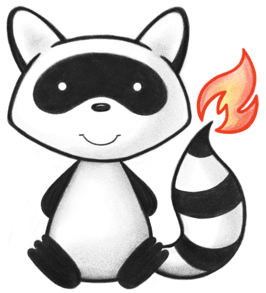
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * The formal description of a single piece of information that can be gathered and reported. 053 */ 054@ResourceDef(name="DataElement", profile="http://hl7.org/fhir/Profile/DataElement") 055@ChildOrder(names={"url", "identifier", "version", "status", "experimental", "date", "publisher", "name", "title", "contact", "useContext", "jurisdiction", "copyright", "stringency", "mapping", "element"}) 056public class DataElement extends MetadataResource { 057 058 public enum DataElementStringency { 059 /** 060 * The data element is sufficiently well-constrained that multiple pieces of data captured according to the constraints of the data element will be comparable (though in some cases, a degree of automated conversion/normalization may be required). 061 */ 062 COMPARABLE, 063 /** 064 * The data element is fully specified down to a single value set, single unit of measure, single data type, etc. Multiple pieces of data associated with this data element are fully comparable. 065 */ 066 FULLYSPECIFIED, 067 /** 068 * The data element allows multiple units of measure having equivalent meaning; e.g. "cc" (cubic centimeter) and "mL" (milliliter). 069 */ 070 EQUIVALENT, 071 /** 072 * The data element allows multiple units of measure that are convertable between each other (e.g. inches and centimeters) and/or allows data to be captured in multiple value sets for which a known mapping exists allowing conversion of meaning. 073 */ 074 CONVERTABLE, 075 /** 076 * A convertable data element where unit conversions are different only by a power of 10; e.g. g, mg, kg. 077 */ 078 SCALEABLE, 079 /** 080 * The data element is unconstrained in units, choice of data types and/or choice of vocabulary such that automated comparison of data captured using the data element is not possible. 081 */ 082 FLEXIBLE, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static DataElementStringency fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("comparable".equals(codeString)) 091 return COMPARABLE; 092 if ("fully-specified".equals(codeString)) 093 return FULLYSPECIFIED; 094 if ("equivalent".equals(codeString)) 095 return EQUIVALENT; 096 if ("convertable".equals(codeString)) 097 return CONVERTABLE; 098 if ("scaleable".equals(codeString)) 099 return SCALEABLE; 100 if ("flexible".equals(codeString)) 101 return FLEXIBLE; 102 if (Configuration.isAcceptInvalidEnums()) 103 return null; 104 else 105 throw new FHIRException("Unknown DataElementStringency code '"+codeString+"'"); 106 } 107 public String toCode() { 108 switch (this) { 109 case COMPARABLE: return "comparable"; 110 case FULLYSPECIFIED: return "fully-specified"; 111 case EQUIVALENT: return "equivalent"; 112 case CONVERTABLE: return "convertable"; 113 case SCALEABLE: return "scaleable"; 114 case FLEXIBLE: return "flexible"; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getSystem() { 120 switch (this) { 121 case COMPARABLE: return "http://hl7.org/fhir/dataelement-stringency"; 122 case FULLYSPECIFIED: return "http://hl7.org/fhir/dataelement-stringency"; 123 case EQUIVALENT: return "http://hl7.org/fhir/dataelement-stringency"; 124 case CONVERTABLE: return "http://hl7.org/fhir/dataelement-stringency"; 125 case SCALEABLE: return "http://hl7.org/fhir/dataelement-stringency"; 126 case FLEXIBLE: return "http://hl7.org/fhir/dataelement-stringency"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getDefinition() { 132 switch (this) { 133 case COMPARABLE: return "The data element is sufficiently well-constrained that multiple pieces of data captured according to the constraints of the data element will be comparable (though in some cases, a degree of automated conversion/normalization may be required)."; 134 case FULLYSPECIFIED: return "The data element is fully specified down to a single value set, single unit of measure, single data type, etc. Multiple pieces of data associated with this data element are fully comparable."; 135 case EQUIVALENT: return "The data element allows multiple units of measure having equivalent meaning; e.g. \"cc\" (cubic centimeter) and \"mL\" (milliliter)."; 136 case CONVERTABLE: return "The data element allows multiple units of measure that are convertable between each other (e.g. inches and centimeters) and/or allows data to be captured in multiple value sets for which a known mapping exists allowing conversion of meaning."; 137 case SCALEABLE: return "A convertable data element where unit conversions are different only by a power of 10; e.g. g, mg, kg."; 138 case FLEXIBLE: return "The data element is unconstrained in units, choice of data types and/or choice of vocabulary such that automated comparison of data captured using the data element is not possible."; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDisplay() { 144 switch (this) { 145 case COMPARABLE: return "Comparable"; 146 case FULLYSPECIFIED: return "Fully Specified"; 147 case EQUIVALENT: return "Equivalent"; 148 case CONVERTABLE: return "Convertable"; 149 case SCALEABLE: return "Scaleable"; 150 case FLEXIBLE: return "Flexible"; 151 case NULL: return null; 152 default: return "?"; 153 } 154 } 155 } 156 157 public static class DataElementStringencyEnumFactory implements EnumFactory<DataElementStringency> { 158 public DataElementStringency fromCode(String codeString) throws IllegalArgumentException { 159 if (codeString == null || "".equals(codeString)) 160 if (codeString == null || "".equals(codeString)) 161 return null; 162 if ("comparable".equals(codeString)) 163 return DataElementStringency.COMPARABLE; 164 if ("fully-specified".equals(codeString)) 165 return DataElementStringency.FULLYSPECIFIED; 166 if ("equivalent".equals(codeString)) 167 return DataElementStringency.EQUIVALENT; 168 if ("convertable".equals(codeString)) 169 return DataElementStringency.CONVERTABLE; 170 if ("scaleable".equals(codeString)) 171 return DataElementStringency.SCALEABLE; 172 if ("flexible".equals(codeString)) 173 return DataElementStringency.FLEXIBLE; 174 throw new IllegalArgumentException("Unknown DataElementStringency code '"+codeString+"'"); 175 } 176 public Enumeration<DataElementStringency> fromType(PrimitiveType<?> code) throws FHIRException { 177 if (code == null) 178 return null; 179 if (code.isEmpty()) 180 return new Enumeration<DataElementStringency>(this); 181 String codeString = code.asStringValue(); 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("comparable".equals(codeString)) 185 return new Enumeration<DataElementStringency>(this, DataElementStringency.COMPARABLE); 186 if ("fully-specified".equals(codeString)) 187 return new Enumeration<DataElementStringency>(this, DataElementStringency.FULLYSPECIFIED); 188 if ("equivalent".equals(codeString)) 189 return new Enumeration<DataElementStringency>(this, DataElementStringency.EQUIVALENT); 190 if ("convertable".equals(codeString)) 191 return new Enumeration<DataElementStringency>(this, DataElementStringency.CONVERTABLE); 192 if ("scaleable".equals(codeString)) 193 return new Enumeration<DataElementStringency>(this, DataElementStringency.SCALEABLE); 194 if ("flexible".equals(codeString)) 195 return new Enumeration<DataElementStringency>(this, DataElementStringency.FLEXIBLE); 196 throw new FHIRException("Unknown DataElementStringency code '"+codeString+"'"); 197 } 198 public String toCode(DataElementStringency code) { 199 if (code == DataElementStringency.NULL) 200 return null; 201 if (code == DataElementStringency.COMPARABLE) 202 return "comparable"; 203 if (code == DataElementStringency.FULLYSPECIFIED) 204 return "fully-specified"; 205 if (code == DataElementStringency.EQUIVALENT) 206 return "equivalent"; 207 if (code == DataElementStringency.CONVERTABLE) 208 return "convertable"; 209 if (code == DataElementStringency.SCALEABLE) 210 return "scaleable"; 211 if (code == DataElementStringency.FLEXIBLE) 212 return "flexible"; 213 return "?"; 214 } 215 public String toSystem(DataElementStringency code) { 216 return code.getSystem(); 217 } 218 } 219 220 @Block() 221 public static class DataElementMappingComponent extends BackboneElement implements IBaseBackboneElement { 222 /** 223 * An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis. 224 */ 225 @Child(name = "identity", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 226 @Description(shortDefinition="Internal id when this mapping is used", formalDefinition="An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis." ) 227 protected IdType identity; 228 229 /** 230 * An absolute URI that identifies the specification that this mapping is expressed to. 231 */ 232 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 233 @Description(shortDefinition="Identifies what this mapping refers to", formalDefinition="An absolute URI that identifies the specification that this mapping is expressed to." ) 234 protected UriType uri; 235 236 /** 237 * A name for the specification that is being mapped to. 238 */ 239 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 240 @Description(shortDefinition="Names what this mapping refers to", formalDefinition="A name for the specification that is being mapped to." ) 241 protected StringType name; 242 243 /** 244 * Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 245 */ 246 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 247 @Description(shortDefinition="Versions, issues, scope limitations, etc.", formalDefinition="Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage." ) 248 protected StringType comment; 249 250 private static final long serialVersionUID = 9610265L; 251 252 /** 253 * Constructor 254 */ 255 public DataElementMappingComponent() { 256 super(); 257 } 258 259 /** 260 * Constructor 261 */ 262 public DataElementMappingComponent(IdType identity) { 263 super(); 264 this.identity = identity; 265 } 266 267 /** 268 * @return {@link #identity} (An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 269 */ 270 public IdType getIdentityElement() { 271 if (this.identity == null) 272 if (Configuration.errorOnAutoCreate()) 273 throw new Error("Attempt to auto-create DataElementMappingComponent.identity"); 274 else if (Configuration.doAutoCreate()) 275 this.identity = new IdType(); // bb 276 return this.identity; 277 } 278 279 public boolean hasIdentityElement() { 280 return this.identity != null && !this.identity.isEmpty(); 281 } 282 283 public boolean hasIdentity() { 284 return this.identity != null && !this.identity.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #identity} (An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 289 */ 290 public DataElementMappingComponent setIdentityElement(IdType value) { 291 this.identity = value; 292 return this; 293 } 294 295 /** 296 * @return An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis. 297 */ 298 public String getIdentity() { 299 return this.identity == null ? null : this.identity.getValue(); 300 } 301 302 /** 303 * @param value An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis. 304 */ 305 public DataElementMappingComponent setIdentity(String value) { 306 if (this.identity == null) 307 this.identity = new IdType(); 308 this.identity.setValue(value); 309 return this; 310 } 311 312 /** 313 * @return {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 314 */ 315 public UriType getUriElement() { 316 if (this.uri == null) 317 if (Configuration.errorOnAutoCreate()) 318 throw new Error("Attempt to auto-create DataElementMappingComponent.uri"); 319 else if (Configuration.doAutoCreate()) 320 this.uri = new UriType(); // bb 321 return this.uri; 322 } 323 324 public boolean hasUriElement() { 325 return this.uri != null && !this.uri.isEmpty(); 326 } 327 328 public boolean hasUri() { 329 return this.uri != null && !this.uri.isEmpty(); 330 } 331 332 /** 333 * @param value {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 334 */ 335 public DataElementMappingComponent setUriElement(UriType value) { 336 this.uri = value; 337 return this; 338 } 339 340 /** 341 * @return An absolute URI that identifies the specification that this mapping is expressed to. 342 */ 343 public String getUri() { 344 return this.uri == null ? null : this.uri.getValue(); 345 } 346 347 /** 348 * @param value An absolute URI that identifies the specification that this mapping is expressed to. 349 */ 350 public DataElementMappingComponent setUri(String value) { 351 if (Utilities.noString(value)) 352 this.uri = null; 353 else { 354 if (this.uri == null) 355 this.uri = new UriType(); 356 this.uri.setValue(value); 357 } 358 return this; 359 } 360 361 /** 362 * @return {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 363 */ 364 public StringType getNameElement() { 365 if (this.name == null) 366 if (Configuration.errorOnAutoCreate()) 367 throw new Error("Attempt to auto-create DataElementMappingComponent.name"); 368 else if (Configuration.doAutoCreate()) 369 this.name = new StringType(); // bb 370 return this.name; 371 } 372 373 public boolean hasNameElement() { 374 return this.name != null && !this.name.isEmpty(); 375 } 376 377 public boolean hasName() { 378 return this.name != null && !this.name.isEmpty(); 379 } 380 381 /** 382 * @param value {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 383 */ 384 public DataElementMappingComponent setNameElement(StringType value) { 385 this.name = value; 386 return this; 387 } 388 389 /** 390 * @return A name for the specification that is being mapped to. 391 */ 392 public String getName() { 393 return this.name == null ? null : this.name.getValue(); 394 } 395 396 /** 397 * @param value A name for the specification that is being mapped to. 398 */ 399 public DataElementMappingComponent setName(String value) { 400 if (Utilities.noString(value)) 401 this.name = null; 402 else { 403 if (this.name == null) 404 this.name = new StringType(); 405 this.name.setValue(value); 406 } 407 return this; 408 } 409 410 /** 411 * @return {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 412 */ 413 public StringType getCommentElement() { 414 if (this.comment == null) 415 if (Configuration.errorOnAutoCreate()) 416 throw new Error("Attempt to auto-create DataElementMappingComponent.comment"); 417 else if (Configuration.doAutoCreate()) 418 this.comment = new StringType(); // bb 419 return this.comment; 420 } 421 422 public boolean hasCommentElement() { 423 return this.comment != null && !this.comment.isEmpty(); 424 } 425 426 public boolean hasComment() { 427 return this.comment != null && !this.comment.isEmpty(); 428 } 429 430 /** 431 * @param value {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 432 */ 433 public DataElementMappingComponent setCommentElement(StringType value) { 434 this.comment = value; 435 return this; 436 } 437 438 /** 439 * @return Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 440 */ 441 public String getComment() { 442 return this.comment == null ? null : this.comment.getValue(); 443 } 444 445 /** 446 * @param value Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 447 */ 448 public DataElementMappingComponent setComment(String value) { 449 if (Utilities.noString(value)) 450 this.comment = null; 451 else { 452 if (this.comment == null) 453 this.comment = new StringType(); 454 this.comment.setValue(value); 455 } 456 return this; 457 } 458 459 protected void listChildren(List<Property> children) { 460 super.listChildren(children); 461 children.add(new Property("identity", "id", "An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.", 0, 1, identity)); 462 children.add(new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri)); 463 children.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name)); 464 children.add(new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment)); 465 } 466 467 @Override 468 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 469 switch (_hash) { 470 case -135761730: /*identity*/ return new Property("identity", "id", "An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.", 0, 1, identity); 471 case 116076: /*uri*/ return new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri); 472 case 3373707: /*name*/ return new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name); 473 case 950398559: /*comment*/ return new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment); 474 default: return super.getNamedProperty(_hash, _name, _checkValid); 475 } 476 477 } 478 479 @Override 480 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 481 switch (hash) { 482 case -135761730: /*identity*/ return this.identity == null ? new Base[0] : new Base[] {this.identity}; // IdType 483 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 484 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 485 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 486 default: return super.getProperty(hash, name, checkValid); 487 } 488 489 } 490 491 @Override 492 public Base setProperty(int hash, String name, Base value) throws FHIRException { 493 switch (hash) { 494 case -135761730: // identity 495 this.identity = castToId(value); // IdType 496 return value; 497 case 116076: // uri 498 this.uri = castToUri(value); // UriType 499 return value; 500 case 3373707: // name 501 this.name = castToString(value); // StringType 502 return value; 503 case 950398559: // comment 504 this.comment = castToString(value); // StringType 505 return value; 506 default: return super.setProperty(hash, name, value); 507 } 508 509 } 510 511 @Override 512 public Base setProperty(String name, Base value) throws FHIRException { 513 if (name.equals("identity")) { 514 this.identity = castToId(value); // IdType 515 } else if (name.equals("uri")) { 516 this.uri = castToUri(value); // UriType 517 } else if (name.equals("name")) { 518 this.name = castToString(value); // StringType 519 } else if (name.equals("comment")) { 520 this.comment = castToString(value); // StringType 521 } else 522 return super.setProperty(name, value); 523 return value; 524 } 525 526 @Override 527 public Base makeProperty(int hash, String name) throws FHIRException { 528 switch (hash) { 529 case -135761730: return getIdentityElement(); 530 case 116076: return getUriElement(); 531 case 3373707: return getNameElement(); 532 case 950398559: return getCommentElement(); 533 default: return super.makeProperty(hash, name); 534 } 535 536 } 537 538 @Override 539 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 540 switch (hash) { 541 case -135761730: /*identity*/ return new String[] {"id"}; 542 case 116076: /*uri*/ return new String[] {"uri"}; 543 case 3373707: /*name*/ return new String[] {"string"}; 544 case 950398559: /*comment*/ return new String[] {"string"}; 545 default: return super.getTypesForProperty(hash, name); 546 } 547 548 } 549 550 @Override 551 public Base addChild(String name) throws FHIRException { 552 if (name.equals("identity")) { 553 throw new FHIRException("Cannot call addChild on a singleton property DataElement.identity"); 554 } 555 else if (name.equals("uri")) { 556 throw new FHIRException("Cannot call addChild on a singleton property DataElement.uri"); 557 } 558 else if (name.equals("name")) { 559 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 560 } 561 else if (name.equals("comment")) { 562 throw new FHIRException("Cannot call addChild on a singleton property DataElement.comment"); 563 } 564 else 565 return super.addChild(name); 566 } 567 568 public DataElementMappingComponent copy() { 569 DataElementMappingComponent dst = new DataElementMappingComponent(); 570 copyValues(dst); 571 dst.identity = identity == null ? null : identity.copy(); 572 dst.uri = uri == null ? null : uri.copy(); 573 dst.name = name == null ? null : name.copy(); 574 dst.comment = comment == null ? null : comment.copy(); 575 return dst; 576 } 577 578 @Override 579 public boolean equalsDeep(Base other_) { 580 if (!super.equalsDeep(other_)) 581 return false; 582 if (!(other_ instanceof DataElementMappingComponent)) 583 return false; 584 DataElementMappingComponent o = (DataElementMappingComponent) other_; 585 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 586 && compareDeep(comment, o.comment, true); 587 } 588 589 @Override 590 public boolean equalsShallow(Base other_) { 591 if (!super.equalsShallow(other_)) 592 return false; 593 if (!(other_ instanceof DataElementMappingComponent)) 594 return false; 595 DataElementMappingComponent o = (DataElementMappingComponent) other_; 596 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) && compareValues(name, o.name, true) 597 && compareValues(comment, o.comment, true); 598 } 599 600 public boolean isEmpty() { 601 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, uri, name, comment 602 ); 603 } 604 605 public String fhirType() { 606 return "DataElement.mapping"; 607 608 } 609 610 } 611 612 /** 613 * A formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance. 614 */ 615 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 616 @Description(shortDefinition="Additional identifier for the data element", formalDefinition="A formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 617 protected List<Identifier> identifier; 618 619 /** 620 * A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element. 621 */ 622 @Child(name = "copyright", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 623 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element." ) 624 protected MarkdownType copyright; 625 626 /** 627 * Identifies how precise the data element is in its definition. 628 */ 629 @Child(name = "stringency", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 630 @Description(shortDefinition="comparable | fully-specified | equivalent | convertable | scaleable | flexible", formalDefinition="Identifies how precise the data element is in its definition." ) 631 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/dataelement-stringency") 632 protected Enumeration<DataElementStringency> stringency; 633 634 /** 635 * Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with. 636 */ 637 @Child(name = "mapping", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 638 @Description(shortDefinition="External specification mapped to", formalDefinition="Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with." ) 639 protected List<DataElementMappingComponent> mapping; 640 641 /** 642 * Defines the structure, type, allowed values and other constraining characteristics of the data element. 643 */ 644 @Child(name = "element", type = {ElementDefinition.class}, order=4, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 645 @Description(shortDefinition="Definition of element", formalDefinition="Defines the structure, type, allowed values and other constraining characteristics of the data element." ) 646 protected List<ElementDefinition> element; 647 648 private static final long serialVersionUID = 634422795L; 649 650 /** 651 * Constructor 652 */ 653 public DataElement() { 654 super(); 655 } 656 657 /** 658 * Constructor 659 */ 660 public DataElement(Enumeration<PublicationStatus> status) { 661 super(); 662 this.status = status; 663 } 664 665 /** 666 * @return {@link #url} (An absolute URI that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published. The URL SHOULD include the major version of the data element. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 667 */ 668 public UriType getUrlElement() { 669 if (this.url == null) 670 if (Configuration.errorOnAutoCreate()) 671 throw new Error("Attempt to auto-create DataElement.url"); 672 else if (Configuration.doAutoCreate()) 673 this.url = new UriType(); // bb 674 return this.url; 675 } 676 677 public boolean hasUrlElement() { 678 return this.url != null && !this.url.isEmpty(); 679 } 680 681 public boolean hasUrl() { 682 return this.url != null && !this.url.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #url} (An absolute URI that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published. The URL SHOULD include the major version of the data element. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 687 */ 688 public DataElement setUrlElement(UriType value) { 689 this.url = value; 690 return this; 691 } 692 693 /** 694 * @return An absolute URI that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published. The URL SHOULD include the major version of the data element. For more information see [Technical and Business Versions](resource.html#versions). 695 */ 696 public String getUrl() { 697 return this.url == null ? null : this.url.getValue(); 698 } 699 700 /** 701 * @param value An absolute URI that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published. The URL SHOULD include the major version of the data element. For more information see [Technical and Business Versions](resource.html#versions). 702 */ 703 public DataElement setUrl(String value) { 704 if (Utilities.noString(value)) 705 this.url = null; 706 else { 707 if (this.url == null) 708 this.url = new UriType(); 709 this.url.setValue(value); 710 } 711 return this; 712 } 713 714 /** 715 * @return {@link #identifier} (A formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance.) 716 */ 717 public List<Identifier> getIdentifier() { 718 if (this.identifier == null) 719 this.identifier = new ArrayList<Identifier>(); 720 return this.identifier; 721 } 722 723 /** 724 * @return Returns a reference to <code>this</code> for easy method chaining 725 */ 726 public DataElement setIdentifier(List<Identifier> theIdentifier) { 727 this.identifier = theIdentifier; 728 return this; 729 } 730 731 public boolean hasIdentifier() { 732 if (this.identifier == null) 733 return false; 734 for (Identifier item : this.identifier) 735 if (!item.isEmpty()) 736 return true; 737 return false; 738 } 739 740 public Identifier addIdentifier() { //3 741 Identifier t = new Identifier(); 742 if (this.identifier == null) 743 this.identifier = new ArrayList<Identifier>(); 744 this.identifier.add(t); 745 return t; 746 } 747 748 public DataElement addIdentifier(Identifier t) { //3 749 if (t == null) 750 return this; 751 if (this.identifier == null) 752 this.identifier = new ArrayList<Identifier>(); 753 this.identifier.add(t); 754 return this; 755 } 756 757 /** 758 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 759 */ 760 public Identifier getIdentifierFirstRep() { 761 if (getIdentifier().isEmpty()) { 762 addIdentifier(); 763 } 764 return getIdentifier().get(0); 765 } 766 767 /** 768 * @return {@link #version} (The identifier that is used to identify this version of the data element when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the data element author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 769 */ 770 public StringType getVersionElement() { 771 if (this.version == null) 772 if (Configuration.errorOnAutoCreate()) 773 throw new Error("Attempt to auto-create DataElement.version"); 774 else if (Configuration.doAutoCreate()) 775 this.version = new StringType(); // bb 776 return this.version; 777 } 778 779 public boolean hasVersionElement() { 780 return this.version != null && !this.version.isEmpty(); 781 } 782 783 public boolean hasVersion() { 784 return this.version != null && !this.version.isEmpty(); 785 } 786 787 /** 788 * @param value {@link #version} (The identifier that is used to identify this version of the data element when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the data element author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 789 */ 790 public DataElement setVersionElement(StringType value) { 791 this.version = value; 792 return this; 793 } 794 795 /** 796 * @return The identifier that is used to identify this version of the data element when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the data element author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 797 */ 798 public String getVersion() { 799 return this.version == null ? null : this.version.getValue(); 800 } 801 802 /** 803 * @param value The identifier that is used to identify this version of the data element when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the data element author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 804 */ 805 public DataElement setVersion(String value) { 806 if (Utilities.noString(value)) 807 this.version = null; 808 else { 809 if (this.version == null) 810 this.version = new StringType(); 811 this.version.setValue(value); 812 } 813 return this; 814 } 815 816 /** 817 * @return {@link #status} (The status of this data element. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 818 */ 819 public Enumeration<PublicationStatus> getStatusElement() { 820 if (this.status == null) 821 if (Configuration.errorOnAutoCreate()) 822 throw new Error("Attempt to auto-create DataElement.status"); 823 else if (Configuration.doAutoCreate()) 824 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 825 return this.status; 826 } 827 828 public boolean hasStatusElement() { 829 return this.status != null && !this.status.isEmpty(); 830 } 831 832 public boolean hasStatus() { 833 return this.status != null && !this.status.isEmpty(); 834 } 835 836 /** 837 * @param value {@link #status} (The status of this data element. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 838 */ 839 public DataElement setStatusElement(Enumeration<PublicationStatus> value) { 840 this.status = value; 841 return this; 842 } 843 844 /** 845 * @return The status of this data element. Enables tracking the life-cycle of the content. 846 */ 847 public PublicationStatus getStatus() { 848 return this.status == null ? null : this.status.getValue(); 849 } 850 851 /** 852 * @param value The status of this data element. Enables tracking the life-cycle of the content. 853 */ 854 public DataElement setStatus(PublicationStatus value) { 855 if (this.status == null) 856 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 857 this.status.setValue(value); 858 return this; 859 } 860 861 /** 862 * @return {@link #experimental} (A boolean value to indicate that this data element is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 863 */ 864 public BooleanType getExperimentalElement() { 865 if (this.experimental == null) 866 if (Configuration.errorOnAutoCreate()) 867 throw new Error("Attempt to auto-create DataElement.experimental"); 868 else if (Configuration.doAutoCreate()) 869 this.experimental = new BooleanType(); // bb 870 return this.experimental; 871 } 872 873 public boolean hasExperimentalElement() { 874 return this.experimental != null && !this.experimental.isEmpty(); 875 } 876 877 public boolean hasExperimental() { 878 return this.experimental != null && !this.experimental.isEmpty(); 879 } 880 881 /** 882 * @param value {@link #experimental} (A boolean value to indicate that this data element is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 883 */ 884 public DataElement setExperimentalElement(BooleanType value) { 885 this.experimental = value; 886 return this; 887 } 888 889 /** 890 * @return A boolean value to indicate that this data element is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 891 */ 892 public boolean getExperimental() { 893 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 894 } 895 896 /** 897 * @param value A boolean value to indicate that this data element is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 898 */ 899 public DataElement setExperimental(boolean value) { 900 if (this.experimental == null) 901 this.experimental = new BooleanType(); 902 this.experimental.setValue(value); 903 return this; 904 } 905 906 /** 907 * @return {@link #date} (The date (and optionally time) when the data element was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 908 */ 909 public DateTimeType getDateElement() { 910 if (this.date == null) 911 if (Configuration.errorOnAutoCreate()) 912 throw new Error("Attempt to auto-create DataElement.date"); 913 else if (Configuration.doAutoCreate()) 914 this.date = new DateTimeType(); // bb 915 return this.date; 916 } 917 918 public boolean hasDateElement() { 919 return this.date != null && !this.date.isEmpty(); 920 } 921 922 public boolean hasDate() { 923 return this.date != null && !this.date.isEmpty(); 924 } 925 926 /** 927 * @param value {@link #date} (The date (and optionally time) when the data element was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 928 */ 929 public DataElement setDateElement(DateTimeType value) { 930 this.date = value; 931 return this; 932 } 933 934 /** 935 * @return The date (and optionally time) when the data element was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes. 936 */ 937 public Date getDate() { 938 return this.date == null ? null : this.date.getValue(); 939 } 940 941 /** 942 * @param value The date (and optionally time) when the data element was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes. 943 */ 944 public DataElement setDate(Date value) { 945 if (value == null) 946 this.date = null; 947 else { 948 if (this.date == null) 949 this.date = new DateTimeType(); 950 this.date.setValue(value); 951 } 952 return this; 953 } 954 955 /** 956 * @return {@link #publisher} (The name of the individual or organization that published the data element.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 957 */ 958 public StringType getPublisherElement() { 959 if (this.publisher == null) 960 if (Configuration.errorOnAutoCreate()) 961 throw new Error("Attempt to auto-create DataElement.publisher"); 962 else if (Configuration.doAutoCreate()) 963 this.publisher = new StringType(); // bb 964 return this.publisher; 965 } 966 967 public boolean hasPublisherElement() { 968 return this.publisher != null && !this.publisher.isEmpty(); 969 } 970 971 public boolean hasPublisher() { 972 return this.publisher != null && !this.publisher.isEmpty(); 973 } 974 975 /** 976 * @param value {@link #publisher} (The name of the individual or organization that published the data element.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 977 */ 978 public DataElement setPublisherElement(StringType value) { 979 this.publisher = value; 980 return this; 981 } 982 983 /** 984 * @return The name of the individual or organization that published the data element. 985 */ 986 public String getPublisher() { 987 return this.publisher == null ? null : this.publisher.getValue(); 988 } 989 990 /** 991 * @param value The name of the individual or organization that published the data element. 992 */ 993 public DataElement setPublisher(String value) { 994 if (Utilities.noString(value)) 995 this.publisher = null; 996 else { 997 if (this.publisher == null) 998 this.publisher = new StringType(); 999 this.publisher.setValue(value); 1000 } 1001 return this; 1002 } 1003 1004 /** 1005 * @return {@link #name} (A natural language name identifying the data element. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1006 */ 1007 public StringType getNameElement() { 1008 if (this.name == null) 1009 if (Configuration.errorOnAutoCreate()) 1010 throw new Error("Attempt to auto-create DataElement.name"); 1011 else if (Configuration.doAutoCreate()) 1012 this.name = new StringType(); // bb 1013 return this.name; 1014 } 1015 1016 public boolean hasNameElement() { 1017 return this.name != null && !this.name.isEmpty(); 1018 } 1019 1020 public boolean hasName() { 1021 return this.name != null && !this.name.isEmpty(); 1022 } 1023 1024 /** 1025 * @param value {@link #name} (A natural language name identifying the data element. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1026 */ 1027 public DataElement setNameElement(StringType value) { 1028 this.name = value; 1029 return this; 1030 } 1031 1032 /** 1033 * @return A natural language name identifying the data element. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1034 */ 1035 public String getName() { 1036 return this.name == null ? null : this.name.getValue(); 1037 } 1038 1039 /** 1040 * @param value A natural language name identifying the data element. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1041 */ 1042 public DataElement setName(String value) { 1043 if (Utilities.noString(value)) 1044 this.name = null; 1045 else { 1046 if (this.name == null) 1047 this.name = new StringType(); 1048 this.name.setValue(value); 1049 } 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #title} (A short, descriptive, user-friendly title for the data element.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1055 */ 1056 public StringType getTitleElement() { 1057 if (this.title == null) 1058 if (Configuration.errorOnAutoCreate()) 1059 throw new Error("Attempt to auto-create DataElement.title"); 1060 else if (Configuration.doAutoCreate()) 1061 this.title = new StringType(); // bb 1062 return this.title; 1063 } 1064 1065 public boolean hasTitleElement() { 1066 return this.title != null && !this.title.isEmpty(); 1067 } 1068 1069 public boolean hasTitle() { 1070 return this.title != null && !this.title.isEmpty(); 1071 } 1072 1073 /** 1074 * @param value {@link #title} (A short, descriptive, user-friendly title for the data element.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1075 */ 1076 public DataElement setTitleElement(StringType value) { 1077 this.title = value; 1078 return this; 1079 } 1080 1081 /** 1082 * @return A short, descriptive, user-friendly title for the data element. 1083 */ 1084 public String getTitle() { 1085 return this.title == null ? null : this.title.getValue(); 1086 } 1087 1088 /** 1089 * @param value A short, descriptive, user-friendly title for the data element. 1090 */ 1091 public DataElement setTitle(String value) { 1092 if (Utilities.noString(value)) 1093 this.title = null; 1094 else { 1095 if (this.title == null) 1096 this.title = new StringType(); 1097 this.title.setValue(value); 1098 } 1099 return this; 1100 } 1101 1102 /** 1103 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1104 */ 1105 public List<ContactDetail> getContact() { 1106 if (this.contact == null) 1107 this.contact = new ArrayList<ContactDetail>(); 1108 return this.contact; 1109 } 1110 1111 /** 1112 * @return Returns a reference to <code>this</code> for easy method chaining 1113 */ 1114 public DataElement setContact(List<ContactDetail> theContact) { 1115 this.contact = theContact; 1116 return this; 1117 } 1118 1119 public boolean hasContact() { 1120 if (this.contact == null) 1121 return false; 1122 for (ContactDetail item : this.contact) 1123 if (!item.isEmpty()) 1124 return true; 1125 return false; 1126 } 1127 1128 public ContactDetail addContact() { //3 1129 ContactDetail t = new ContactDetail(); 1130 if (this.contact == null) 1131 this.contact = new ArrayList<ContactDetail>(); 1132 this.contact.add(t); 1133 return t; 1134 } 1135 1136 public DataElement addContact(ContactDetail t) { //3 1137 if (t == null) 1138 return this; 1139 if (this.contact == null) 1140 this.contact = new ArrayList<ContactDetail>(); 1141 this.contact.add(t); 1142 return this; 1143 } 1144 1145 /** 1146 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1147 */ 1148 public ContactDetail getContactFirstRep() { 1149 if (getContact().isEmpty()) { 1150 addContact(); 1151 } 1152 return getContact().get(0); 1153 } 1154 1155 /** 1156 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate data element instances.) 1157 */ 1158 public List<UsageContext> getUseContext() { 1159 if (this.useContext == null) 1160 this.useContext = new ArrayList<UsageContext>(); 1161 return this.useContext; 1162 } 1163 1164 /** 1165 * @return Returns a reference to <code>this</code> for easy method chaining 1166 */ 1167 public DataElement setUseContext(List<UsageContext> theUseContext) { 1168 this.useContext = theUseContext; 1169 return this; 1170 } 1171 1172 public boolean hasUseContext() { 1173 if (this.useContext == null) 1174 return false; 1175 for (UsageContext item : this.useContext) 1176 if (!item.isEmpty()) 1177 return true; 1178 return false; 1179 } 1180 1181 public UsageContext addUseContext() { //3 1182 UsageContext t = new UsageContext(); 1183 if (this.useContext == null) 1184 this.useContext = new ArrayList<UsageContext>(); 1185 this.useContext.add(t); 1186 return t; 1187 } 1188 1189 public DataElement addUseContext(UsageContext t) { //3 1190 if (t == null) 1191 return this; 1192 if (this.useContext == null) 1193 this.useContext = new ArrayList<UsageContext>(); 1194 this.useContext.add(t); 1195 return this; 1196 } 1197 1198 /** 1199 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 1200 */ 1201 public UsageContext getUseContextFirstRep() { 1202 if (getUseContext().isEmpty()) { 1203 addUseContext(); 1204 } 1205 return getUseContext().get(0); 1206 } 1207 1208 /** 1209 * @return {@link #jurisdiction} (A legal or geographic region in which the data element is intended to be used.) 1210 */ 1211 public List<CodeableConcept> getJurisdiction() { 1212 if (this.jurisdiction == null) 1213 this.jurisdiction = new ArrayList<CodeableConcept>(); 1214 return this.jurisdiction; 1215 } 1216 1217 /** 1218 * @return Returns a reference to <code>this</code> for easy method chaining 1219 */ 1220 public DataElement setJurisdiction(List<CodeableConcept> theJurisdiction) { 1221 this.jurisdiction = theJurisdiction; 1222 return this; 1223 } 1224 1225 public boolean hasJurisdiction() { 1226 if (this.jurisdiction == null) 1227 return false; 1228 for (CodeableConcept item : this.jurisdiction) 1229 if (!item.isEmpty()) 1230 return true; 1231 return false; 1232 } 1233 1234 public CodeableConcept addJurisdiction() { //3 1235 CodeableConcept t = new CodeableConcept(); 1236 if (this.jurisdiction == null) 1237 this.jurisdiction = new ArrayList<CodeableConcept>(); 1238 this.jurisdiction.add(t); 1239 return t; 1240 } 1241 1242 public DataElement addJurisdiction(CodeableConcept t) { //3 1243 if (t == null) 1244 return this; 1245 if (this.jurisdiction == null) 1246 this.jurisdiction = new ArrayList<CodeableConcept>(); 1247 this.jurisdiction.add(t); 1248 return this; 1249 } 1250 1251 /** 1252 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1253 */ 1254 public CodeableConcept getJurisdictionFirstRep() { 1255 if (getJurisdiction().isEmpty()) { 1256 addJurisdiction(); 1257 } 1258 return getJurisdiction().get(0); 1259 } 1260 1261 /** 1262 * @return {@link #copyright} (A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1263 */ 1264 public MarkdownType getCopyrightElement() { 1265 if (this.copyright == null) 1266 if (Configuration.errorOnAutoCreate()) 1267 throw new Error("Attempt to auto-create DataElement.copyright"); 1268 else if (Configuration.doAutoCreate()) 1269 this.copyright = new MarkdownType(); // bb 1270 return this.copyright; 1271 } 1272 1273 public boolean hasCopyrightElement() { 1274 return this.copyright != null && !this.copyright.isEmpty(); 1275 } 1276 1277 public boolean hasCopyright() { 1278 return this.copyright != null && !this.copyright.isEmpty(); 1279 } 1280 1281 /** 1282 * @param value {@link #copyright} (A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1283 */ 1284 public DataElement setCopyrightElement(MarkdownType value) { 1285 this.copyright = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element. 1291 */ 1292 public String getCopyright() { 1293 return this.copyright == null ? null : this.copyright.getValue(); 1294 } 1295 1296 /** 1297 * @param value A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element. 1298 */ 1299 public DataElement setCopyright(String value) { 1300 if (value == null) 1301 this.copyright = null; 1302 else { 1303 if (this.copyright == null) 1304 this.copyright = new MarkdownType(); 1305 this.copyright.setValue(value); 1306 } 1307 return this; 1308 } 1309 1310 /** 1311 * @return {@link #stringency} (Identifies how precise the data element is in its definition.). This is the underlying object with id, value and extensions. The accessor "getStringency" gives direct access to the value 1312 */ 1313 public Enumeration<DataElementStringency> getStringencyElement() { 1314 if (this.stringency == null) 1315 if (Configuration.errorOnAutoCreate()) 1316 throw new Error("Attempt to auto-create DataElement.stringency"); 1317 else if (Configuration.doAutoCreate()) 1318 this.stringency = new Enumeration<DataElementStringency>(new DataElementStringencyEnumFactory()); // bb 1319 return this.stringency; 1320 } 1321 1322 public boolean hasStringencyElement() { 1323 return this.stringency != null && !this.stringency.isEmpty(); 1324 } 1325 1326 public boolean hasStringency() { 1327 return this.stringency != null && !this.stringency.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #stringency} (Identifies how precise the data element is in its definition.). This is the underlying object with id, value and extensions. The accessor "getStringency" gives direct access to the value 1332 */ 1333 public DataElement setStringencyElement(Enumeration<DataElementStringency> value) { 1334 this.stringency = value; 1335 return this; 1336 } 1337 1338 /** 1339 * @return Identifies how precise the data element is in its definition. 1340 */ 1341 public DataElementStringency getStringency() { 1342 return this.stringency == null ? null : this.stringency.getValue(); 1343 } 1344 1345 /** 1346 * @param value Identifies how precise the data element is in its definition. 1347 */ 1348 public DataElement setStringency(DataElementStringency value) { 1349 if (value == null) 1350 this.stringency = null; 1351 else { 1352 if (this.stringency == null) 1353 this.stringency = new Enumeration<DataElementStringency>(new DataElementStringencyEnumFactory()); 1354 this.stringency.setValue(value); 1355 } 1356 return this; 1357 } 1358 1359 /** 1360 * @return {@link #mapping} (Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with.) 1361 */ 1362 public List<DataElementMappingComponent> getMapping() { 1363 if (this.mapping == null) 1364 this.mapping = new ArrayList<DataElementMappingComponent>(); 1365 return this.mapping; 1366 } 1367 1368 /** 1369 * @return Returns a reference to <code>this</code> for easy method chaining 1370 */ 1371 public DataElement setMapping(List<DataElementMappingComponent> theMapping) { 1372 this.mapping = theMapping; 1373 return this; 1374 } 1375 1376 public boolean hasMapping() { 1377 if (this.mapping == null) 1378 return false; 1379 for (DataElementMappingComponent item : this.mapping) 1380 if (!item.isEmpty()) 1381 return true; 1382 return false; 1383 } 1384 1385 public DataElementMappingComponent addMapping() { //3 1386 DataElementMappingComponent t = new DataElementMappingComponent(); 1387 if (this.mapping == null) 1388 this.mapping = new ArrayList<DataElementMappingComponent>(); 1389 this.mapping.add(t); 1390 return t; 1391 } 1392 1393 public DataElement addMapping(DataElementMappingComponent t) { //3 1394 if (t == null) 1395 return this; 1396 if (this.mapping == null) 1397 this.mapping = new ArrayList<DataElementMappingComponent>(); 1398 this.mapping.add(t); 1399 return this; 1400 } 1401 1402 /** 1403 * @return The first repetition of repeating field {@link #mapping}, creating it if it does not already exist 1404 */ 1405 public DataElementMappingComponent getMappingFirstRep() { 1406 if (getMapping().isEmpty()) { 1407 addMapping(); 1408 } 1409 return getMapping().get(0); 1410 } 1411 1412 /** 1413 * @return {@link #element} (Defines the structure, type, allowed values and other constraining characteristics of the data element.) 1414 */ 1415 public List<ElementDefinition> getElement() { 1416 if (this.element == null) 1417 this.element = new ArrayList<ElementDefinition>(); 1418 return this.element; 1419 } 1420 1421 /** 1422 * @return Returns a reference to <code>this</code> for easy method chaining 1423 */ 1424 public DataElement setElement(List<ElementDefinition> theElement) { 1425 this.element = theElement; 1426 return this; 1427 } 1428 1429 public boolean hasElement() { 1430 if (this.element == null) 1431 return false; 1432 for (ElementDefinition item : this.element) 1433 if (!item.isEmpty()) 1434 return true; 1435 return false; 1436 } 1437 1438 public ElementDefinition addElement() { //3 1439 ElementDefinition t = new ElementDefinition(); 1440 if (this.element == null) 1441 this.element = new ArrayList<ElementDefinition>(); 1442 this.element.add(t); 1443 return t; 1444 } 1445 1446 public DataElement addElement(ElementDefinition t) { //3 1447 if (t == null) 1448 return this; 1449 if (this.element == null) 1450 this.element = new ArrayList<ElementDefinition>(); 1451 this.element.add(t); 1452 return this; 1453 } 1454 1455 /** 1456 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist 1457 */ 1458 public ElementDefinition getElementFirstRep() { 1459 if (getElement().isEmpty()) { 1460 addElement(); 1461 } 1462 return getElement().get(0); 1463 } 1464 1465 protected void listChildren(List<Property> children) { 1466 super.listChildren(children); 1467 children.add(new Property("url", "uri", "An absolute URI that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published. The URL SHOULD include the major version of the data element. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 1468 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1469 children.add(new Property("version", "string", "The identifier that is used to identify this version of the data element when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the data element author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 1470 children.add(new Property("status", "code", "The status of this data element. Enables tracking the life-cycle of the content.", 0, 1, status)); 1471 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this data element is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 1472 children.add(new Property("date", "dateTime", "The date (and optionally time) when the data element was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.", 0, 1, date)); 1473 children.add(new Property("publisher", "string", "The name of the individual or organization that published the data element.", 0, 1, publisher)); 1474 children.add(new Property("name", "string", "A natural language name identifying the data element. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1475 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the data element.", 0, 1, title)); 1476 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1477 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate data element instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1478 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the data element is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1479 children.add(new Property("copyright", "markdown", "A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element.", 0, 1, copyright)); 1480 children.add(new Property("stringency", "code", "Identifies how precise the data element is in its definition.", 0, 1, stringency)); 1481 children.add(new Property("mapping", "", "Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with.", 0, java.lang.Integer.MAX_VALUE, mapping)); 1482 children.add(new Property("element", "ElementDefinition", "Defines the structure, type, allowed values and other constraining characteristics of the data element.", 0, java.lang.Integer.MAX_VALUE, element)); 1483 } 1484 1485 @Override 1486 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1487 switch (_hash) { 1488 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published. The URL SHOULD include the major version of the data element. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 1489 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 1490 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the data element when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the data element author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 1491 case -892481550: /*status*/ return new Property("status", "code", "The status of this data element. Enables tracking the life-cycle of the content.", 0, 1, status); 1492 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this data element is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 1493 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the data element was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.", 0, 1, date); 1494 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the data element.", 0, 1, publisher); 1495 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the data element. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1496 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the data element.", 0, 1, title); 1497 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1498 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate data element instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1499 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the data element is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1500 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the data element and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the data element.", 0, 1, copyright); 1501 case -1572568464: /*stringency*/ return new Property("stringency", "code", "Identifies how precise the data element is in its definition.", 0, 1, stringency); 1502 case 837556430: /*mapping*/ return new Property("mapping", "", "Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with.", 0, java.lang.Integer.MAX_VALUE, mapping); 1503 case -1662836996: /*element*/ return new Property("element", "ElementDefinition", "Defines the structure, type, allowed values and other constraining characteristics of the data element.", 0, java.lang.Integer.MAX_VALUE, element); 1504 default: return super.getNamedProperty(_hash, _name, _checkValid); 1505 } 1506 1507 } 1508 1509 @Override 1510 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1511 switch (hash) { 1512 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1513 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1514 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1515 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1516 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 1517 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1518 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1519 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1520 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1521 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1522 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1523 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1524 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 1525 case -1572568464: /*stringency*/ return this.stringency == null ? new Base[0] : new Base[] {this.stringency}; // Enumeration<DataElementStringency> 1526 case 837556430: /*mapping*/ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // DataElementMappingComponent 1527 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1528 default: return super.getProperty(hash, name, checkValid); 1529 } 1530 1531 } 1532 1533 @Override 1534 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1535 switch (hash) { 1536 case 116079: // url 1537 this.url = castToUri(value); // UriType 1538 return value; 1539 case -1618432855: // identifier 1540 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1541 return value; 1542 case 351608024: // version 1543 this.version = castToString(value); // StringType 1544 return value; 1545 case -892481550: // status 1546 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1547 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1548 return value; 1549 case -404562712: // experimental 1550 this.experimental = castToBoolean(value); // BooleanType 1551 return value; 1552 case 3076014: // date 1553 this.date = castToDateTime(value); // DateTimeType 1554 return value; 1555 case 1447404028: // publisher 1556 this.publisher = castToString(value); // StringType 1557 return value; 1558 case 3373707: // name 1559 this.name = castToString(value); // StringType 1560 return value; 1561 case 110371416: // title 1562 this.title = castToString(value); // StringType 1563 return value; 1564 case 951526432: // contact 1565 this.getContact().add(castToContactDetail(value)); // ContactDetail 1566 return value; 1567 case -669707736: // useContext 1568 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1569 return value; 1570 case -507075711: // jurisdiction 1571 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1572 return value; 1573 case 1522889671: // copyright 1574 this.copyright = castToMarkdown(value); // MarkdownType 1575 return value; 1576 case -1572568464: // stringency 1577 value = new DataElementStringencyEnumFactory().fromType(castToCode(value)); 1578 this.stringency = (Enumeration) value; // Enumeration<DataElementStringency> 1579 return value; 1580 case 837556430: // mapping 1581 this.getMapping().add((DataElementMappingComponent) value); // DataElementMappingComponent 1582 return value; 1583 case -1662836996: // element 1584 this.getElement().add(castToElementDefinition(value)); // ElementDefinition 1585 return value; 1586 default: return super.setProperty(hash, name, value); 1587 } 1588 1589 } 1590 1591 @Override 1592 public Base setProperty(String name, Base value) throws FHIRException { 1593 if (name.equals("url")) { 1594 this.url = castToUri(value); // UriType 1595 } else if (name.equals("identifier")) { 1596 this.getIdentifier().add(castToIdentifier(value)); 1597 } else if (name.equals("version")) { 1598 this.version = castToString(value); // StringType 1599 } else if (name.equals("status")) { 1600 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1601 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1602 } else if (name.equals("experimental")) { 1603 this.experimental = castToBoolean(value); // BooleanType 1604 } else if (name.equals("date")) { 1605 this.date = castToDateTime(value); // DateTimeType 1606 } else if (name.equals("publisher")) { 1607 this.publisher = castToString(value); // StringType 1608 } else if (name.equals("name")) { 1609 this.name = castToString(value); // StringType 1610 } else if (name.equals("title")) { 1611 this.title = castToString(value); // StringType 1612 } else if (name.equals("contact")) { 1613 this.getContact().add(castToContactDetail(value)); 1614 } else if (name.equals("useContext")) { 1615 this.getUseContext().add(castToUsageContext(value)); 1616 } else if (name.equals("jurisdiction")) { 1617 this.getJurisdiction().add(castToCodeableConcept(value)); 1618 } else if (name.equals("copyright")) { 1619 this.copyright = castToMarkdown(value); // MarkdownType 1620 } else if (name.equals("stringency")) { 1621 value = new DataElementStringencyEnumFactory().fromType(castToCode(value)); 1622 this.stringency = (Enumeration) value; // Enumeration<DataElementStringency> 1623 } else if (name.equals("mapping")) { 1624 this.getMapping().add((DataElementMappingComponent) value); 1625 } else if (name.equals("element")) { 1626 this.getElement().add(castToElementDefinition(value)); 1627 } else 1628 return super.setProperty(name, value); 1629 return value; 1630 } 1631 1632 @Override 1633 public Base makeProperty(int hash, String name) throws FHIRException { 1634 switch (hash) { 1635 case 116079: return getUrlElement(); 1636 case -1618432855: return addIdentifier(); 1637 case 351608024: return getVersionElement(); 1638 case -892481550: return getStatusElement(); 1639 case -404562712: return getExperimentalElement(); 1640 case 3076014: return getDateElement(); 1641 case 1447404028: return getPublisherElement(); 1642 case 3373707: return getNameElement(); 1643 case 110371416: return getTitleElement(); 1644 case 951526432: return addContact(); 1645 case -669707736: return addUseContext(); 1646 case -507075711: return addJurisdiction(); 1647 case 1522889671: return getCopyrightElement(); 1648 case -1572568464: return getStringencyElement(); 1649 case 837556430: return addMapping(); 1650 case -1662836996: return addElement(); 1651 default: return super.makeProperty(hash, name); 1652 } 1653 1654 } 1655 1656 @Override 1657 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1658 switch (hash) { 1659 case 116079: /*url*/ return new String[] {"uri"}; 1660 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1661 case 351608024: /*version*/ return new String[] {"string"}; 1662 case -892481550: /*status*/ return new String[] {"code"}; 1663 case -404562712: /*experimental*/ return new String[] {"boolean"}; 1664 case 3076014: /*date*/ return new String[] {"dateTime"}; 1665 case 1447404028: /*publisher*/ return new String[] {"string"}; 1666 case 3373707: /*name*/ return new String[] {"string"}; 1667 case 110371416: /*title*/ return new String[] {"string"}; 1668 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1669 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 1670 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1671 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 1672 case -1572568464: /*stringency*/ return new String[] {"code"}; 1673 case 837556430: /*mapping*/ return new String[] {}; 1674 case -1662836996: /*element*/ return new String[] {"ElementDefinition"}; 1675 default: return super.getTypesForProperty(hash, name); 1676 } 1677 1678 } 1679 1680 @Override 1681 public Base addChild(String name) throws FHIRException { 1682 if (name.equals("url")) { 1683 throw new FHIRException("Cannot call addChild on a singleton property DataElement.url"); 1684 } 1685 else if (name.equals("identifier")) { 1686 return addIdentifier(); 1687 } 1688 else if (name.equals("version")) { 1689 throw new FHIRException("Cannot call addChild on a singleton property DataElement.version"); 1690 } 1691 else if (name.equals("status")) { 1692 throw new FHIRException("Cannot call addChild on a singleton property DataElement.status"); 1693 } 1694 else if (name.equals("experimental")) { 1695 throw new FHIRException("Cannot call addChild on a singleton property DataElement.experimental"); 1696 } 1697 else if (name.equals("date")) { 1698 throw new FHIRException("Cannot call addChild on a singleton property DataElement.date"); 1699 } 1700 else if (name.equals("publisher")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property DataElement.publisher"); 1702 } 1703 else if (name.equals("name")) { 1704 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 1705 } 1706 else if (name.equals("title")) { 1707 throw new FHIRException("Cannot call addChild on a singleton property DataElement.title"); 1708 } 1709 else if (name.equals("contact")) { 1710 return addContact(); 1711 } 1712 else if (name.equals("useContext")) { 1713 return addUseContext(); 1714 } 1715 else if (name.equals("jurisdiction")) { 1716 return addJurisdiction(); 1717 } 1718 else if (name.equals("copyright")) { 1719 throw new FHIRException("Cannot call addChild on a singleton property DataElement.copyright"); 1720 } 1721 else if (name.equals("stringency")) { 1722 throw new FHIRException("Cannot call addChild on a singleton property DataElement.stringency"); 1723 } 1724 else if (name.equals("mapping")) { 1725 return addMapping(); 1726 } 1727 else if (name.equals("element")) { 1728 return addElement(); 1729 } 1730 else 1731 return super.addChild(name); 1732 } 1733 1734 public String fhirType() { 1735 return "DataElement"; 1736 1737 } 1738 1739 public DataElement copy() { 1740 DataElement dst = new DataElement(); 1741 copyValues(dst); 1742 dst.url = url == null ? null : url.copy(); 1743 if (identifier != null) { 1744 dst.identifier = new ArrayList<Identifier>(); 1745 for (Identifier i : identifier) 1746 dst.identifier.add(i.copy()); 1747 }; 1748 dst.version = version == null ? null : version.copy(); 1749 dst.status = status == null ? null : status.copy(); 1750 dst.experimental = experimental == null ? null : experimental.copy(); 1751 dst.date = date == null ? null : date.copy(); 1752 dst.publisher = publisher == null ? null : publisher.copy(); 1753 dst.name = name == null ? null : name.copy(); 1754 dst.title = title == null ? null : title.copy(); 1755 if (contact != null) { 1756 dst.contact = new ArrayList<ContactDetail>(); 1757 for (ContactDetail i : contact) 1758 dst.contact.add(i.copy()); 1759 }; 1760 if (useContext != null) { 1761 dst.useContext = new ArrayList<UsageContext>(); 1762 for (UsageContext i : useContext) 1763 dst.useContext.add(i.copy()); 1764 }; 1765 if (jurisdiction != null) { 1766 dst.jurisdiction = new ArrayList<CodeableConcept>(); 1767 for (CodeableConcept i : jurisdiction) 1768 dst.jurisdiction.add(i.copy()); 1769 }; 1770 dst.copyright = copyright == null ? null : copyright.copy(); 1771 dst.stringency = stringency == null ? null : stringency.copy(); 1772 if (mapping != null) { 1773 dst.mapping = new ArrayList<DataElementMappingComponent>(); 1774 for (DataElementMappingComponent i : mapping) 1775 dst.mapping.add(i.copy()); 1776 }; 1777 if (element != null) { 1778 dst.element = new ArrayList<ElementDefinition>(); 1779 for (ElementDefinition i : element) 1780 dst.element.add(i.copy()); 1781 }; 1782 return dst; 1783 } 1784 1785 protected DataElement typedCopy() { 1786 return copy(); 1787 } 1788 1789 @Override 1790 public boolean equalsDeep(Base other_) { 1791 if (!super.equalsDeep(other_)) 1792 return false; 1793 if (!(other_ instanceof DataElement)) 1794 return false; 1795 DataElement o = (DataElement) other_; 1796 return compareDeep(identifier, o.identifier, true) && compareDeep(copyright, o.copyright, true) 1797 && compareDeep(stringency, o.stringency, true) && compareDeep(mapping, o.mapping, true) && compareDeep(element, o.element, true) 1798 ; 1799 } 1800 1801 @Override 1802 public boolean equalsShallow(Base other_) { 1803 if (!super.equalsShallow(other_)) 1804 return false; 1805 if (!(other_ instanceof DataElement)) 1806 return false; 1807 DataElement o = (DataElement) other_; 1808 return compareValues(copyright, o.copyright, true) && compareValues(stringency, o.stringency, true) 1809 ; 1810 } 1811 1812 public boolean isEmpty() { 1813 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, copyright, stringency 1814 , mapping, element); 1815 } 1816 1817 @Override 1818 public ResourceType getResourceType() { 1819 return ResourceType.DataElement; 1820 } 1821 1822 /** 1823 * Search parameter: <b>date</b> 1824 * <p> 1825 * Description: <b>The data element publication date</b><br> 1826 * Type: <b>date</b><br> 1827 * Path: <b>DataElement.date</b><br> 1828 * </p> 1829 */ 1830 @SearchParamDefinition(name="date", path="DataElement.date", description="The data element publication date", type="date" ) 1831 public static final String SP_DATE = "date"; 1832 /** 1833 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1834 * <p> 1835 * Description: <b>The data element publication date</b><br> 1836 * Type: <b>date</b><br> 1837 * Path: <b>DataElement.date</b><br> 1838 * </p> 1839 */ 1840 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1841 1842 /** 1843 * Search parameter: <b>identifier</b> 1844 * <p> 1845 * Description: <b>External identifier for the data element</b><br> 1846 * Type: <b>token</b><br> 1847 * Path: <b>DataElement.identifier</b><br> 1848 * </p> 1849 */ 1850 @SearchParamDefinition(name="identifier", path="DataElement.identifier", description="External identifier for the data element", type="token" ) 1851 public static final String SP_IDENTIFIER = "identifier"; 1852 /** 1853 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1854 * <p> 1855 * Description: <b>External identifier for the data element</b><br> 1856 * Type: <b>token</b><br> 1857 * Path: <b>DataElement.identifier</b><br> 1858 * </p> 1859 */ 1860 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1861 1862 /** 1863 * Search parameter: <b>code</b> 1864 * <p> 1865 * Description: <b>A code for the data element (server may choose to do subsumption)</b><br> 1866 * Type: <b>token</b><br> 1867 * Path: <b>DataElement.element.code</b><br> 1868 * </p> 1869 */ 1870 @SearchParamDefinition(name="code", path="DataElement.element.code", description="A code for the data element (server may choose to do subsumption)", type="token" ) 1871 public static final String SP_CODE = "code"; 1872 /** 1873 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1874 * <p> 1875 * Description: <b>A code for the data element (server may choose to do subsumption)</b><br> 1876 * Type: <b>token</b><br> 1877 * Path: <b>DataElement.element.code</b><br> 1878 * </p> 1879 */ 1880 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1881 1882 /** 1883 * Search parameter: <b>stringency</b> 1884 * <p> 1885 * Description: <b>The stringency of the data element definition</b><br> 1886 * Type: <b>token</b><br> 1887 * Path: <b>DataElement.stringency</b><br> 1888 * </p> 1889 */ 1890 @SearchParamDefinition(name="stringency", path="DataElement.stringency", description="The stringency of the data element definition", type="token" ) 1891 public static final String SP_STRINGENCY = "stringency"; 1892 /** 1893 * <b>Fluent Client</b> search parameter constant for <b>stringency</b> 1894 * <p> 1895 * Description: <b>The stringency of the data element definition</b><br> 1896 * Type: <b>token</b><br> 1897 * Path: <b>DataElement.stringency</b><br> 1898 * </p> 1899 */ 1900 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STRINGENCY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STRINGENCY); 1901 1902 /** 1903 * Search parameter: <b>jurisdiction</b> 1904 * <p> 1905 * Description: <b>Intended jurisdiction for the data element</b><br> 1906 * Type: <b>token</b><br> 1907 * Path: <b>DataElement.jurisdiction</b><br> 1908 * </p> 1909 */ 1910 @SearchParamDefinition(name="jurisdiction", path="DataElement.jurisdiction", description="Intended jurisdiction for the data element", type="token" ) 1911 public static final String SP_JURISDICTION = "jurisdiction"; 1912 /** 1913 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 1914 * <p> 1915 * Description: <b>Intended jurisdiction for the data element</b><br> 1916 * Type: <b>token</b><br> 1917 * Path: <b>DataElement.jurisdiction</b><br> 1918 * </p> 1919 */ 1920 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 1921 1922 /** 1923 * Search parameter: <b>name</b> 1924 * <p> 1925 * Description: <b>Computationally friendly name of the data element</b><br> 1926 * Type: <b>string</b><br> 1927 * Path: <b>DataElement.name</b><br> 1928 * </p> 1929 */ 1930 @SearchParamDefinition(name="name", path="DataElement.name", description="Computationally friendly name of the data element", type="string" ) 1931 public static final String SP_NAME = "name"; 1932 /** 1933 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1934 * <p> 1935 * Description: <b>Computationally friendly name of the data element</b><br> 1936 * Type: <b>string</b><br> 1937 * Path: <b>DataElement.name</b><br> 1938 * </p> 1939 */ 1940 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1941 1942 /** 1943 * Search parameter: <b>publisher</b> 1944 * <p> 1945 * Description: <b>Name of the publisher of the data element</b><br> 1946 * Type: <b>string</b><br> 1947 * Path: <b>DataElement.publisher</b><br> 1948 * </p> 1949 */ 1950 @SearchParamDefinition(name="publisher", path="DataElement.publisher", description="Name of the publisher of the data element", type="string" ) 1951 public static final String SP_PUBLISHER = "publisher"; 1952 /** 1953 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 1954 * <p> 1955 * Description: <b>Name of the publisher of the data element</b><br> 1956 * Type: <b>string</b><br> 1957 * Path: <b>DataElement.publisher</b><br> 1958 * </p> 1959 */ 1960 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 1961 1962 /** 1963 * Search parameter: <b>description</b> 1964 * <p> 1965 * Description: <b>Text search in the description of the data element. This corresponds to the definition of the first DataElement.element.</b><br> 1966 * Type: <b>string</b><br> 1967 * Path: <b>DataElement.element.definition</b><br> 1968 * </p> 1969 */ 1970 @SearchParamDefinition(name="description", path="DataElement.element.definition", description="Text search in the description of the data element. This corresponds to the definition of the first DataElement.element.", type="string" ) 1971 public static final String SP_DESCRIPTION = "description"; 1972 /** 1973 * <b>Fluent Client</b> search parameter constant for <b>description</b> 1974 * <p> 1975 * Description: <b>Text search in the description of the data element. This corresponds to the definition of the first DataElement.element.</b><br> 1976 * Type: <b>string</b><br> 1977 * Path: <b>DataElement.element.definition</b><br> 1978 * </p> 1979 */ 1980 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 1981 1982 /** 1983 * Search parameter: <b>title</b> 1984 * <p> 1985 * Description: <b>The human-friendly name of the data element</b><br> 1986 * Type: <b>string</b><br> 1987 * Path: <b>DataElement.title</b><br> 1988 * </p> 1989 */ 1990 @SearchParamDefinition(name="title", path="DataElement.title", description="The human-friendly name of the data element", type="string" ) 1991 public static final String SP_TITLE = "title"; 1992 /** 1993 * <b>Fluent Client</b> search parameter constant for <b>title</b> 1994 * <p> 1995 * Description: <b>The human-friendly name of the data element</b><br> 1996 * Type: <b>string</b><br> 1997 * Path: <b>DataElement.title</b><br> 1998 * </p> 1999 */ 2000 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 2001 2002 /** 2003 * Search parameter: <b>version</b> 2004 * <p> 2005 * Description: <b>The business version of the data element</b><br> 2006 * Type: <b>token</b><br> 2007 * Path: <b>DataElement.version</b><br> 2008 * </p> 2009 */ 2010 @SearchParamDefinition(name="version", path="DataElement.version", description="The business version of the data element", type="token" ) 2011 public static final String SP_VERSION = "version"; 2012 /** 2013 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2014 * <p> 2015 * Description: <b>The business version of the data element</b><br> 2016 * Type: <b>token</b><br> 2017 * Path: <b>DataElement.version</b><br> 2018 * </p> 2019 */ 2020 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 2021 2022 /** 2023 * Search parameter: <b>url</b> 2024 * <p> 2025 * Description: <b>The uri that identifies the data element</b><br> 2026 * Type: <b>uri</b><br> 2027 * Path: <b>DataElement.url</b><br> 2028 * </p> 2029 */ 2030 @SearchParamDefinition(name="url", path="DataElement.url", description="The uri that identifies the data element", type="uri" ) 2031 public static final String SP_URL = "url"; 2032 /** 2033 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2034 * <p> 2035 * Description: <b>The uri that identifies the data element</b><br> 2036 * Type: <b>uri</b><br> 2037 * Path: <b>DataElement.url</b><br> 2038 * </p> 2039 */ 2040 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2041 2042 /** 2043 * Search parameter: <b>status</b> 2044 * <p> 2045 * Description: <b>The current status of the data element</b><br> 2046 * Type: <b>token</b><br> 2047 * Path: <b>DataElement.status</b><br> 2048 * </p> 2049 */ 2050 @SearchParamDefinition(name="status", path="DataElement.status", description="The current status of the data element", type="token" ) 2051 public static final String SP_STATUS = "status"; 2052 /** 2053 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2054 * <p> 2055 * Description: <b>The current status of the data element</b><br> 2056 * Type: <b>token</b><br> 2057 * Path: <b>DataElement.status</b><br> 2058 * </p> 2059 */ 2060 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2061 2062 2063}