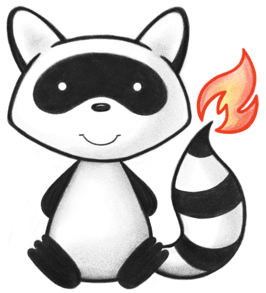
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 047 */ 048@DatatypeDef(name="Contributor") 049public class Contributor extends Type implements ICompositeType { 050 051 public enum ContributorType { 052 /** 053 * An author of the content of the module 054 */ 055 AUTHOR, 056 /** 057 * An editor of the content of the module 058 */ 059 EDITOR, 060 /** 061 * A reviewer of the content of the module 062 */ 063 REVIEWER, 064 /** 065 * An endorser of the content of the module 066 */ 067 ENDORSER, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static ContributorType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("author".equals(codeString)) 076 return AUTHOR; 077 if ("editor".equals(codeString)) 078 return EDITOR; 079 if ("reviewer".equals(codeString)) 080 return REVIEWER; 081 if ("endorser".equals(codeString)) 082 return ENDORSER; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown ContributorType code '"+codeString+"'"); 087 } 088 public String toCode() { 089 switch (this) { 090 case AUTHOR: return "author"; 091 case EDITOR: return "editor"; 092 case REVIEWER: return "reviewer"; 093 case ENDORSER: return "endorser"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case AUTHOR: return "http://hl7.org/fhir/contributor-type"; 101 case EDITOR: return "http://hl7.org/fhir/contributor-type"; 102 case REVIEWER: return "http://hl7.org/fhir/contributor-type"; 103 case ENDORSER: return "http://hl7.org/fhir/contributor-type"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getDefinition() { 109 switch (this) { 110 case AUTHOR: return "An author of the content of the module"; 111 case EDITOR: return "An editor of the content of the module"; 112 case REVIEWER: return "A reviewer of the content of the module"; 113 case ENDORSER: return "An endorser of the content of the module"; 114 case NULL: return null; 115 default: return "?"; 116 } 117 } 118 public String getDisplay() { 119 switch (this) { 120 case AUTHOR: return "Author"; 121 case EDITOR: return "Editor"; 122 case REVIEWER: return "Reviewer"; 123 case ENDORSER: return "Endorser"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 } 129 130 public static class ContributorTypeEnumFactory implements EnumFactory<ContributorType> { 131 public ContributorType fromCode(String codeString) throws IllegalArgumentException { 132 if (codeString == null || "".equals(codeString)) 133 if (codeString == null || "".equals(codeString)) 134 return null; 135 if ("author".equals(codeString)) 136 return ContributorType.AUTHOR; 137 if ("editor".equals(codeString)) 138 return ContributorType.EDITOR; 139 if ("reviewer".equals(codeString)) 140 return ContributorType.REVIEWER; 141 if ("endorser".equals(codeString)) 142 return ContributorType.ENDORSER; 143 throw new IllegalArgumentException("Unknown ContributorType code '"+codeString+"'"); 144 } 145 public Enumeration<ContributorType> fromType(PrimitiveType<?> code) throws FHIRException { 146 if (code == null) 147 return null; 148 if (code.isEmpty()) 149 return new Enumeration<ContributorType>(this); 150 String codeString = code.asStringValue(); 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("author".equals(codeString)) 154 return new Enumeration<ContributorType>(this, ContributorType.AUTHOR); 155 if ("editor".equals(codeString)) 156 return new Enumeration<ContributorType>(this, ContributorType.EDITOR); 157 if ("reviewer".equals(codeString)) 158 return new Enumeration<ContributorType>(this, ContributorType.REVIEWER); 159 if ("endorser".equals(codeString)) 160 return new Enumeration<ContributorType>(this, ContributorType.ENDORSER); 161 throw new FHIRException("Unknown ContributorType code '"+codeString+"'"); 162 } 163 public String toCode(ContributorType code) { 164 if (code == ContributorType.NULL) 165 return null; 166 if (code == ContributorType.AUTHOR) 167 return "author"; 168 if (code == ContributorType.EDITOR) 169 return "editor"; 170 if (code == ContributorType.REVIEWER) 171 return "reviewer"; 172 if (code == ContributorType.ENDORSER) 173 return "endorser"; 174 return "?"; 175 } 176 public String toSystem(ContributorType code) { 177 return code.getSystem(); 178 } 179 } 180 181 /** 182 * The type of contributor. 183 */ 184 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 185 @Description(shortDefinition="author | editor | reviewer | endorser", formalDefinition="The type of contributor." ) 186 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contributor-type") 187 protected Enumeration<ContributorType> type; 188 189 /** 190 * The name of the individual or organization responsible for the contribution. 191 */ 192 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 193 @Description(shortDefinition="Who contributed the content", formalDefinition="The name of the individual or organization responsible for the contribution." ) 194 protected StringType name; 195 196 /** 197 * Contact details to assist a user in finding and communicating with the contributor. 198 */ 199 @Child(name = "contact", type = {ContactDetail.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 200 @Description(shortDefinition="Contact details of the contributor", formalDefinition="Contact details to assist a user in finding and communicating with the contributor." ) 201 protected List<ContactDetail> contact; 202 203 private static final long serialVersionUID = -609887113L; 204 205 /** 206 * Constructor 207 */ 208 public Contributor() { 209 super(); 210 } 211 212 /** 213 * Constructor 214 */ 215 public Contributor(Enumeration<ContributorType> type, StringType name) { 216 super(); 217 this.type = type; 218 this.name = name; 219 } 220 221 /** 222 * @return {@link #type} (The type of contributor.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 223 */ 224 public Enumeration<ContributorType> getTypeElement() { 225 if (this.type == null) 226 if (Configuration.errorOnAutoCreate()) 227 throw new Error("Attempt to auto-create Contributor.type"); 228 else if (Configuration.doAutoCreate()) 229 this.type = new Enumeration<ContributorType>(new ContributorTypeEnumFactory()); // bb 230 return this.type; 231 } 232 233 public boolean hasTypeElement() { 234 return this.type != null && !this.type.isEmpty(); 235 } 236 237 public boolean hasType() { 238 return this.type != null && !this.type.isEmpty(); 239 } 240 241 /** 242 * @param value {@link #type} (The type of contributor.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 243 */ 244 public Contributor setTypeElement(Enumeration<ContributorType> value) { 245 this.type = value; 246 return this; 247 } 248 249 /** 250 * @return The type of contributor. 251 */ 252 public ContributorType getType() { 253 return this.type == null ? null : this.type.getValue(); 254 } 255 256 /** 257 * @param value The type of contributor. 258 */ 259 public Contributor setType(ContributorType value) { 260 if (this.type == null) 261 this.type = new Enumeration<ContributorType>(new ContributorTypeEnumFactory()); 262 this.type.setValue(value); 263 return this; 264 } 265 266 /** 267 * @return {@link #name} (The name of the individual or organization responsible for the contribution.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 268 */ 269 public StringType getNameElement() { 270 if (this.name == null) 271 if (Configuration.errorOnAutoCreate()) 272 throw new Error("Attempt to auto-create Contributor.name"); 273 else if (Configuration.doAutoCreate()) 274 this.name = new StringType(); // bb 275 return this.name; 276 } 277 278 public boolean hasNameElement() { 279 return this.name != null && !this.name.isEmpty(); 280 } 281 282 public boolean hasName() { 283 return this.name != null && !this.name.isEmpty(); 284 } 285 286 /** 287 * @param value {@link #name} (The name of the individual or organization responsible for the contribution.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 288 */ 289 public Contributor setNameElement(StringType value) { 290 this.name = value; 291 return this; 292 } 293 294 /** 295 * @return The name of the individual or organization responsible for the contribution. 296 */ 297 public String getName() { 298 return this.name == null ? null : this.name.getValue(); 299 } 300 301 /** 302 * @param value The name of the individual or organization responsible for the contribution. 303 */ 304 public Contributor setName(String value) { 305 if (this.name == null) 306 this.name = new StringType(); 307 this.name.setValue(value); 308 return this; 309 } 310 311 /** 312 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the contributor.) 313 */ 314 public List<ContactDetail> getContact() { 315 if (this.contact == null) 316 this.contact = new ArrayList<ContactDetail>(); 317 return this.contact; 318 } 319 320 /** 321 * @return Returns a reference to <code>this</code> for easy method chaining 322 */ 323 public Contributor setContact(List<ContactDetail> theContact) { 324 this.contact = theContact; 325 return this; 326 } 327 328 public boolean hasContact() { 329 if (this.contact == null) 330 return false; 331 for (ContactDetail item : this.contact) 332 if (!item.isEmpty()) 333 return true; 334 return false; 335 } 336 337 public ContactDetail addContact() { //3 338 ContactDetail t = new ContactDetail(); 339 if (this.contact == null) 340 this.contact = new ArrayList<ContactDetail>(); 341 this.contact.add(t); 342 return t; 343 } 344 345 public Contributor addContact(ContactDetail t) { //3 346 if (t == null) 347 return this; 348 if (this.contact == null) 349 this.contact = new ArrayList<ContactDetail>(); 350 this.contact.add(t); 351 return this; 352 } 353 354 /** 355 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 356 */ 357 public ContactDetail getContactFirstRep() { 358 if (getContact().isEmpty()) { 359 addContact(); 360 } 361 return getContact().get(0); 362 } 363 364 protected void listChildren(List<Property> children) { 365 super.listChildren(children); 366 children.add(new Property("type", "code", "The type of contributor.", 0, 1, type)); 367 children.add(new Property("name", "string", "The name of the individual or organization responsible for the contribution.", 0, 1, name)); 368 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the contributor.", 0, java.lang.Integer.MAX_VALUE, contact)); 369 } 370 371 @Override 372 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 373 switch (_hash) { 374 case 3575610: /*type*/ return new Property("type", "code", "The type of contributor.", 0, 1, type); 375 case 3373707: /*name*/ return new Property("name", "string", "The name of the individual or organization responsible for the contribution.", 0, 1, name); 376 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the contributor.", 0, java.lang.Integer.MAX_VALUE, contact); 377 default: return super.getNamedProperty(_hash, _name, _checkValid); 378 } 379 380 } 381 382 @Override 383 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 384 switch (hash) { 385 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ContributorType> 386 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 387 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 388 default: return super.getProperty(hash, name, checkValid); 389 } 390 391 } 392 393 @Override 394 public Base setProperty(int hash, String name, Base value) throws FHIRException { 395 switch (hash) { 396 case 3575610: // type 397 value = new ContributorTypeEnumFactory().fromType(castToCode(value)); 398 this.type = (Enumeration) value; // Enumeration<ContributorType> 399 return value; 400 case 3373707: // name 401 this.name = castToString(value); // StringType 402 return value; 403 case 951526432: // contact 404 this.getContact().add(castToContactDetail(value)); // ContactDetail 405 return value; 406 default: return super.setProperty(hash, name, value); 407 } 408 409 } 410 411 @Override 412 public Base setProperty(String name, Base value) throws FHIRException { 413 if (name.equals("type")) { 414 value = new ContributorTypeEnumFactory().fromType(castToCode(value)); 415 this.type = (Enumeration) value; // Enumeration<ContributorType> 416 } else if (name.equals("name")) { 417 this.name = castToString(value); // StringType 418 } else if (name.equals("contact")) { 419 this.getContact().add(castToContactDetail(value)); 420 } else 421 return super.setProperty(name, value); 422 return value; 423 } 424 425 @Override 426 public Base makeProperty(int hash, String name) throws FHIRException { 427 switch (hash) { 428 case 3575610: return getTypeElement(); 429 case 3373707: return getNameElement(); 430 case 951526432: return addContact(); 431 default: return super.makeProperty(hash, name); 432 } 433 434 } 435 436 @Override 437 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 438 switch (hash) { 439 case 3575610: /*type*/ return new String[] {"code"}; 440 case 3373707: /*name*/ return new String[] {"string"}; 441 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 442 default: return super.getTypesForProperty(hash, name); 443 } 444 445 } 446 447 @Override 448 public Base addChild(String name) throws FHIRException { 449 if (name.equals("type")) { 450 throw new FHIRException("Cannot call addChild on a singleton property Contributor.type"); 451 } 452 else if (name.equals("name")) { 453 throw new FHIRException("Cannot call addChild on a singleton property Contributor.name"); 454 } 455 else if (name.equals("contact")) { 456 return addContact(); 457 } 458 else 459 return super.addChild(name); 460 } 461 462 public String fhirType() { 463 return "Contributor"; 464 465 } 466 467 public Contributor copy() { 468 Contributor dst = new Contributor(); 469 copyValues(dst); 470 dst.type = type == null ? null : type.copy(); 471 dst.name = name == null ? null : name.copy(); 472 if (contact != null) { 473 dst.contact = new ArrayList<ContactDetail>(); 474 for (ContactDetail i : contact) 475 dst.contact.add(i.copy()); 476 }; 477 return dst; 478 } 479 480 protected Contributor typedCopy() { 481 return copy(); 482 } 483 484 @Override 485 public boolean equalsDeep(Base other_) { 486 if (!super.equalsDeep(other_)) 487 return false; 488 if (!(other_ instanceof Contributor)) 489 return false; 490 Contributor o = (Contributor) other_; 491 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(contact, o.contact, true) 492 ; 493 } 494 495 @Override 496 public boolean equalsShallow(Base other_) { 497 if (!super.equalsShallow(other_)) 498 return false; 499 if (!(other_ instanceof Contributor)) 500 return false; 501 Contributor o = (Contributor) other_; 502 return compareValues(type, o.type, true) && compareValues(name, o.name, true); 503 } 504 505 public boolean isEmpty() { 506 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name, contact); 507 } 508 509 510}