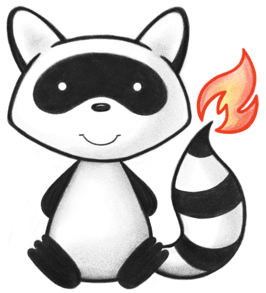
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 050 */ 051@ResourceDef(name="DeviceComponent", profile="http://hl7.org/fhir/Profile/DeviceComponent") 052public class DeviceComponent extends DomainResource { 053 054 public enum MeasmntPrinciple { 055 /** 056 * Measurement principle isn't in the list. 057 */ 058 OTHER, 059 /** 060 * Measurement is done using the chemical principle. 061 */ 062 CHEMICAL, 063 /** 064 * Measurement is done using the electrical principle. 065 */ 066 ELECTRICAL, 067 /** 068 * Measurement is done using the impedance principle. 069 */ 070 IMPEDANCE, 071 /** 072 * Measurement is done using the nuclear principle. 073 */ 074 NUCLEAR, 075 /** 076 * Measurement is done using the optical principle. 077 */ 078 OPTICAL, 079 /** 080 * Measurement is done using the thermal principle. 081 */ 082 THERMAL, 083 /** 084 * Measurement is done using the biological principle. 085 */ 086 BIOLOGICAL, 087 /** 088 * Measurement is done using the mechanical principle. 089 */ 090 MECHANICAL, 091 /** 092 * Measurement is done using the acoustical principle. 093 */ 094 ACOUSTICAL, 095 /** 096 * Measurement is done using the manual principle. 097 */ 098 MANUAL, 099 /** 100 * added to help the parsers with the generic types 101 */ 102 NULL; 103 public static MeasmntPrinciple fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("other".equals(codeString)) 107 return OTHER; 108 if ("chemical".equals(codeString)) 109 return CHEMICAL; 110 if ("electrical".equals(codeString)) 111 return ELECTRICAL; 112 if ("impedance".equals(codeString)) 113 return IMPEDANCE; 114 if ("nuclear".equals(codeString)) 115 return NUCLEAR; 116 if ("optical".equals(codeString)) 117 return OPTICAL; 118 if ("thermal".equals(codeString)) 119 return THERMAL; 120 if ("biological".equals(codeString)) 121 return BIOLOGICAL; 122 if ("mechanical".equals(codeString)) 123 return MECHANICAL; 124 if ("acoustical".equals(codeString)) 125 return ACOUSTICAL; 126 if ("manual".equals(codeString)) 127 return MANUAL; 128 if (Configuration.isAcceptInvalidEnums()) 129 return null; 130 else 131 throw new FHIRException("Unknown MeasmntPrinciple code '"+codeString+"'"); 132 } 133 public String toCode() { 134 switch (this) { 135 case OTHER: return "other"; 136 case CHEMICAL: return "chemical"; 137 case ELECTRICAL: return "electrical"; 138 case IMPEDANCE: return "impedance"; 139 case NUCLEAR: return "nuclear"; 140 case OPTICAL: return "optical"; 141 case THERMAL: return "thermal"; 142 case BIOLOGICAL: return "biological"; 143 case MECHANICAL: return "mechanical"; 144 case ACOUSTICAL: return "acoustical"; 145 case MANUAL: return "manual"; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getSystem() { 151 switch (this) { 152 case OTHER: return "http://hl7.org/fhir/measurement-principle"; 153 case CHEMICAL: return "http://hl7.org/fhir/measurement-principle"; 154 case ELECTRICAL: return "http://hl7.org/fhir/measurement-principle"; 155 case IMPEDANCE: return "http://hl7.org/fhir/measurement-principle"; 156 case NUCLEAR: return "http://hl7.org/fhir/measurement-principle"; 157 case OPTICAL: return "http://hl7.org/fhir/measurement-principle"; 158 case THERMAL: return "http://hl7.org/fhir/measurement-principle"; 159 case BIOLOGICAL: return "http://hl7.org/fhir/measurement-principle"; 160 case MECHANICAL: return "http://hl7.org/fhir/measurement-principle"; 161 case ACOUSTICAL: return "http://hl7.org/fhir/measurement-principle"; 162 case MANUAL: return "http://hl7.org/fhir/measurement-principle"; 163 case NULL: return null; 164 default: return "?"; 165 } 166 } 167 public String getDefinition() { 168 switch (this) { 169 case OTHER: return "Measurement principle isn't in the list."; 170 case CHEMICAL: return "Measurement is done using the chemical principle."; 171 case ELECTRICAL: return "Measurement is done using the electrical principle."; 172 case IMPEDANCE: return "Measurement is done using the impedance principle."; 173 case NUCLEAR: return "Measurement is done using the nuclear principle."; 174 case OPTICAL: return "Measurement is done using the optical principle."; 175 case THERMAL: return "Measurement is done using the thermal principle."; 176 case BIOLOGICAL: return "Measurement is done using the biological principle."; 177 case MECHANICAL: return "Measurement is done using the mechanical principle."; 178 case ACOUSTICAL: return "Measurement is done using the acoustical principle."; 179 case MANUAL: return "Measurement is done using the manual principle."; 180 case NULL: return null; 181 default: return "?"; 182 } 183 } 184 public String getDisplay() { 185 switch (this) { 186 case OTHER: return "MSP Other"; 187 case CHEMICAL: return "MSP Chemical"; 188 case ELECTRICAL: return "MSP Electrical"; 189 case IMPEDANCE: return "MSP Impedance"; 190 case NUCLEAR: return "MSP Nuclear"; 191 case OPTICAL: return "MSP Optical"; 192 case THERMAL: return "MSP Thermal"; 193 case BIOLOGICAL: return "MSP Biological"; 194 case MECHANICAL: return "MSP Mechanical"; 195 case ACOUSTICAL: return "MSP Acoustical"; 196 case MANUAL: return "MSP Manual"; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 } 202 203 public static class MeasmntPrincipleEnumFactory implements EnumFactory<MeasmntPrinciple> { 204 public MeasmntPrinciple fromCode(String codeString) throws IllegalArgumentException { 205 if (codeString == null || "".equals(codeString)) 206 if (codeString == null || "".equals(codeString)) 207 return null; 208 if ("other".equals(codeString)) 209 return MeasmntPrinciple.OTHER; 210 if ("chemical".equals(codeString)) 211 return MeasmntPrinciple.CHEMICAL; 212 if ("electrical".equals(codeString)) 213 return MeasmntPrinciple.ELECTRICAL; 214 if ("impedance".equals(codeString)) 215 return MeasmntPrinciple.IMPEDANCE; 216 if ("nuclear".equals(codeString)) 217 return MeasmntPrinciple.NUCLEAR; 218 if ("optical".equals(codeString)) 219 return MeasmntPrinciple.OPTICAL; 220 if ("thermal".equals(codeString)) 221 return MeasmntPrinciple.THERMAL; 222 if ("biological".equals(codeString)) 223 return MeasmntPrinciple.BIOLOGICAL; 224 if ("mechanical".equals(codeString)) 225 return MeasmntPrinciple.MECHANICAL; 226 if ("acoustical".equals(codeString)) 227 return MeasmntPrinciple.ACOUSTICAL; 228 if ("manual".equals(codeString)) 229 return MeasmntPrinciple.MANUAL; 230 throw new IllegalArgumentException("Unknown MeasmntPrinciple code '"+codeString+"'"); 231 } 232 public Enumeration<MeasmntPrinciple> fromType(PrimitiveType<?> code) throws FHIRException { 233 if (code == null) 234 return null; 235 if (code.isEmpty()) 236 return new Enumeration<MeasmntPrinciple>(this); 237 String codeString = code.asStringValue(); 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("other".equals(codeString)) 241 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.OTHER); 242 if ("chemical".equals(codeString)) 243 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.CHEMICAL); 244 if ("electrical".equals(codeString)) 245 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.ELECTRICAL); 246 if ("impedance".equals(codeString)) 247 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.IMPEDANCE); 248 if ("nuclear".equals(codeString)) 249 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.NUCLEAR); 250 if ("optical".equals(codeString)) 251 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.OPTICAL); 252 if ("thermal".equals(codeString)) 253 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.THERMAL); 254 if ("biological".equals(codeString)) 255 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.BIOLOGICAL); 256 if ("mechanical".equals(codeString)) 257 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.MECHANICAL); 258 if ("acoustical".equals(codeString)) 259 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.ACOUSTICAL); 260 if ("manual".equals(codeString)) 261 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.MANUAL); 262 throw new FHIRException("Unknown MeasmntPrinciple code '"+codeString+"'"); 263 } 264 public String toCode(MeasmntPrinciple code) { 265 if (code == MeasmntPrinciple.NULL) 266 return null; 267 if (code == MeasmntPrinciple.OTHER) 268 return "other"; 269 if (code == MeasmntPrinciple.CHEMICAL) 270 return "chemical"; 271 if (code == MeasmntPrinciple.ELECTRICAL) 272 return "electrical"; 273 if (code == MeasmntPrinciple.IMPEDANCE) 274 return "impedance"; 275 if (code == MeasmntPrinciple.NUCLEAR) 276 return "nuclear"; 277 if (code == MeasmntPrinciple.OPTICAL) 278 return "optical"; 279 if (code == MeasmntPrinciple.THERMAL) 280 return "thermal"; 281 if (code == MeasmntPrinciple.BIOLOGICAL) 282 return "biological"; 283 if (code == MeasmntPrinciple.MECHANICAL) 284 return "mechanical"; 285 if (code == MeasmntPrinciple.ACOUSTICAL) 286 return "acoustical"; 287 if (code == MeasmntPrinciple.MANUAL) 288 return "manual"; 289 return "?"; 290 } 291 public String toSystem(MeasmntPrinciple code) { 292 return code.getSystem(); 293 } 294 } 295 296 @Block() 297 public static class DeviceComponentProductionSpecificationComponent extends BackboneElement implements IBaseBackboneElement { 298 /** 299 * The specification type, such as, serial number, part number, hardware revision, software revision, etc. 300 */ 301 @Child(name = "specType", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 302 @Description(shortDefinition="Type or kind of production specification, for example serial number or software revision", formalDefinition="The specification type, such as, serial number, part number, hardware revision, software revision, etc." ) 303 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specification-type") 304 protected CodeableConcept specType; 305 306 /** 307 * The internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacturer can make use of. 308 */ 309 @Child(name = "componentId", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=true) 310 @Description(shortDefinition="Internal component unique identification", formalDefinition="The internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacturer can make use of." ) 311 protected Identifier componentId; 312 313 /** 314 * The printable string defining the component. 315 */ 316 @Child(name = "productionSpec", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 317 @Description(shortDefinition="A printable string defining the component", formalDefinition="The printable string defining the component." ) 318 protected StringType productionSpec; 319 320 private static final long serialVersionUID = -1476597516L; 321 322 /** 323 * Constructor 324 */ 325 public DeviceComponentProductionSpecificationComponent() { 326 super(); 327 } 328 329 /** 330 * @return {@link #specType} (The specification type, such as, serial number, part number, hardware revision, software revision, etc.) 331 */ 332 public CodeableConcept getSpecType() { 333 if (this.specType == null) 334 if (Configuration.errorOnAutoCreate()) 335 throw new Error("Attempt to auto-create DeviceComponentProductionSpecificationComponent.specType"); 336 else if (Configuration.doAutoCreate()) 337 this.specType = new CodeableConcept(); // cc 338 return this.specType; 339 } 340 341 public boolean hasSpecType() { 342 return this.specType != null && !this.specType.isEmpty(); 343 } 344 345 /** 346 * @param value {@link #specType} (The specification type, such as, serial number, part number, hardware revision, software revision, etc.) 347 */ 348 public DeviceComponentProductionSpecificationComponent setSpecType(CodeableConcept value) { 349 this.specType = value; 350 return this; 351 } 352 353 /** 354 * @return {@link #componentId} (The internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacturer can make use of.) 355 */ 356 public Identifier getComponentId() { 357 if (this.componentId == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create DeviceComponentProductionSpecificationComponent.componentId"); 360 else if (Configuration.doAutoCreate()) 361 this.componentId = new Identifier(); // cc 362 return this.componentId; 363 } 364 365 public boolean hasComponentId() { 366 return this.componentId != null && !this.componentId.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #componentId} (The internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacturer can make use of.) 371 */ 372 public DeviceComponentProductionSpecificationComponent setComponentId(Identifier value) { 373 this.componentId = value; 374 return this; 375 } 376 377 /** 378 * @return {@link #productionSpec} (The printable string defining the component.). This is the underlying object with id, value and extensions. The accessor "getProductionSpec" gives direct access to the value 379 */ 380 public StringType getProductionSpecElement() { 381 if (this.productionSpec == null) 382 if (Configuration.errorOnAutoCreate()) 383 throw new Error("Attempt to auto-create DeviceComponentProductionSpecificationComponent.productionSpec"); 384 else if (Configuration.doAutoCreate()) 385 this.productionSpec = new StringType(); // bb 386 return this.productionSpec; 387 } 388 389 public boolean hasProductionSpecElement() { 390 return this.productionSpec != null && !this.productionSpec.isEmpty(); 391 } 392 393 public boolean hasProductionSpec() { 394 return this.productionSpec != null && !this.productionSpec.isEmpty(); 395 } 396 397 /** 398 * @param value {@link #productionSpec} (The printable string defining the component.). This is the underlying object with id, value and extensions. The accessor "getProductionSpec" gives direct access to the value 399 */ 400 public DeviceComponentProductionSpecificationComponent setProductionSpecElement(StringType value) { 401 this.productionSpec = value; 402 return this; 403 } 404 405 /** 406 * @return The printable string defining the component. 407 */ 408 public String getProductionSpec() { 409 return this.productionSpec == null ? null : this.productionSpec.getValue(); 410 } 411 412 /** 413 * @param value The printable string defining the component. 414 */ 415 public DeviceComponentProductionSpecificationComponent setProductionSpec(String value) { 416 if (Utilities.noString(value)) 417 this.productionSpec = null; 418 else { 419 if (this.productionSpec == null) 420 this.productionSpec = new StringType(); 421 this.productionSpec.setValue(value); 422 } 423 return this; 424 } 425 426 protected void listChildren(List<Property> children) { 427 super.listChildren(children); 428 children.add(new Property("specType", "CodeableConcept", "The specification type, such as, serial number, part number, hardware revision, software revision, etc.", 0, 1, specType)); 429 children.add(new Property("componentId", "Identifier", "The internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacturer can make use of.", 0, 1, componentId)); 430 children.add(new Property("productionSpec", "string", "The printable string defining the component.", 0, 1, productionSpec)); 431 } 432 433 @Override 434 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 435 switch (_hash) { 436 case -2133482091: /*specType*/ return new Property("specType", "CodeableConcept", "The specification type, such as, serial number, part number, hardware revision, software revision, etc.", 0, 1, specType); 437 case -985933064: /*componentId*/ return new Property("componentId", "Identifier", "The internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacturer can make use of.", 0, 1, componentId); 438 case 182147092: /*productionSpec*/ return new Property("productionSpec", "string", "The printable string defining the component.", 0, 1, productionSpec); 439 default: return super.getNamedProperty(_hash, _name, _checkValid); 440 } 441 442 } 443 444 @Override 445 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 446 switch (hash) { 447 case -2133482091: /*specType*/ return this.specType == null ? new Base[0] : new Base[] {this.specType}; // CodeableConcept 448 case -985933064: /*componentId*/ return this.componentId == null ? new Base[0] : new Base[] {this.componentId}; // Identifier 449 case 182147092: /*productionSpec*/ return this.productionSpec == null ? new Base[0] : new Base[] {this.productionSpec}; // StringType 450 default: return super.getProperty(hash, name, checkValid); 451 } 452 453 } 454 455 @Override 456 public Base setProperty(int hash, String name, Base value) throws FHIRException { 457 switch (hash) { 458 case -2133482091: // specType 459 this.specType = castToCodeableConcept(value); // CodeableConcept 460 return value; 461 case -985933064: // componentId 462 this.componentId = castToIdentifier(value); // Identifier 463 return value; 464 case 182147092: // productionSpec 465 this.productionSpec = castToString(value); // StringType 466 return value; 467 default: return super.setProperty(hash, name, value); 468 } 469 470 } 471 472 @Override 473 public Base setProperty(String name, Base value) throws FHIRException { 474 if (name.equals("specType")) { 475 this.specType = castToCodeableConcept(value); // CodeableConcept 476 } else if (name.equals("componentId")) { 477 this.componentId = castToIdentifier(value); // Identifier 478 } else if (name.equals("productionSpec")) { 479 this.productionSpec = castToString(value); // StringType 480 } else 481 return super.setProperty(name, value); 482 return value; 483 } 484 485 @Override 486 public Base makeProperty(int hash, String name) throws FHIRException { 487 switch (hash) { 488 case -2133482091: return getSpecType(); 489 case -985933064: return getComponentId(); 490 case 182147092: return getProductionSpecElement(); 491 default: return super.makeProperty(hash, name); 492 } 493 494 } 495 496 @Override 497 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 498 switch (hash) { 499 case -2133482091: /*specType*/ return new String[] {"CodeableConcept"}; 500 case -985933064: /*componentId*/ return new String[] {"Identifier"}; 501 case 182147092: /*productionSpec*/ return new String[] {"string"}; 502 default: return super.getTypesForProperty(hash, name); 503 } 504 505 } 506 507 @Override 508 public Base addChild(String name) throws FHIRException { 509 if (name.equals("specType")) { 510 this.specType = new CodeableConcept(); 511 return this.specType; 512 } 513 else if (name.equals("componentId")) { 514 this.componentId = new Identifier(); 515 return this.componentId; 516 } 517 else if (name.equals("productionSpec")) { 518 throw new FHIRException("Cannot call addChild on a singleton property DeviceComponent.productionSpec"); 519 } 520 else 521 return super.addChild(name); 522 } 523 524 public DeviceComponentProductionSpecificationComponent copy() { 525 DeviceComponentProductionSpecificationComponent dst = new DeviceComponentProductionSpecificationComponent(); 526 copyValues(dst); 527 dst.specType = specType == null ? null : specType.copy(); 528 dst.componentId = componentId == null ? null : componentId.copy(); 529 dst.productionSpec = productionSpec == null ? null : productionSpec.copy(); 530 return dst; 531 } 532 533 @Override 534 public boolean equalsDeep(Base other_) { 535 if (!super.equalsDeep(other_)) 536 return false; 537 if (!(other_ instanceof DeviceComponentProductionSpecificationComponent)) 538 return false; 539 DeviceComponentProductionSpecificationComponent o = (DeviceComponentProductionSpecificationComponent) other_; 540 return compareDeep(specType, o.specType, true) && compareDeep(componentId, o.componentId, true) 541 && compareDeep(productionSpec, o.productionSpec, true); 542 } 543 544 @Override 545 public boolean equalsShallow(Base other_) { 546 if (!super.equalsShallow(other_)) 547 return false; 548 if (!(other_ instanceof DeviceComponentProductionSpecificationComponent)) 549 return false; 550 DeviceComponentProductionSpecificationComponent o = (DeviceComponentProductionSpecificationComponent) other_; 551 return compareValues(productionSpec, o.productionSpec, true); 552 } 553 554 public boolean isEmpty() { 555 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(specType, componentId, productionSpec 556 ); 557 } 558 559 public String fhirType() { 560 return "DeviceComponent.productionSpecification"; 561 562 } 563 564 } 565 566 /** 567 * The locally assigned unique identification by the software. For example: handle ID. 568 */ 569 @Child(name = "identifier", type = {Identifier.class}, order=0, min=1, max=1, modifier=false, summary=true) 570 @Description(shortDefinition="Instance id assigned by the software stack", formalDefinition="The locally assigned unique identification by the software. For example: handle ID." ) 571 protected Identifier identifier; 572 573 /** 574 * The component type as defined in the object-oriented or metric nomenclature partition. 575 */ 576 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 577 @Description(shortDefinition="What kind of component it is", formalDefinition="The component type as defined in the object-oriented or metric nomenclature partition." ) 578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-kind") 579 protected CodeableConcept type; 580 581 /** 582 * The timestamp for the most recent system change which includes device configuration or setting change. 583 */ 584 @Child(name = "lastSystemChange", type = {InstantType.class}, order=2, min=0, max=1, modifier=false, summary=true) 585 @Description(shortDefinition="Recent system change timestamp", formalDefinition="The timestamp for the most recent system change which includes device configuration or setting change." ) 586 protected InstantType lastSystemChange; 587 588 /** 589 * The link to the source Device that contains administrative device information such as manufacture, serial number, etc. 590 */ 591 @Child(name = "source", type = {Device.class}, order=3, min=0, max=1, modifier=false, summary=true) 592 @Description(shortDefinition="Top-level device resource link", formalDefinition="The link to the source Device that contains administrative device information such as manufacture, serial number, etc." ) 593 protected Reference source; 594 595 /** 596 * The actual object that is the target of the reference (The link to the source Device that contains administrative device information such as manufacture, serial number, etc.) 597 */ 598 protected Device sourceTarget; 599 600 /** 601 * The link to the parent resource. For example: Channel is linked to its VMD parent. 602 */ 603 @Child(name = "parent", type = {DeviceComponent.class}, order=4, min=0, max=1, modifier=false, summary=true) 604 @Description(shortDefinition="Parent resource link", formalDefinition="The link to the parent resource. For example: Channel is linked to its VMD parent." ) 605 protected Reference parent; 606 607 /** 608 * The actual object that is the target of the reference (The link to the parent resource. For example: Channel is linked to its VMD parent.) 609 */ 610 protected DeviceComponent parentTarget; 611 612 /** 613 * The current operational status of the device. For example: On, Off, Standby, etc. 614 */ 615 @Child(name = "operationalStatus", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 616 @Description(shortDefinition="Current operational status of the component, for example On, Off or Standby", formalDefinition="The current operational status of the device. For example: On, Off, Standby, etc." ) 617 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operational-status") 618 protected List<CodeableConcept> operationalStatus; 619 620 /** 621 * The parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular. 622 */ 623 @Child(name = "parameterGroup", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 624 @Description(shortDefinition="Current supported parameter group", formalDefinition="The parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular." ) 625 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/parameter-group") 626 protected CodeableConcept parameterGroup; 627 628 /** 629 * The physical principle of the measurement. For example: thermal, chemical, acoustical, etc. 630 */ 631 @Child(name = "measurementPrinciple", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 632 @Description(shortDefinition="other | chemical | electrical | impedance | nuclear | optical | thermal | biological | mechanical | acoustical | manual+", formalDefinition="The physical principle of the measurement. For example: thermal, chemical, acoustical, etc." ) 633 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measurement-principle") 634 protected Enumeration<MeasmntPrinciple> measurementPrinciple; 635 636 /** 637 * The production specification such as component revision, serial number, etc. 638 */ 639 @Child(name = "productionSpecification", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 640 @Description(shortDefinition="Specification details such as Component Revisions, or Serial Numbers", formalDefinition="The production specification such as component revision, serial number, etc." ) 641 protected List<DeviceComponentProductionSpecificationComponent> productionSpecification; 642 643 /** 644 * The language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US. 645 */ 646 @Child(name = "languageCode", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=true) 647 @Description(shortDefinition="Language code for the human-readable text strings produced by the device", formalDefinition="The language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US." ) 648 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 649 protected CodeableConcept languageCode; 650 651 private static final long serialVersionUID = 32987426L; 652 653 /** 654 * Constructor 655 */ 656 public DeviceComponent() { 657 super(); 658 } 659 660 /** 661 * Constructor 662 */ 663 public DeviceComponent(Identifier identifier, CodeableConcept type) { 664 super(); 665 this.identifier = identifier; 666 this.type = type; 667 } 668 669 /** 670 * @return {@link #identifier} (The locally assigned unique identification by the software. For example: handle ID.) 671 */ 672 public Identifier getIdentifier() { 673 if (this.identifier == null) 674 if (Configuration.errorOnAutoCreate()) 675 throw new Error("Attempt to auto-create DeviceComponent.identifier"); 676 else if (Configuration.doAutoCreate()) 677 this.identifier = new Identifier(); // cc 678 return this.identifier; 679 } 680 681 public boolean hasIdentifier() { 682 return this.identifier != null && !this.identifier.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #identifier} (The locally assigned unique identification by the software. For example: handle ID.) 687 */ 688 public DeviceComponent setIdentifier(Identifier value) { 689 this.identifier = value; 690 return this; 691 } 692 693 /** 694 * @return {@link #type} (The component type as defined in the object-oriented or metric nomenclature partition.) 695 */ 696 public CodeableConcept getType() { 697 if (this.type == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create DeviceComponent.type"); 700 else if (Configuration.doAutoCreate()) 701 this.type = new CodeableConcept(); // cc 702 return this.type; 703 } 704 705 public boolean hasType() { 706 return this.type != null && !this.type.isEmpty(); 707 } 708 709 /** 710 * @param value {@link #type} (The component type as defined in the object-oriented or metric nomenclature partition.) 711 */ 712 public DeviceComponent setType(CodeableConcept value) { 713 this.type = value; 714 return this; 715 } 716 717 /** 718 * @return {@link #lastSystemChange} (The timestamp for the most recent system change which includes device configuration or setting change.). This is the underlying object with id, value and extensions. The accessor "getLastSystemChange" gives direct access to the value 719 */ 720 public InstantType getLastSystemChangeElement() { 721 if (this.lastSystemChange == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create DeviceComponent.lastSystemChange"); 724 else if (Configuration.doAutoCreate()) 725 this.lastSystemChange = new InstantType(); // bb 726 return this.lastSystemChange; 727 } 728 729 public boolean hasLastSystemChangeElement() { 730 return this.lastSystemChange != null && !this.lastSystemChange.isEmpty(); 731 } 732 733 public boolean hasLastSystemChange() { 734 return this.lastSystemChange != null && !this.lastSystemChange.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #lastSystemChange} (The timestamp for the most recent system change which includes device configuration or setting change.). This is the underlying object with id, value and extensions. The accessor "getLastSystemChange" gives direct access to the value 739 */ 740 public DeviceComponent setLastSystemChangeElement(InstantType value) { 741 this.lastSystemChange = value; 742 return this; 743 } 744 745 /** 746 * @return The timestamp for the most recent system change which includes device configuration or setting change. 747 */ 748 public Date getLastSystemChange() { 749 return this.lastSystemChange == null ? null : this.lastSystemChange.getValue(); 750 } 751 752 /** 753 * @param value The timestamp for the most recent system change which includes device configuration or setting change. 754 */ 755 public DeviceComponent setLastSystemChange(Date value) { 756 if (value == null) 757 this.lastSystemChange = null; 758 else { 759 if (this.lastSystemChange == null) 760 this.lastSystemChange = new InstantType(); 761 this.lastSystemChange.setValue(value); 762 } 763 return this; 764 } 765 766 /** 767 * @return {@link #source} (The link to the source Device that contains administrative device information such as manufacture, serial number, etc.) 768 */ 769 public Reference getSource() { 770 if (this.source == null) 771 if (Configuration.errorOnAutoCreate()) 772 throw new Error("Attempt to auto-create DeviceComponent.source"); 773 else if (Configuration.doAutoCreate()) 774 this.source = new Reference(); // cc 775 return this.source; 776 } 777 778 public boolean hasSource() { 779 return this.source != null && !this.source.isEmpty(); 780 } 781 782 /** 783 * @param value {@link #source} (The link to the source Device that contains administrative device information such as manufacture, serial number, etc.) 784 */ 785 public DeviceComponent setSource(Reference value) { 786 this.source = value; 787 return this; 788 } 789 790 /** 791 * @return {@link #source} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The link to the source Device that contains administrative device information such as manufacture, serial number, etc.) 792 */ 793 public Device getSourceTarget() { 794 if (this.sourceTarget == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create DeviceComponent.source"); 797 else if (Configuration.doAutoCreate()) 798 this.sourceTarget = new Device(); // aa 799 return this.sourceTarget; 800 } 801 802 /** 803 * @param value {@link #source} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The link to the source Device that contains administrative device information such as manufacture, serial number, etc.) 804 */ 805 public DeviceComponent setSourceTarget(Device value) { 806 this.sourceTarget = value; 807 return this; 808 } 809 810 /** 811 * @return {@link #parent} (The link to the parent resource. For example: Channel is linked to its VMD parent.) 812 */ 813 public Reference getParent() { 814 if (this.parent == null) 815 if (Configuration.errorOnAutoCreate()) 816 throw new Error("Attempt to auto-create DeviceComponent.parent"); 817 else if (Configuration.doAutoCreate()) 818 this.parent = new Reference(); // cc 819 return this.parent; 820 } 821 822 public boolean hasParent() { 823 return this.parent != null && !this.parent.isEmpty(); 824 } 825 826 /** 827 * @param value {@link #parent} (The link to the parent resource. For example: Channel is linked to its VMD parent.) 828 */ 829 public DeviceComponent setParent(Reference value) { 830 this.parent = value; 831 return this; 832 } 833 834 /** 835 * @return {@link #parent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The link to the parent resource. For example: Channel is linked to its VMD parent.) 836 */ 837 public DeviceComponent getParentTarget() { 838 if (this.parentTarget == null) 839 if (Configuration.errorOnAutoCreate()) 840 throw new Error("Attempt to auto-create DeviceComponent.parent"); 841 else if (Configuration.doAutoCreate()) 842 this.parentTarget = new DeviceComponent(); // aa 843 return this.parentTarget; 844 } 845 846 /** 847 * @param value {@link #parent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The link to the parent resource. For example: Channel is linked to its VMD parent.) 848 */ 849 public DeviceComponent setParentTarget(DeviceComponent value) { 850 this.parentTarget = value; 851 return this; 852 } 853 854 /** 855 * @return {@link #operationalStatus} (The current operational status of the device. For example: On, Off, Standby, etc.) 856 */ 857 public List<CodeableConcept> getOperationalStatus() { 858 if (this.operationalStatus == null) 859 this.operationalStatus = new ArrayList<CodeableConcept>(); 860 return this.operationalStatus; 861 } 862 863 /** 864 * @return Returns a reference to <code>this</code> for easy method chaining 865 */ 866 public DeviceComponent setOperationalStatus(List<CodeableConcept> theOperationalStatus) { 867 this.operationalStatus = theOperationalStatus; 868 return this; 869 } 870 871 public boolean hasOperationalStatus() { 872 if (this.operationalStatus == null) 873 return false; 874 for (CodeableConcept item : this.operationalStatus) 875 if (!item.isEmpty()) 876 return true; 877 return false; 878 } 879 880 public CodeableConcept addOperationalStatus() { //3 881 CodeableConcept t = new CodeableConcept(); 882 if (this.operationalStatus == null) 883 this.operationalStatus = new ArrayList<CodeableConcept>(); 884 this.operationalStatus.add(t); 885 return t; 886 } 887 888 public DeviceComponent addOperationalStatus(CodeableConcept t) { //3 889 if (t == null) 890 return this; 891 if (this.operationalStatus == null) 892 this.operationalStatus = new ArrayList<CodeableConcept>(); 893 this.operationalStatus.add(t); 894 return this; 895 } 896 897 /** 898 * @return The first repetition of repeating field {@link #operationalStatus}, creating it if it does not already exist 899 */ 900 public CodeableConcept getOperationalStatusFirstRep() { 901 if (getOperationalStatus().isEmpty()) { 902 addOperationalStatus(); 903 } 904 return getOperationalStatus().get(0); 905 } 906 907 /** 908 * @return {@link #parameterGroup} (The parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular.) 909 */ 910 public CodeableConcept getParameterGroup() { 911 if (this.parameterGroup == null) 912 if (Configuration.errorOnAutoCreate()) 913 throw new Error("Attempt to auto-create DeviceComponent.parameterGroup"); 914 else if (Configuration.doAutoCreate()) 915 this.parameterGroup = new CodeableConcept(); // cc 916 return this.parameterGroup; 917 } 918 919 public boolean hasParameterGroup() { 920 return this.parameterGroup != null && !this.parameterGroup.isEmpty(); 921 } 922 923 /** 924 * @param value {@link #parameterGroup} (The parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular.) 925 */ 926 public DeviceComponent setParameterGroup(CodeableConcept value) { 927 this.parameterGroup = value; 928 return this; 929 } 930 931 /** 932 * @return {@link #measurementPrinciple} (The physical principle of the measurement. For example: thermal, chemical, acoustical, etc.). This is the underlying object with id, value and extensions. The accessor "getMeasurementPrinciple" gives direct access to the value 933 */ 934 public Enumeration<MeasmntPrinciple> getMeasurementPrincipleElement() { 935 if (this.measurementPrinciple == null) 936 if (Configuration.errorOnAutoCreate()) 937 throw new Error("Attempt to auto-create DeviceComponent.measurementPrinciple"); 938 else if (Configuration.doAutoCreate()) 939 this.measurementPrinciple = new Enumeration<MeasmntPrinciple>(new MeasmntPrincipleEnumFactory()); // bb 940 return this.measurementPrinciple; 941 } 942 943 public boolean hasMeasurementPrincipleElement() { 944 return this.measurementPrinciple != null && !this.measurementPrinciple.isEmpty(); 945 } 946 947 public boolean hasMeasurementPrinciple() { 948 return this.measurementPrinciple != null && !this.measurementPrinciple.isEmpty(); 949 } 950 951 /** 952 * @param value {@link #measurementPrinciple} (The physical principle of the measurement. For example: thermal, chemical, acoustical, etc.). This is the underlying object with id, value and extensions. The accessor "getMeasurementPrinciple" gives direct access to the value 953 */ 954 public DeviceComponent setMeasurementPrincipleElement(Enumeration<MeasmntPrinciple> value) { 955 this.measurementPrinciple = value; 956 return this; 957 } 958 959 /** 960 * @return The physical principle of the measurement. For example: thermal, chemical, acoustical, etc. 961 */ 962 public MeasmntPrinciple getMeasurementPrinciple() { 963 return this.measurementPrinciple == null ? null : this.measurementPrinciple.getValue(); 964 } 965 966 /** 967 * @param value The physical principle of the measurement. For example: thermal, chemical, acoustical, etc. 968 */ 969 public DeviceComponent setMeasurementPrinciple(MeasmntPrinciple value) { 970 if (value == null) 971 this.measurementPrinciple = null; 972 else { 973 if (this.measurementPrinciple == null) 974 this.measurementPrinciple = new Enumeration<MeasmntPrinciple>(new MeasmntPrincipleEnumFactory()); 975 this.measurementPrinciple.setValue(value); 976 } 977 return this; 978 } 979 980 /** 981 * @return {@link #productionSpecification} (The production specification such as component revision, serial number, etc.) 982 */ 983 public List<DeviceComponentProductionSpecificationComponent> getProductionSpecification() { 984 if (this.productionSpecification == null) 985 this.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 986 return this.productionSpecification; 987 } 988 989 /** 990 * @return Returns a reference to <code>this</code> for easy method chaining 991 */ 992 public DeviceComponent setProductionSpecification(List<DeviceComponentProductionSpecificationComponent> theProductionSpecification) { 993 this.productionSpecification = theProductionSpecification; 994 return this; 995 } 996 997 public boolean hasProductionSpecification() { 998 if (this.productionSpecification == null) 999 return false; 1000 for (DeviceComponentProductionSpecificationComponent item : this.productionSpecification) 1001 if (!item.isEmpty()) 1002 return true; 1003 return false; 1004 } 1005 1006 public DeviceComponentProductionSpecificationComponent addProductionSpecification() { //3 1007 DeviceComponentProductionSpecificationComponent t = new DeviceComponentProductionSpecificationComponent(); 1008 if (this.productionSpecification == null) 1009 this.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 1010 this.productionSpecification.add(t); 1011 return t; 1012 } 1013 1014 public DeviceComponent addProductionSpecification(DeviceComponentProductionSpecificationComponent t) { //3 1015 if (t == null) 1016 return this; 1017 if (this.productionSpecification == null) 1018 this.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 1019 this.productionSpecification.add(t); 1020 return this; 1021 } 1022 1023 /** 1024 * @return The first repetition of repeating field {@link #productionSpecification}, creating it if it does not already exist 1025 */ 1026 public DeviceComponentProductionSpecificationComponent getProductionSpecificationFirstRep() { 1027 if (getProductionSpecification().isEmpty()) { 1028 addProductionSpecification(); 1029 } 1030 return getProductionSpecification().get(0); 1031 } 1032 1033 /** 1034 * @return {@link #languageCode} (The language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US.) 1035 */ 1036 public CodeableConcept getLanguageCode() { 1037 if (this.languageCode == null) 1038 if (Configuration.errorOnAutoCreate()) 1039 throw new Error("Attempt to auto-create DeviceComponent.languageCode"); 1040 else if (Configuration.doAutoCreate()) 1041 this.languageCode = new CodeableConcept(); // cc 1042 return this.languageCode; 1043 } 1044 1045 public boolean hasLanguageCode() { 1046 return this.languageCode != null && !this.languageCode.isEmpty(); 1047 } 1048 1049 /** 1050 * @param value {@link #languageCode} (The language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US.) 1051 */ 1052 public DeviceComponent setLanguageCode(CodeableConcept value) { 1053 this.languageCode = value; 1054 return this; 1055 } 1056 1057 protected void listChildren(List<Property> children) { 1058 super.listChildren(children); 1059 children.add(new Property("identifier", "Identifier", "The locally assigned unique identification by the software. For example: handle ID.", 0, 1, identifier)); 1060 children.add(new Property("type", "CodeableConcept", "The component type as defined in the object-oriented or metric nomenclature partition.", 0, 1, type)); 1061 children.add(new Property("lastSystemChange", "instant", "The timestamp for the most recent system change which includes device configuration or setting change.", 0, 1, lastSystemChange)); 1062 children.add(new Property("source", "Reference(Device)", "The link to the source Device that contains administrative device information such as manufacture, serial number, etc.", 0, 1, source)); 1063 children.add(new Property("parent", "Reference(DeviceComponent)", "The link to the parent resource. For example: Channel is linked to its VMD parent.", 0, 1, parent)); 1064 children.add(new Property("operationalStatus", "CodeableConcept", "The current operational status of the device. For example: On, Off, Standby, etc.", 0, java.lang.Integer.MAX_VALUE, operationalStatus)); 1065 children.add(new Property("parameterGroup", "CodeableConcept", "The parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular.", 0, 1, parameterGroup)); 1066 children.add(new Property("measurementPrinciple", "code", "The physical principle of the measurement. For example: thermal, chemical, acoustical, etc.", 0, 1, measurementPrinciple)); 1067 children.add(new Property("productionSpecification", "", "The production specification such as component revision, serial number, etc.", 0, java.lang.Integer.MAX_VALUE, productionSpecification)); 1068 children.add(new Property("languageCode", "CodeableConcept", "The language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US.", 0, 1, languageCode)); 1069 } 1070 1071 @Override 1072 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1073 switch (_hash) { 1074 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The locally assigned unique identification by the software. For example: handle ID.", 0, 1, identifier); 1075 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The component type as defined in the object-oriented or metric nomenclature partition.", 0, 1, type); 1076 case -2072475531: /*lastSystemChange*/ return new Property("lastSystemChange", "instant", "The timestamp for the most recent system change which includes device configuration or setting change.", 0, 1, lastSystemChange); 1077 case -896505829: /*source*/ return new Property("source", "Reference(Device)", "The link to the source Device that contains administrative device information such as manufacture, serial number, etc.", 0, 1, source); 1078 case -995424086: /*parent*/ return new Property("parent", "Reference(DeviceComponent)", "The link to the parent resource. For example: Channel is linked to its VMD parent.", 0, 1, parent); 1079 case -2103166364: /*operationalStatus*/ return new Property("operationalStatus", "CodeableConcept", "The current operational status of the device. For example: On, Off, Standby, etc.", 0, java.lang.Integer.MAX_VALUE, operationalStatus); 1080 case 1111110742: /*parameterGroup*/ return new Property("parameterGroup", "CodeableConcept", "The parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular.", 0, 1, parameterGroup); 1081 case 24324384: /*measurementPrinciple*/ return new Property("measurementPrinciple", "code", "The physical principle of the measurement. For example: thermal, chemical, acoustical, etc.", 0, 1, measurementPrinciple); 1082 case -455527222: /*productionSpecification*/ return new Property("productionSpecification", "", "The production specification such as component revision, serial number, etc.", 0, java.lang.Integer.MAX_VALUE, productionSpecification); 1083 case -2092349083: /*languageCode*/ return new Property("languageCode", "CodeableConcept", "The language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US.", 0, 1, languageCode); 1084 default: return super.getNamedProperty(_hash, _name, _checkValid); 1085 } 1086 1087 } 1088 1089 @Override 1090 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1091 switch (hash) { 1092 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1093 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1094 case -2072475531: /*lastSystemChange*/ return this.lastSystemChange == null ? new Base[0] : new Base[] {this.lastSystemChange}; // InstantType 1095 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1096 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // Reference 1097 case -2103166364: /*operationalStatus*/ return this.operationalStatus == null ? new Base[0] : this.operationalStatus.toArray(new Base[this.operationalStatus.size()]); // CodeableConcept 1098 case 1111110742: /*parameterGroup*/ return this.parameterGroup == null ? new Base[0] : new Base[] {this.parameterGroup}; // CodeableConcept 1099 case 24324384: /*measurementPrinciple*/ return this.measurementPrinciple == null ? new Base[0] : new Base[] {this.measurementPrinciple}; // Enumeration<MeasmntPrinciple> 1100 case -455527222: /*productionSpecification*/ return this.productionSpecification == null ? new Base[0] : this.productionSpecification.toArray(new Base[this.productionSpecification.size()]); // DeviceComponentProductionSpecificationComponent 1101 case -2092349083: /*languageCode*/ return this.languageCode == null ? new Base[0] : new Base[] {this.languageCode}; // CodeableConcept 1102 default: return super.getProperty(hash, name, checkValid); 1103 } 1104 1105 } 1106 1107 @Override 1108 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1109 switch (hash) { 1110 case -1618432855: // identifier 1111 this.identifier = castToIdentifier(value); // Identifier 1112 return value; 1113 case 3575610: // type 1114 this.type = castToCodeableConcept(value); // CodeableConcept 1115 return value; 1116 case -2072475531: // lastSystemChange 1117 this.lastSystemChange = castToInstant(value); // InstantType 1118 return value; 1119 case -896505829: // source 1120 this.source = castToReference(value); // Reference 1121 return value; 1122 case -995424086: // parent 1123 this.parent = castToReference(value); // Reference 1124 return value; 1125 case -2103166364: // operationalStatus 1126 this.getOperationalStatus().add(castToCodeableConcept(value)); // CodeableConcept 1127 return value; 1128 case 1111110742: // parameterGroup 1129 this.parameterGroup = castToCodeableConcept(value); // CodeableConcept 1130 return value; 1131 case 24324384: // measurementPrinciple 1132 value = new MeasmntPrincipleEnumFactory().fromType(castToCode(value)); 1133 this.measurementPrinciple = (Enumeration) value; // Enumeration<MeasmntPrinciple> 1134 return value; 1135 case -455527222: // productionSpecification 1136 this.getProductionSpecification().add((DeviceComponentProductionSpecificationComponent) value); // DeviceComponentProductionSpecificationComponent 1137 return value; 1138 case -2092349083: // languageCode 1139 this.languageCode = castToCodeableConcept(value); // CodeableConcept 1140 return value; 1141 default: return super.setProperty(hash, name, value); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base setProperty(String name, Base value) throws FHIRException { 1148 if (name.equals("identifier")) { 1149 this.identifier = castToIdentifier(value); // Identifier 1150 } else if (name.equals("type")) { 1151 this.type = castToCodeableConcept(value); // CodeableConcept 1152 } else if (name.equals("lastSystemChange")) { 1153 this.lastSystemChange = castToInstant(value); // InstantType 1154 } else if (name.equals("source")) { 1155 this.source = castToReference(value); // Reference 1156 } else if (name.equals("parent")) { 1157 this.parent = castToReference(value); // Reference 1158 } else if (name.equals("operationalStatus")) { 1159 this.getOperationalStatus().add(castToCodeableConcept(value)); 1160 } else if (name.equals("parameterGroup")) { 1161 this.parameterGroup = castToCodeableConcept(value); // CodeableConcept 1162 } else if (name.equals("measurementPrinciple")) { 1163 value = new MeasmntPrincipleEnumFactory().fromType(castToCode(value)); 1164 this.measurementPrinciple = (Enumeration) value; // Enumeration<MeasmntPrinciple> 1165 } else if (name.equals("productionSpecification")) { 1166 this.getProductionSpecification().add((DeviceComponentProductionSpecificationComponent) value); 1167 } else if (name.equals("languageCode")) { 1168 this.languageCode = castToCodeableConcept(value); // CodeableConcept 1169 } else 1170 return super.setProperty(name, value); 1171 return value; 1172 } 1173 1174 @Override 1175 public Base makeProperty(int hash, String name) throws FHIRException { 1176 switch (hash) { 1177 case -1618432855: return getIdentifier(); 1178 case 3575610: return getType(); 1179 case -2072475531: return getLastSystemChangeElement(); 1180 case -896505829: return getSource(); 1181 case -995424086: return getParent(); 1182 case -2103166364: return addOperationalStatus(); 1183 case 1111110742: return getParameterGroup(); 1184 case 24324384: return getMeasurementPrincipleElement(); 1185 case -455527222: return addProductionSpecification(); 1186 case -2092349083: return getLanguageCode(); 1187 default: return super.makeProperty(hash, name); 1188 } 1189 1190 } 1191 1192 @Override 1193 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1194 switch (hash) { 1195 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1196 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1197 case -2072475531: /*lastSystemChange*/ return new String[] {"instant"}; 1198 case -896505829: /*source*/ return new String[] {"Reference"}; 1199 case -995424086: /*parent*/ return new String[] {"Reference"}; 1200 case -2103166364: /*operationalStatus*/ return new String[] {"CodeableConcept"}; 1201 case 1111110742: /*parameterGroup*/ return new String[] {"CodeableConcept"}; 1202 case 24324384: /*measurementPrinciple*/ return new String[] {"code"}; 1203 case -455527222: /*productionSpecification*/ return new String[] {}; 1204 case -2092349083: /*languageCode*/ return new String[] {"CodeableConcept"}; 1205 default: return super.getTypesForProperty(hash, name); 1206 } 1207 1208 } 1209 1210 @Override 1211 public Base addChild(String name) throws FHIRException { 1212 if (name.equals("identifier")) { 1213 this.identifier = new Identifier(); 1214 return this.identifier; 1215 } 1216 else if (name.equals("type")) { 1217 this.type = new CodeableConcept(); 1218 return this.type; 1219 } 1220 else if (name.equals("lastSystemChange")) { 1221 throw new FHIRException("Cannot call addChild on a singleton property DeviceComponent.lastSystemChange"); 1222 } 1223 else if (name.equals("source")) { 1224 this.source = new Reference(); 1225 return this.source; 1226 } 1227 else if (name.equals("parent")) { 1228 this.parent = new Reference(); 1229 return this.parent; 1230 } 1231 else if (name.equals("operationalStatus")) { 1232 return addOperationalStatus(); 1233 } 1234 else if (name.equals("parameterGroup")) { 1235 this.parameterGroup = new CodeableConcept(); 1236 return this.parameterGroup; 1237 } 1238 else if (name.equals("measurementPrinciple")) { 1239 throw new FHIRException("Cannot call addChild on a singleton property DeviceComponent.measurementPrinciple"); 1240 } 1241 else if (name.equals("productionSpecification")) { 1242 return addProductionSpecification(); 1243 } 1244 else if (name.equals("languageCode")) { 1245 this.languageCode = new CodeableConcept(); 1246 return this.languageCode; 1247 } 1248 else 1249 return super.addChild(name); 1250 } 1251 1252 public String fhirType() { 1253 return "DeviceComponent"; 1254 1255 } 1256 1257 public DeviceComponent copy() { 1258 DeviceComponent dst = new DeviceComponent(); 1259 copyValues(dst); 1260 dst.identifier = identifier == null ? null : identifier.copy(); 1261 dst.type = type == null ? null : type.copy(); 1262 dst.lastSystemChange = lastSystemChange == null ? null : lastSystemChange.copy(); 1263 dst.source = source == null ? null : source.copy(); 1264 dst.parent = parent == null ? null : parent.copy(); 1265 if (operationalStatus != null) { 1266 dst.operationalStatus = new ArrayList<CodeableConcept>(); 1267 for (CodeableConcept i : operationalStatus) 1268 dst.operationalStatus.add(i.copy()); 1269 }; 1270 dst.parameterGroup = parameterGroup == null ? null : parameterGroup.copy(); 1271 dst.measurementPrinciple = measurementPrinciple == null ? null : measurementPrinciple.copy(); 1272 if (productionSpecification != null) { 1273 dst.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 1274 for (DeviceComponentProductionSpecificationComponent i : productionSpecification) 1275 dst.productionSpecification.add(i.copy()); 1276 }; 1277 dst.languageCode = languageCode == null ? null : languageCode.copy(); 1278 return dst; 1279 } 1280 1281 protected DeviceComponent typedCopy() { 1282 return copy(); 1283 } 1284 1285 @Override 1286 public boolean equalsDeep(Base other_) { 1287 if (!super.equalsDeep(other_)) 1288 return false; 1289 if (!(other_ instanceof DeviceComponent)) 1290 return false; 1291 DeviceComponent o = (DeviceComponent) other_; 1292 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(lastSystemChange, o.lastSystemChange, true) 1293 && compareDeep(source, o.source, true) && compareDeep(parent, o.parent, true) && compareDeep(operationalStatus, o.operationalStatus, true) 1294 && compareDeep(parameterGroup, o.parameterGroup, true) && compareDeep(measurementPrinciple, o.measurementPrinciple, true) 1295 && compareDeep(productionSpecification, o.productionSpecification, true) && compareDeep(languageCode, o.languageCode, true) 1296 ; 1297 } 1298 1299 @Override 1300 public boolean equalsShallow(Base other_) { 1301 if (!super.equalsShallow(other_)) 1302 return false; 1303 if (!(other_ instanceof DeviceComponent)) 1304 return false; 1305 DeviceComponent o = (DeviceComponent) other_; 1306 return compareValues(lastSystemChange, o.lastSystemChange, true) && compareValues(measurementPrinciple, o.measurementPrinciple, true) 1307 ; 1308 } 1309 1310 public boolean isEmpty() { 1311 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, lastSystemChange 1312 , source, parent, operationalStatus, parameterGroup, measurementPrinciple, productionSpecification 1313 , languageCode); 1314 } 1315 1316 @Override 1317 public ResourceType getResourceType() { 1318 return ResourceType.DeviceComponent; 1319 } 1320 1321 /** 1322 * Search parameter: <b>parent</b> 1323 * <p> 1324 * Description: <b>The parent DeviceComponent resource</b><br> 1325 * Type: <b>reference</b><br> 1326 * Path: <b>DeviceComponent.parent</b><br> 1327 * </p> 1328 */ 1329 @SearchParamDefinition(name="parent", path="DeviceComponent.parent", description="The parent DeviceComponent resource", type="reference", target={DeviceComponent.class } ) 1330 public static final String SP_PARENT = "parent"; 1331 /** 1332 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 1333 * <p> 1334 * Description: <b>The parent DeviceComponent resource</b><br> 1335 * Type: <b>reference</b><br> 1336 * Path: <b>DeviceComponent.parent</b><br> 1337 * </p> 1338 */ 1339 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 1340 1341/** 1342 * Constant for fluent queries to be used to add include statements. Specifies 1343 * the path value of "<b>DeviceComponent:parent</b>". 1344 */ 1345 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("DeviceComponent:parent").toLocked(); 1346 1347 /** 1348 * Search parameter: <b>identifier</b> 1349 * <p> 1350 * Description: <b>The identifier of the component</b><br> 1351 * Type: <b>token</b><br> 1352 * Path: <b>DeviceComponent.identifier</b><br> 1353 * </p> 1354 */ 1355 @SearchParamDefinition(name="identifier", path="DeviceComponent.identifier", description="The identifier of the component", type="token" ) 1356 public static final String SP_IDENTIFIER = "identifier"; 1357 /** 1358 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1359 * <p> 1360 * Description: <b>The identifier of the component</b><br> 1361 * Type: <b>token</b><br> 1362 * Path: <b>DeviceComponent.identifier</b><br> 1363 * </p> 1364 */ 1365 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1366 1367 /** 1368 * Search parameter: <b>source</b> 1369 * <p> 1370 * Description: <b>The device source</b><br> 1371 * Type: <b>reference</b><br> 1372 * Path: <b>DeviceComponent.source</b><br> 1373 * </p> 1374 */ 1375 @SearchParamDefinition(name="source", path="DeviceComponent.source", description="The device source", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class } ) 1376 public static final String SP_SOURCE = "source"; 1377 /** 1378 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1379 * <p> 1380 * Description: <b>The device source</b><br> 1381 * Type: <b>reference</b><br> 1382 * Path: <b>DeviceComponent.source</b><br> 1383 * </p> 1384 */ 1385 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 1386 1387/** 1388 * Constant for fluent queries to be used to add include statements. Specifies 1389 * the path value of "<b>DeviceComponent:source</b>". 1390 */ 1391 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("DeviceComponent:source").toLocked(); 1392 1393 /** 1394 * Search parameter: <b>type</b> 1395 * <p> 1396 * Description: <b>The device component type</b><br> 1397 * Type: <b>token</b><br> 1398 * Path: <b>DeviceComponent.type</b><br> 1399 * </p> 1400 */ 1401 @SearchParamDefinition(name="type", path="DeviceComponent.type", description="The device component type", type="token" ) 1402 public static final String SP_TYPE = "type"; 1403 /** 1404 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1405 * <p> 1406 * Description: <b>The device component type</b><br> 1407 * Type: <b>token</b><br> 1408 * Path: <b>DeviceComponent.type</b><br> 1409 * </p> 1410 */ 1411 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1412 1413 1414}