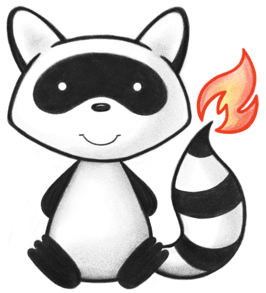
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * A reference to a document. 052 */ 053@ResourceDef(name="DocumentReference", profile="http://hl7.org/fhir/Profile/DocumentReference") 054public class DocumentReference extends DomainResource { 055 056 public enum ReferredDocumentStatus { 057 /** 058 * This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified. 059 */ 060 PRELIMINARY, 061 /** 062 * This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition. 063 */ 064 FINAL, 065 /** 066 * The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as "final" and the composition is complete and verified by an authorized person. 067 */ 068 AMENDED, 069 /** 070 * The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid. 071 */ 072 ENTEREDINERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static ReferredDocumentStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("preliminary".equals(codeString)) 081 return PRELIMINARY; 082 if ("final".equals(codeString)) 083 return FINAL; 084 if ("amended".equals(codeString)) 085 return AMENDED; 086 if ("entered-in-error".equals(codeString)) 087 return ENTEREDINERROR; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown ReferredDocumentStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case PRELIMINARY: return "preliminary"; 096 case FINAL: return "final"; 097 case AMENDED: return "amended"; 098 case ENTEREDINERROR: return "entered-in-error"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case PRELIMINARY: return "http://hl7.org/fhir/composition-status"; 106 case FINAL: return "http://hl7.org/fhir/composition-status"; 107 case AMENDED: return "http://hl7.org/fhir/composition-status"; 108 case ENTEREDINERROR: return "http://hl7.org/fhir/composition-status"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case PRELIMINARY: return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 116 case FINAL: return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 117 case AMENDED: return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 118 case ENTEREDINERROR: return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case PRELIMINARY: return "Preliminary"; 126 case FINAL: return "Final"; 127 case AMENDED: return "Amended"; 128 case ENTEREDINERROR: return "Entered in Error"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class ReferredDocumentStatusEnumFactory implements EnumFactory<ReferredDocumentStatus> { 136 public ReferredDocumentStatus fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("preliminary".equals(codeString)) 141 return ReferredDocumentStatus.PRELIMINARY; 142 if ("final".equals(codeString)) 143 return ReferredDocumentStatus.FINAL; 144 if ("amended".equals(codeString)) 145 return ReferredDocumentStatus.AMENDED; 146 if ("entered-in-error".equals(codeString)) 147 return ReferredDocumentStatus.ENTEREDINERROR; 148 throw new IllegalArgumentException("Unknown ReferredDocumentStatus code '"+codeString+"'"); 149 } 150 public Enumeration<ReferredDocumentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<ReferredDocumentStatus>(this); 155 String codeString = code.asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("preliminary".equals(codeString)) 159 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.PRELIMINARY); 160 if ("final".equals(codeString)) 161 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.FINAL); 162 if ("amended".equals(codeString)) 163 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.AMENDED); 164 if ("entered-in-error".equals(codeString)) 165 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.ENTEREDINERROR); 166 throw new FHIRException("Unknown ReferredDocumentStatus code '"+codeString+"'"); 167 } 168 public String toCode(ReferredDocumentStatus code) { 169 if (code == ReferredDocumentStatus.NULL) 170 return null; 171 if (code == ReferredDocumentStatus.PRELIMINARY) 172 return "preliminary"; 173 if (code == ReferredDocumentStatus.FINAL) 174 return "final"; 175 if (code == ReferredDocumentStatus.AMENDED) 176 return "amended"; 177 if (code == ReferredDocumentStatus.ENTEREDINERROR) 178 return "entered-in-error"; 179 return "?"; 180 } 181 public String toSystem(ReferredDocumentStatus code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum DocumentRelationshipType { 187 /** 188 * This document logically replaces or supersedes the target document. 189 */ 190 REPLACES, 191 /** 192 * This document was generated by transforming the target document (e.g. format or language conversion). 193 */ 194 TRANSFORMS, 195 /** 196 * This document is a signature of the target document. 197 */ 198 SIGNS, 199 /** 200 * This document adds additional information to the target document. 201 */ 202 APPENDS, 203 /** 204 * added to help the parsers with the generic types 205 */ 206 NULL; 207 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("replaces".equals(codeString)) 211 return REPLACES; 212 if ("transforms".equals(codeString)) 213 return TRANSFORMS; 214 if ("signs".equals(codeString)) 215 return SIGNS; 216 if ("appends".equals(codeString)) 217 return APPENDS; 218 if (Configuration.isAcceptInvalidEnums()) 219 return null; 220 else 221 throw new FHIRException("Unknown DocumentRelationshipType code '"+codeString+"'"); 222 } 223 public String toCode() { 224 switch (this) { 225 case REPLACES: return "replaces"; 226 case TRANSFORMS: return "transforms"; 227 case SIGNS: return "signs"; 228 case APPENDS: return "appends"; 229 case NULL: return null; 230 default: return "?"; 231 } 232 } 233 public String getSystem() { 234 switch (this) { 235 case REPLACES: return "http://hl7.org/fhir/document-relationship-type"; 236 case TRANSFORMS: return "http://hl7.org/fhir/document-relationship-type"; 237 case SIGNS: return "http://hl7.org/fhir/document-relationship-type"; 238 case APPENDS: return "http://hl7.org/fhir/document-relationship-type"; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 public String getDefinition() { 244 switch (this) { 245 case REPLACES: return "This document logically replaces or supersedes the target document."; 246 case TRANSFORMS: return "This document was generated by transforming the target document (e.g. format or language conversion)."; 247 case SIGNS: return "This document is a signature of the target document."; 248 case APPENDS: return "This document adds additional information to the target document."; 249 case NULL: return null; 250 default: return "?"; 251 } 252 } 253 public String getDisplay() { 254 switch (this) { 255 case REPLACES: return "Replaces"; 256 case TRANSFORMS: return "Transforms"; 257 case SIGNS: return "Signs"; 258 case APPENDS: return "Appends"; 259 case NULL: return null; 260 default: return "?"; 261 } 262 } 263 } 264 265 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 266 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 267 if (codeString == null || "".equals(codeString)) 268 if (codeString == null || "".equals(codeString)) 269 return null; 270 if ("replaces".equals(codeString)) 271 return DocumentRelationshipType.REPLACES; 272 if ("transforms".equals(codeString)) 273 return DocumentRelationshipType.TRANSFORMS; 274 if ("signs".equals(codeString)) 275 return DocumentRelationshipType.SIGNS; 276 if ("appends".equals(codeString)) 277 return DocumentRelationshipType.APPENDS; 278 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '"+codeString+"'"); 279 } 280 public Enumeration<DocumentRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 281 if (code == null) 282 return null; 283 if (code.isEmpty()) 284 return new Enumeration<DocumentRelationshipType>(this); 285 String codeString = code.asStringValue(); 286 if (codeString == null || "".equals(codeString)) 287 return null; 288 if ("replaces".equals(codeString)) 289 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES); 290 if ("transforms".equals(codeString)) 291 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS); 292 if ("signs".equals(codeString)) 293 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS); 294 if ("appends".equals(codeString)) 295 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS); 296 throw new FHIRException("Unknown DocumentRelationshipType code '"+codeString+"'"); 297 } 298 public String toCode(DocumentRelationshipType code) { 299 if (code == DocumentRelationshipType.NULL) 300 return null; 301 if (code == DocumentRelationshipType.REPLACES) 302 return "replaces"; 303 if (code == DocumentRelationshipType.TRANSFORMS) 304 return "transforms"; 305 if (code == DocumentRelationshipType.SIGNS) 306 return "signs"; 307 if (code == DocumentRelationshipType.APPENDS) 308 return "appends"; 309 return "?"; 310 } 311 public String toSystem(DocumentRelationshipType code) { 312 return code.getSystem(); 313 } 314 } 315 316 @Block() 317 public static class DocumentReferenceRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 318 /** 319 * The type of relationship that this document has with anther document. 320 */ 321 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 322 @Description(shortDefinition="replaces | transforms | signs | appends", formalDefinition="The type of relationship that this document has with anther document." ) 323 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-relationship-type") 324 protected Enumeration<DocumentRelationshipType> code; 325 326 /** 327 * The target document of this relationship. 328 */ 329 @Child(name = "target", type = {DocumentReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 330 @Description(shortDefinition="Target of the relationship", formalDefinition="The target document of this relationship." ) 331 protected Reference target; 332 333 /** 334 * The actual object that is the target of the reference (The target document of this relationship.) 335 */ 336 protected DocumentReference targetTarget; 337 338 private static final long serialVersionUID = -347257495L; 339 340 /** 341 * Constructor 342 */ 343 public DocumentReferenceRelatesToComponent() { 344 super(); 345 } 346 347 /** 348 * Constructor 349 */ 350 public DocumentReferenceRelatesToComponent(Enumeration<DocumentRelationshipType> code, Reference target) { 351 super(); 352 this.code = code; 353 this.target = target; 354 } 355 356 /** 357 * @return {@link #code} (The type of relationship that this document has with anther document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 358 */ 359 public Enumeration<DocumentRelationshipType> getCodeElement() { 360 if (this.code == null) 361 if (Configuration.errorOnAutoCreate()) 362 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.code"); 363 else if (Configuration.doAutoCreate()) 364 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 365 return this.code; 366 } 367 368 public boolean hasCodeElement() { 369 return this.code != null && !this.code.isEmpty(); 370 } 371 372 public boolean hasCode() { 373 return this.code != null && !this.code.isEmpty(); 374 } 375 376 /** 377 * @param value {@link #code} (The type of relationship that this document has with anther document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 378 */ 379 public DocumentReferenceRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 380 this.code = value; 381 return this; 382 } 383 384 /** 385 * @return The type of relationship that this document has with anther document. 386 */ 387 public DocumentRelationshipType getCode() { 388 return this.code == null ? null : this.code.getValue(); 389 } 390 391 /** 392 * @param value The type of relationship that this document has with anther document. 393 */ 394 public DocumentReferenceRelatesToComponent setCode(DocumentRelationshipType value) { 395 if (this.code == null) 396 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 397 this.code.setValue(value); 398 return this; 399 } 400 401 /** 402 * @return {@link #target} (The target document of this relationship.) 403 */ 404 public Reference getTarget() { 405 if (this.target == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 408 else if (Configuration.doAutoCreate()) 409 this.target = new Reference(); // cc 410 return this.target; 411 } 412 413 public boolean hasTarget() { 414 return this.target != null && !this.target.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #target} (The target document of this relationship.) 419 */ 420 public DocumentReferenceRelatesToComponent setTarget(Reference value) { 421 this.target = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #target} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The target document of this relationship.) 427 */ 428 public DocumentReference getTargetTarget() { 429 if (this.targetTarget == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 432 else if (Configuration.doAutoCreate()) 433 this.targetTarget = new DocumentReference(); // aa 434 return this.targetTarget; 435 } 436 437 /** 438 * @param value {@link #target} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The target document of this relationship.) 439 */ 440 public DocumentReferenceRelatesToComponent setTargetTarget(DocumentReference value) { 441 this.targetTarget = value; 442 return this; 443 } 444 445 protected void listChildren(List<Property> children) { 446 super.listChildren(children); 447 children.add(new Property("code", "code", "The type of relationship that this document has with anther document.", 0, 1, code)); 448 children.add(new Property("target", "Reference(DocumentReference)", "The target document of this relationship.", 0, 1, target)); 449 } 450 451 @Override 452 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 453 switch (_hash) { 454 case 3059181: /*code*/ return new Property("code", "code", "The type of relationship that this document has with anther document.", 0, 1, code); 455 case -880905839: /*target*/ return new Property("target", "Reference(DocumentReference)", "The target document of this relationship.", 0, 1, target); 456 default: return super.getNamedProperty(_hash, _name, _checkValid); 457 } 458 459 } 460 461 @Override 462 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 463 switch (hash) { 464 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<DocumentRelationshipType> 465 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 466 default: return super.getProperty(hash, name, checkValid); 467 } 468 469 } 470 471 @Override 472 public Base setProperty(int hash, String name, Base value) throws FHIRException { 473 switch (hash) { 474 case 3059181: // code 475 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 476 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 477 return value; 478 case -880905839: // target 479 this.target = castToReference(value); // Reference 480 return value; 481 default: return super.setProperty(hash, name, value); 482 } 483 484 } 485 486 @Override 487 public Base setProperty(String name, Base value) throws FHIRException { 488 if (name.equals("code")) { 489 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 490 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 491 } else if (name.equals("target")) { 492 this.target = castToReference(value); // Reference 493 } else 494 return super.setProperty(name, value); 495 return value; 496 } 497 498 @Override 499 public Base makeProperty(int hash, String name) throws FHIRException { 500 switch (hash) { 501 case 3059181: return getCodeElement(); 502 case -880905839: return getTarget(); 503 default: return super.makeProperty(hash, name); 504 } 505 506 } 507 508 @Override 509 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 510 switch (hash) { 511 case 3059181: /*code*/ return new String[] {"code"}; 512 case -880905839: /*target*/ return new String[] {"Reference"}; 513 default: return super.getTypesForProperty(hash, name); 514 } 515 516 } 517 518 @Override 519 public Base addChild(String name) throws FHIRException { 520 if (name.equals("code")) { 521 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.code"); 522 } 523 else if (name.equals("target")) { 524 this.target = new Reference(); 525 return this.target; 526 } 527 else 528 return super.addChild(name); 529 } 530 531 public DocumentReferenceRelatesToComponent copy() { 532 DocumentReferenceRelatesToComponent dst = new DocumentReferenceRelatesToComponent(); 533 copyValues(dst); 534 dst.code = code == null ? null : code.copy(); 535 dst.target = target == null ? null : target.copy(); 536 return dst; 537 } 538 539 @Override 540 public boolean equalsDeep(Base other_) { 541 if (!super.equalsDeep(other_)) 542 return false; 543 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 544 return false; 545 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 546 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 547 } 548 549 @Override 550 public boolean equalsShallow(Base other_) { 551 if (!super.equalsShallow(other_)) 552 return false; 553 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 554 return false; 555 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 556 return compareValues(code, o.code, true); 557 } 558 559 public boolean isEmpty() { 560 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 561 } 562 563 public String fhirType() { 564 return "DocumentReference.relatesTo"; 565 566 } 567 568 } 569 570 @Block() 571 public static class DocumentReferenceContentComponent extends BackboneElement implements IBaseBackboneElement { 572 /** 573 * The document or URL of the document along with critical metadata to prove content has integrity. 574 */ 575 @Child(name = "attachment", type = {Attachment.class}, order=1, min=1, max=1, modifier=false, summary=true) 576 @Description(shortDefinition="Where to access the document", formalDefinition="The document or URL of the document along with critical metadata to prove content has integrity." ) 577 protected Attachment attachment; 578 579 /** 580 * An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType. 581 */ 582 @Child(name = "format", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 583 @Description(shortDefinition="Format/content rules for the document", formalDefinition="An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType." ) 584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/formatcodes") 585 protected Coding format; 586 587 private static final long serialVersionUID = -1313860217L; 588 589 /** 590 * Constructor 591 */ 592 public DocumentReferenceContentComponent() { 593 super(); 594 } 595 596 /** 597 * Constructor 598 */ 599 public DocumentReferenceContentComponent(Attachment attachment) { 600 super(); 601 this.attachment = attachment; 602 } 603 604 /** 605 * @return {@link #attachment} (The document or URL of the document along with critical metadata to prove content has integrity.) 606 */ 607 public Attachment getAttachment() { 608 if (this.attachment == null) 609 if (Configuration.errorOnAutoCreate()) 610 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.attachment"); 611 else if (Configuration.doAutoCreate()) 612 this.attachment = new Attachment(); // cc 613 return this.attachment; 614 } 615 616 public boolean hasAttachment() { 617 return this.attachment != null && !this.attachment.isEmpty(); 618 } 619 620 /** 621 * @param value {@link #attachment} (The document or URL of the document along with critical metadata to prove content has integrity.) 622 */ 623 public DocumentReferenceContentComponent setAttachment(Attachment value) { 624 this.attachment = value; 625 return this; 626 } 627 628 /** 629 * @return {@link #format} (An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.) 630 */ 631 public Coding getFormat() { 632 if (this.format == null) 633 if (Configuration.errorOnAutoCreate()) 634 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.format"); 635 else if (Configuration.doAutoCreate()) 636 this.format = new Coding(); // cc 637 return this.format; 638 } 639 640 public boolean hasFormat() { 641 return this.format != null && !this.format.isEmpty(); 642 } 643 644 /** 645 * @param value {@link #format} (An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.) 646 */ 647 public DocumentReferenceContentComponent setFormat(Coding value) { 648 this.format = value; 649 return this; 650 } 651 652 protected void listChildren(List<Property> children) { 653 super.listChildren(children); 654 children.add(new Property("attachment", "Attachment", "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, attachment)); 655 children.add(new Property("format", "Coding", "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 0, 1, format)); 656 } 657 658 @Override 659 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 660 switch (_hash) { 661 case -1963501277: /*attachment*/ return new Property("attachment", "Attachment", "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, attachment); 662 case -1268779017: /*format*/ return new Property("format", "Coding", "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 0, 1, format); 663 default: return super.getNamedProperty(_hash, _name, _checkValid); 664 } 665 666 } 667 668 @Override 669 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 670 switch (hash) { 671 case -1963501277: /*attachment*/ return this.attachment == null ? new Base[0] : new Base[] {this.attachment}; // Attachment 672 case -1268779017: /*format*/ return this.format == null ? new Base[0] : new Base[] {this.format}; // Coding 673 default: return super.getProperty(hash, name, checkValid); 674 } 675 676 } 677 678 @Override 679 public Base setProperty(int hash, String name, Base value) throws FHIRException { 680 switch (hash) { 681 case -1963501277: // attachment 682 this.attachment = castToAttachment(value); // Attachment 683 return value; 684 case -1268779017: // format 685 this.format = castToCoding(value); // Coding 686 return value; 687 default: return super.setProperty(hash, name, value); 688 } 689 690 } 691 692 @Override 693 public Base setProperty(String name, Base value) throws FHIRException { 694 if (name.equals("attachment")) { 695 this.attachment = castToAttachment(value); // Attachment 696 } else if (name.equals("format")) { 697 this.format = castToCoding(value); // Coding 698 } else 699 return super.setProperty(name, value); 700 return value; 701 } 702 703 @Override 704 public Base makeProperty(int hash, String name) throws FHIRException { 705 switch (hash) { 706 case -1963501277: return getAttachment(); 707 case -1268779017: return getFormat(); 708 default: return super.makeProperty(hash, name); 709 } 710 711 } 712 713 @Override 714 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 715 switch (hash) { 716 case -1963501277: /*attachment*/ return new String[] {"Attachment"}; 717 case -1268779017: /*format*/ return new String[] {"Coding"}; 718 default: return super.getTypesForProperty(hash, name); 719 } 720 721 } 722 723 @Override 724 public Base addChild(String name) throws FHIRException { 725 if (name.equals("attachment")) { 726 this.attachment = new Attachment(); 727 return this.attachment; 728 } 729 else if (name.equals("format")) { 730 this.format = new Coding(); 731 return this.format; 732 } 733 else 734 return super.addChild(name); 735 } 736 737 public DocumentReferenceContentComponent copy() { 738 DocumentReferenceContentComponent dst = new DocumentReferenceContentComponent(); 739 copyValues(dst); 740 dst.attachment = attachment == null ? null : attachment.copy(); 741 dst.format = format == null ? null : format.copy(); 742 return dst; 743 } 744 745 @Override 746 public boolean equalsDeep(Base other_) { 747 if (!super.equalsDeep(other_)) 748 return false; 749 if (!(other_ instanceof DocumentReferenceContentComponent)) 750 return false; 751 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 752 return compareDeep(attachment, o.attachment, true) && compareDeep(format, o.format, true); 753 } 754 755 @Override 756 public boolean equalsShallow(Base other_) { 757 if (!super.equalsShallow(other_)) 758 return false; 759 if (!(other_ instanceof DocumentReferenceContentComponent)) 760 return false; 761 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 762 return true; 763 } 764 765 public boolean isEmpty() { 766 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(attachment, format); 767 } 768 769 public String fhirType() { 770 return "DocumentReference.content"; 771 772 } 773 774 } 775 776 @Block() 777 public static class DocumentReferenceContextComponent extends BackboneElement implements IBaseBackboneElement { 778 /** 779 * Describes the clinical encounter or type of care that the document content is associated with. 780 */ 781 @Child(name = "encounter", type = {Encounter.class}, order=1, min=0, max=1, modifier=false, summary=true) 782 @Description(shortDefinition="Context of the document content", formalDefinition="Describes the clinical encounter or type of care that the document content is associated with." ) 783 protected Reference encounter; 784 785 /** 786 * The actual object that is the target of the reference (Describes the clinical encounter or type of care that the document content is associated with.) 787 */ 788 protected Encounter encounterTarget; 789 790 /** 791 * This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a "History and Physical Report" in which the procedure being documented is necessarily a "History and Physical" act. 792 */ 793 @Child(name = "event", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 794 @Description(shortDefinition="Main clinical acts documented", formalDefinition="This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act." ) 795 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActCode") 796 protected List<CodeableConcept> event; 797 798 /** 799 * The time period over which the service that is described by the document was provided. 800 */ 801 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 802 @Description(shortDefinition="Time of service that is being documented", formalDefinition="The time period over which the service that is described by the document was provided." ) 803 protected Period period; 804 805 /** 806 * The kind of facility where the patient was seen. 807 */ 808 @Child(name = "facilityType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 809 @Description(shortDefinition="Kind of facility where patient was seen", formalDefinition="The kind of facility where the patient was seen." ) 810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-facilitycodes") 811 protected CodeableConcept facilityType; 812 813 /** 814 * This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty. 815 */ 816 @Child(name = "practiceSetting", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 817 @Description(shortDefinition="Additional details about where the content was created (e.g. clinical specialty)", formalDefinition="This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty." ) 818 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 819 protected CodeableConcept practiceSetting; 820 821 /** 822 * The Patient Information as known when the document was published. May be a reference to a version specific, or contained. 823 */ 824 @Child(name = "sourcePatientInfo", type = {Patient.class}, order=6, min=0, max=1, modifier=false, summary=true) 825 @Description(shortDefinition="Patient demographics from source", formalDefinition="The Patient Information as known when the document was published. May be a reference to a version specific, or contained." ) 826 protected Reference sourcePatientInfo; 827 828 /** 829 * The actual object that is the target of the reference (The Patient Information as known when the document was published. May be a reference to a version specific, or contained.) 830 */ 831 protected Patient sourcePatientInfoTarget; 832 833 /** 834 * Related identifiers or resources associated with the DocumentReference. 835 */ 836 @Child(name = "related", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 837 @Description(shortDefinition="Related identifiers or resources", formalDefinition="Related identifiers or resources associated with the DocumentReference." ) 838 protected List<DocumentReferenceContextRelatedComponent> related; 839 840 private static final long serialVersionUID = 994799273L; 841 842 /** 843 * Constructor 844 */ 845 public DocumentReferenceContextComponent() { 846 super(); 847 } 848 849 /** 850 * @return {@link #encounter} (Describes the clinical encounter or type of care that the document content is associated with.) 851 */ 852 public Reference getEncounter() { 853 if (this.encounter == null) 854 if (Configuration.errorOnAutoCreate()) 855 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.encounter"); 856 else if (Configuration.doAutoCreate()) 857 this.encounter = new Reference(); // cc 858 return this.encounter; 859 } 860 861 public boolean hasEncounter() { 862 return this.encounter != null && !this.encounter.isEmpty(); 863 } 864 865 /** 866 * @param value {@link #encounter} (Describes the clinical encounter or type of care that the document content is associated with.) 867 */ 868 public DocumentReferenceContextComponent setEncounter(Reference value) { 869 this.encounter = value; 870 return this; 871 } 872 873 /** 874 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Describes the clinical encounter or type of care that the document content is associated with.) 875 */ 876 public Encounter getEncounterTarget() { 877 if (this.encounterTarget == null) 878 if (Configuration.errorOnAutoCreate()) 879 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.encounter"); 880 else if (Configuration.doAutoCreate()) 881 this.encounterTarget = new Encounter(); // aa 882 return this.encounterTarget; 883 } 884 885 /** 886 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Describes the clinical encounter or type of care that the document content is associated with.) 887 */ 888 public DocumentReferenceContextComponent setEncounterTarget(Encounter value) { 889 this.encounterTarget = value; 890 return this; 891 } 892 893 /** 894 * @return {@link #event} (This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a "History and Physical Report" in which the procedure being documented is necessarily a "History and Physical" act.) 895 */ 896 public List<CodeableConcept> getEvent() { 897 if (this.event == null) 898 this.event = new ArrayList<CodeableConcept>(); 899 return this.event; 900 } 901 902 /** 903 * @return Returns a reference to <code>this</code> for easy method chaining 904 */ 905 public DocumentReferenceContextComponent setEvent(List<CodeableConcept> theEvent) { 906 this.event = theEvent; 907 return this; 908 } 909 910 public boolean hasEvent() { 911 if (this.event == null) 912 return false; 913 for (CodeableConcept item : this.event) 914 if (!item.isEmpty()) 915 return true; 916 return false; 917 } 918 919 public CodeableConcept addEvent() { //3 920 CodeableConcept t = new CodeableConcept(); 921 if (this.event == null) 922 this.event = new ArrayList<CodeableConcept>(); 923 this.event.add(t); 924 return t; 925 } 926 927 public DocumentReferenceContextComponent addEvent(CodeableConcept t) { //3 928 if (t == null) 929 return this; 930 if (this.event == null) 931 this.event = new ArrayList<CodeableConcept>(); 932 this.event.add(t); 933 return this; 934 } 935 936 /** 937 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist 938 */ 939 public CodeableConcept getEventFirstRep() { 940 if (getEvent().isEmpty()) { 941 addEvent(); 942 } 943 return getEvent().get(0); 944 } 945 946 /** 947 * @return {@link #period} (The time period over which the service that is described by the document was provided.) 948 */ 949 public Period getPeriod() { 950 if (this.period == null) 951 if (Configuration.errorOnAutoCreate()) 952 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.period"); 953 else if (Configuration.doAutoCreate()) 954 this.period = new Period(); // cc 955 return this.period; 956 } 957 958 public boolean hasPeriod() { 959 return this.period != null && !this.period.isEmpty(); 960 } 961 962 /** 963 * @param value {@link #period} (The time period over which the service that is described by the document was provided.) 964 */ 965 public DocumentReferenceContextComponent setPeriod(Period value) { 966 this.period = value; 967 return this; 968 } 969 970 /** 971 * @return {@link #facilityType} (The kind of facility where the patient was seen.) 972 */ 973 public CodeableConcept getFacilityType() { 974 if (this.facilityType == null) 975 if (Configuration.errorOnAutoCreate()) 976 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.facilityType"); 977 else if (Configuration.doAutoCreate()) 978 this.facilityType = new CodeableConcept(); // cc 979 return this.facilityType; 980 } 981 982 public boolean hasFacilityType() { 983 return this.facilityType != null && !this.facilityType.isEmpty(); 984 } 985 986 /** 987 * @param value {@link #facilityType} (The kind of facility where the patient was seen.) 988 */ 989 public DocumentReferenceContextComponent setFacilityType(CodeableConcept value) { 990 this.facilityType = value; 991 return this; 992 } 993 994 /** 995 * @return {@link #practiceSetting} (This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.) 996 */ 997 public CodeableConcept getPracticeSetting() { 998 if (this.practiceSetting == null) 999 if (Configuration.errorOnAutoCreate()) 1000 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.practiceSetting"); 1001 else if (Configuration.doAutoCreate()) 1002 this.practiceSetting = new CodeableConcept(); // cc 1003 return this.practiceSetting; 1004 } 1005 1006 public boolean hasPracticeSetting() { 1007 return this.practiceSetting != null && !this.practiceSetting.isEmpty(); 1008 } 1009 1010 /** 1011 * @param value {@link #practiceSetting} (This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.) 1012 */ 1013 public DocumentReferenceContextComponent setPracticeSetting(CodeableConcept value) { 1014 this.practiceSetting = value; 1015 return this; 1016 } 1017 1018 /** 1019 * @return {@link #sourcePatientInfo} (The Patient Information as known when the document was published. May be a reference to a version specific, or contained.) 1020 */ 1021 public Reference getSourcePatientInfo() { 1022 if (this.sourcePatientInfo == null) 1023 if (Configuration.errorOnAutoCreate()) 1024 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1025 else if (Configuration.doAutoCreate()) 1026 this.sourcePatientInfo = new Reference(); // cc 1027 return this.sourcePatientInfo; 1028 } 1029 1030 public boolean hasSourcePatientInfo() { 1031 return this.sourcePatientInfo != null && !this.sourcePatientInfo.isEmpty(); 1032 } 1033 1034 /** 1035 * @param value {@link #sourcePatientInfo} (The Patient Information as known when the document was published. May be a reference to a version specific, or contained.) 1036 */ 1037 public DocumentReferenceContextComponent setSourcePatientInfo(Reference value) { 1038 this.sourcePatientInfo = value; 1039 return this; 1040 } 1041 1042 /** 1043 * @return {@link #sourcePatientInfo} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Patient Information as known when the document was published. May be a reference to a version specific, or contained.) 1044 */ 1045 public Patient getSourcePatientInfoTarget() { 1046 if (this.sourcePatientInfoTarget == null) 1047 if (Configuration.errorOnAutoCreate()) 1048 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1049 else if (Configuration.doAutoCreate()) 1050 this.sourcePatientInfoTarget = new Patient(); // aa 1051 return this.sourcePatientInfoTarget; 1052 } 1053 1054 /** 1055 * @param value {@link #sourcePatientInfo} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Patient Information as known when the document was published. May be a reference to a version specific, or contained.) 1056 */ 1057 public DocumentReferenceContextComponent setSourcePatientInfoTarget(Patient value) { 1058 this.sourcePatientInfoTarget = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return {@link #related} (Related identifiers or resources associated with the DocumentReference.) 1064 */ 1065 public List<DocumentReferenceContextRelatedComponent> getRelated() { 1066 if (this.related == null) 1067 this.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 1068 return this.related; 1069 } 1070 1071 /** 1072 * @return Returns a reference to <code>this</code> for easy method chaining 1073 */ 1074 public DocumentReferenceContextComponent setRelated(List<DocumentReferenceContextRelatedComponent> theRelated) { 1075 this.related = theRelated; 1076 return this; 1077 } 1078 1079 public boolean hasRelated() { 1080 if (this.related == null) 1081 return false; 1082 for (DocumentReferenceContextRelatedComponent item : this.related) 1083 if (!item.isEmpty()) 1084 return true; 1085 return false; 1086 } 1087 1088 public DocumentReferenceContextRelatedComponent addRelated() { //3 1089 DocumentReferenceContextRelatedComponent t = new DocumentReferenceContextRelatedComponent(); 1090 if (this.related == null) 1091 this.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 1092 this.related.add(t); 1093 return t; 1094 } 1095 1096 public DocumentReferenceContextComponent addRelated(DocumentReferenceContextRelatedComponent t) { //3 1097 if (t == null) 1098 return this; 1099 if (this.related == null) 1100 this.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 1101 this.related.add(t); 1102 return this; 1103 } 1104 1105 /** 1106 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist 1107 */ 1108 public DocumentReferenceContextRelatedComponent getRelatedFirstRep() { 1109 if (getRelated().isEmpty()) { 1110 addRelated(); 1111 } 1112 return getRelated().get(0); 1113 } 1114 1115 protected void listChildren(List<Property> children) { 1116 super.listChildren(children); 1117 children.add(new Property("encounter", "Reference(Encounter)", "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1, encounter)); 1118 children.add(new Property("event", "CodeableConcept", "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 0, java.lang.Integer.MAX_VALUE, event)); 1119 children.add(new Property("period", "Period", "The time period over which the service that is described by the document was provided.", 0, 1, period)); 1120 children.add(new Property("facilityType", "CodeableConcept", "The kind of facility where the patient was seen.", 0, 1, facilityType)); 1121 children.add(new Property("practiceSetting", "CodeableConcept", "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 0, 1, practiceSetting)); 1122 children.add(new Property("sourcePatientInfo", "Reference(Patient)", "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 0, 1, sourcePatientInfo)); 1123 children.add(new Property("related", "", "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, related)); 1124 } 1125 1126 @Override 1127 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1128 switch (_hash) { 1129 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1, encounter); 1130 case 96891546: /*event*/ return new Property("event", "CodeableConcept", "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 0, java.lang.Integer.MAX_VALUE, event); 1131 case -991726143: /*period*/ return new Property("period", "Period", "The time period over which the service that is described by the document was provided.", 0, 1, period); 1132 case 370698365: /*facilityType*/ return new Property("facilityType", "CodeableConcept", "The kind of facility where the patient was seen.", 0, 1, facilityType); 1133 case 331373717: /*practiceSetting*/ return new Property("practiceSetting", "CodeableConcept", "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 0, 1, practiceSetting); 1134 case 2031381048: /*sourcePatientInfo*/ return new Property("sourcePatientInfo", "Reference(Patient)", "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 0, 1, sourcePatientInfo); 1135 case 1090493483: /*related*/ return new Property("related", "", "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, related); 1136 default: return super.getNamedProperty(_hash, _name, _checkValid); 1137 } 1138 1139 } 1140 1141 @Override 1142 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1143 switch (hash) { 1144 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1145 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CodeableConcept 1146 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1147 case 370698365: /*facilityType*/ return this.facilityType == null ? new Base[0] : new Base[] {this.facilityType}; // CodeableConcept 1148 case 331373717: /*practiceSetting*/ return this.practiceSetting == null ? new Base[0] : new Base[] {this.practiceSetting}; // CodeableConcept 1149 case 2031381048: /*sourcePatientInfo*/ return this.sourcePatientInfo == null ? new Base[0] : new Base[] {this.sourcePatientInfo}; // Reference 1150 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // DocumentReferenceContextRelatedComponent 1151 default: return super.getProperty(hash, name, checkValid); 1152 } 1153 1154 } 1155 1156 @Override 1157 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1158 switch (hash) { 1159 case 1524132147: // encounter 1160 this.encounter = castToReference(value); // Reference 1161 return value; 1162 case 96891546: // event 1163 this.getEvent().add(castToCodeableConcept(value)); // CodeableConcept 1164 return value; 1165 case -991726143: // period 1166 this.period = castToPeriod(value); // Period 1167 return value; 1168 case 370698365: // facilityType 1169 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1170 return value; 1171 case 331373717: // practiceSetting 1172 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1173 return value; 1174 case 2031381048: // sourcePatientInfo 1175 this.sourcePatientInfo = castToReference(value); // Reference 1176 return value; 1177 case 1090493483: // related 1178 this.getRelated().add((DocumentReferenceContextRelatedComponent) value); // DocumentReferenceContextRelatedComponent 1179 return value; 1180 default: return super.setProperty(hash, name, value); 1181 } 1182 1183 } 1184 1185 @Override 1186 public Base setProperty(String name, Base value) throws FHIRException { 1187 if (name.equals("encounter")) { 1188 this.encounter = castToReference(value); // Reference 1189 } else if (name.equals("event")) { 1190 this.getEvent().add(castToCodeableConcept(value)); 1191 } else if (name.equals("period")) { 1192 this.period = castToPeriod(value); // Period 1193 } else if (name.equals("facilityType")) { 1194 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1195 } else if (name.equals("practiceSetting")) { 1196 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1197 } else if (name.equals("sourcePatientInfo")) { 1198 this.sourcePatientInfo = castToReference(value); // Reference 1199 } else if (name.equals("related")) { 1200 this.getRelated().add((DocumentReferenceContextRelatedComponent) value); 1201 } else 1202 return super.setProperty(name, value); 1203 return value; 1204 } 1205 1206 @Override 1207 public Base makeProperty(int hash, String name) throws FHIRException { 1208 switch (hash) { 1209 case 1524132147: return getEncounter(); 1210 case 96891546: return addEvent(); 1211 case -991726143: return getPeriod(); 1212 case 370698365: return getFacilityType(); 1213 case 331373717: return getPracticeSetting(); 1214 case 2031381048: return getSourcePatientInfo(); 1215 case 1090493483: return addRelated(); 1216 default: return super.makeProperty(hash, name); 1217 } 1218 1219 } 1220 1221 @Override 1222 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1223 switch (hash) { 1224 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1225 case 96891546: /*event*/ return new String[] {"CodeableConcept"}; 1226 case -991726143: /*period*/ return new String[] {"Period"}; 1227 case 370698365: /*facilityType*/ return new String[] {"CodeableConcept"}; 1228 case 331373717: /*practiceSetting*/ return new String[] {"CodeableConcept"}; 1229 case 2031381048: /*sourcePatientInfo*/ return new String[] {"Reference"}; 1230 case 1090493483: /*related*/ return new String[] {}; 1231 default: return super.getTypesForProperty(hash, name); 1232 } 1233 1234 } 1235 1236 @Override 1237 public Base addChild(String name) throws FHIRException { 1238 if (name.equals("encounter")) { 1239 this.encounter = new Reference(); 1240 return this.encounter; 1241 } 1242 else if (name.equals("event")) { 1243 return addEvent(); 1244 } 1245 else if (name.equals("period")) { 1246 this.period = new Period(); 1247 return this.period; 1248 } 1249 else if (name.equals("facilityType")) { 1250 this.facilityType = new CodeableConcept(); 1251 return this.facilityType; 1252 } 1253 else if (name.equals("practiceSetting")) { 1254 this.practiceSetting = new CodeableConcept(); 1255 return this.practiceSetting; 1256 } 1257 else if (name.equals("sourcePatientInfo")) { 1258 this.sourcePatientInfo = new Reference(); 1259 return this.sourcePatientInfo; 1260 } 1261 else if (name.equals("related")) { 1262 return addRelated(); 1263 } 1264 else 1265 return super.addChild(name); 1266 } 1267 1268 public DocumentReferenceContextComponent copy() { 1269 DocumentReferenceContextComponent dst = new DocumentReferenceContextComponent(); 1270 copyValues(dst); 1271 dst.encounter = encounter == null ? null : encounter.copy(); 1272 if (event != null) { 1273 dst.event = new ArrayList<CodeableConcept>(); 1274 for (CodeableConcept i : event) 1275 dst.event.add(i.copy()); 1276 }; 1277 dst.period = period == null ? null : period.copy(); 1278 dst.facilityType = facilityType == null ? null : facilityType.copy(); 1279 dst.practiceSetting = practiceSetting == null ? null : practiceSetting.copy(); 1280 dst.sourcePatientInfo = sourcePatientInfo == null ? null : sourcePatientInfo.copy(); 1281 if (related != null) { 1282 dst.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 1283 for (DocumentReferenceContextRelatedComponent i : related) 1284 dst.related.add(i.copy()); 1285 }; 1286 return dst; 1287 } 1288 1289 @Override 1290 public boolean equalsDeep(Base other_) { 1291 if (!super.equalsDeep(other_)) 1292 return false; 1293 if (!(other_ instanceof DocumentReferenceContextComponent)) 1294 return false; 1295 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1296 return compareDeep(encounter, o.encounter, true) && compareDeep(event, o.event, true) && compareDeep(period, o.period, true) 1297 && compareDeep(facilityType, o.facilityType, true) && compareDeep(practiceSetting, o.practiceSetting, true) 1298 && compareDeep(sourcePatientInfo, o.sourcePatientInfo, true) && compareDeep(related, o.related, true) 1299 ; 1300 } 1301 1302 @Override 1303 public boolean equalsShallow(Base other_) { 1304 if (!super.equalsShallow(other_)) 1305 return false; 1306 if (!(other_ instanceof DocumentReferenceContextComponent)) 1307 return false; 1308 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1309 return true; 1310 } 1311 1312 public boolean isEmpty() { 1313 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(encounter, event, period 1314 , facilityType, practiceSetting, sourcePatientInfo, related); 1315 } 1316 1317 public String fhirType() { 1318 return "DocumentReference.context"; 1319 1320 } 1321 1322 } 1323 1324 @Block() 1325 public static class DocumentReferenceContextRelatedComponent extends BackboneElement implements IBaseBackboneElement { 1326 /** 1327 * Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing. 1328 */ 1329 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1330 @Description(shortDefinition="Identifier of related objects or events", formalDefinition="Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing." ) 1331 protected Identifier identifier; 1332 1333 /** 1334 * Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing. 1335 */ 1336 @Child(name = "ref", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=true) 1337 @Description(shortDefinition="Related Resource", formalDefinition="Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing." ) 1338 protected Reference ref; 1339 1340 /** 1341 * The actual object that is the target of the reference (Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.) 1342 */ 1343 protected Resource refTarget; 1344 1345 private static final long serialVersionUID = -1670123330L; 1346 1347 /** 1348 * Constructor 1349 */ 1350 public DocumentReferenceContextRelatedComponent() { 1351 super(); 1352 } 1353 1354 /** 1355 * @return {@link #identifier} (Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing.) 1356 */ 1357 public Identifier getIdentifier() { 1358 if (this.identifier == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create DocumentReferenceContextRelatedComponent.identifier"); 1361 else if (Configuration.doAutoCreate()) 1362 this.identifier = new Identifier(); // cc 1363 return this.identifier; 1364 } 1365 1366 public boolean hasIdentifier() { 1367 return this.identifier != null && !this.identifier.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #identifier} (Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing.) 1372 */ 1373 public DocumentReferenceContextRelatedComponent setIdentifier(Identifier value) { 1374 this.identifier = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #ref} (Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.) 1380 */ 1381 public Reference getRef() { 1382 if (this.ref == null) 1383 if (Configuration.errorOnAutoCreate()) 1384 throw new Error("Attempt to auto-create DocumentReferenceContextRelatedComponent.ref"); 1385 else if (Configuration.doAutoCreate()) 1386 this.ref = new Reference(); // cc 1387 return this.ref; 1388 } 1389 1390 public boolean hasRef() { 1391 return this.ref != null && !this.ref.isEmpty(); 1392 } 1393 1394 /** 1395 * @param value {@link #ref} (Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.) 1396 */ 1397 public DocumentReferenceContextRelatedComponent setRef(Reference value) { 1398 this.ref = value; 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #ref} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.) 1404 */ 1405 public Resource getRefTarget() { 1406 return this.refTarget; 1407 } 1408 1409 /** 1410 * @param value {@link #ref} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.) 1411 */ 1412 public DocumentReferenceContextRelatedComponent setRefTarget(Resource value) { 1413 this.refTarget = value; 1414 return this; 1415 } 1416 1417 protected void listChildren(List<Property> children) { 1418 super.listChildren(children); 1419 children.add(new Property("identifier", "Identifier", "Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing.", 0, 1, identifier)); 1420 children.add(new Property("ref", "Reference(Any)", "Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.", 0, 1, ref)); 1421 } 1422 1423 @Override 1424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1425 switch (_hash) { 1426 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing.", 0, 1, identifier); 1427 case 112787: /*ref*/ return new Property("ref", "Reference(Any)", "Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.", 0, 1, ref); 1428 default: return super.getNamedProperty(_hash, _name, _checkValid); 1429 } 1430 1431 } 1432 1433 @Override 1434 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1435 switch (hash) { 1436 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1437 case 112787: /*ref*/ return this.ref == null ? new Base[0] : new Base[] {this.ref}; // Reference 1438 default: return super.getProperty(hash, name, checkValid); 1439 } 1440 1441 } 1442 1443 @Override 1444 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1445 switch (hash) { 1446 case -1618432855: // identifier 1447 this.identifier = castToIdentifier(value); // Identifier 1448 return value; 1449 case 112787: // ref 1450 this.ref = castToReference(value); // Reference 1451 return value; 1452 default: return super.setProperty(hash, name, value); 1453 } 1454 1455 } 1456 1457 @Override 1458 public Base setProperty(String name, Base value) throws FHIRException { 1459 if (name.equals("identifier")) { 1460 this.identifier = castToIdentifier(value); // Identifier 1461 } else if (name.equals("ref")) { 1462 this.ref = castToReference(value); // Reference 1463 } else 1464 return super.setProperty(name, value); 1465 return value; 1466 } 1467 1468 @Override 1469 public Base makeProperty(int hash, String name) throws FHIRException { 1470 switch (hash) { 1471 case -1618432855: return getIdentifier(); 1472 case 112787: return getRef(); 1473 default: return super.makeProperty(hash, name); 1474 } 1475 1476 } 1477 1478 @Override 1479 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1480 switch (hash) { 1481 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1482 case 112787: /*ref*/ return new String[] {"Reference"}; 1483 default: return super.getTypesForProperty(hash, name); 1484 } 1485 1486 } 1487 1488 @Override 1489 public Base addChild(String name) throws FHIRException { 1490 if (name.equals("identifier")) { 1491 this.identifier = new Identifier(); 1492 return this.identifier; 1493 } 1494 else if (name.equals("ref")) { 1495 this.ref = new Reference(); 1496 return this.ref; 1497 } 1498 else 1499 return super.addChild(name); 1500 } 1501 1502 public DocumentReferenceContextRelatedComponent copy() { 1503 DocumentReferenceContextRelatedComponent dst = new DocumentReferenceContextRelatedComponent(); 1504 copyValues(dst); 1505 dst.identifier = identifier == null ? null : identifier.copy(); 1506 dst.ref = ref == null ? null : ref.copy(); 1507 return dst; 1508 } 1509 1510 @Override 1511 public boolean equalsDeep(Base other_) { 1512 if (!super.equalsDeep(other_)) 1513 return false; 1514 if (!(other_ instanceof DocumentReferenceContextRelatedComponent)) 1515 return false; 1516 DocumentReferenceContextRelatedComponent o = (DocumentReferenceContextRelatedComponent) other_; 1517 return compareDeep(identifier, o.identifier, true) && compareDeep(ref, o.ref, true); 1518 } 1519 1520 @Override 1521 public boolean equalsShallow(Base other_) { 1522 if (!super.equalsShallow(other_)) 1523 return false; 1524 if (!(other_ instanceof DocumentReferenceContextRelatedComponent)) 1525 return false; 1526 DocumentReferenceContextRelatedComponent o = (DocumentReferenceContextRelatedComponent) other_; 1527 return true; 1528 } 1529 1530 public boolean isEmpty() { 1531 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, ref); 1532 } 1533 1534 public String fhirType() { 1535 return "DocumentReference.context.related"; 1536 1537 } 1538 1539 } 1540 1541 /** 1542 * Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document. 1543 */ 1544 @Child(name = "masterIdentifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 1545 @Description(shortDefinition="Master Version Specific Identifier", formalDefinition="Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document." ) 1546 protected Identifier masterIdentifier; 1547 1548 /** 1549 * Other identifiers associated with the document, including version independent identifiers. 1550 */ 1551 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1552 @Description(shortDefinition="Other identifiers for the document", formalDefinition="Other identifiers associated with the document, including version independent identifiers." ) 1553 protected List<Identifier> identifier; 1554 1555 /** 1556 * The status of this document reference. 1557 */ 1558 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1559 @Description(shortDefinition="current | superseded | entered-in-error", formalDefinition="The status of this document reference." ) 1560 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-reference-status") 1561 protected Enumeration<DocumentReferenceStatus> status; 1562 1563 /** 1564 * The status of the underlying document. 1565 */ 1566 @Child(name = "docStatus", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1567 @Description(shortDefinition="preliminary | final | appended | amended | entered-in-error", formalDefinition="The status of the underlying document." ) 1568 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composition-status") 1569 protected Enumeration<ReferredDocumentStatus> docStatus; 1570 1571 /** 1572 * Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced. 1573 */ 1574 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 1575 @Description(shortDefinition="Kind of document (LOINC if possible)", formalDefinition="Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced." ) 1576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-doc-typecodes") 1577 protected CodeableConcept type; 1578 1579 /** 1580 * A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type. 1581 */ 1582 @Child(name = "class", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 1583 @Description(shortDefinition="Categorization of document", formalDefinition="A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type." ) 1584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-doc-classcodes") 1585 protected CodeableConcept class_; 1586 1587 /** 1588 * Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). 1589 */ 1590 @Child(name = "subject", type = {Patient.class, Practitioner.class, Group.class, Device.class}, order=6, min=0, max=1, modifier=false, summary=true) 1591 @Description(shortDefinition="Who/what is the subject of the document", formalDefinition="Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure)." ) 1592 protected Reference subject; 1593 1594 /** 1595 * The actual object that is the target of the reference (Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).) 1596 */ 1597 protected Resource subjectTarget; 1598 1599 /** 1600 * When the document was created. 1601 */ 1602 @Child(name = "created", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1603 @Description(shortDefinition="Document creation time", formalDefinition="When the document was created." ) 1604 protected DateTimeType created; 1605 1606 /** 1607 * When the document reference was created. 1608 */ 1609 @Child(name = "indexed", type = {InstantType.class}, order=8, min=1, max=1, modifier=false, summary=true) 1610 @Description(shortDefinition="When this document reference was created", formalDefinition="When the document reference was created." ) 1611 protected InstantType indexed; 1612 1613 /** 1614 * Identifies who is responsible for adding the information to the document. 1615 */ 1616 @Child(name = "author", type = {Practitioner.class, Organization.class, Device.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1617 @Description(shortDefinition="Who and/or what authored the document", formalDefinition="Identifies who is responsible for adding the information to the document." ) 1618 protected List<Reference> author; 1619 /** 1620 * The actual objects that are the target of the reference (Identifies who is responsible for adding the information to the document.) 1621 */ 1622 protected List<Resource> authorTarget; 1623 1624 1625 /** 1626 * Which person or organization authenticates that this document is valid. 1627 */ 1628 @Child(name = "authenticator", type = {Practitioner.class, Organization.class}, order=10, min=0, max=1, modifier=false, summary=true) 1629 @Description(shortDefinition="Who/what authenticated the document", formalDefinition="Which person or organization authenticates that this document is valid." ) 1630 protected Reference authenticator; 1631 1632 /** 1633 * The actual object that is the target of the reference (Which person or organization authenticates that this document is valid.) 1634 */ 1635 protected Resource authenticatorTarget; 1636 1637 /** 1638 * Identifies the organization or group who is responsible for ongoing maintenance of and access to the document. 1639 */ 1640 @Child(name = "custodian", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 1641 @Description(shortDefinition="Organization which maintains the document", formalDefinition="Identifies the organization or group who is responsible for ongoing maintenance of and access to the document." ) 1642 protected Reference custodian; 1643 1644 /** 1645 * The actual object that is the target of the reference (Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.) 1646 */ 1647 protected Organization custodianTarget; 1648 1649 /** 1650 * Relationships that this document has with other document references that already exist. 1651 */ 1652 @Child(name = "relatesTo", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 1653 @Description(shortDefinition="Relationships to other documents", formalDefinition="Relationships that this document has with other document references that already exist." ) 1654 protected List<DocumentReferenceRelatesToComponent> relatesTo; 1655 1656 /** 1657 * Human-readable description of the source document. This is sometimes known as the "title". 1658 */ 1659 @Child(name = "description", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1660 @Description(shortDefinition="Human-readable description (title)", formalDefinition="Human-readable description of the source document. This is sometimes known as the \"title\"." ) 1661 protected StringType description; 1662 1663 /** 1664 * A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the "reference" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to. 1665 */ 1666 @Child(name = "securityLabel", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1667 @Description(shortDefinition="Document security-tags", formalDefinition="A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to." ) 1668 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 1669 protected List<CodeableConcept> securityLabel; 1670 1671 /** 1672 * The document and format referenced. There may be multiple content element repetitions, each with a different format. 1673 */ 1674 @Child(name = "content", type = {}, order=15, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1675 @Description(shortDefinition="Document referenced", formalDefinition="The document and format referenced. There may be multiple content element repetitions, each with a different format." ) 1676 protected List<DocumentReferenceContentComponent> content; 1677 1678 /** 1679 * The clinical context in which the document was prepared. 1680 */ 1681 @Child(name = "context", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 1682 @Description(shortDefinition="Clinical context of document", formalDefinition="The clinical context in which the document was prepared." ) 1683 protected DocumentReferenceContextComponent context; 1684 1685 private static final long serialVersionUID = 1465770040L; 1686 1687 /** 1688 * Constructor 1689 */ 1690 public DocumentReference() { 1691 super(); 1692 } 1693 1694 /** 1695 * Constructor 1696 */ 1697 public DocumentReference(Enumeration<DocumentReferenceStatus> status, CodeableConcept type, InstantType indexed) { 1698 super(); 1699 this.status = status; 1700 this.type = type; 1701 this.indexed = indexed; 1702 } 1703 1704 /** 1705 * @return {@link #masterIdentifier} (Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.) 1706 */ 1707 public Identifier getMasterIdentifier() { 1708 if (this.masterIdentifier == null) 1709 if (Configuration.errorOnAutoCreate()) 1710 throw new Error("Attempt to auto-create DocumentReference.masterIdentifier"); 1711 else if (Configuration.doAutoCreate()) 1712 this.masterIdentifier = new Identifier(); // cc 1713 return this.masterIdentifier; 1714 } 1715 1716 public boolean hasMasterIdentifier() { 1717 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 1718 } 1719 1720 /** 1721 * @param value {@link #masterIdentifier} (Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.) 1722 */ 1723 public DocumentReference setMasterIdentifier(Identifier value) { 1724 this.masterIdentifier = value; 1725 return this; 1726 } 1727 1728 /** 1729 * @return {@link #identifier} (Other identifiers associated with the document, including version independent identifiers.) 1730 */ 1731 public List<Identifier> getIdentifier() { 1732 if (this.identifier == null) 1733 this.identifier = new ArrayList<Identifier>(); 1734 return this.identifier; 1735 } 1736 1737 /** 1738 * @return Returns a reference to <code>this</code> for easy method chaining 1739 */ 1740 public DocumentReference setIdentifier(List<Identifier> theIdentifier) { 1741 this.identifier = theIdentifier; 1742 return this; 1743 } 1744 1745 public boolean hasIdentifier() { 1746 if (this.identifier == null) 1747 return false; 1748 for (Identifier item : this.identifier) 1749 if (!item.isEmpty()) 1750 return true; 1751 return false; 1752 } 1753 1754 public Identifier addIdentifier() { //3 1755 Identifier t = new Identifier(); 1756 if (this.identifier == null) 1757 this.identifier = new ArrayList<Identifier>(); 1758 this.identifier.add(t); 1759 return t; 1760 } 1761 1762 public DocumentReference addIdentifier(Identifier t) { //3 1763 if (t == null) 1764 return this; 1765 if (this.identifier == null) 1766 this.identifier = new ArrayList<Identifier>(); 1767 this.identifier.add(t); 1768 return this; 1769 } 1770 1771 /** 1772 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1773 */ 1774 public Identifier getIdentifierFirstRep() { 1775 if (getIdentifier().isEmpty()) { 1776 addIdentifier(); 1777 } 1778 return getIdentifier().get(0); 1779 } 1780 1781 /** 1782 * @return {@link #status} (The status of this document reference.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1783 */ 1784 public Enumeration<DocumentReferenceStatus> getStatusElement() { 1785 if (this.status == null) 1786 if (Configuration.errorOnAutoCreate()) 1787 throw new Error("Attempt to auto-create DocumentReference.status"); 1788 else if (Configuration.doAutoCreate()) 1789 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 1790 return this.status; 1791 } 1792 1793 public boolean hasStatusElement() { 1794 return this.status != null && !this.status.isEmpty(); 1795 } 1796 1797 public boolean hasStatus() { 1798 return this.status != null && !this.status.isEmpty(); 1799 } 1800 1801 /** 1802 * @param value {@link #status} (The status of this document reference.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1803 */ 1804 public DocumentReference setStatusElement(Enumeration<DocumentReferenceStatus> value) { 1805 this.status = value; 1806 return this; 1807 } 1808 1809 /** 1810 * @return The status of this document reference. 1811 */ 1812 public DocumentReferenceStatus getStatus() { 1813 return this.status == null ? null : this.status.getValue(); 1814 } 1815 1816 /** 1817 * @param value The status of this document reference. 1818 */ 1819 public DocumentReference setStatus(DocumentReferenceStatus value) { 1820 if (this.status == null) 1821 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 1822 this.status.setValue(value); 1823 return this; 1824 } 1825 1826 /** 1827 * @return {@link #docStatus} (The status of the underlying document.). This is the underlying object with id, value and extensions. The accessor "getDocStatus" gives direct access to the value 1828 */ 1829 public Enumeration<ReferredDocumentStatus> getDocStatusElement() { 1830 if (this.docStatus == null) 1831 if (Configuration.errorOnAutoCreate()) 1832 throw new Error("Attempt to auto-create DocumentReference.docStatus"); 1833 else if (Configuration.doAutoCreate()) 1834 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); // bb 1835 return this.docStatus; 1836 } 1837 1838 public boolean hasDocStatusElement() { 1839 return this.docStatus != null && !this.docStatus.isEmpty(); 1840 } 1841 1842 public boolean hasDocStatus() { 1843 return this.docStatus != null && !this.docStatus.isEmpty(); 1844 } 1845 1846 /** 1847 * @param value {@link #docStatus} (The status of the underlying document.). This is the underlying object with id, value and extensions. The accessor "getDocStatus" gives direct access to the value 1848 */ 1849 public DocumentReference setDocStatusElement(Enumeration<ReferredDocumentStatus> value) { 1850 this.docStatus = value; 1851 return this; 1852 } 1853 1854 /** 1855 * @return The status of the underlying document. 1856 */ 1857 public ReferredDocumentStatus getDocStatus() { 1858 return this.docStatus == null ? null : this.docStatus.getValue(); 1859 } 1860 1861 /** 1862 * @param value The status of the underlying document. 1863 */ 1864 public DocumentReference setDocStatus(ReferredDocumentStatus value) { 1865 if (value == null) 1866 this.docStatus = null; 1867 else { 1868 if (this.docStatus == null) 1869 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); 1870 this.docStatus.setValue(value); 1871 } 1872 return this; 1873 } 1874 1875 /** 1876 * @return {@link #type} (Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.) 1877 */ 1878 public CodeableConcept getType() { 1879 if (this.type == null) 1880 if (Configuration.errorOnAutoCreate()) 1881 throw new Error("Attempt to auto-create DocumentReference.type"); 1882 else if (Configuration.doAutoCreate()) 1883 this.type = new CodeableConcept(); // cc 1884 return this.type; 1885 } 1886 1887 public boolean hasType() { 1888 return this.type != null && !this.type.isEmpty(); 1889 } 1890 1891 /** 1892 * @param value {@link #type} (Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.) 1893 */ 1894 public DocumentReference setType(CodeableConcept value) { 1895 this.type = value; 1896 return this; 1897 } 1898 1899 /** 1900 * @return {@link #class_} (A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.) 1901 */ 1902 public CodeableConcept getClass_() { 1903 if (this.class_ == null) 1904 if (Configuration.errorOnAutoCreate()) 1905 throw new Error("Attempt to auto-create DocumentReference.class_"); 1906 else if (Configuration.doAutoCreate()) 1907 this.class_ = new CodeableConcept(); // cc 1908 return this.class_; 1909 } 1910 1911 public boolean hasClass_() { 1912 return this.class_ != null && !this.class_.isEmpty(); 1913 } 1914 1915 /** 1916 * @param value {@link #class_} (A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.) 1917 */ 1918 public DocumentReference setClass_(CodeableConcept value) { 1919 this.class_ = value; 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #subject} (Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).) 1925 */ 1926 public Reference getSubject() { 1927 if (this.subject == null) 1928 if (Configuration.errorOnAutoCreate()) 1929 throw new Error("Attempt to auto-create DocumentReference.subject"); 1930 else if (Configuration.doAutoCreate()) 1931 this.subject = new Reference(); // cc 1932 return this.subject; 1933 } 1934 1935 public boolean hasSubject() { 1936 return this.subject != null && !this.subject.isEmpty(); 1937 } 1938 1939 /** 1940 * @param value {@link #subject} (Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).) 1941 */ 1942 public DocumentReference setSubject(Reference value) { 1943 this.subject = value; 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).) 1949 */ 1950 public Resource getSubjectTarget() { 1951 return this.subjectTarget; 1952 } 1953 1954 /** 1955 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).) 1956 */ 1957 public DocumentReference setSubjectTarget(Resource value) { 1958 this.subjectTarget = value; 1959 return this; 1960 } 1961 1962 /** 1963 * @return {@link #created} (When the document was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1964 */ 1965 public DateTimeType getCreatedElement() { 1966 if (this.created == null) 1967 if (Configuration.errorOnAutoCreate()) 1968 throw new Error("Attempt to auto-create DocumentReference.created"); 1969 else if (Configuration.doAutoCreate()) 1970 this.created = new DateTimeType(); // bb 1971 return this.created; 1972 } 1973 1974 public boolean hasCreatedElement() { 1975 return this.created != null && !this.created.isEmpty(); 1976 } 1977 1978 public boolean hasCreated() { 1979 return this.created != null && !this.created.isEmpty(); 1980 } 1981 1982 /** 1983 * @param value {@link #created} (When the document was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1984 */ 1985 public DocumentReference setCreatedElement(DateTimeType value) { 1986 this.created = value; 1987 return this; 1988 } 1989 1990 /** 1991 * @return When the document was created. 1992 */ 1993 public Date getCreated() { 1994 return this.created == null ? null : this.created.getValue(); 1995 } 1996 1997 /** 1998 * @param value When the document was created. 1999 */ 2000 public DocumentReference setCreated(Date value) { 2001 if (value == null) 2002 this.created = null; 2003 else { 2004 if (this.created == null) 2005 this.created = new DateTimeType(); 2006 this.created.setValue(value); 2007 } 2008 return this; 2009 } 2010 2011 /** 2012 * @return {@link #indexed} (When the document reference was created.). This is the underlying object with id, value and extensions. The accessor "getIndexed" gives direct access to the value 2013 */ 2014 public InstantType getIndexedElement() { 2015 if (this.indexed == null) 2016 if (Configuration.errorOnAutoCreate()) 2017 throw new Error("Attempt to auto-create DocumentReference.indexed"); 2018 else if (Configuration.doAutoCreate()) 2019 this.indexed = new InstantType(); // bb 2020 return this.indexed; 2021 } 2022 2023 public boolean hasIndexedElement() { 2024 return this.indexed != null && !this.indexed.isEmpty(); 2025 } 2026 2027 public boolean hasIndexed() { 2028 return this.indexed != null && !this.indexed.isEmpty(); 2029 } 2030 2031 /** 2032 * @param value {@link #indexed} (When the document reference was created.). This is the underlying object with id, value and extensions. The accessor "getIndexed" gives direct access to the value 2033 */ 2034 public DocumentReference setIndexedElement(InstantType value) { 2035 this.indexed = value; 2036 return this; 2037 } 2038 2039 /** 2040 * @return When the document reference was created. 2041 */ 2042 public Date getIndexed() { 2043 return this.indexed == null ? null : this.indexed.getValue(); 2044 } 2045 2046 /** 2047 * @param value When the document reference was created. 2048 */ 2049 public DocumentReference setIndexed(Date value) { 2050 if (this.indexed == null) 2051 this.indexed = new InstantType(); 2052 this.indexed.setValue(value); 2053 return this; 2054 } 2055 2056 /** 2057 * @return {@link #author} (Identifies who is responsible for adding the information to the document.) 2058 */ 2059 public List<Reference> getAuthor() { 2060 if (this.author == null) 2061 this.author = new ArrayList<Reference>(); 2062 return this.author; 2063 } 2064 2065 /** 2066 * @return Returns a reference to <code>this</code> for easy method chaining 2067 */ 2068 public DocumentReference setAuthor(List<Reference> theAuthor) { 2069 this.author = theAuthor; 2070 return this; 2071 } 2072 2073 public boolean hasAuthor() { 2074 if (this.author == null) 2075 return false; 2076 for (Reference item : this.author) 2077 if (!item.isEmpty()) 2078 return true; 2079 return false; 2080 } 2081 2082 public Reference addAuthor() { //3 2083 Reference t = new Reference(); 2084 if (this.author == null) 2085 this.author = new ArrayList<Reference>(); 2086 this.author.add(t); 2087 return t; 2088 } 2089 2090 public DocumentReference addAuthor(Reference t) { //3 2091 if (t == null) 2092 return this; 2093 if (this.author == null) 2094 this.author = new ArrayList<Reference>(); 2095 this.author.add(t); 2096 return this; 2097 } 2098 2099 /** 2100 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist 2101 */ 2102 public Reference getAuthorFirstRep() { 2103 if (getAuthor().isEmpty()) { 2104 addAuthor(); 2105 } 2106 return getAuthor().get(0); 2107 } 2108 2109 /** 2110 * @deprecated Use Reference#setResource(IBaseResource) instead 2111 */ 2112 @Deprecated 2113 public List<Resource> getAuthorTarget() { 2114 if (this.authorTarget == null) 2115 this.authorTarget = new ArrayList<Resource>(); 2116 return this.authorTarget; 2117 } 2118 2119 /** 2120 * @return {@link #authenticator} (Which person or organization authenticates that this document is valid.) 2121 */ 2122 public Reference getAuthenticator() { 2123 if (this.authenticator == null) 2124 if (Configuration.errorOnAutoCreate()) 2125 throw new Error("Attempt to auto-create DocumentReference.authenticator"); 2126 else if (Configuration.doAutoCreate()) 2127 this.authenticator = new Reference(); // cc 2128 return this.authenticator; 2129 } 2130 2131 public boolean hasAuthenticator() { 2132 return this.authenticator != null && !this.authenticator.isEmpty(); 2133 } 2134 2135 /** 2136 * @param value {@link #authenticator} (Which person or organization authenticates that this document is valid.) 2137 */ 2138 public DocumentReference setAuthenticator(Reference value) { 2139 this.authenticator = value; 2140 return this; 2141 } 2142 2143 /** 2144 * @return {@link #authenticator} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Which person or organization authenticates that this document is valid.) 2145 */ 2146 public Resource getAuthenticatorTarget() { 2147 return this.authenticatorTarget; 2148 } 2149 2150 /** 2151 * @param value {@link #authenticator} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Which person or organization authenticates that this document is valid.) 2152 */ 2153 public DocumentReference setAuthenticatorTarget(Resource value) { 2154 this.authenticatorTarget = value; 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.) 2160 */ 2161 public Reference getCustodian() { 2162 if (this.custodian == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2165 else if (Configuration.doAutoCreate()) 2166 this.custodian = new Reference(); // cc 2167 return this.custodian; 2168 } 2169 2170 public boolean hasCustodian() { 2171 return this.custodian != null && !this.custodian.isEmpty(); 2172 } 2173 2174 /** 2175 * @param value {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.) 2176 */ 2177 public DocumentReference setCustodian(Reference value) { 2178 this.custodian = value; 2179 return this; 2180 } 2181 2182 /** 2183 * @return {@link #custodian} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.) 2184 */ 2185 public Organization getCustodianTarget() { 2186 if (this.custodianTarget == null) 2187 if (Configuration.errorOnAutoCreate()) 2188 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2189 else if (Configuration.doAutoCreate()) 2190 this.custodianTarget = new Organization(); // aa 2191 return this.custodianTarget; 2192 } 2193 2194 /** 2195 * @param value {@link #custodian} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.) 2196 */ 2197 public DocumentReference setCustodianTarget(Organization value) { 2198 this.custodianTarget = value; 2199 return this; 2200 } 2201 2202 /** 2203 * @return {@link #relatesTo} (Relationships that this document has with other document references that already exist.) 2204 */ 2205 public List<DocumentReferenceRelatesToComponent> getRelatesTo() { 2206 if (this.relatesTo == null) 2207 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2208 return this.relatesTo; 2209 } 2210 2211 /** 2212 * @return Returns a reference to <code>this</code> for easy method chaining 2213 */ 2214 public DocumentReference setRelatesTo(List<DocumentReferenceRelatesToComponent> theRelatesTo) { 2215 this.relatesTo = theRelatesTo; 2216 return this; 2217 } 2218 2219 public boolean hasRelatesTo() { 2220 if (this.relatesTo == null) 2221 return false; 2222 for (DocumentReferenceRelatesToComponent item : this.relatesTo) 2223 if (!item.isEmpty()) 2224 return true; 2225 return false; 2226 } 2227 2228 public DocumentReferenceRelatesToComponent addRelatesTo() { //3 2229 DocumentReferenceRelatesToComponent t = new DocumentReferenceRelatesToComponent(); 2230 if (this.relatesTo == null) 2231 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2232 this.relatesTo.add(t); 2233 return t; 2234 } 2235 2236 public DocumentReference addRelatesTo(DocumentReferenceRelatesToComponent t) { //3 2237 if (t == null) 2238 return this; 2239 if (this.relatesTo == null) 2240 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2241 this.relatesTo.add(t); 2242 return this; 2243 } 2244 2245 /** 2246 * @return The first repetition of repeating field {@link #relatesTo}, creating it if it does not already exist 2247 */ 2248 public DocumentReferenceRelatesToComponent getRelatesToFirstRep() { 2249 if (getRelatesTo().isEmpty()) { 2250 addRelatesTo(); 2251 } 2252 return getRelatesTo().get(0); 2253 } 2254 2255 /** 2256 * @return {@link #description} (Human-readable description of the source document. This is sometimes known as the "title".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2257 */ 2258 public StringType getDescriptionElement() { 2259 if (this.description == null) 2260 if (Configuration.errorOnAutoCreate()) 2261 throw new Error("Attempt to auto-create DocumentReference.description"); 2262 else if (Configuration.doAutoCreate()) 2263 this.description = new StringType(); // bb 2264 return this.description; 2265 } 2266 2267 public boolean hasDescriptionElement() { 2268 return this.description != null && !this.description.isEmpty(); 2269 } 2270 2271 public boolean hasDescription() { 2272 return this.description != null && !this.description.isEmpty(); 2273 } 2274 2275 /** 2276 * @param value {@link #description} (Human-readable description of the source document. This is sometimes known as the "title".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2277 */ 2278 public DocumentReference setDescriptionElement(StringType value) { 2279 this.description = value; 2280 return this; 2281 } 2282 2283 /** 2284 * @return Human-readable description of the source document. This is sometimes known as the "title". 2285 */ 2286 public String getDescription() { 2287 return this.description == null ? null : this.description.getValue(); 2288 } 2289 2290 /** 2291 * @param value Human-readable description of the source document. This is sometimes known as the "title". 2292 */ 2293 public DocumentReference setDescription(String value) { 2294 if (Utilities.noString(value)) 2295 this.description = null; 2296 else { 2297 if (this.description == null) 2298 this.description = new StringType(); 2299 this.description.setValue(value); 2300 } 2301 return this; 2302 } 2303 2304 /** 2305 * @return {@link #securityLabel} (A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the "reference" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.) 2306 */ 2307 public List<CodeableConcept> getSecurityLabel() { 2308 if (this.securityLabel == null) 2309 this.securityLabel = new ArrayList<CodeableConcept>(); 2310 return this.securityLabel; 2311 } 2312 2313 /** 2314 * @return Returns a reference to <code>this</code> for easy method chaining 2315 */ 2316 public DocumentReference setSecurityLabel(List<CodeableConcept> theSecurityLabel) { 2317 this.securityLabel = theSecurityLabel; 2318 return this; 2319 } 2320 2321 public boolean hasSecurityLabel() { 2322 if (this.securityLabel == null) 2323 return false; 2324 for (CodeableConcept item : this.securityLabel) 2325 if (!item.isEmpty()) 2326 return true; 2327 return false; 2328 } 2329 2330 public CodeableConcept addSecurityLabel() { //3 2331 CodeableConcept t = new CodeableConcept(); 2332 if (this.securityLabel == null) 2333 this.securityLabel = new ArrayList<CodeableConcept>(); 2334 this.securityLabel.add(t); 2335 return t; 2336 } 2337 2338 public DocumentReference addSecurityLabel(CodeableConcept t) { //3 2339 if (t == null) 2340 return this; 2341 if (this.securityLabel == null) 2342 this.securityLabel = new ArrayList<CodeableConcept>(); 2343 this.securityLabel.add(t); 2344 return this; 2345 } 2346 2347 /** 2348 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist 2349 */ 2350 public CodeableConcept getSecurityLabelFirstRep() { 2351 if (getSecurityLabel().isEmpty()) { 2352 addSecurityLabel(); 2353 } 2354 return getSecurityLabel().get(0); 2355 } 2356 2357 /** 2358 * @return {@link #content} (The document and format referenced. There may be multiple content element repetitions, each with a different format.) 2359 */ 2360 public List<DocumentReferenceContentComponent> getContent() { 2361 if (this.content == null) 2362 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2363 return this.content; 2364 } 2365 2366 /** 2367 * @return Returns a reference to <code>this</code> for easy method chaining 2368 */ 2369 public DocumentReference setContent(List<DocumentReferenceContentComponent> theContent) { 2370 this.content = theContent; 2371 return this; 2372 } 2373 2374 public boolean hasContent() { 2375 if (this.content == null) 2376 return false; 2377 for (DocumentReferenceContentComponent item : this.content) 2378 if (!item.isEmpty()) 2379 return true; 2380 return false; 2381 } 2382 2383 public DocumentReferenceContentComponent addContent() { //3 2384 DocumentReferenceContentComponent t = new DocumentReferenceContentComponent(); 2385 if (this.content == null) 2386 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2387 this.content.add(t); 2388 return t; 2389 } 2390 2391 public DocumentReference addContent(DocumentReferenceContentComponent t) { //3 2392 if (t == null) 2393 return this; 2394 if (this.content == null) 2395 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2396 this.content.add(t); 2397 return this; 2398 } 2399 2400 /** 2401 * @return The first repetition of repeating field {@link #content}, creating it if it does not already exist 2402 */ 2403 public DocumentReferenceContentComponent getContentFirstRep() { 2404 if (getContent().isEmpty()) { 2405 addContent(); 2406 } 2407 return getContent().get(0); 2408 } 2409 2410 /** 2411 * @return {@link #context} (The clinical context in which the document was prepared.) 2412 */ 2413 public DocumentReferenceContextComponent getContext() { 2414 if (this.context == null) 2415 if (Configuration.errorOnAutoCreate()) 2416 throw new Error("Attempt to auto-create DocumentReference.context"); 2417 else if (Configuration.doAutoCreate()) 2418 this.context = new DocumentReferenceContextComponent(); // cc 2419 return this.context; 2420 } 2421 2422 public boolean hasContext() { 2423 return this.context != null && !this.context.isEmpty(); 2424 } 2425 2426 /** 2427 * @param value {@link #context} (The clinical context in which the document was prepared.) 2428 */ 2429 public DocumentReference setContext(DocumentReferenceContextComponent value) { 2430 this.context = value; 2431 return this; 2432 } 2433 2434 protected void listChildren(List<Property> children) { 2435 super.listChildren(children); 2436 children.add(new Property("masterIdentifier", "Identifier", "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 0, 1, masterIdentifier)); 2437 children.add(new Property("identifier", "Identifier", "Other identifiers associated with the document, including version independent identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2438 children.add(new Property("status", "code", "The status of this document reference.", 0, 1, status)); 2439 children.add(new Property("docStatus", "code", "The status of the underlying document.", 0, 1, docStatus)); 2440 children.add(new Property("type", "CodeableConcept", "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 0, 1, type)); 2441 children.add(new Property("class", "CodeableConcept", "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 0, 1, class_)); 2442 children.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 0, 1, subject)); 2443 children.add(new Property("created", "dateTime", "When the document was created.", 0, 1, created)); 2444 children.add(new Property("indexed", "instant", "When the document reference was created.", 0, 1, indexed)); 2445 children.add(new Property("author", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, author)); 2446 children.add(new Property("authenticator", "Reference(Practitioner|Organization)", "Which person or organization authenticates that this document is valid.", 0, 1, authenticator)); 2447 children.add(new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 0, 1, custodian)); 2448 children.add(new Property("relatesTo", "", "Relationships that this document has with other document references that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo)); 2449 children.add(new Property("description", "string", "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 1, description)); 2450 children.add(new Property("securityLabel", "CodeableConcept", "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2451 children.add(new Property("content", "", "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 0, java.lang.Integer.MAX_VALUE, content)); 2452 children.add(new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, context)); 2453 } 2454 2455 @Override 2456 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2457 switch (_hash) { 2458 case 243769515: /*masterIdentifier*/ return new Property("masterIdentifier", "Identifier", "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 0, 1, masterIdentifier); 2459 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Other identifiers associated with the document, including version independent identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier); 2460 case -892481550: /*status*/ return new Property("status", "code", "The status of this document reference.", 0, 1, status); 2461 case -23496886: /*docStatus*/ return new Property("docStatus", "code", "The status of the underlying document.", 0, 1, docStatus); 2462 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 0, 1, type); 2463 case 94742904: /*class*/ return new Property("class", "CodeableConcept", "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 0, 1, class_); 2464 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Practitioner|Group|Device)", "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 0, 1, subject); 2465 case 1028554472: /*created*/ return new Property("created", "dateTime", "When the document was created.", 0, 1, created); 2466 case 1943292145: /*indexed*/ return new Property("indexed", "instant", "When the document reference was created.", 0, 1, indexed); 2467 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, author); 2468 case 1815000435: /*authenticator*/ return new Property("authenticator", "Reference(Practitioner|Organization)", "Which person or organization authenticates that this document is valid.", 0, 1, authenticator); 2469 case 1611297262: /*custodian*/ return new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 0, 1, custodian); 2470 case -7765931: /*relatesTo*/ return new Property("relatesTo", "", "Relationships that this document has with other document references that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo); 2471 case -1724546052: /*description*/ return new Property("description", "string", "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 1, description); 2472 case -722296940: /*securityLabel*/ return new Property("securityLabel", "CodeableConcept", "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 2473 case 951530617: /*content*/ return new Property("content", "", "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 0, java.lang.Integer.MAX_VALUE, content); 2474 case 951530927: /*context*/ return new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, context); 2475 default: return super.getNamedProperty(_hash, _name, _checkValid); 2476 } 2477 2478 } 2479 2480 @Override 2481 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2482 switch (hash) { 2483 case 243769515: /*masterIdentifier*/ return this.masterIdentifier == null ? new Base[0] : new Base[] {this.masterIdentifier}; // Identifier 2484 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2485 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DocumentReferenceStatus> 2486 case -23496886: /*docStatus*/ return this.docStatus == null ? new Base[0] : new Base[] {this.docStatus}; // Enumeration<ReferredDocumentStatus> 2487 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2488 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // CodeableConcept 2489 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2490 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 2491 case 1943292145: /*indexed*/ return this.indexed == null ? new Base[0] : new Base[] {this.indexed}; // InstantType 2492 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2493 case 1815000435: /*authenticator*/ return this.authenticator == null ? new Base[0] : new Base[] {this.authenticator}; // Reference 2494 case 1611297262: /*custodian*/ return this.custodian == null ? new Base[0] : new Base[] {this.custodian}; // Reference 2495 case -7765931: /*relatesTo*/ return this.relatesTo == null ? new Base[0] : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // DocumentReferenceRelatesToComponent 2496 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2497 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // CodeableConcept 2498 case 951530617: /*content*/ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // DocumentReferenceContentComponent 2499 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // DocumentReferenceContextComponent 2500 default: return super.getProperty(hash, name, checkValid); 2501 } 2502 2503 } 2504 2505 @Override 2506 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2507 switch (hash) { 2508 case 243769515: // masterIdentifier 2509 this.masterIdentifier = castToIdentifier(value); // Identifier 2510 return value; 2511 case -1618432855: // identifier 2512 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2513 return value; 2514 case -892481550: // status 2515 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2516 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2517 return value; 2518 case -23496886: // docStatus 2519 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2520 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2521 return value; 2522 case 3575610: // type 2523 this.type = castToCodeableConcept(value); // CodeableConcept 2524 return value; 2525 case 94742904: // class 2526 this.class_ = castToCodeableConcept(value); // CodeableConcept 2527 return value; 2528 case -1867885268: // subject 2529 this.subject = castToReference(value); // Reference 2530 return value; 2531 case 1028554472: // created 2532 this.created = castToDateTime(value); // DateTimeType 2533 return value; 2534 case 1943292145: // indexed 2535 this.indexed = castToInstant(value); // InstantType 2536 return value; 2537 case -1406328437: // author 2538 this.getAuthor().add(castToReference(value)); // Reference 2539 return value; 2540 case 1815000435: // authenticator 2541 this.authenticator = castToReference(value); // Reference 2542 return value; 2543 case 1611297262: // custodian 2544 this.custodian = castToReference(value); // Reference 2545 return value; 2546 case -7765931: // relatesTo 2547 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); // DocumentReferenceRelatesToComponent 2548 return value; 2549 case -1724546052: // description 2550 this.description = castToString(value); // StringType 2551 return value; 2552 case -722296940: // securityLabel 2553 this.getSecurityLabel().add(castToCodeableConcept(value)); // CodeableConcept 2554 return value; 2555 case 951530617: // content 2556 this.getContent().add((DocumentReferenceContentComponent) value); // DocumentReferenceContentComponent 2557 return value; 2558 case 951530927: // context 2559 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2560 return value; 2561 default: return super.setProperty(hash, name, value); 2562 } 2563 2564 } 2565 2566 @Override 2567 public Base setProperty(String name, Base value) throws FHIRException { 2568 if (name.equals("masterIdentifier")) { 2569 this.masterIdentifier = castToIdentifier(value); // Identifier 2570 } else if (name.equals("identifier")) { 2571 this.getIdentifier().add(castToIdentifier(value)); 2572 } else if (name.equals("status")) { 2573 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2574 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2575 } else if (name.equals("docStatus")) { 2576 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2577 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2578 } else if (name.equals("type")) { 2579 this.type = castToCodeableConcept(value); // CodeableConcept 2580 } else if (name.equals("class")) { 2581 this.class_ = castToCodeableConcept(value); // CodeableConcept 2582 } else if (name.equals("subject")) { 2583 this.subject = castToReference(value); // Reference 2584 } else if (name.equals("created")) { 2585 this.created = castToDateTime(value); // DateTimeType 2586 } else if (name.equals("indexed")) { 2587 this.indexed = castToInstant(value); // InstantType 2588 } else if (name.equals("author")) { 2589 this.getAuthor().add(castToReference(value)); 2590 } else if (name.equals("authenticator")) { 2591 this.authenticator = castToReference(value); // Reference 2592 } else if (name.equals("custodian")) { 2593 this.custodian = castToReference(value); // Reference 2594 } else if (name.equals("relatesTo")) { 2595 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); 2596 } else if (name.equals("description")) { 2597 this.description = castToString(value); // StringType 2598 } else if (name.equals("securityLabel")) { 2599 this.getSecurityLabel().add(castToCodeableConcept(value)); 2600 } else if (name.equals("content")) { 2601 this.getContent().add((DocumentReferenceContentComponent) value); 2602 } else if (name.equals("context")) { 2603 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2604 } else 2605 return super.setProperty(name, value); 2606 return value; 2607 } 2608 2609 @Override 2610 public Base makeProperty(int hash, String name) throws FHIRException { 2611 switch (hash) { 2612 case 243769515: return getMasterIdentifier(); 2613 case -1618432855: return addIdentifier(); 2614 case -892481550: return getStatusElement(); 2615 case -23496886: return getDocStatusElement(); 2616 case 3575610: return getType(); 2617 case 94742904: return getClass_(); 2618 case -1867885268: return getSubject(); 2619 case 1028554472: return getCreatedElement(); 2620 case 1943292145: return getIndexedElement(); 2621 case -1406328437: return addAuthor(); 2622 case 1815000435: return getAuthenticator(); 2623 case 1611297262: return getCustodian(); 2624 case -7765931: return addRelatesTo(); 2625 case -1724546052: return getDescriptionElement(); 2626 case -722296940: return addSecurityLabel(); 2627 case 951530617: return addContent(); 2628 case 951530927: return getContext(); 2629 default: return super.makeProperty(hash, name); 2630 } 2631 2632 } 2633 2634 @Override 2635 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2636 switch (hash) { 2637 case 243769515: /*masterIdentifier*/ return new String[] {"Identifier"}; 2638 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2639 case -892481550: /*status*/ return new String[] {"code"}; 2640 case -23496886: /*docStatus*/ return new String[] {"code"}; 2641 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2642 case 94742904: /*class*/ return new String[] {"CodeableConcept"}; 2643 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2644 case 1028554472: /*created*/ return new String[] {"dateTime"}; 2645 case 1943292145: /*indexed*/ return new String[] {"instant"}; 2646 case -1406328437: /*author*/ return new String[] {"Reference"}; 2647 case 1815000435: /*authenticator*/ return new String[] {"Reference"}; 2648 case 1611297262: /*custodian*/ return new String[] {"Reference"}; 2649 case -7765931: /*relatesTo*/ return new String[] {}; 2650 case -1724546052: /*description*/ return new String[] {"string"}; 2651 case -722296940: /*securityLabel*/ return new String[] {"CodeableConcept"}; 2652 case 951530617: /*content*/ return new String[] {}; 2653 case 951530927: /*context*/ return new String[] {}; 2654 default: return super.getTypesForProperty(hash, name); 2655 } 2656 2657 } 2658 2659 @Override 2660 public Base addChild(String name) throws FHIRException { 2661 if (name.equals("masterIdentifier")) { 2662 this.masterIdentifier = new Identifier(); 2663 return this.masterIdentifier; 2664 } 2665 else if (name.equals("identifier")) { 2666 return addIdentifier(); 2667 } 2668 else if (name.equals("status")) { 2669 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.status"); 2670 } 2671 else if (name.equals("docStatus")) { 2672 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.docStatus"); 2673 } 2674 else if (name.equals("type")) { 2675 this.type = new CodeableConcept(); 2676 return this.type; 2677 } 2678 else if (name.equals("class")) { 2679 this.class_ = new CodeableConcept(); 2680 return this.class_; 2681 } 2682 else if (name.equals("subject")) { 2683 this.subject = new Reference(); 2684 return this.subject; 2685 } 2686 else if (name.equals("created")) { 2687 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.created"); 2688 } 2689 else if (name.equals("indexed")) { 2690 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.indexed"); 2691 } 2692 else if (name.equals("author")) { 2693 return addAuthor(); 2694 } 2695 else if (name.equals("authenticator")) { 2696 this.authenticator = new Reference(); 2697 return this.authenticator; 2698 } 2699 else if (name.equals("custodian")) { 2700 this.custodian = new Reference(); 2701 return this.custodian; 2702 } 2703 else if (name.equals("relatesTo")) { 2704 return addRelatesTo(); 2705 } 2706 else if (name.equals("description")) { 2707 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.description"); 2708 } 2709 else if (name.equals("securityLabel")) { 2710 return addSecurityLabel(); 2711 } 2712 else if (name.equals("content")) { 2713 return addContent(); 2714 } 2715 else if (name.equals("context")) { 2716 this.context = new DocumentReferenceContextComponent(); 2717 return this.context; 2718 } 2719 else 2720 return super.addChild(name); 2721 } 2722 2723 public String fhirType() { 2724 return "DocumentReference"; 2725 2726 } 2727 2728 public DocumentReference copy() { 2729 DocumentReference dst = new DocumentReference(); 2730 copyValues(dst); 2731 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 2732 if (identifier != null) { 2733 dst.identifier = new ArrayList<Identifier>(); 2734 for (Identifier i : identifier) 2735 dst.identifier.add(i.copy()); 2736 }; 2737 dst.status = status == null ? null : status.copy(); 2738 dst.docStatus = docStatus == null ? null : docStatus.copy(); 2739 dst.type = type == null ? null : type.copy(); 2740 dst.class_ = class_ == null ? null : class_.copy(); 2741 dst.subject = subject == null ? null : subject.copy(); 2742 dst.created = created == null ? null : created.copy(); 2743 dst.indexed = indexed == null ? null : indexed.copy(); 2744 if (author != null) { 2745 dst.author = new ArrayList<Reference>(); 2746 for (Reference i : author) 2747 dst.author.add(i.copy()); 2748 }; 2749 dst.authenticator = authenticator == null ? null : authenticator.copy(); 2750 dst.custodian = custodian == null ? null : custodian.copy(); 2751 if (relatesTo != null) { 2752 dst.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2753 for (DocumentReferenceRelatesToComponent i : relatesTo) 2754 dst.relatesTo.add(i.copy()); 2755 }; 2756 dst.description = description == null ? null : description.copy(); 2757 if (securityLabel != null) { 2758 dst.securityLabel = new ArrayList<CodeableConcept>(); 2759 for (CodeableConcept i : securityLabel) 2760 dst.securityLabel.add(i.copy()); 2761 }; 2762 if (content != null) { 2763 dst.content = new ArrayList<DocumentReferenceContentComponent>(); 2764 for (DocumentReferenceContentComponent i : content) 2765 dst.content.add(i.copy()); 2766 }; 2767 dst.context = context == null ? null : context.copy(); 2768 return dst; 2769 } 2770 2771 protected DocumentReference typedCopy() { 2772 return copy(); 2773 } 2774 2775 @Override 2776 public boolean equalsDeep(Base other_) { 2777 if (!super.equalsDeep(other_)) 2778 return false; 2779 if (!(other_ instanceof DocumentReference)) 2780 return false; 2781 DocumentReference o = (DocumentReference) other_; 2782 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 2783 && compareDeep(status, o.status, true) && compareDeep(docStatus, o.docStatus, true) && compareDeep(type, o.type, true) 2784 && compareDeep(class_, o.class_, true) && compareDeep(subject, o.subject, true) && compareDeep(created, o.created, true) 2785 && compareDeep(indexed, o.indexed, true) && compareDeep(author, o.author, true) && compareDeep(authenticator, o.authenticator, true) 2786 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) && compareDeep(description, o.description, true) 2787 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(content, o.content, true) && compareDeep(context, o.context, true) 2788 ; 2789 } 2790 2791 @Override 2792 public boolean equalsShallow(Base other_) { 2793 if (!super.equalsShallow(other_)) 2794 return false; 2795 if (!(other_ instanceof DocumentReference)) 2796 return false; 2797 DocumentReference o = (DocumentReference) other_; 2798 return compareValues(status, o.status, true) && compareValues(docStatus, o.docStatus, true) && compareValues(created, o.created, true) 2799 && compareValues(indexed, o.indexed, true) && compareValues(description, o.description, true); 2800 } 2801 2802 public boolean isEmpty() { 2803 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(masterIdentifier, identifier 2804 , status, docStatus, type, class_, subject, created, indexed, author, authenticator 2805 , custodian, relatesTo, description, securityLabel, content, context); 2806 } 2807 2808 @Override 2809 public ResourceType getResourceType() { 2810 return ResourceType.DocumentReference; 2811 } 2812 2813 /** 2814 * Search parameter: <b>securitylabel</b> 2815 * <p> 2816 * Description: <b>Document security-tags</b><br> 2817 * Type: <b>token</b><br> 2818 * Path: <b>DocumentReference.securityLabel</b><br> 2819 * </p> 2820 */ 2821 @SearchParamDefinition(name="securitylabel", path="DocumentReference.securityLabel", description="Document security-tags", type="token" ) 2822 public static final String SP_SECURITYLABEL = "securitylabel"; 2823 /** 2824 * <b>Fluent Client</b> search parameter constant for <b>securitylabel</b> 2825 * <p> 2826 * Description: <b>Document security-tags</b><br> 2827 * Type: <b>token</b><br> 2828 * Path: <b>DocumentReference.securityLabel</b><br> 2829 * </p> 2830 */ 2831 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITYLABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITYLABEL); 2832 2833 /** 2834 * Search parameter: <b>subject</b> 2835 * <p> 2836 * Description: <b>Who/what is the subject of the document</b><br> 2837 * Type: <b>reference</b><br> 2838 * Path: <b>DocumentReference.subject</b><br> 2839 * </p> 2840 */ 2841 @SearchParamDefinition(name="subject", path="DocumentReference.subject", description="Who/what is the subject of the document", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Group.class, Patient.class, Practitioner.class } ) 2842 public static final String SP_SUBJECT = "subject"; 2843 /** 2844 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2845 * <p> 2846 * Description: <b>Who/what is the subject of the document</b><br> 2847 * Type: <b>reference</b><br> 2848 * Path: <b>DocumentReference.subject</b><br> 2849 * </p> 2850 */ 2851 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2852 2853/** 2854 * Constant for fluent queries to be used to add include statements. Specifies 2855 * the path value of "<b>DocumentReference:subject</b>". 2856 */ 2857 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DocumentReference:subject").toLocked(); 2858 2859 /** 2860 * Search parameter: <b>description</b> 2861 * <p> 2862 * Description: <b>Human-readable description (title)</b><br> 2863 * Type: <b>string</b><br> 2864 * Path: <b>DocumentReference.description</b><br> 2865 * </p> 2866 */ 2867 @SearchParamDefinition(name="description", path="DocumentReference.description", description="Human-readable description (title)", type="string" ) 2868 public static final String SP_DESCRIPTION = "description"; 2869 /** 2870 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2871 * <p> 2872 * Description: <b>Human-readable description (title)</b><br> 2873 * Type: <b>string</b><br> 2874 * Path: <b>DocumentReference.description</b><br> 2875 * </p> 2876 */ 2877 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2878 2879 /** 2880 * Search parameter: <b>language</b> 2881 * <p> 2882 * Description: <b>Human language of the content (BCP-47)</b><br> 2883 * Type: <b>token</b><br> 2884 * Path: <b>DocumentReference.content.attachment.language</b><br> 2885 * </p> 2886 */ 2887 @SearchParamDefinition(name="language", path="DocumentReference.content.attachment.language", description="Human language of the content (BCP-47)", type="token" ) 2888 public static final String SP_LANGUAGE = "language"; 2889 /** 2890 * <b>Fluent Client</b> search parameter constant for <b>language</b> 2891 * <p> 2892 * Description: <b>Human language of the content (BCP-47)</b><br> 2893 * Type: <b>token</b><br> 2894 * Path: <b>DocumentReference.content.attachment.language</b><br> 2895 * </p> 2896 */ 2897 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LANGUAGE); 2898 2899 /** 2900 * Search parameter: <b>type</b> 2901 * <p> 2902 * Description: <b>Kind of document (LOINC if possible)</b><br> 2903 * Type: <b>token</b><br> 2904 * Path: <b>DocumentReference.type</b><br> 2905 * </p> 2906 */ 2907 @SearchParamDefinition(name="type", path="DocumentReference.type", description="Kind of document (LOINC if possible)", type="token" ) 2908 public static final String SP_TYPE = "type"; 2909 /** 2910 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2911 * <p> 2912 * Description: <b>Kind of document (LOINC if possible)</b><br> 2913 * Type: <b>token</b><br> 2914 * Path: <b>DocumentReference.type</b><br> 2915 * </p> 2916 */ 2917 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2918 2919 /** 2920 * Search parameter: <b>relation</b> 2921 * <p> 2922 * Description: <b>replaces | transforms | signs | appends</b><br> 2923 * Type: <b>token</b><br> 2924 * Path: <b>DocumentReference.relatesTo.code</b><br> 2925 * </p> 2926 */ 2927 @SearchParamDefinition(name="relation", path="DocumentReference.relatesTo.code", description="replaces | transforms | signs | appends", type="token" ) 2928 public static final String SP_RELATION = "relation"; 2929 /** 2930 * <b>Fluent Client</b> search parameter constant for <b>relation</b> 2931 * <p> 2932 * Description: <b>replaces | transforms | signs | appends</b><br> 2933 * Type: <b>token</b><br> 2934 * Path: <b>DocumentReference.relatesTo.code</b><br> 2935 * </p> 2936 */ 2937 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATION); 2938 2939 /** 2940 * Search parameter: <b>setting</b> 2941 * <p> 2942 * Description: <b>Additional details about where the content was created (e.g. clinical specialty)</b><br> 2943 * Type: <b>token</b><br> 2944 * Path: <b>DocumentReference.context.practiceSetting</b><br> 2945 * </p> 2946 */ 2947 @SearchParamDefinition(name="setting", path="DocumentReference.context.practiceSetting", description="Additional details about where the content was created (e.g. clinical specialty)", type="token" ) 2948 public static final String SP_SETTING = "setting"; 2949 /** 2950 * <b>Fluent Client</b> search parameter constant for <b>setting</b> 2951 * <p> 2952 * Description: <b>Additional details about where the content was created (e.g. clinical specialty)</b><br> 2953 * Type: <b>token</b><br> 2954 * Path: <b>DocumentReference.context.practiceSetting</b><br> 2955 * </p> 2956 */ 2957 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SETTING = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SETTING); 2958 2959 /** 2960 * Search parameter: <b>patient</b> 2961 * <p> 2962 * Description: <b>Who/what is the subject of the document</b><br> 2963 * Type: <b>reference</b><br> 2964 * Path: <b>DocumentReference.subject</b><br> 2965 * </p> 2966 */ 2967 @SearchParamDefinition(name="patient", path="DocumentReference.subject", description="Who/what is the subject of the document", type="reference", target={Patient.class } ) 2968 public static final String SP_PATIENT = "patient"; 2969 /** 2970 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2971 * <p> 2972 * Description: <b>Who/what is the subject of the document</b><br> 2973 * Type: <b>reference</b><br> 2974 * Path: <b>DocumentReference.subject</b><br> 2975 * </p> 2976 */ 2977 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2978 2979/** 2980 * Constant for fluent queries to be used to add include statements. Specifies 2981 * the path value of "<b>DocumentReference:patient</b>". 2982 */ 2983 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DocumentReference:patient").toLocked(); 2984 2985 /** 2986 * Search parameter: <b>relationship</b> 2987 * <p> 2988 * Description: <b>Combination of relation and relatesTo</b><br> 2989 * Type: <b>composite</b><br> 2990 * Path: <b></b><br> 2991 * </p> 2992 */ 2993 @SearchParamDefinition(name="relationship", path="DocumentReference.relatesTo", description="Combination of relation and relatesTo", type="composite", compositeOf={"relatesto", "relation"} ) 2994 public static final String SP_RELATIONSHIP = "relationship"; 2995 /** 2996 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 2997 * <p> 2998 * Description: <b>Combination of relation and relatesTo</b><br> 2999 * Type: <b>composite</b><br> 3000 * Path: <b></b><br> 3001 * </p> 3002 */ 3003 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> RELATIONSHIP = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_RELATIONSHIP); 3004 3005 /** 3006 * Search parameter: <b>event</b> 3007 * <p> 3008 * Description: <b>Main clinical acts documented</b><br> 3009 * Type: <b>token</b><br> 3010 * Path: <b>DocumentReference.context.event</b><br> 3011 * </p> 3012 */ 3013 @SearchParamDefinition(name="event", path="DocumentReference.context.event", description="Main clinical acts documented", type="token" ) 3014 public static final String SP_EVENT = "event"; 3015 /** 3016 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3017 * <p> 3018 * Description: <b>Main clinical acts documented</b><br> 3019 * Type: <b>token</b><br> 3020 * Path: <b>DocumentReference.context.event</b><br> 3021 * </p> 3022 */ 3023 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 3024 3025 /** 3026 * Search parameter: <b>class</b> 3027 * <p> 3028 * Description: <b>Categorization of document</b><br> 3029 * Type: <b>token</b><br> 3030 * Path: <b>DocumentReference.class</b><br> 3031 * </p> 3032 */ 3033 @SearchParamDefinition(name="class", path="DocumentReference.class", description="Categorization of document", type="token" ) 3034 public static final String SP_CLASS = "class"; 3035 /** 3036 * <b>Fluent Client</b> search parameter constant for <b>class</b> 3037 * <p> 3038 * Description: <b>Categorization of document</b><br> 3039 * Type: <b>token</b><br> 3040 * Path: <b>DocumentReference.class</b><br> 3041 * </p> 3042 */ 3043 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASS); 3044 3045 /** 3046 * Search parameter: <b>authenticator</b> 3047 * <p> 3048 * Description: <b>Who/what authenticated the document</b><br> 3049 * Type: <b>reference</b><br> 3050 * Path: <b>DocumentReference.authenticator</b><br> 3051 * </p> 3052 */ 3053 @SearchParamDefinition(name="authenticator", path="DocumentReference.authenticator", description="Who/what authenticated the document", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 3054 public static final String SP_AUTHENTICATOR = "authenticator"; 3055 /** 3056 * <b>Fluent Client</b> search parameter constant for <b>authenticator</b> 3057 * <p> 3058 * Description: <b>Who/what authenticated the document</b><br> 3059 * Type: <b>reference</b><br> 3060 * Path: <b>DocumentReference.authenticator</b><br> 3061 * </p> 3062 */ 3063 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHENTICATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHENTICATOR); 3064 3065/** 3066 * Constant for fluent queries to be used to add include statements. Specifies 3067 * the path value of "<b>DocumentReference:authenticator</b>". 3068 */ 3069 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHENTICATOR = new ca.uhn.fhir.model.api.Include("DocumentReference:authenticator").toLocked(); 3070 3071 /** 3072 * Search parameter: <b>identifier</b> 3073 * <p> 3074 * Description: <b>Master Version Specific Identifier</b><br> 3075 * Type: <b>token</b><br> 3076 * Path: <b>DocumentReference.masterIdentifier, DocumentReference.identifier</b><br> 3077 * </p> 3078 */ 3079 @SearchParamDefinition(name="identifier", path="DocumentReference.masterIdentifier | DocumentReference.identifier", description="Master Version Specific Identifier", type="token" ) 3080 public static final String SP_IDENTIFIER = "identifier"; 3081 /** 3082 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3083 * <p> 3084 * Description: <b>Master Version Specific Identifier</b><br> 3085 * Type: <b>token</b><br> 3086 * Path: <b>DocumentReference.masterIdentifier, DocumentReference.identifier</b><br> 3087 * </p> 3088 */ 3089 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3090 3091 /** 3092 * Search parameter: <b>period</b> 3093 * <p> 3094 * Description: <b>Time of service that is being documented</b><br> 3095 * Type: <b>date</b><br> 3096 * Path: <b>DocumentReference.context.period</b><br> 3097 * </p> 3098 */ 3099 @SearchParamDefinition(name="period", path="DocumentReference.context.period", description="Time of service that is being documented", type="date" ) 3100 public static final String SP_PERIOD = "period"; 3101 /** 3102 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3103 * <p> 3104 * Description: <b>Time of service that is being documented</b><br> 3105 * Type: <b>date</b><br> 3106 * Path: <b>DocumentReference.context.period</b><br> 3107 * </p> 3108 */ 3109 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 3110 3111 /** 3112 * Search parameter: <b>related-id</b> 3113 * <p> 3114 * Description: <b>Identifier of related objects or events</b><br> 3115 * Type: <b>token</b><br> 3116 * Path: <b>DocumentReference.context.related.identifier</b><br> 3117 * </p> 3118 */ 3119 @SearchParamDefinition(name="related-id", path="DocumentReference.context.related.identifier", description="Identifier of related objects or events", type="token" ) 3120 public static final String SP_RELATED_ID = "related-id"; 3121 /** 3122 * <b>Fluent Client</b> search parameter constant for <b>related-id</b> 3123 * <p> 3124 * Description: <b>Identifier of related objects or events</b><br> 3125 * Type: <b>token</b><br> 3126 * Path: <b>DocumentReference.context.related.identifier</b><br> 3127 * </p> 3128 */ 3129 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATED_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATED_ID); 3130 3131 /** 3132 * Search parameter: <b>custodian</b> 3133 * <p> 3134 * Description: <b>Organization which maintains the document</b><br> 3135 * Type: <b>reference</b><br> 3136 * Path: <b>DocumentReference.custodian</b><br> 3137 * </p> 3138 */ 3139 @SearchParamDefinition(name="custodian", path="DocumentReference.custodian", description="Organization which maintains the document", type="reference", target={Organization.class } ) 3140 public static final String SP_CUSTODIAN = "custodian"; 3141 /** 3142 * <b>Fluent Client</b> search parameter constant for <b>custodian</b> 3143 * <p> 3144 * Description: <b>Organization which maintains the document</b><br> 3145 * Type: <b>reference</b><br> 3146 * Path: <b>DocumentReference.custodian</b><br> 3147 * </p> 3148 */ 3149 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CUSTODIAN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CUSTODIAN); 3150 3151/** 3152 * Constant for fluent queries to be used to add include statements. Specifies 3153 * the path value of "<b>DocumentReference:custodian</b>". 3154 */ 3155 public static final ca.uhn.fhir.model.api.Include INCLUDE_CUSTODIAN = new ca.uhn.fhir.model.api.Include("DocumentReference:custodian").toLocked(); 3156 3157 /** 3158 * Search parameter: <b>indexed</b> 3159 * <p> 3160 * Description: <b>When this document reference was created</b><br> 3161 * Type: <b>date</b><br> 3162 * Path: <b>DocumentReference.indexed</b><br> 3163 * </p> 3164 */ 3165 @SearchParamDefinition(name="indexed", path="DocumentReference.indexed", description="When this document reference was created", type="date" ) 3166 public static final String SP_INDEXED = "indexed"; 3167 /** 3168 * <b>Fluent Client</b> search parameter constant for <b>indexed</b> 3169 * <p> 3170 * Description: <b>When this document reference was created</b><br> 3171 * Type: <b>date</b><br> 3172 * Path: <b>DocumentReference.indexed</b><br> 3173 * </p> 3174 */ 3175 public static final ca.uhn.fhir.rest.gclient.DateClientParam INDEXED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_INDEXED); 3176 3177 /** 3178 * Search parameter: <b>author</b> 3179 * <p> 3180 * Description: <b>Who and/or what authored the document</b><br> 3181 * Type: <b>reference</b><br> 3182 * Path: <b>DocumentReference.author</b><br> 3183 * </p> 3184 */ 3185 @SearchParamDefinition(name="author", path="DocumentReference.author", description="Who and/or what authored the document", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3186 public static final String SP_AUTHOR = "author"; 3187 /** 3188 * <b>Fluent Client</b> search parameter constant for <b>author</b> 3189 * <p> 3190 * Description: <b>Who and/or what authored the document</b><br> 3191 * Type: <b>reference</b><br> 3192 * Path: <b>DocumentReference.author</b><br> 3193 * </p> 3194 */ 3195 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 3196 3197/** 3198 * Constant for fluent queries to be used to add include statements. Specifies 3199 * the path value of "<b>DocumentReference:author</b>". 3200 */ 3201 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("DocumentReference:author").toLocked(); 3202 3203 /** 3204 * Search parameter: <b>created</b> 3205 * <p> 3206 * Description: <b>Document creation time</b><br> 3207 * Type: <b>date</b><br> 3208 * Path: <b>DocumentReference.created</b><br> 3209 * </p> 3210 */ 3211 @SearchParamDefinition(name="created", path="DocumentReference.created", description="Document creation time", type="date" ) 3212 public static final String SP_CREATED = "created"; 3213 /** 3214 * <b>Fluent Client</b> search parameter constant for <b>created</b> 3215 * <p> 3216 * Description: <b>Document creation time</b><br> 3217 * Type: <b>date</b><br> 3218 * Path: <b>DocumentReference.created</b><br> 3219 * </p> 3220 */ 3221 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 3222 3223 /** 3224 * Search parameter: <b>format</b> 3225 * <p> 3226 * Description: <b>Format/content rules for the document</b><br> 3227 * Type: <b>token</b><br> 3228 * Path: <b>DocumentReference.content.format</b><br> 3229 * </p> 3230 */ 3231 @SearchParamDefinition(name="format", path="DocumentReference.content.format", description="Format/content rules for the document", type="token" ) 3232 public static final String SP_FORMAT = "format"; 3233 /** 3234 * <b>Fluent Client</b> search parameter constant for <b>format</b> 3235 * <p> 3236 * Description: <b>Format/content rules for the document</b><br> 3237 * Type: <b>token</b><br> 3238 * Path: <b>DocumentReference.content.format</b><br> 3239 * </p> 3240 */ 3241 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORMAT); 3242 3243 /** 3244 * Search parameter: <b>encounter</b> 3245 * <p> 3246 * Description: <b>Context of the document content</b><br> 3247 * Type: <b>reference</b><br> 3248 * Path: <b>DocumentReference.context.encounter</b><br> 3249 * </p> 3250 */ 3251 @SearchParamDefinition(name="encounter", path="DocumentReference.context.encounter", description="Context of the document content", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 3252 public static final String SP_ENCOUNTER = "encounter"; 3253 /** 3254 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3255 * <p> 3256 * Description: <b>Context of the document content</b><br> 3257 * Type: <b>reference</b><br> 3258 * Path: <b>DocumentReference.context.encounter</b><br> 3259 * </p> 3260 */ 3261 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3262 3263/** 3264 * Constant for fluent queries to be used to add include statements. Specifies 3265 * the path value of "<b>DocumentReference:encounter</b>". 3266 */ 3267 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("DocumentReference:encounter").toLocked(); 3268 3269 /** 3270 * Search parameter: <b>related-ref</b> 3271 * <p> 3272 * Description: <b>Related Resource</b><br> 3273 * Type: <b>reference</b><br> 3274 * Path: <b>DocumentReference.context.related.ref</b><br> 3275 * </p> 3276 */ 3277 @SearchParamDefinition(name="related-ref", path="DocumentReference.context.related.ref", description="Related Resource", type="reference" ) 3278 public static final String SP_RELATED_REF = "related-ref"; 3279 /** 3280 * <b>Fluent Client</b> search parameter constant for <b>related-ref</b> 3281 * <p> 3282 * Description: <b>Related Resource</b><br> 3283 * Type: <b>reference</b><br> 3284 * Path: <b>DocumentReference.context.related.ref</b><br> 3285 * </p> 3286 */ 3287 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATED_REF); 3288 3289/** 3290 * Constant for fluent queries to be used to add include statements. Specifies 3291 * the path value of "<b>DocumentReference:related-ref</b>". 3292 */ 3293 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED_REF = new ca.uhn.fhir.model.api.Include("DocumentReference:related-ref").toLocked(); 3294 3295 /** 3296 * Search parameter: <b>location</b> 3297 * <p> 3298 * Description: <b>Uri where the data can be found</b><br> 3299 * Type: <b>uri</b><br> 3300 * Path: <b>DocumentReference.content.attachment.url</b><br> 3301 * </p> 3302 */ 3303 @SearchParamDefinition(name="location", path="DocumentReference.content.attachment.url", description="Uri where the data can be found", type="uri" ) 3304 public static final String SP_LOCATION = "location"; 3305 /** 3306 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3307 * <p> 3308 * Description: <b>Uri where the data can be found</b><br> 3309 * Type: <b>uri</b><br> 3310 * Path: <b>DocumentReference.content.attachment.url</b><br> 3311 * </p> 3312 */ 3313 public static final ca.uhn.fhir.rest.gclient.UriClientParam LOCATION = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_LOCATION); 3314 3315 /** 3316 * Search parameter: <b>relatesto</b> 3317 * <p> 3318 * Description: <b>Target of the relationship</b><br> 3319 * Type: <b>reference</b><br> 3320 * Path: <b>DocumentReference.relatesTo.target</b><br> 3321 * </p> 3322 */ 3323 @SearchParamDefinition(name="relatesto", path="DocumentReference.relatesTo.target", description="Target of the relationship", type="reference", target={DocumentReference.class } ) 3324 public static final String SP_RELATESTO = "relatesto"; 3325 /** 3326 * <b>Fluent Client</b> search parameter constant for <b>relatesto</b> 3327 * <p> 3328 * Description: <b>Target of the relationship</b><br> 3329 * Type: <b>reference</b><br> 3330 * Path: <b>DocumentReference.relatesTo.target</b><br> 3331 * </p> 3332 */ 3333 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATESTO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATESTO); 3334 3335/** 3336 * Constant for fluent queries to be used to add include statements. Specifies 3337 * the path value of "<b>DocumentReference:relatesto</b>". 3338 */ 3339 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATESTO = new ca.uhn.fhir.model.api.Include("DocumentReference:relatesto").toLocked(); 3340 3341 /** 3342 * Search parameter: <b>facility</b> 3343 * <p> 3344 * Description: <b>Kind of facility where patient was seen</b><br> 3345 * Type: <b>token</b><br> 3346 * Path: <b>DocumentReference.context.facilityType</b><br> 3347 * </p> 3348 */ 3349 @SearchParamDefinition(name="facility", path="DocumentReference.context.facilityType", description="Kind of facility where patient was seen", type="token" ) 3350 public static final String SP_FACILITY = "facility"; 3351 /** 3352 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 3353 * <p> 3354 * Description: <b>Kind of facility where patient was seen</b><br> 3355 * Type: <b>token</b><br> 3356 * Path: <b>DocumentReference.context.facilityType</b><br> 3357 * </p> 3358 */ 3359 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FACILITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FACILITY); 3360 3361 /** 3362 * Search parameter: <b>status</b> 3363 * <p> 3364 * Description: <b>current | superseded | entered-in-error</b><br> 3365 * Type: <b>token</b><br> 3366 * Path: <b>DocumentReference.status</b><br> 3367 * </p> 3368 */ 3369 @SearchParamDefinition(name="status", path="DocumentReference.status", description="current | superseded | entered-in-error", type="token" ) 3370 public static final String SP_STATUS = "status"; 3371 /** 3372 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3373 * <p> 3374 * Description: <b>current | superseded | entered-in-error</b><br> 3375 * Type: <b>token</b><br> 3376 * Path: <b>DocumentReference.status</b><br> 3377 * </p> 3378 */ 3379 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3380 3381 3382}