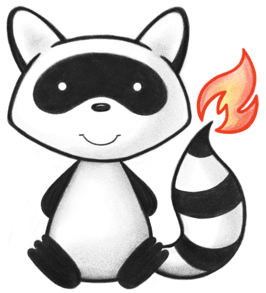
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 048 */ 049@ResourceDef(name="DeviceUseStatement", profile="http://hl7.org/fhir/Profile/DeviceUseStatement") 050public class DeviceUseStatement extends DomainResource { 051 052 public enum DeviceUseStatementStatus { 053 /** 054 * The device is still being used. 055 */ 056 ACTIVE, 057 /** 058 * The device is no longer being used. 059 */ 060 COMPLETED, 061 /** 062 * The statement was recorded incorrectly. 063 */ 064 ENTEREDINERROR, 065 /** 066 * The device may be used at some time in the future. 067 */ 068 INTENDED, 069 /** 070 * Actions implied by the statement have been permanently halted, before all of them occurred. 071 */ 072 STOPPED, 073 /** 074 * Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called "suspended". 075 */ 076 ONHOLD, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static DeviceUseStatementStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("active".equals(codeString)) 085 return ACTIVE; 086 if ("completed".equals(codeString)) 087 return COMPLETED; 088 if ("entered-in-error".equals(codeString)) 089 return ENTEREDINERROR; 090 if ("intended".equals(codeString)) 091 return INTENDED; 092 if ("stopped".equals(codeString)) 093 return STOPPED; 094 if ("on-hold".equals(codeString)) 095 return ONHOLD; 096 if (Configuration.isAcceptInvalidEnums()) 097 return null; 098 else 099 throw new FHIRException("Unknown DeviceUseStatementStatus code '"+codeString+"'"); 100 } 101 public String toCode() { 102 switch (this) { 103 case ACTIVE: return "active"; 104 case COMPLETED: return "completed"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case INTENDED: return "intended"; 107 case STOPPED: return "stopped"; 108 case ONHOLD: return "on-hold"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getSystem() { 114 switch (this) { 115 case ACTIVE: return "http://hl7.org/fhir/device-statement-status"; 116 case COMPLETED: return "http://hl7.org/fhir/device-statement-status"; 117 case ENTEREDINERROR: return "http://hl7.org/fhir/device-statement-status"; 118 case INTENDED: return "http://hl7.org/fhir/device-statement-status"; 119 case STOPPED: return "http://hl7.org/fhir/device-statement-status"; 120 case ONHOLD: return "http://hl7.org/fhir/device-statement-status"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 public String getDefinition() { 126 switch (this) { 127 case ACTIVE: return "The device is still being used."; 128 case COMPLETED: return "The device is no longer being used."; 129 case ENTEREDINERROR: return "The statement was recorded incorrectly."; 130 case INTENDED: return "The device may be used at some time in the future."; 131 case STOPPED: return "Actions implied by the statement have been permanently halted, before all of them occurred."; 132 case ONHOLD: return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDisplay() { 138 switch (this) { 139 case ACTIVE: return "Active"; 140 case COMPLETED: return "Completed"; 141 case ENTEREDINERROR: return "Entered in Error"; 142 case INTENDED: return "Intended"; 143 case STOPPED: return "Stopped"; 144 case ONHOLD: return "On Hold"; 145 case NULL: return null; 146 default: return "?"; 147 } 148 } 149 } 150 151 public static class DeviceUseStatementStatusEnumFactory implements EnumFactory<DeviceUseStatementStatus> { 152 public DeviceUseStatementStatus fromCode(String codeString) throws IllegalArgumentException { 153 if (codeString == null || "".equals(codeString)) 154 if (codeString == null || "".equals(codeString)) 155 return null; 156 if ("active".equals(codeString)) 157 return DeviceUseStatementStatus.ACTIVE; 158 if ("completed".equals(codeString)) 159 return DeviceUseStatementStatus.COMPLETED; 160 if ("entered-in-error".equals(codeString)) 161 return DeviceUseStatementStatus.ENTEREDINERROR; 162 if ("intended".equals(codeString)) 163 return DeviceUseStatementStatus.INTENDED; 164 if ("stopped".equals(codeString)) 165 return DeviceUseStatementStatus.STOPPED; 166 if ("on-hold".equals(codeString)) 167 return DeviceUseStatementStatus.ONHOLD; 168 throw new IllegalArgumentException("Unknown DeviceUseStatementStatus code '"+codeString+"'"); 169 } 170 public Enumeration<DeviceUseStatementStatus> fromType(PrimitiveType<?> code) throws FHIRException { 171 if (code == null) 172 return null; 173 if (code.isEmpty()) 174 return new Enumeration<DeviceUseStatementStatus>(this); 175 String codeString = code.asStringValue(); 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("active".equals(codeString)) 179 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ACTIVE); 180 if ("completed".equals(codeString)) 181 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.COMPLETED); 182 if ("entered-in-error".equals(codeString)) 183 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ENTEREDINERROR); 184 if ("intended".equals(codeString)) 185 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.INTENDED); 186 if ("stopped".equals(codeString)) 187 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.STOPPED); 188 if ("on-hold".equals(codeString)) 189 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ONHOLD); 190 throw new FHIRException("Unknown DeviceUseStatementStatus code '"+codeString+"'"); 191 } 192 public String toCode(DeviceUseStatementStatus code) { 193 if (code == DeviceUseStatementStatus.NULL) 194 return null; 195 if (code == DeviceUseStatementStatus.ACTIVE) 196 return "active"; 197 if (code == DeviceUseStatementStatus.COMPLETED) 198 return "completed"; 199 if (code == DeviceUseStatementStatus.ENTEREDINERROR) 200 return "entered-in-error"; 201 if (code == DeviceUseStatementStatus.INTENDED) 202 return "intended"; 203 if (code == DeviceUseStatementStatus.STOPPED) 204 return "stopped"; 205 if (code == DeviceUseStatementStatus.ONHOLD) 206 return "on-hold"; 207 return "?"; 208 } 209 public String toSystem(DeviceUseStatementStatus code) { 210 return code.getSystem(); 211 } 212 } 213 214 /** 215 * An external identifier for this statement such as an IRI. 216 */ 217 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 218 @Description(shortDefinition="External identifier for this record", formalDefinition="An external identifier for this statement such as an IRI." ) 219 protected List<Identifier> identifier; 220 221 /** 222 * A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed. 223 */ 224 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 225 @Description(shortDefinition="active | completed | entered-in-error +", formalDefinition="A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed." ) 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-statement-status") 227 protected Enumeration<DeviceUseStatementStatus> status; 228 229 /** 230 * The patient who used the device. 231 */ 232 @Child(name = "subject", type = {Patient.class, Group.class}, order=2, min=1, max=1, modifier=false, summary=false) 233 @Description(shortDefinition="Patient using device", formalDefinition="The patient who used the device." ) 234 protected Reference subject; 235 236 /** 237 * The actual object that is the target of the reference (The patient who used the device.) 238 */ 239 protected Resource subjectTarget; 240 241 /** 242 * The time period over which the device was used. 243 */ 244 @Child(name = "whenUsed", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 245 @Description(shortDefinition="Period device was used", formalDefinition="The time period over which the device was used." ) 246 protected Period whenUsed; 247 248 /** 249 * How often the device was used. 250 */ 251 @Child(name = "timing", type = {Timing.class, Period.class, DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 252 @Description(shortDefinition="How often the device was used", formalDefinition="How often the device was used." ) 253 protected Type timing; 254 255 /** 256 * The time at which the statement was made/recorded. 257 */ 258 @Child(name = "recordedOn", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 259 @Description(shortDefinition="When statement was recorded", formalDefinition="The time at which the statement was made/recorded." ) 260 protected DateTimeType recordedOn; 261 262 /** 263 * Who reported the device was being used by the patient. 264 */ 265 @Child(name = "source", type = {Patient.class, Practitioner.class, RelatedPerson.class}, order=6, min=0, max=1, modifier=false, summary=false) 266 @Description(shortDefinition="Who made the statement", formalDefinition="Who reported the device was being used by the patient." ) 267 protected Reference source; 268 269 /** 270 * The actual object that is the target of the reference (Who reported the device was being used by the patient.) 271 */ 272 protected Resource sourceTarget; 273 274 /** 275 * The details of the device used. 276 */ 277 @Child(name = "device", type = {Device.class}, order=7, min=1, max=1, modifier=false, summary=false) 278 @Description(shortDefinition="Reference to device used", formalDefinition="The details of the device used." ) 279 protected Reference device; 280 281 /** 282 * The actual object that is the target of the reference (The details of the device used.) 283 */ 284 protected Device deviceTarget; 285 286 /** 287 * Reason or justification for the use of the device. 288 */ 289 @Child(name = "indication", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 290 @Description(shortDefinition="Why device was used", formalDefinition="Reason or justification for the use of the device." ) 291 protected List<CodeableConcept> indication; 292 293 /** 294 * Indicates the site on the subject's body where the device was used ( i.e. the target site). 295 */ 296 @Child(name = "bodySite", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 297 @Description(shortDefinition="Target body site", formalDefinition="Indicates the site on the subject's body where the device was used ( i.e. the target site)." ) 298 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 299 protected CodeableConcept bodySite; 300 301 /** 302 * Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement. 303 */ 304 @Child(name = "note", type = {Annotation.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 305 @Description(shortDefinition="Addition details (comments, instructions)", formalDefinition="Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement." ) 306 protected List<Annotation> note; 307 308 private static final long serialVersionUID = 2144163845L; 309 310 /** 311 * Constructor 312 */ 313 public DeviceUseStatement() { 314 super(); 315 } 316 317 /** 318 * Constructor 319 */ 320 public DeviceUseStatement(Enumeration<DeviceUseStatementStatus> status, Reference subject, Reference device) { 321 super(); 322 this.status = status; 323 this.subject = subject; 324 this.device = device; 325 } 326 327 /** 328 * @return {@link #identifier} (An external identifier for this statement such as an IRI.) 329 */ 330 public List<Identifier> getIdentifier() { 331 if (this.identifier == null) 332 this.identifier = new ArrayList<Identifier>(); 333 return this.identifier; 334 } 335 336 /** 337 * @return Returns a reference to <code>this</code> for easy method chaining 338 */ 339 public DeviceUseStatement setIdentifier(List<Identifier> theIdentifier) { 340 this.identifier = theIdentifier; 341 return this; 342 } 343 344 public boolean hasIdentifier() { 345 if (this.identifier == null) 346 return false; 347 for (Identifier item : this.identifier) 348 if (!item.isEmpty()) 349 return true; 350 return false; 351 } 352 353 public Identifier addIdentifier() { //3 354 Identifier t = new Identifier(); 355 if (this.identifier == null) 356 this.identifier = new ArrayList<Identifier>(); 357 this.identifier.add(t); 358 return t; 359 } 360 361 public DeviceUseStatement addIdentifier(Identifier t) { //3 362 if (t == null) 363 return this; 364 if (this.identifier == null) 365 this.identifier = new ArrayList<Identifier>(); 366 this.identifier.add(t); 367 return this; 368 } 369 370 /** 371 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 372 */ 373 public Identifier getIdentifierFirstRep() { 374 if (getIdentifier().isEmpty()) { 375 addIdentifier(); 376 } 377 return getIdentifier().get(0); 378 } 379 380 /** 381 * @return {@link #status} (A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 382 */ 383 public Enumeration<DeviceUseStatementStatus> getStatusElement() { 384 if (this.status == null) 385 if (Configuration.errorOnAutoCreate()) 386 throw new Error("Attempt to auto-create DeviceUseStatement.status"); 387 else if (Configuration.doAutoCreate()) 388 this.status = new Enumeration<DeviceUseStatementStatus>(new DeviceUseStatementStatusEnumFactory()); // bb 389 return this.status; 390 } 391 392 public boolean hasStatusElement() { 393 return this.status != null && !this.status.isEmpty(); 394 } 395 396 public boolean hasStatus() { 397 return this.status != null && !this.status.isEmpty(); 398 } 399 400 /** 401 * @param value {@link #status} (A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 402 */ 403 public DeviceUseStatement setStatusElement(Enumeration<DeviceUseStatementStatus> value) { 404 this.status = value; 405 return this; 406 } 407 408 /** 409 * @return A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed. 410 */ 411 public DeviceUseStatementStatus getStatus() { 412 return this.status == null ? null : this.status.getValue(); 413 } 414 415 /** 416 * @param value A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed. 417 */ 418 public DeviceUseStatement setStatus(DeviceUseStatementStatus value) { 419 if (this.status == null) 420 this.status = new Enumeration<DeviceUseStatementStatus>(new DeviceUseStatementStatusEnumFactory()); 421 this.status.setValue(value); 422 return this; 423 } 424 425 /** 426 * @return {@link #subject} (The patient who used the device.) 427 */ 428 public Reference getSubject() { 429 if (this.subject == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create DeviceUseStatement.subject"); 432 else if (Configuration.doAutoCreate()) 433 this.subject = new Reference(); // cc 434 return this.subject; 435 } 436 437 public boolean hasSubject() { 438 return this.subject != null && !this.subject.isEmpty(); 439 } 440 441 /** 442 * @param value {@link #subject} (The patient who used the device.) 443 */ 444 public DeviceUseStatement setSubject(Reference value) { 445 this.subject = value; 446 return this; 447 } 448 449 /** 450 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who used the device.) 451 */ 452 public Resource getSubjectTarget() { 453 return this.subjectTarget; 454 } 455 456 /** 457 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who used the device.) 458 */ 459 public DeviceUseStatement setSubjectTarget(Resource value) { 460 this.subjectTarget = value; 461 return this; 462 } 463 464 /** 465 * @return {@link #whenUsed} (The time period over which the device was used.) 466 */ 467 public Period getWhenUsed() { 468 if (this.whenUsed == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create DeviceUseStatement.whenUsed"); 471 else if (Configuration.doAutoCreate()) 472 this.whenUsed = new Period(); // cc 473 return this.whenUsed; 474 } 475 476 public boolean hasWhenUsed() { 477 return this.whenUsed != null && !this.whenUsed.isEmpty(); 478 } 479 480 /** 481 * @param value {@link #whenUsed} (The time period over which the device was used.) 482 */ 483 public DeviceUseStatement setWhenUsed(Period value) { 484 this.whenUsed = value; 485 return this; 486 } 487 488 /** 489 * @return {@link #timing} (How often the device was used.) 490 */ 491 public Type getTiming() { 492 return this.timing; 493 } 494 495 /** 496 * @return {@link #timing} (How often the device was used.) 497 */ 498 public Timing getTimingTiming() throws FHIRException { 499 if (this.timing == null) 500 return null; 501 if (!(this.timing instanceof Timing)) 502 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 503 return (Timing) this.timing; 504 } 505 506 public boolean hasTimingTiming() { 507 return this != null && this.timing instanceof Timing; 508 } 509 510 /** 511 * @return {@link #timing} (How often the device was used.) 512 */ 513 public Period getTimingPeriod() throws FHIRException { 514 if (this.timing == null) 515 return null; 516 if (!(this.timing instanceof Period)) 517 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 518 return (Period) this.timing; 519 } 520 521 public boolean hasTimingPeriod() { 522 return this != null && this.timing instanceof Period; 523 } 524 525 /** 526 * @return {@link #timing} (How often the device was used.) 527 */ 528 public DateTimeType getTimingDateTimeType() throws FHIRException { 529 if (this.timing == null) 530 return null; 531 if (!(this.timing instanceof DateTimeType)) 532 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 533 return (DateTimeType) this.timing; 534 } 535 536 public boolean hasTimingDateTimeType() { 537 return this != null && this.timing instanceof DateTimeType; 538 } 539 540 public boolean hasTiming() { 541 return this.timing != null && !this.timing.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #timing} (How often the device was used.) 546 */ 547 public DeviceUseStatement setTiming(Type value) throws FHIRFormatError { 548 if (value != null && !(value instanceof Timing || value instanceof Period || value instanceof DateTimeType)) 549 throw new FHIRFormatError("Not the right type for DeviceUseStatement.timing[x]: "+value.fhirType()); 550 this.timing = value; 551 return this; 552 } 553 554 /** 555 * @return {@link #recordedOn} (The time at which the statement was made/recorded.). This is the underlying object with id, value and extensions. The accessor "getRecordedOn" gives direct access to the value 556 */ 557 public DateTimeType getRecordedOnElement() { 558 if (this.recordedOn == null) 559 if (Configuration.errorOnAutoCreate()) 560 throw new Error("Attempt to auto-create DeviceUseStatement.recordedOn"); 561 else if (Configuration.doAutoCreate()) 562 this.recordedOn = new DateTimeType(); // bb 563 return this.recordedOn; 564 } 565 566 public boolean hasRecordedOnElement() { 567 return this.recordedOn != null && !this.recordedOn.isEmpty(); 568 } 569 570 public boolean hasRecordedOn() { 571 return this.recordedOn != null && !this.recordedOn.isEmpty(); 572 } 573 574 /** 575 * @param value {@link #recordedOn} (The time at which the statement was made/recorded.). This is the underlying object with id, value and extensions. The accessor "getRecordedOn" gives direct access to the value 576 */ 577 public DeviceUseStatement setRecordedOnElement(DateTimeType value) { 578 this.recordedOn = value; 579 return this; 580 } 581 582 /** 583 * @return The time at which the statement was made/recorded. 584 */ 585 public Date getRecordedOn() { 586 return this.recordedOn == null ? null : this.recordedOn.getValue(); 587 } 588 589 /** 590 * @param value The time at which the statement was made/recorded. 591 */ 592 public DeviceUseStatement setRecordedOn(Date value) { 593 if (value == null) 594 this.recordedOn = null; 595 else { 596 if (this.recordedOn == null) 597 this.recordedOn = new DateTimeType(); 598 this.recordedOn.setValue(value); 599 } 600 return this; 601 } 602 603 /** 604 * @return {@link #source} (Who reported the device was being used by the patient.) 605 */ 606 public Reference getSource() { 607 if (this.source == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create DeviceUseStatement.source"); 610 else if (Configuration.doAutoCreate()) 611 this.source = new Reference(); // cc 612 return this.source; 613 } 614 615 public boolean hasSource() { 616 return this.source != null && !this.source.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #source} (Who reported the device was being used by the patient.) 621 */ 622 public DeviceUseStatement setSource(Reference value) { 623 this.source = value; 624 return this; 625 } 626 627 /** 628 * @return {@link #source} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Who reported the device was being used by the patient.) 629 */ 630 public Resource getSourceTarget() { 631 return this.sourceTarget; 632 } 633 634 /** 635 * @param value {@link #source} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Who reported the device was being used by the patient.) 636 */ 637 public DeviceUseStatement setSourceTarget(Resource value) { 638 this.sourceTarget = value; 639 return this; 640 } 641 642 /** 643 * @return {@link #device} (The details of the device used.) 644 */ 645 public Reference getDevice() { 646 if (this.device == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 649 else if (Configuration.doAutoCreate()) 650 this.device = new Reference(); // cc 651 return this.device; 652 } 653 654 public boolean hasDevice() { 655 return this.device != null && !this.device.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #device} (The details of the device used.) 660 */ 661 public DeviceUseStatement setDevice(Reference value) { 662 this.device = value; 663 return this; 664 } 665 666 /** 667 * @return {@link #device} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The details of the device used.) 668 */ 669 public Device getDeviceTarget() { 670 if (this.deviceTarget == null) 671 if (Configuration.errorOnAutoCreate()) 672 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 673 else if (Configuration.doAutoCreate()) 674 this.deviceTarget = new Device(); // aa 675 return this.deviceTarget; 676 } 677 678 /** 679 * @param value {@link #device} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The details of the device used.) 680 */ 681 public DeviceUseStatement setDeviceTarget(Device value) { 682 this.deviceTarget = value; 683 return this; 684 } 685 686 /** 687 * @return {@link #indication} (Reason or justification for the use of the device.) 688 */ 689 public List<CodeableConcept> getIndication() { 690 if (this.indication == null) 691 this.indication = new ArrayList<CodeableConcept>(); 692 return this.indication; 693 } 694 695 /** 696 * @return Returns a reference to <code>this</code> for easy method chaining 697 */ 698 public DeviceUseStatement setIndication(List<CodeableConcept> theIndication) { 699 this.indication = theIndication; 700 return this; 701 } 702 703 public boolean hasIndication() { 704 if (this.indication == null) 705 return false; 706 for (CodeableConcept item : this.indication) 707 if (!item.isEmpty()) 708 return true; 709 return false; 710 } 711 712 public CodeableConcept addIndication() { //3 713 CodeableConcept t = new CodeableConcept(); 714 if (this.indication == null) 715 this.indication = new ArrayList<CodeableConcept>(); 716 this.indication.add(t); 717 return t; 718 } 719 720 public DeviceUseStatement addIndication(CodeableConcept t) { //3 721 if (t == null) 722 return this; 723 if (this.indication == null) 724 this.indication = new ArrayList<CodeableConcept>(); 725 this.indication.add(t); 726 return this; 727 } 728 729 /** 730 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist 731 */ 732 public CodeableConcept getIndicationFirstRep() { 733 if (getIndication().isEmpty()) { 734 addIndication(); 735 } 736 return getIndication().get(0); 737 } 738 739 /** 740 * @return {@link #bodySite} (Indicates the site on the subject's body where the device was used ( i.e. the target site).) 741 */ 742 public CodeableConcept getBodySite() { 743 if (this.bodySite == null) 744 if (Configuration.errorOnAutoCreate()) 745 throw new Error("Attempt to auto-create DeviceUseStatement.bodySite"); 746 else if (Configuration.doAutoCreate()) 747 this.bodySite = new CodeableConcept(); // cc 748 return this.bodySite; 749 } 750 751 public boolean hasBodySite() { 752 return this.bodySite != null && !this.bodySite.isEmpty(); 753 } 754 755 /** 756 * @param value {@link #bodySite} (Indicates the site on the subject's body where the device was used ( i.e. the target site).) 757 */ 758 public DeviceUseStatement setBodySite(CodeableConcept value) { 759 this.bodySite = value; 760 return this; 761 } 762 763 /** 764 * @return {@link #note} (Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.) 765 */ 766 public List<Annotation> getNote() { 767 if (this.note == null) 768 this.note = new ArrayList<Annotation>(); 769 return this.note; 770 } 771 772 /** 773 * @return Returns a reference to <code>this</code> for easy method chaining 774 */ 775 public DeviceUseStatement setNote(List<Annotation> theNote) { 776 this.note = theNote; 777 return this; 778 } 779 780 public boolean hasNote() { 781 if (this.note == null) 782 return false; 783 for (Annotation item : this.note) 784 if (!item.isEmpty()) 785 return true; 786 return false; 787 } 788 789 public Annotation addNote() { //3 790 Annotation t = new Annotation(); 791 if (this.note == null) 792 this.note = new ArrayList<Annotation>(); 793 this.note.add(t); 794 return t; 795 } 796 797 public DeviceUseStatement addNote(Annotation t) { //3 798 if (t == null) 799 return this; 800 if (this.note == null) 801 this.note = new ArrayList<Annotation>(); 802 this.note.add(t); 803 return this; 804 } 805 806 /** 807 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 808 */ 809 public Annotation getNoteFirstRep() { 810 if (getNote().isEmpty()) { 811 addNote(); 812 } 813 return getNote().get(0); 814 } 815 816 protected void listChildren(List<Property> children) { 817 super.listChildren(children); 818 children.add(new Property("identifier", "Identifier", "An external identifier for this statement such as an IRI.", 0, java.lang.Integer.MAX_VALUE, identifier)); 819 children.add(new Property("status", "code", "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 0, 1, status)); 820 children.add(new Property("subject", "Reference(Patient|Group)", "The patient who used the device.", 0, 1, subject)); 821 children.add(new Property("whenUsed", "Period", "The time period over which the device was used.", 0, 1, whenUsed)); 822 children.add(new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing)); 823 children.add(new Property("recordedOn", "dateTime", "The time at which the statement was made/recorded.", 0, 1, recordedOn)); 824 children.add(new Property("source", "Reference(Patient|Practitioner|RelatedPerson)", "Who reported the device was being used by the patient.", 0, 1, source)); 825 children.add(new Property("device", "Reference(Device)", "The details of the device used.", 0, 1, device)); 826 children.add(new Property("indication", "CodeableConcept", "Reason or justification for the use of the device.", 0, java.lang.Integer.MAX_VALUE, indication)); 827 children.add(new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the device was used ( i.e. the target site).", 0, 1, bodySite)); 828 children.add(new Property("note", "Annotation", "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note)); 829 } 830 831 @Override 832 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 833 switch (_hash) { 834 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An external identifier for this statement such as an IRI.", 0, java.lang.Integer.MAX_VALUE, identifier); 835 case -892481550: /*status*/ return new Property("status", "code", "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 0, 1, status); 836 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient who used the device.", 0, 1, subject); 837 case 2042879511: /*whenUsed*/ return new Property("whenUsed", "Period", "The time period over which the device was used.", 0, 1, whenUsed); 838 case 164632566: /*timing[x]*/ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing); 839 case -873664438: /*timing*/ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing); 840 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing); 841 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing); 842 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing); 843 case 735397551: /*recordedOn*/ return new Property("recordedOn", "dateTime", "The time at which the statement was made/recorded.", 0, 1, recordedOn); 844 case -896505829: /*source*/ return new Property("source", "Reference(Patient|Practitioner|RelatedPerson)", "Who reported the device was being used by the patient.", 0, 1, source); 845 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "The details of the device used.", 0, 1, device); 846 case -597168804: /*indication*/ return new Property("indication", "CodeableConcept", "Reason or justification for the use of the device.", 0, java.lang.Integer.MAX_VALUE, indication); 847 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the device was used ( i.e. the target site).", 0, 1, bodySite); 848 case 3387378: /*note*/ return new Property("note", "Annotation", "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note); 849 default: return super.getNamedProperty(_hash, _name, _checkValid); 850 } 851 852 } 853 854 @Override 855 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 856 switch (hash) { 857 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 858 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DeviceUseStatementStatus> 859 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 860 case 2042879511: /*whenUsed*/ return this.whenUsed == null ? new Base[0] : new Base[] {this.whenUsed}; // Period 861 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 862 case 735397551: /*recordedOn*/ return this.recordedOn == null ? new Base[0] : new Base[] {this.recordedOn}; // DateTimeType 863 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 864 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 865 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // CodeableConcept 866 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 867 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 868 default: return super.getProperty(hash, name, checkValid); 869 } 870 871 } 872 873 @Override 874 public Base setProperty(int hash, String name, Base value) throws FHIRException { 875 switch (hash) { 876 case -1618432855: // identifier 877 this.getIdentifier().add(castToIdentifier(value)); // Identifier 878 return value; 879 case -892481550: // status 880 value = new DeviceUseStatementStatusEnumFactory().fromType(castToCode(value)); 881 this.status = (Enumeration) value; // Enumeration<DeviceUseStatementStatus> 882 return value; 883 case -1867885268: // subject 884 this.subject = castToReference(value); // Reference 885 return value; 886 case 2042879511: // whenUsed 887 this.whenUsed = castToPeriod(value); // Period 888 return value; 889 case -873664438: // timing 890 this.timing = castToType(value); // Type 891 return value; 892 case 735397551: // recordedOn 893 this.recordedOn = castToDateTime(value); // DateTimeType 894 return value; 895 case -896505829: // source 896 this.source = castToReference(value); // Reference 897 return value; 898 case -1335157162: // device 899 this.device = castToReference(value); // Reference 900 return value; 901 case -597168804: // indication 902 this.getIndication().add(castToCodeableConcept(value)); // CodeableConcept 903 return value; 904 case 1702620169: // bodySite 905 this.bodySite = castToCodeableConcept(value); // CodeableConcept 906 return value; 907 case 3387378: // note 908 this.getNote().add(castToAnnotation(value)); // Annotation 909 return value; 910 default: return super.setProperty(hash, name, value); 911 } 912 913 } 914 915 @Override 916 public Base setProperty(String name, Base value) throws FHIRException { 917 if (name.equals("identifier")) { 918 this.getIdentifier().add(castToIdentifier(value)); 919 } else if (name.equals("status")) { 920 value = new DeviceUseStatementStatusEnumFactory().fromType(castToCode(value)); 921 this.status = (Enumeration) value; // Enumeration<DeviceUseStatementStatus> 922 } else if (name.equals("subject")) { 923 this.subject = castToReference(value); // Reference 924 } else if (name.equals("whenUsed")) { 925 this.whenUsed = castToPeriod(value); // Period 926 } else if (name.equals("timing[x]")) { 927 this.timing = castToType(value); // Type 928 } else if (name.equals("recordedOn")) { 929 this.recordedOn = castToDateTime(value); // DateTimeType 930 } else if (name.equals("source")) { 931 this.source = castToReference(value); // Reference 932 } else if (name.equals("device")) { 933 this.device = castToReference(value); // Reference 934 } else if (name.equals("indication")) { 935 this.getIndication().add(castToCodeableConcept(value)); 936 } else if (name.equals("bodySite")) { 937 this.bodySite = castToCodeableConcept(value); // CodeableConcept 938 } else if (name.equals("note")) { 939 this.getNote().add(castToAnnotation(value)); 940 } else 941 return super.setProperty(name, value); 942 return value; 943 } 944 945 @Override 946 public Base makeProperty(int hash, String name) throws FHIRException { 947 switch (hash) { 948 case -1618432855: return addIdentifier(); 949 case -892481550: return getStatusElement(); 950 case -1867885268: return getSubject(); 951 case 2042879511: return getWhenUsed(); 952 case 164632566: return getTiming(); 953 case -873664438: return getTiming(); 954 case 735397551: return getRecordedOnElement(); 955 case -896505829: return getSource(); 956 case -1335157162: return getDevice(); 957 case -597168804: return addIndication(); 958 case 1702620169: return getBodySite(); 959 case 3387378: return addNote(); 960 default: return super.makeProperty(hash, name); 961 } 962 963 } 964 965 @Override 966 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 967 switch (hash) { 968 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 969 case -892481550: /*status*/ return new String[] {"code"}; 970 case -1867885268: /*subject*/ return new String[] {"Reference"}; 971 case 2042879511: /*whenUsed*/ return new String[] {"Period"}; 972 case -873664438: /*timing*/ return new String[] {"Timing", "Period", "dateTime"}; 973 case 735397551: /*recordedOn*/ return new String[] {"dateTime"}; 974 case -896505829: /*source*/ return new String[] {"Reference"}; 975 case -1335157162: /*device*/ return new String[] {"Reference"}; 976 case -597168804: /*indication*/ return new String[] {"CodeableConcept"}; 977 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 978 case 3387378: /*note*/ return new String[] {"Annotation"}; 979 default: return super.getTypesForProperty(hash, name); 980 } 981 982 } 983 984 @Override 985 public Base addChild(String name) throws FHIRException { 986 if (name.equals("identifier")) { 987 return addIdentifier(); 988 } 989 else if (name.equals("status")) { 990 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.status"); 991 } 992 else if (name.equals("subject")) { 993 this.subject = new Reference(); 994 return this.subject; 995 } 996 else if (name.equals("whenUsed")) { 997 this.whenUsed = new Period(); 998 return this.whenUsed; 999 } 1000 else if (name.equals("timingTiming")) { 1001 this.timing = new Timing(); 1002 return this.timing; 1003 } 1004 else if (name.equals("timingPeriod")) { 1005 this.timing = new Period(); 1006 return this.timing; 1007 } 1008 else if (name.equals("timingDateTime")) { 1009 this.timing = new DateTimeType(); 1010 return this.timing; 1011 } 1012 else if (name.equals("recordedOn")) { 1013 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.recordedOn"); 1014 } 1015 else if (name.equals("source")) { 1016 this.source = new Reference(); 1017 return this.source; 1018 } 1019 else if (name.equals("device")) { 1020 this.device = new Reference(); 1021 return this.device; 1022 } 1023 else if (name.equals("indication")) { 1024 return addIndication(); 1025 } 1026 else if (name.equals("bodySite")) { 1027 this.bodySite = new CodeableConcept(); 1028 return this.bodySite; 1029 } 1030 else if (name.equals("note")) { 1031 return addNote(); 1032 } 1033 else 1034 return super.addChild(name); 1035 } 1036 1037 public String fhirType() { 1038 return "DeviceUseStatement"; 1039 1040 } 1041 1042 public DeviceUseStatement copy() { 1043 DeviceUseStatement dst = new DeviceUseStatement(); 1044 copyValues(dst); 1045 if (identifier != null) { 1046 dst.identifier = new ArrayList<Identifier>(); 1047 for (Identifier i : identifier) 1048 dst.identifier.add(i.copy()); 1049 }; 1050 dst.status = status == null ? null : status.copy(); 1051 dst.subject = subject == null ? null : subject.copy(); 1052 dst.whenUsed = whenUsed == null ? null : whenUsed.copy(); 1053 dst.timing = timing == null ? null : timing.copy(); 1054 dst.recordedOn = recordedOn == null ? null : recordedOn.copy(); 1055 dst.source = source == null ? null : source.copy(); 1056 dst.device = device == null ? null : device.copy(); 1057 if (indication != null) { 1058 dst.indication = new ArrayList<CodeableConcept>(); 1059 for (CodeableConcept i : indication) 1060 dst.indication.add(i.copy()); 1061 }; 1062 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1063 if (note != null) { 1064 dst.note = new ArrayList<Annotation>(); 1065 for (Annotation i : note) 1066 dst.note.add(i.copy()); 1067 }; 1068 return dst; 1069 } 1070 1071 protected DeviceUseStatement typedCopy() { 1072 return copy(); 1073 } 1074 1075 @Override 1076 public boolean equalsDeep(Base other_) { 1077 if (!super.equalsDeep(other_)) 1078 return false; 1079 if (!(other_ instanceof DeviceUseStatement)) 1080 return false; 1081 DeviceUseStatement o = (DeviceUseStatement) other_; 1082 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 1083 && compareDeep(whenUsed, o.whenUsed, true) && compareDeep(timing, o.timing, true) && compareDeep(recordedOn, o.recordedOn, true) 1084 && compareDeep(source, o.source, true) && compareDeep(device, o.device, true) && compareDeep(indication, o.indication, true) 1085 && compareDeep(bodySite, o.bodySite, true) && compareDeep(note, o.note, true); 1086 } 1087 1088 @Override 1089 public boolean equalsShallow(Base other_) { 1090 if (!super.equalsShallow(other_)) 1091 return false; 1092 if (!(other_ instanceof DeviceUseStatement)) 1093 return false; 1094 DeviceUseStatement o = (DeviceUseStatement) other_; 1095 return compareValues(status, o.status, true) && compareValues(recordedOn, o.recordedOn, true); 1096 } 1097 1098 public boolean isEmpty() { 1099 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, subject 1100 , whenUsed, timing, recordedOn, source, device, indication, bodySite, note); 1101 } 1102 1103 @Override 1104 public ResourceType getResourceType() { 1105 return ResourceType.DeviceUseStatement; 1106 } 1107 1108 /** 1109 * Search parameter: <b>identifier</b> 1110 * <p> 1111 * Description: <b>Search by identifier</b><br> 1112 * Type: <b>token</b><br> 1113 * Path: <b>DeviceUseStatement.identifier</b><br> 1114 * </p> 1115 */ 1116 @SearchParamDefinition(name="identifier", path="DeviceUseStatement.identifier", description="Search by identifier", type="token" ) 1117 public static final String SP_IDENTIFIER = "identifier"; 1118 /** 1119 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1120 * <p> 1121 * Description: <b>Search by identifier</b><br> 1122 * Type: <b>token</b><br> 1123 * Path: <b>DeviceUseStatement.identifier</b><br> 1124 * </p> 1125 */ 1126 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1127 1128 /** 1129 * Search parameter: <b>subject</b> 1130 * <p> 1131 * Description: <b>Search by subject</b><br> 1132 * Type: <b>reference</b><br> 1133 * Path: <b>DeviceUseStatement.subject</b><br> 1134 * </p> 1135 */ 1136 @SearchParamDefinition(name="subject", path="DeviceUseStatement.subject", description="Search by subject", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 1137 public static final String SP_SUBJECT = "subject"; 1138 /** 1139 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1140 * <p> 1141 * Description: <b>Search by subject</b><br> 1142 * Type: <b>reference</b><br> 1143 * Path: <b>DeviceUseStatement.subject</b><br> 1144 * </p> 1145 */ 1146 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1147 1148/** 1149 * Constant for fluent queries to be used to add include statements. Specifies 1150 * the path value of "<b>DeviceUseStatement:subject</b>". 1151 */ 1152 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DeviceUseStatement:subject").toLocked(); 1153 1154 /** 1155 * Search parameter: <b>patient</b> 1156 * <p> 1157 * Description: <b>Search by subject - a patient</b><br> 1158 * Type: <b>reference</b><br> 1159 * Path: <b>DeviceUseStatement.subject</b><br> 1160 * </p> 1161 */ 1162 @SearchParamDefinition(name="patient", path="DeviceUseStatement.subject", description="Search by subject - a patient", type="reference", target={Group.class, Patient.class } ) 1163 public static final String SP_PATIENT = "patient"; 1164 /** 1165 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1166 * <p> 1167 * Description: <b>Search by subject - a patient</b><br> 1168 * Type: <b>reference</b><br> 1169 * Path: <b>DeviceUseStatement.subject</b><br> 1170 * </p> 1171 */ 1172 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1173 1174/** 1175 * Constant for fluent queries to be used to add include statements. Specifies 1176 * the path value of "<b>DeviceUseStatement:patient</b>". 1177 */ 1178 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceUseStatement:patient").toLocked(); 1179 1180 /** 1181 * Search parameter: <b>device</b> 1182 * <p> 1183 * Description: <b>Search by device</b><br> 1184 * Type: <b>reference</b><br> 1185 * Path: <b>DeviceUseStatement.device</b><br> 1186 * </p> 1187 */ 1188 @SearchParamDefinition(name="device", path="DeviceUseStatement.device", description="Search by device", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class } ) 1189 public static final String SP_DEVICE = "device"; 1190 /** 1191 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1192 * <p> 1193 * Description: <b>Search by device</b><br> 1194 * Type: <b>reference</b><br> 1195 * Path: <b>DeviceUseStatement.device</b><br> 1196 * </p> 1197 */ 1198 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 1199 1200/** 1201 * Constant for fluent queries to be used to add include statements. Specifies 1202 * the path value of "<b>DeviceUseStatement:device</b>". 1203 */ 1204 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceUseStatement:device").toLocked(); 1205 1206 1207}