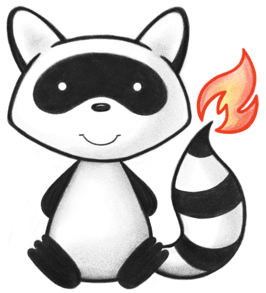
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 050 */ 051@ResourceDef(name="DeviceRequest", profile="http://hl7.org/fhir/Profile/DeviceRequest") 052public class DeviceRequest extends DomainResource { 053 054 public enum DeviceRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action 057 */ 058 DRAFT, 059 /** 060 * The request is ready to be acted upon 061 */ 062 ACTIVE, 063 /** 064 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 065 */ 066 SUSPENDED, 067 /** 068 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 069 */ 070 CANCELLED, 071 /** 072 * Activity against the request has been sufficiently completed to the satisfaction of the requester 073 */ 074 COMPLETED, 075 /** 076 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static DeviceRequestStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("draft".equals(codeString)) 091 return DRAFT; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("cancelled".equals(codeString)) 097 return CANCELLED; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown DeviceRequestStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case DRAFT: return "draft"; 112 case ACTIVE: return "active"; 113 case SUSPENDED: return "suspended"; 114 case CANCELLED: return "cancelled"; 115 case COMPLETED: return "completed"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case DRAFT: return "http://hl7.org/fhir/request-status"; 125 case ACTIVE: return "http://hl7.org/fhir/request-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 127 case CANCELLED: return "http://hl7.org/fhir/request-status"; 128 case COMPLETED: return "http://hl7.org/fhir/request-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 138 case ACTIVE: return "The request is ready to be acted upon"; 139 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 140 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 141 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 142 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 143 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case DRAFT: return "Draft"; 151 case ACTIVE: return "Active"; 152 case SUSPENDED: return "Suspended"; 153 case CANCELLED: return "Cancelled"; 154 case COMPLETED: return "Completed"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class DeviceRequestStatusEnumFactory implements EnumFactory<DeviceRequestStatus> { 164 public DeviceRequestStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("draft".equals(codeString)) 169 return DeviceRequestStatus.DRAFT; 170 if ("active".equals(codeString)) 171 return DeviceRequestStatus.ACTIVE; 172 if ("suspended".equals(codeString)) 173 return DeviceRequestStatus.SUSPENDED; 174 if ("cancelled".equals(codeString)) 175 return DeviceRequestStatus.CANCELLED; 176 if ("completed".equals(codeString)) 177 return DeviceRequestStatus.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return DeviceRequestStatus.ENTEREDINERROR; 180 if ("unknown".equals(codeString)) 181 return DeviceRequestStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown DeviceRequestStatus code '"+codeString+"'"); 183 } 184 public Enumeration<DeviceRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<DeviceRequestStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("draft".equals(codeString)) 193 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.DRAFT); 194 if ("active".equals(codeString)) 195 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.ACTIVE); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.SUSPENDED); 198 if ("cancelled".equals(codeString)) 199 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.CANCELLED); 200 if ("completed".equals(codeString)) 201 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.COMPLETED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.ENTEREDINERROR); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.UNKNOWN); 206 throw new FHIRException("Unknown DeviceRequestStatus code '"+codeString+"'"); 207 } 208 public String toCode(DeviceRequestStatus code) { 209 if (code == DeviceRequestStatus.NULL) 210 return null; 211 if (code == DeviceRequestStatus.DRAFT) 212 return "draft"; 213 if (code == DeviceRequestStatus.ACTIVE) 214 return "active"; 215 if (code == DeviceRequestStatus.SUSPENDED) 216 return "suspended"; 217 if (code == DeviceRequestStatus.CANCELLED) 218 return "cancelled"; 219 if (code == DeviceRequestStatus.COMPLETED) 220 return "completed"; 221 if (code == DeviceRequestStatus.ENTEREDINERROR) 222 return "entered-in-error"; 223 if (code == DeviceRequestStatus.UNKNOWN) 224 return "unknown"; 225 return "?"; 226 } 227 public String toSystem(DeviceRequestStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 public enum RequestPriority { 233 /** 234 * The request has normal priority 235 */ 236 ROUTINE, 237 /** 238 * The request should be actioned promptly - higher priority than routine 239 */ 240 URGENT, 241 /** 242 * The request should be actioned as soon as possible - higher priority than urgent 243 */ 244 ASAP, 245 /** 246 * The request should be actioned immediately - highest possible priority. E.g. an emergency 247 */ 248 STAT, 249 /** 250 * added to help the parsers with the generic types 251 */ 252 NULL; 253 public static RequestPriority fromCode(String codeString) throws FHIRException { 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("routine".equals(codeString)) 257 return ROUTINE; 258 if ("urgent".equals(codeString)) 259 return URGENT; 260 if ("asap".equals(codeString)) 261 return ASAP; 262 if ("stat".equals(codeString)) 263 return STAT; 264 if (Configuration.isAcceptInvalidEnums()) 265 return null; 266 else 267 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 268 } 269 public String toCode() { 270 switch (this) { 271 case ROUTINE: return "routine"; 272 case URGENT: return "urgent"; 273 case ASAP: return "asap"; 274 case STAT: return "stat"; 275 case NULL: return null; 276 default: return "?"; 277 } 278 } 279 public String getSystem() { 280 switch (this) { 281 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 282 case URGENT: return "http://hl7.org/fhir/request-priority"; 283 case ASAP: return "http://hl7.org/fhir/request-priority"; 284 case STAT: return "http://hl7.org/fhir/request-priority"; 285 case NULL: return null; 286 default: return "?"; 287 } 288 } 289 public String getDefinition() { 290 switch (this) { 291 case ROUTINE: return "The request has normal priority"; 292 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 293 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 294 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 295 case NULL: return null; 296 default: return "?"; 297 } 298 } 299 public String getDisplay() { 300 switch (this) { 301 case ROUTINE: return "Routine"; 302 case URGENT: return "Urgent"; 303 case ASAP: return "ASAP"; 304 case STAT: return "STAT"; 305 case NULL: return null; 306 default: return "?"; 307 } 308 } 309 } 310 311 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 312 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 313 if (codeString == null || "".equals(codeString)) 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("routine".equals(codeString)) 317 return RequestPriority.ROUTINE; 318 if ("urgent".equals(codeString)) 319 return RequestPriority.URGENT; 320 if ("asap".equals(codeString)) 321 return RequestPriority.ASAP; 322 if ("stat".equals(codeString)) 323 return RequestPriority.STAT; 324 throw new IllegalArgumentException("Unknown RequestPriority code '"+codeString+"'"); 325 } 326 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 327 if (code == null) 328 return null; 329 if (code.isEmpty()) 330 return new Enumeration<RequestPriority>(this); 331 String codeString = code.asStringValue(); 332 if (codeString == null || "".equals(codeString)) 333 return null; 334 if ("routine".equals(codeString)) 335 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE); 336 if ("urgent".equals(codeString)) 337 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT); 338 if ("asap".equals(codeString)) 339 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP); 340 if ("stat".equals(codeString)) 341 return new Enumeration<RequestPriority>(this, RequestPriority.STAT); 342 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 343 } 344 public String toCode(RequestPriority code) { 345 if (code == RequestPriority.NULL) 346 return null; 347 if (code == RequestPriority.ROUTINE) 348 return "routine"; 349 if (code == RequestPriority.URGENT) 350 return "urgent"; 351 if (code == RequestPriority.ASAP) 352 return "asap"; 353 if (code == RequestPriority.STAT) 354 return "stat"; 355 return "?"; 356 } 357 public String toSystem(RequestPriority code) { 358 return code.getSystem(); 359 } 360 } 361 362 @Block() 363 public static class DeviceRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 364 /** 365 * The device, practitioner, etc. who initiated the request. 366 */ 367 @Child(name = "agent", type = {Device.class, Practitioner.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=true) 368 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner, etc. who initiated the request." ) 369 protected Reference agent; 370 371 /** 372 * The actual object that is the target of the reference (The device, practitioner, etc. who initiated the request.) 373 */ 374 protected Resource agentTarget; 375 376 /** 377 * The organization the device or practitioner was acting on behalf of. 378 */ 379 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 380 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 381 protected Reference onBehalfOf; 382 383 /** 384 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 385 */ 386 protected Organization onBehalfOfTarget; 387 388 private static final long serialVersionUID = -71453027L; 389 390 /** 391 * Constructor 392 */ 393 public DeviceRequestRequesterComponent() { 394 super(); 395 } 396 397 /** 398 * Constructor 399 */ 400 public DeviceRequestRequesterComponent(Reference agent) { 401 super(); 402 this.agent = agent; 403 } 404 405 /** 406 * @return {@link #agent} (The device, practitioner, etc. who initiated the request.) 407 */ 408 public Reference getAgent() { 409 if (this.agent == null) 410 if (Configuration.errorOnAutoCreate()) 411 throw new Error("Attempt to auto-create DeviceRequestRequesterComponent.agent"); 412 else if (Configuration.doAutoCreate()) 413 this.agent = new Reference(); // cc 414 return this.agent; 415 } 416 417 public boolean hasAgent() { 418 return this.agent != null && !this.agent.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #agent} (The device, practitioner, etc. who initiated the request.) 423 */ 424 public DeviceRequestRequesterComponent setAgent(Reference value) { 425 this.agent = value; 426 return this; 427 } 428 429 /** 430 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 431 */ 432 public Resource getAgentTarget() { 433 return this.agentTarget; 434 } 435 436 /** 437 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 438 */ 439 public DeviceRequestRequesterComponent setAgentTarget(Resource value) { 440 this.agentTarget = value; 441 return this; 442 } 443 444 /** 445 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 446 */ 447 public Reference getOnBehalfOf() { 448 if (this.onBehalfOf == null) 449 if (Configuration.errorOnAutoCreate()) 450 throw new Error("Attempt to auto-create DeviceRequestRequesterComponent.onBehalfOf"); 451 else if (Configuration.doAutoCreate()) 452 this.onBehalfOf = new Reference(); // cc 453 return this.onBehalfOf; 454 } 455 456 public boolean hasOnBehalfOf() { 457 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 458 } 459 460 /** 461 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 462 */ 463 public DeviceRequestRequesterComponent setOnBehalfOf(Reference value) { 464 this.onBehalfOf = value; 465 return this; 466 } 467 468 /** 469 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 470 */ 471 public Organization getOnBehalfOfTarget() { 472 if (this.onBehalfOfTarget == null) 473 if (Configuration.errorOnAutoCreate()) 474 throw new Error("Attempt to auto-create DeviceRequestRequesterComponent.onBehalfOf"); 475 else if (Configuration.doAutoCreate()) 476 this.onBehalfOfTarget = new Organization(); // aa 477 return this.onBehalfOfTarget; 478 } 479 480 /** 481 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 482 */ 483 public DeviceRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 484 this.onBehalfOfTarget = value; 485 return this; 486 } 487 488 protected void listChildren(List<Property> children) { 489 super.listChildren(children); 490 children.add(new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent)); 491 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 492 } 493 494 @Override 495 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 496 switch (_hash) { 497 case 92750597: /*agent*/ return new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent); 498 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 499 default: return super.getNamedProperty(_hash, _name, _checkValid); 500 } 501 502 } 503 504 @Override 505 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 506 switch (hash) { 507 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 508 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 509 default: return super.getProperty(hash, name, checkValid); 510 } 511 512 } 513 514 @Override 515 public Base setProperty(int hash, String name, Base value) throws FHIRException { 516 switch (hash) { 517 case 92750597: // agent 518 this.agent = castToReference(value); // Reference 519 return value; 520 case -14402964: // onBehalfOf 521 this.onBehalfOf = castToReference(value); // Reference 522 return value; 523 default: return super.setProperty(hash, name, value); 524 } 525 526 } 527 528 @Override 529 public Base setProperty(String name, Base value) throws FHIRException { 530 if (name.equals("agent")) { 531 this.agent = castToReference(value); // Reference 532 } else if (name.equals("onBehalfOf")) { 533 this.onBehalfOf = castToReference(value); // Reference 534 } else 535 return super.setProperty(name, value); 536 return value; 537 } 538 539 @Override 540 public Base makeProperty(int hash, String name) throws FHIRException { 541 switch (hash) { 542 case 92750597: return getAgent(); 543 case -14402964: return getOnBehalfOf(); 544 default: return super.makeProperty(hash, name); 545 } 546 547 } 548 549 @Override 550 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 551 switch (hash) { 552 case 92750597: /*agent*/ return new String[] {"Reference"}; 553 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 554 default: return super.getTypesForProperty(hash, name); 555 } 556 557 } 558 559 @Override 560 public Base addChild(String name) throws FHIRException { 561 if (name.equals("agent")) { 562 this.agent = new Reference(); 563 return this.agent; 564 } 565 else if (name.equals("onBehalfOf")) { 566 this.onBehalfOf = new Reference(); 567 return this.onBehalfOf; 568 } 569 else 570 return super.addChild(name); 571 } 572 573 public DeviceRequestRequesterComponent copy() { 574 DeviceRequestRequesterComponent dst = new DeviceRequestRequesterComponent(); 575 copyValues(dst); 576 dst.agent = agent == null ? null : agent.copy(); 577 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 578 return dst; 579 } 580 581 @Override 582 public boolean equalsDeep(Base other_) { 583 if (!super.equalsDeep(other_)) 584 return false; 585 if (!(other_ instanceof DeviceRequestRequesterComponent)) 586 return false; 587 DeviceRequestRequesterComponent o = (DeviceRequestRequesterComponent) other_; 588 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 589 } 590 591 @Override 592 public boolean equalsShallow(Base other_) { 593 if (!super.equalsShallow(other_)) 594 return false; 595 if (!(other_ instanceof DeviceRequestRequesterComponent)) 596 return false; 597 DeviceRequestRequesterComponent o = (DeviceRequestRequesterComponent) other_; 598 return true; 599 } 600 601 public boolean isEmpty() { 602 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 603 } 604 605 public String fhirType() { 606 return "DeviceRequest.requester"; 607 608 } 609 610 } 611 612 /** 613 * Identifiers assigned to this order by the orderer or by the receiver. 614 */ 615 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 616 @Description(shortDefinition="External Request identifier", formalDefinition="Identifiers assigned to this order by the orderer or by the receiver." ) 617 protected List<Identifier> identifier; 618 619 /** 620 * Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%. 621 */ 622 @Child(name = "definition", type = {ActivityDefinition.class, PlanDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 623 @Description(shortDefinition="Protocol or definition", formalDefinition="Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%." ) 624 protected List<Reference> definition; 625 /** 626 * The actual objects that are the target of the reference (Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.) 627 */ 628 protected List<Resource> definitionTarget; 629 630 631 /** 632 * Plan/proposal/order fulfilled by this request. 633 */ 634 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 635 @Description(shortDefinition="What request fulfills", formalDefinition="Plan/proposal/order fulfilled by this request." ) 636 protected List<Reference> basedOn; 637 /** 638 * The actual objects that are the target of the reference (Plan/proposal/order fulfilled by this request.) 639 */ 640 protected List<Resource> basedOnTarget; 641 642 643 /** 644 * The request takes the place of the referenced completed or terminated request(s). 645 */ 646 @Child(name = "priorRequest", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 647 @Description(shortDefinition="What request replaces", formalDefinition="The request takes the place of the referenced completed or terminated request(s)." ) 648 protected List<Reference> priorRequest; 649 /** 650 * The actual objects that are the target of the reference (The request takes the place of the referenced completed or terminated request(s).) 651 */ 652 protected List<Resource> priorRequestTarget; 653 654 655 /** 656 * Composite request this is part of. 657 */ 658 @Child(name = "groupIdentifier", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 659 @Description(shortDefinition="Identifier of composite request", formalDefinition="Composite request this is part of." ) 660 protected Identifier groupIdentifier; 661 662 /** 663 * The status of the request. 664 */ 665 @Child(name = "status", type = {CodeType.class}, order=5, min=0, max=1, modifier=true, summary=true) 666 @Description(shortDefinition="draft | active | suspended | completed | entered-in-error | cancelled", formalDefinition="The status of the request." ) 667 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 668 protected Enumeration<DeviceRequestStatus> status; 669 670 /** 671 * Whether the request is a proposal, plan, an original order or a reflex order. 672 */ 673 @Child(name = "intent", type = {CodeableConcept.class}, order=6, min=1, max=1, modifier=true, summary=true) 674 @Description(shortDefinition="proposal | plan | original-order | encoded | reflex-order", formalDefinition="Whether the request is a proposal, plan, an original order or a reflex order." ) 675 protected CodeableConcept intent; 676 677 /** 678 * Indicates how quickly the {{title}} should be addressed with respect to other requests. 679 */ 680 @Child(name = "priority", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 681 @Description(shortDefinition="Indicates how quickly the {{title}} should be addressed with respect to other requests", formalDefinition="Indicates how quickly the {{title}} should be addressed with respect to other requests." ) 682 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 683 protected Enumeration<RequestPriority> priority; 684 685 /** 686 * The details of the device to be used. 687 */ 688 @Child(name = "code", type = {Device.class, CodeableConcept.class}, order=8, min=1, max=1, modifier=false, summary=true) 689 @Description(shortDefinition="Device requested", formalDefinition="The details of the device to be used." ) 690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-kind") 691 protected Type code; 692 693 /** 694 * The patient who will use the device. 695 */ 696 @Child(name = "subject", type = {Patient.class, Group.class, Location.class, Device.class}, order=9, min=1, max=1, modifier=false, summary=true) 697 @Description(shortDefinition="Focus of request", formalDefinition="The patient who will use the device." ) 698 protected Reference subject; 699 700 /** 701 * The actual object that is the target of the reference (The patient who will use the device.) 702 */ 703 protected Resource subjectTarget; 704 705 /** 706 * An encounter that provides additional context in which this request is made. 707 */ 708 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=10, min=0, max=1, modifier=false, summary=true) 709 @Description(shortDefinition="Encounter or Episode motivating request", formalDefinition="An encounter that provides additional context in which this request is made." ) 710 protected Reference context; 711 712 /** 713 * The actual object that is the target of the reference (An encounter that provides additional context in which this request is made.) 714 */ 715 protected Resource contextTarget; 716 717 /** 718 * The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013". 719 */ 720 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=11, min=0, max=1, modifier=false, summary=true) 721 @Description(shortDefinition="Desired time or schedule for use", formalDefinition="The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\"." ) 722 protected Type occurrence; 723 724 /** 725 * When the request transitioned to being actionable. 726 */ 727 @Child(name = "authoredOn", type = {DateTimeType.class}, order=12, min=0, max=1, modifier=false, summary=true) 728 @Description(shortDefinition="When recorded", formalDefinition="When the request transitioned to being actionable." ) 729 protected DateTimeType authoredOn; 730 731 /** 732 * The individual who initiated the request and has responsibility for its activation. 733 */ 734 @Child(name = "requester", type = {}, order=13, min=0, max=1, modifier=false, summary=true) 735 @Description(shortDefinition="Who/what is requesting diagnostics", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 736 protected DeviceRequestRequesterComponent requester; 737 738 /** 739 * Desired type of performer for doing the diagnostic testing. 740 */ 741 @Child(name = "performerType", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=true) 742 @Description(shortDefinition="Fille role", formalDefinition="Desired type of performer for doing the diagnostic testing." ) 743 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 744 protected CodeableConcept performerType; 745 746 /** 747 * The desired perfomer for doing the diagnostic testing. 748 */ 749 @Child(name = "performer", type = {Practitioner.class, Organization.class, Patient.class, Device.class, RelatedPerson.class, HealthcareService.class}, order=15, min=0, max=1, modifier=false, summary=true) 750 @Description(shortDefinition="Requested Filler", formalDefinition="The desired perfomer for doing the diagnostic testing." ) 751 protected Reference performer; 752 753 /** 754 * The actual object that is the target of the reference (The desired perfomer for doing the diagnostic testing.) 755 */ 756 protected Resource performerTarget; 757 758 /** 759 * Reason or justification for the use of this device. 760 */ 761 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 762 @Description(shortDefinition="Coded Reason for request", formalDefinition="Reason or justification for the use of this device." ) 763 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 764 protected List<CodeableConcept> reasonCode; 765 766 /** 767 * Reason or justification for the use of this device. 768 */ 769 @Child(name = "reasonReference", type = {Reference.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 770 @Description(shortDefinition="Linked Reason for request", formalDefinition="Reason or justification for the use of this device." ) 771 protected List<Reference> reasonReference; 772 /** 773 * The actual objects that are the target of the reference (Reason or justification for the use of this device.) 774 */ 775 protected List<Resource> reasonReferenceTarget; 776 777 778 /** 779 * Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site). 780 */ 781 @Child(name = "supportingInfo", type = {Reference.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 782 @Description(shortDefinition="Additional clinical information", formalDefinition="Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site)." ) 783 protected List<Reference> supportingInfo; 784 /** 785 * The actual objects that are the target of the reference (Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).) 786 */ 787 protected List<Resource> supportingInfoTarget; 788 789 790 /** 791 * Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement. 792 */ 793 @Child(name = "note", type = {Annotation.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 794 @Description(shortDefinition="Notes or comments", formalDefinition="Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement." ) 795 protected List<Annotation> note; 796 797 /** 798 * Key events in the history of the request. 799 */ 800 @Child(name = "relevantHistory", type = {Provenance.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 801 @Description(shortDefinition="Request provenance", formalDefinition="Key events in the history of the request." ) 802 protected List<Reference> relevantHistory; 803 /** 804 * The actual objects that are the target of the reference (Key events in the history of the request.) 805 */ 806 protected List<Provenance> relevantHistoryTarget; 807 808 809 private static final long serialVersionUID = -2002514925L; 810 811 /** 812 * Constructor 813 */ 814 public DeviceRequest() { 815 super(); 816 } 817 818 /** 819 * Constructor 820 */ 821 public DeviceRequest(CodeableConcept intent, Type code, Reference subject) { 822 super(); 823 this.intent = intent; 824 this.code = code; 825 this.subject = subject; 826 } 827 828 /** 829 * @return {@link #identifier} (Identifiers assigned to this order by the orderer or by the receiver.) 830 */ 831 public List<Identifier> getIdentifier() { 832 if (this.identifier == null) 833 this.identifier = new ArrayList<Identifier>(); 834 return this.identifier; 835 } 836 837 /** 838 * @return Returns a reference to <code>this</code> for easy method chaining 839 */ 840 public DeviceRequest setIdentifier(List<Identifier> theIdentifier) { 841 this.identifier = theIdentifier; 842 return this; 843 } 844 845 public boolean hasIdentifier() { 846 if (this.identifier == null) 847 return false; 848 for (Identifier item : this.identifier) 849 if (!item.isEmpty()) 850 return true; 851 return false; 852 } 853 854 public Identifier addIdentifier() { //3 855 Identifier t = new Identifier(); 856 if (this.identifier == null) 857 this.identifier = new ArrayList<Identifier>(); 858 this.identifier.add(t); 859 return t; 860 } 861 862 public DeviceRequest addIdentifier(Identifier t) { //3 863 if (t == null) 864 return this; 865 if (this.identifier == null) 866 this.identifier = new ArrayList<Identifier>(); 867 this.identifier.add(t); 868 return this; 869 } 870 871 /** 872 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 873 */ 874 public Identifier getIdentifierFirstRep() { 875 if (getIdentifier().isEmpty()) { 876 addIdentifier(); 877 } 878 return getIdentifier().get(0); 879 } 880 881 /** 882 * @return {@link #definition} (Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.) 883 */ 884 public List<Reference> getDefinition() { 885 if (this.definition == null) 886 this.definition = new ArrayList<Reference>(); 887 return this.definition; 888 } 889 890 /** 891 * @return Returns a reference to <code>this</code> for easy method chaining 892 */ 893 public DeviceRequest setDefinition(List<Reference> theDefinition) { 894 this.definition = theDefinition; 895 return this; 896 } 897 898 public boolean hasDefinition() { 899 if (this.definition == null) 900 return false; 901 for (Reference item : this.definition) 902 if (!item.isEmpty()) 903 return true; 904 return false; 905 } 906 907 public Reference addDefinition() { //3 908 Reference t = new Reference(); 909 if (this.definition == null) 910 this.definition = new ArrayList<Reference>(); 911 this.definition.add(t); 912 return t; 913 } 914 915 public DeviceRequest addDefinition(Reference t) { //3 916 if (t == null) 917 return this; 918 if (this.definition == null) 919 this.definition = new ArrayList<Reference>(); 920 this.definition.add(t); 921 return this; 922 } 923 924 /** 925 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 926 */ 927 public Reference getDefinitionFirstRep() { 928 if (getDefinition().isEmpty()) { 929 addDefinition(); 930 } 931 return getDefinition().get(0); 932 } 933 934 /** 935 * @deprecated Use Reference#setResource(IBaseResource) instead 936 */ 937 @Deprecated 938 public List<Resource> getDefinitionTarget() { 939 if (this.definitionTarget == null) 940 this.definitionTarget = new ArrayList<Resource>(); 941 return this.definitionTarget; 942 } 943 944 /** 945 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 946 */ 947 public List<Reference> getBasedOn() { 948 if (this.basedOn == null) 949 this.basedOn = new ArrayList<Reference>(); 950 return this.basedOn; 951 } 952 953 /** 954 * @return Returns a reference to <code>this</code> for easy method chaining 955 */ 956 public DeviceRequest setBasedOn(List<Reference> theBasedOn) { 957 this.basedOn = theBasedOn; 958 return this; 959 } 960 961 public boolean hasBasedOn() { 962 if (this.basedOn == null) 963 return false; 964 for (Reference item : this.basedOn) 965 if (!item.isEmpty()) 966 return true; 967 return false; 968 } 969 970 public Reference addBasedOn() { //3 971 Reference t = new Reference(); 972 if (this.basedOn == null) 973 this.basedOn = new ArrayList<Reference>(); 974 this.basedOn.add(t); 975 return t; 976 } 977 978 public DeviceRequest addBasedOn(Reference t) { //3 979 if (t == null) 980 return this; 981 if (this.basedOn == null) 982 this.basedOn = new ArrayList<Reference>(); 983 this.basedOn.add(t); 984 return this; 985 } 986 987 /** 988 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 989 */ 990 public Reference getBasedOnFirstRep() { 991 if (getBasedOn().isEmpty()) { 992 addBasedOn(); 993 } 994 return getBasedOn().get(0); 995 } 996 997 /** 998 * @deprecated Use Reference#setResource(IBaseResource) instead 999 */ 1000 @Deprecated 1001 public List<Resource> getBasedOnTarget() { 1002 if (this.basedOnTarget == null) 1003 this.basedOnTarget = new ArrayList<Resource>(); 1004 return this.basedOnTarget; 1005 } 1006 1007 /** 1008 * @return {@link #priorRequest} (The request takes the place of the referenced completed or terminated request(s).) 1009 */ 1010 public List<Reference> getPriorRequest() { 1011 if (this.priorRequest == null) 1012 this.priorRequest = new ArrayList<Reference>(); 1013 return this.priorRequest; 1014 } 1015 1016 /** 1017 * @return Returns a reference to <code>this</code> for easy method chaining 1018 */ 1019 public DeviceRequest setPriorRequest(List<Reference> thePriorRequest) { 1020 this.priorRequest = thePriorRequest; 1021 return this; 1022 } 1023 1024 public boolean hasPriorRequest() { 1025 if (this.priorRequest == null) 1026 return false; 1027 for (Reference item : this.priorRequest) 1028 if (!item.isEmpty()) 1029 return true; 1030 return false; 1031 } 1032 1033 public Reference addPriorRequest() { //3 1034 Reference t = new Reference(); 1035 if (this.priorRequest == null) 1036 this.priorRequest = new ArrayList<Reference>(); 1037 this.priorRequest.add(t); 1038 return t; 1039 } 1040 1041 public DeviceRequest addPriorRequest(Reference t) { //3 1042 if (t == null) 1043 return this; 1044 if (this.priorRequest == null) 1045 this.priorRequest = new ArrayList<Reference>(); 1046 this.priorRequest.add(t); 1047 return this; 1048 } 1049 1050 /** 1051 * @return The first repetition of repeating field {@link #priorRequest}, creating it if it does not already exist 1052 */ 1053 public Reference getPriorRequestFirstRep() { 1054 if (getPriorRequest().isEmpty()) { 1055 addPriorRequest(); 1056 } 1057 return getPriorRequest().get(0); 1058 } 1059 1060 /** 1061 * @deprecated Use Reference#setResource(IBaseResource) instead 1062 */ 1063 @Deprecated 1064 public List<Resource> getPriorRequestTarget() { 1065 if (this.priorRequestTarget == null) 1066 this.priorRequestTarget = new ArrayList<Resource>(); 1067 return this.priorRequestTarget; 1068 } 1069 1070 /** 1071 * @return {@link #groupIdentifier} (Composite request this is part of.) 1072 */ 1073 public Identifier getGroupIdentifier() { 1074 if (this.groupIdentifier == null) 1075 if (Configuration.errorOnAutoCreate()) 1076 throw new Error("Attempt to auto-create DeviceRequest.groupIdentifier"); 1077 else if (Configuration.doAutoCreate()) 1078 this.groupIdentifier = new Identifier(); // cc 1079 return this.groupIdentifier; 1080 } 1081 1082 public boolean hasGroupIdentifier() { 1083 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1084 } 1085 1086 /** 1087 * @param value {@link #groupIdentifier} (Composite request this is part of.) 1088 */ 1089 public DeviceRequest setGroupIdentifier(Identifier value) { 1090 this.groupIdentifier = value; 1091 return this; 1092 } 1093 1094 /** 1095 * @return {@link #status} (The status of the request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1096 */ 1097 public Enumeration<DeviceRequestStatus> getStatusElement() { 1098 if (this.status == null) 1099 if (Configuration.errorOnAutoCreate()) 1100 throw new Error("Attempt to auto-create DeviceRequest.status"); 1101 else if (Configuration.doAutoCreate()) 1102 this.status = new Enumeration<DeviceRequestStatus>(new DeviceRequestStatusEnumFactory()); // bb 1103 return this.status; 1104 } 1105 1106 public boolean hasStatusElement() { 1107 return this.status != null && !this.status.isEmpty(); 1108 } 1109 1110 public boolean hasStatus() { 1111 return this.status != null && !this.status.isEmpty(); 1112 } 1113 1114 /** 1115 * @param value {@link #status} (The status of the request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1116 */ 1117 public DeviceRequest setStatusElement(Enumeration<DeviceRequestStatus> value) { 1118 this.status = value; 1119 return this; 1120 } 1121 1122 /** 1123 * @return The status of the request. 1124 */ 1125 public DeviceRequestStatus getStatus() { 1126 return this.status == null ? null : this.status.getValue(); 1127 } 1128 1129 /** 1130 * @param value The status of the request. 1131 */ 1132 public DeviceRequest setStatus(DeviceRequestStatus value) { 1133 if (value == null) 1134 this.status = null; 1135 else { 1136 if (this.status == null) 1137 this.status = new Enumeration<DeviceRequestStatus>(new DeviceRequestStatusEnumFactory()); 1138 this.status.setValue(value); 1139 } 1140 return this; 1141 } 1142 1143 /** 1144 * @return {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.) 1145 */ 1146 public CodeableConcept getIntent() { 1147 if (this.intent == null) 1148 if (Configuration.errorOnAutoCreate()) 1149 throw new Error("Attempt to auto-create DeviceRequest.intent"); 1150 else if (Configuration.doAutoCreate()) 1151 this.intent = new CodeableConcept(); // cc 1152 return this.intent; 1153 } 1154 1155 public boolean hasIntent() { 1156 return this.intent != null && !this.intent.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.) 1161 */ 1162 public DeviceRequest setIntent(CodeableConcept value) { 1163 this.intent = value; 1164 return this; 1165 } 1166 1167 /** 1168 * @return {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1169 */ 1170 public Enumeration<RequestPriority> getPriorityElement() { 1171 if (this.priority == null) 1172 if (Configuration.errorOnAutoCreate()) 1173 throw new Error("Attempt to auto-create DeviceRequest.priority"); 1174 else if (Configuration.doAutoCreate()) 1175 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 1176 return this.priority; 1177 } 1178 1179 public boolean hasPriorityElement() { 1180 return this.priority != null && !this.priority.isEmpty(); 1181 } 1182 1183 public boolean hasPriority() { 1184 return this.priority != null && !this.priority.isEmpty(); 1185 } 1186 1187 /** 1188 * @param value {@link #priority} (Indicates how quickly the {{title}} should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1189 */ 1190 public DeviceRequest setPriorityElement(Enumeration<RequestPriority> value) { 1191 this.priority = value; 1192 return this; 1193 } 1194 1195 /** 1196 * @return Indicates how quickly the {{title}} should be addressed with respect to other requests. 1197 */ 1198 public RequestPriority getPriority() { 1199 return this.priority == null ? null : this.priority.getValue(); 1200 } 1201 1202 /** 1203 * @param value Indicates how quickly the {{title}} should be addressed with respect to other requests. 1204 */ 1205 public DeviceRequest setPriority(RequestPriority value) { 1206 if (value == null) 1207 this.priority = null; 1208 else { 1209 if (this.priority == null) 1210 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 1211 this.priority.setValue(value); 1212 } 1213 return this; 1214 } 1215 1216 /** 1217 * @return {@link #code} (The details of the device to be used.) 1218 */ 1219 public Type getCode() { 1220 return this.code; 1221 } 1222 1223 /** 1224 * @return {@link #code} (The details of the device to be used.) 1225 */ 1226 public Reference getCodeReference() throws FHIRException { 1227 if (this.code == null) 1228 return null; 1229 if (!(this.code instanceof Reference)) 1230 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.code.getClass().getName()+" was encountered"); 1231 return (Reference) this.code; 1232 } 1233 1234 public boolean hasCodeReference() { 1235 return this != null && this.code instanceof Reference; 1236 } 1237 1238 /** 1239 * @return {@link #code} (The details of the device to be used.) 1240 */ 1241 public CodeableConcept getCodeCodeableConcept() throws FHIRException { 1242 if (this.code == null) 1243 return null; 1244 if (!(this.code instanceof CodeableConcept)) 1245 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.code.getClass().getName()+" was encountered"); 1246 return (CodeableConcept) this.code; 1247 } 1248 1249 public boolean hasCodeCodeableConcept() { 1250 return this != null && this.code instanceof CodeableConcept; 1251 } 1252 1253 public boolean hasCode() { 1254 return this.code != null && !this.code.isEmpty(); 1255 } 1256 1257 /** 1258 * @param value {@link #code} (The details of the device to be used.) 1259 */ 1260 public DeviceRequest setCode(Type value) throws FHIRFormatError { 1261 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1262 throw new FHIRFormatError("Not the right type for DeviceRequest.code[x]: "+value.fhirType()); 1263 this.code = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return {@link #subject} (The patient who will use the device.) 1269 */ 1270 public Reference getSubject() { 1271 if (this.subject == null) 1272 if (Configuration.errorOnAutoCreate()) 1273 throw new Error("Attempt to auto-create DeviceRequest.subject"); 1274 else if (Configuration.doAutoCreate()) 1275 this.subject = new Reference(); // cc 1276 return this.subject; 1277 } 1278 1279 public boolean hasSubject() { 1280 return this.subject != null && !this.subject.isEmpty(); 1281 } 1282 1283 /** 1284 * @param value {@link #subject} (The patient who will use the device.) 1285 */ 1286 public DeviceRequest setSubject(Reference value) { 1287 this.subject = value; 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who will use the device.) 1293 */ 1294 public Resource getSubjectTarget() { 1295 return this.subjectTarget; 1296 } 1297 1298 /** 1299 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who will use the device.) 1300 */ 1301 public DeviceRequest setSubjectTarget(Resource value) { 1302 this.subjectTarget = value; 1303 return this; 1304 } 1305 1306 /** 1307 * @return {@link #context} (An encounter that provides additional context in which this request is made.) 1308 */ 1309 public Reference getContext() { 1310 if (this.context == null) 1311 if (Configuration.errorOnAutoCreate()) 1312 throw new Error("Attempt to auto-create DeviceRequest.context"); 1313 else if (Configuration.doAutoCreate()) 1314 this.context = new Reference(); // cc 1315 return this.context; 1316 } 1317 1318 public boolean hasContext() { 1319 return this.context != null && !this.context.isEmpty(); 1320 } 1321 1322 /** 1323 * @param value {@link #context} (An encounter that provides additional context in which this request is made.) 1324 */ 1325 public DeviceRequest setContext(Reference value) { 1326 this.context = value; 1327 return this; 1328 } 1329 1330 /** 1331 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An encounter that provides additional context in which this request is made.) 1332 */ 1333 public Resource getContextTarget() { 1334 return this.contextTarget; 1335 } 1336 1337 /** 1338 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An encounter that provides additional context in which this request is made.) 1339 */ 1340 public DeviceRequest setContextTarget(Resource value) { 1341 this.contextTarget = value; 1342 return this; 1343 } 1344 1345 /** 1346 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1347 */ 1348 public Type getOccurrence() { 1349 return this.occurrence; 1350 } 1351 1352 /** 1353 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1354 */ 1355 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1356 if (this.occurrence == null) 1357 return null; 1358 if (!(this.occurrence instanceof DateTimeType)) 1359 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1360 return (DateTimeType) this.occurrence; 1361 } 1362 1363 public boolean hasOccurrenceDateTimeType() { 1364 return this != null && this.occurrence instanceof DateTimeType; 1365 } 1366 1367 /** 1368 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1369 */ 1370 public Period getOccurrencePeriod() throws FHIRException { 1371 if (this.occurrence == null) 1372 return null; 1373 if (!(this.occurrence instanceof Period)) 1374 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1375 return (Period) this.occurrence; 1376 } 1377 1378 public boolean hasOccurrencePeriod() { 1379 return this != null && this.occurrence instanceof Period; 1380 } 1381 1382 /** 1383 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1384 */ 1385 public Timing getOccurrenceTiming() throws FHIRException { 1386 if (this.occurrence == null) 1387 return null; 1388 if (!(this.occurrence instanceof Timing)) 1389 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1390 return (Timing) this.occurrence; 1391 } 1392 1393 public boolean hasOccurrenceTiming() { 1394 return this != null && this.occurrence instanceof Timing; 1395 } 1396 1397 public boolean hasOccurrence() { 1398 return this.occurrence != null && !this.occurrence.isEmpty(); 1399 } 1400 1401 /** 1402 * @param value {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1403 */ 1404 public DeviceRequest setOccurrence(Type value) throws FHIRFormatError { 1405 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1406 throw new FHIRFormatError("Not the right type for DeviceRequest.occurrence[x]: "+value.fhirType()); 1407 this.occurrence = value; 1408 return this; 1409 } 1410 1411 /** 1412 * @return {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1413 */ 1414 public DateTimeType getAuthoredOnElement() { 1415 if (this.authoredOn == null) 1416 if (Configuration.errorOnAutoCreate()) 1417 throw new Error("Attempt to auto-create DeviceRequest.authoredOn"); 1418 else if (Configuration.doAutoCreate()) 1419 this.authoredOn = new DateTimeType(); // bb 1420 return this.authoredOn; 1421 } 1422 1423 public boolean hasAuthoredOnElement() { 1424 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1425 } 1426 1427 public boolean hasAuthoredOn() { 1428 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1429 } 1430 1431 /** 1432 * @param value {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1433 */ 1434 public DeviceRequest setAuthoredOnElement(DateTimeType value) { 1435 this.authoredOn = value; 1436 return this; 1437 } 1438 1439 /** 1440 * @return When the request transitioned to being actionable. 1441 */ 1442 public Date getAuthoredOn() { 1443 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1444 } 1445 1446 /** 1447 * @param value When the request transitioned to being actionable. 1448 */ 1449 public DeviceRequest setAuthoredOn(Date value) { 1450 if (value == null) 1451 this.authoredOn = null; 1452 else { 1453 if (this.authoredOn == null) 1454 this.authoredOn = new DateTimeType(); 1455 this.authoredOn.setValue(value); 1456 } 1457 return this; 1458 } 1459 1460 /** 1461 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1462 */ 1463 public DeviceRequestRequesterComponent getRequester() { 1464 if (this.requester == null) 1465 if (Configuration.errorOnAutoCreate()) 1466 throw new Error("Attempt to auto-create DeviceRequest.requester"); 1467 else if (Configuration.doAutoCreate()) 1468 this.requester = new DeviceRequestRequesterComponent(); // cc 1469 return this.requester; 1470 } 1471 1472 public boolean hasRequester() { 1473 return this.requester != null && !this.requester.isEmpty(); 1474 } 1475 1476 /** 1477 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1478 */ 1479 public DeviceRequest setRequester(DeviceRequestRequesterComponent value) { 1480 this.requester = value; 1481 return this; 1482 } 1483 1484 /** 1485 * @return {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1486 */ 1487 public CodeableConcept getPerformerType() { 1488 if (this.performerType == null) 1489 if (Configuration.errorOnAutoCreate()) 1490 throw new Error("Attempt to auto-create DeviceRequest.performerType"); 1491 else if (Configuration.doAutoCreate()) 1492 this.performerType = new CodeableConcept(); // cc 1493 return this.performerType; 1494 } 1495 1496 public boolean hasPerformerType() { 1497 return this.performerType != null && !this.performerType.isEmpty(); 1498 } 1499 1500 /** 1501 * @param value {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1502 */ 1503 public DeviceRequest setPerformerType(CodeableConcept value) { 1504 this.performerType = value; 1505 return this; 1506 } 1507 1508 /** 1509 * @return {@link #performer} (The desired perfomer for doing the diagnostic testing.) 1510 */ 1511 public Reference getPerformer() { 1512 if (this.performer == null) 1513 if (Configuration.errorOnAutoCreate()) 1514 throw new Error("Attempt to auto-create DeviceRequest.performer"); 1515 else if (Configuration.doAutoCreate()) 1516 this.performer = new Reference(); // cc 1517 return this.performer; 1518 } 1519 1520 public boolean hasPerformer() { 1521 return this.performer != null && !this.performer.isEmpty(); 1522 } 1523 1524 /** 1525 * @param value {@link #performer} (The desired perfomer for doing the diagnostic testing.) 1526 */ 1527 public DeviceRequest setPerformer(Reference value) { 1528 this.performer = value; 1529 return this; 1530 } 1531 1532 /** 1533 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing.) 1534 */ 1535 public Resource getPerformerTarget() { 1536 return this.performerTarget; 1537 } 1538 1539 /** 1540 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing.) 1541 */ 1542 public DeviceRequest setPerformerTarget(Resource value) { 1543 this.performerTarget = value; 1544 return this; 1545 } 1546 1547 /** 1548 * @return {@link #reasonCode} (Reason or justification for the use of this device.) 1549 */ 1550 public List<CodeableConcept> getReasonCode() { 1551 if (this.reasonCode == null) 1552 this.reasonCode = new ArrayList<CodeableConcept>(); 1553 return this.reasonCode; 1554 } 1555 1556 /** 1557 * @return Returns a reference to <code>this</code> for easy method chaining 1558 */ 1559 public DeviceRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1560 this.reasonCode = theReasonCode; 1561 return this; 1562 } 1563 1564 public boolean hasReasonCode() { 1565 if (this.reasonCode == null) 1566 return false; 1567 for (CodeableConcept item : this.reasonCode) 1568 if (!item.isEmpty()) 1569 return true; 1570 return false; 1571 } 1572 1573 public CodeableConcept addReasonCode() { //3 1574 CodeableConcept t = new CodeableConcept(); 1575 if (this.reasonCode == null) 1576 this.reasonCode = new ArrayList<CodeableConcept>(); 1577 this.reasonCode.add(t); 1578 return t; 1579 } 1580 1581 public DeviceRequest addReasonCode(CodeableConcept t) { //3 1582 if (t == null) 1583 return this; 1584 if (this.reasonCode == null) 1585 this.reasonCode = new ArrayList<CodeableConcept>(); 1586 this.reasonCode.add(t); 1587 return this; 1588 } 1589 1590 /** 1591 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1592 */ 1593 public CodeableConcept getReasonCodeFirstRep() { 1594 if (getReasonCode().isEmpty()) { 1595 addReasonCode(); 1596 } 1597 return getReasonCode().get(0); 1598 } 1599 1600 /** 1601 * @return {@link #reasonReference} (Reason or justification for the use of this device.) 1602 */ 1603 public List<Reference> getReasonReference() { 1604 if (this.reasonReference == null) 1605 this.reasonReference = new ArrayList<Reference>(); 1606 return this.reasonReference; 1607 } 1608 1609 /** 1610 * @return Returns a reference to <code>this</code> for easy method chaining 1611 */ 1612 public DeviceRequest setReasonReference(List<Reference> theReasonReference) { 1613 this.reasonReference = theReasonReference; 1614 return this; 1615 } 1616 1617 public boolean hasReasonReference() { 1618 if (this.reasonReference == null) 1619 return false; 1620 for (Reference item : this.reasonReference) 1621 if (!item.isEmpty()) 1622 return true; 1623 return false; 1624 } 1625 1626 public Reference addReasonReference() { //3 1627 Reference t = new Reference(); 1628 if (this.reasonReference == null) 1629 this.reasonReference = new ArrayList<Reference>(); 1630 this.reasonReference.add(t); 1631 return t; 1632 } 1633 1634 public DeviceRequest addReasonReference(Reference t) { //3 1635 if (t == null) 1636 return this; 1637 if (this.reasonReference == null) 1638 this.reasonReference = new ArrayList<Reference>(); 1639 this.reasonReference.add(t); 1640 return this; 1641 } 1642 1643 /** 1644 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1645 */ 1646 public Reference getReasonReferenceFirstRep() { 1647 if (getReasonReference().isEmpty()) { 1648 addReasonReference(); 1649 } 1650 return getReasonReference().get(0); 1651 } 1652 1653 /** 1654 * @deprecated Use Reference#setResource(IBaseResource) instead 1655 */ 1656 @Deprecated 1657 public List<Resource> getReasonReferenceTarget() { 1658 if (this.reasonReferenceTarget == null) 1659 this.reasonReferenceTarget = new ArrayList<Resource>(); 1660 return this.reasonReferenceTarget; 1661 } 1662 1663 /** 1664 * @return {@link #supportingInfo} (Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).) 1665 */ 1666 public List<Reference> getSupportingInfo() { 1667 if (this.supportingInfo == null) 1668 this.supportingInfo = new ArrayList<Reference>(); 1669 return this.supportingInfo; 1670 } 1671 1672 /** 1673 * @return Returns a reference to <code>this</code> for easy method chaining 1674 */ 1675 public DeviceRequest setSupportingInfo(List<Reference> theSupportingInfo) { 1676 this.supportingInfo = theSupportingInfo; 1677 return this; 1678 } 1679 1680 public boolean hasSupportingInfo() { 1681 if (this.supportingInfo == null) 1682 return false; 1683 for (Reference item : this.supportingInfo) 1684 if (!item.isEmpty()) 1685 return true; 1686 return false; 1687 } 1688 1689 public Reference addSupportingInfo() { //3 1690 Reference t = new Reference(); 1691 if (this.supportingInfo == null) 1692 this.supportingInfo = new ArrayList<Reference>(); 1693 this.supportingInfo.add(t); 1694 return t; 1695 } 1696 1697 public DeviceRequest addSupportingInfo(Reference t) { //3 1698 if (t == null) 1699 return this; 1700 if (this.supportingInfo == null) 1701 this.supportingInfo = new ArrayList<Reference>(); 1702 this.supportingInfo.add(t); 1703 return this; 1704 } 1705 1706 /** 1707 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 1708 */ 1709 public Reference getSupportingInfoFirstRep() { 1710 if (getSupportingInfo().isEmpty()) { 1711 addSupportingInfo(); 1712 } 1713 return getSupportingInfo().get(0); 1714 } 1715 1716 /** 1717 * @deprecated Use Reference#setResource(IBaseResource) instead 1718 */ 1719 @Deprecated 1720 public List<Resource> getSupportingInfoTarget() { 1721 if (this.supportingInfoTarget == null) 1722 this.supportingInfoTarget = new ArrayList<Resource>(); 1723 return this.supportingInfoTarget; 1724 } 1725 1726 /** 1727 * @return {@link #note} (Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.) 1728 */ 1729 public List<Annotation> getNote() { 1730 if (this.note == null) 1731 this.note = new ArrayList<Annotation>(); 1732 return this.note; 1733 } 1734 1735 /** 1736 * @return Returns a reference to <code>this</code> for easy method chaining 1737 */ 1738 public DeviceRequest setNote(List<Annotation> theNote) { 1739 this.note = theNote; 1740 return this; 1741 } 1742 1743 public boolean hasNote() { 1744 if (this.note == null) 1745 return false; 1746 for (Annotation item : this.note) 1747 if (!item.isEmpty()) 1748 return true; 1749 return false; 1750 } 1751 1752 public Annotation addNote() { //3 1753 Annotation t = new Annotation(); 1754 if (this.note == null) 1755 this.note = new ArrayList<Annotation>(); 1756 this.note.add(t); 1757 return t; 1758 } 1759 1760 public DeviceRequest addNote(Annotation t) { //3 1761 if (t == null) 1762 return this; 1763 if (this.note == null) 1764 this.note = new ArrayList<Annotation>(); 1765 this.note.add(t); 1766 return this; 1767 } 1768 1769 /** 1770 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1771 */ 1772 public Annotation getNoteFirstRep() { 1773 if (getNote().isEmpty()) { 1774 addNote(); 1775 } 1776 return getNote().get(0); 1777 } 1778 1779 /** 1780 * @return {@link #relevantHistory} (Key events in the history of the request.) 1781 */ 1782 public List<Reference> getRelevantHistory() { 1783 if (this.relevantHistory == null) 1784 this.relevantHistory = new ArrayList<Reference>(); 1785 return this.relevantHistory; 1786 } 1787 1788 /** 1789 * @return Returns a reference to <code>this</code> for easy method chaining 1790 */ 1791 public DeviceRequest setRelevantHistory(List<Reference> theRelevantHistory) { 1792 this.relevantHistory = theRelevantHistory; 1793 return this; 1794 } 1795 1796 public boolean hasRelevantHistory() { 1797 if (this.relevantHistory == null) 1798 return false; 1799 for (Reference item : this.relevantHistory) 1800 if (!item.isEmpty()) 1801 return true; 1802 return false; 1803 } 1804 1805 public Reference addRelevantHistory() { //3 1806 Reference t = new Reference(); 1807 if (this.relevantHistory == null) 1808 this.relevantHistory = new ArrayList<Reference>(); 1809 this.relevantHistory.add(t); 1810 return t; 1811 } 1812 1813 public DeviceRequest addRelevantHistory(Reference t) { //3 1814 if (t == null) 1815 return this; 1816 if (this.relevantHistory == null) 1817 this.relevantHistory = new ArrayList<Reference>(); 1818 this.relevantHistory.add(t); 1819 return this; 1820 } 1821 1822 /** 1823 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 1824 */ 1825 public Reference getRelevantHistoryFirstRep() { 1826 if (getRelevantHistory().isEmpty()) { 1827 addRelevantHistory(); 1828 } 1829 return getRelevantHistory().get(0); 1830 } 1831 1832 /** 1833 * @deprecated Use Reference#setResource(IBaseResource) instead 1834 */ 1835 @Deprecated 1836 public List<Provenance> getRelevantHistoryTarget() { 1837 if (this.relevantHistoryTarget == null) 1838 this.relevantHistoryTarget = new ArrayList<Provenance>(); 1839 return this.relevantHistoryTarget; 1840 } 1841 1842 /** 1843 * @deprecated Use Reference#setResource(IBaseResource) instead 1844 */ 1845 @Deprecated 1846 public Provenance addRelevantHistoryTarget() { 1847 Provenance r = new Provenance(); 1848 if (this.relevantHistoryTarget == null) 1849 this.relevantHistoryTarget = new ArrayList<Provenance>(); 1850 this.relevantHistoryTarget.add(r); 1851 return r; 1852 } 1853 1854 protected void listChildren(List<Property> children) { 1855 super.listChildren(children); 1856 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1857 children.add(new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.", 0, java.lang.Integer.MAX_VALUE, definition)); 1858 children.add(new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1859 children.add(new Property("priorRequest", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, priorRequest)); 1860 children.add(new Property("groupIdentifier", "Identifier", "Composite request this is part of.", 0, 1, groupIdentifier)); 1861 children.add(new Property("status", "code", "The status of the request.", 0, 1, status)); 1862 children.add(new Property("intent", "CodeableConcept", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 1863 children.add(new Property("priority", "code", "Indicates how quickly the {{title}} should be addressed with respect to other requests.", 0, 1, priority)); 1864 children.add(new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code)); 1865 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", "The patient who will use the device.", 0, 1, subject)); 1866 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter that provides additional context in which this request is made.", 0, 1, context)); 1867 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence)); 1868 children.add(new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 1869 children.add(new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 1870 children.add(new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType)); 1871 children.add(new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing.", 0, 1, performer)); 1872 children.add(new Property("reasonCode", "CodeableConcept", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1873 children.add(new Property("reasonReference", "Reference(Any)", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1874 children.add(new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 1875 children.add(new Property("note", "Annotation", "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note)); 1876 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 1877 } 1878 1879 @Override 1880 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1881 switch (_hash) { 1882 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, identifier); 1883 case -1014418093: /*definition*/ return new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request. For example: The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.", 0, java.lang.Integer.MAX_VALUE, definition); 1884 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1885 case 237568101: /*priorRequest*/ return new Property("priorRequest", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, priorRequest); 1886 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "Composite request this is part of.", 0, 1, groupIdentifier); 1887 case -892481550: /*status*/ return new Property("status", "code", "The status of the request.", 0, 1, status); 1888 case -1183762788: /*intent*/ return new Property("intent", "CodeableConcept", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 1889 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the {{title}} should be addressed with respect to other requests.", 0, 1, priority); 1890 case 941839219: /*code[x]*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1891 case 3059181: /*code*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1892 case 1565461470: /*codeReference*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1893 case 4899316: /*codeCodeableConcept*/ return new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 0, 1, code); 1894 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Location|Device)", "The patient who will use the device.", 0, 1, subject); 1895 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter that provides additional context in which this request is made.", 0, 1, context); 1896 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1897 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1898 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1899 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1900 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1901 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn); 1902 case 693933948: /*requester*/ return new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 1903 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType); 1904 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing.", 0, 1, performer); 1905 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1906 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Any)", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1907 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient that may influence the request fulfilment. For example, this may includes body where on the subject's the device will be used ( i.e. the target site).", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 1908 case 3387378: /*note*/ return new Property("note", "Annotation", "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note); 1909 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 1910 default: return super.getNamedProperty(_hash, _name, _checkValid); 1911 } 1912 1913 } 1914 1915 @Override 1916 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1917 switch (hash) { 1918 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1919 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 1920 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1921 case 237568101: /*priorRequest*/ return this.priorRequest == null ? new Base[0] : this.priorRequest.toArray(new Base[this.priorRequest.size()]); // Reference 1922 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 1923 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DeviceRequestStatus> 1924 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // CodeableConcept 1925 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1926 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Type 1927 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1928 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1929 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 1930 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 1931 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // DeviceRequestRequesterComponent 1932 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 1933 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 1934 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1935 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1936 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 1937 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1938 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 1939 default: return super.getProperty(hash, name, checkValid); 1940 } 1941 1942 } 1943 1944 @Override 1945 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1946 switch (hash) { 1947 case -1618432855: // identifier 1948 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1949 return value; 1950 case -1014418093: // definition 1951 this.getDefinition().add(castToReference(value)); // Reference 1952 return value; 1953 case -332612366: // basedOn 1954 this.getBasedOn().add(castToReference(value)); // Reference 1955 return value; 1956 case 237568101: // priorRequest 1957 this.getPriorRequest().add(castToReference(value)); // Reference 1958 return value; 1959 case -445338488: // groupIdentifier 1960 this.groupIdentifier = castToIdentifier(value); // Identifier 1961 return value; 1962 case -892481550: // status 1963 value = new DeviceRequestStatusEnumFactory().fromType(castToCode(value)); 1964 this.status = (Enumeration) value; // Enumeration<DeviceRequestStatus> 1965 return value; 1966 case -1183762788: // intent 1967 this.intent = castToCodeableConcept(value); // CodeableConcept 1968 return value; 1969 case -1165461084: // priority 1970 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1971 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1972 return value; 1973 case 3059181: // code 1974 this.code = castToType(value); // Type 1975 return value; 1976 case -1867885268: // subject 1977 this.subject = castToReference(value); // Reference 1978 return value; 1979 case 951530927: // context 1980 this.context = castToReference(value); // Reference 1981 return value; 1982 case 1687874001: // occurrence 1983 this.occurrence = castToType(value); // Type 1984 return value; 1985 case -1500852503: // authoredOn 1986 this.authoredOn = castToDateTime(value); // DateTimeType 1987 return value; 1988 case 693933948: // requester 1989 this.requester = (DeviceRequestRequesterComponent) value; // DeviceRequestRequesterComponent 1990 return value; 1991 case -901444568: // performerType 1992 this.performerType = castToCodeableConcept(value); // CodeableConcept 1993 return value; 1994 case 481140686: // performer 1995 this.performer = castToReference(value); // Reference 1996 return value; 1997 case 722137681: // reasonCode 1998 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1999 return value; 2000 case -1146218137: // reasonReference 2001 this.getReasonReference().add(castToReference(value)); // Reference 2002 return value; 2003 case 1922406657: // supportingInfo 2004 this.getSupportingInfo().add(castToReference(value)); // Reference 2005 return value; 2006 case 3387378: // note 2007 this.getNote().add(castToAnnotation(value)); // Annotation 2008 return value; 2009 case 1538891575: // relevantHistory 2010 this.getRelevantHistory().add(castToReference(value)); // Reference 2011 return value; 2012 default: return super.setProperty(hash, name, value); 2013 } 2014 2015 } 2016 2017 @Override 2018 public Base setProperty(String name, Base value) throws FHIRException { 2019 if (name.equals("identifier")) { 2020 this.getIdentifier().add(castToIdentifier(value)); 2021 } else if (name.equals("definition")) { 2022 this.getDefinition().add(castToReference(value)); 2023 } else if (name.equals("basedOn")) { 2024 this.getBasedOn().add(castToReference(value)); 2025 } else if (name.equals("priorRequest")) { 2026 this.getPriorRequest().add(castToReference(value)); 2027 } else if (name.equals("groupIdentifier")) { 2028 this.groupIdentifier = castToIdentifier(value); // Identifier 2029 } else if (name.equals("status")) { 2030 value = new DeviceRequestStatusEnumFactory().fromType(castToCode(value)); 2031 this.status = (Enumeration) value; // Enumeration<DeviceRequestStatus> 2032 } else if (name.equals("intent")) { 2033 this.intent = castToCodeableConcept(value); // CodeableConcept 2034 } else if (name.equals("priority")) { 2035 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 2036 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 2037 } else if (name.equals("code[x]")) { 2038 this.code = castToType(value); // Type 2039 } else if (name.equals("subject")) { 2040 this.subject = castToReference(value); // Reference 2041 } else if (name.equals("context")) { 2042 this.context = castToReference(value); // Reference 2043 } else if (name.equals("occurrence[x]")) { 2044 this.occurrence = castToType(value); // Type 2045 } else if (name.equals("authoredOn")) { 2046 this.authoredOn = castToDateTime(value); // DateTimeType 2047 } else if (name.equals("requester")) { 2048 this.requester = (DeviceRequestRequesterComponent) value; // DeviceRequestRequesterComponent 2049 } else if (name.equals("performerType")) { 2050 this.performerType = castToCodeableConcept(value); // CodeableConcept 2051 } else if (name.equals("performer")) { 2052 this.performer = castToReference(value); // Reference 2053 } else if (name.equals("reasonCode")) { 2054 this.getReasonCode().add(castToCodeableConcept(value)); 2055 } else if (name.equals("reasonReference")) { 2056 this.getReasonReference().add(castToReference(value)); 2057 } else if (name.equals("supportingInfo")) { 2058 this.getSupportingInfo().add(castToReference(value)); 2059 } else if (name.equals("note")) { 2060 this.getNote().add(castToAnnotation(value)); 2061 } else if (name.equals("relevantHistory")) { 2062 this.getRelevantHistory().add(castToReference(value)); 2063 } else 2064 return super.setProperty(name, value); 2065 return value; 2066 } 2067 2068 @Override 2069 public Base makeProperty(int hash, String name) throws FHIRException { 2070 switch (hash) { 2071 case -1618432855: return addIdentifier(); 2072 case -1014418093: return addDefinition(); 2073 case -332612366: return addBasedOn(); 2074 case 237568101: return addPriorRequest(); 2075 case -445338488: return getGroupIdentifier(); 2076 case -892481550: return getStatusElement(); 2077 case -1183762788: return getIntent(); 2078 case -1165461084: return getPriorityElement(); 2079 case 941839219: return getCode(); 2080 case 3059181: return getCode(); 2081 case -1867885268: return getSubject(); 2082 case 951530927: return getContext(); 2083 case -2022646513: return getOccurrence(); 2084 case 1687874001: return getOccurrence(); 2085 case -1500852503: return getAuthoredOnElement(); 2086 case 693933948: return getRequester(); 2087 case -901444568: return getPerformerType(); 2088 case 481140686: return getPerformer(); 2089 case 722137681: return addReasonCode(); 2090 case -1146218137: return addReasonReference(); 2091 case 1922406657: return addSupportingInfo(); 2092 case 3387378: return addNote(); 2093 case 1538891575: return addRelevantHistory(); 2094 default: return super.makeProperty(hash, name); 2095 } 2096 2097 } 2098 2099 @Override 2100 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2101 switch (hash) { 2102 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2103 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2104 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2105 case 237568101: /*priorRequest*/ return new String[] {"Reference"}; 2106 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 2107 case -892481550: /*status*/ return new String[] {"code"}; 2108 case -1183762788: /*intent*/ return new String[] {"CodeableConcept"}; 2109 case -1165461084: /*priority*/ return new String[] {"code"}; 2110 case 3059181: /*code*/ return new String[] {"Reference", "CodeableConcept"}; 2111 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2112 case 951530927: /*context*/ return new String[] {"Reference"}; 2113 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 2114 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 2115 case 693933948: /*requester*/ return new String[] {}; 2116 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 2117 case 481140686: /*performer*/ return new String[] {"Reference"}; 2118 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2119 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2120 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2121 case 3387378: /*note*/ return new String[] {"Annotation"}; 2122 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 2123 default: return super.getTypesForProperty(hash, name); 2124 } 2125 2126 } 2127 2128 @Override 2129 public Base addChild(String name) throws FHIRException { 2130 if (name.equals("identifier")) { 2131 return addIdentifier(); 2132 } 2133 else if (name.equals("definition")) { 2134 return addDefinition(); 2135 } 2136 else if (name.equals("basedOn")) { 2137 return addBasedOn(); 2138 } 2139 else if (name.equals("priorRequest")) { 2140 return addPriorRequest(); 2141 } 2142 else if (name.equals("groupIdentifier")) { 2143 this.groupIdentifier = new Identifier(); 2144 return this.groupIdentifier; 2145 } 2146 else if (name.equals("status")) { 2147 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.status"); 2148 } 2149 else if (name.equals("intent")) { 2150 this.intent = new CodeableConcept(); 2151 return this.intent; 2152 } 2153 else if (name.equals("priority")) { 2154 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.priority"); 2155 } 2156 else if (name.equals("codeReference")) { 2157 this.code = new Reference(); 2158 return this.code; 2159 } 2160 else if (name.equals("codeCodeableConcept")) { 2161 this.code = new CodeableConcept(); 2162 return this.code; 2163 } 2164 else if (name.equals("subject")) { 2165 this.subject = new Reference(); 2166 return this.subject; 2167 } 2168 else if (name.equals("context")) { 2169 this.context = new Reference(); 2170 return this.context; 2171 } 2172 else if (name.equals("occurrenceDateTime")) { 2173 this.occurrence = new DateTimeType(); 2174 return this.occurrence; 2175 } 2176 else if (name.equals("occurrencePeriod")) { 2177 this.occurrence = new Period(); 2178 return this.occurrence; 2179 } 2180 else if (name.equals("occurrenceTiming")) { 2181 this.occurrence = new Timing(); 2182 return this.occurrence; 2183 } 2184 else if (name.equals("authoredOn")) { 2185 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.authoredOn"); 2186 } 2187 else if (name.equals("requester")) { 2188 this.requester = new DeviceRequestRequesterComponent(); 2189 return this.requester; 2190 } 2191 else if (name.equals("performerType")) { 2192 this.performerType = new CodeableConcept(); 2193 return this.performerType; 2194 } 2195 else if (name.equals("performer")) { 2196 this.performer = new Reference(); 2197 return this.performer; 2198 } 2199 else if (name.equals("reasonCode")) { 2200 return addReasonCode(); 2201 } 2202 else if (name.equals("reasonReference")) { 2203 return addReasonReference(); 2204 } 2205 else if (name.equals("supportingInfo")) { 2206 return addSupportingInfo(); 2207 } 2208 else if (name.equals("note")) { 2209 return addNote(); 2210 } 2211 else if (name.equals("relevantHistory")) { 2212 return addRelevantHistory(); 2213 } 2214 else 2215 return super.addChild(name); 2216 } 2217 2218 public String fhirType() { 2219 return "DeviceRequest"; 2220 2221 } 2222 2223 public DeviceRequest copy() { 2224 DeviceRequest dst = new DeviceRequest(); 2225 copyValues(dst); 2226 if (identifier != null) { 2227 dst.identifier = new ArrayList<Identifier>(); 2228 for (Identifier i : identifier) 2229 dst.identifier.add(i.copy()); 2230 }; 2231 if (definition != null) { 2232 dst.definition = new ArrayList<Reference>(); 2233 for (Reference i : definition) 2234 dst.definition.add(i.copy()); 2235 }; 2236 if (basedOn != null) { 2237 dst.basedOn = new ArrayList<Reference>(); 2238 for (Reference i : basedOn) 2239 dst.basedOn.add(i.copy()); 2240 }; 2241 if (priorRequest != null) { 2242 dst.priorRequest = new ArrayList<Reference>(); 2243 for (Reference i : priorRequest) 2244 dst.priorRequest.add(i.copy()); 2245 }; 2246 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2247 dst.status = status == null ? null : status.copy(); 2248 dst.intent = intent == null ? null : intent.copy(); 2249 dst.priority = priority == null ? null : priority.copy(); 2250 dst.code = code == null ? null : code.copy(); 2251 dst.subject = subject == null ? null : subject.copy(); 2252 dst.context = context == null ? null : context.copy(); 2253 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2254 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2255 dst.requester = requester == null ? null : requester.copy(); 2256 dst.performerType = performerType == null ? null : performerType.copy(); 2257 dst.performer = performer == null ? null : performer.copy(); 2258 if (reasonCode != null) { 2259 dst.reasonCode = new ArrayList<CodeableConcept>(); 2260 for (CodeableConcept i : reasonCode) 2261 dst.reasonCode.add(i.copy()); 2262 }; 2263 if (reasonReference != null) { 2264 dst.reasonReference = new ArrayList<Reference>(); 2265 for (Reference i : reasonReference) 2266 dst.reasonReference.add(i.copy()); 2267 }; 2268 if (supportingInfo != null) { 2269 dst.supportingInfo = new ArrayList<Reference>(); 2270 for (Reference i : supportingInfo) 2271 dst.supportingInfo.add(i.copy()); 2272 }; 2273 if (note != null) { 2274 dst.note = new ArrayList<Annotation>(); 2275 for (Annotation i : note) 2276 dst.note.add(i.copy()); 2277 }; 2278 if (relevantHistory != null) { 2279 dst.relevantHistory = new ArrayList<Reference>(); 2280 for (Reference i : relevantHistory) 2281 dst.relevantHistory.add(i.copy()); 2282 }; 2283 return dst; 2284 } 2285 2286 protected DeviceRequest typedCopy() { 2287 return copy(); 2288 } 2289 2290 @Override 2291 public boolean equalsDeep(Base other_) { 2292 if (!super.equalsDeep(other_)) 2293 return false; 2294 if (!(other_ instanceof DeviceRequest)) 2295 return false; 2296 DeviceRequest o = (DeviceRequest) other_; 2297 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2298 && compareDeep(basedOn, o.basedOn, true) && compareDeep(priorRequest, o.priorRequest, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 2299 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 2300 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 2301 && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 2302 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 2303 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2304 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 2305 ; 2306 } 2307 2308 @Override 2309 public boolean equalsShallow(Base other_) { 2310 if (!super.equalsShallow(other_)) 2311 return false; 2312 if (!(other_ instanceof DeviceRequest)) 2313 return false; 2314 DeviceRequest o = (DeviceRequest) other_; 2315 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) && compareValues(authoredOn, o.authoredOn, true) 2316 ; 2317 } 2318 2319 public boolean isEmpty() { 2320 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2321 , priorRequest, groupIdentifier, status, intent, priority, code, subject, context 2322 , occurrence, authoredOn, requester, performerType, performer, reasonCode, reasonReference 2323 , supportingInfo, note, relevantHistory); 2324 } 2325 2326 @Override 2327 public ResourceType getResourceType() { 2328 return ResourceType.DeviceRequest; 2329 } 2330 2331 /** 2332 * Search parameter: <b>requester</b> 2333 * <p> 2334 * Description: <b>Who/what is requesting service </b><br> 2335 * Type: <b>reference</b><br> 2336 * Path: <b>DeviceRequest.requester.agent</b><br> 2337 * </p> 2338 */ 2339 @SearchParamDefinition(name="requester", path="DeviceRequest.requester.agent", description="Who/what is requesting service ", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Practitioner.class } ) 2340 public static final String SP_REQUESTER = "requester"; 2341 /** 2342 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2343 * <p> 2344 * Description: <b>Who/what is requesting service </b><br> 2345 * Type: <b>reference</b><br> 2346 * Path: <b>DeviceRequest.requester.agent</b><br> 2347 * </p> 2348 */ 2349 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2350 2351/** 2352 * Constant for fluent queries to be used to add include statements. Specifies 2353 * the path value of "<b>DeviceRequest:requester</b>". 2354 */ 2355 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("DeviceRequest:requester").toLocked(); 2356 2357 /** 2358 * Search parameter: <b>identifier</b> 2359 * <p> 2360 * Description: <b>Business identifier for request/order</b><br> 2361 * Type: <b>token</b><br> 2362 * Path: <b>DeviceRequest.identifier</b><br> 2363 * </p> 2364 */ 2365 @SearchParamDefinition(name="identifier", path="DeviceRequest.identifier", description="Business identifier for request/order", type="token" ) 2366 public static final String SP_IDENTIFIER = "identifier"; 2367 /** 2368 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2369 * <p> 2370 * Description: <b>Business identifier for request/order</b><br> 2371 * Type: <b>token</b><br> 2372 * Path: <b>DeviceRequest.identifier</b><br> 2373 * </p> 2374 */ 2375 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2376 2377 /** 2378 * Search parameter: <b>code</b> 2379 * <p> 2380 * Description: <b>Code for what is being requested/ordered</b><br> 2381 * Type: <b>token</b><br> 2382 * Path: <b>DeviceRequest.codeCodeableConcept</b><br> 2383 * </p> 2384 */ 2385 @SearchParamDefinition(name="code", path="DeviceRequest.code.as(CodeableConcept)", description="Code for what is being requested/ordered", type="token" ) 2386 public static final String SP_CODE = "code"; 2387 /** 2388 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2389 * <p> 2390 * Description: <b>Code for what is being requested/ordered</b><br> 2391 * Type: <b>token</b><br> 2392 * Path: <b>DeviceRequest.codeCodeableConcept</b><br> 2393 * </p> 2394 */ 2395 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2396 2397 /** 2398 * Search parameter: <b>performer</b> 2399 * <p> 2400 * Description: <b>Desired performer for service</b><br> 2401 * Type: <b>reference</b><br> 2402 * Path: <b>DeviceRequest.performer</b><br> 2403 * </p> 2404 */ 2405 @SearchParamDefinition(name="performer", path="DeviceRequest.performer", description="Desired performer for service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2406 public static final String SP_PERFORMER = "performer"; 2407 /** 2408 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2409 * <p> 2410 * Description: <b>Desired performer for service</b><br> 2411 * Type: <b>reference</b><br> 2412 * Path: <b>DeviceRequest.performer</b><br> 2413 * </p> 2414 */ 2415 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2416 2417/** 2418 * Constant for fluent queries to be used to add include statements. Specifies 2419 * the path value of "<b>DeviceRequest:performer</b>". 2420 */ 2421 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("DeviceRequest:performer").toLocked(); 2422 2423 /** 2424 * Search parameter: <b>event-date</b> 2425 * <p> 2426 * Description: <b>When service should occur</b><br> 2427 * Type: <b>date</b><br> 2428 * Path: <b>DeviceRequest.occurrenceDateTime, DeviceRequest.occurrencePeriod</b><br> 2429 * </p> 2430 */ 2431 @SearchParamDefinition(name="event-date", path="DeviceRequest.occurrence.as(DateTime) | DeviceRequest.occurrence.as(Period)", description="When service should occur", type="date" ) 2432 public static final String SP_EVENT_DATE = "event-date"; 2433 /** 2434 * <b>Fluent Client</b> search parameter constant for <b>event-date</b> 2435 * <p> 2436 * Description: <b>When service should occur</b><br> 2437 * Type: <b>date</b><br> 2438 * Path: <b>DeviceRequest.occurrenceDateTime, DeviceRequest.occurrencePeriod</b><br> 2439 * </p> 2440 */ 2441 public static final ca.uhn.fhir.rest.gclient.DateClientParam EVENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EVENT_DATE); 2442 2443 /** 2444 * Search parameter: <b>subject</b> 2445 * <p> 2446 * Description: <b>Individual the service is ordered for</b><br> 2447 * Type: <b>reference</b><br> 2448 * Path: <b>DeviceRequest.subject</b><br> 2449 * </p> 2450 */ 2451 @SearchParamDefinition(name="subject", path="DeviceRequest.subject", description="Individual the service is ordered for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 2452 public static final String SP_SUBJECT = "subject"; 2453 /** 2454 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2455 * <p> 2456 * Description: <b>Individual the service is ordered for</b><br> 2457 * Type: <b>reference</b><br> 2458 * Path: <b>DeviceRequest.subject</b><br> 2459 * </p> 2460 */ 2461 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2462 2463/** 2464 * Constant for fluent queries to be used to add include statements. Specifies 2465 * the path value of "<b>DeviceRequest:subject</b>". 2466 */ 2467 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DeviceRequest:subject").toLocked(); 2468 2469 /** 2470 * Search parameter: <b>encounter</b> 2471 * <p> 2472 * Description: <b>Encounter or Episode during which request was created</b><br> 2473 * Type: <b>reference</b><br> 2474 * Path: <b>DeviceRequest.context</b><br> 2475 * </p> 2476 */ 2477 @SearchParamDefinition(name="encounter", path="DeviceRequest.context", description="Encounter or Episode during which request was created", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2478 public static final String SP_ENCOUNTER = "encounter"; 2479 /** 2480 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2481 * <p> 2482 * Description: <b>Encounter or Episode during which request was created</b><br> 2483 * Type: <b>reference</b><br> 2484 * Path: <b>DeviceRequest.context</b><br> 2485 * </p> 2486 */ 2487 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2488 2489/** 2490 * Constant for fluent queries to be used to add include statements. Specifies 2491 * the path value of "<b>DeviceRequest:encounter</b>". 2492 */ 2493 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("DeviceRequest:encounter").toLocked(); 2494 2495 /** 2496 * Search parameter: <b>authored-on</b> 2497 * <p> 2498 * Description: <b>When the request transitioned to being actionable</b><br> 2499 * Type: <b>date</b><br> 2500 * Path: <b>DeviceRequest.authoredOn</b><br> 2501 * </p> 2502 */ 2503 @SearchParamDefinition(name="authored-on", path="DeviceRequest.authoredOn", description="When the request transitioned to being actionable", type="date" ) 2504 public static final String SP_AUTHORED_ON = "authored-on"; 2505 /** 2506 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 2507 * <p> 2508 * Description: <b>When the request transitioned to being actionable</b><br> 2509 * Type: <b>date</b><br> 2510 * Path: <b>DeviceRequest.authoredOn</b><br> 2511 * </p> 2512 */ 2513 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED_ON); 2514 2515 /** 2516 * Search parameter: <b>intent</b> 2517 * <p> 2518 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 2519 * Type: <b>token</b><br> 2520 * Path: <b>DeviceRequest.intent</b><br> 2521 * </p> 2522 */ 2523 @SearchParamDefinition(name="intent", path="DeviceRequest.intent", description="proposal | plan | original-order |reflex-order", type="token" ) 2524 public static final String SP_INTENT = "intent"; 2525 /** 2526 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 2527 * <p> 2528 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 2529 * Type: <b>token</b><br> 2530 * Path: <b>DeviceRequest.intent</b><br> 2531 * </p> 2532 */ 2533 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 2534 2535 /** 2536 * Search parameter: <b>group-identifier</b> 2537 * <p> 2538 * Description: <b>Composite request this is part of</b><br> 2539 * Type: <b>token</b><br> 2540 * Path: <b>DeviceRequest.groupIdentifier</b><br> 2541 * </p> 2542 */ 2543 @SearchParamDefinition(name="group-identifier", path="DeviceRequest.groupIdentifier", description="Composite request this is part of", type="token" ) 2544 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 2545 /** 2546 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 2547 * <p> 2548 * Description: <b>Composite request this is part of</b><br> 2549 * Type: <b>token</b><br> 2550 * Path: <b>DeviceRequest.groupIdentifier</b><br> 2551 * </p> 2552 */ 2553 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 2554 2555 /** 2556 * Search parameter: <b>based-on</b> 2557 * <p> 2558 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 2559 * Type: <b>reference</b><br> 2560 * Path: <b>DeviceRequest.basedOn</b><br> 2561 * </p> 2562 */ 2563 @SearchParamDefinition(name="based-on", path="DeviceRequest.basedOn", description="Plan/proposal/order fulfilled by this request", type="reference" ) 2564 public static final String SP_BASED_ON = "based-on"; 2565 /** 2566 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2567 * <p> 2568 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 2569 * Type: <b>reference</b><br> 2570 * Path: <b>DeviceRequest.basedOn</b><br> 2571 * </p> 2572 */ 2573 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2574 2575/** 2576 * Constant for fluent queries to be used to add include statements. Specifies 2577 * the path value of "<b>DeviceRequest:based-on</b>". 2578 */ 2579 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("DeviceRequest:based-on").toLocked(); 2580 2581 /** 2582 * Search parameter: <b>priorrequest</b> 2583 * <p> 2584 * Description: <b>Request takes the place of referenced completed or terminated requests</b><br> 2585 * Type: <b>reference</b><br> 2586 * Path: <b>DeviceRequest.priorRequest</b><br> 2587 * </p> 2588 */ 2589 @SearchParamDefinition(name="priorrequest", path="DeviceRequest.priorRequest", description="Request takes the place of referenced completed or terminated requests", type="reference" ) 2590 public static final String SP_PRIORREQUEST = "priorrequest"; 2591 /** 2592 * <b>Fluent Client</b> search parameter constant for <b>priorrequest</b> 2593 * <p> 2594 * Description: <b>Request takes the place of referenced completed or terminated requests</b><br> 2595 * Type: <b>reference</b><br> 2596 * Path: <b>DeviceRequest.priorRequest</b><br> 2597 * </p> 2598 */ 2599 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRIORREQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRIORREQUEST); 2600 2601/** 2602 * Constant for fluent queries to be used to add include statements. Specifies 2603 * the path value of "<b>DeviceRequest:priorrequest</b>". 2604 */ 2605 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRIORREQUEST = new ca.uhn.fhir.model.api.Include("DeviceRequest:priorrequest").toLocked(); 2606 2607 /** 2608 * Search parameter: <b>patient</b> 2609 * <p> 2610 * Description: <b>Individual the service is ordered for</b><br> 2611 * Type: <b>reference</b><br> 2612 * Path: <b>DeviceRequest.subject</b><br> 2613 * </p> 2614 */ 2615 @SearchParamDefinition(name="patient", path="DeviceRequest.subject", description="Individual the service is ordered for", type="reference", target={Patient.class } ) 2616 public static final String SP_PATIENT = "patient"; 2617 /** 2618 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2619 * <p> 2620 * Description: <b>Individual the service is ordered for</b><br> 2621 * Type: <b>reference</b><br> 2622 * Path: <b>DeviceRequest.subject</b><br> 2623 * </p> 2624 */ 2625 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2626 2627/** 2628 * Constant for fluent queries to be used to add include statements. Specifies 2629 * the path value of "<b>DeviceRequest:patient</b>". 2630 */ 2631 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceRequest:patient").toLocked(); 2632 2633 /** 2634 * Search parameter: <b>definition</b> 2635 * <p> 2636 * Description: <b>Protocol or definition followed by this request</b><br> 2637 * Type: <b>reference</b><br> 2638 * Path: <b>DeviceRequest.definition</b><br> 2639 * </p> 2640 */ 2641 @SearchParamDefinition(name="definition", path="DeviceRequest.definition", description="Protocol or definition followed by this request", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 2642 public static final String SP_DEFINITION = "definition"; 2643 /** 2644 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 2645 * <p> 2646 * Description: <b>Protocol or definition followed by this request</b><br> 2647 * Type: <b>reference</b><br> 2648 * Path: <b>DeviceRequest.definition</b><br> 2649 * </p> 2650 */ 2651 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 2652 2653/** 2654 * Constant for fluent queries to be used to add include statements. Specifies 2655 * the path value of "<b>DeviceRequest:definition</b>". 2656 */ 2657 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("DeviceRequest:definition").toLocked(); 2658 2659 /** 2660 * Search parameter: <b>device</b> 2661 * <p> 2662 * Description: <b>Reference to resource that is being requested/ordered</b><br> 2663 * Type: <b>reference</b><br> 2664 * Path: <b>DeviceRequest.codeReference</b><br> 2665 * </p> 2666 */ 2667 @SearchParamDefinition(name="device", path="DeviceRequest.code.as(Reference)", description="Reference to resource that is being requested/ordered", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class } ) 2668 public static final String SP_DEVICE = "device"; 2669 /** 2670 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2671 * <p> 2672 * Description: <b>Reference to resource that is being requested/ordered</b><br> 2673 * Type: <b>reference</b><br> 2674 * Path: <b>DeviceRequest.codeReference</b><br> 2675 * </p> 2676 */ 2677 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 2678 2679/** 2680 * Constant for fluent queries to be used to add include statements. Specifies 2681 * the path value of "<b>DeviceRequest:device</b>". 2682 */ 2683 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceRequest:device").toLocked(); 2684 2685 /** 2686 * Search parameter: <b>status</b> 2687 * <p> 2688 * Description: <b>entered-in-error | draft | active |suspended | completed </b><br> 2689 * Type: <b>token</b><br> 2690 * Path: <b>DeviceRequest.status</b><br> 2691 * </p> 2692 */ 2693 @SearchParamDefinition(name="status", path="DeviceRequest.status", description="entered-in-error | draft | active |suspended | completed ", type="token" ) 2694 public static final String SP_STATUS = "status"; 2695 /** 2696 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2697 * <p> 2698 * Description: <b>entered-in-error | draft | active |suspended | completed </b><br> 2699 * Type: <b>token</b><br> 2700 * Path: <b>DeviceRequest.status</b><br> 2701 * </p> 2702 */ 2703 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2704 2705 2706}