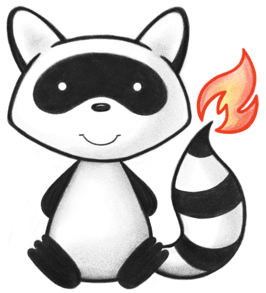
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 051 */ 052@ResourceDef(name="DiagnosticReport", profile="http://hl7.org/fhir/Profile/DiagnosticReport") 053public class DiagnosticReport extends DomainResource { 054 055 public enum DiagnosticReportStatus { 056 /** 057 * The existence of the report is registered, but there is nothing yet available. 058 */ 059 REGISTERED, 060 /** 061 * This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified. 062 */ 063 PARTIAL, 064 /** 065 * Verified early results are available, but not all results are final. 066 */ 067 PRELIMINARY, 068 /** 069 * The report is complete and verified by an authorized person. 070 */ 071 FINAL, 072 /** 073 * Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued. 074 */ 075 AMENDED, 076 /** 077 * Subsequent to being final, the report has been modified to correct an error in the report or referenced results. 078 */ 079 CORRECTED, 080 /** 081 * Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged. 082 */ 083 APPENDED, 084 /** 085 * The report is unavailable because the measurement was not started or not completed (also sometimes called "aborted"). 086 */ 087 CANCELLED, 088 /** 089 * The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 090 */ 091 ENTEREDINERROR, 092 /** 093 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 094 */ 095 UNKNOWN, 096 /** 097 * added to help the parsers with the generic types 098 */ 099 NULL; 100 public static DiagnosticReportStatus fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("registered".equals(codeString)) 104 return REGISTERED; 105 if ("partial".equals(codeString)) 106 return PARTIAL; 107 if ("preliminary".equals(codeString)) 108 return PRELIMINARY; 109 if ("final".equals(codeString)) 110 return FINAL; 111 if ("amended".equals(codeString)) 112 return AMENDED; 113 if ("corrected".equals(codeString)) 114 return CORRECTED; 115 if ("appended".equals(codeString)) 116 return APPENDED; 117 if ("cancelled".equals(codeString)) 118 return CANCELLED; 119 if ("entered-in-error".equals(codeString)) 120 return ENTEREDINERROR; 121 if ("unknown".equals(codeString)) 122 return UNKNOWN; 123 if (Configuration.isAcceptInvalidEnums()) 124 return null; 125 else 126 throw new FHIRException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 127 } 128 public String toCode() { 129 switch (this) { 130 case REGISTERED: return "registered"; 131 case PARTIAL: return "partial"; 132 case PRELIMINARY: return "preliminary"; 133 case FINAL: return "final"; 134 case AMENDED: return "amended"; 135 case CORRECTED: return "corrected"; 136 case APPENDED: return "appended"; 137 case CANCELLED: return "cancelled"; 138 case ENTEREDINERROR: return "entered-in-error"; 139 case UNKNOWN: return "unknown"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 public String getSystem() { 145 switch (this) { 146 case REGISTERED: return "http://hl7.org/fhir/diagnostic-report-status"; 147 case PARTIAL: return "http://hl7.org/fhir/diagnostic-report-status"; 148 case PRELIMINARY: return "http://hl7.org/fhir/diagnostic-report-status"; 149 case FINAL: return "http://hl7.org/fhir/diagnostic-report-status"; 150 case AMENDED: return "http://hl7.org/fhir/diagnostic-report-status"; 151 case CORRECTED: return "http://hl7.org/fhir/diagnostic-report-status"; 152 case APPENDED: return "http://hl7.org/fhir/diagnostic-report-status"; 153 case CANCELLED: return "http://hl7.org/fhir/diagnostic-report-status"; 154 case ENTEREDINERROR: return "http://hl7.org/fhir/diagnostic-report-status"; 155 case UNKNOWN: return "http://hl7.org/fhir/diagnostic-report-status"; 156 case NULL: return null; 157 default: return "?"; 158 } 159 } 160 public String getDefinition() { 161 switch (this) { 162 case REGISTERED: return "The existence of the report is registered, but there is nothing yet available."; 163 case PARTIAL: return "This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified."; 164 case PRELIMINARY: return "Verified early results are available, but not all results are final."; 165 case FINAL: return "The report is complete and verified by an authorized person."; 166 case AMENDED: return "Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued."; 167 case CORRECTED: return "Subsequent to being final, the report has been modified to correct an error in the report or referenced results."; 168 case APPENDED: return "Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged."; 169 case CANCELLED: return "The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 170 case ENTEREDINERROR: return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 171 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 172 case NULL: return null; 173 default: return "?"; 174 } 175 } 176 public String getDisplay() { 177 switch (this) { 178 case REGISTERED: return "Registered"; 179 case PARTIAL: return "Partial"; 180 case PRELIMINARY: return "Preliminary"; 181 case FINAL: return "Final"; 182 case AMENDED: return "Amended"; 183 case CORRECTED: return "Corrected"; 184 case APPENDED: return "Appended"; 185 case CANCELLED: return "Cancelled"; 186 case ENTEREDINERROR: return "Entered in Error"; 187 case UNKNOWN: return "Unknown"; 188 case NULL: return null; 189 default: return "?"; 190 } 191 } 192 } 193 194 public static class DiagnosticReportStatusEnumFactory implements EnumFactory<DiagnosticReportStatus> { 195 public DiagnosticReportStatus fromCode(String codeString) throws IllegalArgumentException { 196 if (codeString == null || "".equals(codeString)) 197 if (codeString == null || "".equals(codeString)) 198 return null; 199 if ("registered".equals(codeString)) 200 return DiagnosticReportStatus.REGISTERED; 201 if ("partial".equals(codeString)) 202 return DiagnosticReportStatus.PARTIAL; 203 if ("preliminary".equals(codeString)) 204 return DiagnosticReportStatus.PRELIMINARY; 205 if ("final".equals(codeString)) 206 return DiagnosticReportStatus.FINAL; 207 if ("amended".equals(codeString)) 208 return DiagnosticReportStatus.AMENDED; 209 if ("corrected".equals(codeString)) 210 return DiagnosticReportStatus.CORRECTED; 211 if ("appended".equals(codeString)) 212 return DiagnosticReportStatus.APPENDED; 213 if ("cancelled".equals(codeString)) 214 return DiagnosticReportStatus.CANCELLED; 215 if ("entered-in-error".equals(codeString)) 216 return DiagnosticReportStatus.ENTEREDINERROR; 217 if ("unknown".equals(codeString)) 218 return DiagnosticReportStatus.UNKNOWN; 219 throw new IllegalArgumentException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 220 } 221 public Enumeration<DiagnosticReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 222 if (code == null) 223 return null; 224 if (code.isEmpty()) 225 return new Enumeration<DiagnosticReportStatus>(this); 226 String codeString = code.asStringValue(); 227 if (codeString == null || "".equals(codeString)) 228 return null; 229 if ("registered".equals(codeString)) 230 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.REGISTERED); 231 if ("partial".equals(codeString)) 232 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PARTIAL); 233 if ("preliminary".equals(codeString)) 234 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PRELIMINARY); 235 if ("final".equals(codeString)) 236 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.FINAL); 237 if ("amended".equals(codeString)) 238 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.AMENDED); 239 if ("corrected".equals(codeString)) 240 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CORRECTED); 241 if ("appended".equals(codeString)) 242 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.APPENDED); 243 if ("cancelled".equals(codeString)) 244 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CANCELLED); 245 if ("entered-in-error".equals(codeString)) 246 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.ENTEREDINERROR); 247 if ("unknown".equals(codeString)) 248 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.UNKNOWN); 249 throw new FHIRException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 250 } 251 public String toCode(DiagnosticReportStatus code) { 252 if (code == DiagnosticReportStatus.NULL) 253 return null; 254 if (code == DiagnosticReportStatus.REGISTERED) 255 return "registered"; 256 if (code == DiagnosticReportStatus.PARTIAL) 257 return "partial"; 258 if (code == DiagnosticReportStatus.PRELIMINARY) 259 return "preliminary"; 260 if (code == DiagnosticReportStatus.FINAL) 261 return "final"; 262 if (code == DiagnosticReportStatus.AMENDED) 263 return "amended"; 264 if (code == DiagnosticReportStatus.CORRECTED) 265 return "corrected"; 266 if (code == DiagnosticReportStatus.APPENDED) 267 return "appended"; 268 if (code == DiagnosticReportStatus.CANCELLED) 269 return "cancelled"; 270 if (code == DiagnosticReportStatus.ENTEREDINERROR) 271 return "entered-in-error"; 272 if (code == DiagnosticReportStatus.UNKNOWN) 273 return "unknown"; 274 return "?"; 275 } 276 public String toSystem(DiagnosticReportStatus code) { 277 return code.getSystem(); 278 } 279 } 280 281 @Block() 282 public static class DiagnosticReportPerformerComponent extends BackboneElement implements IBaseBackboneElement { 283 /** 284 * Describes the type of participation (e.g. a responsible party, author, or verifier). 285 */ 286 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 287 @Description(shortDefinition="Type of performer", formalDefinition="Describes the type of participation (e.g. a responsible party, author, or verifier)." ) 288 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 289 protected CodeableConcept role; 290 291 /** 292 * The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report. 293 */ 294 @Child(name = "actor", type = {Practitioner.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=true) 295 @Description(shortDefinition="Practitioner or Organization participant", formalDefinition="The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report." ) 296 protected Reference actor; 297 298 /** 299 * The actual object that is the target of the reference (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 300 */ 301 protected Resource actorTarget; 302 303 private static final long serialVersionUID = 805521719L; 304 305 /** 306 * Constructor 307 */ 308 public DiagnosticReportPerformerComponent() { 309 super(); 310 } 311 312 /** 313 * Constructor 314 */ 315 public DiagnosticReportPerformerComponent(Reference actor) { 316 super(); 317 this.actor = actor; 318 } 319 320 /** 321 * @return {@link #role} (Describes the type of participation (e.g. a responsible party, author, or verifier).) 322 */ 323 public CodeableConcept getRole() { 324 if (this.role == null) 325 if (Configuration.errorOnAutoCreate()) 326 throw new Error("Attempt to auto-create DiagnosticReportPerformerComponent.role"); 327 else if (Configuration.doAutoCreate()) 328 this.role = new CodeableConcept(); // cc 329 return this.role; 330 } 331 332 public boolean hasRole() { 333 return this.role != null && !this.role.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #role} (Describes the type of participation (e.g. a responsible party, author, or verifier).) 338 */ 339 public DiagnosticReportPerformerComponent setRole(CodeableConcept value) { 340 this.role = value; 341 return this; 342 } 343 344 /** 345 * @return {@link #actor} (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 346 */ 347 public Reference getActor() { 348 if (this.actor == null) 349 if (Configuration.errorOnAutoCreate()) 350 throw new Error("Attempt to auto-create DiagnosticReportPerformerComponent.actor"); 351 else if (Configuration.doAutoCreate()) 352 this.actor = new Reference(); // cc 353 return this.actor; 354 } 355 356 public boolean hasActor() { 357 return this.actor != null && !this.actor.isEmpty(); 358 } 359 360 /** 361 * @param value {@link #actor} (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 362 */ 363 public DiagnosticReportPerformerComponent setActor(Reference value) { 364 this.actor = value; 365 return this; 366 } 367 368 /** 369 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 370 */ 371 public Resource getActorTarget() { 372 return this.actorTarget; 373 } 374 375 /** 376 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 377 */ 378 public DiagnosticReportPerformerComponent setActorTarget(Resource value) { 379 this.actorTarget = value; 380 return this; 381 } 382 383 protected void listChildren(List<Property> children) { 384 super.listChildren(children); 385 children.add(new Property("role", "CodeableConcept", "Describes the type of participation (e.g. a responsible party, author, or verifier).", 0, 1, role)); 386 children.add(new Property("actor", "Reference(Practitioner|Organization)", "The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.", 0, 1, actor)); 387 } 388 389 @Override 390 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 391 switch (_hash) { 392 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Describes the type of participation (e.g. a responsible party, author, or verifier).", 0, 1, role); 393 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Organization)", "The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.", 0, 1, actor); 394 default: return super.getNamedProperty(_hash, _name, _checkValid); 395 } 396 397 } 398 399 @Override 400 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 401 switch (hash) { 402 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 403 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 404 default: return super.getProperty(hash, name, checkValid); 405 } 406 407 } 408 409 @Override 410 public Base setProperty(int hash, String name, Base value) throws FHIRException { 411 switch (hash) { 412 case 3506294: // role 413 this.role = castToCodeableConcept(value); // CodeableConcept 414 return value; 415 case 92645877: // actor 416 this.actor = castToReference(value); // Reference 417 return value; 418 default: return super.setProperty(hash, name, value); 419 } 420 421 } 422 423 @Override 424 public Base setProperty(String name, Base value) throws FHIRException { 425 if (name.equals("role")) { 426 this.role = castToCodeableConcept(value); // CodeableConcept 427 } else if (name.equals("actor")) { 428 this.actor = castToReference(value); // Reference 429 } else 430 return super.setProperty(name, value); 431 return value; 432 } 433 434 @Override 435 public Base makeProperty(int hash, String name) throws FHIRException { 436 switch (hash) { 437 case 3506294: return getRole(); 438 case 92645877: return getActor(); 439 default: return super.makeProperty(hash, name); 440 } 441 442 } 443 444 @Override 445 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 446 switch (hash) { 447 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 448 case 92645877: /*actor*/ return new String[] {"Reference"}; 449 default: return super.getTypesForProperty(hash, name); 450 } 451 452 } 453 454 @Override 455 public Base addChild(String name) throws FHIRException { 456 if (name.equals("role")) { 457 this.role = new CodeableConcept(); 458 return this.role; 459 } 460 else if (name.equals("actor")) { 461 this.actor = new Reference(); 462 return this.actor; 463 } 464 else 465 return super.addChild(name); 466 } 467 468 public DiagnosticReportPerformerComponent copy() { 469 DiagnosticReportPerformerComponent dst = new DiagnosticReportPerformerComponent(); 470 copyValues(dst); 471 dst.role = role == null ? null : role.copy(); 472 dst.actor = actor == null ? null : actor.copy(); 473 return dst; 474 } 475 476 @Override 477 public boolean equalsDeep(Base other_) { 478 if (!super.equalsDeep(other_)) 479 return false; 480 if (!(other_ instanceof DiagnosticReportPerformerComponent)) 481 return false; 482 DiagnosticReportPerformerComponent o = (DiagnosticReportPerformerComponent) other_; 483 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 484 } 485 486 @Override 487 public boolean equalsShallow(Base other_) { 488 if (!super.equalsShallow(other_)) 489 return false; 490 if (!(other_ instanceof DiagnosticReportPerformerComponent)) 491 return false; 492 DiagnosticReportPerformerComponent o = (DiagnosticReportPerformerComponent) other_; 493 return true; 494 } 495 496 public boolean isEmpty() { 497 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 498 } 499 500 public String fhirType() { 501 return "DiagnosticReport.performer"; 502 503 } 504 505 } 506 507 @Block() 508 public static class DiagnosticReportImageComponent extends BackboneElement implements IBaseBackboneElement { 509 /** 510 * A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features. 511 */ 512 @Child(name = "comment", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 513 @Description(shortDefinition="Comment about the image (e.g. explanation)", formalDefinition="A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features." ) 514 protected StringType comment; 515 516 /** 517 * Reference to the image source. 518 */ 519 @Child(name = "link", type = {Media.class}, order=2, min=1, max=1, modifier=false, summary=true) 520 @Description(shortDefinition="Reference to the image source", formalDefinition="Reference to the image source." ) 521 protected Reference link; 522 523 /** 524 * The actual object that is the target of the reference (Reference to the image source.) 525 */ 526 protected Media linkTarget; 527 528 private static final long serialVersionUID = 935791940L; 529 530 /** 531 * Constructor 532 */ 533 public DiagnosticReportImageComponent() { 534 super(); 535 } 536 537 /** 538 * Constructor 539 */ 540 public DiagnosticReportImageComponent(Reference link) { 541 super(); 542 this.link = link; 543 } 544 545 /** 546 * @return {@link #comment} (A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 547 */ 548 public StringType getCommentElement() { 549 if (this.comment == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.comment"); 552 else if (Configuration.doAutoCreate()) 553 this.comment = new StringType(); // bb 554 return this.comment; 555 } 556 557 public boolean hasCommentElement() { 558 return this.comment != null && !this.comment.isEmpty(); 559 } 560 561 public boolean hasComment() { 562 return this.comment != null && !this.comment.isEmpty(); 563 } 564 565 /** 566 * @param value {@link #comment} (A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 567 */ 568 public DiagnosticReportImageComponent setCommentElement(StringType value) { 569 this.comment = value; 570 return this; 571 } 572 573 /** 574 * @return A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features. 575 */ 576 public String getComment() { 577 return this.comment == null ? null : this.comment.getValue(); 578 } 579 580 /** 581 * @param value A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features. 582 */ 583 public DiagnosticReportImageComponent setComment(String value) { 584 if (Utilities.noString(value)) 585 this.comment = null; 586 else { 587 if (this.comment == null) 588 this.comment = new StringType(); 589 this.comment.setValue(value); 590 } 591 return this; 592 } 593 594 /** 595 * @return {@link #link} (Reference to the image source.) 596 */ 597 public Reference getLink() { 598 if (this.link == null) 599 if (Configuration.errorOnAutoCreate()) 600 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.link"); 601 else if (Configuration.doAutoCreate()) 602 this.link = new Reference(); // cc 603 return this.link; 604 } 605 606 public boolean hasLink() { 607 return this.link != null && !this.link.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #link} (Reference to the image source.) 612 */ 613 public DiagnosticReportImageComponent setLink(Reference value) { 614 this.link = value; 615 return this; 616 } 617 618 /** 619 * @return {@link #link} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the image source.) 620 */ 621 public Media getLinkTarget() { 622 if (this.linkTarget == null) 623 if (Configuration.errorOnAutoCreate()) 624 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.link"); 625 else if (Configuration.doAutoCreate()) 626 this.linkTarget = new Media(); // aa 627 return this.linkTarget; 628 } 629 630 /** 631 * @param value {@link #link} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the image source.) 632 */ 633 public DiagnosticReportImageComponent setLinkTarget(Media value) { 634 this.linkTarget = value; 635 return this; 636 } 637 638 protected void listChildren(List<Property> children) { 639 super.listChildren(children); 640 children.add(new Property("comment", "string", "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 0, 1, comment)); 641 children.add(new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link)); 642 } 643 644 @Override 645 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 646 switch (_hash) { 647 case 950398559: /*comment*/ return new Property("comment", "string", "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 0, 1, comment); 648 case 3321850: /*link*/ return new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link); 649 default: return super.getNamedProperty(_hash, _name, _checkValid); 650 } 651 652 } 653 654 @Override 655 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 656 switch (hash) { 657 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 658 case 3321850: /*link*/ return this.link == null ? new Base[0] : new Base[] {this.link}; // Reference 659 default: return super.getProperty(hash, name, checkValid); 660 } 661 662 } 663 664 @Override 665 public Base setProperty(int hash, String name, Base value) throws FHIRException { 666 switch (hash) { 667 case 950398559: // comment 668 this.comment = castToString(value); // StringType 669 return value; 670 case 3321850: // link 671 this.link = castToReference(value); // Reference 672 return value; 673 default: return super.setProperty(hash, name, value); 674 } 675 676 } 677 678 @Override 679 public Base setProperty(String name, Base value) throws FHIRException { 680 if (name.equals("comment")) { 681 this.comment = castToString(value); // StringType 682 } else if (name.equals("link")) { 683 this.link = castToReference(value); // Reference 684 } else 685 return super.setProperty(name, value); 686 return value; 687 } 688 689 @Override 690 public Base makeProperty(int hash, String name) throws FHIRException { 691 switch (hash) { 692 case 950398559: return getCommentElement(); 693 case 3321850: return getLink(); 694 default: return super.makeProperty(hash, name); 695 } 696 697 } 698 699 @Override 700 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 701 switch (hash) { 702 case 950398559: /*comment*/ return new String[] {"string"}; 703 case 3321850: /*link*/ return new String[] {"Reference"}; 704 default: return super.getTypesForProperty(hash, name); 705 } 706 707 } 708 709 @Override 710 public Base addChild(String name) throws FHIRException { 711 if (name.equals("comment")) { 712 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.comment"); 713 } 714 else if (name.equals("link")) { 715 this.link = new Reference(); 716 return this.link; 717 } 718 else 719 return super.addChild(name); 720 } 721 722 public DiagnosticReportImageComponent copy() { 723 DiagnosticReportImageComponent dst = new DiagnosticReportImageComponent(); 724 copyValues(dst); 725 dst.comment = comment == null ? null : comment.copy(); 726 dst.link = link == null ? null : link.copy(); 727 return dst; 728 } 729 730 @Override 731 public boolean equalsDeep(Base other_) { 732 if (!super.equalsDeep(other_)) 733 return false; 734 if (!(other_ instanceof DiagnosticReportImageComponent)) 735 return false; 736 DiagnosticReportImageComponent o = (DiagnosticReportImageComponent) other_; 737 return compareDeep(comment, o.comment, true) && compareDeep(link, o.link, true); 738 } 739 740 @Override 741 public boolean equalsShallow(Base other_) { 742 if (!super.equalsShallow(other_)) 743 return false; 744 if (!(other_ instanceof DiagnosticReportImageComponent)) 745 return false; 746 DiagnosticReportImageComponent o = (DiagnosticReportImageComponent) other_; 747 return compareValues(comment, o.comment, true); 748 } 749 750 public boolean isEmpty() { 751 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, link); 752 } 753 754 public String fhirType() { 755 return "DiagnosticReport.image"; 756 757 } 758 759 } 760 761 /** 762 * Identifiers assigned to this report by the performer or other systems. 763 */ 764 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 765 @Description(shortDefinition="Business identifier for report", formalDefinition="Identifiers assigned to this report by the performer or other systems." ) 766 protected List<Identifier> identifier; 767 768 /** 769 * Details concerning a test or procedure requested. 770 */ 771 @Child(name = "basedOn", type = {CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ProcedureRequest.class, ReferralRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 772 @Description(shortDefinition="What was requested", formalDefinition="Details concerning a test or procedure requested." ) 773 protected List<Reference> basedOn; 774 /** 775 * The actual objects that are the target of the reference (Details concerning a test or procedure requested.) 776 */ 777 protected List<Resource> basedOnTarget; 778 779 780 /** 781 * The status of the diagnostic report as a whole. 782 */ 783 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 784 @Description(shortDefinition="registered | partial | preliminary | final +", formalDefinition="The status of the diagnostic report as a whole." ) 785 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnostic-report-status") 786 protected Enumeration<DiagnosticReportStatus> status; 787 788 /** 789 * A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes. 790 */ 791 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 792 @Description(shortDefinition="Service category", formalDefinition="A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes." ) 793 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnostic-service-sections") 794 protected CodeableConcept category; 795 796 /** 797 * A code or name that describes this diagnostic report. 798 */ 799 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 800 @Description(shortDefinition="Name/Code for this diagnostic report", formalDefinition="A code or name that describes this diagnostic report." ) 801 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-codes") 802 protected CodeableConcept code; 803 804 /** 805 * The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources. 806 */ 807 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class}, order=5, min=0, max=1, modifier=false, summary=true) 808 @Description(shortDefinition="The subject of the report - usually, but not always, the patient", formalDefinition="The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources." ) 809 protected Reference subject; 810 811 /** 812 * The actual object that is the target of the reference (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 813 */ 814 protected Resource subjectTarget; 815 816 /** 817 * The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about. 818 */ 819 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=true) 820 @Description(shortDefinition="Health care event when test ordered", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about." ) 821 protected Reference context; 822 823 /** 824 * The actual object that is the target of the reference (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 825 */ 826 protected Resource contextTarget; 827 828 /** 829 * The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself. 830 */ 831 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 832 @Description(shortDefinition="Clinically relevant time/time-period for report", formalDefinition="The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself." ) 833 protected Type effective; 834 835 /** 836 * The date and time that this version of the report was released from the source diagnostic service. 837 */ 838 @Child(name = "issued", type = {InstantType.class}, order=8, min=0, max=1, modifier=false, summary=true) 839 @Description(shortDefinition="DateTime this version was released", formalDefinition="The date and time that this version of the report was released from the source diagnostic service." ) 840 protected InstantType issued; 841 842 /** 843 * Indicates who or what participated in producing the report. 844 */ 845 @Child(name = "performer", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 846 @Description(shortDefinition="Participants in producing the report", formalDefinition="Indicates who or what participated in producing the report." ) 847 protected List<DiagnosticReportPerformerComponent> performer; 848 849 /** 850 * Details about the specimens on which this diagnostic report is based. 851 */ 852 @Child(name = "specimen", type = {Specimen.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 853 @Description(shortDefinition="Specimens this report is based on", formalDefinition="Details about the specimens on which this diagnostic report is based." ) 854 protected List<Reference> specimen; 855 /** 856 * The actual objects that are the target of the reference (Details about the specimens on which this diagnostic report is based.) 857 */ 858 protected List<Specimen> specimenTarget; 859 860 861 /** 862 * Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. "atomic" results), or they can be grouping observations that include references to other members of the group (e.g. "panels"). 863 */ 864 @Child(name = "result", type = {Observation.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 865 @Description(shortDefinition="Observations - simple, or complex nested groups", formalDefinition="Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\")." ) 866 protected List<Reference> result; 867 /** 868 * The actual objects that are the target of the reference (Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. "atomic" results), or they can be grouping observations that include references to other members of the group (e.g. "panels").) 869 */ 870 protected List<Observation> resultTarget; 871 872 873 /** 874 * One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images. 875 */ 876 @Child(name = "imagingStudy", type = {ImagingStudy.class, ImagingManifest.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 877 @Description(shortDefinition="Reference to full details of imaging associated with the diagnostic report", formalDefinition="One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images." ) 878 protected List<Reference> imagingStudy; 879 /** 880 * The actual objects that are the target of the reference (One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.) 881 */ 882 protected List<Resource> imagingStudyTarget; 883 884 885 /** 886 * A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest). 887 */ 888 @Child(name = "image", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 889 @Description(shortDefinition="Key images associated with this report", formalDefinition="A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest)." ) 890 protected List<DiagnosticReportImageComponent> image; 891 892 /** 893 * Concise and clinically contextualized impression / summary of the diagnostic report. 894 */ 895 @Child(name = "conclusion", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 896 @Description(shortDefinition="Clinical Interpretation of test results", formalDefinition="Concise and clinically contextualized impression / summary of the diagnostic report." ) 897 protected StringType conclusion; 898 899 /** 900 * Codes for the conclusion. 901 */ 902 @Child(name = "codedDiagnosis", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 903 @Description(shortDefinition="Codes for the conclusion", formalDefinition="Codes for the conclusion." ) 904 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 905 protected List<CodeableConcept> codedDiagnosis; 906 907 /** 908 * Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent. 909 */ 910 @Child(name = "presentedForm", type = {Attachment.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 911 @Description(shortDefinition="Entire report as issued", formalDefinition="Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent." ) 912 protected List<Attachment> presentedForm; 913 914 private static final long serialVersionUID = 989012294L; 915 916 /** 917 * Constructor 918 */ 919 public DiagnosticReport() { 920 super(); 921 } 922 923 /** 924 * Constructor 925 */ 926 public DiagnosticReport(Enumeration<DiagnosticReportStatus> status, CodeableConcept code) { 927 super(); 928 this.status = status; 929 this.code = code; 930 } 931 932 /** 933 * @return {@link #identifier} (Identifiers assigned to this report by the performer or other systems.) 934 */ 935 public List<Identifier> getIdentifier() { 936 if (this.identifier == null) 937 this.identifier = new ArrayList<Identifier>(); 938 return this.identifier; 939 } 940 941 /** 942 * @return Returns a reference to <code>this</code> for easy method chaining 943 */ 944 public DiagnosticReport setIdentifier(List<Identifier> theIdentifier) { 945 this.identifier = theIdentifier; 946 return this; 947 } 948 949 public boolean hasIdentifier() { 950 if (this.identifier == null) 951 return false; 952 for (Identifier item : this.identifier) 953 if (!item.isEmpty()) 954 return true; 955 return false; 956 } 957 958 public Identifier addIdentifier() { //3 959 Identifier t = new Identifier(); 960 if (this.identifier == null) 961 this.identifier = new ArrayList<Identifier>(); 962 this.identifier.add(t); 963 return t; 964 } 965 966 public DiagnosticReport addIdentifier(Identifier t) { //3 967 if (t == null) 968 return this; 969 if (this.identifier == null) 970 this.identifier = new ArrayList<Identifier>(); 971 this.identifier.add(t); 972 return this; 973 } 974 975 /** 976 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 977 */ 978 public Identifier getIdentifierFirstRep() { 979 if (getIdentifier().isEmpty()) { 980 addIdentifier(); 981 } 982 return getIdentifier().get(0); 983 } 984 985 /** 986 * @return {@link #basedOn} (Details concerning a test or procedure requested.) 987 */ 988 public List<Reference> getBasedOn() { 989 if (this.basedOn == null) 990 this.basedOn = new ArrayList<Reference>(); 991 return this.basedOn; 992 } 993 994 /** 995 * @return Returns a reference to <code>this</code> for easy method chaining 996 */ 997 public DiagnosticReport setBasedOn(List<Reference> theBasedOn) { 998 this.basedOn = theBasedOn; 999 return this; 1000 } 1001 1002 public boolean hasBasedOn() { 1003 if (this.basedOn == null) 1004 return false; 1005 for (Reference item : this.basedOn) 1006 if (!item.isEmpty()) 1007 return true; 1008 return false; 1009 } 1010 1011 public Reference addBasedOn() { //3 1012 Reference t = new Reference(); 1013 if (this.basedOn == null) 1014 this.basedOn = new ArrayList<Reference>(); 1015 this.basedOn.add(t); 1016 return t; 1017 } 1018 1019 public DiagnosticReport addBasedOn(Reference t) { //3 1020 if (t == null) 1021 return this; 1022 if (this.basedOn == null) 1023 this.basedOn = new ArrayList<Reference>(); 1024 this.basedOn.add(t); 1025 return this; 1026 } 1027 1028 /** 1029 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1030 */ 1031 public Reference getBasedOnFirstRep() { 1032 if (getBasedOn().isEmpty()) { 1033 addBasedOn(); 1034 } 1035 return getBasedOn().get(0); 1036 } 1037 1038 /** 1039 * @deprecated Use Reference#setResource(IBaseResource) instead 1040 */ 1041 @Deprecated 1042 public List<Resource> getBasedOnTarget() { 1043 if (this.basedOnTarget == null) 1044 this.basedOnTarget = new ArrayList<Resource>(); 1045 return this.basedOnTarget; 1046 } 1047 1048 /** 1049 * @return {@link #status} (The status of the diagnostic report as a whole.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1050 */ 1051 public Enumeration<DiagnosticReportStatus> getStatusElement() { 1052 if (this.status == null) 1053 if (Configuration.errorOnAutoCreate()) 1054 throw new Error("Attempt to auto-create DiagnosticReport.status"); 1055 else if (Configuration.doAutoCreate()) 1056 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); // bb 1057 return this.status; 1058 } 1059 1060 public boolean hasStatusElement() { 1061 return this.status != null && !this.status.isEmpty(); 1062 } 1063 1064 public boolean hasStatus() { 1065 return this.status != null && !this.status.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #status} (The status of the diagnostic report as a whole.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1070 */ 1071 public DiagnosticReport setStatusElement(Enumeration<DiagnosticReportStatus> value) { 1072 this.status = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return The status of the diagnostic report as a whole. 1078 */ 1079 public DiagnosticReportStatus getStatus() { 1080 return this.status == null ? null : this.status.getValue(); 1081 } 1082 1083 /** 1084 * @param value The status of the diagnostic report as a whole. 1085 */ 1086 public DiagnosticReport setStatus(DiagnosticReportStatus value) { 1087 if (this.status == null) 1088 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); 1089 this.status.setValue(value); 1090 return this; 1091 } 1092 1093 /** 1094 * @return {@link #category} (A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.) 1095 */ 1096 public CodeableConcept getCategory() { 1097 if (this.category == null) 1098 if (Configuration.errorOnAutoCreate()) 1099 throw new Error("Attempt to auto-create DiagnosticReport.category"); 1100 else if (Configuration.doAutoCreate()) 1101 this.category = new CodeableConcept(); // cc 1102 return this.category; 1103 } 1104 1105 public boolean hasCategory() { 1106 return this.category != null && !this.category.isEmpty(); 1107 } 1108 1109 /** 1110 * @param value {@link #category} (A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.) 1111 */ 1112 public DiagnosticReport setCategory(CodeableConcept value) { 1113 this.category = value; 1114 return this; 1115 } 1116 1117 /** 1118 * @return {@link #code} (A code or name that describes this diagnostic report.) 1119 */ 1120 public CodeableConcept getCode() { 1121 if (this.code == null) 1122 if (Configuration.errorOnAutoCreate()) 1123 throw new Error("Attempt to auto-create DiagnosticReport.code"); 1124 else if (Configuration.doAutoCreate()) 1125 this.code = new CodeableConcept(); // cc 1126 return this.code; 1127 } 1128 1129 public boolean hasCode() { 1130 return this.code != null && !this.code.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #code} (A code or name that describes this diagnostic report.) 1135 */ 1136 public DiagnosticReport setCode(CodeableConcept value) { 1137 this.code = value; 1138 return this; 1139 } 1140 1141 /** 1142 * @return {@link #subject} (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1143 */ 1144 public Reference getSubject() { 1145 if (this.subject == null) 1146 if (Configuration.errorOnAutoCreate()) 1147 throw new Error("Attempt to auto-create DiagnosticReport.subject"); 1148 else if (Configuration.doAutoCreate()) 1149 this.subject = new Reference(); // cc 1150 return this.subject; 1151 } 1152 1153 public boolean hasSubject() { 1154 return this.subject != null && !this.subject.isEmpty(); 1155 } 1156 1157 /** 1158 * @param value {@link #subject} (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1159 */ 1160 public DiagnosticReport setSubject(Reference value) { 1161 this.subject = value; 1162 return this; 1163 } 1164 1165 /** 1166 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1167 */ 1168 public Resource getSubjectTarget() { 1169 return this.subjectTarget; 1170 } 1171 1172 /** 1173 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1174 */ 1175 public DiagnosticReport setSubjectTarget(Resource value) { 1176 this.subjectTarget = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1182 */ 1183 public Reference getContext() { 1184 if (this.context == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create DiagnosticReport.context"); 1187 else if (Configuration.doAutoCreate()) 1188 this.context = new Reference(); // cc 1189 return this.context; 1190 } 1191 1192 public boolean hasContext() { 1193 return this.context != null && !this.context.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1198 */ 1199 public DiagnosticReport setContext(Reference value) { 1200 this.context = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1206 */ 1207 public Resource getContextTarget() { 1208 return this.contextTarget; 1209 } 1210 1211 /** 1212 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1213 */ 1214 public DiagnosticReport setContextTarget(Resource value) { 1215 this.contextTarget = value; 1216 return this; 1217 } 1218 1219 /** 1220 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1221 */ 1222 public Type getEffective() { 1223 return this.effective; 1224 } 1225 1226 /** 1227 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1228 */ 1229 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1230 if (this.effective == null) 1231 return null; 1232 if (!(this.effective instanceof DateTimeType)) 1233 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 1234 return (DateTimeType) this.effective; 1235 } 1236 1237 public boolean hasEffectiveDateTimeType() { 1238 return this != null && this.effective instanceof DateTimeType; 1239 } 1240 1241 /** 1242 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1243 */ 1244 public Period getEffectivePeriod() throws FHIRException { 1245 if (this.effective == null) 1246 return null; 1247 if (!(this.effective instanceof Period)) 1248 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 1249 return (Period) this.effective; 1250 } 1251 1252 public boolean hasEffectivePeriod() { 1253 return this != null && this.effective instanceof Period; 1254 } 1255 1256 public boolean hasEffective() { 1257 return this.effective != null && !this.effective.isEmpty(); 1258 } 1259 1260 /** 1261 * @param value {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1262 */ 1263 public DiagnosticReport setEffective(Type value) throws FHIRFormatError { 1264 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1265 throw new FHIRFormatError("Not the right type for DiagnosticReport.effective[x]: "+value.fhirType()); 1266 this.effective = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return {@link #issued} (The date and time that this version of the report was released from the source diagnostic service.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1272 */ 1273 public InstantType getIssuedElement() { 1274 if (this.issued == null) 1275 if (Configuration.errorOnAutoCreate()) 1276 throw new Error("Attempt to auto-create DiagnosticReport.issued"); 1277 else if (Configuration.doAutoCreate()) 1278 this.issued = new InstantType(); // bb 1279 return this.issued; 1280 } 1281 1282 public boolean hasIssuedElement() { 1283 return this.issued != null && !this.issued.isEmpty(); 1284 } 1285 1286 public boolean hasIssued() { 1287 return this.issued != null && !this.issued.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #issued} (The date and time that this version of the report was released from the source diagnostic service.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1292 */ 1293 public DiagnosticReport setIssuedElement(InstantType value) { 1294 this.issued = value; 1295 return this; 1296 } 1297 1298 /** 1299 * @return The date and time that this version of the report was released from the source diagnostic service. 1300 */ 1301 public Date getIssued() { 1302 return this.issued == null ? null : this.issued.getValue(); 1303 } 1304 1305 /** 1306 * @param value The date and time that this version of the report was released from the source diagnostic service. 1307 */ 1308 public DiagnosticReport setIssued(Date value) { 1309 if (value == null) 1310 this.issued = null; 1311 else { 1312 if (this.issued == null) 1313 this.issued = new InstantType(); 1314 this.issued.setValue(value); 1315 } 1316 return this; 1317 } 1318 1319 /** 1320 * @return {@link #performer} (Indicates who or what participated in producing the report.) 1321 */ 1322 public List<DiagnosticReportPerformerComponent> getPerformer() { 1323 if (this.performer == null) 1324 this.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 1325 return this.performer; 1326 } 1327 1328 /** 1329 * @return Returns a reference to <code>this</code> for easy method chaining 1330 */ 1331 public DiagnosticReport setPerformer(List<DiagnosticReportPerformerComponent> thePerformer) { 1332 this.performer = thePerformer; 1333 return this; 1334 } 1335 1336 public boolean hasPerformer() { 1337 if (this.performer == null) 1338 return false; 1339 for (DiagnosticReportPerformerComponent item : this.performer) 1340 if (!item.isEmpty()) 1341 return true; 1342 return false; 1343 } 1344 1345 public DiagnosticReportPerformerComponent addPerformer() { //3 1346 DiagnosticReportPerformerComponent t = new DiagnosticReportPerformerComponent(); 1347 if (this.performer == null) 1348 this.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 1349 this.performer.add(t); 1350 return t; 1351 } 1352 1353 public DiagnosticReport addPerformer(DiagnosticReportPerformerComponent t) { //3 1354 if (t == null) 1355 return this; 1356 if (this.performer == null) 1357 this.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 1358 this.performer.add(t); 1359 return this; 1360 } 1361 1362 /** 1363 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 1364 */ 1365 public DiagnosticReportPerformerComponent getPerformerFirstRep() { 1366 if (getPerformer().isEmpty()) { 1367 addPerformer(); 1368 } 1369 return getPerformer().get(0); 1370 } 1371 1372 /** 1373 * @return {@link #specimen} (Details about the specimens on which this diagnostic report is based.) 1374 */ 1375 public List<Reference> getSpecimen() { 1376 if (this.specimen == null) 1377 this.specimen = new ArrayList<Reference>(); 1378 return this.specimen; 1379 } 1380 1381 /** 1382 * @return Returns a reference to <code>this</code> for easy method chaining 1383 */ 1384 public DiagnosticReport setSpecimen(List<Reference> theSpecimen) { 1385 this.specimen = theSpecimen; 1386 return this; 1387 } 1388 1389 public boolean hasSpecimen() { 1390 if (this.specimen == null) 1391 return false; 1392 for (Reference item : this.specimen) 1393 if (!item.isEmpty()) 1394 return true; 1395 return false; 1396 } 1397 1398 public Reference addSpecimen() { //3 1399 Reference t = new Reference(); 1400 if (this.specimen == null) 1401 this.specimen = new ArrayList<Reference>(); 1402 this.specimen.add(t); 1403 return t; 1404 } 1405 1406 public DiagnosticReport addSpecimen(Reference t) { //3 1407 if (t == null) 1408 return this; 1409 if (this.specimen == null) 1410 this.specimen = new ArrayList<Reference>(); 1411 this.specimen.add(t); 1412 return this; 1413 } 1414 1415 /** 1416 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist 1417 */ 1418 public Reference getSpecimenFirstRep() { 1419 if (getSpecimen().isEmpty()) { 1420 addSpecimen(); 1421 } 1422 return getSpecimen().get(0); 1423 } 1424 1425 /** 1426 * @deprecated Use Reference#setResource(IBaseResource) instead 1427 */ 1428 @Deprecated 1429 public List<Specimen> getSpecimenTarget() { 1430 if (this.specimenTarget == null) 1431 this.specimenTarget = new ArrayList<Specimen>(); 1432 return this.specimenTarget; 1433 } 1434 1435 /** 1436 * @deprecated Use Reference#setResource(IBaseResource) instead 1437 */ 1438 @Deprecated 1439 public Specimen addSpecimenTarget() { 1440 Specimen r = new Specimen(); 1441 if (this.specimenTarget == null) 1442 this.specimenTarget = new ArrayList<Specimen>(); 1443 this.specimenTarget.add(r); 1444 return r; 1445 } 1446 1447 /** 1448 * @return {@link #result} (Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. "atomic" results), or they can be grouping observations that include references to other members of the group (e.g. "panels").) 1449 */ 1450 public List<Reference> getResult() { 1451 if (this.result == null) 1452 this.result = new ArrayList<Reference>(); 1453 return this.result; 1454 } 1455 1456 /** 1457 * @return Returns a reference to <code>this</code> for easy method chaining 1458 */ 1459 public DiagnosticReport setResult(List<Reference> theResult) { 1460 this.result = theResult; 1461 return this; 1462 } 1463 1464 public boolean hasResult() { 1465 if (this.result == null) 1466 return false; 1467 for (Reference item : this.result) 1468 if (!item.isEmpty()) 1469 return true; 1470 return false; 1471 } 1472 1473 public Reference addResult() { //3 1474 Reference t = new Reference(); 1475 if (this.result == null) 1476 this.result = new ArrayList<Reference>(); 1477 this.result.add(t); 1478 return t; 1479 } 1480 1481 public DiagnosticReport addResult(Reference t) { //3 1482 if (t == null) 1483 return this; 1484 if (this.result == null) 1485 this.result = new ArrayList<Reference>(); 1486 this.result.add(t); 1487 return this; 1488 } 1489 1490 /** 1491 * @return The first repetition of repeating field {@link #result}, creating it if it does not already exist 1492 */ 1493 public Reference getResultFirstRep() { 1494 if (getResult().isEmpty()) { 1495 addResult(); 1496 } 1497 return getResult().get(0); 1498 } 1499 1500 /** 1501 * @deprecated Use Reference#setResource(IBaseResource) instead 1502 */ 1503 @Deprecated 1504 public List<Observation> getResultTarget() { 1505 if (this.resultTarget == null) 1506 this.resultTarget = new ArrayList<Observation>(); 1507 return this.resultTarget; 1508 } 1509 1510 /** 1511 * @deprecated Use Reference#setResource(IBaseResource) instead 1512 */ 1513 @Deprecated 1514 public Observation addResultTarget() { 1515 Observation r = new Observation(); 1516 if (this.resultTarget == null) 1517 this.resultTarget = new ArrayList<Observation>(); 1518 this.resultTarget.add(r); 1519 return r; 1520 } 1521 1522 /** 1523 * @return {@link #imagingStudy} (One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.) 1524 */ 1525 public List<Reference> getImagingStudy() { 1526 if (this.imagingStudy == null) 1527 this.imagingStudy = new ArrayList<Reference>(); 1528 return this.imagingStudy; 1529 } 1530 1531 /** 1532 * @return Returns a reference to <code>this</code> for easy method chaining 1533 */ 1534 public DiagnosticReport setImagingStudy(List<Reference> theImagingStudy) { 1535 this.imagingStudy = theImagingStudy; 1536 return this; 1537 } 1538 1539 public boolean hasImagingStudy() { 1540 if (this.imagingStudy == null) 1541 return false; 1542 for (Reference item : this.imagingStudy) 1543 if (!item.isEmpty()) 1544 return true; 1545 return false; 1546 } 1547 1548 public Reference addImagingStudy() { //3 1549 Reference t = new Reference(); 1550 if (this.imagingStudy == null) 1551 this.imagingStudy = new ArrayList<Reference>(); 1552 this.imagingStudy.add(t); 1553 return t; 1554 } 1555 1556 public DiagnosticReport addImagingStudy(Reference t) { //3 1557 if (t == null) 1558 return this; 1559 if (this.imagingStudy == null) 1560 this.imagingStudy = new ArrayList<Reference>(); 1561 this.imagingStudy.add(t); 1562 return this; 1563 } 1564 1565 /** 1566 * @return The first repetition of repeating field {@link #imagingStudy}, creating it if it does not already exist 1567 */ 1568 public Reference getImagingStudyFirstRep() { 1569 if (getImagingStudy().isEmpty()) { 1570 addImagingStudy(); 1571 } 1572 return getImagingStudy().get(0); 1573 } 1574 1575 /** 1576 * @deprecated Use Reference#setResource(IBaseResource) instead 1577 */ 1578 @Deprecated 1579 public List<Resource> getImagingStudyTarget() { 1580 if (this.imagingStudyTarget == null) 1581 this.imagingStudyTarget = new ArrayList<Resource>(); 1582 return this.imagingStudyTarget; 1583 } 1584 1585 /** 1586 * @return {@link #image} (A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).) 1587 */ 1588 public List<DiagnosticReportImageComponent> getImage() { 1589 if (this.image == null) 1590 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1591 return this.image; 1592 } 1593 1594 /** 1595 * @return Returns a reference to <code>this</code> for easy method chaining 1596 */ 1597 public DiagnosticReport setImage(List<DiagnosticReportImageComponent> theImage) { 1598 this.image = theImage; 1599 return this; 1600 } 1601 1602 public boolean hasImage() { 1603 if (this.image == null) 1604 return false; 1605 for (DiagnosticReportImageComponent item : this.image) 1606 if (!item.isEmpty()) 1607 return true; 1608 return false; 1609 } 1610 1611 public DiagnosticReportImageComponent addImage() { //3 1612 DiagnosticReportImageComponent t = new DiagnosticReportImageComponent(); 1613 if (this.image == null) 1614 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1615 this.image.add(t); 1616 return t; 1617 } 1618 1619 public DiagnosticReport addImage(DiagnosticReportImageComponent t) { //3 1620 if (t == null) 1621 return this; 1622 if (this.image == null) 1623 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1624 this.image.add(t); 1625 return this; 1626 } 1627 1628 /** 1629 * @return The first repetition of repeating field {@link #image}, creating it if it does not already exist 1630 */ 1631 public DiagnosticReportImageComponent getImageFirstRep() { 1632 if (getImage().isEmpty()) { 1633 addImage(); 1634 } 1635 return getImage().get(0); 1636 } 1637 1638 /** 1639 * @return {@link #conclusion} (Concise and clinically contextualized impression / summary of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getConclusion" gives direct access to the value 1640 */ 1641 public StringType getConclusionElement() { 1642 if (this.conclusion == null) 1643 if (Configuration.errorOnAutoCreate()) 1644 throw new Error("Attempt to auto-create DiagnosticReport.conclusion"); 1645 else if (Configuration.doAutoCreate()) 1646 this.conclusion = new StringType(); // bb 1647 return this.conclusion; 1648 } 1649 1650 public boolean hasConclusionElement() { 1651 return this.conclusion != null && !this.conclusion.isEmpty(); 1652 } 1653 1654 public boolean hasConclusion() { 1655 return this.conclusion != null && !this.conclusion.isEmpty(); 1656 } 1657 1658 /** 1659 * @param value {@link #conclusion} (Concise and clinically contextualized impression / summary of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getConclusion" gives direct access to the value 1660 */ 1661 public DiagnosticReport setConclusionElement(StringType value) { 1662 this.conclusion = value; 1663 return this; 1664 } 1665 1666 /** 1667 * @return Concise and clinically contextualized impression / summary of the diagnostic report. 1668 */ 1669 public String getConclusion() { 1670 return this.conclusion == null ? null : this.conclusion.getValue(); 1671 } 1672 1673 /** 1674 * @param value Concise and clinically contextualized impression / summary of the diagnostic report. 1675 */ 1676 public DiagnosticReport setConclusion(String value) { 1677 if (Utilities.noString(value)) 1678 this.conclusion = null; 1679 else { 1680 if (this.conclusion == null) 1681 this.conclusion = new StringType(); 1682 this.conclusion.setValue(value); 1683 } 1684 return this; 1685 } 1686 1687 /** 1688 * @return {@link #codedDiagnosis} (Codes for the conclusion.) 1689 */ 1690 public List<CodeableConcept> getCodedDiagnosis() { 1691 if (this.codedDiagnosis == null) 1692 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1693 return this.codedDiagnosis; 1694 } 1695 1696 /** 1697 * @return Returns a reference to <code>this</code> for easy method chaining 1698 */ 1699 public DiagnosticReport setCodedDiagnosis(List<CodeableConcept> theCodedDiagnosis) { 1700 this.codedDiagnosis = theCodedDiagnosis; 1701 return this; 1702 } 1703 1704 public boolean hasCodedDiagnosis() { 1705 if (this.codedDiagnosis == null) 1706 return false; 1707 for (CodeableConcept item : this.codedDiagnosis) 1708 if (!item.isEmpty()) 1709 return true; 1710 return false; 1711 } 1712 1713 public CodeableConcept addCodedDiagnosis() { //3 1714 CodeableConcept t = new CodeableConcept(); 1715 if (this.codedDiagnosis == null) 1716 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1717 this.codedDiagnosis.add(t); 1718 return t; 1719 } 1720 1721 public DiagnosticReport addCodedDiagnosis(CodeableConcept t) { //3 1722 if (t == null) 1723 return this; 1724 if (this.codedDiagnosis == null) 1725 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1726 this.codedDiagnosis.add(t); 1727 return this; 1728 } 1729 1730 /** 1731 * @return The first repetition of repeating field {@link #codedDiagnosis}, creating it if it does not already exist 1732 */ 1733 public CodeableConcept getCodedDiagnosisFirstRep() { 1734 if (getCodedDiagnosis().isEmpty()) { 1735 addCodedDiagnosis(); 1736 } 1737 return getCodedDiagnosis().get(0); 1738 } 1739 1740 /** 1741 * @return {@link #presentedForm} (Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.) 1742 */ 1743 public List<Attachment> getPresentedForm() { 1744 if (this.presentedForm == null) 1745 this.presentedForm = new ArrayList<Attachment>(); 1746 return this.presentedForm; 1747 } 1748 1749 /** 1750 * @return Returns a reference to <code>this</code> for easy method chaining 1751 */ 1752 public DiagnosticReport setPresentedForm(List<Attachment> thePresentedForm) { 1753 this.presentedForm = thePresentedForm; 1754 return this; 1755 } 1756 1757 public boolean hasPresentedForm() { 1758 if (this.presentedForm == null) 1759 return false; 1760 for (Attachment item : this.presentedForm) 1761 if (!item.isEmpty()) 1762 return true; 1763 return false; 1764 } 1765 1766 public Attachment addPresentedForm() { //3 1767 Attachment t = new Attachment(); 1768 if (this.presentedForm == null) 1769 this.presentedForm = new ArrayList<Attachment>(); 1770 this.presentedForm.add(t); 1771 return t; 1772 } 1773 1774 public DiagnosticReport addPresentedForm(Attachment t) { //3 1775 if (t == null) 1776 return this; 1777 if (this.presentedForm == null) 1778 this.presentedForm = new ArrayList<Attachment>(); 1779 this.presentedForm.add(t); 1780 return this; 1781 } 1782 1783 /** 1784 * @return The first repetition of repeating field {@link #presentedForm}, creating it if it does not already exist 1785 */ 1786 public Attachment getPresentedFormFirstRep() { 1787 if (getPresentedForm().isEmpty()) { 1788 addPresentedForm(); 1789 } 1790 return getPresentedForm().get(0); 1791 } 1792 1793 protected void listChildren(List<Property> children) { 1794 super.listChildren(children); 1795 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1796 children.add(new Property("basedOn", "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ProcedureRequest|ReferralRequest)", "Details concerning a test or procedure requested.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1797 children.add(new Property("status", "code", "The status of the diagnostic report as a whole.", 0, 1, status)); 1798 children.add(new Property("category", "CodeableConcept", "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 0, 1, category)); 1799 children.add(new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code)); 1800 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", "The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.", 0, 1, subject)); 1801 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.", 0, 1, context)); 1802 children.add(new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective)); 1803 children.add(new Property("issued", "instant", "The date and time that this version of the report was released from the source diagnostic service.", 0, 1, issued)); 1804 children.add(new Property("performer", "", "Indicates who or what participated in producing the report.", 0, java.lang.Integer.MAX_VALUE, performer)); 1805 children.add(new Property("specimen", "Reference(Specimen)", "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, specimen)); 1806 children.add(new Property("result", "Reference(Observation)", "Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\").", 0, java.lang.Integer.MAX_VALUE, result)); 1807 children.add(new Property("imagingStudy", "Reference(ImagingStudy|ImagingManifest)", "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 0, java.lang.Integer.MAX_VALUE, imagingStudy)); 1808 children.add(new Property("image", "", "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 0, java.lang.Integer.MAX_VALUE, image)); 1809 children.add(new Property("conclusion", "string", "Concise and clinically contextualized impression / summary of the diagnostic report.", 0, 1, conclusion)); 1810 children.add(new Property("codedDiagnosis", "CodeableConcept", "Codes for the conclusion.", 0, java.lang.Integer.MAX_VALUE, codedDiagnosis)); 1811 children.add(new Property("presentedForm", "Attachment", "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 0, java.lang.Integer.MAX_VALUE, presentedForm)); 1812 } 1813 1814 @Override 1815 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1816 switch (_hash) { 1817 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1818 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ProcedureRequest|ReferralRequest)", "Details concerning a test or procedure requested.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1819 case -892481550: /*status*/ return new Property("status", "code", "The status of the diagnostic report as a whole.", 0, 1, status); 1820 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 0, 1, category); 1821 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code); 1822 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location)", "The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.", 0, 1, subject); 1823 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.", 0, 1, context); 1824 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1825 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1826 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1827 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1828 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time that this version of the report was released from the source diagnostic service.", 0, 1, issued); 1829 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what participated in producing the report.", 0, java.lang.Integer.MAX_VALUE, performer); 1830 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, specimen); 1831 case -934426595: /*result*/ return new Property("result", "Reference(Observation)", "Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\").", 0, java.lang.Integer.MAX_VALUE, result); 1832 case -814900911: /*imagingStudy*/ return new Property("imagingStudy", "Reference(ImagingStudy|ImagingManifest)", "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 0, java.lang.Integer.MAX_VALUE, imagingStudy); 1833 case 100313435: /*image*/ return new Property("image", "", "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 0, java.lang.Integer.MAX_VALUE, image); 1834 case -1731259873: /*conclusion*/ return new Property("conclusion", "string", "Concise and clinically contextualized impression / summary of the diagnostic report.", 0, 1, conclusion); 1835 case -1364269926: /*codedDiagnosis*/ return new Property("codedDiagnosis", "CodeableConcept", "Codes for the conclusion.", 0, java.lang.Integer.MAX_VALUE, codedDiagnosis); 1836 case 230090366: /*presentedForm*/ return new Property("presentedForm", "Attachment", "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 0, java.lang.Integer.MAX_VALUE, presentedForm); 1837 default: return super.getNamedProperty(_hash, _name, _checkValid); 1838 } 1839 1840 } 1841 1842 @Override 1843 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1844 switch (hash) { 1845 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1846 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1847 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DiagnosticReportStatus> 1848 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1849 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1850 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1851 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1852 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // Type 1853 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 1854 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // DiagnosticReportPerformerComponent 1855 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 1856 case -934426595: /*result*/ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 1857 case -814900911: /*imagingStudy*/ return this.imagingStudy == null ? new Base[0] : this.imagingStudy.toArray(new Base[this.imagingStudy.size()]); // Reference 1858 case 100313435: /*image*/ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // DiagnosticReportImageComponent 1859 case -1731259873: /*conclusion*/ return this.conclusion == null ? new Base[0] : new Base[] {this.conclusion}; // StringType 1860 case -1364269926: /*codedDiagnosis*/ return this.codedDiagnosis == null ? new Base[0] : this.codedDiagnosis.toArray(new Base[this.codedDiagnosis.size()]); // CodeableConcept 1861 case 230090366: /*presentedForm*/ return this.presentedForm == null ? new Base[0] : this.presentedForm.toArray(new Base[this.presentedForm.size()]); // Attachment 1862 default: return super.getProperty(hash, name, checkValid); 1863 } 1864 1865 } 1866 1867 @Override 1868 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1869 switch (hash) { 1870 case -1618432855: // identifier 1871 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1872 return value; 1873 case -332612366: // basedOn 1874 this.getBasedOn().add(castToReference(value)); // Reference 1875 return value; 1876 case -892481550: // status 1877 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 1878 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 1879 return value; 1880 case 50511102: // category 1881 this.category = castToCodeableConcept(value); // CodeableConcept 1882 return value; 1883 case 3059181: // code 1884 this.code = castToCodeableConcept(value); // CodeableConcept 1885 return value; 1886 case -1867885268: // subject 1887 this.subject = castToReference(value); // Reference 1888 return value; 1889 case 951530927: // context 1890 this.context = castToReference(value); // Reference 1891 return value; 1892 case -1468651097: // effective 1893 this.effective = castToType(value); // Type 1894 return value; 1895 case -1179159893: // issued 1896 this.issued = castToInstant(value); // InstantType 1897 return value; 1898 case 481140686: // performer 1899 this.getPerformer().add((DiagnosticReportPerformerComponent) value); // DiagnosticReportPerformerComponent 1900 return value; 1901 case -2132868344: // specimen 1902 this.getSpecimen().add(castToReference(value)); // Reference 1903 return value; 1904 case -934426595: // result 1905 this.getResult().add(castToReference(value)); // Reference 1906 return value; 1907 case -814900911: // imagingStudy 1908 this.getImagingStudy().add(castToReference(value)); // Reference 1909 return value; 1910 case 100313435: // image 1911 this.getImage().add((DiagnosticReportImageComponent) value); // DiagnosticReportImageComponent 1912 return value; 1913 case -1731259873: // conclusion 1914 this.conclusion = castToString(value); // StringType 1915 return value; 1916 case -1364269926: // codedDiagnosis 1917 this.getCodedDiagnosis().add(castToCodeableConcept(value)); // CodeableConcept 1918 return value; 1919 case 230090366: // presentedForm 1920 this.getPresentedForm().add(castToAttachment(value)); // Attachment 1921 return value; 1922 default: return super.setProperty(hash, name, value); 1923 } 1924 1925 } 1926 1927 @Override 1928 public Base setProperty(String name, Base value) throws FHIRException { 1929 if (name.equals("identifier")) { 1930 this.getIdentifier().add(castToIdentifier(value)); 1931 } else if (name.equals("basedOn")) { 1932 this.getBasedOn().add(castToReference(value)); 1933 } else if (name.equals("status")) { 1934 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 1935 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 1936 } else if (name.equals("category")) { 1937 this.category = castToCodeableConcept(value); // CodeableConcept 1938 } else if (name.equals("code")) { 1939 this.code = castToCodeableConcept(value); // CodeableConcept 1940 } else if (name.equals("subject")) { 1941 this.subject = castToReference(value); // Reference 1942 } else if (name.equals("context")) { 1943 this.context = castToReference(value); // Reference 1944 } else if (name.equals("effective[x]")) { 1945 this.effective = castToType(value); // Type 1946 } else if (name.equals("issued")) { 1947 this.issued = castToInstant(value); // InstantType 1948 } else if (name.equals("performer")) { 1949 this.getPerformer().add((DiagnosticReportPerformerComponent) value); 1950 } else if (name.equals("specimen")) { 1951 this.getSpecimen().add(castToReference(value)); 1952 } else if (name.equals("result")) { 1953 this.getResult().add(castToReference(value)); 1954 } else if (name.equals("imagingStudy")) { 1955 this.getImagingStudy().add(castToReference(value)); 1956 } else if (name.equals("image")) { 1957 this.getImage().add((DiagnosticReportImageComponent) value); 1958 } else if (name.equals("conclusion")) { 1959 this.conclusion = castToString(value); // StringType 1960 } else if (name.equals("codedDiagnosis")) { 1961 this.getCodedDiagnosis().add(castToCodeableConcept(value)); 1962 } else if (name.equals("presentedForm")) { 1963 this.getPresentedForm().add(castToAttachment(value)); 1964 } else 1965 return super.setProperty(name, value); 1966 return value; 1967 } 1968 1969 @Override 1970 public Base makeProperty(int hash, String name) throws FHIRException { 1971 switch (hash) { 1972 case -1618432855: return addIdentifier(); 1973 case -332612366: return addBasedOn(); 1974 case -892481550: return getStatusElement(); 1975 case 50511102: return getCategory(); 1976 case 3059181: return getCode(); 1977 case -1867885268: return getSubject(); 1978 case 951530927: return getContext(); 1979 case 247104889: return getEffective(); 1980 case -1468651097: return getEffective(); 1981 case -1179159893: return getIssuedElement(); 1982 case 481140686: return addPerformer(); 1983 case -2132868344: return addSpecimen(); 1984 case -934426595: return addResult(); 1985 case -814900911: return addImagingStudy(); 1986 case 100313435: return addImage(); 1987 case -1731259873: return getConclusionElement(); 1988 case -1364269926: return addCodedDiagnosis(); 1989 case 230090366: return addPresentedForm(); 1990 default: return super.makeProperty(hash, name); 1991 } 1992 1993 } 1994 1995 @Override 1996 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1997 switch (hash) { 1998 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1999 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2000 case -892481550: /*status*/ return new String[] {"code"}; 2001 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2002 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2003 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2004 case 951530927: /*context*/ return new String[] {"Reference"}; 2005 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 2006 case -1179159893: /*issued*/ return new String[] {"instant"}; 2007 case 481140686: /*performer*/ return new String[] {}; 2008 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 2009 case -934426595: /*result*/ return new String[] {"Reference"}; 2010 case -814900911: /*imagingStudy*/ return new String[] {"Reference"}; 2011 case 100313435: /*image*/ return new String[] {}; 2012 case -1731259873: /*conclusion*/ return new String[] {"string"}; 2013 case -1364269926: /*codedDiagnosis*/ return new String[] {"CodeableConcept"}; 2014 case 230090366: /*presentedForm*/ return new String[] {"Attachment"}; 2015 default: return super.getTypesForProperty(hash, name); 2016 } 2017 2018 } 2019 2020 @Override 2021 public Base addChild(String name) throws FHIRException { 2022 if (name.equals("identifier")) { 2023 return addIdentifier(); 2024 } 2025 else if (name.equals("basedOn")) { 2026 return addBasedOn(); 2027 } 2028 else if (name.equals("status")) { 2029 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.status"); 2030 } 2031 else if (name.equals("category")) { 2032 this.category = new CodeableConcept(); 2033 return this.category; 2034 } 2035 else if (name.equals("code")) { 2036 this.code = new CodeableConcept(); 2037 return this.code; 2038 } 2039 else if (name.equals("subject")) { 2040 this.subject = new Reference(); 2041 return this.subject; 2042 } 2043 else if (name.equals("context")) { 2044 this.context = new Reference(); 2045 return this.context; 2046 } 2047 else if (name.equals("effectiveDateTime")) { 2048 this.effective = new DateTimeType(); 2049 return this.effective; 2050 } 2051 else if (name.equals("effectivePeriod")) { 2052 this.effective = new Period(); 2053 return this.effective; 2054 } 2055 else if (name.equals("issued")) { 2056 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.issued"); 2057 } 2058 else if (name.equals("performer")) { 2059 return addPerformer(); 2060 } 2061 else if (name.equals("specimen")) { 2062 return addSpecimen(); 2063 } 2064 else if (name.equals("result")) { 2065 return addResult(); 2066 } 2067 else if (name.equals("imagingStudy")) { 2068 return addImagingStudy(); 2069 } 2070 else if (name.equals("image")) { 2071 return addImage(); 2072 } 2073 else if (name.equals("conclusion")) { 2074 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.conclusion"); 2075 } 2076 else if (name.equals("codedDiagnosis")) { 2077 return addCodedDiagnosis(); 2078 } 2079 else if (name.equals("presentedForm")) { 2080 return addPresentedForm(); 2081 } 2082 else 2083 return super.addChild(name); 2084 } 2085 2086 public String fhirType() { 2087 return "DiagnosticReport"; 2088 2089 } 2090 2091 public DiagnosticReport copy() { 2092 DiagnosticReport dst = new DiagnosticReport(); 2093 copyValues(dst); 2094 if (identifier != null) { 2095 dst.identifier = new ArrayList<Identifier>(); 2096 for (Identifier i : identifier) 2097 dst.identifier.add(i.copy()); 2098 }; 2099 if (basedOn != null) { 2100 dst.basedOn = new ArrayList<Reference>(); 2101 for (Reference i : basedOn) 2102 dst.basedOn.add(i.copy()); 2103 }; 2104 dst.status = status == null ? null : status.copy(); 2105 dst.category = category == null ? null : category.copy(); 2106 dst.code = code == null ? null : code.copy(); 2107 dst.subject = subject == null ? null : subject.copy(); 2108 dst.context = context == null ? null : context.copy(); 2109 dst.effective = effective == null ? null : effective.copy(); 2110 dst.issued = issued == null ? null : issued.copy(); 2111 if (performer != null) { 2112 dst.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 2113 for (DiagnosticReportPerformerComponent i : performer) 2114 dst.performer.add(i.copy()); 2115 }; 2116 if (specimen != null) { 2117 dst.specimen = new ArrayList<Reference>(); 2118 for (Reference i : specimen) 2119 dst.specimen.add(i.copy()); 2120 }; 2121 if (result != null) { 2122 dst.result = new ArrayList<Reference>(); 2123 for (Reference i : result) 2124 dst.result.add(i.copy()); 2125 }; 2126 if (imagingStudy != null) { 2127 dst.imagingStudy = new ArrayList<Reference>(); 2128 for (Reference i : imagingStudy) 2129 dst.imagingStudy.add(i.copy()); 2130 }; 2131 if (image != null) { 2132 dst.image = new ArrayList<DiagnosticReportImageComponent>(); 2133 for (DiagnosticReportImageComponent i : image) 2134 dst.image.add(i.copy()); 2135 }; 2136 dst.conclusion = conclusion == null ? null : conclusion.copy(); 2137 if (codedDiagnosis != null) { 2138 dst.codedDiagnosis = new ArrayList<CodeableConcept>(); 2139 for (CodeableConcept i : codedDiagnosis) 2140 dst.codedDiagnosis.add(i.copy()); 2141 }; 2142 if (presentedForm != null) { 2143 dst.presentedForm = new ArrayList<Attachment>(); 2144 for (Attachment i : presentedForm) 2145 dst.presentedForm.add(i.copy()); 2146 }; 2147 return dst; 2148 } 2149 2150 protected DiagnosticReport typedCopy() { 2151 return copy(); 2152 } 2153 2154 @Override 2155 public boolean equalsDeep(Base other_) { 2156 if (!super.equalsDeep(other_)) 2157 return false; 2158 if (!(other_ instanceof DiagnosticReport)) 2159 return false; 2160 DiagnosticReport o = (DiagnosticReport) other_; 2161 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(status, o.status, true) 2162 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 2163 && compareDeep(context, o.context, true) && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) 2164 && compareDeep(performer, o.performer, true) && compareDeep(specimen, o.specimen, true) && compareDeep(result, o.result, true) 2165 && compareDeep(imagingStudy, o.imagingStudy, true) && compareDeep(image, o.image, true) && compareDeep(conclusion, o.conclusion, true) 2166 && compareDeep(codedDiagnosis, o.codedDiagnosis, true) && compareDeep(presentedForm, o.presentedForm, true) 2167 ; 2168 } 2169 2170 @Override 2171 public boolean equalsShallow(Base other_) { 2172 if (!super.equalsShallow(other_)) 2173 return false; 2174 if (!(other_ instanceof DiagnosticReport)) 2175 return false; 2176 DiagnosticReport o = (DiagnosticReport) other_; 2177 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) && compareValues(conclusion, o.conclusion, true) 2178 ; 2179 } 2180 2181 public boolean isEmpty() { 2182 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status 2183 , category, code, subject, context, effective, issued, performer, specimen, result 2184 , imagingStudy, image, conclusion, codedDiagnosis, presentedForm); 2185 } 2186 2187 @Override 2188 public ResourceType getResourceType() { 2189 return ResourceType.DiagnosticReport; 2190 } 2191 2192 /** 2193 * Search parameter: <b>date</b> 2194 * <p> 2195 * Description: <b>The clinically relevant time of the report</b><br> 2196 * Type: <b>date</b><br> 2197 * Path: <b>DiagnosticReport.effective[x]</b><br> 2198 * </p> 2199 */ 2200 @SearchParamDefinition(name="date", path="DiagnosticReport.effective", description="The clinically relevant time of the report", type="date" ) 2201 public static final String SP_DATE = "date"; 2202 /** 2203 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2204 * <p> 2205 * Description: <b>The clinically relevant time of the report</b><br> 2206 * Type: <b>date</b><br> 2207 * Path: <b>DiagnosticReport.effective[x]</b><br> 2208 * </p> 2209 */ 2210 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2211 2212 /** 2213 * Search parameter: <b>identifier</b> 2214 * <p> 2215 * Description: <b>An identifier for the report</b><br> 2216 * Type: <b>token</b><br> 2217 * Path: <b>DiagnosticReport.identifier</b><br> 2218 * </p> 2219 */ 2220 @SearchParamDefinition(name="identifier", path="DiagnosticReport.identifier", description="An identifier for the report", type="token" ) 2221 public static final String SP_IDENTIFIER = "identifier"; 2222 /** 2223 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2224 * <p> 2225 * Description: <b>An identifier for the report</b><br> 2226 * Type: <b>token</b><br> 2227 * Path: <b>DiagnosticReport.identifier</b><br> 2228 * </p> 2229 */ 2230 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2231 2232 /** 2233 * Search parameter: <b>image</b> 2234 * <p> 2235 * Description: <b>A reference to the image source.</b><br> 2236 * Type: <b>reference</b><br> 2237 * Path: <b>DiagnosticReport.image.link</b><br> 2238 * </p> 2239 */ 2240 @SearchParamDefinition(name="image", path="DiagnosticReport.image.link", description="A reference to the image source.", type="reference", target={Media.class } ) 2241 public static final String SP_IMAGE = "image"; 2242 /** 2243 * <b>Fluent Client</b> search parameter constant for <b>image</b> 2244 * <p> 2245 * Description: <b>A reference to the image source.</b><br> 2246 * Type: <b>reference</b><br> 2247 * Path: <b>DiagnosticReport.image.link</b><br> 2248 * </p> 2249 */ 2250 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_IMAGE); 2251 2252/** 2253 * Constant for fluent queries to be used to add include statements. Specifies 2254 * the path value of "<b>DiagnosticReport:image</b>". 2255 */ 2256 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMAGE = new ca.uhn.fhir.model.api.Include("DiagnosticReport:image").toLocked(); 2257 2258 /** 2259 * Search parameter: <b>performer</b> 2260 * <p> 2261 * Description: <b>Who was the source of the report (organization)</b><br> 2262 * Type: <b>reference</b><br> 2263 * Path: <b>DiagnosticReport.performer.actor</b><br> 2264 * </p> 2265 */ 2266 @SearchParamDefinition(name="performer", path="DiagnosticReport.performer.actor", description="Who was the source of the report (organization)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 2267 public static final String SP_PERFORMER = "performer"; 2268 /** 2269 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2270 * <p> 2271 * Description: <b>Who was the source of the report (organization)</b><br> 2272 * Type: <b>reference</b><br> 2273 * Path: <b>DiagnosticReport.performer.actor</b><br> 2274 * </p> 2275 */ 2276 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2277 2278/** 2279 * Constant for fluent queries to be used to add include statements. Specifies 2280 * the path value of "<b>DiagnosticReport:performer</b>". 2281 */ 2282 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("DiagnosticReport:performer").toLocked(); 2283 2284 /** 2285 * Search parameter: <b>code</b> 2286 * <p> 2287 * Description: <b>The code for the report as a whole, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result</b><br> 2288 * Type: <b>token</b><br> 2289 * Path: <b>DiagnosticReport.code</b><br> 2290 * </p> 2291 */ 2292 @SearchParamDefinition(name="code", path="DiagnosticReport.code", description="The code for the report as a whole, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result", type="token" ) 2293 public static final String SP_CODE = "code"; 2294 /** 2295 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2296 * <p> 2297 * Description: <b>The code for the report as a whole, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result</b><br> 2298 * Type: <b>token</b><br> 2299 * Path: <b>DiagnosticReport.code</b><br> 2300 * </p> 2301 */ 2302 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2303 2304 /** 2305 * Search parameter: <b>subject</b> 2306 * <p> 2307 * Description: <b>The subject of the report</b><br> 2308 * Type: <b>reference</b><br> 2309 * Path: <b>DiagnosticReport.subject</b><br> 2310 * </p> 2311 */ 2312 @SearchParamDefinition(name="subject", path="DiagnosticReport.subject", description="The subject of the report", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 2313 public static final String SP_SUBJECT = "subject"; 2314 /** 2315 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2316 * <p> 2317 * Description: <b>The subject of the report</b><br> 2318 * Type: <b>reference</b><br> 2319 * Path: <b>DiagnosticReport.subject</b><br> 2320 * </p> 2321 */ 2322 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2323 2324/** 2325 * Constant for fluent queries to be used to add include statements. Specifies 2326 * the path value of "<b>DiagnosticReport:subject</b>". 2327 */ 2328 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:subject").toLocked(); 2329 2330 /** 2331 * Search parameter: <b>diagnosis</b> 2332 * <p> 2333 * Description: <b>A coded diagnosis on the report</b><br> 2334 * Type: <b>token</b><br> 2335 * Path: <b>DiagnosticReport.codedDiagnosis</b><br> 2336 * </p> 2337 */ 2338 @SearchParamDefinition(name="diagnosis", path="DiagnosticReport.codedDiagnosis", description="A coded diagnosis on the report", type="token" ) 2339 public static final String SP_DIAGNOSIS = "diagnosis"; 2340 /** 2341 * <b>Fluent Client</b> search parameter constant for <b>diagnosis</b> 2342 * <p> 2343 * Description: <b>A coded diagnosis on the report</b><br> 2344 * Type: <b>token</b><br> 2345 * Path: <b>DiagnosticReport.codedDiagnosis</b><br> 2346 * </p> 2347 */ 2348 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DIAGNOSIS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DIAGNOSIS); 2349 2350 /** 2351 * Search parameter: <b>encounter</b> 2352 * <p> 2353 * Description: <b>The Encounter when the order was made</b><br> 2354 * Type: <b>reference</b><br> 2355 * Path: <b>DiagnosticReport.context</b><br> 2356 * </p> 2357 */ 2358 @SearchParamDefinition(name="encounter", path="DiagnosticReport.context", description="The Encounter when the order was made", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 2359 public static final String SP_ENCOUNTER = "encounter"; 2360 /** 2361 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2362 * <p> 2363 * Description: <b>The Encounter when the order was made</b><br> 2364 * Type: <b>reference</b><br> 2365 * Path: <b>DiagnosticReport.context</b><br> 2366 * </p> 2367 */ 2368 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2369 2370/** 2371 * Constant for fluent queries to be used to add include statements. Specifies 2372 * the path value of "<b>DiagnosticReport:encounter</b>". 2373 */ 2374 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("DiagnosticReport:encounter").toLocked(); 2375 2376 /** 2377 * Search parameter: <b>result</b> 2378 * <p> 2379 * Description: <b>Link to an atomic result (observation resource)</b><br> 2380 * Type: <b>reference</b><br> 2381 * Path: <b>DiagnosticReport.result</b><br> 2382 * </p> 2383 */ 2384 @SearchParamDefinition(name="result", path="DiagnosticReport.result", description="Link to an atomic result (observation resource)", type="reference", target={Observation.class } ) 2385 public static final String SP_RESULT = "result"; 2386 /** 2387 * <b>Fluent Client</b> search parameter constant for <b>result</b> 2388 * <p> 2389 * Description: <b>Link to an atomic result (observation resource)</b><br> 2390 * Type: <b>reference</b><br> 2391 * Path: <b>DiagnosticReport.result</b><br> 2392 * </p> 2393 */ 2394 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESULT); 2395 2396/** 2397 * Constant for fluent queries to be used to add include statements. Specifies 2398 * the path value of "<b>DiagnosticReport:result</b>". 2399 */ 2400 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:result").toLocked(); 2401 2402 /** 2403 * Search parameter: <b>based-on</b> 2404 * <p> 2405 * Description: <b>Reference to the procedure request.</b><br> 2406 * Type: <b>reference</b><br> 2407 * Path: <b>DiagnosticReport.basedOn</b><br> 2408 * </p> 2409 */ 2410 @SearchParamDefinition(name="based-on", path="DiagnosticReport.basedOn", description="Reference to the procedure request.", type="reference", target={CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ProcedureRequest.class, ReferralRequest.class } ) 2411 public static final String SP_BASED_ON = "based-on"; 2412 /** 2413 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2414 * <p> 2415 * Description: <b>Reference to the procedure request.</b><br> 2416 * Type: <b>reference</b><br> 2417 * Path: <b>DiagnosticReport.basedOn</b><br> 2418 * </p> 2419 */ 2420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2421 2422/** 2423 * Constant for fluent queries to be used to add include statements. Specifies 2424 * the path value of "<b>DiagnosticReport:based-on</b>". 2425 */ 2426 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("DiagnosticReport:based-on").toLocked(); 2427 2428 /** 2429 * Search parameter: <b>patient</b> 2430 * <p> 2431 * Description: <b>The subject of the report if a patient</b><br> 2432 * Type: <b>reference</b><br> 2433 * Path: <b>DiagnosticReport.subject</b><br> 2434 * </p> 2435 */ 2436 @SearchParamDefinition(name="patient", path="DiagnosticReport.subject", description="The subject of the report if a patient", type="reference", target={Patient.class } ) 2437 public static final String SP_PATIENT = "patient"; 2438 /** 2439 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2440 * <p> 2441 * Description: <b>The subject of the report if a patient</b><br> 2442 * Type: <b>reference</b><br> 2443 * Path: <b>DiagnosticReport.subject</b><br> 2444 * </p> 2445 */ 2446 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2447 2448/** 2449 * Constant for fluent queries to be used to add include statements. Specifies 2450 * the path value of "<b>DiagnosticReport:patient</b>". 2451 */ 2452 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:patient").toLocked(); 2453 2454 /** 2455 * Search parameter: <b>specimen</b> 2456 * <p> 2457 * Description: <b>The specimen details</b><br> 2458 * Type: <b>reference</b><br> 2459 * Path: <b>DiagnosticReport.specimen</b><br> 2460 * </p> 2461 */ 2462 @SearchParamDefinition(name="specimen", path="DiagnosticReport.specimen", description="The specimen details", type="reference", target={Specimen.class } ) 2463 public static final String SP_SPECIMEN = "specimen"; 2464 /** 2465 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 2466 * <p> 2467 * Description: <b>The specimen details</b><br> 2468 * Type: <b>reference</b><br> 2469 * Path: <b>DiagnosticReport.specimen</b><br> 2470 * </p> 2471 */ 2472 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 2473 2474/** 2475 * Constant for fluent queries to be used to add include statements. Specifies 2476 * the path value of "<b>DiagnosticReport:specimen</b>". 2477 */ 2478 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("DiagnosticReport:specimen").toLocked(); 2479 2480 /** 2481 * Search parameter: <b>context</b> 2482 * <p> 2483 * Description: <b>Healthcare event (Episode of Care or Encounter) related to the report</b><br> 2484 * Type: <b>reference</b><br> 2485 * Path: <b>DiagnosticReport.context</b><br> 2486 * </p> 2487 */ 2488 @SearchParamDefinition(name="context", path="DiagnosticReport.context", description="Healthcare event (Episode of Care or Encounter) related to the report", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 2489 public static final String SP_CONTEXT = "context"; 2490 /** 2491 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2492 * <p> 2493 * Description: <b>Healthcare event (Episode of Care or Encounter) related to the report</b><br> 2494 * Type: <b>reference</b><br> 2495 * Path: <b>DiagnosticReport.context</b><br> 2496 * </p> 2497 */ 2498 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2499 2500/** 2501 * Constant for fluent queries to be used to add include statements. Specifies 2502 * the path value of "<b>DiagnosticReport:context</b>". 2503 */ 2504 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:context").toLocked(); 2505 2506 /** 2507 * Search parameter: <b>issued</b> 2508 * <p> 2509 * Description: <b>When the report was issued</b><br> 2510 * Type: <b>date</b><br> 2511 * Path: <b>DiagnosticReport.issued</b><br> 2512 * </p> 2513 */ 2514 @SearchParamDefinition(name="issued", path="DiagnosticReport.issued", description="When the report was issued", type="date" ) 2515 public static final String SP_ISSUED = "issued"; 2516 /** 2517 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 2518 * <p> 2519 * Description: <b>When the report was issued</b><br> 2520 * Type: <b>date</b><br> 2521 * Path: <b>DiagnosticReport.issued</b><br> 2522 * </p> 2523 */ 2524 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 2525 2526 /** 2527 * Search parameter: <b>category</b> 2528 * <p> 2529 * Description: <b>Which diagnostic discipline/department created the report</b><br> 2530 * Type: <b>token</b><br> 2531 * Path: <b>DiagnosticReport.category</b><br> 2532 * </p> 2533 */ 2534 @SearchParamDefinition(name="category", path="DiagnosticReport.category", description="Which diagnostic discipline/department created the report", type="token" ) 2535 public static final String SP_CATEGORY = "category"; 2536 /** 2537 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2538 * <p> 2539 * Description: <b>Which diagnostic discipline/department created the report</b><br> 2540 * Type: <b>token</b><br> 2541 * Path: <b>DiagnosticReport.category</b><br> 2542 * </p> 2543 */ 2544 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2545 2546 /** 2547 * Search parameter: <b>status</b> 2548 * <p> 2549 * Description: <b>The status of the report</b><br> 2550 * Type: <b>token</b><br> 2551 * Path: <b>DiagnosticReport.status</b><br> 2552 * </p> 2553 */ 2554 @SearchParamDefinition(name="status", path="DiagnosticReport.status", description="The status of the report", type="token" ) 2555 public static final String SP_STATUS = "status"; 2556 /** 2557 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2558 * <p> 2559 * Description: <b>The status of the report</b><br> 2560 * Type: <b>token</b><br> 2561 * Path: <b>DiagnosticReport.status</b><br> 2562 * </p> 2563 */ 2564 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2565 2566 2567}