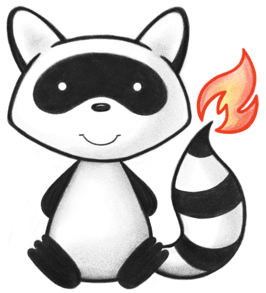
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Describes a measurement, calculation or setting capability of a medical device. 049 */ 050@ResourceDef(name="DeviceMetric", profile="http://hl7.org/fhir/Profile/DeviceMetric") 051public class DeviceMetric extends DomainResource { 052 053 public enum DeviceMetricOperationalStatus { 054 /** 055 * The DeviceMetric is operating and will generate DeviceObservations. 056 */ 057 ON, 058 /** 059 * The DeviceMetric is not operating. 060 */ 061 OFF, 062 /** 063 * The DeviceMetric is operating, but will not generate any DeviceObservations. 064 */ 065 STANDBY, 066 /** 067 * The DeviceMetric was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 public static DeviceMetricOperationalStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("on".equals(codeString)) 078 return ON; 079 if ("off".equals(codeString)) 080 return OFF; 081 if ("standby".equals(codeString)) 082 return STANDBY; 083 if ("entered-in-error".equals(codeString)) 084 return ENTEREDINERROR; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 089 } 090 public String toCode() { 091 switch (this) { 092 case ON: return "on"; 093 case OFF: return "off"; 094 case STANDBY: return "standby"; 095 case ENTEREDINERROR: return "entered-in-error"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getSystem() { 101 switch (this) { 102 case ON: return "http://hl7.org/fhir/metric-operational-status"; 103 case OFF: return "http://hl7.org/fhir/metric-operational-status"; 104 case STANDBY: return "http://hl7.org/fhir/metric-operational-status"; 105 case ENTEREDINERROR: return "http://hl7.org/fhir/metric-operational-status"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getDefinition() { 111 switch (this) { 112 case ON: return "The DeviceMetric is operating and will generate DeviceObservations."; 113 case OFF: return "The DeviceMetric is not operating."; 114 case STANDBY: return "The DeviceMetric is operating, but will not generate any DeviceObservations."; 115 case ENTEREDINERROR: return "The DeviceMetric was entered in error."; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDisplay() { 121 switch (this) { 122 case ON: return "On"; 123 case OFF: return "Off"; 124 case STANDBY: return "Standby"; 125 case ENTEREDINERROR: return "Entered In Error"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 } 131 132 public static class DeviceMetricOperationalStatusEnumFactory implements EnumFactory<DeviceMetricOperationalStatus> { 133 public DeviceMetricOperationalStatus fromCode(String codeString) throws IllegalArgumentException { 134 if (codeString == null || "".equals(codeString)) 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("on".equals(codeString)) 138 return DeviceMetricOperationalStatus.ON; 139 if ("off".equals(codeString)) 140 return DeviceMetricOperationalStatus.OFF; 141 if ("standby".equals(codeString)) 142 return DeviceMetricOperationalStatus.STANDBY; 143 if ("entered-in-error".equals(codeString)) 144 return DeviceMetricOperationalStatus.ENTEREDINERROR; 145 throw new IllegalArgumentException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 146 } 147 public Enumeration<DeviceMetricOperationalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 148 if (code == null) 149 return null; 150 if (code.isEmpty()) 151 return new Enumeration<DeviceMetricOperationalStatus>(this); 152 String codeString = code.asStringValue(); 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("on".equals(codeString)) 156 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ON); 157 if ("off".equals(codeString)) 158 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.OFF); 159 if ("standby".equals(codeString)) 160 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.STANDBY); 161 if ("entered-in-error".equals(codeString)) 162 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ENTEREDINERROR); 163 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 164 } 165 public String toCode(DeviceMetricOperationalStatus code) { 166 if (code == DeviceMetricOperationalStatus.NULL) 167 return null; 168 if (code == DeviceMetricOperationalStatus.ON) 169 return "on"; 170 if (code == DeviceMetricOperationalStatus.OFF) 171 return "off"; 172 if (code == DeviceMetricOperationalStatus.STANDBY) 173 return "standby"; 174 if (code == DeviceMetricOperationalStatus.ENTEREDINERROR) 175 return "entered-in-error"; 176 return "?"; 177 } 178 public String toSystem(DeviceMetricOperationalStatus code) { 179 return code.getSystem(); 180 } 181 } 182 183 public enum DeviceMetricColor { 184 /** 185 * Color for representation - black. 186 */ 187 BLACK, 188 /** 189 * Color for representation - red. 190 */ 191 RED, 192 /** 193 * Color for representation - green. 194 */ 195 GREEN, 196 /** 197 * Color for representation - yellow. 198 */ 199 YELLOW, 200 /** 201 * Color for representation - blue. 202 */ 203 BLUE, 204 /** 205 * Color for representation - magenta. 206 */ 207 MAGENTA, 208 /** 209 * Color for representation - cyan. 210 */ 211 CYAN, 212 /** 213 * Color for representation - white. 214 */ 215 WHITE, 216 /** 217 * added to help the parsers with the generic types 218 */ 219 NULL; 220 public static DeviceMetricColor fromCode(String codeString) throws FHIRException { 221 if (codeString == null || "".equals(codeString)) 222 return null; 223 if ("black".equals(codeString)) 224 return BLACK; 225 if ("red".equals(codeString)) 226 return RED; 227 if ("green".equals(codeString)) 228 return GREEN; 229 if ("yellow".equals(codeString)) 230 return YELLOW; 231 if ("blue".equals(codeString)) 232 return BLUE; 233 if ("magenta".equals(codeString)) 234 return MAGENTA; 235 if ("cyan".equals(codeString)) 236 return CYAN; 237 if ("white".equals(codeString)) 238 return WHITE; 239 if (Configuration.isAcceptInvalidEnums()) 240 return null; 241 else 242 throw new FHIRException("Unknown DeviceMetricColor code '"+codeString+"'"); 243 } 244 public String toCode() { 245 switch (this) { 246 case BLACK: return "black"; 247 case RED: return "red"; 248 case GREEN: return "green"; 249 case YELLOW: return "yellow"; 250 case BLUE: return "blue"; 251 case MAGENTA: return "magenta"; 252 case CYAN: return "cyan"; 253 case WHITE: return "white"; 254 case NULL: return null; 255 default: return "?"; 256 } 257 } 258 public String getSystem() { 259 switch (this) { 260 case BLACK: return "http://hl7.org/fhir/metric-color"; 261 case RED: return "http://hl7.org/fhir/metric-color"; 262 case GREEN: return "http://hl7.org/fhir/metric-color"; 263 case YELLOW: return "http://hl7.org/fhir/metric-color"; 264 case BLUE: return "http://hl7.org/fhir/metric-color"; 265 case MAGENTA: return "http://hl7.org/fhir/metric-color"; 266 case CYAN: return "http://hl7.org/fhir/metric-color"; 267 case WHITE: return "http://hl7.org/fhir/metric-color"; 268 case NULL: return null; 269 default: return "?"; 270 } 271 } 272 public String getDefinition() { 273 switch (this) { 274 case BLACK: return "Color for representation - black."; 275 case RED: return "Color for representation - red."; 276 case GREEN: return "Color for representation - green."; 277 case YELLOW: return "Color for representation - yellow."; 278 case BLUE: return "Color for representation - blue."; 279 case MAGENTA: return "Color for representation - magenta."; 280 case CYAN: return "Color for representation - cyan."; 281 case WHITE: return "Color for representation - white."; 282 case NULL: return null; 283 default: return "?"; 284 } 285 } 286 public String getDisplay() { 287 switch (this) { 288 case BLACK: return "Color Black"; 289 case RED: return "Color Red"; 290 case GREEN: return "Color Green"; 291 case YELLOW: return "Color Yellow"; 292 case BLUE: return "Color Blue"; 293 case MAGENTA: return "Color Magenta"; 294 case CYAN: return "Color Cyan"; 295 case WHITE: return "Color White"; 296 case NULL: return null; 297 default: return "?"; 298 } 299 } 300 } 301 302 public static class DeviceMetricColorEnumFactory implements EnumFactory<DeviceMetricColor> { 303 public DeviceMetricColor fromCode(String codeString) throws IllegalArgumentException { 304 if (codeString == null || "".equals(codeString)) 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("black".equals(codeString)) 308 return DeviceMetricColor.BLACK; 309 if ("red".equals(codeString)) 310 return DeviceMetricColor.RED; 311 if ("green".equals(codeString)) 312 return DeviceMetricColor.GREEN; 313 if ("yellow".equals(codeString)) 314 return DeviceMetricColor.YELLOW; 315 if ("blue".equals(codeString)) 316 return DeviceMetricColor.BLUE; 317 if ("magenta".equals(codeString)) 318 return DeviceMetricColor.MAGENTA; 319 if ("cyan".equals(codeString)) 320 return DeviceMetricColor.CYAN; 321 if ("white".equals(codeString)) 322 return DeviceMetricColor.WHITE; 323 throw new IllegalArgumentException("Unknown DeviceMetricColor code '"+codeString+"'"); 324 } 325 public Enumeration<DeviceMetricColor> fromType(PrimitiveType<?> code) throws FHIRException { 326 if (code == null) 327 return null; 328 if (code.isEmpty()) 329 return new Enumeration<DeviceMetricColor>(this); 330 String codeString = code.asStringValue(); 331 if (codeString == null || "".equals(codeString)) 332 return null; 333 if ("black".equals(codeString)) 334 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLACK); 335 if ("red".equals(codeString)) 336 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.RED); 337 if ("green".equals(codeString)) 338 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.GREEN); 339 if ("yellow".equals(codeString)) 340 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.YELLOW); 341 if ("blue".equals(codeString)) 342 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLUE); 343 if ("magenta".equals(codeString)) 344 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.MAGENTA); 345 if ("cyan".equals(codeString)) 346 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.CYAN); 347 if ("white".equals(codeString)) 348 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.WHITE); 349 throw new FHIRException("Unknown DeviceMetricColor code '"+codeString+"'"); 350 } 351 public String toCode(DeviceMetricColor code) { 352 if (code == DeviceMetricColor.NULL) 353 return null; 354 if (code == DeviceMetricColor.BLACK) 355 return "black"; 356 if (code == DeviceMetricColor.RED) 357 return "red"; 358 if (code == DeviceMetricColor.GREEN) 359 return "green"; 360 if (code == DeviceMetricColor.YELLOW) 361 return "yellow"; 362 if (code == DeviceMetricColor.BLUE) 363 return "blue"; 364 if (code == DeviceMetricColor.MAGENTA) 365 return "magenta"; 366 if (code == DeviceMetricColor.CYAN) 367 return "cyan"; 368 if (code == DeviceMetricColor.WHITE) 369 return "white"; 370 return "?"; 371 } 372 public String toSystem(DeviceMetricColor code) { 373 return code.getSystem(); 374 } 375 } 376 377 public enum DeviceMetricCategory { 378 /** 379 * DeviceObservations generated for this DeviceMetric are measured. 380 */ 381 MEASUREMENT, 382 /** 383 * DeviceObservations generated for this DeviceMetric is a setting that will influence the behavior of the Device. 384 */ 385 SETTING, 386 /** 387 * DeviceObservations generated for this DeviceMetric are calculated. 388 */ 389 CALCULATION, 390 /** 391 * The category of this DeviceMetric is unspecified. 392 */ 393 UNSPECIFIED, 394 /** 395 * added to help the parsers with the generic types 396 */ 397 NULL; 398 public static DeviceMetricCategory fromCode(String codeString) throws FHIRException { 399 if (codeString == null || "".equals(codeString)) 400 return null; 401 if ("measurement".equals(codeString)) 402 return MEASUREMENT; 403 if ("setting".equals(codeString)) 404 return SETTING; 405 if ("calculation".equals(codeString)) 406 return CALCULATION; 407 if ("unspecified".equals(codeString)) 408 return UNSPECIFIED; 409 if (Configuration.isAcceptInvalidEnums()) 410 return null; 411 else 412 throw new FHIRException("Unknown DeviceMetricCategory code '"+codeString+"'"); 413 } 414 public String toCode() { 415 switch (this) { 416 case MEASUREMENT: return "measurement"; 417 case SETTING: return "setting"; 418 case CALCULATION: return "calculation"; 419 case UNSPECIFIED: return "unspecified"; 420 case NULL: return null; 421 default: return "?"; 422 } 423 } 424 public String getSystem() { 425 switch (this) { 426 case MEASUREMENT: return "http://hl7.org/fhir/metric-category"; 427 case SETTING: return "http://hl7.org/fhir/metric-category"; 428 case CALCULATION: return "http://hl7.org/fhir/metric-category"; 429 case UNSPECIFIED: return "http://hl7.org/fhir/metric-category"; 430 case NULL: return null; 431 default: return "?"; 432 } 433 } 434 public String getDefinition() { 435 switch (this) { 436 case MEASUREMENT: return "DeviceObservations generated for this DeviceMetric are measured."; 437 case SETTING: return "DeviceObservations generated for this DeviceMetric is a setting that will influence the behavior of the Device."; 438 case CALCULATION: return "DeviceObservations generated for this DeviceMetric are calculated."; 439 case UNSPECIFIED: return "The category of this DeviceMetric is unspecified."; 440 case NULL: return null; 441 default: return "?"; 442 } 443 } 444 public String getDisplay() { 445 switch (this) { 446 case MEASUREMENT: return "Measurement"; 447 case SETTING: return "Setting"; 448 case CALCULATION: return "Calculation"; 449 case UNSPECIFIED: return "Unspecified"; 450 case NULL: return null; 451 default: return "?"; 452 } 453 } 454 } 455 456 public static class DeviceMetricCategoryEnumFactory implements EnumFactory<DeviceMetricCategory> { 457 public DeviceMetricCategory fromCode(String codeString) throws IllegalArgumentException { 458 if (codeString == null || "".equals(codeString)) 459 if (codeString == null || "".equals(codeString)) 460 return null; 461 if ("measurement".equals(codeString)) 462 return DeviceMetricCategory.MEASUREMENT; 463 if ("setting".equals(codeString)) 464 return DeviceMetricCategory.SETTING; 465 if ("calculation".equals(codeString)) 466 return DeviceMetricCategory.CALCULATION; 467 if ("unspecified".equals(codeString)) 468 return DeviceMetricCategory.UNSPECIFIED; 469 throw new IllegalArgumentException("Unknown DeviceMetricCategory code '"+codeString+"'"); 470 } 471 public Enumeration<DeviceMetricCategory> fromType(PrimitiveType<?> code) throws FHIRException { 472 if (code == null) 473 return null; 474 if (code.isEmpty()) 475 return new Enumeration<DeviceMetricCategory>(this); 476 String codeString = code.asStringValue(); 477 if (codeString == null || "".equals(codeString)) 478 return null; 479 if ("measurement".equals(codeString)) 480 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.MEASUREMENT); 481 if ("setting".equals(codeString)) 482 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.SETTING); 483 if ("calculation".equals(codeString)) 484 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.CALCULATION); 485 if ("unspecified".equals(codeString)) 486 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.UNSPECIFIED); 487 throw new FHIRException("Unknown DeviceMetricCategory code '"+codeString+"'"); 488 } 489 public String toCode(DeviceMetricCategory code) { 490 if (code == DeviceMetricCategory.NULL) 491 return null; 492 if (code == DeviceMetricCategory.MEASUREMENT) 493 return "measurement"; 494 if (code == DeviceMetricCategory.SETTING) 495 return "setting"; 496 if (code == DeviceMetricCategory.CALCULATION) 497 return "calculation"; 498 if (code == DeviceMetricCategory.UNSPECIFIED) 499 return "unspecified"; 500 return "?"; 501 } 502 public String toSystem(DeviceMetricCategory code) { 503 return code.getSystem(); 504 } 505 } 506 507 public enum DeviceMetricCalibrationType { 508 /** 509 * Metric calibration method has not been identified. 510 */ 511 UNSPECIFIED, 512 /** 513 * Offset metric calibration method 514 */ 515 OFFSET, 516 /** 517 * Gain metric calibration method 518 */ 519 GAIN, 520 /** 521 * Two-point metric calibration method 522 */ 523 TWOPOINT, 524 /** 525 * added to help the parsers with the generic types 526 */ 527 NULL; 528 public static DeviceMetricCalibrationType fromCode(String codeString) throws FHIRException { 529 if (codeString == null || "".equals(codeString)) 530 return null; 531 if ("unspecified".equals(codeString)) 532 return UNSPECIFIED; 533 if ("offset".equals(codeString)) 534 return OFFSET; 535 if ("gain".equals(codeString)) 536 return GAIN; 537 if ("two-point".equals(codeString)) 538 return TWOPOINT; 539 if (Configuration.isAcceptInvalidEnums()) 540 return null; 541 else 542 throw new FHIRException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 543 } 544 public String toCode() { 545 switch (this) { 546 case UNSPECIFIED: return "unspecified"; 547 case OFFSET: return "offset"; 548 case GAIN: return "gain"; 549 case TWOPOINT: return "two-point"; 550 case NULL: return null; 551 default: return "?"; 552 } 553 } 554 public String getSystem() { 555 switch (this) { 556 case UNSPECIFIED: return "http://hl7.org/fhir/metric-calibration-type"; 557 case OFFSET: return "http://hl7.org/fhir/metric-calibration-type"; 558 case GAIN: return "http://hl7.org/fhir/metric-calibration-type"; 559 case TWOPOINT: return "http://hl7.org/fhir/metric-calibration-type"; 560 case NULL: return null; 561 default: return "?"; 562 } 563 } 564 public String getDefinition() { 565 switch (this) { 566 case UNSPECIFIED: return "Metric calibration method has not been identified."; 567 case OFFSET: return "Offset metric calibration method"; 568 case GAIN: return "Gain metric calibration method"; 569 case TWOPOINT: return "Two-point metric calibration method"; 570 case NULL: return null; 571 default: return "?"; 572 } 573 } 574 public String getDisplay() { 575 switch (this) { 576 case UNSPECIFIED: return "Unspecified"; 577 case OFFSET: return "Offset"; 578 case GAIN: return "Gain"; 579 case TWOPOINT: return "Two Point"; 580 case NULL: return null; 581 default: return "?"; 582 } 583 } 584 } 585 586 public static class DeviceMetricCalibrationTypeEnumFactory implements EnumFactory<DeviceMetricCalibrationType> { 587 public DeviceMetricCalibrationType fromCode(String codeString) throws IllegalArgumentException { 588 if (codeString == null || "".equals(codeString)) 589 if (codeString == null || "".equals(codeString)) 590 return null; 591 if ("unspecified".equals(codeString)) 592 return DeviceMetricCalibrationType.UNSPECIFIED; 593 if ("offset".equals(codeString)) 594 return DeviceMetricCalibrationType.OFFSET; 595 if ("gain".equals(codeString)) 596 return DeviceMetricCalibrationType.GAIN; 597 if ("two-point".equals(codeString)) 598 return DeviceMetricCalibrationType.TWOPOINT; 599 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 600 } 601 public Enumeration<DeviceMetricCalibrationType> fromType(PrimitiveType<?> code) throws FHIRException { 602 if (code == null) 603 return null; 604 if (code.isEmpty()) 605 return new Enumeration<DeviceMetricCalibrationType>(this); 606 String codeString = code.asStringValue(); 607 if (codeString == null || "".equals(codeString)) 608 return null; 609 if ("unspecified".equals(codeString)) 610 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.UNSPECIFIED); 611 if ("offset".equals(codeString)) 612 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.OFFSET); 613 if ("gain".equals(codeString)) 614 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.GAIN); 615 if ("two-point".equals(codeString)) 616 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.TWOPOINT); 617 throw new FHIRException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 618 } 619 public String toCode(DeviceMetricCalibrationType code) { 620 if (code == DeviceMetricCalibrationType.NULL) 621 return null; 622 if (code == DeviceMetricCalibrationType.UNSPECIFIED) 623 return "unspecified"; 624 if (code == DeviceMetricCalibrationType.OFFSET) 625 return "offset"; 626 if (code == DeviceMetricCalibrationType.GAIN) 627 return "gain"; 628 if (code == DeviceMetricCalibrationType.TWOPOINT) 629 return "two-point"; 630 return "?"; 631 } 632 public String toSystem(DeviceMetricCalibrationType code) { 633 return code.getSystem(); 634 } 635 } 636 637 public enum DeviceMetricCalibrationState { 638 /** 639 * The metric has not been calibrated. 640 */ 641 NOTCALIBRATED, 642 /** 643 * The metric needs to be calibrated. 644 */ 645 CALIBRATIONREQUIRED, 646 /** 647 * The metric has been calibrated. 648 */ 649 CALIBRATED, 650 /** 651 * The state of calibration of this metric is unspecified. 652 */ 653 UNSPECIFIED, 654 /** 655 * added to help the parsers with the generic types 656 */ 657 NULL; 658 public static DeviceMetricCalibrationState fromCode(String codeString) throws FHIRException { 659 if (codeString == null || "".equals(codeString)) 660 return null; 661 if ("not-calibrated".equals(codeString)) 662 return NOTCALIBRATED; 663 if ("calibration-required".equals(codeString)) 664 return CALIBRATIONREQUIRED; 665 if ("calibrated".equals(codeString)) 666 return CALIBRATED; 667 if ("unspecified".equals(codeString)) 668 return UNSPECIFIED; 669 if (Configuration.isAcceptInvalidEnums()) 670 return null; 671 else 672 throw new FHIRException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 673 } 674 public String toCode() { 675 switch (this) { 676 case NOTCALIBRATED: return "not-calibrated"; 677 case CALIBRATIONREQUIRED: return "calibration-required"; 678 case CALIBRATED: return "calibrated"; 679 case UNSPECIFIED: return "unspecified"; 680 case NULL: return null; 681 default: return "?"; 682 } 683 } 684 public String getSystem() { 685 switch (this) { 686 case NOTCALIBRATED: return "http://hl7.org/fhir/metric-calibration-state"; 687 case CALIBRATIONREQUIRED: return "http://hl7.org/fhir/metric-calibration-state"; 688 case CALIBRATED: return "http://hl7.org/fhir/metric-calibration-state"; 689 case UNSPECIFIED: return "http://hl7.org/fhir/metric-calibration-state"; 690 case NULL: return null; 691 default: return "?"; 692 } 693 } 694 public String getDefinition() { 695 switch (this) { 696 case NOTCALIBRATED: return "The metric has not been calibrated."; 697 case CALIBRATIONREQUIRED: return "The metric needs to be calibrated."; 698 case CALIBRATED: return "The metric has been calibrated."; 699 case UNSPECIFIED: return "The state of calibration of this metric is unspecified."; 700 case NULL: return null; 701 default: return "?"; 702 } 703 } 704 public String getDisplay() { 705 switch (this) { 706 case NOTCALIBRATED: return "Not Calibrated"; 707 case CALIBRATIONREQUIRED: return "Calibration Required"; 708 case CALIBRATED: return "Calibrated"; 709 case UNSPECIFIED: return "Unspecified"; 710 case NULL: return null; 711 default: return "?"; 712 } 713 } 714 } 715 716 public static class DeviceMetricCalibrationStateEnumFactory implements EnumFactory<DeviceMetricCalibrationState> { 717 public DeviceMetricCalibrationState fromCode(String codeString) throws IllegalArgumentException { 718 if (codeString == null || "".equals(codeString)) 719 if (codeString == null || "".equals(codeString)) 720 return null; 721 if ("not-calibrated".equals(codeString)) 722 return DeviceMetricCalibrationState.NOTCALIBRATED; 723 if ("calibration-required".equals(codeString)) 724 return DeviceMetricCalibrationState.CALIBRATIONREQUIRED; 725 if ("calibrated".equals(codeString)) 726 return DeviceMetricCalibrationState.CALIBRATED; 727 if ("unspecified".equals(codeString)) 728 return DeviceMetricCalibrationState.UNSPECIFIED; 729 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 730 } 731 public Enumeration<DeviceMetricCalibrationState> fromType(PrimitiveType<?> code) throws FHIRException { 732 if (code == null) 733 return null; 734 if (code.isEmpty()) 735 return new Enumeration<DeviceMetricCalibrationState>(this); 736 String codeString = code.asStringValue(); 737 if (codeString == null || "".equals(codeString)) 738 return null; 739 if ("not-calibrated".equals(codeString)) 740 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NOTCALIBRATED); 741 if ("calibration-required".equals(codeString)) 742 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATIONREQUIRED); 743 if ("calibrated".equals(codeString)) 744 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATED); 745 if ("unspecified".equals(codeString)) 746 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.UNSPECIFIED); 747 throw new FHIRException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 748 } 749 public String toCode(DeviceMetricCalibrationState code) { 750 if (code == DeviceMetricCalibrationState.NULL) 751 return null; 752 if (code == DeviceMetricCalibrationState.NOTCALIBRATED) 753 return "not-calibrated"; 754 if (code == DeviceMetricCalibrationState.CALIBRATIONREQUIRED) 755 return "calibration-required"; 756 if (code == DeviceMetricCalibrationState.CALIBRATED) 757 return "calibrated"; 758 if (code == DeviceMetricCalibrationState.UNSPECIFIED) 759 return "unspecified"; 760 return "?"; 761 } 762 public String toSystem(DeviceMetricCalibrationState code) { 763 return code.getSystem(); 764 } 765 } 766 767 @Block() 768 public static class DeviceMetricCalibrationComponent extends BackboneElement implements IBaseBackboneElement { 769 /** 770 * Describes the type of the calibration method. 771 */ 772 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 773 @Description(shortDefinition="unspecified | offset | gain | two-point", formalDefinition="Describes the type of the calibration method." ) 774 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-calibration-type") 775 protected Enumeration<DeviceMetricCalibrationType> type; 776 777 /** 778 * Describes the state of the calibration. 779 */ 780 @Child(name = "state", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 781 @Description(shortDefinition="not-calibrated | calibration-required | calibrated | unspecified", formalDefinition="Describes the state of the calibration." ) 782 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-calibration-state") 783 protected Enumeration<DeviceMetricCalibrationState> state; 784 785 /** 786 * Describes the time last calibration has been performed. 787 */ 788 @Child(name = "time", type = {InstantType.class}, order=3, min=0, max=1, modifier=false, summary=true) 789 @Description(shortDefinition="Describes the time last calibration has been performed", formalDefinition="Describes the time last calibration has been performed." ) 790 protected InstantType time; 791 792 private static final long serialVersionUID = 1163986578L; 793 794 /** 795 * Constructor 796 */ 797 public DeviceMetricCalibrationComponent() { 798 super(); 799 } 800 801 /** 802 * @return {@link #type} (Describes the type of the calibration method.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 803 */ 804 public Enumeration<DeviceMetricCalibrationType> getTypeElement() { 805 if (this.type == null) 806 if (Configuration.errorOnAutoCreate()) 807 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.type"); 808 else if (Configuration.doAutoCreate()) 809 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); // bb 810 return this.type; 811 } 812 813 public boolean hasTypeElement() { 814 return this.type != null && !this.type.isEmpty(); 815 } 816 817 public boolean hasType() { 818 return this.type != null && !this.type.isEmpty(); 819 } 820 821 /** 822 * @param value {@link #type} (Describes the type of the calibration method.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 823 */ 824 public DeviceMetricCalibrationComponent setTypeElement(Enumeration<DeviceMetricCalibrationType> value) { 825 this.type = value; 826 return this; 827 } 828 829 /** 830 * @return Describes the type of the calibration method. 831 */ 832 public DeviceMetricCalibrationType getType() { 833 return this.type == null ? null : this.type.getValue(); 834 } 835 836 /** 837 * @param value Describes the type of the calibration method. 838 */ 839 public DeviceMetricCalibrationComponent setType(DeviceMetricCalibrationType value) { 840 if (value == null) 841 this.type = null; 842 else { 843 if (this.type == null) 844 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); 845 this.type.setValue(value); 846 } 847 return this; 848 } 849 850 /** 851 * @return {@link #state} (Describes the state of the calibration.). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 852 */ 853 public Enumeration<DeviceMetricCalibrationState> getStateElement() { 854 if (this.state == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.state"); 857 else if (Configuration.doAutoCreate()) 858 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); // bb 859 return this.state; 860 } 861 862 public boolean hasStateElement() { 863 return this.state != null && !this.state.isEmpty(); 864 } 865 866 public boolean hasState() { 867 return this.state != null && !this.state.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #state} (Describes the state of the calibration.). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 872 */ 873 public DeviceMetricCalibrationComponent setStateElement(Enumeration<DeviceMetricCalibrationState> value) { 874 this.state = value; 875 return this; 876 } 877 878 /** 879 * @return Describes the state of the calibration. 880 */ 881 public DeviceMetricCalibrationState getState() { 882 return this.state == null ? null : this.state.getValue(); 883 } 884 885 /** 886 * @param value Describes the state of the calibration. 887 */ 888 public DeviceMetricCalibrationComponent setState(DeviceMetricCalibrationState value) { 889 if (value == null) 890 this.state = null; 891 else { 892 if (this.state == null) 893 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); 894 this.state.setValue(value); 895 } 896 return this; 897 } 898 899 /** 900 * @return {@link #time} (Describes the time last calibration has been performed.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 901 */ 902 public InstantType getTimeElement() { 903 if (this.time == null) 904 if (Configuration.errorOnAutoCreate()) 905 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.time"); 906 else if (Configuration.doAutoCreate()) 907 this.time = new InstantType(); // bb 908 return this.time; 909 } 910 911 public boolean hasTimeElement() { 912 return this.time != null && !this.time.isEmpty(); 913 } 914 915 public boolean hasTime() { 916 return this.time != null && !this.time.isEmpty(); 917 } 918 919 /** 920 * @param value {@link #time} (Describes the time last calibration has been performed.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 921 */ 922 public DeviceMetricCalibrationComponent setTimeElement(InstantType value) { 923 this.time = value; 924 return this; 925 } 926 927 /** 928 * @return Describes the time last calibration has been performed. 929 */ 930 public Date getTime() { 931 return this.time == null ? null : this.time.getValue(); 932 } 933 934 /** 935 * @param value Describes the time last calibration has been performed. 936 */ 937 public DeviceMetricCalibrationComponent setTime(Date value) { 938 if (value == null) 939 this.time = null; 940 else { 941 if (this.time == null) 942 this.time = new InstantType(); 943 this.time.setValue(value); 944 } 945 return this; 946 } 947 948 protected void listChildren(List<Property> children) { 949 super.listChildren(children); 950 children.add(new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type)); 951 children.add(new Property("state", "code", "Describes the state of the calibration.", 0, 1, state)); 952 children.add(new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time)); 953 } 954 955 @Override 956 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 957 switch (_hash) { 958 case 3575610: /*type*/ return new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type); 959 case 109757585: /*state*/ return new Property("state", "code", "Describes the state of the calibration.", 0, 1, state); 960 case 3560141: /*time*/ return new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time); 961 default: return super.getNamedProperty(_hash, _name, _checkValid); 962 } 963 964 } 965 966 @Override 967 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 968 switch (hash) { 969 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DeviceMetricCalibrationType> 970 case 109757585: /*state*/ return this.state == null ? new Base[0] : new Base[] {this.state}; // Enumeration<DeviceMetricCalibrationState> 971 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // InstantType 972 default: return super.getProperty(hash, name, checkValid); 973 } 974 975 } 976 977 @Override 978 public Base setProperty(int hash, String name, Base value) throws FHIRException { 979 switch (hash) { 980 case 3575610: // type 981 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(castToCode(value)); 982 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 983 return value; 984 case 109757585: // state 985 value = new DeviceMetricCalibrationStateEnumFactory().fromType(castToCode(value)); 986 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 987 return value; 988 case 3560141: // time 989 this.time = castToInstant(value); // InstantType 990 return value; 991 default: return super.setProperty(hash, name, value); 992 } 993 994 } 995 996 @Override 997 public Base setProperty(String name, Base value) throws FHIRException { 998 if (name.equals("type")) { 999 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(castToCode(value)); 1000 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 1001 } else if (name.equals("state")) { 1002 value = new DeviceMetricCalibrationStateEnumFactory().fromType(castToCode(value)); 1003 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 1004 } else if (name.equals("time")) { 1005 this.time = castToInstant(value); // InstantType 1006 } else 1007 return super.setProperty(name, value); 1008 return value; 1009 } 1010 1011 @Override 1012 public Base makeProperty(int hash, String name) throws FHIRException { 1013 switch (hash) { 1014 case 3575610: return getTypeElement(); 1015 case 109757585: return getStateElement(); 1016 case 3560141: return getTimeElement(); 1017 default: return super.makeProperty(hash, name); 1018 } 1019 1020 } 1021 1022 @Override 1023 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1024 switch (hash) { 1025 case 3575610: /*type*/ return new String[] {"code"}; 1026 case 109757585: /*state*/ return new String[] {"code"}; 1027 case 3560141: /*time*/ return new String[] {"instant"}; 1028 default: return super.getTypesForProperty(hash, name); 1029 } 1030 1031 } 1032 1033 @Override 1034 public Base addChild(String name) throws FHIRException { 1035 if (name.equals("type")) { 1036 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.type"); 1037 } 1038 else if (name.equals("state")) { 1039 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.state"); 1040 } 1041 else if (name.equals("time")) { 1042 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.time"); 1043 } 1044 else 1045 return super.addChild(name); 1046 } 1047 1048 public DeviceMetricCalibrationComponent copy() { 1049 DeviceMetricCalibrationComponent dst = new DeviceMetricCalibrationComponent(); 1050 copyValues(dst); 1051 dst.type = type == null ? null : type.copy(); 1052 dst.state = state == null ? null : state.copy(); 1053 dst.time = time == null ? null : time.copy(); 1054 return dst; 1055 } 1056 1057 @Override 1058 public boolean equalsDeep(Base other_) { 1059 if (!super.equalsDeep(other_)) 1060 return false; 1061 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 1062 return false; 1063 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 1064 return compareDeep(type, o.type, true) && compareDeep(state, o.state, true) && compareDeep(time, o.time, true) 1065 ; 1066 } 1067 1068 @Override 1069 public boolean equalsShallow(Base other_) { 1070 if (!super.equalsShallow(other_)) 1071 return false; 1072 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 1073 return false; 1074 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 1075 return compareValues(type, o.type, true) && compareValues(state, o.state, true) && compareValues(time, o.time, true) 1076 ; 1077 } 1078 1079 public boolean isEmpty() { 1080 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, state, time); 1081 } 1082 1083 public String fhirType() { 1084 return "DeviceMetric.calibration"; 1085 1086 } 1087 1088 } 1089 1090 /** 1091 * Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device. 1092 */ 1093 @Child(name = "identifier", type = {Identifier.class}, order=0, min=1, max=1, modifier=false, summary=true) 1094 @Description(shortDefinition="Unique identifier of this DeviceMetric", formalDefinition="Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device." ) 1095 protected Identifier identifier; 1096 1097 /** 1098 * Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc. 1099 */ 1100 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1101 @Description(shortDefinition="Identity of metric, for example Heart Rate or PEEP Setting", formalDefinition="Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc." ) 1102 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicemetric-type") 1103 protected CodeableConcept type; 1104 1105 /** 1106 * Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc. 1107 */ 1108 @Child(name = "unit", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1109 @Description(shortDefinition="Unit of Measure for the Metric", formalDefinition="Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc." ) 1110 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicemetric-type") 1111 protected CodeableConcept unit; 1112 1113 /** 1114 * Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc. 1115 */ 1116 @Child(name = "source", type = {Device.class}, order=3, min=0, max=1, modifier=false, summary=true) 1117 @Description(shortDefinition="Describes the link to the source Device", formalDefinition="Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc." ) 1118 protected Reference source; 1119 1120 /** 1121 * The actual object that is the target of the reference (Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.) 1122 */ 1123 protected Device sourceTarget; 1124 1125 /** 1126 * Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location. 1127 */ 1128 @Child(name = "parent", type = {DeviceComponent.class}, order=4, min=0, max=1, modifier=false, summary=true) 1129 @Description(shortDefinition="Describes the link to the parent DeviceComponent", formalDefinition="Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location." ) 1130 protected Reference parent; 1131 1132 /** 1133 * The actual object that is the target of the reference (Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.) 1134 */ 1135 protected DeviceComponent parentTarget; 1136 1137 /** 1138 * Indicates current operational state of the device. For example: On, Off, Standby, etc. 1139 */ 1140 @Child(name = "operationalStatus", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1141 @Description(shortDefinition="on | off | standby | entered-in-error", formalDefinition="Indicates current operational state of the device. For example: On, Off, Standby, etc." ) 1142 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-operational-status") 1143 protected Enumeration<DeviceMetricOperationalStatus> operationalStatus; 1144 1145 /** 1146 * Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta. 1147 */ 1148 @Child(name = "color", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 1149 @Description(shortDefinition="black | red | green | yellow | blue | magenta | cyan | white", formalDefinition="Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta." ) 1150 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-color") 1151 protected Enumeration<DeviceMetricColor> color; 1152 1153 /** 1154 * Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 1155 */ 1156 @Child(name = "category", type = {CodeType.class}, order=7, min=1, max=1, modifier=false, summary=true) 1157 @Description(shortDefinition="measurement | setting | calculation | unspecified", formalDefinition="Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation." ) 1158 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-category") 1159 protected Enumeration<DeviceMetricCategory> category; 1160 1161 /** 1162 * Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured. 1163 */ 1164 @Child(name = "measurementPeriod", type = {Timing.class}, order=8, min=0, max=1, modifier=false, summary=true) 1165 @Description(shortDefinition="Describes the measurement repetition time", formalDefinition="Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured." ) 1166 protected Timing measurementPeriod; 1167 1168 /** 1169 * Describes the calibrations that have been performed or that are required to be performed. 1170 */ 1171 @Child(name = "calibration", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1172 @Description(shortDefinition="Describes the calibrations that have been performed or that are required to be performed", formalDefinition="Describes the calibrations that have been performed or that are required to be performed." ) 1173 protected List<DeviceMetricCalibrationComponent> calibration; 1174 1175 private static final long serialVersionUID = -380567474L; 1176 1177 /** 1178 * Constructor 1179 */ 1180 public DeviceMetric() { 1181 super(); 1182 } 1183 1184 /** 1185 * Constructor 1186 */ 1187 public DeviceMetric(Identifier identifier, CodeableConcept type, Enumeration<DeviceMetricCategory> category) { 1188 super(); 1189 this.identifier = identifier; 1190 this.type = type; 1191 this.category = category; 1192 } 1193 1194 /** 1195 * @return {@link #identifier} (Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device.) 1196 */ 1197 public Identifier getIdentifier() { 1198 if (this.identifier == null) 1199 if (Configuration.errorOnAutoCreate()) 1200 throw new Error("Attempt to auto-create DeviceMetric.identifier"); 1201 else if (Configuration.doAutoCreate()) 1202 this.identifier = new Identifier(); // cc 1203 return this.identifier; 1204 } 1205 1206 public boolean hasIdentifier() { 1207 return this.identifier != null && !this.identifier.isEmpty(); 1208 } 1209 1210 /** 1211 * @param value {@link #identifier} (Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device.) 1212 */ 1213 public DeviceMetric setIdentifier(Identifier value) { 1214 this.identifier = value; 1215 return this; 1216 } 1217 1218 /** 1219 * @return {@link #type} (Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.) 1220 */ 1221 public CodeableConcept getType() { 1222 if (this.type == null) 1223 if (Configuration.errorOnAutoCreate()) 1224 throw new Error("Attempt to auto-create DeviceMetric.type"); 1225 else if (Configuration.doAutoCreate()) 1226 this.type = new CodeableConcept(); // cc 1227 return this.type; 1228 } 1229 1230 public boolean hasType() { 1231 return this.type != null && !this.type.isEmpty(); 1232 } 1233 1234 /** 1235 * @param value {@link #type} (Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.) 1236 */ 1237 public DeviceMetric setType(CodeableConcept value) { 1238 this.type = value; 1239 return this; 1240 } 1241 1242 /** 1243 * @return {@link #unit} (Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.) 1244 */ 1245 public CodeableConcept getUnit() { 1246 if (this.unit == null) 1247 if (Configuration.errorOnAutoCreate()) 1248 throw new Error("Attempt to auto-create DeviceMetric.unit"); 1249 else if (Configuration.doAutoCreate()) 1250 this.unit = new CodeableConcept(); // cc 1251 return this.unit; 1252 } 1253 1254 public boolean hasUnit() { 1255 return this.unit != null && !this.unit.isEmpty(); 1256 } 1257 1258 /** 1259 * @param value {@link #unit} (Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.) 1260 */ 1261 public DeviceMetric setUnit(CodeableConcept value) { 1262 this.unit = value; 1263 return this; 1264 } 1265 1266 /** 1267 * @return {@link #source} (Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.) 1268 */ 1269 public Reference getSource() { 1270 if (this.source == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create DeviceMetric.source"); 1273 else if (Configuration.doAutoCreate()) 1274 this.source = new Reference(); // cc 1275 return this.source; 1276 } 1277 1278 public boolean hasSource() { 1279 return this.source != null && !this.source.isEmpty(); 1280 } 1281 1282 /** 1283 * @param value {@link #source} (Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.) 1284 */ 1285 public DeviceMetric setSource(Reference value) { 1286 this.source = value; 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #source} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.) 1292 */ 1293 public Device getSourceTarget() { 1294 if (this.sourceTarget == null) 1295 if (Configuration.errorOnAutoCreate()) 1296 throw new Error("Attempt to auto-create DeviceMetric.source"); 1297 else if (Configuration.doAutoCreate()) 1298 this.sourceTarget = new Device(); // aa 1299 return this.sourceTarget; 1300 } 1301 1302 /** 1303 * @param value {@link #source} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.) 1304 */ 1305 public DeviceMetric setSourceTarget(Device value) { 1306 this.sourceTarget = value; 1307 return this; 1308 } 1309 1310 /** 1311 * @return {@link #parent} (Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.) 1312 */ 1313 public Reference getParent() { 1314 if (this.parent == null) 1315 if (Configuration.errorOnAutoCreate()) 1316 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1317 else if (Configuration.doAutoCreate()) 1318 this.parent = new Reference(); // cc 1319 return this.parent; 1320 } 1321 1322 public boolean hasParent() { 1323 return this.parent != null && !this.parent.isEmpty(); 1324 } 1325 1326 /** 1327 * @param value {@link #parent} (Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.) 1328 */ 1329 public DeviceMetric setParent(Reference value) { 1330 this.parent = value; 1331 return this; 1332 } 1333 1334 /** 1335 * @return {@link #parent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.) 1336 */ 1337 public DeviceComponent getParentTarget() { 1338 if (this.parentTarget == null) 1339 if (Configuration.errorOnAutoCreate()) 1340 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1341 else if (Configuration.doAutoCreate()) 1342 this.parentTarget = new DeviceComponent(); // aa 1343 return this.parentTarget; 1344 } 1345 1346 /** 1347 * @param value {@link #parent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.) 1348 */ 1349 public DeviceMetric setParentTarget(DeviceComponent value) { 1350 this.parentTarget = value; 1351 return this; 1352 } 1353 1354 /** 1355 * @return {@link #operationalStatus} (Indicates current operational state of the device. For example: On, Off, Standby, etc.). This is the underlying object with id, value and extensions. The accessor "getOperationalStatus" gives direct access to the value 1356 */ 1357 public Enumeration<DeviceMetricOperationalStatus> getOperationalStatusElement() { 1358 if (this.operationalStatus == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create DeviceMetric.operationalStatus"); 1361 else if (Configuration.doAutoCreate()) 1362 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>(new DeviceMetricOperationalStatusEnumFactory()); // bb 1363 return this.operationalStatus; 1364 } 1365 1366 public boolean hasOperationalStatusElement() { 1367 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1368 } 1369 1370 public boolean hasOperationalStatus() { 1371 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1372 } 1373 1374 /** 1375 * @param value {@link #operationalStatus} (Indicates current operational state of the device. For example: On, Off, Standby, etc.). This is the underlying object with id, value and extensions. The accessor "getOperationalStatus" gives direct access to the value 1376 */ 1377 public DeviceMetric setOperationalStatusElement(Enumeration<DeviceMetricOperationalStatus> value) { 1378 this.operationalStatus = value; 1379 return this; 1380 } 1381 1382 /** 1383 * @return Indicates current operational state of the device. For example: On, Off, Standby, etc. 1384 */ 1385 public DeviceMetricOperationalStatus getOperationalStatus() { 1386 return this.operationalStatus == null ? null : this.operationalStatus.getValue(); 1387 } 1388 1389 /** 1390 * @param value Indicates current operational state of the device. For example: On, Off, Standby, etc. 1391 */ 1392 public DeviceMetric setOperationalStatus(DeviceMetricOperationalStatus value) { 1393 if (value == null) 1394 this.operationalStatus = null; 1395 else { 1396 if (this.operationalStatus == null) 1397 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>(new DeviceMetricOperationalStatusEnumFactory()); 1398 this.operationalStatus.setValue(value); 1399 } 1400 return this; 1401 } 1402 1403 /** 1404 * @return {@link #color} (Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1405 */ 1406 public Enumeration<DeviceMetricColor> getColorElement() { 1407 if (this.color == null) 1408 if (Configuration.errorOnAutoCreate()) 1409 throw new Error("Attempt to auto-create DeviceMetric.color"); 1410 else if (Configuration.doAutoCreate()) 1411 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); // bb 1412 return this.color; 1413 } 1414 1415 public boolean hasColorElement() { 1416 return this.color != null && !this.color.isEmpty(); 1417 } 1418 1419 public boolean hasColor() { 1420 return this.color != null && !this.color.isEmpty(); 1421 } 1422 1423 /** 1424 * @param value {@link #color} (Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1425 */ 1426 public DeviceMetric setColorElement(Enumeration<DeviceMetricColor> value) { 1427 this.color = value; 1428 return this; 1429 } 1430 1431 /** 1432 * @return Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta. 1433 */ 1434 public DeviceMetricColor getColor() { 1435 return this.color == null ? null : this.color.getValue(); 1436 } 1437 1438 /** 1439 * @param value Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta. 1440 */ 1441 public DeviceMetric setColor(DeviceMetricColor value) { 1442 if (value == null) 1443 this.color = null; 1444 else { 1445 if (this.color == null) 1446 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); 1447 this.color.setValue(value); 1448 } 1449 return this; 1450 } 1451 1452 /** 1453 * @return {@link #category} (Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1454 */ 1455 public Enumeration<DeviceMetricCategory> getCategoryElement() { 1456 if (this.category == null) 1457 if (Configuration.errorOnAutoCreate()) 1458 throw new Error("Attempt to auto-create DeviceMetric.category"); 1459 else if (Configuration.doAutoCreate()) 1460 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); // bb 1461 return this.category; 1462 } 1463 1464 public boolean hasCategoryElement() { 1465 return this.category != null && !this.category.isEmpty(); 1466 } 1467 1468 public boolean hasCategory() { 1469 return this.category != null && !this.category.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #category} (Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1474 */ 1475 public DeviceMetric setCategoryElement(Enumeration<DeviceMetricCategory> value) { 1476 this.category = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 1482 */ 1483 public DeviceMetricCategory getCategory() { 1484 return this.category == null ? null : this.category.getValue(); 1485 } 1486 1487 /** 1488 * @param value Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 1489 */ 1490 public DeviceMetric setCategory(DeviceMetricCategory value) { 1491 if (this.category == null) 1492 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); 1493 this.category.setValue(value); 1494 return this; 1495 } 1496 1497 /** 1498 * @return {@link #measurementPeriod} (Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.) 1499 */ 1500 public Timing getMeasurementPeriod() { 1501 if (this.measurementPeriod == null) 1502 if (Configuration.errorOnAutoCreate()) 1503 throw new Error("Attempt to auto-create DeviceMetric.measurementPeriod"); 1504 else if (Configuration.doAutoCreate()) 1505 this.measurementPeriod = new Timing(); // cc 1506 return this.measurementPeriod; 1507 } 1508 1509 public boolean hasMeasurementPeriod() { 1510 return this.measurementPeriod != null && !this.measurementPeriod.isEmpty(); 1511 } 1512 1513 /** 1514 * @param value {@link #measurementPeriod} (Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.) 1515 */ 1516 public DeviceMetric setMeasurementPeriod(Timing value) { 1517 this.measurementPeriod = value; 1518 return this; 1519 } 1520 1521 /** 1522 * @return {@link #calibration} (Describes the calibrations that have been performed or that are required to be performed.) 1523 */ 1524 public List<DeviceMetricCalibrationComponent> getCalibration() { 1525 if (this.calibration == null) 1526 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1527 return this.calibration; 1528 } 1529 1530 /** 1531 * @return Returns a reference to <code>this</code> for easy method chaining 1532 */ 1533 public DeviceMetric setCalibration(List<DeviceMetricCalibrationComponent> theCalibration) { 1534 this.calibration = theCalibration; 1535 return this; 1536 } 1537 1538 public boolean hasCalibration() { 1539 if (this.calibration == null) 1540 return false; 1541 for (DeviceMetricCalibrationComponent item : this.calibration) 1542 if (!item.isEmpty()) 1543 return true; 1544 return false; 1545 } 1546 1547 public DeviceMetricCalibrationComponent addCalibration() { //3 1548 DeviceMetricCalibrationComponent t = new DeviceMetricCalibrationComponent(); 1549 if (this.calibration == null) 1550 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1551 this.calibration.add(t); 1552 return t; 1553 } 1554 1555 public DeviceMetric addCalibration(DeviceMetricCalibrationComponent t) { //3 1556 if (t == null) 1557 return this; 1558 if (this.calibration == null) 1559 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1560 this.calibration.add(t); 1561 return this; 1562 } 1563 1564 /** 1565 * @return The first repetition of repeating field {@link #calibration}, creating it if it does not already exist 1566 */ 1567 public DeviceMetricCalibrationComponent getCalibrationFirstRep() { 1568 if (getCalibration().isEmpty()) { 1569 addCalibration(); 1570 } 1571 return getCalibration().get(0); 1572 } 1573 1574 protected void listChildren(List<Property> children) { 1575 super.listChildren(children); 1576 children.add(new Property("identifier", "Identifier", "Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device.", 0, 1, identifier)); 1577 children.add(new Property("type", "CodeableConcept", "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type)); 1578 children.add(new Property("unit", "CodeableConcept", "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 0, 1, unit)); 1579 children.add(new Property("source", "Reference(Device)", "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.", 0, 1, source)); 1580 children.add(new Property("parent", "Reference(DeviceComponent)", "Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.", 0, 1, parent)); 1581 children.add(new Property("operationalStatus", "code", "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, operationalStatus)); 1582 children.add(new Property("color", "code", "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.", 0, 1, color)); 1583 children.add(new Property("category", "code", "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 0, 1, category)); 1584 children.add(new Property("measurementPeriod", "Timing", "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.", 0, 1, measurementPeriod)); 1585 children.add(new Property("calibration", "", "Describes the calibrations that have been performed or that are required to be performed.", 0, java.lang.Integer.MAX_VALUE, calibration)); 1586 } 1587 1588 @Override 1589 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1590 switch (_hash) { 1591 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device.", 0, 1, identifier); 1592 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type); 1593 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 0, 1, unit); 1594 case -896505829: /*source*/ return new Property("source", "Reference(Device)", "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.", 0, 1, source); 1595 case -995424086: /*parent*/ return new Property("parent", "Reference(DeviceComponent)", "Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.", 0, 1, parent); 1596 case -2103166364: /*operationalStatus*/ return new Property("operationalStatus", "code", "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, operationalStatus); 1597 case 94842723: /*color*/ return new Property("color", "code", "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.", 0, 1, color); 1598 case 50511102: /*category*/ return new Property("category", "code", "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 0, 1, category); 1599 case -1300332387: /*measurementPeriod*/ return new Property("measurementPeriod", "Timing", "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.", 0, 1, measurementPeriod); 1600 case 1421318634: /*calibration*/ return new Property("calibration", "", "Describes the calibrations that have been performed or that are required to be performed.", 0, java.lang.Integer.MAX_VALUE, calibration); 1601 default: return super.getNamedProperty(_hash, _name, _checkValid); 1602 } 1603 1604 } 1605 1606 @Override 1607 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1608 switch (hash) { 1609 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1610 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1611 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 1612 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1613 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // Reference 1614 case -2103166364: /*operationalStatus*/ return this.operationalStatus == null ? new Base[0] : new Base[] {this.operationalStatus}; // Enumeration<DeviceMetricOperationalStatus> 1615 case 94842723: /*color*/ return this.color == null ? new Base[0] : new Base[] {this.color}; // Enumeration<DeviceMetricColor> 1616 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<DeviceMetricCategory> 1617 case -1300332387: /*measurementPeriod*/ return this.measurementPeriod == null ? new Base[0] : new Base[] {this.measurementPeriod}; // Timing 1618 case 1421318634: /*calibration*/ return this.calibration == null ? new Base[0] : this.calibration.toArray(new Base[this.calibration.size()]); // DeviceMetricCalibrationComponent 1619 default: return super.getProperty(hash, name, checkValid); 1620 } 1621 1622 } 1623 1624 @Override 1625 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1626 switch (hash) { 1627 case -1618432855: // identifier 1628 this.identifier = castToIdentifier(value); // Identifier 1629 return value; 1630 case 3575610: // type 1631 this.type = castToCodeableConcept(value); // CodeableConcept 1632 return value; 1633 case 3594628: // unit 1634 this.unit = castToCodeableConcept(value); // CodeableConcept 1635 return value; 1636 case -896505829: // source 1637 this.source = castToReference(value); // Reference 1638 return value; 1639 case -995424086: // parent 1640 this.parent = castToReference(value); // Reference 1641 return value; 1642 case -2103166364: // operationalStatus 1643 value = new DeviceMetricOperationalStatusEnumFactory().fromType(castToCode(value)); 1644 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1645 return value; 1646 case 94842723: // color 1647 value = new DeviceMetricColorEnumFactory().fromType(castToCode(value)); 1648 this.color = (Enumeration) value; // Enumeration<DeviceMetricColor> 1649 return value; 1650 case 50511102: // category 1651 value = new DeviceMetricCategoryEnumFactory().fromType(castToCode(value)); 1652 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1653 return value; 1654 case -1300332387: // measurementPeriod 1655 this.measurementPeriod = castToTiming(value); // Timing 1656 return value; 1657 case 1421318634: // calibration 1658 this.getCalibration().add((DeviceMetricCalibrationComponent) value); // DeviceMetricCalibrationComponent 1659 return value; 1660 default: return super.setProperty(hash, name, value); 1661 } 1662 1663 } 1664 1665 @Override 1666 public Base setProperty(String name, Base value) throws FHIRException { 1667 if (name.equals("identifier")) { 1668 this.identifier = castToIdentifier(value); // Identifier 1669 } else if (name.equals("type")) { 1670 this.type = castToCodeableConcept(value); // CodeableConcept 1671 } else if (name.equals("unit")) { 1672 this.unit = castToCodeableConcept(value); // CodeableConcept 1673 } else if (name.equals("source")) { 1674 this.source = castToReference(value); // Reference 1675 } else if (name.equals("parent")) { 1676 this.parent = castToReference(value); // Reference 1677 } else if (name.equals("operationalStatus")) { 1678 value = new DeviceMetricOperationalStatusEnumFactory().fromType(castToCode(value)); 1679 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1680 } else if (name.equals("color")) { 1681 value = new DeviceMetricColorEnumFactory().fromType(castToCode(value)); 1682 this.color = (Enumeration) value; // Enumeration<DeviceMetricColor> 1683 } else if (name.equals("category")) { 1684 value = new DeviceMetricCategoryEnumFactory().fromType(castToCode(value)); 1685 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1686 } else if (name.equals("measurementPeriod")) { 1687 this.measurementPeriod = castToTiming(value); // Timing 1688 } else if (name.equals("calibration")) { 1689 this.getCalibration().add((DeviceMetricCalibrationComponent) value); 1690 } else 1691 return super.setProperty(name, value); 1692 return value; 1693 } 1694 1695 @Override 1696 public Base makeProperty(int hash, String name) throws FHIRException { 1697 switch (hash) { 1698 case -1618432855: return getIdentifier(); 1699 case 3575610: return getType(); 1700 case 3594628: return getUnit(); 1701 case -896505829: return getSource(); 1702 case -995424086: return getParent(); 1703 case -2103166364: return getOperationalStatusElement(); 1704 case 94842723: return getColorElement(); 1705 case 50511102: return getCategoryElement(); 1706 case -1300332387: return getMeasurementPeriod(); 1707 case 1421318634: return addCalibration(); 1708 default: return super.makeProperty(hash, name); 1709 } 1710 1711 } 1712 1713 @Override 1714 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1715 switch (hash) { 1716 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1717 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1718 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 1719 case -896505829: /*source*/ return new String[] {"Reference"}; 1720 case -995424086: /*parent*/ return new String[] {"Reference"}; 1721 case -2103166364: /*operationalStatus*/ return new String[] {"code"}; 1722 case 94842723: /*color*/ return new String[] {"code"}; 1723 case 50511102: /*category*/ return new String[] {"code"}; 1724 case -1300332387: /*measurementPeriod*/ return new String[] {"Timing"}; 1725 case 1421318634: /*calibration*/ return new String[] {}; 1726 default: return super.getTypesForProperty(hash, name); 1727 } 1728 1729 } 1730 1731 @Override 1732 public Base addChild(String name) throws FHIRException { 1733 if (name.equals("identifier")) { 1734 this.identifier = new Identifier(); 1735 return this.identifier; 1736 } 1737 else if (name.equals("type")) { 1738 this.type = new CodeableConcept(); 1739 return this.type; 1740 } 1741 else if (name.equals("unit")) { 1742 this.unit = new CodeableConcept(); 1743 return this.unit; 1744 } 1745 else if (name.equals("source")) { 1746 this.source = new Reference(); 1747 return this.source; 1748 } 1749 else if (name.equals("parent")) { 1750 this.parent = new Reference(); 1751 return this.parent; 1752 } 1753 else if (name.equals("operationalStatus")) { 1754 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.operationalStatus"); 1755 } 1756 else if (name.equals("color")) { 1757 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.color"); 1758 } 1759 else if (name.equals("category")) { 1760 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.category"); 1761 } 1762 else if (name.equals("measurementPeriod")) { 1763 this.measurementPeriod = new Timing(); 1764 return this.measurementPeriod; 1765 } 1766 else if (name.equals("calibration")) { 1767 return addCalibration(); 1768 } 1769 else 1770 return super.addChild(name); 1771 } 1772 1773 public String fhirType() { 1774 return "DeviceMetric"; 1775 1776 } 1777 1778 public DeviceMetric copy() { 1779 DeviceMetric dst = new DeviceMetric(); 1780 copyValues(dst); 1781 dst.identifier = identifier == null ? null : identifier.copy(); 1782 dst.type = type == null ? null : type.copy(); 1783 dst.unit = unit == null ? null : unit.copy(); 1784 dst.source = source == null ? null : source.copy(); 1785 dst.parent = parent == null ? null : parent.copy(); 1786 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 1787 dst.color = color == null ? null : color.copy(); 1788 dst.category = category == null ? null : category.copy(); 1789 dst.measurementPeriod = measurementPeriod == null ? null : measurementPeriod.copy(); 1790 if (calibration != null) { 1791 dst.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1792 for (DeviceMetricCalibrationComponent i : calibration) 1793 dst.calibration.add(i.copy()); 1794 }; 1795 return dst; 1796 } 1797 1798 protected DeviceMetric typedCopy() { 1799 return copy(); 1800 } 1801 1802 @Override 1803 public boolean equalsDeep(Base other_) { 1804 if (!super.equalsDeep(other_)) 1805 return false; 1806 if (!(other_ instanceof DeviceMetric)) 1807 return false; 1808 DeviceMetric o = (DeviceMetric) other_; 1809 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(unit, o.unit, true) 1810 && compareDeep(source, o.source, true) && compareDeep(parent, o.parent, true) && compareDeep(operationalStatus, o.operationalStatus, true) 1811 && compareDeep(color, o.color, true) && compareDeep(category, o.category, true) && compareDeep(measurementPeriod, o.measurementPeriod, true) 1812 && compareDeep(calibration, o.calibration, true); 1813 } 1814 1815 @Override 1816 public boolean equalsShallow(Base other_) { 1817 if (!super.equalsShallow(other_)) 1818 return false; 1819 if (!(other_ instanceof DeviceMetric)) 1820 return false; 1821 DeviceMetric o = (DeviceMetric) other_; 1822 return compareValues(operationalStatus, o.operationalStatus, true) && compareValues(color, o.color, true) 1823 && compareValues(category, o.category, true); 1824 } 1825 1826 public boolean isEmpty() { 1827 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, unit, source 1828 , parent, operationalStatus, color, category, measurementPeriod, calibration); 1829 } 1830 1831 @Override 1832 public ResourceType getResourceType() { 1833 return ResourceType.DeviceMetric; 1834 } 1835 1836 /** 1837 * Search parameter: <b>parent</b> 1838 * <p> 1839 * Description: <b>The parent DeviceMetric resource</b><br> 1840 * Type: <b>reference</b><br> 1841 * Path: <b>DeviceMetric.parent</b><br> 1842 * </p> 1843 */ 1844 @SearchParamDefinition(name="parent", path="DeviceMetric.parent", description="The parent DeviceMetric resource", type="reference", target={DeviceComponent.class } ) 1845 public static final String SP_PARENT = "parent"; 1846 /** 1847 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 1848 * <p> 1849 * Description: <b>The parent DeviceMetric resource</b><br> 1850 * Type: <b>reference</b><br> 1851 * Path: <b>DeviceMetric.parent</b><br> 1852 * </p> 1853 */ 1854 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 1855 1856/** 1857 * Constant for fluent queries to be used to add include statements. Specifies 1858 * the path value of "<b>DeviceMetric:parent</b>". 1859 */ 1860 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("DeviceMetric:parent").toLocked(); 1861 1862 /** 1863 * Search parameter: <b>identifier</b> 1864 * <p> 1865 * Description: <b>The identifier of the metric</b><br> 1866 * Type: <b>token</b><br> 1867 * Path: <b>DeviceMetric.identifier</b><br> 1868 * </p> 1869 */ 1870 @SearchParamDefinition(name="identifier", path="DeviceMetric.identifier", description="The identifier of the metric", type="token" ) 1871 public static final String SP_IDENTIFIER = "identifier"; 1872 /** 1873 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1874 * <p> 1875 * Description: <b>The identifier of the metric</b><br> 1876 * Type: <b>token</b><br> 1877 * Path: <b>DeviceMetric.identifier</b><br> 1878 * </p> 1879 */ 1880 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1881 1882 /** 1883 * Search parameter: <b>source</b> 1884 * <p> 1885 * Description: <b>The device resource</b><br> 1886 * Type: <b>reference</b><br> 1887 * Path: <b>DeviceMetric.source</b><br> 1888 * </p> 1889 */ 1890 @SearchParamDefinition(name="source", path="DeviceMetric.source", description="The device resource", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class } ) 1891 public static final String SP_SOURCE = "source"; 1892 /** 1893 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1894 * <p> 1895 * Description: <b>The device resource</b><br> 1896 * Type: <b>reference</b><br> 1897 * Path: <b>DeviceMetric.source</b><br> 1898 * </p> 1899 */ 1900 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 1901 1902/** 1903 * Constant for fluent queries to be used to add include statements. Specifies 1904 * the path value of "<b>DeviceMetric:source</b>". 1905 */ 1906 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("DeviceMetric:source").toLocked(); 1907 1908 /** 1909 * Search parameter: <b>type</b> 1910 * <p> 1911 * Description: <b>The component type</b><br> 1912 * Type: <b>token</b><br> 1913 * Path: <b>DeviceMetric.type</b><br> 1914 * </p> 1915 */ 1916 @SearchParamDefinition(name="type", path="DeviceMetric.type", description="The component type", type="token" ) 1917 public static final String SP_TYPE = "type"; 1918 /** 1919 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1920 * <p> 1921 * Description: <b>The component type</b><br> 1922 * Type: <b>token</b><br> 1923 * Path: <b>DeviceMetric.type</b><br> 1924 * </p> 1925 */ 1926 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1927 1928 /** 1929 * Search parameter: <b>category</b> 1930 * <p> 1931 * Description: <b>The category of the metric</b><br> 1932 * Type: <b>token</b><br> 1933 * Path: <b>DeviceMetric.category</b><br> 1934 * </p> 1935 */ 1936 @SearchParamDefinition(name="category", path="DeviceMetric.category", description="The category of the metric", type="token" ) 1937 public static final String SP_CATEGORY = "category"; 1938 /** 1939 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1940 * <p> 1941 * Description: <b>The category of the metric</b><br> 1942 * Type: <b>token</b><br> 1943 * Path: <b>DeviceMetric.category</b><br> 1944 * </p> 1945 */ 1946 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1947 1948 1949}