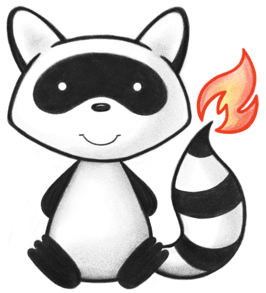
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041/** 042 * A length - a value with a unit that is a physical distance. 043 */ 044@DatatypeDef(name="Distance") 045public class Distance extends Quantity implements ICompositeType { 046 047 private static final long serialVersionUID = 0L; 048 049 /** 050 * Constructor 051 */ 052 public Distance() { 053 super(); 054 } 055 056 protected void listChildren(List<Property> children) { 057 super.listChildren(children); 058 } 059 060 @Override 061 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 062 switch (_hash) { 063 default: return super.getNamedProperty(_hash, _name, _checkValid); 064 } 065 066 } 067 068 @Override 069 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 070 switch (hash) { 071 default: return super.getProperty(hash, name, checkValid); 072 } 073 074 } 075 076 @Override 077 public Base setProperty(int hash, String name, Base value) throws FHIRException { 078 switch (hash) { 079 default: return super.setProperty(hash, name, value); 080 } 081 082 } 083 084 @Override 085 public Base setProperty(String name, Base value) throws FHIRException { 086 return super.setProperty(name, value); 087 } 088 089 @Override 090 public Base makeProperty(int hash, String name) throws FHIRException { 091 switch (hash) { 092 default: return super.makeProperty(hash, name); 093 } 094 095 } 096 097 @Override 098 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 099 switch (hash) { 100 default: return super.getTypesForProperty(hash, name); 101 } 102 103 } 104 105 @Override 106 public Base addChild(String name) throws FHIRException { 107 return super.addChild(name); 108 } 109 110 public String fhirType() { 111 return "Distance"; 112 113 } 114 115 public Distance copy() { 116 Distance dst = new Distance(); 117 copyValues(dst); 118 return dst; 119 } 120 121 protected Distance typedCopy() { 122 return copy(); 123 } 124 125 @Override 126 public boolean equalsDeep(Base other_) { 127 if (!super.equalsDeep(other_)) 128 return false; 129 if (!(other_ instanceof Distance)) 130 return false; 131 Distance o = (Distance) other_; 132 return true; 133 } 134 135 @Override 136 public boolean equalsShallow(Base other_) { 137 if (!super.equalsShallow(other_)) 138 return false; 139 if (!(other_ instanceof Distance)) 140 return false; 141 Distance o = (Distance) other_; 142 return true; 143 } 144 145 public boolean isEmpty() { 146 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(); 147 } 148 149 150}