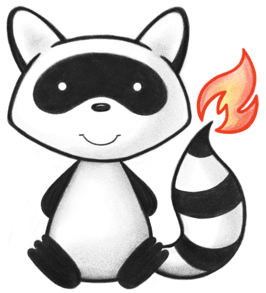
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 050 */ 051@ResourceDef(name="DetectedIssue", profile="http://hl7.org/fhir/Profile/DetectedIssue") 052public class DetectedIssue extends DomainResource { 053 054 public enum DetectedIssueStatus { 055 /** 056 * The existence of the observation is registered, but there is no result yet available. 057 */ 058 REGISTERED, 059 /** 060 * This is an initial or interim observation: data may be incomplete or unverified. 061 */ 062 PRELIMINARY, 063 /** 064 * The observation is complete. 065 */ 066 FINAL, 067 /** 068 * Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections. 069 */ 070 AMENDED, 071 /** 072 * Subsequent to being Final, the observation has been modified to correct an error in the test result. 073 */ 074 CORRECTED, 075 /** 076 * The observation is unavailable because the measurement was not started or not completed (also sometimes called "aborted"). 077 */ 078 CANCELLED, 079 /** 080 * The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 081 */ 082 ENTEREDINERROR, 083 /** 084 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, but the authoring system does not know which. 085 */ 086 UNKNOWN, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 public static DetectedIssueStatus fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("registered".equals(codeString)) 095 return REGISTERED; 096 if ("preliminary".equals(codeString)) 097 return PRELIMINARY; 098 if ("final".equals(codeString)) 099 return FINAL; 100 if ("amended".equals(codeString)) 101 return AMENDED; 102 if ("corrected".equals(codeString)) 103 return CORRECTED; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("entered-in-error".equals(codeString)) 107 return ENTEREDINERROR; 108 if ("unknown".equals(codeString)) 109 return UNKNOWN; 110 if (Configuration.isAcceptInvalidEnums()) 111 return null; 112 else 113 throw new FHIRException("Unknown DetectedIssueStatus code '"+codeString+"'"); 114 } 115 public String toCode() { 116 switch (this) { 117 case REGISTERED: return "registered"; 118 case PRELIMINARY: return "preliminary"; 119 case FINAL: return "final"; 120 case AMENDED: return "amended"; 121 case CORRECTED: return "corrected"; 122 case CANCELLED: return "cancelled"; 123 case ENTEREDINERROR: return "entered-in-error"; 124 case UNKNOWN: return "unknown"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getSystem() { 130 switch (this) { 131 case REGISTERED: return "http://hl7.org/fhir/observation-status"; 132 case PRELIMINARY: return "http://hl7.org/fhir/observation-status"; 133 case FINAL: return "http://hl7.org/fhir/observation-status"; 134 case AMENDED: return "http://hl7.org/fhir/observation-status"; 135 case CORRECTED: return "http://hl7.org/fhir/observation-status"; 136 case CANCELLED: return "http://hl7.org/fhir/observation-status"; 137 case ENTEREDINERROR: return "http://hl7.org/fhir/observation-status"; 138 case UNKNOWN: return "http://hl7.org/fhir/observation-status"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDefinition() { 144 switch (this) { 145 case REGISTERED: return "The existence of the observation is registered, but there is no result yet available."; 146 case PRELIMINARY: return "This is an initial or interim observation: data may be incomplete or unverified."; 147 case FINAL: return "The observation is complete."; 148 case AMENDED: return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 149 case CORRECTED: return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 150 case CANCELLED: return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 151 case ENTEREDINERROR: return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 152 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring system does not know which."; 153 case NULL: return null; 154 default: return "?"; 155 } 156 } 157 public String getDisplay() { 158 switch (this) { 159 case REGISTERED: return "Registered"; 160 case PRELIMINARY: return "Preliminary"; 161 case FINAL: return "Final"; 162 case AMENDED: return "Amended"; 163 case CORRECTED: return "Corrected"; 164 case CANCELLED: return "Cancelled"; 165 case ENTEREDINERROR: return "Entered in Error"; 166 case UNKNOWN: return "Unknown"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 } 172 173 public static class DetectedIssueStatusEnumFactory implements EnumFactory<DetectedIssueStatus> { 174 public DetectedIssueStatus fromCode(String codeString) throws IllegalArgumentException { 175 if (codeString == null || "".equals(codeString)) 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("registered".equals(codeString)) 179 return DetectedIssueStatus.REGISTERED; 180 if ("preliminary".equals(codeString)) 181 return DetectedIssueStatus.PRELIMINARY; 182 if ("final".equals(codeString)) 183 return DetectedIssueStatus.FINAL; 184 if ("amended".equals(codeString)) 185 return DetectedIssueStatus.AMENDED; 186 if ("corrected".equals(codeString)) 187 return DetectedIssueStatus.CORRECTED; 188 if ("cancelled".equals(codeString)) 189 return DetectedIssueStatus.CANCELLED; 190 if ("entered-in-error".equals(codeString)) 191 return DetectedIssueStatus.ENTEREDINERROR; 192 if ("unknown".equals(codeString)) 193 return DetectedIssueStatus.UNKNOWN; 194 throw new IllegalArgumentException("Unknown DetectedIssueStatus code '"+codeString+"'"); 195 } 196 public Enumeration<DetectedIssueStatus> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<DetectedIssueStatus>(this); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("registered".equals(codeString)) 205 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.REGISTERED); 206 if ("preliminary".equals(codeString)) 207 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.PRELIMINARY); 208 if ("final".equals(codeString)) 209 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.FINAL); 210 if ("amended".equals(codeString)) 211 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.AMENDED); 212 if ("corrected".equals(codeString)) 213 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CORRECTED); 214 if ("cancelled".equals(codeString)) 215 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CANCELLED); 216 if ("entered-in-error".equals(codeString)) 217 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.ENTEREDINERROR); 218 if ("unknown".equals(codeString)) 219 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.UNKNOWN); 220 throw new FHIRException("Unknown DetectedIssueStatus code '"+codeString+"'"); 221 } 222 public String toCode(DetectedIssueStatus code) { 223 if (code == DetectedIssueStatus.NULL) 224 return null; 225 if (code == DetectedIssueStatus.REGISTERED) 226 return "registered"; 227 if (code == DetectedIssueStatus.PRELIMINARY) 228 return "preliminary"; 229 if (code == DetectedIssueStatus.FINAL) 230 return "final"; 231 if (code == DetectedIssueStatus.AMENDED) 232 return "amended"; 233 if (code == DetectedIssueStatus.CORRECTED) 234 return "corrected"; 235 if (code == DetectedIssueStatus.CANCELLED) 236 return "cancelled"; 237 if (code == DetectedIssueStatus.ENTEREDINERROR) 238 return "entered-in-error"; 239 if (code == DetectedIssueStatus.UNKNOWN) 240 return "unknown"; 241 return "?"; 242 } 243 public String toSystem(DetectedIssueStatus code) { 244 return code.getSystem(); 245 } 246 } 247 248 public enum DetectedIssueSeverity { 249 /** 250 * Indicates the issue may be life-threatening or has the potential to cause permanent injury. 251 */ 252 HIGH, 253 /** 254 * Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury. 255 */ 256 MODERATE, 257 /** 258 * Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject. 259 */ 260 LOW, 261 /** 262 * added to help the parsers with the generic types 263 */ 264 NULL; 265 public static DetectedIssueSeverity fromCode(String codeString) throws FHIRException { 266 if (codeString == null || "".equals(codeString)) 267 return null; 268 if ("high".equals(codeString)) 269 return HIGH; 270 if ("moderate".equals(codeString)) 271 return MODERATE; 272 if ("low".equals(codeString)) 273 return LOW; 274 if (Configuration.isAcceptInvalidEnums()) 275 return null; 276 else 277 throw new FHIRException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 278 } 279 public String toCode() { 280 switch (this) { 281 case HIGH: return "high"; 282 case MODERATE: return "moderate"; 283 case LOW: return "low"; 284 case NULL: return null; 285 default: return "?"; 286 } 287 } 288 public String getSystem() { 289 switch (this) { 290 case HIGH: return "http://hl7.org/fhir/detectedissue-severity"; 291 case MODERATE: return "http://hl7.org/fhir/detectedissue-severity"; 292 case LOW: return "http://hl7.org/fhir/detectedissue-severity"; 293 case NULL: return null; 294 default: return "?"; 295 } 296 } 297 public String getDefinition() { 298 switch (this) { 299 case HIGH: return "Indicates the issue may be life-threatening or has the potential to cause permanent injury."; 300 case MODERATE: return "Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 301 case LOW: return "Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 302 case NULL: return null; 303 default: return "?"; 304 } 305 } 306 public String getDisplay() { 307 switch (this) { 308 case HIGH: return "High"; 309 case MODERATE: return "Moderate"; 310 case LOW: return "Low"; 311 case NULL: return null; 312 default: return "?"; 313 } 314 } 315 } 316 317 public static class DetectedIssueSeverityEnumFactory implements EnumFactory<DetectedIssueSeverity> { 318 public DetectedIssueSeverity fromCode(String codeString) throws IllegalArgumentException { 319 if (codeString == null || "".equals(codeString)) 320 if (codeString == null || "".equals(codeString)) 321 return null; 322 if ("high".equals(codeString)) 323 return DetectedIssueSeverity.HIGH; 324 if ("moderate".equals(codeString)) 325 return DetectedIssueSeverity.MODERATE; 326 if ("low".equals(codeString)) 327 return DetectedIssueSeverity.LOW; 328 throw new IllegalArgumentException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 329 } 330 public Enumeration<DetectedIssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 331 if (code == null) 332 return null; 333 if (code.isEmpty()) 334 return new Enumeration<DetectedIssueSeverity>(this); 335 String codeString = code.asStringValue(); 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("high".equals(codeString)) 339 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.HIGH); 340 if ("moderate".equals(codeString)) 341 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.MODERATE); 342 if ("low".equals(codeString)) 343 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.LOW); 344 throw new FHIRException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 345 } 346 public String toCode(DetectedIssueSeverity code) { 347 if (code == DetectedIssueSeverity.NULL) 348 return null; 349 if (code == DetectedIssueSeverity.HIGH) 350 return "high"; 351 if (code == DetectedIssueSeverity.MODERATE) 352 return "moderate"; 353 if (code == DetectedIssueSeverity.LOW) 354 return "low"; 355 return "?"; 356 } 357 public String toSystem(DetectedIssueSeverity code) { 358 return code.getSystem(); 359 } 360 } 361 362 @Block() 363 public static class DetectedIssueMitigationComponent extends BackboneElement implements IBaseBackboneElement { 364 /** 365 * Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue. 366 */ 367 @Child(name = "action", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 368 @Description(shortDefinition="What mitigation?", formalDefinition="Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue." ) 369 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-mitigation-action") 370 protected CodeableConcept action; 371 372 /** 373 * Indicates when the mitigating action was documented. 374 */ 375 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 376 @Description(shortDefinition="Date committed", formalDefinition="Indicates when the mitigating action was documented." ) 377 protected DateTimeType date; 378 379 /** 380 * Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring. 381 */ 382 @Child(name = "author", type = {Practitioner.class}, order=3, min=0, max=1, modifier=false, summary=false) 383 @Description(shortDefinition="Who is committing?", formalDefinition="Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring." ) 384 protected Reference author; 385 386 /** 387 * The actual object that is the target of the reference (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 388 */ 389 protected Practitioner authorTarget; 390 391 private static final long serialVersionUID = -1994768436L; 392 393 /** 394 * Constructor 395 */ 396 public DetectedIssueMitigationComponent() { 397 super(); 398 } 399 400 /** 401 * Constructor 402 */ 403 public DetectedIssueMitigationComponent(CodeableConcept action) { 404 super(); 405 this.action = action; 406 } 407 408 /** 409 * @return {@link #action} (Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.) 410 */ 411 public CodeableConcept getAction() { 412 if (this.action == null) 413 if (Configuration.errorOnAutoCreate()) 414 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.action"); 415 else if (Configuration.doAutoCreate()) 416 this.action = new CodeableConcept(); // cc 417 return this.action; 418 } 419 420 public boolean hasAction() { 421 return this.action != null && !this.action.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #action} (Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.) 426 */ 427 public DetectedIssueMitigationComponent setAction(CodeableConcept value) { 428 this.action = value; 429 return this; 430 } 431 432 /** 433 * @return {@link #date} (Indicates when the mitigating action was documented.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 434 */ 435 public DateTimeType getDateElement() { 436 if (this.date == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.date"); 439 else if (Configuration.doAutoCreate()) 440 this.date = new DateTimeType(); // bb 441 return this.date; 442 } 443 444 public boolean hasDateElement() { 445 return this.date != null && !this.date.isEmpty(); 446 } 447 448 public boolean hasDate() { 449 return this.date != null && !this.date.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #date} (Indicates when the mitigating action was documented.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 454 */ 455 public DetectedIssueMitigationComponent setDateElement(DateTimeType value) { 456 this.date = value; 457 return this; 458 } 459 460 /** 461 * @return Indicates when the mitigating action was documented. 462 */ 463 public Date getDate() { 464 return this.date == null ? null : this.date.getValue(); 465 } 466 467 /** 468 * @param value Indicates when the mitigating action was documented. 469 */ 470 public DetectedIssueMitigationComponent setDate(Date value) { 471 if (value == null) 472 this.date = null; 473 else { 474 if (this.date == null) 475 this.date = new DateTimeType(); 476 this.date.setValue(value); 477 } 478 return this; 479 } 480 481 /** 482 * @return {@link #author} (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 483 */ 484 public Reference getAuthor() { 485 if (this.author == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 488 else if (Configuration.doAutoCreate()) 489 this.author = new Reference(); // cc 490 return this.author; 491 } 492 493 public boolean hasAuthor() { 494 return this.author != null && !this.author.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #author} (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 499 */ 500 public DetectedIssueMitigationComponent setAuthor(Reference value) { 501 this.author = value; 502 return this; 503 } 504 505 /** 506 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 507 */ 508 public Practitioner getAuthorTarget() { 509 if (this.authorTarget == null) 510 if (Configuration.errorOnAutoCreate()) 511 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 512 else if (Configuration.doAutoCreate()) 513 this.authorTarget = new Practitioner(); // aa 514 return this.authorTarget; 515 } 516 517 /** 518 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 519 */ 520 public DetectedIssueMitigationComponent setAuthorTarget(Practitioner value) { 521 this.authorTarget = value; 522 return this; 523 } 524 525 protected void listChildren(List<Property> children) { 526 super.listChildren(children); 527 children.add(new Property("action", "CodeableConcept", "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 0, 1, action)); 528 children.add(new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date)); 529 children.add(new Property("author", "Reference(Practitioner)", "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 0, 1, author)); 530 } 531 532 @Override 533 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 534 switch (_hash) { 535 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 0, 1, action); 536 case 3076014: /*date*/ return new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date); 537 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner)", "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 0, 1, author); 538 default: return super.getNamedProperty(_hash, _name, _checkValid); 539 } 540 541 } 542 543 @Override 544 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 545 switch (hash) { 546 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // CodeableConcept 547 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 548 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 549 default: return super.getProperty(hash, name, checkValid); 550 } 551 552 } 553 554 @Override 555 public Base setProperty(int hash, String name, Base value) throws FHIRException { 556 switch (hash) { 557 case -1422950858: // action 558 this.action = castToCodeableConcept(value); // CodeableConcept 559 return value; 560 case 3076014: // date 561 this.date = castToDateTime(value); // DateTimeType 562 return value; 563 case -1406328437: // author 564 this.author = castToReference(value); // Reference 565 return value; 566 default: return super.setProperty(hash, name, value); 567 } 568 569 } 570 571 @Override 572 public Base setProperty(String name, Base value) throws FHIRException { 573 if (name.equals("action")) { 574 this.action = castToCodeableConcept(value); // CodeableConcept 575 } else if (name.equals("date")) { 576 this.date = castToDateTime(value); // DateTimeType 577 } else if (name.equals("author")) { 578 this.author = castToReference(value); // Reference 579 } else 580 return super.setProperty(name, value); 581 return value; 582 } 583 584 @Override 585 public Base makeProperty(int hash, String name) throws FHIRException { 586 switch (hash) { 587 case -1422950858: return getAction(); 588 case 3076014: return getDateElement(); 589 case -1406328437: return getAuthor(); 590 default: return super.makeProperty(hash, name); 591 } 592 593 } 594 595 @Override 596 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 597 switch (hash) { 598 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 599 case 3076014: /*date*/ return new String[] {"dateTime"}; 600 case -1406328437: /*author*/ return new String[] {"Reference"}; 601 default: return super.getTypesForProperty(hash, name); 602 } 603 604 } 605 606 @Override 607 public Base addChild(String name) throws FHIRException { 608 if (name.equals("action")) { 609 this.action = new CodeableConcept(); 610 return this.action; 611 } 612 else if (name.equals("date")) { 613 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 614 } 615 else if (name.equals("author")) { 616 this.author = new Reference(); 617 return this.author; 618 } 619 else 620 return super.addChild(name); 621 } 622 623 public DetectedIssueMitigationComponent copy() { 624 DetectedIssueMitigationComponent dst = new DetectedIssueMitigationComponent(); 625 copyValues(dst); 626 dst.action = action == null ? null : action.copy(); 627 dst.date = date == null ? null : date.copy(); 628 dst.author = author == null ? null : author.copy(); 629 return dst; 630 } 631 632 @Override 633 public boolean equalsDeep(Base other_) { 634 if (!super.equalsDeep(other_)) 635 return false; 636 if (!(other_ instanceof DetectedIssueMitigationComponent)) 637 return false; 638 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 639 return compareDeep(action, o.action, true) && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) 640 ; 641 } 642 643 @Override 644 public boolean equalsShallow(Base other_) { 645 if (!super.equalsShallow(other_)) 646 return false; 647 if (!(other_ instanceof DetectedIssueMitigationComponent)) 648 return false; 649 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 650 return compareValues(date, o.date, true); 651 } 652 653 public boolean isEmpty() { 654 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, date, author); 655 } 656 657 public String fhirType() { 658 return "DetectedIssue.mitigation"; 659 660 } 661 662 } 663 664 /** 665 * Business identifier associated with the detected issue record. 666 */ 667 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 668 @Description(shortDefinition="Unique id for the detected issue", formalDefinition="Business identifier associated with the detected issue record." ) 669 protected Identifier identifier; 670 671 /** 672 * Indicates the status of the detected issue. 673 */ 674 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 675 @Description(shortDefinition="registered | preliminary | final | amended +", formalDefinition="Indicates the status of the detected issue." ) 676 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-status") 677 protected Enumeration<DetectedIssueStatus> status; 678 679 /** 680 * Identifies the general type of issue identified. 681 */ 682 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 683 @Description(shortDefinition="Issue Category, e.g. drug-drug, duplicate therapy, etc.", formalDefinition="Identifies the general type of issue identified." ) 684 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-category") 685 protected CodeableConcept category; 686 687 /** 688 * Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 689 */ 690 @Child(name = "severity", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 691 @Description(shortDefinition="high | moderate | low", formalDefinition="Indicates the degree of importance associated with the identified issue based on the potential impact on the patient." ) 692 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-severity") 693 protected Enumeration<DetectedIssueSeverity> severity; 694 695 /** 696 * Indicates the patient whose record the detected issue is associated with. 697 */ 698 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=true) 699 @Description(shortDefinition="Associated patient", formalDefinition="Indicates the patient whose record the detected issue is associated with." ) 700 protected Reference patient; 701 702 /** 703 * The actual object that is the target of the reference (Indicates the patient whose record the detected issue is associated with.) 704 */ 705 protected Patient patientTarget; 706 707 /** 708 * The date or date-time when the detected issue was initially identified. 709 */ 710 @Child(name = "date", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 711 @Description(shortDefinition="When identified", formalDefinition="The date or date-time when the detected issue was initially identified." ) 712 protected DateTimeType date; 713 714 /** 715 * Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review. 716 */ 717 @Child(name = "author", type = {Practitioner.class, Device.class}, order=6, min=0, max=1, modifier=false, summary=true) 718 @Description(shortDefinition="The provider or device that identified the issue", formalDefinition="Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review." ) 719 protected Reference author; 720 721 /** 722 * The actual object that is the target of the reference (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 723 */ 724 protected Resource authorTarget; 725 726 /** 727 * Indicates the resource representing the current activity or proposed activity that is potentially problematic. 728 */ 729 @Child(name = "implicated", type = {Reference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 730 @Description(shortDefinition="Problem resource", formalDefinition="Indicates the resource representing the current activity or proposed activity that is potentially problematic." ) 731 protected List<Reference> implicated; 732 /** 733 * The actual objects that are the target of the reference (Indicates the resource representing the current activity or proposed activity that is potentially problematic.) 734 */ 735 protected List<Resource> implicatedTarget; 736 737 738 /** 739 * A textual explanation of the detected issue. 740 */ 741 @Child(name = "detail", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 742 @Description(shortDefinition="Description and context", formalDefinition="A textual explanation of the detected issue." ) 743 protected StringType detail; 744 745 /** 746 * The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 747 */ 748 @Child(name = "reference", type = {UriType.class}, order=9, min=0, max=1, modifier=false, summary=false) 749 @Description(shortDefinition="Authority for issue", formalDefinition="The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified." ) 750 protected UriType reference; 751 752 /** 753 * Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action. 754 */ 755 @Child(name = "mitigation", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 756 @Description(shortDefinition="Step taken to address", formalDefinition="Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action." ) 757 protected List<DetectedIssueMitigationComponent> mitigation; 758 759 private static final long serialVersionUID = -1002889332L; 760 761 /** 762 * Constructor 763 */ 764 public DetectedIssue() { 765 super(); 766 } 767 768 /** 769 * Constructor 770 */ 771 public DetectedIssue(Enumeration<DetectedIssueStatus> status) { 772 super(); 773 this.status = status; 774 } 775 776 /** 777 * @return {@link #identifier} (Business identifier associated with the detected issue record.) 778 */ 779 public Identifier getIdentifier() { 780 if (this.identifier == null) 781 if (Configuration.errorOnAutoCreate()) 782 throw new Error("Attempt to auto-create DetectedIssue.identifier"); 783 else if (Configuration.doAutoCreate()) 784 this.identifier = new Identifier(); // cc 785 return this.identifier; 786 } 787 788 public boolean hasIdentifier() { 789 return this.identifier != null && !this.identifier.isEmpty(); 790 } 791 792 /** 793 * @param value {@link #identifier} (Business identifier associated with the detected issue record.) 794 */ 795 public DetectedIssue setIdentifier(Identifier value) { 796 this.identifier = value; 797 return this; 798 } 799 800 /** 801 * @return {@link #status} (Indicates the status of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 802 */ 803 public Enumeration<DetectedIssueStatus> getStatusElement() { 804 if (this.status == null) 805 if (Configuration.errorOnAutoCreate()) 806 throw new Error("Attempt to auto-create DetectedIssue.status"); 807 else if (Configuration.doAutoCreate()) 808 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); // bb 809 return this.status; 810 } 811 812 public boolean hasStatusElement() { 813 return this.status != null && !this.status.isEmpty(); 814 } 815 816 public boolean hasStatus() { 817 return this.status != null && !this.status.isEmpty(); 818 } 819 820 /** 821 * @param value {@link #status} (Indicates the status of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 822 */ 823 public DetectedIssue setStatusElement(Enumeration<DetectedIssueStatus> value) { 824 this.status = value; 825 return this; 826 } 827 828 /** 829 * @return Indicates the status of the detected issue. 830 */ 831 public DetectedIssueStatus getStatus() { 832 return this.status == null ? null : this.status.getValue(); 833 } 834 835 /** 836 * @param value Indicates the status of the detected issue. 837 */ 838 public DetectedIssue setStatus(DetectedIssueStatus value) { 839 if (this.status == null) 840 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); 841 this.status.setValue(value); 842 return this; 843 } 844 845 /** 846 * @return {@link #category} (Identifies the general type of issue identified.) 847 */ 848 public CodeableConcept getCategory() { 849 if (this.category == null) 850 if (Configuration.errorOnAutoCreate()) 851 throw new Error("Attempt to auto-create DetectedIssue.category"); 852 else if (Configuration.doAutoCreate()) 853 this.category = new CodeableConcept(); // cc 854 return this.category; 855 } 856 857 public boolean hasCategory() { 858 return this.category != null && !this.category.isEmpty(); 859 } 860 861 /** 862 * @param value {@link #category} (Identifies the general type of issue identified.) 863 */ 864 public DetectedIssue setCategory(CodeableConcept value) { 865 this.category = value; 866 return this; 867 } 868 869 /** 870 * @return {@link #severity} (Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 871 */ 872 public Enumeration<DetectedIssueSeverity> getSeverityElement() { 873 if (this.severity == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create DetectedIssue.severity"); 876 else if (Configuration.doAutoCreate()) 877 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); // bb 878 return this.severity; 879 } 880 881 public boolean hasSeverityElement() { 882 return this.severity != null && !this.severity.isEmpty(); 883 } 884 885 public boolean hasSeverity() { 886 return this.severity != null && !this.severity.isEmpty(); 887 } 888 889 /** 890 * @param value {@link #severity} (Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 891 */ 892 public DetectedIssue setSeverityElement(Enumeration<DetectedIssueSeverity> value) { 893 this.severity = value; 894 return this; 895 } 896 897 /** 898 * @return Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 899 */ 900 public DetectedIssueSeverity getSeverity() { 901 return this.severity == null ? null : this.severity.getValue(); 902 } 903 904 /** 905 * @param value Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 906 */ 907 public DetectedIssue setSeverity(DetectedIssueSeverity value) { 908 if (value == null) 909 this.severity = null; 910 else { 911 if (this.severity == null) 912 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); 913 this.severity.setValue(value); 914 } 915 return this; 916 } 917 918 /** 919 * @return {@link #patient} (Indicates the patient whose record the detected issue is associated with.) 920 */ 921 public Reference getPatient() { 922 if (this.patient == null) 923 if (Configuration.errorOnAutoCreate()) 924 throw new Error("Attempt to auto-create DetectedIssue.patient"); 925 else if (Configuration.doAutoCreate()) 926 this.patient = new Reference(); // cc 927 return this.patient; 928 } 929 930 public boolean hasPatient() { 931 return this.patient != null && !this.patient.isEmpty(); 932 } 933 934 /** 935 * @param value {@link #patient} (Indicates the patient whose record the detected issue is associated with.) 936 */ 937 public DetectedIssue setPatient(Reference value) { 938 this.patient = value; 939 return this; 940 } 941 942 /** 943 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the patient whose record the detected issue is associated with.) 944 */ 945 public Patient getPatientTarget() { 946 if (this.patientTarget == null) 947 if (Configuration.errorOnAutoCreate()) 948 throw new Error("Attempt to auto-create DetectedIssue.patient"); 949 else if (Configuration.doAutoCreate()) 950 this.patientTarget = new Patient(); // aa 951 return this.patientTarget; 952 } 953 954 /** 955 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the patient whose record the detected issue is associated with.) 956 */ 957 public DetectedIssue setPatientTarget(Patient value) { 958 this.patientTarget = value; 959 return this; 960 } 961 962 /** 963 * @return {@link #date} (The date or date-time when the detected issue was initially identified.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 964 */ 965 public DateTimeType getDateElement() { 966 if (this.date == null) 967 if (Configuration.errorOnAutoCreate()) 968 throw new Error("Attempt to auto-create DetectedIssue.date"); 969 else if (Configuration.doAutoCreate()) 970 this.date = new DateTimeType(); // bb 971 return this.date; 972 } 973 974 public boolean hasDateElement() { 975 return this.date != null && !this.date.isEmpty(); 976 } 977 978 public boolean hasDate() { 979 return this.date != null && !this.date.isEmpty(); 980 } 981 982 /** 983 * @param value {@link #date} (The date or date-time when the detected issue was initially identified.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 984 */ 985 public DetectedIssue setDateElement(DateTimeType value) { 986 this.date = value; 987 return this; 988 } 989 990 /** 991 * @return The date or date-time when the detected issue was initially identified. 992 */ 993 public Date getDate() { 994 return this.date == null ? null : this.date.getValue(); 995 } 996 997 /** 998 * @param value The date or date-time when the detected issue was initially identified. 999 */ 1000 public DetectedIssue setDate(Date value) { 1001 if (value == null) 1002 this.date = null; 1003 else { 1004 if (this.date == null) 1005 this.date = new DateTimeType(); 1006 this.date.setValue(value); 1007 } 1008 return this; 1009 } 1010 1011 /** 1012 * @return {@link #author} (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1013 */ 1014 public Reference getAuthor() { 1015 if (this.author == null) 1016 if (Configuration.errorOnAutoCreate()) 1017 throw new Error("Attempt to auto-create DetectedIssue.author"); 1018 else if (Configuration.doAutoCreate()) 1019 this.author = new Reference(); // cc 1020 return this.author; 1021 } 1022 1023 public boolean hasAuthor() { 1024 return this.author != null && !this.author.isEmpty(); 1025 } 1026 1027 /** 1028 * @param value {@link #author} (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1029 */ 1030 public DetectedIssue setAuthor(Reference value) { 1031 this.author = value; 1032 return this; 1033 } 1034 1035 /** 1036 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1037 */ 1038 public Resource getAuthorTarget() { 1039 return this.authorTarget; 1040 } 1041 1042 /** 1043 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1044 */ 1045 public DetectedIssue setAuthorTarget(Resource value) { 1046 this.authorTarget = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #implicated} (Indicates the resource representing the current activity or proposed activity that is potentially problematic.) 1052 */ 1053 public List<Reference> getImplicated() { 1054 if (this.implicated == null) 1055 this.implicated = new ArrayList<Reference>(); 1056 return this.implicated; 1057 } 1058 1059 /** 1060 * @return Returns a reference to <code>this</code> for easy method chaining 1061 */ 1062 public DetectedIssue setImplicated(List<Reference> theImplicated) { 1063 this.implicated = theImplicated; 1064 return this; 1065 } 1066 1067 public boolean hasImplicated() { 1068 if (this.implicated == null) 1069 return false; 1070 for (Reference item : this.implicated) 1071 if (!item.isEmpty()) 1072 return true; 1073 return false; 1074 } 1075 1076 public Reference addImplicated() { //3 1077 Reference t = new Reference(); 1078 if (this.implicated == null) 1079 this.implicated = new ArrayList<Reference>(); 1080 this.implicated.add(t); 1081 return t; 1082 } 1083 1084 public DetectedIssue addImplicated(Reference t) { //3 1085 if (t == null) 1086 return this; 1087 if (this.implicated == null) 1088 this.implicated = new ArrayList<Reference>(); 1089 this.implicated.add(t); 1090 return this; 1091 } 1092 1093 /** 1094 * @return The first repetition of repeating field {@link #implicated}, creating it if it does not already exist 1095 */ 1096 public Reference getImplicatedFirstRep() { 1097 if (getImplicated().isEmpty()) { 1098 addImplicated(); 1099 } 1100 return getImplicated().get(0); 1101 } 1102 1103 /** 1104 * @deprecated Use Reference#setResource(IBaseResource) instead 1105 */ 1106 @Deprecated 1107 public List<Resource> getImplicatedTarget() { 1108 if (this.implicatedTarget == null) 1109 this.implicatedTarget = new ArrayList<Resource>(); 1110 return this.implicatedTarget; 1111 } 1112 1113 /** 1114 * @return {@link #detail} (A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1115 */ 1116 public StringType getDetailElement() { 1117 if (this.detail == null) 1118 if (Configuration.errorOnAutoCreate()) 1119 throw new Error("Attempt to auto-create DetectedIssue.detail"); 1120 else if (Configuration.doAutoCreate()) 1121 this.detail = new StringType(); // bb 1122 return this.detail; 1123 } 1124 1125 public boolean hasDetailElement() { 1126 return this.detail != null && !this.detail.isEmpty(); 1127 } 1128 1129 public boolean hasDetail() { 1130 return this.detail != null && !this.detail.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #detail} (A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1135 */ 1136 public DetectedIssue setDetailElement(StringType value) { 1137 this.detail = value; 1138 return this; 1139 } 1140 1141 /** 1142 * @return A textual explanation of the detected issue. 1143 */ 1144 public String getDetail() { 1145 return this.detail == null ? null : this.detail.getValue(); 1146 } 1147 1148 /** 1149 * @param value A textual explanation of the detected issue. 1150 */ 1151 public DetectedIssue setDetail(String value) { 1152 if (Utilities.noString(value)) 1153 this.detail = null; 1154 else { 1155 if (this.detail == null) 1156 this.detail = new StringType(); 1157 this.detail.setValue(value); 1158 } 1159 return this; 1160 } 1161 1162 /** 1163 * @return {@link #reference} (The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1164 */ 1165 public UriType getReferenceElement() { 1166 if (this.reference == null) 1167 if (Configuration.errorOnAutoCreate()) 1168 throw new Error("Attempt to auto-create DetectedIssue.reference"); 1169 else if (Configuration.doAutoCreate()) 1170 this.reference = new UriType(); // bb 1171 return this.reference; 1172 } 1173 1174 public boolean hasReferenceElement() { 1175 return this.reference != null && !this.reference.isEmpty(); 1176 } 1177 1178 public boolean hasReference() { 1179 return this.reference != null && !this.reference.isEmpty(); 1180 } 1181 1182 /** 1183 * @param value {@link #reference} (The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1184 */ 1185 public DetectedIssue setReferenceElement(UriType value) { 1186 this.reference = value; 1187 return this; 1188 } 1189 1190 /** 1191 * @return The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 1192 */ 1193 public String getReference() { 1194 return this.reference == null ? null : this.reference.getValue(); 1195 } 1196 1197 /** 1198 * @param value The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 1199 */ 1200 public DetectedIssue setReference(String value) { 1201 if (Utilities.noString(value)) 1202 this.reference = null; 1203 else { 1204 if (this.reference == null) 1205 this.reference = new UriType(); 1206 this.reference.setValue(value); 1207 } 1208 return this; 1209 } 1210 1211 /** 1212 * @return {@link #mitigation} (Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.) 1213 */ 1214 public List<DetectedIssueMitigationComponent> getMitigation() { 1215 if (this.mitigation == null) 1216 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1217 return this.mitigation; 1218 } 1219 1220 /** 1221 * @return Returns a reference to <code>this</code> for easy method chaining 1222 */ 1223 public DetectedIssue setMitigation(List<DetectedIssueMitigationComponent> theMitigation) { 1224 this.mitigation = theMitigation; 1225 return this; 1226 } 1227 1228 public boolean hasMitigation() { 1229 if (this.mitigation == null) 1230 return false; 1231 for (DetectedIssueMitigationComponent item : this.mitigation) 1232 if (!item.isEmpty()) 1233 return true; 1234 return false; 1235 } 1236 1237 public DetectedIssueMitigationComponent addMitigation() { //3 1238 DetectedIssueMitigationComponent t = new DetectedIssueMitigationComponent(); 1239 if (this.mitigation == null) 1240 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1241 this.mitigation.add(t); 1242 return t; 1243 } 1244 1245 public DetectedIssue addMitigation(DetectedIssueMitigationComponent t) { //3 1246 if (t == null) 1247 return this; 1248 if (this.mitigation == null) 1249 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1250 this.mitigation.add(t); 1251 return this; 1252 } 1253 1254 /** 1255 * @return The first repetition of repeating field {@link #mitigation}, creating it if it does not already exist 1256 */ 1257 public DetectedIssueMitigationComponent getMitigationFirstRep() { 1258 if (getMitigation().isEmpty()) { 1259 addMitigation(); 1260 } 1261 return getMitigation().get(0); 1262 } 1263 1264 protected void listChildren(List<Property> children) { 1265 super.listChildren(children); 1266 children.add(new Property("identifier", "Identifier", "Business identifier associated with the detected issue record.", 0, 1, identifier)); 1267 children.add(new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status)); 1268 children.add(new Property("category", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1, category)); 1269 children.add(new Property("severity", "code", "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 0, 1, severity)); 1270 children.add(new Property("patient", "Reference(Patient)", "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient)); 1271 children.add(new Property("date", "dateTime", "The date or date-time when the detected issue was initially identified.", 0, 1, date)); 1272 children.add(new Property("author", "Reference(Practitioner|Device)", "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 0, 1, author)); 1273 children.add(new Property("implicated", "Reference(Any)", "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 0, java.lang.Integer.MAX_VALUE, implicated)); 1274 children.add(new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, detail)); 1275 children.add(new Property("reference", "uri", "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 0, 1, reference)); 1276 children.add(new Property("mitigation", "", "Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 0, java.lang.Integer.MAX_VALUE, mitigation)); 1277 } 1278 1279 @Override 1280 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1281 switch (_hash) { 1282 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier associated with the detected issue record.", 0, 1, identifier); 1283 case -892481550: /*status*/ return new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status); 1284 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1, category); 1285 case 1478300413: /*severity*/ return new Property("severity", "code", "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 0, 1, severity); 1286 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient); 1287 case 3076014: /*date*/ return new Property("date", "dateTime", "The date or date-time when the detected issue was initially identified.", 0, 1, date); 1288 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Device)", "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 0, 1, author); 1289 case -810216884: /*implicated*/ return new Property("implicated", "Reference(Any)", "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 0, java.lang.Integer.MAX_VALUE, implicated); 1290 case -1335224239: /*detail*/ return new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, detail); 1291 case -925155509: /*reference*/ return new Property("reference", "uri", "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 0, 1, reference); 1292 case 1293793087: /*mitigation*/ return new Property("mitigation", "", "Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 0, java.lang.Integer.MAX_VALUE, mitigation); 1293 default: return super.getNamedProperty(_hash, _name, _checkValid); 1294 } 1295 1296 } 1297 1298 @Override 1299 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1300 switch (hash) { 1301 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1302 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DetectedIssueStatus> 1303 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1304 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<DetectedIssueSeverity> 1305 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1306 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1307 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1308 case -810216884: /*implicated*/ return this.implicated == null ? new Base[0] : this.implicated.toArray(new Base[this.implicated.size()]); // Reference 1309 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // StringType 1310 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // UriType 1311 case 1293793087: /*mitigation*/ return this.mitigation == null ? new Base[0] : this.mitigation.toArray(new Base[this.mitigation.size()]); // DetectedIssueMitigationComponent 1312 default: return super.getProperty(hash, name, checkValid); 1313 } 1314 1315 } 1316 1317 @Override 1318 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1319 switch (hash) { 1320 case -1618432855: // identifier 1321 this.identifier = castToIdentifier(value); // Identifier 1322 return value; 1323 case -892481550: // status 1324 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 1325 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 1326 return value; 1327 case 50511102: // category 1328 this.category = castToCodeableConcept(value); // CodeableConcept 1329 return value; 1330 case 1478300413: // severity 1331 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 1332 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 1333 return value; 1334 case -791418107: // patient 1335 this.patient = castToReference(value); // Reference 1336 return value; 1337 case 3076014: // date 1338 this.date = castToDateTime(value); // DateTimeType 1339 return value; 1340 case -1406328437: // author 1341 this.author = castToReference(value); // Reference 1342 return value; 1343 case -810216884: // implicated 1344 this.getImplicated().add(castToReference(value)); // Reference 1345 return value; 1346 case -1335224239: // detail 1347 this.detail = castToString(value); // StringType 1348 return value; 1349 case -925155509: // reference 1350 this.reference = castToUri(value); // UriType 1351 return value; 1352 case 1293793087: // mitigation 1353 this.getMitigation().add((DetectedIssueMitigationComponent) value); // DetectedIssueMitigationComponent 1354 return value; 1355 default: return super.setProperty(hash, name, value); 1356 } 1357 1358 } 1359 1360 @Override 1361 public Base setProperty(String name, Base value) throws FHIRException { 1362 if (name.equals("identifier")) { 1363 this.identifier = castToIdentifier(value); // Identifier 1364 } else if (name.equals("status")) { 1365 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 1366 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 1367 } else if (name.equals("category")) { 1368 this.category = castToCodeableConcept(value); // CodeableConcept 1369 } else if (name.equals("severity")) { 1370 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 1371 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 1372 } else if (name.equals("patient")) { 1373 this.patient = castToReference(value); // Reference 1374 } else if (name.equals("date")) { 1375 this.date = castToDateTime(value); // DateTimeType 1376 } else if (name.equals("author")) { 1377 this.author = castToReference(value); // Reference 1378 } else if (name.equals("implicated")) { 1379 this.getImplicated().add(castToReference(value)); 1380 } else if (name.equals("detail")) { 1381 this.detail = castToString(value); // StringType 1382 } else if (name.equals("reference")) { 1383 this.reference = castToUri(value); // UriType 1384 } else if (name.equals("mitigation")) { 1385 this.getMitigation().add((DetectedIssueMitigationComponent) value); 1386 } else 1387 return super.setProperty(name, value); 1388 return value; 1389 } 1390 1391 @Override 1392 public Base makeProperty(int hash, String name) throws FHIRException { 1393 switch (hash) { 1394 case -1618432855: return getIdentifier(); 1395 case -892481550: return getStatusElement(); 1396 case 50511102: return getCategory(); 1397 case 1478300413: return getSeverityElement(); 1398 case -791418107: return getPatient(); 1399 case 3076014: return getDateElement(); 1400 case -1406328437: return getAuthor(); 1401 case -810216884: return addImplicated(); 1402 case -1335224239: return getDetailElement(); 1403 case -925155509: return getReferenceElement(); 1404 case 1293793087: return addMitigation(); 1405 default: return super.makeProperty(hash, name); 1406 } 1407 1408 } 1409 1410 @Override 1411 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1412 switch (hash) { 1413 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1414 case -892481550: /*status*/ return new String[] {"code"}; 1415 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1416 case 1478300413: /*severity*/ return new String[] {"code"}; 1417 case -791418107: /*patient*/ return new String[] {"Reference"}; 1418 case 3076014: /*date*/ return new String[] {"dateTime"}; 1419 case -1406328437: /*author*/ return new String[] {"Reference"}; 1420 case -810216884: /*implicated*/ return new String[] {"Reference"}; 1421 case -1335224239: /*detail*/ return new String[] {"string"}; 1422 case -925155509: /*reference*/ return new String[] {"uri"}; 1423 case 1293793087: /*mitigation*/ return new String[] {}; 1424 default: return super.getTypesForProperty(hash, name); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base addChild(String name) throws FHIRException { 1431 if (name.equals("identifier")) { 1432 this.identifier = new Identifier(); 1433 return this.identifier; 1434 } 1435 else if (name.equals("status")) { 1436 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.status"); 1437 } 1438 else if (name.equals("category")) { 1439 this.category = new CodeableConcept(); 1440 return this.category; 1441 } 1442 else if (name.equals("severity")) { 1443 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.severity"); 1444 } 1445 else if (name.equals("patient")) { 1446 this.patient = new Reference(); 1447 return this.patient; 1448 } 1449 else if (name.equals("date")) { 1450 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 1451 } 1452 else if (name.equals("author")) { 1453 this.author = new Reference(); 1454 return this.author; 1455 } 1456 else if (name.equals("implicated")) { 1457 return addImplicated(); 1458 } 1459 else if (name.equals("detail")) { 1460 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.detail"); 1461 } 1462 else if (name.equals("reference")) { 1463 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.reference"); 1464 } 1465 else if (name.equals("mitigation")) { 1466 return addMitigation(); 1467 } 1468 else 1469 return super.addChild(name); 1470 } 1471 1472 public String fhirType() { 1473 return "DetectedIssue"; 1474 1475 } 1476 1477 public DetectedIssue copy() { 1478 DetectedIssue dst = new DetectedIssue(); 1479 copyValues(dst); 1480 dst.identifier = identifier == null ? null : identifier.copy(); 1481 dst.status = status == null ? null : status.copy(); 1482 dst.category = category == null ? null : category.copy(); 1483 dst.severity = severity == null ? null : severity.copy(); 1484 dst.patient = patient == null ? null : patient.copy(); 1485 dst.date = date == null ? null : date.copy(); 1486 dst.author = author == null ? null : author.copy(); 1487 if (implicated != null) { 1488 dst.implicated = new ArrayList<Reference>(); 1489 for (Reference i : implicated) 1490 dst.implicated.add(i.copy()); 1491 }; 1492 dst.detail = detail == null ? null : detail.copy(); 1493 dst.reference = reference == null ? null : reference.copy(); 1494 if (mitigation != null) { 1495 dst.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1496 for (DetectedIssueMitigationComponent i : mitigation) 1497 dst.mitigation.add(i.copy()); 1498 }; 1499 return dst; 1500 } 1501 1502 protected DetectedIssue typedCopy() { 1503 return copy(); 1504 } 1505 1506 @Override 1507 public boolean equalsDeep(Base other_) { 1508 if (!super.equalsDeep(other_)) 1509 return false; 1510 if (!(other_ instanceof DetectedIssue)) 1511 return false; 1512 DetectedIssue o = (DetectedIssue) other_; 1513 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1514 && compareDeep(severity, o.severity, true) && compareDeep(patient, o.patient, true) && compareDeep(date, o.date, true) 1515 && compareDeep(author, o.author, true) && compareDeep(implicated, o.implicated, true) && compareDeep(detail, o.detail, true) 1516 && compareDeep(reference, o.reference, true) && compareDeep(mitigation, o.mitigation, true); 1517 } 1518 1519 @Override 1520 public boolean equalsShallow(Base other_) { 1521 if (!super.equalsShallow(other_)) 1522 return false; 1523 if (!(other_ instanceof DetectedIssue)) 1524 return false; 1525 DetectedIssue o = (DetectedIssue) other_; 1526 return compareValues(status, o.status, true) && compareValues(severity, o.severity, true) && compareValues(date, o.date, true) 1527 && compareValues(detail, o.detail, true) && compareValues(reference, o.reference, true); 1528 } 1529 1530 public boolean isEmpty() { 1531 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1532 , severity, patient, date, author, implicated, detail, reference, mitigation 1533 ); 1534 } 1535 1536 @Override 1537 public ResourceType getResourceType() { 1538 return ResourceType.DetectedIssue; 1539 } 1540 1541 /** 1542 * Search parameter: <b>date</b> 1543 * <p> 1544 * Description: <b>When identified</b><br> 1545 * Type: <b>date</b><br> 1546 * Path: <b>DetectedIssue.date</b><br> 1547 * </p> 1548 */ 1549 @SearchParamDefinition(name="date", path="DetectedIssue.date", description="When identified", type="date" ) 1550 public static final String SP_DATE = "date"; 1551 /** 1552 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1553 * <p> 1554 * Description: <b>When identified</b><br> 1555 * Type: <b>date</b><br> 1556 * Path: <b>DetectedIssue.date</b><br> 1557 * </p> 1558 */ 1559 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1560 1561 /** 1562 * Search parameter: <b>identifier</b> 1563 * <p> 1564 * Description: <b>Unique id for the detected issue</b><br> 1565 * Type: <b>token</b><br> 1566 * Path: <b>DetectedIssue.identifier</b><br> 1567 * </p> 1568 */ 1569 @SearchParamDefinition(name="identifier", path="DetectedIssue.identifier", description="Unique id for the detected issue", type="token" ) 1570 public static final String SP_IDENTIFIER = "identifier"; 1571 /** 1572 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1573 * <p> 1574 * Description: <b>Unique id for the detected issue</b><br> 1575 * Type: <b>token</b><br> 1576 * Path: <b>DetectedIssue.identifier</b><br> 1577 * </p> 1578 */ 1579 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1580 1581 /** 1582 * Search parameter: <b>patient</b> 1583 * <p> 1584 * Description: <b>Associated patient</b><br> 1585 * Type: <b>reference</b><br> 1586 * Path: <b>DetectedIssue.patient</b><br> 1587 * </p> 1588 */ 1589 @SearchParamDefinition(name="patient", path="DetectedIssue.patient", description="Associated patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1590 public static final String SP_PATIENT = "patient"; 1591 /** 1592 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1593 * <p> 1594 * Description: <b>Associated patient</b><br> 1595 * Type: <b>reference</b><br> 1596 * Path: <b>DetectedIssue.patient</b><br> 1597 * </p> 1598 */ 1599 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1600 1601/** 1602 * Constant for fluent queries to be used to add include statements. Specifies 1603 * the path value of "<b>DetectedIssue:patient</b>". 1604 */ 1605 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DetectedIssue:patient").toLocked(); 1606 1607 /** 1608 * Search parameter: <b>author</b> 1609 * <p> 1610 * Description: <b>The provider or device that identified the issue</b><br> 1611 * Type: <b>reference</b><br> 1612 * Path: <b>DetectedIssue.author</b><br> 1613 * </p> 1614 */ 1615 @SearchParamDefinition(name="author", path="DetectedIssue.author", description="The provider or device that identified the issue", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Practitioner.class } ) 1616 public static final String SP_AUTHOR = "author"; 1617 /** 1618 * <b>Fluent Client</b> search parameter constant for <b>author</b> 1619 * <p> 1620 * Description: <b>The provider or device that identified the issue</b><br> 1621 * Type: <b>reference</b><br> 1622 * Path: <b>DetectedIssue.author</b><br> 1623 * </p> 1624 */ 1625 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 1626 1627/** 1628 * Constant for fluent queries to be used to add include statements. Specifies 1629 * the path value of "<b>DetectedIssue:author</b>". 1630 */ 1631 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("DetectedIssue:author").toLocked(); 1632 1633 /** 1634 * Search parameter: <b>implicated</b> 1635 * <p> 1636 * Description: <b>Problem resource</b><br> 1637 * Type: <b>reference</b><br> 1638 * Path: <b>DetectedIssue.implicated</b><br> 1639 * </p> 1640 */ 1641 @SearchParamDefinition(name="implicated", path="DetectedIssue.implicated", description="Problem resource", type="reference" ) 1642 public static final String SP_IMPLICATED = "implicated"; 1643 /** 1644 * <b>Fluent Client</b> search parameter constant for <b>implicated</b> 1645 * <p> 1646 * Description: <b>Problem resource</b><br> 1647 * Type: <b>reference</b><br> 1648 * Path: <b>DetectedIssue.implicated</b><br> 1649 * </p> 1650 */ 1651 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMPLICATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_IMPLICATED); 1652 1653/** 1654 * Constant for fluent queries to be used to add include statements. Specifies 1655 * the path value of "<b>DetectedIssue:implicated</b>". 1656 */ 1657 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMPLICATED = new ca.uhn.fhir.model.api.Include("DetectedIssue:implicated").toLocked(); 1658 1659 /** 1660 * Search parameter: <b>category</b> 1661 * <p> 1662 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, etc.</b><br> 1663 * Type: <b>token</b><br> 1664 * Path: <b>DetectedIssue.category</b><br> 1665 * </p> 1666 */ 1667 @SearchParamDefinition(name="category", path="DetectedIssue.category", description="Issue Category, e.g. drug-drug, duplicate therapy, etc.", type="token" ) 1668 public static final String SP_CATEGORY = "category"; 1669 /** 1670 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1671 * <p> 1672 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, etc.</b><br> 1673 * Type: <b>token</b><br> 1674 * Path: <b>DetectedIssue.category</b><br> 1675 * </p> 1676 */ 1677 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1678 1679 1680}