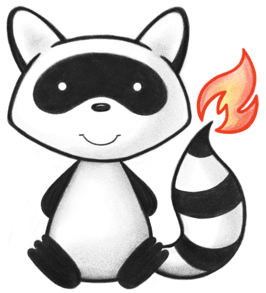
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 051 */ 052@ResourceDef(name="Device", profile="http://hl7.org/fhir/Profile/Device") 053public class Device extends DomainResource { 054 055 public enum UDIEntryType { 056 /** 057 * A Barcode scanner captured the data from the device label 058 */ 059 BARCODE, 060 /** 061 * An RFID chip reader captured the data from the device label 062 */ 063 RFID, 064 /** 065 * The data was read from the label by a person and manually entered. (e.g. via a keyboard) 066 */ 067 MANUAL, 068 /** 069 * The data originated from a patient's implant card and read by an operator. 070 */ 071 CARD, 072 /** 073 * The data originated from a patient source and not directly scanned or read from a label or card. 074 */ 075 SELFREPORTED, 076 /** 077 * The method of data capture has not been determined 078 */ 079 UNKNOWN, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static UDIEntryType fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("barcode".equals(codeString)) 088 return BARCODE; 089 if ("rfid".equals(codeString)) 090 return RFID; 091 if ("manual".equals(codeString)) 092 return MANUAL; 093 if ("card".equals(codeString)) 094 return CARD; 095 if ("self-reported".equals(codeString)) 096 return SELFREPORTED; 097 if ("unknown".equals(codeString)) 098 return UNKNOWN; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown UDIEntryType code '"+codeString+"'"); 103 } 104 public String toCode() { 105 switch (this) { 106 case BARCODE: return "barcode"; 107 case RFID: return "rfid"; 108 case MANUAL: return "manual"; 109 case CARD: return "card"; 110 case SELFREPORTED: return "self-reported"; 111 case UNKNOWN: return "unknown"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getSystem() { 117 switch (this) { 118 case BARCODE: return "http://hl7.org/fhir/udi-entry-type"; 119 case RFID: return "http://hl7.org/fhir/udi-entry-type"; 120 case MANUAL: return "http://hl7.org/fhir/udi-entry-type"; 121 case CARD: return "http://hl7.org/fhir/udi-entry-type"; 122 case SELFREPORTED: return "http://hl7.org/fhir/udi-entry-type"; 123 case UNKNOWN: return "http://hl7.org/fhir/udi-entry-type"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 public String getDefinition() { 129 switch (this) { 130 case BARCODE: return "A Barcode scanner captured the data from the device label"; 131 case RFID: return "An RFID chip reader captured the data from the device label"; 132 case MANUAL: return "The data was read from the label by a person and manually entered. (e.g. via a keyboard)"; 133 case CARD: return "The data originated from a patient's implant card and read by an operator."; 134 case SELFREPORTED: return "The data originated from a patient source and not directly scanned or read from a label or card."; 135 case UNKNOWN: return "The method of data capture has not been determined"; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 public String getDisplay() { 141 switch (this) { 142 case BARCODE: return "BarCode"; 143 case RFID: return "RFID"; 144 case MANUAL: return "Manual"; 145 case CARD: return "Card"; 146 case SELFREPORTED: return "Self Reported"; 147 case UNKNOWN: return "Unknown"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 } 153 154 public static class UDIEntryTypeEnumFactory implements EnumFactory<UDIEntryType> { 155 public UDIEntryType fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("barcode".equals(codeString)) 160 return UDIEntryType.BARCODE; 161 if ("rfid".equals(codeString)) 162 return UDIEntryType.RFID; 163 if ("manual".equals(codeString)) 164 return UDIEntryType.MANUAL; 165 if ("card".equals(codeString)) 166 return UDIEntryType.CARD; 167 if ("self-reported".equals(codeString)) 168 return UDIEntryType.SELFREPORTED; 169 if ("unknown".equals(codeString)) 170 return UDIEntryType.UNKNOWN; 171 throw new IllegalArgumentException("Unknown UDIEntryType code '"+codeString+"'"); 172 } 173 public Enumeration<UDIEntryType> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<UDIEntryType>(this); 178 String codeString = code.asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("barcode".equals(codeString)) 182 return new Enumeration<UDIEntryType>(this, UDIEntryType.BARCODE); 183 if ("rfid".equals(codeString)) 184 return new Enumeration<UDIEntryType>(this, UDIEntryType.RFID); 185 if ("manual".equals(codeString)) 186 return new Enumeration<UDIEntryType>(this, UDIEntryType.MANUAL); 187 if ("card".equals(codeString)) 188 return new Enumeration<UDIEntryType>(this, UDIEntryType.CARD); 189 if ("self-reported".equals(codeString)) 190 return new Enumeration<UDIEntryType>(this, UDIEntryType.SELFREPORTED); 191 if ("unknown".equals(codeString)) 192 return new Enumeration<UDIEntryType>(this, UDIEntryType.UNKNOWN); 193 throw new FHIRException("Unknown UDIEntryType code '"+codeString+"'"); 194 } 195 public String toCode(UDIEntryType code) { 196 if (code == UDIEntryType.NULL) 197 return null; 198 if (code == UDIEntryType.BARCODE) 199 return "barcode"; 200 if (code == UDIEntryType.RFID) 201 return "rfid"; 202 if (code == UDIEntryType.MANUAL) 203 return "manual"; 204 if (code == UDIEntryType.CARD) 205 return "card"; 206 if (code == UDIEntryType.SELFREPORTED) 207 return "self-reported"; 208 if (code == UDIEntryType.UNKNOWN) 209 return "unknown"; 210 return "?"; 211 } 212 public String toSystem(UDIEntryType code) { 213 return code.getSystem(); 214 } 215 } 216 217 public enum FHIRDeviceStatus { 218 /** 219 * The Device is available for use. Note: This means for *implanted devices* the device is implanted in the patient. 220 */ 221 ACTIVE, 222 /** 223 * The Device is no longer available for use (e.g. lost, expired, damaged). Note: This means for *implanted devices* the device has been removed from the patient. 224 */ 225 INACTIVE, 226 /** 227 * The Device was entered in error and voided. 228 */ 229 ENTEREDINERROR, 230 /** 231 * The status of the device has not been determined. 232 */ 233 UNKNOWN, 234 /** 235 * added to help the parsers with the generic types 236 */ 237 NULL; 238 public static FHIRDeviceStatus fromCode(String codeString) throws FHIRException { 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("active".equals(codeString)) 242 return ACTIVE; 243 if ("inactive".equals(codeString)) 244 return INACTIVE; 245 if ("entered-in-error".equals(codeString)) 246 return ENTEREDINERROR; 247 if ("unknown".equals(codeString)) 248 return UNKNOWN; 249 if (Configuration.isAcceptInvalidEnums()) 250 return null; 251 else 252 throw new FHIRException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 253 } 254 public String toCode() { 255 switch (this) { 256 case ACTIVE: return "active"; 257 case INACTIVE: return "inactive"; 258 case ENTEREDINERROR: return "entered-in-error"; 259 case UNKNOWN: return "unknown"; 260 case NULL: return null; 261 default: return "?"; 262 } 263 } 264 public String getSystem() { 265 switch (this) { 266 case ACTIVE: return "http://hl7.org/fhir/device-status"; 267 case INACTIVE: return "http://hl7.org/fhir/device-status"; 268 case ENTEREDINERROR: return "http://hl7.org/fhir/device-status"; 269 case UNKNOWN: return "http://hl7.org/fhir/device-status"; 270 case NULL: return null; 271 default: return "?"; 272 } 273 } 274 public String getDefinition() { 275 switch (this) { 276 case ACTIVE: return "The Device is available for use. Note: This means for *implanted devices* the device is implanted in the patient."; 277 case INACTIVE: return "The Device is no longer available for use (e.g. lost, expired, damaged). Note: This means for *implanted devices* the device has been removed from the patient."; 278 case ENTEREDINERROR: return "The Device was entered in error and voided."; 279 case UNKNOWN: return "The status of the device has not been determined."; 280 case NULL: return null; 281 default: return "?"; 282 } 283 } 284 public String getDisplay() { 285 switch (this) { 286 case ACTIVE: return "Active"; 287 case INACTIVE: return "Inactive"; 288 case ENTEREDINERROR: return "Entered in Error"; 289 case UNKNOWN: return "Unknown"; 290 case NULL: return null; 291 default: return "?"; 292 } 293 } 294 } 295 296 public static class FHIRDeviceStatusEnumFactory implements EnumFactory<FHIRDeviceStatus> { 297 public FHIRDeviceStatus fromCode(String codeString) throws IllegalArgumentException { 298 if (codeString == null || "".equals(codeString)) 299 if (codeString == null || "".equals(codeString)) 300 return null; 301 if ("active".equals(codeString)) 302 return FHIRDeviceStatus.ACTIVE; 303 if ("inactive".equals(codeString)) 304 return FHIRDeviceStatus.INACTIVE; 305 if ("entered-in-error".equals(codeString)) 306 return FHIRDeviceStatus.ENTEREDINERROR; 307 if ("unknown".equals(codeString)) 308 return FHIRDeviceStatus.UNKNOWN; 309 throw new IllegalArgumentException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 310 } 311 public Enumeration<FHIRDeviceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 312 if (code == null) 313 return null; 314 if (code.isEmpty()) 315 return new Enumeration<FHIRDeviceStatus>(this); 316 String codeString = code.asStringValue(); 317 if (codeString == null || "".equals(codeString)) 318 return null; 319 if ("active".equals(codeString)) 320 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ACTIVE); 321 if ("inactive".equals(codeString)) 322 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.INACTIVE); 323 if ("entered-in-error".equals(codeString)) 324 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ENTEREDINERROR); 325 if ("unknown".equals(codeString)) 326 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.UNKNOWN); 327 throw new FHIRException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 328 } 329 public String toCode(FHIRDeviceStatus code) { 330 if (code == FHIRDeviceStatus.NULL) 331 return null; 332 if (code == FHIRDeviceStatus.ACTIVE) 333 return "active"; 334 if (code == FHIRDeviceStatus.INACTIVE) 335 return "inactive"; 336 if (code == FHIRDeviceStatus.ENTEREDINERROR) 337 return "entered-in-error"; 338 if (code == FHIRDeviceStatus.UNKNOWN) 339 return "unknown"; 340 return "?"; 341 } 342 public String toSystem(FHIRDeviceStatus code) { 343 return code.getSystem(); 344 } 345 } 346 347 @Block() 348 public static class DeviceUdiComponent extends BackboneElement implements IBaseBackboneElement { 349 /** 350 * The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 351 */ 352 @Child(name = "deviceIdentifier", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 353 @Description(shortDefinition="Mandatory fixed portion of UDI", formalDefinition="The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device." ) 354 protected StringType deviceIdentifier; 355 356 /** 357 * Name of device as used in labeling or catalog. 358 */ 359 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 360 @Description(shortDefinition="Device Name as appears on UDI label", formalDefinition="Name of device as used in labeling or catalog." ) 361 protected StringType name; 362 363 /** 364 * The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi. 365 */ 366 @Child(name = "jurisdiction", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=false) 367 @Description(shortDefinition="Regional UDI authority", formalDefinition="The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi." ) 368 protected UriType jurisdiction; 369 370 /** 371 * The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 372 */ 373 @Child(name = "carrierHRF", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 374 @Description(shortDefinition="UDI Human Readable Barcode String", formalDefinition="The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device." ) 375 protected StringType carrierHRF; 376 377 /** 378 * The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 379 */ 380 @Child(name = "carrierAIDC", type = {Base64BinaryType.class}, order=5, min=0, max=1, modifier=false, summary=true) 381 @Description(shortDefinition="UDI Machine Readable Barcode String", formalDefinition="The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded." ) 382 protected Base64BinaryType carrierAIDC; 383 384 /** 385 * Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include : 3861) GS1: 387http://hl7.org/fhir/NamingSystem/gs1-di, 3882) HIBCC: 389http://hl7.org/fhir/NamingSystem/hibcc-dI, 3903) ICCBBA for blood containers: 391http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 3924) ICCBA for other devices: 393http://hl7.org/fhir/NamingSystem/iccbba-other-di. 394 */ 395 @Child(name = "issuer", type = {UriType.class}, order=6, min=0, max=1, modifier=false, summary=false) 396 @Description(shortDefinition="UDI Issuing Organization", formalDefinition="Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include :\n1) GS1: \nhttp://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC:\nhttp://hl7.org/fhir/NamingSystem/hibcc-dI, \n3) ICCBBA for blood containers:\nhttp://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices:\nhttp://hl7.org/fhir/NamingSystem/iccbba-other-di." ) 397 protected UriType issuer; 398 399 /** 400 * A coded entry to indicate how the data was entered. 401 */ 402 @Child(name = "entryType", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 403 @Description(shortDefinition="barcode | rfid | manual +", formalDefinition="A coded entry to indicate how the data was entered." ) 404 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/udi-entry-type") 405 protected Enumeration<UDIEntryType> entryType; 406 407 private static final long serialVersionUID = -1105798343L; 408 409 /** 410 * Constructor 411 */ 412 public DeviceUdiComponent() { 413 super(); 414 } 415 416 /** 417 * @return {@link #deviceIdentifier} (The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 418 */ 419 public StringType getDeviceIdentifierElement() { 420 if (this.deviceIdentifier == null) 421 if (Configuration.errorOnAutoCreate()) 422 throw new Error("Attempt to auto-create DeviceUdiComponent.deviceIdentifier"); 423 else if (Configuration.doAutoCreate()) 424 this.deviceIdentifier = new StringType(); // bb 425 return this.deviceIdentifier; 426 } 427 428 public boolean hasDeviceIdentifierElement() { 429 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 430 } 431 432 public boolean hasDeviceIdentifier() { 433 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 434 } 435 436 /** 437 * @param value {@link #deviceIdentifier} (The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 438 */ 439 public DeviceUdiComponent setDeviceIdentifierElement(StringType value) { 440 this.deviceIdentifier = value; 441 return this; 442 } 443 444 /** 445 * @return The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 446 */ 447 public String getDeviceIdentifier() { 448 return this.deviceIdentifier == null ? null : this.deviceIdentifier.getValue(); 449 } 450 451 /** 452 * @param value The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 453 */ 454 public DeviceUdiComponent setDeviceIdentifier(String value) { 455 if (Utilities.noString(value)) 456 this.deviceIdentifier = null; 457 else { 458 if (this.deviceIdentifier == null) 459 this.deviceIdentifier = new StringType(); 460 this.deviceIdentifier.setValue(value); 461 } 462 return this; 463 } 464 465 /** 466 * @return {@link #name} (Name of device as used in labeling or catalog.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 467 */ 468 public StringType getNameElement() { 469 if (this.name == null) 470 if (Configuration.errorOnAutoCreate()) 471 throw new Error("Attempt to auto-create DeviceUdiComponent.name"); 472 else if (Configuration.doAutoCreate()) 473 this.name = new StringType(); // bb 474 return this.name; 475 } 476 477 public boolean hasNameElement() { 478 return this.name != null && !this.name.isEmpty(); 479 } 480 481 public boolean hasName() { 482 return this.name != null && !this.name.isEmpty(); 483 } 484 485 /** 486 * @param value {@link #name} (Name of device as used in labeling or catalog.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 487 */ 488 public DeviceUdiComponent setNameElement(StringType value) { 489 this.name = value; 490 return this; 491 } 492 493 /** 494 * @return Name of device as used in labeling or catalog. 495 */ 496 public String getName() { 497 return this.name == null ? null : this.name.getValue(); 498 } 499 500 /** 501 * @param value Name of device as used in labeling or catalog. 502 */ 503 public DeviceUdiComponent setName(String value) { 504 if (Utilities.noString(value)) 505 this.name = null; 506 else { 507 if (this.name == null) 508 this.name = new StringType(); 509 this.name.setValue(value); 510 } 511 return this; 512 } 513 514 /** 515 * @return {@link #jurisdiction} (The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 516 */ 517 public UriType getJurisdictionElement() { 518 if (this.jurisdiction == null) 519 if (Configuration.errorOnAutoCreate()) 520 throw new Error("Attempt to auto-create DeviceUdiComponent.jurisdiction"); 521 else if (Configuration.doAutoCreate()) 522 this.jurisdiction = new UriType(); // bb 523 return this.jurisdiction; 524 } 525 526 public boolean hasJurisdictionElement() { 527 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 528 } 529 530 public boolean hasJurisdiction() { 531 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 532 } 533 534 /** 535 * @param value {@link #jurisdiction} (The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 536 */ 537 public DeviceUdiComponent setJurisdictionElement(UriType value) { 538 this.jurisdiction = value; 539 return this; 540 } 541 542 /** 543 * @return The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi. 544 */ 545 public String getJurisdiction() { 546 return this.jurisdiction == null ? null : this.jurisdiction.getValue(); 547 } 548 549 /** 550 * @param value The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi. 551 */ 552 public DeviceUdiComponent setJurisdiction(String value) { 553 if (Utilities.noString(value)) 554 this.jurisdiction = null; 555 else { 556 if (this.jurisdiction == null) 557 this.jurisdiction = new UriType(); 558 this.jurisdiction.setValue(value); 559 } 560 return this; 561 } 562 563 /** 564 * @return {@link #carrierHRF} (The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.). This is the underlying object with id, value and extensions. The accessor "getCarrierHRF" gives direct access to the value 565 */ 566 public StringType getCarrierHRFElement() { 567 if (this.carrierHRF == null) 568 if (Configuration.errorOnAutoCreate()) 569 throw new Error("Attempt to auto-create DeviceUdiComponent.carrierHRF"); 570 else if (Configuration.doAutoCreate()) 571 this.carrierHRF = new StringType(); // bb 572 return this.carrierHRF; 573 } 574 575 public boolean hasCarrierHRFElement() { 576 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 577 } 578 579 public boolean hasCarrierHRF() { 580 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 581 } 582 583 /** 584 * @param value {@link #carrierHRF} (The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.). This is the underlying object with id, value and extensions. The accessor "getCarrierHRF" gives direct access to the value 585 */ 586 public DeviceUdiComponent setCarrierHRFElement(StringType value) { 587 this.carrierHRF = value; 588 return this; 589 } 590 591 /** 592 * @return The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 593 */ 594 public String getCarrierHRF() { 595 return this.carrierHRF == null ? null : this.carrierHRF.getValue(); 596 } 597 598 /** 599 * @param value The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 600 */ 601 public DeviceUdiComponent setCarrierHRF(String value) { 602 if (Utilities.noString(value)) 603 this.carrierHRF = null; 604 else { 605 if (this.carrierHRF == null) 606 this.carrierHRF = new StringType(); 607 this.carrierHRF.setValue(value); 608 } 609 return this; 610 } 611 612 /** 613 * @return {@link #carrierAIDC} (The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getCarrierAIDC" gives direct access to the value 614 */ 615 public Base64BinaryType getCarrierAIDCElement() { 616 if (this.carrierAIDC == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create DeviceUdiComponent.carrierAIDC"); 619 else if (Configuration.doAutoCreate()) 620 this.carrierAIDC = new Base64BinaryType(); // bb 621 return this.carrierAIDC; 622 } 623 624 public boolean hasCarrierAIDCElement() { 625 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 626 } 627 628 public boolean hasCarrierAIDC() { 629 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 630 } 631 632 /** 633 * @param value {@link #carrierAIDC} (The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getCarrierAIDC" gives direct access to the value 634 */ 635 public DeviceUdiComponent setCarrierAIDCElement(Base64BinaryType value) { 636 this.carrierAIDC = value; 637 return this; 638 } 639 640 /** 641 * @return The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 642 */ 643 public byte[] getCarrierAIDC() { 644 return this.carrierAIDC == null ? null : this.carrierAIDC.getValue(); 645 } 646 647 /** 648 * @param value The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 649 */ 650 public DeviceUdiComponent setCarrierAIDC(byte[] value) { 651 if (value == null) 652 this.carrierAIDC = null; 653 else { 654 if (this.carrierAIDC == null) 655 this.carrierAIDC = new Base64BinaryType(); 656 this.carrierAIDC.setValue(value); 657 } 658 return this; 659 } 660 661 /** 662 * @return {@link #issuer} (Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include : 6631) GS1: 664http://hl7.org/fhir/NamingSystem/gs1-di, 6652) HIBCC: 666http://hl7.org/fhir/NamingSystem/hibcc-dI, 6673) ICCBBA for blood containers: 668http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 6694) ICCBA for other devices: 670http://hl7.org/fhir/NamingSystem/iccbba-other-di.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 671 */ 672 public UriType getIssuerElement() { 673 if (this.issuer == null) 674 if (Configuration.errorOnAutoCreate()) 675 throw new Error("Attempt to auto-create DeviceUdiComponent.issuer"); 676 else if (Configuration.doAutoCreate()) 677 this.issuer = new UriType(); // bb 678 return this.issuer; 679 } 680 681 public boolean hasIssuerElement() { 682 return this.issuer != null && !this.issuer.isEmpty(); 683 } 684 685 public boolean hasIssuer() { 686 return this.issuer != null && !this.issuer.isEmpty(); 687 } 688 689 /** 690 * @param value {@link #issuer} (Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include : 6911) GS1: 692http://hl7.org/fhir/NamingSystem/gs1-di, 6932) HIBCC: 694http://hl7.org/fhir/NamingSystem/hibcc-dI, 6953) ICCBBA for blood containers: 696http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 6974) ICCBA for other devices: 698http://hl7.org/fhir/NamingSystem/iccbba-other-di.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 699 */ 700 public DeviceUdiComponent setIssuerElement(UriType value) { 701 this.issuer = value; 702 return this; 703 } 704 705 /** 706 * @return Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include : 7071) GS1: 708http://hl7.org/fhir/NamingSystem/gs1-di, 7092) HIBCC: 710http://hl7.org/fhir/NamingSystem/hibcc-dI, 7113) ICCBBA for blood containers: 712http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 7134) ICCBA for other devices: 714http://hl7.org/fhir/NamingSystem/iccbba-other-di. 715 */ 716 public String getIssuer() { 717 return this.issuer == null ? null : this.issuer.getValue(); 718 } 719 720 /** 721 * @param value Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include : 7221) GS1: 723http://hl7.org/fhir/NamingSystem/gs1-di, 7242) HIBCC: 725http://hl7.org/fhir/NamingSystem/hibcc-dI, 7263) ICCBBA for blood containers: 727http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 7284) ICCBA for other devices: 729http://hl7.org/fhir/NamingSystem/iccbba-other-di. 730 */ 731 public DeviceUdiComponent setIssuer(String value) { 732 if (Utilities.noString(value)) 733 this.issuer = null; 734 else { 735 if (this.issuer == null) 736 this.issuer = new UriType(); 737 this.issuer.setValue(value); 738 } 739 return this; 740 } 741 742 /** 743 * @return {@link #entryType} (A coded entry to indicate how the data was entered.). This is the underlying object with id, value and extensions. The accessor "getEntryType" gives direct access to the value 744 */ 745 public Enumeration<UDIEntryType> getEntryTypeElement() { 746 if (this.entryType == null) 747 if (Configuration.errorOnAutoCreate()) 748 throw new Error("Attempt to auto-create DeviceUdiComponent.entryType"); 749 else if (Configuration.doAutoCreate()) 750 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); // bb 751 return this.entryType; 752 } 753 754 public boolean hasEntryTypeElement() { 755 return this.entryType != null && !this.entryType.isEmpty(); 756 } 757 758 public boolean hasEntryType() { 759 return this.entryType != null && !this.entryType.isEmpty(); 760 } 761 762 /** 763 * @param value {@link #entryType} (A coded entry to indicate how the data was entered.). This is the underlying object with id, value and extensions. The accessor "getEntryType" gives direct access to the value 764 */ 765 public DeviceUdiComponent setEntryTypeElement(Enumeration<UDIEntryType> value) { 766 this.entryType = value; 767 return this; 768 } 769 770 /** 771 * @return A coded entry to indicate how the data was entered. 772 */ 773 public UDIEntryType getEntryType() { 774 return this.entryType == null ? null : this.entryType.getValue(); 775 } 776 777 /** 778 * @param value A coded entry to indicate how the data was entered. 779 */ 780 public DeviceUdiComponent setEntryType(UDIEntryType value) { 781 if (value == null) 782 this.entryType = null; 783 else { 784 if (this.entryType == null) 785 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); 786 this.entryType.setValue(value); 787 } 788 return this; 789 } 790 791 protected void listChildren(List<Property> children) { 792 super.listChildren(children); 793 children.add(new Property("deviceIdentifier", "string", "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 0, 1, deviceIdentifier)); 794 children.add(new Property("name", "string", "Name of device as used in labeling or catalog.", 0, 1, name)); 795 children.add(new Property("jurisdiction", "uri", "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi.", 0, 1, jurisdiction)); 796 children.add(new Property("carrierHRF", "string", "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 0, 1, carrierHRF)); 797 children.add(new Property("carrierAIDC", "base64Binary", "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 0, 1, carrierAIDC)); 798 children.add(new Property("issuer", "uri", "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include :\n1) GS1: \nhttp://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC:\nhttp://hl7.org/fhir/NamingSystem/hibcc-dI, \n3) ICCBBA for blood containers:\nhttp://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices:\nhttp://hl7.org/fhir/NamingSystem/iccbba-other-di.", 0, 1, issuer)); 799 children.add(new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 0, 1, entryType)); 800 } 801 802 @Override 803 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 804 switch (_hash) { 805 case 1322005407: /*deviceIdentifier*/ return new Property("deviceIdentifier", "string", "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 0, 1, deviceIdentifier); 806 case 3373707: /*name*/ return new Property("name", "string", "Name of device as used in labeling or catalog.", 0, 1, name); 807 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "uri", "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace. with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi.", 0, 1, jurisdiction); 808 case 806499972: /*carrierHRF*/ return new Property("carrierHRF", "string", "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 0, 1, carrierHRF); 809 case -768521825: /*carrierAIDC*/ return new Property("carrierAIDC", "base64Binary", "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - E.g a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 0, 1, carrierAIDC); 810 case -1179159879: /*issuer*/ return new Property("issuer", "uri", "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include :\n1) GS1: \nhttp://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC:\nhttp://hl7.org/fhir/NamingSystem/hibcc-dI, \n3) ICCBBA for blood containers:\nhttp://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices:\nhttp://hl7.org/fhir/NamingSystem/iccbba-other-di.", 0, 1, issuer); 811 case -479362356: /*entryType*/ return new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 0, 1, entryType); 812 default: return super.getNamedProperty(_hash, _name, _checkValid); 813 } 814 815 } 816 817 @Override 818 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 819 switch (hash) { 820 case 1322005407: /*deviceIdentifier*/ return this.deviceIdentifier == null ? new Base[0] : new Base[] {this.deviceIdentifier}; // StringType 821 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 822 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // UriType 823 case 806499972: /*carrierHRF*/ return this.carrierHRF == null ? new Base[0] : new Base[] {this.carrierHRF}; // StringType 824 case -768521825: /*carrierAIDC*/ return this.carrierAIDC == null ? new Base[0] : new Base[] {this.carrierAIDC}; // Base64BinaryType 825 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // UriType 826 case -479362356: /*entryType*/ return this.entryType == null ? new Base[0] : new Base[] {this.entryType}; // Enumeration<UDIEntryType> 827 default: return super.getProperty(hash, name, checkValid); 828 } 829 830 } 831 832 @Override 833 public Base setProperty(int hash, String name, Base value) throws FHIRException { 834 switch (hash) { 835 case 1322005407: // deviceIdentifier 836 this.deviceIdentifier = castToString(value); // StringType 837 return value; 838 case 3373707: // name 839 this.name = castToString(value); // StringType 840 return value; 841 case -507075711: // jurisdiction 842 this.jurisdiction = castToUri(value); // UriType 843 return value; 844 case 806499972: // carrierHRF 845 this.carrierHRF = castToString(value); // StringType 846 return value; 847 case -768521825: // carrierAIDC 848 this.carrierAIDC = castToBase64Binary(value); // Base64BinaryType 849 return value; 850 case -1179159879: // issuer 851 this.issuer = castToUri(value); // UriType 852 return value; 853 case -479362356: // entryType 854 value = new UDIEntryTypeEnumFactory().fromType(castToCode(value)); 855 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 856 return value; 857 default: return super.setProperty(hash, name, value); 858 } 859 860 } 861 862 @Override 863 public Base setProperty(String name, Base value) throws FHIRException { 864 if (name.equals("deviceIdentifier")) { 865 this.deviceIdentifier = castToString(value); // StringType 866 } else if (name.equals("name")) { 867 this.name = castToString(value); // StringType 868 } else if (name.equals("jurisdiction")) { 869 this.jurisdiction = castToUri(value); // UriType 870 } else if (name.equals("carrierHRF")) { 871 this.carrierHRF = castToString(value); // StringType 872 } else if (name.equals("carrierAIDC")) { 873 this.carrierAIDC = castToBase64Binary(value); // Base64BinaryType 874 } else if (name.equals("issuer")) { 875 this.issuer = castToUri(value); // UriType 876 } else if (name.equals("entryType")) { 877 value = new UDIEntryTypeEnumFactory().fromType(castToCode(value)); 878 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 879 } else 880 return super.setProperty(name, value); 881 return value; 882 } 883 884 @Override 885 public Base makeProperty(int hash, String name) throws FHIRException { 886 switch (hash) { 887 case 1322005407: return getDeviceIdentifierElement(); 888 case 3373707: return getNameElement(); 889 case -507075711: return getJurisdictionElement(); 890 case 806499972: return getCarrierHRFElement(); 891 case -768521825: return getCarrierAIDCElement(); 892 case -1179159879: return getIssuerElement(); 893 case -479362356: return getEntryTypeElement(); 894 default: return super.makeProperty(hash, name); 895 } 896 897 } 898 899 @Override 900 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 901 switch (hash) { 902 case 1322005407: /*deviceIdentifier*/ return new String[] {"string"}; 903 case 3373707: /*name*/ return new String[] {"string"}; 904 case -507075711: /*jurisdiction*/ return new String[] {"uri"}; 905 case 806499972: /*carrierHRF*/ return new String[] {"string"}; 906 case -768521825: /*carrierAIDC*/ return new String[] {"base64Binary"}; 907 case -1179159879: /*issuer*/ return new String[] {"uri"}; 908 case -479362356: /*entryType*/ return new String[] {"code"}; 909 default: return super.getTypesForProperty(hash, name); 910 } 911 912 } 913 914 @Override 915 public Base addChild(String name) throws FHIRException { 916 if (name.equals("deviceIdentifier")) { 917 throw new FHIRException("Cannot call addChild on a singleton property Device.deviceIdentifier"); 918 } 919 else if (name.equals("name")) { 920 throw new FHIRException("Cannot call addChild on a singleton property Device.name"); 921 } 922 else if (name.equals("jurisdiction")) { 923 throw new FHIRException("Cannot call addChild on a singleton property Device.jurisdiction"); 924 } 925 else if (name.equals("carrierHRF")) { 926 throw new FHIRException("Cannot call addChild on a singleton property Device.carrierHRF"); 927 } 928 else if (name.equals("carrierAIDC")) { 929 throw new FHIRException("Cannot call addChild on a singleton property Device.carrierAIDC"); 930 } 931 else if (name.equals("issuer")) { 932 throw new FHIRException("Cannot call addChild on a singleton property Device.issuer"); 933 } 934 else if (name.equals("entryType")) { 935 throw new FHIRException("Cannot call addChild on a singleton property Device.entryType"); 936 } 937 else 938 return super.addChild(name); 939 } 940 941 public DeviceUdiComponent copy() { 942 DeviceUdiComponent dst = new DeviceUdiComponent(); 943 copyValues(dst); 944 dst.deviceIdentifier = deviceIdentifier == null ? null : deviceIdentifier.copy(); 945 dst.name = name == null ? null : name.copy(); 946 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 947 dst.carrierHRF = carrierHRF == null ? null : carrierHRF.copy(); 948 dst.carrierAIDC = carrierAIDC == null ? null : carrierAIDC.copy(); 949 dst.issuer = issuer == null ? null : issuer.copy(); 950 dst.entryType = entryType == null ? null : entryType.copy(); 951 return dst; 952 } 953 954 @Override 955 public boolean equalsDeep(Base other_) { 956 if (!super.equalsDeep(other_)) 957 return false; 958 if (!(other_ instanceof DeviceUdiComponent)) 959 return false; 960 DeviceUdiComponent o = (DeviceUdiComponent) other_; 961 return compareDeep(deviceIdentifier, o.deviceIdentifier, true) && compareDeep(name, o.name, true) 962 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(carrierHRF, o.carrierHRF, true) 963 && compareDeep(carrierAIDC, o.carrierAIDC, true) && compareDeep(issuer, o.issuer, true) && compareDeep(entryType, o.entryType, true) 964 ; 965 } 966 967 @Override 968 public boolean equalsShallow(Base other_) { 969 if (!super.equalsShallow(other_)) 970 return false; 971 if (!(other_ instanceof DeviceUdiComponent)) 972 return false; 973 DeviceUdiComponent o = (DeviceUdiComponent) other_; 974 return compareValues(deviceIdentifier, o.deviceIdentifier, true) && compareValues(name, o.name, true) 975 && compareValues(jurisdiction, o.jurisdiction, true) && compareValues(carrierHRF, o.carrierHRF, true) 976 && compareValues(carrierAIDC, o.carrierAIDC, true) && compareValues(issuer, o.issuer, true) && compareValues(entryType, o.entryType, true) 977 ; 978 } 979 980 public boolean isEmpty() { 981 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(deviceIdentifier, name, jurisdiction 982 , carrierHRF, carrierAIDC, issuer, entryType); 983 } 984 985 public String fhirType() { 986 return "Device.udi"; 987 988 } 989 990 } 991 992 /** 993 * Unique instance identifiers assigned to a device by manufacturers other organizations or owners. 994 */ 995 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 996 @Description(shortDefinition="Instance identifier", formalDefinition="Unique instance identifiers assigned to a device by manufacturers other organizations or owners." ) 997 protected List<Identifier> identifier; 998 999 /** 1000 * [Unique device identifier (UDI)](device.html#5.11.3.2.2) assigned to device label or package. 1001 */ 1002 @Child(name = "udi", type = {}, order=1, min=0, max=1, modifier=false, summary=true) 1003 @Description(shortDefinition="Unique Device Identifier (UDI) Barcode string", formalDefinition="[Unique device identifier (UDI)](device.html#5.11.3.2.2) assigned to device label or package." ) 1004 protected DeviceUdiComponent udi; 1005 1006 /** 1007 * Status of the Device availability. 1008 */ 1009 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 1010 @Description(shortDefinition="active | inactive | entered-in-error | unknown", formalDefinition="Status of the Device availability." ) 1011 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-status") 1012 protected Enumeration<FHIRDeviceStatus> status; 1013 1014 /** 1015 * Code or identifier to identify a kind of device. 1016 */ 1017 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1018 @Description(shortDefinition="What kind of device this is", formalDefinition="Code or identifier to identify a kind of device." ) 1019 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-kind") 1020 protected CodeableConcept type; 1021 1022 /** 1023 * Lot number assigned by the manufacturer. 1024 */ 1025 @Child(name = "lotNumber", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1026 @Description(shortDefinition="Lot number of manufacture", formalDefinition="Lot number assigned by the manufacturer." ) 1027 protected StringType lotNumber; 1028 1029 /** 1030 * A name of the manufacturer. 1031 */ 1032 @Child(name = "manufacturer", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1033 @Description(shortDefinition="Name of device manufacturer", formalDefinition="A name of the manufacturer." ) 1034 protected StringType manufacturer; 1035 1036 /** 1037 * The date and time when the device was manufactured. 1038 */ 1039 @Child(name = "manufactureDate", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1040 @Description(shortDefinition="Date when the device was made", formalDefinition="The date and time when the device was manufactured." ) 1041 protected DateTimeType manufactureDate; 1042 1043 /** 1044 * The date and time beyond which this device is no longer valid or should not be used (if applicable). 1045 */ 1046 @Child(name = "expirationDate", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 1047 @Description(shortDefinition="Date and time of expiry of this device (if applicable)", formalDefinition="The date and time beyond which this device is no longer valid or should not be used (if applicable)." ) 1048 protected DateTimeType expirationDate; 1049 1050 /** 1051 * The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type. 1052 */ 1053 @Child(name = "model", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1054 @Description(shortDefinition="Model id assigned by the manufacturer", formalDefinition="The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type." ) 1055 protected StringType model; 1056 1057 /** 1058 * The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware. 1059 */ 1060 @Child(name = "version", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1061 @Description(shortDefinition="Version number (i.e. software)", formalDefinition="The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware." ) 1062 protected StringType version; 1063 1064 /** 1065 * Patient information, If the device is affixed to a person. 1066 */ 1067 @Child(name = "patient", type = {Patient.class}, order=10, min=0, max=1, modifier=false, summary=false) 1068 @Description(shortDefinition="Patient to whom Device is affixed", formalDefinition="Patient information, If the device is affixed to a person." ) 1069 protected Reference patient; 1070 1071 /** 1072 * The actual object that is the target of the reference (Patient information, If the device is affixed to a person.) 1073 */ 1074 protected Patient patientTarget; 1075 1076 /** 1077 * An organization that is responsible for the provision and ongoing maintenance of the device. 1078 */ 1079 @Child(name = "owner", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=false) 1080 @Description(shortDefinition="Organization responsible for device", formalDefinition="An organization that is responsible for the provision and ongoing maintenance of the device." ) 1081 protected Reference owner; 1082 1083 /** 1084 * The actual object that is the target of the reference (An organization that is responsible for the provision and ongoing maintenance of the device.) 1085 */ 1086 protected Organization ownerTarget; 1087 1088 /** 1089 * Contact details for an organization or a particular human that is responsible for the device. 1090 */ 1091 @Child(name = "contact", type = {ContactPoint.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1092 @Description(shortDefinition="Details for human/organization for support", formalDefinition="Contact details for an organization or a particular human that is responsible for the device." ) 1093 protected List<ContactPoint> contact; 1094 1095 /** 1096 * The place where the device can be found. 1097 */ 1098 @Child(name = "location", type = {Location.class}, order=13, min=0, max=1, modifier=false, summary=false) 1099 @Description(shortDefinition="Where the resource is found", formalDefinition="The place where the device can be found." ) 1100 protected Reference location; 1101 1102 /** 1103 * The actual object that is the target of the reference (The place where the device can be found.) 1104 */ 1105 protected Location locationTarget; 1106 1107 /** 1108 * A network address on which the device may be contacted directly. 1109 */ 1110 @Child(name = "url", type = {UriType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1111 @Description(shortDefinition="Network address to contact device", formalDefinition="A network address on which the device may be contacted directly." ) 1112 protected UriType url; 1113 1114 /** 1115 * Descriptive information, usage information or implantation information that is not captured in an existing element. 1116 */ 1117 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1118 @Description(shortDefinition="Device notes and comments", formalDefinition="Descriptive information, usage information or implantation information that is not captured in an existing element." ) 1119 protected List<Annotation> note; 1120 1121 /** 1122 * Provides additional safety characteristics about a medical device. For example devices containing latex. 1123 */ 1124 @Child(name = "safety", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1125 @Description(shortDefinition="Safety Characteristics of Device", formalDefinition="Provides additional safety characteristics about a medical device. For example devices containing latex." ) 1126 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-safety") 1127 protected List<CodeableConcept> safety; 1128 1129 private static final long serialVersionUID = -1056263930L; 1130 1131 /** 1132 * Constructor 1133 */ 1134 public Device() { 1135 super(); 1136 } 1137 1138 /** 1139 * @return {@link #identifier} (Unique instance identifiers assigned to a device by manufacturers other organizations or owners.) 1140 */ 1141 public List<Identifier> getIdentifier() { 1142 if (this.identifier == null) 1143 this.identifier = new ArrayList<Identifier>(); 1144 return this.identifier; 1145 } 1146 1147 /** 1148 * @return Returns a reference to <code>this</code> for easy method chaining 1149 */ 1150 public Device setIdentifier(List<Identifier> theIdentifier) { 1151 this.identifier = theIdentifier; 1152 return this; 1153 } 1154 1155 public boolean hasIdentifier() { 1156 if (this.identifier == null) 1157 return false; 1158 for (Identifier item : this.identifier) 1159 if (!item.isEmpty()) 1160 return true; 1161 return false; 1162 } 1163 1164 public Identifier addIdentifier() { //3 1165 Identifier t = new Identifier(); 1166 if (this.identifier == null) 1167 this.identifier = new ArrayList<Identifier>(); 1168 this.identifier.add(t); 1169 return t; 1170 } 1171 1172 public Device addIdentifier(Identifier t) { //3 1173 if (t == null) 1174 return this; 1175 if (this.identifier == null) 1176 this.identifier = new ArrayList<Identifier>(); 1177 this.identifier.add(t); 1178 return this; 1179 } 1180 1181 /** 1182 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1183 */ 1184 public Identifier getIdentifierFirstRep() { 1185 if (getIdentifier().isEmpty()) { 1186 addIdentifier(); 1187 } 1188 return getIdentifier().get(0); 1189 } 1190 1191 /** 1192 * @return {@link #udi} ([Unique device identifier (UDI)](device.html#5.11.3.2.2) assigned to device label or package.) 1193 */ 1194 public DeviceUdiComponent getUdi() { 1195 if (this.udi == null) 1196 if (Configuration.errorOnAutoCreate()) 1197 throw new Error("Attempt to auto-create Device.udi"); 1198 else if (Configuration.doAutoCreate()) 1199 this.udi = new DeviceUdiComponent(); // cc 1200 return this.udi; 1201 } 1202 1203 public boolean hasUdi() { 1204 return this.udi != null && !this.udi.isEmpty(); 1205 } 1206 1207 /** 1208 * @param value {@link #udi} ([Unique device identifier (UDI)](device.html#5.11.3.2.2) assigned to device label or package.) 1209 */ 1210 public Device setUdi(DeviceUdiComponent value) { 1211 this.udi = value; 1212 return this; 1213 } 1214 1215 /** 1216 * @return {@link #status} (Status of the Device availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1217 */ 1218 public Enumeration<FHIRDeviceStatus> getStatusElement() { 1219 if (this.status == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create Device.status"); 1222 else if (Configuration.doAutoCreate()) 1223 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); // bb 1224 return this.status; 1225 } 1226 1227 public boolean hasStatusElement() { 1228 return this.status != null && !this.status.isEmpty(); 1229 } 1230 1231 public boolean hasStatus() { 1232 return this.status != null && !this.status.isEmpty(); 1233 } 1234 1235 /** 1236 * @param value {@link #status} (Status of the Device availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1237 */ 1238 public Device setStatusElement(Enumeration<FHIRDeviceStatus> value) { 1239 this.status = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return Status of the Device availability. 1245 */ 1246 public FHIRDeviceStatus getStatus() { 1247 return this.status == null ? null : this.status.getValue(); 1248 } 1249 1250 /** 1251 * @param value Status of the Device availability. 1252 */ 1253 public Device setStatus(FHIRDeviceStatus value) { 1254 if (value == null) 1255 this.status = null; 1256 else { 1257 if (this.status == null) 1258 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); 1259 this.status.setValue(value); 1260 } 1261 return this; 1262 } 1263 1264 /** 1265 * @return {@link #type} (Code or identifier to identify a kind of device.) 1266 */ 1267 public CodeableConcept getType() { 1268 if (this.type == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create Device.type"); 1271 else if (Configuration.doAutoCreate()) 1272 this.type = new CodeableConcept(); // cc 1273 return this.type; 1274 } 1275 1276 public boolean hasType() { 1277 return this.type != null && !this.type.isEmpty(); 1278 } 1279 1280 /** 1281 * @param value {@link #type} (Code or identifier to identify a kind of device.) 1282 */ 1283 public Device setType(CodeableConcept value) { 1284 this.type = value; 1285 return this; 1286 } 1287 1288 /** 1289 * @return {@link #lotNumber} (Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1290 */ 1291 public StringType getLotNumberElement() { 1292 if (this.lotNumber == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create Device.lotNumber"); 1295 else if (Configuration.doAutoCreate()) 1296 this.lotNumber = new StringType(); // bb 1297 return this.lotNumber; 1298 } 1299 1300 public boolean hasLotNumberElement() { 1301 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1302 } 1303 1304 public boolean hasLotNumber() { 1305 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1306 } 1307 1308 /** 1309 * @param value {@link #lotNumber} (Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1310 */ 1311 public Device setLotNumberElement(StringType value) { 1312 this.lotNumber = value; 1313 return this; 1314 } 1315 1316 /** 1317 * @return Lot number assigned by the manufacturer. 1318 */ 1319 public String getLotNumber() { 1320 return this.lotNumber == null ? null : this.lotNumber.getValue(); 1321 } 1322 1323 /** 1324 * @param value Lot number assigned by the manufacturer. 1325 */ 1326 public Device setLotNumber(String value) { 1327 if (Utilities.noString(value)) 1328 this.lotNumber = null; 1329 else { 1330 if (this.lotNumber == null) 1331 this.lotNumber = new StringType(); 1332 this.lotNumber.setValue(value); 1333 } 1334 return this; 1335 } 1336 1337 /** 1338 * @return {@link #manufacturer} (A name of the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value 1339 */ 1340 public StringType getManufacturerElement() { 1341 if (this.manufacturer == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create Device.manufacturer"); 1344 else if (Configuration.doAutoCreate()) 1345 this.manufacturer = new StringType(); // bb 1346 return this.manufacturer; 1347 } 1348 1349 public boolean hasManufacturerElement() { 1350 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1351 } 1352 1353 public boolean hasManufacturer() { 1354 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1355 } 1356 1357 /** 1358 * @param value {@link #manufacturer} (A name of the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value 1359 */ 1360 public Device setManufacturerElement(StringType value) { 1361 this.manufacturer = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return A name of the manufacturer. 1367 */ 1368 public String getManufacturer() { 1369 return this.manufacturer == null ? null : this.manufacturer.getValue(); 1370 } 1371 1372 /** 1373 * @param value A name of the manufacturer. 1374 */ 1375 public Device setManufacturer(String value) { 1376 if (Utilities.noString(value)) 1377 this.manufacturer = null; 1378 else { 1379 if (this.manufacturer == null) 1380 this.manufacturer = new StringType(); 1381 this.manufacturer.setValue(value); 1382 } 1383 return this; 1384 } 1385 1386 /** 1387 * @return {@link #manufactureDate} (The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value 1388 */ 1389 public DateTimeType getManufactureDateElement() { 1390 if (this.manufactureDate == null) 1391 if (Configuration.errorOnAutoCreate()) 1392 throw new Error("Attempt to auto-create Device.manufactureDate"); 1393 else if (Configuration.doAutoCreate()) 1394 this.manufactureDate = new DateTimeType(); // bb 1395 return this.manufactureDate; 1396 } 1397 1398 public boolean hasManufactureDateElement() { 1399 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 1400 } 1401 1402 public boolean hasManufactureDate() { 1403 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 1404 } 1405 1406 /** 1407 * @param value {@link #manufactureDate} (The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value 1408 */ 1409 public Device setManufactureDateElement(DateTimeType value) { 1410 this.manufactureDate = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return The date and time when the device was manufactured. 1416 */ 1417 public Date getManufactureDate() { 1418 return this.manufactureDate == null ? null : this.manufactureDate.getValue(); 1419 } 1420 1421 /** 1422 * @param value The date and time when the device was manufactured. 1423 */ 1424 public Device setManufactureDate(Date value) { 1425 if (value == null) 1426 this.manufactureDate = null; 1427 else { 1428 if (this.manufactureDate == null) 1429 this.manufactureDate = new DateTimeType(); 1430 this.manufactureDate.setValue(value); 1431 } 1432 return this; 1433 } 1434 1435 /** 1436 * @return {@link #expirationDate} (The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1437 */ 1438 public DateTimeType getExpirationDateElement() { 1439 if (this.expirationDate == null) 1440 if (Configuration.errorOnAutoCreate()) 1441 throw new Error("Attempt to auto-create Device.expirationDate"); 1442 else if (Configuration.doAutoCreate()) 1443 this.expirationDate = new DateTimeType(); // bb 1444 return this.expirationDate; 1445 } 1446 1447 public boolean hasExpirationDateElement() { 1448 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1449 } 1450 1451 public boolean hasExpirationDate() { 1452 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1453 } 1454 1455 /** 1456 * @param value {@link #expirationDate} (The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1457 */ 1458 public Device setExpirationDateElement(DateTimeType value) { 1459 this.expirationDate = value; 1460 return this; 1461 } 1462 1463 /** 1464 * @return The date and time beyond which this device is no longer valid or should not be used (if applicable). 1465 */ 1466 public Date getExpirationDate() { 1467 return this.expirationDate == null ? null : this.expirationDate.getValue(); 1468 } 1469 1470 /** 1471 * @param value The date and time beyond which this device is no longer valid or should not be used (if applicable). 1472 */ 1473 public Device setExpirationDate(Date value) { 1474 if (value == null) 1475 this.expirationDate = null; 1476 else { 1477 if (this.expirationDate == null) 1478 this.expirationDate = new DateTimeType(); 1479 this.expirationDate.setValue(value); 1480 } 1481 return this; 1482 } 1483 1484 /** 1485 * @return {@link #model} (The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.). This is the underlying object with id, value and extensions. The accessor "getModel" gives direct access to the value 1486 */ 1487 public StringType getModelElement() { 1488 if (this.model == null) 1489 if (Configuration.errorOnAutoCreate()) 1490 throw new Error("Attempt to auto-create Device.model"); 1491 else if (Configuration.doAutoCreate()) 1492 this.model = new StringType(); // bb 1493 return this.model; 1494 } 1495 1496 public boolean hasModelElement() { 1497 return this.model != null && !this.model.isEmpty(); 1498 } 1499 1500 public boolean hasModel() { 1501 return this.model != null && !this.model.isEmpty(); 1502 } 1503 1504 /** 1505 * @param value {@link #model} (The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.). This is the underlying object with id, value and extensions. The accessor "getModel" gives direct access to the value 1506 */ 1507 public Device setModelElement(StringType value) { 1508 this.model = value; 1509 return this; 1510 } 1511 1512 /** 1513 * @return The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type. 1514 */ 1515 public String getModel() { 1516 return this.model == null ? null : this.model.getValue(); 1517 } 1518 1519 /** 1520 * @param value The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type. 1521 */ 1522 public Device setModel(String value) { 1523 if (Utilities.noString(value)) 1524 this.model = null; 1525 else { 1526 if (this.model == null) 1527 this.model = new StringType(); 1528 this.model.setValue(value); 1529 } 1530 return this; 1531 } 1532 1533 /** 1534 * @return {@link #version} (The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1535 */ 1536 public StringType getVersionElement() { 1537 if (this.version == null) 1538 if (Configuration.errorOnAutoCreate()) 1539 throw new Error("Attempt to auto-create Device.version"); 1540 else if (Configuration.doAutoCreate()) 1541 this.version = new StringType(); // bb 1542 return this.version; 1543 } 1544 1545 public boolean hasVersionElement() { 1546 return this.version != null && !this.version.isEmpty(); 1547 } 1548 1549 public boolean hasVersion() { 1550 return this.version != null && !this.version.isEmpty(); 1551 } 1552 1553 /** 1554 * @param value {@link #version} (The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1555 */ 1556 public Device setVersionElement(StringType value) { 1557 this.version = value; 1558 return this; 1559 } 1560 1561 /** 1562 * @return The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware. 1563 */ 1564 public String getVersion() { 1565 return this.version == null ? null : this.version.getValue(); 1566 } 1567 1568 /** 1569 * @param value The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware. 1570 */ 1571 public Device setVersion(String value) { 1572 if (Utilities.noString(value)) 1573 this.version = null; 1574 else { 1575 if (this.version == null) 1576 this.version = new StringType(); 1577 this.version.setValue(value); 1578 } 1579 return this; 1580 } 1581 1582 /** 1583 * @return {@link #patient} (Patient information, If the device is affixed to a person.) 1584 */ 1585 public Reference getPatient() { 1586 if (this.patient == null) 1587 if (Configuration.errorOnAutoCreate()) 1588 throw new Error("Attempt to auto-create Device.patient"); 1589 else if (Configuration.doAutoCreate()) 1590 this.patient = new Reference(); // cc 1591 return this.patient; 1592 } 1593 1594 public boolean hasPatient() { 1595 return this.patient != null && !this.patient.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #patient} (Patient information, If the device is affixed to a person.) 1600 */ 1601 public Device setPatient(Reference value) { 1602 this.patient = value; 1603 return this; 1604 } 1605 1606 /** 1607 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient information, If the device is affixed to a person.) 1608 */ 1609 public Patient getPatientTarget() { 1610 if (this.patientTarget == null) 1611 if (Configuration.errorOnAutoCreate()) 1612 throw new Error("Attempt to auto-create Device.patient"); 1613 else if (Configuration.doAutoCreate()) 1614 this.patientTarget = new Patient(); // aa 1615 return this.patientTarget; 1616 } 1617 1618 /** 1619 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient information, If the device is affixed to a person.) 1620 */ 1621 public Device setPatientTarget(Patient value) { 1622 this.patientTarget = value; 1623 return this; 1624 } 1625 1626 /** 1627 * @return {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 1628 */ 1629 public Reference getOwner() { 1630 if (this.owner == null) 1631 if (Configuration.errorOnAutoCreate()) 1632 throw new Error("Attempt to auto-create Device.owner"); 1633 else if (Configuration.doAutoCreate()) 1634 this.owner = new Reference(); // cc 1635 return this.owner; 1636 } 1637 1638 public boolean hasOwner() { 1639 return this.owner != null && !this.owner.isEmpty(); 1640 } 1641 1642 /** 1643 * @param value {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 1644 */ 1645 public Device setOwner(Reference value) { 1646 this.owner = value; 1647 return this; 1648 } 1649 1650 /** 1651 * @return {@link #owner} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An organization that is responsible for the provision and ongoing maintenance of the device.) 1652 */ 1653 public Organization getOwnerTarget() { 1654 if (this.ownerTarget == null) 1655 if (Configuration.errorOnAutoCreate()) 1656 throw new Error("Attempt to auto-create Device.owner"); 1657 else if (Configuration.doAutoCreate()) 1658 this.ownerTarget = new Organization(); // aa 1659 return this.ownerTarget; 1660 } 1661 1662 /** 1663 * @param value {@link #owner} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An organization that is responsible for the provision and ongoing maintenance of the device.) 1664 */ 1665 public Device setOwnerTarget(Organization value) { 1666 this.ownerTarget = value; 1667 return this; 1668 } 1669 1670 /** 1671 * @return {@link #contact} (Contact details for an organization or a particular human that is responsible for the device.) 1672 */ 1673 public List<ContactPoint> getContact() { 1674 if (this.contact == null) 1675 this.contact = new ArrayList<ContactPoint>(); 1676 return this.contact; 1677 } 1678 1679 /** 1680 * @return Returns a reference to <code>this</code> for easy method chaining 1681 */ 1682 public Device setContact(List<ContactPoint> theContact) { 1683 this.contact = theContact; 1684 return this; 1685 } 1686 1687 public boolean hasContact() { 1688 if (this.contact == null) 1689 return false; 1690 for (ContactPoint item : this.contact) 1691 if (!item.isEmpty()) 1692 return true; 1693 return false; 1694 } 1695 1696 public ContactPoint addContact() { //3 1697 ContactPoint t = new ContactPoint(); 1698 if (this.contact == null) 1699 this.contact = new ArrayList<ContactPoint>(); 1700 this.contact.add(t); 1701 return t; 1702 } 1703 1704 public Device addContact(ContactPoint t) { //3 1705 if (t == null) 1706 return this; 1707 if (this.contact == null) 1708 this.contact = new ArrayList<ContactPoint>(); 1709 this.contact.add(t); 1710 return this; 1711 } 1712 1713 /** 1714 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1715 */ 1716 public ContactPoint getContactFirstRep() { 1717 if (getContact().isEmpty()) { 1718 addContact(); 1719 } 1720 return getContact().get(0); 1721 } 1722 1723 /** 1724 * @return {@link #location} (The place where the device can be found.) 1725 */ 1726 public Reference getLocation() { 1727 if (this.location == null) 1728 if (Configuration.errorOnAutoCreate()) 1729 throw new Error("Attempt to auto-create Device.location"); 1730 else if (Configuration.doAutoCreate()) 1731 this.location = new Reference(); // cc 1732 return this.location; 1733 } 1734 1735 public boolean hasLocation() { 1736 return this.location != null && !this.location.isEmpty(); 1737 } 1738 1739 /** 1740 * @param value {@link #location} (The place where the device can be found.) 1741 */ 1742 public Device setLocation(Reference value) { 1743 this.location = value; 1744 return this; 1745 } 1746 1747 /** 1748 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The place where the device can be found.) 1749 */ 1750 public Location getLocationTarget() { 1751 if (this.locationTarget == null) 1752 if (Configuration.errorOnAutoCreate()) 1753 throw new Error("Attempt to auto-create Device.location"); 1754 else if (Configuration.doAutoCreate()) 1755 this.locationTarget = new Location(); // aa 1756 return this.locationTarget; 1757 } 1758 1759 /** 1760 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The place where the device can be found.) 1761 */ 1762 public Device setLocationTarget(Location value) { 1763 this.locationTarget = value; 1764 return this; 1765 } 1766 1767 /** 1768 * @return {@link #url} (A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1769 */ 1770 public UriType getUrlElement() { 1771 if (this.url == null) 1772 if (Configuration.errorOnAutoCreate()) 1773 throw new Error("Attempt to auto-create Device.url"); 1774 else if (Configuration.doAutoCreate()) 1775 this.url = new UriType(); // bb 1776 return this.url; 1777 } 1778 1779 public boolean hasUrlElement() { 1780 return this.url != null && !this.url.isEmpty(); 1781 } 1782 1783 public boolean hasUrl() { 1784 return this.url != null && !this.url.isEmpty(); 1785 } 1786 1787 /** 1788 * @param value {@link #url} (A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1789 */ 1790 public Device setUrlElement(UriType value) { 1791 this.url = value; 1792 return this; 1793 } 1794 1795 /** 1796 * @return A network address on which the device may be contacted directly. 1797 */ 1798 public String getUrl() { 1799 return this.url == null ? null : this.url.getValue(); 1800 } 1801 1802 /** 1803 * @param value A network address on which the device may be contacted directly. 1804 */ 1805 public Device setUrl(String value) { 1806 if (Utilities.noString(value)) 1807 this.url = null; 1808 else { 1809 if (this.url == null) 1810 this.url = new UriType(); 1811 this.url.setValue(value); 1812 } 1813 return this; 1814 } 1815 1816 /** 1817 * @return {@link #note} (Descriptive information, usage information or implantation information that is not captured in an existing element.) 1818 */ 1819 public List<Annotation> getNote() { 1820 if (this.note == null) 1821 this.note = new ArrayList<Annotation>(); 1822 return this.note; 1823 } 1824 1825 /** 1826 * @return Returns a reference to <code>this</code> for easy method chaining 1827 */ 1828 public Device setNote(List<Annotation> theNote) { 1829 this.note = theNote; 1830 return this; 1831 } 1832 1833 public boolean hasNote() { 1834 if (this.note == null) 1835 return false; 1836 for (Annotation item : this.note) 1837 if (!item.isEmpty()) 1838 return true; 1839 return false; 1840 } 1841 1842 public Annotation addNote() { //3 1843 Annotation t = new Annotation(); 1844 if (this.note == null) 1845 this.note = new ArrayList<Annotation>(); 1846 this.note.add(t); 1847 return t; 1848 } 1849 1850 public Device addNote(Annotation t) { //3 1851 if (t == null) 1852 return this; 1853 if (this.note == null) 1854 this.note = new ArrayList<Annotation>(); 1855 this.note.add(t); 1856 return this; 1857 } 1858 1859 /** 1860 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1861 */ 1862 public Annotation getNoteFirstRep() { 1863 if (getNote().isEmpty()) { 1864 addNote(); 1865 } 1866 return getNote().get(0); 1867 } 1868 1869 /** 1870 * @return {@link #safety} (Provides additional safety characteristics about a medical device. For example devices containing latex.) 1871 */ 1872 public List<CodeableConcept> getSafety() { 1873 if (this.safety == null) 1874 this.safety = new ArrayList<CodeableConcept>(); 1875 return this.safety; 1876 } 1877 1878 /** 1879 * @return Returns a reference to <code>this</code> for easy method chaining 1880 */ 1881 public Device setSafety(List<CodeableConcept> theSafety) { 1882 this.safety = theSafety; 1883 return this; 1884 } 1885 1886 public boolean hasSafety() { 1887 if (this.safety == null) 1888 return false; 1889 for (CodeableConcept item : this.safety) 1890 if (!item.isEmpty()) 1891 return true; 1892 return false; 1893 } 1894 1895 public CodeableConcept addSafety() { //3 1896 CodeableConcept t = new CodeableConcept(); 1897 if (this.safety == null) 1898 this.safety = new ArrayList<CodeableConcept>(); 1899 this.safety.add(t); 1900 return t; 1901 } 1902 1903 public Device addSafety(CodeableConcept t) { //3 1904 if (t == null) 1905 return this; 1906 if (this.safety == null) 1907 this.safety = new ArrayList<CodeableConcept>(); 1908 this.safety.add(t); 1909 return this; 1910 } 1911 1912 /** 1913 * @return The first repetition of repeating field {@link #safety}, creating it if it does not already exist 1914 */ 1915 public CodeableConcept getSafetyFirstRep() { 1916 if (getSafety().isEmpty()) { 1917 addSafety(); 1918 } 1919 return getSafety().get(0); 1920 } 1921 1922 protected void listChildren(List<Property> children) { 1923 super.listChildren(children); 1924 children.add(new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1925 children.add(new Property("udi", "", "[Unique device identifier (UDI)](device.html#5.11.3.2.2) assigned to device label or package.", 0, 1, udi)); 1926 children.add(new Property("status", "code", "Status of the Device availability.", 0, 1, status)); 1927 children.add(new Property("type", "CodeableConcept", "Code or identifier to identify a kind of device.", 0, 1, type)); 1928 children.add(new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, lotNumber)); 1929 children.add(new Property("manufacturer", "string", "A name of the manufacturer.", 0, 1, manufacturer)); 1930 children.add(new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 0, 1, manufactureDate)); 1931 children.add(new Property("expirationDate", "dateTime", "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, expirationDate)); 1932 children.add(new Property("model", "string", "The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.", 0, 1, model)); 1933 children.add(new Property("version", "string", "The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.", 0, 1, version)); 1934 children.add(new Property("patient", "Reference(Patient)", "Patient information, If the device is affixed to a person.", 0, 1, patient)); 1935 children.add(new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner)); 1936 children.add(new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact)); 1937 children.add(new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1, location)); 1938 children.add(new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url)); 1939 children.add(new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note)); 1940 children.add(new Property("safety", "CodeableConcept", "Provides additional safety characteristics about a medical device. For example devices containing latex.", 0, java.lang.Integer.MAX_VALUE, safety)); 1941 } 1942 1943 @Override 1944 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1945 switch (_hash) { 1946 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, java.lang.Integer.MAX_VALUE, identifier); 1947 case 115642: /*udi*/ return new Property("udi", "", "[Unique device identifier (UDI)](device.html#5.11.3.2.2) assigned to device label or package.", 0, 1, udi); 1948 case -892481550: /*status*/ return new Property("status", "code", "Status of the Device availability.", 0, 1, status); 1949 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code or identifier to identify a kind of device.", 0, 1, type); 1950 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, lotNumber); 1951 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "string", "A name of the manufacturer.", 0, 1, manufacturer); 1952 case 416714767: /*manufactureDate*/ return new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 0, 1, manufactureDate); 1953 case -668811523: /*expirationDate*/ return new Property("expirationDate", "dateTime", "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, expirationDate); 1954 case 104069929: /*model*/ return new Property("model", "string", "The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.", 0, 1, model); 1955 case 351608024: /*version*/ return new Property("version", "string", "The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.", 0, 1, version); 1956 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Patient information, If the device is affixed to a person.", 0, 1, patient); 1957 case 106164915: /*owner*/ return new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner); 1958 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact); 1959 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1, location); 1960 case 116079: /*url*/ return new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url); 1961 case 3387378: /*note*/ return new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note); 1962 case -909893934: /*safety*/ return new Property("safety", "CodeableConcept", "Provides additional safety characteristics about a medical device. For example devices containing latex.", 0, java.lang.Integer.MAX_VALUE, safety); 1963 default: return super.getNamedProperty(_hash, _name, _checkValid); 1964 } 1965 1966 } 1967 1968 @Override 1969 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1970 switch (hash) { 1971 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1972 case 115642: /*udi*/ return this.udi == null ? new Base[0] : new Base[] {this.udi}; // DeviceUdiComponent 1973 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FHIRDeviceStatus> 1974 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1975 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 1976 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // StringType 1977 case 416714767: /*manufactureDate*/ return this.manufactureDate == null ? new Base[0] : new Base[] {this.manufactureDate}; // DateTimeType 1978 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateTimeType 1979 case 104069929: /*model*/ return this.model == null ? new Base[0] : new Base[] {this.model}; // StringType 1980 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1981 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1982 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 1983 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 1984 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1985 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1986 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1987 case -909893934: /*safety*/ return this.safety == null ? new Base[0] : this.safety.toArray(new Base[this.safety.size()]); // CodeableConcept 1988 default: return super.getProperty(hash, name, checkValid); 1989 } 1990 1991 } 1992 1993 @Override 1994 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1995 switch (hash) { 1996 case -1618432855: // identifier 1997 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1998 return value; 1999 case 115642: // udi 2000 this.udi = (DeviceUdiComponent) value; // DeviceUdiComponent 2001 return value; 2002 case -892481550: // status 2003 value = new FHIRDeviceStatusEnumFactory().fromType(castToCode(value)); 2004 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 2005 return value; 2006 case 3575610: // type 2007 this.type = castToCodeableConcept(value); // CodeableConcept 2008 return value; 2009 case 462547450: // lotNumber 2010 this.lotNumber = castToString(value); // StringType 2011 return value; 2012 case -1969347631: // manufacturer 2013 this.manufacturer = castToString(value); // StringType 2014 return value; 2015 case 416714767: // manufactureDate 2016 this.manufactureDate = castToDateTime(value); // DateTimeType 2017 return value; 2018 case -668811523: // expirationDate 2019 this.expirationDate = castToDateTime(value); // DateTimeType 2020 return value; 2021 case 104069929: // model 2022 this.model = castToString(value); // StringType 2023 return value; 2024 case 351608024: // version 2025 this.version = castToString(value); // StringType 2026 return value; 2027 case -791418107: // patient 2028 this.patient = castToReference(value); // Reference 2029 return value; 2030 case 106164915: // owner 2031 this.owner = castToReference(value); // Reference 2032 return value; 2033 case 951526432: // contact 2034 this.getContact().add(castToContactPoint(value)); // ContactPoint 2035 return value; 2036 case 1901043637: // location 2037 this.location = castToReference(value); // Reference 2038 return value; 2039 case 116079: // url 2040 this.url = castToUri(value); // UriType 2041 return value; 2042 case 3387378: // note 2043 this.getNote().add(castToAnnotation(value)); // Annotation 2044 return value; 2045 case -909893934: // safety 2046 this.getSafety().add(castToCodeableConcept(value)); // CodeableConcept 2047 return value; 2048 default: return super.setProperty(hash, name, value); 2049 } 2050 2051 } 2052 2053 @Override 2054 public Base setProperty(String name, Base value) throws FHIRException { 2055 if (name.equals("identifier")) { 2056 this.getIdentifier().add(castToIdentifier(value)); 2057 } else if (name.equals("udi")) { 2058 this.udi = (DeviceUdiComponent) value; // DeviceUdiComponent 2059 } else if (name.equals("status")) { 2060 value = new FHIRDeviceStatusEnumFactory().fromType(castToCode(value)); 2061 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 2062 } else if (name.equals("type")) { 2063 this.type = castToCodeableConcept(value); // CodeableConcept 2064 } else if (name.equals("lotNumber")) { 2065 this.lotNumber = castToString(value); // StringType 2066 } else if (name.equals("manufacturer")) { 2067 this.manufacturer = castToString(value); // StringType 2068 } else if (name.equals("manufactureDate")) { 2069 this.manufactureDate = castToDateTime(value); // DateTimeType 2070 } else if (name.equals("expirationDate")) { 2071 this.expirationDate = castToDateTime(value); // DateTimeType 2072 } else if (name.equals("model")) { 2073 this.model = castToString(value); // StringType 2074 } else if (name.equals("version")) { 2075 this.version = castToString(value); // StringType 2076 } else if (name.equals("patient")) { 2077 this.patient = castToReference(value); // Reference 2078 } else if (name.equals("owner")) { 2079 this.owner = castToReference(value); // Reference 2080 } else if (name.equals("contact")) { 2081 this.getContact().add(castToContactPoint(value)); 2082 } else if (name.equals("location")) { 2083 this.location = castToReference(value); // Reference 2084 } else if (name.equals("url")) { 2085 this.url = castToUri(value); // UriType 2086 } else if (name.equals("note")) { 2087 this.getNote().add(castToAnnotation(value)); 2088 } else if (name.equals("safety")) { 2089 this.getSafety().add(castToCodeableConcept(value)); 2090 } else 2091 return super.setProperty(name, value); 2092 return value; 2093 } 2094 2095 @Override 2096 public Base makeProperty(int hash, String name) throws FHIRException { 2097 switch (hash) { 2098 case -1618432855: return addIdentifier(); 2099 case 115642: return getUdi(); 2100 case -892481550: return getStatusElement(); 2101 case 3575610: return getType(); 2102 case 462547450: return getLotNumberElement(); 2103 case -1969347631: return getManufacturerElement(); 2104 case 416714767: return getManufactureDateElement(); 2105 case -668811523: return getExpirationDateElement(); 2106 case 104069929: return getModelElement(); 2107 case 351608024: return getVersionElement(); 2108 case -791418107: return getPatient(); 2109 case 106164915: return getOwner(); 2110 case 951526432: return addContact(); 2111 case 1901043637: return getLocation(); 2112 case 116079: return getUrlElement(); 2113 case 3387378: return addNote(); 2114 case -909893934: return addSafety(); 2115 default: return super.makeProperty(hash, name); 2116 } 2117 2118 } 2119 2120 @Override 2121 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2122 switch (hash) { 2123 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2124 case 115642: /*udi*/ return new String[] {}; 2125 case -892481550: /*status*/ return new String[] {"code"}; 2126 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2127 case 462547450: /*lotNumber*/ return new String[] {"string"}; 2128 case -1969347631: /*manufacturer*/ return new String[] {"string"}; 2129 case 416714767: /*manufactureDate*/ return new String[] {"dateTime"}; 2130 case -668811523: /*expirationDate*/ return new String[] {"dateTime"}; 2131 case 104069929: /*model*/ return new String[] {"string"}; 2132 case 351608024: /*version*/ return new String[] {"string"}; 2133 case -791418107: /*patient*/ return new String[] {"Reference"}; 2134 case 106164915: /*owner*/ return new String[] {"Reference"}; 2135 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 2136 case 1901043637: /*location*/ return new String[] {"Reference"}; 2137 case 116079: /*url*/ return new String[] {"uri"}; 2138 case 3387378: /*note*/ return new String[] {"Annotation"}; 2139 case -909893934: /*safety*/ return new String[] {"CodeableConcept"}; 2140 default: return super.getTypesForProperty(hash, name); 2141 } 2142 2143 } 2144 2145 @Override 2146 public Base addChild(String name) throws FHIRException { 2147 if (name.equals("identifier")) { 2148 return addIdentifier(); 2149 } 2150 else if (name.equals("udi")) { 2151 this.udi = new DeviceUdiComponent(); 2152 return this.udi; 2153 } 2154 else if (name.equals("status")) { 2155 throw new FHIRException("Cannot call addChild on a singleton property Device.status"); 2156 } 2157 else if (name.equals("type")) { 2158 this.type = new CodeableConcept(); 2159 return this.type; 2160 } 2161 else if (name.equals("lotNumber")) { 2162 throw new FHIRException("Cannot call addChild on a singleton property Device.lotNumber"); 2163 } 2164 else if (name.equals("manufacturer")) { 2165 throw new FHIRException("Cannot call addChild on a singleton property Device.manufacturer"); 2166 } 2167 else if (name.equals("manufactureDate")) { 2168 throw new FHIRException("Cannot call addChild on a singleton property Device.manufactureDate"); 2169 } 2170 else if (name.equals("expirationDate")) { 2171 throw new FHIRException("Cannot call addChild on a singleton property Device.expirationDate"); 2172 } 2173 else if (name.equals("model")) { 2174 throw new FHIRException("Cannot call addChild on a singleton property Device.model"); 2175 } 2176 else if (name.equals("version")) { 2177 throw new FHIRException("Cannot call addChild on a singleton property Device.version"); 2178 } 2179 else if (name.equals("patient")) { 2180 this.patient = new Reference(); 2181 return this.patient; 2182 } 2183 else if (name.equals("owner")) { 2184 this.owner = new Reference(); 2185 return this.owner; 2186 } 2187 else if (name.equals("contact")) { 2188 return addContact(); 2189 } 2190 else if (name.equals("location")) { 2191 this.location = new Reference(); 2192 return this.location; 2193 } 2194 else if (name.equals("url")) { 2195 throw new FHIRException("Cannot call addChild on a singleton property Device.url"); 2196 } 2197 else if (name.equals("note")) { 2198 return addNote(); 2199 } 2200 else if (name.equals("safety")) { 2201 return addSafety(); 2202 } 2203 else 2204 return super.addChild(name); 2205 } 2206 2207 public String fhirType() { 2208 return "Device"; 2209 2210 } 2211 2212 public Device copy() { 2213 Device dst = new Device(); 2214 copyValues(dst); 2215 if (identifier != null) { 2216 dst.identifier = new ArrayList<Identifier>(); 2217 for (Identifier i : identifier) 2218 dst.identifier.add(i.copy()); 2219 }; 2220 dst.udi = udi == null ? null : udi.copy(); 2221 dst.status = status == null ? null : status.copy(); 2222 dst.type = type == null ? null : type.copy(); 2223 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 2224 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 2225 dst.manufactureDate = manufactureDate == null ? null : manufactureDate.copy(); 2226 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 2227 dst.model = model == null ? null : model.copy(); 2228 dst.version = version == null ? null : version.copy(); 2229 dst.patient = patient == null ? null : patient.copy(); 2230 dst.owner = owner == null ? null : owner.copy(); 2231 if (contact != null) { 2232 dst.contact = new ArrayList<ContactPoint>(); 2233 for (ContactPoint i : contact) 2234 dst.contact.add(i.copy()); 2235 }; 2236 dst.location = location == null ? null : location.copy(); 2237 dst.url = url == null ? null : url.copy(); 2238 if (note != null) { 2239 dst.note = new ArrayList<Annotation>(); 2240 for (Annotation i : note) 2241 dst.note.add(i.copy()); 2242 }; 2243 if (safety != null) { 2244 dst.safety = new ArrayList<CodeableConcept>(); 2245 for (CodeableConcept i : safety) 2246 dst.safety.add(i.copy()); 2247 }; 2248 return dst; 2249 } 2250 2251 protected Device typedCopy() { 2252 return copy(); 2253 } 2254 2255 @Override 2256 public boolean equalsDeep(Base other_) { 2257 if (!super.equalsDeep(other_)) 2258 return false; 2259 if (!(other_ instanceof Device)) 2260 return false; 2261 Device o = (Device) other_; 2262 return compareDeep(identifier, o.identifier, true) && compareDeep(udi, o.udi, true) && compareDeep(status, o.status, true) 2263 && compareDeep(type, o.type, true) && compareDeep(lotNumber, o.lotNumber, true) && compareDeep(manufacturer, o.manufacturer, true) 2264 && compareDeep(manufactureDate, o.manufactureDate, true) && compareDeep(expirationDate, o.expirationDate, true) 2265 && compareDeep(model, o.model, true) && compareDeep(version, o.version, true) && compareDeep(patient, o.patient, true) 2266 && compareDeep(owner, o.owner, true) && compareDeep(contact, o.contact, true) && compareDeep(location, o.location, true) 2267 && compareDeep(url, o.url, true) && compareDeep(note, o.note, true) && compareDeep(safety, o.safety, true) 2268 ; 2269 } 2270 2271 @Override 2272 public boolean equalsShallow(Base other_) { 2273 if (!super.equalsShallow(other_)) 2274 return false; 2275 if (!(other_ instanceof Device)) 2276 return false; 2277 Device o = (Device) other_; 2278 return compareValues(status, o.status, true) && compareValues(lotNumber, o.lotNumber, true) && compareValues(manufacturer, o.manufacturer, true) 2279 && compareValues(manufactureDate, o.manufactureDate, true) && compareValues(expirationDate, o.expirationDate, true) 2280 && compareValues(model, o.model, true) && compareValues(version, o.version, true) && compareValues(url, o.url, true) 2281 ; 2282 } 2283 2284 public boolean isEmpty() { 2285 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, udi, status 2286 , type, lotNumber, manufacturer, manufactureDate, expirationDate, model, version 2287 , patient, owner, contact, location, url, note, safety); 2288 } 2289 2290 @Override 2291 public ResourceType getResourceType() { 2292 return ResourceType.Device; 2293 } 2294 2295 /** 2296 * Search parameter: <b>udi-di</b> 2297 * <p> 2298 * Description: <b>The udi Device Identifier (DI)</b><br> 2299 * Type: <b>string</b><br> 2300 * Path: <b>Device.udi.deviceIdentifier</b><br> 2301 * </p> 2302 */ 2303 @SearchParamDefinition(name="udi-di", path="Device.udi.deviceIdentifier", description="The udi Device Identifier (DI)", type="string" ) 2304 public static final String SP_UDI_DI = "udi-di"; 2305 /** 2306 * <b>Fluent Client</b> search parameter constant for <b>udi-di</b> 2307 * <p> 2308 * Description: <b>The udi Device Identifier (DI)</b><br> 2309 * Type: <b>string</b><br> 2310 * Path: <b>Device.udi.deviceIdentifier</b><br> 2311 * </p> 2312 */ 2313 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_DI = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_UDI_DI); 2314 2315 /** 2316 * Search parameter: <b>identifier</b> 2317 * <p> 2318 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 2319 * Type: <b>token</b><br> 2320 * Path: <b>Device.identifier</b><br> 2321 * </p> 2322 */ 2323 @SearchParamDefinition(name="identifier", path="Device.identifier", description="Instance id from manufacturer, owner, and others", type="token" ) 2324 public static final String SP_IDENTIFIER = "identifier"; 2325 /** 2326 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2327 * <p> 2328 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 2329 * Type: <b>token</b><br> 2330 * Path: <b>Device.identifier</b><br> 2331 * </p> 2332 */ 2333 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2334 2335 /** 2336 * Search parameter: <b>udi-carrier</b> 2337 * <p> 2338 * Description: <b>UDI Barcode (RFID or other technology) string either in HRF format or AIDC format converted to base64 string.</b><br> 2339 * Type: <b>string</b><br> 2340 * Path: <b>Device.udi.carrierHRF, Device.udi.carrierAIDC</b><br> 2341 * </p> 2342 */ 2343 @SearchParamDefinition(name="udi-carrier", path="Device.udi.carrierHRF | Device.udi.carrierAIDC", description="UDI Barcode (RFID or other technology) string either in HRF format or AIDC format converted to base64 string.", type="string" ) 2344 public static final String SP_UDI_CARRIER = "udi-carrier"; 2345 /** 2346 * <b>Fluent Client</b> search parameter constant for <b>udi-carrier</b> 2347 * <p> 2348 * Description: <b>UDI Barcode (RFID or other technology) string either in HRF format or AIDC format converted to base64 string.</b><br> 2349 * Type: <b>string</b><br> 2350 * Path: <b>Device.udi.carrierHRF, Device.udi.carrierAIDC</b><br> 2351 * </p> 2352 */ 2353 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_CARRIER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_UDI_CARRIER); 2354 2355 /** 2356 * Search parameter: <b>device-name</b> 2357 * <p> 2358 * Description: <b>A server defined search that may match any of the string fields in the Device.udi.name or Device.type.coding.display or Device.type.text</b><br> 2359 * Type: <b>string</b><br> 2360 * Path: <b>Device.udi.name, Device.type.text, Device.type.coding.display</b><br> 2361 * </p> 2362 */ 2363 @SearchParamDefinition(name="device-name", path="Device.udi.name | Device.type.text | Device.type.coding.display", description="A server defined search that may match any of the string fields in the Device.udi.name or Device.type.coding.display or Device.type.text", type="string" ) 2364 public static final String SP_DEVICE_NAME = "device-name"; 2365 /** 2366 * <b>Fluent Client</b> search parameter constant for <b>device-name</b> 2367 * <p> 2368 * Description: <b>A server defined search that may match any of the string fields in the Device.udi.name or Device.type.coding.display or Device.type.text</b><br> 2369 * Type: <b>string</b><br> 2370 * Path: <b>Device.udi.name, Device.type.text, Device.type.coding.display</b><br> 2371 * </p> 2372 */ 2373 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEVICE_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DEVICE_NAME); 2374 2375 /** 2376 * Search parameter: <b>patient</b> 2377 * <p> 2378 * Description: <b>Patient information, if the resource is affixed to a person</b><br> 2379 * Type: <b>reference</b><br> 2380 * Path: <b>Device.patient</b><br> 2381 * </p> 2382 */ 2383 @SearchParamDefinition(name="patient", path="Device.patient", description="Patient information, if the resource is affixed to a person", type="reference", target={Patient.class } ) 2384 public static final String SP_PATIENT = "patient"; 2385 /** 2386 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2387 * <p> 2388 * Description: <b>Patient information, if the resource is affixed to a person</b><br> 2389 * Type: <b>reference</b><br> 2390 * Path: <b>Device.patient</b><br> 2391 * </p> 2392 */ 2393 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2394 2395/** 2396 * Constant for fluent queries to be used to add include statements. Specifies 2397 * the path value of "<b>Device:patient</b>". 2398 */ 2399 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Device:patient").toLocked(); 2400 2401 /** 2402 * Search parameter: <b>organization</b> 2403 * <p> 2404 * Description: <b>The organization responsible for the device</b><br> 2405 * Type: <b>reference</b><br> 2406 * Path: <b>Device.owner</b><br> 2407 * </p> 2408 */ 2409 @SearchParamDefinition(name="organization", path="Device.owner", description="The organization responsible for the device", type="reference", target={Organization.class } ) 2410 public static final String SP_ORGANIZATION = "organization"; 2411 /** 2412 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2413 * <p> 2414 * Description: <b>The organization responsible for the device</b><br> 2415 * Type: <b>reference</b><br> 2416 * Path: <b>Device.owner</b><br> 2417 * </p> 2418 */ 2419 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2420 2421/** 2422 * Constant for fluent queries to be used to add include statements. Specifies 2423 * the path value of "<b>Device:organization</b>". 2424 */ 2425 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Device:organization").toLocked(); 2426 2427 /** 2428 * Search parameter: <b>model</b> 2429 * <p> 2430 * Description: <b>The model of the device</b><br> 2431 * Type: <b>string</b><br> 2432 * Path: <b>Device.model</b><br> 2433 * </p> 2434 */ 2435 @SearchParamDefinition(name="model", path="Device.model", description="The model of the device", type="string" ) 2436 public static final String SP_MODEL = "model"; 2437 /** 2438 * <b>Fluent Client</b> search parameter constant for <b>model</b> 2439 * <p> 2440 * Description: <b>The model of the device</b><br> 2441 * Type: <b>string</b><br> 2442 * Path: <b>Device.model</b><br> 2443 * </p> 2444 */ 2445 public static final ca.uhn.fhir.rest.gclient.StringClientParam MODEL = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_MODEL); 2446 2447 /** 2448 * Search parameter: <b>location</b> 2449 * <p> 2450 * Description: <b>A location, where the resource is found</b><br> 2451 * Type: <b>reference</b><br> 2452 * Path: <b>Device.location</b><br> 2453 * </p> 2454 */ 2455 @SearchParamDefinition(name="location", path="Device.location", description="A location, where the resource is found", type="reference", target={Location.class } ) 2456 public static final String SP_LOCATION = "location"; 2457 /** 2458 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2459 * <p> 2460 * Description: <b>A location, where the resource is found</b><br> 2461 * Type: <b>reference</b><br> 2462 * Path: <b>Device.location</b><br> 2463 * </p> 2464 */ 2465 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2466 2467/** 2468 * Constant for fluent queries to be used to add include statements. Specifies 2469 * the path value of "<b>Device:location</b>". 2470 */ 2471 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Device:location").toLocked(); 2472 2473 /** 2474 * Search parameter: <b>type</b> 2475 * <p> 2476 * Description: <b>The type of the device</b><br> 2477 * Type: <b>token</b><br> 2478 * Path: <b>Device.type</b><br> 2479 * </p> 2480 */ 2481 @SearchParamDefinition(name="type", path="Device.type", description="The type of the device", type="token" ) 2482 public static final String SP_TYPE = "type"; 2483 /** 2484 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2485 * <p> 2486 * Description: <b>The type of the device</b><br> 2487 * Type: <b>token</b><br> 2488 * Path: <b>Device.type</b><br> 2489 * </p> 2490 */ 2491 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2492 2493 /** 2494 * Search parameter: <b>url</b> 2495 * <p> 2496 * Description: <b>Network address to contact device</b><br> 2497 * Type: <b>uri</b><br> 2498 * Path: <b>Device.url</b><br> 2499 * </p> 2500 */ 2501 @SearchParamDefinition(name="url", path="Device.url", description="Network address to contact device", type="uri" ) 2502 public static final String SP_URL = "url"; 2503 /** 2504 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2505 * <p> 2506 * Description: <b>Network address to contact device</b><br> 2507 * Type: <b>uri</b><br> 2508 * Path: <b>Device.url</b><br> 2509 * </p> 2510 */ 2511 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2512 2513 /** 2514 * Search parameter: <b>manufacturer</b> 2515 * <p> 2516 * Description: <b>The manufacturer of the device</b><br> 2517 * Type: <b>string</b><br> 2518 * Path: <b>Device.manufacturer</b><br> 2519 * </p> 2520 */ 2521 @SearchParamDefinition(name="manufacturer", path="Device.manufacturer", description="The manufacturer of the device", type="string" ) 2522 public static final String SP_MANUFACTURER = "manufacturer"; 2523 /** 2524 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 2525 * <p> 2526 * Description: <b>The manufacturer of the device</b><br> 2527 * Type: <b>string</b><br> 2528 * Path: <b>Device.manufacturer</b><br> 2529 * </p> 2530 */ 2531 public static final ca.uhn.fhir.rest.gclient.StringClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_MANUFACTURER); 2532 2533 /** 2534 * Search parameter: <b>status</b> 2535 * <p> 2536 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 2537 * Type: <b>token</b><br> 2538 * Path: <b>Device.status</b><br> 2539 * </p> 2540 */ 2541 @SearchParamDefinition(name="status", path="Device.status", description="active | inactive | entered-in-error | unknown", type="token" ) 2542 public static final String SP_STATUS = "status"; 2543 /** 2544 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2545 * <p> 2546 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 2547 * Type: <b>token</b><br> 2548 * Path: <b>Device.status</b><br> 2549 * </p> 2550 */ 2551 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2552 2553 2554}