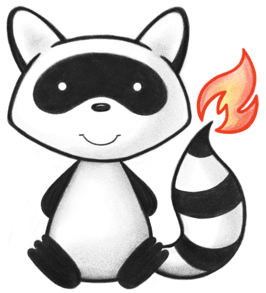
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 051 */ 052@ResourceDef(name="DiagnosticReport", profile="http://hl7.org/fhir/Profile/DiagnosticReport") 053public class DiagnosticReport extends DomainResource { 054 055 public enum DiagnosticReportStatus { 056 /** 057 * The existence of the report is registered, but there is nothing yet available. 058 */ 059 REGISTERED, 060 /** 061 * This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified. 062 */ 063 PARTIAL, 064 /** 065 * Verified early results are available, but not all results are final. 066 */ 067 PRELIMINARY, 068 /** 069 * The report is complete and verified by an authorized person. 070 */ 071 FINAL, 072 /** 073 * Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued. 074 */ 075 AMENDED, 076 /** 077 * Subsequent to being final, the report has been modified to correct an error in the report or referenced results. 078 */ 079 CORRECTED, 080 /** 081 * Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged. 082 */ 083 APPENDED, 084 /** 085 * The report is unavailable because the measurement was not started or not completed (also sometimes called "aborted"). 086 */ 087 CANCELLED, 088 /** 089 * The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 090 */ 091 ENTEREDINERROR, 092 /** 093 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 094 */ 095 UNKNOWN, 096 /** 097 * added to help the parsers with the generic types 098 */ 099 NULL; 100 public static DiagnosticReportStatus fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("registered".equals(codeString)) 104 return REGISTERED; 105 if ("partial".equals(codeString)) 106 return PARTIAL; 107 if ("preliminary".equals(codeString)) 108 return PRELIMINARY; 109 if ("final".equals(codeString)) 110 return FINAL; 111 if ("amended".equals(codeString)) 112 return AMENDED; 113 if ("corrected".equals(codeString)) 114 return CORRECTED; 115 if ("appended".equals(codeString)) 116 return APPENDED; 117 if ("cancelled".equals(codeString)) 118 return CANCELLED; 119 if ("entered-in-error".equals(codeString)) 120 return ENTEREDINERROR; 121 if ("unknown".equals(codeString)) 122 return UNKNOWN; 123 if (Configuration.isAcceptInvalidEnums()) 124 return null; 125 else 126 throw new FHIRException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 127 } 128 public String toCode() { 129 switch (this) { 130 case REGISTERED: return "registered"; 131 case PARTIAL: return "partial"; 132 case PRELIMINARY: return "preliminary"; 133 case FINAL: return "final"; 134 case AMENDED: return "amended"; 135 case CORRECTED: return "corrected"; 136 case APPENDED: return "appended"; 137 case CANCELLED: return "cancelled"; 138 case ENTEREDINERROR: return "entered-in-error"; 139 case UNKNOWN: return "unknown"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 public String getSystem() { 145 switch (this) { 146 case REGISTERED: return "http://hl7.org/fhir/diagnostic-report-status"; 147 case PARTIAL: return "http://hl7.org/fhir/diagnostic-report-status"; 148 case PRELIMINARY: return "http://hl7.org/fhir/diagnostic-report-status"; 149 case FINAL: return "http://hl7.org/fhir/diagnostic-report-status"; 150 case AMENDED: return "http://hl7.org/fhir/diagnostic-report-status"; 151 case CORRECTED: return "http://hl7.org/fhir/diagnostic-report-status"; 152 case APPENDED: return "http://hl7.org/fhir/diagnostic-report-status"; 153 case CANCELLED: return "http://hl7.org/fhir/diagnostic-report-status"; 154 case ENTEREDINERROR: return "http://hl7.org/fhir/diagnostic-report-status"; 155 case UNKNOWN: return "http://hl7.org/fhir/diagnostic-report-status"; 156 case NULL: return null; 157 default: return "?"; 158 } 159 } 160 public String getDefinition() { 161 switch (this) { 162 case REGISTERED: return "The existence of the report is registered, but there is nothing yet available."; 163 case PARTIAL: return "This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified."; 164 case PRELIMINARY: return "Verified early results are available, but not all results are final."; 165 case FINAL: return "The report is complete and verified by an authorized person."; 166 case AMENDED: return "Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued."; 167 case CORRECTED: return "Subsequent to being final, the report has been modified to correct an error in the report or referenced results."; 168 case APPENDED: return "Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged."; 169 case CANCELLED: return "The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 170 case ENTEREDINERROR: return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 171 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 172 case NULL: return null; 173 default: return "?"; 174 } 175 } 176 public String getDisplay() { 177 switch (this) { 178 case REGISTERED: return "Registered"; 179 case PARTIAL: return "Partial"; 180 case PRELIMINARY: return "Preliminary"; 181 case FINAL: return "Final"; 182 case AMENDED: return "Amended"; 183 case CORRECTED: return "Corrected"; 184 case APPENDED: return "Appended"; 185 case CANCELLED: return "Cancelled"; 186 case ENTEREDINERROR: return "Entered in Error"; 187 case UNKNOWN: return "Unknown"; 188 case NULL: return null; 189 default: return "?"; 190 } 191 } 192 } 193 194 public static class DiagnosticReportStatusEnumFactory implements EnumFactory<DiagnosticReportStatus> { 195 public DiagnosticReportStatus fromCode(String codeString) throws IllegalArgumentException { 196 if (codeString == null || "".equals(codeString)) 197 if (codeString == null || "".equals(codeString)) 198 return null; 199 if ("registered".equals(codeString)) 200 return DiagnosticReportStatus.REGISTERED; 201 if ("partial".equals(codeString)) 202 return DiagnosticReportStatus.PARTIAL; 203 if ("preliminary".equals(codeString)) 204 return DiagnosticReportStatus.PRELIMINARY; 205 if ("final".equals(codeString)) 206 return DiagnosticReportStatus.FINAL; 207 if ("amended".equals(codeString)) 208 return DiagnosticReportStatus.AMENDED; 209 if ("corrected".equals(codeString)) 210 return DiagnosticReportStatus.CORRECTED; 211 if ("appended".equals(codeString)) 212 return DiagnosticReportStatus.APPENDED; 213 if ("cancelled".equals(codeString)) 214 return DiagnosticReportStatus.CANCELLED; 215 if ("entered-in-error".equals(codeString)) 216 return DiagnosticReportStatus.ENTEREDINERROR; 217 if ("unknown".equals(codeString)) 218 return DiagnosticReportStatus.UNKNOWN; 219 throw new IllegalArgumentException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 220 } 221 public Enumeration<DiagnosticReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 222 if (code == null) 223 return null; 224 if (code.isEmpty()) 225 return new Enumeration<DiagnosticReportStatus>(this); 226 String codeString = code.asStringValue(); 227 if (codeString == null || "".equals(codeString)) 228 return null; 229 if ("registered".equals(codeString)) 230 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.REGISTERED); 231 if ("partial".equals(codeString)) 232 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PARTIAL); 233 if ("preliminary".equals(codeString)) 234 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PRELIMINARY); 235 if ("final".equals(codeString)) 236 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.FINAL); 237 if ("amended".equals(codeString)) 238 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.AMENDED); 239 if ("corrected".equals(codeString)) 240 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CORRECTED); 241 if ("appended".equals(codeString)) 242 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.APPENDED); 243 if ("cancelled".equals(codeString)) 244 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CANCELLED); 245 if ("entered-in-error".equals(codeString)) 246 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.ENTEREDINERROR); 247 if ("unknown".equals(codeString)) 248 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.UNKNOWN); 249 throw new FHIRException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 250 } 251 public String toCode(DiagnosticReportStatus code) { 252 if (code == DiagnosticReportStatus.REGISTERED) 253 return "registered"; 254 if (code == DiagnosticReportStatus.PARTIAL) 255 return "partial"; 256 if (code == DiagnosticReportStatus.PRELIMINARY) 257 return "preliminary"; 258 if (code == DiagnosticReportStatus.FINAL) 259 return "final"; 260 if (code == DiagnosticReportStatus.AMENDED) 261 return "amended"; 262 if (code == DiagnosticReportStatus.CORRECTED) 263 return "corrected"; 264 if (code == DiagnosticReportStatus.APPENDED) 265 return "appended"; 266 if (code == DiagnosticReportStatus.CANCELLED) 267 return "cancelled"; 268 if (code == DiagnosticReportStatus.ENTEREDINERROR) 269 return "entered-in-error"; 270 if (code == DiagnosticReportStatus.UNKNOWN) 271 return "unknown"; 272 return "?"; 273 } 274 public String toSystem(DiagnosticReportStatus code) { 275 return code.getSystem(); 276 } 277 } 278 279 @Block() 280 public static class DiagnosticReportPerformerComponent extends BackboneElement implements IBaseBackboneElement { 281 /** 282 * Describes the type of participation (e.g. a responsible party, author, or verifier). 283 */ 284 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 285 @Description(shortDefinition="Type of performer", formalDefinition="Describes the type of participation (e.g. a responsible party, author, or verifier)." ) 286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 287 protected CodeableConcept role; 288 289 /** 290 * The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report. 291 */ 292 @Child(name = "actor", type = {Practitioner.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=true) 293 @Description(shortDefinition="Practitioner or Organization participant", formalDefinition="The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report." ) 294 protected Reference actor; 295 296 /** 297 * The actual object that is the target of the reference (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 298 */ 299 protected Resource actorTarget; 300 301 private static final long serialVersionUID = 805521719L; 302 303 /** 304 * Constructor 305 */ 306 public DiagnosticReportPerformerComponent() { 307 super(); 308 } 309 310 /** 311 * Constructor 312 */ 313 public DiagnosticReportPerformerComponent(Reference actor) { 314 super(); 315 this.actor = actor; 316 } 317 318 /** 319 * @return {@link #role} (Describes the type of participation (e.g. a responsible party, author, or verifier).) 320 */ 321 public CodeableConcept getRole() { 322 if (this.role == null) 323 if (Configuration.errorOnAutoCreate()) 324 throw new Error("Attempt to auto-create DiagnosticReportPerformerComponent.role"); 325 else if (Configuration.doAutoCreate()) 326 this.role = new CodeableConcept(); // cc 327 return this.role; 328 } 329 330 public boolean hasRole() { 331 return this.role != null && !this.role.isEmpty(); 332 } 333 334 /** 335 * @param value {@link #role} (Describes the type of participation (e.g. a responsible party, author, or verifier).) 336 */ 337 public DiagnosticReportPerformerComponent setRole(CodeableConcept value) { 338 this.role = value; 339 return this; 340 } 341 342 /** 343 * @return {@link #actor} (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 344 */ 345 public Reference getActor() { 346 if (this.actor == null) 347 if (Configuration.errorOnAutoCreate()) 348 throw new Error("Attempt to auto-create DiagnosticReportPerformerComponent.actor"); 349 else if (Configuration.doAutoCreate()) 350 this.actor = new Reference(); // cc 351 return this.actor; 352 } 353 354 public boolean hasActor() { 355 return this.actor != null && !this.actor.isEmpty(); 356 } 357 358 /** 359 * @param value {@link #actor} (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 360 */ 361 public DiagnosticReportPerformerComponent setActor(Reference value) { 362 this.actor = value; 363 return this; 364 } 365 366 /** 367 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 368 */ 369 public Resource getActorTarget() { 370 return this.actorTarget; 371 } 372 373 /** 374 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.) 375 */ 376 public DiagnosticReportPerformerComponent setActorTarget(Resource value) { 377 this.actorTarget = value; 378 return this; 379 } 380 381 protected void listChildren(List<Property> children) { 382 super.listChildren(children); 383 children.add(new Property("role", "CodeableConcept", "Describes the type of participation (e.g. a responsible party, author, or verifier).", 0, 1, role)); 384 children.add(new Property("actor", "Reference(Practitioner|Organization)", "The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.", 0, 1, actor)); 385 } 386 387 @Override 388 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 389 switch (_hash) { 390 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Describes the type of participation (e.g. a responsible party, author, or verifier).", 0, 1, role); 391 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Organization)", "The reference to the practitioner or organization involved in producing the report. For example, the diagnostic service that is responsible for issuing the report.", 0, 1, actor); 392 default: return super.getNamedProperty(_hash, _name, _checkValid); 393 } 394 395 } 396 397 @Override 398 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 399 switch (hash) { 400 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 401 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 402 default: return super.getProperty(hash, name, checkValid); 403 } 404 405 } 406 407 @Override 408 public Base setProperty(int hash, String name, Base value) throws FHIRException { 409 switch (hash) { 410 case 3506294: // role 411 this.role = castToCodeableConcept(value); // CodeableConcept 412 return value; 413 case 92645877: // actor 414 this.actor = castToReference(value); // Reference 415 return value; 416 default: return super.setProperty(hash, name, value); 417 } 418 419 } 420 421 @Override 422 public Base setProperty(String name, Base value) throws FHIRException { 423 if (name.equals("role")) { 424 this.role = castToCodeableConcept(value); // CodeableConcept 425 } else if (name.equals("actor")) { 426 this.actor = castToReference(value); // Reference 427 } else 428 return super.setProperty(name, value); 429 return value; 430 } 431 432 @Override 433 public Base makeProperty(int hash, String name) throws FHIRException { 434 switch (hash) { 435 case 3506294: return getRole(); 436 case 92645877: return getActor(); 437 default: return super.makeProperty(hash, name); 438 } 439 440 } 441 442 @Override 443 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 444 switch (hash) { 445 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 446 case 92645877: /*actor*/ return new String[] {"Reference"}; 447 default: return super.getTypesForProperty(hash, name); 448 } 449 450 } 451 452 @Override 453 public Base addChild(String name) throws FHIRException { 454 if (name.equals("role")) { 455 this.role = new CodeableConcept(); 456 return this.role; 457 } 458 else if (name.equals("actor")) { 459 this.actor = new Reference(); 460 return this.actor; 461 } 462 else 463 return super.addChild(name); 464 } 465 466 public DiagnosticReportPerformerComponent copy() { 467 DiagnosticReportPerformerComponent dst = new DiagnosticReportPerformerComponent(); 468 copyValues(dst); 469 dst.role = role == null ? null : role.copy(); 470 dst.actor = actor == null ? null : actor.copy(); 471 return dst; 472 } 473 474 @Override 475 public boolean equalsDeep(Base other_) { 476 if (!super.equalsDeep(other_)) 477 return false; 478 if (!(other_ instanceof DiagnosticReportPerformerComponent)) 479 return false; 480 DiagnosticReportPerformerComponent o = (DiagnosticReportPerformerComponent) other_; 481 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 482 } 483 484 @Override 485 public boolean equalsShallow(Base other_) { 486 if (!super.equalsShallow(other_)) 487 return false; 488 if (!(other_ instanceof DiagnosticReportPerformerComponent)) 489 return false; 490 DiagnosticReportPerformerComponent o = (DiagnosticReportPerformerComponent) other_; 491 return true; 492 } 493 494 public boolean isEmpty() { 495 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 496 } 497 498 public String fhirType() { 499 return "DiagnosticReport.performer"; 500 501 } 502 503 } 504 505 @Block() 506 public static class DiagnosticReportImageComponent extends BackboneElement implements IBaseBackboneElement { 507 /** 508 * A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features. 509 */ 510 @Child(name = "comment", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 511 @Description(shortDefinition="Comment about the image (e.g. explanation)", formalDefinition="A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features." ) 512 protected StringType comment; 513 514 /** 515 * Reference to the image source. 516 */ 517 @Child(name = "link", type = {Media.class}, order=2, min=1, max=1, modifier=false, summary=true) 518 @Description(shortDefinition="Reference to the image source", formalDefinition="Reference to the image source." ) 519 protected Reference link; 520 521 /** 522 * The actual object that is the target of the reference (Reference to the image source.) 523 */ 524 protected Media linkTarget; 525 526 private static final long serialVersionUID = 935791940L; 527 528 /** 529 * Constructor 530 */ 531 public DiagnosticReportImageComponent() { 532 super(); 533 } 534 535 /** 536 * Constructor 537 */ 538 public DiagnosticReportImageComponent(Reference link) { 539 super(); 540 this.link = link; 541 } 542 543 /** 544 * @return {@link #comment} (A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 545 */ 546 public StringType getCommentElement() { 547 if (this.comment == null) 548 if (Configuration.errorOnAutoCreate()) 549 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.comment"); 550 else if (Configuration.doAutoCreate()) 551 this.comment = new StringType(); // bb 552 return this.comment; 553 } 554 555 public boolean hasCommentElement() { 556 return this.comment != null && !this.comment.isEmpty(); 557 } 558 559 public boolean hasComment() { 560 return this.comment != null && !this.comment.isEmpty(); 561 } 562 563 /** 564 * @param value {@link #comment} (A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 565 */ 566 public DiagnosticReportImageComponent setCommentElement(StringType value) { 567 this.comment = value; 568 return this; 569 } 570 571 /** 572 * @return A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features. 573 */ 574 public String getComment() { 575 return this.comment == null ? null : this.comment.getValue(); 576 } 577 578 /** 579 * @param value A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features. 580 */ 581 public DiagnosticReportImageComponent setComment(String value) { 582 if (Utilities.noString(value)) 583 this.comment = null; 584 else { 585 if (this.comment == null) 586 this.comment = new StringType(); 587 this.comment.setValue(value); 588 } 589 return this; 590 } 591 592 /** 593 * @return {@link #link} (Reference to the image source.) 594 */ 595 public Reference getLink() { 596 if (this.link == null) 597 if (Configuration.errorOnAutoCreate()) 598 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.link"); 599 else if (Configuration.doAutoCreate()) 600 this.link = new Reference(); // cc 601 return this.link; 602 } 603 604 public boolean hasLink() { 605 return this.link != null && !this.link.isEmpty(); 606 } 607 608 /** 609 * @param value {@link #link} (Reference to the image source.) 610 */ 611 public DiagnosticReportImageComponent setLink(Reference value) { 612 this.link = value; 613 return this; 614 } 615 616 /** 617 * @return {@link #link} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the image source.) 618 */ 619 public Media getLinkTarget() { 620 if (this.linkTarget == null) 621 if (Configuration.errorOnAutoCreate()) 622 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.link"); 623 else if (Configuration.doAutoCreate()) 624 this.linkTarget = new Media(); // aa 625 return this.linkTarget; 626 } 627 628 /** 629 * @param value {@link #link} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the image source.) 630 */ 631 public DiagnosticReportImageComponent setLinkTarget(Media value) { 632 this.linkTarget = value; 633 return this; 634 } 635 636 protected void listChildren(List<Property> children) { 637 super.listChildren(children); 638 children.add(new Property("comment", "string", "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 0, 1, comment)); 639 children.add(new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link)); 640 } 641 642 @Override 643 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 644 switch (_hash) { 645 case 950398559: /*comment*/ return new Property("comment", "string", "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 0, 1, comment); 646 case 3321850: /*link*/ return new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link); 647 default: return super.getNamedProperty(_hash, _name, _checkValid); 648 } 649 650 } 651 652 @Override 653 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 654 switch (hash) { 655 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 656 case 3321850: /*link*/ return this.link == null ? new Base[0] : new Base[] {this.link}; // Reference 657 default: return super.getProperty(hash, name, checkValid); 658 } 659 660 } 661 662 @Override 663 public Base setProperty(int hash, String name, Base value) throws FHIRException { 664 switch (hash) { 665 case 950398559: // comment 666 this.comment = castToString(value); // StringType 667 return value; 668 case 3321850: // link 669 this.link = castToReference(value); // Reference 670 return value; 671 default: return super.setProperty(hash, name, value); 672 } 673 674 } 675 676 @Override 677 public Base setProperty(String name, Base value) throws FHIRException { 678 if (name.equals("comment")) { 679 this.comment = castToString(value); // StringType 680 } else if (name.equals("link")) { 681 this.link = castToReference(value); // Reference 682 } else 683 return super.setProperty(name, value); 684 return value; 685 } 686 687 @Override 688 public Base makeProperty(int hash, String name) throws FHIRException { 689 switch (hash) { 690 case 950398559: return getCommentElement(); 691 case 3321850: return getLink(); 692 default: return super.makeProperty(hash, name); 693 } 694 695 } 696 697 @Override 698 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 699 switch (hash) { 700 case 950398559: /*comment*/ return new String[] {"string"}; 701 case 3321850: /*link*/ return new String[] {"Reference"}; 702 default: return super.getTypesForProperty(hash, name); 703 } 704 705 } 706 707 @Override 708 public Base addChild(String name) throws FHIRException { 709 if (name.equals("comment")) { 710 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.comment"); 711 } 712 else if (name.equals("link")) { 713 this.link = new Reference(); 714 return this.link; 715 } 716 else 717 return super.addChild(name); 718 } 719 720 public DiagnosticReportImageComponent copy() { 721 DiagnosticReportImageComponent dst = new DiagnosticReportImageComponent(); 722 copyValues(dst); 723 dst.comment = comment == null ? null : comment.copy(); 724 dst.link = link == null ? null : link.copy(); 725 return dst; 726 } 727 728 @Override 729 public boolean equalsDeep(Base other_) { 730 if (!super.equalsDeep(other_)) 731 return false; 732 if (!(other_ instanceof DiagnosticReportImageComponent)) 733 return false; 734 DiagnosticReportImageComponent o = (DiagnosticReportImageComponent) other_; 735 return compareDeep(comment, o.comment, true) && compareDeep(link, o.link, true); 736 } 737 738 @Override 739 public boolean equalsShallow(Base other_) { 740 if (!super.equalsShallow(other_)) 741 return false; 742 if (!(other_ instanceof DiagnosticReportImageComponent)) 743 return false; 744 DiagnosticReportImageComponent o = (DiagnosticReportImageComponent) other_; 745 return compareValues(comment, o.comment, true); 746 } 747 748 public boolean isEmpty() { 749 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, link); 750 } 751 752 public String fhirType() { 753 return "DiagnosticReport.image"; 754 755 } 756 757 } 758 759 /** 760 * Identifiers assigned to this report by the performer or other systems. 761 */ 762 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 763 @Description(shortDefinition="Business identifier for report", formalDefinition="Identifiers assigned to this report by the performer or other systems." ) 764 protected List<Identifier> identifier; 765 766 /** 767 * Details concerning a test or procedure requested. 768 */ 769 @Child(name = "basedOn", type = {CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ProcedureRequest.class, ReferralRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 770 @Description(shortDefinition="What was requested", formalDefinition="Details concerning a test or procedure requested." ) 771 protected List<Reference> basedOn; 772 /** 773 * The actual objects that are the target of the reference (Details concerning a test or procedure requested.) 774 */ 775 protected List<Resource> basedOnTarget; 776 777 778 /** 779 * The status of the diagnostic report as a whole. 780 */ 781 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 782 @Description(shortDefinition="registered | partial | preliminary | final +", formalDefinition="The status of the diagnostic report as a whole." ) 783 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnostic-report-status") 784 protected Enumeration<DiagnosticReportStatus> status; 785 786 /** 787 * A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes. 788 */ 789 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 790 @Description(shortDefinition="Service category", formalDefinition="A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes." ) 791 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnostic-service-sections") 792 protected CodeableConcept category; 793 794 /** 795 * A code or name that describes this diagnostic report. 796 */ 797 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 798 @Description(shortDefinition="Name/Code for this diagnostic report", formalDefinition="A code or name that describes this diagnostic report." ) 799 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-codes") 800 protected CodeableConcept code; 801 802 /** 803 * The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources. 804 */ 805 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class}, order=5, min=0, max=1, modifier=false, summary=true) 806 @Description(shortDefinition="The subject of the report - usually, but not always, the patient", formalDefinition="The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources." ) 807 protected Reference subject; 808 809 /** 810 * The actual object that is the target of the reference (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 811 */ 812 protected Resource subjectTarget; 813 814 /** 815 * The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about. 816 */ 817 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=true) 818 @Description(shortDefinition="Health care event when test ordered", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about." ) 819 protected Reference context; 820 821 /** 822 * The actual object that is the target of the reference (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 823 */ 824 protected Resource contextTarget; 825 826 /** 827 * The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself. 828 */ 829 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 830 @Description(shortDefinition="Clinically relevant time/time-period for report", formalDefinition="The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself." ) 831 protected Type effective; 832 833 /** 834 * The date and time that this version of the report was released from the source diagnostic service. 835 */ 836 @Child(name = "issued", type = {InstantType.class}, order=8, min=0, max=1, modifier=false, summary=true) 837 @Description(shortDefinition="DateTime this version was released", formalDefinition="The date and time that this version of the report was released from the source diagnostic service." ) 838 protected InstantType issued; 839 840 /** 841 * Indicates who or what participated in producing the report. 842 */ 843 @Child(name = "performer", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 844 @Description(shortDefinition="Participants in producing the report", formalDefinition="Indicates who or what participated in producing the report." ) 845 protected List<DiagnosticReportPerformerComponent> performer; 846 847 /** 848 * Details about the specimens on which this diagnostic report is based. 849 */ 850 @Child(name = "specimen", type = {Specimen.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 851 @Description(shortDefinition="Specimens this report is based on", formalDefinition="Details about the specimens on which this diagnostic report is based." ) 852 protected List<Reference> specimen; 853 /** 854 * The actual objects that are the target of the reference (Details about the specimens on which this diagnostic report is based.) 855 */ 856 protected List<Specimen> specimenTarget; 857 858 859 /** 860 * Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. "atomic" results), or they can be grouping observations that include references to other members of the group (e.g. "panels"). 861 */ 862 @Child(name = "result", type = {Observation.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 863 @Description(shortDefinition="Observations - simple, or complex nested groups", formalDefinition="Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\")." ) 864 protected List<Reference> result; 865 /** 866 * The actual objects that are the target of the reference (Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. "atomic" results), or they can be grouping observations that include references to other members of the group (e.g. "panels").) 867 */ 868 protected List<Observation> resultTarget; 869 870 871 /** 872 * One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images. 873 */ 874 @Child(name = "imagingStudy", type = {ImagingStudy.class, ImagingManifest.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 875 @Description(shortDefinition="Reference to full details of imaging associated with the diagnostic report", formalDefinition="One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images." ) 876 protected List<Reference> imagingStudy; 877 /** 878 * The actual objects that are the target of the reference (One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.) 879 */ 880 protected List<Resource> imagingStudyTarget; 881 882 883 /** 884 * A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest). 885 */ 886 @Child(name = "image", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 887 @Description(shortDefinition="Key images associated with this report", formalDefinition="A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest)." ) 888 protected List<DiagnosticReportImageComponent> image; 889 890 /** 891 * Concise and clinically contextualized impression / summary of the diagnostic report. 892 */ 893 @Child(name = "conclusion", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 894 @Description(shortDefinition="Clinical Interpretation of test results", formalDefinition="Concise and clinically contextualized impression / summary of the diagnostic report." ) 895 protected StringType conclusion; 896 897 /** 898 * Codes for the conclusion. 899 */ 900 @Child(name = "codedDiagnosis", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 901 @Description(shortDefinition="Codes for the conclusion", formalDefinition="Codes for the conclusion." ) 902 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 903 protected List<CodeableConcept> codedDiagnosis; 904 905 /** 906 * Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent. 907 */ 908 @Child(name = "presentedForm", type = {Attachment.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 909 @Description(shortDefinition="Entire report as issued", formalDefinition="Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent." ) 910 protected List<Attachment> presentedForm; 911 912 private static final long serialVersionUID = 989012294L; 913 914 /** 915 * Constructor 916 */ 917 public DiagnosticReport() { 918 super(); 919 } 920 921 /** 922 * Constructor 923 */ 924 public DiagnosticReport(Enumeration<DiagnosticReportStatus> status, CodeableConcept code) { 925 super(); 926 this.status = status; 927 this.code = code; 928 } 929 930 /** 931 * @return {@link #identifier} (Identifiers assigned to this report by the performer or other systems.) 932 */ 933 public List<Identifier> getIdentifier() { 934 if (this.identifier == null) 935 this.identifier = new ArrayList<Identifier>(); 936 return this.identifier; 937 } 938 939 /** 940 * @return Returns a reference to <code>this</code> for easy method chaining 941 */ 942 public DiagnosticReport setIdentifier(List<Identifier> theIdentifier) { 943 this.identifier = theIdentifier; 944 return this; 945 } 946 947 public boolean hasIdentifier() { 948 if (this.identifier == null) 949 return false; 950 for (Identifier item : this.identifier) 951 if (!item.isEmpty()) 952 return true; 953 return false; 954 } 955 956 public Identifier addIdentifier() { //3 957 Identifier t = new Identifier(); 958 if (this.identifier == null) 959 this.identifier = new ArrayList<Identifier>(); 960 this.identifier.add(t); 961 return t; 962 } 963 964 public DiagnosticReport addIdentifier(Identifier t) { //3 965 if (t == null) 966 return this; 967 if (this.identifier == null) 968 this.identifier = new ArrayList<Identifier>(); 969 this.identifier.add(t); 970 return this; 971 } 972 973 /** 974 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 975 */ 976 public Identifier getIdentifierFirstRep() { 977 if (getIdentifier().isEmpty()) { 978 addIdentifier(); 979 } 980 return getIdentifier().get(0); 981 } 982 983 /** 984 * @return {@link #basedOn} (Details concerning a test or procedure requested.) 985 */ 986 public List<Reference> getBasedOn() { 987 if (this.basedOn == null) 988 this.basedOn = new ArrayList<Reference>(); 989 return this.basedOn; 990 } 991 992 /** 993 * @return Returns a reference to <code>this</code> for easy method chaining 994 */ 995 public DiagnosticReport setBasedOn(List<Reference> theBasedOn) { 996 this.basedOn = theBasedOn; 997 return this; 998 } 999 1000 public boolean hasBasedOn() { 1001 if (this.basedOn == null) 1002 return false; 1003 for (Reference item : this.basedOn) 1004 if (!item.isEmpty()) 1005 return true; 1006 return false; 1007 } 1008 1009 public Reference addBasedOn() { //3 1010 Reference t = new Reference(); 1011 if (this.basedOn == null) 1012 this.basedOn = new ArrayList<Reference>(); 1013 this.basedOn.add(t); 1014 return t; 1015 } 1016 1017 public DiagnosticReport addBasedOn(Reference t) { //3 1018 if (t == null) 1019 return this; 1020 if (this.basedOn == null) 1021 this.basedOn = new ArrayList<Reference>(); 1022 this.basedOn.add(t); 1023 return this; 1024 } 1025 1026 /** 1027 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1028 */ 1029 public Reference getBasedOnFirstRep() { 1030 if (getBasedOn().isEmpty()) { 1031 addBasedOn(); 1032 } 1033 return getBasedOn().get(0); 1034 } 1035 1036 /** 1037 * @deprecated Use Reference#setResource(IBaseResource) instead 1038 */ 1039 @Deprecated 1040 public List<Resource> getBasedOnTarget() { 1041 if (this.basedOnTarget == null) 1042 this.basedOnTarget = new ArrayList<Resource>(); 1043 return this.basedOnTarget; 1044 } 1045 1046 /** 1047 * @return {@link #status} (The status of the diagnostic report as a whole.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1048 */ 1049 public Enumeration<DiagnosticReportStatus> getStatusElement() { 1050 if (this.status == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create DiagnosticReport.status"); 1053 else if (Configuration.doAutoCreate()) 1054 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); // bb 1055 return this.status; 1056 } 1057 1058 public boolean hasStatusElement() { 1059 return this.status != null && !this.status.isEmpty(); 1060 } 1061 1062 public boolean hasStatus() { 1063 return this.status != null && !this.status.isEmpty(); 1064 } 1065 1066 /** 1067 * @param value {@link #status} (The status of the diagnostic report as a whole.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1068 */ 1069 public DiagnosticReport setStatusElement(Enumeration<DiagnosticReportStatus> value) { 1070 this.status = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return The status of the diagnostic report as a whole. 1076 */ 1077 public DiagnosticReportStatus getStatus() { 1078 return this.status == null ? null : this.status.getValue(); 1079 } 1080 1081 /** 1082 * @param value The status of the diagnostic report as a whole. 1083 */ 1084 public DiagnosticReport setStatus(DiagnosticReportStatus value) { 1085 if (this.status == null) 1086 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); 1087 this.status.setValue(value); 1088 return this; 1089 } 1090 1091 /** 1092 * @return {@link #category} (A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.) 1093 */ 1094 public CodeableConcept getCategory() { 1095 if (this.category == null) 1096 if (Configuration.errorOnAutoCreate()) 1097 throw new Error("Attempt to auto-create DiagnosticReport.category"); 1098 else if (Configuration.doAutoCreate()) 1099 this.category = new CodeableConcept(); // cc 1100 return this.category; 1101 } 1102 1103 public boolean hasCategory() { 1104 return this.category != null && !this.category.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #category} (A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.) 1109 */ 1110 public DiagnosticReport setCategory(CodeableConcept value) { 1111 this.category = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return {@link #code} (A code or name that describes this diagnostic report.) 1117 */ 1118 public CodeableConcept getCode() { 1119 if (this.code == null) 1120 if (Configuration.errorOnAutoCreate()) 1121 throw new Error("Attempt to auto-create DiagnosticReport.code"); 1122 else if (Configuration.doAutoCreate()) 1123 this.code = new CodeableConcept(); // cc 1124 return this.code; 1125 } 1126 1127 public boolean hasCode() { 1128 return this.code != null && !this.code.isEmpty(); 1129 } 1130 1131 /** 1132 * @param value {@link #code} (A code or name that describes this diagnostic report.) 1133 */ 1134 public DiagnosticReport setCode(CodeableConcept value) { 1135 this.code = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #subject} (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1141 */ 1142 public Reference getSubject() { 1143 if (this.subject == null) 1144 if (Configuration.errorOnAutoCreate()) 1145 throw new Error("Attempt to auto-create DiagnosticReport.subject"); 1146 else if (Configuration.doAutoCreate()) 1147 this.subject = new Reference(); // cc 1148 return this.subject; 1149 } 1150 1151 public boolean hasSubject() { 1152 return this.subject != null && !this.subject.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #subject} (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1157 */ 1158 public DiagnosticReport setSubject(Reference value) { 1159 this.subject = value; 1160 return this; 1161 } 1162 1163 /** 1164 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1165 */ 1166 public Resource getSubjectTarget() { 1167 return this.subjectTarget; 1168 } 1169 1170 /** 1171 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1172 */ 1173 public DiagnosticReport setSubjectTarget(Resource value) { 1174 this.subjectTarget = value; 1175 return this; 1176 } 1177 1178 /** 1179 * @return {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1180 */ 1181 public Reference getContext() { 1182 if (this.context == null) 1183 if (Configuration.errorOnAutoCreate()) 1184 throw new Error("Attempt to auto-create DiagnosticReport.context"); 1185 else if (Configuration.doAutoCreate()) 1186 this.context = new Reference(); // cc 1187 return this.context; 1188 } 1189 1190 public boolean hasContext() { 1191 return this.context != null && !this.context.isEmpty(); 1192 } 1193 1194 /** 1195 * @param value {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1196 */ 1197 public DiagnosticReport setContext(Reference value) { 1198 this.context = value; 1199 return this; 1200 } 1201 1202 /** 1203 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1204 */ 1205 public Resource getContextTarget() { 1206 return this.contextTarget; 1207 } 1208 1209 /** 1210 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.) 1211 */ 1212 public DiagnosticReport setContextTarget(Resource value) { 1213 this.contextTarget = value; 1214 return this; 1215 } 1216 1217 /** 1218 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1219 */ 1220 public Type getEffective() { 1221 return this.effective; 1222 } 1223 1224 /** 1225 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1226 */ 1227 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1228 if (this.effective == null) 1229 return null; 1230 if (!(this.effective instanceof DateTimeType)) 1231 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 1232 return (DateTimeType) this.effective; 1233 } 1234 1235 public boolean hasEffectiveDateTimeType() { 1236 return this != null && this.effective instanceof DateTimeType; 1237 } 1238 1239 /** 1240 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1241 */ 1242 public Period getEffectivePeriod() throws FHIRException { 1243 if (this.effective == null) 1244 return null; 1245 if (!(this.effective instanceof Period)) 1246 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 1247 return (Period) this.effective; 1248 } 1249 1250 public boolean hasEffectivePeriod() { 1251 return this != null && this.effective instanceof Period; 1252 } 1253 1254 public boolean hasEffective() { 1255 return this.effective != null && !this.effective.isEmpty(); 1256 } 1257 1258 /** 1259 * @param value {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1260 */ 1261 public DiagnosticReport setEffective(Type value) throws FHIRFormatError { 1262 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1263 throw new FHIRFormatError("Not the right type for DiagnosticReport.effective[x]: "+value.fhirType()); 1264 this.effective = value; 1265 return this; 1266 } 1267 1268 /** 1269 * @return {@link #issued} (The date and time that this version of the report was released from the source diagnostic service.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1270 */ 1271 public InstantType getIssuedElement() { 1272 if (this.issued == null) 1273 if (Configuration.errorOnAutoCreate()) 1274 throw new Error("Attempt to auto-create DiagnosticReport.issued"); 1275 else if (Configuration.doAutoCreate()) 1276 this.issued = new InstantType(); // bb 1277 return this.issued; 1278 } 1279 1280 public boolean hasIssuedElement() { 1281 return this.issued != null && !this.issued.isEmpty(); 1282 } 1283 1284 public boolean hasIssued() { 1285 return this.issued != null && !this.issued.isEmpty(); 1286 } 1287 1288 /** 1289 * @param value {@link #issued} (The date and time that this version of the report was released from the source diagnostic service.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1290 */ 1291 public DiagnosticReport setIssuedElement(InstantType value) { 1292 this.issued = value; 1293 return this; 1294 } 1295 1296 /** 1297 * @return The date and time that this version of the report was released from the source diagnostic service. 1298 */ 1299 public Date getIssued() { 1300 return this.issued == null ? null : this.issued.getValue(); 1301 } 1302 1303 /** 1304 * @param value The date and time that this version of the report was released from the source diagnostic service. 1305 */ 1306 public DiagnosticReport setIssued(Date value) { 1307 if (value == null) 1308 this.issued = null; 1309 else { 1310 if (this.issued == null) 1311 this.issued = new InstantType(); 1312 this.issued.setValue(value); 1313 } 1314 return this; 1315 } 1316 1317 /** 1318 * @return {@link #performer} (Indicates who or what participated in producing the report.) 1319 */ 1320 public List<DiagnosticReportPerformerComponent> getPerformer() { 1321 if (this.performer == null) 1322 this.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 1323 return this.performer; 1324 } 1325 1326 /** 1327 * @return Returns a reference to <code>this</code> for easy method chaining 1328 */ 1329 public DiagnosticReport setPerformer(List<DiagnosticReportPerformerComponent> thePerformer) { 1330 this.performer = thePerformer; 1331 return this; 1332 } 1333 1334 public boolean hasPerformer() { 1335 if (this.performer == null) 1336 return false; 1337 for (DiagnosticReportPerformerComponent item : this.performer) 1338 if (!item.isEmpty()) 1339 return true; 1340 return false; 1341 } 1342 1343 public DiagnosticReportPerformerComponent addPerformer() { //3 1344 DiagnosticReportPerformerComponent t = new DiagnosticReportPerformerComponent(); 1345 if (this.performer == null) 1346 this.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 1347 this.performer.add(t); 1348 return t; 1349 } 1350 1351 public DiagnosticReport addPerformer(DiagnosticReportPerformerComponent t) { //3 1352 if (t == null) 1353 return this; 1354 if (this.performer == null) 1355 this.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 1356 this.performer.add(t); 1357 return this; 1358 } 1359 1360 /** 1361 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 1362 */ 1363 public DiagnosticReportPerformerComponent getPerformerFirstRep() { 1364 if (getPerformer().isEmpty()) { 1365 addPerformer(); 1366 } 1367 return getPerformer().get(0); 1368 } 1369 1370 /** 1371 * @return {@link #specimen} (Details about the specimens on which this diagnostic report is based.) 1372 */ 1373 public List<Reference> getSpecimen() { 1374 if (this.specimen == null) 1375 this.specimen = new ArrayList<Reference>(); 1376 return this.specimen; 1377 } 1378 1379 /** 1380 * @return Returns a reference to <code>this</code> for easy method chaining 1381 */ 1382 public DiagnosticReport setSpecimen(List<Reference> theSpecimen) { 1383 this.specimen = theSpecimen; 1384 return this; 1385 } 1386 1387 public boolean hasSpecimen() { 1388 if (this.specimen == null) 1389 return false; 1390 for (Reference item : this.specimen) 1391 if (!item.isEmpty()) 1392 return true; 1393 return false; 1394 } 1395 1396 public Reference addSpecimen() { //3 1397 Reference t = new Reference(); 1398 if (this.specimen == null) 1399 this.specimen = new ArrayList<Reference>(); 1400 this.specimen.add(t); 1401 return t; 1402 } 1403 1404 public DiagnosticReport addSpecimen(Reference t) { //3 1405 if (t == null) 1406 return this; 1407 if (this.specimen == null) 1408 this.specimen = new ArrayList<Reference>(); 1409 this.specimen.add(t); 1410 return this; 1411 } 1412 1413 /** 1414 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist 1415 */ 1416 public Reference getSpecimenFirstRep() { 1417 if (getSpecimen().isEmpty()) { 1418 addSpecimen(); 1419 } 1420 return getSpecimen().get(0); 1421 } 1422 1423 /** 1424 * @deprecated Use Reference#setResource(IBaseResource) instead 1425 */ 1426 @Deprecated 1427 public List<Specimen> getSpecimenTarget() { 1428 if (this.specimenTarget == null) 1429 this.specimenTarget = new ArrayList<Specimen>(); 1430 return this.specimenTarget; 1431 } 1432 1433 /** 1434 * @deprecated Use Reference#setResource(IBaseResource) instead 1435 */ 1436 @Deprecated 1437 public Specimen addSpecimenTarget() { 1438 Specimen r = new Specimen(); 1439 if (this.specimenTarget == null) 1440 this.specimenTarget = new ArrayList<Specimen>(); 1441 this.specimenTarget.add(r); 1442 return r; 1443 } 1444 1445 /** 1446 * @return {@link #result} (Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. "atomic" results), or they can be grouping observations that include references to other members of the group (e.g. "panels").) 1447 */ 1448 public List<Reference> getResult() { 1449 if (this.result == null) 1450 this.result = new ArrayList<Reference>(); 1451 return this.result; 1452 } 1453 1454 /** 1455 * @return Returns a reference to <code>this</code> for easy method chaining 1456 */ 1457 public DiagnosticReport setResult(List<Reference> theResult) { 1458 this.result = theResult; 1459 return this; 1460 } 1461 1462 public boolean hasResult() { 1463 if (this.result == null) 1464 return false; 1465 for (Reference item : this.result) 1466 if (!item.isEmpty()) 1467 return true; 1468 return false; 1469 } 1470 1471 public Reference addResult() { //3 1472 Reference t = new Reference(); 1473 if (this.result == null) 1474 this.result = new ArrayList<Reference>(); 1475 this.result.add(t); 1476 return t; 1477 } 1478 1479 public DiagnosticReport addResult(Reference t) { //3 1480 if (t == null) 1481 return this; 1482 if (this.result == null) 1483 this.result = new ArrayList<Reference>(); 1484 this.result.add(t); 1485 return this; 1486 } 1487 1488 /** 1489 * @return The first repetition of repeating field {@link #result}, creating it if it does not already exist 1490 */ 1491 public Reference getResultFirstRep() { 1492 if (getResult().isEmpty()) { 1493 addResult(); 1494 } 1495 return getResult().get(0); 1496 } 1497 1498 /** 1499 * @deprecated Use Reference#setResource(IBaseResource) instead 1500 */ 1501 @Deprecated 1502 public List<Observation> getResultTarget() { 1503 if (this.resultTarget == null) 1504 this.resultTarget = new ArrayList<Observation>(); 1505 return this.resultTarget; 1506 } 1507 1508 /** 1509 * @deprecated Use Reference#setResource(IBaseResource) instead 1510 */ 1511 @Deprecated 1512 public Observation addResultTarget() { 1513 Observation r = new Observation(); 1514 if (this.resultTarget == null) 1515 this.resultTarget = new ArrayList<Observation>(); 1516 this.resultTarget.add(r); 1517 return r; 1518 } 1519 1520 /** 1521 * @return {@link #imagingStudy} (One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.) 1522 */ 1523 public List<Reference> getImagingStudy() { 1524 if (this.imagingStudy == null) 1525 this.imagingStudy = new ArrayList<Reference>(); 1526 return this.imagingStudy; 1527 } 1528 1529 /** 1530 * @return Returns a reference to <code>this</code> for easy method chaining 1531 */ 1532 public DiagnosticReport setImagingStudy(List<Reference> theImagingStudy) { 1533 this.imagingStudy = theImagingStudy; 1534 return this; 1535 } 1536 1537 public boolean hasImagingStudy() { 1538 if (this.imagingStudy == null) 1539 return false; 1540 for (Reference item : this.imagingStudy) 1541 if (!item.isEmpty()) 1542 return true; 1543 return false; 1544 } 1545 1546 public Reference addImagingStudy() { //3 1547 Reference t = new Reference(); 1548 if (this.imagingStudy == null) 1549 this.imagingStudy = new ArrayList<Reference>(); 1550 this.imagingStudy.add(t); 1551 return t; 1552 } 1553 1554 public DiagnosticReport addImagingStudy(Reference t) { //3 1555 if (t == null) 1556 return this; 1557 if (this.imagingStudy == null) 1558 this.imagingStudy = new ArrayList<Reference>(); 1559 this.imagingStudy.add(t); 1560 return this; 1561 } 1562 1563 /** 1564 * @return The first repetition of repeating field {@link #imagingStudy}, creating it if it does not already exist 1565 */ 1566 public Reference getImagingStudyFirstRep() { 1567 if (getImagingStudy().isEmpty()) { 1568 addImagingStudy(); 1569 } 1570 return getImagingStudy().get(0); 1571 } 1572 1573 /** 1574 * @deprecated Use Reference#setResource(IBaseResource) instead 1575 */ 1576 @Deprecated 1577 public List<Resource> getImagingStudyTarget() { 1578 if (this.imagingStudyTarget == null) 1579 this.imagingStudyTarget = new ArrayList<Resource>(); 1580 return this.imagingStudyTarget; 1581 } 1582 1583 /** 1584 * @return {@link #image} (A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).) 1585 */ 1586 public List<DiagnosticReportImageComponent> getImage() { 1587 if (this.image == null) 1588 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1589 return this.image; 1590 } 1591 1592 /** 1593 * @return Returns a reference to <code>this</code> for easy method chaining 1594 */ 1595 public DiagnosticReport setImage(List<DiagnosticReportImageComponent> theImage) { 1596 this.image = theImage; 1597 return this; 1598 } 1599 1600 public boolean hasImage() { 1601 if (this.image == null) 1602 return false; 1603 for (DiagnosticReportImageComponent item : this.image) 1604 if (!item.isEmpty()) 1605 return true; 1606 return false; 1607 } 1608 1609 public DiagnosticReportImageComponent addImage() { //3 1610 DiagnosticReportImageComponent t = new DiagnosticReportImageComponent(); 1611 if (this.image == null) 1612 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1613 this.image.add(t); 1614 return t; 1615 } 1616 1617 public DiagnosticReport addImage(DiagnosticReportImageComponent t) { //3 1618 if (t == null) 1619 return this; 1620 if (this.image == null) 1621 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1622 this.image.add(t); 1623 return this; 1624 } 1625 1626 /** 1627 * @return The first repetition of repeating field {@link #image}, creating it if it does not already exist 1628 */ 1629 public DiagnosticReportImageComponent getImageFirstRep() { 1630 if (getImage().isEmpty()) { 1631 addImage(); 1632 } 1633 return getImage().get(0); 1634 } 1635 1636 /** 1637 * @return {@link #conclusion} (Concise and clinically contextualized impression / summary of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getConclusion" gives direct access to the value 1638 */ 1639 public StringType getConclusionElement() { 1640 if (this.conclusion == null) 1641 if (Configuration.errorOnAutoCreate()) 1642 throw new Error("Attempt to auto-create DiagnosticReport.conclusion"); 1643 else if (Configuration.doAutoCreate()) 1644 this.conclusion = new StringType(); // bb 1645 return this.conclusion; 1646 } 1647 1648 public boolean hasConclusionElement() { 1649 return this.conclusion != null && !this.conclusion.isEmpty(); 1650 } 1651 1652 public boolean hasConclusion() { 1653 return this.conclusion != null && !this.conclusion.isEmpty(); 1654 } 1655 1656 /** 1657 * @param value {@link #conclusion} (Concise and clinically contextualized impression / summary of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getConclusion" gives direct access to the value 1658 */ 1659 public DiagnosticReport setConclusionElement(StringType value) { 1660 this.conclusion = value; 1661 return this; 1662 } 1663 1664 /** 1665 * @return Concise and clinically contextualized impression / summary of the diagnostic report. 1666 */ 1667 public String getConclusion() { 1668 return this.conclusion == null ? null : this.conclusion.getValue(); 1669 } 1670 1671 /** 1672 * @param value Concise and clinically contextualized impression / summary of the diagnostic report. 1673 */ 1674 public DiagnosticReport setConclusion(String value) { 1675 if (Utilities.noString(value)) 1676 this.conclusion = null; 1677 else { 1678 if (this.conclusion == null) 1679 this.conclusion = new StringType(); 1680 this.conclusion.setValue(value); 1681 } 1682 return this; 1683 } 1684 1685 /** 1686 * @return {@link #codedDiagnosis} (Codes for the conclusion.) 1687 */ 1688 public List<CodeableConcept> getCodedDiagnosis() { 1689 if (this.codedDiagnosis == null) 1690 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1691 return this.codedDiagnosis; 1692 } 1693 1694 /** 1695 * @return Returns a reference to <code>this</code> for easy method chaining 1696 */ 1697 public DiagnosticReport setCodedDiagnosis(List<CodeableConcept> theCodedDiagnosis) { 1698 this.codedDiagnosis = theCodedDiagnosis; 1699 return this; 1700 } 1701 1702 public boolean hasCodedDiagnosis() { 1703 if (this.codedDiagnosis == null) 1704 return false; 1705 for (CodeableConcept item : this.codedDiagnosis) 1706 if (!item.isEmpty()) 1707 return true; 1708 return false; 1709 } 1710 1711 public CodeableConcept addCodedDiagnosis() { //3 1712 CodeableConcept t = new CodeableConcept(); 1713 if (this.codedDiagnosis == null) 1714 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1715 this.codedDiagnosis.add(t); 1716 return t; 1717 } 1718 1719 public DiagnosticReport addCodedDiagnosis(CodeableConcept t) { //3 1720 if (t == null) 1721 return this; 1722 if (this.codedDiagnosis == null) 1723 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1724 this.codedDiagnosis.add(t); 1725 return this; 1726 } 1727 1728 /** 1729 * @return The first repetition of repeating field {@link #codedDiagnosis}, creating it if it does not already exist 1730 */ 1731 public CodeableConcept getCodedDiagnosisFirstRep() { 1732 if (getCodedDiagnosis().isEmpty()) { 1733 addCodedDiagnosis(); 1734 } 1735 return getCodedDiagnosis().get(0); 1736 } 1737 1738 /** 1739 * @return {@link #presentedForm} (Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.) 1740 */ 1741 public List<Attachment> getPresentedForm() { 1742 if (this.presentedForm == null) 1743 this.presentedForm = new ArrayList<Attachment>(); 1744 return this.presentedForm; 1745 } 1746 1747 /** 1748 * @return Returns a reference to <code>this</code> for easy method chaining 1749 */ 1750 public DiagnosticReport setPresentedForm(List<Attachment> thePresentedForm) { 1751 this.presentedForm = thePresentedForm; 1752 return this; 1753 } 1754 1755 public boolean hasPresentedForm() { 1756 if (this.presentedForm == null) 1757 return false; 1758 for (Attachment item : this.presentedForm) 1759 if (!item.isEmpty()) 1760 return true; 1761 return false; 1762 } 1763 1764 public Attachment addPresentedForm() { //3 1765 Attachment t = new Attachment(); 1766 if (this.presentedForm == null) 1767 this.presentedForm = new ArrayList<Attachment>(); 1768 this.presentedForm.add(t); 1769 return t; 1770 } 1771 1772 public DiagnosticReport addPresentedForm(Attachment t) { //3 1773 if (t == null) 1774 return this; 1775 if (this.presentedForm == null) 1776 this.presentedForm = new ArrayList<Attachment>(); 1777 this.presentedForm.add(t); 1778 return this; 1779 } 1780 1781 /** 1782 * @return The first repetition of repeating field {@link #presentedForm}, creating it if it does not already exist 1783 */ 1784 public Attachment getPresentedFormFirstRep() { 1785 if (getPresentedForm().isEmpty()) { 1786 addPresentedForm(); 1787 } 1788 return getPresentedForm().get(0); 1789 } 1790 1791 protected void listChildren(List<Property> children) { 1792 super.listChildren(children); 1793 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1794 children.add(new Property("basedOn", "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ProcedureRequest|ReferralRequest)", "Details concerning a test or procedure requested.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1795 children.add(new Property("status", "code", "The status of the diagnostic report as a whole.", 0, 1, status)); 1796 children.add(new Property("category", "CodeableConcept", "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 0, 1, category)); 1797 children.add(new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code)); 1798 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", "The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.", 0, 1, subject)); 1799 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.", 0, 1, context)); 1800 children.add(new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective)); 1801 children.add(new Property("issued", "instant", "The date and time that this version of the report was released from the source diagnostic service.", 0, 1, issued)); 1802 children.add(new Property("performer", "", "Indicates who or what participated in producing the report.", 0, java.lang.Integer.MAX_VALUE, performer)); 1803 children.add(new Property("specimen", "Reference(Specimen)", "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, specimen)); 1804 children.add(new Property("result", "Reference(Observation)", "Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\").", 0, java.lang.Integer.MAX_VALUE, result)); 1805 children.add(new Property("imagingStudy", "Reference(ImagingStudy|ImagingManifest)", "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 0, java.lang.Integer.MAX_VALUE, imagingStudy)); 1806 children.add(new Property("image", "", "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 0, java.lang.Integer.MAX_VALUE, image)); 1807 children.add(new Property("conclusion", "string", "Concise and clinically contextualized impression / summary of the diagnostic report.", 0, 1, conclusion)); 1808 children.add(new Property("codedDiagnosis", "CodeableConcept", "Codes for the conclusion.", 0, java.lang.Integer.MAX_VALUE, codedDiagnosis)); 1809 children.add(new Property("presentedForm", "Attachment", "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 0, java.lang.Integer.MAX_VALUE, presentedForm)); 1810 } 1811 1812 @Override 1813 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1814 switch (_hash) { 1815 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1816 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ProcedureRequest|ReferralRequest)", "Details concerning a test or procedure requested.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1817 case -892481550: /*status*/ return new Property("status", "code", "The status of the diagnostic report as a whole.", 0, 1, status); 1818 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 0, 1, category); 1819 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code); 1820 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location)", "The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.", 0, 1, subject); 1821 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport per is about.", 0, 1, context); 1822 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1823 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1824 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1825 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1826 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time that this version of the report was released from the source diagnostic service.", 0, 1, issued); 1827 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what participated in producing the report.", 0, java.lang.Integer.MAX_VALUE, performer); 1828 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, specimen); 1829 case -934426595: /*result*/ return new Property("result", "Reference(Observation)", "Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\").", 0, java.lang.Integer.MAX_VALUE, result); 1830 case -814900911: /*imagingStudy*/ return new Property("imagingStudy", "Reference(ImagingStudy|ImagingManifest)", "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 0, java.lang.Integer.MAX_VALUE, imagingStudy); 1831 case 100313435: /*image*/ return new Property("image", "", "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 0, java.lang.Integer.MAX_VALUE, image); 1832 case -1731259873: /*conclusion*/ return new Property("conclusion", "string", "Concise and clinically contextualized impression / summary of the diagnostic report.", 0, 1, conclusion); 1833 case -1364269926: /*codedDiagnosis*/ return new Property("codedDiagnosis", "CodeableConcept", "Codes for the conclusion.", 0, java.lang.Integer.MAX_VALUE, codedDiagnosis); 1834 case 230090366: /*presentedForm*/ return new Property("presentedForm", "Attachment", "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 0, java.lang.Integer.MAX_VALUE, presentedForm); 1835 default: return super.getNamedProperty(_hash, _name, _checkValid); 1836 } 1837 1838 } 1839 1840 @Override 1841 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1842 switch (hash) { 1843 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1844 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1845 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DiagnosticReportStatus> 1846 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1847 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1848 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1849 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1850 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // Type 1851 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 1852 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // DiagnosticReportPerformerComponent 1853 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 1854 case -934426595: /*result*/ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 1855 case -814900911: /*imagingStudy*/ return this.imagingStudy == null ? new Base[0] : this.imagingStudy.toArray(new Base[this.imagingStudy.size()]); // Reference 1856 case 100313435: /*image*/ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // DiagnosticReportImageComponent 1857 case -1731259873: /*conclusion*/ return this.conclusion == null ? new Base[0] : new Base[] {this.conclusion}; // StringType 1858 case -1364269926: /*codedDiagnosis*/ return this.codedDiagnosis == null ? new Base[0] : this.codedDiagnosis.toArray(new Base[this.codedDiagnosis.size()]); // CodeableConcept 1859 case 230090366: /*presentedForm*/ return this.presentedForm == null ? new Base[0] : this.presentedForm.toArray(new Base[this.presentedForm.size()]); // Attachment 1860 default: return super.getProperty(hash, name, checkValid); 1861 } 1862 1863 } 1864 1865 @Override 1866 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1867 switch (hash) { 1868 case -1618432855: // identifier 1869 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1870 return value; 1871 case -332612366: // basedOn 1872 this.getBasedOn().add(castToReference(value)); // Reference 1873 return value; 1874 case -892481550: // status 1875 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 1876 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 1877 return value; 1878 case 50511102: // category 1879 this.category = castToCodeableConcept(value); // CodeableConcept 1880 return value; 1881 case 3059181: // code 1882 this.code = castToCodeableConcept(value); // CodeableConcept 1883 return value; 1884 case -1867885268: // subject 1885 this.subject = castToReference(value); // Reference 1886 return value; 1887 case 951530927: // context 1888 this.context = castToReference(value); // Reference 1889 return value; 1890 case -1468651097: // effective 1891 this.effective = castToType(value); // Type 1892 return value; 1893 case -1179159893: // issued 1894 this.issued = castToInstant(value); // InstantType 1895 return value; 1896 case 481140686: // performer 1897 this.getPerformer().add((DiagnosticReportPerformerComponent) value); // DiagnosticReportPerformerComponent 1898 return value; 1899 case -2132868344: // specimen 1900 this.getSpecimen().add(castToReference(value)); // Reference 1901 return value; 1902 case -934426595: // result 1903 this.getResult().add(castToReference(value)); // Reference 1904 return value; 1905 case -814900911: // imagingStudy 1906 this.getImagingStudy().add(castToReference(value)); // Reference 1907 return value; 1908 case 100313435: // image 1909 this.getImage().add((DiagnosticReportImageComponent) value); // DiagnosticReportImageComponent 1910 return value; 1911 case -1731259873: // conclusion 1912 this.conclusion = castToString(value); // StringType 1913 return value; 1914 case -1364269926: // codedDiagnosis 1915 this.getCodedDiagnosis().add(castToCodeableConcept(value)); // CodeableConcept 1916 return value; 1917 case 230090366: // presentedForm 1918 this.getPresentedForm().add(castToAttachment(value)); // Attachment 1919 return value; 1920 default: return super.setProperty(hash, name, value); 1921 } 1922 1923 } 1924 1925 @Override 1926 public Base setProperty(String name, Base value) throws FHIRException { 1927 if (name.equals("identifier")) { 1928 this.getIdentifier().add(castToIdentifier(value)); 1929 } else if (name.equals("basedOn")) { 1930 this.getBasedOn().add(castToReference(value)); 1931 } else if (name.equals("status")) { 1932 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 1933 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 1934 } else if (name.equals("category")) { 1935 this.category = castToCodeableConcept(value); // CodeableConcept 1936 } else if (name.equals("code")) { 1937 this.code = castToCodeableConcept(value); // CodeableConcept 1938 } else if (name.equals("subject")) { 1939 this.subject = castToReference(value); // Reference 1940 } else if (name.equals("context")) { 1941 this.context = castToReference(value); // Reference 1942 } else if (name.equals("effective[x]")) { 1943 this.effective = castToType(value); // Type 1944 } else if (name.equals("issued")) { 1945 this.issued = castToInstant(value); // InstantType 1946 } else if (name.equals("performer")) { 1947 this.getPerformer().add((DiagnosticReportPerformerComponent) value); 1948 } else if (name.equals("specimen")) { 1949 this.getSpecimen().add(castToReference(value)); 1950 } else if (name.equals("result")) { 1951 this.getResult().add(castToReference(value)); 1952 } else if (name.equals("imagingStudy")) { 1953 this.getImagingStudy().add(castToReference(value)); 1954 } else if (name.equals("image")) { 1955 this.getImage().add((DiagnosticReportImageComponent) value); 1956 } else if (name.equals("conclusion")) { 1957 this.conclusion = castToString(value); // StringType 1958 } else if (name.equals("codedDiagnosis")) { 1959 this.getCodedDiagnosis().add(castToCodeableConcept(value)); 1960 } else if (name.equals("presentedForm")) { 1961 this.getPresentedForm().add(castToAttachment(value)); 1962 } else 1963 return super.setProperty(name, value); 1964 return value; 1965 } 1966 1967 @Override 1968 public Base makeProperty(int hash, String name) throws FHIRException { 1969 switch (hash) { 1970 case -1618432855: return addIdentifier(); 1971 case -332612366: return addBasedOn(); 1972 case -892481550: return getStatusElement(); 1973 case 50511102: return getCategory(); 1974 case 3059181: return getCode(); 1975 case -1867885268: return getSubject(); 1976 case 951530927: return getContext(); 1977 case 247104889: return getEffective(); 1978 case -1468651097: return getEffective(); 1979 case -1179159893: return getIssuedElement(); 1980 case 481140686: return addPerformer(); 1981 case -2132868344: return addSpecimen(); 1982 case -934426595: return addResult(); 1983 case -814900911: return addImagingStudy(); 1984 case 100313435: return addImage(); 1985 case -1731259873: return getConclusionElement(); 1986 case -1364269926: return addCodedDiagnosis(); 1987 case 230090366: return addPresentedForm(); 1988 default: return super.makeProperty(hash, name); 1989 } 1990 1991 } 1992 1993 @Override 1994 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1995 switch (hash) { 1996 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1997 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1998 case -892481550: /*status*/ return new String[] {"code"}; 1999 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2000 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2001 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2002 case 951530927: /*context*/ return new String[] {"Reference"}; 2003 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 2004 case -1179159893: /*issued*/ return new String[] {"instant"}; 2005 case 481140686: /*performer*/ return new String[] {}; 2006 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 2007 case -934426595: /*result*/ return new String[] {"Reference"}; 2008 case -814900911: /*imagingStudy*/ return new String[] {"Reference"}; 2009 case 100313435: /*image*/ return new String[] {}; 2010 case -1731259873: /*conclusion*/ return new String[] {"string"}; 2011 case -1364269926: /*codedDiagnosis*/ return new String[] {"CodeableConcept"}; 2012 case 230090366: /*presentedForm*/ return new String[] {"Attachment"}; 2013 default: return super.getTypesForProperty(hash, name); 2014 } 2015 2016 } 2017 2018 @Override 2019 public Base addChild(String name) throws FHIRException { 2020 if (name.equals("identifier")) { 2021 return addIdentifier(); 2022 } 2023 else if (name.equals("basedOn")) { 2024 return addBasedOn(); 2025 } 2026 else if (name.equals("status")) { 2027 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.status"); 2028 } 2029 else if (name.equals("category")) { 2030 this.category = new CodeableConcept(); 2031 return this.category; 2032 } 2033 else if (name.equals("code")) { 2034 this.code = new CodeableConcept(); 2035 return this.code; 2036 } 2037 else if (name.equals("subject")) { 2038 this.subject = new Reference(); 2039 return this.subject; 2040 } 2041 else if (name.equals("context")) { 2042 this.context = new Reference(); 2043 return this.context; 2044 } 2045 else if (name.equals("effectiveDateTime")) { 2046 this.effective = new DateTimeType(); 2047 return this.effective; 2048 } 2049 else if (name.equals("effectivePeriod")) { 2050 this.effective = new Period(); 2051 return this.effective; 2052 } 2053 else if (name.equals("issued")) { 2054 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.issued"); 2055 } 2056 else if (name.equals("performer")) { 2057 return addPerformer(); 2058 } 2059 else if (name.equals("specimen")) { 2060 return addSpecimen(); 2061 } 2062 else if (name.equals("result")) { 2063 return addResult(); 2064 } 2065 else if (name.equals("imagingStudy")) { 2066 return addImagingStudy(); 2067 } 2068 else if (name.equals("image")) { 2069 return addImage(); 2070 } 2071 else if (name.equals("conclusion")) { 2072 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.conclusion"); 2073 } 2074 else if (name.equals("codedDiagnosis")) { 2075 return addCodedDiagnosis(); 2076 } 2077 else if (name.equals("presentedForm")) { 2078 return addPresentedForm(); 2079 } 2080 else 2081 return super.addChild(name); 2082 } 2083 2084 public String fhirType() { 2085 return "DiagnosticReport"; 2086 2087 } 2088 2089 public DiagnosticReport copy() { 2090 DiagnosticReport dst = new DiagnosticReport(); 2091 copyValues(dst); 2092 if (identifier != null) { 2093 dst.identifier = new ArrayList<Identifier>(); 2094 for (Identifier i : identifier) 2095 dst.identifier.add(i.copy()); 2096 }; 2097 if (basedOn != null) { 2098 dst.basedOn = new ArrayList<Reference>(); 2099 for (Reference i : basedOn) 2100 dst.basedOn.add(i.copy()); 2101 }; 2102 dst.status = status == null ? null : status.copy(); 2103 dst.category = category == null ? null : category.copy(); 2104 dst.code = code == null ? null : code.copy(); 2105 dst.subject = subject == null ? null : subject.copy(); 2106 dst.context = context == null ? null : context.copy(); 2107 dst.effective = effective == null ? null : effective.copy(); 2108 dst.issued = issued == null ? null : issued.copy(); 2109 if (performer != null) { 2110 dst.performer = new ArrayList<DiagnosticReportPerformerComponent>(); 2111 for (DiagnosticReportPerformerComponent i : performer) 2112 dst.performer.add(i.copy()); 2113 }; 2114 if (specimen != null) { 2115 dst.specimen = new ArrayList<Reference>(); 2116 for (Reference i : specimen) 2117 dst.specimen.add(i.copy()); 2118 }; 2119 if (result != null) { 2120 dst.result = new ArrayList<Reference>(); 2121 for (Reference i : result) 2122 dst.result.add(i.copy()); 2123 }; 2124 if (imagingStudy != null) { 2125 dst.imagingStudy = new ArrayList<Reference>(); 2126 for (Reference i : imagingStudy) 2127 dst.imagingStudy.add(i.copy()); 2128 }; 2129 if (image != null) { 2130 dst.image = new ArrayList<DiagnosticReportImageComponent>(); 2131 for (DiagnosticReportImageComponent i : image) 2132 dst.image.add(i.copy()); 2133 }; 2134 dst.conclusion = conclusion == null ? null : conclusion.copy(); 2135 if (codedDiagnosis != null) { 2136 dst.codedDiagnosis = new ArrayList<CodeableConcept>(); 2137 for (CodeableConcept i : codedDiagnosis) 2138 dst.codedDiagnosis.add(i.copy()); 2139 }; 2140 if (presentedForm != null) { 2141 dst.presentedForm = new ArrayList<Attachment>(); 2142 for (Attachment i : presentedForm) 2143 dst.presentedForm.add(i.copy()); 2144 }; 2145 return dst; 2146 } 2147 2148 protected DiagnosticReport typedCopy() { 2149 return copy(); 2150 } 2151 2152 @Override 2153 public boolean equalsDeep(Base other_) { 2154 if (!super.equalsDeep(other_)) 2155 return false; 2156 if (!(other_ instanceof DiagnosticReport)) 2157 return false; 2158 DiagnosticReport o = (DiagnosticReport) other_; 2159 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(status, o.status, true) 2160 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 2161 && compareDeep(context, o.context, true) && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) 2162 && compareDeep(performer, o.performer, true) && compareDeep(specimen, o.specimen, true) && compareDeep(result, o.result, true) 2163 && compareDeep(imagingStudy, o.imagingStudy, true) && compareDeep(image, o.image, true) && compareDeep(conclusion, o.conclusion, true) 2164 && compareDeep(codedDiagnosis, o.codedDiagnosis, true) && compareDeep(presentedForm, o.presentedForm, true) 2165 ; 2166 } 2167 2168 @Override 2169 public boolean equalsShallow(Base other_) { 2170 if (!super.equalsShallow(other_)) 2171 return false; 2172 if (!(other_ instanceof DiagnosticReport)) 2173 return false; 2174 DiagnosticReport o = (DiagnosticReport) other_; 2175 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) && compareValues(conclusion, o.conclusion, true) 2176 ; 2177 } 2178 2179 public boolean isEmpty() { 2180 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status 2181 , category, code, subject, context, effective, issued, performer, specimen, result 2182 , imagingStudy, image, conclusion, codedDiagnosis, presentedForm); 2183 } 2184 2185 @Override 2186 public ResourceType getResourceType() { 2187 return ResourceType.DiagnosticReport; 2188 } 2189 2190 /** 2191 * Search parameter: <b>date</b> 2192 * <p> 2193 * Description: <b>The clinically relevant time of the report</b><br> 2194 * Type: <b>date</b><br> 2195 * Path: <b>DiagnosticReport.effective[x]</b><br> 2196 * </p> 2197 */ 2198 @SearchParamDefinition(name="date", path="DiagnosticReport.effective", description="The clinically relevant time of the report", type="date" ) 2199 public static final String SP_DATE = "date"; 2200 /** 2201 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2202 * <p> 2203 * Description: <b>The clinically relevant time of the report</b><br> 2204 * Type: <b>date</b><br> 2205 * Path: <b>DiagnosticReport.effective[x]</b><br> 2206 * </p> 2207 */ 2208 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2209 2210 /** 2211 * Search parameter: <b>identifier</b> 2212 * <p> 2213 * Description: <b>An identifier for the report</b><br> 2214 * Type: <b>token</b><br> 2215 * Path: <b>DiagnosticReport.identifier</b><br> 2216 * </p> 2217 */ 2218 @SearchParamDefinition(name="identifier", path="DiagnosticReport.identifier", description="An identifier for the report", type="token" ) 2219 public static final String SP_IDENTIFIER = "identifier"; 2220 /** 2221 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2222 * <p> 2223 * Description: <b>An identifier for the report</b><br> 2224 * Type: <b>token</b><br> 2225 * Path: <b>DiagnosticReport.identifier</b><br> 2226 * </p> 2227 */ 2228 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2229 2230 /** 2231 * Search parameter: <b>image</b> 2232 * <p> 2233 * Description: <b>A reference to the image source.</b><br> 2234 * Type: <b>reference</b><br> 2235 * Path: <b>DiagnosticReport.image.link</b><br> 2236 * </p> 2237 */ 2238 @SearchParamDefinition(name="image", path="DiagnosticReport.image.link", description="A reference to the image source.", type="reference", target={Media.class } ) 2239 public static final String SP_IMAGE = "image"; 2240 /** 2241 * <b>Fluent Client</b> search parameter constant for <b>image</b> 2242 * <p> 2243 * Description: <b>A reference to the image source.</b><br> 2244 * Type: <b>reference</b><br> 2245 * Path: <b>DiagnosticReport.image.link</b><br> 2246 * </p> 2247 */ 2248 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_IMAGE); 2249 2250/** 2251 * Constant for fluent queries to be used to add include statements. Specifies 2252 * the path value of "<b>DiagnosticReport:image</b>". 2253 */ 2254 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMAGE = new ca.uhn.fhir.model.api.Include("DiagnosticReport:image").toLocked(); 2255 2256 /** 2257 * Search parameter: <b>performer</b> 2258 * <p> 2259 * Description: <b>Who was the source of the report (organization)</b><br> 2260 * Type: <b>reference</b><br> 2261 * Path: <b>DiagnosticReport.performer.actor</b><br> 2262 * </p> 2263 */ 2264 @SearchParamDefinition(name="performer", path="DiagnosticReport.performer.actor", description="Who was the source of the report (organization)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 2265 public static final String SP_PERFORMER = "performer"; 2266 /** 2267 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2268 * <p> 2269 * Description: <b>Who was the source of the report (organization)</b><br> 2270 * Type: <b>reference</b><br> 2271 * Path: <b>DiagnosticReport.performer.actor</b><br> 2272 * </p> 2273 */ 2274 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2275 2276/** 2277 * Constant for fluent queries to be used to add include statements. Specifies 2278 * the path value of "<b>DiagnosticReport:performer</b>". 2279 */ 2280 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("DiagnosticReport:performer").toLocked(); 2281 2282 /** 2283 * Search parameter: <b>code</b> 2284 * <p> 2285 * Description: <b>The code for the report as a whole, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result</b><br> 2286 * Type: <b>token</b><br> 2287 * Path: <b>DiagnosticReport.code</b><br> 2288 * </p> 2289 */ 2290 @SearchParamDefinition(name="code", path="DiagnosticReport.code", description="The code for the report as a whole, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result", type="token" ) 2291 public static final String SP_CODE = "code"; 2292 /** 2293 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2294 * <p> 2295 * Description: <b>The code for the report as a whole, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result</b><br> 2296 * Type: <b>token</b><br> 2297 * Path: <b>DiagnosticReport.code</b><br> 2298 * </p> 2299 */ 2300 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2301 2302 /** 2303 * Search parameter: <b>subject</b> 2304 * <p> 2305 * Description: <b>The subject of the report</b><br> 2306 * Type: <b>reference</b><br> 2307 * Path: <b>DiagnosticReport.subject</b><br> 2308 * </p> 2309 */ 2310 @SearchParamDefinition(name="subject", path="DiagnosticReport.subject", description="The subject of the report", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 2311 public static final String SP_SUBJECT = "subject"; 2312 /** 2313 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2314 * <p> 2315 * Description: <b>The subject of the report</b><br> 2316 * Type: <b>reference</b><br> 2317 * Path: <b>DiagnosticReport.subject</b><br> 2318 * </p> 2319 */ 2320 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2321 2322/** 2323 * Constant for fluent queries to be used to add include statements. Specifies 2324 * the path value of "<b>DiagnosticReport:subject</b>". 2325 */ 2326 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:subject").toLocked(); 2327 2328 /** 2329 * Search parameter: <b>diagnosis</b> 2330 * <p> 2331 * Description: <b>A coded diagnosis on the report</b><br> 2332 * Type: <b>token</b><br> 2333 * Path: <b>DiagnosticReport.codedDiagnosis</b><br> 2334 * </p> 2335 */ 2336 @SearchParamDefinition(name="diagnosis", path="DiagnosticReport.codedDiagnosis", description="A coded diagnosis on the report", type="token" ) 2337 public static final String SP_DIAGNOSIS = "diagnosis"; 2338 /** 2339 * <b>Fluent Client</b> search parameter constant for <b>diagnosis</b> 2340 * <p> 2341 * Description: <b>A coded diagnosis on the report</b><br> 2342 * Type: <b>token</b><br> 2343 * Path: <b>DiagnosticReport.codedDiagnosis</b><br> 2344 * </p> 2345 */ 2346 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DIAGNOSIS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DIAGNOSIS); 2347 2348 /** 2349 * Search parameter: <b>encounter</b> 2350 * <p> 2351 * Description: <b>The Encounter when the order was made</b><br> 2352 * Type: <b>reference</b><br> 2353 * Path: <b>DiagnosticReport.context</b><br> 2354 * </p> 2355 */ 2356 @SearchParamDefinition(name="encounter", path="DiagnosticReport.context", description="The Encounter when the order was made", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 2357 public static final String SP_ENCOUNTER = "encounter"; 2358 /** 2359 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2360 * <p> 2361 * Description: <b>The Encounter when the order was made</b><br> 2362 * Type: <b>reference</b><br> 2363 * Path: <b>DiagnosticReport.context</b><br> 2364 * </p> 2365 */ 2366 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2367 2368/** 2369 * Constant for fluent queries to be used to add include statements. Specifies 2370 * the path value of "<b>DiagnosticReport:encounter</b>". 2371 */ 2372 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("DiagnosticReport:encounter").toLocked(); 2373 2374 /** 2375 * Search parameter: <b>result</b> 2376 * <p> 2377 * Description: <b>Link to an atomic result (observation resource)</b><br> 2378 * Type: <b>reference</b><br> 2379 * Path: <b>DiagnosticReport.result</b><br> 2380 * </p> 2381 */ 2382 @SearchParamDefinition(name="result", path="DiagnosticReport.result", description="Link to an atomic result (observation resource)", type="reference", target={Observation.class } ) 2383 public static final String SP_RESULT = "result"; 2384 /** 2385 * <b>Fluent Client</b> search parameter constant for <b>result</b> 2386 * <p> 2387 * Description: <b>Link to an atomic result (observation resource)</b><br> 2388 * Type: <b>reference</b><br> 2389 * Path: <b>DiagnosticReport.result</b><br> 2390 * </p> 2391 */ 2392 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESULT); 2393 2394/** 2395 * Constant for fluent queries to be used to add include statements. Specifies 2396 * the path value of "<b>DiagnosticReport:result</b>". 2397 */ 2398 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:result").toLocked(); 2399 2400 /** 2401 * Search parameter: <b>based-on</b> 2402 * <p> 2403 * Description: <b>Reference to the procedure request.</b><br> 2404 * Type: <b>reference</b><br> 2405 * Path: <b>DiagnosticReport.basedOn</b><br> 2406 * </p> 2407 */ 2408 @SearchParamDefinition(name="based-on", path="DiagnosticReport.basedOn", description="Reference to the procedure request.", type="reference", target={CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ProcedureRequest.class, ReferralRequest.class } ) 2409 public static final String SP_BASED_ON = "based-on"; 2410 /** 2411 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2412 * <p> 2413 * Description: <b>Reference to the procedure request.</b><br> 2414 * Type: <b>reference</b><br> 2415 * Path: <b>DiagnosticReport.basedOn</b><br> 2416 * </p> 2417 */ 2418 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2419 2420/** 2421 * Constant for fluent queries to be used to add include statements. Specifies 2422 * the path value of "<b>DiagnosticReport:based-on</b>". 2423 */ 2424 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("DiagnosticReport:based-on").toLocked(); 2425 2426 /** 2427 * Search parameter: <b>patient</b> 2428 * <p> 2429 * Description: <b>The subject of the report if a patient</b><br> 2430 * Type: <b>reference</b><br> 2431 * Path: <b>DiagnosticReport.subject</b><br> 2432 * </p> 2433 */ 2434 @SearchParamDefinition(name="patient", path="DiagnosticReport.subject", description="The subject of the report if a patient", type="reference", target={Patient.class } ) 2435 public static final String SP_PATIENT = "patient"; 2436 /** 2437 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2438 * <p> 2439 * Description: <b>The subject of the report if a patient</b><br> 2440 * Type: <b>reference</b><br> 2441 * Path: <b>DiagnosticReport.subject</b><br> 2442 * </p> 2443 */ 2444 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2445 2446/** 2447 * Constant for fluent queries to be used to add include statements. Specifies 2448 * the path value of "<b>DiagnosticReport:patient</b>". 2449 */ 2450 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:patient").toLocked(); 2451 2452 /** 2453 * Search parameter: <b>specimen</b> 2454 * <p> 2455 * Description: <b>The specimen details</b><br> 2456 * Type: <b>reference</b><br> 2457 * Path: <b>DiagnosticReport.specimen</b><br> 2458 * </p> 2459 */ 2460 @SearchParamDefinition(name="specimen", path="DiagnosticReport.specimen", description="The specimen details", type="reference", target={Specimen.class } ) 2461 public static final String SP_SPECIMEN = "specimen"; 2462 /** 2463 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 2464 * <p> 2465 * Description: <b>The specimen details</b><br> 2466 * Type: <b>reference</b><br> 2467 * Path: <b>DiagnosticReport.specimen</b><br> 2468 * </p> 2469 */ 2470 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 2471 2472/** 2473 * Constant for fluent queries to be used to add include statements. Specifies 2474 * the path value of "<b>DiagnosticReport:specimen</b>". 2475 */ 2476 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("DiagnosticReport:specimen").toLocked(); 2477 2478 /** 2479 * Search parameter: <b>context</b> 2480 * <p> 2481 * Description: <b>Healthcare event (Episode of Care or Encounter) related to the report</b><br> 2482 * Type: <b>reference</b><br> 2483 * Path: <b>DiagnosticReport.context</b><br> 2484 * </p> 2485 */ 2486 @SearchParamDefinition(name="context", path="DiagnosticReport.context", description="Healthcare event (Episode of Care or Encounter) related to the report", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 2487 public static final String SP_CONTEXT = "context"; 2488 /** 2489 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2490 * <p> 2491 * Description: <b>Healthcare event (Episode of Care or Encounter) related to the report</b><br> 2492 * Type: <b>reference</b><br> 2493 * Path: <b>DiagnosticReport.context</b><br> 2494 * </p> 2495 */ 2496 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2497 2498/** 2499 * Constant for fluent queries to be used to add include statements. Specifies 2500 * the path value of "<b>DiagnosticReport:context</b>". 2501 */ 2502 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:context").toLocked(); 2503 2504 /** 2505 * Search parameter: <b>issued</b> 2506 * <p> 2507 * Description: <b>When the report was issued</b><br> 2508 * Type: <b>date</b><br> 2509 * Path: <b>DiagnosticReport.issued</b><br> 2510 * </p> 2511 */ 2512 @SearchParamDefinition(name="issued", path="DiagnosticReport.issued", description="When the report was issued", type="date" ) 2513 public static final String SP_ISSUED = "issued"; 2514 /** 2515 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 2516 * <p> 2517 * Description: <b>When the report was issued</b><br> 2518 * Type: <b>date</b><br> 2519 * Path: <b>DiagnosticReport.issued</b><br> 2520 * </p> 2521 */ 2522 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 2523 2524 /** 2525 * Search parameter: <b>category</b> 2526 * <p> 2527 * Description: <b>Which diagnostic discipline/department created the report</b><br> 2528 * Type: <b>token</b><br> 2529 * Path: <b>DiagnosticReport.category</b><br> 2530 * </p> 2531 */ 2532 @SearchParamDefinition(name="category", path="DiagnosticReport.category", description="Which diagnostic discipline/department created the report", type="token" ) 2533 public static final String SP_CATEGORY = "category"; 2534 /** 2535 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2536 * <p> 2537 * Description: <b>Which diagnostic discipline/department created the report</b><br> 2538 * Type: <b>token</b><br> 2539 * Path: <b>DiagnosticReport.category</b><br> 2540 * </p> 2541 */ 2542 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2543 2544 /** 2545 * Search parameter: <b>status</b> 2546 * <p> 2547 * Description: <b>The status of the report</b><br> 2548 * Type: <b>token</b><br> 2549 * Path: <b>DiagnosticReport.status</b><br> 2550 * </p> 2551 */ 2552 @SearchParamDefinition(name="status", path="DiagnosticReport.status", description="The status of the report", type="token" ) 2553 public static final String SP_STATUS = "status"; 2554 /** 2555 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2556 * <p> 2557 * Description: <b>The status of the report</b><br> 2558 * Type: <b>token</b><br> 2559 * Path: <b>DiagnosticReport.status</b><br> 2560 * </p> 2561 */ 2562 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2563 2564 2565}