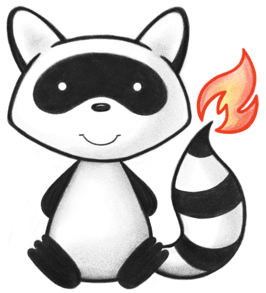
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 053 */ 054@ResourceDef(name="DocumentManifest", profile="http://hl7.org/fhir/Profile/DocumentManifest") 055public class DocumentManifest extends DomainResource { 056 057 @Block() 058 public static class DocumentManifestContentComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed. 061 */ 062 @Child(name = "p", type = {Attachment.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=true) 063 @Description(shortDefinition="Contents of this set of documents", formalDefinition="The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed." ) 064 protected Type p; 065 066 private static final long serialVersionUID = -347538500L; 067 068 /** 069 * Constructor 070 */ 071 public DocumentManifestContentComponent() { 072 super(); 073 } 074 075 /** 076 * Constructor 077 */ 078 public DocumentManifestContentComponent(Type p) { 079 super(); 080 this.p = p; 081 } 082 083 /** 084 * @return {@link #p} (The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.) 085 */ 086 public Type getP() { 087 return this.p; 088 } 089 090 /** 091 * @return {@link #p} (The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.) 092 */ 093 public Attachment getPAttachment() throws FHIRException { 094 if (this.p == null) 095 return null; 096 if (!(this.p instanceof Attachment)) 097 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.p.getClass().getName()+" was encountered"); 098 return (Attachment) this.p; 099 } 100 101 public boolean hasPAttachment() { 102 return this != null && this.p instanceof Attachment; 103 } 104 105 /** 106 * @return {@link #p} (The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.) 107 */ 108 public Reference getPReference() throws FHIRException { 109 if (this.p == null) 110 return null; 111 if (!(this.p instanceof Reference)) 112 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.p.getClass().getName()+" was encountered"); 113 return (Reference) this.p; 114 } 115 116 public boolean hasPReference() { 117 return this != null && this.p instanceof Reference; 118 } 119 120 public boolean hasP() { 121 return this.p != null && !this.p.isEmpty(); 122 } 123 124 /** 125 * @param value {@link #p} (The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.) 126 */ 127 public DocumentManifestContentComponent setP(Type value) throws FHIRFormatError { 128 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 129 throw new FHIRFormatError("Not the right type for DocumentManifest.content.p[x]: "+value.fhirType()); 130 this.p = value; 131 return this; 132 } 133 134 protected void listChildren(List<Property> children) { 135 super.listChildren(children); 136 children.add(new Property("p[x]", "Attachment|Reference(Any)", "The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.", 0, 1, p)); 137 } 138 139 @Override 140 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 141 switch (_hash) { 142 case 3427856: /*p[x]*/ return new Property("p[x]", "Attachment|Reference(Any)", "The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.", 0, 1, p); 143 case 112: /*p*/ return new Property("p[x]", "Attachment|Reference(Any)", "The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.", 0, 1, p); 144 case 634874291: /*pAttachment*/ return new Property("p[x]", "Attachment|Reference(Any)", "The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.", 0, 1, p); 145 case 544136379: /*pReference*/ return new Property("p[x]", "Attachment|Reference(Any)", "The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.", 0, 1, p); 146 default: return super.getNamedProperty(_hash, _name, _checkValid); 147 } 148 149 } 150 151 @Override 152 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 153 switch (hash) { 154 case 112: /*p*/ return this.p == null ? new Base[0] : new Base[] {this.p}; // Type 155 default: return super.getProperty(hash, name, checkValid); 156 } 157 158 } 159 160 @Override 161 public Base setProperty(int hash, String name, Base value) throws FHIRException { 162 switch (hash) { 163 case 112: // p 164 this.p = castToType(value); // Type 165 return value; 166 default: return super.setProperty(hash, name, value); 167 } 168 169 } 170 171 @Override 172 public Base setProperty(String name, Base value) throws FHIRException { 173 if (name.equals("p[x]")) { 174 this.p = castToType(value); // Type 175 } else 176 return super.setProperty(name, value); 177 return value; 178 } 179 180 @Override 181 public Base makeProperty(int hash, String name) throws FHIRException { 182 switch (hash) { 183 case 3427856: return getP(); 184 case 112: return getP(); 185 default: return super.makeProperty(hash, name); 186 } 187 188 } 189 190 @Override 191 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 192 switch (hash) { 193 case 112: /*p*/ return new String[] {"Attachment", "Reference"}; 194 default: return super.getTypesForProperty(hash, name); 195 } 196 197 } 198 199 @Override 200 public Base addChild(String name) throws FHIRException { 201 if (name.equals("pAttachment")) { 202 this.p = new Attachment(); 203 return this.p; 204 } 205 else if (name.equals("pReference")) { 206 this.p = new Reference(); 207 return this.p; 208 } 209 else 210 return super.addChild(name); 211 } 212 213 public DocumentManifestContentComponent copy() { 214 DocumentManifestContentComponent dst = new DocumentManifestContentComponent(); 215 copyValues(dst); 216 dst.p = p == null ? null : p.copy(); 217 return dst; 218 } 219 220 @Override 221 public boolean equalsDeep(Base other_) { 222 if (!super.equalsDeep(other_)) 223 return false; 224 if (!(other_ instanceof DocumentManifestContentComponent)) 225 return false; 226 DocumentManifestContentComponent o = (DocumentManifestContentComponent) other_; 227 return compareDeep(p, o.p, true); 228 } 229 230 @Override 231 public boolean equalsShallow(Base other_) { 232 if (!super.equalsShallow(other_)) 233 return false; 234 if (!(other_ instanceof DocumentManifestContentComponent)) 235 return false; 236 DocumentManifestContentComponent o = (DocumentManifestContentComponent) other_; 237 return true; 238 } 239 240 public boolean isEmpty() { 241 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(p); 242 } 243 244 public String fhirType() { 245 return "DocumentManifest.content"; 246 247 } 248 249 } 250 251 @Block() 252 public static class DocumentManifestRelatedComponent extends BackboneElement implements IBaseBackboneElement { 253 /** 254 * Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers. 255 */ 256 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 257 @Description(shortDefinition="Identifiers of things that are related", formalDefinition="Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers." ) 258 protected Identifier identifier; 259 260 /** 261 * Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc. 262 */ 263 @Child(name = "ref", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=true) 264 @Description(shortDefinition="Related Resource", formalDefinition="Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc." ) 265 protected Reference ref; 266 267 /** 268 * The actual object that is the target of the reference (Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc.) 269 */ 270 protected Resource refTarget; 271 272 private static final long serialVersionUID = -1670123330L; 273 274 /** 275 * Constructor 276 */ 277 public DocumentManifestRelatedComponent() { 278 super(); 279 } 280 281 /** 282 * @return {@link #identifier} (Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.) 283 */ 284 public Identifier getIdentifier() { 285 if (this.identifier == null) 286 if (Configuration.errorOnAutoCreate()) 287 throw new Error("Attempt to auto-create DocumentManifestRelatedComponent.identifier"); 288 else if (Configuration.doAutoCreate()) 289 this.identifier = new Identifier(); // cc 290 return this.identifier; 291 } 292 293 public boolean hasIdentifier() { 294 return this.identifier != null && !this.identifier.isEmpty(); 295 } 296 297 /** 298 * @param value {@link #identifier} (Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.) 299 */ 300 public DocumentManifestRelatedComponent setIdentifier(Identifier value) { 301 this.identifier = value; 302 return this; 303 } 304 305 /** 306 * @return {@link #ref} (Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc.) 307 */ 308 public Reference getRef() { 309 if (this.ref == null) 310 if (Configuration.errorOnAutoCreate()) 311 throw new Error("Attempt to auto-create DocumentManifestRelatedComponent.ref"); 312 else if (Configuration.doAutoCreate()) 313 this.ref = new Reference(); // cc 314 return this.ref; 315 } 316 317 public boolean hasRef() { 318 return this.ref != null && !this.ref.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #ref} (Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc.) 323 */ 324 public DocumentManifestRelatedComponent setRef(Reference value) { 325 this.ref = value; 326 return this; 327 } 328 329 /** 330 * @return {@link #ref} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc.) 331 */ 332 public Resource getRefTarget() { 333 return this.refTarget; 334 } 335 336 /** 337 * @param value {@link #ref} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc.) 338 */ 339 public DocumentManifestRelatedComponent setRefTarget(Resource value) { 340 this.refTarget = value; 341 return this; 342 } 343 344 protected void listChildren(List<Property> children) { 345 super.listChildren(children); 346 children.add(new Property("identifier", "Identifier", "Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.", 0, 1, identifier)); 347 children.add(new Property("ref", "Reference(Any)", "Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc.", 0, 1, ref)); 348 } 349 350 @Override 351 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 352 switch (_hash) { 353 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.", 0, 1, identifier); 354 case 112787: /*ref*/ return new Property("ref", "Reference(Any)", "Related Resource to this DocumentManifest. For example, Order, ProcedureRequest, Procedure, EligibilityRequest, etc.", 0, 1, ref); 355 default: return super.getNamedProperty(_hash, _name, _checkValid); 356 } 357 358 } 359 360 @Override 361 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 362 switch (hash) { 363 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 364 case 112787: /*ref*/ return this.ref == null ? new Base[0] : new Base[] {this.ref}; // Reference 365 default: return super.getProperty(hash, name, checkValid); 366 } 367 368 } 369 370 @Override 371 public Base setProperty(int hash, String name, Base value) throws FHIRException { 372 switch (hash) { 373 case -1618432855: // identifier 374 this.identifier = castToIdentifier(value); // Identifier 375 return value; 376 case 112787: // ref 377 this.ref = castToReference(value); // Reference 378 return value; 379 default: return super.setProperty(hash, name, value); 380 } 381 382 } 383 384 @Override 385 public Base setProperty(String name, Base value) throws FHIRException { 386 if (name.equals("identifier")) { 387 this.identifier = castToIdentifier(value); // Identifier 388 } else if (name.equals("ref")) { 389 this.ref = castToReference(value); // Reference 390 } else 391 return super.setProperty(name, value); 392 return value; 393 } 394 395 @Override 396 public Base makeProperty(int hash, String name) throws FHIRException { 397 switch (hash) { 398 case -1618432855: return getIdentifier(); 399 case 112787: return getRef(); 400 default: return super.makeProperty(hash, name); 401 } 402 403 } 404 405 @Override 406 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 407 switch (hash) { 408 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 409 case 112787: /*ref*/ return new String[] {"Reference"}; 410 default: return super.getTypesForProperty(hash, name); 411 } 412 413 } 414 415 @Override 416 public Base addChild(String name) throws FHIRException { 417 if (name.equals("identifier")) { 418 this.identifier = new Identifier(); 419 return this.identifier; 420 } 421 else if (name.equals("ref")) { 422 this.ref = new Reference(); 423 return this.ref; 424 } 425 else 426 return super.addChild(name); 427 } 428 429 public DocumentManifestRelatedComponent copy() { 430 DocumentManifestRelatedComponent dst = new DocumentManifestRelatedComponent(); 431 copyValues(dst); 432 dst.identifier = identifier == null ? null : identifier.copy(); 433 dst.ref = ref == null ? null : ref.copy(); 434 return dst; 435 } 436 437 @Override 438 public boolean equalsDeep(Base other_) { 439 if (!super.equalsDeep(other_)) 440 return false; 441 if (!(other_ instanceof DocumentManifestRelatedComponent)) 442 return false; 443 DocumentManifestRelatedComponent o = (DocumentManifestRelatedComponent) other_; 444 return compareDeep(identifier, o.identifier, true) && compareDeep(ref, o.ref, true); 445 } 446 447 @Override 448 public boolean equalsShallow(Base other_) { 449 if (!super.equalsShallow(other_)) 450 return false; 451 if (!(other_ instanceof DocumentManifestRelatedComponent)) 452 return false; 453 DocumentManifestRelatedComponent o = (DocumentManifestRelatedComponent) other_; 454 return true; 455 } 456 457 public boolean isEmpty() { 458 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, ref); 459 } 460 461 public String fhirType() { 462 return "DocumentManifest.related"; 463 464 } 465 466 } 467 468 /** 469 * A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts. 470 */ 471 @Child(name = "masterIdentifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 472 @Description(shortDefinition="Unique Identifier for the set of documents", formalDefinition="A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts." ) 473 protected Identifier masterIdentifier; 474 475 /** 476 * Other identifiers associated with the document manifest, including version independent identifiers. 477 */ 478 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 479 @Description(shortDefinition="Other identifiers for the manifest", formalDefinition="Other identifiers associated with the document manifest, including version independent identifiers." ) 480 protected List<Identifier> identifier; 481 482 /** 483 * The status of this document manifest. 484 */ 485 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 486 @Description(shortDefinition="current | superseded | entered-in-error", formalDefinition="The status of this document manifest." ) 487 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-reference-status") 488 protected Enumeration<DocumentReferenceStatus> status; 489 490 /** 491 * Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider. 492 */ 493 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 494 @Description(shortDefinition="Kind of document set", formalDefinition="Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider." ) 495 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-doc-typecodes") 496 protected CodeableConcept type; 497 498 /** 499 * Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case). 500 */ 501 @Child(name = "subject", type = {Patient.class, Practitioner.class, Group.class, Device.class}, order=4, min=0, max=1, modifier=false, summary=true) 502 @Description(shortDefinition="The subject of the set of documents", formalDefinition="Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case)." ) 503 protected Reference subject; 504 505 /** 506 * The actual object that is the target of the reference (Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).) 507 */ 508 protected Resource subjectTarget; 509 510 /** 511 * When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.). 512 */ 513 @Child(name = "created", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 514 @Description(shortDefinition="When this document manifest created", formalDefinition="When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.)." ) 515 protected DateTimeType created; 516 517 /** 518 * Identifies who is responsible for creating the manifest, and adding documents to it. 519 */ 520 @Child(name = "author", type = {Practitioner.class, Organization.class, Device.class, Patient.class, RelatedPerson.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 521 @Description(shortDefinition="Who and/or what authored the manifest", formalDefinition="Identifies who is responsible for creating the manifest, and adding documents to it." ) 522 protected List<Reference> author; 523 /** 524 * The actual objects that are the target of the reference (Identifies who is responsible for creating the manifest, and adding documents to it.) 525 */ 526 protected List<Resource> authorTarget; 527 528 529 /** 530 * A patient, practitioner, or organization for which this set of documents is intended. 531 */ 532 @Child(name = "recipient", type = {Patient.class, Practitioner.class, RelatedPerson.class, Organization.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 533 @Description(shortDefinition="Intended to get notified about this set of documents", formalDefinition="A patient, practitioner, or organization for which this set of documents is intended." ) 534 protected List<Reference> recipient; 535 /** 536 * The actual objects that are the target of the reference (A patient, practitioner, or organization for which this set of documents is intended.) 537 */ 538 protected List<Resource> recipientTarget; 539 540 541 /** 542 * Identifies the source system, application, or software that produced the document manifest. 543 */ 544 @Child(name = "source", type = {UriType.class}, order=8, min=0, max=1, modifier=false, summary=true) 545 @Description(shortDefinition="The source system/application/software", formalDefinition="Identifies the source system, application, or software that produced the document manifest." ) 546 protected UriType source; 547 548 /** 549 * Human-readable description of the source document. This is sometimes known as the "title". 550 */ 551 @Child(name = "description", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 552 @Description(shortDefinition="Human-readable description (title)", formalDefinition="Human-readable description of the source document. This is sometimes known as the \"title\"." ) 553 protected StringType description; 554 555 /** 556 * The list of Documents included in the manifest. 557 */ 558 @Child(name = "content", type = {}, order=10, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 559 @Description(shortDefinition="The items included", formalDefinition="The list of Documents included in the manifest." ) 560 protected List<DocumentManifestContentComponent> content; 561 562 /** 563 * Related identifiers or resources associated with the DocumentManifest. 564 */ 565 @Child(name = "related", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 566 @Description(shortDefinition="Related things", formalDefinition="Related identifiers or resources associated with the DocumentManifest." ) 567 protected List<DocumentManifestRelatedComponent> related; 568 569 private static final long serialVersionUID = -2081196115L; 570 571 /** 572 * Constructor 573 */ 574 public DocumentManifest() { 575 super(); 576 } 577 578 /** 579 * Constructor 580 */ 581 public DocumentManifest(Enumeration<DocumentReferenceStatus> status) { 582 super(); 583 this.status = status; 584 } 585 586 /** 587 * @return {@link #masterIdentifier} (A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.) 588 */ 589 public Identifier getMasterIdentifier() { 590 if (this.masterIdentifier == null) 591 if (Configuration.errorOnAutoCreate()) 592 throw new Error("Attempt to auto-create DocumentManifest.masterIdentifier"); 593 else if (Configuration.doAutoCreate()) 594 this.masterIdentifier = new Identifier(); // cc 595 return this.masterIdentifier; 596 } 597 598 public boolean hasMasterIdentifier() { 599 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 600 } 601 602 /** 603 * @param value {@link #masterIdentifier} (A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.) 604 */ 605 public DocumentManifest setMasterIdentifier(Identifier value) { 606 this.masterIdentifier = value; 607 return this; 608 } 609 610 /** 611 * @return {@link #identifier} (Other identifiers associated with the document manifest, including version independent identifiers.) 612 */ 613 public List<Identifier> getIdentifier() { 614 if (this.identifier == null) 615 this.identifier = new ArrayList<Identifier>(); 616 return this.identifier; 617 } 618 619 /** 620 * @return Returns a reference to <code>this</code> for easy method chaining 621 */ 622 public DocumentManifest setIdentifier(List<Identifier> theIdentifier) { 623 this.identifier = theIdentifier; 624 return this; 625 } 626 627 public boolean hasIdentifier() { 628 if (this.identifier == null) 629 return false; 630 for (Identifier item : this.identifier) 631 if (!item.isEmpty()) 632 return true; 633 return false; 634 } 635 636 public Identifier addIdentifier() { //3 637 Identifier t = new Identifier(); 638 if (this.identifier == null) 639 this.identifier = new ArrayList<Identifier>(); 640 this.identifier.add(t); 641 return t; 642 } 643 644 public DocumentManifest addIdentifier(Identifier t) { //3 645 if (t == null) 646 return this; 647 if (this.identifier == null) 648 this.identifier = new ArrayList<Identifier>(); 649 this.identifier.add(t); 650 return this; 651 } 652 653 /** 654 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 655 */ 656 public Identifier getIdentifierFirstRep() { 657 if (getIdentifier().isEmpty()) { 658 addIdentifier(); 659 } 660 return getIdentifier().get(0); 661 } 662 663 /** 664 * @return {@link #status} (The status of this document manifest.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 665 */ 666 public Enumeration<DocumentReferenceStatus> getStatusElement() { 667 if (this.status == null) 668 if (Configuration.errorOnAutoCreate()) 669 throw new Error("Attempt to auto-create DocumentManifest.status"); 670 else if (Configuration.doAutoCreate()) 671 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 672 return this.status; 673 } 674 675 public boolean hasStatusElement() { 676 return this.status != null && !this.status.isEmpty(); 677 } 678 679 public boolean hasStatus() { 680 return this.status != null && !this.status.isEmpty(); 681 } 682 683 /** 684 * @param value {@link #status} (The status of this document manifest.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 685 */ 686 public DocumentManifest setStatusElement(Enumeration<DocumentReferenceStatus> value) { 687 this.status = value; 688 return this; 689 } 690 691 /** 692 * @return The status of this document manifest. 693 */ 694 public DocumentReferenceStatus getStatus() { 695 return this.status == null ? null : this.status.getValue(); 696 } 697 698 /** 699 * @param value The status of this document manifest. 700 */ 701 public DocumentManifest setStatus(DocumentReferenceStatus value) { 702 if (this.status == null) 703 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 704 this.status.setValue(value); 705 return this; 706 } 707 708 /** 709 * @return {@link #type} (Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider.) 710 */ 711 public CodeableConcept getType() { 712 if (this.type == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create DocumentManifest.type"); 715 else if (Configuration.doAutoCreate()) 716 this.type = new CodeableConcept(); // cc 717 return this.type; 718 } 719 720 public boolean hasType() { 721 return this.type != null && !this.type.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #type} (Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider.) 726 */ 727 public DocumentManifest setType(CodeableConcept value) { 728 this.type = value; 729 return this; 730 } 731 732 /** 733 * @return {@link #subject} (Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).) 734 */ 735 public Reference getSubject() { 736 if (this.subject == null) 737 if (Configuration.errorOnAutoCreate()) 738 throw new Error("Attempt to auto-create DocumentManifest.subject"); 739 else if (Configuration.doAutoCreate()) 740 this.subject = new Reference(); // cc 741 return this.subject; 742 } 743 744 public boolean hasSubject() { 745 return this.subject != null && !this.subject.isEmpty(); 746 } 747 748 /** 749 * @param value {@link #subject} (Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).) 750 */ 751 public DocumentManifest setSubject(Reference value) { 752 this.subject = value; 753 return this; 754 } 755 756 /** 757 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).) 758 */ 759 public Resource getSubjectTarget() { 760 return this.subjectTarget; 761 } 762 763 /** 764 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).) 765 */ 766 public DocumentManifest setSubjectTarget(Resource value) { 767 this.subjectTarget = value; 768 return this; 769 } 770 771 /** 772 * @return {@link #created} (When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 773 */ 774 public DateTimeType getCreatedElement() { 775 if (this.created == null) 776 if (Configuration.errorOnAutoCreate()) 777 throw new Error("Attempt to auto-create DocumentManifest.created"); 778 else if (Configuration.doAutoCreate()) 779 this.created = new DateTimeType(); // bb 780 return this.created; 781 } 782 783 public boolean hasCreatedElement() { 784 return this.created != null && !this.created.isEmpty(); 785 } 786 787 public boolean hasCreated() { 788 return this.created != null && !this.created.isEmpty(); 789 } 790 791 /** 792 * @param value {@link #created} (When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 793 */ 794 public DocumentManifest setCreatedElement(DateTimeType value) { 795 this.created = value; 796 return this; 797 } 798 799 /** 800 * @return When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.). 801 */ 802 public Date getCreated() { 803 return this.created == null ? null : this.created.getValue(); 804 } 805 806 /** 807 * @param value When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.). 808 */ 809 public DocumentManifest setCreated(Date value) { 810 if (value == null) 811 this.created = null; 812 else { 813 if (this.created == null) 814 this.created = new DateTimeType(); 815 this.created.setValue(value); 816 } 817 return this; 818 } 819 820 /** 821 * @return {@link #author} (Identifies who is responsible for creating the manifest, and adding documents to it.) 822 */ 823 public List<Reference> getAuthor() { 824 if (this.author == null) 825 this.author = new ArrayList<Reference>(); 826 return this.author; 827 } 828 829 /** 830 * @return Returns a reference to <code>this</code> for easy method chaining 831 */ 832 public DocumentManifest setAuthor(List<Reference> theAuthor) { 833 this.author = theAuthor; 834 return this; 835 } 836 837 public boolean hasAuthor() { 838 if (this.author == null) 839 return false; 840 for (Reference item : this.author) 841 if (!item.isEmpty()) 842 return true; 843 return false; 844 } 845 846 public Reference addAuthor() { //3 847 Reference t = new Reference(); 848 if (this.author == null) 849 this.author = new ArrayList<Reference>(); 850 this.author.add(t); 851 return t; 852 } 853 854 public DocumentManifest addAuthor(Reference t) { //3 855 if (t == null) 856 return this; 857 if (this.author == null) 858 this.author = new ArrayList<Reference>(); 859 this.author.add(t); 860 return this; 861 } 862 863 /** 864 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist 865 */ 866 public Reference getAuthorFirstRep() { 867 if (getAuthor().isEmpty()) { 868 addAuthor(); 869 } 870 return getAuthor().get(0); 871 } 872 873 /** 874 * @deprecated Use Reference#setResource(IBaseResource) instead 875 */ 876 @Deprecated 877 public List<Resource> getAuthorTarget() { 878 if (this.authorTarget == null) 879 this.authorTarget = new ArrayList<Resource>(); 880 return this.authorTarget; 881 } 882 883 /** 884 * @return {@link #recipient} (A patient, practitioner, or organization for which this set of documents is intended.) 885 */ 886 public List<Reference> getRecipient() { 887 if (this.recipient == null) 888 this.recipient = new ArrayList<Reference>(); 889 return this.recipient; 890 } 891 892 /** 893 * @return Returns a reference to <code>this</code> for easy method chaining 894 */ 895 public DocumentManifest setRecipient(List<Reference> theRecipient) { 896 this.recipient = theRecipient; 897 return this; 898 } 899 900 public boolean hasRecipient() { 901 if (this.recipient == null) 902 return false; 903 for (Reference item : this.recipient) 904 if (!item.isEmpty()) 905 return true; 906 return false; 907 } 908 909 public Reference addRecipient() { //3 910 Reference t = new Reference(); 911 if (this.recipient == null) 912 this.recipient = new ArrayList<Reference>(); 913 this.recipient.add(t); 914 return t; 915 } 916 917 public DocumentManifest addRecipient(Reference t) { //3 918 if (t == null) 919 return this; 920 if (this.recipient == null) 921 this.recipient = new ArrayList<Reference>(); 922 this.recipient.add(t); 923 return this; 924 } 925 926 /** 927 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist 928 */ 929 public Reference getRecipientFirstRep() { 930 if (getRecipient().isEmpty()) { 931 addRecipient(); 932 } 933 return getRecipient().get(0); 934 } 935 936 /** 937 * @deprecated Use Reference#setResource(IBaseResource) instead 938 */ 939 @Deprecated 940 public List<Resource> getRecipientTarget() { 941 if (this.recipientTarget == null) 942 this.recipientTarget = new ArrayList<Resource>(); 943 return this.recipientTarget; 944 } 945 946 /** 947 * @return {@link #source} (Identifies the source system, application, or software that produced the document manifest.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 948 */ 949 public UriType getSourceElement() { 950 if (this.source == null) 951 if (Configuration.errorOnAutoCreate()) 952 throw new Error("Attempt to auto-create DocumentManifest.source"); 953 else if (Configuration.doAutoCreate()) 954 this.source = new UriType(); // bb 955 return this.source; 956 } 957 958 public boolean hasSourceElement() { 959 return this.source != null && !this.source.isEmpty(); 960 } 961 962 public boolean hasSource() { 963 return this.source != null && !this.source.isEmpty(); 964 } 965 966 /** 967 * @param value {@link #source} (Identifies the source system, application, or software that produced the document manifest.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 968 */ 969 public DocumentManifest setSourceElement(UriType value) { 970 this.source = value; 971 return this; 972 } 973 974 /** 975 * @return Identifies the source system, application, or software that produced the document manifest. 976 */ 977 public String getSource() { 978 return this.source == null ? null : this.source.getValue(); 979 } 980 981 /** 982 * @param value Identifies the source system, application, or software that produced the document manifest. 983 */ 984 public DocumentManifest setSource(String value) { 985 if (Utilities.noString(value)) 986 this.source = null; 987 else { 988 if (this.source == null) 989 this.source = new UriType(); 990 this.source.setValue(value); 991 } 992 return this; 993 } 994 995 /** 996 * @return {@link #description} (Human-readable description of the source document. This is sometimes known as the "title".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 997 */ 998 public StringType getDescriptionElement() { 999 if (this.description == null) 1000 if (Configuration.errorOnAutoCreate()) 1001 throw new Error("Attempt to auto-create DocumentManifest.description"); 1002 else if (Configuration.doAutoCreate()) 1003 this.description = new StringType(); // bb 1004 return this.description; 1005 } 1006 1007 public boolean hasDescriptionElement() { 1008 return this.description != null && !this.description.isEmpty(); 1009 } 1010 1011 public boolean hasDescription() { 1012 return this.description != null && !this.description.isEmpty(); 1013 } 1014 1015 /** 1016 * @param value {@link #description} (Human-readable description of the source document. This is sometimes known as the "title".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1017 */ 1018 public DocumentManifest setDescriptionElement(StringType value) { 1019 this.description = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return Human-readable description of the source document. This is sometimes known as the "title". 1025 */ 1026 public String getDescription() { 1027 return this.description == null ? null : this.description.getValue(); 1028 } 1029 1030 /** 1031 * @param value Human-readable description of the source document. This is sometimes known as the "title". 1032 */ 1033 public DocumentManifest setDescription(String value) { 1034 if (Utilities.noString(value)) 1035 this.description = null; 1036 else { 1037 if (this.description == null) 1038 this.description = new StringType(); 1039 this.description.setValue(value); 1040 } 1041 return this; 1042 } 1043 1044 /** 1045 * @return {@link #content} (The list of Documents included in the manifest.) 1046 */ 1047 public List<DocumentManifestContentComponent> getContent() { 1048 if (this.content == null) 1049 this.content = new ArrayList<DocumentManifestContentComponent>(); 1050 return this.content; 1051 } 1052 1053 /** 1054 * @return Returns a reference to <code>this</code> for easy method chaining 1055 */ 1056 public DocumentManifest setContent(List<DocumentManifestContentComponent> theContent) { 1057 this.content = theContent; 1058 return this; 1059 } 1060 1061 public boolean hasContent() { 1062 if (this.content == null) 1063 return false; 1064 for (DocumentManifestContentComponent item : this.content) 1065 if (!item.isEmpty()) 1066 return true; 1067 return false; 1068 } 1069 1070 public DocumentManifestContentComponent addContent() { //3 1071 DocumentManifestContentComponent t = new DocumentManifestContentComponent(); 1072 if (this.content == null) 1073 this.content = new ArrayList<DocumentManifestContentComponent>(); 1074 this.content.add(t); 1075 return t; 1076 } 1077 1078 public DocumentManifest addContent(DocumentManifestContentComponent t) { //3 1079 if (t == null) 1080 return this; 1081 if (this.content == null) 1082 this.content = new ArrayList<DocumentManifestContentComponent>(); 1083 this.content.add(t); 1084 return this; 1085 } 1086 1087 /** 1088 * @return The first repetition of repeating field {@link #content}, creating it if it does not already exist 1089 */ 1090 public DocumentManifestContentComponent getContentFirstRep() { 1091 if (getContent().isEmpty()) { 1092 addContent(); 1093 } 1094 return getContent().get(0); 1095 } 1096 1097 /** 1098 * @return {@link #related} (Related identifiers or resources associated with the DocumentManifest.) 1099 */ 1100 public List<DocumentManifestRelatedComponent> getRelated() { 1101 if (this.related == null) 1102 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1103 return this.related; 1104 } 1105 1106 /** 1107 * @return Returns a reference to <code>this</code> for easy method chaining 1108 */ 1109 public DocumentManifest setRelated(List<DocumentManifestRelatedComponent> theRelated) { 1110 this.related = theRelated; 1111 return this; 1112 } 1113 1114 public boolean hasRelated() { 1115 if (this.related == null) 1116 return false; 1117 for (DocumentManifestRelatedComponent item : this.related) 1118 if (!item.isEmpty()) 1119 return true; 1120 return false; 1121 } 1122 1123 public DocumentManifestRelatedComponent addRelated() { //3 1124 DocumentManifestRelatedComponent t = new DocumentManifestRelatedComponent(); 1125 if (this.related == null) 1126 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1127 this.related.add(t); 1128 return t; 1129 } 1130 1131 public DocumentManifest addRelated(DocumentManifestRelatedComponent t) { //3 1132 if (t == null) 1133 return this; 1134 if (this.related == null) 1135 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1136 this.related.add(t); 1137 return this; 1138 } 1139 1140 /** 1141 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist 1142 */ 1143 public DocumentManifestRelatedComponent getRelatedFirstRep() { 1144 if (getRelated().isEmpty()) { 1145 addRelated(); 1146 } 1147 return getRelated().get(0); 1148 } 1149 1150 protected void listChildren(List<Property> children) { 1151 super.listChildren(children); 1152 children.add(new Property("masterIdentifier", "Identifier", "A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.", 0, 1, masterIdentifier)); 1153 children.add(new Property("identifier", "Identifier", "Other identifiers associated with the document manifest, including version independent identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1154 children.add(new Property("status", "code", "The status of this document manifest.", 0, 1, status)); 1155 children.add(new Property("type", "CodeableConcept", "Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider.", 0, 1, type)); 1156 children.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", "Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).", 0, 1, subject)); 1157 children.add(new Property("created", "dateTime", "When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).", 0, 1, created)); 1158 children.add(new Property("author", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", "Identifies who is responsible for creating the manifest, and adding documents to it.", 0, java.lang.Integer.MAX_VALUE, author)); 1159 children.add(new Property("recipient", "Reference(Patient|Practitioner|RelatedPerson|Organization)", "A patient, practitioner, or organization for which this set of documents is intended.", 0, java.lang.Integer.MAX_VALUE, recipient)); 1160 children.add(new Property("source", "uri", "Identifies the source system, application, or software that produced the document manifest.", 0, 1, source)); 1161 children.add(new Property("description", "string", "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 1, description)); 1162 children.add(new Property("content", "", "The list of Documents included in the manifest.", 0, java.lang.Integer.MAX_VALUE, content)); 1163 children.add(new Property("related", "", "Related identifiers or resources associated with the DocumentManifest.", 0, java.lang.Integer.MAX_VALUE, related)); 1164 } 1165 1166 @Override 1167 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1168 switch (_hash) { 1169 case 243769515: /*masterIdentifier*/ return new Property("masterIdentifier", "Identifier", "A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.", 0, 1, masterIdentifier); 1170 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Other identifiers associated with the document manifest, including version independent identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier); 1171 case -892481550: /*status*/ return new Property("status", "code", "The status of this document manifest.", 0, 1, status); 1172 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider.", 0, 1, type); 1173 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Practitioner|Group|Device)", "Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).", 0, 1, subject); 1174 case 1028554472: /*created*/ return new Property("created", "dateTime", "When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).", 0, 1, created); 1175 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", "Identifies who is responsible for creating the manifest, and adding documents to it.", 0, java.lang.Integer.MAX_VALUE, author); 1176 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Patient|Practitioner|RelatedPerson|Organization)", "A patient, practitioner, or organization for which this set of documents is intended.", 0, java.lang.Integer.MAX_VALUE, recipient); 1177 case -896505829: /*source*/ return new Property("source", "uri", "Identifies the source system, application, or software that produced the document manifest.", 0, 1, source); 1178 case -1724546052: /*description*/ return new Property("description", "string", "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 1, description); 1179 case 951530617: /*content*/ return new Property("content", "", "The list of Documents included in the manifest.", 0, java.lang.Integer.MAX_VALUE, content); 1180 case 1090493483: /*related*/ return new Property("related", "", "Related identifiers or resources associated with the DocumentManifest.", 0, java.lang.Integer.MAX_VALUE, related); 1181 default: return super.getNamedProperty(_hash, _name, _checkValid); 1182 } 1183 1184 } 1185 1186 @Override 1187 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1188 switch (hash) { 1189 case 243769515: /*masterIdentifier*/ return this.masterIdentifier == null ? new Base[0] : new Base[] {this.masterIdentifier}; // Identifier 1190 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1191 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DocumentReferenceStatus> 1192 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1193 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1194 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 1195 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 1196 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1197 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // UriType 1198 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1199 case 951530617: /*content*/ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // DocumentManifestContentComponent 1200 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // DocumentManifestRelatedComponent 1201 default: return super.getProperty(hash, name, checkValid); 1202 } 1203 1204 } 1205 1206 @Override 1207 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1208 switch (hash) { 1209 case 243769515: // masterIdentifier 1210 this.masterIdentifier = castToIdentifier(value); // Identifier 1211 return value; 1212 case -1618432855: // identifier 1213 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1214 return value; 1215 case -892481550: // status 1216 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 1217 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 1218 return value; 1219 case 3575610: // type 1220 this.type = castToCodeableConcept(value); // CodeableConcept 1221 return value; 1222 case -1867885268: // subject 1223 this.subject = castToReference(value); // Reference 1224 return value; 1225 case 1028554472: // created 1226 this.created = castToDateTime(value); // DateTimeType 1227 return value; 1228 case -1406328437: // author 1229 this.getAuthor().add(castToReference(value)); // Reference 1230 return value; 1231 case 820081177: // recipient 1232 this.getRecipient().add(castToReference(value)); // Reference 1233 return value; 1234 case -896505829: // source 1235 this.source = castToUri(value); // UriType 1236 return value; 1237 case -1724546052: // description 1238 this.description = castToString(value); // StringType 1239 return value; 1240 case 951530617: // content 1241 this.getContent().add((DocumentManifestContentComponent) value); // DocumentManifestContentComponent 1242 return value; 1243 case 1090493483: // related 1244 this.getRelated().add((DocumentManifestRelatedComponent) value); // DocumentManifestRelatedComponent 1245 return value; 1246 default: return super.setProperty(hash, name, value); 1247 } 1248 1249 } 1250 1251 @Override 1252 public Base setProperty(String name, Base value) throws FHIRException { 1253 if (name.equals("masterIdentifier")) { 1254 this.masterIdentifier = castToIdentifier(value); // Identifier 1255 } else if (name.equals("identifier")) { 1256 this.getIdentifier().add(castToIdentifier(value)); 1257 } else if (name.equals("status")) { 1258 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 1259 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 1260 } else if (name.equals("type")) { 1261 this.type = castToCodeableConcept(value); // CodeableConcept 1262 } else if (name.equals("subject")) { 1263 this.subject = castToReference(value); // Reference 1264 } else if (name.equals("created")) { 1265 this.created = castToDateTime(value); // DateTimeType 1266 } else if (name.equals("author")) { 1267 this.getAuthor().add(castToReference(value)); 1268 } else if (name.equals("recipient")) { 1269 this.getRecipient().add(castToReference(value)); 1270 } else if (name.equals("source")) { 1271 this.source = castToUri(value); // UriType 1272 } else if (name.equals("description")) { 1273 this.description = castToString(value); // StringType 1274 } else if (name.equals("content")) { 1275 this.getContent().add((DocumentManifestContentComponent) value); 1276 } else if (name.equals("related")) { 1277 this.getRelated().add((DocumentManifestRelatedComponent) value); 1278 } else 1279 return super.setProperty(name, value); 1280 return value; 1281 } 1282 1283 @Override 1284 public Base makeProperty(int hash, String name) throws FHIRException { 1285 switch (hash) { 1286 case 243769515: return getMasterIdentifier(); 1287 case -1618432855: return addIdentifier(); 1288 case -892481550: return getStatusElement(); 1289 case 3575610: return getType(); 1290 case -1867885268: return getSubject(); 1291 case 1028554472: return getCreatedElement(); 1292 case -1406328437: return addAuthor(); 1293 case 820081177: return addRecipient(); 1294 case -896505829: return getSourceElement(); 1295 case -1724546052: return getDescriptionElement(); 1296 case 951530617: return addContent(); 1297 case 1090493483: return addRelated(); 1298 default: return super.makeProperty(hash, name); 1299 } 1300 1301 } 1302 1303 @Override 1304 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1305 switch (hash) { 1306 case 243769515: /*masterIdentifier*/ return new String[] {"Identifier"}; 1307 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1308 case -892481550: /*status*/ return new String[] {"code"}; 1309 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1310 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1311 case 1028554472: /*created*/ return new String[] {"dateTime"}; 1312 case -1406328437: /*author*/ return new String[] {"Reference"}; 1313 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1314 case -896505829: /*source*/ return new String[] {"uri"}; 1315 case -1724546052: /*description*/ return new String[] {"string"}; 1316 case 951530617: /*content*/ return new String[] {}; 1317 case 1090493483: /*related*/ return new String[] {}; 1318 default: return super.getTypesForProperty(hash, name); 1319 } 1320 1321 } 1322 1323 @Override 1324 public Base addChild(String name) throws FHIRException { 1325 if (name.equals("masterIdentifier")) { 1326 this.masterIdentifier = new Identifier(); 1327 return this.masterIdentifier; 1328 } 1329 else if (name.equals("identifier")) { 1330 return addIdentifier(); 1331 } 1332 else if (name.equals("status")) { 1333 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.status"); 1334 } 1335 else if (name.equals("type")) { 1336 this.type = new CodeableConcept(); 1337 return this.type; 1338 } 1339 else if (name.equals("subject")) { 1340 this.subject = new Reference(); 1341 return this.subject; 1342 } 1343 else if (name.equals("created")) { 1344 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.created"); 1345 } 1346 else if (name.equals("author")) { 1347 return addAuthor(); 1348 } 1349 else if (name.equals("recipient")) { 1350 return addRecipient(); 1351 } 1352 else if (name.equals("source")) { 1353 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.source"); 1354 } 1355 else if (name.equals("description")) { 1356 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.description"); 1357 } 1358 else if (name.equals("content")) { 1359 return addContent(); 1360 } 1361 else if (name.equals("related")) { 1362 return addRelated(); 1363 } 1364 else 1365 return super.addChild(name); 1366 } 1367 1368 public String fhirType() { 1369 return "DocumentManifest"; 1370 1371 } 1372 1373 public DocumentManifest copy() { 1374 DocumentManifest dst = new DocumentManifest(); 1375 copyValues(dst); 1376 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 1377 if (identifier != null) { 1378 dst.identifier = new ArrayList<Identifier>(); 1379 for (Identifier i : identifier) 1380 dst.identifier.add(i.copy()); 1381 }; 1382 dst.status = status == null ? null : status.copy(); 1383 dst.type = type == null ? null : type.copy(); 1384 dst.subject = subject == null ? null : subject.copy(); 1385 dst.created = created == null ? null : created.copy(); 1386 if (author != null) { 1387 dst.author = new ArrayList<Reference>(); 1388 for (Reference i : author) 1389 dst.author.add(i.copy()); 1390 }; 1391 if (recipient != null) { 1392 dst.recipient = new ArrayList<Reference>(); 1393 for (Reference i : recipient) 1394 dst.recipient.add(i.copy()); 1395 }; 1396 dst.source = source == null ? null : source.copy(); 1397 dst.description = description == null ? null : description.copy(); 1398 if (content != null) { 1399 dst.content = new ArrayList<DocumentManifestContentComponent>(); 1400 for (DocumentManifestContentComponent i : content) 1401 dst.content.add(i.copy()); 1402 }; 1403 if (related != null) { 1404 dst.related = new ArrayList<DocumentManifestRelatedComponent>(); 1405 for (DocumentManifestRelatedComponent i : related) 1406 dst.related.add(i.copy()); 1407 }; 1408 return dst; 1409 } 1410 1411 protected DocumentManifest typedCopy() { 1412 return copy(); 1413 } 1414 1415 @Override 1416 public boolean equalsDeep(Base other_) { 1417 if (!super.equalsDeep(other_)) 1418 return false; 1419 if (!(other_ instanceof DocumentManifest)) 1420 return false; 1421 DocumentManifest o = (DocumentManifest) other_; 1422 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 1423 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 1424 && compareDeep(created, o.created, true) && compareDeep(author, o.author, true) && compareDeep(recipient, o.recipient, true) 1425 && compareDeep(source, o.source, true) && compareDeep(description, o.description, true) && compareDeep(content, o.content, true) 1426 && compareDeep(related, o.related, true); 1427 } 1428 1429 @Override 1430 public boolean equalsShallow(Base other_) { 1431 if (!super.equalsShallow(other_)) 1432 return false; 1433 if (!(other_ instanceof DocumentManifest)) 1434 return false; 1435 DocumentManifest o = (DocumentManifest) other_; 1436 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(source, o.source, true) 1437 && compareValues(description, o.description, true); 1438 } 1439 1440 public boolean isEmpty() { 1441 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(masterIdentifier, identifier 1442 , status, type, subject, created, author, recipient, source, description, content 1443 , related); 1444 } 1445 1446 @Override 1447 public ResourceType getResourceType() { 1448 return ResourceType.DocumentManifest; 1449 } 1450 1451 /** 1452 * Search parameter: <b>identifier</b> 1453 * <p> 1454 * Description: <b>Unique Identifier for the set of documents</b><br> 1455 * Type: <b>token</b><br> 1456 * Path: <b>DocumentManifest.masterIdentifier, DocumentManifest.identifier</b><br> 1457 * </p> 1458 */ 1459 @SearchParamDefinition(name="identifier", path="DocumentManifest.masterIdentifier | DocumentManifest.identifier", description="Unique Identifier for the set of documents", type="token" ) 1460 public static final String SP_IDENTIFIER = "identifier"; 1461 /** 1462 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1463 * <p> 1464 * Description: <b>Unique Identifier for the set of documents</b><br> 1465 * Type: <b>token</b><br> 1466 * Path: <b>DocumentManifest.masterIdentifier, DocumentManifest.identifier</b><br> 1467 * </p> 1468 */ 1469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1470 1471 /** 1472 * Search parameter: <b>related-id</b> 1473 * <p> 1474 * Description: <b>Identifiers of things that are related</b><br> 1475 * Type: <b>token</b><br> 1476 * Path: <b>DocumentManifest.related.identifier</b><br> 1477 * </p> 1478 */ 1479 @SearchParamDefinition(name="related-id", path="DocumentManifest.related.identifier", description="Identifiers of things that are related", type="token" ) 1480 public static final String SP_RELATED_ID = "related-id"; 1481 /** 1482 * <b>Fluent Client</b> search parameter constant for <b>related-id</b> 1483 * <p> 1484 * Description: <b>Identifiers of things that are related</b><br> 1485 * Type: <b>token</b><br> 1486 * Path: <b>DocumentManifest.related.identifier</b><br> 1487 * </p> 1488 */ 1489 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATED_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATED_ID); 1490 1491 /** 1492 * Search parameter: <b>content-ref</b> 1493 * <p> 1494 * Description: <b>Contents of this set of documents</b><br> 1495 * Type: <b>reference</b><br> 1496 * Path: <b>DocumentManifest.content.pReference</b><br> 1497 * </p> 1498 */ 1499 @SearchParamDefinition(name="content-ref", path="DocumentManifest.content.p.as(Reference)", description="Contents of this set of documents", type="reference" ) 1500 public static final String SP_CONTENT_REF = "content-ref"; 1501 /** 1502 * <b>Fluent Client</b> search parameter constant for <b>content-ref</b> 1503 * <p> 1504 * Description: <b>Contents of this set of documents</b><br> 1505 * Type: <b>reference</b><br> 1506 * Path: <b>DocumentManifest.content.pReference</b><br> 1507 * </p> 1508 */ 1509 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTENT_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTENT_REF); 1510 1511/** 1512 * Constant for fluent queries to be used to add include statements. Specifies 1513 * the path value of "<b>DocumentManifest:content-ref</b>". 1514 */ 1515 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTENT_REF = new ca.uhn.fhir.model.api.Include("DocumentManifest:content-ref").toLocked(); 1516 1517 /** 1518 * Search parameter: <b>subject</b> 1519 * <p> 1520 * Description: <b>The subject of the set of documents</b><br> 1521 * Type: <b>reference</b><br> 1522 * Path: <b>DocumentManifest.subject</b><br> 1523 * </p> 1524 */ 1525 @SearchParamDefinition(name="subject", path="DocumentManifest.subject", description="The subject of the set of documents", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Group.class, Patient.class, Practitioner.class } ) 1526 public static final String SP_SUBJECT = "subject"; 1527 /** 1528 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1529 * <p> 1530 * Description: <b>The subject of the set of documents</b><br> 1531 * Type: <b>reference</b><br> 1532 * Path: <b>DocumentManifest.subject</b><br> 1533 * </p> 1534 */ 1535 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1536 1537/** 1538 * Constant for fluent queries to be used to add include statements. Specifies 1539 * the path value of "<b>DocumentManifest:subject</b>". 1540 */ 1541 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DocumentManifest:subject").toLocked(); 1542 1543 /** 1544 * Search parameter: <b>author</b> 1545 * <p> 1546 * Description: <b>Who and/or what authored the manifest</b><br> 1547 * Type: <b>reference</b><br> 1548 * Path: <b>DocumentManifest.author</b><br> 1549 * </p> 1550 */ 1551 @SearchParamDefinition(name="author", path="DocumentManifest.author", description="Who and/or what authored the manifest", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 1552 public static final String SP_AUTHOR = "author"; 1553 /** 1554 * <b>Fluent Client</b> search parameter constant for <b>author</b> 1555 * <p> 1556 * Description: <b>Who and/or what authored the manifest</b><br> 1557 * Type: <b>reference</b><br> 1558 * Path: <b>DocumentManifest.author</b><br> 1559 * </p> 1560 */ 1561 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 1562 1563/** 1564 * Constant for fluent queries to be used to add include statements. Specifies 1565 * the path value of "<b>DocumentManifest:author</b>". 1566 */ 1567 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("DocumentManifest:author").toLocked(); 1568 1569 /** 1570 * Search parameter: <b>created</b> 1571 * <p> 1572 * Description: <b>When this document manifest created</b><br> 1573 * Type: <b>date</b><br> 1574 * Path: <b>DocumentManifest.created</b><br> 1575 * </p> 1576 */ 1577 @SearchParamDefinition(name="created", path="DocumentManifest.created", description="When this document manifest created", type="date" ) 1578 public static final String SP_CREATED = "created"; 1579 /** 1580 * <b>Fluent Client</b> search parameter constant for <b>created</b> 1581 * <p> 1582 * Description: <b>When this document manifest created</b><br> 1583 * Type: <b>date</b><br> 1584 * Path: <b>DocumentManifest.created</b><br> 1585 * </p> 1586 */ 1587 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 1588 1589 /** 1590 * Search parameter: <b>description</b> 1591 * <p> 1592 * Description: <b>Human-readable description (title)</b><br> 1593 * Type: <b>string</b><br> 1594 * Path: <b>DocumentManifest.description</b><br> 1595 * </p> 1596 */ 1597 @SearchParamDefinition(name="description", path="DocumentManifest.description", description="Human-readable description (title)", type="string" ) 1598 public static final String SP_DESCRIPTION = "description"; 1599 /** 1600 * <b>Fluent Client</b> search parameter constant for <b>description</b> 1601 * <p> 1602 * Description: <b>Human-readable description (title)</b><br> 1603 * Type: <b>string</b><br> 1604 * Path: <b>DocumentManifest.description</b><br> 1605 * </p> 1606 */ 1607 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 1608 1609 /** 1610 * Search parameter: <b>source</b> 1611 * <p> 1612 * Description: <b>The source system/application/software</b><br> 1613 * Type: <b>uri</b><br> 1614 * Path: <b>DocumentManifest.source</b><br> 1615 * </p> 1616 */ 1617 @SearchParamDefinition(name="source", path="DocumentManifest.source", description="The source system/application/software", type="uri" ) 1618 public static final String SP_SOURCE = "source"; 1619 /** 1620 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1621 * <p> 1622 * Description: <b>The source system/application/software</b><br> 1623 * Type: <b>uri</b><br> 1624 * Path: <b>DocumentManifest.source</b><br> 1625 * </p> 1626 */ 1627 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SOURCE); 1628 1629 /** 1630 * Search parameter: <b>type</b> 1631 * <p> 1632 * Description: <b>Kind of document set</b><br> 1633 * Type: <b>token</b><br> 1634 * Path: <b>DocumentManifest.type</b><br> 1635 * </p> 1636 */ 1637 @SearchParamDefinition(name="type", path="DocumentManifest.type", description="Kind of document set", type="token" ) 1638 public static final String SP_TYPE = "type"; 1639 /** 1640 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1641 * <p> 1642 * Description: <b>Kind of document set</b><br> 1643 * Type: <b>token</b><br> 1644 * Path: <b>DocumentManifest.type</b><br> 1645 * </p> 1646 */ 1647 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1648 1649 /** 1650 * Search parameter: <b>related-ref</b> 1651 * <p> 1652 * Description: <b>Related Resource</b><br> 1653 * Type: <b>reference</b><br> 1654 * Path: <b>DocumentManifest.related.ref</b><br> 1655 * </p> 1656 */ 1657 @SearchParamDefinition(name="related-ref", path="DocumentManifest.related.ref", description="Related Resource", type="reference" ) 1658 public static final String SP_RELATED_REF = "related-ref"; 1659 /** 1660 * <b>Fluent Client</b> search parameter constant for <b>related-ref</b> 1661 * <p> 1662 * Description: <b>Related Resource</b><br> 1663 * Type: <b>reference</b><br> 1664 * Path: <b>DocumentManifest.related.ref</b><br> 1665 * </p> 1666 */ 1667 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATED_REF); 1668 1669/** 1670 * Constant for fluent queries to be used to add include statements. Specifies 1671 * the path value of "<b>DocumentManifest:related-ref</b>". 1672 */ 1673 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED_REF = new ca.uhn.fhir.model.api.Include("DocumentManifest:related-ref").toLocked(); 1674 1675 /** 1676 * Search parameter: <b>patient</b> 1677 * <p> 1678 * Description: <b>The subject of the set of documents</b><br> 1679 * Type: <b>reference</b><br> 1680 * Path: <b>DocumentManifest.subject</b><br> 1681 * </p> 1682 */ 1683 @SearchParamDefinition(name="patient", path="DocumentManifest.subject", description="The subject of the set of documents", type="reference", target={Patient.class } ) 1684 public static final String SP_PATIENT = "patient"; 1685 /** 1686 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1687 * <p> 1688 * Description: <b>The subject of the set of documents</b><br> 1689 * Type: <b>reference</b><br> 1690 * Path: <b>DocumentManifest.subject</b><br> 1691 * </p> 1692 */ 1693 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1694 1695/** 1696 * Constant for fluent queries to be used to add include statements. Specifies 1697 * the path value of "<b>DocumentManifest:patient</b>". 1698 */ 1699 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DocumentManifest:patient").toLocked(); 1700 1701 /** 1702 * Search parameter: <b>recipient</b> 1703 * <p> 1704 * Description: <b>Intended to get notified about this set of documents</b><br> 1705 * Type: <b>reference</b><br> 1706 * Path: <b>DocumentManifest.recipient</b><br> 1707 * </p> 1708 */ 1709 @SearchParamDefinition(name="recipient", path="DocumentManifest.recipient", description="Intended to get notified about this set of documents", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 1710 public static final String SP_RECIPIENT = "recipient"; 1711 /** 1712 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 1713 * <p> 1714 * Description: <b>Intended to get notified about this set of documents</b><br> 1715 * Type: <b>reference</b><br> 1716 * Path: <b>DocumentManifest.recipient</b><br> 1717 * </p> 1718 */ 1719 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 1720 1721/** 1722 * Constant for fluent queries to be used to add include statements. Specifies 1723 * the path value of "<b>DocumentManifest:recipient</b>". 1724 */ 1725 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("DocumentManifest:recipient").toLocked(); 1726 1727 /** 1728 * Search parameter: <b>status</b> 1729 * <p> 1730 * Description: <b>current | superseded | entered-in-error</b><br> 1731 * Type: <b>token</b><br> 1732 * Path: <b>DocumentManifest.status</b><br> 1733 * </p> 1734 */ 1735 @SearchParamDefinition(name="status", path="DocumentManifest.status", description="current | superseded | entered-in-error", type="token" ) 1736 public static final String SP_STATUS = "status"; 1737 /** 1738 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1739 * <p> 1740 * Description: <b>current | superseded | entered-in-error</b><br> 1741 * Type: <b>token</b><br> 1742 * Path: <b>DocumentManifest.status</b><br> 1743 * </p> 1744 */ 1745 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1746 1747 1748}