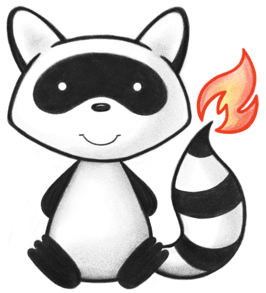
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Financial instrument which may be used to reimburse or pay for health care products and services. 049 */ 050@ResourceDef(name="Coverage", profile="http://hl7.org/fhir/Profile/Coverage") 051public class Coverage extends DomainResource { 052 053 public enum CoverageStatus { 054 /** 055 * The instance is currently in-force. 056 */ 057 ACTIVE, 058 /** 059 * The instance is withdrawn, rescinded or reversed. 060 */ 061 CANCELLED, 062 /** 063 * A new instance the contents of which is not complete. 064 */ 065 DRAFT, 066 /** 067 * The instance was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 public static CoverageStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("active".equals(codeString)) 078 return ACTIVE; 079 if ("cancelled".equals(codeString)) 080 return CANCELLED; 081 if ("draft".equals(codeString)) 082 return DRAFT; 083 if ("entered-in-error".equals(codeString)) 084 return ENTEREDINERROR; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown CoverageStatus code '"+codeString+"'"); 089 } 090 public String toCode() { 091 switch (this) { 092 case ACTIVE: return "active"; 093 case CANCELLED: return "cancelled"; 094 case DRAFT: return "draft"; 095 case ENTEREDINERROR: return "entered-in-error"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getSystem() { 101 switch (this) { 102 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 103 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 104 case DRAFT: return "http://hl7.org/fhir/fm-status"; 105 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getDefinition() { 111 switch (this) { 112 case ACTIVE: return "The instance is currently in-force."; 113 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 114 case DRAFT: return "A new instance the contents of which is not complete."; 115 case ENTEREDINERROR: return "The instance was entered in error."; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDisplay() { 121 switch (this) { 122 case ACTIVE: return "Active"; 123 case CANCELLED: return "Cancelled"; 124 case DRAFT: return "Draft"; 125 case ENTEREDINERROR: return "Entered in Error"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 } 131 132 public static class CoverageStatusEnumFactory implements EnumFactory<CoverageStatus> { 133 public CoverageStatus fromCode(String codeString) throws IllegalArgumentException { 134 if (codeString == null || "".equals(codeString)) 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("active".equals(codeString)) 138 return CoverageStatus.ACTIVE; 139 if ("cancelled".equals(codeString)) 140 return CoverageStatus.CANCELLED; 141 if ("draft".equals(codeString)) 142 return CoverageStatus.DRAFT; 143 if ("entered-in-error".equals(codeString)) 144 return CoverageStatus.ENTEREDINERROR; 145 throw new IllegalArgumentException("Unknown CoverageStatus code '"+codeString+"'"); 146 } 147 public Enumeration<CoverageStatus> fromType(PrimitiveType<?> code) throws FHIRException { 148 if (code == null) 149 return null; 150 if (code.isEmpty()) 151 return new Enumeration<CoverageStatus>(this); 152 String codeString = code.asStringValue(); 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("active".equals(codeString)) 156 return new Enumeration<CoverageStatus>(this, CoverageStatus.ACTIVE); 157 if ("cancelled".equals(codeString)) 158 return new Enumeration<CoverageStatus>(this, CoverageStatus.CANCELLED); 159 if ("draft".equals(codeString)) 160 return new Enumeration<CoverageStatus>(this, CoverageStatus.DRAFT); 161 if ("entered-in-error".equals(codeString)) 162 return new Enumeration<CoverageStatus>(this, CoverageStatus.ENTEREDINERROR); 163 throw new FHIRException("Unknown CoverageStatus code '"+codeString+"'"); 164 } 165 public String toCode(CoverageStatus code) { 166 if (code == CoverageStatus.ACTIVE) 167 return "active"; 168 if (code == CoverageStatus.CANCELLED) 169 return "cancelled"; 170 if (code == CoverageStatus.DRAFT) 171 return "draft"; 172 if (code == CoverageStatus.ENTEREDINERROR) 173 return "entered-in-error"; 174 return "?"; 175 } 176 public String toSystem(CoverageStatus code) { 177 return code.getSystem(); 178 } 179 } 180 181 @Block() 182 public static class GroupComponent extends BackboneElement implements IBaseBackboneElement { 183 /** 184 * Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID. 185 */ 186 @Child(name = "group", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 187 @Description(shortDefinition="An identifier for the group", formalDefinition="Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID." ) 188 protected StringType group; 189 190 /** 191 * A short description for the group. 192 */ 193 @Child(name = "groupDisplay", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 194 @Description(shortDefinition="Display text for an identifier for the group", formalDefinition="A short description for the group." ) 195 protected StringType groupDisplay; 196 197 /** 198 * Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group. 199 */ 200 @Child(name = "subGroup", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 201 @Description(shortDefinition="An identifier for the subsection of the group", formalDefinition="Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group." ) 202 protected StringType subGroup; 203 204 /** 205 * A short description for the subgroup. 206 */ 207 @Child(name = "subGroupDisplay", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 208 @Description(shortDefinition="Display text for the subsection of the group", formalDefinition="A short description for the subgroup." ) 209 protected StringType subGroupDisplay; 210 211 /** 212 * Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID. 213 */ 214 @Child(name = "plan", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 215 @Description(shortDefinition="An identifier for the plan", formalDefinition="Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID." ) 216 protected StringType plan; 217 218 /** 219 * A short description for the plan. 220 */ 221 @Child(name = "planDisplay", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 222 @Description(shortDefinition="Display text for the plan", formalDefinition="A short description for the plan." ) 223 protected StringType planDisplay; 224 225 /** 226 * Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees. 227 */ 228 @Child(name = "subPlan", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 229 @Description(shortDefinition="An identifier for the subsection of the plan", formalDefinition="Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees." ) 230 protected StringType subPlan; 231 232 /** 233 * A short description for the subplan. 234 */ 235 @Child(name = "subPlanDisplay", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 236 @Description(shortDefinition="Display text for the subsection of the plan", formalDefinition="A short description for the subplan." ) 237 protected StringType subPlanDisplay; 238 239 /** 240 * Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment. 241 */ 242 @Child(name = "class", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 243 @Description(shortDefinition="An identifier for the class", formalDefinition="Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment." ) 244 protected StringType class_; 245 246 /** 247 * A short description for the class. 248 */ 249 @Child(name = "classDisplay", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 250 @Description(shortDefinition="Display text for the class", formalDefinition="A short description for the class." ) 251 protected StringType classDisplay; 252 253 /** 254 * Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment. 255 */ 256 @Child(name = "subClass", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 257 @Description(shortDefinition="An identifier for the subsection of the class", formalDefinition="Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment." ) 258 protected StringType subClass; 259 260 /** 261 * A short description for the subclass. 262 */ 263 @Child(name = "subClassDisplay", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 264 @Description(shortDefinition="Display text for the subsection of the subclass", formalDefinition="A short description for the subclass." ) 265 protected StringType subClassDisplay; 266 267 private static final long serialVersionUID = -13147121L; 268 269 /** 270 * Constructor 271 */ 272 public GroupComponent() { 273 super(); 274 } 275 276 /** 277 * @return {@link #group} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.). This is the underlying object with id, value and extensions. The accessor "getGroup" gives direct access to the value 278 */ 279 public StringType getGroupElement() { 280 if (this.group == null) 281 if (Configuration.errorOnAutoCreate()) 282 throw new Error("Attempt to auto-create GroupComponent.group"); 283 else if (Configuration.doAutoCreate()) 284 this.group = new StringType(); // bb 285 return this.group; 286 } 287 288 public boolean hasGroupElement() { 289 return this.group != null && !this.group.isEmpty(); 290 } 291 292 public boolean hasGroup() { 293 return this.group != null && !this.group.isEmpty(); 294 } 295 296 /** 297 * @param value {@link #group} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.). This is the underlying object with id, value and extensions. The accessor "getGroup" gives direct access to the value 298 */ 299 public GroupComponent setGroupElement(StringType value) { 300 this.group = value; 301 return this; 302 } 303 304 /** 305 * @return Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID. 306 */ 307 public String getGroup() { 308 return this.group == null ? null : this.group.getValue(); 309 } 310 311 /** 312 * @param value Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID. 313 */ 314 public GroupComponent setGroup(String value) { 315 if (Utilities.noString(value)) 316 this.group = null; 317 else { 318 if (this.group == null) 319 this.group = new StringType(); 320 this.group.setValue(value); 321 } 322 return this; 323 } 324 325 /** 326 * @return {@link #groupDisplay} (A short description for the group.). This is the underlying object with id, value and extensions. The accessor "getGroupDisplay" gives direct access to the value 327 */ 328 public StringType getGroupDisplayElement() { 329 if (this.groupDisplay == null) 330 if (Configuration.errorOnAutoCreate()) 331 throw new Error("Attempt to auto-create GroupComponent.groupDisplay"); 332 else if (Configuration.doAutoCreate()) 333 this.groupDisplay = new StringType(); // bb 334 return this.groupDisplay; 335 } 336 337 public boolean hasGroupDisplayElement() { 338 return this.groupDisplay != null && !this.groupDisplay.isEmpty(); 339 } 340 341 public boolean hasGroupDisplay() { 342 return this.groupDisplay != null && !this.groupDisplay.isEmpty(); 343 } 344 345 /** 346 * @param value {@link #groupDisplay} (A short description for the group.). This is the underlying object with id, value and extensions. The accessor "getGroupDisplay" gives direct access to the value 347 */ 348 public GroupComponent setGroupDisplayElement(StringType value) { 349 this.groupDisplay = value; 350 return this; 351 } 352 353 /** 354 * @return A short description for the group. 355 */ 356 public String getGroupDisplay() { 357 return this.groupDisplay == null ? null : this.groupDisplay.getValue(); 358 } 359 360 /** 361 * @param value A short description for the group. 362 */ 363 public GroupComponent setGroupDisplay(String value) { 364 if (Utilities.noString(value)) 365 this.groupDisplay = null; 366 else { 367 if (this.groupDisplay == null) 368 this.groupDisplay = new StringType(); 369 this.groupDisplay.setValue(value); 370 } 371 return this; 372 } 373 374 /** 375 * @return {@link #subGroup} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.). This is the underlying object with id, value and extensions. The accessor "getSubGroup" gives direct access to the value 376 */ 377 public StringType getSubGroupElement() { 378 if (this.subGroup == null) 379 if (Configuration.errorOnAutoCreate()) 380 throw new Error("Attempt to auto-create GroupComponent.subGroup"); 381 else if (Configuration.doAutoCreate()) 382 this.subGroup = new StringType(); // bb 383 return this.subGroup; 384 } 385 386 public boolean hasSubGroupElement() { 387 return this.subGroup != null && !this.subGroup.isEmpty(); 388 } 389 390 public boolean hasSubGroup() { 391 return this.subGroup != null && !this.subGroup.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #subGroup} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.). This is the underlying object with id, value and extensions. The accessor "getSubGroup" gives direct access to the value 396 */ 397 public GroupComponent setSubGroupElement(StringType value) { 398 this.subGroup = value; 399 return this; 400 } 401 402 /** 403 * @return Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group. 404 */ 405 public String getSubGroup() { 406 return this.subGroup == null ? null : this.subGroup.getValue(); 407 } 408 409 /** 410 * @param value Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group. 411 */ 412 public GroupComponent setSubGroup(String value) { 413 if (Utilities.noString(value)) 414 this.subGroup = null; 415 else { 416 if (this.subGroup == null) 417 this.subGroup = new StringType(); 418 this.subGroup.setValue(value); 419 } 420 return this; 421 } 422 423 /** 424 * @return {@link #subGroupDisplay} (A short description for the subgroup.). This is the underlying object with id, value and extensions. The accessor "getSubGroupDisplay" gives direct access to the value 425 */ 426 public StringType getSubGroupDisplayElement() { 427 if (this.subGroupDisplay == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create GroupComponent.subGroupDisplay"); 430 else if (Configuration.doAutoCreate()) 431 this.subGroupDisplay = new StringType(); // bb 432 return this.subGroupDisplay; 433 } 434 435 public boolean hasSubGroupDisplayElement() { 436 return this.subGroupDisplay != null && !this.subGroupDisplay.isEmpty(); 437 } 438 439 public boolean hasSubGroupDisplay() { 440 return this.subGroupDisplay != null && !this.subGroupDisplay.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #subGroupDisplay} (A short description for the subgroup.). This is the underlying object with id, value and extensions. The accessor "getSubGroupDisplay" gives direct access to the value 445 */ 446 public GroupComponent setSubGroupDisplayElement(StringType value) { 447 this.subGroupDisplay = value; 448 return this; 449 } 450 451 /** 452 * @return A short description for the subgroup. 453 */ 454 public String getSubGroupDisplay() { 455 return this.subGroupDisplay == null ? null : this.subGroupDisplay.getValue(); 456 } 457 458 /** 459 * @param value A short description for the subgroup. 460 */ 461 public GroupComponent setSubGroupDisplay(String value) { 462 if (Utilities.noString(value)) 463 this.subGroupDisplay = null; 464 else { 465 if (this.subGroupDisplay == null) 466 this.subGroupDisplay = new StringType(); 467 this.subGroupDisplay.setValue(value); 468 } 469 return this; 470 } 471 472 /** 473 * @return {@link #plan} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.). This is the underlying object with id, value and extensions. The accessor "getPlan" gives direct access to the value 474 */ 475 public StringType getPlanElement() { 476 if (this.plan == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create GroupComponent.plan"); 479 else if (Configuration.doAutoCreate()) 480 this.plan = new StringType(); // bb 481 return this.plan; 482 } 483 484 public boolean hasPlanElement() { 485 return this.plan != null && !this.plan.isEmpty(); 486 } 487 488 public boolean hasPlan() { 489 return this.plan != null && !this.plan.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #plan} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.). This is the underlying object with id, value and extensions. The accessor "getPlan" gives direct access to the value 494 */ 495 public GroupComponent setPlanElement(StringType value) { 496 this.plan = value; 497 return this; 498 } 499 500 /** 501 * @return Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID. 502 */ 503 public String getPlan() { 504 return this.plan == null ? null : this.plan.getValue(); 505 } 506 507 /** 508 * @param value Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID. 509 */ 510 public GroupComponent setPlan(String value) { 511 if (Utilities.noString(value)) 512 this.plan = null; 513 else { 514 if (this.plan == null) 515 this.plan = new StringType(); 516 this.plan.setValue(value); 517 } 518 return this; 519 } 520 521 /** 522 * @return {@link #planDisplay} (A short description for the plan.). This is the underlying object with id, value and extensions. The accessor "getPlanDisplay" gives direct access to the value 523 */ 524 public StringType getPlanDisplayElement() { 525 if (this.planDisplay == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create GroupComponent.planDisplay"); 528 else if (Configuration.doAutoCreate()) 529 this.planDisplay = new StringType(); // bb 530 return this.planDisplay; 531 } 532 533 public boolean hasPlanDisplayElement() { 534 return this.planDisplay != null && !this.planDisplay.isEmpty(); 535 } 536 537 public boolean hasPlanDisplay() { 538 return this.planDisplay != null && !this.planDisplay.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #planDisplay} (A short description for the plan.). This is the underlying object with id, value and extensions. The accessor "getPlanDisplay" gives direct access to the value 543 */ 544 public GroupComponent setPlanDisplayElement(StringType value) { 545 this.planDisplay = value; 546 return this; 547 } 548 549 /** 550 * @return A short description for the plan. 551 */ 552 public String getPlanDisplay() { 553 return this.planDisplay == null ? null : this.planDisplay.getValue(); 554 } 555 556 /** 557 * @param value A short description for the plan. 558 */ 559 public GroupComponent setPlanDisplay(String value) { 560 if (Utilities.noString(value)) 561 this.planDisplay = null; 562 else { 563 if (this.planDisplay == null) 564 this.planDisplay = new StringType(); 565 this.planDisplay.setValue(value); 566 } 567 return this; 568 } 569 570 /** 571 * @return {@link #subPlan} (Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.). This is the underlying object with id, value and extensions. The accessor "getSubPlan" gives direct access to the value 572 */ 573 public StringType getSubPlanElement() { 574 if (this.subPlan == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create GroupComponent.subPlan"); 577 else if (Configuration.doAutoCreate()) 578 this.subPlan = new StringType(); // bb 579 return this.subPlan; 580 } 581 582 public boolean hasSubPlanElement() { 583 return this.subPlan != null && !this.subPlan.isEmpty(); 584 } 585 586 public boolean hasSubPlan() { 587 return this.subPlan != null && !this.subPlan.isEmpty(); 588 } 589 590 /** 591 * @param value {@link #subPlan} (Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.). This is the underlying object with id, value and extensions. The accessor "getSubPlan" gives direct access to the value 592 */ 593 public GroupComponent setSubPlanElement(StringType value) { 594 this.subPlan = value; 595 return this; 596 } 597 598 /** 599 * @return Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees. 600 */ 601 public String getSubPlan() { 602 return this.subPlan == null ? null : this.subPlan.getValue(); 603 } 604 605 /** 606 * @param value Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees. 607 */ 608 public GroupComponent setSubPlan(String value) { 609 if (Utilities.noString(value)) 610 this.subPlan = null; 611 else { 612 if (this.subPlan == null) 613 this.subPlan = new StringType(); 614 this.subPlan.setValue(value); 615 } 616 return this; 617 } 618 619 /** 620 * @return {@link #subPlanDisplay} (A short description for the subplan.). This is the underlying object with id, value and extensions. The accessor "getSubPlanDisplay" gives direct access to the value 621 */ 622 public StringType getSubPlanDisplayElement() { 623 if (this.subPlanDisplay == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create GroupComponent.subPlanDisplay"); 626 else if (Configuration.doAutoCreate()) 627 this.subPlanDisplay = new StringType(); // bb 628 return this.subPlanDisplay; 629 } 630 631 public boolean hasSubPlanDisplayElement() { 632 return this.subPlanDisplay != null && !this.subPlanDisplay.isEmpty(); 633 } 634 635 public boolean hasSubPlanDisplay() { 636 return this.subPlanDisplay != null && !this.subPlanDisplay.isEmpty(); 637 } 638 639 /** 640 * @param value {@link #subPlanDisplay} (A short description for the subplan.). This is the underlying object with id, value and extensions. The accessor "getSubPlanDisplay" gives direct access to the value 641 */ 642 public GroupComponent setSubPlanDisplayElement(StringType value) { 643 this.subPlanDisplay = value; 644 return this; 645 } 646 647 /** 648 * @return A short description for the subplan. 649 */ 650 public String getSubPlanDisplay() { 651 return this.subPlanDisplay == null ? null : this.subPlanDisplay.getValue(); 652 } 653 654 /** 655 * @param value A short description for the subplan. 656 */ 657 public GroupComponent setSubPlanDisplay(String value) { 658 if (Utilities.noString(value)) 659 this.subPlanDisplay = null; 660 else { 661 if (this.subPlanDisplay == null) 662 this.subPlanDisplay = new StringType(); 663 this.subPlanDisplay.setValue(value); 664 } 665 return this; 666 } 667 668 /** 669 * @return {@link #class_} (Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getClass_" gives direct access to the value 670 */ 671 public StringType getClass_Element() { 672 if (this.class_ == null) 673 if (Configuration.errorOnAutoCreate()) 674 throw new Error("Attempt to auto-create GroupComponent.class_"); 675 else if (Configuration.doAutoCreate()) 676 this.class_ = new StringType(); // bb 677 return this.class_; 678 } 679 680 public boolean hasClass_Element() { 681 return this.class_ != null && !this.class_.isEmpty(); 682 } 683 684 public boolean hasClass_() { 685 return this.class_ != null && !this.class_.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #class_} (Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getClass_" gives direct access to the value 690 */ 691 public GroupComponent setClass_Element(StringType value) { 692 this.class_ = value; 693 return this; 694 } 695 696 /** 697 * @return Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment. 698 */ 699 public String getClass_() { 700 return this.class_ == null ? null : this.class_.getValue(); 701 } 702 703 /** 704 * @param value Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment. 705 */ 706 public GroupComponent setClass_(String value) { 707 if (Utilities.noString(value)) 708 this.class_ = null; 709 else { 710 if (this.class_ == null) 711 this.class_ = new StringType(); 712 this.class_.setValue(value); 713 } 714 return this; 715 } 716 717 /** 718 * @return {@link #classDisplay} (A short description for the class.). This is the underlying object with id, value and extensions. The accessor "getClassDisplay" gives direct access to the value 719 */ 720 public StringType getClassDisplayElement() { 721 if (this.classDisplay == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create GroupComponent.classDisplay"); 724 else if (Configuration.doAutoCreate()) 725 this.classDisplay = new StringType(); // bb 726 return this.classDisplay; 727 } 728 729 public boolean hasClassDisplayElement() { 730 return this.classDisplay != null && !this.classDisplay.isEmpty(); 731 } 732 733 public boolean hasClassDisplay() { 734 return this.classDisplay != null && !this.classDisplay.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #classDisplay} (A short description for the class.). This is the underlying object with id, value and extensions. The accessor "getClassDisplay" gives direct access to the value 739 */ 740 public GroupComponent setClassDisplayElement(StringType value) { 741 this.classDisplay = value; 742 return this; 743 } 744 745 /** 746 * @return A short description for the class. 747 */ 748 public String getClassDisplay() { 749 return this.classDisplay == null ? null : this.classDisplay.getValue(); 750 } 751 752 /** 753 * @param value A short description for the class. 754 */ 755 public GroupComponent setClassDisplay(String value) { 756 if (Utilities.noString(value)) 757 this.classDisplay = null; 758 else { 759 if (this.classDisplay == null) 760 this.classDisplay = new StringType(); 761 this.classDisplay.setValue(value); 762 } 763 return this; 764 } 765 766 /** 767 * @return {@link #subClass} (Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getSubClass" gives direct access to the value 768 */ 769 public StringType getSubClassElement() { 770 if (this.subClass == null) 771 if (Configuration.errorOnAutoCreate()) 772 throw new Error("Attempt to auto-create GroupComponent.subClass"); 773 else if (Configuration.doAutoCreate()) 774 this.subClass = new StringType(); // bb 775 return this.subClass; 776 } 777 778 public boolean hasSubClassElement() { 779 return this.subClass != null && !this.subClass.isEmpty(); 780 } 781 782 public boolean hasSubClass() { 783 return this.subClass != null && !this.subClass.isEmpty(); 784 } 785 786 /** 787 * @param value {@link #subClass} (Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getSubClass" gives direct access to the value 788 */ 789 public GroupComponent setSubClassElement(StringType value) { 790 this.subClass = value; 791 return this; 792 } 793 794 /** 795 * @return Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment. 796 */ 797 public String getSubClass() { 798 return this.subClass == null ? null : this.subClass.getValue(); 799 } 800 801 /** 802 * @param value Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment. 803 */ 804 public GroupComponent setSubClass(String value) { 805 if (Utilities.noString(value)) 806 this.subClass = null; 807 else { 808 if (this.subClass == null) 809 this.subClass = new StringType(); 810 this.subClass.setValue(value); 811 } 812 return this; 813 } 814 815 /** 816 * @return {@link #subClassDisplay} (A short description for the subclass.). This is the underlying object with id, value and extensions. The accessor "getSubClassDisplay" gives direct access to the value 817 */ 818 public StringType getSubClassDisplayElement() { 819 if (this.subClassDisplay == null) 820 if (Configuration.errorOnAutoCreate()) 821 throw new Error("Attempt to auto-create GroupComponent.subClassDisplay"); 822 else if (Configuration.doAutoCreate()) 823 this.subClassDisplay = new StringType(); // bb 824 return this.subClassDisplay; 825 } 826 827 public boolean hasSubClassDisplayElement() { 828 return this.subClassDisplay != null && !this.subClassDisplay.isEmpty(); 829 } 830 831 public boolean hasSubClassDisplay() { 832 return this.subClassDisplay != null && !this.subClassDisplay.isEmpty(); 833 } 834 835 /** 836 * @param value {@link #subClassDisplay} (A short description for the subclass.). This is the underlying object with id, value and extensions. The accessor "getSubClassDisplay" gives direct access to the value 837 */ 838 public GroupComponent setSubClassDisplayElement(StringType value) { 839 this.subClassDisplay = value; 840 return this; 841 } 842 843 /** 844 * @return A short description for the subclass. 845 */ 846 public String getSubClassDisplay() { 847 return this.subClassDisplay == null ? null : this.subClassDisplay.getValue(); 848 } 849 850 /** 851 * @param value A short description for the subclass. 852 */ 853 public GroupComponent setSubClassDisplay(String value) { 854 if (Utilities.noString(value)) 855 this.subClassDisplay = null; 856 else { 857 if (this.subClassDisplay == null) 858 this.subClassDisplay = new StringType(); 859 this.subClassDisplay.setValue(value); 860 } 861 return this; 862 } 863 864 protected void listChildren(List<Property> children) { 865 super.listChildren(children); 866 children.add(new Property("group", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.", 0, 1, group)); 867 children.add(new Property("groupDisplay", "string", "A short description for the group.", 0, 1, groupDisplay)); 868 children.add(new Property("subGroup", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.", 0, 1, subGroup)); 869 children.add(new Property("subGroupDisplay", "string", "A short description for the subgroup.", 0, 1, subGroupDisplay)); 870 children.add(new Property("plan", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.", 0, 1, plan)); 871 children.add(new Property("planDisplay", "string", "A short description for the plan.", 0, 1, planDisplay)); 872 children.add(new Property("subPlan", "string", "Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.", 0, 1, subPlan)); 873 children.add(new Property("subPlanDisplay", "string", "A short description for the subplan.", 0, 1, subPlanDisplay)); 874 children.add(new Property("class", "string", "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.", 0, 1, class_)); 875 children.add(new Property("classDisplay", "string", "A short description for the class.", 0, 1, classDisplay)); 876 children.add(new Property("subClass", "string", "Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.", 0, 1, subClass)); 877 children.add(new Property("subClassDisplay", "string", "A short description for the subclass.", 0, 1, subClassDisplay)); 878 } 879 880 @Override 881 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 882 switch (_hash) { 883 case 98629247: /*group*/ return new Property("group", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.", 0, 1, group); 884 case 1322335555: /*groupDisplay*/ return new Property("groupDisplay", "string", "A short description for the group.", 0, 1, groupDisplay); 885 case -2101792737: /*subGroup*/ return new Property("subGroup", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.", 0, 1, subGroup); 886 case 1051914147: /*subGroupDisplay*/ return new Property("subGroupDisplay", "string", "A short description for the subgroup.", 0, 1, subGroupDisplay); 887 case 3443497: /*plan*/ return new Property("plan", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.", 0, 1, plan); 888 case -896076455: /*planDisplay*/ return new Property("planDisplay", "string", "A short description for the plan.", 0, 1, planDisplay); 889 case -1868653175: /*subPlan*/ return new Property("subPlan", "string", "Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.", 0, 1, subPlan); 890 case -1736083719: /*subPlanDisplay*/ return new Property("subPlanDisplay", "string", "A short description for the subplan.", 0, 1, subPlanDisplay); 891 case 94742904: /*class*/ return new Property("class", "string", "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.", 0, 1, class_); 892 case 1707405354: /*classDisplay*/ return new Property("classDisplay", "string", "A short description for the class.", 0, 1, classDisplay); 893 case -2105679080: /*subClass*/ return new Property("subClass", "string", "Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.", 0, 1, subClass); 894 case 1436983946: /*subClassDisplay*/ return new Property("subClassDisplay", "string", "A short description for the subclass.", 0, 1, subClassDisplay); 895 default: return super.getNamedProperty(_hash, _name, _checkValid); 896 } 897 898 } 899 900 @Override 901 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 902 switch (hash) { 903 case 98629247: /*group*/ return this.group == null ? new Base[0] : new Base[] {this.group}; // StringType 904 case 1322335555: /*groupDisplay*/ return this.groupDisplay == null ? new Base[0] : new Base[] {this.groupDisplay}; // StringType 905 case -2101792737: /*subGroup*/ return this.subGroup == null ? new Base[0] : new Base[] {this.subGroup}; // StringType 906 case 1051914147: /*subGroupDisplay*/ return this.subGroupDisplay == null ? new Base[0] : new Base[] {this.subGroupDisplay}; // StringType 907 case 3443497: /*plan*/ return this.plan == null ? new Base[0] : new Base[] {this.plan}; // StringType 908 case -896076455: /*planDisplay*/ return this.planDisplay == null ? new Base[0] : new Base[] {this.planDisplay}; // StringType 909 case -1868653175: /*subPlan*/ return this.subPlan == null ? new Base[0] : new Base[] {this.subPlan}; // StringType 910 case -1736083719: /*subPlanDisplay*/ return this.subPlanDisplay == null ? new Base[0] : new Base[] {this.subPlanDisplay}; // StringType 911 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // StringType 912 case 1707405354: /*classDisplay*/ return this.classDisplay == null ? new Base[0] : new Base[] {this.classDisplay}; // StringType 913 case -2105679080: /*subClass*/ return this.subClass == null ? new Base[0] : new Base[] {this.subClass}; // StringType 914 case 1436983946: /*subClassDisplay*/ return this.subClassDisplay == null ? new Base[0] : new Base[] {this.subClassDisplay}; // StringType 915 default: return super.getProperty(hash, name, checkValid); 916 } 917 918 } 919 920 @Override 921 public Base setProperty(int hash, String name, Base value) throws FHIRException { 922 switch (hash) { 923 case 98629247: // group 924 this.group = castToString(value); // StringType 925 return value; 926 case 1322335555: // groupDisplay 927 this.groupDisplay = castToString(value); // StringType 928 return value; 929 case -2101792737: // subGroup 930 this.subGroup = castToString(value); // StringType 931 return value; 932 case 1051914147: // subGroupDisplay 933 this.subGroupDisplay = castToString(value); // StringType 934 return value; 935 case 3443497: // plan 936 this.plan = castToString(value); // StringType 937 return value; 938 case -896076455: // planDisplay 939 this.planDisplay = castToString(value); // StringType 940 return value; 941 case -1868653175: // subPlan 942 this.subPlan = castToString(value); // StringType 943 return value; 944 case -1736083719: // subPlanDisplay 945 this.subPlanDisplay = castToString(value); // StringType 946 return value; 947 case 94742904: // class 948 this.class_ = castToString(value); // StringType 949 return value; 950 case 1707405354: // classDisplay 951 this.classDisplay = castToString(value); // StringType 952 return value; 953 case -2105679080: // subClass 954 this.subClass = castToString(value); // StringType 955 return value; 956 case 1436983946: // subClassDisplay 957 this.subClassDisplay = castToString(value); // StringType 958 return value; 959 default: return super.setProperty(hash, name, value); 960 } 961 962 } 963 964 @Override 965 public Base setProperty(String name, Base value) throws FHIRException { 966 if (name.equals("group")) { 967 this.group = castToString(value); // StringType 968 } else if (name.equals("groupDisplay")) { 969 this.groupDisplay = castToString(value); // StringType 970 } else if (name.equals("subGroup")) { 971 this.subGroup = castToString(value); // StringType 972 } else if (name.equals("subGroupDisplay")) { 973 this.subGroupDisplay = castToString(value); // StringType 974 } else if (name.equals("plan")) { 975 this.plan = castToString(value); // StringType 976 } else if (name.equals("planDisplay")) { 977 this.planDisplay = castToString(value); // StringType 978 } else if (name.equals("subPlan")) { 979 this.subPlan = castToString(value); // StringType 980 } else if (name.equals("subPlanDisplay")) { 981 this.subPlanDisplay = castToString(value); // StringType 982 } else if (name.equals("class")) { 983 this.class_ = castToString(value); // StringType 984 } else if (name.equals("classDisplay")) { 985 this.classDisplay = castToString(value); // StringType 986 } else if (name.equals("subClass")) { 987 this.subClass = castToString(value); // StringType 988 } else if (name.equals("subClassDisplay")) { 989 this.subClassDisplay = castToString(value); // StringType 990 } else 991 return super.setProperty(name, value); 992 return value; 993 } 994 995 @Override 996 public Base makeProperty(int hash, String name) throws FHIRException { 997 switch (hash) { 998 case 98629247: return getGroupElement(); 999 case 1322335555: return getGroupDisplayElement(); 1000 case -2101792737: return getSubGroupElement(); 1001 case 1051914147: return getSubGroupDisplayElement(); 1002 case 3443497: return getPlanElement(); 1003 case -896076455: return getPlanDisplayElement(); 1004 case -1868653175: return getSubPlanElement(); 1005 case -1736083719: return getSubPlanDisplayElement(); 1006 case 94742904: return getClass_Element(); 1007 case 1707405354: return getClassDisplayElement(); 1008 case -2105679080: return getSubClassElement(); 1009 case 1436983946: return getSubClassDisplayElement(); 1010 default: return super.makeProperty(hash, name); 1011 } 1012 1013 } 1014 1015 @Override 1016 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1017 switch (hash) { 1018 case 98629247: /*group*/ return new String[] {"string"}; 1019 case 1322335555: /*groupDisplay*/ return new String[] {"string"}; 1020 case -2101792737: /*subGroup*/ return new String[] {"string"}; 1021 case 1051914147: /*subGroupDisplay*/ return new String[] {"string"}; 1022 case 3443497: /*plan*/ return new String[] {"string"}; 1023 case -896076455: /*planDisplay*/ return new String[] {"string"}; 1024 case -1868653175: /*subPlan*/ return new String[] {"string"}; 1025 case -1736083719: /*subPlanDisplay*/ return new String[] {"string"}; 1026 case 94742904: /*class*/ return new String[] {"string"}; 1027 case 1707405354: /*classDisplay*/ return new String[] {"string"}; 1028 case -2105679080: /*subClass*/ return new String[] {"string"}; 1029 case 1436983946: /*subClassDisplay*/ return new String[] {"string"}; 1030 default: return super.getTypesForProperty(hash, name); 1031 } 1032 1033 } 1034 1035 @Override 1036 public Base addChild(String name) throws FHIRException { 1037 if (name.equals("group")) { 1038 throw new FHIRException("Cannot call addChild on a singleton property Coverage.group"); 1039 } 1040 else if (name.equals("groupDisplay")) { 1041 throw new FHIRException("Cannot call addChild on a singleton property Coverage.groupDisplay"); 1042 } 1043 else if (name.equals("subGroup")) { 1044 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subGroup"); 1045 } 1046 else if (name.equals("subGroupDisplay")) { 1047 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subGroupDisplay"); 1048 } 1049 else if (name.equals("plan")) { 1050 throw new FHIRException("Cannot call addChild on a singleton property Coverage.plan"); 1051 } 1052 else if (name.equals("planDisplay")) { 1053 throw new FHIRException("Cannot call addChild on a singleton property Coverage.planDisplay"); 1054 } 1055 else if (name.equals("subPlan")) { 1056 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subPlan"); 1057 } 1058 else if (name.equals("subPlanDisplay")) { 1059 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subPlanDisplay"); 1060 } 1061 else if (name.equals("class")) { 1062 throw new FHIRException("Cannot call addChild on a singleton property Coverage.class"); 1063 } 1064 else if (name.equals("classDisplay")) { 1065 throw new FHIRException("Cannot call addChild on a singleton property Coverage.classDisplay"); 1066 } 1067 else if (name.equals("subClass")) { 1068 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subClass"); 1069 } 1070 else if (name.equals("subClassDisplay")) { 1071 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subClassDisplay"); 1072 } 1073 else 1074 return super.addChild(name); 1075 } 1076 1077 public GroupComponent copy() { 1078 GroupComponent dst = new GroupComponent(); 1079 copyValues(dst); 1080 dst.group = group == null ? null : group.copy(); 1081 dst.groupDisplay = groupDisplay == null ? null : groupDisplay.copy(); 1082 dst.subGroup = subGroup == null ? null : subGroup.copy(); 1083 dst.subGroupDisplay = subGroupDisplay == null ? null : subGroupDisplay.copy(); 1084 dst.plan = plan == null ? null : plan.copy(); 1085 dst.planDisplay = planDisplay == null ? null : planDisplay.copy(); 1086 dst.subPlan = subPlan == null ? null : subPlan.copy(); 1087 dst.subPlanDisplay = subPlanDisplay == null ? null : subPlanDisplay.copy(); 1088 dst.class_ = class_ == null ? null : class_.copy(); 1089 dst.classDisplay = classDisplay == null ? null : classDisplay.copy(); 1090 dst.subClass = subClass == null ? null : subClass.copy(); 1091 dst.subClassDisplay = subClassDisplay == null ? null : subClassDisplay.copy(); 1092 return dst; 1093 } 1094 1095 @Override 1096 public boolean equalsDeep(Base other_) { 1097 if (!super.equalsDeep(other_)) 1098 return false; 1099 if (!(other_ instanceof GroupComponent)) 1100 return false; 1101 GroupComponent o = (GroupComponent) other_; 1102 return compareDeep(group, o.group, true) && compareDeep(groupDisplay, o.groupDisplay, true) && compareDeep(subGroup, o.subGroup, true) 1103 && compareDeep(subGroupDisplay, o.subGroupDisplay, true) && compareDeep(plan, o.plan, true) && compareDeep(planDisplay, o.planDisplay, true) 1104 && compareDeep(subPlan, o.subPlan, true) && compareDeep(subPlanDisplay, o.subPlanDisplay, true) 1105 && compareDeep(class_, o.class_, true) && compareDeep(classDisplay, o.classDisplay, true) && compareDeep(subClass, o.subClass, true) 1106 && compareDeep(subClassDisplay, o.subClassDisplay, true); 1107 } 1108 1109 @Override 1110 public boolean equalsShallow(Base other_) { 1111 if (!super.equalsShallow(other_)) 1112 return false; 1113 if (!(other_ instanceof GroupComponent)) 1114 return false; 1115 GroupComponent o = (GroupComponent) other_; 1116 return compareValues(group, o.group, true) && compareValues(groupDisplay, o.groupDisplay, true) && compareValues(subGroup, o.subGroup, true) 1117 && compareValues(subGroupDisplay, o.subGroupDisplay, true) && compareValues(plan, o.plan, true) && compareValues(planDisplay, o.planDisplay, true) 1118 && compareValues(subPlan, o.subPlan, true) && compareValues(subPlanDisplay, o.subPlanDisplay, true) 1119 && compareValues(class_, o.class_, true) && compareValues(classDisplay, o.classDisplay, true) && compareValues(subClass, o.subClass, true) 1120 && compareValues(subClassDisplay, o.subClassDisplay, true); 1121 } 1122 1123 public boolean isEmpty() { 1124 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(group, groupDisplay, subGroup 1125 , subGroupDisplay, plan, planDisplay, subPlan, subPlanDisplay, class_, classDisplay 1126 , subClass, subClassDisplay); 1127 } 1128 1129 public String fhirType() { 1130 return "Coverage.grouping"; 1131 1132 } 1133 1134 } 1135 1136 /** 1137 * The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant. 1138 */ 1139 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1140 @Description(shortDefinition="The primary coverage ID", formalDefinition="The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant." ) 1141 protected List<Identifier> identifier; 1142 1143 /** 1144 * The status of the resource instance. 1145 */ 1146 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1147 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 1148 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 1149 protected Enumeration<CoverageStatus> status; 1150 1151 /** 1152 * The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization. 1153 */ 1154 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1155 @Description(shortDefinition="Type of coverage such as medical or accident", formalDefinition="The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization." ) 1156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverage-type") 1157 protected CodeableConcept type; 1158 1159 /** 1160 * The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer. 1161 */ 1162 @Child(name = "policyHolder", type = {Patient.class, RelatedPerson.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 1163 @Description(shortDefinition="Owner of the policy", formalDefinition="The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer." ) 1164 protected Reference policyHolder; 1165 1166 /** 1167 * The actual object that is the target of the reference (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1168 */ 1169 protected Resource policyHolderTarget; 1170 1171 /** 1172 * The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due. 1173 */ 1174 @Child(name = "subscriber", type = {Patient.class, RelatedPerson.class}, order=4, min=0, max=1, modifier=false, summary=true) 1175 @Description(shortDefinition="Subscriber to the policy", formalDefinition="The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due." ) 1176 protected Reference subscriber; 1177 1178 /** 1179 * The actual object that is the target of the reference (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1180 */ 1181 protected Resource subscriberTarget; 1182 1183 /** 1184 * The insurer assigned ID for the Subscriber. 1185 */ 1186 @Child(name = "subscriberId", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1187 @Description(shortDefinition="ID assigned to the Subscriber", formalDefinition="The insurer assigned ID for the Subscriber." ) 1188 protected StringType subscriberId; 1189 1190 /** 1191 * The party who benefits from the insurance coverage., the patient when services are provided. 1192 */ 1193 @Child(name = "beneficiary", type = {Patient.class}, order=6, min=0, max=1, modifier=false, summary=true) 1194 @Description(shortDefinition="Plan Beneficiary", formalDefinition="The party who benefits from the insurance coverage., the patient when services are provided." ) 1195 protected Reference beneficiary; 1196 1197 /** 1198 * The actual object that is the target of the reference (The party who benefits from the insurance coverage., the patient when services are provided.) 1199 */ 1200 protected Patient beneficiaryTarget; 1201 1202 /** 1203 * The relationship of beneficiary (patient) to the subscriber. 1204 */ 1205 @Child(name = "relationship", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1206 @Description(shortDefinition="Beneficiary relationship to the Subscriber", formalDefinition="The relationship of beneficiary (patient) to the subscriber." ) 1207 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/policyholder-relationship") 1208 protected CodeableConcept relationship; 1209 1210 /** 1211 * Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force. 1212 */ 1213 @Child(name = "period", type = {Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 1214 @Description(shortDefinition="Coverage start and end dates", formalDefinition="Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force." ) 1215 protected Period period; 1216 1217 /** 1218 * The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number). 1219 */ 1220 @Child(name = "payor", type = {Organization.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1221 @Description(shortDefinition="Identifier for the plan or agreement issuer", formalDefinition="The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number)." ) 1222 protected List<Reference> payor; 1223 /** 1224 * The actual objects that are the target of the reference (The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).) 1225 */ 1226 protected List<Resource> payorTarget; 1227 1228 1229 /** 1230 * A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan. 1231 */ 1232 @Child(name = "grouping", type = {}, order=10, min=0, max=1, modifier=false, summary=false) 1233 @Description(shortDefinition="Additional coverage classifications", formalDefinition="A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan." ) 1234 protected GroupComponent grouping; 1235 1236 /** 1237 * A unique identifier for a dependent under the coverage. 1238 */ 1239 @Child(name = "dependent", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1240 @Description(shortDefinition="Dependent number", formalDefinition="A unique identifier for a dependent under the coverage." ) 1241 protected StringType dependent; 1242 1243 /** 1244 * An optional counter for a particular instance of the identified coverage which increments upon each renewal. 1245 */ 1246 @Child(name = "sequence", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1247 @Description(shortDefinition="The plan instance or sequence counter", formalDefinition="An optional counter for a particular instance of the identified coverage which increments upon each renewal." ) 1248 protected StringType sequence; 1249 1250 /** 1251 * The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care. 1252 */ 1253 @Child(name = "order", type = {PositiveIntType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1254 @Description(shortDefinition="Relative order of the coverage", formalDefinition="The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care." ) 1255 protected PositiveIntType order; 1256 1257 /** 1258 * The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1259 */ 1260 @Child(name = "network", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=true) 1261 @Description(shortDefinition="Insurer network", formalDefinition="The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply." ) 1262 protected StringType network; 1263 1264 /** 1265 * The policy(s) which constitute this insurance coverage. 1266 */ 1267 @Child(name = "contract", type = {Contract.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1268 @Description(shortDefinition="Contract details", formalDefinition="The policy(s) which constitute this insurance coverage." ) 1269 protected List<Reference> contract; 1270 /** 1271 * The actual objects that are the target of the reference (The policy(s) which constitute this insurance coverage.) 1272 */ 1273 protected List<Contract> contractTarget; 1274 1275 1276 private static final long serialVersionUID = -1719168406L; 1277 1278 /** 1279 * Constructor 1280 */ 1281 public Coverage() { 1282 super(); 1283 } 1284 1285 /** 1286 * @return {@link #identifier} (The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant.) 1287 */ 1288 public List<Identifier> getIdentifier() { 1289 if (this.identifier == null) 1290 this.identifier = new ArrayList<Identifier>(); 1291 return this.identifier; 1292 } 1293 1294 /** 1295 * @return Returns a reference to <code>this</code> for easy method chaining 1296 */ 1297 public Coverage setIdentifier(List<Identifier> theIdentifier) { 1298 this.identifier = theIdentifier; 1299 return this; 1300 } 1301 1302 public boolean hasIdentifier() { 1303 if (this.identifier == null) 1304 return false; 1305 for (Identifier item : this.identifier) 1306 if (!item.isEmpty()) 1307 return true; 1308 return false; 1309 } 1310 1311 public Identifier addIdentifier() { //3 1312 Identifier t = new Identifier(); 1313 if (this.identifier == null) 1314 this.identifier = new ArrayList<Identifier>(); 1315 this.identifier.add(t); 1316 return t; 1317 } 1318 1319 public Coverage addIdentifier(Identifier t) { //3 1320 if (t == null) 1321 return this; 1322 if (this.identifier == null) 1323 this.identifier = new ArrayList<Identifier>(); 1324 this.identifier.add(t); 1325 return this; 1326 } 1327 1328 /** 1329 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1330 */ 1331 public Identifier getIdentifierFirstRep() { 1332 if (getIdentifier().isEmpty()) { 1333 addIdentifier(); 1334 } 1335 return getIdentifier().get(0); 1336 } 1337 1338 /** 1339 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1340 */ 1341 public Enumeration<CoverageStatus> getStatusElement() { 1342 if (this.status == null) 1343 if (Configuration.errorOnAutoCreate()) 1344 throw new Error("Attempt to auto-create Coverage.status"); 1345 else if (Configuration.doAutoCreate()) 1346 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); // bb 1347 return this.status; 1348 } 1349 1350 public boolean hasStatusElement() { 1351 return this.status != null && !this.status.isEmpty(); 1352 } 1353 1354 public boolean hasStatus() { 1355 return this.status != null && !this.status.isEmpty(); 1356 } 1357 1358 /** 1359 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1360 */ 1361 public Coverage setStatusElement(Enumeration<CoverageStatus> value) { 1362 this.status = value; 1363 return this; 1364 } 1365 1366 /** 1367 * @return The status of the resource instance. 1368 */ 1369 public CoverageStatus getStatus() { 1370 return this.status == null ? null : this.status.getValue(); 1371 } 1372 1373 /** 1374 * @param value The status of the resource instance. 1375 */ 1376 public Coverage setStatus(CoverageStatus value) { 1377 if (value == null) 1378 this.status = null; 1379 else { 1380 if (this.status == null) 1381 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); 1382 this.status.setValue(value); 1383 } 1384 return this; 1385 } 1386 1387 /** 1388 * @return {@link #type} (The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.) 1389 */ 1390 public CodeableConcept getType() { 1391 if (this.type == null) 1392 if (Configuration.errorOnAutoCreate()) 1393 throw new Error("Attempt to auto-create Coverage.type"); 1394 else if (Configuration.doAutoCreate()) 1395 this.type = new CodeableConcept(); // cc 1396 return this.type; 1397 } 1398 1399 public boolean hasType() { 1400 return this.type != null && !this.type.isEmpty(); 1401 } 1402 1403 /** 1404 * @param value {@link #type} (The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.) 1405 */ 1406 public Coverage setType(CodeableConcept value) { 1407 this.type = value; 1408 return this; 1409 } 1410 1411 /** 1412 * @return {@link #policyHolder} (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1413 */ 1414 public Reference getPolicyHolder() { 1415 if (this.policyHolder == null) 1416 if (Configuration.errorOnAutoCreate()) 1417 throw new Error("Attempt to auto-create Coverage.policyHolder"); 1418 else if (Configuration.doAutoCreate()) 1419 this.policyHolder = new Reference(); // cc 1420 return this.policyHolder; 1421 } 1422 1423 public boolean hasPolicyHolder() { 1424 return this.policyHolder != null && !this.policyHolder.isEmpty(); 1425 } 1426 1427 /** 1428 * @param value {@link #policyHolder} (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1429 */ 1430 public Coverage setPolicyHolder(Reference value) { 1431 this.policyHolder = value; 1432 return this; 1433 } 1434 1435 /** 1436 * @return {@link #policyHolder} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1437 */ 1438 public Resource getPolicyHolderTarget() { 1439 return this.policyHolderTarget; 1440 } 1441 1442 /** 1443 * @param value {@link #policyHolder} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1444 */ 1445 public Coverage setPolicyHolderTarget(Resource value) { 1446 this.policyHolderTarget = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return {@link #subscriber} (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1452 */ 1453 public Reference getSubscriber() { 1454 if (this.subscriber == null) 1455 if (Configuration.errorOnAutoCreate()) 1456 throw new Error("Attempt to auto-create Coverage.subscriber"); 1457 else if (Configuration.doAutoCreate()) 1458 this.subscriber = new Reference(); // cc 1459 return this.subscriber; 1460 } 1461 1462 public boolean hasSubscriber() { 1463 return this.subscriber != null && !this.subscriber.isEmpty(); 1464 } 1465 1466 /** 1467 * @param value {@link #subscriber} (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1468 */ 1469 public Coverage setSubscriber(Reference value) { 1470 this.subscriber = value; 1471 return this; 1472 } 1473 1474 /** 1475 * @return {@link #subscriber} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1476 */ 1477 public Resource getSubscriberTarget() { 1478 return this.subscriberTarget; 1479 } 1480 1481 /** 1482 * @param value {@link #subscriber} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1483 */ 1484 public Coverage setSubscriberTarget(Resource value) { 1485 this.subscriberTarget = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #subscriberId} (The insurer assigned ID for the Subscriber.). This is the underlying object with id, value and extensions. The accessor "getSubscriberId" gives direct access to the value 1491 */ 1492 public StringType getSubscriberIdElement() { 1493 if (this.subscriberId == null) 1494 if (Configuration.errorOnAutoCreate()) 1495 throw new Error("Attempt to auto-create Coverage.subscriberId"); 1496 else if (Configuration.doAutoCreate()) 1497 this.subscriberId = new StringType(); // bb 1498 return this.subscriberId; 1499 } 1500 1501 public boolean hasSubscriberIdElement() { 1502 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1503 } 1504 1505 public boolean hasSubscriberId() { 1506 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1507 } 1508 1509 /** 1510 * @param value {@link #subscriberId} (The insurer assigned ID for the Subscriber.). This is the underlying object with id, value and extensions. The accessor "getSubscriberId" gives direct access to the value 1511 */ 1512 public Coverage setSubscriberIdElement(StringType value) { 1513 this.subscriberId = value; 1514 return this; 1515 } 1516 1517 /** 1518 * @return The insurer assigned ID for the Subscriber. 1519 */ 1520 public String getSubscriberId() { 1521 return this.subscriberId == null ? null : this.subscriberId.getValue(); 1522 } 1523 1524 /** 1525 * @param value The insurer assigned ID for the Subscriber. 1526 */ 1527 public Coverage setSubscriberId(String value) { 1528 if (Utilities.noString(value)) 1529 this.subscriberId = null; 1530 else { 1531 if (this.subscriberId == null) 1532 this.subscriberId = new StringType(); 1533 this.subscriberId.setValue(value); 1534 } 1535 return this; 1536 } 1537 1538 /** 1539 * @return {@link #beneficiary} (The party who benefits from the insurance coverage., the patient when services are provided.) 1540 */ 1541 public Reference getBeneficiary() { 1542 if (this.beneficiary == null) 1543 if (Configuration.errorOnAutoCreate()) 1544 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1545 else if (Configuration.doAutoCreate()) 1546 this.beneficiary = new Reference(); // cc 1547 return this.beneficiary; 1548 } 1549 1550 public boolean hasBeneficiary() { 1551 return this.beneficiary != null && !this.beneficiary.isEmpty(); 1552 } 1553 1554 /** 1555 * @param value {@link #beneficiary} (The party who benefits from the insurance coverage., the patient when services are provided.) 1556 */ 1557 public Coverage setBeneficiary(Reference value) { 1558 this.beneficiary = value; 1559 return this; 1560 } 1561 1562 /** 1563 * @return {@link #beneficiary} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The party who benefits from the insurance coverage., the patient when services are provided.) 1564 */ 1565 public Patient getBeneficiaryTarget() { 1566 if (this.beneficiaryTarget == null) 1567 if (Configuration.errorOnAutoCreate()) 1568 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1569 else if (Configuration.doAutoCreate()) 1570 this.beneficiaryTarget = new Patient(); // aa 1571 return this.beneficiaryTarget; 1572 } 1573 1574 /** 1575 * @param value {@link #beneficiary} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The party who benefits from the insurance coverage., the patient when services are provided.) 1576 */ 1577 public Coverage setBeneficiaryTarget(Patient value) { 1578 this.beneficiaryTarget = value; 1579 return this; 1580 } 1581 1582 /** 1583 * @return {@link #relationship} (The relationship of beneficiary (patient) to the subscriber.) 1584 */ 1585 public CodeableConcept getRelationship() { 1586 if (this.relationship == null) 1587 if (Configuration.errorOnAutoCreate()) 1588 throw new Error("Attempt to auto-create Coverage.relationship"); 1589 else if (Configuration.doAutoCreate()) 1590 this.relationship = new CodeableConcept(); // cc 1591 return this.relationship; 1592 } 1593 1594 public boolean hasRelationship() { 1595 return this.relationship != null && !this.relationship.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #relationship} (The relationship of beneficiary (patient) to the subscriber.) 1600 */ 1601 public Coverage setRelationship(CodeableConcept value) { 1602 this.relationship = value; 1603 return this; 1604 } 1605 1606 /** 1607 * @return {@link #period} (Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.) 1608 */ 1609 public Period getPeriod() { 1610 if (this.period == null) 1611 if (Configuration.errorOnAutoCreate()) 1612 throw new Error("Attempt to auto-create Coverage.period"); 1613 else if (Configuration.doAutoCreate()) 1614 this.period = new Period(); // cc 1615 return this.period; 1616 } 1617 1618 public boolean hasPeriod() { 1619 return this.period != null && !this.period.isEmpty(); 1620 } 1621 1622 /** 1623 * @param value {@link #period} (Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.) 1624 */ 1625 public Coverage setPeriod(Period value) { 1626 this.period = value; 1627 return this; 1628 } 1629 1630 /** 1631 * @return {@link #payor} (The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).) 1632 */ 1633 public List<Reference> getPayor() { 1634 if (this.payor == null) 1635 this.payor = new ArrayList<Reference>(); 1636 return this.payor; 1637 } 1638 1639 /** 1640 * @return Returns a reference to <code>this</code> for easy method chaining 1641 */ 1642 public Coverage setPayor(List<Reference> thePayor) { 1643 this.payor = thePayor; 1644 return this; 1645 } 1646 1647 public boolean hasPayor() { 1648 if (this.payor == null) 1649 return false; 1650 for (Reference item : this.payor) 1651 if (!item.isEmpty()) 1652 return true; 1653 return false; 1654 } 1655 1656 public Reference addPayor() { //3 1657 Reference t = new Reference(); 1658 if (this.payor == null) 1659 this.payor = new ArrayList<Reference>(); 1660 this.payor.add(t); 1661 return t; 1662 } 1663 1664 public Coverage addPayor(Reference t) { //3 1665 if (t == null) 1666 return this; 1667 if (this.payor == null) 1668 this.payor = new ArrayList<Reference>(); 1669 this.payor.add(t); 1670 return this; 1671 } 1672 1673 /** 1674 * @return The first repetition of repeating field {@link #payor}, creating it if it does not already exist 1675 */ 1676 public Reference getPayorFirstRep() { 1677 if (getPayor().isEmpty()) { 1678 addPayor(); 1679 } 1680 return getPayor().get(0); 1681 } 1682 1683 /** 1684 * @deprecated Use Reference#setResource(IBaseResource) instead 1685 */ 1686 @Deprecated 1687 public List<Resource> getPayorTarget() { 1688 if (this.payorTarget == null) 1689 this.payorTarget = new ArrayList<Resource>(); 1690 return this.payorTarget; 1691 } 1692 1693 /** 1694 * @return {@link #grouping} (A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.) 1695 */ 1696 public GroupComponent getGrouping() { 1697 if (this.grouping == null) 1698 if (Configuration.errorOnAutoCreate()) 1699 throw new Error("Attempt to auto-create Coverage.grouping"); 1700 else if (Configuration.doAutoCreate()) 1701 this.grouping = new GroupComponent(); // cc 1702 return this.grouping; 1703 } 1704 1705 public boolean hasGrouping() { 1706 return this.grouping != null && !this.grouping.isEmpty(); 1707 } 1708 1709 /** 1710 * @param value {@link #grouping} (A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.) 1711 */ 1712 public Coverage setGrouping(GroupComponent value) { 1713 this.grouping = value; 1714 return this; 1715 } 1716 1717 /** 1718 * @return {@link #dependent} (A unique identifier for a dependent under the coverage.). This is the underlying object with id, value and extensions. The accessor "getDependent" gives direct access to the value 1719 */ 1720 public StringType getDependentElement() { 1721 if (this.dependent == null) 1722 if (Configuration.errorOnAutoCreate()) 1723 throw new Error("Attempt to auto-create Coverage.dependent"); 1724 else if (Configuration.doAutoCreate()) 1725 this.dependent = new StringType(); // bb 1726 return this.dependent; 1727 } 1728 1729 public boolean hasDependentElement() { 1730 return this.dependent != null && !this.dependent.isEmpty(); 1731 } 1732 1733 public boolean hasDependent() { 1734 return this.dependent != null && !this.dependent.isEmpty(); 1735 } 1736 1737 /** 1738 * @param value {@link #dependent} (A unique identifier for a dependent under the coverage.). This is the underlying object with id, value and extensions. The accessor "getDependent" gives direct access to the value 1739 */ 1740 public Coverage setDependentElement(StringType value) { 1741 this.dependent = value; 1742 return this; 1743 } 1744 1745 /** 1746 * @return A unique identifier for a dependent under the coverage. 1747 */ 1748 public String getDependent() { 1749 return this.dependent == null ? null : this.dependent.getValue(); 1750 } 1751 1752 /** 1753 * @param value A unique identifier for a dependent under the coverage. 1754 */ 1755 public Coverage setDependent(String value) { 1756 if (Utilities.noString(value)) 1757 this.dependent = null; 1758 else { 1759 if (this.dependent == null) 1760 this.dependent = new StringType(); 1761 this.dependent.setValue(value); 1762 } 1763 return this; 1764 } 1765 1766 /** 1767 * @return {@link #sequence} (An optional counter for a particular instance of the identified coverage which increments upon each renewal.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1768 */ 1769 public StringType getSequenceElement() { 1770 if (this.sequence == null) 1771 if (Configuration.errorOnAutoCreate()) 1772 throw new Error("Attempt to auto-create Coverage.sequence"); 1773 else if (Configuration.doAutoCreate()) 1774 this.sequence = new StringType(); // bb 1775 return this.sequence; 1776 } 1777 1778 public boolean hasSequenceElement() { 1779 return this.sequence != null && !this.sequence.isEmpty(); 1780 } 1781 1782 public boolean hasSequence() { 1783 return this.sequence != null && !this.sequence.isEmpty(); 1784 } 1785 1786 /** 1787 * @param value {@link #sequence} (An optional counter for a particular instance of the identified coverage which increments upon each renewal.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1788 */ 1789 public Coverage setSequenceElement(StringType value) { 1790 this.sequence = value; 1791 return this; 1792 } 1793 1794 /** 1795 * @return An optional counter for a particular instance of the identified coverage which increments upon each renewal. 1796 */ 1797 public String getSequence() { 1798 return this.sequence == null ? null : this.sequence.getValue(); 1799 } 1800 1801 /** 1802 * @param value An optional counter for a particular instance of the identified coverage which increments upon each renewal. 1803 */ 1804 public Coverage setSequence(String value) { 1805 if (Utilities.noString(value)) 1806 this.sequence = null; 1807 else { 1808 if (this.sequence == null) 1809 this.sequence = new StringType(); 1810 this.sequence.setValue(value); 1811 } 1812 return this; 1813 } 1814 1815 /** 1816 * @return {@link #order} (The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.). This is the underlying object with id, value and extensions. The accessor "getOrder" gives direct access to the value 1817 */ 1818 public PositiveIntType getOrderElement() { 1819 if (this.order == null) 1820 if (Configuration.errorOnAutoCreate()) 1821 throw new Error("Attempt to auto-create Coverage.order"); 1822 else if (Configuration.doAutoCreate()) 1823 this.order = new PositiveIntType(); // bb 1824 return this.order; 1825 } 1826 1827 public boolean hasOrderElement() { 1828 return this.order != null && !this.order.isEmpty(); 1829 } 1830 1831 public boolean hasOrder() { 1832 return this.order != null && !this.order.isEmpty(); 1833 } 1834 1835 /** 1836 * @param value {@link #order} (The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.). This is the underlying object with id, value and extensions. The accessor "getOrder" gives direct access to the value 1837 */ 1838 public Coverage setOrderElement(PositiveIntType value) { 1839 this.order = value; 1840 return this; 1841 } 1842 1843 /** 1844 * @return The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care. 1845 */ 1846 public int getOrder() { 1847 return this.order == null || this.order.isEmpty() ? 0 : this.order.getValue(); 1848 } 1849 1850 /** 1851 * @param value The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care. 1852 */ 1853 public Coverage setOrder(int value) { 1854 if (this.order == null) 1855 this.order = new PositiveIntType(); 1856 this.order.setValue(value); 1857 return this; 1858 } 1859 1860 /** 1861 * @return {@link #network} (The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.). This is the underlying object with id, value and extensions. The accessor "getNetwork" gives direct access to the value 1862 */ 1863 public StringType getNetworkElement() { 1864 if (this.network == null) 1865 if (Configuration.errorOnAutoCreate()) 1866 throw new Error("Attempt to auto-create Coverage.network"); 1867 else if (Configuration.doAutoCreate()) 1868 this.network = new StringType(); // bb 1869 return this.network; 1870 } 1871 1872 public boolean hasNetworkElement() { 1873 return this.network != null && !this.network.isEmpty(); 1874 } 1875 1876 public boolean hasNetwork() { 1877 return this.network != null && !this.network.isEmpty(); 1878 } 1879 1880 /** 1881 * @param value {@link #network} (The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.). This is the underlying object with id, value and extensions. The accessor "getNetwork" gives direct access to the value 1882 */ 1883 public Coverage setNetworkElement(StringType value) { 1884 this.network = value; 1885 return this; 1886 } 1887 1888 /** 1889 * @return The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1890 */ 1891 public String getNetwork() { 1892 return this.network == null ? null : this.network.getValue(); 1893 } 1894 1895 /** 1896 * @param value The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1897 */ 1898 public Coverage setNetwork(String value) { 1899 if (Utilities.noString(value)) 1900 this.network = null; 1901 else { 1902 if (this.network == null) 1903 this.network = new StringType(); 1904 this.network.setValue(value); 1905 } 1906 return this; 1907 } 1908 1909 /** 1910 * @return {@link #contract} (The policy(s) which constitute this insurance coverage.) 1911 */ 1912 public List<Reference> getContract() { 1913 if (this.contract == null) 1914 this.contract = new ArrayList<Reference>(); 1915 return this.contract; 1916 } 1917 1918 /** 1919 * @return Returns a reference to <code>this</code> for easy method chaining 1920 */ 1921 public Coverage setContract(List<Reference> theContract) { 1922 this.contract = theContract; 1923 return this; 1924 } 1925 1926 public boolean hasContract() { 1927 if (this.contract == null) 1928 return false; 1929 for (Reference item : this.contract) 1930 if (!item.isEmpty()) 1931 return true; 1932 return false; 1933 } 1934 1935 public Reference addContract() { //3 1936 Reference t = new Reference(); 1937 if (this.contract == null) 1938 this.contract = new ArrayList<Reference>(); 1939 this.contract.add(t); 1940 return t; 1941 } 1942 1943 public Coverage addContract(Reference t) { //3 1944 if (t == null) 1945 return this; 1946 if (this.contract == null) 1947 this.contract = new ArrayList<Reference>(); 1948 this.contract.add(t); 1949 return this; 1950 } 1951 1952 /** 1953 * @return The first repetition of repeating field {@link #contract}, creating it if it does not already exist 1954 */ 1955 public Reference getContractFirstRep() { 1956 if (getContract().isEmpty()) { 1957 addContract(); 1958 } 1959 return getContract().get(0); 1960 } 1961 1962 /** 1963 * @deprecated Use Reference#setResource(IBaseResource) instead 1964 */ 1965 @Deprecated 1966 public List<Contract> getContractTarget() { 1967 if (this.contractTarget == null) 1968 this.contractTarget = new ArrayList<Contract>(); 1969 return this.contractTarget; 1970 } 1971 1972 /** 1973 * @deprecated Use Reference#setResource(IBaseResource) instead 1974 */ 1975 @Deprecated 1976 public Contract addContractTarget() { 1977 Contract r = new Contract(); 1978 if (this.contractTarget == null) 1979 this.contractTarget = new ArrayList<Contract>(); 1980 this.contractTarget.add(r); 1981 return r; 1982 } 1983 1984 protected void listChildren(List<Property> children) { 1985 super.listChildren(children); 1986 children.add(new Property("identifier", "Identifier", "The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1987 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 1988 children.add(new Property("type", "CodeableConcept", "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 0, 1, type)); 1989 children.add(new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", "The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.", 0, 1, policyHolder)); 1990 children.add(new Property("subscriber", "Reference(Patient|RelatedPerson)", "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 0, 1, subscriber)); 1991 children.add(new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 1, subscriberId)); 1992 children.add(new Property("beneficiary", "Reference(Patient)", "The party who benefits from the insurance coverage., the patient when services are provided.", 0, 1, beneficiary)); 1993 children.add(new Property("relationship", "CodeableConcept", "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship)); 1994 children.add(new Property("period", "Period", "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 0, 1, period)); 1995 children.add(new Property("payor", "Reference(Organization|Patient|RelatedPerson)", "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).", 0, java.lang.Integer.MAX_VALUE, payor)); 1996 children.add(new Property("grouping", "", "A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 0, 1, grouping)); 1997 children.add(new Property("dependent", "string", "A unique identifier for a dependent under the coverage.", 0, 1, dependent)); 1998 children.add(new Property("sequence", "string", "An optional counter for a particular instance of the identified coverage which increments upon each renewal.", 0, 1, sequence)); 1999 children.add(new Property("order", "positiveInt", "The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.", 0, 1, order)); 2000 children.add(new Property("network", "string", "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 0, 1, network)); 2001 children.add(new Property("contract", "Reference(Contract)", "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract)); 2002 } 2003 2004 @Override 2005 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2006 switch (_hash) { 2007 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant.", 0, java.lang.Integer.MAX_VALUE, identifier); 2008 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2009 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 0, 1, type); 2010 case 2046898558: /*policyHolder*/ return new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", "The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.", 0, 1, policyHolder); 2011 case -1219769240: /*subscriber*/ return new Property("subscriber", "Reference(Patient|RelatedPerson)", "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 0, 1, subscriber); 2012 case 327834531: /*subscriberId*/ return new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 1, subscriberId); 2013 case -565102875: /*beneficiary*/ return new Property("beneficiary", "Reference(Patient)", "The party who benefits from the insurance coverage., the patient when services are provided.", 0, 1, beneficiary); 2014 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship); 2015 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 0, 1, period); 2016 case 106443915: /*payor*/ return new Property("payor", "Reference(Organization|Patient|RelatedPerson)", "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).", 0, java.lang.Integer.MAX_VALUE, payor); 2017 case 506371331: /*grouping*/ return new Property("grouping", "", "A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 0, 1, grouping); 2018 case -1109226753: /*dependent*/ return new Property("dependent", "string", "A unique identifier for a dependent under the coverage.", 0, 1, dependent); 2019 case 1349547969: /*sequence*/ return new Property("sequence", "string", "An optional counter for a particular instance of the identified coverage which increments upon each renewal.", 0, 1, sequence); 2020 case 106006350: /*order*/ return new Property("order", "positiveInt", "The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.", 0, 1, order); 2021 case 1843485230: /*network*/ return new Property("network", "string", "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 0, 1, network); 2022 case -566947566: /*contract*/ return new Property("contract", "Reference(Contract)", "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract); 2023 default: return super.getNamedProperty(_hash, _name, _checkValid); 2024 } 2025 2026 } 2027 2028 @Override 2029 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2030 switch (hash) { 2031 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2032 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CoverageStatus> 2033 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2034 case 2046898558: /*policyHolder*/ return this.policyHolder == null ? new Base[0] : new Base[] {this.policyHolder}; // Reference 2035 case -1219769240: /*subscriber*/ return this.subscriber == null ? new Base[0] : new Base[] {this.subscriber}; // Reference 2036 case 327834531: /*subscriberId*/ return this.subscriberId == null ? new Base[0] : new Base[] {this.subscriberId}; // StringType 2037 case -565102875: /*beneficiary*/ return this.beneficiary == null ? new Base[0] : new Base[] {this.beneficiary}; // Reference 2038 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 2039 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 2040 case 106443915: /*payor*/ return this.payor == null ? new Base[0] : this.payor.toArray(new Base[this.payor.size()]); // Reference 2041 case 506371331: /*grouping*/ return this.grouping == null ? new Base[0] : new Base[] {this.grouping}; // GroupComponent 2042 case -1109226753: /*dependent*/ return this.dependent == null ? new Base[0] : new Base[] {this.dependent}; // StringType 2043 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // StringType 2044 case 106006350: /*order*/ return this.order == null ? new Base[0] : new Base[] {this.order}; // PositiveIntType 2045 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // StringType 2046 case -566947566: /*contract*/ return this.contract == null ? new Base[0] : this.contract.toArray(new Base[this.contract.size()]); // Reference 2047 default: return super.getProperty(hash, name, checkValid); 2048 } 2049 2050 } 2051 2052 @Override 2053 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2054 switch (hash) { 2055 case -1618432855: // identifier 2056 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2057 return value; 2058 case -892481550: // status 2059 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2060 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2061 return value; 2062 case 3575610: // type 2063 this.type = castToCodeableConcept(value); // CodeableConcept 2064 return value; 2065 case 2046898558: // policyHolder 2066 this.policyHolder = castToReference(value); // Reference 2067 return value; 2068 case -1219769240: // subscriber 2069 this.subscriber = castToReference(value); // Reference 2070 return value; 2071 case 327834531: // subscriberId 2072 this.subscriberId = castToString(value); // StringType 2073 return value; 2074 case -565102875: // beneficiary 2075 this.beneficiary = castToReference(value); // Reference 2076 return value; 2077 case -261851592: // relationship 2078 this.relationship = castToCodeableConcept(value); // CodeableConcept 2079 return value; 2080 case -991726143: // period 2081 this.period = castToPeriod(value); // Period 2082 return value; 2083 case 106443915: // payor 2084 this.getPayor().add(castToReference(value)); // Reference 2085 return value; 2086 case 506371331: // grouping 2087 this.grouping = (GroupComponent) value; // GroupComponent 2088 return value; 2089 case -1109226753: // dependent 2090 this.dependent = castToString(value); // StringType 2091 return value; 2092 case 1349547969: // sequence 2093 this.sequence = castToString(value); // StringType 2094 return value; 2095 case 106006350: // order 2096 this.order = castToPositiveInt(value); // PositiveIntType 2097 return value; 2098 case 1843485230: // network 2099 this.network = castToString(value); // StringType 2100 return value; 2101 case -566947566: // contract 2102 this.getContract().add(castToReference(value)); // Reference 2103 return value; 2104 default: return super.setProperty(hash, name, value); 2105 } 2106 2107 } 2108 2109 @Override 2110 public Base setProperty(String name, Base value) throws FHIRException { 2111 if (name.equals("identifier")) { 2112 this.getIdentifier().add(castToIdentifier(value)); 2113 } else if (name.equals("status")) { 2114 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2115 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2116 } else if (name.equals("type")) { 2117 this.type = castToCodeableConcept(value); // CodeableConcept 2118 } else if (name.equals("policyHolder")) { 2119 this.policyHolder = castToReference(value); // Reference 2120 } else if (name.equals("subscriber")) { 2121 this.subscriber = castToReference(value); // Reference 2122 } else if (name.equals("subscriberId")) { 2123 this.subscriberId = castToString(value); // StringType 2124 } else if (name.equals("beneficiary")) { 2125 this.beneficiary = castToReference(value); // Reference 2126 } else if (name.equals("relationship")) { 2127 this.relationship = castToCodeableConcept(value); // CodeableConcept 2128 } else if (name.equals("period")) { 2129 this.period = castToPeriod(value); // Period 2130 } else if (name.equals("payor")) { 2131 this.getPayor().add(castToReference(value)); 2132 } else if (name.equals("grouping")) { 2133 this.grouping = (GroupComponent) value; // GroupComponent 2134 } else if (name.equals("dependent")) { 2135 this.dependent = castToString(value); // StringType 2136 } else if (name.equals("sequence")) { 2137 this.sequence = castToString(value); // StringType 2138 } else if (name.equals("order")) { 2139 this.order = castToPositiveInt(value); // PositiveIntType 2140 } else if (name.equals("network")) { 2141 this.network = castToString(value); // StringType 2142 } else if (name.equals("contract")) { 2143 this.getContract().add(castToReference(value)); 2144 } else 2145 return super.setProperty(name, value); 2146 return value; 2147 } 2148 2149 @Override 2150 public Base makeProperty(int hash, String name) throws FHIRException { 2151 switch (hash) { 2152 case -1618432855: return addIdentifier(); 2153 case -892481550: return getStatusElement(); 2154 case 3575610: return getType(); 2155 case 2046898558: return getPolicyHolder(); 2156 case -1219769240: return getSubscriber(); 2157 case 327834531: return getSubscriberIdElement(); 2158 case -565102875: return getBeneficiary(); 2159 case -261851592: return getRelationship(); 2160 case -991726143: return getPeriod(); 2161 case 106443915: return addPayor(); 2162 case 506371331: return getGrouping(); 2163 case -1109226753: return getDependentElement(); 2164 case 1349547969: return getSequenceElement(); 2165 case 106006350: return getOrderElement(); 2166 case 1843485230: return getNetworkElement(); 2167 case -566947566: return addContract(); 2168 default: return super.makeProperty(hash, name); 2169 } 2170 2171 } 2172 2173 @Override 2174 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2175 switch (hash) { 2176 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2177 case -892481550: /*status*/ return new String[] {"code"}; 2178 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2179 case 2046898558: /*policyHolder*/ return new String[] {"Reference"}; 2180 case -1219769240: /*subscriber*/ return new String[] {"Reference"}; 2181 case 327834531: /*subscriberId*/ return new String[] {"string"}; 2182 case -565102875: /*beneficiary*/ return new String[] {"Reference"}; 2183 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 2184 case -991726143: /*period*/ return new String[] {"Period"}; 2185 case 106443915: /*payor*/ return new String[] {"Reference"}; 2186 case 506371331: /*grouping*/ return new String[] {}; 2187 case -1109226753: /*dependent*/ return new String[] {"string"}; 2188 case 1349547969: /*sequence*/ return new String[] {"string"}; 2189 case 106006350: /*order*/ return new String[] {"positiveInt"}; 2190 case 1843485230: /*network*/ return new String[] {"string"}; 2191 case -566947566: /*contract*/ return new String[] {"Reference"}; 2192 default: return super.getTypesForProperty(hash, name); 2193 } 2194 2195 } 2196 2197 @Override 2198 public Base addChild(String name) throws FHIRException { 2199 if (name.equals("identifier")) { 2200 return addIdentifier(); 2201 } 2202 else if (name.equals("status")) { 2203 throw new FHIRException("Cannot call addChild on a singleton property Coverage.status"); 2204 } 2205 else if (name.equals("type")) { 2206 this.type = new CodeableConcept(); 2207 return this.type; 2208 } 2209 else if (name.equals("policyHolder")) { 2210 this.policyHolder = new Reference(); 2211 return this.policyHolder; 2212 } 2213 else if (name.equals("subscriber")) { 2214 this.subscriber = new Reference(); 2215 return this.subscriber; 2216 } 2217 else if (name.equals("subscriberId")) { 2218 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subscriberId"); 2219 } 2220 else if (name.equals("beneficiary")) { 2221 this.beneficiary = new Reference(); 2222 return this.beneficiary; 2223 } 2224 else if (name.equals("relationship")) { 2225 this.relationship = new CodeableConcept(); 2226 return this.relationship; 2227 } 2228 else if (name.equals("period")) { 2229 this.period = new Period(); 2230 return this.period; 2231 } 2232 else if (name.equals("payor")) { 2233 return addPayor(); 2234 } 2235 else if (name.equals("grouping")) { 2236 this.grouping = new GroupComponent(); 2237 return this.grouping; 2238 } 2239 else if (name.equals("dependent")) { 2240 throw new FHIRException("Cannot call addChild on a singleton property Coverage.dependent"); 2241 } 2242 else if (name.equals("sequence")) { 2243 throw new FHIRException("Cannot call addChild on a singleton property Coverage.sequence"); 2244 } 2245 else if (name.equals("order")) { 2246 throw new FHIRException("Cannot call addChild on a singleton property Coverage.order"); 2247 } 2248 else if (name.equals("network")) { 2249 throw new FHIRException("Cannot call addChild on a singleton property Coverage.network"); 2250 } 2251 else if (name.equals("contract")) { 2252 return addContract(); 2253 } 2254 else 2255 return super.addChild(name); 2256 } 2257 2258 public String fhirType() { 2259 return "Coverage"; 2260 2261 } 2262 2263 public Coverage copy() { 2264 Coverage dst = new Coverage(); 2265 copyValues(dst); 2266 if (identifier != null) { 2267 dst.identifier = new ArrayList<Identifier>(); 2268 for (Identifier i : identifier) 2269 dst.identifier.add(i.copy()); 2270 }; 2271 dst.status = status == null ? null : status.copy(); 2272 dst.type = type == null ? null : type.copy(); 2273 dst.policyHolder = policyHolder == null ? null : policyHolder.copy(); 2274 dst.subscriber = subscriber == null ? null : subscriber.copy(); 2275 dst.subscriberId = subscriberId == null ? null : subscriberId.copy(); 2276 dst.beneficiary = beneficiary == null ? null : beneficiary.copy(); 2277 dst.relationship = relationship == null ? null : relationship.copy(); 2278 dst.period = period == null ? null : period.copy(); 2279 if (payor != null) { 2280 dst.payor = new ArrayList<Reference>(); 2281 for (Reference i : payor) 2282 dst.payor.add(i.copy()); 2283 }; 2284 dst.grouping = grouping == null ? null : grouping.copy(); 2285 dst.dependent = dependent == null ? null : dependent.copy(); 2286 dst.sequence = sequence == null ? null : sequence.copy(); 2287 dst.order = order == null ? null : order.copy(); 2288 dst.network = network == null ? null : network.copy(); 2289 if (contract != null) { 2290 dst.contract = new ArrayList<Reference>(); 2291 for (Reference i : contract) 2292 dst.contract.add(i.copy()); 2293 }; 2294 return dst; 2295 } 2296 2297 protected Coverage typedCopy() { 2298 return copy(); 2299 } 2300 2301 @Override 2302 public boolean equalsDeep(Base other_) { 2303 if (!super.equalsDeep(other_)) 2304 return false; 2305 if (!(other_ instanceof Coverage)) 2306 return false; 2307 Coverage o = (Coverage) other_; 2308 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 2309 && compareDeep(policyHolder, o.policyHolder, true) && compareDeep(subscriber, o.subscriber, true) 2310 && compareDeep(subscriberId, o.subscriberId, true) && compareDeep(beneficiary, o.beneficiary, true) 2311 && compareDeep(relationship, o.relationship, true) && compareDeep(period, o.period, true) && compareDeep(payor, o.payor, true) 2312 && compareDeep(grouping, o.grouping, true) && compareDeep(dependent, o.dependent, true) && compareDeep(sequence, o.sequence, true) 2313 && compareDeep(order, o.order, true) && compareDeep(network, o.network, true) && compareDeep(contract, o.contract, true) 2314 ; 2315 } 2316 2317 @Override 2318 public boolean equalsShallow(Base other_) { 2319 if (!super.equalsShallow(other_)) 2320 return false; 2321 if (!(other_ instanceof Coverage)) 2322 return false; 2323 Coverage o = (Coverage) other_; 2324 return compareValues(status, o.status, true) && compareValues(subscriberId, o.subscriberId, true) && compareValues(dependent, o.dependent, true) 2325 && compareValues(sequence, o.sequence, true) && compareValues(order, o.order, true) && compareValues(network, o.network, true) 2326 ; 2327 } 2328 2329 public boolean isEmpty() { 2330 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 2331 , policyHolder, subscriber, subscriberId, beneficiary, relationship, period, payor 2332 , grouping, dependent, sequence, order, network, contract); 2333 } 2334 2335 @Override 2336 public ResourceType getResourceType() { 2337 return ResourceType.Coverage; 2338 } 2339 2340 /** 2341 * Search parameter: <b>identifier</b> 2342 * <p> 2343 * Description: <b>The primary identifier of the insured and the coverage</b><br> 2344 * Type: <b>token</b><br> 2345 * Path: <b>Coverage.identifier</b><br> 2346 * </p> 2347 */ 2348 @SearchParamDefinition(name="identifier", path="Coverage.identifier", description="The primary identifier of the insured and the coverage", type="token" ) 2349 public static final String SP_IDENTIFIER = "identifier"; 2350 /** 2351 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2352 * <p> 2353 * Description: <b>The primary identifier of the insured and the coverage</b><br> 2354 * Type: <b>token</b><br> 2355 * Path: <b>Coverage.identifier</b><br> 2356 * </p> 2357 */ 2358 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2359 2360 /** 2361 * Search parameter: <b>subgroup</b> 2362 * <p> 2363 * Description: <b>Sub-group identifier</b><br> 2364 * Type: <b>string</b><br> 2365 * Path: <b>Coverage.grouping.subGroup</b><br> 2366 * </p> 2367 */ 2368 @SearchParamDefinition(name="subgroup", path="Coverage.grouping.subGroup", description="Sub-group identifier", type="string" ) 2369 public static final String SP_SUBGROUP = "subgroup"; 2370 /** 2371 * <b>Fluent Client</b> search parameter constant for <b>subgroup</b> 2372 * <p> 2373 * Description: <b>Sub-group identifier</b><br> 2374 * Type: <b>string</b><br> 2375 * Path: <b>Coverage.grouping.subGroup</b><br> 2376 * </p> 2377 */ 2378 public static final ca.uhn.fhir.rest.gclient.StringClientParam SUBGROUP = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SUBGROUP); 2379 2380 /** 2381 * Search parameter: <b>subscriber</b> 2382 * <p> 2383 * Description: <b>Reference to the subscriber</b><br> 2384 * Type: <b>reference</b><br> 2385 * Path: <b>Coverage.subscriber</b><br> 2386 * </p> 2387 */ 2388 @SearchParamDefinition(name="subscriber", path="Coverage.subscriber", description="Reference to the subscriber", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, RelatedPerson.class } ) 2389 public static final String SP_SUBSCRIBER = "subscriber"; 2390 /** 2391 * <b>Fluent Client</b> search parameter constant for <b>subscriber</b> 2392 * <p> 2393 * Description: <b>Reference to the subscriber</b><br> 2394 * Type: <b>reference</b><br> 2395 * Path: <b>Coverage.subscriber</b><br> 2396 * </p> 2397 */ 2398 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSCRIBER); 2399 2400/** 2401 * Constant for fluent queries to be used to add include statements. Specifies 2402 * the path value of "<b>Coverage:subscriber</b>". 2403 */ 2404 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSCRIBER = new ca.uhn.fhir.model.api.Include("Coverage:subscriber").toLocked(); 2405 2406 /** 2407 * Search parameter: <b>subplan</b> 2408 * <p> 2409 * Description: <b>Sub-plan identifier</b><br> 2410 * Type: <b>string</b><br> 2411 * Path: <b>Coverage.grouping.subPlan</b><br> 2412 * </p> 2413 */ 2414 @SearchParamDefinition(name="subplan", path="Coverage.grouping.subPlan", description="Sub-plan identifier", type="string" ) 2415 public static final String SP_SUBPLAN = "subplan"; 2416 /** 2417 * <b>Fluent Client</b> search parameter constant for <b>subplan</b> 2418 * <p> 2419 * Description: <b>Sub-plan identifier</b><br> 2420 * Type: <b>string</b><br> 2421 * Path: <b>Coverage.grouping.subPlan</b><br> 2422 * </p> 2423 */ 2424 public static final ca.uhn.fhir.rest.gclient.StringClientParam SUBPLAN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SUBPLAN); 2425 2426 /** 2427 * Search parameter: <b>type</b> 2428 * <p> 2429 * Description: <b>The kind of coverage (health plan, auto, Workers Compensation)</b><br> 2430 * Type: <b>token</b><br> 2431 * Path: <b>Coverage.type</b><br> 2432 * </p> 2433 */ 2434 @SearchParamDefinition(name="type", path="Coverage.type", description="The kind of coverage (health plan, auto, Workers Compensation)", type="token" ) 2435 public static final String SP_TYPE = "type"; 2436 /** 2437 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2438 * <p> 2439 * Description: <b>The kind of coverage (health plan, auto, Workers Compensation)</b><br> 2440 * Type: <b>token</b><br> 2441 * Path: <b>Coverage.type</b><br> 2442 * </p> 2443 */ 2444 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2445 2446 /** 2447 * Search parameter: <b>sequence</b> 2448 * <p> 2449 * Description: <b>Sequence number</b><br> 2450 * Type: <b>string</b><br> 2451 * Path: <b>Coverage.sequence</b><br> 2452 * </p> 2453 */ 2454 @SearchParamDefinition(name="sequence", path="Coverage.sequence", description="Sequence number", type="string" ) 2455 public static final String SP_SEQUENCE = "sequence"; 2456 /** 2457 * <b>Fluent Client</b> search parameter constant for <b>sequence</b> 2458 * <p> 2459 * Description: <b>Sequence number</b><br> 2460 * Type: <b>string</b><br> 2461 * Path: <b>Coverage.sequence</b><br> 2462 * </p> 2463 */ 2464 public static final ca.uhn.fhir.rest.gclient.StringClientParam SEQUENCE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SEQUENCE); 2465 2466 /** 2467 * Search parameter: <b>payor</b> 2468 * <p> 2469 * Description: <b>The identity of the insurer or party paying for services</b><br> 2470 * Type: <b>reference</b><br> 2471 * Path: <b>Coverage.payor</b><br> 2472 * </p> 2473 */ 2474 @SearchParamDefinition(name="payor", path="Coverage.payor", description="The identity of the insurer or party paying for services", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 2475 public static final String SP_PAYOR = "payor"; 2476 /** 2477 * <b>Fluent Client</b> search parameter constant for <b>payor</b> 2478 * <p> 2479 * Description: <b>The identity of the insurer or party paying for services</b><br> 2480 * Type: <b>reference</b><br> 2481 * Path: <b>Coverage.payor</b><br> 2482 * </p> 2483 */ 2484 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYOR); 2485 2486/** 2487 * Constant for fluent queries to be used to add include statements. Specifies 2488 * the path value of "<b>Coverage:payor</b>". 2489 */ 2490 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYOR = new ca.uhn.fhir.model.api.Include("Coverage:payor").toLocked(); 2491 2492 /** 2493 * Search parameter: <b>beneficiary</b> 2494 * <p> 2495 * Description: <b>Covered party</b><br> 2496 * Type: <b>reference</b><br> 2497 * Path: <b>Coverage.beneficiary</b><br> 2498 * </p> 2499 */ 2500 @SearchParamDefinition(name="beneficiary", path="Coverage.beneficiary", description="Covered party", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2501 public static final String SP_BENEFICIARY = "beneficiary"; 2502 /** 2503 * <b>Fluent Client</b> search parameter constant for <b>beneficiary</b> 2504 * <p> 2505 * Description: <b>Covered party</b><br> 2506 * Type: <b>reference</b><br> 2507 * Path: <b>Coverage.beneficiary</b><br> 2508 * </p> 2509 */ 2510 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BENEFICIARY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BENEFICIARY); 2511 2512/** 2513 * Constant for fluent queries to be used to add include statements. Specifies 2514 * the path value of "<b>Coverage:beneficiary</b>". 2515 */ 2516 public static final ca.uhn.fhir.model.api.Include INCLUDE_BENEFICIARY = new ca.uhn.fhir.model.api.Include("Coverage:beneficiary").toLocked(); 2517 2518 /** 2519 * Search parameter: <b>subclass</b> 2520 * <p> 2521 * Description: <b>Sub-class identifier</b><br> 2522 * Type: <b>string</b><br> 2523 * Path: <b>Coverage.grouping.subClass</b><br> 2524 * </p> 2525 */ 2526 @SearchParamDefinition(name="subclass", path="Coverage.grouping.subClass", description="Sub-class identifier", type="string" ) 2527 public static final String SP_SUBCLASS = "subclass"; 2528 /** 2529 * <b>Fluent Client</b> search parameter constant for <b>subclass</b> 2530 * <p> 2531 * Description: <b>Sub-class identifier</b><br> 2532 * Type: <b>string</b><br> 2533 * Path: <b>Coverage.grouping.subClass</b><br> 2534 * </p> 2535 */ 2536 public static final ca.uhn.fhir.rest.gclient.StringClientParam SUBCLASS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SUBCLASS); 2537 2538 /** 2539 * Search parameter: <b>plan</b> 2540 * <p> 2541 * Description: <b>A plan or policy identifier</b><br> 2542 * Type: <b>string</b><br> 2543 * Path: <b>Coverage.grouping.plan</b><br> 2544 * </p> 2545 */ 2546 @SearchParamDefinition(name="plan", path="Coverage.grouping.plan", description="A plan or policy identifier", type="string" ) 2547 public static final String SP_PLAN = "plan"; 2548 /** 2549 * <b>Fluent Client</b> search parameter constant for <b>plan</b> 2550 * <p> 2551 * Description: <b>A plan or policy identifier</b><br> 2552 * Type: <b>string</b><br> 2553 * Path: <b>Coverage.grouping.plan</b><br> 2554 * </p> 2555 */ 2556 public static final ca.uhn.fhir.rest.gclient.StringClientParam PLAN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PLAN); 2557 2558 /** 2559 * Search parameter: <b>class</b> 2560 * <p> 2561 * Description: <b>Class identifier</b><br> 2562 * Type: <b>string</b><br> 2563 * Path: <b>Coverage.grouping.class</b><br> 2564 * </p> 2565 */ 2566 @SearchParamDefinition(name="class", path="Coverage.grouping.class", description="Class identifier", type="string" ) 2567 public static final String SP_CLASS = "class"; 2568 /** 2569 * <b>Fluent Client</b> search parameter constant for <b>class</b> 2570 * <p> 2571 * Description: <b>Class identifier</b><br> 2572 * Type: <b>string</b><br> 2573 * Path: <b>Coverage.grouping.class</b><br> 2574 * </p> 2575 */ 2576 public static final ca.uhn.fhir.rest.gclient.StringClientParam CLASS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_CLASS); 2577 2578 /** 2579 * Search parameter: <b>dependent</b> 2580 * <p> 2581 * Description: <b>Dependent number</b><br> 2582 * Type: <b>string</b><br> 2583 * Path: <b>Coverage.dependent</b><br> 2584 * </p> 2585 */ 2586 @SearchParamDefinition(name="dependent", path="Coverage.dependent", description="Dependent number", type="string" ) 2587 public static final String SP_DEPENDENT = "dependent"; 2588 /** 2589 * <b>Fluent Client</b> search parameter constant for <b>dependent</b> 2590 * <p> 2591 * Description: <b>Dependent number</b><br> 2592 * Type: <b>string</b><br> 2593 * Path: <b>Coverage.dependent</b><br> 2594 * </p> 2595 */ 2596 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEPENDENT = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DEPENDENT); 2597 2598 /** 2599 * Search parameter: <b>group</b> 2600 * <p> 2601 * Description: <b>Group identifier</b><br> 2602 * Type: <b>string</b><br> 2603 * Path: <b>Coverage.grouping.group</b><br> 2604 * </p> 2605 */ 2606 @SearchParamDefinition(name="group", path="Coverage.grouping.group", description="Group identifier", type="string" ) 2607 public static final String SP_GROUP = "group"; 2608 /** 2609 * <b>Fluent Client</b> search parameter constant for <b>group</b> 2610 * <p> 2611 * Description: <b>Group identifier</b><br> 2612 * Type: <b>string</b><br> 2613 * Path: <b>Coverage.grouping.group</b><br> 2614 * </p> 2615 */ 2616 public static final ca.uhn.fhir.rest.gclient.StringClientParam GROUP = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GROUP); 2617 2618 /** 2619 * Search parameter: <b>policy-holder</b> 2620 * <p> 2621 * Description: <b>Reference to the policyholder</b><br> 2622 * Type: <b>reference</b><br> 2623 * Path: <b>Coverage.policyHolder</b><br> 2624 * </p> 2625 */ 2626 @SearchParamDefinition(name="policy-holder", path="Coverage.policyHolder", description="Reference to the policyholder", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 2627 public static final String SP_POLICY_HOLDER = "policy-holder"; 2628 /** 2629 * <b>Fluent Client</b> search parameter constant for <b>policy-holder</b> 2630 * <p> 2631 * Description: <b>Reference to the policyholder</b><br> 2632 * Type: <b>reference</b><br> 2633 * Path: <b>Coverage.policyHolder</b><br> 2634 * </p> 2635 */ 2636 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam POLICY_HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_POLICY_HOLDER); 2637 2638/** 2639 * Constant for fluent queries to be used to add include statements. Specifies 2640 * the path value of "<b>Coverage:policy-holder</b>". 2641 */ 2642 public static final ca.uhn.fhir.model.api.Include INCLUDE_POLICY_HOLDER = new ca.uhn.fhir.model.api.Include("Coverage:policy-holder").toLocked(); 2643 2644 2645}