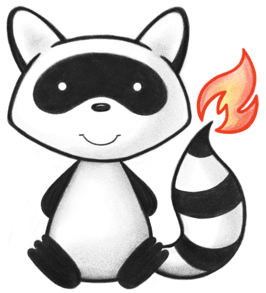
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Financial instrument which may be used to reimburse or pay for health care products and services. 049 */ 050@ResourceDef(name="Coverage", profile="http://hl7.org/fhir/Profile/Coverage") 051public class Coverage extends DomainResource { 052 053 public enum CoverageStatus { 054 /** 055 * The instance is currently in-force. 056 */ 057 ACTIVE, 058 /** 059 * The instance is withdrawn, rescinded or reversed. 060 */ 061 CANCELLED, 062 /** 063 * A new instance the contents of which is not complete. 064 */ 065 DRAFT, 066 /** 067 * The instance was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 public static CoverageStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("active".equals(codeString)) 078 return ACTIVE; 079 if ("cancelled".equals(codeString)) 080 return CANCELLED; 081 if ("draft".equals(codeString)) 082 return DRAFT; 083 if ("entered-in-error".equals(codeString)) 084 return ENTEREDINERROR; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown CoverageStatus code '"+codeString+"'"); 089 } 090 public String toCode() { 091 switch (this) { 092 case ACTIVE: return "active"; 093 case CANCELLED: return "cancelled"; 094 case DRAFT: return "draft"; 095 case ENTEREDINERROR: return "entered-in-error"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getSystem() { 101 switch (this) { 102 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 103 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 104 case DRAFT: return "http://hl7.org/fhir/fm-status"; 105 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getDefinition() { 111 switch (this) { 112 case ACTIVE: return "The instance is currently in-force."; 113 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 114 case DRAFT: return "A new instance the contents of which is not complete."; 115 case ENTEREDINERROR: return "The instance was entered in error."; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDisplay() { 121 switch (this) { 122 case ACTIVE: return "Active"; 123 case CANCELLED: return "Cancelled"; 124 case DRAFT: return "Draft"; 125 case ENTEREDINERROR: return "Entered in Error"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 } 131 132 public static class CoverageStatusEnumFactory implements EnumFactory<CoverageStatus> { 133 public CoverageStatus fromCode(String codeString) throws IllegalArgumentException { 134 if (codeString == null || "".equals(codeString)) 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("active".equals(codeString)) 138 return CoverageStatus.ACTIVE; 139 if ("cancelled".equals(codeString)) 140 return CoverageStatus.CANCELLED; 141 if ("draft".equals(codeString)) 142 return CoverageStatus.DRAFT; 143 if ("entered-in-error".equals(codeString)) 144 return CoverageStatus.ENTEREDINERROR; 145 throw new IllegalArgumentException("Unknown CoverageStatus code '"+codeString+"'"); 146 } 147 public Enumeration<CoverageStatus> fromType(PrimitiveType<?> code) throws FHIRException { 148 if (code == null) 149 return null; 150 if (code.isEmpty()) 151 return new Enumeration<CoverageStatus>(this); 152 String codeString = code.asStringValue(); 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("active".equals(codeString)) 156 return new Enumeration<CoverageStatus>(this, CoverageStatus.ACTIVE); 157 if ("cancelled".equals(codeString)) 158 return new Enumeration<CoverageStatus>(this, CoverageStatus.CANCELLED); 159 if ("draft".equals(codeString)) 160 return new Enumeration<CoverageStatus>(this, CoverageStatus.DRAFT); 161 if ("entered-in-error".equals(codeString)) 162 return new Enumeration<CoverageStatus>(this, CoverageStatus.ENTEREDINERROR); 163 throw new FHIRException("Unknown CoverageStatus code '"+codeString+"'"); 164 } 165 public String toCode(CoverageStatus code) { 166 if (code == CoverageStatus.NULL) 167 return null; 168 if (code == CoverageStatus.ACTIVE) 169 return "active"; 170 if (code == CoverageStatus.CANCELLED) 171 return "cancelled"; 172 if (code == CoverageStatus.DRAFT) 173 return "draft"; 174 if (code == CoverageStatus.ENTEREDINERROR) 175 return "entered-in-error"; 176 return "?"; 177 } 178 public String toSystem(CoverageStatus code) { 179 return code.getSystem(); 180 } 181 } 182 183 @Block() 184 public static class GroupComponent extends BackboneElement implements IBaseBackboneElement { 185 /** 186 * Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID. 187 */ 188 @Child(name = "group", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 189 @Description(shortDefinition="An identifier for the group", formalDefinition="Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID." ) 190 protected StringType group; 191 192 /** 193 * A short description for the group. 194 */ 195 @Child(name = "groupDisplay", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 196 @Description(shortDefinition="Display text for an identifier for the group", formalDefinition="A short description for the group." ) 197 protected StringType groupDisplay; 198 199 /** 200 * Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group. 201 */ 202 @Child(name = "subGroup", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 203 @Description(shortDefinition="An identifier for the subsection of the group", formalDefinition="Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group." ) 204 protected StringType subGroup; 205 206 /** 207 * A short description for the subgroup. 208 */ 209 @Child(name = "subGroupDisplay", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 210 @Description(shortDefinition="Display text for the subsection of the group", formalDefinition="A short description for the subgroup." ) 211 protected StringType subGroupDisplay; 212 213 /** 214 * Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID. 215 */ 216 @Child(name = "plan", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 217 @Description(shortDefinition="An identifier for the plan", formalDefinition="Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID." ) 218 protected StringType plan; 219 220 /** 221 * A short description for the plan. 222 */ 223 @Child(name = "planDisplay", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 224 @Description(shortDefinition="Display text for the plan", formalDefinition="A short description for the plan." ) 225 protected StringType planDisplay; 226 227 /** 228 * Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees. 229 */ 230 @Child(name = "subPlan", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 231 @Description(shortDefinition="An identifier for the subsection of the plan", formalDefinition="Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees." ) 232 protected StringType subPlan; 233 234 /** 235 * A short description for the subplan. 236 */ 237 @Child(name = "subPlanDisplay", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 238 @Description(shortDefinition="Display text for the subsection of the plan", formalDefinition="A short description for the subplan." ) 239 protected StringType subPlanDisplay; 240 241 /** 242 * Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment. 243 */ 244 @Child(name = "class", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 245 @Description(shortDefinition="An identifier for the class", formalDefinition="Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment." ) 246 protected StringType class_; 247 248 /** 249 * A short description for the class. 250 */ 251 @Child(name = "classDisplay", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 252 @Description(shortDefinition="Display text for the class", formalDefinition="A short description for the class." ) 253 protected StringType classDisplay; 254 255 /** 256 * Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment. 257 */ 258 @Child(name = "subClass", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 259 @Description(shortDefinition="An identifier for the subsection of the class", formalDefinition="Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment." ) 260 protected StringType subClass; 261 262 /** 263 * A short description for the subclass. 264 */ 265 @Child(name = "subClassDisplay", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 266 @Description(shortDefinition="Display text for the subsection of the subclass", formalDefinition="A short description for the subclass." ) 267 protected StringType subClassDisplay; 268 269 private static final long serialVersionUID = -13147121L; 270 271 /** 272 * Constructor 273 */ 274 public GroupComponent() { 275 super(); 276 } 277 278 /** 279 * @return {@link #group} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.). This is the underlying object with id, value and extensions. The accessor "getGroup" gives direct access to the value 280 */ 281 public StringType getGroupElement() { 282 if (this.group == null) 283 if (Configuration.errorOnAutoCreate()) 284 throw new Error("Attempt to auto-create GroupComponent.group"); 285 else if (Configuration.doAutoCreate()) 286 this.group = new StringType(); // bb 287 return this.group; 288 } 289 290 public boolean hasGroupElement() { 291 return this.group != null && !this.group.isEmpty(); 292 } 293 294 public boolean hasGroup() { 295 return this.group != null && !this.group.isEmpty(); 296 } 297 298 /** 299 * @param value {@link #group} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.). This is the underlying object with id, value and extensions. The accessor "getGroup" gives direct access to the value 300 */ 301 public GroupComponent setGroupElement(StringType value) { 302 this.group = value; 303 return this; 304 } 305 306 /** 307 * @return Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID. 308 */ 309 public String getGroup() { 310 return this.group == null ? null : this.group.getValue(); 311 } 312 313 /** 314 * @param value Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID. 315 */ 316 public GroupComponent setGroup(String value) { 317 if (Utilities.noString(value)) 318 this.group = null; 319 else { 320 if (this.group == null) 321 this.group = new StringType(); 322 this.group.setValue(value); 323 } 324 return this; 325 } 326 327 /** 328 * @return {@link #groupDisplay} (A short description for the group.). This is the underlying object with id, value and extensions. The accessor "getGroupDisplay" gives direct access to the value 329 */ 330 public StringType getGroupDisplayElement() { 331 if (this.groupDisplay == null) 332 if (Configuration.errorOnAutoCreate()) 333 throw new Error("Attempt to auto-create GroupComponent.groupDisplay"); 334 else if (Configuration.doAutoCreate()) 335 this.groupDisplay = new StringType(); // bb 336 return this.groupDisplay; 337 } 338 339 public boolean hasGroupDisplayElement() { 340 return this.groupDisplay != null && !this.groupDisplay.isEmpty(); 341 } 342 343 public boolean hasGroupDisplay() { 344 return this.groupDisplay != null && !this.groupDisplay.isEmpty(); 345 } 346 347 /** 348 * @param value {@link #groupDisplay} (A short description for the group.). This is the underlying object with id, value and extensions. The accessor "getGroupDisplay" gives direct access to the value 349 */ 350 public GroupComponent setGroupDisplayElement(StringType value) { 351 this.groupDisplay = value; 352 return this; 353 } 354 355 /** 356 * @return A short description for the group. 357 */ 358 public String getGroupDisplay() { 359 return this.groupDisplay == null ? null : this.groupDisplay.getValue(); 360 } 361 362 /** 363 * @param value A short description for the group. 364 */ 365 public GroupComponent setGroupDisplay(String value) { 366 if (Utilities.noString(value)) 367 this.groupDisplay = null; 368 else { 369 if (this.groupDisplay == null) 370 this.groupDisplay = new StringType(); 371 this.groupDisplay.setValue(value); 372 } 373 return this; 374 } 375 376 /** 377 * @return {@link #subGroup} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.). This is the underlying object with id, value and extensions. The accessor "getSubGroup" gives direct access to the value 378 */ 379 public StringType getSubGroupElement() { 380 if (this.subGroup == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create GroupComponent.subGroup"); 383 else if (Configuration.doAutoCreate()) 384 this.subGroup = new StringType(); // bb 385 return this.subGroup; 386 } 387 388 public boolean hasSubGroupElement() { 389 return this.subGroup != null && !this.subGroup.isEmpty(); 390 } 391 392 public boolean hasSubGroup() { 393 return this.subGroup != null && !this.subGroup.isEmpty(); 394 } 395 396 /** 397 * @param value {@link #subGroup} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.). This is the underlying object with id, value and extensions. The accessor "getSubGroup" gives direct access to the value 398 */ 399 public GroupComponent setSubGroupElement(StringType value) { 400 this.subGroup = value; 401 return this; 402 } 403 404 /** 405 * @return Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group. 406 */ 407 public String getSubGroup() { 408 return this.subGroup == null ? null : this.subGroup.getValue(); 409 } 410 411 /** 412 * @param value Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group. 413 */ 414 public GroupComponent setSubGroup(String value) { 415 if (Utilities.noString(value)) 416 this.subGroup = null; 417 else { 418 if (this.subGroup == null) 419 this.subGroup = new StringType(); 420 this.subGroup.setValue(value); 421 } 422 return this; 423 } 424 425 /** 426 * @return {@link #subGroupDisplay} (A short description for the subgroup.). This is the underlying object with id, value and extensions. The accessor "getSubGroupDisplay" gives direct access to the value 427 */ 428 public StringType getSubGroupDisplayElement() { 429 if (this.subGroupDisplay == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create GroupComponent.subGroupDisplay"); 432 else if (Configuration.doAutoCreate()) 433 this.subGroupDisplay = new StringType(); // bb 434 return this.subGroupDisplay; 435 } 436 437 public boolean hasSubGroupDisplayElement() { 438 return this.subGroupDisplay != null && !this.subGroupDisplay.isEmpty(); 439 } 440 441 public boolean hasSubGroupDisplay() { 442 return this.subGroupDisplay != null && !this.subGroupDisplay.isEmpty(); 443 } 444 445 /** 446 * @param value {@link #subGroupDisplay} (A short description for the subgroup.). This is the underlying object with id, value and extensions. The accessor "getSubGroupDisplay" gives direct access to the value 447 */ 448 public GroupComponent setSubGroupDisplayElement(StringType value) { 449 this.subGroupDisplay = value; 450 return this; 451 } 452 453 /** 454 * @return A short description for the subgroup. 455 */ 456 public String getSubGroupDisplay() { 457 return this.subGroupDisplay == null ? null : this.subGroupDisplay.getValue(); 458 } 459 460 /** 461 * @param value A short description for the subgroup. 462 */ 463 public GroupComponent setSubGroupDisplay(String value) { 464 if (Utilities.noString(value)) 465 this.subGroupDisplay = null; 466 else { 467 if (this.subGroupDisplay == null) 468 this.subGroupDisplay = new StringType(); 469 this.subGroupDisplay.setValue(value); 470 } 471 return this; 472 } 473 474 /** 475 * @return {@link #plan} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.). This is the underlying object with id, value and extensions. The accessor "getPlan" gives direct access to the value 476 */ 477 public StringType getPlanElement() { 478 if (this.plan == null) 479 if (Configuration.errorOnAutoCreate()) 480 throw new Error("Attempt to auto-create GroupComponent.plan"); 481 else if (Configuration.doAutoCreate()) 482 this.plan = new StringType(); // bb 483 return this.plan; 484 } 485 486 public boolean hasPlanElement() { 487 return this.plan != null && !this.plan.isEmpty(); 488 } 489 490 public boolean hasPlan() { 491 return this.plan != null && !this.plan.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #plan} (Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.). This is the underlying object with id, value and extensions. The accessor "getPlan" gives direct access to the value 496 */ 497 public GroupComponent setPlanElement(StringType value) { 498 this.plan = value; 499 return this; 500 } 501 502 /** 503 * @return Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID. 504 */ 505 public String getPlan() { 506 return this.plan == null ? null : this.plan.getValue(); 507 } 508 509 /** 510 * @param value Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID. 511 */ 512 public GroupComponent setPlan(String value) { 513 if (Utilities.noString(value)) 514 this.plan = null; 515 else { 516 if (this.plan == null) 517 this.plan = new StringType(); 518 this.plan.setValue(value); 519 } 520 return this; 521 } 522 523 /** 524 * @return {@link #planDisplay} (A short description for the plan.). This is the underlying object with id, value and extensions. The accessor "getPlanDisplay" gives direct access to the value 525 */ 526 public StringType getPlanDisplayElement() { 527 if (this.planDisplay == null) 528 if (Configuration.errorOnAutoCreate()) 529 throw new Error("Attempt to auto-create GroupComponent.planDisplay"); 530 else if (Configuration.doAutoCreate()) 531 this.planDisplay = new StringType(); // bb 532 return this.planDisplay; 533 } 534 535 public boolean hasPlanDisplayElement() { 536 return this.planDisplay != null && !this.planDisplay.isEmpty(); 537 } 538 539 public boolean hasPlanDisplay() { 540 return this.planDisplay != null && !this.planDisplay.isEmpty(); 541 } 542 543 /** 544 * @param value {@link #planDisplay} (A short description for the plan.). This is the underlying object with id, value and extensions. The accessor "getPlanDisplay" gives direct access to the value 545 */ 546 public GroupComponent setPlanDisplayElement(StringType value) { 547 this.planDisplay = value; 548 return this; 549 } 550 551 /** 552 * @return A short description for the plan. 553 */ 554 public String getPlanDisplay() { 555 return this.planDisplay == null ? null : this.planDisplay.getValue(); 556 } 557 558 /** 559 * @param value A short description for the plan. 560 */ 561 public GroupComponent setPlanDisplay(String value) { 562 if (Utilities.noString(value)) 563 this.planDisplay = null; 564 else { 565 if (this.planDisplay == null) 566 this.planDisplay = new StringType(); 567 this.planDisplay.setValue(value); 568 } 569 return this; 570 } 571 572 /** 573 * @return {@link #subPlan} (Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.). This is the underlying object with id, value and extensions. The accessor "getSubPlan" gives direct access to the value 574 */ 575 public StringType getSubPlanElement() { 576 if (this.subPlan == null) 577 if (Configuration.errorOnAutoCreate()) 578 throw new Error("Attempt to auto-create GroupComponent.subPlan"); 579 else if (Configuration.doAutoCreate()) 580 this.subPlan = new StringType(); // bb 581 return this.subPlan; 582 } 583 584 public boolean hasSubPlanElement() { 585 return this.subPlan != null && !this.subPlan.isEmpty(); 586 } 587 588 public boolean hasSubPlan() { 589 return this.subPlan != null && !this.subPlan.isEmpty(); 590 } 591 592 /** 593 * @param value {@link #subPlan} (Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.). This is the underlying object with id, value and extensions. The accessor "getSubPlan" gives direct access to the value 594 */ 595 public GroupComponent setSubPlanElement(StringType value) { 596 this.subPlan = value; 597 return this; 598 } 599 600 /** 601 * @return Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees. 602 */ 603 public String getSubPlan() { 604 return this.subPlan == null ? null : this.subPlan.getValue(); 605 } 606 607 /** 608 * @param value Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees. 609 */ 610 public GroupComponent setSubPlan(String value) { 611 if (Utilities.noString(value)) 612 this.subPlan = null; 613 else { 614 if (this.subPlan == null) 615 this.subPlan = new StringType(); 616 this.subPlan.setValue(value); 617 } 618 return this; 619 } 620 621 /** 622 * @return {@link #subPlanDisplay} (A short description for the subplan.). This is the underlying object with id, value and extensions. The accessor "getSubPlanDisplay" gives direct access to the value 623 */ 624 public StringType getSubPlanDisplayElement() { 625 if (this.subPlanDisplay == null) 626 if (Configuration.errorOnAutoCreate()) 627 throw new Error("Attempt to auto-create GroupComponent.subPlanDisplay"); 628 else if (Configuration.doAutoCreate()) 629 this.subPlanDisplay = new StringType(); // bb 630 return this.subPlanDisplay; 631 } 632 633 public boolean hasSubPlanDisplayElement() { 634 return this.subPlanDisplay != null && !this.subPlanDisplay.isEmpty(); 635 } 636 637 public boolean hasSubPlanDisplay() { 638 return this.subPlanDisplay != null && !this.subPlanDisplay.isEmpty(); 639 } 640 641 /** 642 * @param value {@link #subPlanDisplay} (A short description for the subplan.). This is the underlying object with id, value and extensions. The accessor "getSubPlanDisplay" gives direct access to the value 643 */ 644 public GroupComponent setSubPlanDisplayElement(StringType value) { 645 this.subPlanDisplay = value; 646 return this; 647 } 648 649 /** 650 * @return A short description for the subplan. 651 */ 652 public String getSubPlanDisplay() { 653 return this.subPlanDisplay == null ? null : this.subPlanDisplay.getValue(); 654 } 655 656 /** 657 * @param value A short description for the subplan. 658 */ 659 public GroupComponent setSubPlanDisplay(String value) { 660 if (Utilities.noString(value)) 661 this.subPlanDisplay = null; 662 else { 663 if (this.subPlanDisplay == null) 664 this.subPlanDisplay = new StringType(); 665 this.subPlanDisplay.setValue(value); 666 } 667 return this; 668 } 669 670 /** 671 * @return {@link #class_} (Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getClass_" gives direct access to the value 672 */ 673 public StringType getClass_Element() { 674 if (this.class_ == null) 675 if (Configuration.errorOnAutoCreate()) 676 throw new Error("Attempt to auto-create GroupComponent.class_"); 677 else if (Configuration.doAutoCreate()) 678 this.class_ = new StringType(); // bb 679 return this.class_; 680 } 681 682 public boolean hasClass_Element() { 683 return this.class_ != null && !this.class_.isEmpty(); 684 } 685 686 public boolean hasClass_() { 687 return this.class_ != null && !this.class_.isEmpty(); 688 } 689 690 /** 691 * @param value {@link #class_} (Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getClass_" gives direct access to the value 692 */ 693 public GroupComponent setClass_Element(StringType value) { 694 this.class_ = value; 695 return this; 696 } 697 698 /** 699 * @return Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment. 700 */ 701 public String getClass_() { 702 return this.class_ == null ? null : this.class_.getValue(); 703 } 704 705 /** 706 * @param value Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment. 707 */ 708 public GroupComponent setClass_(String value) { 709 if (Utilities.noString(value)) 710 this.class_ = null; 711 else { 712 if (this.class_ == null) 713 this.class_ = new StringType(); 714 this.class_.setValue(value); 715 } 716 return this; 717 } 718 719 /** 720 * @return {@link #classDisplay} (A short description for the class.). This is the underlying object with id, value and extensions. The accessor "getClassDisplay" gives direct access to the value 721 */ 722 public StringType getClassDisplayElement() { 723 if (this.classDisplay == null) 724 if (Configuration.errorOnAutoCreate()) 725 throw new Error("Attempt to auto-create GroupComponent.classDisplay"); 726 else if (Configuration.doAutoCreate()) 727 this.classDisplay = new StringType(); // bb 728 return this.classDisplay; 729 } 730 731 public boolean hasClassDisplayElement() { 732 return this.classDisplay != null && !this.classDisplay.isEmpty(); 733 } 734 735 public boolean hasClassDisplay() { 736 return this.classDisplay != null && !this.classDisplay.isEmpty(); 737 } 738 739 /** 740 * @param value {@link #classDisplay} (A short description for the class.). This is the underlying object with id, value and extensions. The accessor "getClassDisplay" gives direct access to the value 741 */ 742 public GroupComponent setClassDisplayElement(StringType value) { 743 this.classDisplay = value; 744 return this; 745 } 746 747 /** 748 * @return A short description for the class. 749 */ 750 public String getClassDisplay() { 751 return this.classDisplay == null ? null : this.classDisplay.getValue(); 752 } 753 754 /** 755 * @param value A short description for the class. 756 */ 757 public GroupComponent setClassDisplay(String value) { 758 if (Utilities.noString(value)) 759 this.classDisplay = null; 760 else { 761 if (this.classDisplay == null) 762 this.classDisplay = new StringType(); 763 this.classDisplay.setValue(value); 764 } 765 return this; 766 } 767 768 /** 769 * @return {@link #subClass} (Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getSubClass" gives direct access to the value 770 */ 771 public StringType getSubClassElement() { 772 if (this.subClass == null) 773 if (Configuration.errorOnAutoCreate()) 774 throw new Error("Attempt to auto-create GroupComponent.subClass"); 775 else if (Configuration.doAutoCreate()) 776 this.subClass = new StringType(); // bb 777 return this.subClass; 778 } 779 780 public boolean hasSubClassElement() { 781 return this.subClass != null && !this.subClass.isEmpty(); 782 } 783 784 public boolean hasSubClass() { 785 return this.subClass != null && !this.subClass.isEmpty(); 786 } 787 788 /** 789 * @param value {@link #subClass} (Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.). This is the underlying object with id, value and extensions. The accessor "getSubClass" gives direct access to the value 790 */ 791 public GroupComponent setSubClassElement(StringType value) { 792 this.subClass = value; 793 return this; 794 } 795 796 /** 797 * @return Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment. 798 */ 799 public String getSubClass() { 800 return this.subClass == null ? null : this.subClass.getValue(); 801 } 802 803 /** 804 * @param value Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment. 805 */ 806 public GroupComponent setSubClass(String value) { 807 if (Utilities.noString(value)) 808 this.subClass = null; 809 else { 810 if (this.subClass == null) 811 this.subClass = new StringType(); 812 this.subClass.setValue(value); 813 } 814 return this; 815 } 816 817 /** 818 * @return {@link #subClassDisplay} (A short description for the subclass.). This is the underlying object with id, value and extensions. The accessor "getSubClassDisplay" gives direct access to the value 819 */ 820 public StringType getSubClassDisplayElement() { 821 if (this.subClassDisplay == null) 822 if (Configuration.errorOnAutoCreate()) 823 throw new Error("Attempt to auto-create GroupComponent.subClassDisplay"); 824 else if (Configuration.doAutoCreate()) 825 this.subClassDisplay = new StringType(); // bb 826 return this.subClassDisplay; 827 } 828 829 public boolean hasSubClassDisplayElement() { 830 return this.subClassDisplay != null && !this.subClassDisplay.isEmpty(); 831 } 832 833 public boolean hasSubClassDisplay() { 834 return this.subClassDisplay != null && !this.subClassDisplay.isEmpty(); 835 } 836 837 /** 838 * @param value {@link #subClassDisplay} (A short description for the subclass.). This is the underlying object with id, value and extensions. The accessor "getSubClassDisplay" gives direct access to the value 839 */ 840 public GroupComponent setSubClassDisplayElement(StringType value) { 841 this.subClassDisplay = value; 842 return this; 843 } 844 845 /** 846 * @return A short description for the subclass. 847 */ 848 public String getSubClassDisplay() { 849 return this.subClassDisplay == null ? null : this.subClassDisplay.getValue(); 850 } 851 852 /** 853 * @param value A short description for the subclass. 854 */ 855 public GroupComponent setSubClassDisplay(String value) { 856 if (Utilities.noString(value)) 857 this.subClassDisplay = null; 858 else { 859 if (this.subClassDisplay == null) 860 this.subClassDisplay = new StringType(); 861 this.subClassDisplay.setValue(value); 862 } 863 return this; 864 } 865 866 protected void listChildren(List<Property> children) { 867 super.listChildren(children); 868 children.add(new Property("group", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.", 0, 1, group)); 869 children.add(new Property("groupDisplay", "string", "A short description for the group.", 0, 1, groupDisplay)); 870 children.add(new Property("subGroup", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.", 0, 1, subGroup)); 871 children.add(new Property("subGroupDisplay", "string", "A short description for the subgroup.", 0, 1, subGroupDisplay)); 872 children.add(new Property("plan", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.", 0, 1, plan)); 873 children.add(new Property("planDisplay", "string", "A short description for the plan.", 0, 1, planDisplay)); 874 children.add(new Property("subPlan", "string", "Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.", 0, 1, subPlan)); 875 children.add(new Property("subPlanDisplay", "string", "A short description for the subplan.", 0, 1, subPlanDisplay)); 876 children.add(new Property("class", "string", "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.", 0, 1, class_)); 877 children.add(new Property("classDisplay", "string", "A short description for the class.", 0, 1, classDisplay)); 878 children.add(new Property("subClass", "string", "Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.", 0, 1, subClass)); 879 children.add(new Property("subClassDisplay", "string", "A short description for the subclass.", 0, 1, subClassDisplay)); 880 } 881 882 @Override 883 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 884 switch (_hash) { 885 case 98629247: /*group*/ return new Property("group", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify an employer group. May also be referred to as a Policy or Group ID.", 0, 1, group); 886 case 1322335555: /*groupDisplay*/ return new Property("groupDisplay", "string", "A short description for the group.", 0, 1, groupDisplay); 887 case -2101792737: /*subGroup*/ return new Property("subGroup", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a subset of an employer group.", 0, 1, subGroup); 888 case 1051914147: /*subGroupDisplay*/ return new Property("subGroupDisplay", "string", "A short description for the subgroup.", 0, 1, subGroupDisplay); 889 case 3443497: /*plan*/ return new Property("plan", "string", "Identifies a style or collective of coverage issued by the underwriter, for example may be used to identify a collection of benefits provided to employees. May be referred to as a Section or Division ID.", 0, 1, plan); 890 case -896076455: /*planDisplay*/ return new Property("planDisplay", "string", "A short description for the plan.", 0, 1, planDisplay); 891 case -1868653175: /*subPlan*/ return new Property("subPlan", "string", "Identifies a sub-style or sub-collective of coverage issued by the underwriter, for example may be used to identify a subset of a collection of benefits provided to employees.", 0, 1, subPlan); 892 case -1736083719: /*subPlanDisplay*/ return new Property("subPlanDisplay", "string", "A short description for the subplan.", 0, 1, subPlanDisplay); 893 case 94742904: /*class*/ return new Property("class", "string", "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage such as a level of deductables or co-payment.", 0, 1, class_); 894 case 1707405354: /*classDisplay*/ return new Property("classDisplay", "string", "A short description for the class.", 0, 1, classDisplay); 895 case -2105679080: /*subClass*/ return new Property("subClass", "string", "Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a subclass of coverage such as a sub-level of deductables or co-payment.", 0, 1, subClass); 896 case 1436983946: /*subClassDisplay*/ return new Property("subClassDisplay", "string", "A short description for the subclass.", 0, 1, subClassDisplay); 897 default: return super.getNamedProperty(_hash, _name, _checkValid); 898 } 899 900 } 901 902 @Override 903 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 904 switch (hash) { 905 case 98629247: /*group*/ return this.group == null ? new Base[0] : new Base[] {this.group}; // StringType 906 case 1322335555: /*groupDisplay*/ return this.groupDisplay == null ? new Base[0] : new Base[] {this.groupDisplay}; // StringType 907 case -2101792737: /*subGroup*/ return this.subGroup == null ? new Base[0] : new Base[] {this.subGroup}; // StringType 908 case 1051914147: /*subGroupDisplay*/ return this.subGroupDisplay == null ? new Base[0] : new Base[] {this.subGroupDisplay}; // StringType 909 case 3443497: /*plan*/ return this.plan == null ? new Base[0] : new Base[] {this.plan}; // StringType 910 case -896076455: /*planDisplay*/ return this.planDisplay == null ? new Base[0] : new Base[] {this.planDisplay}; // StringType 911 case -1868653175: /*subPlan*/ return this.subPlan == null ? new Base[0] : new Base[] {this.subPlan}; // StringType 912 case -1736083719: /*subPlanDisplay*/ return this.subPlanDisplay == null ? new Base[0] : new Base[] {this.subPlanDisplay}; // StringType 913 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // StringType 914 case 1707405354: /*classDisplay*/ return this.classDisplay == null ? new Base[0] : new Base[] {this.classDisplay}; // StringType 915 case -2105679080: /*subClass*/ return this.subClass == null ? new Base[0] : new Base[] {this.subClass}; // StringType 916 case 1436983946: /*subClassDisplay*/ return this.subClassDisplay == null ? new Base[0] : new Base[] {this.subClassDisplay}; // StringType 917 default: return super.getProperty(hash, name, checkValid); 918 } 919 920 } 921 922 @Override 923 public Base setProperty(int hash, String name, Base value) throws FHIRException { 924 switch (hash) { 925 case 98629247: // group 926 this.group = castToString(value); // StringType 927 return value; 928 case 1322335555: // groupDisplay 929 this.groupDisplay = castToString(value); // StringType 930 return value; 931 case -2101792737: // subGroup 932 this.subGroup = castToString(value); // StringType 933 return value; 934 case 1051914147: // subGroupDisplay 935 this.subGroupDisplay = castToString(value); // StringType 936 return value; 937 case 3443497: // plan 938 this.plan = castToString(value); // StringType 939 return value; 940 case -896076455: // planDisplay 941 this.planDisplay = castToString(value); // StringType 942 return value; 943 case -1868653175: // subPlan 944 this.subPlan = castToString(value); // StringType 945 return value; 946 case -1736083719: // subPlanDisplay 947 this.subPlanDisplay = castToString(value); // StringType 948 return value; 949 case 94742904: // class 950 this.class_ = castToString(value); // StringType 951 return value; 952 case 1707405354: // classDisplay 953 this.classDisplay = castToString(value); // StringType 954 return value; 955 case -2105679080: // subClass 956 this.subClass = castToString(value); // StringType 957 return value; 958 case 1436983946: // subClassDisplay 959 this.subClassDisplay = castToString(value); // StringType 960 return value; 961 default: return super.setProperty(hash, name, value); 962 } 963 964 } 965 966 @Override 967 public Base setProperty(String name, Base value) throws FHIRException { 968 if (name.equals("group")) { 969 this.group = castToString(value); // StringType 970 } else if (name.equals("groupDisplay")) { 971 this.groupDisplay = castToString(value); // StringType 972 } else if (name.equals("subGroup")) { 973 this.subGroup = castToString(value); // StringType 974 } else if (name.equals("subGroupDisplay")) { 975 this.subGroupDisplay = castToString(value); // StringType 976 } else if (name.equals("plan")) { 977 this.plan = castToString(value); // StringType 978 } else if (name.equals("planDisplay")) { 979 this.planDisplay = castToString(value); // StringType 980 } else if (name.equals("subPlan")) { 981 this.subPlan = castToString(value); // StringType 982 } else if (name.equals("subPlanDisplay")) { 983 this.subPlanDisplay = castToString(value); // StringType 984 } else if (name.equals("class")) { 985 this.class_ = castToString(value); // StringType 986 } else if (name.equals("classDisplay")) { 987 this.classDisplay = castToString(value); // StringType 988 } else if (name.equals("subClass")) { 989 this.subClass = castToString(value); // StringType 990 } else if (name.equals("subClassDisplay")) { 991 this.subClassDisplay = castToString(value); // StringType 992 } else 993 return super.setProperty(name, value); 994 return value; 995 } 996 997 @Override 998 public Base makeProperty(int hash, String name) throws FHIRException { 999 switch (hash) { 1000 case 98629247: return getGroupElement(); 1001 case 1322335555: return getGroupDisplayElement(); 1002 case -2101792737: return getSubGroupElement(); 1003 case 1051914147: return getSubGroupDisplayElement(); 1004 case 3443497: return getPlanElement(); 1005 case -896076455: return getPlanDisplayElement(); 1006 case -1868653175: return getSubPlanElement(); 1007 case -1736083719: return getSubPlanDisplayElement(); 1008 case 94742904: return getClass_Element(); 1009 case 1707405354: return getClassDisplayElement(); 1010 case -2105679080: return getSubClassElement(); 1011 case 1436983946: return getSubClassDisplayElement(); 1012 default: return super.makeProperty(hash, name); 1013 } 1014 1015 } 1016 1017 @Override 1018 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1019 switch (hash) { 1020 case 98629247: /*group*/ return new String[] {"string"}; 1021 case 1322335555: /*groupDisplay*/ return new String[] {"string"}; 1022 case -2101792737: /*subGroup*/ return new String[] {"string"}; 1023 case 1051914147: /*subGroupDisplay*/ return new String[] {"string"}; 1024 case 3443497: /*plan*/ return new String[] {"string"}; 1025 case -896076455: /*planDisplay*/ return new String[] {"string"}; 1026 case -1868653175: /*subPlan*/ return new String[] {"string"}; 1027 case -1736083719: /*subPlanDisplay*/ return new String[] {"string"}; 1028 case 94742904: /*class*/ return new String[] {"string"}; 1029 case 1707405354: /*classDisplay*/ return new String[] {"string"}; 1030 case -2105679080: /*subClass*/ return new String[] {"string"}; 1031 case 1436983946: /*subClassDisplay*/ return new String[] {"string"}; 1032 default: return super.getTypesForProperty(hash, name); 1033 } 1034 1035 } 1036 1037 @Override 1038 public Base addChild(String name) throws FHIRException { 1039 if (name.equals("group")) { 1040 throw new FHIRException("Cannot call addChild on a singleton property Coverage.group"); 1041 } 1042 else if (name.equals("groupDisplay")) { 1043 throw new FHIRException("Cannot call addChild on a singleton property Coverage.groupDisplay"); 1044 } 1045 else if (name.equals("subGroup")) { 1046 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subGroup"); 1047 } 1048 else if (name.equals("subGroupDisplay")) { 1049 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subGroupDisplay"); 1050 } 1051 else if (name.equals("plan")) { 1052 throw new FHIRException("Cannot call addChild on a singleton property Coverage.plan"); 1053 } 1054 else if (name.equals("planDisplay")) { 1055 throw new FHIRException("Cannot call addChild on a singleton property Coverage.planDisplay"); 1056 } 1057 else if (name.equals("subPlan")) { 1058 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subPlan"); 1059 } 1060 else if (name.equals("subPlanDisplay")) { 1061 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subPlanDisplay"); 1062 } 1063 else if (name.equals("class")) { 1064 throw new FHIRException("Cannot call addChild on a singleton property Coverage.class"); 1065 } 1066 else if (name.equals("classDisplay")) { 1067 throw new FHIRException("Cannot call addChild on a singleton property Coverage.classDisplay"); 1068 } 1069 else if (name.equals("subClass")) { 1070 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subClass"); 1071 } 1072 else if (name.equals("subClassDisplay")) { 1073 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subClassDisplay"); 1074 } 1075 else 1076 return super.addChild(name); 1077 } 1078 1079 public GroupComponent copy() { 1080 GroupComponent dst = new GroupComponent(); 1081 copyValues(dst); 1082 dst.group = group == null ? null : group.copy(); 1083 dst.groupDisplay = groupDisplay == null ? null : groupDisplay.copy(); 1084 dst.subGroup = subGroup == null ? null : subGroup.copy(); 1085 dst.subGroupDisplay = subGroupDisplay == null ? null : subGroupDisplay.copy(); 1086 dst.plan = plan == null ? null : plan.copy(); 1087 dst.planDisplay = planDisplay == null ? null : planDisplay.copy(); 1088 dst.subPlan = subPlan == null ? null : subPlan.copy(); 1089 dst.subPlanDisplay = subPlanDisplay == null ? null : subPlanDisplay.copy(); 1090 dst.class_ = class_ == null ? null : class_.copy(); 1091 dst.classDisplay = classDisplay == null ? null : classDisplay.copy(); 1092 dst.subClass = subClass == null ? null : subClass.copy(); 1093 dst.subClassDisplay = subClassDisplay == null ? null : subClassDisplay.copy(); 1094 return dst; 1095 } 1096 1097 @Override 1098 public boolean equalsDeep(Base other_) { 1099 if (!super.equalsDeep(other_)) 1100 return false; 1101 if (!(other_ instanceof GroupComponent)) 1102 return false; 1103 GroupComponent o = (GroupComponent) other_; 1104 return compareDeep(group, o.group, true) && compareDeep(groupDisplay, o.groupDisplay, true) && compareDeep(subGroup, o.subGroup, true) 1105 && compareDeep(subGroupDisplay, o.subGroupDisplay, true) && compareDeep(plan, o.plan, true) && compareDeep(planDisplay, o.planDisplay, true) 1106 && compareDeep(subPlan, o.subPlan, true) && compareDeep(subPlanDisplay, o.subPlanDisplay, true) 1107 && compareDeep(class_, o.class_, true) && compareDeep(classDisplay, o.classDisplay, true) && compareDeep(subClass, o.subClass, true) 1108 && compareDeep(subClassDisplay, o.subClassDisplay, true); 1109 } 1110 1111 @Override 1112 public boolean equalsShallow(Base other_) { 1113 if (!super.equalsShallow(other_)) 1114 return false; 1115 if (!(other_ instanceof GroupComponent)) 1116 return false; 1117 GroupComponent o = (GroupComponent) other_; 1118 return compareValues(group, o.group, true) && compareValues(groupDisplay, o.groupDisplay, true) && compareValues(subGroup, o.subGroup, true) 1119 && compareValues(subGroupDisplay, o.subGroupDisplay, true) && compareValues(plan, o.plan, true) && compareValues(planDisplay, o.planDisplay, true) 1120 && compareValues(subPlan, o.subPlan, true) && compareValues(subPlanDisplay, o.subPlanDisplay, true) 1121 && compareValues(class_, o.class_, true) && compareValues(classDisplay, o.classDisplay, true) && compareValues(subClass, o.subClass, true) 1122 && compareValues(subClassDisplay, o.subClassDisplay, true); 1123 } 1124 1125 public boolean isEmpty() { 1126 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(group, groupDisplay, subGroup 1127 , subGroupDisplay, plan, planDisplay, subPlan, subPlanDisplay, class_, classDisplay 1128 , subClass, subClassDisplay); 1129 } 1130 1131 public String fhirType() { 1132 return "Coverage.grouping"; 1133 1134 } 1135 1136 } 1137 1138 /** 1139 * The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant. 1140 */ 1141 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1142 @Description(shortDefinition="The primary coverage ID", formalDefinition="The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant." ) 1143 protected List<Identifier> identifier; 1144 1145 /** 1146 * The status of the resource instance. 1147 */ 1148 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1149 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 1150 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 1151 protected Enumeration<CoverageStatus> status; 1152 1153 /** 1154 * The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization. 1155 */ 1156 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1157 @Description(shortDefinition="Type of coverage such as medical or accident", formalDefinition="The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization." ) 1158 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverage-type") 1159 protected CodeableConcept type; 1160 1161 /** 1162 * The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer. 1163 */ 1164 @Child(name = "policyHolder", type = {Patient.class, RelatedPerson.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 1165 @Description(shortDefinition="Owner of the policy", formalDefinition="The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer." ) 1166 protected Reference policyHolder; 1167 1168 /** 1169 * The actual object that is the target of the reference (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1170 */ 1171 protected Resource policyHolderTarget; 1172 1173 /** 1174 * The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due. 1175 */ 1176 @Child(name = "subscriber", type = {Patient.class, RelatedPerson.class}, order=4, min=0, max=1, modifier=false, summary=true) 1177 @Description(shortDefinition="Subscriber to the policy", formalDefinition="The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due." ) 1178 protected Reference subscriber; 1179 1180 /** 1181 * The actual object that is the target of the reference (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1182 */ 1183 protected Resource subscriberTarget; 1184 1185 /** 1186 * The insurer assigned ID for the Subscriber. 1187 */ 1188 @Child(name = "subscriberId", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1189 @Description(shortDefinition="ID assigned to the Subscriber", formalDefinition="The insurer assigned ID for the Subscriber." ) 1190 protected StringType subscriberId; 1191 1192 /** 1193 * The party who benefits from the insurance coverage., the patient when services are provided. 1194 */ 1195 @Child(name = "beneficiary", type = {Patient.class}, order=6, min=0, max=1, modifier=false, summary=true) 1196 @Description(shortDefinition="Plan Beneficiary", formalDefinition="The party who benefits from the insurance coverage., the patient when services are provided." ) 1197 protected Reference beneficiary; 1198 1199 /** 1200 * The actual object that is the target of the reference (The party who benefits from the insurance coverage., the patient when services are provided.) 1201 */ 1202 protected Patient beneficiaryTarget; 1203 1204 /** 1205 * The relationship of beneficiary (patient) to the subscriber. 1206 */ 1207 @Child(name = "relationship", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1208 @Description(shortDefinition="Beneficiary relationship to the Subscriber", formalDefinition="The relationship of beneficiary (patient) to the subscriber." ) 1209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/policyholder-relationship") 1210 protected CodeableConcept relationship; 1211 1212 /** 1213 * Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force. 1214 */ 1215 @Child(name = "period", type = {Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 1216 @Description(shortDefinition="Coverage start and end dates", formalDefinition="Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force." ) 1217 protected Period period; 1218 1219 /** 1220 * The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number). 1221 */ 1222 @Child(name = "payor", type = {Organization.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1223 @Description(shortDefinition="Identifier for the plan or agreement issuer", formalDefinition="The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number)." ) 1224 protected List<Reference> payor; 1225 /** 1226 * The actual objects that are the target of the reference (The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).) 1227 */ 1228 protected List<Resource> payorTarget; 1229 1230 1231 /** 1232 * A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan. 1233 */ 1234 @Child(name = "grouping", type = {}, order=10, min=0, max=1, modifier=false, summary=false) 1235 @Description(shortDefinition="Additional coverage classifications", formalDefinition="A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan." ) 1236 protected GroupComponent grouping; 1237 1238 /** 1239 * A unique identifier for a dependent under the coverage. 1240 */ 1241 @Child(name = "dependent", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1242 @Description(shortDefinition="Dependent number", formalDefinition="A unique identifier for a dependent under the coverage." ) 1243 protected StringType dependent; 1244 1245 /** 1246 * An optional counter for a particular instance of the identified coverage which increments upon each renewal. 1247 */ 1248 @Child(name = "sequence", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1249 @Description(shortDefinition="The plan instance or sequence counter", formalDefinition="An optional counter for a particular instance of the identified coverage which increments upon each renewal." ) 1250 protected StringType sequence; 1251 1252 /** 1253 * The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care. 1254 */ 1255 @Child(name = "order", type = {PositiveIntType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1256 @Description(shortDefinition="Relative order of the coverage", formalDefinition="The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care." ) 1257 protected PositiveIntType order; 1258 1259 /** 1260 * The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1261 */ 1262 @Child(name = "network", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=true) 1263 @Description(shortDefinition="Insurer network", formalDefinition="The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply." ) 1264 protected StringType network; 1265 1266 /** 1267 * The policy(s) which constitute this insurance coverage. 1268 */ 1269 @Child(name = "contract", type = {Contract.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1270 @Description(shortDefinition="Contract details", formalDefinition="The policy(s) which constitute this insurance coverage." ) 1271 protected List<Reference> contract; 1272 /** 1273 * The actual objects that are the target of the reference (The policy(s) which constitute this insurance coverage.) 1274 */ 1275 protected List<Contract> contractTarget; 1276 1277 1278 private static final long serialVersionUID = -1719168406L; 1279 1280 /** 1281 * Constructor 1282 */ 1283 public Coverage() { 1284 super(); 1285 } 1286 1287 /** 1288 * @return {@link #identifier} (The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant.) 1289 */ 1290 public List<Identifier> getIdentifier() { 1291 if (this.identifier == null) 1292 this.identifier = new ArrayList<Identifier>(); 1293 return this.identifier; 1294 } 1295 1296 /** 1297 * @return Returns a reference to <code>this</code> for easy method chaining 1298 */ 1299 public Coverage setIdentifier(List<Identifier> theIdentifier) { 1300 this.identifier = theIdentifier; 1301 return this; 1302 } 1303 1304 public boolean hasIdentifier() { 1305 if (this.identifier == null) 1306 return false; 1307 for (Identifier item : this.identifier) 1308 if (!item.isEmpty()) 1309 return true; 1310 return false; 1311 } 1312 1313 public Identifier addIdentifier() { //3 1314 Identifier t = new Identifier(); 1315 if (this.identifier == null) 1316 this.identifier = new ArrayList<Identifier>(); 1317 this.identifier.add(t); 1318 return t; 1319 } 1320 1321 public Coverage addIdentifier(Identifier t) { //3 1322 if (t == null) 1323 return this; 1324 if (this.identifier == null) 1325 this.identifier = new ArrayList<Identifier>(); 1326 this.identifier.add(t); 1327 return this; 1328 } 1329 1330 /** 1331 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1332 */ 1333 public Identifier getIdentifierFirstRep() { 1334 if (getIdentifier().isEmpty()) { 1335 addIdentifier(); 1336 } 1337 return getIdentifier().get(0); 1338 } 1339 1340 /** 1341 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1342 */ 1343 public Enumeration<CoverageStatus> getStatusElement() { 1344 if (this.status == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error("Attempt to auto-create Coverage.status"); 1347 else if (Configuration.doAutoCreate()) 1348 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); // bb 1349 return this.status; 1350 } 1351 1352 public boolean hasStatusElement() { 1353 return this.status != null && !this.status.isEmpty(); 1354 } 1355 1356 public boolean hasStatus() { 1357 return this.status != null && !this.status.isEmpty(); 1358 } 1359 1360 /** 1361 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1362 */ 1363 public Coverage setStatusElement(Enumeration<CoverageStatus> value) { 1364 this.status = value; 1365 return this; 1366 } 1367 1368 /** 1369 * @return The status of the resource instance. 1370 */ 1371 public CoverageStatus getStatus() { 1372 return this.status == null ? null : this.status.getValue(); 1373 } 1374 1375 /** 1376 * @param value The status of the resource instance. 1377 */ 1378 public Coverage setStatus(CoverageStatus value) { 1379 if (value == null) 1380 this.status = null; 1381 else { 1382 if (this.status == null) 1383 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); 1384 this.status.setValue(value); 1385 } 1386 return this; 1387 } 1388 1389 /** 1390 * @return {@link #type} (The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.) 1391 */ 1392 public CodeableConcept getType() { 1393 if (this.type == null) 1394 if (Configuration.errorOnAutoCreate()) 1395 throw new Error("Attempt to auto-create Coverage.type"); 1396 else if (Configuration.doAutoCreate()) 1397 this.type = new CodeableConcept(); // cc 1398 return this.type; 1399 } 1400 1401 public boolean hasType() { 1402 return this.type != null && !this.type.isEmpty(); 1403 } 1404 1405 /** 1406 * @param value {@link #type} (The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.) 1407 */ 1408 public Coverage setType(CodeableConcept value) { 1409 this.type = value; 1410 return this; 1411 } 1412 1413 /** 1414 * @return {@link #policyHolder} (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1415 */ 1416 public Reference getPolicyHolder() { 1417 if (this.policyHolder == null) 1418 if (Configuration.errorOnAutoCreate()) 1419 throw new Error("Attempt to auto-create Coverage.policyHolder"); 1420 else if (Configuration.doAutoCreate()) 1421 this.policyHolder = new Reference(); // cc 1422 return this.policyHolder; 1423 } 1424 1425 public boolean hasPolicyHolder() { 1426 return this.policyHolder != null && !this.policyHolder.isEmpty(); 1427 } 1428 1429 /** 1430 * @param value {@link #policyHolder} (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1431 */ 1432 public Coverage setPolicyHolder(Reference value) { 1433 this.policyHolder = value; 1434 return this; 1435 } 1436 1437 /** 1438 * @return {@link #policyHolder} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1439 */ 1440 public Resource getPolicyHolderTarget() { 1441 return this.policyHolderTarget; 1442 } 1443 1444 /** 1445 * @param value {@link #policyHolder} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.) 1446 */ 1447 public Coverage setPolicyHolderTarget(Resource value) { 1448 this.policyHolderTarget = value; 1449 return this; 1450 } 1451 1452 /** 1453 * @return {@link #subscriber} (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1454 */ 1455 public Reference getSubscriber() { 1456 if (this.subscriber == null) 1457 if (Configuration.errorOnAutoCreate()) 1458 throw new Error("Attempt to auto-create Coverage.subscriber"); 1459 else if (Configuration.doAutoCreate()) 1460 this.subscriber = new Reference(); // cc 1461 return this.subscriber; 1462 } 1463 1464 public boolean hasSubscriber() { 1465 return this.subscriber != null && !this.subscriber.isEmpty(); 1466 } 1467 1468 /** 1469 * @param value {@link #subscriber} (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1470 */ 1471 public Coverage setSubscriber(Reference value) { 1472 this.subscriber = value; 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #subscriber} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1478 */ 1479 public Resource getSubscriberTarget() { 1480 return this.subscriberTarget; 1481 } 1482 1483 /** 1484 * @param value {@link #subscriber} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1485 */ 1486 public Coverage setSubscriberTarget(Resource value) { 1487 this.subscriberTarget = value; 1488 return this; 1489 } 1490 1491 /** 1492 * @return {@link #subscriberId} (The insurer assigned ID for the Subscriber.). This is the underlying object with id, value and extensions. The accessor "getSubscriberId" gives direct access to the value 1493 */ 1494 public StringType getSubscriberIdElement() { 1495 if (this.subscriberId == null) 1496 if (Configuration.errorOnAutoCreate()) 1497 throw new Error("Attempt to auto-create Coverage.subscriberId"); 1498 else if (Configuration.doAutoCreate()) 1499 this.subscriberId = new StringType(); // bb 1500 return this.subscriberId; 1501 } 1502 1503 public boolean hasSubscriberIdElement() { 1504 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1505 } 1506 1507 public boolean hasSubscriberId() { 1508 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1509 } 1510 1511 /** 1512 * @param value {@link #subscriberId} (The insurer assigned ID for the Subscriber.). This is the underlying object with id, value and extensions. The accessor "getSubscriberId" gives direct access to the value 1513 */ 1514 public Coverage setSubscriberIdElement(StringType value) { 1515 this.subscriberId = value; 1516 return this; 1517 } 1518 1519 /** 1520 * @return The insurer assigned ID for the Subscriber. 1521 */ 1522 public String getSubscriberId() { 1523 return this.subscriberId == null ? null : this.subscriberId.getValue(); 1524 } 1525 1526 /** 1527 * @param value The insurer assigned ID for the Subscriber. 1528 */ 1529 public Coverage setSubscriberId(String value) { 1530 if (Utilities.noString(value)) 1531 this.subscriberId = null; 1532 else { 1533 if (this.subscriberId == null) 1534 this.subscriberId = new StringType(); 1535 this.subscriberId.setValue(value); 1536 } 1537 return this; 1538 } 1539 1540 /** 1541 * @return {@link #beneficiary} (The party who benefits from the insurance coverage., the patient when services are provided.) 1542 */ 1543 public Reference getBeneficiary() { 1544 if (this.beneficiary == null) 1545 if (Configuration.errorOnAutoCreate()) 1546 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1547 else if (Configuration.doAutoCreate()) 1548 this.beneficiary = new Reference(); // cc 1549 return this.beneficiary; 1550 } 1551 1552 public boolean hasBeneficiary() { 1553 return this.beneficiary != null && !this.beneficiary.isEmpty(); 1554 } 1555 1556 /** 1557 * @param value {@link #beneficiary} (The party who benefits from the insurance coverage., the patient when services are provided.) 1558 */ 1559 public Coverage setBeneficiary(Reference value) { 1560 this.beneficiary = value; 1561 return this; 1562 } 1563 1564 /** 1565 * @return {@link #beneficiary} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The party who benefits from the insurance coverage., the patient when services are provided.) 1566 */ 1567 public Patient getBeneficiaryTarget() { 1568 if (this.beneficiaryTarget == null) 1569 if (Configuration.errorOnAutoCreate()) 1570 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1571 else if (Configuration.doAutoCreate()) 1572 this.beneficiaryTarget = new Patient(); // aa 1573 return this.beneficiaryTarget; 1574 } 1575 1576 /** 1577 * @param value {@link #beneficiary} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The party who benefits from the insurance coverage., the patient when services are provided.) 1578 */ 1579 public Coverage setBeneficiaryTarget(Patient value) { 1580 this.beneficiaryTarget = value; 1581 return this; 1582 } 1583 1584 /** 1585 * @return {@link #relationship} (The relationship of beneficiary (patient) to the subscriber.) 1586 */ 1587 public CodeableConcept getRelationship() { 1588 if (this.relationship == null) 1589 if (Configuration.errorOnAutoCreate()) 1590 throw new Error("Attempt to auto-create Coverage.relationship"); 1591 else if (Configuration.doAutoCreate()) 1592 this.relationship = new CodeableConcept(); // cc 1593 return this.relationship; 1594 } 1595 1596 public boolean hasRelationship() { 1597 return this.relationship != null && !this.relationship.isEmpty(); 1598 } 1599 1600 /** 1601 * @param value {@link #relationship} (The relationship of beneficiary (patient) to the subscriber.) 1602 */ 1603 public Coverage setRelationship(CodeableConcept value) { 1604 this.relationship = value; 1605 return this; 1606 } 1607 1608 /** 1609 * @return {@link #period} (Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.) 1610 */ 1611 public Period getPeriod() { 1612 if (this.period == null) 1613 if (Configuration.errorOnAutoCreate()) 1614 throw new Error("Attempt to auto-create Coverage.period"); 1615 else if (Configuration.doAutoCreate()) 1616 this.period = new Period(); // cc 1617 return this.period; 1618 } 1619 1620 public boolean hasPeriod() { 1621 return this.period != null && !this.period.isEmpty(); 1622 } 1623 1624 /** 1625 * @param value {@link #period} (Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.) 1626 */ 1627 public Coverage setPeriod(Period value) { 1628 this.period = value; 1629 return this; 1630 } 1631 1632 /** 1633 * @return {@link #payor} (The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).) 1634 */ 1635 public List<Reference> getPayor() { 1636 if (this.payor == null) 1637 this.payor = new ArrayList<Reference>(); 1638 return this.payor; 1639 } 1640 1641 /** 1642 * @return Returns a reference to <code>this</code> for easy method chaining 1643 */ 1644 public Coverage setPayor(List<Reference> thePayor) { 1645 this.payor = thePayor; 1646 return this; 1647 } 1648 1649 public boolean hasPayor() { 1650 if (this.payor == null) 1651 return false; 1652 for (Reference item : this.payor) 1653 if (!item.isEmpty()) 1654 return true; 1655 return false; 1656 } 1657 1658 public Reference addPayor() { //3 1659 Reference t = new Reference(); 1660 if (this.payor == null) 1661 this.payor = new ArrayList<Reference>(); 1662 this.payor.add(t); 1663 return t; 1664 } 1665 1666 public Coverage addPayor(Reference t) { //3 1667 if (t == null) 1668 return this; 1669 if (this.payor == null) 1670 this.payor = new ArrayList<Reference>(); 1671 this.payor.add(t); 1672 return this; 1673 } 1674 1675 /** 1676 * @return The first repetition of repeating field {@link #payor}, creating it if it does not already exist 1677 */ 1678 public Reference getPayorFirstRep() { 1679 if (getPayor().isEmpty()) { 1680 addPayor(); 1681 } 1682 return getPayor().get(0); 1683 } 1684 1685 /** 1686 * @deprecated Use Reference#setResource(IBaseResource) instead 1687 */ 1688 @Deprecated 1689 public List<Resource> getPayorTarget() { 1690 if (this.payorTarget == null) 1691 this.payorTarget = new ArrayList<Resource>(); 1692 return this.payorTarget; 1693 } 1694 1695 /** 1696 * @return {@link #grouping} (A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.) 1697 */ 1698 public GroupComponent getGrouping() { 1699 if (this.grouping == null) 1700 if (Configuration.errorOnAutoCreate()) 1701 throw new Error("Attempt to auto-create Coverage.grouping"); 1702 else if (Configuration.doAutoCreate()) 1703 this.grouping = new GroupComponent(); // cc 1704 return this.grouping; 1705 } 1706 1707 public boolean hasGrouping() { 1708 return this.grouping != null && !this.grouping.isEmpty(); 1709 } 1710 1711 /** 1712 * @param value {@link #grouping} (A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.) 1713 */ 1714 public Coverage setGrouping(GroupComponent value) { 1715 this.grouping = value; 1716 return this; 1717 } 1718 1719 /** 1720 * @return {@link #dependent} (A unique identifier for a dependent under the coverage.). This is the underlying object with id, value and extensions. The accessor "getDependent" gives direct access to the value 1721 */ 1722 public StringType getDependentElement() { 1723 if (this.dependent == null) 1724 if (Configuration.errorOnAutoCreate()) 1725 throw new Error("Attempt to auto-create Coverage.dependent"); 1726 else if (Configuration.doAutoCreate()) 1727 this.dependent = new StringType(); // bb 1728 return this.dependent; 1729 } 1730 1731 public boolean hasDependentElement() { 1732 return this.dependent != null && !this.dependent.isEmpty(); 1733 } 1734 1735 public boolean hasDependent() { 1736 return this.dependent != null && !this.dependent.isEmpty(); 1737 } 1738 1739 /** 1740 * @param value {@link #dependent} (A unique identifier for a dependent under the coverage.). This is the underlying object with id, value and extensions. The accessor "getDependent" gives direct access to the value 1741 */ 1742 public Coverage setDependentElement(StringType value) { 1743 this.dependent = value; 1744 return this; 1745 } 1746 1747 /** 1748 * @return A unique identifier for a dependent under the coverage. 1749 */ 1750 public String getDependent() { 1751 return this.dependent == null ? null : this.dependent.getValue(); 1752 } 1753 1754 /** 1755 * @param value A unique identifier for a dependent under the coverage. 1756 */ 1757 public Coverage setDependent(String value) { 1758 if (Utilities.noString(value)) 1759 this.dependent = null; 1760 else { 1761 if (this.dependent == null) 1762 this.dependent = new StringType(); 1763 this.dependent.setValue(value); 1764 } 1765 return this; 1766 } 1767 1768 /** 1769 * @return {@link #sequence} (An optional counter for a particular instance of the identified coverage which increments upon each renewal.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1770 */ 1771 public StringType getSequenceElement() { 1772 if (this.sequence == null) 1773 if (Configuration.errorOnAutoCreate()) 1774 throw new Error("Attempt to auto-create Coverage.sequence"); 1775 else if (Configuration.doAutoCreate()) 1776 this.sequence = new StringType(); // bb 1777 return this.sequence; 1778 } 1779 1780 public boolean hasSequenceElement() { 1781 return this.sequence != null && !this.sequence.isEmpty(); 1782 } 1783 1784 public boolean hasSequence() { 1785 return this.sequence != null && !this.sequence.isEmpty(); 1786 } 1787 1788 /** 1789 * @param value {@link #sequence} (An optional counter for a particular instance of the identified coverage which increments upon each renewal.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1790 */ 1791 public Coverage setSequenceElement(StringType value) { 1792 this.sequence = value; 1793 return this; 1794 } 1795 1796 /** 1797 * @return An optional counter for a particular instance of the identified coverage which increments upon each renewal. 1798 */ 1799 public String getSequence() { 1800 return this.sequence == null ? null : this.sequence.getValue(); 1801 } 1802 1803 /** 1804 * @param value An optional counter for a particular instance of the identified coverage which increments upon each renewal. 1805 */ 1806 public Coverage setSequence(String value) { 1807 if (Utilities.noString(value)) 1808 this.sequence = null; 1809 else { 1810 if (this.sequence == null) 1811 this.sequence = new StringType(); 1812 this.sequence.setValue(value); 1813 } 1814 return this; 1815 } 1816 1817 /** 1818 * @return {@link #order} (The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.). This is the underlying object with id, value and extensions. The accessor "getOrder" gives direct access to the value 1819 */ 1820 public PositiveIntType getOrderElement() { 1821 if (this.order == null) 1822 if (Configuration.errorOnAutoCreate()) 1823 throw new Error("Attempt to auto-create Coverage.order"); 1824 else if (Configuration.doAutoCreate()) 1825 this.order = new PositiveIntType(); // bb 1826 return this.order; 1827 } 1828 1829 public boolean hasOrderElement() { 1830 return this.order != null && !this.order.isEmpty(); 1831 } 1832 1833 public boolean hasOrder() { 1834 return this.order != null && !this.order.isEmpty(); 1835 } 1836 1837 /** 1838 * @param value {@link #order} (The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.). This is the underlying object with id, value and extensions. The accessor "getOrder" gives direct access to the value 1839 */ 1840 public Coverage setOrderElement(PositiveIntType value) { 1841 this.order = value; 1842 return this; 1843 } 1844 1845 /** 1846 * @return The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care. 1847 */ 1848 public int getOrder() { 1849 return this.order == null || this.order.isEmpty() ? 0 : this.order.getValue(); 1850 } 1851 1852 /** 1853 * @param value The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care. 1854 */ 1855 public Coverage setOrder(int value) { 1856 if (this.order == null) 1857 this.order = new PositiveIntType(); 1858 this.order.setValue(value); 1859 return this; 1860 } 1861 1862 /** 1863 * @return {@link #network} (The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.). This is the underlying object with id, value and extensions. The accessor "getNetwork" gives direct access to the value 1864 */ 1865 public StringType getNetworkElement() { 1866 if (this.network == null) 1867 if (Configuration.errorOnAutoCreate()) 1868 throw new Error("Attempt to auto-create Coverage.network"); 1869 else if (Configuration.doAutoCreate()) 1870 this.network = new StringType(); // bb 1871 return this.network; 1872 } 1873 1874 public boolean hasNetworkElement() { 1875 return this.network != null && !this.network.isEmpty(); 1876 } 1877 1878 public boolean hasNetwork() { 1879 return this.network != null && !this.network.isEmpty(); 1880 } 1881 1882 /** 1883 * @param value {@link #network} (The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.). This is the underlying object with id, value and extensions. The accessor "getNetwork" gives direct access to the value 1884 */ 1885 public Coverage setNetworkElement(StringType value) { 1886 this.network = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1892 */ 1893 public String getNetwork() { 1894 return this.network == null ? null : this.network.getValue(); 1895 } 1896 1897 /** 1898 * @param value The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1899 */ 1900 public Coverage setNetwork(String value) { 1901 if (Utilities.noString(value)) 1902 this.network = null; 1903 else { 1904 if (this.network == null) 1905 this.network = new StringType(); 1906 this.network.setValue(value); 1907 } 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #contract} (The policy(s) which constitute this insurance coverage.) 1913 */ 1914 public List<Reference> getContract() { 1915 if (this.contract == null) 1916 this.contract = new ArrayList<Reference>(); 1917 return this.contract; 1918 } 1919 1920 /** 1921 * @return Returns a reference to <code>this</code> for easy method chaining 1922 */ 1923 public Coverage setContract(List<Reference> theContract) { 1924 this.contract = theContract; 1925 return this; 1926 } 1927 1928 public boolean hasContract() { 1929 if (this.contract == null) 1930 return false; 1931 for (Reference item : this.contract) 1932 if (!item.isEmpty()) 1933 return true; 1934 return false; 1935 } 1936 1937 public Reference addContract() { //3 1938 Reference t = new Reference(); 1939 if (this.contract == null) 1940 this.contract = new ArrayList<Reference>(); 1941 this.contract.add(t); 1942 return t; 1943 } 1944 1945 public Coverage addContract(Reference t) { //3 1946 if (t == null) 1947 return this; 1948 if (this.contract == null) 1949 this.contract = new ArrayList<Reference>(); 1950 this.contract.add(t); 1951 return this; 1952 } 1953 1954 /** 1955 * @return The first repetition of repeating field {@link #contract}, creating it if it does not already exist 1956 */ 1957 public Reference getContractFirstRep() { 1958 if (getContract().isEmpty()) { 1959 addContract(); 1960 } 1961 return getContract().get(0); 1962 } 1963 1964 /** 1965 * @deprecated Use Reference#setResource(IBaseResource) instead 1966 */ 1967 @Deprecated 1968 public List<Contract> getContractTarget() { 1969 if (this.contractTarget == null) 1970 this.contractTarget = new ArrayList<Contract>(); 1971 return this.contractTarget; 1972 } 1973 1974 /** 1975 * @deprecated Use Reference#setResource(IBaseResource) instead 1976 */ 1977 @Deprecated 1978 public Contract addContractTarget() { 1979 Contract r = new Contract(); 1980 if (this.contractTarget == null) 1981 this.contractTarget = new ArrayList<Contract>(); 1982 this.contractTarget.add(r); 1983 return r; 1984 } 1985 1986 protected void listChildren(List<Property> children) { 1987 super.listChildren(children); 1988 children.add(new Property("identifier", "Identifier", "The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1989 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 1990 children.add(new Property("type", "CodeableConcept", "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 0, 1, type)); 1991 children.add(new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", "The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.", 0, 1, policyHolder)); 1992 children.add(new Property("subscriber", "Reference(Patient|RelatedPerson)", "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 0, 1, subscriber)); 1993 children.add(new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 1, subscriberId)); 1994 children.add(new Property("beneficiary", "Reference(Patient)", "The party who benefits from the insurance coverage., the patient when services are provided.", 0, 1, beneficiary)); 1995 children.add(new Property("relationship", "CodeableConcept", "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship)); 1996 children.add(new Property("period", "Period", "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 0, 1, period)); 1997 children.add(new Property("payor", "Reference(Organization|Patient|RelatedPerson)", "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).", 0, java.lang.Integer.MAX_VALUE, payor)); 1998 children.add(new Property("grouping", "", "A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 0, 1, grouping)); 1999 children.add(new Property("dependent", "string", "A unique identifier for a dependent under the coverage.", 0, 1, dependent)); 2000 children.add(new Property("sequence", "string", "An optional counter for a particular instance of the identified coverage which increments upon each renewal.", 0, 1, sequence)); 2001 children.add(new Property("order", "positiveInt", "The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.", 0, 1, order)); 2002 children.add(new Property("network", "string", "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 0, 1, network)); 2003 children.add(new Property("contract", "Reference(Contract)", "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract)); 2004 } 2005 2006 @Override 2007 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2008 switch (_hash) { 2009 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Certificate number, Personal Health Number or Case ID. May be constructed as the concatination of the Coverage.SubscriberID and the Coverage.dependant.", 0, java.lang.Integer.MAX_VALUE, identifier); 2010 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2011 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 0, 1, type); 2012 case 2046898558: /*policyHolder*/ return new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", "The party who 'owns' the insurance policy, may be an individual, corporation or the subscriber's employer.", 0, 1, policyHolder); 2013 case -1219769240: /*subscriber*/ return new Property("subscriber", "Reference(Patient|RelatedPerson)", "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 0, 1, subscriber); 2014 case 327834531: /*subscriberId*/ return new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 1, subscriberId); 2015 case -565102875: /*beneficiary*/ return new Property("beneficiary", "Reference(Patient)", "The party who benefits from the insurance coverage., the patient when services are provided.", 0, 1, beneficiary); 2016 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship); 2017 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 0, 1, period); 2018 case 106443915: /*payor*/ return new Property("payor", "Reference(Organization|Patient|RelatedPerson)", "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements. May provide multiple identifiers such as insurance company identifier or business identifier (BIN number).", 0, java.lang.Integer.MAX_VALUE, payor); 2019 case 506371331: /*grouping*/ return new Property("grouping", "", "A suite of underwrite specific classifiers, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 0, 1, grouping); 2020 case -1109226753: /*dependent*/ return new Property("dependent", "string", "A unique identifier for a dependent under the coverage.", 0, 1, dependent); 2021 case 1349547969: /*sequence*/ return new Property("sequence", "string", "An optional counter for a particular instance of the identified coverage which increments upon each renewal.", 0, 1, sequence); 2022 case 106006350: /*order*/ return new Property("order", "positiveInt", "The order of applicability of this coverage relative to other coverages which are currently inforce. Note, there may be gaps in the numbering and this does not imply primary, secondard etc. as the specific positioning of coverages depends upon the episode of care.", 0, 1, order); 2023 case 1843485230: /*network*/ return new Property("network", "string", "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 0, 1, network); 2024 case -566947566: /*contract*/ return new Property("contract", "Reference(Contract)", "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract); 2025 default: return super.getNamedProperty(_hash, _name, _checkValid); 2026 } 2027 2028 } 2029 2030 @Override 2031 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2032 switch (hash) { 2033 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2034 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CoverageStatus> 2035 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2036 case 2046898558: /*policyHolder*/ return this.policyHolder == null ? new Base[0] : new Base[] {this.policyHolder}; // Reference 2037 case -1219769240: /*subscriber*/ return this.subscriber == null ? new Base[0] : new Base[] {this.subscriber}; // Reference 2038 case 327834531: /*subscriberId*/ return this.subscriberId == null ? new Base[0] : new Base[] {this.subscriberId}; // StringType 2039 case -565102875: /*beneficiary*/ return this.beneficiary == null ? new Base[0] : new Base[] {this.beneficiary}; // Reference 2040 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 2041 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 2042 case 106443915: /*payor*/ return this.payor == null ? new Base[0] : this.payor.toArray(new Base[this.payor.size()]); // Reference 2043 case 506371331: /*grouping*/ return this.grouping == null ? new Base[0] : new Base[] {this.grouping}; // GroupComponent 2044 case -1109226753: /*dependent*/ return this.dependent == null ? new Base[0] : new Base[] {this.dependent}; // StringType 2045 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // StringType 2046 case 106006350: /*order*/ return this.order == null ? new Base[0] : new Base[] {this.order}; // PositiveIntType 2047 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // StringType 2048 case -566947566: /*contract*/ return this.contract == null ? new Base[0] : this.contract.toArray(new Base[this.contract.size()]); // Reference 2049 default: return super.getProperty(hash, name, checkValid); 2050 } 2051 2052 } 2053 2054 @Override 2055 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2056 switch (hash) { 2057 case -1618432855: // identifier 2058 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2059 return value; 2060 case -892481550: // status 2061 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2062 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2063 return value; 2064 case 3575610: // type 2065 this.type = castToCodeableConcept(value); // CodeableConcept 2066 return value; 2067 case 2046898558: // policyHolder 2068 this.policyHolder = castToReference(value); // Reference 2069 return value; 2070 case -1219769240: // subscriber 2071 this.subscriber = castToReference(value); // Reference 2072 return value; 2073 case 327834531: // subscriberId 2074 this.subscriberId = castToString(value); // StringType 2075 return value; 2076 case -565102875: // beneficiary 2077 this.beneficiary = castToReference(value); // Reference 2078 return value; 2079 case -261851592: // relationship 2080 this.relationship = castToCodeableConcept(value); // CodeableConcept 2081 return value; 2082 case -991726143: // period 2083 this.period = castToPeriod(value); // Period 2084 return value; 2085 case 106443915: // payor 2086 this.getPayor().add(castToReference(value)); // Reference 2087 return value; 2088 case 506371331: // grouping 2089 this.grouping = (GroupComponent) value; // GroupComponent 2090 return value; 2091 case -1109226753: // dependent 2092 this.dependent = castToString(value); // StringType 2093 return value; 2094 case 1349547969: // sequence 2095 this.sequence = castToString(value); // StringType 2096 return value; 2097 case 106006350: // order 2098 this.order = castToPositiveInt(value); // PositiveIntType 2099 return value; 2100 case 1843485230: // network 2101 this.network = castToString(value); // StringType 2102 return value; 2103 case -566947566: // contract 2104 this.getContract().add(castToReference(value)); // Reference 2105 return value; 2106 default: return super.setProperty(hash, name, value); 2107 } 2108 2109 } 2110 2111 @Override 2112 public Base setProperty(String name, Base value) throws FHIRException { 2113 if (name.equals("identifier")) { 2114 this.getIdentifier().add(castToIdentifier(value)); 2115 } else if (name.equals("status")) { 2116 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2117 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2118 } else if (name.equals("type")) { 2119 this.type = castToCodeableConcept(value); // CodeableConcept 2120 } else if (name.equals("policyHolder")) { 2121 this.policyHolder = castToReference(value); // Reference 2122 } else if (name.equals("subscriber")) { 2123 this.subscriber = castToReference(value); // Reference 2124 } else if (name.equals("subscriberId")) { 2125 this.subscriberId = castToString(value); // StringType 2126 } else if (name.equals("beneficiary")) { 2127 this.beneficiary = castToReference(value); // Reference 2128 } else if (name.equals("relationship")) { 2129 this.relationship = castToCodeableConcept(value); // CodeableConcept 2130 } else if (name.equals("period")) { 2131 this.period = castToPeriod(value); // Period 2132 } else if (name.equals("payor")) { 2133 this.getPayor().add(castToReference(value)); 2134 } else if (name.equals("grouping")) { 2135 this.grouping = (GroupComponent) value; // GroupComponent 2136 } else if (name.equals("dependent")) { 2137 this.dependent = castToString(value); // StringType 2138 } else if (name.equals("sequence")) { 2139 this.sequence = castToString(value); // StringType 2140 } else if (name.equals("order")) { 2141 this.order = castToPositiveInt(value); // PositiveIntType 2142 } else if (name.equals("network")) { 2143 this.network = castToString(value); // StringType 2144 } else if (name.equals("contract")) { 2145 this.getContract().add(castToReference(value)); 2146 } else 2147 return super.setProperty(name, value); 2148 return value; 2149 } 2150 2151 @Override 2152 public Base makeProperty(int hash, String name) throws FHIRException { 2153 switch (hash) { 2154 case -1618432855: return addIdentifier(); 2155 case -892481550: return getStatusElement(); 2156 case 3575610: return getType(); 2157 case 2046898558: return getPolicyHolder(); 2158 case -1219769240: return getSubscriber(); 2159 case 327834531: return getSubscriberIdElement(); 2160 case -565102875: return getBeneficiary(); 2161 case -261851592: return getRelationship(); 2162 case -991726143: return getPeriod(); 2163 case 106443915: return addPayor(); 2164 case 506371331: return getGrouping(); 2165 case -1109226753: return getDependentElement(); 2166 case 1349547969: return getSequenceElement(); 2167 case 106006350: return getOrderElement(); 2168 case 1843485230: return getNetworkElement(); 2169 case -566947566: return addContract(); 2170 default: return super.makeProperty(hash, name); 2171 } 2172 2173 } 2174 2175 @Override 2176 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2177 switch (hash) { 2178 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2179 case -892481550: /*status*/ return new String[] {"code"}; 2180 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2181 case 2046898558: /*policyHolder*/ return new String[] {"Reference"}; 2182 case -1219769240: /*subscriber*/ return new String[] {"Reference"}; 2183 case 327834531: /*subscriberId*/ return new String[] {"string"}; 2184 case -565102875: /*beneficiary*/ return new String[] {"Reference"}; 2185 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 2186 case -991726143: /*period*/ return new String[] {"Period"}; 2187 case 106443915: /*payor*/ return new String[] {"Reference"}; 2188 case 506371331: /*grouping*/ return new String[] {}; 2189 case -1109226753: /*dependent*/ return new String[] {"string"}; 2190 case 1349547969: /*sequence*/ return new String[] {"string"}; 2191 case 106006350: /*order*/ return new String[] {"positiveInt"}; 2192 case 1843485230: /*network*/ return new String[] {"string"}; 2193 case -566947566: /*contract*/ return new String[] {"Reference"}; 2194 default: return super.getTypesForProperty(hash, name); 2195 } 2196 2197 } 2198 2199 @Override 2200 public Base addChild(String name) throws FHIRException { 2201 if (name.equals("identifier")) { 2202 return addIdentifier(); 2203 } 2204 else if (name.equals("status")) { 2205 throw new FHIRException("Cannot call addChild on a singleton property Coverage.status"); 2206 } 2207 else if (name.equals("type")) { 2208 this.type = new CodeableConcept(); 2209 return this.type; 2210 } 2211 else if (name.equals("policyHolder")) { 2212 this.policyHolder = new Reference(); 2213 return this.policyHolder; 2214 } 2215 else if (name.equals("subscriber")) { 2216 this.subscriber = new Reference(); 2217 return this.subscriber; 2218 } 2219 else if (name.equals("subscriberId")) { 2220 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subscriberId"); 2221 } 2222 else if (name.equals("beneficiary")) { 2223 this.beneficiary = new Reference(); 2224 return this.beneficiary; 2225 } 2226 else if (name.equals("relationship")) { 2227 this.relationship = new CodeableConcept(); 2228 return this.relationship; 2229 } 2230 else if (name.equals("period")) { 2231 this.period = new Period(); 2232 return this.period; 2233 } 2234 else if (name.equals("payor")) { 2235 return addPayor(); 2236 } 2237 else if (name.equals("grouping")) { 2238 this.grouping = new GroupComponent(); 2239 return this.grouping; 2240 } 2241 else if (name.equals("dependent")) { 2242 throw new FHIRException("Cannot call addChild on a singleton property Coverage.dependent"); 2243 } 2244 else if (name.equals("sequence")) { 2245 throw new FHIRException("Cannot call addChild on a singleton property Coverage.sequence"); 2246 } 2247 else if (name.equals("order")) { 2248 throw new FHIRException("Cannot call addChild on a singleton property Coverage.order"); 2249 } 2250 else if (name.equals("network")) { 2251 throw new FHIRException("Cannot call addChild on a singleton property Coverage.network"); 2252 } 2253 else if (name.equals("contract")) { 2254 return addContract(); 2255 } 2256 else 2257 return super.addChild(name); 2258 } 2259 2260 public String fhirType() { 2261 return "Coverage"; 2262 2263 } 2264 2265 public Coverage copy() { 2266 Coverage dst = new Coverage(); 2267 copyValues(dst); 2268 if (identifier != null) { 2269 dst.identifier = new ArrayList<Identifier>(); 2270 for (Identifier i : identifier) 2271 dst.identifier.add(i.copy()); 2272 }; 2273 dst.status = status == null ? null : status.copy(); 2274 dst.type = type == null ? null : type.copy(); 2275 dst.policyHolder = policyHolder == null ? null : policyHolder.copy(); 2276 dst.subscriber = subscriber == null ? null : subscriber.copy(); 2277 dst.subscriberId = subscriberId == null ? null : subscriberId.copy(); 2278 dst.beneficiary = beneficiary == null ? null : beneficiary.copy(); 2279 dst.relationship = relationship == null ? null : relationship.copy(); 2280 dst.period = period == null ? null : period.copy(); 2281 if (payor != null) { 2282 dst.payor = new ArrayList<Reference>(); 2283 for (Reference i : payor) 2284 dst.payor.add(i.copy()); 2285 }; 2286 dst.grouping = grouping == null ? null : grouping.copy(); 2287 dst.dependent = dependent == null ? null : dependent.copy(); 2288 dst.sequence = sequence == null ? null : sequence.copy(); 2289 dst.order = order == null ? null : order.copy(); 2290 dst.network = network == null ? null : network.copy(); 2291 if (contract != null) { 2292 dst.contract = new ArrayList<Reference>(); 2293 for (Reference i : contract) 2294 dst.contract.add(i.copy()); 2295 }; 2296 return dst; 2297 } 2298 2299 protected Coverage typedCopy() { 2300 return copy(); 2301 } 2302 2303 @Override 2304 public boolean equalsDeep(Base other_) { 2305 if (!super.equalsDeep(other_)) 2306 return false; 2307 if (!(other_ instanceof Coverage)) 2308 return false; 2309 Coverage o = (Coverage) other_; 2310 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 2311 && compareDeep(policyHolder, o.policyHolder, true) && compareDeep(subscriber, o.subscriber, true) 2312 && compareDeep(subscriberId, o.subscriberId, true) && compareDeep(beneficiary, o.beneficiary, true) 2313 && compareDeep(relationship, o.relationship, true) && compareDeep(period, o.period, true) && compareDeep(payor, o.payor, true) 2314 && compareDeep(grouping, o.grouping, true) && compareDeep(dependent, o.dependent, true) && compareDeep(sequence, o.sequence, true) 2315 && compareDeep(order, o.order, true) && compareDeep(network, o.network, true) && compareDeep(contract, o.contract, true) 2316 ; 2317 } 2318 2319 @Override 2320 public boolean equalsShallow(Base other_) { 2321 if (!super.equalsShallow(other_)) 2322 return false; 2323 if (!(other_ instanceof Coverage)) 2324 return false; 2325 Coverage o = (Coverage) other_; 2326 return compareValues(status, o.status, true) && compareValues(subscriberId, o.subscriberId, true) && compareValues(dependent, o.dependent, true) 2327 && compareValues(sequence, o.sequence, true) && compareValues(order, o.order, true) && compareValues(network, o.network, true) 2328 ; 2329 } 2330 2331 public boolean isEmpty() { 2332 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 2333 , policyHolder, subscriber, subscriberId, beneficiary, relationship, period, payor 2334 , grouping, dependent, sequence, order, network, contract); 2335 } 2336 2337 @Override 2338 public ResourceType getResourceType() { 2339 return ResourceType.Coverage; 2340 } 2341 2342 /** 2343 * Search parameter: <b>identifier</b> 2344 * <p> 2345 * Description: <b>The primary identifier of the insured and the coverage</b><br> 2346 * Type: <b>token</b><br> 2347 * Path: <b>Coverage.identifier</b><br> 2348 * </p> 2349 */ 2350 @SearchParamDefinition(name="identifier", path="Coverage.identifier", description="The primary identifier of the insured and the coverage", type="token" ) 2351 public static final String SP_IDENTIFIER = "identifier"; 2352 /** 2353 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2354 * <p> 2355 * Description: <b>The primary identifier of the insured and the coverage</b><br> 2356 * Type: <b>token</b><br> 2357 * Path: <b>Coverage.identifier</b><br> 2358 * </p> 2359 */ 2360 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2361 2362 /** 2363 * Search parameter: <b>subgroup</b> 2364 * <p> 2365 * Description: <b>Sub-group identifier</b><br> 2366 * Type: <b>string</b><br> 2367 * Path: <b>Coverage.grouping.subGroup</b><br> 2368 * </p> 2369 */ 2370 @SearchParamDefinition(name="subgroup", path="Coverage.grouping.subGroup", description="Sub-group identifier", type="string" ) 2371 public static final String SP_SUBGROUP = "subgroup"; 2372 /** 2373 * <b>Fluent Client</b> search parameter constant for <b>subgroup</b> 2374 * <p> 2375 * Description: <b>Sub-group identifier</b><br> 2376 * Type: <b>string</b><br> 2377 * Path: <b>Coverage.grouping.subGroup</b><br> 2378 * </p> 2379 */ 2380 public static final ca.uhn.fhir.rest.gclient.StringClientParam SUBGROUP = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SUBGROUP); 2381 2382 /** 2383 * Search parameter: <b>subscriber</b> 2384 * <p> 2385 * Description: <b>Reference to the subscriber</b><br> 2386 * Type: <b>reference</b><br> 2387 * Path: <b>Coverage.subscriber</b><br> 2388 * </p> 2389 */ 2390 @SearchParamDefinition(name="subscriber", path="Coverage.subscriber", description="Reference to the subscriber", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, RelatedPerson.class } ) 2391 public static final String SP_SUBSCRIBER = "subscriber"; 2392 /** 2393 * <b>Fluent Client</b> search parameter constant for <b>subscriber</b> 2394 * <p> 2395 * Description: <b>Reference to the subscriber</b><br> 2396 * Type: <b>reference</b><br> 2397 * Path: <b>Coverage.subscriber</b><br> 2398 * </p> 2399 */ 2400 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSCRIBER); 2401 2402/** 2403 * Constant for fluent queries to be used to add include statements. Specifies 2404 * the path value of "<b>Coverage:subscriber</b>". 2405 */ 2406 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSCRIBER = new ca.uhn.fhir.model.api.Include("Coverage:subscriber").toLocked(); 2407 2408 /** 2409 * Search parameter: <b>subplan</b> 2410 * <p> 2411 * Description: <b>Sub-plan identifier</b><br> 2412 * Type: <b>string</b><br> 2413 * Path: <b>Coverage.grouping.subPlan</b><br> 2414 * </p> 2415 */ 2416 @SearchParamDefinition(name="subplan", path="Coverage.grouping.subPlan", description="Sub-plan identifier", type="string" ) 2417 public static final String SP_SUBPLAN = "subplan"; 2418 /** 2419 * <b>Fluent Client</b> search parameter constant for <b>subplan</b> 2420 * <p> 2421 * Description: <b>Sub-plan identifier</b><br> 2422 * Type: <b>string</b><br> 2423 * Path: <b>Coverage.grouping.subPlan</b><br> 2424 * </p> 2425 */ 2426 public static final ca.uhn.fhir.rest.gclient.StringClientParam SUBPLAN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SUBPLAN); 2427 2428 /** 2429 * Search parameter: <b>type</b> 2430 * <p> 2431 * Description: <b>The kind of coverage (health plan, auto, Workers Compensation)</b><br> 2432 * Type: <b>token</b><br> 2433 * Path: <b>Coverage.type</b><br> 2434 * </p> 2435 */ 2436 @SearchParamDefinition(name="type", path="Coverage.type", description="The kind of coverage (health plan, auto, Workers Compensation)", type="token" ) 2437 public static final String SP_TYPE = "type"; 2438 /** 2439 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2440 * <p> 2441 * Description: <b>The kind of coverage (health plan, auto, Workers Compensation)</b><br> 2442 * Type: <b>token</b><br> 2443 * Path: <b>Coverage.type</b><br> 2444 * </p> 2445 */ 2446 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2447 2448 /** 2449 * Search parameter: <b>sequence</b> 2450 * <p> 2451 * Description: <b>Sequence number</b><br> 2452 * Type: <b>string</b><br> 2453 * Path: <b>Coverage.sequence</b><br> 2454 * </p> 2455 */ 2456 @SearchParamDefinition(name="sequence", path="Coverage.sequence", description="Sequence number", type="string" ) 2457 public static final String SP_SEQUENCE = "sequence"; 2458 /** 2459 * <b>Fluent Client</b> search parameter constant for <b>sequence</b> 2460 * <p> 2461 * Description: <b>Sequence number</b><br> 2462 * Type: <b>string</b><br> 2463 * Path: <b>Coverage.sequence</b><br> 2464 * </p> 2465 */ 2466 public static final ca.uhn.fhir.rest.gclient.StringClientParam SEQUENCE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SEQUENCE); 2467 2468 /** 2469 * Search parameter: <b>payor</b> 2470 * <p> 2471 * Description: <b>The identity of the insurer or party paying for services</b><br> 2472 * Type: <b>reference</b><br> 2473 * Path: <b>Coverage.payor</b><br> 2474 * </p> 2475 */ 2476 @SearchParamDefinition(name="payor", path="Coverage.payor", description="The identity of the insurer or party paying for services", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 2477 public static final String SP_PAYOR = "payor"; 2478 /** 2479 * <b>Fluent Client</b> search parameter constant for <b>payor</b> 2480 * <p> 2481 * Description: <b>The identity of the insurer or party paying for services</b><br> 2482 * Type: <b>reference</b><br> 2483 * Path: <b>Coverage.payor</b><br> 2484 * </p> 2485 */ 2486 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYOR); 2487 2488/** 2489 * Constant for fluent queries to be used to add include statements. Specifies 2490 * the path value of "<b>Coverage:payor</b>". 2491 */ 2492 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYOR = new ca.uhn.fhir.model.api.Include("Coverage:payor").toLocked(); 2493 2494 /** 2495 * Search parameter: <b>beneficiary</b> 2496 * <p> 2497 * Description: <b>Covered party</b><br> 2498 * Type: <b>reference</b><br> 2499 * Path: <b>Coverage.beneficiary</b><br> 2500 * </p> 2501 */ 2502 @SearchParamDefinition(name="beneficiary", path="Coverage.beneficiary", description="Covered party", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2503 public static final String SP_BENEFICIARY = "beneficiary"; 2504 /** 2505 * <b>Fluent Client</b> search parameter constant for <b>beneficiary</b> 2506 * <p> 2507 * Description: <b>Covered party</b><br> 2508 * Type: <b>reference</b><br> 2509 * Path: <b>Coverage.beneficiary</b><br> 2510 * </p> 2511 */ 2512 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BENEFICIARY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BENEFICIARY); 2513 2514/** 2515 * Constant for fluent queries to be used to add include statements. Specifies 2516 * the path value of "<b>Coverage:beneficiary</b>". 2517 */ 2518 public static final ca.uhn.fhir.model.api.Include INCLUDE_BENEFICIARY = new ca.uhn.fhir.model.api.Include("Coverage:beneficiary").toLocked(); 2519 2520 /** 2521 * Search parameter: <b>subclass</b> 2522 * <p> 2523 * Description: <b>Sub-class identifier</b><br> 2524 * Type: <b>string</b><br> 2525 * Path: <b>Coverage.grouping.subClass</b><br> 2526 * </p> 2527 */ 2528 @SearchParamDefinition(name="subclass", path="Coverage.grouping.subClass", description="Sub-class identifier", type="string" ) 2529 public static final String SP_SUBCLASS = "subclass"; 2530 /** 2531 * <b>Fluent Client</b> search parameter constant for <b>subclass</b> 2532 * <p> 2533 * Description: <b>Sub-class identifier</b><br> 2534 * Type: <b>string</b><br> 2535 * Path: <b>Coverage.grouping.subClass</b><br> 2536 * </p> 2537 */ 2538 public static final ca.uhn.fhir.rest.gclient.StringClientParam SUBCLASS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SUBCLASS); 2539 2540 /** 2541 * Search parameter: <b>plan</b> 2542 * <p> 2543 * Description: <b>A plan or policy identifier</b><br> 2544 * Type: <b>string</b><br> 2545 * Path: <b>Coverage.grouping.plan</b><br> 2546 * </p> 2547 */ 2548 @SearchParamDefinition(name="plan", path="Coverage.grouping.plan", description="A plan or policy identifier", type="string" ) 2549 public static final String SP_PLAN = "plan"; 2550 /** 2551 * <b>Fluent Client</b> search parameter constant for <b>plan</b> 2552 * <p> 2553 * Description: <b>A plan or policy identifier</b><br> 2554 * Type: <b>string</b><br> 2555 * Path: <b>Coverage.grouping.plan</b><br> 2556 * </p> 2557 */ 2558 public static final ca.uhn.fhir.rest.gclient.StringClientParam PLAN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PLAN); 2559 2560 /** 2561 * Search parameter: <b>class</b> 2562 * <p> 2563 * Description: <b>Class identifier</b><br> 2564 * Type: <b>string</b><br> 2565 * Path: <b>Coverage.grouping.class</b><br> 2566 * </p> 2567 */ 2568 @SearchParamDefinition(name="class", path="Coverage.grouping.class", description="Class identifier", type="string" ) 2569 public static final String SP_CLASS = "class"; 2570 /** 2571 * <b>Fluent Client</b> search parameter constant for <b>class</b> 2572 * <p> 2573 * Description: <b>Class identifier</b><br> 2574 * Type: <b>string</b><br> 2575 * Path: <b>Coverage.grouping.class</b><br> 2576 * </p> 2577 */ 2578 public static final ca.uhn.fhir.rest.gclient.StringClientParam CLASS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_CLASS); 2579 2580 /** 2581 * Search parameter: <b>dependent</b> 2582 * <p> 2583 * Description: <b>Dependent number</b><br> 2584 * Type: <b>string</b><br> 2585 * Path: <b>Coverage.dependent</b><br> 2586 * </p> 2587 */ 2588 @SearchParamDefinition(name="dependent", path="Coverage.dependent", description="Dependent number", type="string" ) 2589 public static final String SP_DEPENDENT = "dependent"; 2590 /** 2591 * <b>Fluent Client</b> search parameter constant for <b>dependent</b> 2592 * <p> 2593 * Description: <b>Dependent number</b><br> 2594 * Type: <b>string</b><br> 2595 * Path: <b>Coverage.dependent</b><br> 2596 * </p> 2597 */ 2598 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEPENDENT = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DEPENDENT); 2599 2600 /** 2601 * Search parameter: <b>group</b> 2602 * <p> 2603 * Description: <b>Group identifier</b><br> 2604 * Type: <b>string</b><br> 2605 * Path: <b>Coverage.grouping.group</b><br> 2606 * </p> 2607 */ 2608 @SearchParamDefinition(name="group", path="Coverage.grouping.group", description="Group identifier", type="string" ) 2609 public static final String SP_GROUP = "group"; 2610 /** 2611 * <b>Fluent Client</b> search parameter constant for <b>group</b> 2612 * <p> 2613 * Description: <b>Group identifier</b><br> 2614 * Type: <b>string</b><br> 2615 * Path: <b>Coverage.grouping.group</b><br> 2616 * </p> 2617 */ 2618 public static final ca.uhn.fhir.rest.gclient.StringClientParam GROUP = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GROUP); 2619 2620 /** 2621 * Search parameter: <b>policy-holder</b> 2622 * <p> 2623 * Description: <b>Reference to the policyholder</b><br> 2624 * Type: <b>reference</b><br> 2625 * Path: <b>Coverage.policyHolder</b><br> 2626 * </p> 2627 */ 2628 @SearchParamDefinition(name="policy-holder", path="Coverage.policyHolder", description="Reference to the policyholder", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 2629 public static final String SP_POLICY_HOLDER = "policy-holder"; 2630 /** 2631 * <b>Fluent Client</b> search parameter constant for <b>policy-holder</b> 2632 * <p> 2633 * Description: <b>Reference to the policyholder</b><br> 2634 * Type: <b>reference</b><br> 2635 * Path: <b>Coverage.policyHolder</b><br> 2636 * </p> 2637 */ 2638 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam POLICY_HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_POLICY_HOLDER); 2639 2640/** 2641 * Constant for fluent queries to be used to add include statements. Specifies 2642 * the path value of "<b>Coverage:policy-holder</b>". 2643 */ 2644 public static final ca.uhn.fhir.model.api.Include INCLUDE_POLICY_HOLDER = new ca.uhn.fhir.model.api.Include("Coverage:policy-holder").toLocked(); 2645 2646 2647}