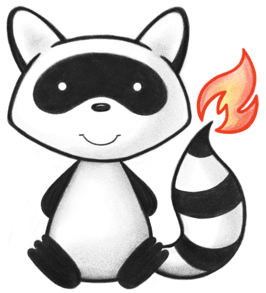
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047/** 048 * Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 049 */ 050@DatatypeDef(name="DataRequirement") 051public class DataRequirement extends Type implements ICompositeType { 052 053 @Block() 054 public static class DataRequirementCodeFilterComponent extends Element implements IBaseDatatypeElement { 055 /** 056 * The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept. 057 */ 058 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="The code-valued attribute of the filter", formalDefinition="The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept." ) 060 protected StringType path; 061 062 /** 063 * The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset. 064 */ 065 @Child(name = "valueSet", type = {StringType.class, ValueSet.class}, order=2, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="Valueset for the filter", formalDefinition="The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset." ) 067 protected Type valueSet; 068 069 /** 070 * The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. 071 */ 072 @Child(name = "valueCode", type = {CodeType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 073 @Description(shortDefinition="What code is expected", formalDefinition="The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes." ) 074 protected List<CodeType> valueCode; 075 076 /** 077 * The Codings for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified Codings. 078 */ 079 @Child(name = "valueCoding", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 080 @Description(shortDefinition="What Coding is expected", formalDefinition="The Codings for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified Codings." ) 081 protected List<Coding> valueCoding; 082 083 /** 084 * The CodeableConcepts for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified CodeableConcepts. 085 */ 086 @Child(name = "valueCodeableConcept", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 087 @Description(shortDefinition="What CodeableConcept is expected", formalDefinition="The CodeableConcepts for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified CodeableConcepts." ) 088 protected List<CodeableConcept> valueCodeableConcept; 089 090 private static final long serialVersionUID = -888422840L; 091 092 /** 093 * Constructor 094 */ 095 public DataRequirementCodeFilterComponent() { 096 super(); 097 } 098 099 /** 100 * Constructor 101 */ 102 public DataRequirementCodeFilterComponent(StringType path) { 103 super(); 104 this.path = path; 105 } 106 107 /** 108 * @return {@link #path} (The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 109 */ 110 public StringType getPathElement() { 111 if (this.path == null) 112 if (Configuration.errorOnAutoCreate()) 113 throw new Error("Attempt to auto-create DataRequirementCodeFilterComponent.path"); 114 else if (Configuration.doAutoCreate()) 115 this.path = new StringType(); // bb 116 return this.path; 117 } 118 119 public boolean hasPathElement() { 120 return this.path != null && !this.path.isEmpty(); 121 } 122 123 public boolean hasPath() { 124 return this.path != null && !this.path.isEmpty(); 125 } 126 127 /** 128 * @param value {@link #path} (The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 129 */ 130 public DataRequirementCodeFilterComponent setPathElement(StringType value) { 131 this.path = value; 132 return this; 133 } 134 135 /** 136 * @return The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept. 137 */ 138 public String getPath() { 139 return this.path == null ? null : this.path.getValue(); 140 } 141 142 /** 143 * @param value The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept. 144 */ 145 public DataRequirementCodeFilterComponent setPath(String value) { 146 if (this.path == null) 147 this.path = new StringType(); 148 this.path.setValue(value); 149 return this; 150 } 151 152 /** 153 * @return {@link #valueSet} (The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.) 154 */ 155 public Type getValueSet() { 156 return this.valueSet; 157 } 158 159 /** 160 * @return {@link #valueSet} (The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.) 161 */ 162 public StringType getValueSetStringType() throws FHIRException { 163 if (this.valueSet == null) 164 return null; 165 if (!(this.valueSet instanceof StringType)) 166 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.valueSet.getClass().getName()+" was encountered"); 167 return (StringType) this.valueSet; 168 } 169 170 public boolean hasValueSetStringType() { 171 return this.valueSet instanceof StringType; 172 } 173 174 /** 175 * @return {@link #valueSet} (The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.) 176 */ 177 public Reference getValueSetReference() throws FHIRException { 178 if (this.valueSet == null) 179 return null; 180 if (!(this.valueSet instanceof Reference)) 181 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.valueSet.getClass().getName()+" was encountered"); 182 return (Reference) this.valueSet; 183 } 184 185 public boolean hasValueSetReference() { 186 return this.valueSet instanceof Reference; 187 } 188 189 public boolean hasValueSet() { 190 return this.valueSet != null && !this.valueSet.isEmpty(); 191 } 192 193 /** 194 * @param value {@link #valueSet} (The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.) 195 */ 196 public DataRequirementCodeFilterComponent setValueSet(Type value) throws FHIRFormatError { 197 if (value != null && !(value instanceof StringType || value instanceof Reference)) 198 throw new FHIRFormatError("Not the right type for DataRequirement.codeFilter.valueSet[x]: "+value.fhirType()); 199 this.valueSet = value; 200 return this; 201 } 202 203 /** 204 * @return {@link #valueCode} (The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes.) 205 */ 206 public List<CodeType> getValueCode() { 207 if (this.valueCode == null) 208 this.valueCode = new ArrayList<CodeType>(); 209 return this.valueCode; 210 } 211 212 /** 213 * @return Returns a reference to <code>this</code> for easy method chaining 214 */ 215 public DataRequirementCodeFilterComponent setValueCode(List<CodeType> theValueCode) { 216 this.valueCode = theValueCode; 217 return this; 218 } 219 220 public boolean hasValueCode() { 221 if (this.valueCode == null) 222 return false; 223 for (CodeType item : this.valueCode) 224 if (!item.isEmpty()) 225 return true; 226 return false; 227 } 228 229 /** 230 * @return {@link #valueCode} (The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes.) 231 */ 232 public CodeType addValueCodeElement() {//2 233 CodeType t = new CodeType(); 234 if (this.valueCode == null) 235 this.valueCode = new ArrayList<CodeType>(); 236 this.valueCode.add(t); 237 return t; 238 } 239 240 /** 241 * @param value {@link #valueCode} (The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes.) 242 */ 243 public DataRequirementCodeFilterComponent addValueCode(String value) { //1 244 CodeType t = new CodeType(); 245 t.setValue(value); 246 if (this.valueCode == null) 247 this.valueCode = new ArrayList<CodeType>(); 248 this.valueCode.add(t); 249 return this; 250 } 251 252 /** 253 * @param value {@link #valueCode} (The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes.) 254 */ 255 public boolean hasValueCode(String value) { 256 if (this.valueCode == null) 257 return false; 258 for (CodeType v : this.valueCode) 259 if (v.getValue().equals(value)) // code 260 return true; 261 return false; 262 } 263 264 /** 265 * @return {@link #valueCoding} (The Codings for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified Codings.) 266 */ 267 public List<Coding> getValueCoding() { 268 if (this.valueCoding == null) 269 this.valueCoding = new ArrayList<Coding>(); 270 return this.valueCoding; 271 } 272 273 /** 274 * @return Returns a reference to <code>this</code> for easy method chaining 275 */ 276 public DataRequirementCodeFilterComponent setValueCoding(List<Coding> theValueCoding) { 277 this.valueCoding = theValueCoding; 278 return this; 279 } 280 281 public boolean hasValueCoding() { 282 if (this.valueCoding == null) 283 return false; 284 for (Coding item : this.valueCoding) 285 if (!item.isEmpty()) 286 return true; 287 return false; 288 } 289 290 public Coding addValueCoding() { //3 291 Coding t = new Coding(); 292 if (this.valueCoding == null) 293 this.valueCoding = new ArrayList<Coding>(); 294 this.valueCoding.add(t); 295 return t; 296 } 297 298 public DataRequirementCodeFilterComponent addValueCoding(Coding t) { //3 299 if (t == null) 300 return this; 301 if (this.valueCoding == null) 302 this.valueCoding = new ArrayList<Coding>(); 303 this.valueCoding.add(t); 304 return this; 305 } 306 307 /** 308 * @return The first repetition of repeating field {@link #valueCoding}, creating it if it does not already exist 309 */ 310 public Coding getValueCodingFirstRep() { 311 if (getValueCoding().isEmpty()) { 312 addValueCoding(); 313 } 314 return getValueCoding().get(0); 315 } 316 317 /** 318 * @return {@link #valueCodeableConcept} (The CodeableConcepts for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified CodeableConcepts.) 319 */ 320 public List<CodeableConcept> getValueCodeableConcept() { 321 if (this.valueCodeableConcept == null) 322 this.valueCodeableConcept = new ArrayList<CodeableConcept>(); 323 return this.valueCodeableConcept; 324 } 325 326 /** 327 * @return Returns a reference to <code>this</code> for easy method chaining 328 */ 329 public DataRequirementCodeFilterComponent setValueCodeableConcept(List<CodeableConcept> theValueCodeableConcept) { 330 this.valueCodeableConcept = theValueCodeableConcept; 331 return this; 332 } 333 334 public boolean hasValueCodeableConcept() { 335 if (this.valueCodeableConcept == null) 336 return false; 337 for (CodeableConcept item : this.valueCodeableConcept) 338 if (!item.isEmpty()) 339 return true; 340 return false; 341 } 342 343 public CodeableConcept addValueCodeableConcept() { //3 344 CodeableConcept t = new CodeableConcept(); 345 if (this.valueCodeableConcept == null) 346 this.valueCodeableConcept = new ArrayList<CodeableConcept>(); 347 this.valueCodeableConcept.add(t); 348 return t; 349 } 350 351 public DataRequirementCodeFilterComponent addValueCodeableConcept(CodeableConcept t) { //3 352 if (t == null) 353 return this; 354 if (this.valueCodeableConcept == null) 355 this.valueCodeableConcept = new ArrayList<CodeableConcept>(); 356 this.valueCodeableConcept.add(t); 357 return this; 358 } 359 360 /** 361 * @return The first repetition of repeating field {@link #valueCodeableConcept}, creating it if it does not already exist 362 */ 363 public CodeableConcept getValueCodeableConceptFirstRep() { 364 if (getValueCodeableConcept().isEmpty()) { 365 addValueCodeableConcept(); 366 } 367 return getValueCodeableConcept().get(0); 368 } 369 370 protected void listChildren(List<Property> children) { 371 super.listChildren(children); 372 children.add(new Property("path", "string", "The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.", 0, 1, path)); 373 children.add(new Property("valueSet[x]", "string|Reference(ValueSet)", "The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 0, 1, valueSet)); 374 children.add(new Property("valueCode", "code", "The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes.", 0, java.lang.Integer.MAX_VALUE, valueCode)); 375 children.add(new Property("valueCoding", "Coding", "The Codings for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified Codings.", 0, java.lang.Integer.MAX_VALUE, valueCoding)); 376 children.add(new Property("valueCodeableConcept", "CodeableConcept", "The CodeableConcepts for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified CodeableConcepts.", 0, java.lang.Integer.MAX_VALUE, valueCodeableConcept)); 377 } 378 379 @Override 380 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 381 switch (_hash) { 382 case 3433509: /*path*/ return new Property("path", "string", "The code-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.", 0, 1, path); 383 case -1438410321: /*valueSet[x]*/ return new Property("valueSet[x]", "string|Reference(ValueSet)", "The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 0, 1, valueSet); 384 case -1410174671: /*valueSet*/ return new Property("valueSet[x]", "string|Reference(ValueSet)", "The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 0, 1, valueSet); 385 case -1025157982: /*valueSetString*/ return new Property("valueSet[x]", "string|Reference(ValueSet)", "The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 0, 1, valueSet); 386 case 295220506: /*valueSetReference*/ return new Property("valueSet[x]", "string|Reference(ValueSet)", "The valueset for the code filter. The valueSet and value elements are exclusive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 0, 1, valueSet); 387 case -766209282: /*valueCode*/ return new Property("valueCode", "code", "The codes for the code filter. Only one of valueSet, valueCode, valueCoding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes.", 0, java.lang.Integer.MAX_VALUE, valueCode); 388 case -1887705029: /*valueCoding*/ return new Property("valueCoding", "Coding", "The Codings for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified Codings.", 0, java.lang.Integer.MAX_VALUE, valueCoding); 389 case 924902896: /*valueCodeableConcept*/ return new Property("valueCodeableConcept", "CodeableConcept", "The CodeableConcepts for the code filter. Only one of valueSet, valueCode, valueConding, or valueCodeableConcept may be specified. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified CodeableConcepts.", 0, java.lang.Integer.MAX_VALUE, valueCodeableConcept); 390 default: return super.getNamedProperty(_hash, _name, _checkValid); 391 } 392 393 } 394 395 @Override 396 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 397 switch (hash) { 398 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 399 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // Type 400 case -766209282: /*valueCode*/ return this.valueCode == null ? new Base[0] : this.valueCode.toArray(new Base[this.valueCode.size()]); // CodeType 401 case -1887705029: /*valueCoding*/ return this.valueCoding == null ? new Base[0] : this.valueCoding.toArray(new Base[this.valueCoding.size()]); // Coding 402 case 924902896: /*valueCodeableConcept*/ return this.valueCodeableConcept == null ? new Base[0] : this.valueCodeableConcept.toArray(new Base[this.valueCodeableConcept.size()]); // CodeableConcept 403 default: return super.getProperty(hash, name, checkValid); 404 } 405 406 } 407 408 @Override 409 public Base setProperty(int hash, String name, Base value) throws FHIRException { 410 switch (hash) { 411 case 3433509: // path 412 this.path = castToString(value); // StringType 413 return value; 414 case -1410174671: // valueSet 415 this.valueSet = castToType(value); // Type 416 return value; 417 case -766209282: // valueCode 418 this.getValueCode().add(castToCode(value)); // CodeType 419 return value; 420 case -1887705029: // valueCoding 421 this.getValueCoding().add(castToCoding(value)); // Coding 422 return value; 423 case 924902896: // valueCodeableConcept 424 this.getValueCodeableConcept().add(castToCodeableConcept(value)); // CodeableConcept 425 return value; 426 default: return super.setProperty(hash, name, value); 427 } 428 429 } 430 431 @Override 432 public Base setProperty(String name, Base value) throws FHIRException { 433 if (name.equals("path")) { 434 this.path = castToString(value); // StringType 435 } else if (name.equals("valueSet[x]")) { 436 this.valueSet = castToType(value); // Type 437 } else if (name.equals("valueCode")) { 438 this.getValueCode().add(castToCode(value)); 439 } else if (name.equals("valueCoding")) { 440 this.getValueCoding().add(castToCoding(value)); 441 } else if (name.equals("valueCodeableConcept")) { 442 this.getValueCodeableConcept().add(castToCodeableConcept(value)); 443 } else 444 return super.setProperty(name, value); 445 return value; 446 } 447 448 @Override 449 public Base makeProperty(int hash, String name) throws FHIRException { 450 switch (hash) { 451 case 3433509: return getPathElement(); 452 case -1438410321: return getValueSet(); 453 case -1410174671: return getValueSet(); 454 case -766209282: return addValueCodeElement(); 455 case -1887705029: return addValueCoding(); 456 case 924902896: return addValueCodeableConcept(); 457 default: return super.makeProperty(hash, name); 458 } 459 460 } 461 462 @Override 463 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 464 switch (hash) { 465 case 3433509: /*path*/ return new String[] {"string"}; 466 case -1410174671: /*valueSet*/ return new String[] {"string", "Reference"}; 467 case -766209282: /*valueCode*/ return new String[] {"code"}; 468 case -1887705029: /*valueCoding*/ return new String[] {"Coding"}; 469 case 924902896: /*valueCodeableConcept*/ return new String[] {"CodeableConcept"}; 470 default: return super.getTypesForProperty(hash, name); 471 } 472 473 } 474 475 @Override 476 public Base addChild(String name) throws FHIRException { 477 if (name.equals("path")) { 478 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.path"); 479 } 480 else if (name.equals("valueSetString")) { 481 this.valueSet = new StringType(); 482 return this.valueSet; 483 } 484 else if (name.equals("valueSetReference")) { 485 this.valueSet = new Reference(); 486 return this.valueSet; 487 } 488 else if (name.equals("valueCode")) { 489 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.valueCode"); 490 } 491 else if (name.equals("valueCoding")) { 492 return addValueCoding(); 493 } 494 else if (name.equals("valueCodeableConcept")) { 495 return addValueCodeableConcept(); 496 } 497 else 498 return super.addChild(name); 499 } 500 501 public DataRequirementCodeFilterComponent copy() { 502 DataRequirementCodeFilterComponent dst = new DataRequirementCodeFilterComponent(); 503 copyValues(dst); 504 dst.path = path == null ? null : path.copy(); 505 dst.valueSet = valueSet == null ? null : valueSet.copy(); 506 if (valueCode != null) { 507 dst.valueCode = new ArrayList<CodeType>(); 508 for (CodeType i : valueCode) 509 dst.valueCode.add(i.copy()); 510 }; 511 if (valueCoding != null) { 512 dst.valueCoding = new ArrayList<Coding>(); 513 for (Coding i : valueCoding) 514 dst.valueCoding.add(i.copy()); 515 }; 516 if (valueCodeableConcept != null) { 517 dst.valueCodeableConcept = new ArrayList<CodeableConcept>(); 518 for (CodeableConcept i : valueCodeableConcept) 519 dst.valueCodeableConcept.add(i.copy()); 520 }; 521 return dst; 522 } 523 524 @Override 525 public boolean equalsDeep(Base other_) { 526 if (!super.equalsDeep(other_)) 527 return false; 528 if (!(other_ instanceof DataRequirementCodeFilterComponent)) 529 return false; 530 DataRequirementCodeFilterComponent o = (DataRequirementCodeFilterComponent) other_; 531 return compareDeep(path, o.path, true) && compareDeep(valueSet, o.valueSet, true) && compareDeep(valueCode, o.valueCode, true) 532 && compareDeep(valueCoding, o.valueCoding, true) && compareDeep(valueCodeableConcept, o.valueCodeableConcept, true) 533 ; 534 } 535 536 @Override 537 public boolean equalsShallow(Base other_) { 538 if (!super.equalsShallow(other_)) 539 return false; 540 if (!(other_ instanceof DataRequirementCodeFilterComponent)) 541 return false; 542 DataRequirementCodeFilterComponent o = (DataRequirementCodeFilterComponent) other_; 543 return compareValues(path, o.path, true) && compareValues(valueCode, o.valueCode, true); 544 } 545 546 public boolean isEmpty() { 547 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, valueSet, valueCode 548 , valueCoding, valueCodeableConcept); 549 } 550 551 public String fhirType() { 552 return "DataRequirement.codeFilter"; 553 554 } 555 556 } 557 558 @Block() 559 public static class DataRequirementDateFilterComponent extends Element implements IBaseDatatypeElement { 560 /** 561 * The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing. 562 */ 563 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 564 @Description(shortDefinition="The date-valued attribute of the filter", formalDefinition="The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing." ) 565 protected StringType path; 566 567 /** 568 * The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now. 569 */ 570 @Child(name = "value", type = {DateTimeType.class, Period.class, Duration.class}, order=2, min=0, max=1, modifier=false, summary=true) 571 @Description(shortDefinition="The value of the filter, as a Period, DateTime, or Duration value", formalDefinition="The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now." ) 572 protected Type value; 573 574 private static final long serialVersionUID = 1791957163L; 575 576 /** 577 * Constructor 578 */ 579 public DataRequirementDateFilterComponent() { 580 super(); 581 } 582 583 /** 584 * Constructor 585 */ 586 public DataRequirementDateFilterComponent(StringType path) { 587 super(); 588 this.path = path; 589 } 590 591 /** 592 * @return {@link #path} (The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 593 */ 594 public StringType getPathElement() { 595 if (this.path == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create DataRequirementDateFilterComponent.path"); 598 else if (Configuration.doAutoCreate()) 599 this.path = new StringType(); // bb 600 return this.path; 601 } 602 603 public boolean hasPathElement() { 604 return this.path != null && !this.path.isEmpty(); 605 } 606 607 public boolean hasPath() { 608 return this.path != null && !this.path.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #path} (The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 613 */ 614 public DataRequirementDateFilterComponent setPathElement(StringType value) { 615 this.path = value; 616 return this; 617 } 618 619 /** 620 * @return The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing. 621 */ 622 public String getPath() { 623 return this.path == null ? null : this.path.getValue(); 624 } 625 626 /** 627 * @param value The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing. 628 */ 629 public DataRequirementDateFilterComponent setPath(String value) { 630 if (this.path == null) 631 this.path = new StringType(); 632 this.path.setValue(value); 633 return this; 634 } 635 636 /** 637 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.) 638 */ 639 public Type getValue() { 640 return this.value; 641 } 642 643 /** 644 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.) 645 */ 646 public DateTimeType getValueDateTimeType() throws FHIRException { 647 if (this.value == null) 648 return null; 649 if (!(this.value instanceof DateTimeType)) 650 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 651 return (DateTimeType) this.value; 652 } 653 654 public boolean hasValueDateTimeType() { 655 return this.value instanceof DateTimeType; 656 } 657 658 /** 659 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.) 660 */ 661 public Period getValuePeriod() throws FHIRException { 662 if (this.value == null) 663 return null; 664 if (!(this.value instanceof Period)) 665 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 666 return (Period) this.value; 667 } 668 669 public boolean hasValuePeriod() { 670 return this.value instanceof Period; 671 } 672 673 /** 674 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.) 675 */ 676 public Duration getValueDuration() throws FHIRException { 677 if (this.value == null) 678 return null; 679 if (!(this.value instanceof Duration)) 680 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 681 return (Duration) this.value; 682 } 683 684 public boolean hasValueDuration() { 685 return this.value instanceof Duration; 686 } 687 688 public boolean hasValue() { 689 return this.value != null && !this.value.isEmpty(); 690 } 691 692 /** 693 * @param value {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.) 694 */ 695 public DataRequirementDateFilterComponent setValue(Type value) throws FHIRFormatError { 696 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration)) 697 throw new FHIRFormatError("Not the right type for DataRequirement.dateFilter.value[x]: "+value.fhirType()); 698 this.value = value; 699 return this; 700 } 701 702 protected void listChildren(List<Property> children) { 703 super.listChildren(children); 704 children.add(new Property("path", "string", "The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing.", 0, 1, path)); 705 children.add(new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.", 0, 1, value)); 706 } 707 708 @Override 709 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 710 switch (_hash) { 711 case 3433509: /*path*/ return new Property("path", "string", "The date-valued attribute of the filter. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. The path must resolve to an element of type dateTime, Period, Schedule, or Timing.", 0, 1, path); 712 case -1410166417: /*value[x]*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.", 0, 1, value); 713 case 111972721: /*value*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.", 0, 1, value); 714 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.", 0, 1, value); 715 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.", 0, 1, value); 716 case 1558135333: /*valueDuration*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration from now.", 0, 1, value); 717 default: return super.getNamedProperty(_hash, _name, _checkValid); 718 } 719 720 } 721 722 @Override 723 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 724 switch (hash) { 725 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 726 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 727 default: return super.getProperty(hash, name, checkValid); 728 } 729 730 } 731 732 @Override 733 public Base setProperty(int hash, String name, Base value) throws FHIRException { 734 switch (hash) { 735 case 3433509: // path 736 this.path = castToString(value); // StringType 737 return value; 738 case 111972721: // value 739 this.value = castToType(value); // Type 740 return value; 741 default: return super.setProperty(hash, name, value); 742 } 743 744 } 745 746 @Override 747 public Base setProperty(String name, Base value) throws FHIRException { 748 if (name.equals("path")) { 749 this.path = castToString(value); // StringType 750 } else if (name.equals("value[x]")) { 751 this.value = castToType(value); // Type 752 } else 753 return super.setProperty(name, value); 754 return value; 755 } 756 757 @Override 758 public Base makeProperty(int hash, String name) throws FHIRException { 759 switch (hash) { 760 case 3433509: return getPathElement(); 761 case -1410166417: return getValue(); 762 case 111972721: return getValue(); 763 default: return super.makeProperty(hash, name); 764 } 765 766 } 767 768 @Override 769 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 770 switch (hash) { 771 case 3433509: /*path*/ return new String[] {"string"}; 772 case 111972721: /*value*/ return new String[] {"dateTime", "Period", "Duration"}; 773 default: return super.getTypesForProperty(hash, name); 774 } 775 776 } 777 778 @Override 779 public Base addChild(String name) throws FHIRException { 780 if (name.equals("path")) { 781 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.path"); 782 } 783 else if (name.equals("valueDateTime")) { 784 this.value = new DateTimeType(); 785 return this.value; 786 } 787 else if (name.equals("valuePeriod")) { 788 this.value = new Period(); 789 return this.value; 790 } 791 else if (name.equals("valueDuration")) { 792 this.value = new Duration(); 793 return this.value; 794 } 795 else 796 return super.addChild(name); 797 } 798 799 public DataRequirementDateFilterComponent copy() { 800 DataRequirementDateFilterComponent dst = new DataRequirementDateFilterComponent(); 801 copyValues(dst); 802 dst.path = path == null ? null : path.copy(); 803 dst.value = value == null ? null : value.copy(); 804 return dst; 805 } 806 807 @Override 808 public boolean equalsDeep(Base other_) { 809 if (!super.equalsDeep(other_)) 810 return false; 811 if (!(other_ instanceof DataRequirementDateFilterComponent)) 812 return false; 813 DataRequirementDateFilterComponent o = (DataRequirementDateFilterComponent) other_; 814 return compareDeep(path, o.path, true) && compareDeep(value, o.value, true); 815 } 816 817 @Override 818 public boolean equalsShallow(Base other_) { 819 if (!super.equalsShallow(other_)) 820 return false; 821 if (!(other_ instanceof DataRequirementDateFilterComponent)) 822 return false; 823 DataRequirementDateFilterComponent o = (DataRequirementDateFilterComponent) other_; 824 return compareValues(path, o.path, true); 825 } 826 827 public boolean isEmpty() { 828 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, value); 829 } 830 831 public String fhirType() { 832 return "DataRequirement.dateFilter"; 833 834 } 835 836 } 837 838 /** 839 * The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile. 840 */ 841 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 842 @Description(shortDefinition="The type of the required data", formalDefinition="The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile." ) 843 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-types") 844 protected CodeType type; 845 846 /** 847 * The profile of the required data, specified as the uri of the profile definition. 848 */ 849 @Child(name = "profile", type = {UriType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 850 @Description(shortDefinition="The profile of the required data", formalDefinition="The profile of the required data, specified as the uri of the profile definition." ) 851 protected List<UriType> profile; 852 853 /** 854 * Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported. 855 */ 856 @Child(name = "mustSupport", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 857 @Description(shortDefinition="Indicates that specific structure elements are referenced by the knowledge module", formalDefinition="Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported." ) 858 protected List<StringType> mustSupport; 859 860 /** 861 * Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. 862 */ 863 @Child(name = "codeFilter", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 864 @Description(shortDefinition="What codes are expected", formalDefinition="Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data." ) 865 protected List<DataRequirementCodeFilterComponent> codeFilter; 866 867 /** 868 * Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. 869 */ 870 @Child(name = "dateFilter", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 871 @Description(shortDefinition="What dates/date ranges are expected", formalDefinition="Date filters specify additional constraints on the data in terms of the applicable date range for specific elements." ) 872 protected List<DataRequirementDateFilterComponent> dateFilter; 873 874 private static final long serialVersionUID = 274786645L; 875 876 /** 877 * Constructor 878 */ 879 public DataRequirement() { 880 super(); 881 } 882 883 /** 884 * Constructor 885 */ 886 public DataRequirement(CodeType type) { 887 super(); 888 this.type = type; 889 } 890 891 /** 892 * @return {@link #type} (The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 893 */ 894 public CodeType getTypeElement() { 895 if (this.type == null) 896 if (Configuration.errorOnAutoCreate()) 897 throw new Error("Attempt to auto-create DataRequirement.type"); 898 else if (Configuration.doAutoCreate()) 899 this.type = new CodeType(); // bb 900 return this.type; 901 } 902 903 public boolean hasTypeElement() { 904 return this.type != null && !this.type.isEmpty(); 905 } 906 907 public boolean hasType() { 908 return this.type != null && !this.type.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #type} (The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 913 */ 914 public DataRequirement setTypeElement(CodeType value) { 915 this.type = value; 916 return this; 917 } 918 919 /** 920 * @return The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile. 921 */ 922 public String getType() { 923 return this.type == null ? null : this.type.getValue(); 924 } 925 926 /** 927 * @param value The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile. 928 */ 929 public DataRequirement setType(String value) { 930 if (this.type == null) 931 this.type = new CodeType(); 932 this.type.setValue(value); 933 return this; 934 } 935 936 /** 937 * @return {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 938 */ 939 public List<UriType> getProfile() { 940 if (this.profile == null) 941 this.profile = new ArrayList<UriType>(); 942 return this.profile; 943 } 944 945 /** 946 * @return Returns a reference to <code>this</code> for easy method chaining 947 */ 948 public DataRequirement setProfile(List<UriType> theProfile) { 949 this.profile = theProfile; 950 return this; 951 } 952 953 public boolean hasProfile() { 954 if (this.profile == null) 955 return false; 956 for (UriType item : this.profile) 957 if (!item.isEmpty()) 958 return true; 959 return false; 960 } 961 962 /** 963 * @return {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 964 */ 965 public UriType addProfileElement() {//2 966 UriType t = new UriType(); 967 if (this.profile == null) 968 this.profile = new ArrayList<UriType>(); 969 this.profile.add(t); 970 return t; 971 } 972 973 /** 974 * @param value {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 975 */ 976 public DataRequirement addProfile(String value) { //1 977 UriType t = new UriType(); 978 t.setValue(value); 979 if (this.profile == null) 980 this.profile = new ArrayList<UriType>(); 981 this.profile.add(t); 982 return this; 983 } 984 985 /** 986 * @param value {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 987 */ 988 public boolean hasProfile(String value) { 989 if (this.profile == null) 990 return false; 991 for (UriType v : this.profile) 992 if (v.getValue().equals(value)) // uri 993 return true; 994 return false; 995 } 996 997 /** 998 * @return {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported.) 999 */ 1000 public List<StringType> getMustSupport() { 1001 if (this.mustSupport == null) 1002 this.mustSupport = new ArrayList<StringType>(); 1003 return this.mustSupport; 1004 } 1005 1006 /** 1007 * @return Returns a reference to <code>this</code> for easy method chaining 1008 */ 1009 public DataRequirement setMustSupport(List<StringType> theMustSupport) { 1010 this.mustSupport = theMustSupport; 1011 return this; 1012 } 1013 1014 public boolean hasMustSupport() { 1015 if (this.mustSupport == null) 1016 return false; 1017 for (StringType item : this.mustSupport) 1018 if (!item.isEmpty()) 1019 return true; 1020 return false; 1021 } 1022 1023 /** 1024 * @return {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported.) 1025 */ 1026 public StringType addMustSupportElement() {//2 1027 StringType t = new StringType(); 1028 if (this.mustSupport == null) 1029 this.mustSupport = new ArrayList<StringType>(); 1030 this.mustSupport.add(t); 1031 return t; 1032 } 1033 1034 /** 1035 * @param value {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported.) 1036 */ 1037 public DataRequirement addMustSupport(String value) { //1 1038 StringType t = new StringType(); 1039 t.setValue(value); 1040 if (this.mustSupport == null) 1041 this.mustSupport = new ArrayList<StringType>(); 1042 this.mustSupport.add(t); 1043 return this; 1044 } 1045 1046 /** 1047 * @param value {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported.) 1048 */ 1049 public boolean hasMustSupport(String value) { 1050 if (this.mustSupport == null) 1051 return false; 1052 for (StringType v : this.mustSupport) 1053 if (v.getValue().equals(value)) // string 1054 return true; 1055 return false; 1056 } 1057 1058 /** 1059 * @return {@link #codeFilter} (Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data.) 1060 */ 1061 public List<DataRequirementCodeFilterComponent> getCodeFilter() { 1062 if (this.codeFilter == null) 1063 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 1064 return this.codeFilter; 1065 } 1066 1067 /** 1068 * @return Returns a reference to <code>this</code> for easy method chaining 1069 */ 1070 public DataRequirement setCodeFilter(List<DataRequirementCodeFilterComponent> theCodeFilter) { 1071 this.codeFilter = theCodeFilter; 1072 return this; 1073 } 1074 1075 public boolean hasCodeFilter() { 1076 if (this.codeFilter == null) 1077 return false; 1078 for (DataRequirementCodeFilterComponent item : this.codeFilter) 1079 if (!item.isEmpty()) 1080 return true; 1081 return false; 1082 } 1083 1084 public DataRequirementCodeFilterComponent addCodeFilter() { //3 1085 DataRequirementCodeFilterComponent t = new DataRequirementCodeFilterComponent(); 1086 if (this.codeFilter == null) 1087 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 1088 this.codeFilter.add(t); 1089 return t; 1090 } 1091 1092 public DataRequirement addCodeFilter(DataRequirementCodeFilterComponent t) { //3 1093 if (t == null) 1094 return this; 1095 if (this.codeFilter == null) 1096 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 1097 this.codeFilter.add(t); 1098 return this; 1099 } 1100 1101 /** 1102 * @return The first repetition of repeating field {@link #codeFilter}, creating it if it does not already exist 1103 */ 1104 public DataRequirementCodeFilterComponent getCodeFilterFirstRep() { 1105 if (getCodeFilter().isEmpty()) { 1106 addCodeFilter(); 1107 } 1108 return getCodeFilter().get(0); 1109 } 1110 1111 /** 1112 * @return {@link #dateFilter} (Date filters specify additional constraints on the data in terms of the applicable date range for specific elements.) 1113 */ 1114 public List<DataRequirementDateFilterComponent> getDateFilter() { 1115 if (this.dateFilter == null) 1116 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 1117 return this.dateFilter; 1118 } 1119 1120 /** 1121 * @return Returns a reference to <code>this</code> for easy method chaining 1122 */ 1123 public DataRequirement setDateFilter(List<DataRequirementDateFilterComponent> theDateFilter) { 1124 this.dateFilter = theDateFilter; 1125 return this; 1126 } 1127 1128 public boolean hasDateFilter() { 1129 if (this.dateFilter == null) 1130 return false; 1131 for (DataRequirementDateFilterComponent item : this.dateFilter) 1132 if (!item.isEmpty()) 1133 return true; 1134 return false; 1135 } 1136 1137 public DataRequirementDateFilterComponent addDateFilter() { //3 1138 DataRequirementDateFilterComponent t = new DataRequirementDateFilterComponent(); 1139 if (this.dateFilter == null) 1140 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 1141 this.dateFilter.add(t); 1142 return t; 1143 } 1144 1145 public DataRequirement addDateFilter(DataRequirementDateFilterComponent t) { //3 1146 if (t == null) 1147 return this; 1148 if (this.dateFilter == null) 1149 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 1150 this.dateFilter.add(t); 1151 return this; 1152 } 1153 1154 /** 1155 * @return The first repetition of repeating field {@link #dateFilter}, creating it if it does not already exist 1156 */ 1157 public DataRequirementDateFilterComponent getDateFilterFirstRep() { 1158 if (getDateFilter().isEmpty()) { 1159 addDateFilter(); 1160 } 1161 return getDateFilter().get(0); 1162 } 1163 1164 protected void listChildren(List<Property> children) { 1165 super.listChildren(children); 1166 children.add(new Property("type", "code", "The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.", 0, 1, type)); 1167 children.add(new Property("profile", "uri", "The profile of the required data, specified as the uri of the profile definition.", 0, java.lang.Integer.MAX_VALUE, profile)); 1168 children.add(new Property("mustSupport", "string", "Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported.", 0, java.lang.Integer.MAX_VALUE, mustSupport)); 1169 children.add(new Property("codeFilter", "", "Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data.", 0, java.lang.Integer.MAX_VALUE, codeFilter)); 1170 children.add(new Property("dateFilter", "", "Date filters specify additional constraints on the data in terms of the applicable date range for specific elements.", 0, java.lang.Integer.MAX_VALUE, dateFilter)); 1171 } 1172 1173 @Override 1174 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1175 switch (_hash) { 1176 case 3575610: /*type*/ return new Property("type", "code", "The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.", 0, 1, type); 1177 case -309425751: /*profile*/ return new Property("profile", "uri", "The profile of the required data, specified as the uri of the profile definition.", 0, java.lang.Integer.MAX_VALUE, profile); 1178 case -1402857082: /*mustSupport*/ return new Property("mustSupport", "string", "Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. Note that the value for this element can be a path to allow references to nested elements. In that case, all the elements along the path must be supported.", 0, java.lang.Integer.MAX_VALUE, mustSupport); 1179 case -1303674939: /*codeFilter*/ return new Property("codeFilter", "", "Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data.", 0, java.lang.Integer.MAX_VALUE, codeFilter); 1180 case 149531846: /*dateFilter*/ return new Property("dateFilter", "", "Date filters specify additional constraints on the data in terms of the applicable date range for specific elements.", 0, java.lang.Integer.MAX_VALUE, dateFilter); 1181 default: return super.getNamedProperty(_hash, _name, _checkValid); 1182 } 1183 1184 } 1185 1186 @Override 1187 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1188 switch (hash) { 1189 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 1190 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // UriType 1191 case -1402857082: /*mustSupport*/ return this.mustSupport == null ? new Base[0] : this.mustSupport.toArray(new Base[this.mustSupport.size()]); // StringType 1192 case -1303674939: /*codeFilter*/ return this.codeFilter == null ? new Base[0] : this.codeFilter.toArray(new Base[this.codeFilter.size()]); // DataRequirementCodeFilterComponent 1193 case 149531846: /*dateFilter*/ return this.dateFilter == null ? new Base[0] : this.dateFilter.toArray(new Base[this.dateFilter.size()]); // DataRequirementDateFilterComponent 1194 default: return super.getProperty(hash, name, checkValid); 1195 } 1196 1197 } 1198 1199 @Override 1200 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1201 switch (hash) { 1202 case 3575610: // type 1203 this.type = castToCode(value); // CodeType 1204 return value; 1205 case -309425751: // profile 1206 this.getProfile().add(castToUri(value)); // UriType 1207 return value; 1208 case -1402857082: // mustSupport 1209 this.getMustSupport().add(castToString(value)); // StringType 1210 return value; 1211 case -1303674939: // codeFilter 1212 this.getCodeFilter().add((DataRequirementCodeFilterComponent) value); // DataRequirementCodeFilterComponent 1213 return value; 1214 case 149531846: // dateFilter 1215 this.getDateFilter().add((DataRequirementDateFilterComponent) value); // DataRequirementDateFilterComponent 1216 return value; 1217 default: return super.setProperty(hash, name, value); 1218 } 1219 1220 } 1221 1222 @Override 1223 public Base setProperty(String name, Base value) throws FHIRException { 1224 if (name.equals("type")) { 1225 this.type = castToCode(value); // CodeType 1226 } else if (name.equals("profile")) { 1227 this.getProfile().add(castToUri(value)); 1228 } else if (name.equals("mustSupport")) { 1229 this.getMustSupport().add(castToString(value)); 1230 } else if (name.equals("codeFilter")) { 1231 this.getCodeFilter().add((DataRequirementCodeFilterComponent) value); 1232 } else if (name.equals("dateFilter")) { 1233 this.getDateFilter().add((DataRequirementDateFilterComponent) value); 1234 } else 1235 return super.setProperty(name, value); 1236 return value; 1237 } 1238 1239 @Override 1240 public Base makeProperty(int hash, String name) throws FHIRException { 1241 switch (hash) { 1242 case 3575610: return getTypeElement(); 1243 case -309425751: return addProfileElement(); 1244 case -1402857082: return addMustSupportElement(); 1245 case -1303674939: return addCodeFilter(); 1246 case 149531846: return addDateFilter(); 1247 default: return super.makeProperty(hash, name); 1248 } 1249 1250 } 1251 1252 @Override 1253 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1254 switch (hash) { 1255 case 3575610: /*type*/ return new String[] {"code"}; 1256 case -309425751: /*profile*/ return new String[] {"uri"}; 1257 case -1402857082: /*mustSupport*/ return new String[] {"string"}; 1258 case -1303674939: /*codeFilter*/ return new String[] {}; 1259 case 149531846: /*dateFilter*/ return new String[] {}; 1260 default: return super.getTypesForProperty(hash, name); 1261 } 1262 1263 } 1264 1265 @Override 1266 public Base addChild(String name) throws FHIRException { 1267 if (name.equals("type")) { 1268 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.type"); 1269 } 1270 else if (name.equals("profile")) { 1271 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.profile"); 1272 } 1273 else if (name.equals("mustSupport")) { 1274 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.mustSupport"); 1275 } 1276 else if (name.equals("codeFilter")) { 1277 return addCodeFilter(); 1278 } 1279 else if (name.equals("dateFilter")) { 1280 return addDateFilter(); 1281 } 1282 else 1283 return super.addChild(name); 1284 } 1285 1286 public String fhirType() { 1287 return "DataRequirement"; 1288 1289 } 1290 1291 public DataRequirement copy() { 1292 DataRequirement dst = new DataRequirement(); 1293 copyValues(dst); 1294 dst.type = type == null ? null : type.copy(); 1295 if (profile != null) { 1296 dst.profile = new ArrayList<UriType>(); 1297 for (UriType i : profile) 1298 dst.profile.add(i.copy()); 1299 }; 1300 if (mustSupport != null) { 1301 dst.mustSupport = new ArrayList<StringType>(); 1302 for (StringType i : mustSupport) 1303 dst.mustSupport.add(i.copy()); 1304 }; 1305 if (codeFilter != null) { 1306 dst.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 1307 for (DataRequirementCodeFilterComponent i : codeFilter) 1308 dst.codeFilter.add(i.copy()); 1309 }; 1310 if (dateFilter != null) { 1311 dst.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 1312 for (DataRequirementDateFilterComponent i : dateFilter) 1313 dst.dateFilter.add(i.copy()); 1314 }; 1315 return dst; 1316 } 1317 1318 protected DataRequirement typedCopy() { 1319 return copy(); 1320 } 1321 1322 @Override 1323 public boolean equalsDeep(Base other_) { 1324 if (!super.equalsDeep(other_)) 1325 return false; 1326 if (!(other_ instanceof DataRequirement)) 1327 return false; 1328 DataRequirement o = (DataRequirement) other_; 1329 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) && compareDeep(mustSupport, o.mustSupport, true) 1330 && compareDeep(codeFilter, o.codeFilter, true) && compareDeep(dateFilter, o.dateFilter, true); 1331 } 1332 1333 @Override 1334 public boolean equalsShallow(Base other_) { 1335 if (!super.equalsShallow(other_)) 1336 return false; 1337 if (!(other_ instanceof DataRequirement)) 1338 return false; 1339 DataRequirement o = (DataRequirement) other_; 1340 return compareValues(type, o.type, true) && compareValues(profile, o.profile, true) && compareValues(mustSupport, o.mustSupport, true) 1341 ; 1342 } 1343 1344 public boolean isEmpty() { 1345 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, mustSupport 1346 , codeFilter, dateFilter); 1347 } 1348 1349 1350}