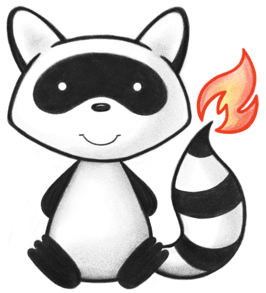
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Collections; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 041import org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions; 042import org.hl7.fhir.instance.model.api.IDomainResource; 043 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046/** 047 * A resource that includes narrative, extensions, and contained resources. 048 */ 049public abstract class DomainResource extends Resource implements IBaseHasExtensions, IBaseHasModifierExtensions, IDomainResource { 050 051 /** 052 * A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety. 053 */ 054 @Child(name = "text", type = {Narrative.class}, order=0, min=0, max=1, modifier=false, summary=false) 055 @Description(shortDefinition="Text summary of the resource, for human interpretation", formalDefinition="A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety." ) 056 protected Narrative text; 057 058 /** 059 * These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope. 060 */ 061 @Child(name = "contained", type = {Resource.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 062 @Description(shortDefinition="Contained, inline Resources", formalDefinition="These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope." ) 063 protected List<Resource> contained; 064 065 /** 066 * May be used to represent additional information that is not part of the basic definition of the resource. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. 067 */ 068 @Child(name = "extension", type = {Extension.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 069 @Description(shortDefinition="Additional Content defined by implementations", formalDefinition="May be used to represent additional information that is not part of the basic definition of the resource. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension." ) 070 protected List<Extension> extension; 071 072 /** 073 * May be used to represent additional information that is not part of the basic definition of the resource, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 074 */ 075 @Child(name = "modifierExtension", type = {Extension.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=false) 076 @Description(shortDefinition="Extensions that cannot be ignored", formalDefinition="May be used to represent additional information that is not part of the basic definition of the resource, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions." ) 077 protected List<Extension> modifierExtension; 078 079 private static final long serialVersionUID = -970285559L; 080 081 /** 082 * Constructor 083 */ 084 public DomainResource() { 085 super(); 086 } 087 088 /** 089 * @return {@link #text} (A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.) 090 */ 091 public Narrative getText() { 092 if (this.text == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create DomainResource.text"); 095 else if (Configuration.doAutoCreate()) 096 this.text = new Narrative(); // cc 097 return this.text; 098 } 099 100 public boolean hasText() { 101 return this.text != null && !this.text.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #text} (A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.) 106 */ 107 public DomainResource setText(Narrative value) { 108 this.text = value; 109 return this; 110 } 111 112 /** 113 * @return {@link #contained} (These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.) 114 */ 115 public List<Resource> getContained() { 116 if (this.contained == null) 117 this.contained = new ArrayList<Resource>(); 118 return this.contained; 119 } 120 121 /** 122 * @return Returns a reference to <code>this</code> for easy method chaining 123 */ 124 public DomainResource setContained(List<Resource> theContained) { 125 this.contained = theContained; 126 return this; 127 } 128 129 public boolean hasContained() { 130 if (this.contained == null) 131 return false; 132 for (Resource item : this.contained) 133 if (!item.isEmpty()) 134 return true; 135 return false; 136 } 137 138 public DomainResource addContained(Resource t) { //3 139 if (t == null) 140 return this; 141 if (this.contained == null) 142 this.contained = new ArrayList<Resource>(); 143 this.contained.add(t); 144 return this; 145 } 146 147 /** 148 * @return {@link #extension} (May be used to represent additional information that is not part of the basic definition of the resource. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.) 149 */ 150 public List<Extension> getExtension() { 151 if (this.extension == null) 152 this.extension = new ArrayList<Extension>(); 153 return this.extension; 154 } 155 156 /** 157 * @return Returns a reference to <code>this</code> for easy method chaining 158 */ 159 public DomainResource setExtension(List<Extension> theExtension) { 160 this.extension = theExtension; 161 return this; 162 } 163 164 public boolean hasExtension() { 165 if (this.extension == null) 166 return false; 167 for (Extension item : this.extension) 168 if (!item.isEmpty()) 169 return true; 170 return false; 171 } 172 173 public Extension addExtension() { //3 174 Extension t = new Extension(); 175 if (this.extension == null) 176 this.extension = new ArrayList<Extension>(); 177 this.extension.add(t); 178 return t; 179 } 180 181 public DomainResource addExtension(Extension t) { //3 182 if (t == null) 183 return this; 184 if (this.extension == null) 185 this.extension = new ArrayList<Extension>(); 186 this.extension.add(t); 187 return this; 188 } 189 190 /** 191 * @return {@link #modifierExtension} (May be used to represent additional information that is not part of the basic definition of the resource, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.) 192 */ 193 public List<Extension> getModifierExtension() { 194 if (this.modifierExtension == null) 195 this.modifierExtension = new ArrayList<Extension>(); 196 return this.modifierExtension; 197 } 198 199 /** 200 * @return Returns a reference to <code>this</code> for easy method chaining 201 */ 202 public DomainResource setModifierExtension(List<Extension> theModifierExtension) { 203 this.modifierExtension = theModifierExtension; 204 return this; 205 } 206 207 public boolean hasModifierExtension() { 208 if (this.modifierExtension == null) 209 return false; 210 for (Extension item : this.modifierExtension) 211 if (!item.isEmpty()) 212 return true; 213 return false; 214 } 215 216 public Extension addModifierExtension() { //3 217 Extension t = new Extension(); 218 if (this.modifierExtension == null) 219 this.modifierExtension = new ArrayList<Extension>(); 220 this.modifierExtension.add(t); 221 return t; 222 } 223 224 public DomainResource addModifierExtension(Extension t) { //3 225 if (t == null) 226 return this; 227 if (this.modifierExtension == null) 228 this.modifierExtension = new ArrayList<Extension>(); 229 this.modifierExtension.add(t); 230 return this; 231 } 232 233 /** 234 * Returns a list of extensions from this element which have the given URL. Note that 235 * this list may not be modified (you can not add or remove elements from it) 236 */ 237 public List<Extension> getExtensionsByUrl(String theUrl) { 238 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 239 ArrayList<Extension> retVal = new ArrayList<Extension>(); 240 for (Extension next : getExtension()) { 241 if (theUrl.equals(next.getUrl())) { 242 retVal.add(next); 243 } 244 } 245 return Collections.unmodifiableList(retVal); 246 } 247 248 /** 249 * Returns a list of modifier extensions from this element which have the given URL. Note that 250 * this list may not be modified (you can not add or remove elements from it) 251 */ 252 public List<Extension> getModifierExtensionsByUrl(String theUrl) { 253 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 254 ArrayList<Extension> retVal = new ArrayList<Extension>(); 255 for (Extension next : getModifierExtension()) { 256 if (theUrl.equals(next.getUrl())) { 257 retVal.add(next); 258 } 259 } 260 return Collections.unmodifiableList(retVal); 261 } 262 263 protected void listChildren(List<Property> children) { 264 children.add(new Property("text", "Narrative", "A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 0, 1, text)); 265 children.add(new Property("contained", "Resource", "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.", 0, java.lang.Integer.MAX_VALUE, contained)); 266 children.add(new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension)); 267 children.add(new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 268 } 269 270 @Override 271 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 272 switch (_hash) { 273 case 3556653: /*text*/ return new Property("text", "Narrative", "A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 0, 1, text); 274 case -410956685: /*contained*/ return new Property("contained", "Resource", "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.", 0, java.lang.Integer.MAX_VALUE, contained); 275 case -612557761: /*extension*/ return new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension); 276 case -298878168: /*modifierExtension*/ return new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 0, java.lang.Integer.MAX_VALUE, modifierExtension); 277 default: return super.getNamedProperty(_hash, _name, _checkValid); 278 } 279 280 } 281 282 @Override 283 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 284 switch (hash) { 285 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // Narrative 286 case -410956685: /*contained*/ return this.contained == null ? new Base[0] : this.contained.toArray(new Base[this.contained.size()]); // Resource 287 case -612557761: /*extension*/ return this.extension == null ? new Base[0] : this.extension.toArray(new Base[this.extension.size()]); // Extension 288 case -298878168: /*modifierExtension*/ return this.modifierExtension == null ? new Base[0] : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 289 default: return super.getProperty(hash, name, checkValid); 290 } 291 292 } 293 294 @Override 295 public Base setProperty(int hash, String name, Base value) throws FHIRException { 296 switch (hash) { 297 case 3556653: // text 298 this.text = castToNarrative(value); // Narrative 299 return value; 300 case -410956685: // contained 301 this.getContained().add(castToResource(value)); // Resource 302 return value; 303 case -612557761: // extension 304 this.getExtension().add(castToExtension(value)); // Extension 305 return value; 306 case -298878168: // modifierExtension 307 this.getModifierExtension().add(castToExtension(value)); // Extension 308 return value; 309 default: return super.setProperty(hash, name, value); 310 } 311 312 } 313 314 @Override 315 public Base setProperty(String name, Base value) throws FHIRException { 316 if (name.equals("text")) { 317 this.text = castToNarrative(value); // Narrative 318 } else if (name.equals("contained")) { 319 this.getContained().add(castToResource(value)); 320 } else if (name.equals("extension")) { 321 this.getExtension().add(castToExtension(value)); 322 } else if (name.equals("modifierExtension")) { 323 this.getModifierExtension().add(castToExtension(value)); 324 } else 325 return super.setProperty(name, value); 326 return value; 327 } 328 329 @Override 330 public Base makeProperty(int hash, String name) throws FHIRException { 331 switch (hash) { 332 case 3556653: return getText(); 333 case -410956685: throw new FHIRException("Cannot make property contained as it is not a complex type"); // Resource 334 case -612557761: return addExtension(); 335 case -298878168: return addModifierExtension(); 336 default: return super.makeProperty(hash, name); 337 } 338 339 } 340 341 @Override 342 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 343 switch (hash) { 344 case 3556653: /*text*/ return new String[] {"Narrative"}; 345 case -410956685: /*contained*/ return new String[] {"Resource"}; 346 case -612557761: /*extension*/ return new String[] {"Extension"}; 347 case -298878168: /*modifierExtension*/ return new String[] {"Extension"}; 348 default: return super.getTypesForProperty(hash, name); 349 } 350 351 } 352 353 @Override 354 public Base addChild(String name) throws FHIRException { 355 if (name.equals("text")) { 356 this.text = new Narrative(); 357 return this.text; 358 } 359 else if (name.equals("contained")) { 360 throw new FHIRException("Cannot call addChild on an abstract type DomainResource.contained"); 361 } 362 else if (name.equals("extension")) { 363 return addExtension(); 364 } 365 else if (name.equals("modifierExtension")) { 366 return addModifierExtension(); 367 } 368 else 369 return super.addChild(name); 370 } 371 372 public String fhirType() { 373 return "DomainResource"; 374 375 } 376 377 public abstract DomainResource copy(); 378 379 public void copyValues(DomainResource dst) { 380 super.copyValues(dst); 381 dst.text = text == null ? null : text.copy(); 382 if (contained != null) { 383 dst.contained = new ArrayList<Resource>(); 384 for (Resource i : contained) 385 dst.contained.add(i.copy()); 386 }; 387 if (extension != null) { 388 dst.extension = new ArrayList<Extension>(); 389 for (Extension i : extension) 390 dst.extension.add(i.copy()); 391 }; 392 if (modifierExtension != null) { 393 dst.modifierExtension = new ArrayList<Extension>(); 394 for (Extension i : modifierExtension) 395 dst.modifierExtension.add(i.copy()); 396 }; 397 } 398 399 @Override 400 public boolean equalsDeep(Base other_) { 401 if (!super.equalsDeep(other_)) 402 return false; 403 if (!(other_ instanceof DomainResource)) 404 return false; 405 DomainResource o = (DomainResource) other_; 406 return compareDeep(text, o.text, true) && compareDeep(contained, o.contained, true) && compareDeep(extension, o.extension, true) 407 && compareDeep(modifierExtension, o.modifierExtension, true); 408 } 409 410 @Override 411 public boolean equalsShallow(Base other_) { 412 if (!super.equalsShallow(other_)) 413 return false; 414 if (!(other_ instanceof DomainResource)) 415 return false; 416 DomainResource o = (DomainResource) other_; 417 return true; 418 } 419 420 public boolean isEmpty() { 421 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, contained, extension 422 , modifierExtension); 423 } 424 425 426 public void addExtension(String url, Type value) { 427 Extension ex = new Extension(); 428 ex.setUrl(url); 429 ex.setValue(value); 430 getExtension().add(ex); 431 } 432 433 434 public boolean hasExtension(String url) { 435 for (Extension e : getExtension()) 436 if (url.equals(e.getUrl())) 437 return true; 438 return false; 439 } 440 441 public Extension getExtensionByUrl(String theUrl) { 442 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 443 ArrayList<Extension> retVal = new ArrayList<Extension>(); 444 for (Extension next : getExtension()) { 445 if (theUrl.equals(next.getUrl())) { 446 retVal.add(next); 447 } 448 } 449 if (retVal.size() == 0) 450 return null; 451 else { 452 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url "+theUrl+" must have only one match"); 453 return retVal.get(0); 454 } 455 } 456 457 458}