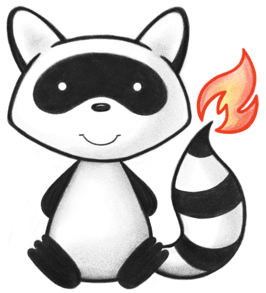
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 050 */ 051@ResourceDef(name="DetectedIssue", profile="http://hl7.org/fhir/Profile/DetectedIssue") 052public class DetectedIssue extends DomainResource { 053 054 public enum DetectedIssueStatus { 055 /** 056 * The existence of the observation is registered, but there is no result yet available. 057 */ 058 REGISTERED, 059 /** 060 * This is an initial or interim observation: data may be incomplete or unverified. 061 */ 062 PRELIMINARY, 063 /** 064 * The observation is complete. 065 */ 066 FINAL, 067 /** 068 * Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections. 069 */ 070 AMENDED, 071 /** 072 * Subsequent to being Final, the observation has been modified to correct an error in the test result. 073 */ 074 CORRECTED, 075 /** 076 * The observation is unavailable because the measurement was not started or not completed (also sometimes called "aborted"). 077 */ 078 CANCELLED, 079 /** 080 * The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 081 */ 082 ENTEREDINERROR, 083 /** 084 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, but the authoring system does not know which. 085 */ 086 UNKNOWN, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 public static DetectedIssueStatus fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("registered".equals(codeString)) 095 return REGISTERED; 096 if ("preliminary".equals(codeString)) 097 return PRELIMINARY; 098 if ("final".equals(codeString)) 099 return FINAL; 100 if ("amended".equals(codeString)) 101 return AMENDED; 102 if ("corrected".equals(codeString)) 103 return CORRECTED; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("entered-in-error".equals(codeString)) 107 return ENTEREDINERROR; 108 if ("unknown".equals(codeString)) 109 return UNKNOWN; 110 if (Configuration.isAcceptInvalidEnums()) 111 return null; 112 else 113 throw new FHIRException("Unknown DetectedIssueStatus code '"+codeString+"'"); 114 } 115 public String toCode() { 116 switch (this) { 117 case REGISTERED: return "registered"; 118 case PRELIMINARY: return "preliminary"; 119 case FINAL: return "final"; 120 case AMENDED: return "amended"; 121 case CORRECTED: return "corrected"; 122 case CANCELLED: return "cancelled"; 123 case ENTEREDINERROR: return "entered-in-error"; 124 case UNKNOWN: return "unknown"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getSystem() { 130 switch (this) { 131 case REGISTERED: return "http://hl7.org/fhir/observation-status"; 132 case PRELIMINARY: return "http://hl7.org/fhir/observation-status"; 133 case FINAL: return "http://hl7.org/fhir/observation-status"; 134 case AMENDED: return "http://hl7.org/fhir/observation-status"; 135 case CORRECTED: return "http://hl7.org/fhir/observation-status"; 136 case CANCELLED: return "http://hl7.org/fhir/observation-status"; 137 case ENTEREDINERROR: return "http://hl7.org/fhir/observation-status"; 138 case UNKNOWN: return "http://hl7.org/fhir/observation-status"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 public String getDefinition() { 144 switch (this) { 145 case REGISTERED: return "The existence of the observation is registered, but there is no result yet available."; 146 case PRELIMINARY: return "This is an initial or interim observation: data may be incomplete or unverified."; 147 case FINAL: return "The observation is complete."; 148 case AMENDED: return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 149 case CORRECTED: return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 150 case CANCELLED: return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 151 case ENTEREDINERROR: return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 152 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring system does not know which."; 153 case NULL: return null; 154 default: return "?"; 155 } 156 } 157 public String getDisplay() { 158 switch (this) { 159 case REGISTERED: return "Registered"; 160 case PRELIMINARY: return "Preliminary"; 161 case FINAL: return "Final"; 162 case AMENDED: return "Amended"; 163 case CORRECTED: return "Corrected"; 164 case CANCELLED: return "Cancelled"; 165 case ENTEREDINERROR: return "Entered in Error"; 166 case UNKNOWN: return "Unknown"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 } 172 173 public static class DetectedIssueStatusEnumFactory implements EnumFactory<DetectedIssueStatus> { 174 public DetectedIssueStatus fromCode(String codeString) throws IllegalArgumentException { 175 if (codeString == null || "".equals(codeString)) 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("registered".equals(codeString)) 179 return DetectedIssueStatus.REGISTERED; 180 if ("preliminary".equals(codeString)) 181 return DetectedIssueStatus.PRELIMINARY; 182 if ("final".equals(codeString)) 183 return DetectedIssueStatus.FINAL; 184 if ("amended".equals(codeString)) 185 return DetectedIssueStatus.AMENDED; 186 if ("corrected".equals(codeString)) 187 return DetectedIssueStatus.CORRECTED; 188 if ("cancelled".equals(codeString)) 189 return DetectedIssueStatus.CANCELLED; 190 if ("entered-in-error".equals(codeString)) 191 return DetectedIssueStatus.ENTEREDINERROR; 192 if ("unknown".equals(codeString)) 193 return DetectedIssueStatus.UNKNOWN; 194 throw new IllegalArgumentException("Unknown DetectedIssueStatus code '"+codeString+"'"); 195 } 196 public Enumeration<DetectedIssueStatus> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<DetectedIssueStatus>(this); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("registered".equals(codeString)) 205 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.REGISTERED); 206 if ("preliminary".equals(codeString)) 207 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.PRELIMINARY); 208 if ("final".equals(codeString)) 209 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.FINAL); 210 if ("amended".equals(codeString)) 211 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.AMENDED); 212 if ("corrected".equals(codeString)) 213 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CORRECTED); 214 if ("cancelled".equals(codeString)) 215 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CANCELLED); 216 if ("entered-in-error".equals(codeString)) 217 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.ENTEREDINERROR); 218 if ("unknown".equals(codeString)) 219 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.UNKNOWN); 220 throw new FHIRException("Unknown DetectedIssueStatus code '"+codeString+"'"); 221 } 222 public String toCode(DetectedIssueStatus code) { 223 if (code == DetectedIssueStatus.REGISTERED) 224 return "registered"; 225 if (code == DetectedIssueStatus.PRELIMINARY) 226 return "preliminary"; 227 if (code == DetectedIssueStatus.FINAL) 228 return "final"; 229 if (code == DetectedIssueStatus.AMENDED) 230 return "amended"; 231 if (code == DetectedIssueStatus.CORRECTED) 232 return "corrected"; 233 if (code == DetectedIssueStatus.CANCELLED) 234 return "cancelled"; 235 if (code == DetectedIssueStatus.ENTEREDINERROR) 236 return "entered-in-error"; 237 if (code == DetectedIssueStatus.UNKNOWN) 238 return "unknown"; 239 return "?"; 240 } 241 public String toSystem(DetectedIssueStatus code) { 242 return code.getSystem(); 243 } 244 } 245 246 public enum DetectedIssueSeverity { 247 /** 248 * Indicates the issue may be life-threatening or has the potential to cause permanent injury. 249 */ 250 HIGH, 251 /** 252 * Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury. 253 */ 254 MODERATE, 255 /** 256 * Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject. 257 */ 258 LOW, 259 /** 260 * added to help the parsers with the generic types 261 */ 262 NULL; 263 public static DetectedIssueSeverity fromCode(String codeString) throws FHIRException { 264 if (codeString == null || "".equals(codeString)) 265 return null; 266 if ("high".equals(codeString)) 267 return HIGH; 268 if ("moderate".equals(codeString)) 269 return MODERATE; 270 if ("low".equals(codeString)) 271 return LOW; 272 if (Configuration.isAcceptInvalidEnums()) 273 return null; 274 else 275 throw new FHIRException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 276 } 277 public String toCode() { 278 switch (this) { 279 case HIGH: return "high"; 280 case MODERATE: return "moderate"; 281 case LOW: return "low"; 282 case NULL: return null; 283 default: return "?"; 284 } 285 } 286 public String getSystem() { 287 switch (this) { 288 case HIGH: return "http://hl7.org/fhir/detectedissue-severity"; 289 case MODERATE: return "http://hl7.org/fhir/detectedissue-severity"; 290 case LOW: return "http://hl7.org/fhir/detectedissue-severity"; 291 case NULL: return null; 292 default: return "?"; 293 } 294 } 295 public String getDefinition() { 296 switch (this) { 297 case HIGH: return "Indicates the issue may be life-threatening or has the potential to cause permanent injury."; 298 case MODERATE: return "Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 299 case LOW: return "Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 300 case NULL: return null; 301 default: return "?"; 302 } 303 } 304 public String getDisplay() { 305 switch (this) { 306 case HIGH: return "High"; 307 case MODERATE: return "Moderate"; 308 case LOW: return "Low"; 309 case NULL: return null; 310 default: return "?"; 311 } 312 } 313 } 314 315 public static class DetectedIssueSeverityEnumFactory implements EnumFactory<DetectedIssueSeverity> { 316 public DetectedIssueSeverity fromCode(String codeString) throws IllegalArgumentException { 317 if (codeString == null || "".equals(codeString)) 318 if (codeString == null || "".equals(codeString)) 319 return null; 320 if ("high".equals(codeString)) 321 return DetectedIssueSeverity.HIGH; 322 if ("moderate".equals(codeString)) 323 return DetectedIssueSeverity.MODERATE; 324 if ("low".equals(codeString)) 325 return DetectedIssueSeverity.LOW; 326 throw new IllegalArgumentException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 327 } 328 public Enumeration<DetectedIssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 329 if (code == null) 330 return null; 331 if (code.isEmpty()) 332 return new Enumeration<DetectedIssueSeverity>(this); 333 String codeString = code.asStringValue(); 334 if (codeString == null || "".equals(codeString)) 335 return null; 336 if ("high".equals(codeString)) 337 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.HIGH); 338 if ("moderate".equals(codeString)) 339 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.MODERATE); 340 if ("low".equals(codeString)) 341 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.LOW); 342 throw new FHIRException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 343 } 344 public String toCode(DetectedIssueSeverity code) { 345 if (code == DetectedIssueSeverity.HIGH) 346 return "high"; 347 if (code == DetectedIssueSeverity.MODERATE) 348 return "moderate"; 349 if (code == DetectedIssueSeverity.LOW) 350 return "low"; 351 return "?"; 352 } 353 public String toSystem(DetectedIssueSeverity code) { 354 return code.getSystem(); 355 } 356 } 357 358 @Block() 359 public static class DetectedIssueMitigationComponent extends BackboneElement implements IBaseBackboneElement { 360 /** 361 * Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue. 362 */ 363 @Child(name = "action", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 364 @Description(shortDefinition="What mitigation?", formalDefinition="Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue." ) 365 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-mitigation-action") 366 protected CodeableConcept action; 367 368 /** 369 * Indicates when the mitigating action was documented. 370 */ 371 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 372 @Description(shortDefinition="Date committed", formalDefinition="Indicates when the mitigating action was documented." ) 373 protected DateTimeType date; 374 375 /** 376 * Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring. 377 */ 378 @Child(name = "author", type = {Practitioner.class}, order=3, min=0, max=1, modifier=false, summary=false) 379 @Description(shortDefinition="Who is committing?", formalDefinition="Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring." ) 380 protected Reference author; 381 382 /** 383 * The actual object that is the target of the reference (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 384 */ 385 protected Practitioner authorTarget; 386 387 private static final long serialVersionUID = -1994768436L; 388 389 /** 390 * Constructor 391 */ 392 public DetectedIssueMitigationComponent() { 393 super(); 394 } 395 396 /** 397 * Constructor 398 */ 399 public DetectedIssueMitigationComponent(CodeableConcept action) { 400 super(); 401 this.action = action; 402 } 403 404 /** 405 * @return {@link #action} (Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.) 406 */ 407 public CodeableConcept getAction() { 408 if (this.action == null) 409 if (Configuration.errorOnAutoCreate()) 410 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.action"); 411 else if (Configuration.doAutoCreate()) 412 this.action = new CodeableConcept(); // cc 413 return this.action; 414 } 415 416 public boolean hasAction() { 417 return this.action != null && !this.action.isEmpty(); 418 } 419 420 /** 421 * @param value {@link #action} (Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.) 422 */ 423 public DetectedIssueMitigationComponent setAction(CodeableConcept value) { 424 this.action = value; 425 return this; 426 } 427 428 /** 429 * @return {@link #date} (Indicates when the mitigating action was documented.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 430 */ 431 public DateTimeType getDateElement() { 432 if (this.date == null) 433 if (Configuration.errorOnAutoCreate()) 434 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.date"); 435 else if (Configuration.doAutoCreate()) 436 this.date = new DateTimeType(); // bb 437 return this.date; 438 } 439 440 public boolean hasDateElement() { 441 return this.date != null && !this.date.isEmpty(); 442 } 443 444 public boolean hasDate() { 445 return this.date != null && !this.date.isEmpty(); 446 } 447 448 /** 449 * @param value {@link #date} (Indicates when the mitigating action was documented.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 450 */ 451 public DetectedIssueMitigationComponent setDateElement(DateTimeType value) { 452 this.date = value; 453 return this; 454 } 455 456 /** 457 * @return Indicates when the mitigating action was documented. 458 */ 459 public Date getDate() { 460 return this.date == null ? null : this.date.getValue(); 461 } 462 463 /** 464 * @param value Indicates when the mitigating action was documented. 465 */ 466 public DetectedIssueMitigationComponent setDate(Date value) { 467 if (value == null) 468 this.date = null; 469 else { 470 if (this.date == null) 471 this.date = new DateTimeType(); 472 this.date.setValue(value); 473 } 474 return this; 475 } 476 477 /** 478 * @return {@link #author} (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 479 */ 480 public Reference getAuthor() { 481 if (this.author == null) 482 if (Configuration.errorOnAutoCreate()) 483 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 484 else if (Configuration.doAutoCreate()) 485 this.author = new Reference(); // cc 486 return this.author; 487 } 488 489 public boolean hasAuthor() { 490 return this.author != null && !this.author.isEmpty(); 491 } 492 493 /** 494 * @param value {@link #author} (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 495 */ 496 public DetectedIssueMitigationComponent setAuthor(Reference value) { 497 this.author = value; 498 return this; 499 } 500 501 /** 502 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 503 */ 504 public Practitioner getAuthorTarget() { 505 if (this.authorTarget == null) 506 if (Configuration.errorOnAutoCreate()) 507 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 508 else if (Configuration.doAutoCreate()) 509 this.authorTarget = new Practitioner(); // aa 510 return this.authorTarget; 511 } 512 513 /** 514 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 515 */ 516 public DetectedIssueMitigationComponent setAuthorTarget(Practitioner value) { 517 this.authorTarget = value; 518 return this; 519 } 520 521 protected void listChildren(List<Property> children) { 522 super.listChildren(children); 523 children.add(new Property("action", "CodeableConcept", "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 0, 1, action)); 524 children.add(new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date)); 525 children.add(new Property("author", "Reference(Practitioner)", "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 0, 1, author)); 526 } 527 528 @Override 529 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 530 switch (_hash) { 531 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 0, 1, action); 532 case 3076014: /*date*/ return new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date); 533 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner)", "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 0, 1, author); 534 default: return super.getNamedProperty(_hash, _name, _checkValid); 535 } 536 537 } 538 539 @Override 540 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 541 switch (hash) { 542 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // CodeableConcept 543 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 544 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 545 default: return super.getProperty(hash, name, checkValid); 546 } 547 548 } 549 550 @Override 551 public Base setProperty(int hash, String name, Base value) throws FHIRException { 552 switch (hash) { 553 case -1422950858: // action 554 this.action = castToCodeableConcept(value); // CodeableConcept 555 return value; 556 case 3076014: // date 557 this.date = castToDateTime(value); // DateTimeType 558 return value; 559 case -1406328437: // author 560 this.author = castToReference(value); // Reference 561 return value; 562 default: return super.setProperty(hash, name, value); 563 } 564 565 } 566 567 @Override 568 public Base setProperty(String name, Base value) throws FHIRException { 569 if (name.equals("action")) { 570 this.action = castToCodeableConcept(value); // CodeableConcept 571 } else if (name.equals("date")) { 572 this.date = castToDateTime(value); // DateTimeType 573 } else if (name.equals("author")) { 574 this.author = castToReference(value); // Reference 575 } else 576 return super.setProperty(name, value); 577 return value; 578 } 579 580 @Override 581 public Base makeProperty(int hash, String name) throws FHIRException { 582 switch (hash) { 583 case -1422950858: return getAction(); 584 case 3076014: return getDateElement(); 585 case -1406328437: return getAuthor(); 586 default: return super.makeProperty(hash, name); 587 } 588 589 } 590 591 @Override 592 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 593 switch (hash) { 594 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 595 case 3076014: /*date*/ return new String[] {"dateTime"}; 596 case -1406328437: /*author*/ return new String[] {"Reference"}; 597 default: return super.getTypesForProperty(hash, name); 598 } 599 600 } 601 602 @Override 603 public Base addChild(String name) throws FHIRException { 604 if (name.equals("action")) { 605 this.action = new CodeableConcept(); 606 return this.action; 607 } 608 else if (name.equals("date")) { 609 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 610 } 611 else if (name.equals("author")) { 612 this.author = new Reference(); 613 return this.author; 614 } 615 else 616 return super.addChild(name); 617 } 618 619 public DetectedIssueMitigationComponent copy() { 620 DetectedIssueMitigationComponent dst = new DetectedIssueMitigationComponent(); 621 copyValues(dst); 622 dst.action = action == null ? null : action.copy(); 623 dst.date = date == null ? null : date.copy(); 624 dst.author = author == null ? null : author.copy(); 625 return dst; 626 } 627 628 @Override 629 public boolean equalsDeep(Base other_) { 630 if (!super.equalsDeep(other_)) 631 return false; 632 if (!(other_ instanceof DetectedIssueMitigationComponent)) 633 return false; 634 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 635 return compareDeep(action, o.action, true) && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) 636 ; 637 } 638 639 @Override 640 public boolean equalsShallow(Base other_) { 641 if (!super.equalsShallow(other_)) 642 return false; 643 if (!(other_ instanceof DetectedIssueMitigationComponent)) 644 return false; 645 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 646 return compareValues(date, o.date, true); 647 } 648 649 public boolean isEmpty() { 650 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, date, author); 651 } 652 653 public String fhirType() { 654 return "DetectedIssue.mitigation"; 655 656 } 657 658 } 659 660 /** 661 * Business identifier associated with the detected issue record. 662 */ 663 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 664 @Description(shortDefinition="Unique id for the detected issue", formalDefinition="Business identifier associated with the detected issue record." ) 665 protected Identifier identifier; 666 667 /** 668 * Indicates the status of the detected issue. 669 */ 670 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 671 @Description(shortDefinition="registered | preliminary | final | amended +", formalDefinition="Indicates the status of the detected issue." ) 672 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-status") 673 protected Enumeration<DetectedIssueStatus> status; 674 675 /** 676 * Identifies the general type of issue identified. 677 */ 678 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 679 @Description(shortDefinition="Issue Category, e.g. drug-drug, duplicate therapy, etc.", formalDefinition="Identifies the general type of issue identified." ) 680 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-category") 681 protected CodeableConcept category; 682 683 /** 684 * Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 685 */ 686 @Child(name = "severity", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 687 @Description(shortDefinition="high | moderate | low", formalDefinition="Indicates the degree of importance associated with the identified issue based on the potential impact on the patient." ) 688 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-severity") 689 protected Enumeration<DetectedIssueSeverity> severity; 690 691 /** 692 * Indicates the patient whose record the detected issue is associated with. 693 */ 694 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=true) 695 @Description(shortDefinition="Associated patient", formalDefinition="Indicates the patient whose record the detected issue is associated with." ) 696 protected Reference patient; 697 698 /** 699 * The actual object that is the target of the reference (Indicates the patient whose record the detected issue is associated with.) 700 */ 701 protected Patient patientTarget; 702 703 /** 704 * The date or date-time when the detected issue was initially identified. 705 */ 706 @Child(name = "date", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 707 @Description(shortDefinition="When identified", formalDefinition="The date or date-time when the detected issue was initially identified." ) 708 protected DateTimeType date; 709 710 /** 711 * Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review. 712 */ 713 @Child(name = "author", type = {Practitioner.class, Device.class}, order=6, min=0, max=1, modifier=false, summary=true) 714 @Description(shortDefinition="The provider or device that identified the issue", formalDefinition="Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review." ) 715 protected Reference author; 716 717 /** 718 * The actual object that is the target of the reference (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 719 */ 720 protected Resource authorTarget; 721 722 /** 723 * Indicates the resource representing the current activity or proposed activity that is potentially problematic. 724 */ 725 @Child(name = "implicated", type = {Reference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 726 @Description(shortDefinition="Problem resource", formalDefinition="Indicates the resource representing the current activity or proposed activity that is potentially problematic." ) 727 protected List<Reference> implicated; 728 /** 729 * The actual objects that are the target of the reference (Indicates the resource representing the current activity or proposed activity that is potentially problematic.) 730 */ 731 protected List<Resource> implicatedTarget; 732 733 734 /** 735 * A textual explanation of the detected issue. 736 */ 737 @Child(name = "detail", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 738 @Description(shortDefinition="Description and context", formalDefinition="A textual explanation of the detected issue." ) 739 protected StringType detail; 740 741 /** 742 * The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 743 */ 744 @Child(name = "reference", type = {UriType.class}, order=9, min=0, max=1, modifier=false, summary=false) 745 @Description(shortDefinition="Authority for issue", formalDefinition="The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified." ) 746 protected UriType reference; 747 748 /** 749 * Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action. 750 */ 751 @Child(name = "mitigation", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 752 @Description(shortDefinition="Step taken to address", formalDefinition="Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action." ) 753 protected List<DetectedIssueMitigationComponent> mitigation; 754 755 private static final long serialVersionUID = -1002889332L; 756 757 /** 758 * Constructor 759 */ 760 public DetectedIssue() { 761 super(); 762 } 763 764 /** 765 * Constructor 766 */ 767 public DetectedIssue(Enumeration<DetectedIssueStatus> status) { 768 super(); 769 this.status = status; 770 } 771 772 /** 773 * @return {@link #identifier} (Business identifier associated with the detected issue record.) 774 */ 775 public Identifier getIdentifier() { 776 if (this.identifier == null) 777 if (Configuration.errorOnAutoCreate()) 778 throw new Error("Attempt to auto-create DetectedIssue.identifier"); 779 else if (Configuration.doAutoCreate()) 780 this.identifier = new Identifier(); // cc 781 return this.identifier; 782 } 783 784 public boolean hasIdentifier() { 785 return this.identifier != null && !this.identifier.isEmpty(); 786 } 787 788 /** 789 * @param value {@link #identifier} (Business identifier associated with the detected issue record.) 790 */ 791 public DetectedIssue setIdentifier(Identifier value) { 792 this.identifier = value; 793 return this; 794 } 795 796 /** 797 * @return {@link #status} (Indicates the status of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 798 */ 799 public Enumeration<DetectedIssueStatus> getStatusElement() { 800 if (this.status == null) 801 if (Configuration.errorOnAutoCreate()) 802 throw new Error("Attempt to auto-create DetectedIssue.status"); 803 else if (Configuration.doAutoCreate()) 804 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); // bb 805 return this.status; 806 } 807 808 public boolean hasStatusElement() { 809 return this.status != null && !this.status.isEmpty(); 810 } 811 812 public boolean hasStatus() { 813 return this.status != null && !this.status.isEmpty(); 814 } 815 816 /** 817 * @param value {@link #status} (Indicates the status of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 818 */ 819 public DetectedIssue setStatusElement(Enumeration<DetectedIssueStatus> value) { 820 this.status = value; 821 return this; 822 } 823 824 /** 825 * @return Indicates the status of the detected issue. 826 */ 827 public DetectedIssueStatus getStatus() { 828 return this.status == null ? null : this.status.getValue(); 829 } 830 831 /** 832 * @param value Indicates the status of the detected issue. 833 */ 834 public DetectedIssue setStatus(DetectedIssueStatus value) { 835 if (this.status == null) 836 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); 837 this.status.setValue(value); 838 return this; 839 } 840 841 /** 842 * @return {@link #category} (Identifies the general type of issue identified.) 843 */ 844 public CodeableConcept getCategory() { 845 if (this.category == null) 846 if (Configuration.errorOnAutoCreate()) 847 throw new Error("Attempt to auto-create DetectedIssue.category"); 848 else if (Configuration.doAutoCreate()) 849 this.category = new CodeableConcept(); // cc 850 return this.category; 851 } 852 853 public boolean hasCategory() { 854 return this.category != null && !this.category.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #category} (Identifies the general type of issue identified.) 859 */ 860 public DetectedIssue setCategory(CodeableConcept value) { 861 this.category = value; 862 return this; 863 } 864 865 /** 866 * @return {@link #severity} (Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 867 */ 868 public Enumeration<DetectedIssueSeverity> getSeverityElement() { 869 if (this.severity == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create DetectedIssue.severity"); 872 else if (Configuration.doAutoCreate()) 873 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); // bb 874 return this.severity; 875 } 876 877 public boolean hasSeverityElement() { 878 return this.severity != null && !this.severity.isEmpty(); 879 } 880 881 public boolean hasSeverity() { 882 return this.severity != null && !this.severity.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #severity} (Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 887 */ 888 public DetectedIssue setSeverityElement(Enumeration<DetectedIssueSeverity> value) { 889 this.severity = value; 890 return this; 891 } 892 893 /** 894 * @return Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 895 */ 896 public DetectedIssueSeverity getSeverity() { 897 return this.severity == null ? null : this.severity.getValue(); 898 } 899 900 /** 901 * @param value Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 902 */ 903 public DetectedIssue setSeverity(DetectedIssueSeverity value) { 904 if (value == null) 905 this.severity = null; 906 else { 907 if (this.severity == null) 908 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); 909 this.severity.setValue(value); 910 } 911 return this; 912 } 913 914 /** 915 * @return {@link #patient} (Indicates the patient whose record the detected issue is associated with.) 916 */ 917 public Reference getPatient() { 918 if (this.patient == null) 919 if (Configuration.errorOnAutoCreate()) 920 throw new Error("Attempt to auto-create DetectedIssue.patient"); 921 else if (Configuration.doAutoCreate()) 922 this.patient = new Reference(); // cc 923 return this.patient; 924 } 925 926 public boolean hasPatient() { 927 return this.patient != null && !this.patient.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #patient} (Indicates the patient whose record the detected issue is associated with.) 932 */ 933 public DetectedIssue setPatient(Reference value) { 934 this.patient = value; 935 return this; 936 } 937 938 /** 939 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the patient whose record the detected issue is associated with.) 940 */ 941 public Patient getPatientTarget() { 942 if (this.patientTarget == null) 943 if (Configuration.errorOnAutoCreate()) 944 throw new Error("Attempt to auto-create DetectedIssue.patient"); 945 else if (Configuration.doAutoCreate()) 946 this.patientTarget = new Patient(); // aa 947 return this.patientTarget; 948 } 949 950 /** 951 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the patient whose record the detected issue is associated with.) 952 */ 953 public DetectedIssue setPatientTarget(Patient value) { 954 this.patientTarget = value; 955 return this; 956 } 957 958 /** 959 * @return {@link #date} (The date or date-time when the detected issue was initially identified.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 960 */ 961 public DateTimeType getDateElement() { 962 if (this.date == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create DetectedIssue.date"); 965 else if (Configuration.doAutoCreate()) 966 this.date = new DateTimeType(); // bb 967 return this.date; 968 } 969 970 public boolean hasDateElement() { 971 return this.date != null && !this.date.isEmpty(); 972 } 973 974 public boolean hasDate() { 975 return this.date != null && !this.date.isEmpty(); 976 } 977 978 /** 979 * @param value {@link #date} (The date or date-time when the detected issue was initially identified.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 980 */ 981 public DetectedIssue setDateElement(DateTimeType value) { 982 this.date = value; 983 return this; 984 } 985 986 /** 987 * @return The date or date-time when the detected issue was initially identified. 988 */ 989 public Date getDate() { 990 return this.date == null ? null : this.date.getValue(); 991 } 992 993 /** 994 * @param value The date or date-time when the detected issue was initially identified. 995 */ 996 public DetectedIssue setDate(Date value) { 997 if (value == null) 998 this.date = null; 999 else { 1000 if (this.date == null) 1001 this.date = new DateTimeType(); 1002 this.date.setValue(value); 1003 } 1004 return this; 1005 } 1006 1007 /** 1008 * @return {@link #author} (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1009 */ 1010 public Reference getAuthor() { 1011 if (this.author == null) 1012 if (Configuration.errorOnAutoCreate()) 1013 throw new Error("Attempt to auto-create DetectedIssue.author"); 1014 else if (Configuration.doAutoCreate()) 1015 this.author = new Reference(); // cc 1016 return this.author; 1017 } 1018 1019 public boolean hasAuthor() { 1020 return this.author != null && !this.author.isEmpty(); 1021 } 1022 1023 /** 1024 * @param value {@link #author} (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1025 */ 1026 public DetectedIssue setAuthor(Reference value) { 1027 this.author = value; 1028 return this; 1029 } 1030 1031 /** 1032 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1033 */ 1034 public Resource getAuthorTarget() { 1035 return this.authorTarget; 1036 } 1037 1038 /** 1039 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1040 */ 1041 public DetectedIssue setAuthorTarget(Resource value) { 1042 this.authorTarget = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #implicated} (Indicates the resource representing the current activity or proposed activity that is potentially problematic.) 1048 */ 1049 public List<Reference> getImplicated() { 1050 if (this.implicated == null) 1051 this.implicated = new ArrayList<Reference>(); 1052 return this.implicated; 1053 } 1054 1055 /** 1056 * @return Returns a reference to <code>this</code> for easy method chaining 1057 */ 1058 public DetectedIssue setImplicated(List<Reference> theImplicated) { 1059 this.implicated = theImplicated; 1060 return this; 1061 } 1062 1063 public boolean hasImplicated() { 1064 if (this.implicated == null) 1065 return false; 1066 for (Reference item : this.implicated) 1067 if (!item.isEmpty()) 1068 return true; 1069 return false; 1070 } 1071 1072 public Reference addImplicated() { //3 1073 Reference t = new Reference(); 1074 if (this.implicated == null) 1075 this.implicated = new ArrayList<Reference>(); 1076 this.implicated.add(t); 1077 return t; 1078 } 1079 1080 public DetectedIssue addImplicated(Reference t) { //3 1081 if (t == null) 1082 return this; 1083 if (this.implicated == null) 1084 this.implicated = new ArrayList<Reference>(); 1085 this.implicated.add(t); 1086 return this; 1087 } 1088 1089 /** 1090 * @return The first repetition of repeating field {@link #implicated}, creating it if it does not already exist 1091 */ 1092 public Reference getImplicatedFirstRep() { 1093 if (getImplicated().isEmpty()) { 1094 addImplicated(); 1095 } 1096 return getImplicated().get(0); 1097 } 1098 1099 /** 1100 * @deprecated Use Reference#setResource(IBaseResource) instead 1101 */ 1102 @Deprecated 1103 public List<Resource> getImplicatedTarget() { 1104 if (this.implicatedTarget == null) 1105 this.implicatedTarget = new ArrayList<Resource>(); 1106 return this.implicatedTarget; 1107 } 1108 1109 /** 1110 * @return {@link #detail} (A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1111 */ 1112 public StringType getDetailElement() { 1113 if (this.detail == null) 1114 if (Configuration.errorOnAutoCreate()) 1115 throw new Error("Attempt to auto-create DetectedIssue.detail"); 1116 else if (Configuration.doAutoCreate()) 1117 this.detail = new StringType(); // bb 1118 return this.detail; 1119 } 1120 1121 public boolean hasDetailElement() { 1122 return this.detail != null && !this.detail.isEmpty(); 1123 } 1124 1125 public boolean hasDetail() { 1126 return this.detail != null && !this.detail.isEmpty(); 1127 } 1128 1129 /** 1130 * @param value {@link #detail} (A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1131 */ 1132 public DetectedIssue setDetailElement(StringType value) { 1133 this.detail = value; 1134 return this; 1135 } 1136 1137 /** 1138 * @return A textual explanation of the detected issue. 1139 */ 1140 public String getDetail() { 1141 return this.detail == null ? null : this.detail.getValue(); 1142 } 1143 1144 /** 1145 * @param value A textual explanation of the detected issue. 1146 */ 1147 public DetectedIssue setDetail(String value) { 1148 if (Utilities.noString(value)) 1149 this.detail = null; 1150 else { 1151 if (this.detail == null) 1152 this.detail = new StringType(); 1153 this.detail.setValue(value); 1154 } 1155 return this; 1156 } 1157 1158 /** 1159 * @return {@link #reference} (The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1160 */ 1161 public UriType getReferenceElement() { 1162 if (this.reference == null) 1163 if (Configuration.errorOnAutoCreate()) 1164 throw new Error("Attempt to auto-create DetectedIssue.reference"); 1165 else if (Configuration.doAutoCreate()) 1166 this.reference = new UriType(); // bb 1167 return this.reference; 1168 } 1169 1170 public boolean hasReferenceElement() { 1171 return this.reference != null && !this.reference.isEmpty(); 1172 } 1173 1174 public boolean hasReference() { 1175 return this.reference != null && !this.reference.isEmpty(); 1176 } 1177 1178 /** 1179 * @param value {@link #reference} (The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1180 */ 1181 public DetectedIssue setReferenceElement(UriType value) { 1182 this.reference = value; 1183 return this; 1184 } 1185 1186 /** 1187 * @return The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 1188 */ 1189 public String getReference() { 1190 return this.reference == null ? null : this.reference.getValue(); 1191 } 1192 1193 /** 1194 * @param value The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 1195 */ 1196 public DetectedIssue setReference(String value) { 1197 if (Utilities.noString(value)) 1198 this.reference = null; 1199 else { 1200 if (this.reference == null) 1201 this.reference = new UriType(); 1202 this.reference.setValue(value); 1203 } 1204 return this; 1205 } 1206 1207 /** 1208 * @return {@link #mitigation} (Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.) 1209 */ 1210 public List<DetectedIssueMitigationComponent> getMitigation() { 1211 if (this.mitigation == null) 1212 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1213 return this.mitigation; 1214 } 1215 1216 /** 1217 * @return Returns a reference to <code>this</code> for easy method chaining 1218 */ 1219 public DetectedIssue setMitigation(List<DetectedIssueMitigationComponent> theMitigation) { 1220 this.mitigation = theMitigation; 1221 return this; 1222 } 1223 1224 public boolean hasMitigation() { 1225 if (this.mitigation == null) 1226 return false; 1227 for (DetectedIssueMitigationComponent item : this.mitigation) 1228 if (!item.isEmpty()) 1229 return true; 1230 return false; 1231 } 1232 1233 public DetectedIssueMitigationComponent addMitigation() { //3 1234 DetectedIssueMitigationComponent t = new DetectedIssueMitigationComponent(); 1235 if (this.mitigation == null) 1236 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1237 this.mitigation.add(t); 1238 return t; 1239 } 1240 1241 public DetectedIssue addMitigation(DetectedIssueMitigationComponent t) { //3 1242 if (t == null) 1243 return this; 1244 if (this.mitigation == null) 1245 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1246 this.mitigation.add(t); 1247 return this; 1248 } 1249 1250 /** 1251 * @return The first repetition of repeating field {@link #mitigation}, creating it if it does not already exist 1252 */ 1253 public DetectedIssueMitigationComponent getMitigationFirstRep() { 1254 if (getMitigation().isEmpty()) { 1255 addMitigation(); 1256 } 1257 return getMitigation().get(0); 1258 } 1259 1260 protected void listChildren(List<Property> children) { 1261 super.listChildren(children); 1262 children.add(new Property("identifier", "Identifier", "Business identifier associated with the detected issue record.", 0, 1, identifier)); 1263 children.add(new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status)); 1264 children.add(new Property("category", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1, category)); 1265 children.add(new Property("severity", "code", "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 0, 1, severity)); 1266 children.add(new Property("patient", "Reference(Patient)", "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient)); 1267 children.add(new Property("date", "dateTime", "The date or date-time when the detected issue was initially identified.", 0, 1, date)); 1268 children.add(new Property("author", "Reference(Practitioner|Device)", "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 0, 1, author)); 1269 children.add(new Property("implicated", "Reference(Any)", "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 0, java.lang.Integer.MAX_VALUE, implicated)); 1270 children.add(new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, detail)); 1271 children.add(new Property("reference", "uri", "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 0, 1, reference)); 1272 children.add(new Property("mitigation", "", "Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 0, java.lang.Integer.MAX_VALUE, mitigation)); 1273 } 1274 1275 @Override 1276 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1277 switch (_hash) { 1278 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier associated with the detected issue record.", 0, 1, identifier); 1279 case -892481550: /*status*/ return new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status); 1280 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1, category); 1281 case 1478300413: /*severity*/ return new Property("severity", "code", "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 0, 1, severity); 1282 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient); 1283 case 3076014: /*date*/ return new Property("date", "dateTime", "The date or date-time when the detected issue was initially identified.", 0, 1, date); 1284 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Device)", "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 0, 1, author); 1285 case -810216884: /*implicated*/ return new Property("implicated", "Reference(Any)", "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 0, java.lang.Integer.MAX_VALUE, implicated); 1286 case -1335224239: /*detail*/ return new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, detail); 1287 case -925155509: /*reference*/ return new Property("reference", "uri", "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 0, 1, reference); 1288 case 1293793087: /*mitigation*/ return new Property("mitigation", "", "Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 0, java.lang.Integer.MAX_VALUE, mitigation); 1289 default: return super.getNamedProperty(_hash, _name, _checkValid); 1290 } 1291 1292 } 1293 1294 @Override 1295 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1296 switch (hash) { 1297 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1298 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DetectedIssueStatus> 1299 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1300 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<DetectedIssueSeverity> 1301 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1302 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1303 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1304 case -810216884: /*implicated*/ return this.implicated == null ? new Base[0] : this.implicated.toArray(new Base[this.implicated.size()]); // Reference 1305 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // StringType 1306 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // UriType 1307 case 1293793087: /*mitigation*/ return this.mitigation == null ? new Base[0] : this.mitigation.toArray(new Base[this.mitigation.size()]); // DetectedIssueMitigationComponent 1308 default: return super.getProperty(hash, name, checkValid); 1309 } 1310 1311 } 1312 1313 @Override 1314 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1315 switch (hash) { 1316 case -1618432855: // identifier 1317 this.identifier = castToIdentifier(value); // Identifier 1318 return value; 1319 case -892481550: // status 1320 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 1321 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 1322 return value; 1323 case 50511102: // category 1324 this.category = castToCodeableConcept(value); // CodeableConcept 1325 return value; 1326 case 1478300413: // severity 1327 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 1328 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 1329 return value; 1330 case -791418107: // patient 1331 this.patient = castToReference(value); // Reference 1332 return value; 1333 case 3076014: // date 1334 this.date = castToDateTime(value); // DateTimeType 1335 return value; 1336 case -1406328437: // author 1337 this.author = castToReference(value); // Reference 1338 return value; 1339 case -810216884: // implicated 1340 this.getImplicated().add(castToReference(value)); // Reference 1341 return value; 1342 case -1335224239: // detail 1343 this.detail = castToString(value); // StringType 1344 return value; 1345 case -925155509: // reference 1346 this.reference = castToUri(value); // UriType 1347 return value; 1348 case 1293793087: // mitigation 1349 this.getMitigation().add((DetectedIssueMitigationComponent) value); // DetectedIssueMitigationComponent 1350 return value; 1351 default: return super.setProperty(hash, name, value); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base setProperty(String name, Base value) throws FHIRException { 1358 if (name.equals("identifier")) { 1359 this.identifier = castToIdentifier(value); // Identifier 1360 } else if (name.equals("status")) { 1361 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 1362 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 1363 } else if (name.equals("category")) { 1364 this.category = castToCodeableConcept(value); // CodeableConcept 1365 } else if (name.equals("severity")) { 1366 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 1367 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 1368 } else if (name.equals("patient")) { 1369 this.patient = castToReference(value); // Reference 1370 } else if (name.equals("date")) { 1371 this.date = castToDateTime(value); // DateTimeType 1372 } else if (name.equals("author")) { 1373 this.author = castToReference(value); // Reference 1374 } else if (name.equals("implicated")) { 1375 this.getImplicated().add(castToReference(value)); 1376 } else if (name.equals("detail")) { 1377 this.detail = castToString(value); // StringType 1378 } else if (name.equals("reference")) { 1379 this.reference = castToUri(value); // UriType 1380 } else if (name.equals("mitigation")) { 1381 this.getMitigation().add((DetectedIssueMitigationComponent) value); 1382 } else 1383 return super.setProperty(name, value); 1384 return value; 1385 } 1386 1387 @Override 1388 public Base makeProperty(int hash, String name) throws FHIRException { 1389 switch (hash) { 1390 case -1618432855: return getIdentifier(); 1391 case -892481550: return getStatusElement(); 1392 case 50511102: return getCategory(); 1393 case 1478300413: return getSeverityElement(); 1394 case -791418107: return getPatient(); 1395 case 3076014: return getDateElement(); 1396 case -1406328437: return getAuthor(); 1397 case -810216884: return addImplicated(); 1398 case -1335224239: return getDetailElement(); 1399 case -925155509: return getReferenceElement(); 1400 case 1293793087: return addMitigation(); 1401 default: return super.makeProperty(hash, name); 1402 } 1403 1404 } 1405 1406 @Override 1407 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1408 switch (hash) { 1409 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1410 case -892481550: /*status*/ return new String[] {"code"}; 1411 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1412 case 1478300413: /*severity*/ return new String[] {"code"}; 1413 case -791418107: /*patient*/ return new String[] {"Reference"}; 1414 case 3076014: /*date*/ return new String[] {"dateTime"}; 1415 case -1406328437: /*author*/ return new String[] {"Reference"}; 1416 case -810216884: /*implicated*/ return new String[] {"Reference"}; 1417 case -1335224239: /*detail*/ return new String[] {"string"}; 1418 case -925155509: /*reference*/ return new String[] {"uri"}; 1419 case 1293793087: /*mitigation*/ return new String[] {}; 1420 default: return super.getTypesForProperty(hash, name); 1421 } 1422 1423 } 1424 1425 @Override 1426 public Base addChild(String name) throws FHIRException { 1427 if (name.equals("identifier")) { 1428 this.identifier = new Identifier(); 1429 return this.identifier; 1430 } 1431 else if (name.equals("status")) { 1432 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.status"); 1433 } 1434 else if (name.equals("category")) { 1435 this.category = new CodeableConcept(); 1436 return this.category; 1437 } 1438 else if (name.equals("severity")) { 1439 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.severity"); 1440 } 1441 else if (name.equals("patient")) { 1442 this.patient = new Reference(); 1443 return this.patient; 1444 } 1445 else if (name.equals("date")) { 1446 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 1447 } 1448 else if (name.equals("author")) { 1449 this.author = new Reference(); 1450 return this.author; 1451 } 1452 else if (name.equals("implicated")) { 1453 return addImplicated(); 1454 } 1455 else if (name.equals("detail")) { 1456 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.detail"); 1457 } 1458 else if (name.equals("reference")) { 1459 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.reference"); 1460 } 1461 else if (name.equals("mitigation")) { 1462 return addMitigation(); 1463 } 1464 else 1465 return super.addChild(name); 1466 } 1467 1468 public String fhirType() { 1469 return "DetectedIssue"; 1470 1471 } 1472 1473 public DetectedIssue copy() { 1474 DetectedIssue dst = new DetectedIssue(); 1475 copyValues(dst); 1476 dst.identifier = identifier == null ? null : identifier.copy(); 1477 dst.status = status == null ? null : status.copy(); 1478 dst.category = category == null ? null : category.copy(); 1479 dst.severity = severity == null ? null : severity.copy(); 1480 dst.patient = patient == null ? null : patient.copy(); 1481 dst.date = date == null ? null : date.copy(); 1482 dst.author = author == null ? null : author.copy(); 1483 if (implicated != null) { 1484 dst.implicated = new ArrayList<Reference>(); 1485 for (Reference i : implicated) 1486 dst.implicated.add(i.copy()); 1487 }; 1488 dst.detail = detail == null ? null : detail.copy(); 1489 dst.reference = reference == null ? null : reference.copy(); 1490 if (mitigation != null) { 1491 dst.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1492 for (DetectedIssueMitigationComponent i : mitigation) 1493 dst.mitigation.add(i.copy()); 1494 }; 1495 return dst; 1496 } 1497 1498 protected DetectedIssue typedCopy() { 1499 return copy(); 1500 } 1501 1502 @Override 1503 public boolean equalsDeep(Base other_) { 1504 if (!super.equalsDeep(other_)) 1505 return false; 1506 if (!(other_ instanceof DetectedIssue)) 1507 return false; 1508 DetectedIssue o = (DetectedIssue) other_; 1509 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1510 && compareDeep(severity, o.severity, true) && compareDeep(patient, o.patient, true) && compareDeep(date, o.date, true) 1511 && compareDeep(author, o.author, true) && compareDeep(implicated, o.implicated, true) && compareDeep(detail, o.detail, true) 1512 && compareDeep(reference, o.reference, true) && compareDeep(mitigation, o.mitigation, true); 1513 } 1514 1515 @Override 1516 public boolean equalsShallow(Base other_) { 1517 if (!super.equalsShallow(other_)) 1518 return false; 1519 if (!(other_ instanceof DetectedIssue)) 1520 return false; 1521 DetectedIssue o = (DetectedIssue) other_; 1522 return compareValues(status, o.status, true) && compareValues(severity, o.severity, true) && compareValues(date, o.date, true) 1523 && compareValues(detail, o.detail, true) && compareValues(reference, o.reference, true); 1524 } 1525 1526 public boolean isEmpty() { 1527 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1528 , severity, patient, date, author, implicated, detail, reference, mitigation 1529 ); 1530 } 1531 1532 @Override 1533 public ResourceType getResourceType() { 1534 return ResourceType.DetectedIssue; 1535 } 1536 1537 /** 1538 * Search parameter: <b>date</b> 1539 * <p> 1540 * Description: <b>When identified</b><br> 1541 * Type: <b>date</b><br> 1542 * Path: <b>DetectedIssue.date</b><br> 1543 * </p> 1544 */ 1545 @SearchParamDefinition(name="date", path="DetectedIssue.date", description="When identified", type="date" ) 1546 public static final String SP_DATE = "date"; 1547 /** 1548 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1549 * <p> 1550 * Description: <b>When identified</b><br> 1551 * Type: <b>date</b><br> 1552 * Path: <b>DetectedIssue.date</b><br> 1553 * </p> 1554 */ 1555 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1556 1557 /** 1558 * Search parameter: <b>identifier</b> 1559 * <p> 1560 * Description: <b>Unique id for the detected issue</b><br> 1561 * Type: <b>token</b><br> 1562 * Path: <b>DetectedIssue.identifier</b><br> 1563 * </p> 1564 */ 1565 @SearchParamDefinition(name="identifier", path="DetectedIssue.identifier", description="Unique id for the detected issue", type="token" ) 1566 public static final String SP_IDENTIFIER = "identifier"; 1567 /** 1568 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1569 * <p> 1570 * Description: <b>Unique id for the detected issue</b><br> 1571 * Type: <b>token</b><br> 1572 * Path: <b>DetectedIssue.identifier</b><br> 1573 * </p> 1574 */ 1575 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1576 1577 /** 1578 * Search parameter: <b>patient</b> 1579 * <p> 1580 * Description: <b>Associated patient</b><br> 1581 * Type: <b>reference</b><br> 1582 * Path: <b>DetectedIssue.patient</b><br> 1583 * </p> 1584 */ 1585 @SearchParamDefinition(name="patient", path="DetectedIssue.patient", description="Associated patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1586 public static final String SP_PATIENT = "patient"; 1587 /** 1588 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1589 * <p> 1590 * Description: <b>Associated patient</b><br> 1591 * Type: <b>reference</b><br> 1592 * Path: <b>DetectedIssue.patient</b><br> 1593 * </p> 1594 */ 1595 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1596 1597/** 1598 * Constant for fluent queries to be used to add include statements. Specifies 1599 * the path value of "<b>DetectedIssue:patient</b>". 1600 */ 1601 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DetectedIssue:patient").toLocked(); 1602 1603 /** 1604 * Search parameter: <b>author</b> 1605 * <p> 1606 * Description: <b>The provider or device that identified the issue</b><br> 1607 * Type: <b>reference</b><br> 1608 * Path: <b>DetectedIssue.author</b><br> 1609 * </p> 1610 */ 1611 @SearchParamDefinition(name="author", path="DetectedIssue.author", description="The provider or device that identified the issue", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Practitioner.class } ) 1612 public static final String SP_AUTHOR = "author"; 1613 /** 1614 * <b>Fluent Client</b> search parameter constant for <b>author</b> 1615 * <p> 1616 * Description: <b>The provider or device that identified the issue</b><br> 1617 * Type: <b>reference</b><br> 1618 * Path: <b>DetectedIssue.author</b><br> 1619 * </p> 1620 */ 1621 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 1622 1623/** 1624 * Constant for fluent queries to be used to add include statements. Specifies 1625 * the path value of "<b>DetectedIssue:author</b>". 1626 */ 1627 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("DetectedIssue:author").toLocked(); 1628 1629 /** 1630 * Search parameter: <b>implicated</b> 1631 * <p> 1632 * Description: <b>Problem resource</b><br> 1633 * Type: <b>reference</b><br> 1634 * Path: <b>DetectedIssue.implicated</b><br> 1635 * </p> 1636 */ 1637 @SearchParamDefinition(name="implicated", path="DetectedIssue.implicated", description="Problem resource", type="reference" ) 1638 public static final String SP_IMPLICATED = "implicated"; 1639 /** 1640 * <b>Fluent Client</b> search parameter constant for <b>implicated</b> 1641 * <p> 1642 * Description: <b>Problem resource</b><br> 1643 * Type: <b>reference</b><br> 1644 * Path: <b>DetectedIssue.implicated</b><br> 1645 * </p> 1646 */ 1647 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMPLICATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_IMPLICATED); 1648 1649/** 1650 * Constant for fluent queries to be used to add include statements. Specifies 1651 * the path value of "<b>DetectedIssue:implicated</b>". 1652 */ 1653 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMPLICATED = new ca.uhn.fhir.model.api.Include("DetectedIssue:implicated").toLocked(); 1654 1655 /** 1656 * Search parameter: <b>category</b> 1657 * <p> 1658 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, etc.</b><br> 1659 * Type: <b>token</b><br> 1660 * Path: <b>DetectedIssue.category</b><br> 1661 * </p> 1662 */ 1663 @SearchParamDefinition(name="category", path="DetectedIssue.category", description="Issue Category, e.g. drug-drug, duplicate therapy, etc.", type="token" ) 1664 public static final String SP_CATEGORY = "category"; 1665 /** 1666 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1667 * <p> 1668 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, etc.</b><br> 1669 * Type: <b>token</b><br> 1670 * Path: <b>DetectedIssue.category</b><br> 1671 * </p> 1672 */ 1673 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1674 1675 1676}