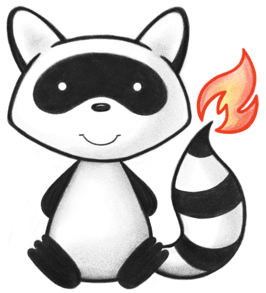
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 050 */ 051@ResourceDef(name="DeviceRequest", profile="http://hl7.org/fhir/Profile/DeviceRequest") 052public class DeviceRequest extends DomainResource { 053 054 public enum DeviceRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action 057 */ 058 DRAFT, 059 /** 060 * The request is ready to be acted upon 061 */ 062 ACTIVE, 063 /** 064 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 065 */ 066 SUSPENDED, 067 /** 068 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 069 */ 070 CANCELLED, 071 /** 072 * Activity against the request has been sufficiently completed to the satisfaction of the requester 073 */ 074 COMPLETED, 075 /** 076 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static DeviceRequestStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("draft".equals(codeString)) 091 return DRAFT; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("cancelled".equals(codeString)) 097 return CANCELLED; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown DeviceRequestStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case DRAFT: return "draft"; 112 case ACTIVE: return "active"; 113 case SUSPENDED: return "suspended"; 114 case CANCELLED: return "cancelled"; 115 case COMPLETED: return "completed"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case DRAFT: return "http://hl7.org/fhir/request-status"; 125 case ACTIVE: return "http://hl7.org/fhir/request-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 127 case CANCELLED: return "http://hl7.org/fhir/request-status"; 128 case COMPLETED: return "http://hl7.org/fhir/request-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 138 case ACTIVE: return "The request is ready to be acted upon"; 139 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 140 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 141 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 142 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 143 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case DRAFT: return "Draft"; 151 case ACTIVE: return "Active"; 152 case SUSPENDED: return "Suspended"; 153 case CANCELLED: return "Cancelled"; 154 case COMPLETED: return "Completed"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class DeviceRequestStatusEnumFactory implements EnumFactory<DeviceRequestStatus> { 164 public DeviceRequestStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("draft".equals(codeString)) 169 return DeviceRequestStatus.DRAFT; 170 if ("active".equals(codeString)) 171 return DeviceRequestStatus.ACTIVE; 172 if ("suspended".equals(codeString)) 173 return DeviceRequestStatus.SUSPENDED; 174 if ("cancelled".equals(codeString)) 175 return DeviceRequestStatus.CANCELLED; 176 if ("completed".equals(codeString)) 177 return DeviceRequestStatus.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return DeviceRequestStatus.ENTEREDINERROR; 180 if ("unknown".equals(codeString)) 181 return DeviceRequestStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown DeviceRequestStatus code '"+codeString+"'"); 183 } 184 public Enumeration<DeviceRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<DeviceRequestStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("draft".equals(codeString)) 193 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.DRAFT); 194 if ("active".equals(codeString)) 195 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.ACTIVE); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.SUSPENDED); 198 if ("cancelled".equals(codeString)) 199 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.CANCELLED); 200 if ("completed".equals(codeString)) 201 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.COMPLETED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.ENTEREDINERROR); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.UNKNOWN); 206 throw new FHIRException("Unknown DeviceRequestStatus code '"+codeString+"'"); 207 } 208 public String toCode(DeviceRequestStatus code) { 209 if (code == DeviceRequestStatus.DRAFT) 210 return "draft"; 211 if (code == DeviceRequestStatus.ACTIVE) 212 return "active"; 213 if (code == DeviceRequestStatus.SUSPENDED) 214 return "suspended"; 215 if (code == DeviceRequestStatus.CANCELLED) 216 return "cancelled"; 217 if (code == DeviceRequestStatus.COMPLETED) 218 return "completed"; 219 if (code == DeviceRequestStatus.ENTEREDINERROR) 220 return "entered-in-error"; 221 if (code == DeviceRequestStatus.UNKNOWN) 222 return "unknown"; 223 return "?"; 224 } 225 public String toSystem(DeviceRequestStatus code) { 226 return code.getSystem(); 227 } 228 } 229 230 public enum RequestPriority { 231 /** 232 * The request has normal priority 233 */ 234 ROUTINE, 235 /** 236 * The request should be actioned promptly - higher priority than routine 237 */ 238 URGENT, 239 /** 240 * The request should be actioned as soon as possible - higher priority than urgent 241 */ 242 ASAP, 243 /** 244 * The request should be actioned immediately - highest possible priority. E.g. an emergency 245 */ 246 STAT, 247 /** 248 * added to help the parsers with the generic types 249 */ 250 NULL; 251 public static RequestPriority fromCode(String codeString) throws FHIRException { 252 if (codeString == null || "".equals(codeString)) 253 return null; 254 if ("routine".equals(codeString)) 255 return ROUTINE; 256 if ("urgent".equals(codeString)) 257 return URGENT; 258 if ("asap".equals(codeString)) 259 return ASAP; 260 if ("stat".equals(codeString)) 261 return STAT; 262 if (Configuration.isAcceptInvalidEnums()) 263 return null; 264 else 265 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 266 } 267 public String toCode() { 268 switch (this) { 269 case ROUTINE: return "routine"; 270 case URGENT: return "urgent"; 271 case ASAP: return "asap"; 272 case STAT: return "stat"; 273 case NULL: return null; 274 default: return "?"; 275 } 276 } 277 public String getSystem() { 278 switch (this) { 279 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 280 case URGENT: return "http://hl7.org/fhir/request-priority"; 281 case ASAP: return "http://hl7.org/fhir/request-priority"; 282 case STAT: return "http://hl7.org/fhir/request-priority"; 283 case NULL: return null; 284 default: return "?"; 285 } 286 } 287 public String getDefinition() { 288 switch (this) { 289 case ROUTINE: return "The request has normal priority"; 290 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 291 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 292 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 293 case NULL: return null; 294 default: return "?"; 295 } 296 } 297 public String getDisplay() { 298 switch (this) { 299 case ROUTINE: return "Routine"; 300 case URGENT: return "Urgent"; 301 case ASAP: return "ASAP"; 302 case STAT: return "STAT"; 303 case NULL: return null; 304 default: return "?"; 305 } 306 } 307 } 308 309 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 310 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 311 if (codeString == null || "".equals(codeString)) 312 if (codeString == null || "".equals(codeString)) 313 return null; 314 if ("routine".equals(codeString)) 315 return RequestPriority.ROUTINE; 316 if ("urgent".equals(codeString)) 317 return RequestPriority.URGENT; 318 if ("asap".equals(codeString)) 319 return RequestPriority.ASAP; 320 if ("stat".equals(codeString)) 321 return RequestPriority.STAT; 322 throw new IllegalArgumentException("Unknown RequestPriority code '"+codeString+"'"); 323 } 324 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 325 if (code == null) 326 return null; 327 if (code.isEmpty()) 328 return new Enumeration<RequestPriority>(this); 329 String codeString = code.asStringValue(); 330 if (codeString == null || "".equals(codeString)) 331 return null; 332 if ("routine".equals(codeString)) 333 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE); 334 if ("urgent".equals(codeString)) 335 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT); 336 if ("asap".equals(codeString)) 337 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP); 338 if ("stat".equals(codeString)) 339 return new Enumeration<RequestPriority>(this, RequestPriority.STAT); 340 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 341 } 342 public String toCode(RequestPriority code) { 343 if (code == RequestPriority.ROUTINE) 344 return "routine"; 345 if (code == RequestPriority.URGENT) 346 return "urgent"; 347 if (code == RequestPriority.ASAP) 348 return "asap"; 349 if (code == RequestPriority.STAT) 350 return "stat"; 351 return "?"; 352 } 353 public String toSystem(RequestPriority code) { 354 return code.getSystem(); 355 } 356 } 357 358 @Block() 359 public static class DeviceRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 360 /** 361 * The device, practitioner, etc. who initiated the request. 362 */ 363 @Child(name = "agent", type = {Device.class, Practitioner.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=true) 364 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner, etc. who initiated the request." ) 365 protected Reference agent; 366 367 /** 368 * The actual object that is the target of the reference (The device, practitioner, etc. who initiated the request.) 369 */ 370 protected Resource agentTarget; 371 372 /** 373 * The organization the device or practitioner was acting on behalf of. 374 */ 375 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 376 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 377 protected Reference onBehalfOf; 378 379 /** 380 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 381 */ 382 protected Organization onBehalfOfTarget; 383 384 private static final long serialVersionUID = -71453027L; 385 386 /** 387 * Constructor 388 */ 389 public DeviceRequestRequesterComponent() { 390 super(); 391 } 392 393 /** 394 * Constructor 395 */ 396 public DeviceRequestRequesterComponent(Reference agent) { 397 super(); 398 this.agent = agent; 399 } 400 401 /** 402 * @return {@link #agent} (The device, practitioner, etc. who initiated the request.) 403 */ 404 public Reference getAgent() { 405 if (this.agent == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create DeviceRequestRequesterComponent.agent"); 408 else if (Configuration.doAutoCreate()) 409 this.agent = new Reference(); // cc 410 return this.agent; 411 } 412 413 public boolean hasAgent() { 414 return this.agent != null && !this.agent.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #agent} (The device, practitioner, etc. who initiated the request.) 419 */ 420 public DeviceRequestRequesterComponent setAgent(Reference value) { 421 this.agent = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 427 */ 428 public Resource getAgentTarget() { 429 return this.agentTarget; 430 } 431 432 /** 433 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 434 */ 435 public DeviceRequestRequesterComponent setAgentTarget(Resource value) { 436 this.agentTarget = value; 437 return this; 438 } 439 440 /** 441 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 442 */ 443 public Reference getOnBehalfOf() { 444 if (this.onBehalfOf == null) 445 if (Configuration.errorOnAutoCreate()) 446 throw new Error("Attempt to auto-create DeviceRequestRequesterComponent.onBehalfOf"); 447 else if (Configuration.doAutoCreate()) 448 this.onBehalfOf = new Reference(); // cc 449 return this.onBehalfOf; 450 } 451 452 public boolean hasOnBehalfOf() { 453 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 454 } 455 456 /** 457 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 458 */ 459 public DeviceRequestRequesterComponent setOnBehalfOf(Reference value) { 460 this.onBehalfOf = value; 461 return this; 462 } 463 464 /** 465 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 466 */ 467 public Organization getOnBehalfOfTarget() { 468 if (this.onBehalfOfTarget == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create DeviceRequestRequesterComponent.onBehalfOf"); 471 else if (Configuration.doAutoCreate()) 472 this.onBehalfOfTarget = new Organization(); // aa 473 return this.onBehalfOfTarget; 474 } 475 476 /** 477 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 478 */ 479 public DeviceRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 480 this.onBehalfOfTarget = value; 481 return this; 482 } 483 484 protected void listChildren(List<Property> children) { 485 super.listChildren(children); 486 children.add(new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent)); 487 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 488 } 489 490 @Override 491 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 492 switch (_hash) { 493 case 92750597: /*agent*/ return new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent); 494 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 495 default: return super.getNamedProperty(_hash, _name, _checkValid); 496 } 497 498 } 499 500 @Override 501 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 502 switch (hash) { 503 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 504 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 505 default: return super.getProperty(hash, name, checkValid); 506 } 507 508 } 509 510 @Override 511 public Base setProperty(int hash, String name, Base value) throws FHIRException { 512 switch (hash) { 513 case 92750597: // agent 514 this.agent = castToReference(value); // Reference 515 return value; 516 case -14402964: // onBehalfOf 517 this.onBehalfOf = castToReference(value); // Reference 518 return value; 519 default: return super.setProperty(hash, name, value); 520 } 521 522 } 523 524 @Override 525 public Base setProperty(String name, Base value) throws FHIRException { 526 if (name.equals("agent")) { 527 this.agent = castToReference(value); // Reference 528 } else if (name.equals("onBehalfOf")) { 529 this.onBehalfOf = castToReference(value); // Reference 530 } else 531 return super.setProperty(name, value); 532 return value; 533 } 534 535 @Override 536 public Base makeProperty(int hash, String name) throws FHIRException { 537 switch (hash) { 538 case 92750597: return getAgent(); 539 case -14402964: return getOnBehalfOf(); 540 default: return super.makeProperty(hash, name); 541 } 542 543 } 544 545 @Override 546 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 547 switch (hash) { 548 case 92750597: /*agent*/ return new String[] {"Reference"}; 549 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 550 default: return super.getTypesForProperty(hash, name); 551 } 552 553 } 554 555 @Override 556 public Base addChild(String name) throws FHIRException { 557 if (name.equals("agent")) { 558 this.agent = new Reference(); 559 return this.agent; 560 } 561 else if (name.equals("onBehalfOf")) { 562 this.onBehalfOf = new Reference(); 563 return this.onBehalfOf; 564 } 565 else 566 return super.addChild(name); 567 } 568 569 public DeviceRequestRequesterComponent copy() { 570 DeviceRequestRequesterComponent dst = new DeviceRequestRequesterComponent(); 571 copyValues(dst); 572 dst.agent = agent == null ? null : agent.copy(); 573 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 574 return dst; 575 } 576 577 @Override 578 public boolean equalsDeep(Base other_) { 579 if (!super.equalsDeep(other_)) 580 return false; 581 if (!(other_ instanceof DeviceRequestRequesterComponent)) 582 return false; 583 DeviceRequestRequesterComponent o = (DeviceRequestRequesterComponent) other_; 584 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 585 } 586 587 @Override 588 public boolean equalsShallow(Base other_) { 589 if (!super.equalsShallow(other_)) 590 return false; 591 if (!(other_ instanceof DeviceRequestRequesterComponent)) 592 return false; 593 DeviceRequestRequesterComponent o = (DeviceRequestRequesterComponent) other_; 594 return true; 595 } 596 597 public boolean isEmpty() { 598 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 599 } 600 601 public String fhirType() { 602 return "DeviceRequest.requester"; 603 604 } 605 606 } 607 608 /** 609 * Identifiers assigned to this order by the orderer or by the receiver. 610 */ 611 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 612 @Description(shortDefinition="External Request identifier", formalDefinition="Identifiers assigned to this order by the orderer or by the receiver." ) 613 protected List<Identifier> identifier; 614 615 /** 616 * Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%. 617 */ 618 @Child(name = "definition", type = {ActivityDefinition.class, PlanDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 619 @Description(shortDefinition="Protocol or definition", formalDefinition="Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%." ) 620 protected List<Reference> definition; 621 /** 622 * The actual objects that are the target of the reference (Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.) 623 */ 624 protected List<Resource> definitionTarget; 625 626 627 /** 628 * Plan/proposal/order fulfilled by this request. 629 */ 630 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 631 @Description(shortDefinition="What request fulfills", formalDefinition="Plan/proposal/order fulfilled by this request." ) 632 protected List<Reference> basedOn; 633 /** 634 * The actual objects that are the target of the reference (Plan/proposal/order fulfilled by this request.) 635 */ 636 protected List<Resource> basedOnTarget; 637 638 639 /** 640 * The request takes the place of the referenced completed or terminated request(s). 641 */ 642 @Child(name = "priorRequest", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 643 @Description(shortDefinition="What request replaces", formalDefinition="The request takes the place of the referenced completed or terminated request(s)." ) 644 protected List<Reference> priorRequest; 645 /** 646 * The actual objects that are the target of the reference (The request takes the place of the referenced completed or terminated request(s).) 647 */ 648 protected List<Resource> priorRequestTarget; 649 650 651 /** 652 * Composite request this is part of. 653 */ 654 @Child(name = "groupIdentifier", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 655 @Description(shortDefinition="Identifier of composite request", formalDefinition="Composite request this is part of." ) 656 protected Identifier groupIdentifier; 657 658 /** 659 * The status of the request. 660 */ 661 @Child(name = "status", type = {CodeType.class}, order=5, min=0, max=1, modifier=true, summary=true) 662 @Description(shortDefinition="draft | active | suspended | completed | entered-in-error | cancelled", formalDefinition="The status of the request." ) 663 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 664 protected Enumeration<DeviceRequestStatus> status; 665 666 /** 667 * Whether the request is a proposal, plan, an original order or a reflex order. 668 */ 669 @Child(name = "intent", type = {CodeableConcept.class}, order=6, min=1, max=1, modifier=true, summary=true) 670 @Description(shortDefinition="proposal | plan | original-order | encoded | reflex-order", formalDefinition="Whether the request is a proposal, plan, an original order or a reflex order." ) 671 protected CodeableConcept intent; 672 673 /** 674 * Indicates how quickly the {{title}} should be addressed with respect to other requests. 675 */ 676 @Child(name = "priority", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 677 @Description(shortDefinition="Indicates how quickly the {{title}} should be addressed with respect to other requests", formalDefinition="Indicates how quickly the {{title}} should be addressed with respect to other requests." ) 678 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 679 protected Enumeration<RequestPriority> priority; 680 681 /** 682 * The details of the device to be used. 683 */ 684 @Child(name = "code", type = {Device.class, CodeableConcept.class}, order=8, min=1, max=1, modifier=false, summary=true) 685 @Description(shortDefinition="Device requested", formalDefinition="The details of the device to be used." ) 686 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-kind") 687 protected Type code; 688 689 /** 690 * The patient who will use the device. 691 */ 692 @Child(name = "subject", type = {Patient.class, Group.class, Location.class, Device.class}, order=9, min=1, max=1, modifier=false, summary=true) 693 @Description(shortDefinition="Focus of request", formalDefinition="The patient who will use the device." ) 694 protected Reference subject; 695 696 /** 697 * The actual object that is the target of the reference (The patient who will use the device.) 698 */ 699 protected Resource subjectTarget; 700 701 /** 702 * An encounter that provides additional context in which this request is made. 703 */ 704 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=10, min=0, max=1, modifier=false, summary=true) 705 @Description(shortDefinition="Encounter or Episode motivating request", formalDefinition="An encounter that provides additional context in which this request is made." ) 706 protected Reference context; 707 708 /** 709 * The actual object that is the target of the reference (An encounter that provides additional context in which this request is made.) 710 */ 711 protected Resource contextTarget; 712 713 /** 714 * The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013". 715 */ 716 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=11, min=0, max=1, modifier=false, summary=true) 717 @Description(shortDefinition="Desired time or schedule for use", formalDefinition="The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\"." ) 718 protected Type occurrence; 719 720 /** 721 * When the request transitioned to being actionable. 722 */ 723 @Child(name = "authoredOn", type = {DateTimeType.class}, order=12, min=0, max=1, modifier=false, summary=true) 724 @Description(shortDefinition="When recorded", formalDefinition="When the request transitioned to being actionable." ) 725 protected DateTimeType authoredOn; 726 727 /** 728 * The individual who initiated the request and has responsibility for its activation. 729 */ 730 @Child(name = "requester", type = {}, order=13, min=0, max=1, modifier=false, summary=true) 731 @Description(shortDefinition="Who/what is requesting diagnostics", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 732 protected DeviceRequestRequesterComponent requester; 733 734 /** 735 * Desired type of performer for doing the diagnostic testing. 736 */ 737 @Child(name = "performerType", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=true) 738 @Description(shortDefinition="Fille role", formalDefinition="Desired type of performer for doing the diagnostic testing." ) 739 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 740 protected CodeableConcept performerType; 741 742 /** 743 * The desired perfomer for doing the diagnostic testing. 744 */ 745 @Child(name = "performer", type = {Practitioner.class, Organization.class, Patient.class, Device.class, RelatedPerson.class, HealthcareService.class}, order=15, min=0, max=1, modifier=false, summary=true) 746 @Description(shortDefinition="Requested Filler", formalDefinition="The desired perfomer for doing the diagnostic testing." ) 747 protected Reference performer; 748 749 /** 750 * The actual object that is the target of the reference (The desired perfomer for doing the diagnostic testing.) 751 */ 752 protected Resource performerTarget; 753 754 /** 755 * Reason or justification for the use of this device. 756 */ 757 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 758 @Description(shortDefinition="Coded Reason for request", formalDefinition="Reason or justification for the use of this device." ) 759 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 760 protected List<CodeableConcept> reasonCode; 761 762 /** 763 * Reason or justification for the use of this device. 764 */ 765 @Child(name = "reasonReference", type = {Reference.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 766 @Description(shortDefinition="Linked Reason for request", formalDefinition="Reason or justification for the use of this device." ) 767 protected List<Reference> reasonReference; 768 /** 769 * The actual objects that are the target of the reference (Reason or justification for the use of this device.) 770 */ 771 protected List<Resource> reasonReferenceTarget; 772 773 774 /** 775 * Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site). 776 */ 777 @Child(name = "supportingInfo", type = {Reference.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 778 @Description(shortDefinition="Additional clinical information", formalDefinition="Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site)." ) 779 protected List<Reference> supportingInfo; 780 /** 781 * The actual objects that are the target of the reference (Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).) 782 */ 783 protected List<Resource> supportingInfoTarget; 784 785 786 /** 787 * Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement. 788 */ 789 @Child(name = "note", type = {Annotation.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 790 @Description(shortDefinition="Notes or comments", formalDefinition="Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement." ) 791 protected List<Annotation> note; 792 793 /** 794 * Key events in the history of the request. 795 */ 796 @Child(name = "relevantHistory", type = {Provenance.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 797 @Description(shortDefinition="Request provenance", formalDefinition="Key events in the history of the request." ) 798 protected List<Reference> relevantHistory; 799 /** 800 * The actual objects that are the target of the reference (Key events in the history of the request.) 801 */ 802 protected List<Provenance> relevantHistoryTarget; 803 804 805 private static final long serialVersionUID = -2002514925L; 806 807 /** 808 * Constructor 809 */ 810 public DeviceRequest() { 811 super(); 812 } 813 814 /** 815 * Constructor 816 */ 817 public DeviceRequest(CodeableConcept intent, Type code, Reference subject) { 818 super(); 819 this.intent = intent; 820 this.code = code; 821 this.subject = subject; 822 } 823 824 /** 825 * @return {@link #identifier} (Identifiers assigned to this order by the orderer or by the receiver.) 826 */ 827 public List<Identifier> getIdentifier() { 828 if (this.identifier == null) 829 this.identifier = new ArrayList<Identifier>(); 830 return this.identifier; 831 } 832 833 /** 834 * @return Returns a reference to <code>this</code> for easy method chaining 835 */ 836 public DeviceRequest setIdentifier(List<Identifier> theIdentifier) { 837 this.identifier = theIdentifier; 838 return this; 839 } 840 841 public boolean hasIdentifier() { 842 if (this.identifier == null) 843 return false; 844 for (Identifier item : this.identifier) 845 if (!item.isEmpty()) 846 return true; 847 return false; 848 } 849 850 public Identifier addIdentifier() { //3 851 Identifier t = new Identifier(); 852 if (this.identifier == null) 853 this.identifier = new ArrayList<Identifier>(); 854 this.identifier.add(t); 855 return t; 856 } 857 858 public DeviceRequest addIdentifier(Identifier t) { //3 859 if (t == null) 860 return this; 861 if (this.identifier == null) 862 this.identifier = new ArrayList<Identifier>(); 863 this.identifier.add(t); 864 return this; 865 } 866 867 /** 868 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 869 */ 870 public Identifier getIdentifierFirstRep() { 871 if (getIdentifier().isEmpty()) { 872 addIdentifier(); 873 } 874 return getIdentifier().get(0); 875 } 876 877 /** 878 * @return {@link #definition} (Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.) 879 */ 880 public List<Reference> getDefinition() { 881 if (this.definition == null) 882 this.definition = new ArrayList<Reference>(); 883 return this.definition; 884 } 885 886 /** 887 * @return Returns a reference to <code>this</code> for easy method chaining 888 */ 889 public DeviceRequest setDefinition(List<Reference> theDefinition) { 890 this.definition = theDefinition; 891 return this; 892 } 893 894 public boolean hasDefinition() { 895 if (this.definition == null) 896 return false; 897 for (Reference item : this.definition) 898 if (!item.isEmpty()) 899 return true; 900 return false; 901 } 902 903 public Reference addDefinition() { //3 904 Reference t = new Reference(); 905 if (this.definition == null) 906 this.definition = new ArrayList<Reference>(); 907 this.definition.add(t); 908 return t; 909 } 910 911 public DeviceRequest addDefinition(Reference t) { //3 912 if (t == null) 913 return this; 914 if (this.definition == null) 915 this.definition = new ArrayList<Reference>(); 916 this.definition.add(t); 917 return this; 918 } 919 920 /** 921 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 922 */ 923 public Reference getDefinitionFirstRep() { 924 if (getDefinition().isEmpty()) { 925 addDefinition(); 926 } 927 return getDefinition().get(0); 928 } 929 930 /** 931 * @deprecated Use Reference#setResource(IBaseResource) instead 932 */ 933 @Deprecated 934 public List<Resource> getDefinitionTarget() { 935 if (this.definitionTarget == null) 936 this.definitionTarget = new ArrayList<Resource>(); 937 return this.definitionTarget; 938 } 939 940 /** 941 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 942 */ 943 public List<Reference> getBasedOn() { 944 if (this.basedOn == null) 945 this.basedOn = new ArrayList<Reference>(); 946 return this.basedOn; 947 } 948 949 /** 950 * @return Returns a reference to <code>this</code> for easy method chaining 951 */ 952 public DeviceRequest setBasedOn(List<Reference> theBasedOn) { 953 this.basedOn = theBasedOn; 954 return this; 955 } 956 957 public boolean hasBasedOn() { 958 if (this.basedOn == null) 959 return false; 960 for (Reference item : this.basedOn) 961 if (!item.isEmpty()) 962 return true; 963 return false; 964 } 965 966 public Reference addBasedOn() { //3 967 Reference t = new Reference(); 968 if (this.basedOn == null) 969 this.basedOn = new ArrayList<Reference>(); 970 this.basedOn.add(t); 971 return t; 972 } 973 974 public DeviceRequest addBasedOn(Reference t) { //3 975 if (t == null) 976 return this; 977 if (this.basedOn == null) 978 this.basedOn = new ArrayList<Reference>(); 979 this.basedOn.add(t); 980 return this; 981 } 982 983 /** 984 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 985 */ 986 public Reference getBasedOnFirstRep() { 987 if (getBasedOn().isEmpty()) { 988 addBasedOn(); 989 } 990 return getBasedOn().get(0); 991 } 992 993 /** 994 * @deprecated Use Reference#setResource(IBaseResource) instead 995 */ 996 @Deprecated 997 public List<Resource> getBasedOnTarget() { 998 if (this.basedOnTarget == null) 999 this.basedOnTarget = new ArrayList<Resource>(); 1000 return this.basedOnTarget; 1001 } 1002 1003 /** 1004 * @return {@link #priorRequest} (The request takes the place of the referenced completed or terminated request(s).) 1005 */ 1006 public List<Reference> getPriorRequest() { 1007 if (this.priorRequest == null) 1008 this.priorRequest = new ArrayList<Reference>(); 1009 return this.priorRequest; 1010 } 1011 1012 /** 1013 * @return Returns a reference to <code>this</code> for easy method chaining 1014 */ 1015 public DeviceRequest setPriorRequest(List<Reference> thePriorRequest) { 1016 this.priorRequest = thePriorRequest; 1017 return this; 1018 } 1019 1020 public boolean hasPriorRequest() { 1021 if (this.priorRequest == null) 1022 return false; 1023 for (Reference item : this.priorRequest) 1024 if (!item.isEmpty()) 1025 return true; 1026 return false; 1027 } 1028 1029 public Reference addPriorRequest() { //3 1030 Reference t = new Reference(); 1031 if (this.priorRequest == null) 1032 this.priorRequest = new ArrayList<Reference>(); 1033 this.priorRequest.add(t); 1034 return t; 1035 } 1036 1037 public DeviceRequest addPriorRequest(Reference t) { //3 1038 if (t == null) 1039 return this; 1040 if (this.priorRequest == null) 1041 this.priorRequest = new ArrayList<Reference>(); 1042 this.priorRequest.add(t); 1043 return this; 1044 } 1045 1046 /** 1047 * @return The first repetition of repeating field {@link #priorRequest}, creating it if it does not already exist 1048 */ 1049 public Reference getPriorRequestFirstRep() { 1050 if (getPriorRequest().isEmpty()) { 1051 addPriorRequest(); 1052 } 1053 return getPriorRequest().get(0); 1054 } 1055 1056 /** 1057 * @deprecated Use Reference#setResource(IBaseResource) instead 1058 */ 1059 @Deprecated 1060 public List<Resource> getPriorRequestTarget() { 1061 if (this.priorRequestTarget == null) 1062 this.priorRequestTarget = new ArrayList<Resource>(); 1063 return this.priorRequestTarget; 1064 } 1065 1066 /** 1067 * @return {@link #groupIdentifier} (Composite request this is part of.) 1068 */ 1069 public Identifier getGroupIdentifier() { 1070 if (this.groupIdentifier == null) 1071 if (Configuration.errorOnAutoCreate()) 1072 throw new Error("Attempt to auto-create DeviceRequest.groupIdentifier"); 1073 else if (Configuration.doAutoCreate()) 1074 this.groupIdentifier = new Identifier(); // cc 1075 return this.groupIdentifier; 1076 } 1077 1078 public boolean hasGroupIdentifier() { 1079 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1080 } 1081 1082 /** 1083 * @param value {@link #groupIdentifier} (Composite request this is part of.) 1084 */ 1085 public DeviceRequest setGroupIdentifier(Identifier value) { 1086 this.groupIdentifier = value; 1087 return this; 1088 } 1089 1090 /** 1091 * @return {@link #status} (The status of the request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1092 */ 1093 public Enumeration<DeviceRequestStatus> getStatusElement() { 1094 if (this.status == null) 1095 if (Configuration.errorOnAutoCreate()) 1096 throw new Error("Attempt to auto-create DeviceRequest.status"); 1097 else if (Configuration.doAutoCreate()) 1098 this.status = new Enumeration<DeviceRequestStatus>(new DeviceRequestStatusEnumFactory()); // bb 1099 return this.status; 1100 } 1101 1102 public boolean hasStatusElement() { 1103 return this.status != null && !this.status.isEmpty(); 1104 } 1105 1106 public boolean hasStatus() { 1107 return this.status != null && !this.status.isEmpty(); 1108 } 1109 1110 /** 1111 * @param value {@link #status} (The status of the request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1112 */ 1113 public DeviceRequest setStatusElement(Enumeration<DeviceRequestStatus> value) { 1114 this.status = value; 1115 return this; 1116 } 1117 1118 /** 1119 * @return The status of the request. 1120 */ 1121 public DeviceRequestStatus getStatus() { 1122 return this.status == null ? null : this.status.getValue(); 1123 } 1124 1125 /** 1126 * @param value The status of the request. 1127 */ 1128 public DeviceRequest setStatus(DeviceRequestStatus value) { 1129 if (value == null) 1130 this.status = null; 1131 else { 1132 if (this.status == null) 1133 this.status = new Enumeration<DeviceRequestStatus>(new DeviceRequestStatusEnumFactory()); 1134 this.status.setValue(value); 1135 } 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.) 1141 */ 1142 public CodeableConcept getIntent() { 1143 if (this.intent == null) 1144 if (Configuration.errorOnAutoCreate()) 1145 throw new Error("Attempt to auto-create DeviceRequest.intent"); 1146 else if (Configuration.doAutoCreate()) 1147 this.intent = new CodeableConcept(); // cc 1148 return this.intent; 1149 } 1150 1151 public boolean hasIntent() { 1152 return this.intent != null && !this.intent.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.) 1157 */ 1158 public DeviceRequest setIntent(CodeableConcept value) { 1159 this.intent = value; 1160 return this; 1161 } 1162 1163 /** 1164 * @return {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1165 */ 1166 public Enumeration<RequestPriority> getPriorityElement() { 1167 if (this.priority == null) 1168 if (Configuration.errorOnAutoCreate()) 1169 throw new Error("Attempt to auto-create DeviceRequest.priority"); 1170 else if (Configuration.doAutoCreate()) 1171 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 1172 return this.priority; 1173 } 1174 1175 public boolean hasPriorityElement() { 1176 return this.priority != null && !this.priority.isEmpty(); 1177 } 1178 1179 public boolean hasPriority() { 1180 return this.priority != null && !this.priority.isEmpty(); 1181 } 1182 1183 /** 1184 * @param value {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1185 */ 1186 public DeviceRequest setPriorityElement(Enumeration<RequestPriority> value) { 1187 this.priority = value; 1188 return this; 1189 } 1190 1191 /** 1192 * @return Indicates how quickly the {{title}} should be addressed with respect to other requests. 1193 */ 1194 public RequestPriority getPriority() { 1195 return this.priority == null ? null : this.priority.getValue(); 1196 } 1197 1198 /** 1199 * @param value Indicates how quickly the {{title}} should be addressed with respect to other requests. 1200 */ 1201 public DeviceRequest setPriority(RequestPriority value) { 1202 if (value == null) 1203 this.priority = null; 1204 else { 1205 if (this.priority == null) 1206 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 1207 this.priority.setValue(value); 1208 } 1209 return this; 1210 } 1211 1212 /** 1213 * @return {@link #code} (The details of the device to be used.) 1214 */ 1215 public Type getCode() { 1216 return this.code; 1217 } 1218 1219 /** 1220 * @return {@link #code} (The details of the device to be used.) 1221 */ 1222 public Reference getCodeReference() throws FHIRException { 1223 if (this.code == null) 1224 return null; 1225 if (!(this.code instanceof Reference)) 1226 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.code.getClass().getName()+" was encountered"); 1227 return (Reference) this.code; 1228 } 1229 1230 public boolean hasCodeReference() { 1231 return this != null && this.code instanceof Reference; 1232 } 1233 1234 /** 1235 * @return {@link #code} (The details of the device to be used.) 1236 */ 1237 public CodeableConcept getCodeCodeableConcept() throws FHIRException { 1238 if (this.code == null) 1239 return null; 1240 if (!(this.code instanceof CodeableConcept)) 1241 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.code.getClass().getName()+" was encountered"); 1242 return (CodeableConcept) this.code; 1243 } 1244 1245 public boolean hasCodeCodeableConcept() { 1246 return this != null && this.code instanceof CodeableConcept; 1247 } 1248 1249 public boolean hasCode() { 1250 return this.code != null && !this.code.isEmpty(); 1251 } 1252 1253 /** 1254 * @param value {@link #code} (The details of the device to be used.) 1255 */ 1256 public DeviceRequest setCode(Type value) throws FHIRFormatError { 1257 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1258 throw new FHIRFormatError("Not the right type for DeviceRequest.code[x]: "+value.fhirType()); 1259 this.code = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return {@link #subject} (The patient who will use the device.) 1265 */ 1266 public Reference getSubject() { 1267 if (this.subject == null) 1268 if (Configuration.errorOnAutoCreate()) 1269 throw new Error("Attempt to auto-create DeviceRequest.subject"); 1270 else if (Configuration.doAutoCreate()) 1271 this.subject = new Reference(); // cc 1272 return this.subject; 1273 } 1274 1275 public boolean hasSubject() { 1276 return this.subject != null && !this.subject.isEmpty(); 1277 } 1278 1279 /** 1280 * @param value {@link #subject} (The patient who will use the device.) 1281 */ 1282 public DeviceRequest setSubject(Reference value) { 1283 this.subject = value; 1284 return this; 1285 } 1286 1287 /** 1288 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who will use the device.) 1289 */ 1290 public Resource getSubjectTarget() { 1291 return this.subjectTarget; 1292 } 1293 1294 /** 1295 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who will use the device.) 1296 */ 1297 public DeviceRequest setSubjectTarget(Resource value) { 1298 this.subjectTarget = value; 1299 return this; 1300 } 1301 1302 /** 1303 * @return {@link #context} (An encounter that provides additional context in which this request is made.) 1304 */ 1305 public Reference getContext() { 1306 if (this.context == null) 1307 if (Configuration.errorOnAutoCreate()) 1308 throw new Error("Attempt to auto-create DeviceRequest.context"); 1309 else if (Configuration.doAutoCreate()) 1310 this.context = new Reference(); // cc 1311 return this.context; 1312 } 1313 1314 public boolean hasContext() { 1315 return this.context != null && !this.context.isEmpty(); 1316 } 1317 1318 /** 1319 * @param value {@link #context} (An encounter that provides additional context in which this request is made.) 1320 */ 1321 public DeviceRequest setContext(Reference value) { 1322 this.context = value; 1323 return this; 1324 } 1325 1326 /** 1327 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An encounter that provides additional context in which this request is made.) 1328 */ 1329 public Resource getContextTarget() { 1330 return this.contextTarget; 1331 } 1332 1333 /** 1334 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An encounter that provides additional context in which this request is made.) 1335 */ 1336 public DeviceRequest setContextTarget(Resource value) { 1337 this.contextTarget = value; 1338 return this; 1339 } 1340 1341 /** 1342 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1343 */ 1344 public Type getOccurrence() { 1345 return this.occurrence; 1346 } 1347 1348 /** 1349 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1350 */ 1351 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1352 if (this.occurrence == null) 1353 return null; 1354 if (!(this.occurrence instanceof DateTimeType)) 1355 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1356 return (DateTimeType) this.occurrence; 1357 } 1358 1359 public boolean hasOccurrenceDateTimeType() { 1360 return this != null && this.occurrence instanceof DateTimeType; 1361 } 1362 1363 /** 1364 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1365 */ 1366 public Period getOccurrencePeriod() throws FHIRException { 1367 if (this.occurrence == null) 1368 return null; 1369 if (!(this.occurrence instanceof Period)) 1370 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1371 return (Period) this.occurrence; 1372 } 1373 1374 public boolean hasOccurrencePeriod() { 1375 return this != null && this.occurrence instanceof Period; 1376 } 1377 1378 /** 1379 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1380 */ 1381 public Timing getOccurrenceTiming() throws FHIRException { 1382 if (this.occurrence == null) 1383 return null; 1384 if (!(this.occurrence instanceof Timing)) 1385 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1386 return (Timing) this.occurrence; 1387 } 1388 1389 public boolean hasOccurrenceTiming() { 1390 return this != null && this.occurrence instanceof Timing; 1391 } 1392 1393 public boolean hasOccurrence() { 1394 return this.occurrence != null && !this.occurrence.isEmpty(); 1395 } 1396 1397 /** 1398 * @param value {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1399 */ 1400 public DeviceRequest setOccurrence(Type value) throws FHIRFormatError { 1401 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1402 throw new FHIRFormatError("Not the right type for DeviceRequest.occurrence[x]: "+value.fhirType()); 1403 this.occurrence = value; 1404 return this; 1405 } 1406 1407 /** 1408 * @return {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1409 */ 1410 public DateTimeType getAuthoredOnElement() { 1411 if (this.authoredOn == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create DeviceRequest.authoredOn"); 1414 else if (Configuration.doAutoCreate()) 1415 this.authoredOn = new DateTimeType(); // bb 1416 return this.authoredOn; 1417 } 1418 1419 public boolean hasAuthoredOnElement() { 1420 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1421 } 1422 1423 public boolean hasAuthoredOn() { 1424 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1425 } 1426 1427 /** 1428 * @param value {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1429 */ 1430 public DeviceRequest setAuthoredOnElement(DateTimeType value) { 1431 this.authoredOn = value; 1432 return this; 1433 } 1434 1435 /** 1436 * @return When the request transitioned to being actionable. 1437 */ 1438 public Date getAuthoredOn() { 1439 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1440 } 1441 1442 /** 1443 * @param value When the request transitioned to being actionable. 1444 */ 1445 public DeviceRequest setAuthoredOn(Date value) { 1446 if (value == null) 1447 this.authoredOn = null; 1448 else { 1449 if (this.authoredOn == null) 1450 this.authoredOn = new DateTimeType(); 1451 this.authoredOn.setValue(value); 1452 } 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1458 */ 1459 public DeviceRequestRequesterComponent getRequester() { 1460 if (this.requester == null) 1461 if (Configuration.errorOnAutoCreate()) 1462 throw new Error("Attempt to auto-create DeviceRequest.requester"); 1463 else if (Configuration.doAutoCreate()) 1464 this.requester = new DeviceRequestRequesterComponent(); // cc 1465 return this.requester; 1466 } 1467 1468 public boolean hasRequester() { 1469 return this.requester != null && !this.requester.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1474 */ 1475 public DeviceRequest setRequester(DeviceRequestRequesterComponent value) { 1476 this.requester = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1482 */ 1483 public CodeableConcept getPerformerType() { 1484 if (this.performerType == null) 1485 if (Configuration.errorOnAutoCreate()) 1486 throw new Error("Attempt to auto-create DeviceRequest.performerType"); 1487 else if (Configuration.doAutoCreate()) 1488 this.performerType = new CodeableConcept(); // cc 1489 return this.performerType; 1490 } 1491 1492 public boolean hasPerformerType() { 1493 return this.performerType != null && !this.performerType.isEmpty(); 1494 } 1495 1496 /** 1497 * @param value {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1498 */ 1499 public DeviceRequest setPerformerType(CodeableConcept value) { 1500 this.performerType = value; 1501 return this; 1502 } 1503 1504 /** 1505 * @return {@link #performer} (The desired perfomer for doing the diagnostic testing.) 1506 */ 1507 public Reference getPerformer() { 1508 if (this.performer == null) 1509 if (Configuration.errorOnAutoCreate()) 1510 throw new Error("Attempt to auto-create DeviceRequest.performer"); 1511 else if (Configuration.doAutoCreate()) 1512 this.performer = new Reference(); // cc 1513 return this.performer; 1514 } 1515 1516 public boolean hasPerformer() { 1517 return this.performer != null && !this.performer.isEmpty(); 1518 } 1519 1520 /** 1521 * @param value {@link #performer} (The desired perfomer for doing the diagnostic testing.) 1522 */ 1523 public DeviceRequest setPerformer(Reference value) { 1524 this.performer = value; 1525 return this; 1526 } 1527 1528 /** 1529 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing.) 1530 */ 1531 public Resource getPerformerTarget() { 1532 return this.performerTarget; 1533 } 1534 1535 /** 1536 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing.) 1537 */ 1538 public DeviceRequest setPerformerTarget(Resource value) { 1539 this.performerTarget = value; 1540 return this; 1541 } 1542 1543 /** 1544 * @return {@link #reasonCode} (Reason or justification for the use of this device.) 1545 */ 1546 public List<CodeableConcept> getReasonCode() { 1547 if (this.reasonCode == null) 1548 this.reasonCode = new ArrayList<CodeableConcept>(); 1549 return this.reasonCode; 1550 } 1551 1552 /** 1553 * @return Returns a reference to <code>this</code> for easy method chaining 1554 */ 1555 public DeviceRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1556 this.reasonCode = theReasonCode; 1557 return this; 1558 } 1559 1560 public boolean hasReasonCode() { 1561 if (this.reasonCode == null) 1562 return false; 1563 for (CodeableConcept item : this.reasonCode) 1564 if (!item.isEmpty()) 1565 return true; 1566 return false; 1567 } 1568 1569 public CodeableConcept addReasonCode() { //3 1570 CodeableConcept t = new CodeableConcept(); 1571 if (this.reasonCode == null) 1572 this.reasonCode = new ArrayList<CodeableConcept>(); 1573 this.reasonCode.add(t); 1574 return t; 1575 } 1576 1577 public DeviceRequest addReasonCode(CodeableConcept t) { //3 1578 if (t == null) 1579 return this; 1580 if (this.reasonCode == null) 1581 this.reasonCode = new ArrayList<CodeableConcept>(); 1582 this.reasonCode.add(t); 1583 return this; 1584 } 1585 1586 /** 1587 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1588 */ 1589 public CodeableConcept getReasonCodeFirstRep() { 1590 if (getReasonCode().isEmpty()) { 1591 addReasonCode(); 1592 } 1593 return getReasonCode().get(0); 1594 } 1595 1596 /** 1597 * @return {@link #reasonReference} (Reason or justification for the use of this device.) 1598 */ 1599 public List<Reference> getReasonReference() { 1600 if (this.reasonReference == null) 1601 this.reasonReference = new ArrayList<Reference>(); 1602 return this.reasonReference; 1603 } 1604 1605 /** 1606 * @return Returns a reference to <code>this</code> for easy method chaining 1607 */ 1608 public DeviceRequest setReasonReference(List<Reference> theReasonReference) { 1609 this.reasonReference = theReasonReference; 1610 return this; 1611 } 1612 1613 public boolean hasReasonReference() { 1614 if (this.reasonReference == null) 1615 return false; 1616 for (Reference item : this.reasonReference) 1617 if (!item.isEmpty()) 1618 return true; 1619 return false; 1620 } 1621 1622 public Reference addReasonReference() { //3 1623 Reference t = new Reference(); 1624 if (this.reasonReference == null) 1625 this.reasonReference = new ArrayList<Reference>(); 1626 this.reasonReference.add(t); 1627 return t; 1628 } 1629 1630 public DeviceRequest addReasonReference(Reference t) { //3 1631 if (t == null) 1632 return this; 1633 if (this.reasonReference == null) 1634 this.reasonReference = new ArrayList<Reference>(); 1635 this.reasonReference.add(t); 1636 return this; 1637 } 1638 1639 /** 1640 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1641 */ 1642 public Reference getReasonReferenceFirstRep() { 1643 if (getReasonReference().isEmpty()) { 1644 addReasonReference(); 1645 } 1646 return getReasonReference().get(0); 1647 } 1648 1649 /** 1650 * @deprecated Use Reference#setResource(IBaseResource) instead 1651 */ 1652 @Deprecated 1653 public List<Resource> getReasonReferenceTarget() { 1654 if (this.reasonReferenceTarget == null) 1655 this.reasonReferenceTarget = new ArrayList<Resource>(); 1656 return this.reasonReferenceTarget; 1657 } 1658 1659 /** 1660 * @return {@link #supportingInfo} (Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).) 1661 */ 1662 public List<Reference> getSupportingInfo() { 1663 if (this.supportingInfo == null) 1664 this.supportingInfo = new ArrayList<Reference>(); 1665 return this.supportingInfo; 1666 } 1667 1668 /** 1669 * @return Returns a reference to <code>this</code> for easy method chaining 1670 */ 1671 public DeviceRequest setSupportingInfo(List<Reference> theSupportingInfo) { 1672 this.supportingInfo = theSupportingInfo; 1673 return this; 1674 } 1675 1676 public boolean hasSupportingInfo() { 1677 if (this.supportingInfo == null) 1678 return false; 1679 for (Reference item : this.supportingInfo) 1680 if (!item.isEmpty()) 1681 return true; 1682 return false; 1683 } 1684 1685 public Reference addSupportingInfo() { //3 1686 Reference t = new Reference(); 1687 if (this.supportingInfo == null) 1688 this.supportingInfo = new ArrayList<Reference>(); 1689 this.supportingInfo.add(t); 1690 return t; 1691 } 1692 1693 public DeviceRequest addSupportingInfo(Reference t) { //3 1694 if (t == null) 1695 return this; 1696 if (this.supportingInfo == null) 1697 this.supportingInfo = new ArrayList<Reference>(); 1698 this.supportingInfo.add(t); 1699 return this; 1700 } 1701 1702 /** 1703 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 1704 */ 1705 public Reference getSupportingInfoFirstRep() { 1706 if (getSupportingInfo().isEmpty()) { 1707 addSupportingInfo(); 1708 } 1709 return getSupportingInfo().get(0); 1710 } 1711 1712 /** 1713 * @deprecated Use Reference#setResource(IBaseResource) instead 1714 */ 1715 @Deprecated 1716 public List<Resource> getSupportingInfoTarget() { 1717 if (this.supportingInfoTarget == null) 1718 this.supportingInfoTarget = new ArrayList<Resource>(); 1719 return this.supportingInfoTarget; 1720 } 1721 1722 /** 1723 * @return {@link #note} (Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.) 1724 */ 1725 public List<Annotation> getNote() { 1726 if (this.note == null) 1727 this.note = new ArrayList<Annotation>(); 1728 return this.note; 1729 } 1730 1731 /** 1732 * @return Returns a reference to <code>this</code> for easy method chaining 1733 */ 1734 public DeviceRequest setNote(List<Annotation> theNote) { 1735 this.note = theNote; 1736 return this; 1737 } 1738 1739 public boolean hasNote() { 1740 if (this.note == null) 1741 return false; 1742 for (Annotation item : this.note) 1743 if (!item.isEmpty()) 1744 return true; 1745 return false; 1746 } 1747 1748 public Annotation addNote() { //3 1749 Annotation t = new Annotation(); 1750 if (this.note == null) 1751 this.note = new ArrayList<Annotation>(); 1752 this.note.add(t); 1753 return t; 1754 } 1755 1756 public DeviceRequest addNote(Annotation t) { //3 1757 if (t == null) 1758 return this; 1759 if (this.note == null) 1760 this.note = new ArrayList<Annotation>(); 1761 this.note.add(t); 1762 return this; 1763 } 1764 1765 /** 1766 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1767 */ 1768 public Annotation getNoteFirstRep() { 1769 if (getNote().isEmpty()) { 1770 addNote(); 1771 } 1772 return getNote().get(0); 1773 } 1774 1775 /** 1776 * @return {@link #relevantHistory} (Key events in the history of the request.) 1777 */ 1778 public List<Reference> getRelevantHistory() { 1779 if (this.relevantHistory == null) 1780 this.relevantHistory = new ArrayList<Reference>(); 1781 return this.relevantHistory; 1782 } 1783 1784 /** 1785 * @return Returns a reference to <code>this</code> for easy method chaining 1786 */ 1787 public DeviceRequest setRelevantHistory(List<Reference> theRelevantHistory) { 1788 this.relevantHistory = theRelevantHistory; 1789 return this; 1790 } 1791 1792 public boolean hasRelevantHistory() { 1793 if (this.relevantHistory == null) 1794 return false; 1795 for (Reference item : this.relevantHistory) 1796 if (!item.isEmpty()) 1797 return true; 1798 return false; 1799 } 1800 1801 public Reference addRelevantHistory() { //3 1802 Reference t = new Reference(); 1803 if (this.relevantHistory == null) 1804 this.relevantHistory = new ArrayList<Reference>(); 1805 this.relevantHistory.add(t); 1806 return t; 1807 } 1808 1809 public DeviceRequest addRelevantHistory(Reference t) { //3 1810 if (t == null) 1811 return this; 1812 if (this.relevantHistory == null) 1813 this.relevantHistory = new ArrayList<Reference>(); 1814 this.relevantHistory.add(t); 1815 return this; 1816 } 1817 1818 /** 1819 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 1820 */ 1821 public Reference getRelevantHistoryFirstRep() { 1822 if (getRelevantHistory().isEmpty()) { 1823 addRelevantHistory(); 1824 } 1825 return getRelevantHistory().get(0); 1826 } 1827 1828 /** 1829 * @deprecated Use Reference#setResource(IBaseResource) instead 1830 */ 1831 @Deprecated 1832 public List<Provenance> getRelevantHistoryTarget() { 1833 if (this.relevantHistoryTarget == null) 1834 this.relevantHistoryTarget = new ArrayList<Provenance>(); 1835 return this.relevantHistoryTarget; 1836 } 1837 1838 /** 1839 * @deprecated Use Reference#setResource(IBaseResource) instead 1840 */ 1841 @Deprecated 1842 public Provenance addRelevantHistoryTarget() { 1843 Provenance r = new Provenance(); 1844 if (this.relevantHistoryTarget == null) 1845 this.relevantHistoryTarget = new ArrayList<Provenance>(); 1846 this.relevantHistoryTarget.add(r); 1847 return r; 1848 } 1849 1850 protected void listChildren(List<Property> children) { 1851 super.listChildren(children); 1852 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1853 children.add(new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.", 0, java.lang.Integer.MAX_VALUE, definition)); 1854 children.add(new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1855 children.add(new Property("priorRequest", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, priorRequest)); 1856 children.add(new Property("groupIdentifier", "Identifier", "Composite request this is part of.", 0, 1, groupIdentifier)); 1857 children.add(new Property("status", "code", "The status of the request.", 0, 1, status)); 1858 children.add(new Property("intent", "CodeableConcept", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 1859 children.add(new Property("priority", "code", "Indicates how quickly the {{title}} should be addressed with respect to other requests.", 0, 1, priority)); 1860 children.add(new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code)); 1861 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", "The patient who will use the device.", 0, 1, subject)); 1862 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter that provides additional context in which this request is made.", 0, 1, context)); 1863 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence)); 1864 children.add(new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 1865 children.add(new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 1866 children.add(new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType)); 1867 children.add(new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing.", 0, 1, performer)); 1868 children.add(new Property("reasonCode", "CodeableConcept", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1869 children.add(new Property("reasonReference", "Reference(Any)", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1870 children.add(new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 1871 children.add(new Property("note", "Annotation", "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note)); 1872 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 1873 } 1874 1875 @Override 1876 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1877 switch (_hash) { 1878 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, identifier); 1879 case -1014418093: /*definition*/ return new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.", 0, java.lang.Integer.MAX_VALUE, definition); 1880 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1881 case 237568101: /*priorRequest*/ return new Property("priorRequest", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, priorRequest); 1882 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "Composite request this is part of.", 0, 1, groupIdentifier); 1883 case -892481550: /*status*/ return new Property("status", "code", "The status of the request.", 0, 1, status); 1884 case -1183762788: /*intent*/ return new Property("intent", "CodeableConcept", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 1885 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the {{title}} should be addressed with respect to other requests.", 0, 1, priority); 1886 case 941839219: /*code[x]*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1887 case 3059181: /*code*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1888 case 1565461470: /*codeReference*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1889 case 4899316: /*codeCodeableConcept*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1890 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Location|Device)", "The patient who will use the device.", 0, 1, subject); 1891 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter that provides additional context in which this request is made.", 0, 1, context); 1892 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1893 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1894 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1895 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1896 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1897 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn); 1898 case 693933948: /*requester*/ return new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 1899 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType); 1900 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing.", 0, 1, performer); 1901 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1902 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Any)", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1903 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 1904 case 3387378: /*note*/ return new Property("note", "Annotation", "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note); 1905 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 1906 default: return super.getNamedProperty(_hash, _name, _checkValid); 1907 } 1908 1909 } 1910 1911 @Override 1912 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1913 switch (hash) { 1914 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1915 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 1916 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1917 case 237568101: /*priorRequest*/ return this.priorRequest == null ? new Base[0] : this.priorRequest.toArray(new Base[this.priorRequest.size()]); // Reference 1918 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 1919 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DeviceRequestStatus> 1920 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // CodeableConcept 1921 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1922 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Type 1923 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1924 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1925 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 1926 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 1927 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // DeviceRequestRequesterComponent 1928 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 1929 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 1930 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1931 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1932 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 1933 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1934 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 1935 default: return super.getProperty(hash, name, checkValid); 1936 } 1937 1938 } 1939 1940 @Override 1941 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1942 switch (hash) { 1943 case -1618432855: // identifier 1944 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1945 return value; 1946 case -1014418093: // definition 1947 this.getDefinition().add(castToReference(value)); // Reference 1948 return value; 1949 case -332612366: // basedOn 1950 this.getBasedOn().add(castToReference(value)); // Reference 1951 return value; 1952 case 237568101: // priorRequest 1953 this.getPriorRequest().add(castToReference(value)); // Reference 1954 return value; 1955 case -445338488: // groupIdentifier 1956 this.groupIdentifier = castToIdentifier(value); // Identifier 1957 return value; 1958 case -892481550: // status 1959 value = new DeviceRequestStatusEnumFactory().fromType(castToCode(value)); 1960 this.status = (Enumeration) value; // Enumeration<DeviceRequestStatus> 1961 return value; 1962 case -1183762788: // intent 1963 this.intent = castToCodeableConcept(value); // CodeableConcept 1964 return value; 1965 case -1165461084: // priority 1966 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1967 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1968 return value; 1969 case 3059181: // code 1970 this.code = castToType(value); // Type 1971 return value; 1972 case -1867885268: // subject 1973 this.subject = castToReference(value); // Reference 1974 return value; 1975 case 951530927: // context 1976 this.context = castToReference(value); // Reference 1977 return value; 1978 case 1687874001: // occurrence 1979 this.occurrence = castToType(value); // Type 1980 return value; 1981 case -1500852503: // authoredOn 1982 this.authoredOn = castToDateTime(value); // DateTimeType 1983 return value; 1984 case 693933948: // requester 1985 this.requester = (DeviceRequestRequesterComponent) value; // DeviceRequestRequesterComponent 1986 return value; 1987 case -901444568: // performerType 1988 this.performerType = castToCodeableConcept(value); // CodeableConcept 1989 return value; 1990 case 481140686: // performer 1991 this.performer = castToReference(value); // Reference 1992 return value; 1993 case 722137681: // reasonCode 1994 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1995 return value; 1996 case -1146218137: // reasonReference 1997 this.getReasonReference().add(castToReference(value)); // Reference 1998 return value; 1999 case 1922406657: // supportingInfo 2000 this.getSupportingInfo().add(castToReference(value)); // Reference 2001 return value; 2002 case 3387378: // note 2003 this.getNote().add(castToAnnotation(value)); // Annotation 2004 return value; 2005 case 1538891575: // relevantHistory 2006 this.getRelevantHistory().add(castToReference(value)); // Reference 2007 return value; 2008 default: return super.setProperty(hash, name, value); 2009 } 2010 2011 } 2012 2013 @Override 2014 public Base setProperty(String name, Base value) throws FHIRException { 2015 if (name.equals("identifier")) { 2016 this.getIdentifier().add(castToIdentifier(value)); 2017 } else if (name.equals("definition")) { 2018 this.getDefinition().add(castToReference(value)); 2019 } else if (name.equals("basedOn")) { 2020 this.getBasedOn().add(castToReference(value)); 2021 } else if (name.equals("priorRequest")) { 2022 this.getPriorRequest().add(castToReference(value)); 2023 } else if (name.equals("groupIdentifier")) { 2024 this.groupIdentifier = castToIdentifier(value); // Identifier 2025 } else if (name.equals("status")) { 2026 value = new DeviceRequestStatusEnumFactory().fromType(castToCode(value)); 2027 this.status = (Enumeration) value; // Enumeration<DeviceRequestStatus> 2028 } else if (name.equals("intent")) { 2029 this.intent = castToCodeableConcept(value); // CodeableConcept 2030 } else if (name.equals("priority")) { 2031 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 2032 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 2033 } else if (name.equals("code[x]")) { 2034 this.code = castToType(value); // Type 2035 } else if (name.equals("subject")) { 2036 this.subject = castToReference(value); // Reference 2037 } else if (name.equals("context")) { 2038 this.context = castToReference(value); // Reference 2039 } else if (name.equals("occurrence[x]")) { 2040 this.occurrence = castToType(value); // Type 2041 } else if (name.equals("authoredOn")) { 2042 this.authoredOn = castToDateTime(value); // DateTimeType 2043 } else if (name.equals("requester")) { 2044 this.requester = (DeviceRequestRequesterComponent) value; // DeviceRequestRequesterComponent 2045 } else if (name.equals("performerType")) { 2046 this.performerType = castToCodeableConcept(value); // CodeableConcept 2047 } else if (name.equals("performer")) { 2048 this.performer = castToReference(value); // Reference 2049 } else if (name.equals("reasonCode")) { 2050 this.getReasonCode().add(castToCodeableConcept(value)); 2051 } else if (name.equals("reasonReference")) { 2052 this.getReasonReference().add(castToReference(value)); 2053 } else if (name.equals("supportingInfo")) { 2054 this.getSupportingInfo().add(castToReference(value)); 2055 } else if (name.equals("note")) { 2056 this.getNote().add(castToAnnotation(value)); 2057 } else if (name.equals("relevantHistory")) { 2058 this.getRelevantHistory().add(castToReference(value)); 2059 } else 2060 return super.setProperty(name, value); 2061 return value; 2062 } 2063 2064 @Override 2065 public Base makeProperty(int hash, String name) throws FHIRException { 2066 switch (hash) { 2067 case -1618432855: return addIdentifier(); 2068 case -1014418093: return addDefinition(); 2069 case -332612366: return addBasedOn(); 2070 case 237568101: return addPriorRequest(); 2071 case -445338488: return getGroupIdentifier(); 2072 case -892481550: return getStatusElement(); 2073 case -1183762788: return getIntent(); 2074 case -1165461084: return getPriorityElement(); 2075 case 941839219: return getCode(); 2076 case 3059181: return getCode(); 2077 case -1867885268: return getSubject(); 2078 case 951530927: return getContext(); 2079 case -2022646513: return getOccurrence(); 2080 case 1687874001: return getOccurrence(); 2081 case -1500852503: return getAuthoredOnElement(); 2082 case 693933948: return getRequester(); 2083 case -901444568: return getPerformerType(); 2084 case 481140686: return getPerformer(); 2085 case 722137681: return addReasonCode(); 2086 case -1146218137: return addReasonReference(); 2087 case 1922406657: return addSupportingInfo(); 2088 case 3387378: return addNote(); 2089 case 1538891575: return addRelevantHistory(); 2090 default: return super.makeProperty(hash, name); 2091 } 2092 2093 } 2094 2095 @Override 2096 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2097 switch (hash) { 2098 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2099 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2100 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2101 case 237568101: /*priorRequest*/ return new String[] {"Reference"}; 2102 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 2103 case -892481550: /*status*/ return new String[] {"code"}; 2104 case -1183762788: /*intent*/ return new String[] {"CodeableConcept"}; 2105 case -1165461084: /*priority*/ return new String[] {"code"}; 2106 case 3059181: /*code*/ return new String[] {"Reference", "CodeableConcept"}; 2107 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2108 case 951530927: /*context*/ return new String[] {"Reference"}; 2109 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 2110 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 2111 case 693933948: /*requester*/ return new String[] {}; 2112 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 2113 case 481140686: /*performer*/ return new String[] {"Reference"}; 2114 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2115 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2116 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2117 case 3387378: /*note*/ return new String[] {"Annotation"}; 2118 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 2119 default: return super.getTypesForProperty(hash, name); 2120 } 2121 2122 } 2123 2124 @Override 2125 public Base addChild(String name) throws FHIRException { 2126 if (name.equals("identifier")) { 2127 return addIdentifier(); 2128 } 2129 else if (name.equals("definition")) { 2130 return addDefinition(); 2131 } 2132 else if (name.equals("basedOn")) { 2133 return addBasedOn(); 2134 } 2135 else if (name.equals("priorRequest")) { 2136 return addPriorRequest(); 2137 } 2138 else if (name.equals("groupIdentifier")) { 2139 this.groupIdentifier = new Identifier(); 2140 return this.groupIdentifier; 2141 } 2142 else if (name.equals("status")) { 2143 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.status"); 2144 } 2145 else if (name.equals("intent")) { 2146 this.intent = new CodeableConcept(); 2147 return this.intent; 2148 } 2149 else if (name.equals("priority")) { 2150 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.priority"); 2151 } 2152 else if (name.equals("codeReference")) { 2153 this.code = new Reference(); 2154 return this.code; 2155 } 2156 else if (name.equals("codeCodeableConcept")) { 2157 this.code = new CodeableConcept(); 2158 return this.code; 2159 } 2160 else if (name.equals("subject")) { 2161 this.subject = new Reference(); 2162 return this.subject; 2163 } 2164 else if (name.equals("context")) { 2165 this.context = new Reference(); 2166 return this.context; 2167 } 2168 else if (name.equals("occurrenceDateTime")) { 2169 this.occurrence = new DateTimeType(); 2170 return this.occurrence; 2171 } 2172 else if (name.equals("occurrencePeriod")) { 2173 this.occurrence = new Period(); 2174 return this.occurrence; 2175 } 2176 else if (name.equals("occurrenceTiming")) { 2177 this.occurrence = new Timing(); 2178 return this.occurrence; 2179 } 2180 else if (name.equals("authoredOn")) { 2181 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.authoredOn"); 2182 } 2183 else if (name.equals("requester")) { 2184 this.requester = new DeviceRequestRequesterComponent(); 2185 return this.requester; 2186 } 2187 else if (name.equals("performerType")) { 2188 this.performerType = new CodeableConcept(); 2189 return this.performerType; 2190 } 2191 else if (name.equals("performer")) { 2192 this.performer = new Reference(); 2193 return this.performer; 2194 } 2195 else if (name.equals("reasonCode")) { 2196 return addReasonCode(); 2197 } 2198 else if (name.equals("reasonReference")) { 2199 return addReasonReference(); 2200 } 2201 else if (name.equals("supportingInfo")) { 2202 return addSupportingInfo(); 2203 } 2204 else if (name.equals("note")) { 2205 return addNote(); 2206 } 2207 else if (name.equals("relevantHistory")) { 2208 return addRelevantHistory(); 2209 } 2210 else 2211 return super.addChild(name); 2212 } 2213 2214 public String fhirType() { 2215 return "DeviceRequest"; 2216 2217 } 2218 2219 public DeviceRequest copy() { 2220 DeviceRequest dst = new DeviceRequest(); 2221 copyValues(dst); 2222 if (identifier != null) { 2223 dst.identifier = new ArrayList<Identifier>(); 2224 for (Identifier i : identifier) 2225 dst.identifier.add(i.copy()); 2226 }; 2227 if (definition != null) { 2228 dst.definition = new ArrayList<Reference>(); 2229 for (Reference i : definition) 2230 dst.definition.add(i.copy()); 2231 }; 2232 if (basedOn != null) { 2233 dst.basedOn = new ArrayList<Reference>(); 2234 for (Reference i : basedOn) 2235 dst.basedOn.add(i.copy()); 2236 }; 2237 if (priorRequest != null) { 2238 dst.priorRequest = new ArrayList<Reference>(); 2239 for (Reference i : priorRequest) 2240 dst.priorRequest.add(i.copy()); 2241 }; 2242 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2243 dst.status = status == null ? null : status.copy(); 2244 dst.intent = intent == null ? null : intent.copy(); 2245 dst.priority = priority == null ? null : priority.copy(); 2246 dst.code = code == null ? null : code.copy(); 2247 dst.subject = subject == null ? null : subject.copy(); 2248 dst.context = context == null ? null : context.copy(); 2249 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2250 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2251 dst.requester = requester == null ? null : requester.copy(); 2252 dst.performerType = performerType == null ? null : performerType.copy(); 2253 dst.performer = performer == null ? null : performer.copy(); 2254 if (reasonCode != null) { 2255 dst.reasonCode = new ArrayList<CodeableConcept>(); 2256 for (CodeableConcept i : reasonCode) 2257 dst.reasonCode.add(i.copy()); 2258 }; 2259 if (reasonReference != null) { 2260 dst.reasonReference = new ArrayList<Reference>(); 2261 for (Reference i : reasonReference) 2262 dst.reasonReference.add(i.copy()); 2263 }; 2264 if (supportingInfo != null) { 2265 dst.supportingInfo = new ArrayList<Reference>(); 2266 for (Reference i : supportingInfo) 2267 dst.supportingInfo.add(i.copy()); 2268 }; 2269 if (note != null) { 2270 dst.note = new ArrayList<Annotation>(); 2271 for (Annotation i : note) 2272 dst.note.add(i.copy()); 2273 }; 2274 if (relevantHistory != null) { 2275 dst.relevantHistory = new ArrayList<Reference>(); 2276 for (Reference i : relevantHistory) 2277 dst.relevantHistory.add(i.copy()); 2278 }; 2279 return dst; 2280 } 2281 2282 protected DeviceRequest typedCopy() { 2283 return copy(); 2284 } 2285 2286 @Override 2287 public boolean equalsDeep(Base other_) { 2288 if (!super.equalsDeep(other_)) 2289 return false; 2290 if (!(other_ instanceof DeviceRequest)) 2291 return false; 2292 DeviceRequest o = (DeviceRequest) other_; 2293 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2294 && compareDeep(basedOn, o.basedOn, true) && compareDeep(priorRequest, o.priorRequest, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 2295 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 2296 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 2297 && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 2298 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 2299 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2300 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 2301 ; 2302 } 2303 2304 @Override 2305 public boolean equalsShallow(Base other_) { 2306 if (!super.equalsShallow(other_)) 2307 return false; 2308 if (!(other_ instanceof DeviceRequest)) 2309 return false; 2310 DeviceRequest o = (DeviceRequest) other_; 2311 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) && compareValues(authoredOn, o.authoredOn, true) 2312 ; 2313 } 2314 2315 public boolean isEmpty() { 2316 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2317 , priorRequest, groupIdentifier, status, intent, priority, code, subject, context 2318 , occurrence, authoredOn, requester, performerType, performer, reasonCode, reasonReference 2319 , supportingInfo, note, relevantHistory); 2320 } 2321 2322 @Override 2323 public ResourceType getResourceType() { 2324 return ResourceType.DeviceRequest; 2325 } 2326 2327 /** 2328 * Search parameter: <b>requester</b> 2329 * <p> 2330 * Description: <b>Who/what is requesting service </b><br> 2331 * Type: <b>reference</b><br> 2332 * Path: <b>DeviceRequest.requester.agent</b><br> 2333 * </p> 2334 */ 2335 @SearchParamDefinition(name="requester", path="DeviceRequest.requester.agent", description="Who/what is requesting service ", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Practitioner.class } ) 2336 public static final String SP_REQUESTER = "requester"; 2337 /** 2338 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2339 * <p> 2340 * Description: <b>Who/what is requesting service </b><br> 2341 * Type: <b>reference</b><br> 2342 * Path: <b>DeviceRequest.requester.agent</b><br> 2343 * </p> 2344 */ 2345 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2346 2347/** 2348 * Constant for fluent queries to be used to add include statements. Specifies 2349 * the path value of "<b>DeviceRequest:requester</b>". 2350 */ 2351 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("DeviceRequest:requester").toLocked(); 2352 2353 /** 2354 * Search parameter: <b>identifier</b> 2355 * <p> 2356 * Description: <b>Business identifier for request/order</b><br> 2357 * Type: <b>token</b><br> 2358 * Path: <b>DeviceRequest.identifier</b><br> 2359 * </p> 2360 */ 2361 @SearchParamDefinition(name="identifier", path="DeviceRequest.identifier", description="Business identifier for request/order", type="token" ) 2362 public static final String SP_IDENTIFIER = "identifier"; 2363 /** 2364 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2365 * <p> 2366 * Description: <b>Business identifier for request/order</b><br> 2367 * Type: <b>token</b><br> 2368 * Path: <b>DeviceRequest.identifier</b><br> 2369 * </p> 2370 */ 2371 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2372 2373 /** 2374 * Search parameter: <b>code</b> 2375 * <p> 2376 * Description: <b>Code for what is being requested/ordered</b><br> 2377 * Type: <b>token</b><br> 2378 * Path: <b>DeviceRequest.codeCodeableConcept</b><br> 2379 * </p> 2380 */ 2381 @SearchParamDefinition(name="code", path="DeviceRequest.code.as(CodeableConcept)", description="Code for what is being requested/ordered", type="token" ) 2382 public static final String SP_CODE = "code"; 2383 /** 2384 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2385 * <p> 2386 * Description: <b>Code for what is being requested/ordered</b><br> 2387 * Type: <b>token</b><br> 2388 * Path: <b>DeviceRequest.codeCodeableConcept</b><br> 2389 * </p> 2390 */ 2391 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2392 2393 /** 2394 * Search parameter: <b>performer</b> 2395 * <p> 2396 * Description: <b>Desired performer for service</b><br> 2397 * Type: <b>reference</b><br> 2398 * Path: <b>DeviceRequest.performer</b><br> 2399 * </p> 2400 */ 2401 @SearchParamDefinition(name="performer", path="DeviceRequest.performer", description="Desired performer for service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2402 public static final String SP_PERFORMER = "performer"; 2403 /** 2404 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2405 * <p> 2406 * Description: <b>Desired performer for service</b><br> 2407 * Type: <b>reference</b><br> 2408 * Path: <b>DeviceRequest.performer</b><br> 2409 * </p> 2410 */ 2411 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2412 2413/** 2414 * Constant for fluent queries to be used to add include statements. Specifies 2415 * the path value of "<b>DeviceRequest:performer</b>". 2416 */ 2417 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("DeviceRequest:performer").toLocked(); 2418 2419 /** 2420 * Search parameter: <b>event-date</b> 2421 * <p> 2422 * Description: <b>When service should occur</b><br> 2423 * Type: <b>date</b><br> 2424 * Path: <b>DeviceRequest.occurrenceDateTime, DeviceRequest.occurrencePeriod</b><br> 2425 * </p> 2426 */ 2427 @SearchParamDefinition(name="event-date", path="DeviceRequest.occurrence.as(DateTime) | DeviceRequest.occurrence.as(Period)", description="When service should occur", type="date" ) 2428 public static final String SP_EVENT_DATE = "event-date"; 2429 /** 2430 * <b>Fluent Client</b> search parameter constant for <b>event-date</b> 2431 * <p> 2432 * Description: <b>When service should occur</b><br> 2433 * Type: <b>date</b><br> 2434 * Path: <b>DeviceRequest.occurrenceDateTime, DeviceRequest.occurrencePeriod</b><br> 2435 * </p> 2436 */ 2437 public static final ca.uhn.fhir.rest.gclient.DateClientParam EVENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EVENT_DATE); 2438 2439 /** 2440 * Search parameter: <b>subject</b> 2441 * <p> 2442 * Description: <b>Individual the service is ordered for</b><br> 2443 * Type: <b>reference</b><br> 2444 * Path: <b>DeviceRequest.subject</b><br> 2445 * </p> 2446 */ 2447 @SearchParamDefinition(name="subject", path="DeviceRequest.subject", description="Individual the service is ordered for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 2448 public static final String SP_SUBJECT = "subject"; 2449 /** 2450 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2451 * <p> 2452 * Description: <b>Individual the service is ordered for</b><br> 2453 * Type: <b>reference</b><br> 2454 * Path: <b>DeviceRequest.subject</b><br> 2455 * </p> 2456 */ 2457 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2458 2459/** 2460 * Constant for fluent queries to be used to add include statements. Specifies 2461 * the path value of "<b>DeviceRequest:subject</b>". 2462 */ 2463 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DeviceRequest:subject").toLocked(); 2464 2465 /** 2466 * Search parameter: <b>encounter</b> 2467 * <p> 2468 * Description: <b>Encounter or Episode during which request was created</b><br> 2469 * Type: <b>reference</b><br> 2470 * Path: <b>DeviceRequest.context</b><br> 2471 * </p> 2472 */ 2473 @SearchParamDefinition(name="encounter", path="DeviceRequest.context", description="Encounter or Episode during which request was created", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2474 public static final String SP_ENCOUNTER = "encounter"; 2475 /** 2476 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2477 * <p> 2478 * Description: <b>Encounter or Episode during which request was created</b><br> 2479 * Type: <b>reference</b><br> 2480 * Path: <b>DeviceRequest.context</b><br> 2481 * </p> 2482 */ 2483 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2484 2485/** 2486 * Constant for fluent queries to be used to add include statements. Specifies 2487 * the path value of "<b>DeviceRequest:encounter</b>". 2488 */ 2489 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("DeviceRequest:encounter").toLocked(); 2490 2491 /** 2492 * Search parameter: <b>authored-on</b> 2493 * <p> 2494 * Description: <b>When the request transitioned to being actionable</b><br> 2495 * Type: <b>date</b><br> 2496 * Path: <b>DeviceRequest.authoredOn</b><br> 2497 * </p> 2498 */ 2499 @SearchParamDefinition(name="authored-on", path="DeviceRequest.authoredOn", description="When the request transitioned to being actionable", type="date" ) 2500 public static final String SP_AUTHORED_ON = "authored-on"; 2501 /** 2502 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 2503 * <p> 2504 * Description: <b>When the request transitioned to being actionable</b><br> 2505 * Type: <b>date</b><br> 2506 * Path: <b>DeviceRequest.authoredOn</b><br> 2507 * </p> 2508 */ 2509 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED_ON); 2510 2511 /** 2512 * Search parameter: <b>intent</b> 2513 * <p> 2514 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 2515 * Type: <b>token</b><br> 2516 * Path: <b>DeviceRequest.intent</b><br> 2517 * </p> 2518 */ 2519 @SearchParamDefinition(name="intent", path="DeviceRequest.intent", description="proposal | plan | original-order |reflex-order", type="token" ) 2520 public static final String SP_INTENT = "intent"; 2521 /** 2522 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 2523 * <p> 2524 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 2525 * Type: <b>token</b><br> 2526 * Path: <b>DeviceRequest.intent</b><br> 2527 * </p> 2528 */ 2529 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 2530 2531 /** 2532 * Search parameter: <b>group-identifier</b> 2533 * <p> 2534 * Description: <b>Composite request this is part of</b><br> 2535 * Type: <b>token</b><br> 2536 * Path: <b>DeviceRequest.groupIdentifier</b><br> 2537 * </p> 2538 */ 2539 @SearchParamDefinition(name="group-identifier", path="DeviceRequest.groupIdentifier", description="Composite request this is part of", type="token" ) 2540 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 2541 /** 2542 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 2543 * <p> 2544 * Description: <b>Composite request this is part of</b><br> 2545 * Type: <b>token</b><br> 2546 * Path: <b>DeviceRequest.groupIdentifier</b><br> 2547 * </p> 2548 */ 2549 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 2550 2551 /** 2552 * Search parameter: <b>based-on</b> 2553 * <p> 2554 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 2555 * Type: <b>reference</b><br> 2556 * Path: <b>DeviceRequest.basedOn</b><br> 2557 * </p> 2558 */ 2559 @SearchParamDefinition(name="based-on", path="DeviceRequest.basedOn", description="Plan/proposal/order fulfilled by this request", type="reference" ) 2560 public static final String SP_BASED_ON = "based-on"; 2561 /** 2562 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2563 * <p> 2564 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 2565 * Type: <b>reference</b><br> 2566 * Path: <b>DeviceRequest.basedOn</b><br> 2567 * </p> 2568 */ 2569 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2570 2571/** 2572 * Constant for fluent queries to be used to add include statements. Specifies 2573 * the path value of "<b>DeviceRequest:based-on</b>". 2574 */ 2575 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("DeviceRequest:based-on").toLocked(); 2576 2577 /** 2578 * Search parameter: <b>priorrequest</b> 2579 * <p> 2580 * Description: <b>Request takes the place of referenced completed or terminated requests</b><br> 2581 * Type: <b>reference</b><br> 2582 * Path: <b>DeviceRequest.priorRequest</b><br> 2583 * </p> 2584 */ 2585 @SearchParamDefinition(name="priorrequest", path="DeviceRequest.priorRequest", description="Request takes the place of referenced completed or terminated requests", type="reference" ) 2586 public static final String SP_PRIORREQUEST = "priorrequest"; 2587 /** 2588 * <b>Fluent Client</b> search parameter constant for <b>priorrequest</b> 2589 * <p> 2590 * Description: <b>Request takes the place of referenced completed or terminated requests</b><br> 2591 * Type: <b>reference</b><br> 2592 * Path: <b>DeviceRequest.priorRequest</b><br> 2593 * </p> 2594 */ 2595 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRIORREQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRIORREQUEST); 2596 2597/** 2598 * Constant for fluent queries to be used to add include statements. Specifies 2599 * the path value of "<b>DeviceRequest:priorrequest</b>". 2600 */ 2601 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRIORREQUEST = new ca.uhn.fhir.model.api.Include("DeviceRequest:priorrequest").toLocked(); 2602 2603 /** 2604 * Search parameter: <b>patient</b> 2605 * <p> 2606 * Description: <b>Individual the service is ordered for</b><br> 2607 * Type: <b>reference</b><br> 2608 * Path: <b>DeviceRequest.subject</b><br> 2609 * </p> 2610 */ 2611 @SearchParamDefinition(name="patient", path="DeviceRequest.subject", description="Individual the service is ordered for", type="reference", target={Patient.class } ) 2612 public static final String SP_PATIENT = "patient"; 2613 /** 2614 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2615 * <p> 2616 * Description: <b>Individual the service is ordered for</b><br> 2617 * Type: <b>reference</b><br> 2618 * Path: <b>DeviceRequest.subject</b><br> 2619 * </p> 2620 */ 2621 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2622 2623/** 2624 * Constant for fluent queries to be used to add include statements. Specifies 2625 * the path value of "<b>DeviceRequest:patient</b>". 2626 */ 2627 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceRequest:patient").toLocked(); 2628 2629 /** 2630 * Search parameter: <b>definition</b> 2631 * <p> 2632 * Description: <b>Protocol or definition followed by this request</b><br> 2633 * Type: <b>reference</b><br> 2634 * Path: <b>DeviceRequest.definition</b><br> 2635 * </p> 2636 */ 2637 @SearchParamDefinition(name="definition", path="DeviceRequest.definition", description="Protocol or definition followed by this request", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 2638 public static final String SP_DEFINITION = "definition"; 2639 /** 2640 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 2641 * <p> 2642 * Description: <b>Protocol or definition followed by this request</b><br> 2643 * Type: <b>reference</b><br> 2644 * Path: <b>DeviceRequest.definition</b><br> 2645 * </p> 2646 */ 2647 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 2648 2649/** 2650 * Constant for fluent queries to be used to add include statements. Specifies 2651 * the path value of "<b>DeviceRequest:definition</b>". 2652 */ 2653 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("DeviceRequest:definition").toLocked(); 2654 2655 /** 2656 * Search parameter: <b>device</b> 2657 * <p> 2658 * Description: <b>Reference to resource that is being requested/ordered</b><br> 2659 * Type: <b>reference</b><br> 2660 * Path: <b>DeviceRequest.codeReference</b><br> 2661 * </p> 2662 */ 2663 @SearchParamDefinition(name="device", path="DeviceRequest.code.as(Reference)", description="Reference to resource that is being requested/ordered", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class } ) 2664 public static final String SP_DEVICE = "device"; 2665 /** 2666 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2667 * <p> 2668 * Description: <b>Reference to resource that is being requested/ordered</b><br> 2669 * Type: <b>reference</b><br> 2670 * Path: <b>DeviceRequest.codeReference</b><br> 2671 * </p> 2672 */ 2673 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 2674 2675/** 2676 * Constant for fluent queries to be used to add include statements. Specifies 2677 * the path value of "<b>DeviceRequest:device</b>". 2678 */ 2679 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceRequest:device").toLocked(); 2680 2681 /** 2682 * Search parameter: <b>status</b> 2683 * <p> 2684 * Description: <b>entered-in-error | draft | active |suspended | completed </b><br> 2685 * Type: <b>token</b><br> 2686 * Path: <b>DeviceRequest.status</b><br> 2687 * </p> 2688 */ 2689 @SearchParamDefinition(name="status", path="DeviceRequest.status", description="entered-in-error | draft | active |suspended | completed ", type="token" ) 2690 public static final String SP_STATUS = "status"; 2691 /** 2692 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2693 * <p> 2694 * Description: <b>entered-in-error | draft | active |suspended | completed </b><br> 2695 * Type: <b>token</b><br> 2696 * Path: <b>DeviceRequest.status</b><br> 2697 * </p> 2698 */ 2699 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2700 2701 2702}