableConcept(value)); // CodeableConcept 2317 return value; 2318 case 1465121818: // actionReason 2319 this.getActionReason().add(castToCodeableConcept(value)); // CodeableConcept 2320 return value; 2321 case -722296940: // securityLabel 2322 this.getSecurityLabel().add(castToCoding(value)); // Coding 2323 return value; 2324 case 92750597: // agent 2325 this.getAgent().add((TermAgentComponent) value); // TermAgentComponent 2326 return value; 2327 case 3556653: // text 2328 this.text = castToString(value); // StringType 2329 return value; 2330 case 2046675654: // valuedItem 2331 this.getValuedItem().add((TermValuedItemComponent) value); // TermValuedItemComponent 2332 return value; 2333 case 98629247: // group 2334 this.getGroup().add((TermComponent) value); // TermComponent 2335 return value; 2336 default: return super.setProperty(hash, name, value); 2337 } 2338 2339 } 2340 2341 @Override 2342 public Base setProperty(String name, Base value) throws FHIRException { 2343 if (name.equals("identifier")) { 2344 this.identifier = castToIdentifier(value); // Identifier 2345 } else if (name.equals("issued")) { 2346 this.issued = castToDateTime(value); // DateTimeType 2347 } else if (name.equals("applies")) { 2348 this.applies = castToPeriod(value); // Period 2349 } else if (name.equals("type")) { 2350 this.type = castToCodeableConcept(value); // CodeableConcept 2351 } else if (name.equals("subType")) { 2352 this.subType = castToCodeableConcept(value); // CodeableConcept 2353 } else if (name.equals("topic")) { 2354 this.getTopic().add(castToReference(value)); 2355 } else if (name.equals("action")) { 2356 this.getAction().add(castToCodeableConcept(value)); 2357 } else if (name.equals("actionReason")) { 2358 this.getActionReason().add(castToCodeableConcept(value)); 2359 } else if (name.equals("securityLabel")) { 2360 this.getSecurityLabel().add(castToCoding(value)); 2361 } else if (name.equals("agent")) { 2362 this.getAgent().add((TermAgentComponent) value); 2363 } else if (name.equals("text")) { 2364 this.text = castToString(value); // StringType 2365 } else if (name.equals("valuedItem")) { 2366 this.getValuedItem().add((TermValuedItemComponent) value); 2367 } else if (name.equals("group")) { 2368 this.getGroup().add((TermComponent) value); 2369 } else 2370 return super.setProperty(name, value); 2371 return value; 2372 } 2373 2374 @Override 2375 public Base makeProperty(int hash, String name) throws FHIRException { 2376 switch (hash) { 2377 case -1618432855: return getIdentifier(); 2378 case -1179159893: return getIssuedElement(); 2379 case -793235316: return getApplies(); 2380 case 3575610: return getType(); 2381 case -1868521062: return getSubType(); 2382 case 110546223: return addTopic(); 2383 case -1422950858: return addAction(); 2384 case 1465121818: return addActionReason(); 2385 case -722296940: return addSecurityLabel(); 2386 case 92750597: return addAgent(); 2387 case 3556653: return getTextElement(); 2388 case 2046675654: return addValuedItem(); 2389 case 98629247: return addGroup(); 2390 default: return super.makeProperty(hash, name); 2391 } 2392 2393 } 2394 2395 @Override 2396 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2397 switch (hash) { 2398 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2399 case -1179159893: /*issued*/ return new String[] {"dateTime"}; 2400 case -793235316: /*applies*/ return new String[] {"Period"}; 2401 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2402 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 2403 case 110546223: /*topic*/ return new String[] {"Reference"}; 2404 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 2405 case 1465121818: /*actionReason*/ return new String[] {"CodeableConcept"}; 2406 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 2407 case 92750597: /*agent*/ return new String[] {}; 2408 case 3556653: /*text*/ return new String[] {"string"}; 2409 case 2046675654: /*valuedItem*/ return new String[] {}; 2410 case 98629247: /*group*/ return new String[] {"@Contract.term"}; 2411 default: return super.getTypesForProperty(hash, name); 2412 } 2413 2414 } 2415 2416 @Override 2417 public Base addChild(String name) throws FHIRException { 2418 if (name.equals("identifier")) { 2419 this.identifier = new Identifier(); 2420 return this.identifier; 2421 } 2422 else if (name.equals("issued")) { 2423 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 2424 } 2425 else if (name.equals("applies")) { 2426 this.applies = new Period(); 2427 return this.applies; 2428 } 2429 else if (name.equals("type")) { 2430 this.type = new CodeableConcept(); 2431 return this.type; 2432 } 2433 else if (name.equals("subType")) { 2434 this.subType = new CodeableConcept(); 2435 return this.subType; 2436 } 2437 else if (name.equals("topic")) { 2438 return addTopic(); 2439 } 2440 else if (name.equals("action")) { 2441 return addAction(); 2442 } 2443 else if (name.equals("actionReason")) { 2444 return addActionReason(); 2445 } 2446 else if (name.equals("securityLabel")) { 2447 return addSecurityLabel(); 2448 } 2449 else if (name.equals("agent")) { 2450 return addAgent(); 2451 } 2452 else if (name.equals("text")) { 2453 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 2454 } 2455 else if (name.equals("valuedItem")) { 2456 return addValuedItem(); 2457 } 2458 else if (name.equals("group")) { 2459 return addGroup(); 2460 } 2461 else 2462 return super.addChild(name); 2463 } 2464 2465 public TermComponent copy() { 2466 TermComponent dst = new TermComponent(); 2467 copyValues(dst); 2468 dst.identifier = identifier == null ? null : identifier.copy(); 2469 dst.issued = issued == null ? null : issued.copy(); 2470 dst.applies = applies == null ? null : applies.copy(); 2471 dst.type = type == null ? null : type.copy(); 2472 dst.subType = subType == null ? null : subType.copy(); 2473 if (topic != null) { 2474 dst.topic = new ArrayList<Reference>(); 2475 for (Reference i : topic) 2476 dst.topic.add(i.copy()); 2477 }; 2478 if (action != null) { 2479 dst.action = new ArrayList<CodeableConcept>(); 2480 for (CodeableConcept i : action) 2481 dst.action.add(i.copy()); 2482 }; 2483 if (actionReason != null) { 2484 dst.actionReason = new ArrayList<CodeableConcept>(); 2485 for (CodeableConcept i : actionReason) 2486 dst.actionReason.add(i.copy()); 2487 }; 2488 if (securityLabel != null) { 2489 dst.securityLabel = new ArrayList<Coding>(); 2490 for (Coding i : securityLabel) 2491 dst.securityLabel.add(i.copy()); 2492 }; 2493 if (agent != null) { 2494 dst.agent = new ArrayList<TermAgentComponent>(); 2495 for (TermAgentComponent i : agent) 2496 dst.agent.add(i.copy()); 2497 }; 2498 dst.text = text == null ? null : text.copy(); 2499 if (valuedItem != null) { 2500 dst.valuedItem = new ArrayList<TermValuedItemComponent>(); 2501 for (TermValuedItemComponent i : valuedItem) 2502 dst.valuedItem.add(i.copy()); 2503 }; 2504 if (group != null) { 2505 dst.group = new ArrayList<TermComponent>(); 2506 for (TermComponent i : group) 2507 dst.group.add(i.copy()); 2508 }; 2509 return dst; 2510 } 2511 2512 @Override 2513 public boolean equalsDeep(Base other_) { 2514 if (!super.equalsDeep(other_)) 2515 return false; 2516 if (!(other_ instanceof TermComponent)) 2517 return false; 2518 TermComponent o = (TermComponent) other_; 2519 return compareDeep(identifier, o.identifier, true) && compareDeep(issued, o.issued, true) && compareDeep(applies, o.applies, true) 2520 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(topic, o.topic, true) 2521 && compareDeep(action, o.action, true) && compareDeep(actionReason, o.actionReason, true) && compareDeep(securityLabel, o.securityLabel, true) 2522 && compareDeep(agent, o.agent, true) && compareDeep(text, o.text, true) && compareDeep(valuedItem, o.valuedItem, true) 2523 && compareDeep(group, o.group, true); 2524 } 2525 2526 @Override 2527 public boolean equalsShallow(Base other_) { 2528 if (!super.equalsShallow(other_)) 2529 return false; 2530 if (!(other_ instanceof TermComponent)) 2531 return false; 2532 TermComponent o = (TermComponent) other_; 2533 return compareValues(issued, o.issued, true) && compareValues(text, o.text, true); 2534 } 2535 2536 public boolean isEmpty() { 2537 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, issued, applies 2538 , type, subType, topic, action, actionReason, securityLabel, agent, text, valuedItem 2539 , group); 2540 } 2541 2542 public String fhirType() { 2543 return "Contract.term"; 2544 2545 } 2546 2547 } 2548 2549 @Block() 2550 public static class TermAgentComponent extends BackboneElement implements IBaseBackboneElement { 2551 /** 2552 * The agent assigned a role in this Contract Provision. 2553 */ 2554 @Child(name = "actor", type = {Contract.class, Device.class, Group.class, Location.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, Substance.class}, order=1, min=1, max=1, modifier=false, summary=false) 2555 @Description(shortDefinition="Contract Term Agent Subject", formalDefinition="The agent assigned a role in this Contract Provision." ) 2556 protected Reference actor; 2557 2558 /** 2559 * The actual object that is the target of the reference (The agent assigned a role in this Contract Provision.) 2560 */ 2561 protected Resource actorTarget; 2562 2563 /** 2564 * Role played by the agent assigned this role in the execution of this Contract Provision. 2565 */ 2566 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2567 @Description(shortDefinition="Type of the Contract Term Agent", formalDefinition="Role played by the agent assigned this role in the execution of this Contract Provision." ) 2568 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-actorrole") 2569 protected List<CodeableConcept> role; 2570 2571 private static final long serialVersionUID = -454551165L; 2572 2573 /** 2574 * Constructor 2575 */ 2576 public TermAgentComponent() { 2577 super(); 2578 } 2579 2580 /** 2581 * Constructor 2582 */ 2583 public TermAgentComponent(Reference actor) { 2584 super(); 2585 this.actor = actor; 2586 } 2587 2588 /** 2589 * @return {@link #actor} (The agent assigned a role in this Contract Provision.) 2590 */ 2591 public Reference getActor() { 2592 if (this.actor == null) 2593 if (Configuration.errorOnAutoCreate()) 2594 throw new Error("Attempt to auto-create TermAgentComponent.actor"); 2595 else if (Configuration.doAutoCreate()) 2596 this.actor = new Reference(); // cc 2597 return this.actor; 2598 } 2599 2600 public boolean hasActor() { 2601 return this.actor != null && !this.actor.isEmpty(); 2602 } 2603 2604 /** 2605 * @param value {@link #actor} (The agent assigned a role in this Contract Provision.) 2606 */ 2607 public TermAgentComponent setActor(Reference value) { 2608 this.actor = value; 2609 return this; 2610 } 2611 2612 /** 2613 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The agent assigned a role in this Contract Provision.) 2614 */ 2615 public Resource getActorTarget() { 2616 return this.actorTarget; 2617 } 2618 2619 /** 2620 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The agent assigned a role in this Contract Provision.) 2621 */ 2622 public TermAgentComponent setActorTarget(Resource value) { 2623 this.actorTarget = value; 2624 return this; 2625 } 2626 2627 /** 2628 * @return {@link #role} (Role played by the agent assigned this role in the execution of this Contract Provision.) 2629 */ 2630 public List<CodeableConcept> getRole() { 2631 if (this.role == null) 2632 this.role = new ArrayList<CodeableConcept>(); 2633 return this.role; 2634 } 2635 2636 /** 2637 * @return Returns a reference to <code>this</code> for easy method chaining 2638 */ 2639 public TermAgentComponent setRole(List<CodeableConcept> theRole) { 2640 this.role = theRole; 2641 return this; 2642 } 2643 2644 public boolean hasRole() { 2645 if (this.role == null) 2646 return false; 2647 for (CodeableConcept item : this.role) 2648 if (!item.isEmpty()) 2649 return true; 2650 return false; 2651 } 2652 2653 public CodeableConcept addRole() { //3 2654 CodeableConcept t = new CodeableConcept(); 2655 if (this.role == null) 2656 this.role = new ArrayList<CodeableConcept>(); 2657 this.role.add(t); 2658 return t; 2659 } 2660 2661 public TermAgentComponent addRole(CodeableConcept t) { //3 2662 if (t == null) 2663 return this; 2664 if (this.role == null) 2665 this.role = new ArrayList<CodeableConcept>(); 2666 this.role.add(t); 2667 return this; 2668 } 2669 2670 /** 2671 * @return The first repetition of repeating field {@link #role}, creating it if it does not already exist 2672 */ 2673 public CodeableConcept getRoleFirstRep() { 2674 if (getRole().isEmpty()) { 2675 addRole(); 2676 } 2677 return getRole().get(0); 2678 } 2679 2680 protected void listChildren(List<Property> children) { 2681 super.listChildren(children); 2682 children.add(new Property("actor", "Reference(Contract|Device|Group|Location|Organization|Patient|Practitioner|RelatedPerson|Substance)", "The agent assigned a role in this Contract Provision.", 0, 1, actor)); 2683 children.add(new Property("role", "CodeableConcept", "Role played by the agent assigned this role in the execution of this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, role)); 2684 } 2685 2686 @Override 2687 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2688 switch (_hash) { 2689 case 92645877: /*actor*/ return new Property("actor", "Reference(Contract|Device|Group|Location|Organization|Patient|Practitioner|RelatedPerson|Substance)", "The agent assigned a role in this Contract Provision.", 0, 1, actor); 2690 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Role played by the agent assigned this role in the execution of this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, role); 2691 default: return super.getNamedProperty(_hash, _name, _checkValid); 2692 } 2693 2694 } 2695 2696 @Override 2697 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2698 switch (hash) { 2699 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 2700 case 3506294: /*role*/ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 2701 default: return super.getProperty(hash, name, checkValid); 2702 } 2703 2704 } 2705 2706 @Override 2707 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2708 switch (hash) { 2709 case 92645877: // actor 2710 this.actor = castToReference(value); // Reference 2711 return value; 2712 case 3506294: // role 2713 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 2714 return value; 2715 default: return super.setProperty(hash, name, value); 2716 } 2717 2718 } 2719 2720 @Override 2721 public Base setProperty(String name, Base value) throws FHIRException { 2722 if (name.equals("actor")) { 2723 this.actor = castToReference(value); // Reference 2724 } else if (name.equals("role")) { 2725 this.getRole().add(castToCodeableConcept(value)); 2726 } else 2727 return super.setProperty(name, value); 2728 return value; 2729 } 2730 2731 @Override 2732 public Base makeProperty(int hash, String name) throws FHIRException { 2733 switch (hash) { 2734 case 92645877: return getActor(); 2735 case 3506294: return addRole(); 2736 default: return super.makeProperty(hash, name); 2737 } 2738 2739 } 2740 2741 @Override 2742 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2743 switch (hash) { 2744 case 92645877: /*actor*/ return new String[] {"Reference"}; 2745 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2746 default: return super.getTypesForProperty(hash, name); 2747 } 2748 2749 } 2750 2751 @Override 2752 public Base addChild(String name) throws FHIRException { 2753 if (name.equals("actor")) { 2754 this.actor = new Reference(); 2755 return this.actor; 2756 } 2757 else if (name.equals("role")) { 2758 return addRole(); 2759 } 2760 else 2761 return super.addChild(name); 2762 } 2763 2764 public TermAgentComponent copy() { 2765 TermAgentComponent dst = new TermAgentComponent(); 2766 copyValues(dst); 2767 dst.actor = actor == null ? null : actor.copy(); 2768 if (role != null) { 2769 dst.role = new ArrayList<CodeableConcept>(); 2770 for (CodeableConcept i : role) 2771 dst.role.add(i.copy()); 2772 }; 2773 return dst; 2774 } 2775 2776 @Override 2777 public boolean equalsDeep(Base other_) { 2778 if (!super.equalsDeep(other_)) 2779 return false; 2780 if (!(other_ instanceof TermAgentComponent)) 2781 return false; 2782 TermAgentComponent o = (TermAgentComponent) other_; 2783 return compareDeep(actor, o.actor, true) && compareDeep(role, o.role, true); 2784 } 2785 2786 @Override 2787 public boolean equalsShallow(Base other_) { 2788 if (!super.equalsShallow(other_)) 2789 return false; 2790 if (!(other_ instanceof TermAgentComponent)) 2791 return false; 2792 TermAgentComponent o = (TermAgentComponent) other_; 2793 return true; 2794 } 2795 2796 public boolean isEmpty() { 2797 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actor, role); 2798 } 2799 2800 public String fhirType() { 2801 return "Contract.term.agent"; 2802 2803 } 2804 2805 } 2806 2807 @Block() 2808 public static class TermValuedItemComponent extends BackboneElement implements IBaseBackboneElement { 2809 /** 2810 * Specific type of Contract Provision Valued Item that may be priced. 2811 */ 2812 @Child(name = "entity", type = {CodeableConcept.class, Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 2813 @Description(shortDefinition="Contract Term Valued Item Type", formalDefinition="Specific type of Contract Provision Valued Item that may be priced." ) 2814 protected Type entity; 2815 2816 /** 2817 * Identifies a Contract Provision Valued Item instance. 2818 */ 2819 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 2820 @Description(shortDefinition="Contract Term Valued Item Number", formalDefinition="Identifies a Contract Provision Valued Item instance." ) 2821 protected Identifier identifier; 2822 2823 /** 2824 * Indicates the time during which this Contract Term ValuedItem information is effective. 2825 */ 2826 @Child(name = "effectiveTime", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2827 @Description(shortDefinition="Contract Term Valued Item Effective Tiem", formalDefinition="Indicates the time during which this Contract Term ValuedItem information is effective." ) 2828 protected DateTimeType effectiveTime; 2829 2830 /** 2831 * Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances. 2832 */ 2833 @Child(name = "quantity", type = {SimpleQuantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 2834 @Description(shortDefinition="Contract Term Valued Item Count", formalDefinition="Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances." ) 2835 protected SimpleQuantity quantity; 2836 2837 /** 2838 * A Contract Provision Valued Item unit valuation measure. 2839 */ 2840 @Child(name = "unitPrice", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 2841 @Description(shortDefinition="Contract Term Valued Item fee, charge, or cost", formalDefinition="A Contract Provision Valued Item unit valuation measure." ) 2842 protected Money unitPrice; 2843 2844 /** 2845 * A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 2846 */ 2847 @Child(name = "factor", type = {DecimalType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2848 @Description(shortDefinition="Contract Term Valued Item Price Scaling Factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 2849 protected DecimalType factor; 2850 2851 /** 2852 * An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point. 2853 */ 2854 @Child(name = "points", type = {DecimalType.class}, order=7, min=0, max=1, modifier=false, summary=false) 2855 @Description(shortDefinition="Contract Term Valued Item Difficulty Scaling Factor", formalDefinition="An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point." ) 2856 protected DecimalType points; 2857 2858 /** 2859 * Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 2860 */ 2861 @Child(name = "net", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 2862 @Description(shortDefinition="Total Contract Term Valued Item Value", formalDefinition="Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 2863 protected Money net; 2864 2865 private static final long serialVersionUID = 1782449516L; 2866 2867 /** 2868 * Constructor 2869 */ 2870 public TermValuedItemComponent() { 2871 super(); 2872 } 2873 2874 /** 2875 * @return {@link #entity} (Specific type of Contract Provision Valued Item that may be priced.) 2876 */ 2877 public Type getEntity() { 2878 return this.entity; 2879 } 2880 2881 /** 2882 * @return {@link #entity} (Specific type of Contract Provision Valued Item that may be priced.) 2883 */ 2884 public CodeableConcept getEntityCodeableConcept() throws FHIRException { 2885 if (this.entity == null) 2886 return null; 2887 if (!(this.entity instanceof CodeableConcept)) 2888 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.entity.getClass().getName()+" was encountered"); 2889 return (CodeableConcept) this.entity; 2890 } 2891 2892 public boolean hasEntityCodeableConcept() { 2893 return this != null && this.entity instanceof CodeableConcept; 2894 } 2895 2896 /** 2897 * @return {@link #entity} (Specific type of Contract Provision Valued Item that may be priced.) 2898 */ 2899 public Reference getEntityReference() throws FHIRException { 2900 if (this.entity == null) 2901 return null; 2902 if (!(this.entity instanceof Reference)) 2903 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.entity.getClass().getName()+" was encountered"); 2904 return (Reference) this.entity; 2905 } 2906 2907 public boolean hasEntityReference() { 2908 return this != null && this.entity instanceof Reference; 2909 } 2910 2911 public boolean hasEntity() { 2912 return this.entity != null && !this.entity.isEmpty(); 2913 } 2914 2915 /** 2916 * @param value {@link #entity} (Specific type of Contract Provision Valued Item that may be priced.) 2917 */ 2918 public TermValuedItemComponent setEntity(Type value) throws FHIRFormatError { 2919 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2920 throw new FHIRFormatError("Not the right type for Contract.term.valuedItem.entity[x]: "+value.fhirType()); 2921 this.entity = value; 2922 return this; 2923 } 2924 2925 /** 2926 * @return {@link #identifier} (Identifies a Contract Provision Valued Item instance.) 2927 */ 2928 public Identifier getIdentifier() { 2929 if (this.identifier == null) 2930 if (Configuration.errorOnAutoCreate()) 2931 throw new Error("Attempt to auto-create TermValuedItemComponent.identifier"); 2932 else if (Configuration.doAutoCreate()) 2933 this.identifier = new Identifier(); // cc 2934 return this.identifier; 2935 } 2936 2937 public boolean hasIdentifier() { 2938 return this.identifier != null && !this.identifier.isEmpty(); 2939 } 2940 2941 /** 2942 * @param value {@link #identifier} (Identifies a Contract Provision Valued Item instance.) 2943 */ 2944 public TermValuedItemComponent setIdentifier(Identifier value) { 2945 this.identifier = value; 2946 return this; 2947 } 2948 2949 /** 2950 * @return {@link #effectiveTime} (Indicates the time during which this Contract Term ValuedItem information is effective.). This is the underlying object with id, value and extensions. The accessor "getEffectiveTime" gives direct access to the value 2951 */ 2952 public DateTimeType getEffectiveTimeElement() { 2953 if (this.effectiveTime == null) 2954 if (Configuration.errorOnAutoCreate()) 2955 throw new Error("Attempt to auto-create TermValuedItemComponent.effectiveTime"); 2956 else if (Configuration.doAutoCreate()) 2957 this.effectiveTime = new DateTimeType(); // bb 2958 return this.effectiveTime; 2959 } 2960 2961 public boolean hasEffectiveTimeElement() { 2962 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 2963 } 2964 2965 public boolean hasEffectiveTime() { 2966 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 2967 } 2968 2969 /** 2970 * @param value {@link #effectiveTime} (Indicates the time during which this Contract Term ValuedItem information is effective.). This is the underlying object with id, value and extensions. The accessor "getEffectiveTime" gives direct access to the value 2971 */ 2972 public TermValuedItemComponent setEffectiveTimeElement(DateTimeType value) { 2973 this.effectiveTime = value; 2974 return this; 2975 } 2976 2977 /** 2978 * @return Indicates the time during which this Contract Term ValuedItem information is effective. 2979 */ 2980 public Date getEffectiveTime() { 2981 return this.effectiveTime == null ? null : this.effectiveTime.getValue(); 2982 } 2983 2984 /** 2985 * @param value Indicates the time during which this Contract Term ValuedItem information is effective. 2986 */ 2987 public TermValuedItemComponent setEffectiveTime(Date value) { 2988 if (value == null) 2989 this.effectiveTime = null; 2990 else { 2991 if (this.effectiveTime == null) 2992 this.effectiveTime = new DateTimeType(); 2993 this.effectiveTime.setValue(value); 2994 } 2995 return this; 2996 } 2997 2998 /** 2999 * @return {@link #quantity} (Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances.) 3000 */ 3001 public SimpleQuantity getQuantity() { 3002 if (this.quantity == null) 3003 if (Configuration.errorOnAutoCreate()) 3004 throw new Error("Attempt to auto-create TermValuedItemComponent.quantity"); 3005 else if (Configuration.doAutoCreate()) 3006 this.quantity = new SimpleQuantity(); // cc 3007 return this.quantity; 3008 } 3009 3010 public boolean hasQuantity() { 3011 return this.quantity != null && !this.quantity.isEmpty(); 3012 } 3013 3014 /** 3015 * @param value {@link #quantity} (Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances.) 3016 */ 3017 public TermValuedItemComponent setQuantity(SimpleQuantity value) { 3018 this.quantity = value; 3019 return this; 3020 } 3021 3022 /** 3023 * @return {@link #unitPrice} (A Contract Provision Valued Item unit valuation measure.) 3024 */ 3025 public Money getUnitPrice() { 3026 if (this.unitPrice == null) 3027 if (Configuration.errorOnAutoCreate()) 3028 throw new Error("Attempt to auto-create TermValuedItemComponent.unitPrice"); 3029 else if (Configuration.doAutoCreate()) 3030 this.unitPrice = new Money(); // cc 3031 return this.unitPrice; 3032 } 3033 3034 public boolean hasUnitPrice() { 3035 return this.unitPrice != null && !this.unitPrice.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #unitPrice} (A Contract Provision Valued Item unit valuation measure.) 3040 */ 3041 public TermValuedItemComponent setUnitPrice(Money value) { 3042 this.unitPrice = value; 3043 return this; 3044 } 3045 3046 /** 3047 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3048 */ 3049 public DecimalType getFactorElement() { 3050 if (this.factor == null) 3051 if (Configuration.errorOnAutoCreate()) 3052 throw new Error("Attempt to auto-create TermValuedItemComponent.factor"); 3053 else if (Configuration.doAutoCreate()) 3054 this.factor = new DecimalType(); // bb 3055 return this.factor; 3056 } 3057 3058 public boolean hasFactorElement() { 3059 return this.factor != null && !this.factor.isEmpty(); 3060 } 3061 3062 public boolean hasFactor() { 3063 return this.factor != null && !this.factor.isEmpty(); 3064 } 3065 3066 /** 3067 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3068 */ 3069 public TermValuedItemComponent setFactorElement(DecimalType value) { 3070 this.factor = value; 3071 return this; 3072 } 3073 3074 /** 3075 * @return A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3076 */ 3077 public BigDecimal getFactor() { 3078 return this.factor == null ? null : this.factor.getValue(); 3079 } 3080 3081 /** 3082 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3083 */ 3084 public TermValuedItemComponent setFactor(BigDecimal value) { 3085 if (value == null) 3086 this.factor = null; 3087 else { 3088 if (this.factor == null) 3089 this.factor = new DecimalType(); 3090 this.factor.setValue(value); 3091 } 3092 return this; 3093 } 3094 3095 /** 3096 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3097 */ 3098 public TermValuedItemComponent setFactor(long value) { 3099 this.factor = new DecimalType(); 3100 this.factor.setValue(value); 3101 return this; 3102 } 3103 3104 /** 3105 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3106 */ 3107 public TermValuedItemComponent setFactor(double value) { 3108 this.factor = new DecimalType(); 3109 this.factor.setValue(value); 3110 return this; 3111 } 3112 3113 /** 3114 * @return {@link #points} (An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point.). This is the underlying object with id, value and extensions. The accessor "getPoints" gives direct access to the value 3115 */ 3116 public DecimalType getPointsElement() { 3117 if (this.points == null) 3118 if (Configuration.errorOnAutoCreate()) 3119 throw new Error("Attempt to auto-create TermValuedItemComponent.points"); 3120 else if (Configuration.doAutoCreate()) 3121 this.points = new DecimalType(); // bb 3122 return this.points; 3123 } 3124 3125 public boolean hasPointsElement() { 3126 return this.points != null && !this.points.isEmpty(); 3127 } 3128 3129 public boolean hasPoints() { 3130 return this.points != null && !this.points.isEmpty(); 3131 } 3132 3133 /** 3134 * @param value {@link #points} (An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point.). This is the underlying object with id, value and extensions. The accessor "getPoints" gives direct access to the value 3135 */ 3136 public TermValuedItemComponent setPointsElement(DecimalType value) { 3137 this.points = value; 3138 return this; 3139 } 3140 3141 /** 3142 * @return An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point. 3143 */ 3144 public BigDecimal getPoints() { 3145 return this.points == null ? null : this.points.getValue(); 3146 } 3147 3148 /** 3149 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point. 3150 */ 3151 public TermValuedItemComponent setPoints(BigDecimal value) { 3152 if (value == null) 3153 this.points = null; 3154 else { 3155 if (this.points == null) 3156 this.points = new DecimalType(); 3157 this.points.setValue(value); 3158 } 3159 return this; 3160 } 3161 3162 /** 3163 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point. 3164 */ 3165 public TermValuedItemComponent setPoints(long value) { 3166 this.points = new DecimalType(); 3167 this.points.setValue(value); 3168 return this; 3169 } 3170 3171 /** 3172 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point. 3173 */ 3174 public TermValuedItemComponent setPoints(double value) { 3175 this.points = new DecimalType(); 3176 this.points.setValue(value); 3177 return this; 3178 } 3179 3180 /** 3181 * @return {@link #net} (Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 3182 */ 3183 public Money getNet() { 3184 if (this.net == null) 3185 if (Configuration.errorOnAutoCreate()) 3186 throw new Error("Attempt to auto-create TermValuedItemComponent.net"); 3187 else if (Configuration.doAutoCreate()) 3188 this.net = new Money(); // cc 3189 return this.net; 3190 } 3191 3192 public boolean hasNet() { 3193 return this.net != null && !this.net.isEmpty(); 3194 } 3195 3196 /** 3197 * @param value {@link #net} (Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 3198 */ 3199 public TermValuedItemComponent setNet(Money value) { 3200 this.net = value; 3201 return this; 3202 } 3203 3204 protected void listChildren(List<Property> children) { 3205 super.listChildren(children); 3206 children.add(new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Provision Valued Item that may be priced.", 0, 1, entity)); 3207 children.add(new Property("identifier", "Identifier", "Identifies a Contract Provision Valued Item instance.", 0, 1, identifier)); 3208 children.add(new Property("effectiveTime", "dateTime", "Indicates the time during which this Contract Term ValuedItem information is effective.", 0, 1, effectiveTime)); 3209 children.add(new Property("quantity", "SimpleQuantity", "Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances.", 0, 1, quantity)); 3210 children.add(new Property("unitPrice", "Money", "A Contract Provision Valued Item unit valuation measure.", 0, 1, unitPrice)); 3211 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 3212 children.add(new Property("points", "decimal", "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point.", 0, 1, points)); 3213 children.add(new Property("net", "Money", "Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 3214 } 3215 3216 @Override 3217 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3218 switch (_hash) { 3219 case -740568643: /*entity[x]*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Provision Valued Item that may be priced.", 0, 1, entity); 3220 case -1298275357: /*entity*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Provision Valued Item that may be priced.", 0, 1, entity); 3221 case 924197182: /*entityCodeableConcept*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Provision Valued Item that may be priced.", 0, 1, entity); 3222 case -356635992: /*entityReference*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Provision Valued Item that may be priced.", 0, 1, entity); 3223 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifies a Contract Provision Valued Item instance.", 0, 1, identifier); 3224 case -929905388: /*effectiveTime*/ return new Property("effectiveTime", "dateTime", "Indicates the time during which this Contract Term ValuedItem information is effective.", 0, 1, effectiveTime); 3225 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances.", 0, 1, quantity); 3226 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "A Contract Provision Valued Item unit valuation measure.", 0, 1, unitPrice); 3227 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 3228 case -982754077: /*points*/ return new Property("points", "decimal", "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point.", 0, 1, points); 3229 case 108957: /*net*/ return new Property("net", "Money", "Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 3230 default: return super.getNamedProperty(_hash, _name, _checkValid); 3231 } 3232 3233 } 3234 3235 @Override 3236 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3237 switch (hash) { 3238 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : new Base[] {this.entity}; // Type 3239 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3240 case -929905388: /*effectiveTime*/ return this.effectiveTime == null ? new Base[0] : new Base[] {this.effectiveTime}; // DateTimeType 3241 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 3242 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 3243 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 3244 case -982754077: /*points*/ return this.points == null ? new Base[0] : new Base[] {this.points}; // DecimalType 3245 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 3246 default: return super.getProperty(hash, name, checkValid); 3247 } 3248 3249 } 3250 3251 @Override 3252 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3253 switch (hash) { 3254 case -1298275357: // entity 3255 this.entity = castToType(value); // Type 3256 return value; 3257 case -1618432855: // identifier 3258 this.identifier = castToIdentifier(value); // Identifier 3259 return value; 3260 case -929905388: // effectiveTime 3261 this.effectiveTime = castToDateTime(value); // DateTimeType 3262 return value; 3263 case -1285004149: // quantity 3264 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 3265 return value; 3266 case -486196699: // unitPrice 3267 this.unitPrice = castToMoney(value); // Money 3268 return value; 3269 case -1282148017: // factor 3270 this.factor = castToDecimal(value); // DecimalType 3271 return value; 3272 case -982754077: // points 3273 this.points = castToDecimal(value); // DecimalType 3274 return value; 3275 case 108957: // net 3276 this.net = castToMoney(value); // Money 3277 return value; 3278 default: return super.setProperty(hash, name, value); 3279 } 3280 3281 } 3282 3283 @Override 3284 public Base setProperty(String name, Base value) throws FHIRException { 3285 if (name.equals("entity[x]")) { 3286 this.entity = castToType(value); // Type 3287 } else if (name.equals("identifier")) { 3288 this.identifier = castToIdentifier(value); // Identifier 3289 } else if (name.equals("effectiveTime")) { 3290 this.effectiveTime = castToDateTime(value); // DateTimeType 3291 } else if (name.equals("quantity")) { 3292 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 3293 } else if (name.equals("unitPrice")) { 3294 this.unitPrice = castToMoney(value); // Money 3295 } else if (name.equals("factor")) { 3296 this.factor = castToDecimal(value); // DecimalType 3297 } else if (name.equals("points")) { 3298 this.points = castToDecimal(value); // DecimalType 3299 } else if (name.equals("net")) { 3300 this.net = castToMoney(value); // Money 3301 } else 3302 return super.setProperty(name, value); 3303 return value; 3304 } 3305 3306 @Override 3307 public Base makeProperty(int hash, String name) throws FHIRException { 3308 switch (hash) { 3309 case -740568643: return getEntity(); 3310 case -1298275357: return getEntity(); 3311 case -1618432855: return getIdentifier(); 3312 case -929905388: return getEffectiveTimeElement(); 3313 case -1285004149: return getQuantity(); 3314 case -486196699: return getUnitPrice(); 3315 case -1282148017: return getFactorElement(); 3316 case -982754077: return getPointsElement(); 3317 case 108957: return getNet(); 3318 default: return super.makeProperty(hash, name); 3319 } 3320 3321 } 3322 3323 @Override 3324 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3325 switch (hash) { 3326 case -1298275357: /*entity*/ return new String[] {"CodeableConcept", "Reference"}; 3327 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3328 case -929905388: /*effectiveTime*/ return new String[] {"dateTime"}; 3329 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 3330 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 3331 case -1282148017: /*factor*/ return new String[] {"decimal"}; 3332 case -982754077: /*points*/ return new String[] {"decimal"}; 3333 case 108957: /*net*/ return new String[] {"Money"}; 3334 default: return super.getTypesForProperty(hash, name); 3335 } 3336 3337 } 3338 3339 @Override 3340 public Base addChild(String name) throws FHIRException { 3341 if (name.equals("entityCodeableConcept")) { 3342 this.entity = new CodeableConcept(); 3343 return this.entity; 3344 } 3345 else if (name.equals("entityReference")) { 3346 this.entity = new Reference(); 3347 return this.entity; 3348 } 3349 else if (name.equals("identifier")) { 3350 this.identifier = new Identifier(); 3351 return this.identifier; 3352 } 3353 else if (name.equals("effectiveTime")) { 3354 throw new FHIRException("Cannot call addChild on a singleton property Contract.effectiveTime"); 3355 } 3356 else if (name.equals("quantity")) { 3357 this.quantity = new SimpleQuantity(); 3358 return this.quantity; 3359 } 3360 else if (name.equals("unitPrice")) { 3361 this.unitPrice = new Money(); 3362 return this.unitPrice; 3363 } 3364 else if (name.equals("factor")) { 3365 throw new FHIRException("Cannot call addChild on a singleton property Contract.factor"); 3366 } 3367 else if (name.equals("points")) { 3368 throw new FHIRException("Cannot call addChild on a singleton property Contract.points"); 3369 } 3370 else if (name.equals("net")) { 3371 this.net = new Money(); 3372 return this.net; 3373 } 3374 else 3375 return super.addChild(name); 3376 } 3377 3378 public TermValuedItemComponent copy() { 3379 TermValuedItemComponent dst = new TermValuedItemComponent(); 3380 copyValues(dst); 3381 dst.entity = entity == null ? null : entity.copy(); 3382 dst.identifier = identifier == null ? null : identifier.copy(); 3383 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 3384 dst.quantity = quantity == null ? null : quantity.copy(); 3385 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 3386 dst.factor = factor == null ? null : factor.copy(); 3387 dst.points = points == null ? null : points.copy(); 3388 dst.net = net == null ? null : net.copy(); 3389 return dst; 3390 } 3391 3392 @Override 3393 public boolean equalsDeep(Base other_) { 3394 if (!super.equalsDeep(other_)) 3395 return false; 3396 if (!(other_ instanceof TermValuedItemComponent)) 3397 return false; 3398 TermValuedItemComponent o = (TermValuedItemComponent) other_; 3399 return compareDeep(entity, o.entity, true) && compareDeep(identifier, o.identifier, true) && compareDeep(effectiveTime, o.effectiveTime, true) 3400 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 3401 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true); 3402 } 3403 3404 @Override 3405 public boolean equalsShallow(Base other_) { 3406 if (!super.equalsShallow(other_)) 3407 return false; 3408 if (!(other_ instanceof TermValuedItemComponent)) 3409 return false; 3410 TermValuedItemComponent o = (TermValuedItemComponent) other_; 3411 return compareValues(effectiveTime, o.effectiveTime, true) && compareValues(factor, o.factor, true) 3412 && compareValues(points, o.points, true); 3413 } 3414 3415 public boolean isEmpty() { 3416 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, identifier, effectiveTime 3417 , quantity, unitPrice, factor, points, net); 3418 } 3419 3420 public String fhirType() { 3421 return "Contract.term.valuedItem"; 3422 3423 } 3424 3425 } 3426 3427 @Block() 3428 public static class FriendlyLanguageComponent extends BackboneElement implements IBaseBackboneElement { 3429 /** 3430 * Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability. 3431 */ 3432 @Child(name = "content", type = {Attachment.class, Composition.class, DocumentReference.class, QuestionnaireResponse.class}, order=1, min=1, max=1, modifier=false, summary=false) 3433 @Description(shortDefinition="Easily comprehended representation of this Contract", formalDefinition="Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability." ) 3434 protected Type content; 3435 3436 private static final long serialVersionUID = -1763459053L; 3437 3438 /** 3439 * Constructor 3440 */ 3441 public FriendlyLanguageComponent() { 3442 super(); 3443 } 3444 3445 /** 3446 * Constructor 3447 */ 3448 public FriendlyLanguageComponent(Type content) { 3449 super(); 3450 this.content = content; 3451 } 3452 3453 /** 3454 * @return {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 3455 */ 3456 public Type getContent() { 3457 return this.content; 3458 } 3459 3460 /** 3461 * @return {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 3462 */ 3463 public Attachment getContentAttachment() throws FHIRException { 3464 if (this.content == null) 3465 return null; 3466 if (!(this.content instanceof Attachment)) 3467 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 3468 return (Attachment) this.content; 3469 } 3470 3471 public boolean hasContentAttachment() { 3472 return this != null && this.content instanceof Attachment; 3473 } 3474 3475 /** 3476 * @return {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 3477 */ 3478 public Reference getContentReference() throws FHIRException { 3479 if (this.content == null) 3480 return null; 3481 if (!(this.content instanceof Reference)) 3482 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 3483 return (Reference) this.content; 3484 } 3485 3486 public boolean hasContentReference() { 3487 return this != null && this.content instanceof Reference; 3488 } 3489 3490 public boolean hasContent() { 3491 return this.content != null && !this.content.isEmpty(); 3492 } 3493 3494 /** 3495 * @param value {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 3496 */ 3497 public FriendlyLanguageComponent setContent(Type value) throws FHIRFormatError { 3498 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 3499 throw new FHIRFormatError("Not the right type for Contract.friendly.content[x]: "+value.fhirType()); 3500 this.content = value; 3501 return this; 3502 } 3503 3504 protected void listChildren(List<Property> children) { 3505 super.listChildren(children); 3506 children.add(new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content)); 3507 } 3508 3509 @Override 3510 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3511 switch (_hash) { 3512 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 3513 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 3514 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 3515 case 1193747154: /*contentReference*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 3516 default: return super.getNamedProperty(_hash, _name, _checkValid); 3517 } 3518 3519 } 3520 3521 @Override 3522 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3523 switch (hash) { 3524 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Type 3525 default: return super.getProperty(hash, name, checkValid); 3526 } 3527 3528 } 3529 3530 @Override 3531 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3532 switch (hash) { 3533 case 951530617: // content 3534 this.content = castToType(value); // Type 3535 return value; 3536 default: return super.setProperty(hash, name, value); 3537 } 3538 3539 } 3540 3541 @Override 3542 public Base setProperty(String name, Base value) throws FHIRException { 3543 if (name.equals("content[x]")) { 3544 this.content = castToType(value); // Type 3545 } else 3546 return super.setProperty(name, value); 3547 return value; 3548 } 3549 3550 @Override 3551 public Base makeProperty(int hash, String name) throws FHIRException { 3552 switch (hash) { 3553 case 264548711: return getContent(); 3554 case 951530617: return getContent(); 3555 default: return super.makeProperty(hash, name); 3556 } 3557 3558 } 3559 3560 @Override 3561 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3562 switch (hash) { 3563 case 951530617: /*content*/ return new String[] {"Attachment", "Reference"}; 3564 default: return super.getTypesForProperty(hash, name); 3565 } 3566 3567 } 3568 3569 @Override 3570 public Base addChild(String name) throws FHIRException { 3571 if (name.equals("contentAttachment")) { 3572 this.content = new Attachment(); 3573 return this.content; 3574 } 3575 else if (name.equals("contentReference")) { 3576 this.content = new Reference(); 3577 return this.content; 3578 } 3579 else 3580 return super.addChild(name); 3581 } 3582 3583 public FriendlyLanguageComponent copy() { 3584 FriendlyLanguageComponent dst = new FriendlyLanguageComponent(); 3585 copyValues(dst); 3586 dst.content = content == null ? null : content.copy(); 3587 return dst; 3588 } 3589 3590 @Override 3591 public boolean equalsDeep(Base other_) { 3592 if (!super.equalsDeep(other_)) 3593 return false; 3594 if (!(other_ instanceof FriendlyLanguageComponent)) 3595 return false; 3596 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 3597 return compareDeep(content, o.content, true); 3598 } 3599 3600 @Override 3601 public boolean equalsShallow(Base other_) { 3602 if (!super.equalsShallow(other_)) 3603 return false; 3604 if (!(other_ instanceof FriendlyLanguageComponent)) 3605 return false; 3606 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 3607 return true; 3608 } 3609 3610 public boolean isEmpty() { 3611 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 3612 } 3613 3614 public String fhirType() { 3615 return "Contract.friendly"; 3616 3617 } 3618 3619 } 3620 3621 @Block() 3622 public static class LegalLanguageComponent extends BackboneElement implements IBaseBackboneElement { 3623 /** 3624 * Contract legal text in human renderable form. 3625 */ 3626 @Child(name = "content", type = {Attachment.class, Composition.class, DocumentReference.class, QuestionnaireResponse.class}, order=1, min=1, max=1, modifier=false, summary=false) 3627 @Description(shortDefinition="Contract Legal Text", formalDefinition="Contract legal text in human renderable form." ) 3628 protected Type content; 3629 3630 private static final long serialVersionUID = -1763459053L; 3631 3632 /** 3633 * Constructor 3634 */ 3635 public LegalLanguageComponent() { 3636 super(); 3637 } 3638 3639 /** 3640 * Constructor 3641 */ 3642 public LegalLanguageComponent(Type content) { 3643 super(); 3644 this.content = content; 3645 } 3646 3647 /** 3648 * @return {@link #content} (Contract legal text in human renderable form.) 3649 */ 3650 public Type getContent() { 3651 return this.content; 3652 } 3653 3654 /** 3655 * @return {@link #content} (Contract legal text in human renderable form.) 3656 */ 3657 public Attachment getContentAttachment() throws FHIRException { 3658 if (this.content == null) 3659 return null; 3660 if (!(this.content instanceof Attachment)) 3661 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 3662 return (Attachment) this.content; 3663 } 3664 3665 public boolean hasContentAttachment() { 3666 return this != null && this.content instanceof Attachment; 3667 } 3668 3669 /** 3670 * @return {@link #content} (Contract legal text in human renderable form.) 3671 */ 3672 public Reference getContentReference() throws FHIRException { 3673 if (this.content == null) 3674 return null; 3675 if (!(this.content instanceof Reference)) 3676 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 3677 return (Reference) this.content; 3678 } 3679 3680 public boolean hasContentReference() { 3681 return this != null && this.content instanceof Reference; 3682 } 3683 3684 public boolean hasContent() { 3685 return this.content != null && !this.content.isEmpty(); 3686 } 3687 3688 /** 3689 * @param value {@link #content} (Contract legal text in human renderable form.) 3690 */ 3691 public LegalLanguageComponent setContent(Type value) throws FHIRFormatError { 3692 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 3693 throw new FHIRFormatError("Not the right type for Contract.legal.content[x]: "+value.fhirType()); 3694 this.content = value; 3695 return this; 3696 } 3697 3698 protected void listChildren(List<Property> children) { 3699 super.listChildren(children); 3700 children.add(new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content)); 3701 } 3702 3703 @Override 3704 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3705 switch (_hash) { 3706 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content); 3707 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content); 3708 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content); 3709 case 1193747154: /*contentReference*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content); 3710 default: return super.getNamedProperty(_hash, _name, _checkValid); 3711 } 3712 3713 } 3714 3715 @Override 3716 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3717 switch (hash) { 3718 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Type 3719 default: return super.getProperty(hash, name, checkValid); 3720 } 3721 3722 } 3723 3724 @Override 3725 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3726 switch (hash) { 3727 case 951530617: // content 3728 this.content = castToType(value); // Type 3729 return value; 3730 default: return super.setProperty(hash, name, value); 3731 } 3732 3733 } 3734 3735 @Override 3736 public Base setProperty(String name, Base value) throws FHIRException { 3737 if (name.equals("content[x]")) { 3738 this.content = castToType(value); // Type 3739 } else 3740 return super.setProperty(name, value); 3741 return value; 3742 } 3743 3744 @Override 3745 public Base makeProperty(int hash, String name) throws FHIRException { 3746 switch (hash) { 3747 case 264548711: return getContent(); 3748 case 951530617: return getContent(); 3749 default: return super.makeProperty(hash, name); 3750 } 3751 3752 } 3753 3754 @Override 3755 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3756 switch (hash) { 3757 case 951530617: /*content*/ return new String[] {"Attachment", "Reference"}; 3758 default: return super.getTypesForProperty(hash, name); 3759 } 3760 3761 } 3762 3763 @Override 3764 public Base addChild(String name) throws FHIRException { 3765 if (name.equals("contentAttachment")) { 3766 this.content = new Attachment(); 3767 return this.content; 3768 } 3769 else if (name.equals("contentReference")) { 3770 this.content = new Reference(); 3771 return this.content; 3772 } 3773 else 3774 return super.addChild(name); 3775 } 3776 3777 public LegalLanguageComponent copy() { 3778 LegalLanguageComponent dst = new LegalLanguageComponent(); 3779 copyValues(dst); 3780 dst.content = content == null ? null : content.copy(); 3781 return dst; 3782 } 3783 3784 @Override 3785 public boolean equalsDeep(Base other_) { 3786 if (!super.equalsDeep(other_)) 3787 return false; 3788 if (!(other_ instanceof LegalLanguageComponent)) 3789 return false; 3790 LegalLanguageComponent o = (LegalLanguageComponent) other_; 3791 return compareDeep(content, o.content, true); 3792 } 3793 3794 @Override 3795 public boolean equalsShallow(Base other_) { 3796 if (!super.equalsShallow(other_)) 3797 return false; 3798 if (!(other_ instanceof LegalLanguageComponent)) 3799 return false; 3800 LegalLanguageComponent o = (LegalLanguageComponent) other_; 3801 return true; 3802 } 3803 3804 public boolean isEmpty() { 3805 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 3806 } 3807 3808 public String fhirType() { 3809 return "Contract.legal"; 3810 3811 } 3812 3813 } 3814 3815 @Block() 3816 public static class ComputableLanguageComponent extends BackboneElement implements IBaseBackboneElement { 3817 /** 3818 * Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal). 3819 */ 3820 @Child(name = "content", type = {Attachment.class, DocumentReference.class}, order=1, min=1, max=1, modifier=false, summary=false) 3821 @Description(shortDefinition="Computable Contract Rules", formalDefinition="Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal)." ) 3822 protected Type content; 3823 3824 private static final long serialVersionUID = -1763459053L; 3825 3826 /** 3827 * Constructor 3828 */ 3829 public ComputableLanguageComponent() { 3830 super(); 3831 } 3832 3833 /** 3834 * Constructor 3835 */ 3836 public ComputableLanguageComponent(Type content) { 3837 super(); 3838 this.content = content; 3839 } 3840 3841 /** 3842 * @return {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 3843 */ 3844 public Type getContent() { 3845 return this.content; 3846 } 3847 3848 /** 3849 * @return {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 3850 */ 3851 public Attachment getContentAttachment() throws FHIRException { 3852 if (this.content == null) 3853 return null; 3854 if (!(this.content instanceof Attachment)) 3855 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 3856 return (Attachment) this.content; 3857 } 3858 3859 public boolean hasContentAttachment() { 3860 return this != null && this.content instanceof Attachment; 3861 } 3862 3863 /** 3864 * @return {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 3865 */ 3866 public Reference getContentReference() throws FHIRException { 3867 if (this.content == null) 3868 return null; 3869 if (!(this.content instanceof Reference)) 3870 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 3871 return (Reference) this.content; 3872 } 3873 3874 public boolean hasContentReference() { 3875 return this != null && this.content instanceof Reference; 3876 } 3877 3878 public boolean hasContent() { 3879 return this.content != null && !this.content.isEmpty(); 3880 } 3881 3882 /** 3883 * @param value {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 3884 */ 3885 public ComputableLanguageComponent setContent(Type value) throws FHIRFormatError { 3886 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 3887 throw new FHIRFormatError("Not the right type for Contract.rule.content[x]: "+value.fhirType()); 3888 this.content = value; 3889 return this; 3890 } 3891 3892 protected void listChildren(List<Property> children) { 3893 super.listChildren(children); 3894 children.add(new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content)); 3895 } 3896 3897 @Override 3898 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3899 switch (_hash) { 3900 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 3901 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 3902 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 3903 case 1193747154: /*contentReference*/ return new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 3904 default: return super.getNamedProperty(_hash, _name, _checkValid); 3905 } 3906 3907 } 3908 3909 @Override 3910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3911 switch (hash) { 3912 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Type 3913 default: return super.getProperty(hash, name, checkValid); 3914 } 3915 3916 } 3917 3918 @Override 3919 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3920 switch (hash) { 3921 case 951530617: // content 3922 this.content = castToType(value); // Type 3923 return value; 3924 default: return super.setProperty(hash, name, value); 3925 } 3926 3927 } 3928 3929 @Override 3930 public Base setProperty(String name, Base value) throws FHIRException { 3931 if (name.equals("content[x]")) { 3932 this.content = castToType(value); // Type 3933 } else 3934 return super.setProperty(name, value); 3935 return value; 3936 } 3937 3938 @Override 3939 public Base makeProperty(int hash, String name) throws FHIRException { 3940 switch (hash) { 3941 case 264548711: return getContent(); 3942 case 951530617: return getContent(); 3943 default: return super.makeProperty(hash, name); 3944 } 3945 3946 } 3947 3948 @Override 3949 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3950 switch (hash) { 3951 case 951530617: /*content*/ return new String[] {"Attachment", "Reference"}; 3952 default: return super.getTypesForProperty(hash, name); 3953 } 3954 3955 } 3956 3957 @Override 3958 public Base addChild(String name) throws FHIRException { 3959 if (name.equals("contentAttachment")) { 3960 this.content = new Attachment(); 3961 return this.content; 3962 } 3963 else if (name.equals("contentReference")) { 3964 this.content = new Reference(); 3965 return this.content; 3966 } 3967 else 3968 return super.addChild(name); 3969 } 3970 3971 public ComputableLanguageComponent copy() { 3972 ComputableLanguageComponent dst = new ComputableLanguageComponent(); 3973 copyValues(dst); 3974 dst.content = content == null ? null : content.copy(); 3975 return dst; 3976 } 3977 3978 @Override 3979 public boolean equalsDeep(Base other_) { 3980 if (!super.equalsDeep(other_)) 3981 return false; 3982 if (!(other_ instanceof ComputableLanguageComponent)) 3983 return false; 3984 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 3985 return compareDeep(content, o.content, true); 3986 } 3987 3988 @Override 3989 public boolean equalsShallow(Base other_) { 3990 if (!super.equalsShallow(other_)) 3991 return false; 3992 if (!(other_ instanceof ComputableLanguageComponent)) 3993 return false; 3994 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 3995 return true; 3996 } 3997 3998 public boolean isEmpty() { 3999 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 4000 } 4001 4002 public String fhirType() { 4003 return "Contract.rule"; 4004 4005 } 4006 4007 } 4008 4009 /** 4010 * Unique identifier for this Contract. 4011 */ 4012 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 4013 @Description(shortDefinition="Contract number", formalDefinition="Unique identifier for this Contract." ) 4014 protected Identifier identifier; 4015 4016 /** 4017 * The status of the resource instance. 4018 */ 4019 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 4020 @Description(shortDefinition="amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated", formalDefinition="The status of the resource instance." ) 4021 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-status") 4022 protected Enumeration<ContractStatus> status; 4023 4024 /** 4025 * When this Contract was issued. 4026 */ 4027 @Child(name = "issued", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4028 @Description(shortDefinition="When this Contract was issued", formalDefinition="When this Contract was issued." ) 4029 protected DateTimeType issued; 4030 4031 /** 4032 * Relevant time or time-period when this Contract is applicable. 4033 */ 4034 @Child(name = "applies", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 4035 @Description(shortDefinition="Effective time", formalDefinition="Relevant time or time-period when this Contract is applicable." ) 4036 protected Period applies; 4037 4038 /** 4039 * The target entity impacted by or of interest to parties to the agreement. 4040 */ 4041 @Child(name = "subject", type = {Reference.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4042 @Description(shortDefinition="Contract Target Entity", formalDefinition="The target entity impacted by or of interest to parties to the agreement." ) 4043 protected List<Reference> subject; 4044 /** 4045 * The actual objects that are the target of the reference (The target entity impacted by or of interest to parties to the agreement.) 4046 */ 4047 protected List<Resource> subjectTarget; 4048 4049 4050 /** 4051 * The matter of concern in the context of this agreement. 4052 */ 4053 @Child(name = "topic", type = {Reference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4054 @Description(shortDefinition="Context of the Contract", formalDefinition="The matter of concern in the context of this agreement." ) 4055 protected List<Reference> topic; 4056 /** 4057 * The actual objects that are the target of the reference (The matter of concern in the context of this agreement.) 4058 */ 4059 protected List<Resource> topicTarget; 4060 4061 4062 /** 4063 * A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies. 4064 */ 4065 @Child(name = "authority", type = {Organization.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4066 @Description(shortDefinition="Authority under which this Contract has standing", formalDefinition="A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies." ) 4067 protected List<Reference> authority; 4068 /** 4069 * The actual objects that are the target of the reference (A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.) 4070 */ 4071 protected List<Organization> authorityTarget; 4072 4073 4074 /** 4075 * Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources. 4076 */ 4077 @Child(name = "domain", type = {Location.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4078 @Description(shortDefinition="Domain in which this Contract applies", formalDefinition="Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources." ) 4079 protected List<Reference> domain; 4080 /** 4081 * The actual objects that are the target of the reference (Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.) 4082 */ 4083 protected List<Location> domainTarget; 4084 4085 4086 /** 4087 * Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc. 4088 */ 4089 @Child(name = "type", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 4090 @Description(shortDefinition="Type or form", formalDefinition="Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc." ) 4091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-type") 4092 protected CodeableConcept type; 4093 4094 /** 4095 * More specific type or specialization of an overarching or more general contract such as auto insurance, home owner insurance, prenupial agreement, Advanced-Directive, or privacy consent. 4096 */ 4097 @Child(name = "subType", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4098 @Description(shortDefinition="Subtype within the context of type", formalDefinition="More specific type or specialization of an overarching or more general contract such as auto insurance, home owner insurance, prenupial agreement, Advanced-Directive, or privacy consent." ) 4099 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-subtype") 4100 protected List<CodeableConcept> subType; 4101 4102 /** 4103 * Action stipulated by this Contract. 4104 */ 4105 @Child(name = "action", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4106 @Description(shortDefinition="Action stipulated by this Contract", formalDefinition="Action stipulated by this Contract." ) 4107 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-action") 4108 protected List<CodeableConcept> action; 4109 4110 /** 4111 * Reason for action stipulated by this Contract. 4112 */ 4113 @Child(name = "actionReason", type = {CodeableConcept.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4114 @Description(shortDefinition="Rationale for the stiplulated action", formalDefinition="Reason for action stipulated by this Contract." ) 4115 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 4116 protected List<CodeableConcept> actionReason; 4117 4118 /** 4119 * The type of decision made by a grantor with respect to an offer made by a grantee. 4120 */ 4121 @Child(name = "decisionType", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 4122 @Description(shortDefinition="Decision by Grantor", formalDefinition="The type of decision made by a grantor with respect to an offer made by a grantee." ) 4123 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActConsentDirective") 4124 protected CodeableConcept decisionType; 4125 4126 /** 4127 * The minimal content derived from the basal information source at a specific stage in its lifecycle. 4128 */ 4129 @Child(name = "contentDerivative", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 4130 @Description(shortDefinition="Content derived from the basal information", formalDefinition="The minimal content derived from the basal information source at a specific stage in its lifecycle." ) 4131 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-content-derivative") 4132 protected CodeableConcept contentDerivative; 4133 4134 /** 4135 * A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels. 4136 */ 4137 @Child(name = "securityLabel", type = {Coding.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4138 @Description(shortDefinition="Security Labels that define affected resources", formalDefinition="A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels." ) 4139 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 4140 protected List<Coding> securityLabel; 4141 4142 /** 4143 * An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place. 4144 */ 4145 @Child(name = "agent", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4146 @Description(shortDefinition="Entity being ascribed responsibility", formalDefinition="An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place." ) 4147 protected List<AgentComponent> agent; 4148 4149 /** 4150 * Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness. 4151 */ 4152 @Child(name = "signer", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4153 @Description(shortDefinition="Contract Signatory", formalDefinition="Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness." ) 4154 protected List<SignatoryComponent> signer; 4155 4156 /** 4157 * Contract Valued Item List. 4158 */ 4159 @Child(name = "valuedItem", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4160 @Description(shortDefinition="Contract Valued Item List", formalDefinition="Contract Valued Item List." ) 4161 protected List<ValuedItemComponent> valuedItem; 4162 4163 /** 4164 * One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups. 4165 */ 4166 @Child(name = "term", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4167 @Description(shortDefinition="Contract Term List", formalDefinition="One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups." ) 4168 protected List<TermComponent> term; 4169 4170 /** 4171 * Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract. 4172 */ 4173 @Child(name = "binding", type = {Attachment.class, Composition.class, DocumentReference.class, QuestionnaireResponse.class}, order=19, min=0, max=1, modifier=false, summary=false) 4174 @Description(shortDefinition="Binding Contract", formalDefinition="Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract." ) 4175 protected Type binding; 4176 4177 /** 4178 * The "patient friendly language" versionof the Contract in whole or in parts. "Patient friendly language" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement. 4179 */ 4180 @Child(name = "friendly", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4181 @Description(shortDefinition="Contract Friendly Language", formalDefinition="The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement." ) 4182 protected List<FriendlyLanguageComponent> friendly; 4183 4184 /** 4185 * List of Legal expressions or representations of this Contract. 4186 */ 4187 @Child(name = "legal", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4188 @Description(shortDefinition="Contract Legal Language", formalDefinition="List of Legal expressions or representations of this Contract." ) 4189 protected List<LegalLanguageComponent> legal; 4190 4191 /** 4192 * List of Computable Policy Rule Language Representations of this Contract. 4193 */ 4194 @Child(name = "rule", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4195 @Description(shortDefinition="Computable Contract Language", formalDefinition="List of Computable Policy Rule Language Representations of this Contract." ) 4196 protected List<ComputableLanguageComponent> rule; 4197 4198 private static final long serialVersionUID = -254555038L; 4199 4200 /** 4201 * Constructor 4202 */ 4203 public Contract() { 4204 super(); 4205 } 4206 4207 /** 4208 * @return {@link #identifier} (Unique identifier for this Contract.) 4209 */ 4210 public Identifier getIdentifier() { 4211 if (this.identifier == null) 4212 if (Configuration.errorOnAutoCreate()) 4213 throw new Error("Attempt to auto-create Contract.identifier"); 4214 else if (Configuration.doAutoCreate()) 4215 this.identifier = new Identifier(); // cc 4216 return this.identifier; 4217 } 4218 4219 public boolean hasIdentifier() { 4220 return this.identifier != null && !this.identifier.isEmpty(); 4221 } 4222 4223 /** 4224 * @param value {@link #identifier} (Unique identifier for this Contract.) 4225 */ 4226 public Contract setIdentifier(Identifier value) { 4227 this.identifier = value; 4228 return this; 4229 } 4230 4231 /** 4232 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4233 */ 4234 public Enumeration<ContractStatus> getStatusElement() { 4235 if (this.status == null) 4236 if (Configuration.errorOnAutoCreate()) 4237 throw new Error("Attempt to auto-create Contract.status"); 4238 else if (Configuration.doAutoCreate()) 4239 this.status = new Enumeration<ContractStatus>(new ContractStatusEnumFactory()); // bb 4240 return this.status; 4241 } 4242 4243 public boolean hasStatusElement() { 4244 return this.status != null && !this.status.isEmpty(); 4245 } 4246 4247 public boolean hasStatus() { 4248 return this.status != null && !this.status.isEmpty(); 4249 } 4250 4251 /** 4252 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4253 */ 4254 public Contract setStatusElement(Enumeration<ContractStatus> value) { 4255 this.status = value; 4256 return this; 4257 } 4258 4259 /** 4260 * @return The status of the resource instance. 4261 */ 4262 public ContractStatus getStatus() { 4263 return this.status == null ? null : this.status.getValue(); 4264 } 4265 4266 /** 4267 * @param value The status of the resource instance. 4268 */ 4269 public Contract setStatus(ContractStatus value) { 4270 if (value == null) 4271 this.status = null; 4272 else { 4273 if (this.status == null) 4274 this.status = new Enumeration<ContractStatus>(new ContractStatusEnumFactory()); 4275 this.status.setValue(value); 4276 } 4277 return this; 4278 } 4279 4280 /** 4281 * @return {@link #issued} (When this Contract was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 4282 */ 4283 public DateTimeType getIssuedElement() { 4284 if (this.issued == null) 4285 if (Configuration.errorOnAutoCreate()) 4286 throw new Error("Attempt to auto-create Contract.issued"); 4287 else if (Configuration.doAutoCreate()) 4288 this.issued = new DateTimeType(); // bb 4289 return this.issued; 4290 } 4291 4292 public boolean hasIssuedElement() { 4293 return this.issued != null && !this.issued.isEmpty(); 4294 } 4295 4296 public boolean hasIssued() { 4297 return this.issued != null && !this.issued.isEmpty(); 4298 } 4299 4300 /** 4301 * @param value {@link #issued} (When this Contract was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 4302 */ 4303 public Contract setIssuedElement(DateTimeType value) { 4304 this.issued = value; 4305 return this; 4306 } 4307 4308 /** 4309 * @return When this Contract was issued. 4310 */ 4311 public Date getIssued() { 4312 return this.issued == null ? null : this.issued.getValue(); 4313 } 4314 4315 /** 4316 * @param value When this Contract was issued. 4317 */ 4318 public Contract setIssued(Date value) { 4319 if (value == null) 4320 this.issued = null; 4321 else { 4322 if (this.issued == null) 4323 this.issued = new DateTimeType(); 4324 this.issued.setValue(value); 4325 } 4326 return this; 4327 } 4328 4329 /** 4330 * @return {@link #applies} (Relevant time or time-period when this Contract is applicable.) 4331 */ 4332 public Period getApplies() { 4333 if (this.applies == null) 4334 if (Configuration.errorOnAutoCreate()) 4335 throw new Error("Attempt to auto-create Contract.applies"); 4336 else if (Configuration.doAutoCreate()) 4337 this.applies = new Period(); // cc 4338 return this.applies; 4339 } 4340 4341 public boolean hasApplies() { 4342 return this.applies != null && !this.applies.isEmpty(); 4343 } 4344 4345 /** 4346 * @param value {@link #applies} (Relevant time or time-period when this Contract is applicable.) 4347 */ 4348 public Contract setApplies(Period value) { 4349 this.applies = value; 4350 return this; 4351 } 4352 4353 /** 4354 * @return {@link #subject} (The target entity impacted by or of interest to parties to the agreement.) 4355 */ 4356 public List<Reference> getSubject() { 4357 if (this.subject == null) 4358 this.subject = new ArrayList<Reference>(); 4359 return this.subject; 4360 } 4361 4362 /** 4363 * @return Returns a reference to <code>this</code> for easy method chaining 4364 */ 4365 public Contract setSubject(List<Reference> theSubject) { 4366 this.subject = theSubject; 4367 return this; 4368 } 4369 4370 public boolean hasSubject() { 4371 if (this.subject == null) 4372 return false; 4373 for (Reference item : this.subject) 4374 if (!item.isEmpty()) 4375 return true; 4376 return false; 4377 } 4378 4379 public Reference addSubject() { //3 4380 Reference t = new Reference(); 4381 if (this.subject == null) 4382 this.subject = new ArrayList<Reference>(); 4383 this.subject.add(t); 4384 return t; 4385 } 4386 4387 public Contract addSubject(Reference t) { //3 4388 if (t == null) 4389 return this; 4390 if (this.subject == null) 4391 this.subject = new ArrayList<Reference>(); 4392 this.subject.add(t); 4393 return this; 4394 } 4395 4396 /** 4397 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist 4398 */ 4399 public Reference getSubjectFirstRep() { 4400 if (getSubject().isEmpty()) { 4401 addSubject(); 4402 } 4403 return getSubject().get(0); 4404 } 4405 4406 /** 4407 * @deprecated Use Reference#setResource(IBaseResource) instead 4408 */ 4409 @Deprecated 4410 public List<Resource> getSubjectTarget() { 4411 if (this.subjectTarget == null) 4412 this.subjectTarget = new ArrayList<Resource>(); 4413 return this.subjectTarget; 4414 } 4415 4416 /** 4417 * @return {@link #topic} (The matter of concern in the context of this agreement.) 4418 */ 4419 public List<Reference> getTopic() { 4420 if (this.topic == null) 4421 this.topic = new ArrayList<Reference>(); 4422 return this.topic; 4423 } 4424 4425 /** 4426 * @return Returns a reference to <code>this</code> for easy method chaining 4427 */ 4428 public Contract setTopic(List<Reference> theTopic) { 4429 this.topic = theTopic; 4430 return this; 4431 } 4432 4433 public boolean hasTopic() { 4434 if (this.topic == null) 4435 return false; 4436 for (Reference item : this.topic) 4437 if (!item.isEmpty()) 4438 return true; 4439 return false; 4440 } 4441 4442 public Reference addTopic() { //3 4443 Reference t = new Reference(); 4444 if (this.topic == null) 4445 this.topic = new ArrayList<Reference>(); 4446 this.topic.add(t); 4447 return t; 4448 } 4449 4450 public Contract addTopic(Reference t) { //3 4451 if (t == null) 4452 return this; 4453 if (this.topic == null) 4454 this.topic = new ArrayList<Reference>(); 4455 this.topic.add(t); 4456 return this; 4457 } 4458 4459 /** 4460 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 4461 */ 4462 public Reference getTopicFirstRep() { 4463 if (getTopic().isEmpty()) { 4464 addTopic(); 4465 } 4466 return getTopic().get(0); 4467 } 4468 4469 /** 4470 * @deprecated Use Reference#setResource(IBaseResource) instead 4471 */ 4472 @Deprecated 4473 public List<Resource> getTopicTarget() { 4474 if (this.topicTarget == null) 4475 this.topicTarget = new ArrayList<Resource>(); 4476 return this.topicTarget; 4477 } 4478 4479 /** 4480 * @return {@link #authority} (A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.) 4481 */ 4482 public List<Reference> getAuthority() { 4483 if (this.authority == null) 4484 this.authority = new ArrayList<Reference>(); 4485 return this.authority; 4486 } 4487 4488 /** 4489 * @return Returns a reference to <code>this</code> for easy method chaining 4490 */ 4491 public Contract setAuthority(List<Reference> theAuthority) { 4492 this.authority = theAuthority; 4493 return this; 4494 } 4495 4496 public boolean hasAuthority() { 4497 if (this.authority == null) 4498 return false; 4499 for (Reference item : this.authority) 4500 if (!item.isEmpty()) 4501 return true; 4502 return false; 4503 } 4504 4505 public Reference addAuthority() { //3 4506 Reference t = new Reference(); 4507 if (this.authority == null) 4508 this.authority = new ArrayList<Reference>(); 4509 this.authority.add(t); 4510 return t; 4511 } 4512 4513 public Contract addAuthority(Reference t) { //3 4514 if (t == null) 4515 return this; 4516 if (this.authority == null) 4517 this.authority = new ArrayList<Reference>(); 4518 this.authority.add(t); 4519 return this; 4520 } 4521 4522 /** 4523 * @return The first repetition of repeating field {@link #authority}, creating it if it does not already exist 4524 */ 4525 public Reference getAuthorityFirstRep() { 4526 if (getAuthority().isEmpty()) { 4527 addAuthority(); 4528 } 4529 return getAuthority().get(0); 4530 } 4531 4532 /** 4533 * @deprecated Use Reference#setResource(IBaseResource) instead 4534 */ 4535 @Deprecated 4536 public List<Organization> getAuthorityTarget() { 4537 if (this.authorityTarget == null) 4538 this.authorityTarget = new ArrayList<Organization>(); 4539 return this.authorityTarget; 4540 } 4541 4542 /** 4543 * @deprecated Use Reference#setResource(IBaseResource) instead 4544 */ 4545 @Deprecated 4546 public Organization addAuthorityTarget() { 4547 Organization r = new Organization(); 4548 if (this.authorityTarget == null) 4549 this.authorityTarget = new ArrayList<Organization>(); 4550 this.authorityTarget.add(r); 4551 return r; 4552 } 4553 4554 /** 4555 * @return {@link #domain} (Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.) 4556 */ 4557 public List<Reference> getDomain() { 4558 if (this.domain == null) 4559 this.domain = new ArrayList<Reference>(); 4560 return this.domain; 4561 } 4562 4563 /** 4564 * @return Returns a reference to <code>this</code> for easy method chaining 4565 */ 4566 public Contract setDomain(List<Reference> theDomain) { 4567 this.domain = theDomain; 4568 return this; 4569 } 4570 4571 public boolean hasDomain() { 4572 if (this.domain == null) 4573 return false; 4574 for (Reference item : this.domain) 4575 if (!item.isEmpty()) 4576 return true; 4577 return false; 4578 } 4579 4580 public Reference addDomain() { //3 4581 Reference t = new Reference(); 4582 if (this.domain == null) 4583 this.domain = new ArrayList<Reference>(); 4584 this.domain.add(t); 4585 return t; 4586 } 4587 4588 public Contract addDomain(Reference t) { //3 4589 if (t == null) 4590 return this; 4591 if (this.domain == null) 4592 this.domain = new ArrayList<Reference>(); 4593 this.domain.add(t); 4594 return this; 4595 } 4596 4597 /** 4598 * @return The first repetition of repeating field {@link #domain}, creating it if it does not already exist 4599 */ 4600 public Reference getDomainFirstRep() { 4601 if (getDomain().isEmpty()) { 4602 addDomain(); 4603 } 4604 return getDomain().get(0); 4605 } 4606 4607 /** 4608 * @deprecated Use Reference#setResource(IBaseResource) instead 4609 */ 4610 @Deprecated 4611 public List<Location> getDomainTarget() { 4612 if (this.domainTarget == null) 4613 this.domainTarget = new ArrayList<Location>(); 4614 return this.domainTarget; 4615 } 4616 4617 /** 4618 * @deprecated Use Reference#setResource(IBaseResource) instead 4619 */ 4620 @Deprecated 4621 public Location addDomainTarget() { 4622 Location r = new Location(); 4623 if (this.domainTarget == null) 4624 this.domainTarget = new ArrayList<Location>(); 4625 this.domainTarget.add(r); 4626 return r; 4627 } 4628 4629 /** 4630 * @return {@link #type} (Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc.) 4631 */ 4632 public CodeableConcept getType() { 4633 if (this.type == null) 4634 if (Configuration.errorOnAutoCreate()) 4635 throw new Error("Attempt to auto-create Contract.type"); 4636 else if (Configuration.doAutoCreate()) 4637 this.type = new CodeableConcept(); // cc 4638 return this.type; 4639 } 4640 4641 public boolean hasType() { 4642 return this.type != null && !this.type.isEmpty(); 4643 } 4644 4645 /** 4646 * @param value {@link #type} (Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc.) 4647 */ 4648 public Contract setType(CodeableConcept value) { 4649 this.type = value; 4650 return this; 4651 } 4652 4653 /** 4654 * @return {@link #subType} (More specific type or specialization of an overarching or more general contract such as auto insurance, home owner insurance, prenupial agreement, Advanced-Directive, or privacy consent.) 4655 */ 4656 public List<CodeableConcept> getSubType() { 4657 if (this.subType == null) 4658 this.subType = new ArrayList<CodeableConcept>(); 4659 return this.subType; 4660 } 4661 4662 /** 4663 * @return Returns a reference to <code>this</code> for easy method chaining 4664 */ 4665 public Contract setSubType(List<CodeableConcept> theSubType) { 4666 this.subType = theSubType; 4667 return this; 4668 } 4669 4670 public boolean hasSubType() { 4671 if (this.subType == null) 4672 return false; 4673 for (CodeableConcept item : this.subType) 4674 if (!item.isEmpty()) 4675 return true; 4676 return false; 4677 } 4678 4679 public CodeableConcept addSubType() { //3 4680 CodeableConcept t = new CodeableConcept(); 4681 if (this.subType == null) 4682 this.subType = new ArrayList<CodeableConcept>(); 4683 this.subType.add(t); 4684 return t; 4685 } 4686 4687 public Contract addSubType(CodeableConcept t) { //3 4688 if (t == null) 4689 return this; 4690 if (this.subType == null) 4691 this.subType = new ArrayList<CodeableConcept>(); 4692 this.subType.add(t); 4693 return this; 4694 } 4695 4696 /** 4697 * @return The first repetition of repeating field {@link #subType}, creating it if it does not already exist 4698 */ 4699 public CodeableConcept getSubTypeFirstRep() { 4700 if (getSubType().isEmpty()) { 4701 addSubType(); 4702 } 4703 return getSubType().get(0); 4704 } 4705 4706 /** 4707 * @return {@link #action} (Action stipulated by this Contract.) 4708 */ 4709 public List<CodeableConcept> getAction() { 4710 if (this.action == null) 4711 this.action = new ArrayList<CodeableConcept>(); 4712 return this.action; 4713 } 4714 4715 /** 4716 * @return Returns a reference to <code>this</code> for easy method chaining 4717 */ 4718 public Contract setAction(List<CodeableConcept> theAction) { 4719 this.action = theAction; 4720 return this; 4721 } 4722 4723 public boolean hasAction() { 4724 if (this.action == null) 4725 return false; 4726 for (CodeableConcept item : this.action) 4727 if (!item.isEmpty()) 4728 return true; 4729 return false; 4730 } 4731 4732 public CodeableConcept addAction() { //3 4733 CodeableConcept t = new CodeableConcept(); 4734 if (this.action == null) 4735 this.action = new ArrayList<CodeableConcept>(); 4736 this.action.add(t); 4737 return t; 4738 } 4739 4740 public Contract addAction(CodeableConcept t) { //3 4741 if (t == null) 4742 return this; 4743 if (this.action == null) 4744 this.action = new ArrayList<CodeableConcept>(); 4745 this.action.add(t); 4746 return this; 4747 } 4748 4749 /** 4750 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 4751 */ 4752 public CodeableConcept getActionFirstRep() { 4753 if (getAction().isEmpty()) { 4754 addAction(); 4755 } 4756 return getAction().get(0); 4757 } 4758 4759 /** 4760 * @return {@link #actionReason} (Reason for action stipulated by this Contract.) 4761 */ 4762 public List<CodeableConcept> getActionReason() { 4763 if (this.actionReason == null) 4764 this.actionReason = new ArrayList<CodeableConcept>(); 4765 return this.actionReason; 4766 } 4767 4768 /** 4769 * @return Returns a reference to <code>this</code> for easy method chaining 4770 */ 4771 public Contract setActionReason(List<CodeableConcept> theActionReason) { 4772 this.actionReason = theActionReason; 4773 return this; 4774 } 4775 4776 public boolean hasActionReason() { 4777 if (this.actionReason == null) 4778 return false; 4779 for (CodeableConcept item : this.actionReason) 4780 if (!item.isEmpty()) 4781 return true; 4782 return false; 4783 } 4784 4785 public CodeableConcept addActionReason() { //3 4786 CodeableConcept t = new CodeableConcept(); 4787 if (this.actionReason == null) 4788 this.actionReason = new ArrayList<CodeableConcept>(); 4789 this.actionReason.add(t); 4790 return t; 4791 } 4792 4793 public Contract addActionReason(CodeableConcept t) { //3 4794 if (t == null) 4795 return this; 4796 if (this.actionReason == null) 4797 this.actionReason = new ArrayList<CodeableConcept>(); 4798 this.actionReason.add(t); 4799 return this; 4800 } 4801 4802 /** 4803 * @return The first repetition of repeating field {@link #actionReason}, creating it if it does not already exist 4804 */ 4805 public CodeableConcept getActionReasonFirstRep() { 4806 if (getActionReason().isEmpty()) { 4807 addActionReason(); 4808 } 4809 return getActionReason().get(0); 4810 } 4811 4812 /** 4813 * @return {@link #decisionType} (The type of decision made by a grantor with respect to an offer made by a grantee.) 4814 */ 4815 public CodeableConcept getDecisionType() { 4816 if (this.decisionType == null) 4817 if (Configuration.errorOnAutoCreate()) 4818 throw new Error("Attempt to auto-create Contract.decisionType"); 4819 else if (Configuration.doAutoCreate()) 4820 this.decisionType = new CodeableConcept(); // cc 4821 return this.decisionType; 4822 } 4823 4824 public boolean hasDecisionType() { 4825 return this.decisionType != null && !this.decisionType.isEmpty(); 4826 } 4827 4828 /** 4829 * @param value {@link #decisionType} (The type of decision made by a grantor with respect to an offer made by a grantee.) 4830 */ 4831 public Contract setDecisionType(CodeableConcept value) { 4832 this.decisionType = value; 4833 return this; 4834 } 4835 4836 /** 4837 * @return {@link #contentDerivative} (The minimal content derived from the basal information source at a specific stage in its lifecycle.) 4838 */ 4839 public CodeableConcept getContentDerivative() { 4840 if (this.contentDerivative == null) 4841 if (Configuration.errorOnAutoCreate()) 4842 throw new Error("Attempt to auto-create Contract.contentDerivative"); 4843 else if (Configuration.doAutoCreate()) 4844 this.contentDerivative = new CodeableConcept(); // cc 4845 return this.contentDerivative; 4846 } 4847 4848 public boolean hasContentDerivative() { 4849 return this.contentDerivative != null && !this.contentDerivative.isEmpty(); 4850 } 4851 4852 /** 4853 * @param value {@link #contentDerivative} (The minimal content derived from the basal information source at a specific stage in its lifecycle.) 4854 */ 4855 public Contract setContentDerivative(CodeableConcept value) { 4856 this.contentDerivative = value; 4857 return this; 4858 } 4859 4860 /** 4861 * @return {@link #securityLabel} (A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.) 4862 */ 4863 public List<Coding> getSecurityLabel() { 4864 if (this.securityLabel == null) 4865 this.securityLabel = new ArrayList<Coding>(); 4866 return this.securityLabel; 4867 } 4868 4869 /** 4870 * @return Returns a reference to <code>this</code> for easy method chaining 4871 */ 4872 public Contract setSecurityLabel(List<Coding> theSecurityLabel) { 4873 this.securityLabel = theSecurityLabel; 4874 return this; 4875 } 4876 4877 public boolean hasSecurityLabel() { 4878 if (this.securityLabel == null) 4879 return false; 4880 for (Coding item : this.securityLabel) 4881 if (!item.isEmpty()) 4882 return true; 4883 return false; 4884 } 4885 4886 public Coding addSecurityLabel() { //3 4887 Coding t = new Coding(); 4888 if (this.securityLabel == null) 4889 this.securityLabel = new ArrayList<Coding>(); 4890 this.securityLabel.add(t); 4891 return t; 4892 } 4893 4894 public Contract addSecurityLabel(Coding t) { //3 4895 if (t == null) 4896 return this; 4897 if (this.securityLabel == null) 4898 this.securityLabel = new ArrayList<Coding>(); 4899 this.securityLabel.add(t); 4900 return this; 4901 } 4902 4903 /** 4904 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 4905 */ 4906 public Coding getSecurityLabelFirstRep() { 4907 if (getSecurityLabel().isEmpty()) { 4908 addSecurityLabel(); 4909 } 4910 return getSecurityLabel().get(0); 4911 } 4912 4913 /** 4914 * @return {@link #agent} (An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.) 4915 */ 4916 public List<AgentComponent> getAgent() { 4917 if (this.agent == null) 4918 this.agent = new ArrayList<AgentComponent>(); 4919 return this.agent; 4920 } 4921 4922 /** 4923 * @return Returns a reference to <code>this</code> for easy method chaining 4924 */ 4925 public Contract setAgent(List<AgentComponent> theAgent) { 4926 this.agent = theAgent; 4927 return this; 4928 } 4929 4930 public boolean hasAgent() { 4931 if (this.agent == null) 4932 return false; 4933 for (AgentComponent item : this.agent) 4934 if (!item.isEmpty()) 4935 return true; 4936 return false; 4937 } 4938 4939 public AgentComponent addAgent() { //3 4940 AgentComponent t = new AgentComponent(); 4941 if (this.agent == null) 4942 this.agent = new ArrayList<AgentComponent>(); 4943 this.agent.add(t); 4944 return t; 4945 } 4946 4947 public Contract addAgent(AgentComponent t) { //3 4948 if (t == null) 4949 return this; 4950 if (this.agent == null) 4951 this.agent = new ArrayList<AgentComponent>(); 4952 this.agent.add(t); 4953 return this; 4954 } 4955 4956 /** 4957 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist 4958 */ 4959 public AgentComponent getAgentFirstRep() { 4960 if (getAgent().isEmpty()) { 4961 addAgent(); 4962 } 4963 return getAgent().get(0); 4964 } 4965 4966 /** 4967 * @return {@link #signer} (Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.) 4968 */ 4969 public List<SignatoryComponent> getSigner() { 4970 if (this.signer == null) 4971 this.signer = new ArrayList<SignatoryComponent>(); 4972 return this.signer; 4973 } 4974 4975 /** 4976 * @return Returns a reference to <code>this</code> for easy method chaining 4977 */ 4978 public Contract setSigner(List<SignatoryComponent> theSigner) { 4979 this.signer = theSigner; 4980 return this; 4981 } 4982 4983 public boolean hasSigner() { 4984 if (this.signer == null) 4985 return false; 4986 for (SignatoryComponent item : this.signer) 4987 if (!item.isEmpty()) 4988 return true; 4989 return false; 4990 } 4991 4992 public SignatoryComponent addSigner() { //3 4993 SignatoryComponent t = new SignatoryComponent(); 4994 if (this.signer == null) 4995 this.signer = new ArrayList<SignatoryComponent>(); 4996 this.signer.add(t); 4997 return t; 4998 } 4999 5000 public Contract addSigner(SignatoryComponent t) { //3 5001 if (t == null) 5002 return this; 5003 if (this.signer == null) 5004 this.signer = new ArrayList<SignatoryComponent>(); 5005 this.signer.add(t); 5006 return this; 5007 } 5008 5009 /** 5010 * @return The first repetition of repeating field {@link #signer}, creating it if it does not already exist 5011 */ 5012 public SignatoryComponent getSignerFirstRep() { 5013 if (getSigner().isEmpty()) { 5014 addSigner(); 5015 } 5016 return getSigner().get(0); 5017 } 5018 5019 /** 5020 * @return {@link #valuedItem} (Contract Valued Item List.) 5021 */ 5022 public List<ValuedItemComponent> getValuedItem() { 5023 if (this.valuedItem == null) 5024 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5025 return this.valuedItem; 5026 } 5027 5028 /** 5029 * @return Returns a reference to <code>this</code> for easy method chaining 5030 */ 5031 public Contract setValuedItem(List<ValuedItemComponent> theValuedItem) { 5032 this.valuedItem = theValuedItem; 5033 return this; 5034 } 5035 5036 public boolean hasValuedItem() { 5037 if (this.valuedItem == null) 5038 return false; 5039 for (ValuedItemComponent item : this.valuedItem) 5040 if (!item.isEmpty()) 5041 return true; 5042 return false; 5043 } 5044 5045 public ValuedItemComponent addValuedItem() { //3 5046 ValuedItemComponent t = new ValuedItemComponent(); 5047 if (this.valuedItem == null) 5048 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5049 this.valuedItem.add(t); 5050 return t; 5051 } 5052 5053 public Contract addValuedItem(ValuedItemComponent t) { //3 5054 if (t == null) 5055 return this; 5056 if (this.valuedItem == null) 5057 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5058 this.valuedItem.add(t); 5059 return this; 5060 } 5061 5062 /** 5063 * @return The first repetition of repeating field {@link #valuedItem}, creating it if it does not already exist 5064 */ 5065 public ValuedItemComponent getValuedItemFirstRep() { 5066 if (getValuedItem().isEmpty()) { 5067 addValuedItem(); 5068 } 5069 return getValuedItem().get(0); 5070 } 5071 5072 /** 5073 * @return {@link #term} (One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.) 5074 */ 5075 public List<TermComponent> getTerm() { 5076 if (this.term == null) 5077 this.term = new ArrayList<TermComponent>(); 5078 return this.term; 5079 } 5080 5081 /** 5082 * @return Returns a reference to <code>this</code> for easy method chaining 5083 */ 5084 public Contract setTerm(List<TermComponent> theTerm) { 5085 this.term = theTerm; 5086 return this; 5087 } 5088 5089 public boolean hasTerm() { 5090 if (this.term == null) 5091 return false; 5092 for (TermComponent item : this.term) 5093 if (!item.isEmpty()) 5094 return true; 5095 return false; 5096 } 5097 5098 public TermComponent addTerm() { //3 5099 TermComponent t = new TermComponent(); 5100 if (this.term == null) 5101 this.term = new ArrayList<TermComponent>(); 5102 this.term.add(t); 5103 return t; 5104 } 5105 5106 public Contract addTerm(TermComponent t) { //3 5107 if (t == null) 5108 return this; 5109 if (this.term == null) 5110 this.term = new ArrayList<TermComponent>(); 5111 this.term.add(t); 5112 return this; 5113 } 5114 5115 /** 5116 * @return The first repetition of repeating field {@link #term}, creating it if it does not already exist 5117 */ 5118 public TermComponent getTermFirstRep() { 5119 if (getTerm().isEmpty()) { 5120 addTerm(); 5121 } 5122 return getTerm().get(0); 5123 } 5124 5125 /** 5126 * @return {@link #binding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 5127 */ 5128 public Type getBinding() { 5129 return this.binding; 5130 } 5131 5132 /** 5133 * @return {@link #binding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 5134 */ 5135 public Attachment getBindingAttachment() throws FHIRException { 5136 if (this.binding == null) 5137 return null; 5138 if (!(this.binding instanceof Attachment)) 5139 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.binding.getClass().getName()+" was encountered"); 5140 return (Attachment) this.binding; 5141 } 5142 5143 public boolean hasBindingAttachment() { 5144 return this != null && this.binding instanceof Attachment; 5145 } 5146 5147 /** 5148 * @return {@link #binding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 5149 */ 5150 public Reference getBindingReference() throws FHIRException { 5151 if (this.binding == null) 5152 return null; 5153 if (!(this.binding instanceof Reference)) 5154 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.binding.getClass().getName()+" was encountered"); 5155 return (Reference) this.binding; 5156 } 5157 5158 public boolean hasBindingReference() { 5159 return this != null && this.binding instanceof Reference; 5160 } 5161 5162 public boolean hasBinding() { 5163 return this.binding != null && !this.binding.isEmpty(); 5164 } 5165 5166 /** 5167 * @param value {@link #binding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 5168 */ 5169 public Contract setBinding(Type value) throws FHIRFormatError { 5170 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 5171 throw new FHIRFormatError("Not the right type for Contract.binding[x]: "+value.fhirType()); 5172 this.binding = value; 5173 return this; 5174 } 5175 5176 /** 5177 * @return {@link #friendly} (The "patient friendly language" versionof the Contract in whole or in parts. "Patient friendly language" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.) 5178 */ 5179 public List<FriendlyLanguageComponent> getFriendly() { 5180 if (this.friendly == null) 5181 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 5182 return this.friendly; 5183 } 5184 5185 /** 5186 * @return Returns a reference to <code>this</code> for easy method chaining 5187 */ 5188 public Contract setFriendly(List<FriendlyLanguageComponent> theFriendly) { 5189 this.friendly = theFriendly; 5190 return this; 5191 } 5192 5193 public boolean hasFriendly() { 5194 if (this.friendly == null) 5195 return false; 5196 for (FriendlyLanguageComponent item : this.friendly) 5197 if (!item.isEmpty()) 5198 return true; 5199 return false; 5200 } 5201 5202 public FriendlyLanguageComponent addFriendly() { //3 5203 FriendlyLanguageComponent t = new FriendlyLanguageComponent(); 5204 if (this.friendly == null) 5205 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 5206 this.friendly.add(t); 5207 return t; 5208 } 5209 5210 public Contract addFriendly(FriendlyLanguageComponent t) { //3 5211 if (t == null) 5212 return this; 5213 if (this.friendly == null) 5214 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 5215 this.friendly.add(t); 5216 return this; 5217 } 5218 5219 /** 5220 * @return The first repetition of repeating field {@link #friendly}, creating it if it does not already exist 5221 */ 5222 public FriendlyLanguageComponent getFriendlyFirstRep() { 5223 if (getFriendly().isEmpty()) { 5224 addFriendly(); 5225 } 5226 return getFriendly().get(0); 5227 } 5228 5229 /** 5230 * @return {@link #legal} (List of Legal expressions or representations of this Contract.) 5231 */ 5232 public List<LegalLanguageComponent> getLegal() { 5233 if (this.legal == null) 5234 this.legal = new ArrayList<LegalLanguageComponent>(); 5235 return this.legal; 5236 } 5237 5238 /** 5239 * @return Returns a reference to <code>this</code> for easy method chaining 5240 */ 5241 public Contract setLegal(List<LegalLanguageComponent> theLegal) { 5242 this.legal = theLegal; 5243 return this; 5244 } 5245 5246 public boolean hasLegal() { 5247 if (this.legal == null) 5248 return false; 5249 for (LegalLanguageComponent item : this.legal) 5250 if (!item.isEmpty()) 5251 return true; 5252 return false; 5253 } 5254 5255 public LegalLanguageComponent addLegal() { //3 5256 LegalLanguageComponent t = new LegalLanguageComponent(); 5257 if (this.legal == null) 5258 this.legal = new ArrayList<LegalLanguageComponent>(); 5259 this.legal.add(t); 5260 return t; 5261 } 5262 5263 public Contract addLegal(LegalLanguageComponent t) { //3 5264 if (t == null) 5265 return this; 5266 if (this.legal == null) 5267 this.legal = new ArrayList<LegalLanguageComponent>(); 5268 this.legal.add(t); 5269 return this; 5270 } 5271 5272 /** 5273 * @return The first repetition of repeating field {@link #legal}, creating it if it does not already exist 5274 */ 5275 public LegalLanguageComponent getLegalFirstRep() { 5276 if (getLegal().isEmpty()) { 5277 addLegal(); 5278 } 5279 return getLegal().get(0); 5280 } 5281 5282 /** 5283 * @return {@link #rule} (List of Computable Policy Rule Language Representations of this Contract.) 5284 */ 5285 public List<ComputableLanguageComponent> getRule() { 5286 if (this.rule == null) 5287 this.rule = new ArrayList<ComputableLanguageComponent>(); 5288 return this.rule; 5289 } 5290 5291 /** 5292 * @return Returns a reference to <code>this</code> for easy method chaining 5293 */ 5294 public Contract setRule(List<ComputableLanguageComponent> theRule) { 5295 this.rule = theRule; 5296 return this; 5297 } 5298 5299 public boolean hasRule() { 5300 if (this.rule == null) 5301 return false; 5302 for (ComputableLanguageComponent item : this.rule) 5303 if (!item.isEmpty()) 5304 return true; 5305 return false; 5306 } 5307 5308 public ComputableLanguageComponent addRule() { //3 5309 ComputableLanguageComponent t = new ComputableLanguageComponent(); 5310 if (this.rule == null) 5311 this.rule = new ArrayList<ComputableLanguageComponent>(); 5312 this.rule.add(t); 5313 return t; 5314 } 5315 5316 public Contract addRule(ComputableLanguageComponent t) { //3 5317 if (t == null) 5318 return this; 5319 if (this.rule == null) 5320 this.rule = new ArrayList<ComputableLanguageComponent>(); 5321 this.rule.add(t); 5322 return this; 5323 } 5324 5325 /** 5326 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist 5327 */ 5328 public ComputableLanguageComponent getRuleFirstRep() { 5329 if (getRule().isEmpty()) { 5330 addRule(); 5331 } 5332 return getRule().get(0); 5333 } 5334 5335 protected void listChildren(List<Property> children) { 5336 super.listChildren(children); 5337 children.add(new Property("identifier", "Identifier", "Unique identifier for this Contract.", 0, 1, identifier)); 5338 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 5339 children.add(new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued)); 5340 children.add(new Property("applies", "Period", "Relevant time or time-period when this Contract is applicable.", 0, 1, applies)); 5341 children.add(new Property("subject", "Reference(Any)", "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, subject)); 5342 children.add(new Property("topic", "Reference(Any)", "The matter of concern in the context of this agreement.", 0, java.lang.Integer.MAX_VALUE, topic)); 5343 children.add(new Property("authority", "Reference(Organization)", "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 0, java.lang.Integer.MAX_VALUE, authority)); 5344 children.add(new Property("domain", "Reference(Location)", "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 0, java.lang.Integer.MAX_VALUE, domain)); 5345 children.add(new Property("type", "CodeableConcept", "Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc.", 0, 1, type)); 5346 children.add(new Property("subType", "CodeableConcept", "More specific type or specialization of an overarching or more general contract such as auto insurance, home owner insurance, prenupial agreement, Advanced-Directive, or privacy consent.", 0, java.lang.Integer.MAX_VALUE, subType)); 5347 children.add(new Property("action", "CodeableConcept", "Action stipulated by this Contract.", 0, java.lang.Integer.MAX_VALUE, action)); 5348 children.add(new Property("actionReason", "CodeableConcept", "Reason for action stipulated by this Contract.", 0, java.lang.Integer.MAX_VALUE, actionReason)); 5349 children.add(new Property("decisionType", "CodeableConcept", "The type of decision made by a grantor with respect to an offer made by a grantee.", 0, 1, decisionType)); 5350 children.add(new Property("contentDerivative", "CodeableConcept", "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, contentDerivative)); 5351 children.add(new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 5352 children.add(new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent)); 5353 children.add(new Property("signer", "", "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 0, java.lang.Integer.MAX_VALUE, signer)); 5354 children.add(new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem)); 5355 children.add(new Property("term", "", "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 0, java.lang.Integer.MAX_VALUE, term)); 5356 children.add(new Property("binding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, binding)); 5357 children.add(new Property("friendly", "", "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 0, java.lang.Integer.MAX_VALUE, friendly)); 5358 children.add(new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, legal)); 5359 children.add(new Property("rule", "", "List of Computable Policy Rule Language Representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, rule)); 5360 } 5361 5362 @Override 5363 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5364 switch (_hash) { 5365 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this Contract.", 0, 1, identifier); 5366 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 5367 case -1179159893: /*issued*/ return new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued); 5368 case -793235316: /*applies*/ return new Property("applies", "Period", "Relevant time or time-period when this Contract is applicable.", 0, 1, applies); 5369 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, subject); 5370 case 110546223: /*topic*/ return new Property("topic", "Reference(Any)", "The matter of concern in the context of this agreement.", 0, java.lang.Integer.MAX_VALUE, topic); 5371 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 0, java.lang.Integer.MAX_VALUE, authority); 5372 case -1326197564: /*domain*/ return new Property("domain", "Reference(Location)", "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 0, java.lang.Integer.MAX_VALUE, domain); 5373 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc.", 0, 1, type); 5374 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "More specific type or specialization of an overarching or more general contract such as auto insurance, home owner insurance, prenupial agreement, Advanced-Directive, or privacy consent.", 0, java.lang.Integer.MAX_VALUE, subType); 5375 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Action stipulated by this Contract.", 0, java.lang.Integer.MAX_VALUE, action); 5376 case 1465121818: /*actionReason*/ return new Property("actionReason", "CodeableConcept", "Reason for action stipulated by this Contract.", 0, java.lang.Integer.MAX_VALUE, actionReason); 5377 case 676128054: /*decisionType*/ return new Property("decisionType", "CodeableConcept", "The type of decision made by a grantor with respect to an offer made by a grantee.", 0, 1, decisionType); 5378 case -92412192: /*contentDerivative*/ return new Property("contentDerivative", "CodeableConcept", "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, contentDerivative); 5379 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A set of security labels that define which resources are controlled by this consent. If more than one label is specified, all resources must have all the specified labels.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 5380 case 92750597: /*agent*/ return new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent); 5381 case -902467798: /*signer*/ return new Property("signer", "", "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 0, java.lang.Integer.MAX_VALUE, signer); 5382 case 2046675654: /*valuedItem*/ return new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem); 5383 case 3556460: /*term*/ return new Property("term", "", "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 0, java.lang.Integer.MAX_VALUE, term); 5384 case 1514826715: /*binding[x]*/ return new Property("binding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, binding); 5385 case -108220795: /*binding*/ return new Property("binding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, binding); 5386 case 1218789768: /*bindingAttachment*/ return new Property("binding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, binding); 5387 case 424425030: /*bindingReference*/ return new Property("binding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, binding); 5388 case -1423054677: /*friendly*/ return new Property("friendly", "", "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 0, java.lang.Integer.MAX_VALUE, friendly); 5389 case 102851257: /*legal*/ return new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, legal); 5390 case 3512060: /*rule*/ return new Property("rule", "", "List of Computable Policy Rule Language Representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, rule); 5391 default: return super.getNamedProperty(_hash, _name, _checkValid); 5392 } 5393 5394 } 5395 5396 @Override 5397 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5398 switch (hash) { 5399 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 5400 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ContractStatus> 5401 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // DateTimeType 5402 case -793235316: /*applies*/ return this.applies == null ? new Base[0] : new Base[] {this.applies}; // Period 5403 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 5404 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // Reference 5405 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : this.authority.toArray(new Base[this.authority.size()]); // Reference 5406 case -1326197564: /*domain*/ return this.domain == null ? new Base[0] : this.domain.toArray(new Base[this.domain.size()]); // Reference 5407 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5408 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 5409 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 5410 case 1465121818: /*actionReason*/ return this.actionReason == null ? new Base[0] : this.actionReason.toArray(new Base[this.actionReason.size()]); // CodeableConcept 5411 case 676128054: /*decisionType*/ return this.decisionType == null ? new Base[0] : new Base[] {this.decisionType}; // CodeableConcept 5412 case -92412192: /*contentDerivative*/ return this.contentDerivative == null ? new Base[0] : new Base[] {this.contentDerivative}; // CodeableConcept 5413 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 5414 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // AgentComponent 5415 case -902467798: /*signer*/ return this.signer == null ? new Base[0] : this.signer.toArray(new Base[this.signer.size()]); // SignatoryComponent 5416 case 2046675654: /*valuedItem*/ return this.valuedItem == null ? new Base[0] : this.valuedItem.toArray(new Base[this.valuedItem.size()]); // ValuedItemComponent 5417 case 3556460: /*term*/ return this.term == null ? new Base[0] : this.term.toArray(new Base[this.term.size()]); // TermComponent 5418 case -108220795: /*binding*/ return this.binding == null ? new Base[0] : new Base[] {this.binding}; // Type 5419 case -1423054677: /*friendly*/ return this.friendly == null ? new Base[0] : this.friendly.toArray(new Base[this.friendly.size()]); // FriendlyLanguageComponent 5420 case 102851257: /*legal*/ return this.legal == null ? new Base[0] : this.legal.toArray(new Base[this.legal.size()]); // LegalLanguageComponent 5421 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // ComputableLanguageComponent 5422 default: return super.getProperty(hash, name, checkValid); 5423 } 5424 5425 } 5426 5427 @Override 5428 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5429 switch (hash) { 5430 case -1618432855: // identifier 5431 this.identifier = castToIdentifier(value); // Identifier 5432 return value; 5433 case -892481550: // status 5434 value = new ContractStatusEnumFactory().fromType(castToCode(value)); 5435 this.status = (Enumeration) value; // Enumeration<ContractStatus> 5436 return value; 5437 case -1179159893: // issued 5438 this.issued = castToDateTime(value); // DateTimeType 5439 return value; 5440 case -793235316: // applies 5441 this.applies = castToPeriod(value); // Period 5442 return value; 5443 case -1867885268: // subject 5444 this.getSubject().add(castToReference(value)); // Reference 5445 return value; 5446 case 110546223: // topic 5447 this.getTopic().add(castToReference(value)); // Reference 5448 return value; 5449 case 1475610435: // authority 5450 this.getAuthority().add(castToReference(value)); // Reference 5451 return value; 5452 case -1326197564: // domain 5453 this.getDomain().add(castToReference(value)); // Reference 5454 return value; 5455 case 3575610: // type 5456 this.type = castToCodeableConcept(value); // CodeableConcept 5457 return value; 5458 case -1868521062: // subType 5459 this.getSubType().add(castToCodeableConcept(value)); // CodeableConcept 5460 return value; 5461 case -1422950858: // action 5462 this.getAction().add(castToCodeableConcept(value)); // CodeableConcept 5463 return value; 5464 case 1465121818: // actionReason 5465 this.getActionReason().add(castToCodeableConcept(value)); // CodeableConcept 5466 return value; 5467 case 676128054: // decisionType 5468 this.decisionType = castToCodeableConcept(value); // CodeableConcept 5469 return value; 5470 case -92412192: // contentDerivative 5471 this.contentDerivative = castToCodeableConcept(value); // CodeableConcept 5472 return value; 5473 case -722296940: // securityLabel 5474 this.getSecurityLabel().add(castToCoding(value)); // Coding 5475 return value; 5476 case 92750597: // agent 5477 this.getAgent().add((AgentComponent) value); // AgentComponent 5478 return value; 5479 case -902467798: // signer 5480 this.getSigner().add((SignatoryComponent) value); // SignatoryComponent 5481 return value; 5482 case 2046675654: // valuedItem 5483 this.getValuedItem().add((ValuedItemComponent) value); // ValuedItemComponent 5484 return value; 5485 case 3556460: // term 5486 this.getTerm().add((TermComponent) value); // TermComponent 5487 return value; 5488 case -108220795: // binding 5489 this.binding = castToType(value); // Type 5490 return value; 5491 case -1423054677: // friendly 5492 this.getFriendly().add((FriendlyLanguageComponent) value); // FriendlyLanguageComponent 5493 return value; 5494 case 102851257: // legal 5495 this.getLegal().add((LegalLanguageComponent) value); // LegalLanguageComponent 5496 return value; 5497 case 3512060: // rule 5498 this.getRule().add((ComputableLanguageComponent) value); // ComputableLanguageComponent 5499 return value; 5500 default: return super.setProperty(hash, name, value); 5501 } 5502 5503 } 5504 5505 @Override 5506 public Base setProperty(String name, Base value) throws FHIRException { 5507 if (name.equals("identifier")) { 5508 this.identifier = castToIdentifier(value); // Identifier 5509 } else if (name.equals("status")) { 5510 value = new ContractStatusEnumFactory().fromType(castToCode(value)); 5511 this.status = (Enumeration) value; // Enumeration<ContractStatus> 5512 } else if (name.equals("issued")) { 5513 this.issued = castToDateTime(value); // DateTimeType 5514 } else if (name.equals("applies")) { 5515 this.applies = castToPeriod(value); // Period 5516 } else if (name.equals("subject")) { 5517 this.getSubject().add(castToReference(value)); 5518 } else if (name.equals("topic")) { 5519 this.getTopic().add(castToReference(value)); 5520 } else if (name.equals("authority")) { 5521 this.getAuthority().add(castToReference(value)); 5522 } else if (name.equals("domain")) { 5523 this.getDomain().add(castToReference(value)); 5524 } else if (name.equals("type")) { 5525 this.type = castToCodeableConcept(value); // CodeableConcept 5526 } else if (name.equals("subType")) { 5527 this.getSubType().add(castToCodeableConcept(value)); 5528 } else if (name.equals("action")) { 5529 this.getAction().add(castToCodeableConcept(value)); 5530 } else if (name.equals("actionReason")) { 5531 this.getActionReason().add(castToCodeableConcept(value)); 5532 } else if (name.equals("decisionType")) { 5533 this.decisionType = castToCodeableConcept(value); // CodeableConcept 5534 } else if (name.equals("contentDerivative")) { 5535 this.contentDerivative = castToCodeableConcept(value); // CodeableConcept 5536 } else if (name.equals("securityLabel")) { 5537 this.getSecurityLabel().add(castToCoding(value)); 5538 } else if (name.equals("agent")) { 5539 this.getAgent().add((AgentComponent) value); 5540 } else if (name.equals("signer")) { 5541 this.getSigner().add((SignatoryComponent) value); 5542 } else if (name.equals("valuedItem")) { 5543 this.getValuedItem().add((ValuedItemComponent) value); 5544 } else if (name.equals("term")) { 5545 this.getTerm().add((TermComponent) value); 5546 } else if (name.equals("binding[x]")) { 5547 this.binding = castToType(value); // Type 5548 } else if (name.equals("friendly")) { 5549 this.getFriendly().add((FriendlyLanguageComponent) value); 5550 } else if (name.equals("legal")) { 5551 this.getLegal().add((LegalLanguageComponent) value); 5552 } else if (name.equals("rule")) { 5553 this.getRule().add((ComputableLanguageComponent) value); 5554 } else 5555 return super.setProperty(name, value); 5556 return value; 5557 } 5558 5559 @Override 5560 public Base makeProperty(int hash, String name) throws FHIRException { 5561 switch (hash) { 5562 case -1618432855: return getIdentifier(); 5563 case -892481550: return getStatusElement(); 5564 case -1179159893: return getIssuedElement(); 5565 case -793235316: return getApplies(); 5566 case -1867885268: return addSubject(); 5567 case 110546223: return addTopic(); 5568 case 1475610435: return addAuthority(); 5569 case -1326197564: return addDomain(); 5570 case 3575610: return getType(); 5571 case -1868521062: return addSubType(); 5572 case -1422950858: return addAction(); 5573 case 1465121818: return addActionReason(); 5574 case 676128054: return getDecisionType(); 5575 case -92412192: return getContentDerivative(); 5576 case -722296940: return addSecurityLabel(); 5577 case 92750597: return addAgent(); 5578 case -902467798: return addSigner(); 5579 case 2046675654: return addValuedItem(); 5580 case 3556460: return addTerm(); 5581 case 1514826715: return getBinding(); 5582 case -108220795: return getBinding(); 5583 case -1423054677: return addFriendly(); 5584 case 102851257: return addLegal(); 5585 case 3512060: return addRule(); 5586 default: return super.makeProperty(hash, name); 5587 } 5588 5589 } 5590 5591 @Override 5592 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5593 switch (hash) { 5594 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5595 case -892481550: /*status*/ return new String[] {"code"}; 5596 case -1179159893: /*issued*/ return new String[] {"dateTime"}; 5597 case -793235316: /*applies*/ return new String[] {"Period"}; 5598 case -1867885268: /*subject*/ return new String[] {"Reference"}; 5599 case 110546223: /*topic*/ return new String[] {"Reference"}; 5600 case 1475610435: /*authority*/ return new String[] {"Reference"}; 5601 case -1326197564: /*domain*/ return new String[] {"Reference"}; 5602 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 5603 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 5604 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 5605 case 1465121818: /*actionReason*/ return new String[] {"CodeableConcept"}; 5606 case 676128054: /*decisionType*/ return new String[] {"CodeableConcept"}; 5607 case -92412192: /*contentDerivative*/ return new String[] {"CodeableConcept"}; 5608 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 5609 case 92750597: /*agent*/ return new String[] {}; 5610 case -902467798: /*signer*/ return new String[] {}; 5611 case 2046675654: /*valuedItem*/ return new String[] {}; 5612 case 3556460: /*term*/ return new String[] {}; 5613 case -108220795: /*binding*/ return new String[] {"Attachment", "Reference"}; 5614 case -1423054677: /*friendly*/ return new String[] {}; 5615 case 102851257: /*legal*/ return new String[] {}; 5616 case 3512060: /*rule*/ return new String[] {}; 5617 default: return super.getTypesForProperty(hash, name); 5618 } 5619 5620 } 5621 5622 @Override 5623 public Base addChild(String name) throws FHIRException { 5624 if (name.equals("identifier")) { 5625 this.identifier = new Identifier(); 5626 return this.identifier; 5627 } 5628 else if (name.equals("status")) { 5629 throw new FHIRException("Cannot call addChild on a singleton property Contract.status"); 5630 } 5631 else if (name.equals("issued")) { 5632 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 5633 } 5634 else if (name.equals("applies")) { 5635 this.applies = new Period(); 5636 return this.applies; 5637 } 5638 else if (name.equals("subject")) { 5639 return addSubject(); 5640 } 5641 else if (name.equals("topic")) { 5642 return addTopic(); 5643 } 5644 else if (name.equals("authority")) { 5645 return addAuthority(); 5646 } 5647 else if (name.equals("domain")) { 5648 return addDomain(); 5649 } 5650 else if (name.equals("type")) { 5651 this.type = new CodeableConcept(); 5652 return this.type; 5653 } 5654 else if (name.equals("subType")) { 5655 return addSubType(); 5656 } 5657 else if (name.equals("action")) { 5658 return addAction(); 5659 } 5660 else if (name.equals("actionReason")) { 5661 return addActionReason(); 5662 } 5663 else if (name.equals("decisionType")) { 5664 this.decisionType = new CodeableConcept(); 5665 return this.decisionType; 5666 } 5667 else if (name.equals("contentDerivative")) { 5668 this.contentDerivative = new CodeableConcept(); 5669 return this.contentDerivative; 5670 } 5671 else if (name.equals("securityLabel")) { 5672 return addSecurityLabel(); 5673 } 5674 else if (name.equals("agent")) { 5675 return addAgent(); 5676 } 5677 else if (name.equals("signer")) { 5678 return addSigner(); 5679 } 5680 else if (name.equals("valuedItem")) { 5681 return addValuedItem(); 5682 } 5683 else if (name.equals("term")) { 5684 return addTerm(); 5685 } 5686 else if (name.equals("bindingAttachment")) { 5687 this.binding = new Attachment(); 5688 return this.binding; 5689 } 5690 else if (name.equals("bindingReference")) { 5691 this.binding = new Reference(); 5692 return this.binding; 5693 } 5694 else if (name.equals("friendly")) { 5695 return addFriendly(); 5696 } 5697 else if (name.equals("legal")) { 5698 return addLegal(); 5699 } 5700 else if (name.equals("rule")) { 5701 return addRule(); 5702 } 5703 else 5704 return super.addChild(name); 5705 } 5706 5707 public String fhirType() { 5708 return "Contract"; 5709 5710 } 5711 5712 public Contract copy() { 5713 Contract dst = new Contract(); 5714 copyValues(dst); 5715 dst.identifier = identifier == null ? null : identifier.copy(); 5716 dst.status = status == null ? null : status.copy(); 5717 dst.issued = issued == null ? null : issued.copy(); 5718 dst.applies = applies == null ? null : applies.copy(); 5719 if (subject != null) { 5720 dst.subject = new ArrayList<Reference>(); 5721 for (Reference i : subject) 5722 dst.subject.add(i.copy()); 5723 }; 5724 if (topic != null) { 5725 dst.topic = new ArrayList<Reference>(); 5726 for (Reference i : topic) 5727 dst.topic.add(i.copy()); 5728 }; 5729 if (authority != null) { 5730 dst.authority = new ArrayList<Reference>(); 5731 for (Reference i : authority) 5732 dst.authority.add(i.copy()); 5733 }; 5734 if (domain != null) { 5735 dst.domain = new ArrayList<Reference>(); 5736 for (Reference i : domain) 5737 dst.domain.add(i.copy()); 5738 }; 5739 dst.type = type == null ? null : type.copy(); 5740 if (subType != null) { 5741 dst.subType = new ArrayList<CodeableConcept>(); 5742 for (CodeableConcept i : subType) 5743 dst.subType.add(i.copy()); 5744 }; 5745 if (action != null) { 5746 dst.action = new ArrayList<CodeableConcept>(); 5747 for (CodeableConcept i : action) 5748 dst.action.add(i.copy()); 5749 }; 5750 if (actionReason != null) { 5751 dst.actionReason = new ArrayList<CodeableConcept>(); 5752 for (CodeableConcept i : actionReason) 5753 dst.actionReason.add(i.copy()); 5754 }; 5755 dst.decisionType = decisionType == null ? null : decisionType.copy(); 5756 dst.contentDerivative = contentDerivative == null ? null : contentDerivative.copy(); 5757 if (securityLabel != null) { 5758 dst.securityLabel = new ArrayList<Coding>(); 5759 for (Coding i : securityLabel) 5760 dst.securityLabel.add(i.copy()); 5761 }; 5762 if (agent != null) { 5763 dst.agent = new ArrayList<AgentComponent>(); 5764 for (AgentComponent i : agent) 5765 dst.agent.add(i.copy()); 5766 }; 5767 if (signer != null) { 5768 dst.signer = new ArrayList<SignatoryComponent>(); 5769 for (SignatoryComponent i : signer) 5770 dst.signer.add(i.copy()); 5771 }; 5772 if (valuedItem != null) { 5773 dst.valuedItem = new ArrayList<ValuedItemComponent>(); 5774 for (ValuedItemComponent i : valuedItem) 5775 dst.valuedItem.add(i.copy()); 5776 }; 5777 if (term != null) { 5778 dst.term = new ArrayList<TermComponent>(); 5779 for (TermComponent i : term) 5780 dst.term.add(i.copy()); 5781 }; 5782 dst.binding = binding == null ? null : binding.copy(); 5783 if (friendly != null) { 5784 dst.friendly = new ArrayList<FriendlyLanguageComponent>(); 5785 for (FriendlyLanguageComponent i : friendly) 5786 dst.friendly.add(i.copy()); 5787 }; 5788 if (legal != null) { 5789 dst.legal = new ArrayList<LegalLanguageComponent>(); 5790 for (LegalLanguageComponent i : legal) 5791 dst.legal.add(i.copy()); 5792 }; 5793 if (rule != null) { 5794 dst.rule = new ArrayList<ComputableLanguageComponent>(); 5795 for (ComputableLanguageComponent i : rule) 5796 dst.rule.add(i.copy()); 5797 }; 5798 return dst; 5799 } 5800 5801 protected Contract typedCopy() { 5802 return copy(); 5803 } 5804 5805 @Override 5806 public boolean equalsDeep(Base other_) { 5807 if (!super.equalsDeep(other_)) 5808 return false; 5809 if (!(other_ instanceof Contract)) 5810 return false; 5811 Contract o = (Contract) other_; 5812 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(issued, o.issued, true) 5813 && compareDeep(applies, o.applies, true) && compareDeep(subject, o.subject, true) && compareDeep(topic, o.topic, true) 5814 && compareDeep(authority, o.authority, true) && compareDeep(domain, o.domain, true) && compareDeep(type, o.type, true) 5815 && compareDeep(subType, o.subType, true) && compareDeep(action, o.action, true) && compareDeep(actionReason, o.actionReason, true) 5816 && compareDeep(decisionType, o.decisionType, true) && compareDeep(contentDerivative, o.contentDerivative, true) 5817 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(agent, o.agent, true) && compareDeep(signer, o.signer, true) 5818 && compareDeep(valuedItem, o.valuedItem, true) && compareDeep(term, o.term, true) && compareDeep(binding, o.binding, true) 5819 && compareDeep(friendly, o.friendly, true) && compareDeep(legal, o.legal, true) && compareDeep(rule, o.rule, true) 5820 ; 5821 } 5822 5823 @Override 5824 public boolean equalsShallow(Base other_) { 5825 if (!super.equalsShallow(other_)) 5826 return false; 5827 if (!(other_ instanceof Contract)) 5828 return false; 5829 Contract o = (Contract) other_; 5830 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true); 5831 } 5832 5833 public boolean isEmpty() { 5834 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, issued 5835 , applies, subject, topic, authority, domain, type, subType, action, actionReason 5836 , decisionType, contentDerivative, securityLabel, agent, signer, valuedItem, term 5837 , binding, friendly, legal, rule); 5838 } 5839 5840 @Override 5841 public ResourceType getResourceType() { 5842 return ResourceType.Contract; 5843 } 5844 5845 /** 5846 * Search parameter: <b>identifier</b> 5847 * <p> 5848 * Description: <b>The identity of the contract</b><br> 5849 * Type: <b>token</b><br> 5850 * Path: <b>Contract.identifier</b><br> 5851 * </p> 5852 */ 5853 @SearchParamDefinition(name="identifier", path="Contract.identifier", description="The identity of the contract", type="token" ) 5854 public static final String SP_IDENTIFIER = "identifier"; 5855 /** 5856 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5857 * <p> 5858 * Description: <b>The identity of the contract</b><br> 5859 * Type: <b>token</b><br> 5860 * Path: <b>Contract.identifier</b><br> 5861 * </p> 5862 */ 5863 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5864 5865 /** 5866 * Search parameter: <b>agent</b> 5867 * <p> 5868 * Description: <b>Agent to the Contact</b><br> 5869 * Type: <b>reference</b><br> 5870 * Path: <b>Contract.agent.actor</b><br> 5871 * </p> 5872 */ 5873 @SearchParamDefinition(name="agent", path="Contract.agent.actor", description="Agent to the Contact", type="reference", target={Contract.class, Device.class, Group.class, Location.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, Substance.class } ) 5874 public static final String SP_AGENT = "agent"; 5875 /** 5876 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 5877 * <p> 5878 * Description: <b>Agent to the Contact</b><br> 5879 * Type: <b>reference</b><br> 5880 * Path: <b>Contract.agent.actor</b><br> 5881 * </p> 5882 */ 5883 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AGENT); 5884 5885/** 5886 * Constant for fluent queries to be used to add include statements. Specifies 5887 * the path value of "<b>Contract:agent</b>". 5888 */ 5889 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include("Contract:agent").toLocked(); 5890 5891 /** 5892 * Search parameter: <b>patient</b> 5893 * <p> 5894 * Description: <b>The identity of the subject of the contract (if a patient)</b><br> 5895 * Type: <b>reference</b><br> 5896 * Path: <b>Contract.subject</b><br> 5897 * </p> 5898 */ 5899 @SearchParamDefinition(name="patient", path="Contract.subject", description="The identity of the subject of the contract (if a patient)", type="reference", target={Patient.class } ) 5900 public static final String SP_PATIENT = "patient"; 5901 /** 5902 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5903 * <p> 5904 * Description: <b>The identity of the subject of the contract (if a patient)</b><br> 5905 * Type: <b>reference</b><br> 5906 * Path: <b>Contract.subject</b><br> 5907 * </p> 5908 */ 5909 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 5910 5911/** 5912 * Constant for fluent queries to be used to add include statements. Specifies 5913 * the path value of "<b>Contract:patient</b>". 5914 */ 5915 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Contract:patient").toLocked(); 5916 5917 /** 5918 * Search parameter: <b>subject</b> 5919 * <p> 5920 * Description: <b>The identity of the subject of the contract</b><br> 5921 * Type: <b>reference</b><br> 5922 * Path: <b>Contract.subject</b><br> 5923 * </p> 5924 */ 5925 @SearchParamDefinition(name="subject", path="Contract.subject", description="The identity of the subject of the contract", type="reference" ) 5926 public static final String SP_SUBJECT = "subject"; 5927 /** 5928 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5929 * <p> 5930 * Description: <b>The identity of the subject of the contract</b><br> 5931 * Type: <b>reference</b><br> 5932 * Path: <b>Contract.subject</b><br> 5933 * </p> 5934 */ 5935 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 5936 5937/** 5938 * Constant for fluent queries to be used to add include statements. Specifies 5939 * the path value of "<b>Contract:subject</b>". 5940 */ 5941 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Contract:subject").toLocked(); 5942 5943 /** 5944 * Search parameter: <b>authority</b> 5945 * <p> 5946 * Description: <b>The authority of the contract</b><br> 5947 * Type: <b>reference</b><br> 5948 * Path: <b>Contract.authority</b><br> 5949 * </p> 5950 */ 5951 @SearchParamDefinition(name="authority", path="Contract.authority", description="The authority of the contract", type="reference", target={Organization.class } ) 5952 public static final String SP_AUTHORITY = "authority"; 5953 /** 5954 * <b>Fluent Client</b> search parameter constant for <b>authority</b> 5955 * <p> 5956 * Description: <b>The authority of the contract</b><br> 5957 * Type: <b>reference</b><br> 5958 * Path: <b>Contract.authority</b><br> 5959 * </p> 5960 */ 5961 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHORITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHORITY); 5962 5963/** 5964 * Constant for fluent queries to be used to add include statements. Specifies 5965 * the path value of "<b>Contract:authority</b>". 5966 */ 5967 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHORITY = new ca.uhn.fhir.model.api.Include("Contract:authority").toLocked(); 5968 5969 /** 5970 * Search parameter: <b>domain</b> 5971 * <p> 5972 * Description: <b>The domain of the contract</b><br> 5973 * Type: <b>reference</b><br> 5974 * Path: <b>Contract.domain</b><br> 5975 * </p> 5976 */ 5977 @SearchParamDefinition(name="domain", path="Contract.domain", description="The domain of the contract", type="reference", target={Location.class } ) 5978 public static final String SP_DOMAIN = "domain"; 5979 /** 5980 * <b>Fluent Client</b> search parameter constant for <b>domain</b> 5981 * <p> 5982 * Description: <b>The domain of the contract</b><br> 5983 * Type: <b>reference</b><br> 5984 * Path: <b>Contract.domain</b><br> 5985 * </p> 5986 */ 5987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DOMAIN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DOMAIN); 5988 5989/** 5990 * Constant for fluent queries to be used to add include statements. Specifies 5991 * the path value of "<b>Contract:domain</b>". 5992 */ 5993 public static final ca.uhn.fhir.model.api.Include INCLUDE_DOMAIN = new ca.uhn.fhir.model.api.Include("Contract:domain").toLocked(); 5994 5995 /** 5996 * Search parameter: <b>term-topic</b> 5997 * <p> 5998 * Description: <b>The identity of the topic of the contract terms</b><br> 5999 * Type: <b>reference</b><br> 6000 * Path: <b>Contract.term.topic</b><br> 6001 * </p> 6002 */ 6003 @SearchParamDefinition(name="term-topic", path="Contract.term.topic", description="The identity of the topic of the contract terms", type="reference" ) 6004 public static final String SP_TERM_TOPIC = "term-topic"; 6005 /** 6006 * <b>Fluent Client</b> search parameter constant for <b>term-topic</b> 6007 * <p> 6008 * Description: <b>The identity of the topic of the contract terms</b><br> 6009 * Type: <b>reference</b><br> 6010 * Path: <b>Contract.term.topic</b><br> 6011 * </p> 6012 */ 6013 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TERM_TOPIC = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TERM_TOPIC); 6014 6015/** 6016 * Constant for fluent queries to be used to add include statements. Specifies 6017 * the path value of "<b>Contract:term-topic</b>". 6018 */ 6019 public static final ca.uhn.fhir.model.api.Include INCLUDE_TERM_TOPIC = new ca.uhn.fhir.model.api.Include("Contract:term-topic").toLocked(); 6020 6021 /** 6022 * Search parameter: <b>issued</b> 6023 * <p> 6024 * Description: <b>The date/time the contract was issued</b><br> 6025 * Type: <b>date</b><br> 6026 * Path: <b>Contract.issued</b><br> 6027 * </p> 6028 */ 6029 @SearchParamDefinition(name="issued", path="Contract.issued", description="The date/time the contract was issued", type="date" ) 6030 public static final String SP_ISSUED = "issued"; 6031 /** 6032 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 6033 * <p> 6034 * Description: <b>The date/time the contract was issued</b><br> 6035 * Type: <b>date</b><br> 6036 * Path: <b>Contract.issued</b><br> 6037 * </p> 6038 */ 6039 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 6040 6041 /** 6042 * Search parameter: <b>signer</b> 6043 * <p> 6044 * Description: <b>Contract Signatory Party</b><br> 6045 * Type: <b>reference</b><br> 6046 * Path: <b>Contract.signer.party</b><br> 6047 * </p> 6048 */ 6049 @SearchParamDefinition(name="signer", path="Contract.signer.party", description="Contract Signatory Party", type="reference", target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 6050 public static final String SP_SIGNER = "signer"; 6051 /** 6052 * <b>Fluent Client</b> search parameter constant for <b>signer</b> 6053 * <p> 6054 * Description: <b>Contract Signatory Party</b><br> 6055 * Type: <b>reference</b><br> 6056 * Path: <b>Contract.signer.party</b><br> 6057 * </p> 6058 */ 6059 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SIGNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SIGNER); 6060 6061/** 6062 * Constant for fluent queries to be used to add include statements. Specifies 6063 * the path value of "<b>Contract:signer</b>". 6064 */ 6065 public static final ca.uhn.fhir.model.api.Include INCLUDE_SIGNER = new ca.uhn.fhir.model.api.Include("Contract:signer").toLocked(); 6066 6067 6068}